CSCI 171 Presentation 12 Files Working with files
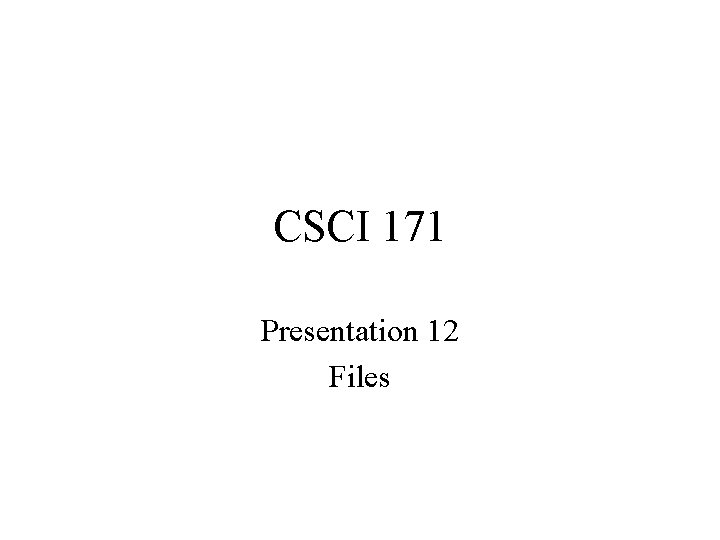
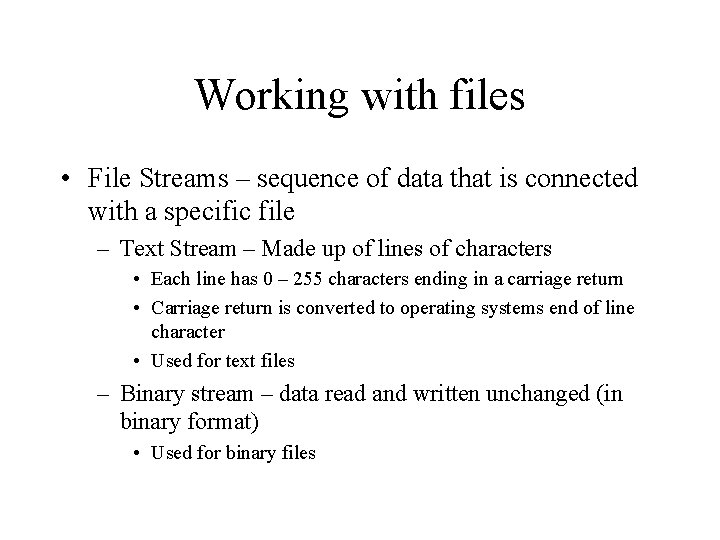
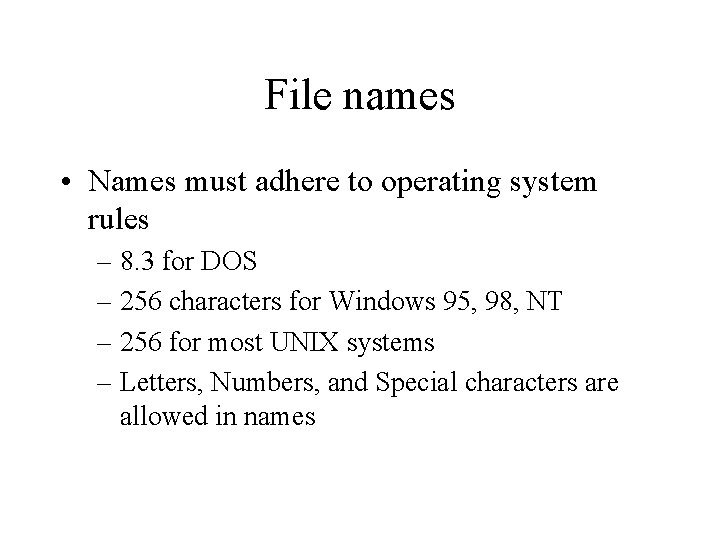
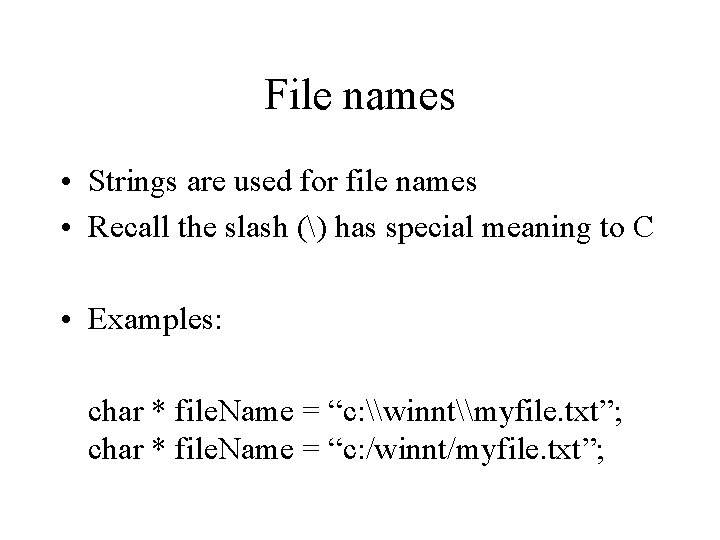
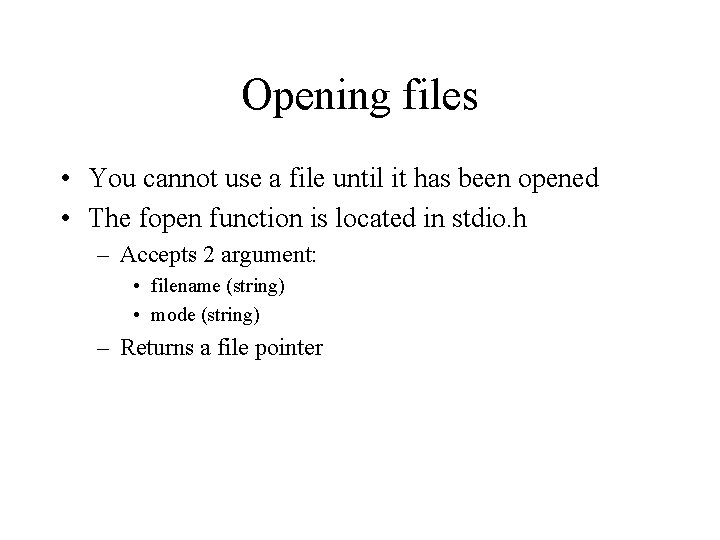
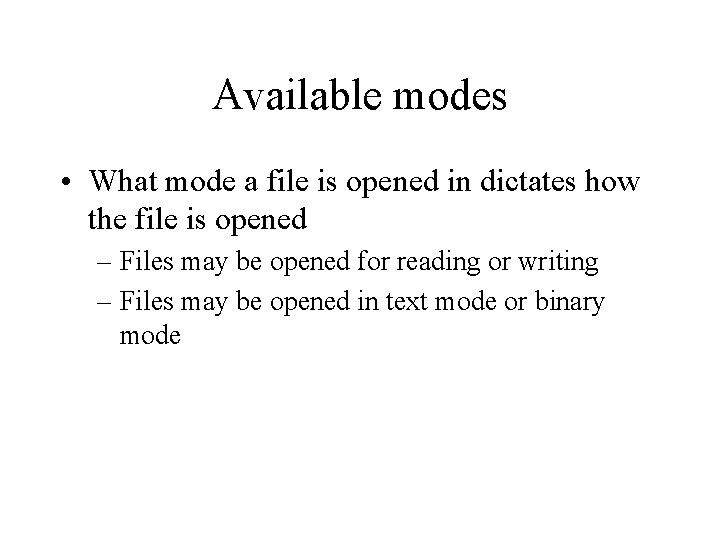
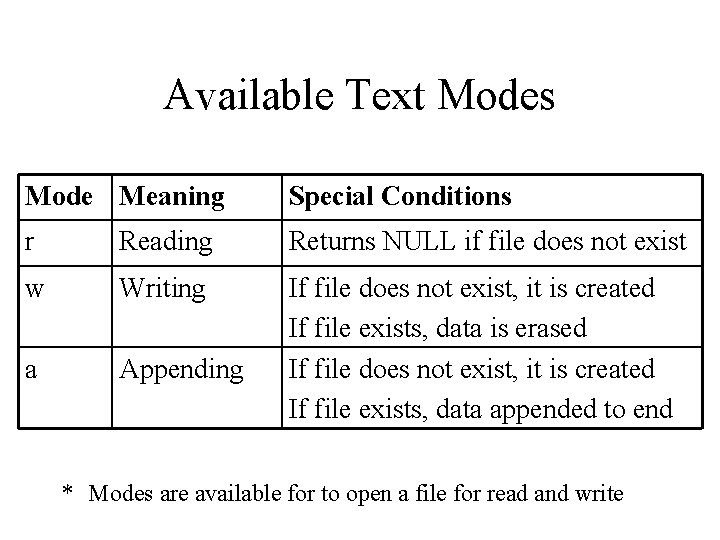
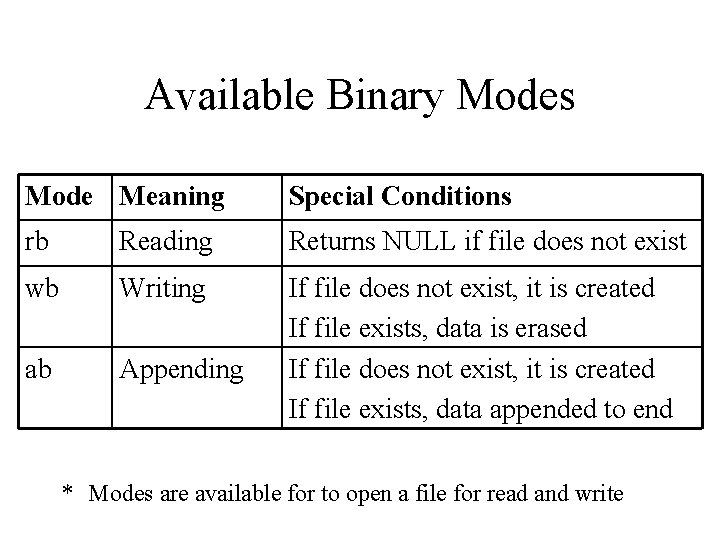
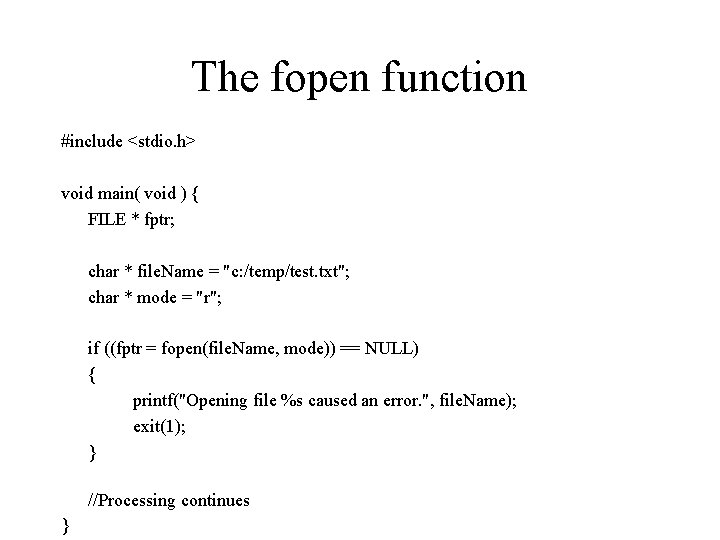
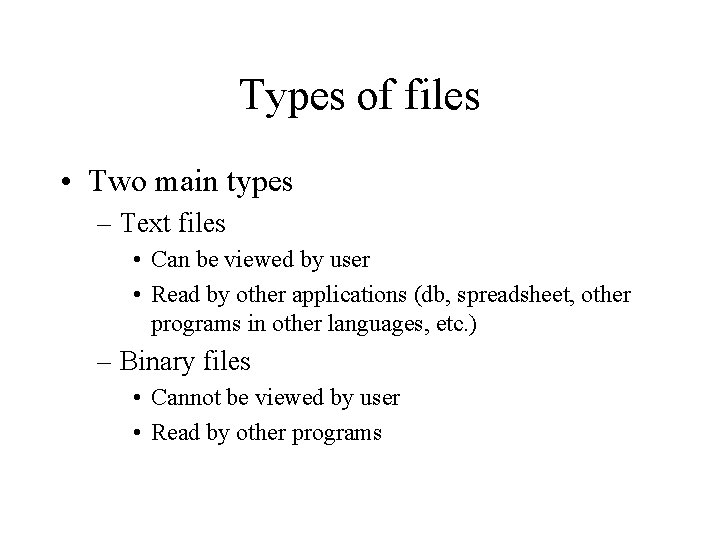
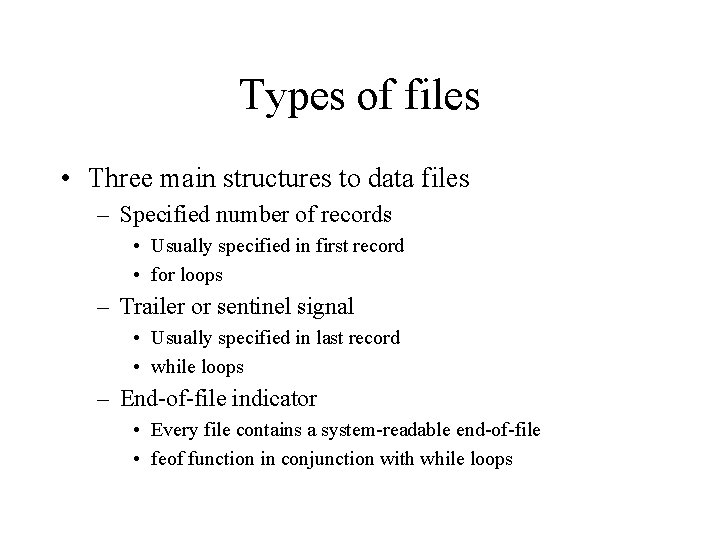
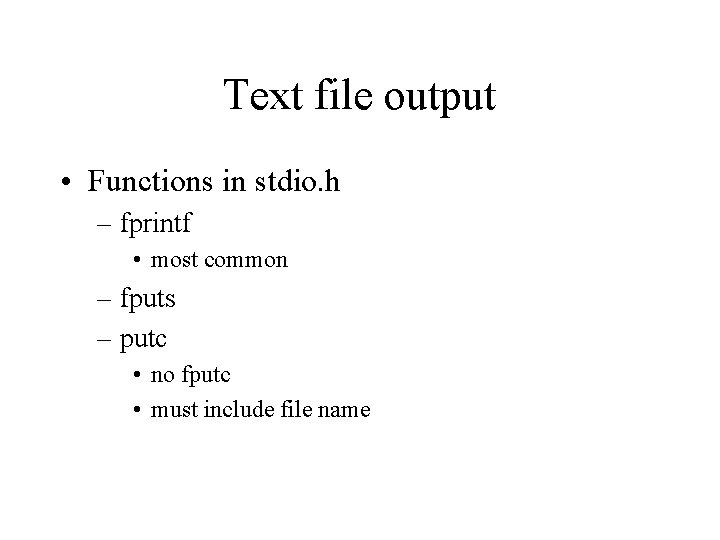
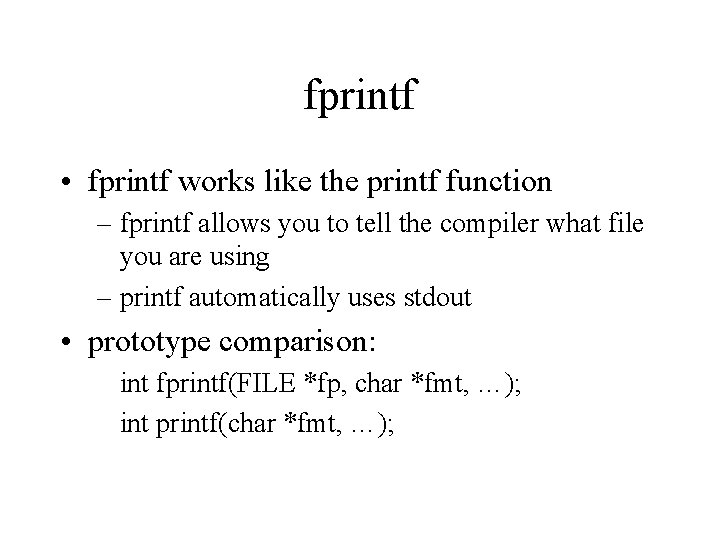
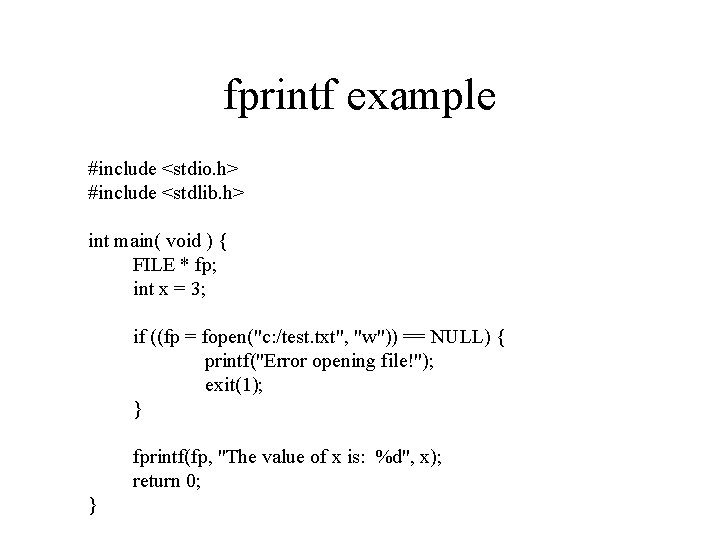
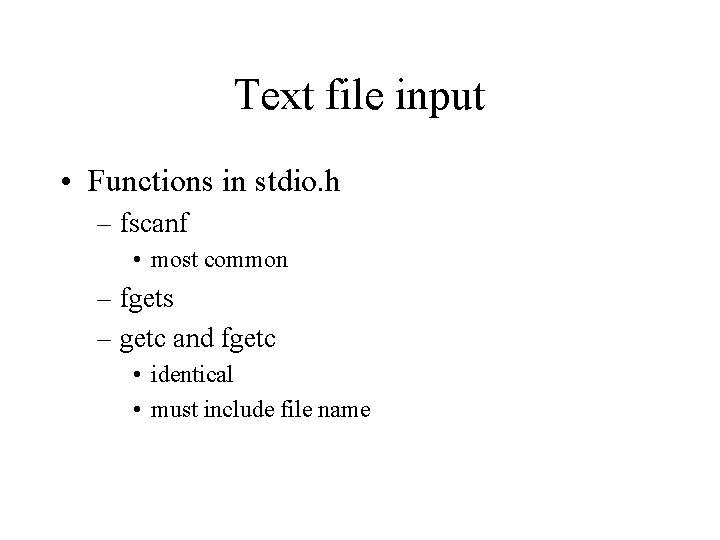
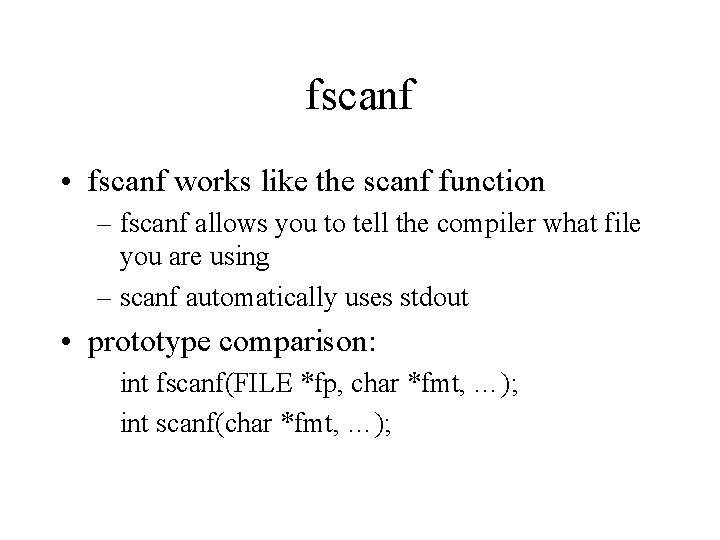
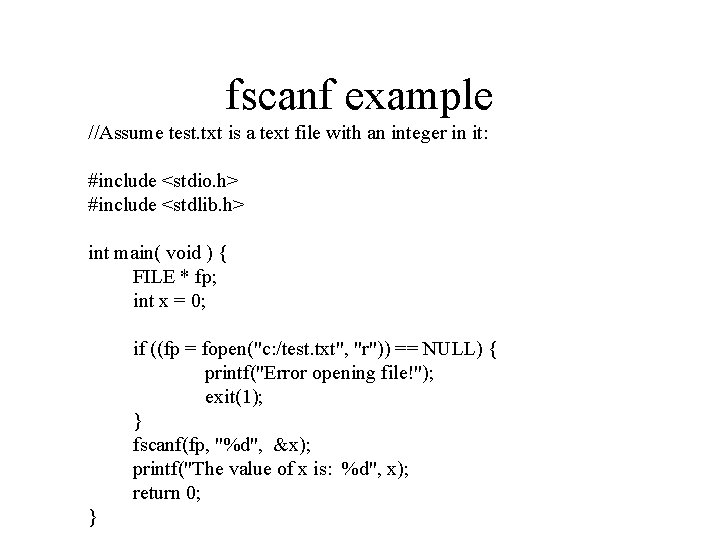
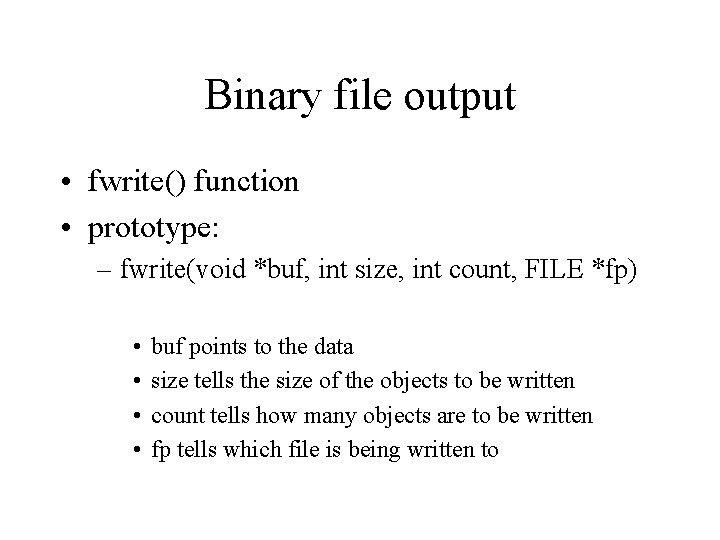
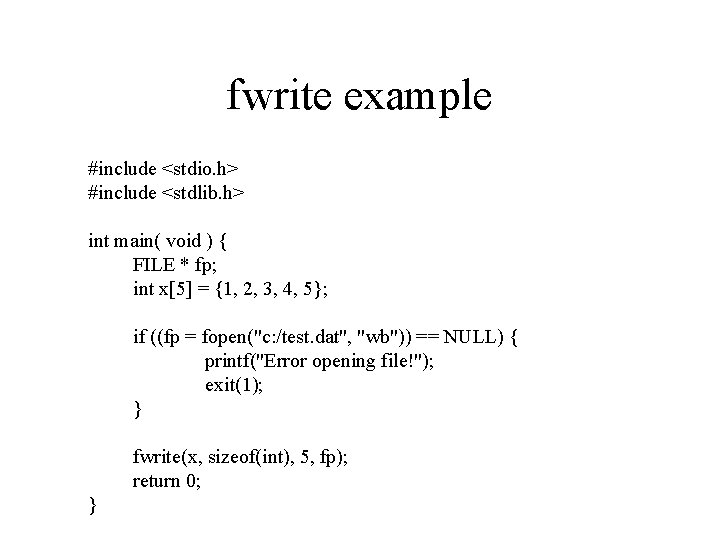
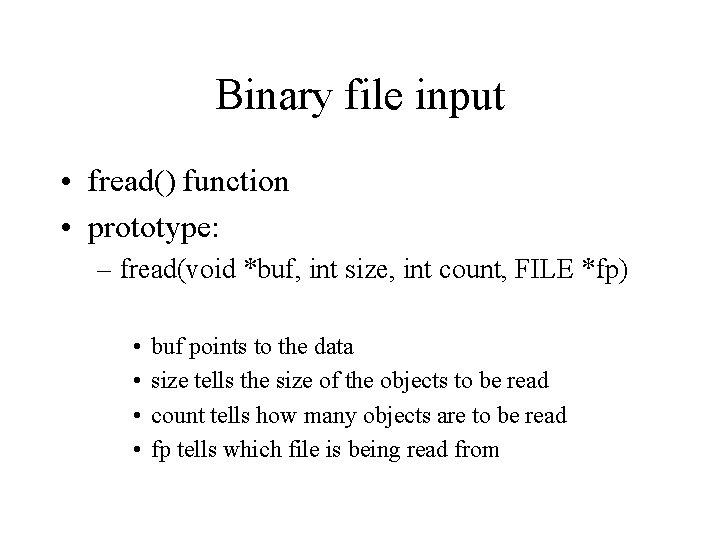
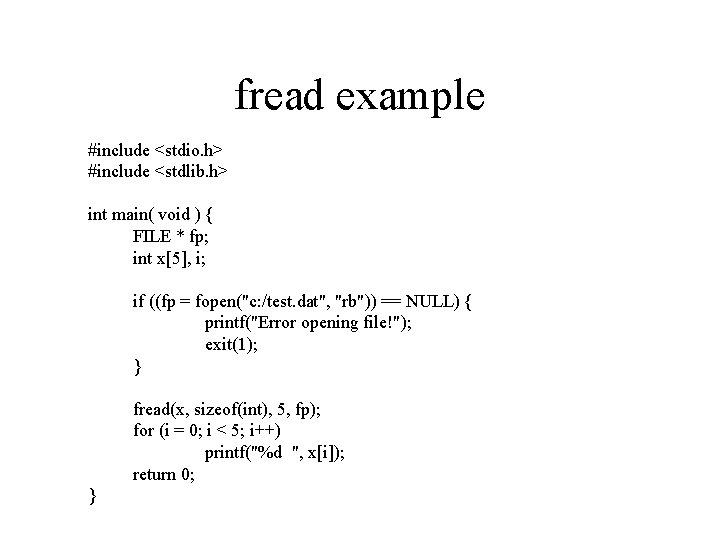
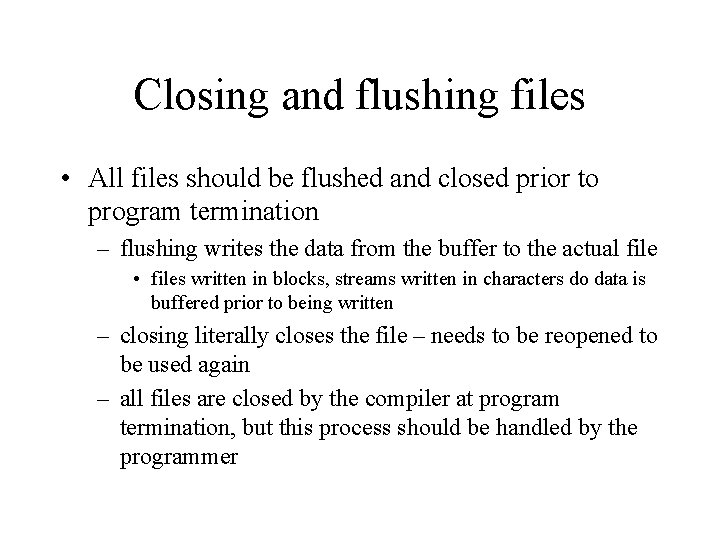
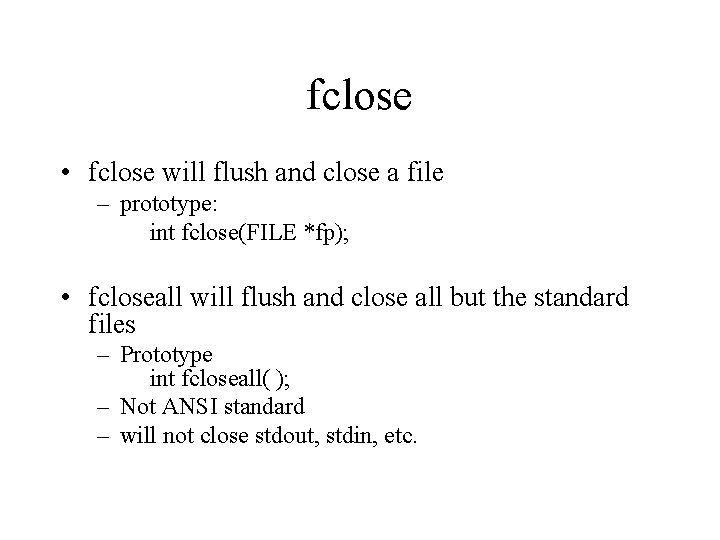
- Slides: 23
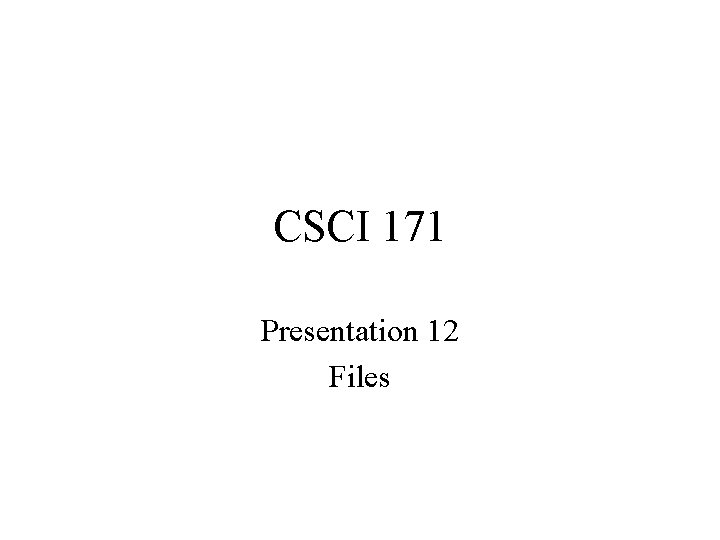
CSCI 171 Presentation 12 Files
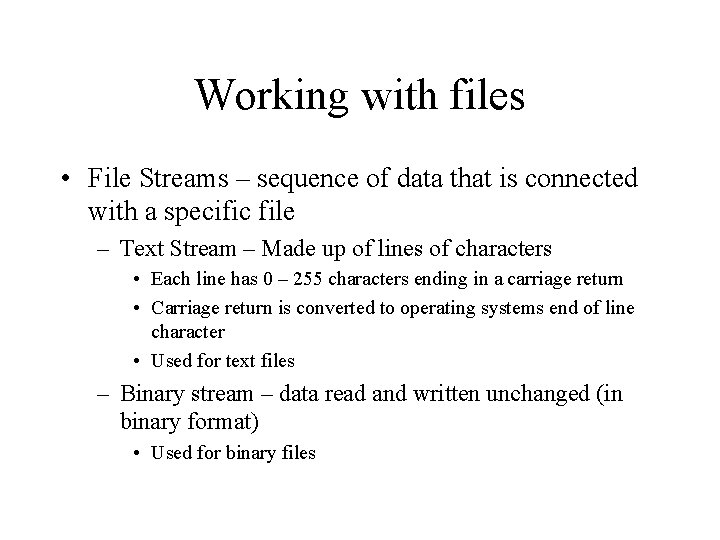
Working with files • File Streams – sequence of data that is connected with a specific file – Text Stream – Made up of lines of characters • Each line has 0 – 255 characters ending in a carriage return • Carriage return is converted to operating systems end of line character • Used for text files – Binary stream – data read and written unchanged (in binary format) • Used for binary files
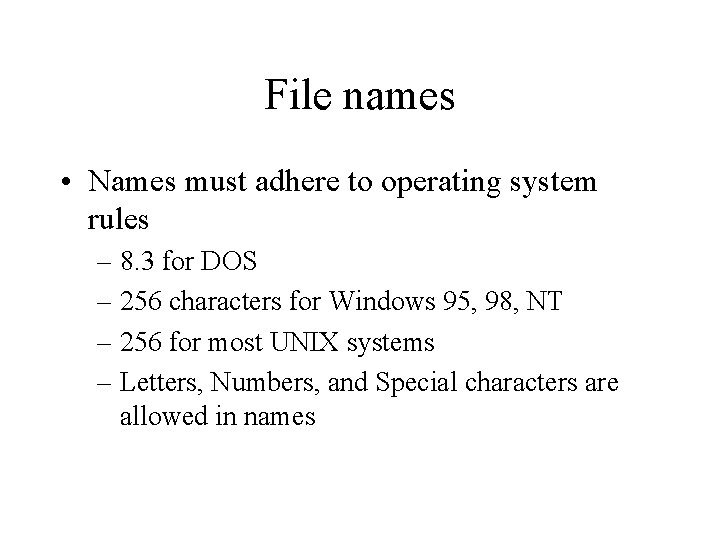
File names • Names must adhere to operating system rules – 8. 3 for DOS – 256 characters for Windows 95, 98, NT – 256 for most UNIX systems – Letters, Numbers, and Special characters are allowed in names
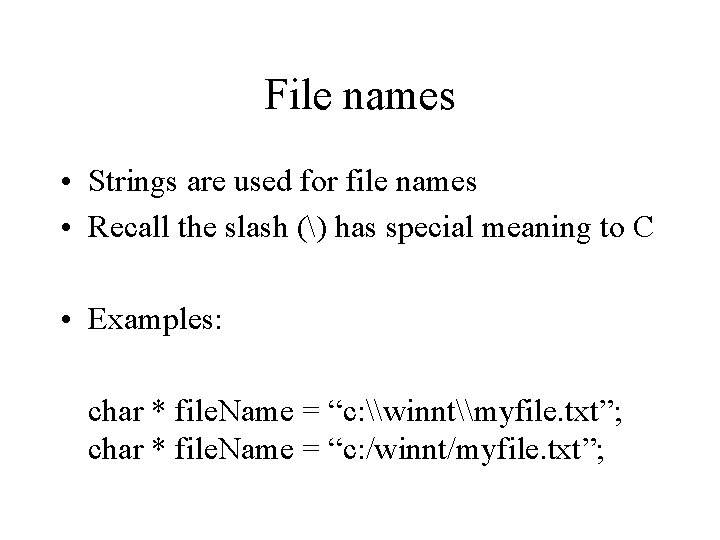
File names • Strings are used for file names • Recall the slash () has special meaning to C • Examples: char * file. Name = “c: \winnt\myfile. txt”; char * file. Name = “c: /winnt/myfile. txt”;
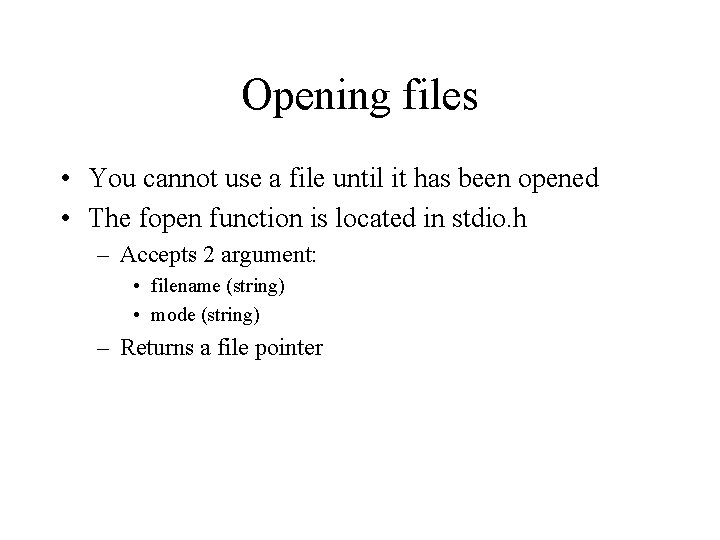
Opening files • You cannot use a file until it has been opened • The fopen function is located in stdio. h – Accepts 2 argument: • filename (string) • mode (string) – Returns a file pointer
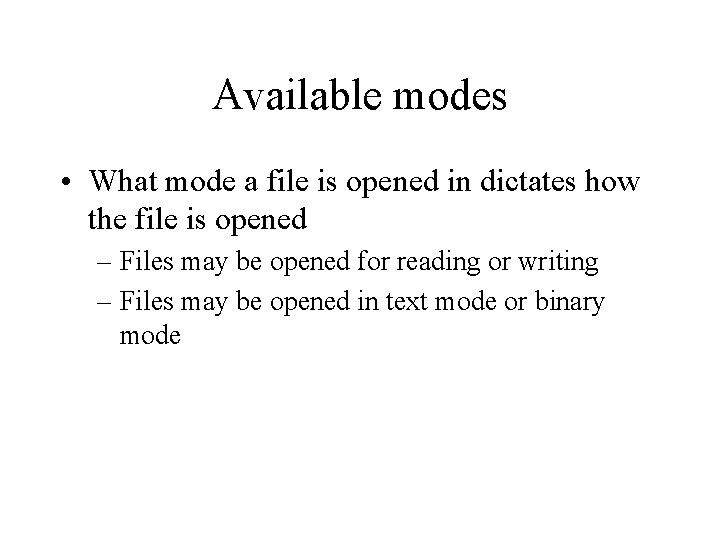
Available modes • What mode a file is opened in dictates how the file is opened – Files may be opened for reading or writing – Files may be opened in text mode or binary mode
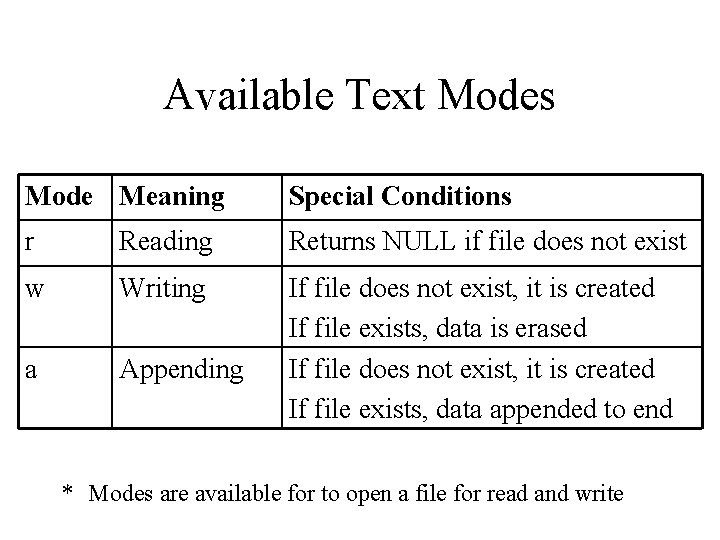
Available Text Modes Mode Meaning Special Conditions r Reading Returns NULL if file does not exist w Writing a Appending If file does not exist, it is created If file exists, data is erased If file does not exist, it is created If file exists, data appended to end * Modes are available for to open a file for read and write
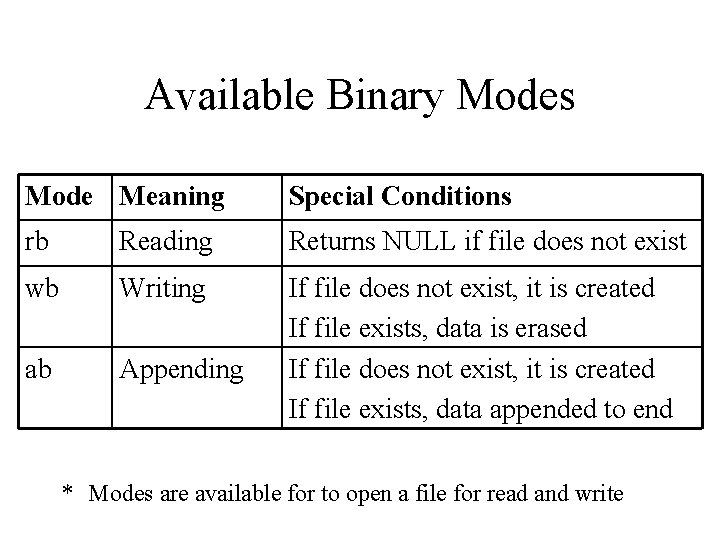
Available Binary Modes Mode Meaning Special Conditions rb Reading Returns NULL if file does not exist wb Writing ab Appending If file does not exist, it is created If file exists, data is erased If file does not exist, it is created If file exists, data appended to end * Modes are available for to open a file for read and write
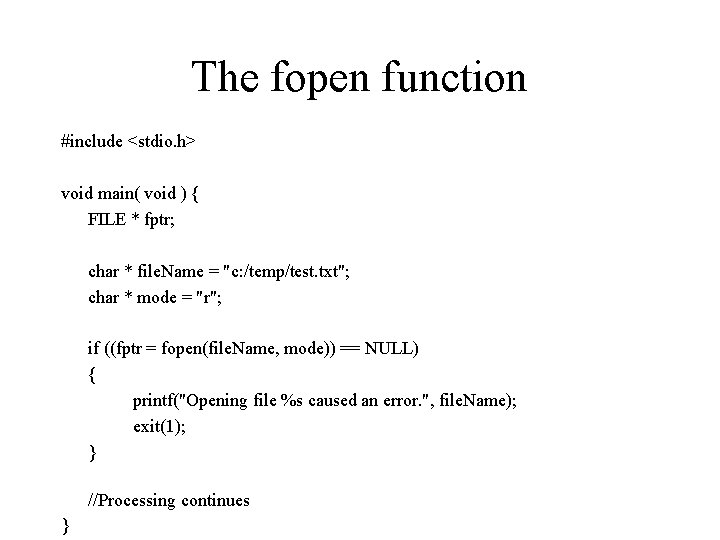
The fopen function #include <stdio. h> void main( void ) { FILE * fptr; char * file. Name = "c: /temp/test. txt"; char * mode = "r"; if ((fptr = fopen(file. Name, mode)) == NULL) { printf("Opening file %s caused an error. ", file. Name); exit(1); } //Processing continues }
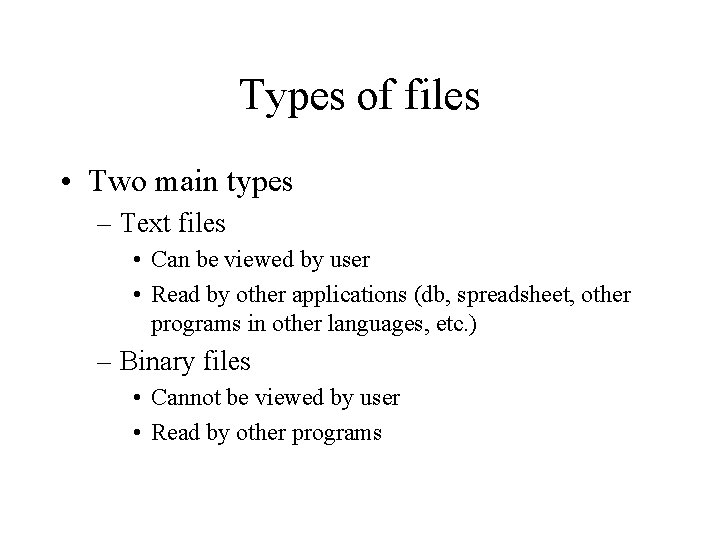
Types of files • Two main types – Text files • Can be viewed by user • Read by other applications (db, spreadsheet, other programs in other languages, etc. ) – Binary files • Cannot be viewed by user • Read by other programs
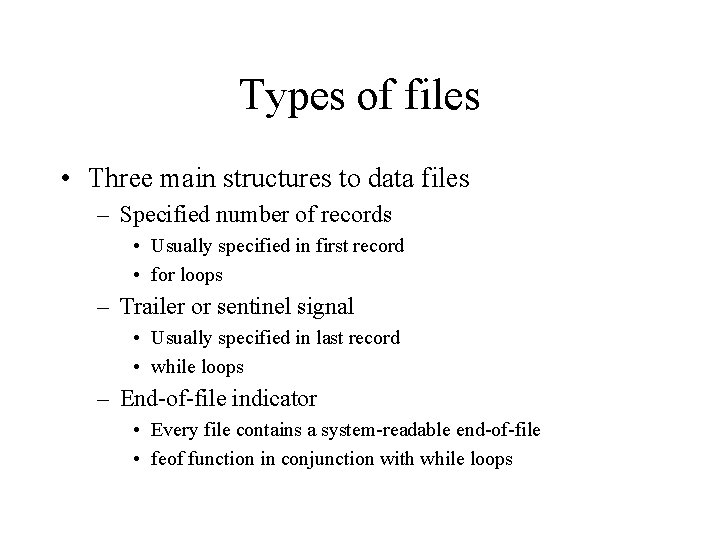
Types of files • Three main structures to data files – Specified number of records • Usually specified in first record • for loops – Trailer or sentinel signal • Usually specified in last record • while loops – End-of-file indicator • Every file contains a system-readable end-of-file • feof function in conjunction with while loops
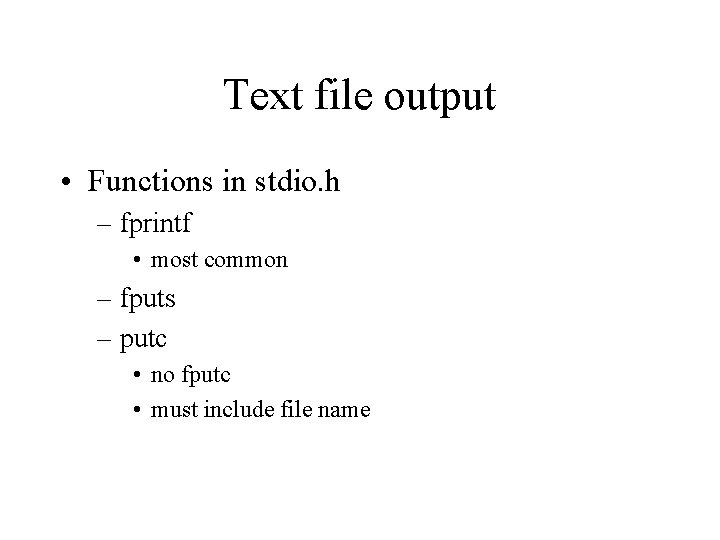
Text file output • Functions in stdio. h – fprintf • most common – fputs – putc • no fputc • must include file name
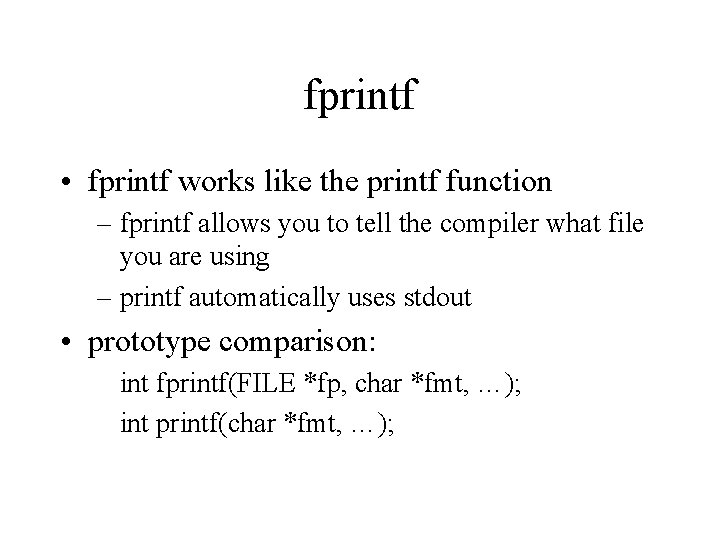
fprintf • fprintf works like the printf function – fprintf allows you to tell the compiler what file you are using – printf automatically uses stdout • prototype comparison: int fprintf(FILE *fp, char *fmt, …); int printf(char *fmt, …);
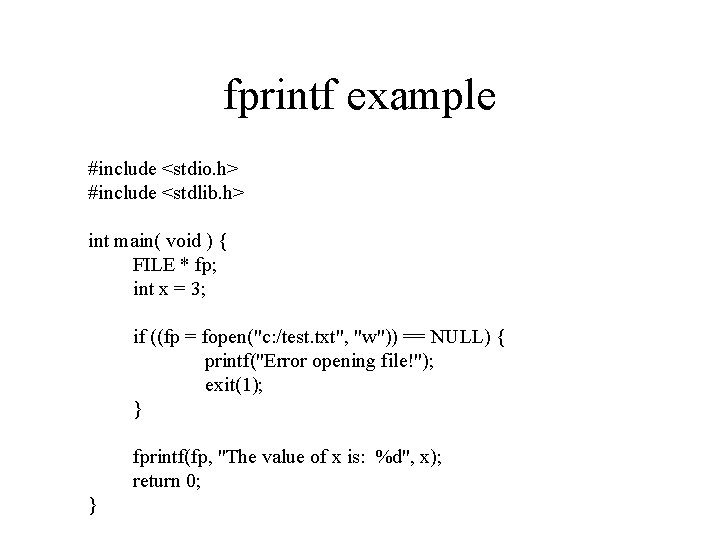
fprintf example #include <stdio. h> #include <stdlib. h> int main( void ) { FILE * fp; int x = 3; if ((fp = fopen("c: /test. txt", "w")) == NULL) { printf("Error opening file!"); exit(1); } fprintf(fp, "The value of x is: %d", x); return 0; }
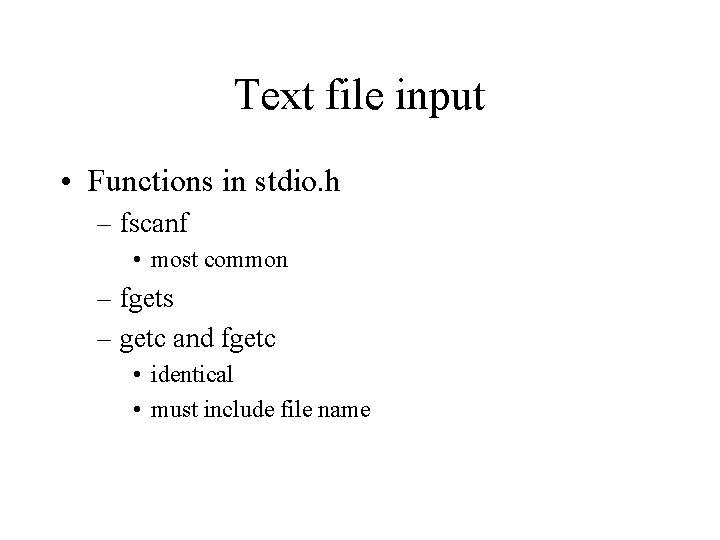
Text file input • Functions in stdio. h – fscanf • most common – fgets – getc and fgetc • identical • must include file name
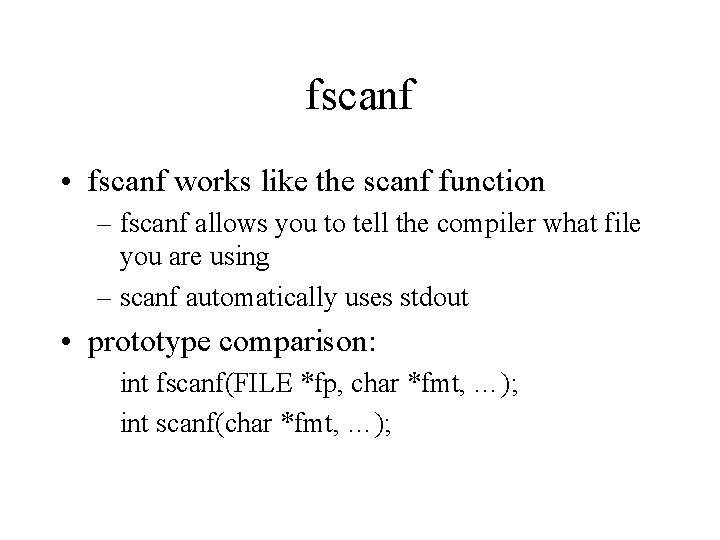
fscanf • fscanf works like the scanf function – fscanf allows you to tell the compiler what file you are using – scanf automatically uses stdout • prototype comparison: int fscanf(FILE *fp, char *fmt, …); int scanf(char *fmt, …);
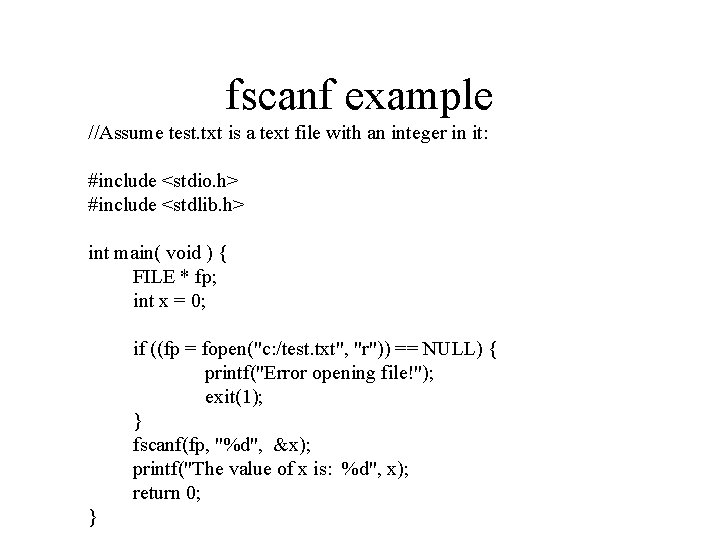
fscanf example //Assume test. txt is a text file with an integer in it: #include <stdio. h> #include <stdlib. h> int main( void ) { FILE * fp; int x = 0; if ((fp = fopen("c: /test. txt", "r")) == NULL) { printf("Error opening file!"); exit(1); } fscanf(fp, "%d", &x); printf("The value of x is: %d", x); return 0; }
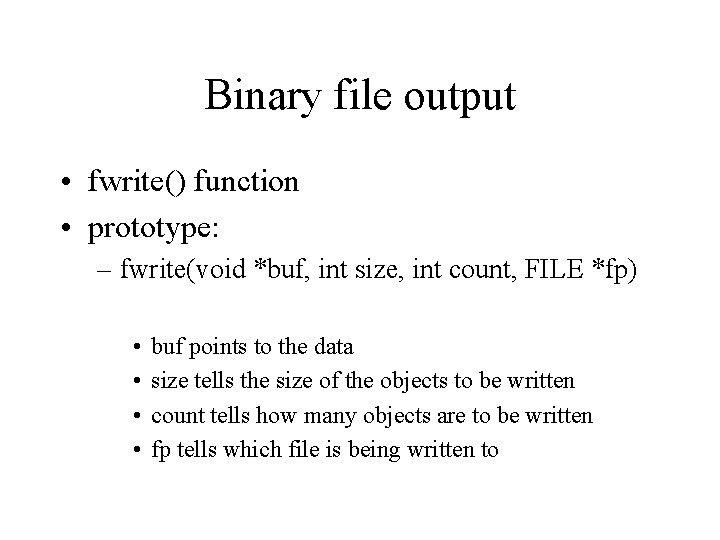
Binary file output • fwrite() function • prototype: – fwrite(void *buf, int size, int count, FILE *fp) • • buf points to the data size tells the size of the objects to be written count tells how many objects are to be written fp tells which file is being written to
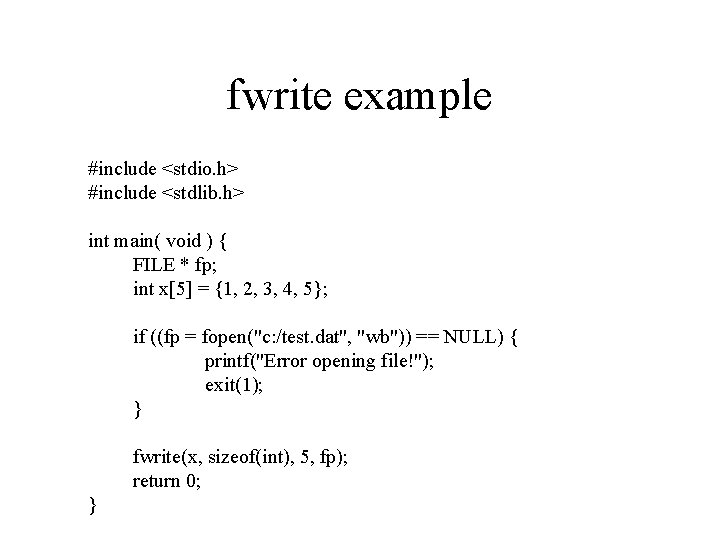
fwrite example #include <stdio. h> #include <stdlib. h> int main( void ) { FILE * fp; int x[5] = {1, 2, 3, 4, 5}; if ((fp = fopen("c: /test. dat", "wb")) == NULL) { printf("Error opening file!"); exit(1); } fwrite(x, sizeof(int), 5, fp); return 0; }
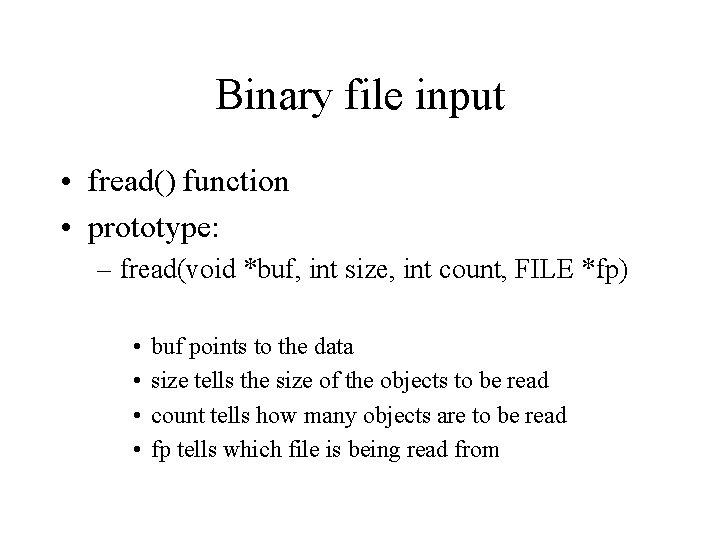
Binary file input • fread() function • prototype: – fread(void *buf, int size, int count, FILE *fp) • • buf points to the data size tells the size of the objects to be read count tells how many objects are to be read fp tells which file is being read from
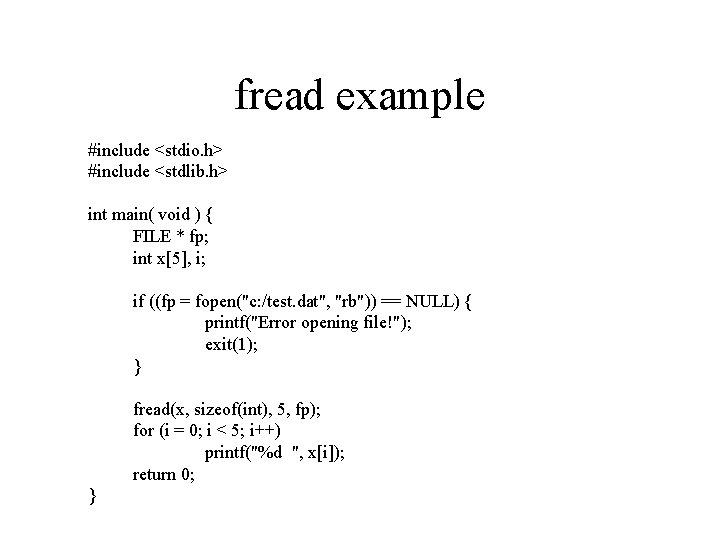
fread example #include <stdio. h> #include <stdlib. h> int main( void ) { FILE * fp; int x[5], i; if ((fp = fopen("c: /test. dat", "rb")) == NULL) { printf("Error opening file!"); exit(1); } fread(x, sizeof(int), 5, fp); for (i = 0; i < 5; i++) printf("%d ", x[i]); return 0; }
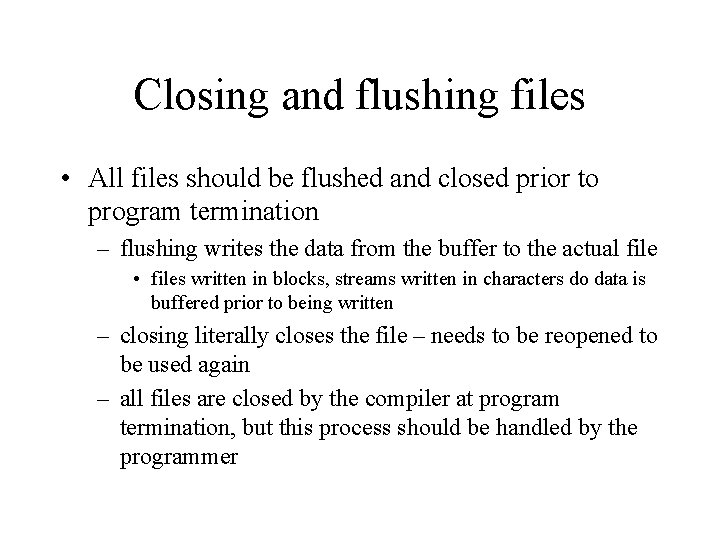
Closing and flushing files • All files should be flushed and closed prior to program termination – flushing writes the data from the buffer to the actual file • files written in blocks, streams written in characters do data is buffered prior to being written – closing literally closes the file – needs to be reopened to be used again – all files are closed by the compiler at program termination, but this process should be handled by the programmer
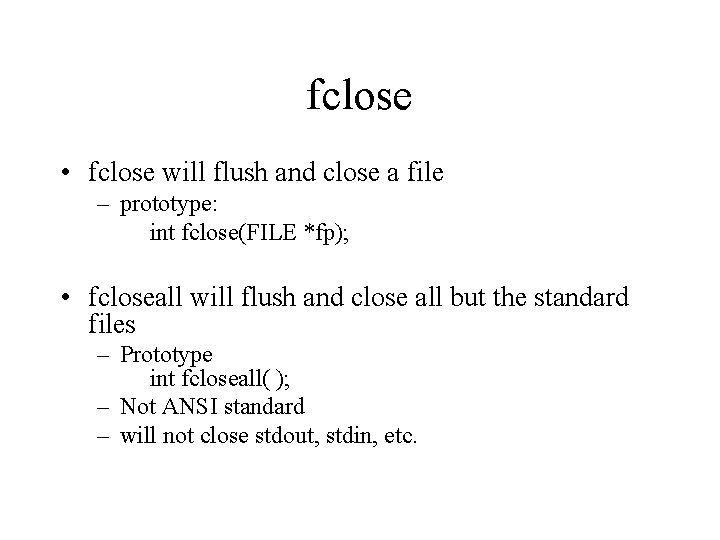
fclose • fclose will flush and close a file – prototype: int fclose(FILE *fp); • fcloseall will flush and close all but the standard files – Prototype int fcloseall( ); – Not ANSI standard – will not close stdout, stdin, etc.
Cjis security levels
Ncic hosts restricted files and non-restricted files
File mode python
171 nomreli mekteb
29052007
Ai 171
171 in binary
Decret 171/2015
The verbs salir decir and venir p 155 answers
Tax allowance meaning
171 sayli mekteb
49 cfr parts 171-179
171
171 nomreli mekteb
171 sayli mekteb
Pg 171
Cs 171 uci
Ai 171
Hard work vs smart work
Prinsip dasar proses pengerjaan panas yang benar adalah
Hot working and cold working
Hot working and cold working difference
Machining operations
Work life balance presentation