Data Structure Linked List Advantages of Link List
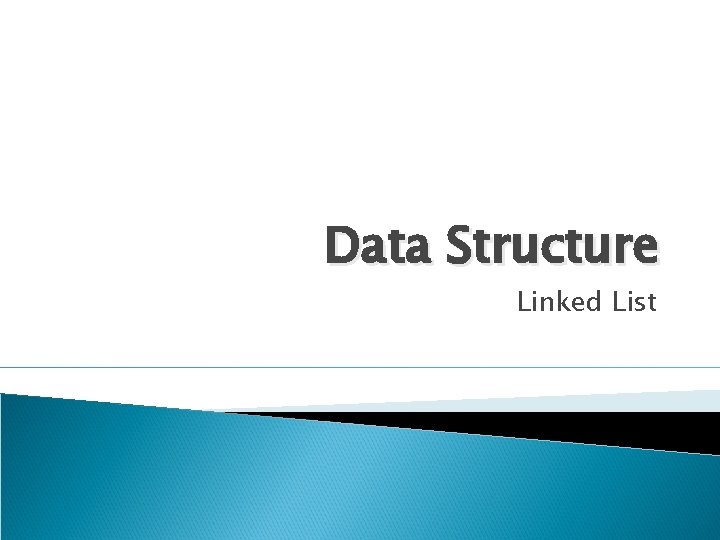
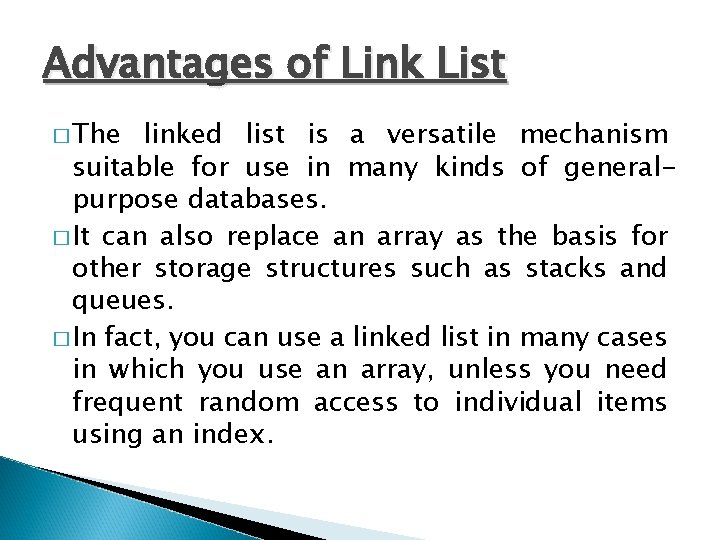
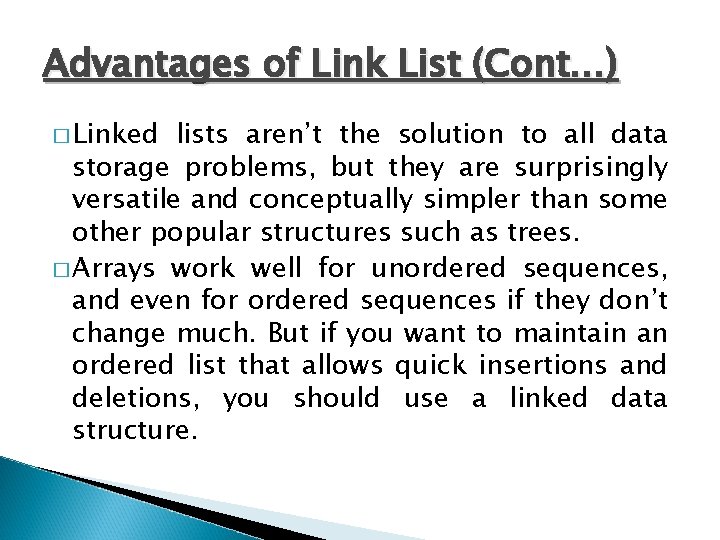
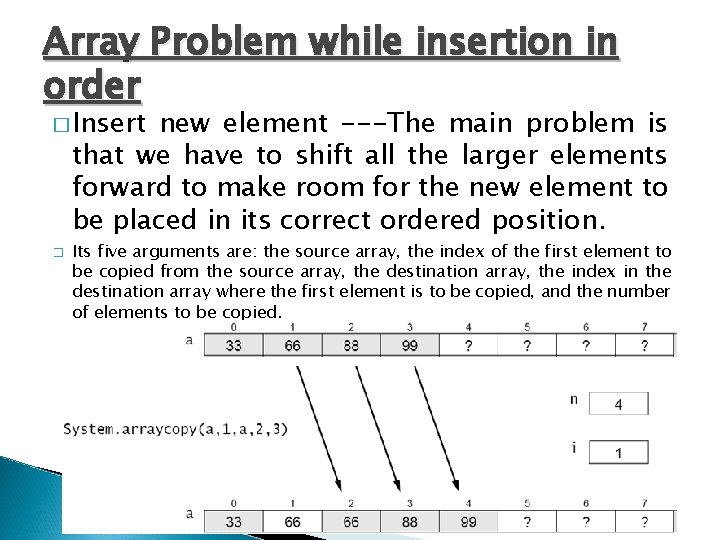
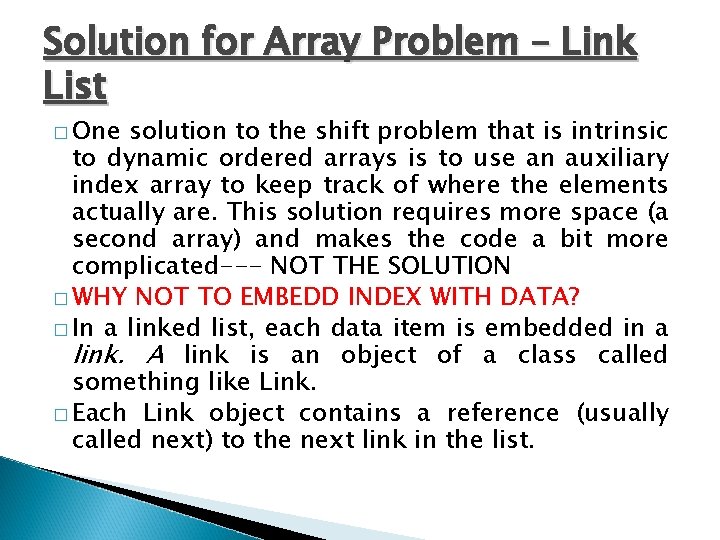
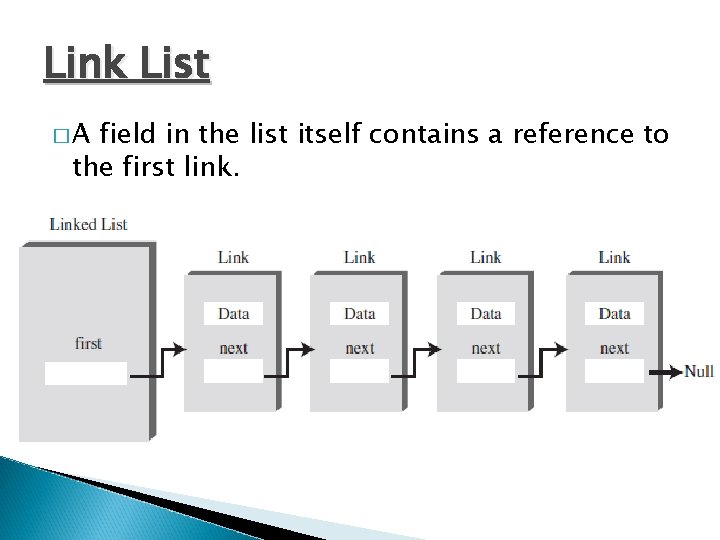
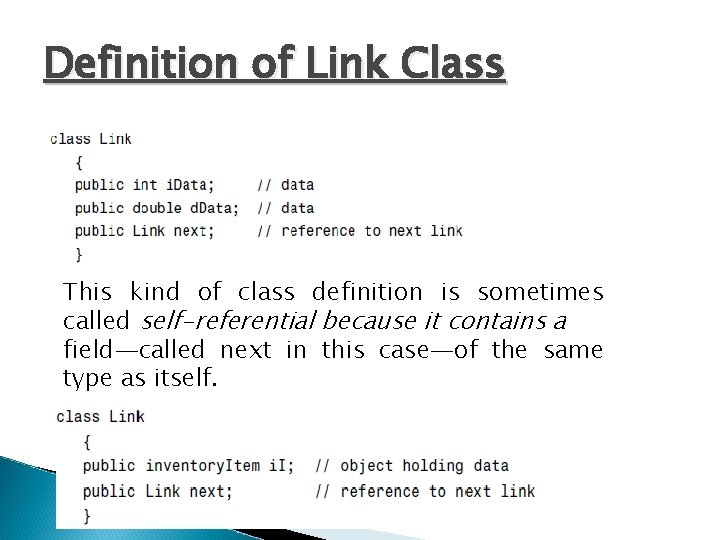
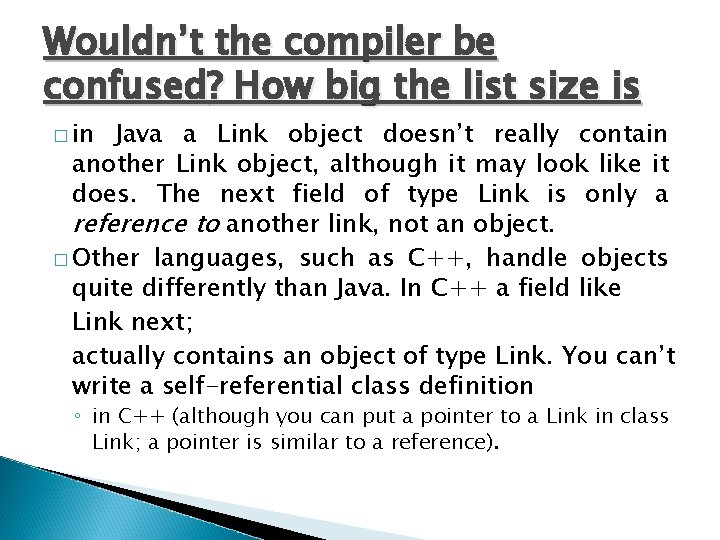
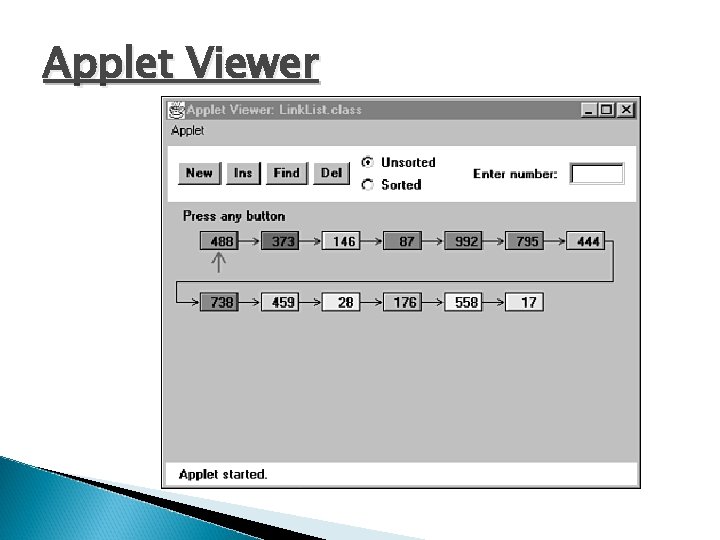
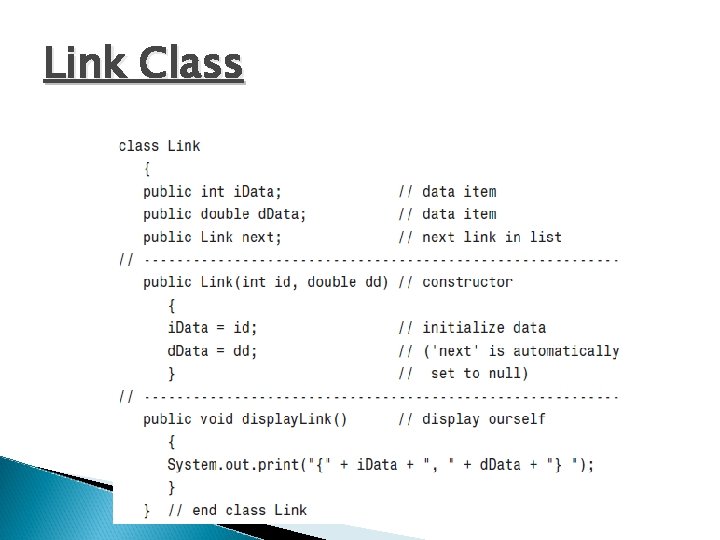
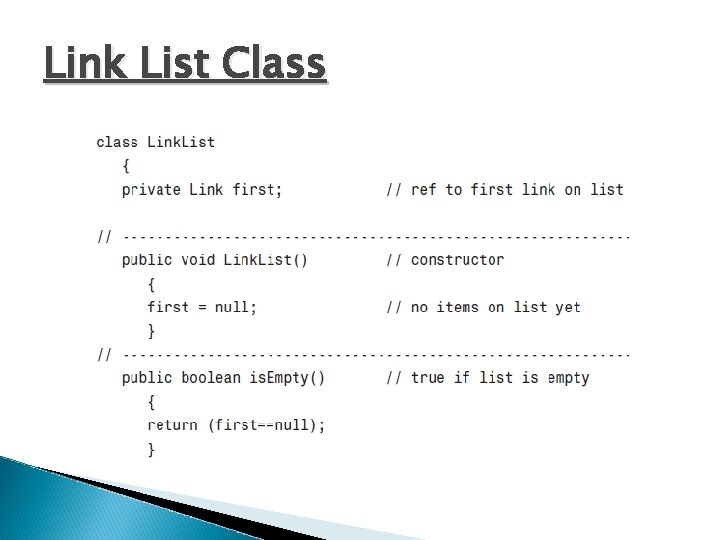
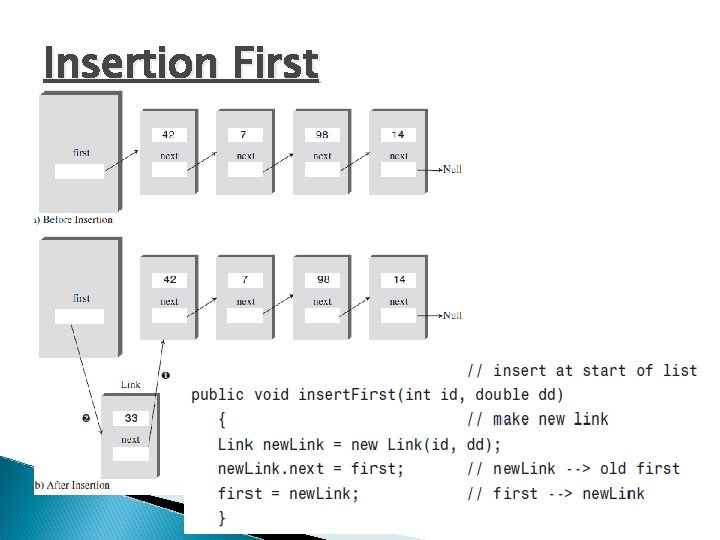
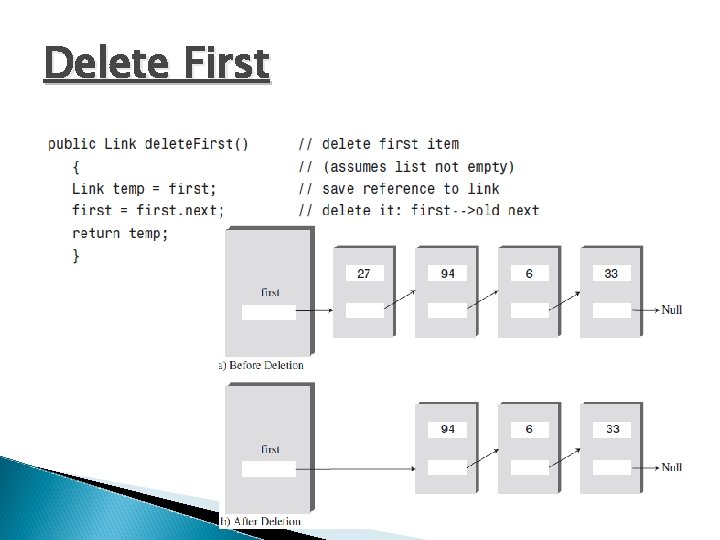
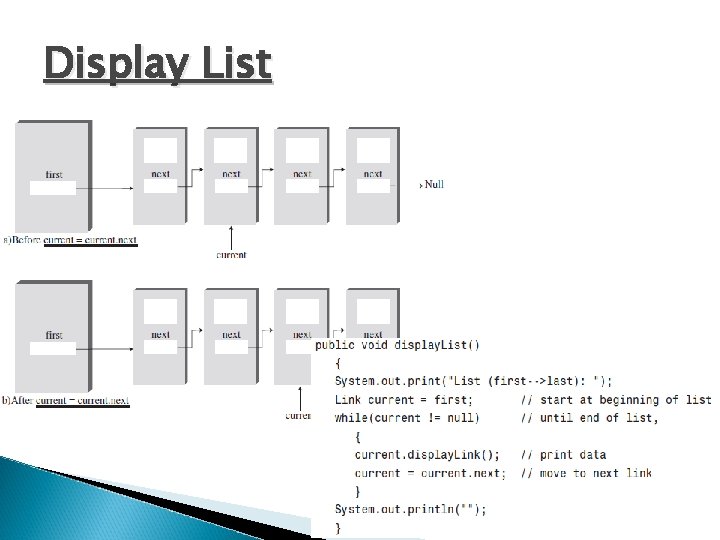
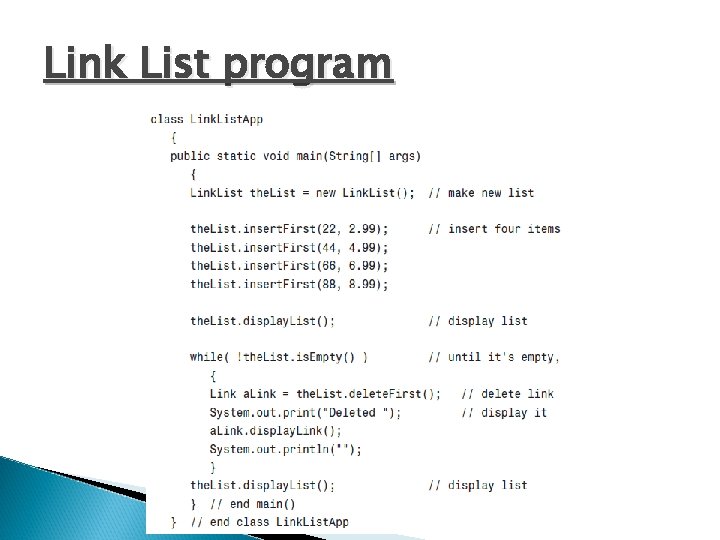
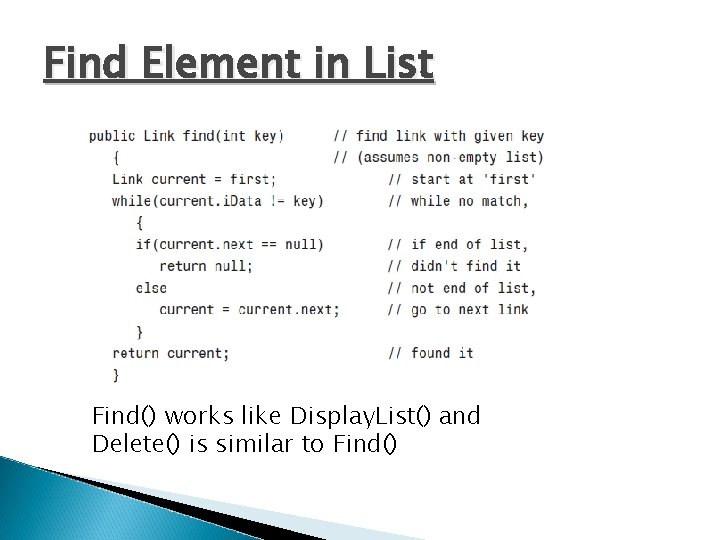
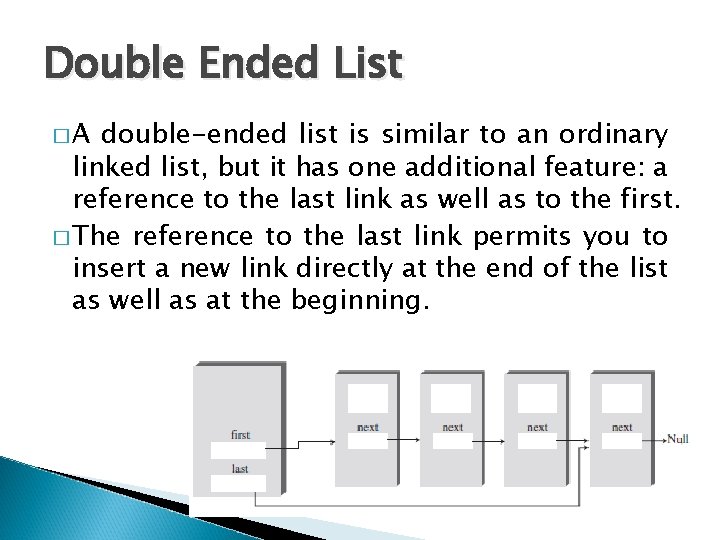
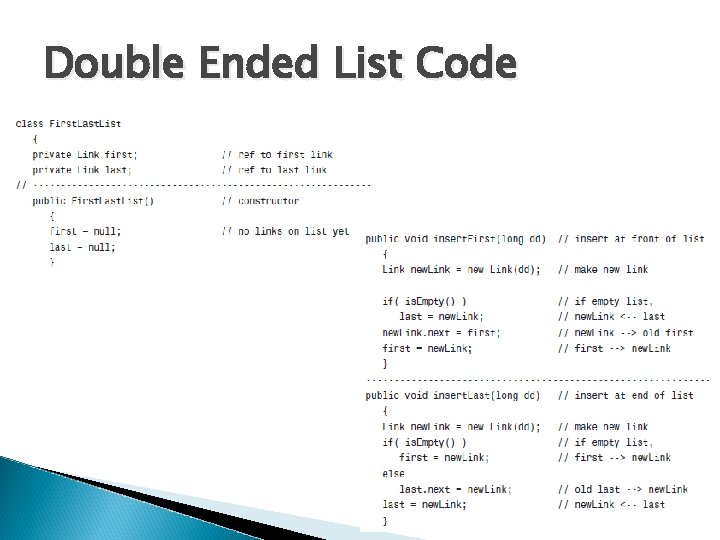
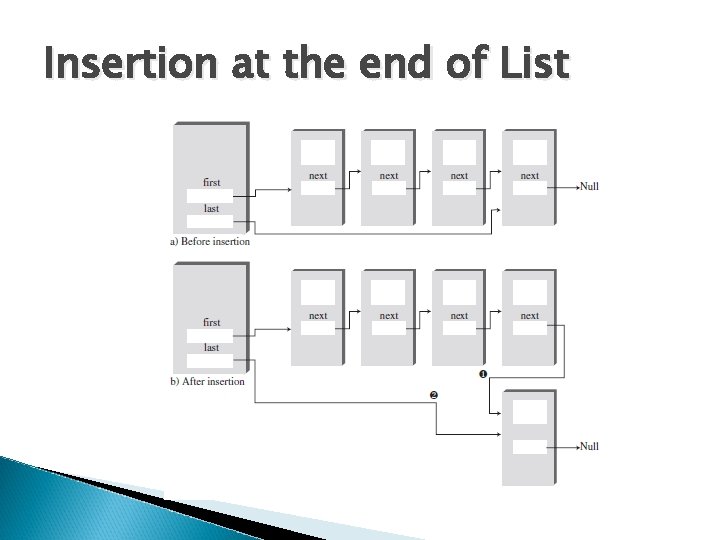
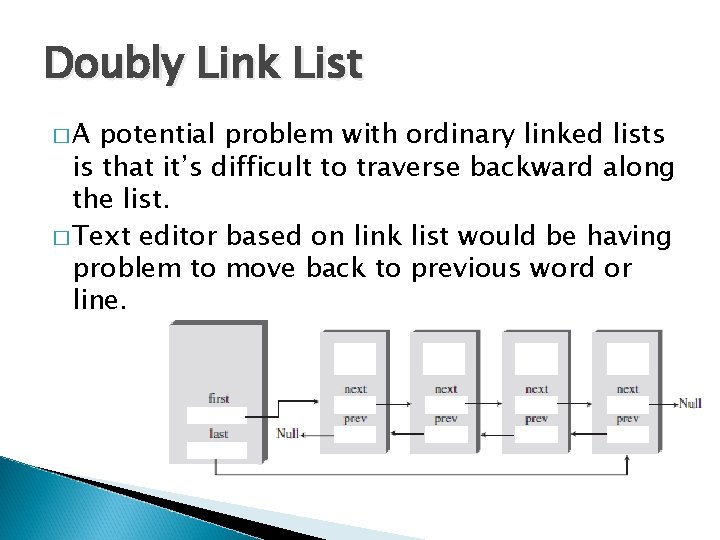
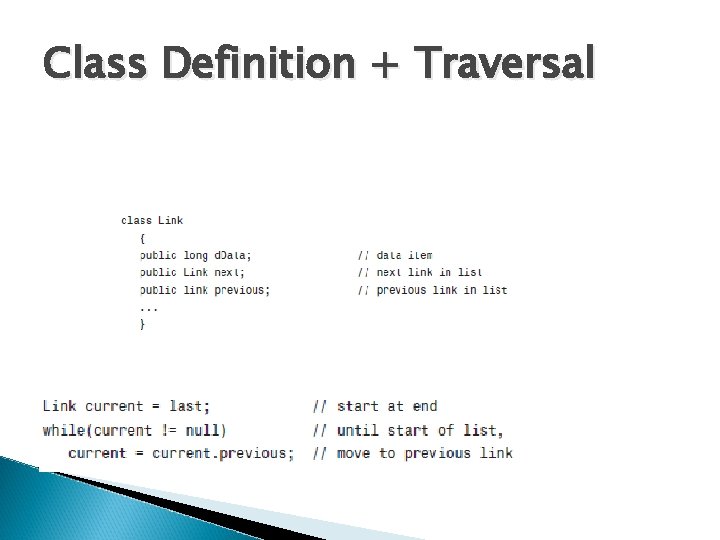
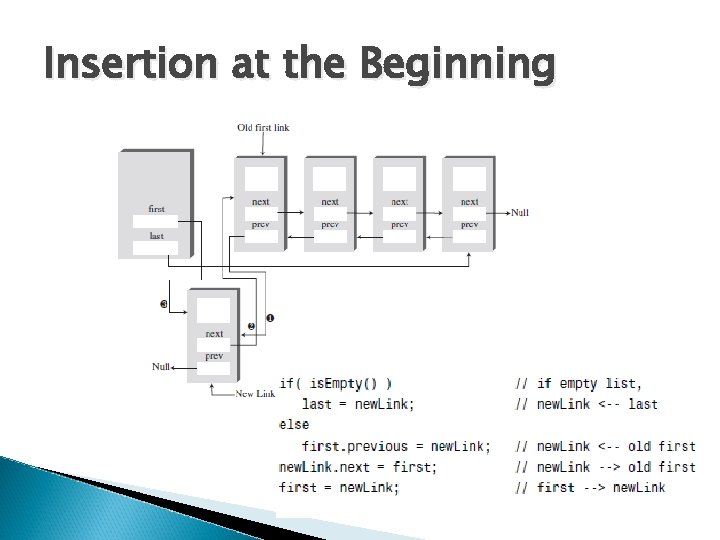
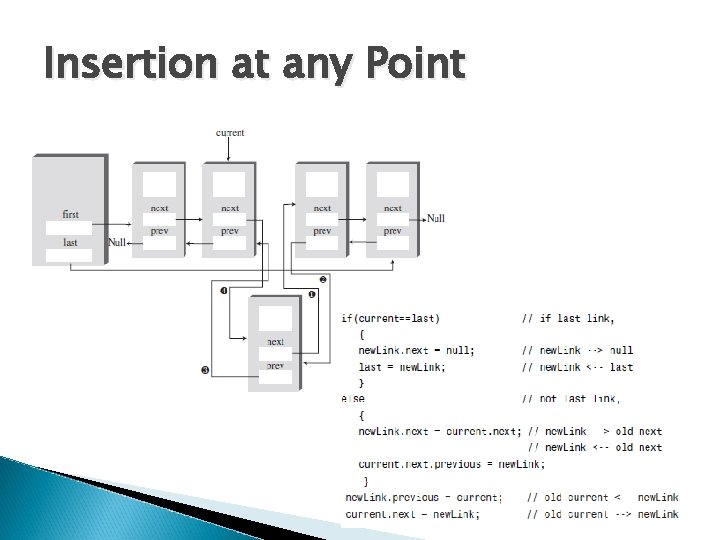
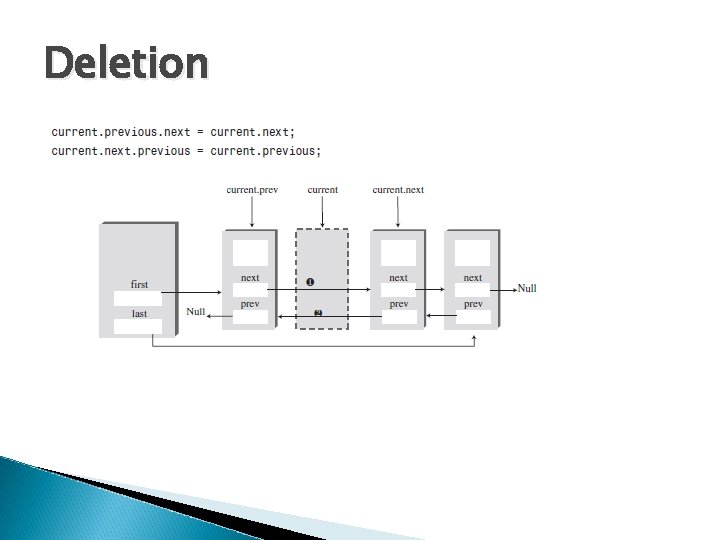
- Slides: 24
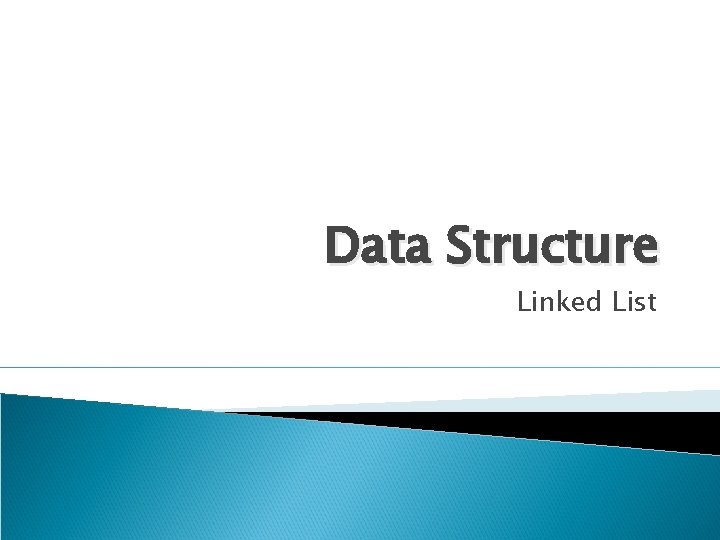
Data Structure Linked List
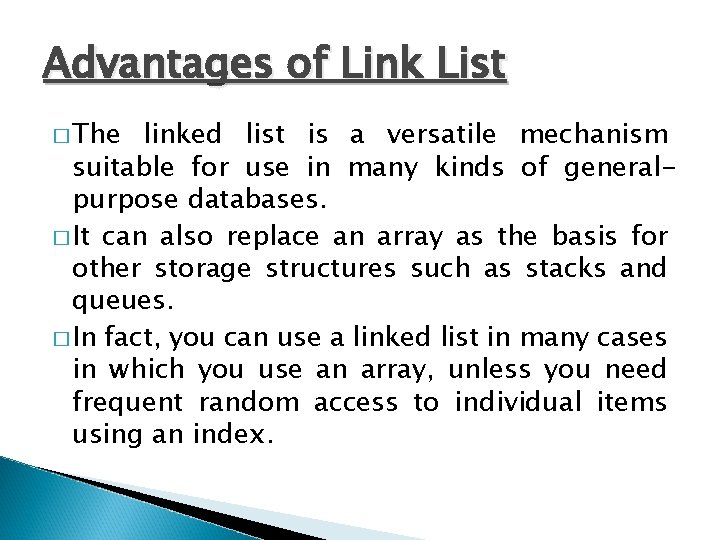
Advantages of Link List � The linked list is a versatile mechanism suitable for use in many kinds of generalpurpose databases. � It can also replace an array as the basis for other storage structures such as stacks and queues. � In fact, you can use a linked list in many cases in which you use an array, unless you need frequent random access to individual items using an index.
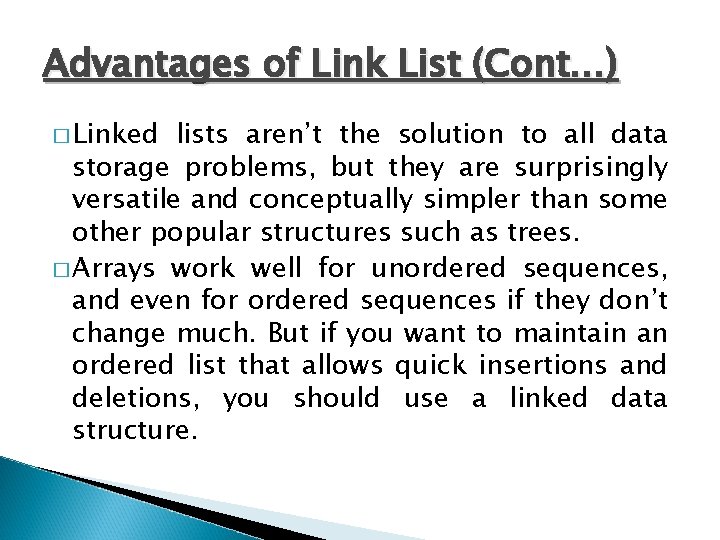
Advantages of Link List (Cont…) � Linked lists aren’t the solution to all data storage problems, but they are surprisingly versatile and conceptually simpler than some other popular structures such as trees. � Arrays work well for unordered sequences, and even for ordered sequences if they don’t change much. But if you want to maintain an ordered list that allows quick insertions and deletions, you should use a linked data structure.
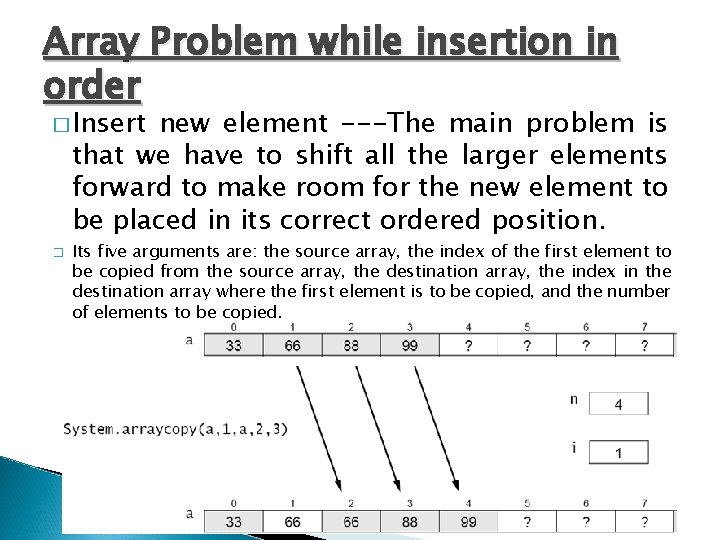
Array Problem while insertion in order � Insert new element ---The main problem is that we have to shift all the larger elements forward to make room for the new element to be placed in its correct ordered position. � Its five arguments are: the source array, the index of the first element to be copied from the source array, the destination array, the index in the destination array where the first element is to be copied, and the number of elements to be copied.
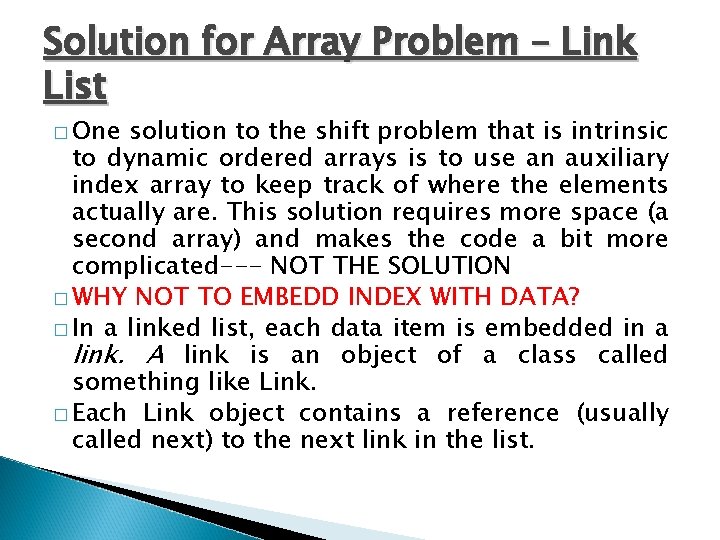
Solution for Array Problem – Link List � One solution to the shift problem that is intrinsic to dynamic ordered arrays is to use an auxiliary index array to keep track of where the elements actually are. This solution requires more space (a second array) and makes the code a bit more complicated--- NOT THE SOLUTION � WHY NOT TO EMBEDD INDEX WITH DATA? � In a linked list, each data item is embedded in a link. A link is an object of a class called something like Link. � Each Link object contains a reference (usually called next) to the next link in the list.
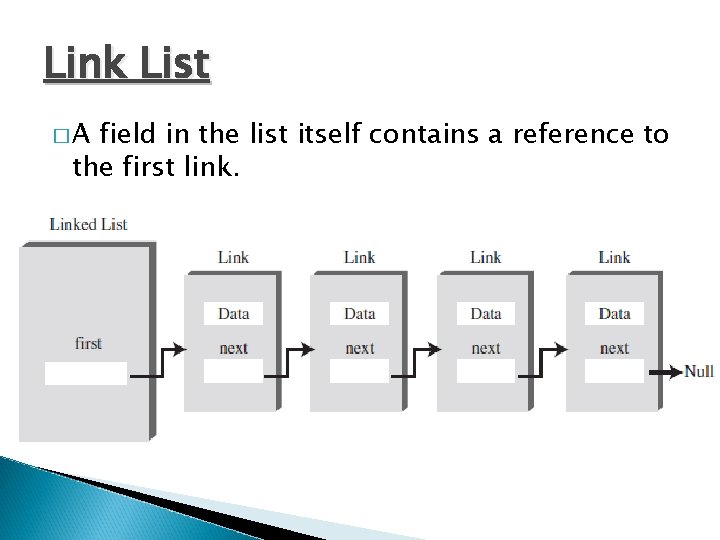
Link List �A field in the list itself contains a reference to the first link.
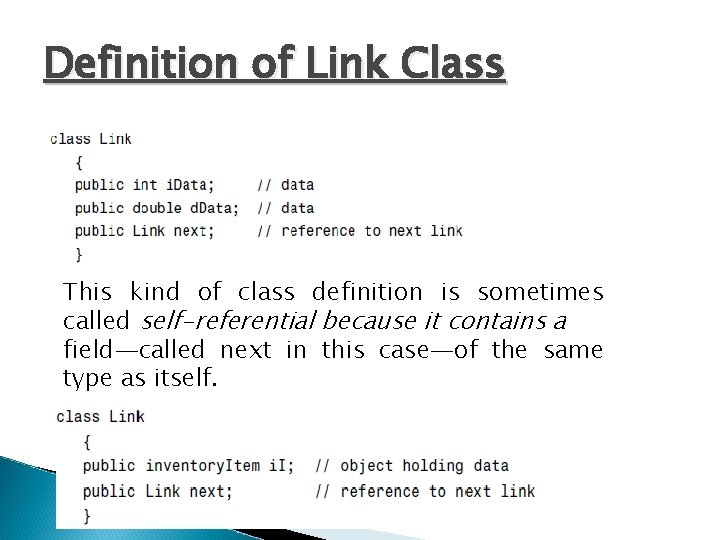
Definition of Link Class This kind of class definition is sometimes called self-referential because it contains a field—called next in this case—of the same type as itself.
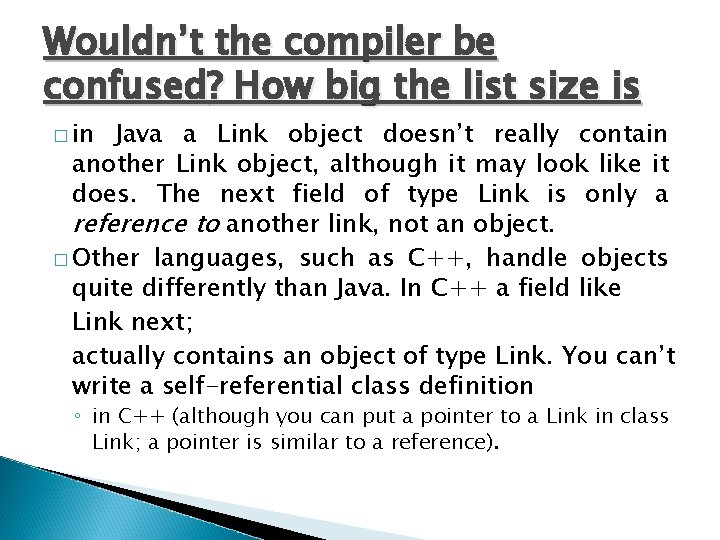
Wouldn’t the compiler be confused? How big the list size is � in Java a Link object doesn’t really contain another Link object, although it may look like it does. The next field of type Link is only a reference to another link, not an object. � Other languages, such as C++, handle objects quite differently than Java. In C++ a field like Link next; actually contains an object of type Link. You can’t write a self-referential class definition ◦ in C++ (although you can put a pointer to a Link in class Link; a pointer is similar to a reference).
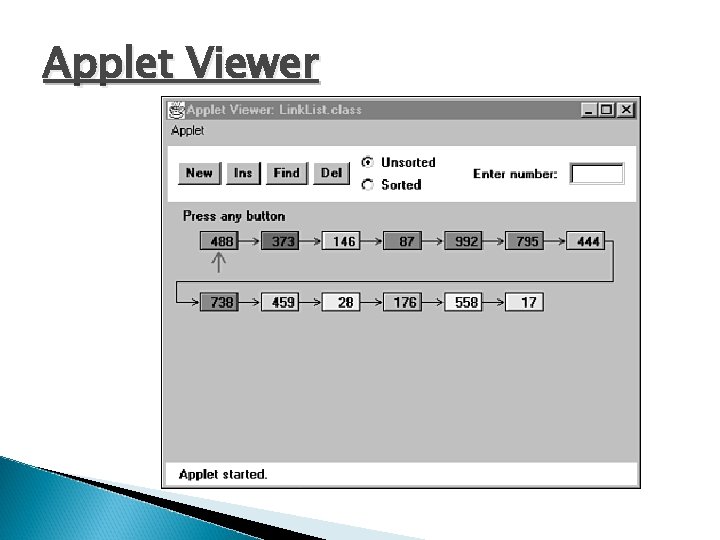
Applet Viewer
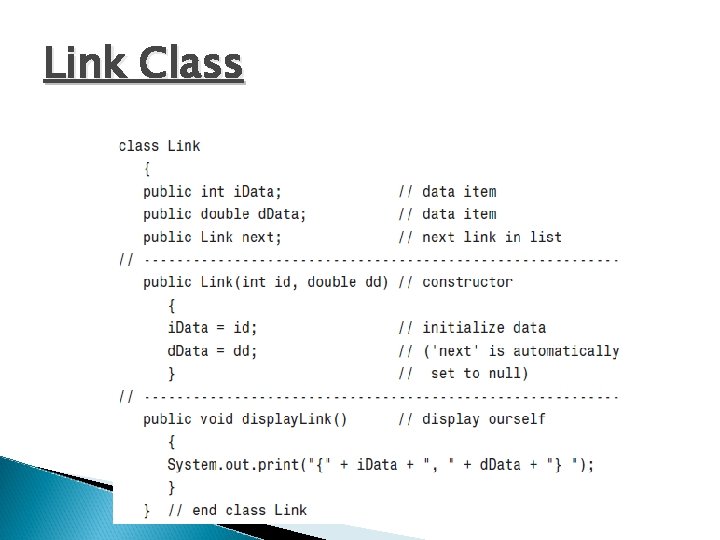
Link Class
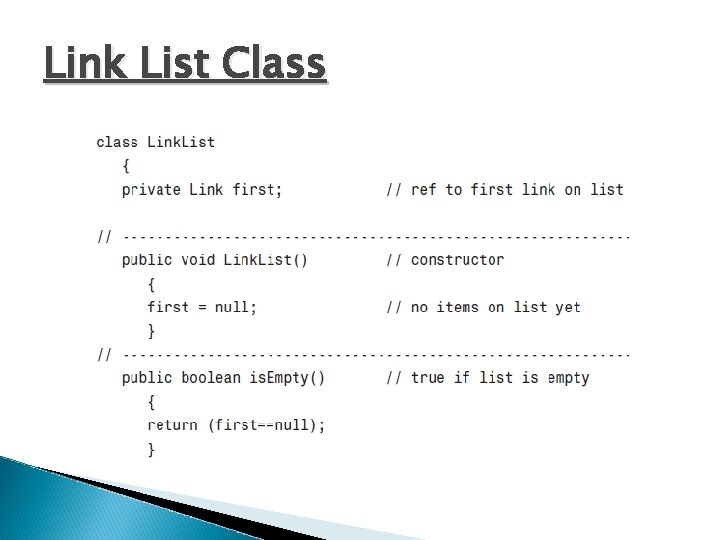
Link List Class
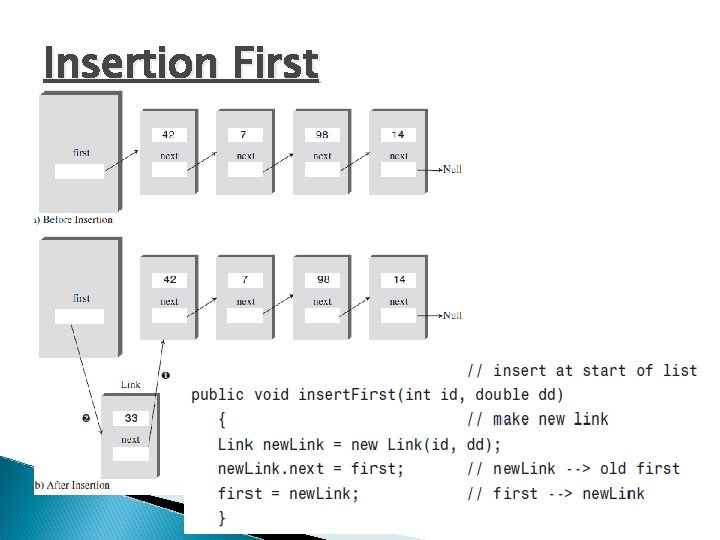
Insertion First
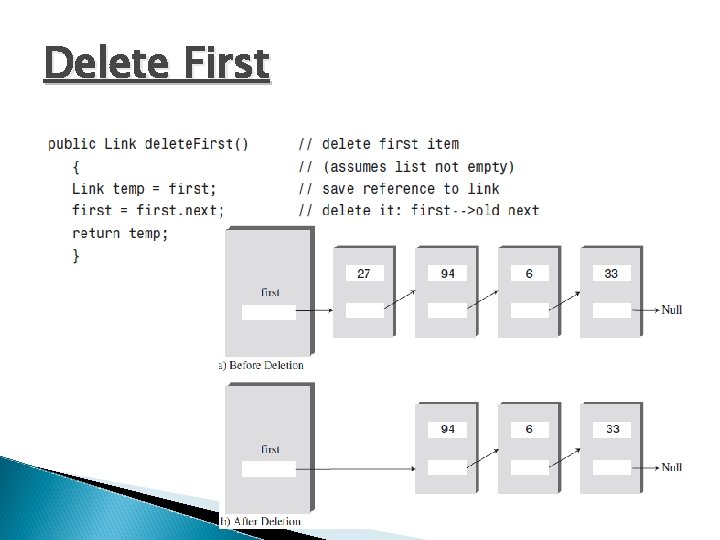
Delete First
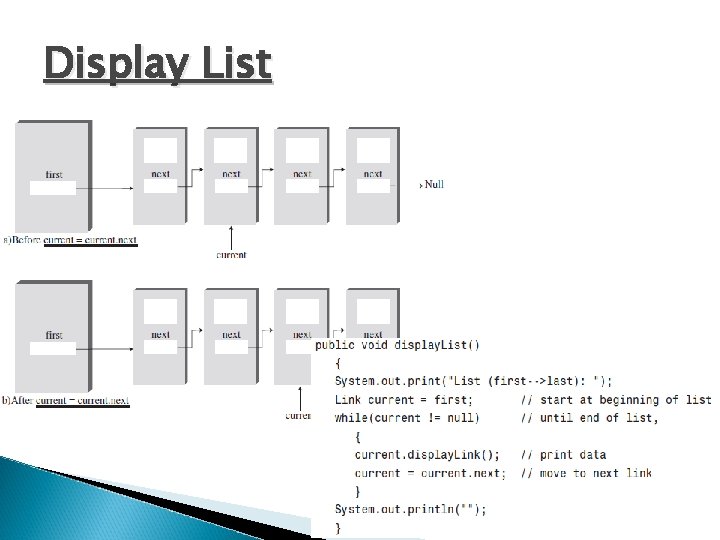
Display List
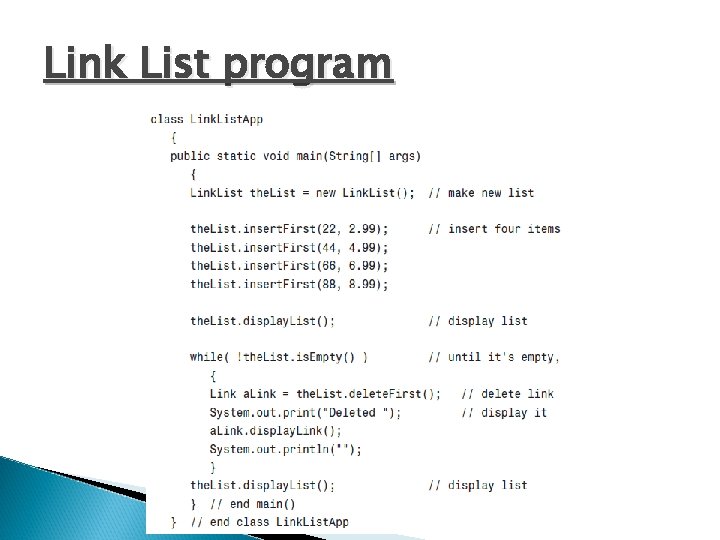
Link List program
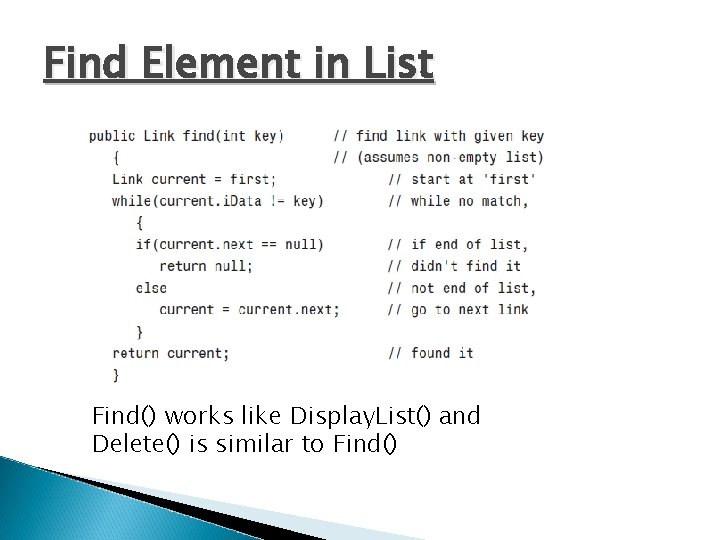
Find Element in List Find() works like Display. List() and Delete() is similar to Find()
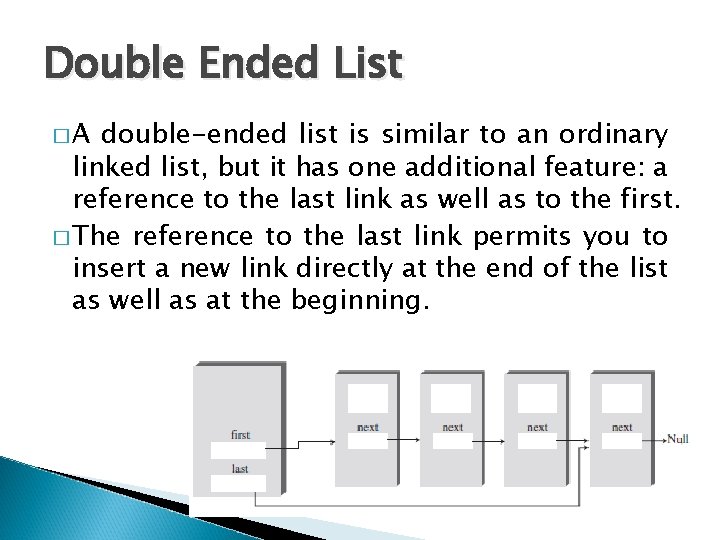
Double Ended List �A double-ended list is similar to an ordinary linked list, but it has one additional feature: a reference to the last link as well as to the first. � The reference to the last link permits you to insert a new link directly at the end of the list as well as at the beginning.
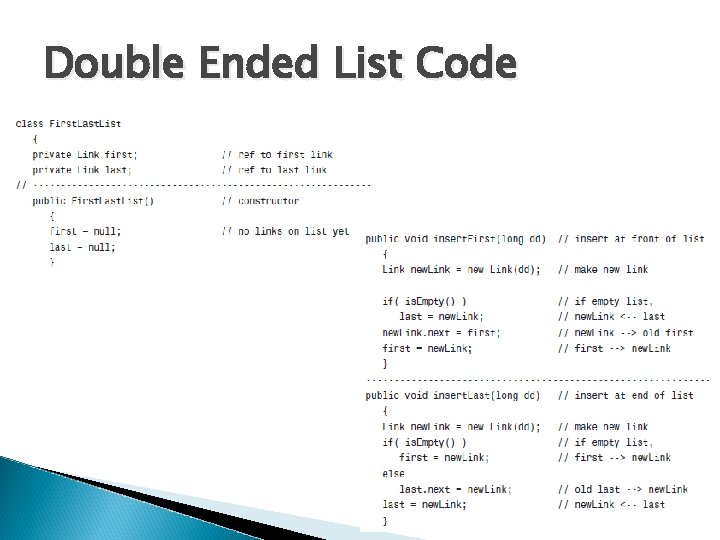
Double Ended List Code
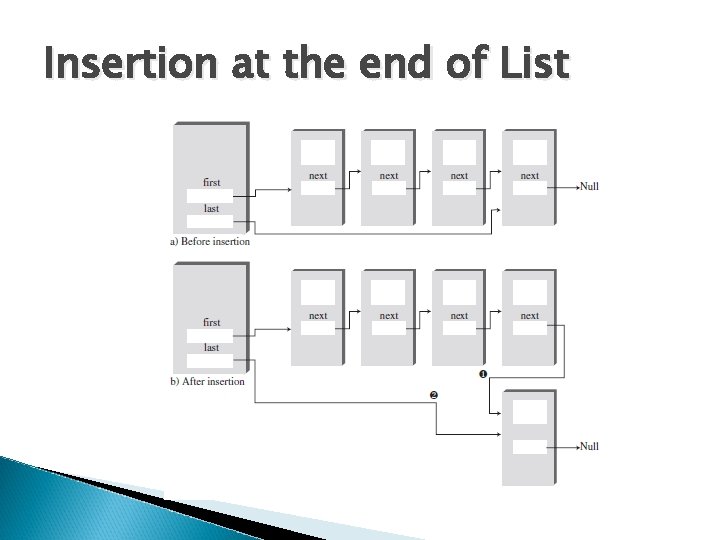
Insertion at the end of List
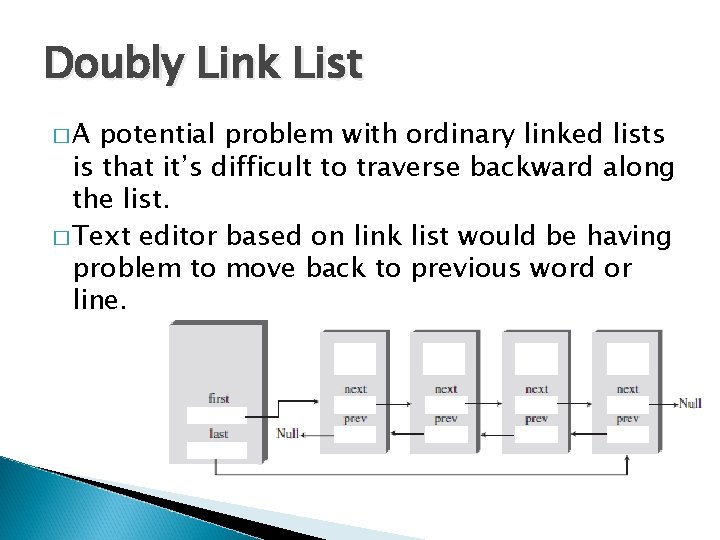
Doubly Link List �A potential problem with ordinary linked lists is that it’s difficult to traverse backward along the list. � Text editor based on link list would be having problem to move back to previous word or line.
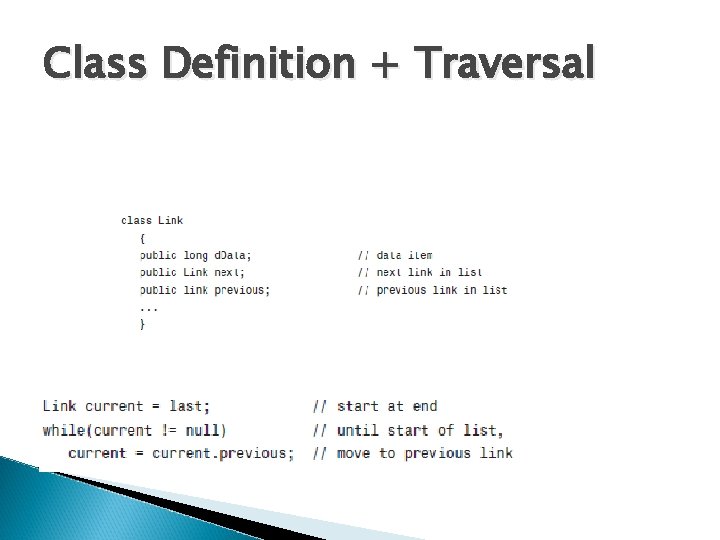
Class Definition + Traversal
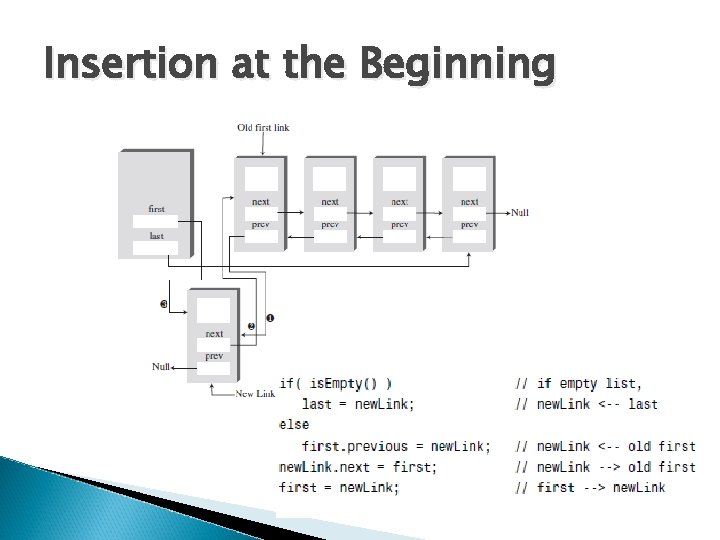
Insertion at the Beginning
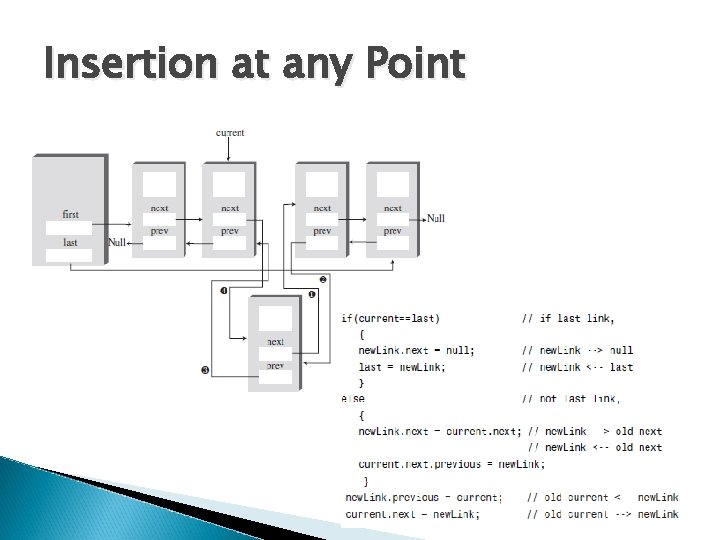
Insertion at any Point
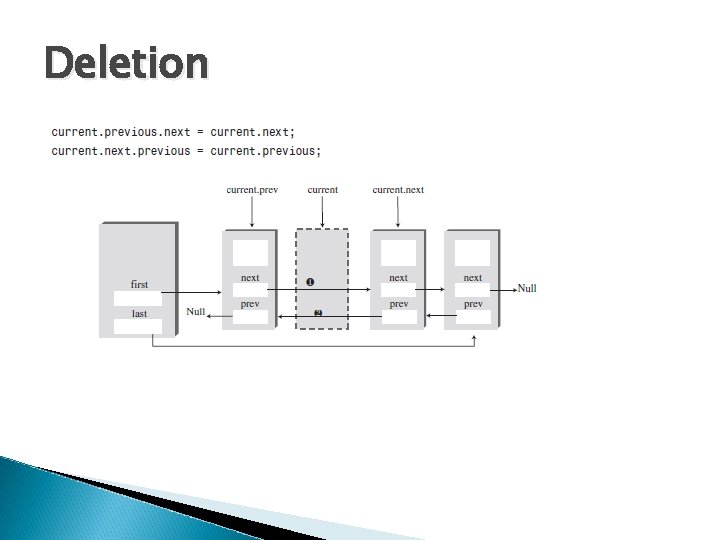
Deletion
Singly linked list vs doubly linked list
Singly vs doubly linked list
List adalah
Java data structures
Polynomial multiplication using linked list in c
Polynomial addition in data structure
Circular linked list advantages
Disadvantages of doubly linked list
Disadvantages of random access memory
Advantages and disadvantages of doubly linked list
Linked list
Advantages of linked list
Smart open
Site:.com "fill link item" "add link"
Stack is a static data structure
Generic linked list in c
Jenis linked list
Singly vs doubly linked list
Market basket analysis
Xor linked list
Traverse a linked list c++
Linked list in mips
Linked list definition
Linked list uml
Language