CSCE 4813 COMPUTER GRAPHICS PROF JOHN GAUCH HIDDEN
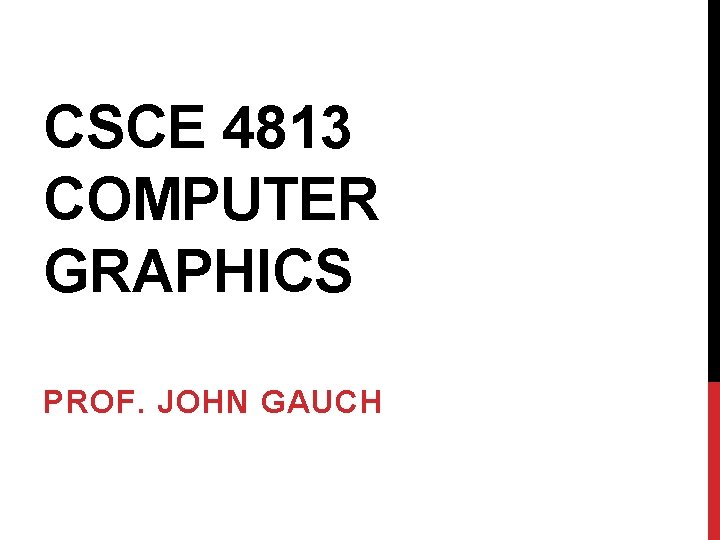
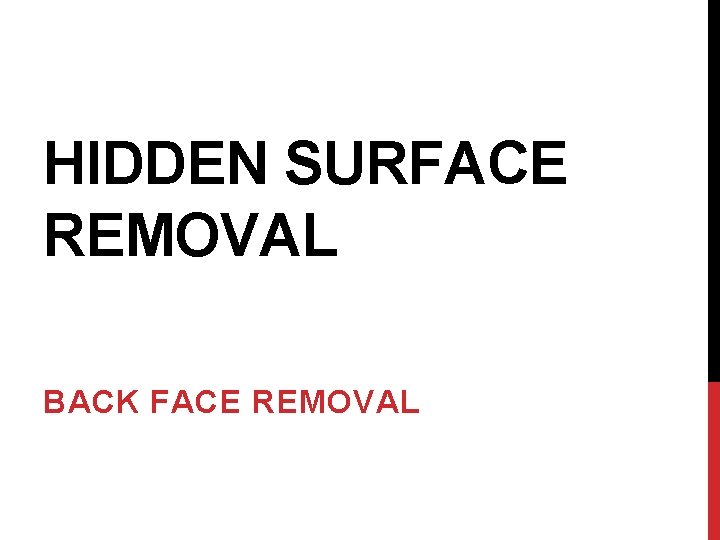
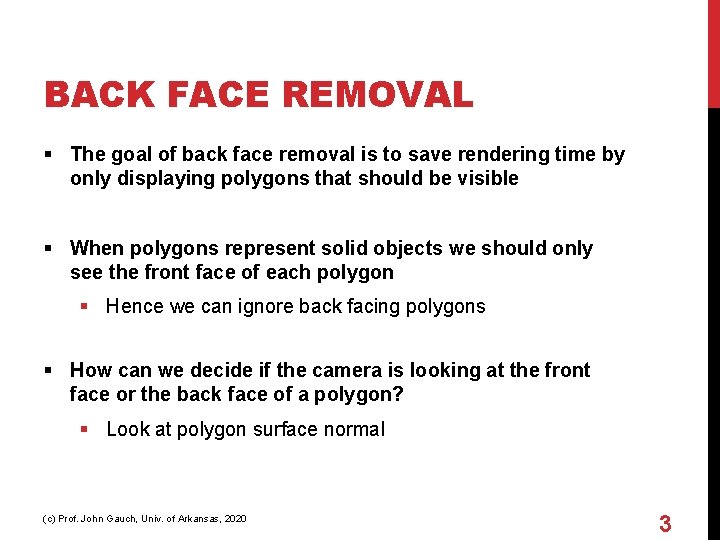
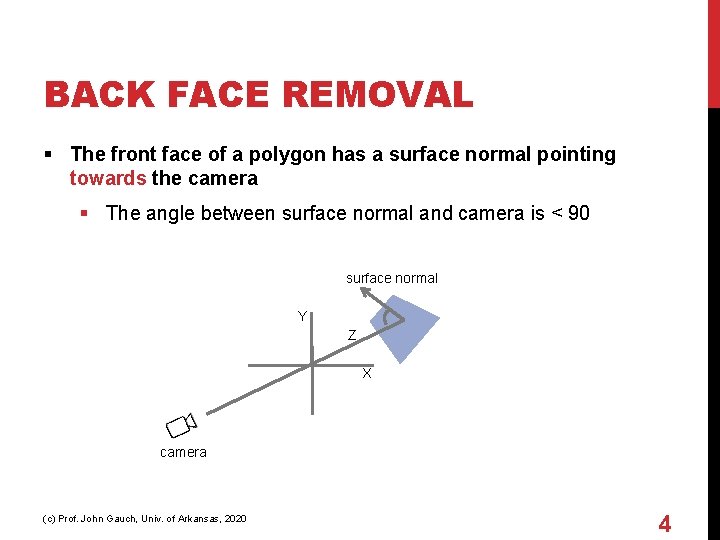
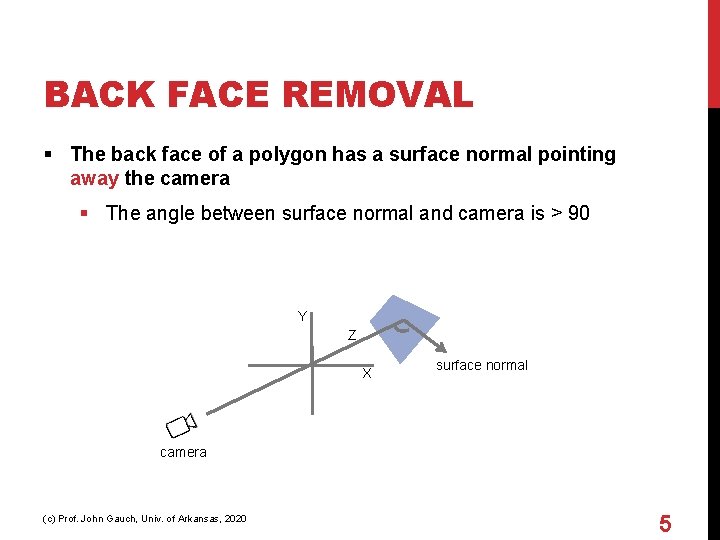
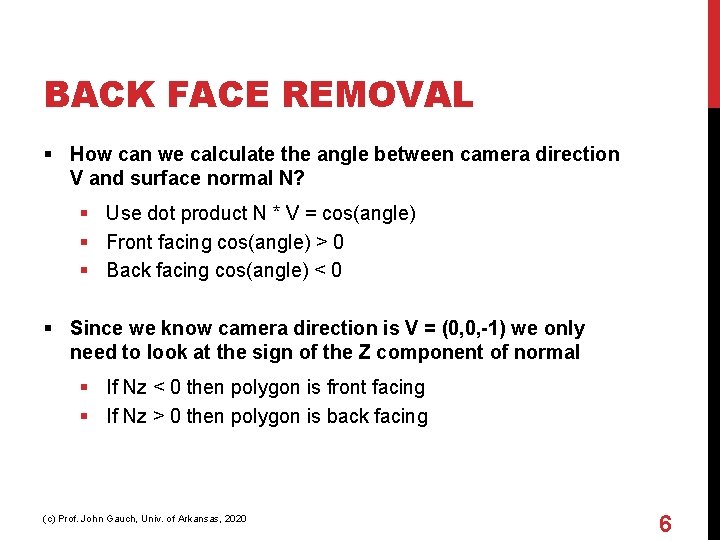
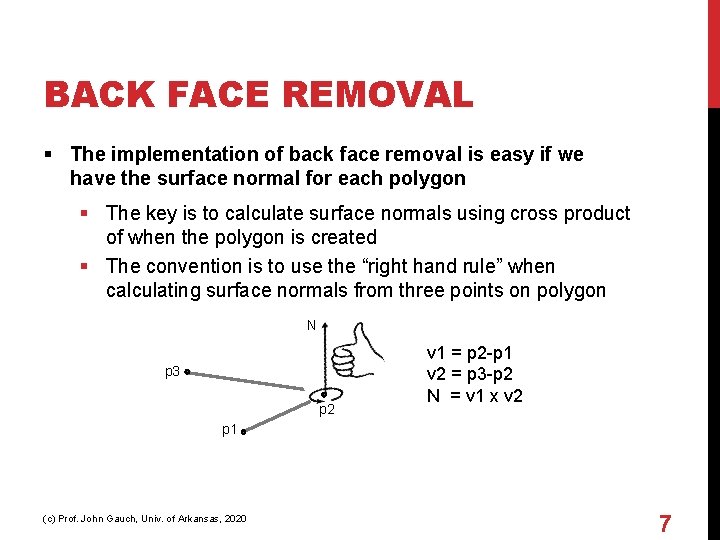
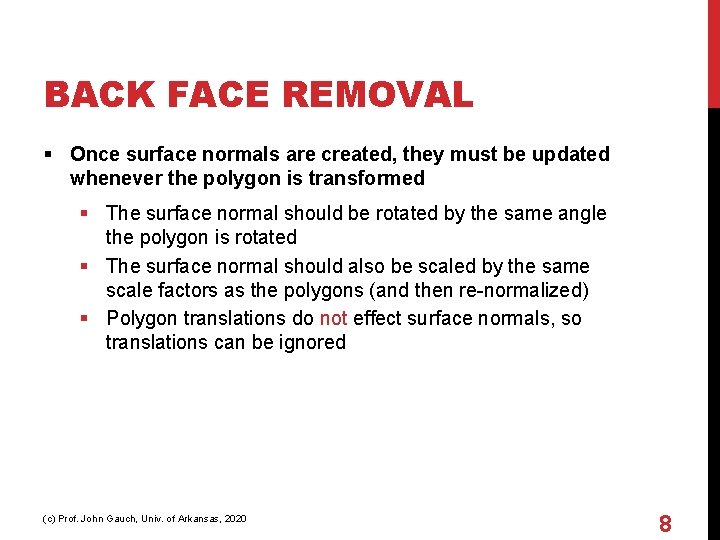
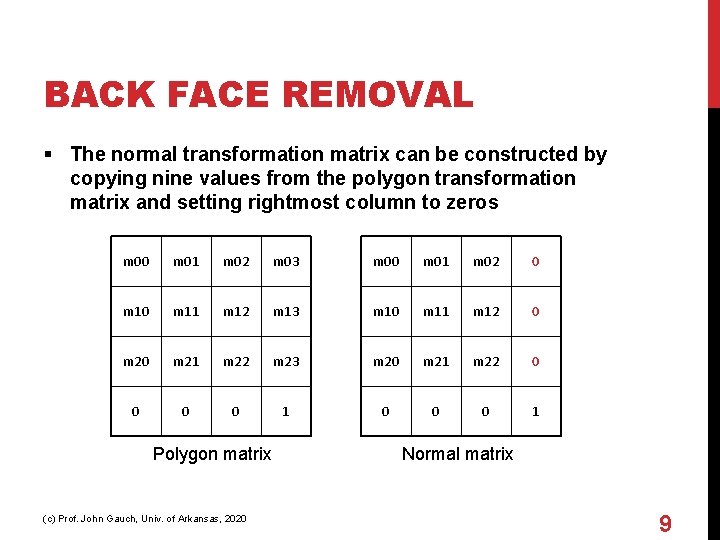
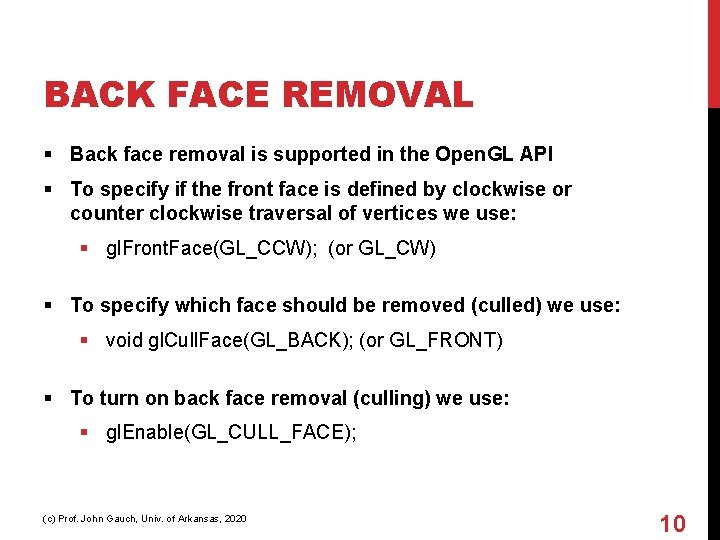
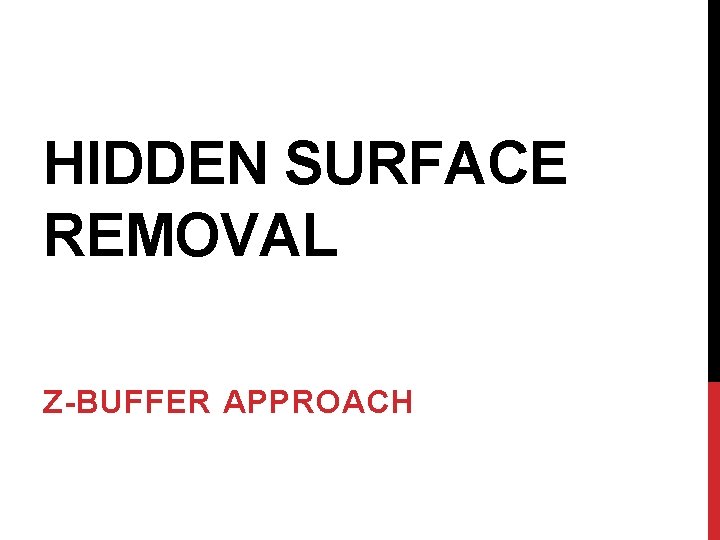
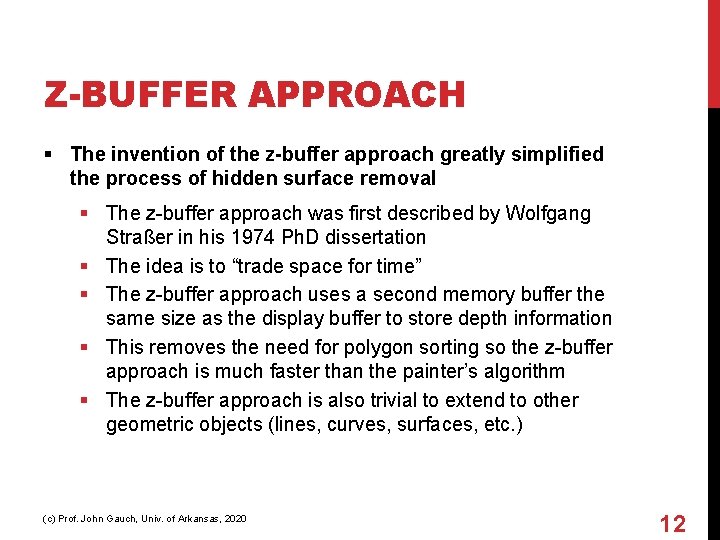
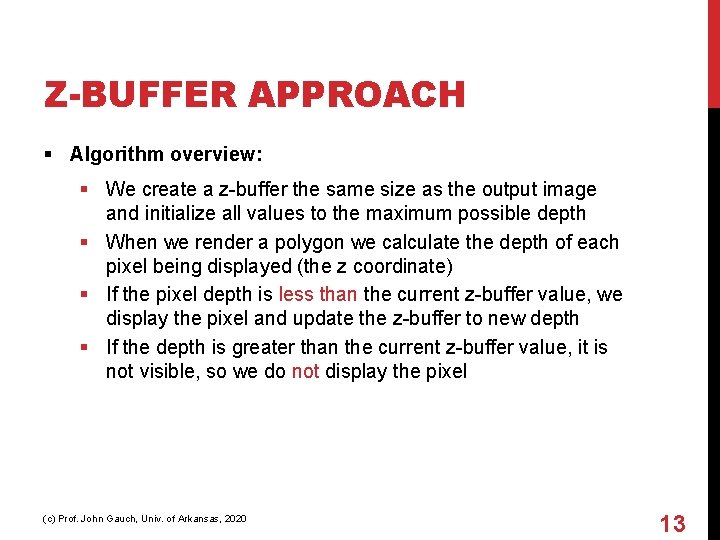
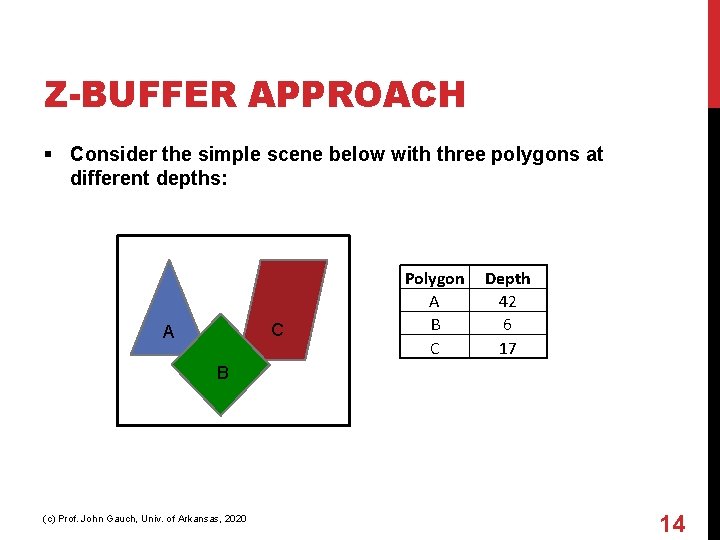
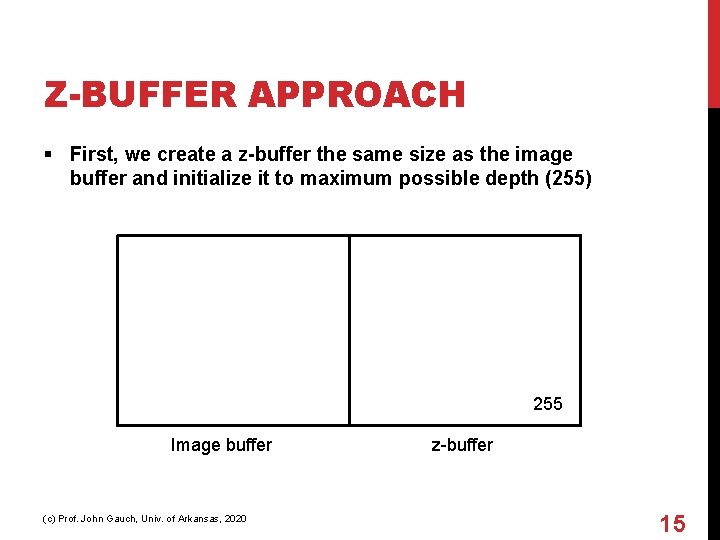
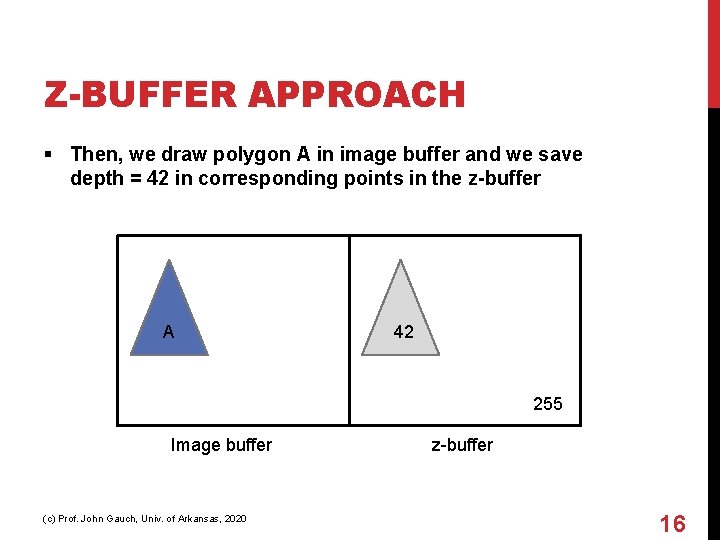
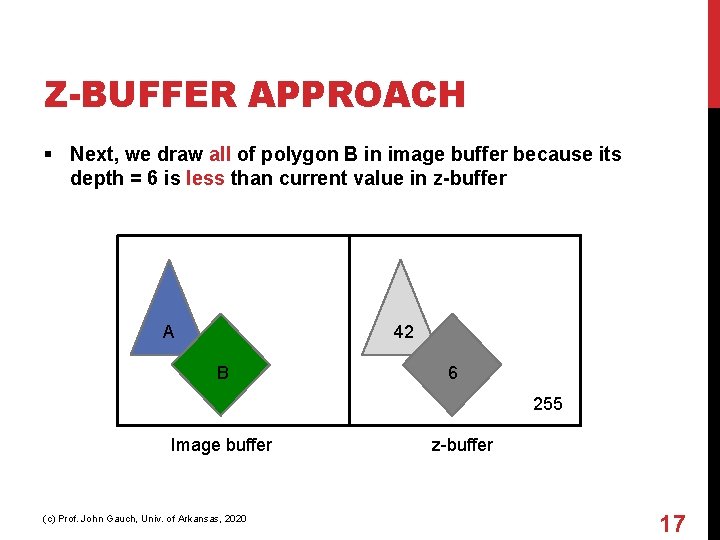
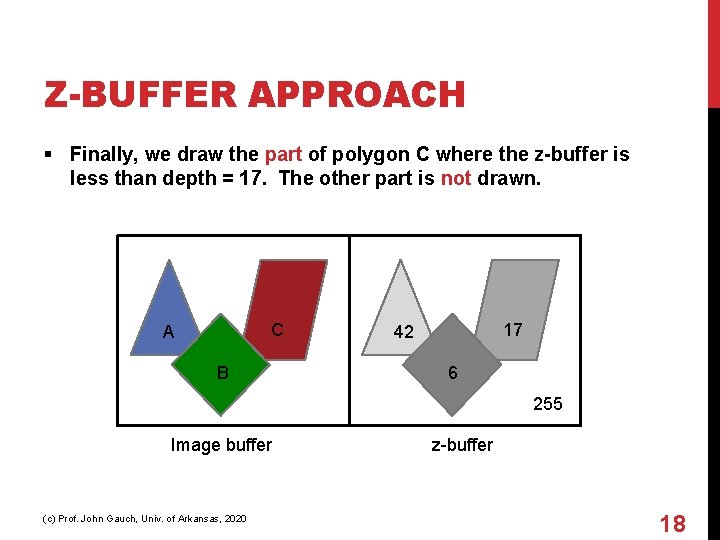
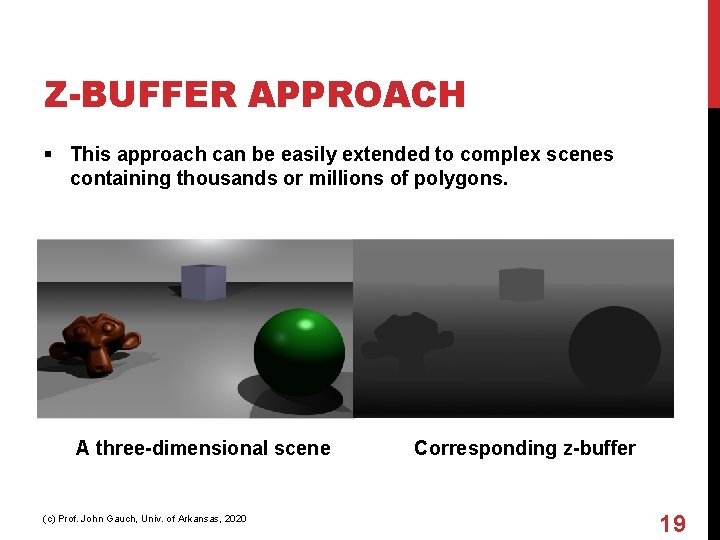
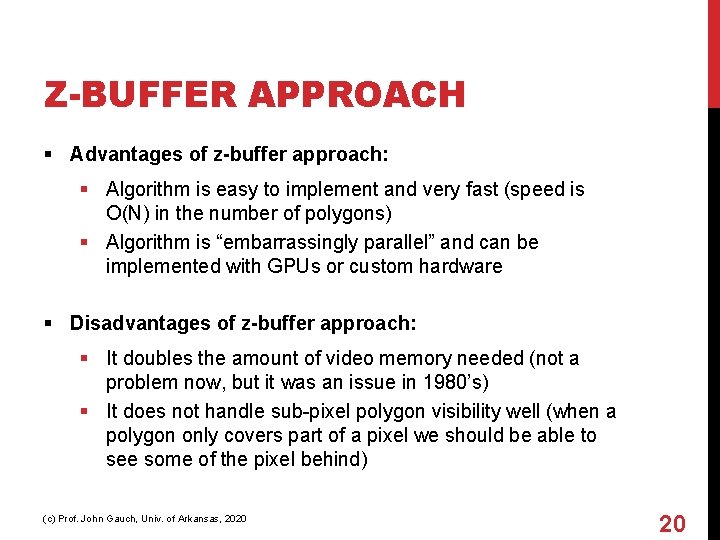
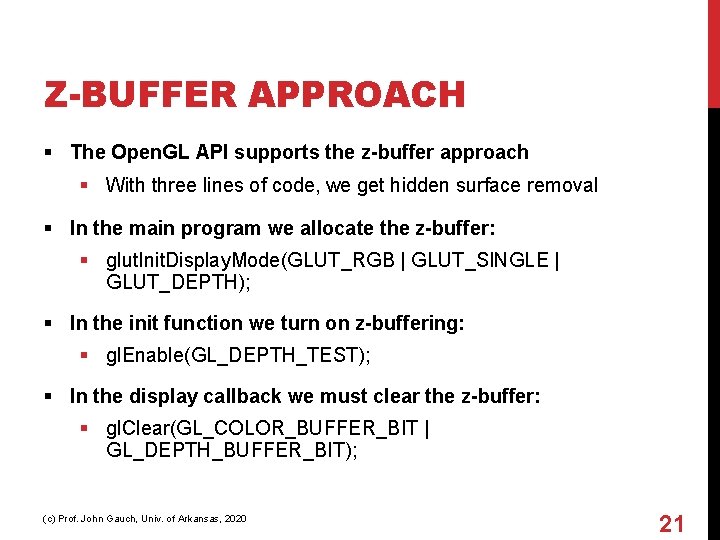
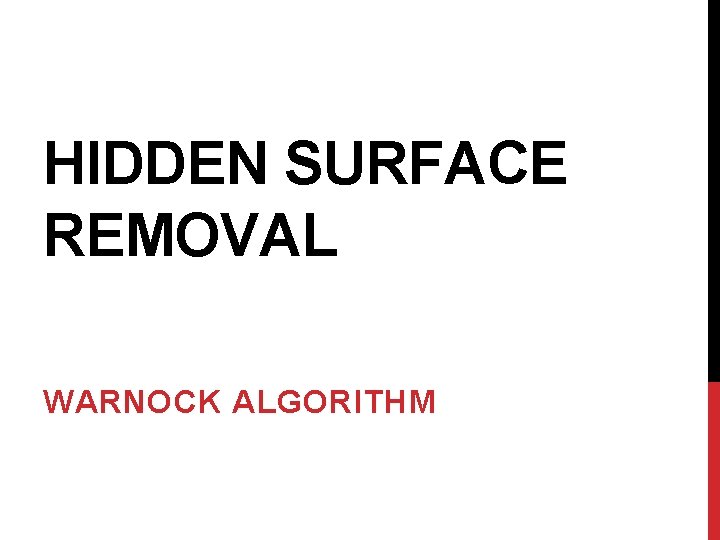
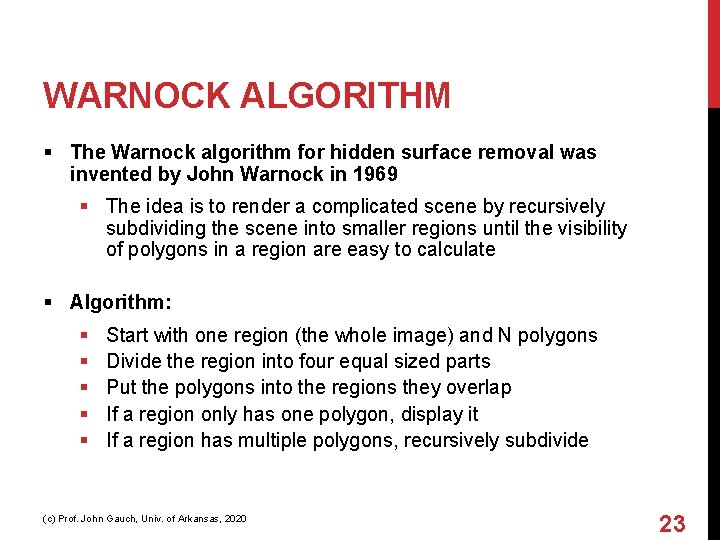
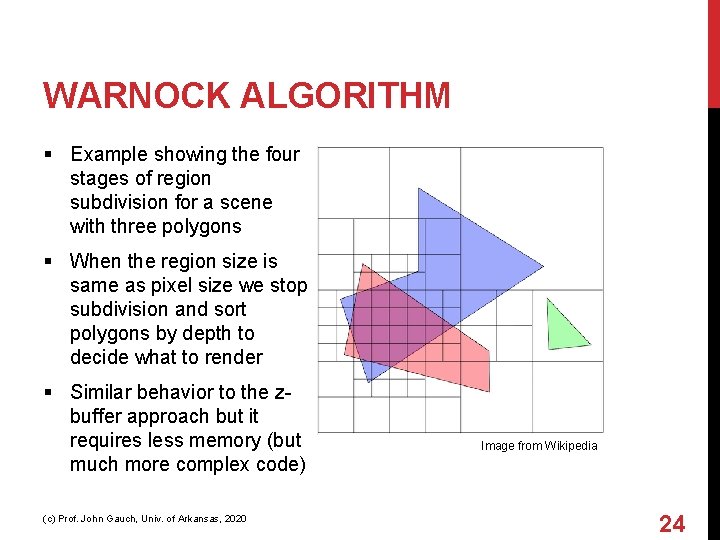
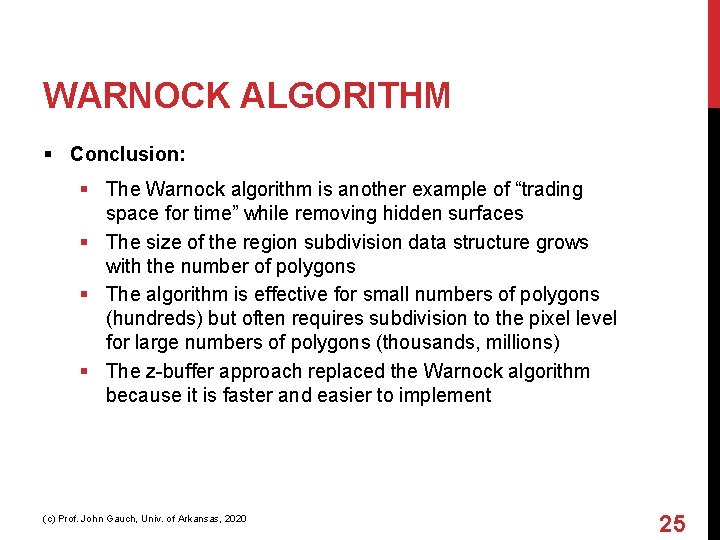
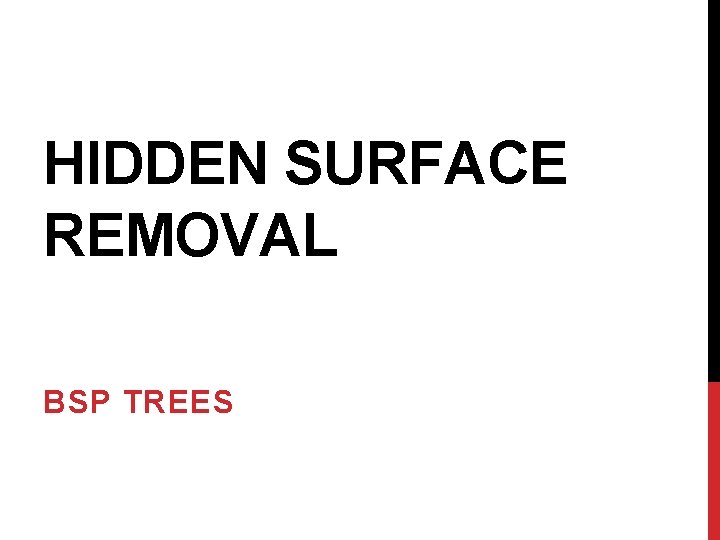
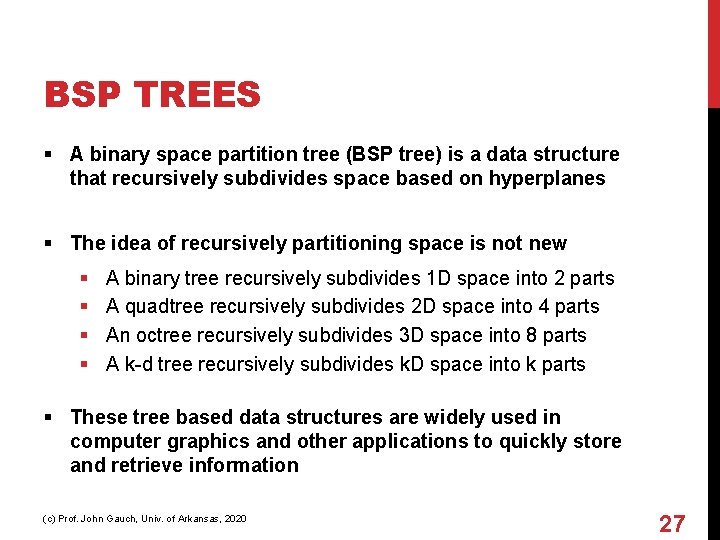
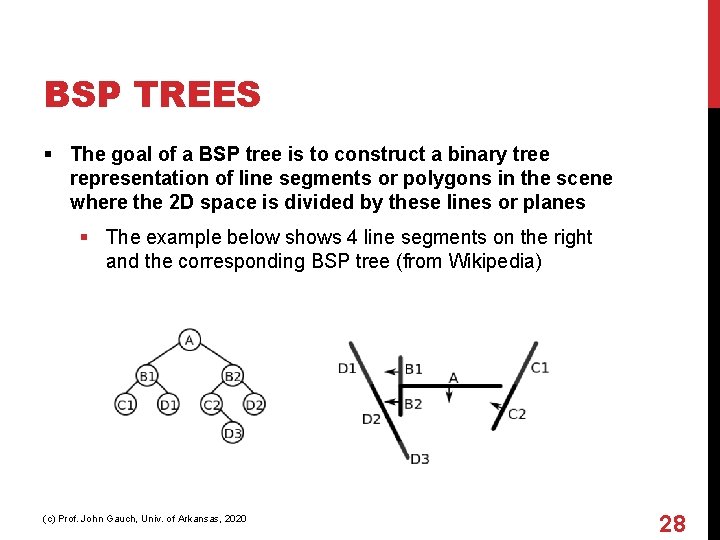
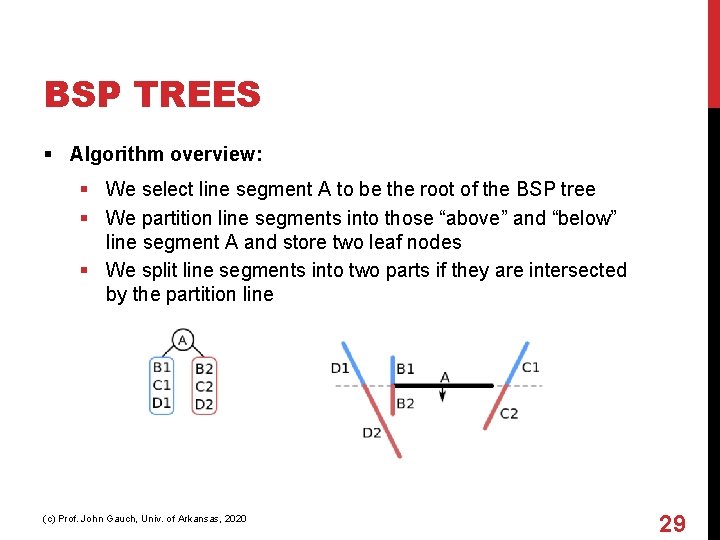
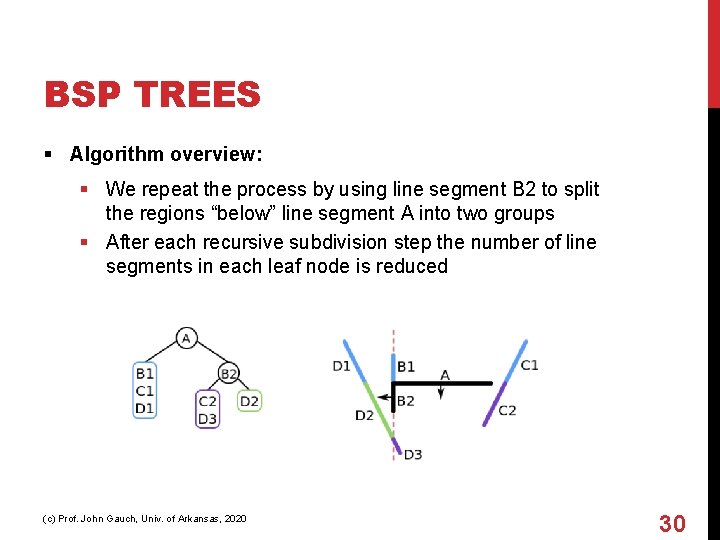
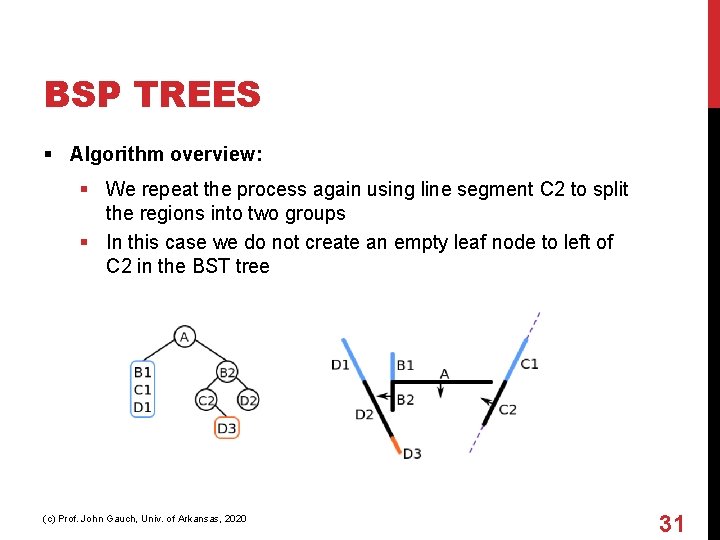
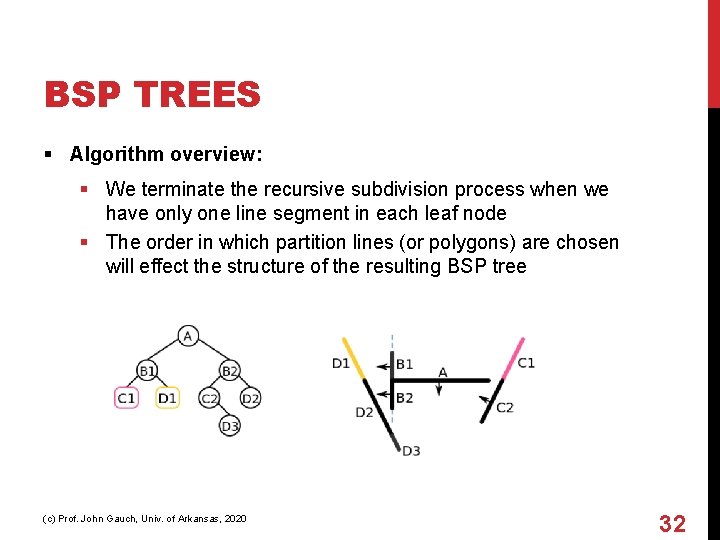
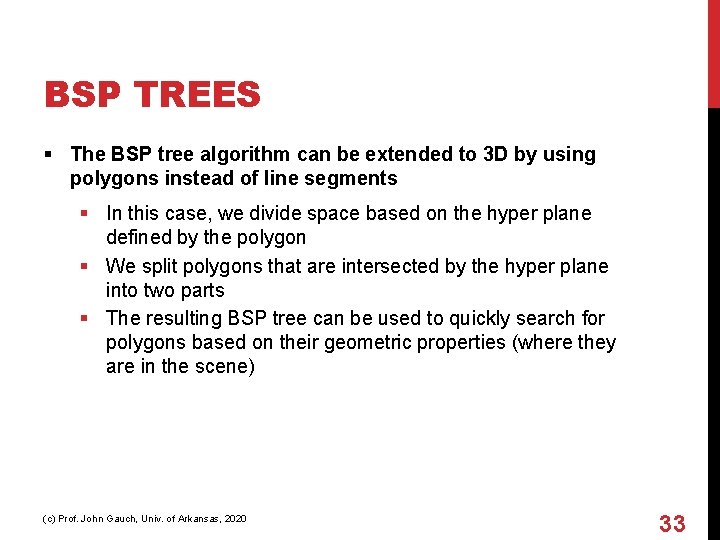
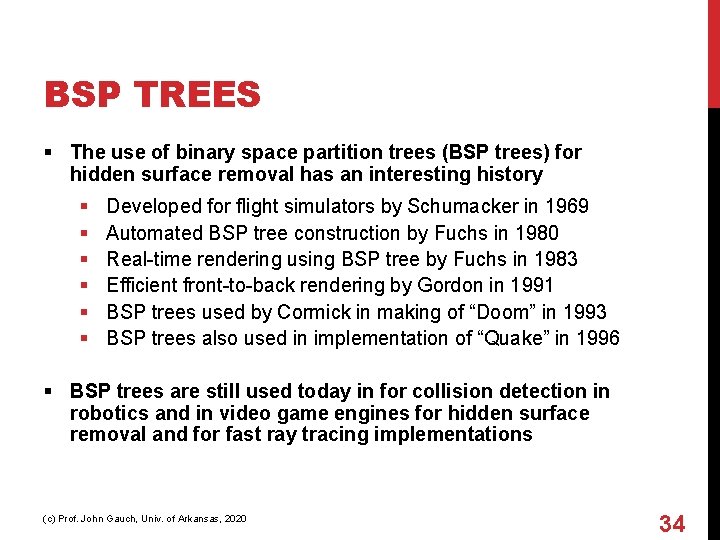
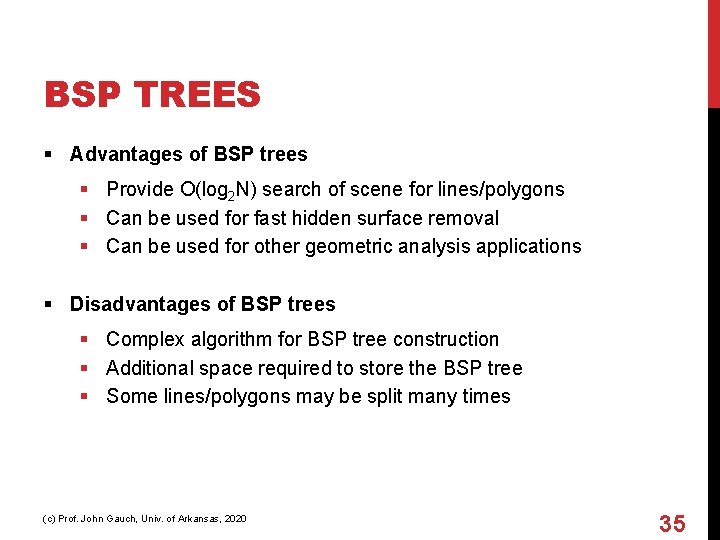
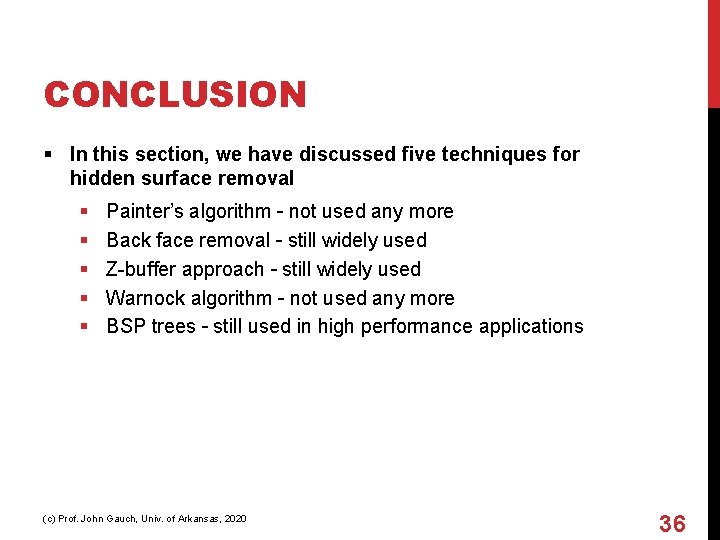
- Slides: 36
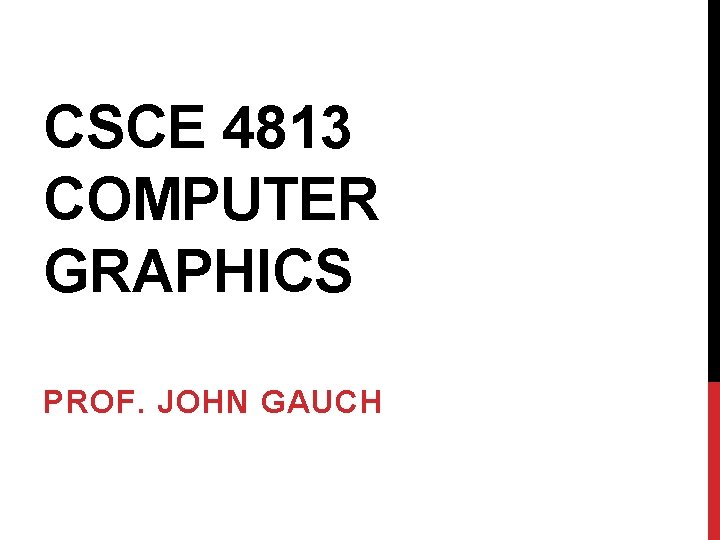
CSCE 4813 COMPUTER GRAPHICS PROF. JOHN GAUCH
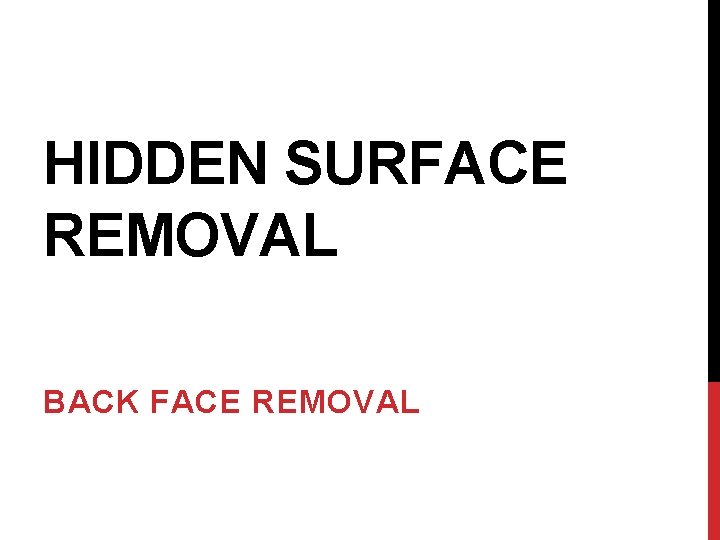
HIDDEN SURFACE REMOVAL BACK FACE REMOVAL
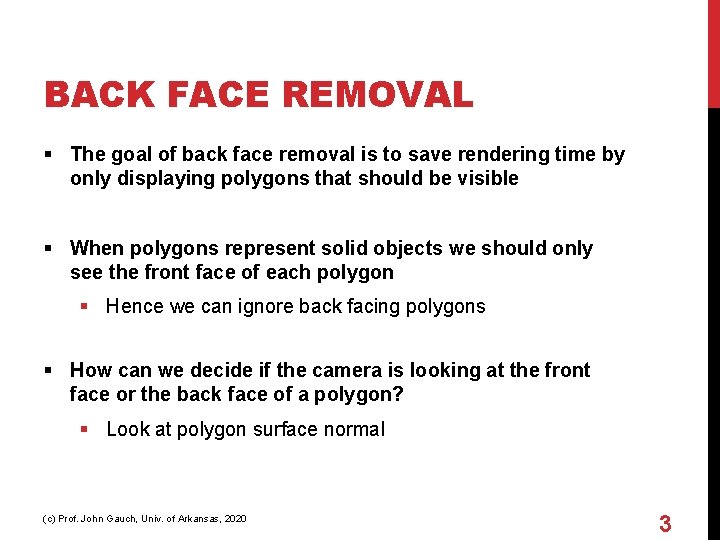
BACK FACE REMOVAL § The goal of back face removal is to save rendering time by only displaying polygons that should be visible § When polygons represent solid objects we should only see the front face of each polygon § Hence we can ignore back facing polygons § How can we decide if the camera is looking at the front face or the back face of a polygon? § Look at polygon surface normal (c) Prof. John Gauch, Univ. of Arkansas, 2020 3
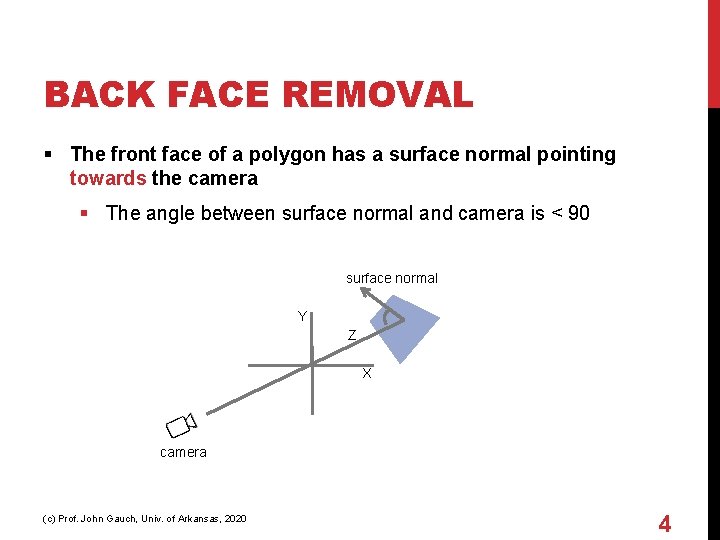
BACK FACE REMOVAL § The front face of a polygon has a surface normal pointing towards the camera § The angle between surface normal and camera is < 90 surface normal Y Z X camera (c) Prof. John Gauch, Univ. of Arkansas, 2020 4
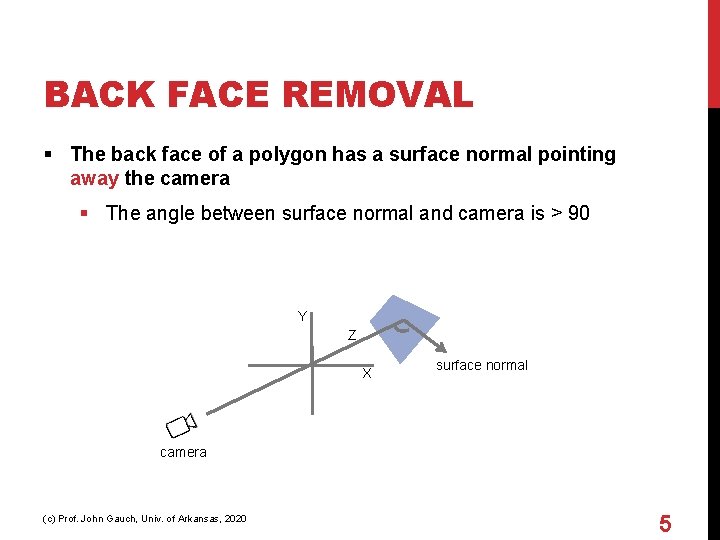
BACK FACE REMOVAL § The back face of a polygon has a surface normal pointing away the camera § The angle between surface normal and camera is > 90 Y Z X surface normal camera (c) Prof. John Gauch, Univ. of Arkansas, 2020 5
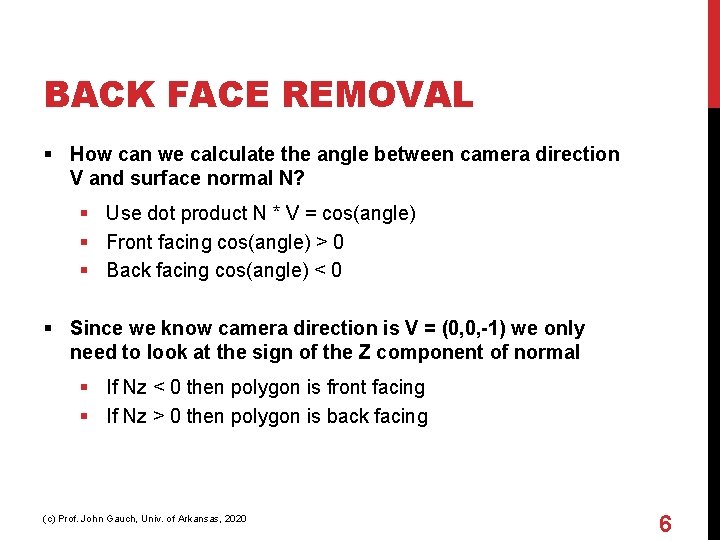
BACK FACE REMOVAL § How can we calculate the angle between camera direction V and surface normal N? § Use dot product N * V = cos(angle) § Front facing cos(angle) > 0 § Back facing cos(angle) < 0 § Since we know camera direction is V = (0, 0, -1) we only need to look at the sign of the Z component of normal § If Nz < 0 then polygon is front facing § If Nz > 0 then polygon is back facing (c) Prof. John Gauch, Univ. of Arkansas, 2020 6
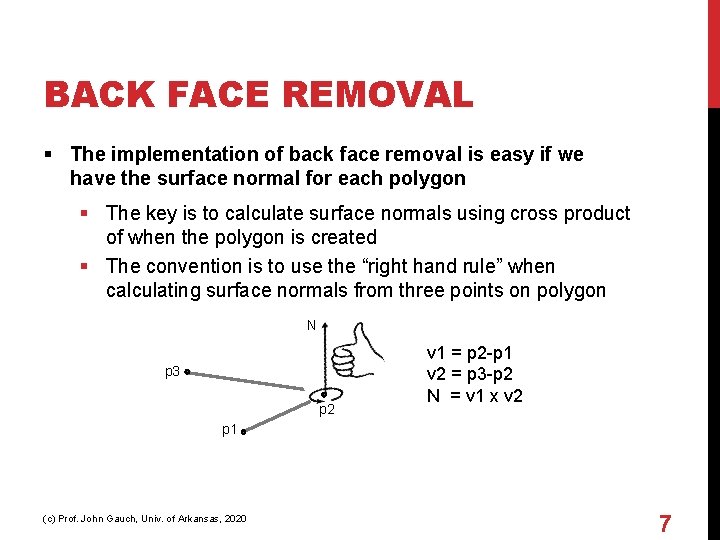
BACK FACE REMOVAL § The implementation of back face removal is easy if we have the surface normal for each polygon § The key is to calculate surface normals using cross product of when the polygon is created § The convention is to use the “right hand rule” when calculating surface normals from three points on polygon N p 3 p 2 v 1 = p 2 -p 1 v 2 = p 3 -p 2 N = v 1 x v 2 p 1 (c) Prof. John Gauch, Univ. of Arkansas, 2020 7
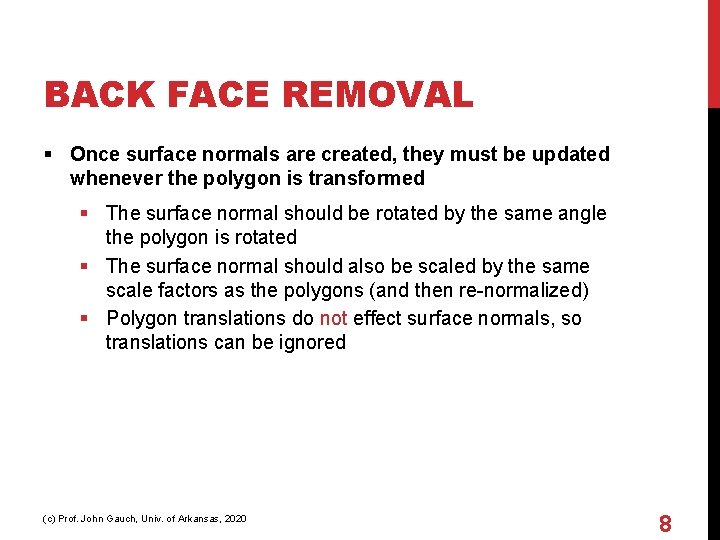
BACK FACE REMOVAL § Once surface normals are created, they must be updated whenever the polygon is transformed § The surface normal should be rotated by the same angle the polygon is rotated § The surface normal should also be scaled by the same scale factors as the polygons (and then re-normalized) § Polygon translations do not effect surface normals, so translations can be ignored (c) Prof. John Gauch, Univ. of Arkansas, 2020 8
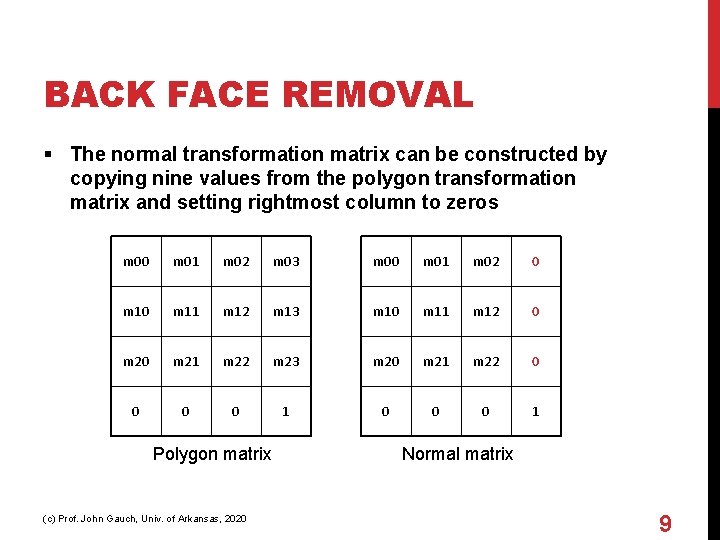
BACK FACE REMOVAL § The normal transformation matrix can be constructed by copying nine values from the polygon transformation matrix and setting rightmost column to zeros m 00 m 01 m 02 m 03 m 00 m 01 m 02 0 m 11 m 12 m 13 m 10 m 11 m 12 0 m 21 m 22 m 23 m 20 m 21 m 22 0 0 1 0 0 0 1 Polygon matrix (c) Prof. John Gauch, Univ. of Arkansas, 2020 Normal matrix 9
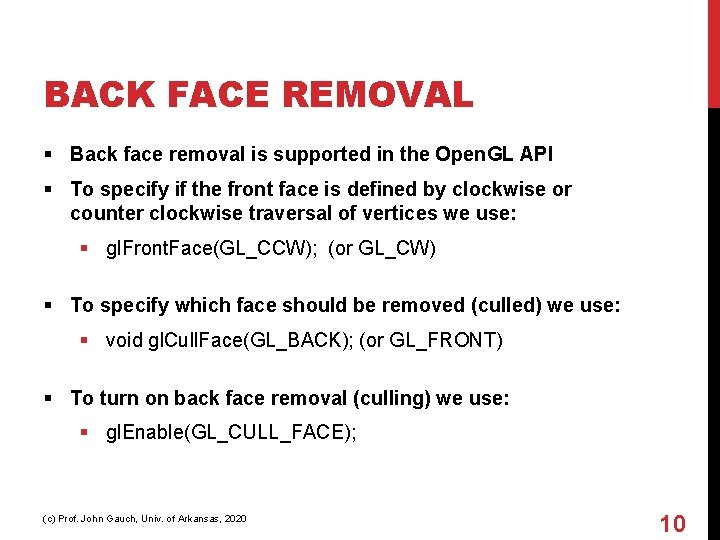
BACK FACE REMOVAL § Back face removal is supported in the Open. GL API § To specify if the front face is defined by clockwise or counter clockwise traversal of vertices we use: § gl. Front. Face(GL_CCW); (or GL_CW) § To specify which face should be removed (culled) we use: § void gl. Cull. Face(GL_BACK); (or GL_FRONT) § To turn on back face removal (culling) we use: § gl. Enable(GL_CULL_FACE); (c) Prof. John Gauch, Univ. of Arkansas, 2020 10
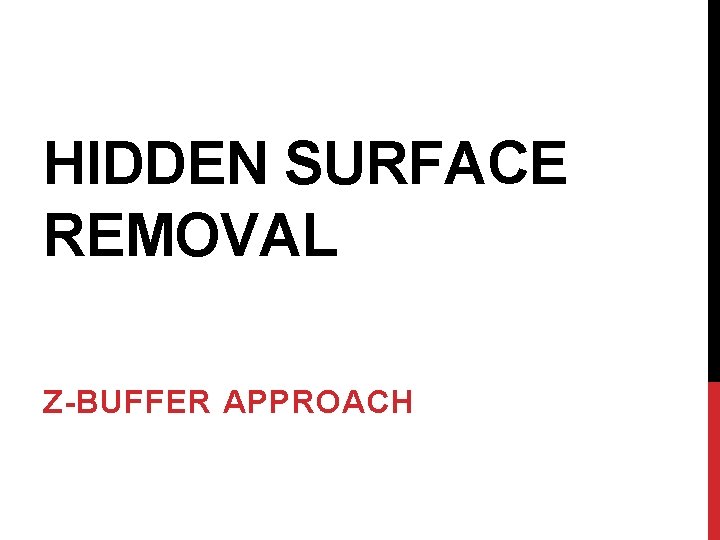
HIDDEN SURFACE REMOVAL Z-BUFFER APPROACH
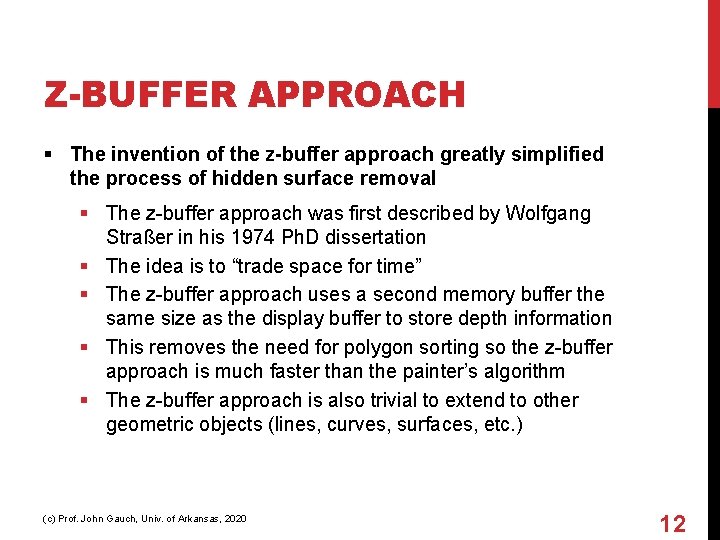
Z-BUFFER APPROACH § The invention of the z-buffer approach greatly simplified the process of hidden surface removal § The z-buffer approach was first described by Wolfgang Straßer in his 1974 Ph. D dissertation § The idea is to “trade space for time” § The z-buffer approach uses a second memory buffer the same size as the display buffer to store depth information § This removes the need for polygon sorting so the z-buffer approach is much faster than the painter’s algorithm § The z-buffer approach is also trivial to extend to other geometric objects (lines, curves, surfaces, etc. ) (c) Prof. John Gauch, Univ. of Arkansas, 2020 12
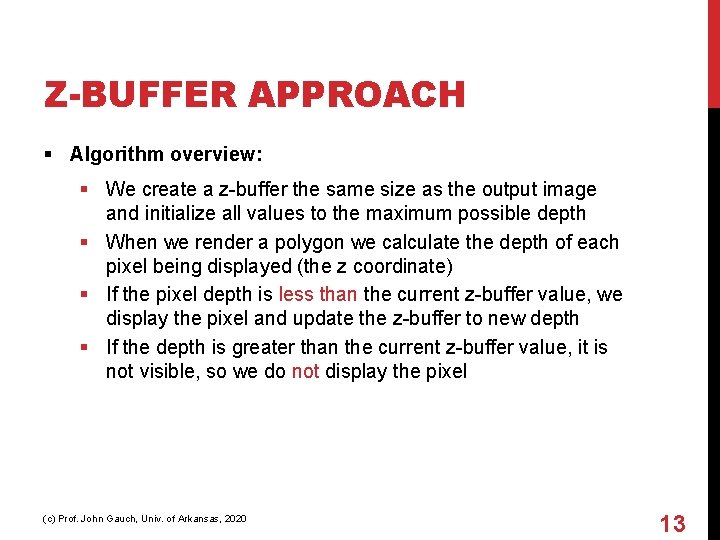
Z-BUFFER APPROACH § Algorithm overview: § We create a z-buffer the same size as the output image and initialize all values to the maximum possible depth § When we render a polygon we calculate the depth of each pixel being displayed (the z coordinate) § If the pixel depth is less than the current z-buffer value, we display the pixel and update the z-buffer to new depth § If the depth is greater than the current z-buffer value, it is not visible, so we do not display the pixel (c) Prof. John Gauch, Univ. of Arkansas, 2020 13
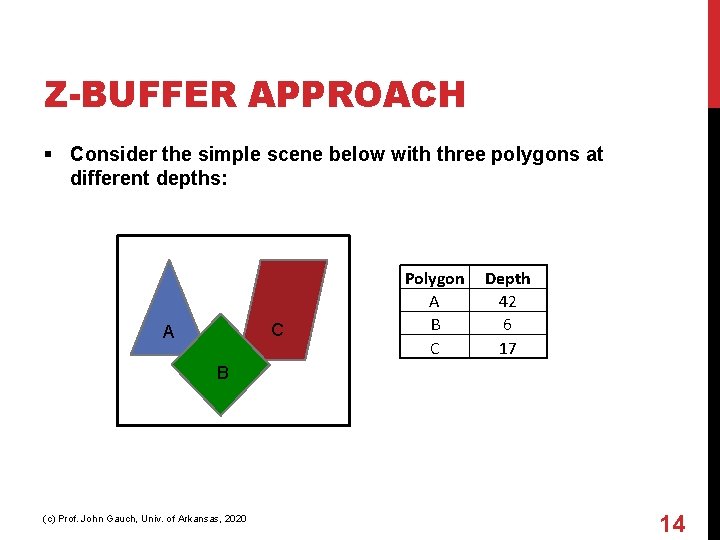
Z-BUFFER APPROACH § Consider the simple scene below with three polygons at different depths: C A Polygon A B C Depth 42 6 17 B (c) Prof. John Gauch, Univ. of Arkansas, 2020 14
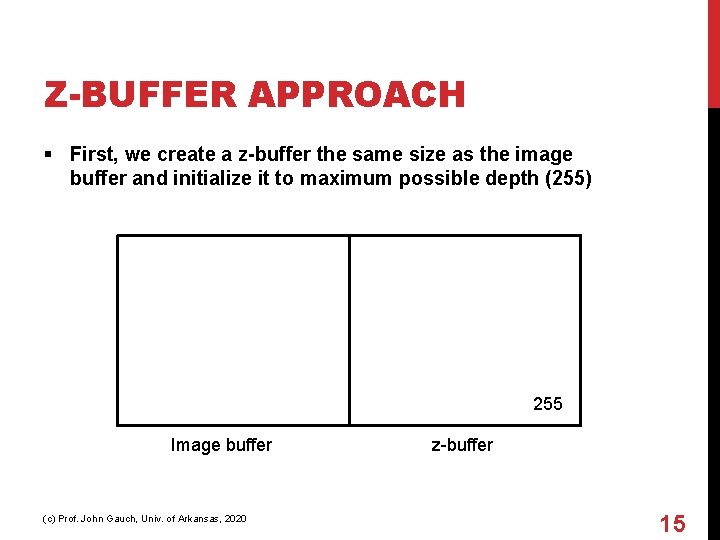
Z-BUFFER APPROACH § First, we create a z-buffer the same size as the image buffer and initialize it to maximum possible depth (255) 255 Image buffer (c) Prof. John Gauch, Univ. of Arkansas, 2020 z-buffer 15
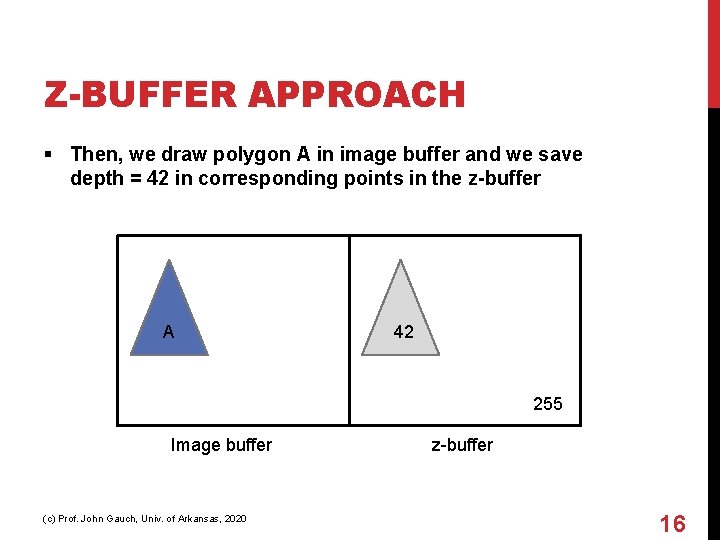
Z-BUFFER APPROACH § Then, we draw polygon A in image buffer and we save depth = 42 in corresponding points in the z-buffer A 42 255 Image buffer (c) Prof. John Gauch, Univ. of Arkansas, 2020 z-buffer 16
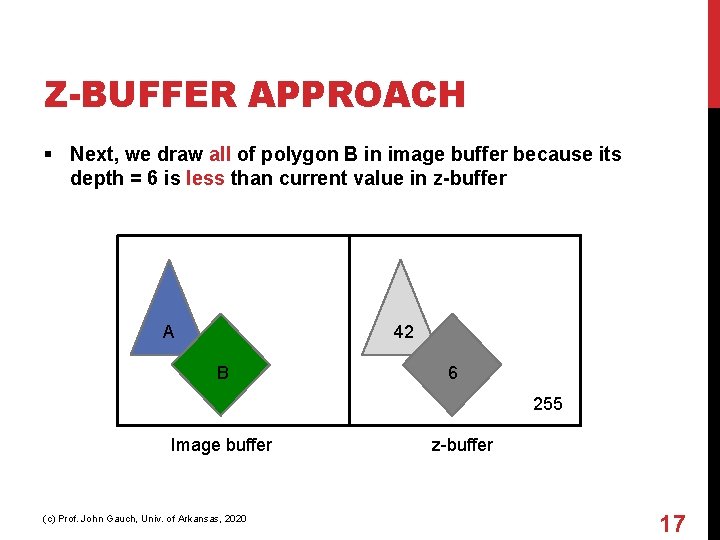
Z-BUFFER APPROACH § Next, we draw all of polygon B in image buffer because its depth = 6 is less than current value in z-buffer A 42 B 6 255 Image buffer (c) Prof. John Gauch, Univ. of Arkansas, 2020 z-buffer 17
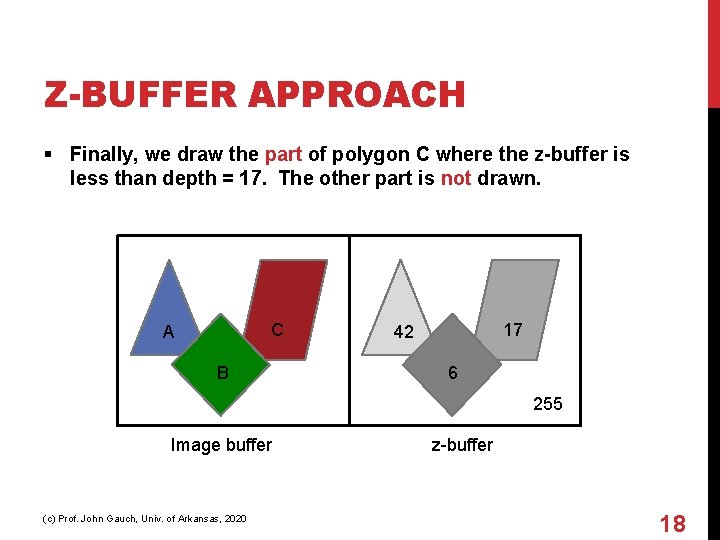
Z-BUFFER APPROACH § Finally, we draw the part of polygon C where the z-buffer is less than depth = 17. The other part is not drawn. C A B 17 42 6 255 Image buffer (c) Prof. John Gauch, Univ. of Arkansas, 2020 z-buffer 18
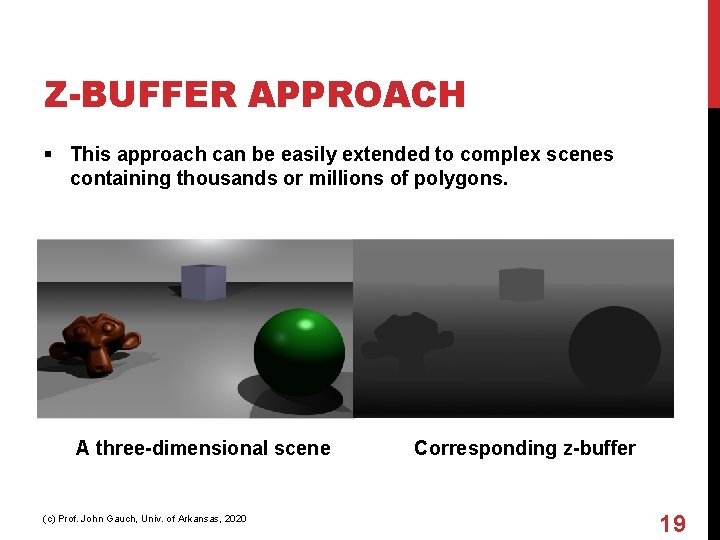
Z-BUFFER APPROACH § This approach can be easily extended to complex scenes containing thousands or millions of polygons. A three-dimensional scene (c) Prof. John Gauch, Univ. of Arkansas, 2020 Corresponding z-buffer 19
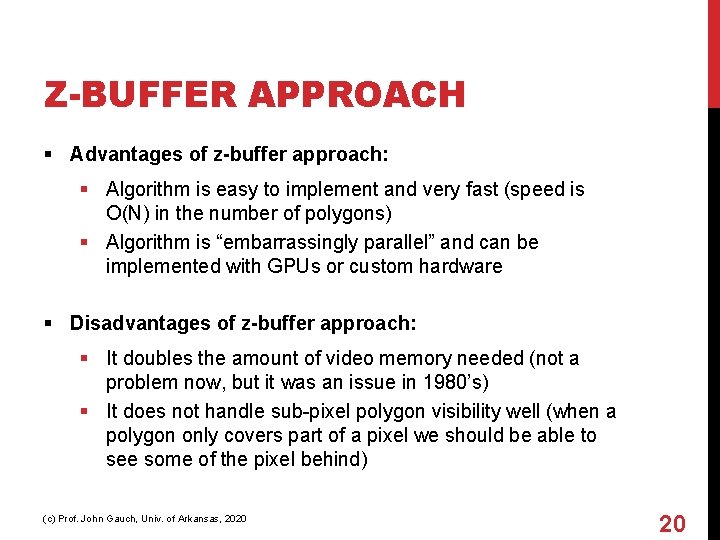
Z-BUFFER APPROACH § Advantages of z-buffer approach: § Algorithm is easy to implement and very fast (speed is O(N) in the number of polygons) § Algorithm is “embarrassingly parallel” and can be implemented with GPUs or custom hardware § Disadvantages of z-buffer approach: § It doubles the amount of video memory needed (not a problem now, but it was an issue in 1980’s) § It does not handle sub-pixel polygon visibility well (when a polygon only covers part of a pixel we should be able to see some of the pixel behind) (c) Prof. John Gauch, Univ. of Arkansas, 2020 20
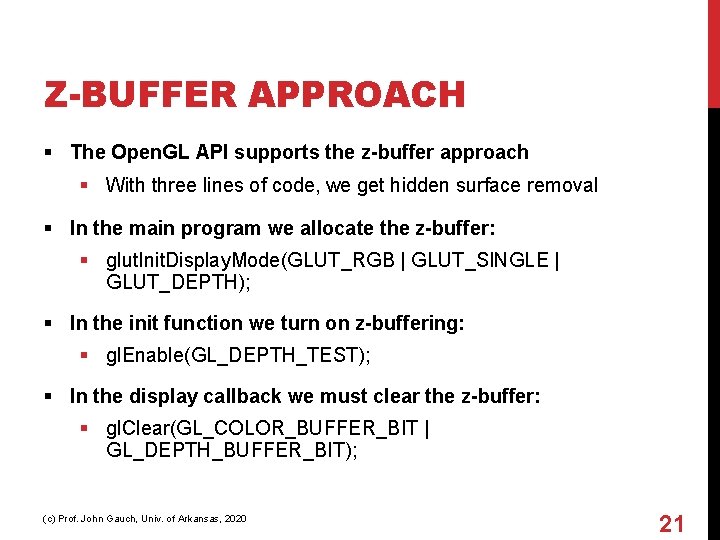
Z-BUFFER APPROACH § The Open. GL API supports the z-buffer approach § With three lines of code, we get hidden surface removal § In the main program we allocate the z-buffer: § glut. Init. Display. Mode(GLUT_RGB | GLUT_SINGLE | GLUT_DEPTH); § In the init function we turn on z-buffering: § gl. Enable(GL_DEPTH_TEST); § In the display callback we must clear the z-buffer: § gl. Clear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT); (c) Prof. John Gauch, Univ. of Arkansas, 2020 21
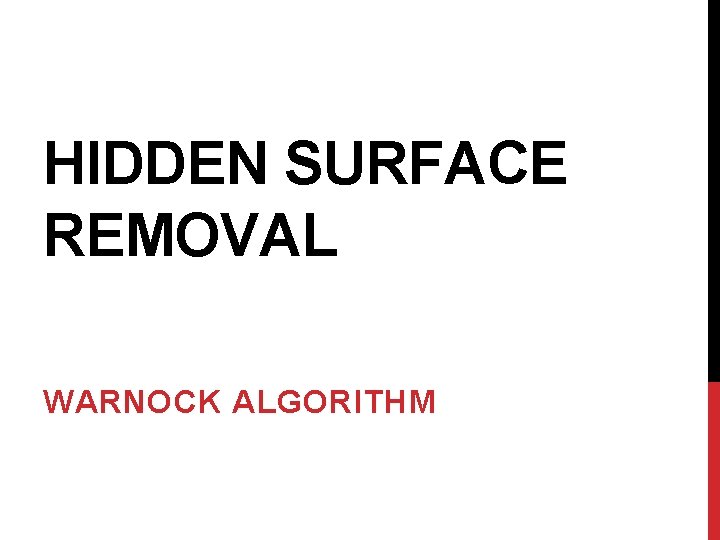
HIDDEN SURFACE REMOVAL WARNOCK ALGORITHM
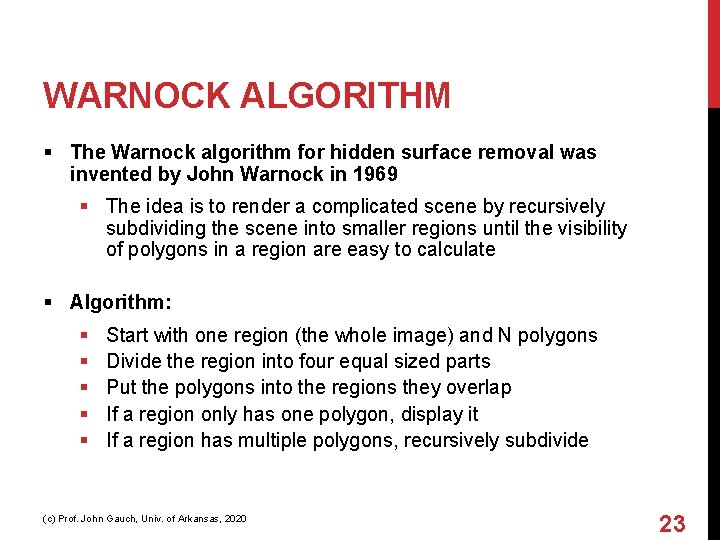
WARNOCK ALGORITHM § The Warnock algorithm for hidden surface removal was invented by John Warnock in 1969 § The idea is to render a complicated scene by recursively subdividing the scene into smaller regions until the visibility of polygons in a region are easy to calculate § Algorithm: § § § Start with one region (the whole image) and N polygons Divide the region into four equal sized parts Put the polygons into the regions they overlap If a region only has one polygon, display it If a region has multiple polygons, recursively subdivide (c) Prof. John Gauch, Univ. of Arkansas, 2020 23
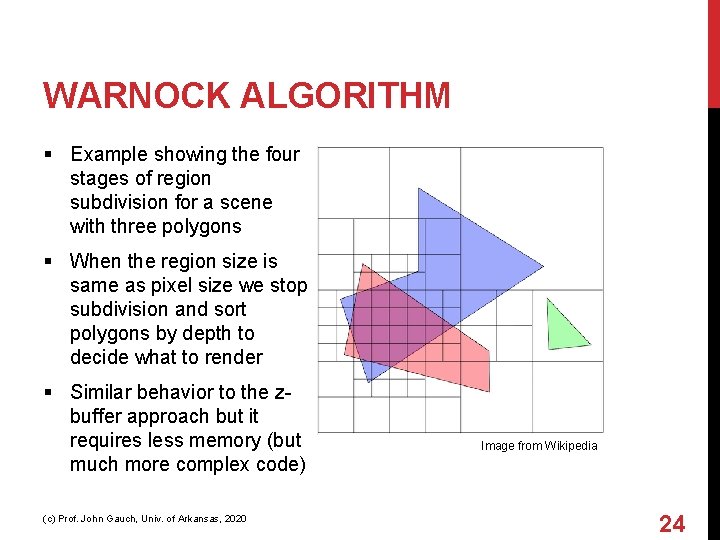
WARNOCK ALGORITHM § Example showing the four stages of region subdivision for a scene with three polygons § When the region size is same as pixel size we stop subdivision and sort polygons by depth to decide what to render § Similar behavior to the zbuffer approach but it requires less memory (but much more complex code) (c) Prof. John Gauch, Univ. of Arkansas, 2020 Image from Wikipedia 24
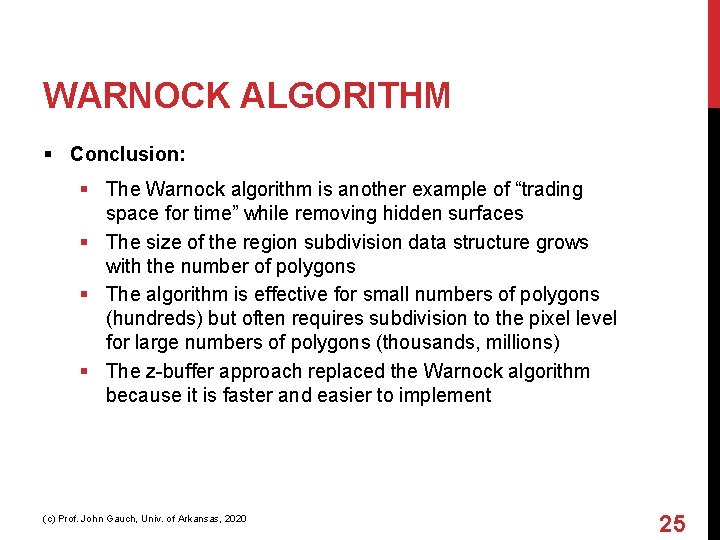
WARNOCK ALGORITHM § Conclusion: § The Warnock algorithm is another example of “trading space for time” while removing hidden surfaces § The size of the region subdivision data structure grows with the number of polygons § The algorithm is effective for small numbers of polygons (hundreds) but often requires subdivision to the pixel level for large numbers of polygons (thousands, millions) § The z-buffer approach replaced the Warnock algorithm because it is faster and easier to implement (c) Prof. John Gauch, Univ. of Arkansas, 2020 25
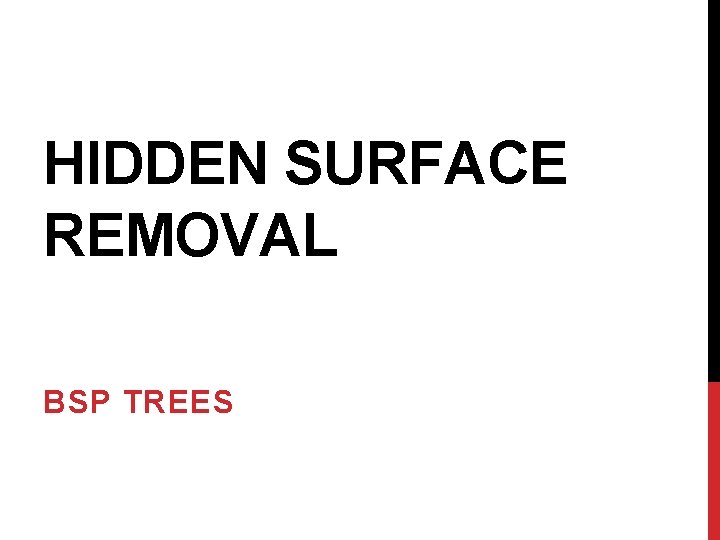
HIDDEN SURFACE REMOVAL BSP TREES
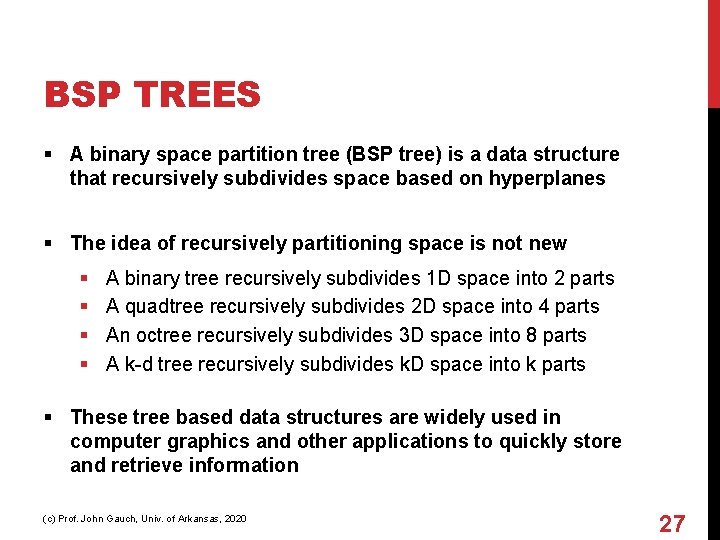
BSP TREES § A binary space partition tree (BSP tree) is a data structure that recursively subdivides space based on hyperplanes § The idea of recursively partitioning space is not new § § A binary tree recursively subdivides 1 D space into 2 parts A quadtree recursively subdivides 2 D space into 4 parts An octree recursively subdivides 3 D space into 8 parts A k-d tree recursively subdivides k. D space into k parts § These tree based data structures are widely used in computer graphics and other applications to quickly store and retrieve information (c) Prof. John Gauch, Univ. of Arkansas, 2020 27
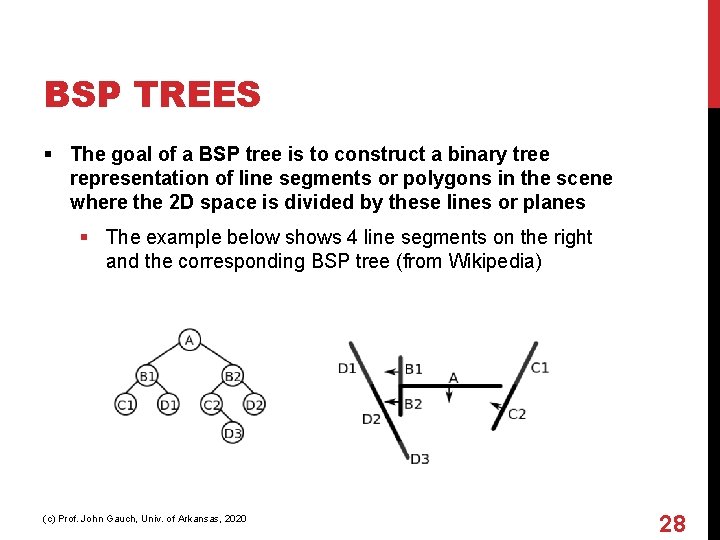
BSP TREES § The goal of a BSP tree is to construct a binary tree representation of line segments or polygons in the scene where the 2 D space is divided by these lines or planes § The example below shows 4 line segments on the right and the corresponding BSP tree (from Wikipedia) (c) Prof. John Gauch, Univ. of Arkansas, 2020 28
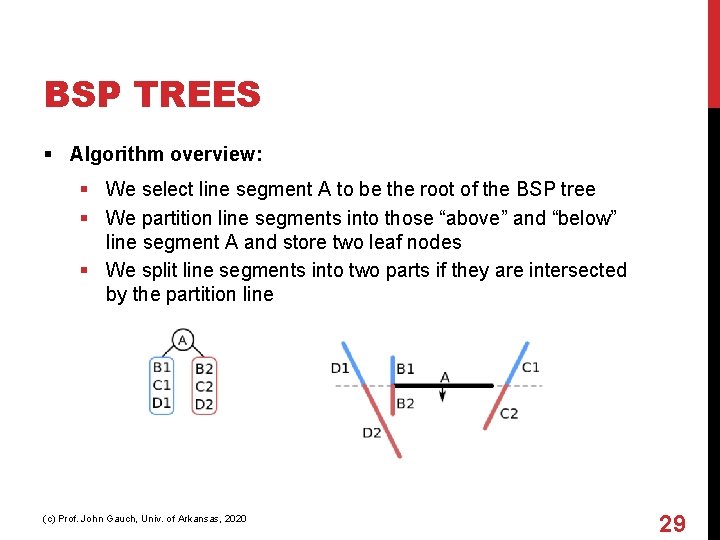
BSP TREES § Algorithm overview: § We select line segment A to be the root of the BSP tree § We partition line segments into those “above” and “below” line segment A and store two leaf nodes § We split line segments into two parts if they are intersected by the partition line (c) Prof. John Gauch, Univ. of Arkansas, 2020 29
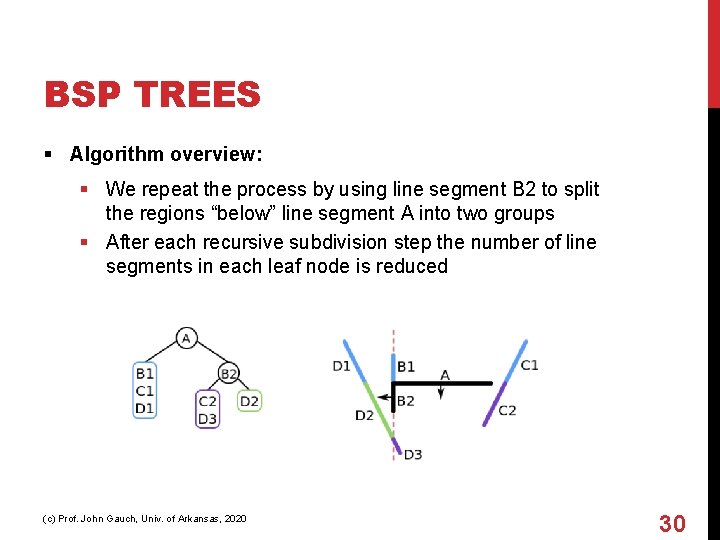
BSP TREES § Algorithm overview: § We repeat the process by using line segment B 2 to split the regions “below” line segment A into two groups § After each recursive subdivision step the number of line segments in each leaf node is reduced (c) Prof. John Gauch, Univ. of Arkansas, 2020 30
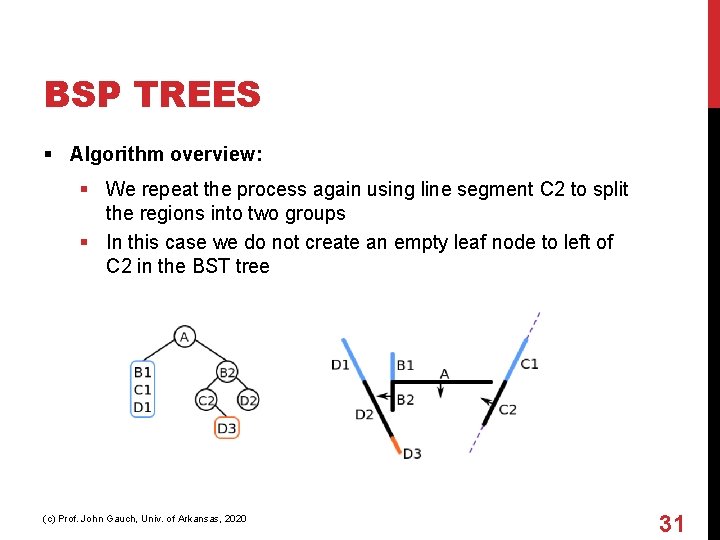
BSP TREES § Algorithm overview: § We repeat the process again using line segment C 2 to split the regions into two groups § In this case we do not create an empty leaf node to left of C 2 in the BST tree (c) Prof. John Gauch, Univ. of Arkansas, 2020 31
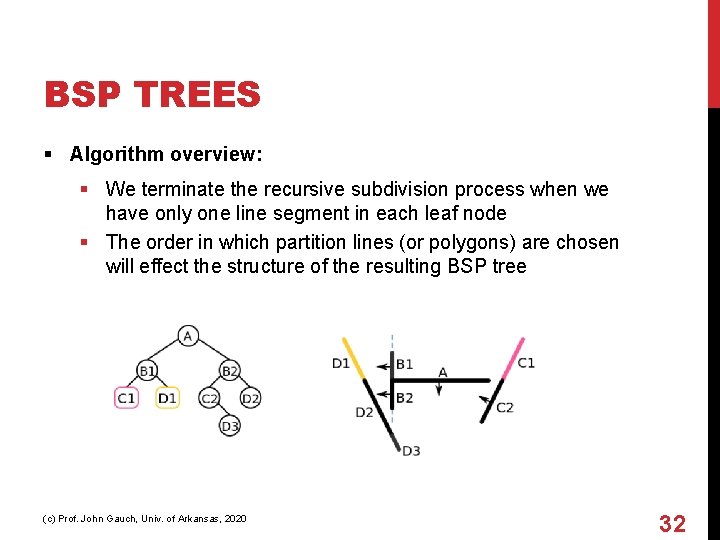
BSP TREES § Algorithm overview: § We terminate the recursive subdivision process when we have only one line segment in each leaf node § The order in which partition lines (or polygons) are chosen will effect the structure of the resulting BSP tree (c) Prof. John Gauch, Univ. of Arkansas, 2020 32
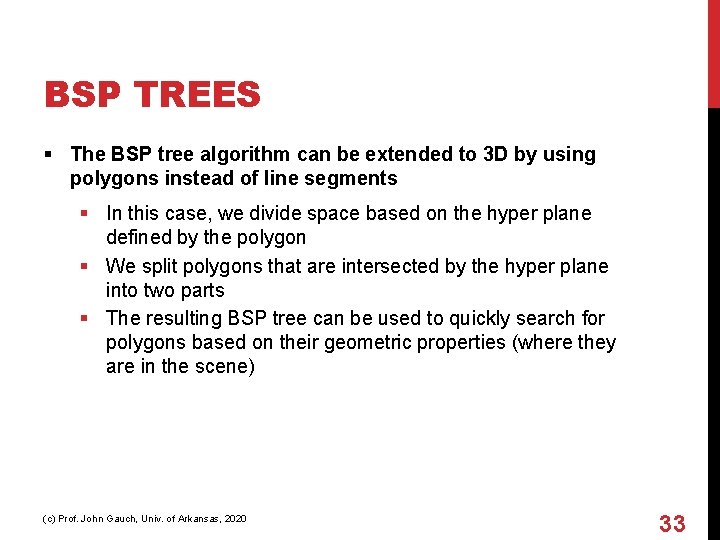
BSP TREES § The BSP tree algorithm can be extended to 3 D by using polygons instead of line segments § In this case, we divide space based on the hyper plane defined by the polygon § We split polygons that are intersected by the hyper plane into two parts § The resulting BSP tree can be used to quickly search for polygons based on their geometric properties (where they are in the scene) (c) Prof. John Gauch, Univ. of Arkansas, 2020 33
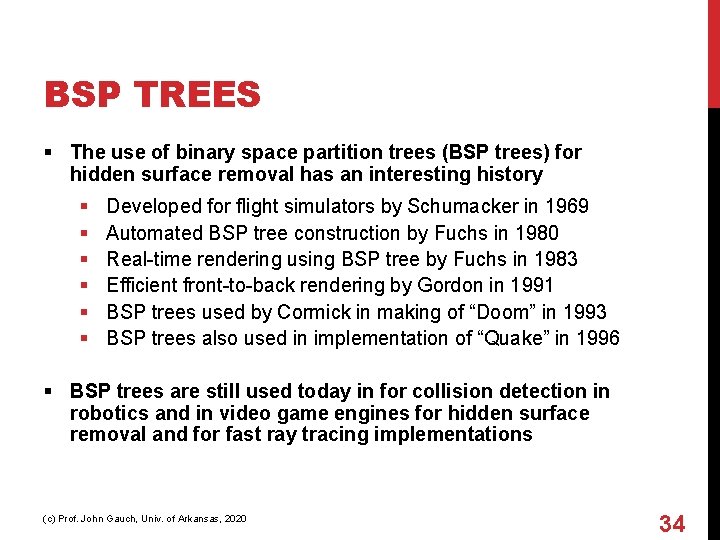
BSP TREES § The use of binary space partition trees (BSP trees) for hidden surface removal has an interesting history § § § Developed for flight simulators by Schumacker in 1969 Automated BSP tree construction by Fuchs in 1980 Real-time rendering using BSP tree by Fuchs in 1983 Efficient front-to-back rendering by Gordon in 1991 BSP trees used by Cormick in making of “Doom” in 1993 BSP trees also used in implementation of “Quake” in 1996 § BSP trees are still used today in for collision detection in robotics and in video game engines for hidden surface removal and for fast ray tracing implementations (c) Prof. John Gauch, Univ. of Arkansas, 2020 34
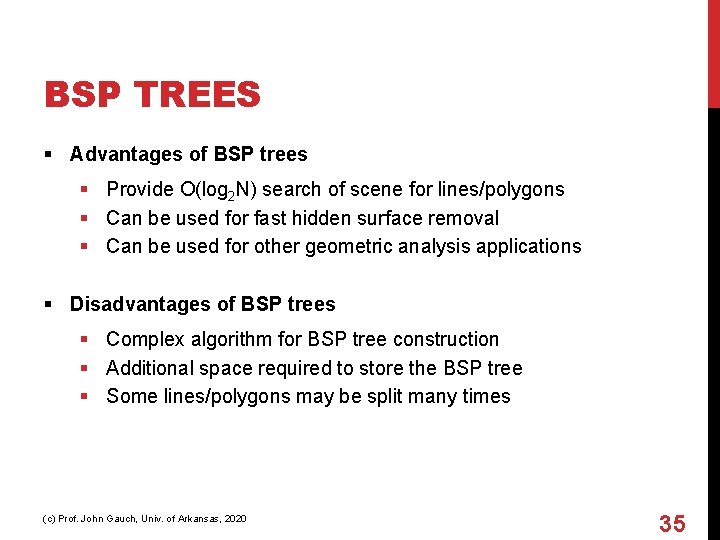
BSP TREES § Advantages of BSP trees § Provide O(log 2 N) search of scene for lines/polygons § Can be used for fast hidden surface removal § Can be used for other geometric analysis applications § Disadvantages of BSP trees § Complex algorithm for BSP tree construction § Additional space required to store the BSP tree § Some lines/polygons may be split many times (c) Prof. John Gauch, Univ. of Arkansas, 2020 35
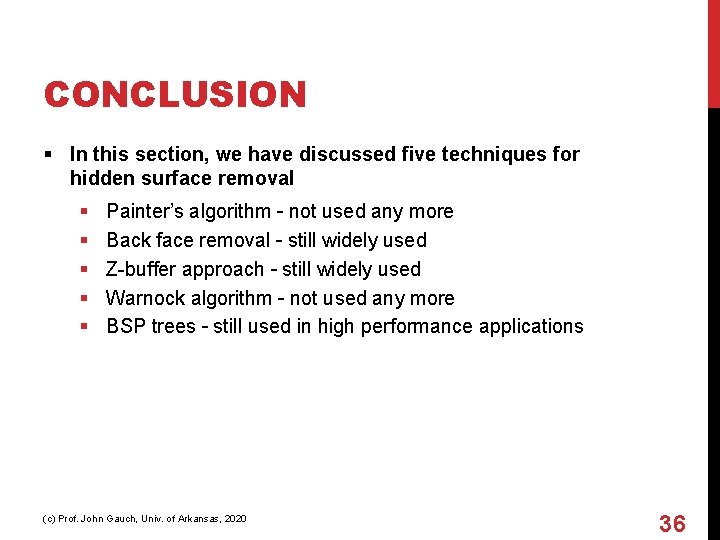
CONCLUSION § In this section, we have discussed five techniques for hidden surface removal § § § Painter’s algorithm – not used any more Back face removal – still widely used Z-buffer approach – still widely used Warnock algorithm – not used any more BSP trees – still used in high performance applications (c) Prof. John Gauch, Univ. of Arkansas, 2020 36
John gauch
Hidden surface removal algorithm in computer graphics
Susan gauch
Susan gauch
Graphic monitor and workstation in computer graphics
Lcd working principle ppt
1stanza
Passenger transport safety
Angel
Define projection in computer graphics
What is plasma panel display in computer graphics
Interior and exterior clipping in computer graphics
Shear transformation in computer graphics
Glsl sincos
Scan conversion of ellipse in computer graphics
Rigid body transformation in computer graphics
Is the process of filling image or region
Starburst method in computer graphics
Polygon filling algorithm in computer graphics
Random scan display is also called as
Computer graphics
Algorithm to draw a line in computer graphics
Cs 418
Glcreatebuffer
Achromatic light in computer graphics
What are the interactive input methods?
Uniform scaling in computer graphics
Uniform scaling in computer graphics
Fundamentals of computer graphics
Logical input devices in computer graphics
Fundamentals of computer graphics
Polygon clipping in computer graphics ppt
Introduction to computer graphics ppt
Dda in computer graphics
What is window and viewport in computer graphics
Keyframe animation in computer graphics
Solid examples