CSCE 4813 COMPUTER GRAPHICS PROF JOHN GAUCH HIDDEN
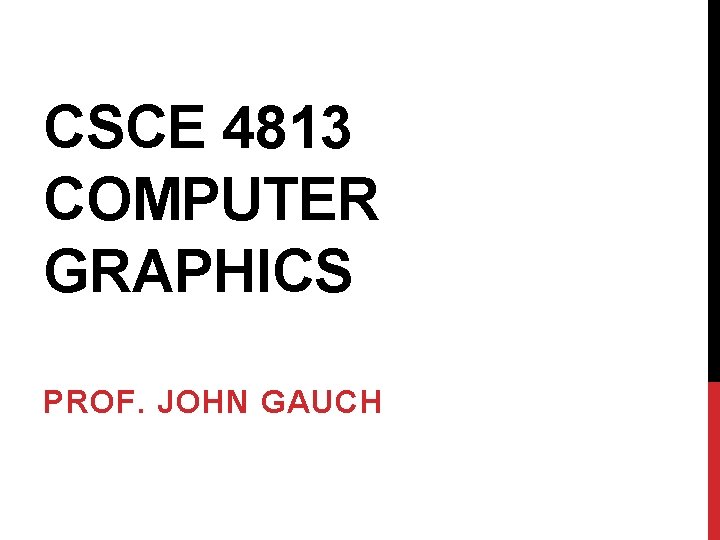
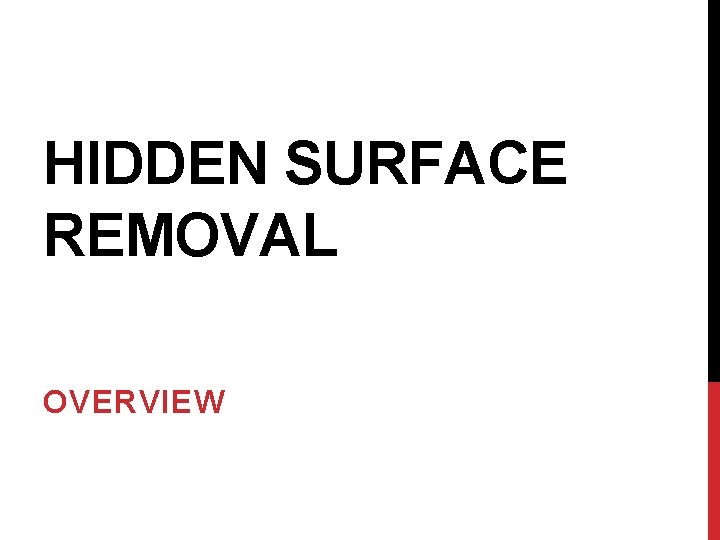
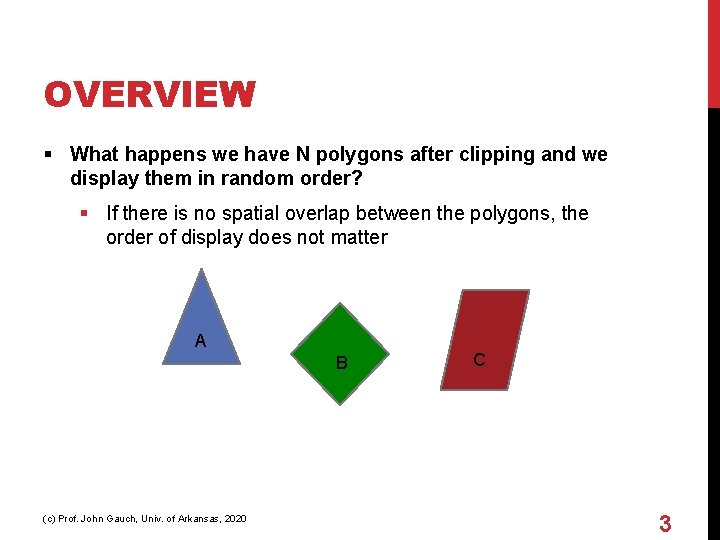
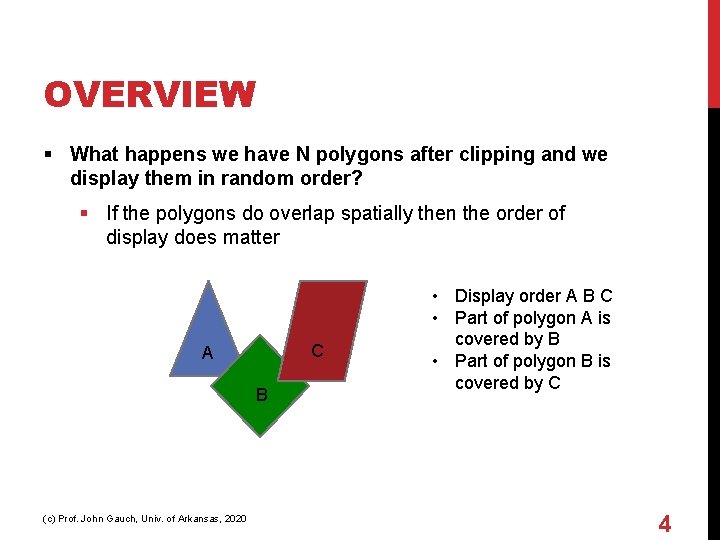
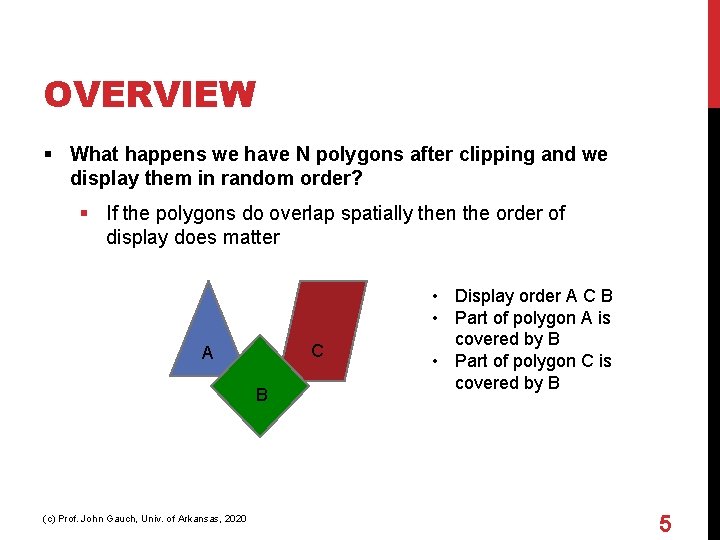
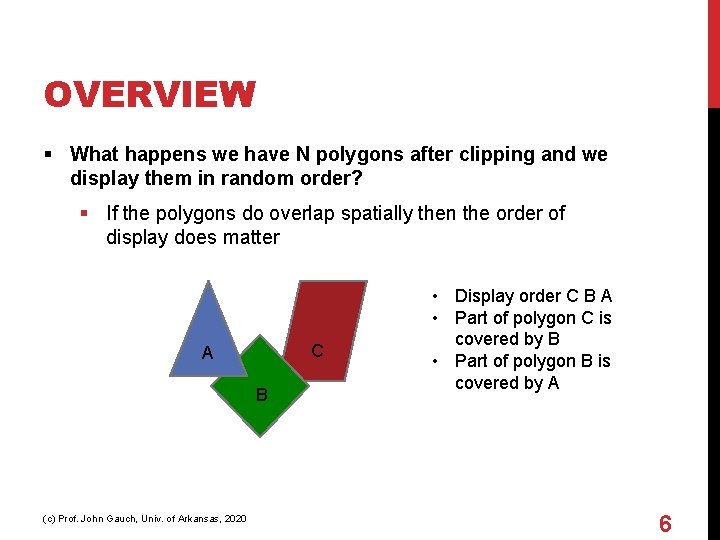
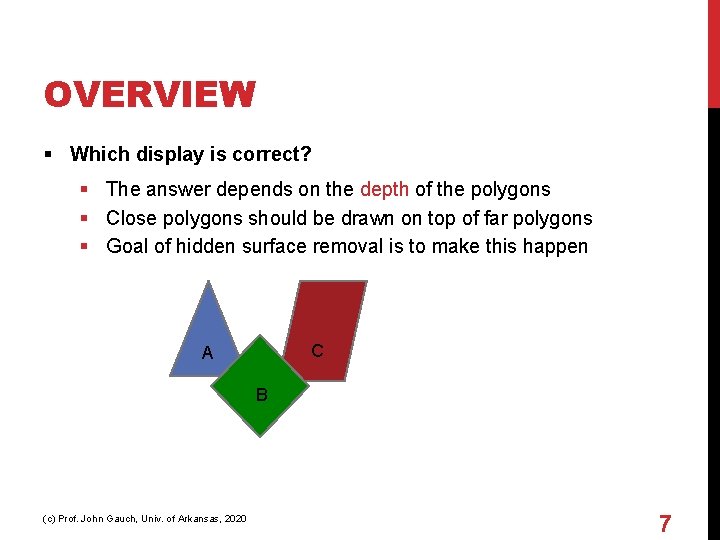
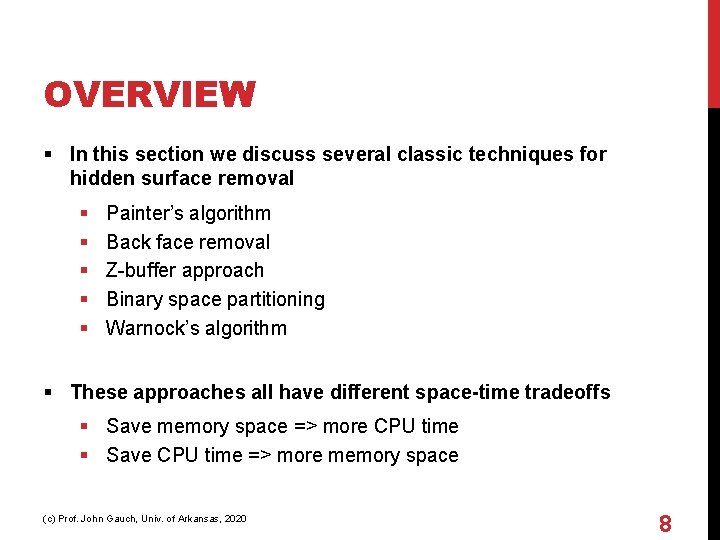
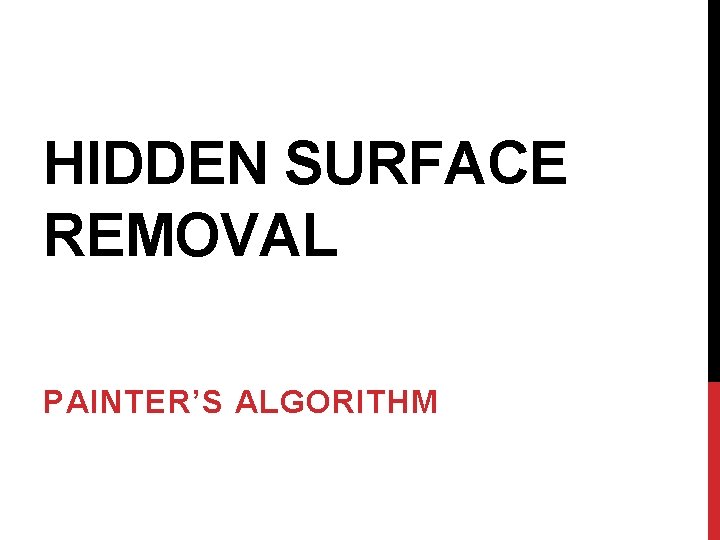
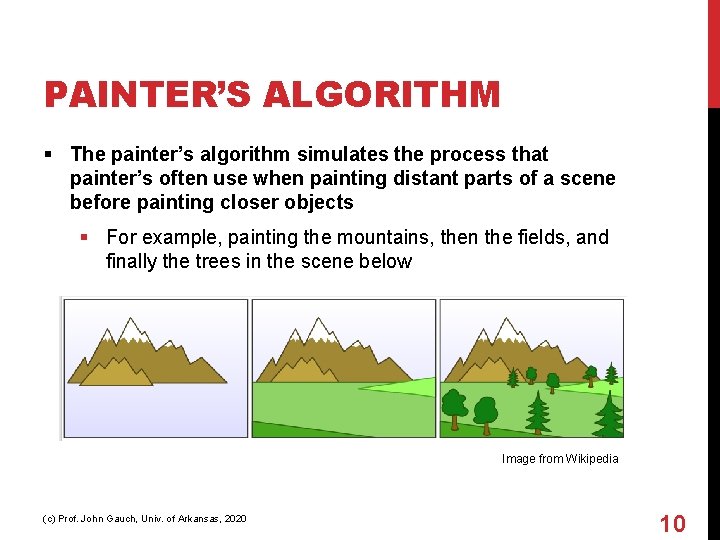
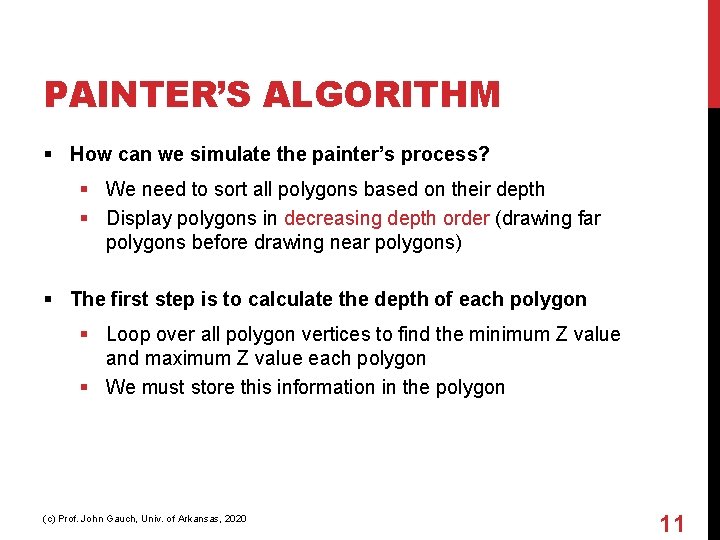
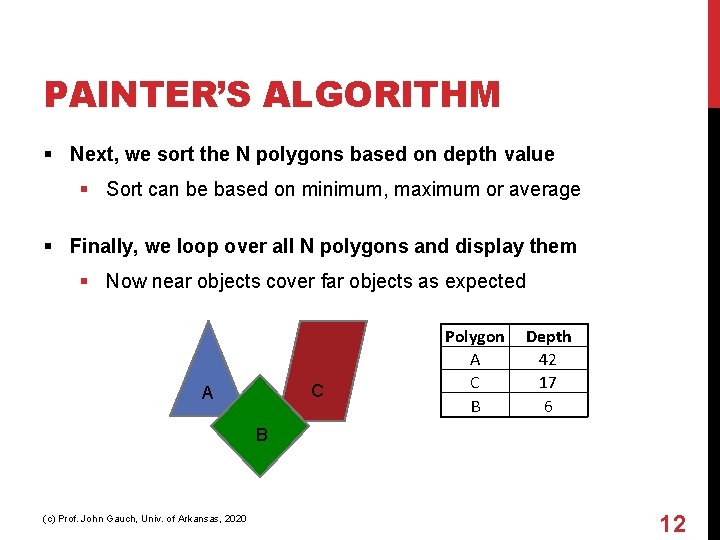
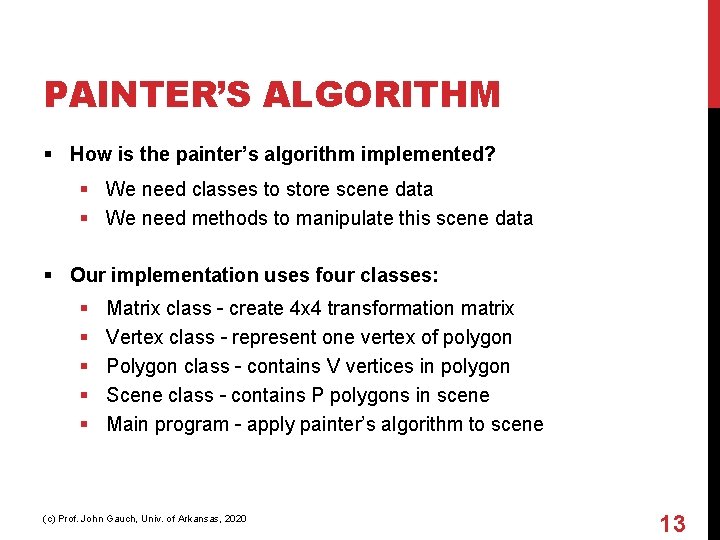
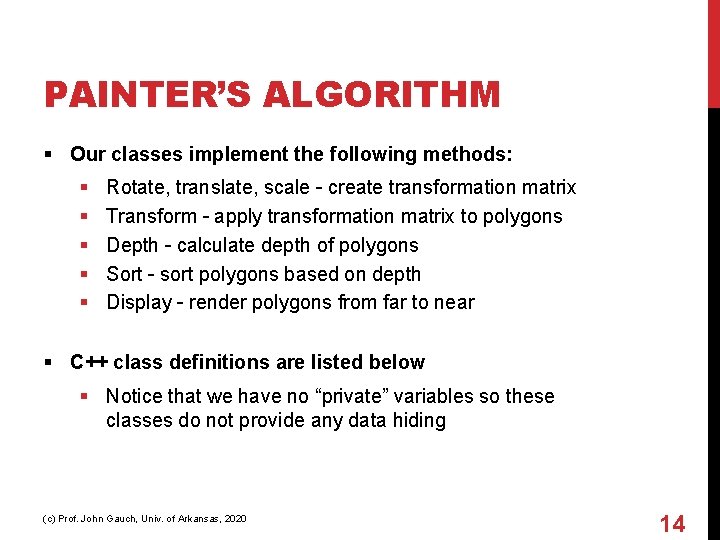
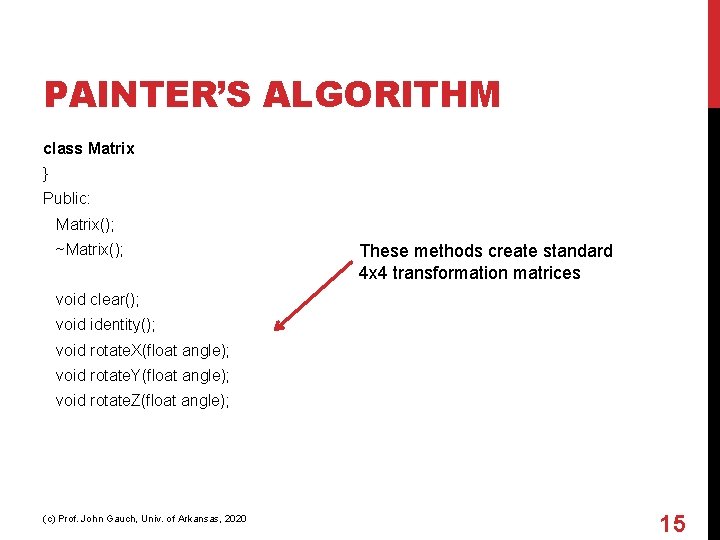
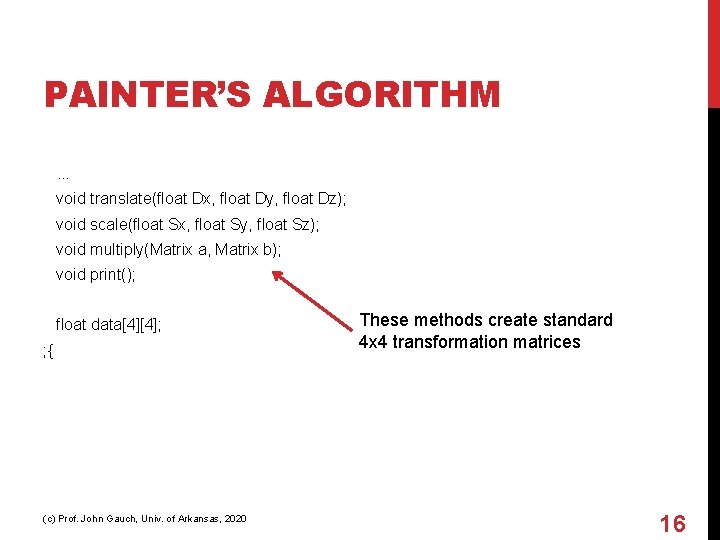
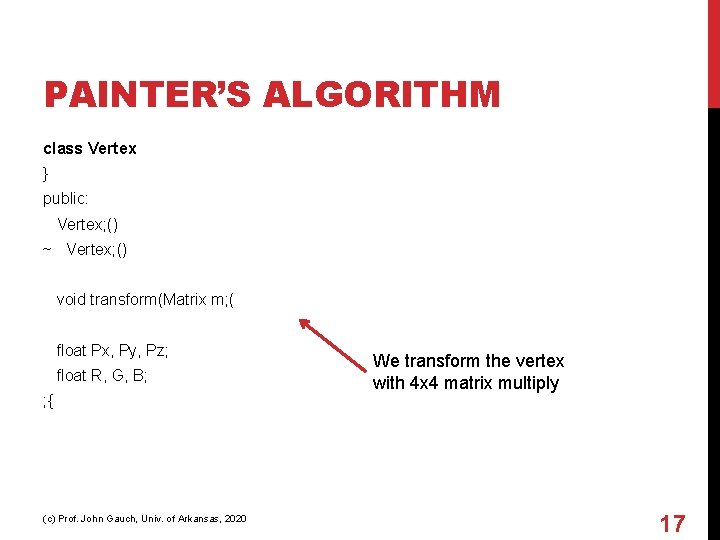
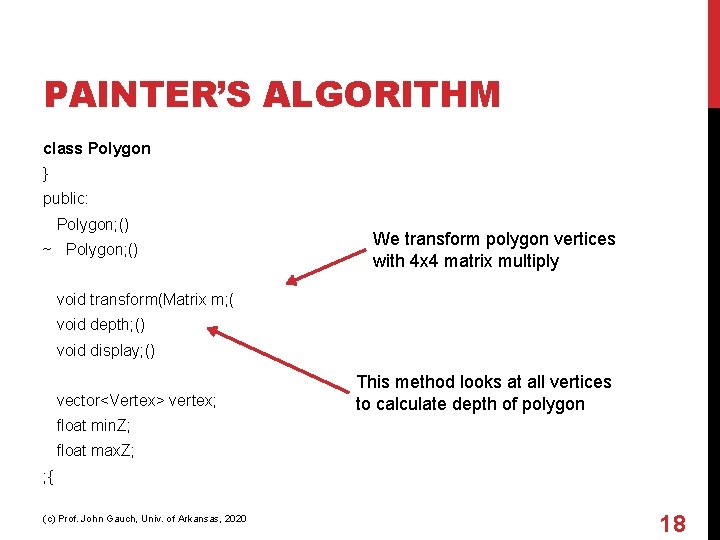
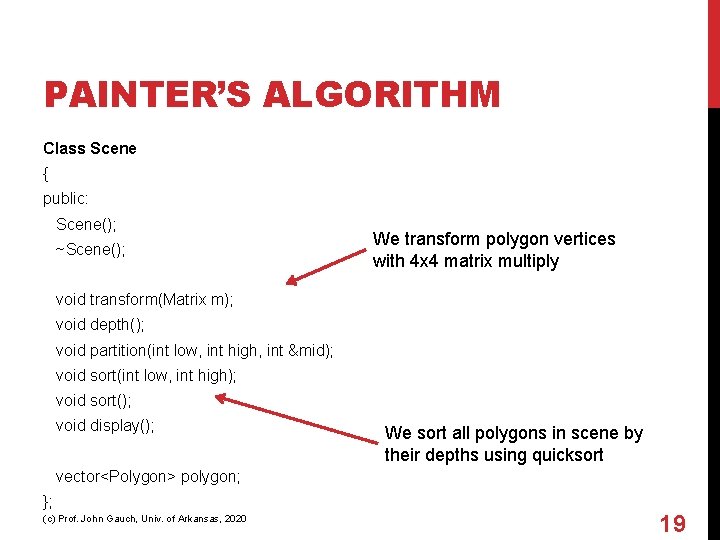
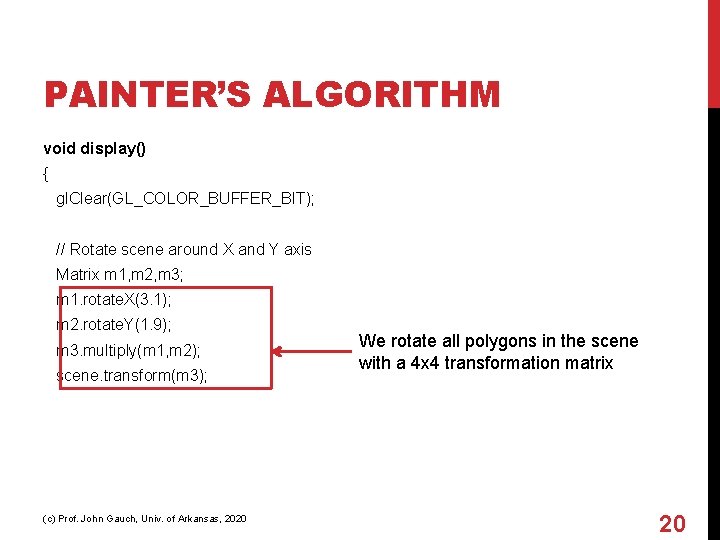
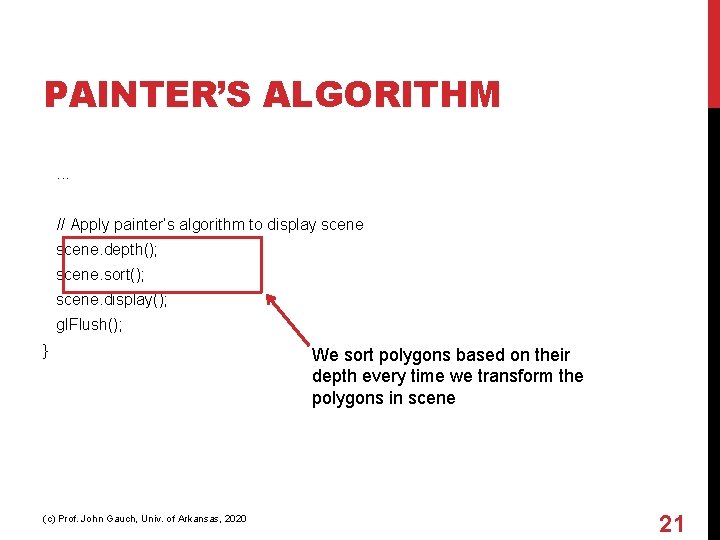
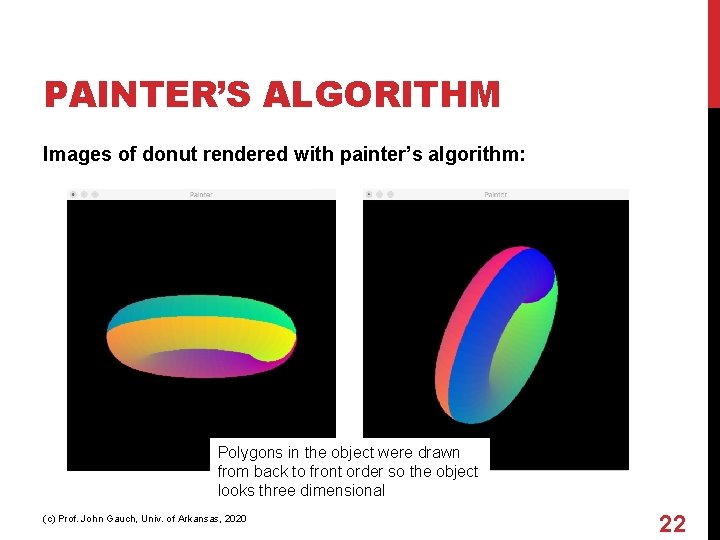
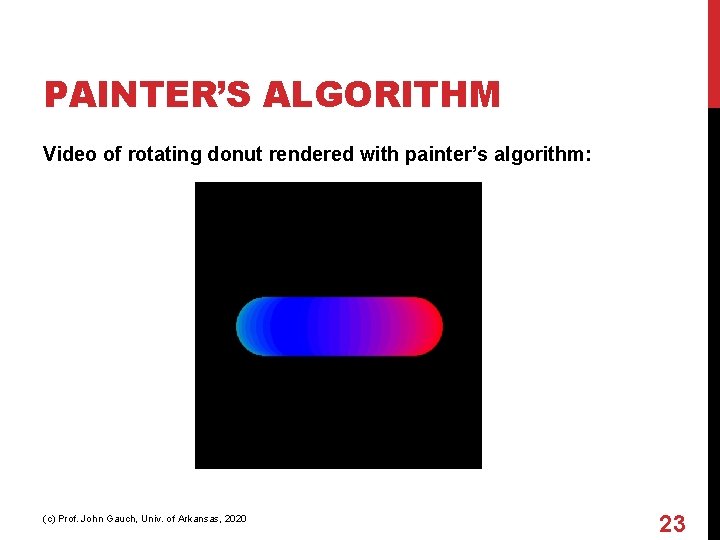
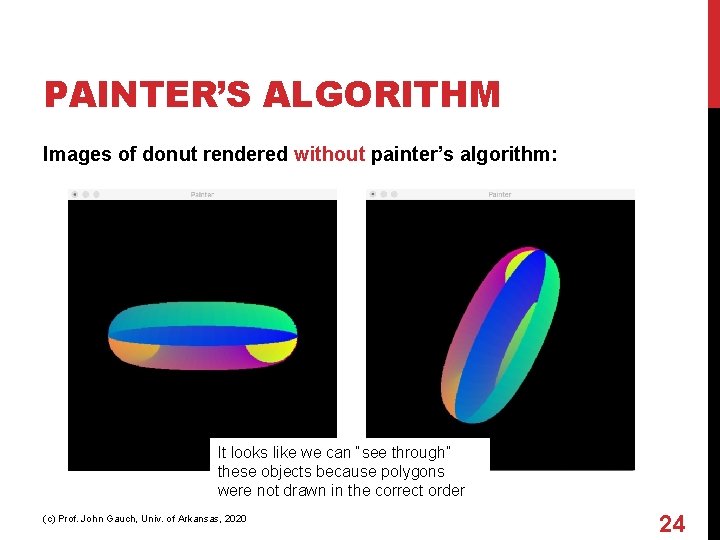
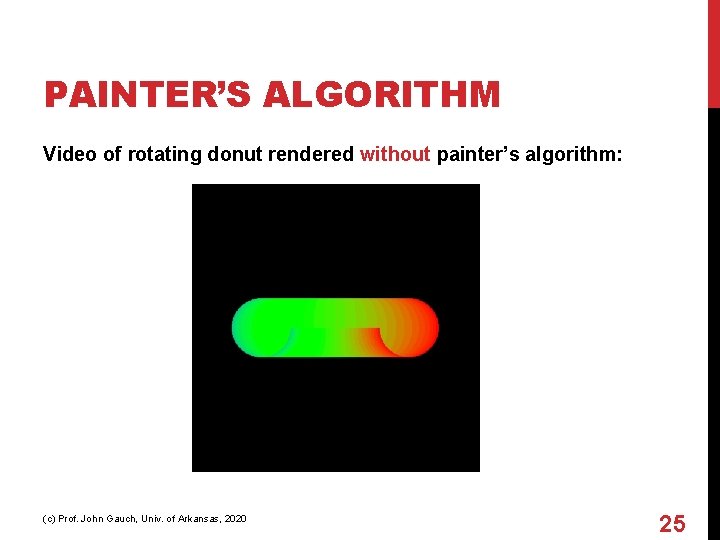
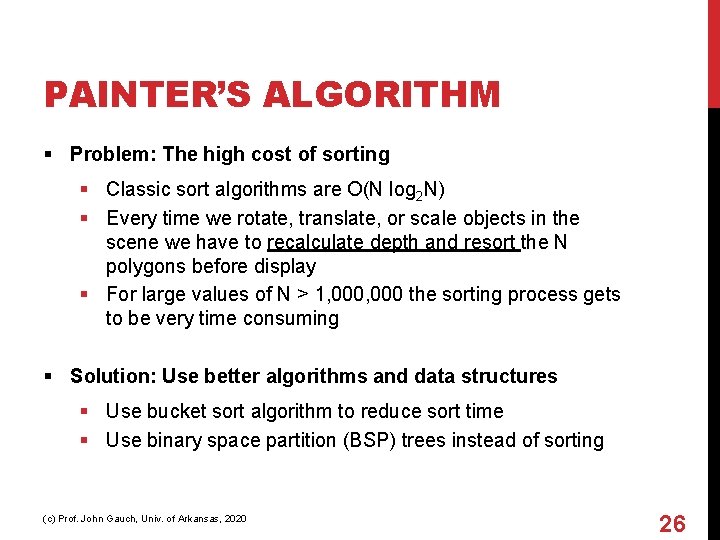
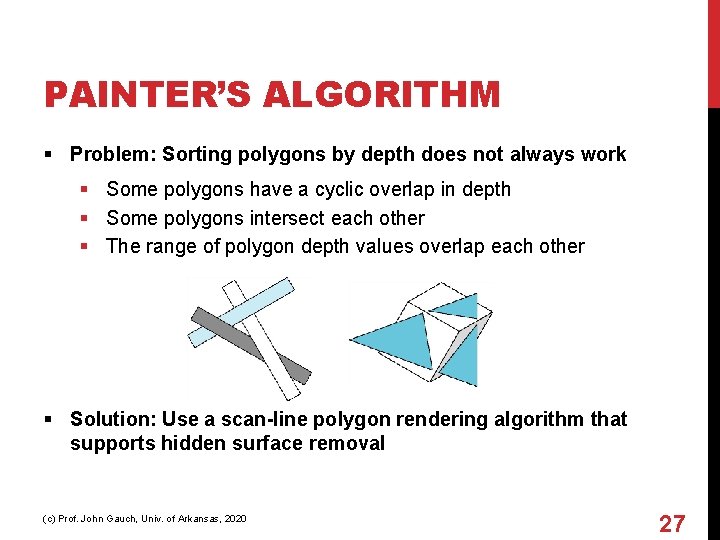
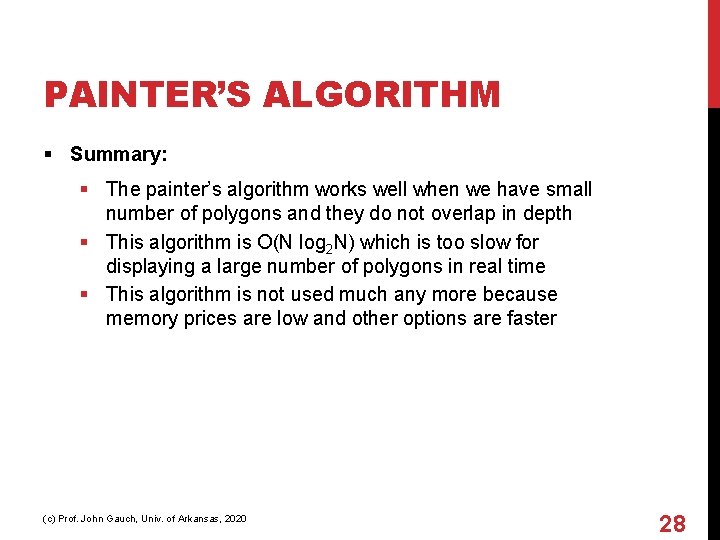
- Slides: 28
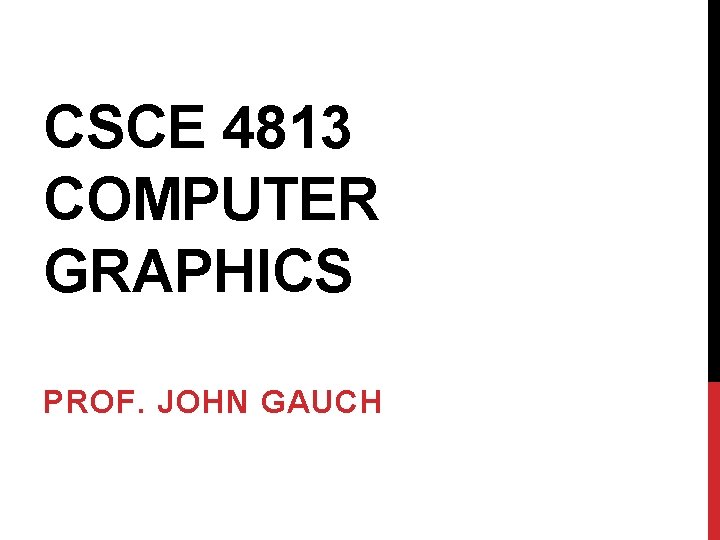
CSCE 4813 COMPUTER GRAPHICS PROF. JOHN GAUCH
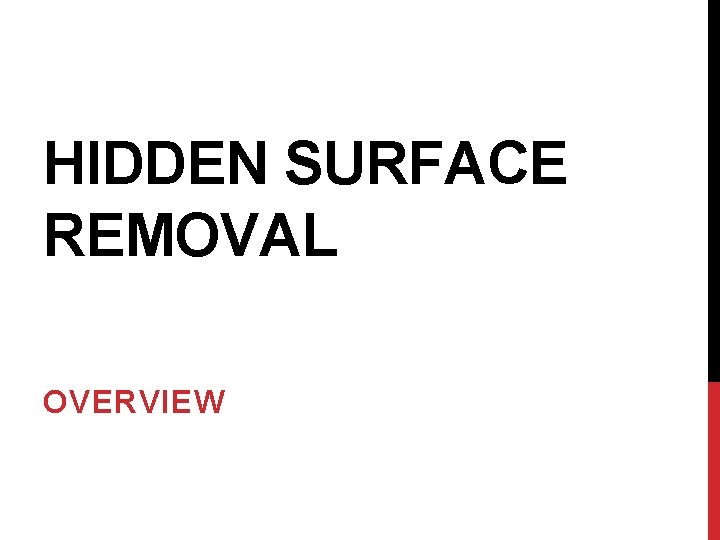
HIDDEN SURFACE REMOVAL OVERVIEW
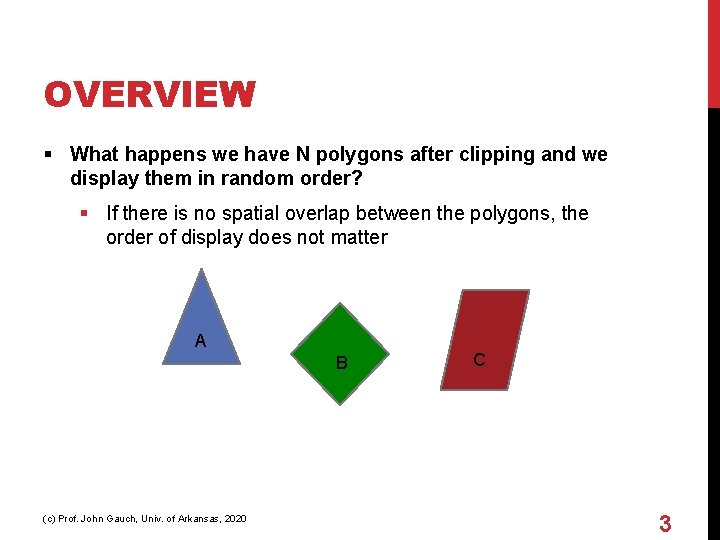
OVERVIEW § What happens we have N polygons after clipping and we display them in random order? § If there is no spatial overlap between the polygons, the order of display does not matter A B (c) Prof. John Gauch, Univ. of Arkansas, 2020 C 3
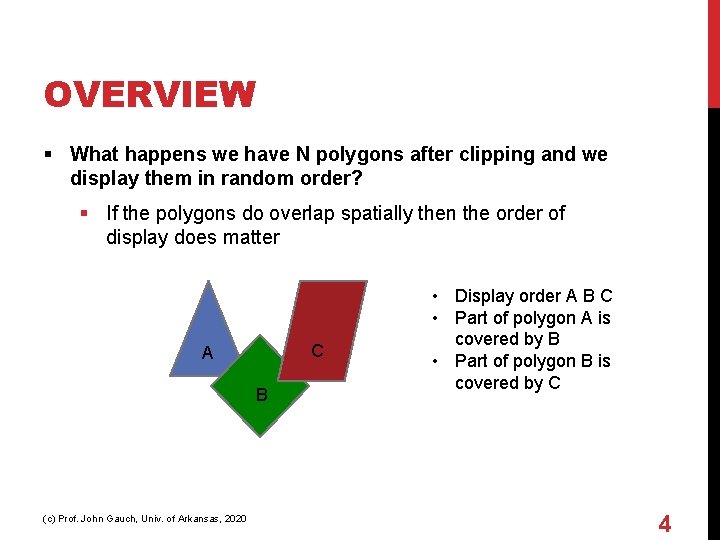
OVERVIEW § What happens we have N polygons after clipping and we display them in random order? § If the polygons do overlap spatially then the order of display does matter C A B (c) Prof. John Gauch, Univ. of Arkansas, 2020 • Display order A B C • Part of polygon A is covered by B • Part of polygon B is covered by C 4
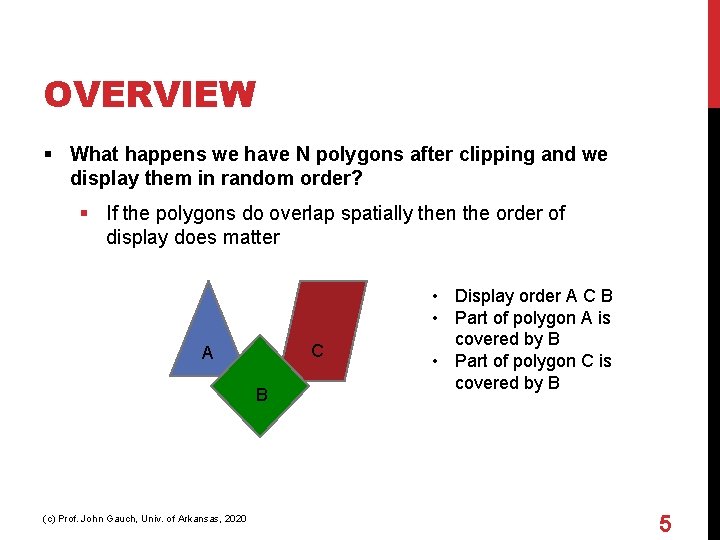
OVERVIEW § What happens we have N polygons after clipping and we display them in random order? § If the polygons do overlap spatially then the order of display does matter C A B (c) Prof. John Gauch, Univ. of Arkansas, 2020 • Display order A C B • Part of polygon A is covered by B • Part of polygon C is covered by B 5
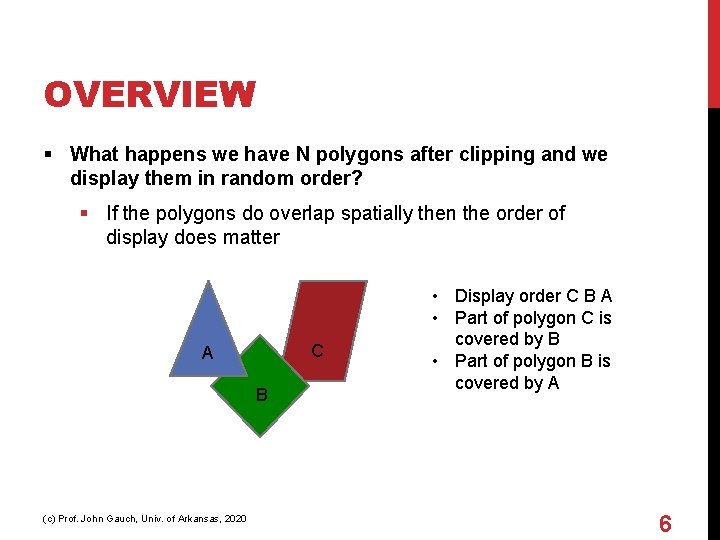
OVERVIEW § What happens we have N polygons after clipping and we display them in random order? § If the polygons do overlap spatially then the order of display does matter C A B (c) Prof. John Gauch, Univ. of Arkansas, 2020 • Display order C B A • Part of polygon C is covered by B • Part of polygon B is covered by A 6
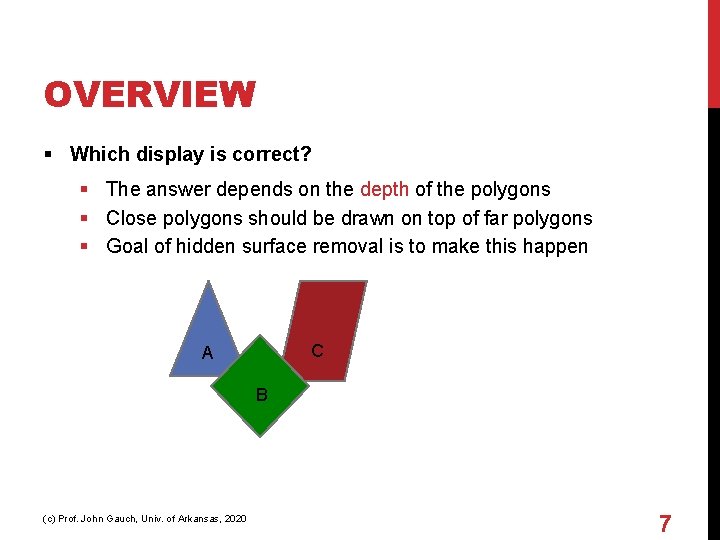
OVERVIEW § Which display is correct? § The answer depends on the depth of the polygons § Close polygons should be drawn on top of far polygons § Goal of hidden surface removal is to make this happen C A B (c) Prof. John Gauch, Univ. of Arkansas, 2020 7
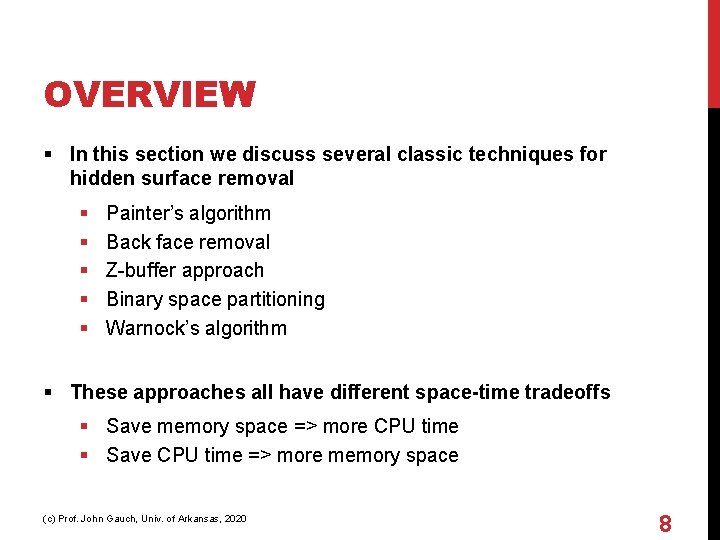
OVERVIEW § In this section we discuss several classic techniques for hidden surface removal § § § Painter’s algorithm Back face removal Z-buffer approach Binary space partitioning Warnock’s algorithm § These approaches all have different space-time tradeoffs § Save memory space => more CPU time § Save CPU time => more memory space (c) Prof. John Gauch, Univ. of Arkansas, 2020 8
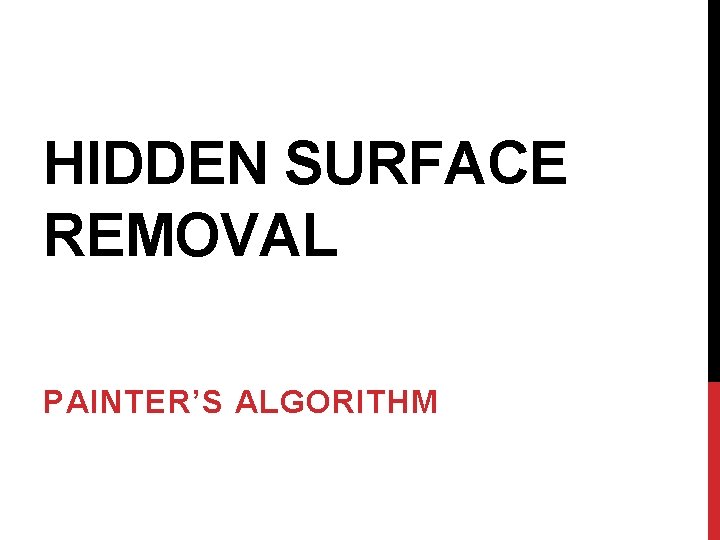
HIDDEN SURFACE REMOVAL PAINTER’S ALGORITHM
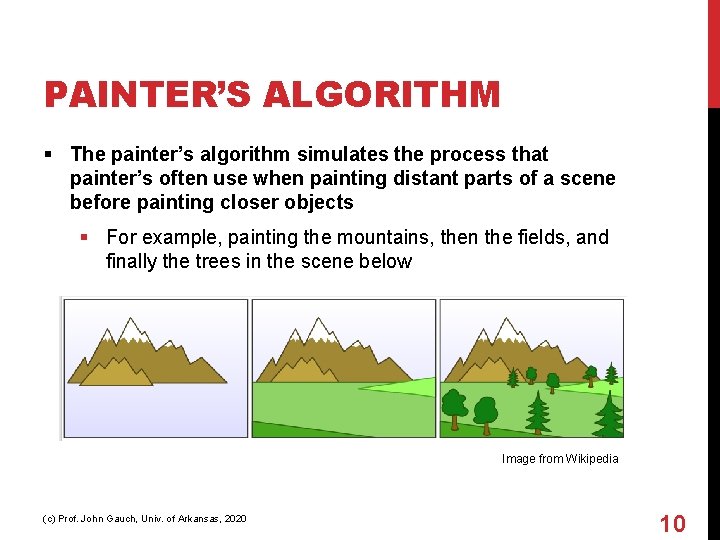
PAINTER’S ALGORITHM § The painter’s algorithm simulates the process that painter’s often use when painting distant parts of a scene before painting closer objects § For example, painting the mountains, then the fields, and finally the trees in the scene below Image from Wikipedia (c) Prof. John Gauch, Univ. of Arkansas, 2020 10
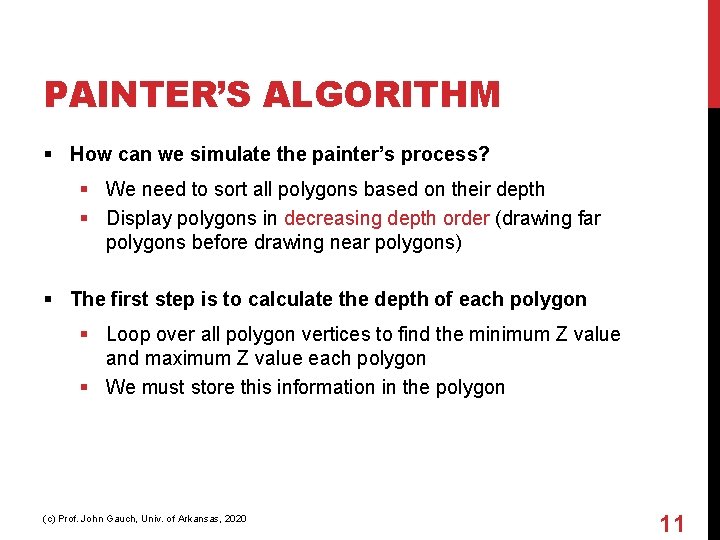
PAINTER’S ALGORITHM § How can we simulate the painter’s process? § We need to sort all polygons based on their depth § Display polygons in decreasing depth order (drawing far polygons before drawing near polygons) § The first step is to calculate the depth of each polygon § Loop over all polygon vertices to find the minimum Z value and maximum Z value each polygon § We must store this information in the polygon (c) Prof. John Gauch, Univ. of Arkansas, 2020 11
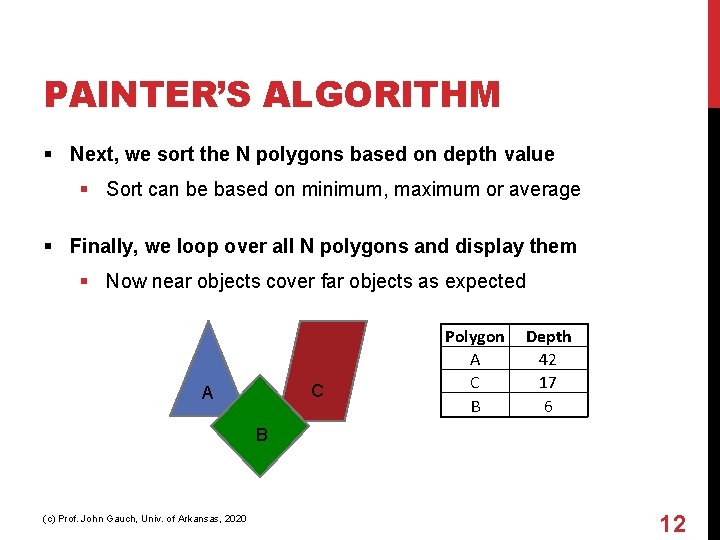
PAINTER’S ALGORITHM § Next, we sort the N polygons based on depth value § Sort can be based on minimum, maximum or average § Finally, we loop over all N polygons and display them § Now near objects cover far objects as expected C A Polygon A C B Depth 42 17 6 B (c) Prof. John Gauch, Univ. of Arkansas, 2020 12
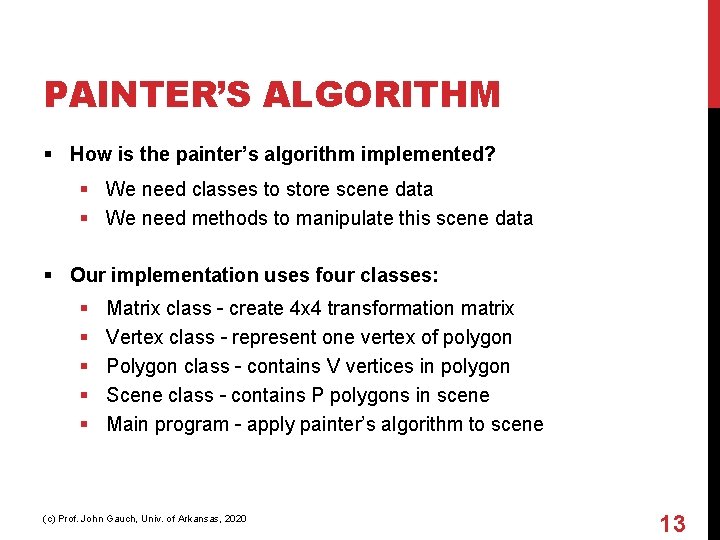
PAINTER’S ALGORITHM § How is the painter’s algorithm implemented? § We need classes to store scene data § We need methods to manipulate this scene data § Our implementation uses four classes: § § § Matrix class – create 4 x 4 transformation matrix Vertex class – represent one vertex of polygon Polygon class – contains V vertices in polygon Scene class – contains P polygons in scene Main program – apply painter’s algorithm to scene (c) Prof. John Gauch, Univ. of Arkansas, 2020 13
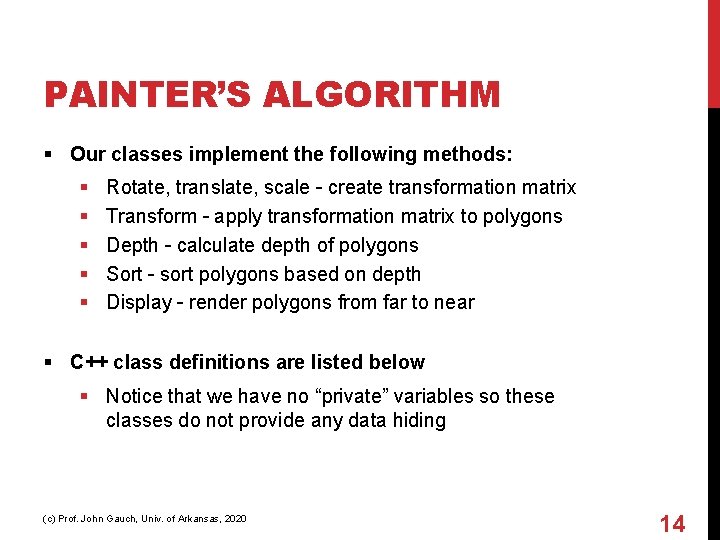
PAINTER’S ALGORITHM § Our classes implement the following methods: § § § Rotate, translate, scale – create transformation matrix Transform – apply transformation matrix to polygons Depth – calculate depth of polygons Sort – sort polygons based on depth Display – render polygons from far to near § C++ class definitions are listed below § Notice that we have no “private” variables so these classes do not provide any data hiding (c) Prof. John Gauch, Univ. of Arkansas, 2020 14
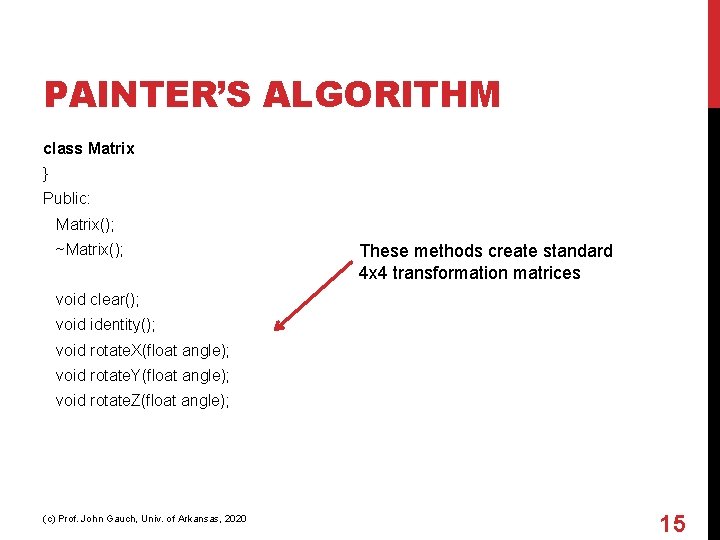
PAINTER’S ALGORITHM class Matrix } Public: Matrix(); ~Matrix(); These methods create standard 4 x 4 transformation matrices void clear(); void identity(); void rotate. X(float angle); void rotate. Y(float angle); void rotate. Z(float angle); (c) Prof. John Gauch, Univ. of Arkansas, 2020 15
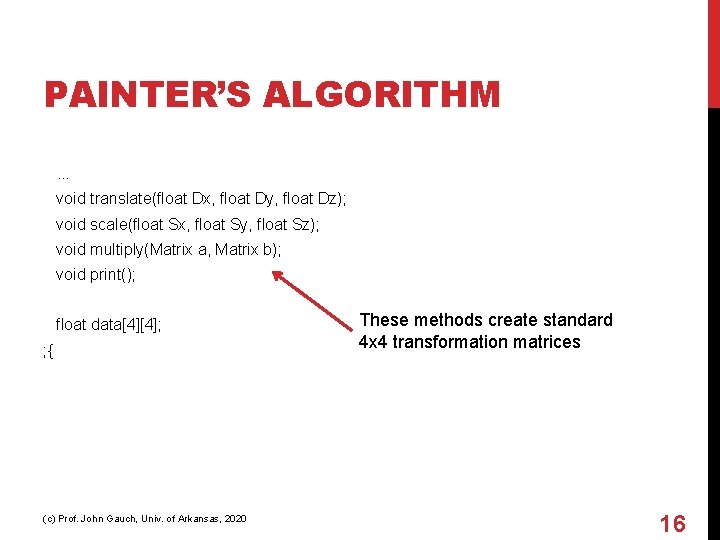
PAINTER’S ALGORITHM. . . void translate(float Dx, float Dy, float Dz); void scale(float Sx, float Sy, float Sz); void multiply(Matrix a, Matrix b); void print(); float data[4][4]; ; { (c) Prof. John Gauch, Univ. of Arkansas, 2020 These methods create standard 4 x 4 transformation matrices 16
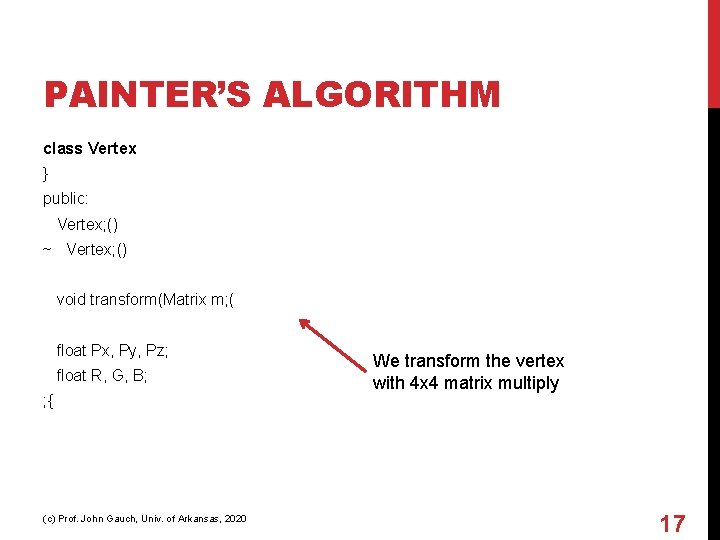
PAINTER’S ALGORITHM class Vertex } public: Vertex; () ~ Vertex; () void transform(Matrix m; ( float Px, Py, Pz; float R, G, B; ; { (c) Prof. John Gauch, Univ. of Arkansas, 2020 We transform the vertex with 4 x 4 matrix multiply 17
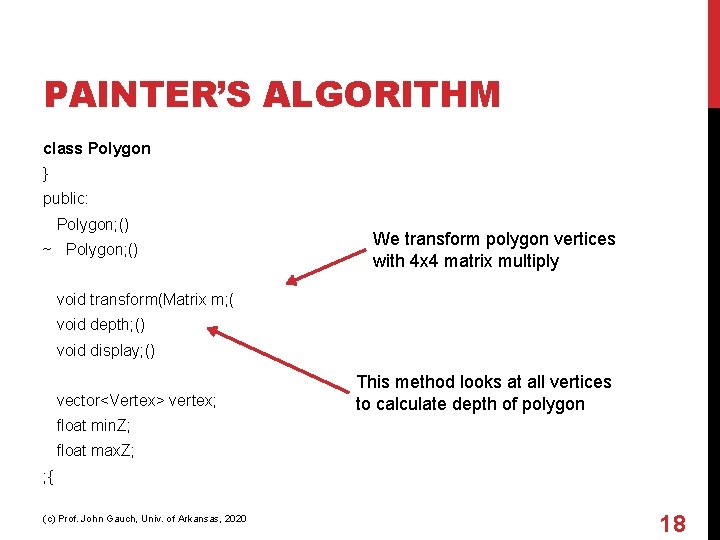
PAINTER’S ALGORITHM class Polygon } public: Polygon; () ~ Polygon; () We transform polygon vertices with 4 x 4 matrix multiply void transform(Matrix m; ( void depth; () void display; () vector<Vertex> vertex; This method looks at all vertices to calculate depth of polygon float min. Z; float max. Z; ; { (c) Prof. John Gauch, Univ. of Arkansas, 2020 18
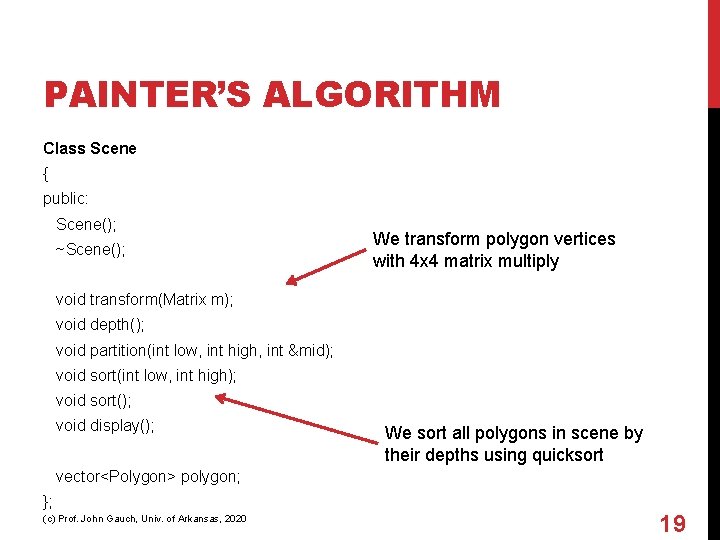
PAINTER’S ALGORITHM Class Scene { public: Scene(); ~Scene(); We transform polygon vertices with 4 x 4 matrix multiply void transform(Matrix m); void depth(); void partition(int low, int high, int &mid); void sort(int low, int high); void sort(); void display(); We sort all polygons in scene by their depths using quicksort vector<Polygon> polygon; }; (c) Prof. John Gauch, Univ. of Arkansas, 2020 19
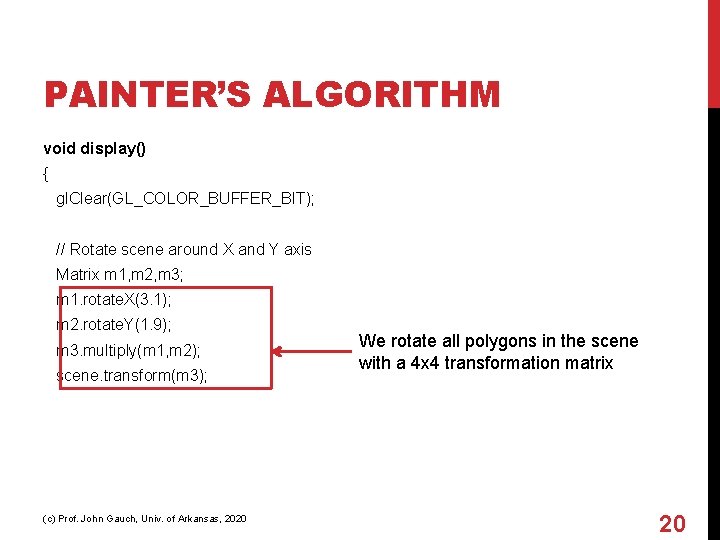
PAINTER’S ALGORITHM void display() { gl. Clear(GL_COLOR_BUFFER_BIT); // Rotate scene around X and Y axis Matrix m 1, m 2, m 3; m 1. rotate. X(3. 1); m 2. rotate. Y(1. 9); m 3. multiply(m 1, m 2); scene. transform(m 3); (c) Prof. John Gauch, Univ. of Arkansas, 2020 We rotate all polygons in the scene with a 4 x 4 transformation matrix 20
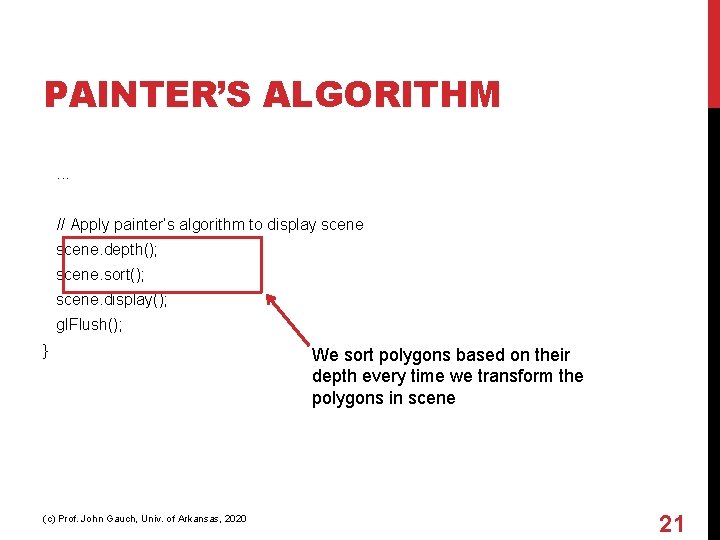
PAINTER’S ALGORITHM. . . // Apply painter’s algorithm to display scene. depth(); scene. sort(); scene. display(); gl. Flush(); } (c) Prof. John Gauch, Univ. of Arkansas, 2020 We sort polygons based on their depth every time we transform the polygons in scene 21
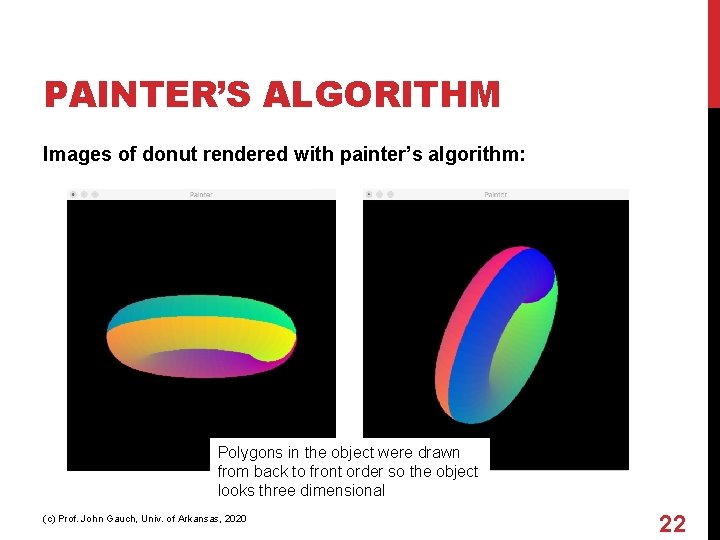
PAINTER’S ALGORITHM Images of donut rendered with painter’s algorithm: Polygons in the object were drawn from back to front order so the object looks three dimensional (c) Prof. John Gauch, Univ. of Arkansas, 2020 22
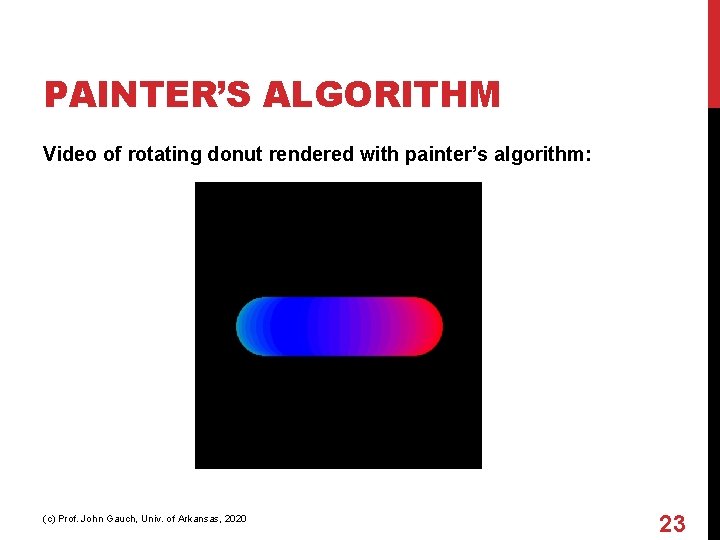
PAINTER’S ALGORITHM Video of rotating donut rendered with painter’s algorithm: (c) Prof. John Gauch, Univ. of Arkansas, 2020 23
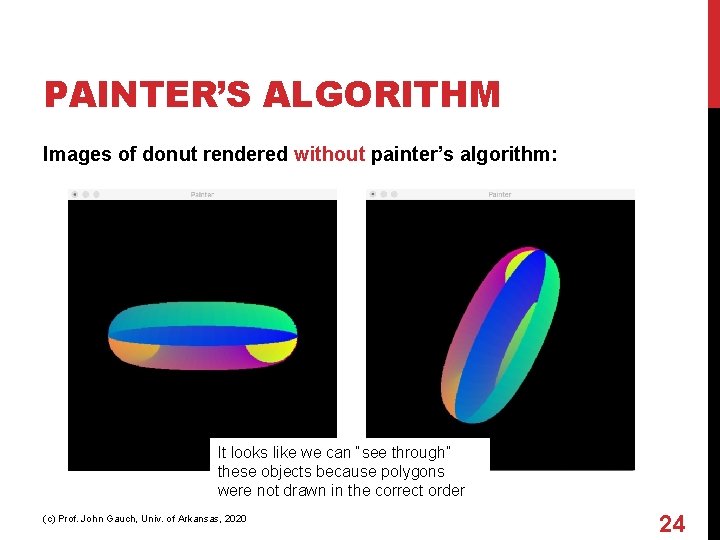
PAINTER’S ALGORITHM Images of donut rendered without painter’s algorithm: It looks like we can “see through” these objects because polygons were not drawn in the correct order (c) Prof. John Gauch, Univ. of Arkansas, 2020 24
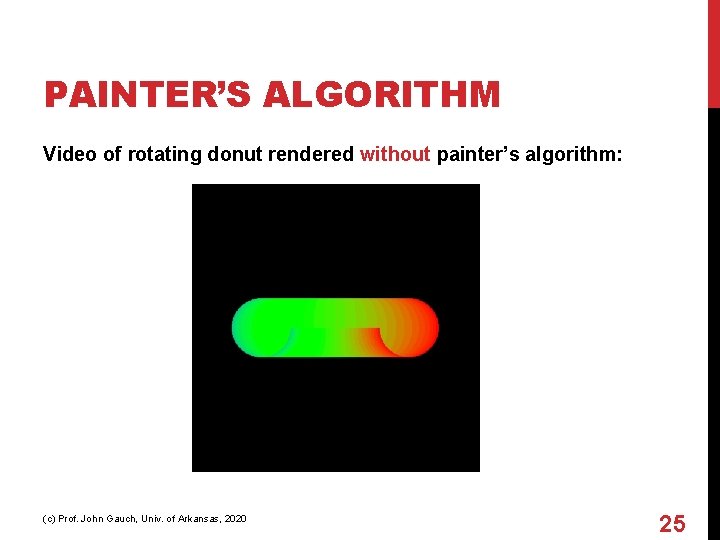
PAINTER’S ALGORITHM Video of rotating donut rendered without painter’s algorithm: (c) Prof. John Gauch, Univ. of Arkansas, 2020 25
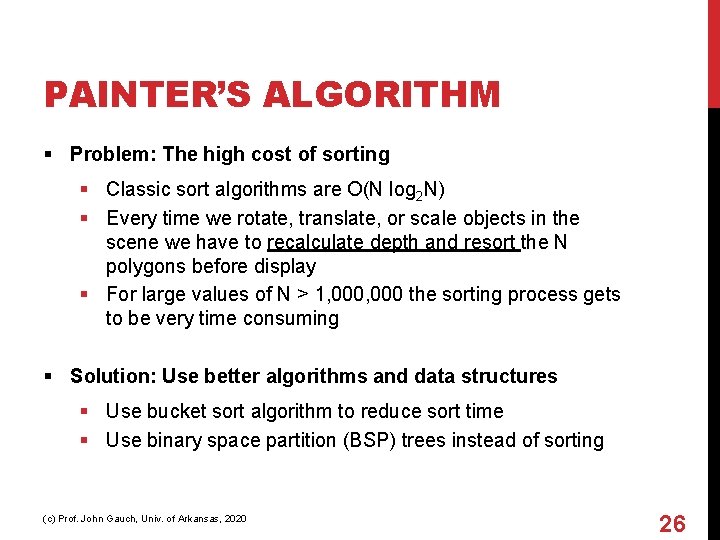
PAINTER’S ALGORITHM § Problem: The high cost of sorting § Classic sort algorithms are O(N log 2 N) § Every time we rotate, translate, or scale objects in the scene we have to recalculate depth and resort the N polygons before display § For large values of N > 1, 000 the sorting process gets to be very time consuming § Solution: Use better algorithms and data structures § Use bucket sort algorithm to reduce sort time § Use binary space partition (BSP) trees instead of sorting (c) Prof. John Gauch, Univ. of Arkansas, 2020 26
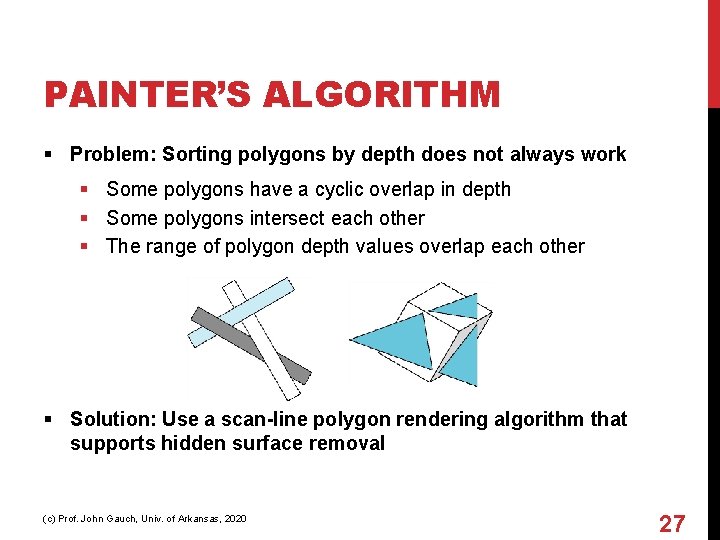
PAINTER’S ALGORITHM § Problem: Sorting polygons by depth does not always work § Some polygons have a cyclic overlap in depth § Some polygons intersect each other § The range of polygon depth values overlap each other § Solution: Use a scan-line polygon rendering algorithm that supports hidden surface removal (c) Prof. John Gauch, Univ. of Arkansas, 2020 27
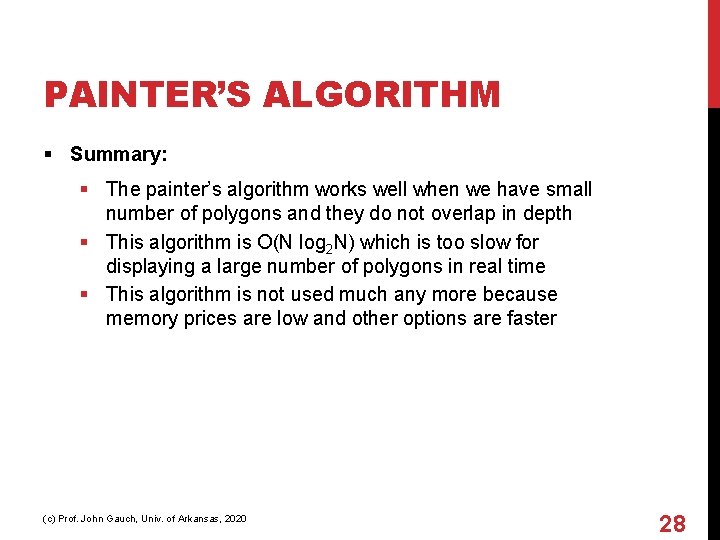
PAINTER’S ALGORITHM § Summary: § The painter’s algorithm works well when we have small number of polygons and they do not overlap in depth § This algorithm is O(N log 2 N) which is too slow for displaying a large number of polygons in real time § This algorithm is not used much any more because memory prices are low and other options are faster (c) Prof. John Gauch, Univ. of Arkansas, 2020 28
John gauch
Hidden surface removal in computer graphics
Susan gauch
Dr gauch
Graphics monitors in computer graphics
Lcd working principle ppt
Hidden flame by john dryden
Passenger transport safety
Angel
What is viewing in computer graphics
Plasma panel display in computer graphics
Interior and exterior clipping in computer graphics
Shear transformation in computer graphics
Glsl sincos
Scan converting ellipse in computer graphics
Rigid body transformation in computer graphics
The region of
Starburst method in computer graphics
Polygon fill algorithm in computer graphics
Raster scan display
Computer graphics
Lines in computer graphics
Cs 418 interactive computer graphics
Cs 418 interactive computer graphics
Achromatic light in computer graphics
Interactive input devices
Uniform scaling in computer graphics
Uniform scaling in computer graphics
Orthogonal projection in computer graphics