CS 101 Introduction to Computing Color replacements and
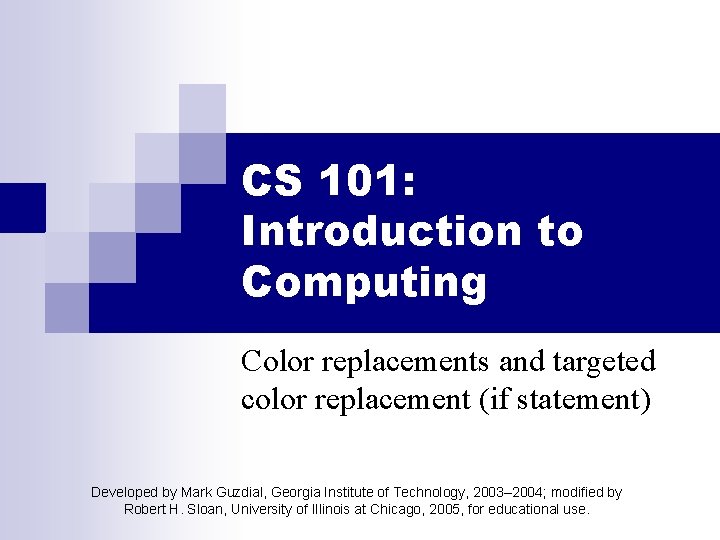
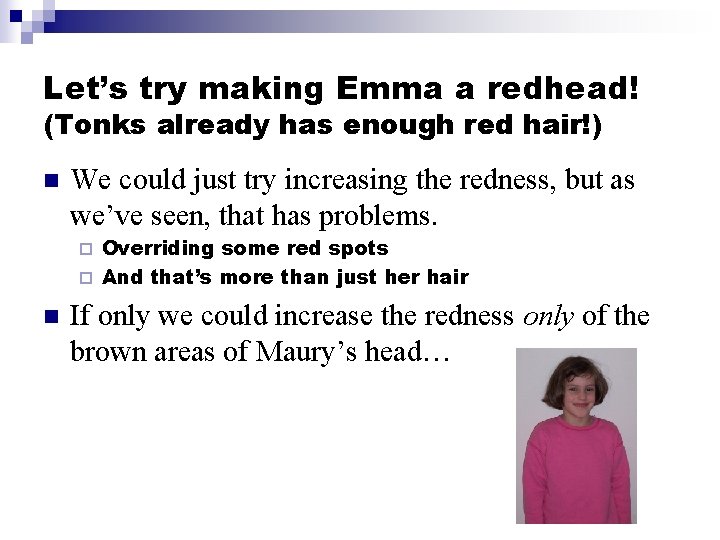
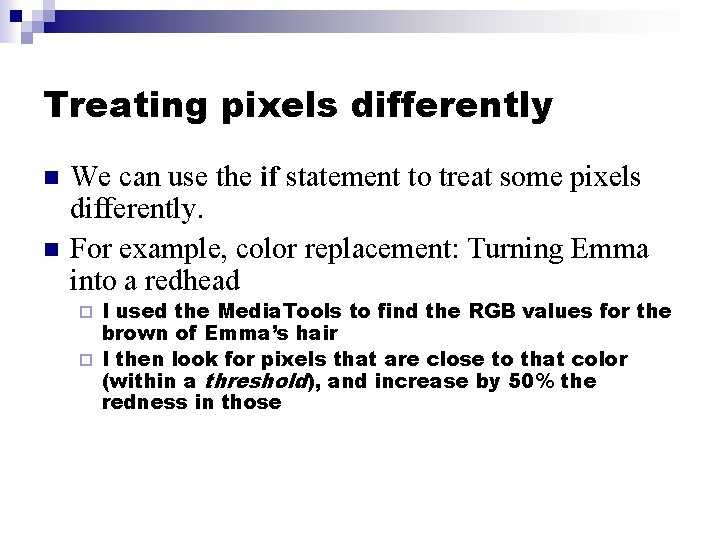
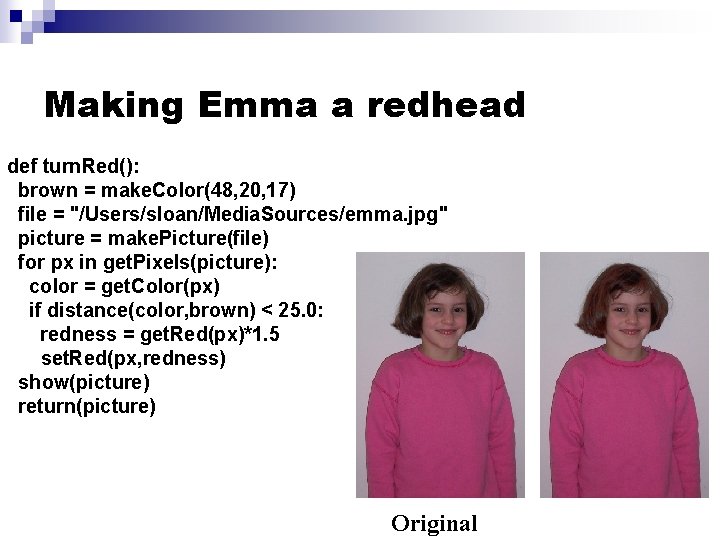
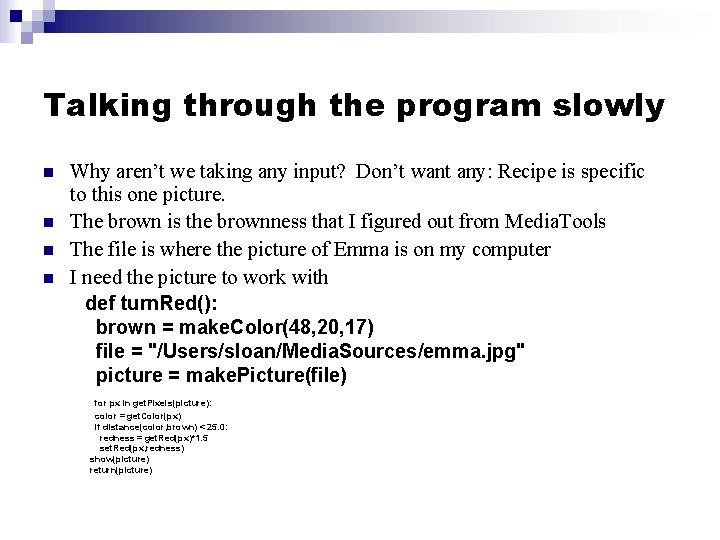
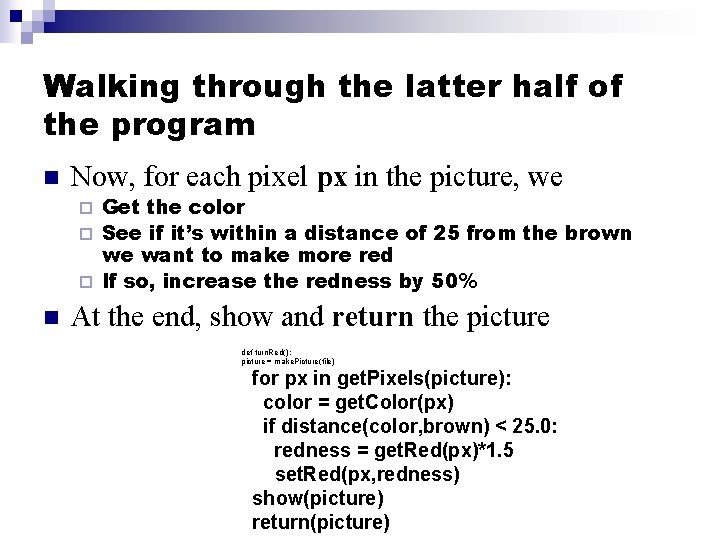
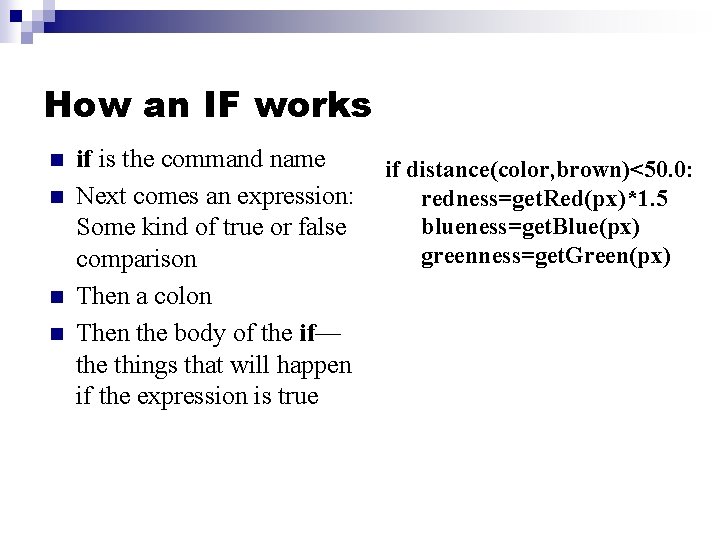
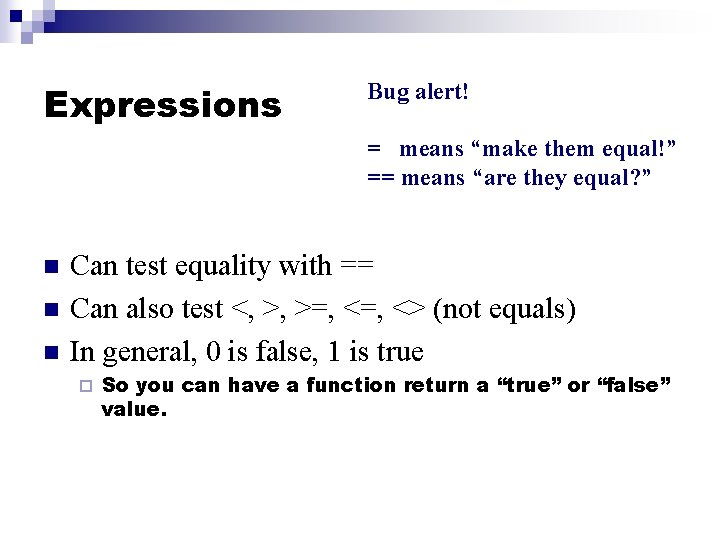
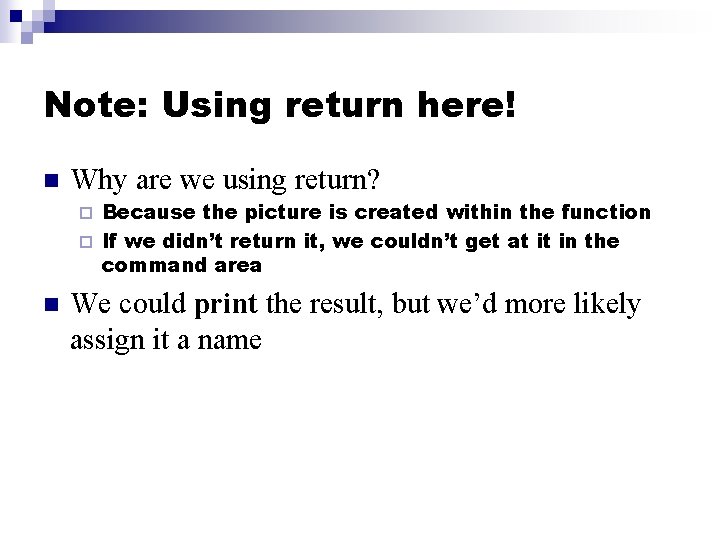
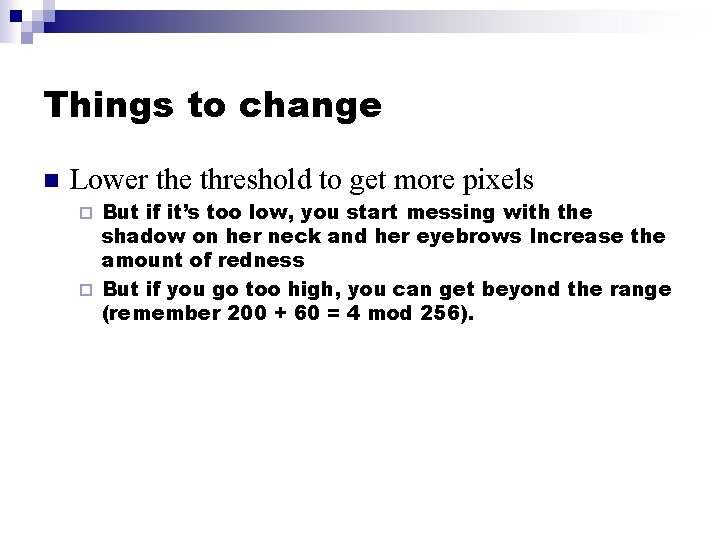
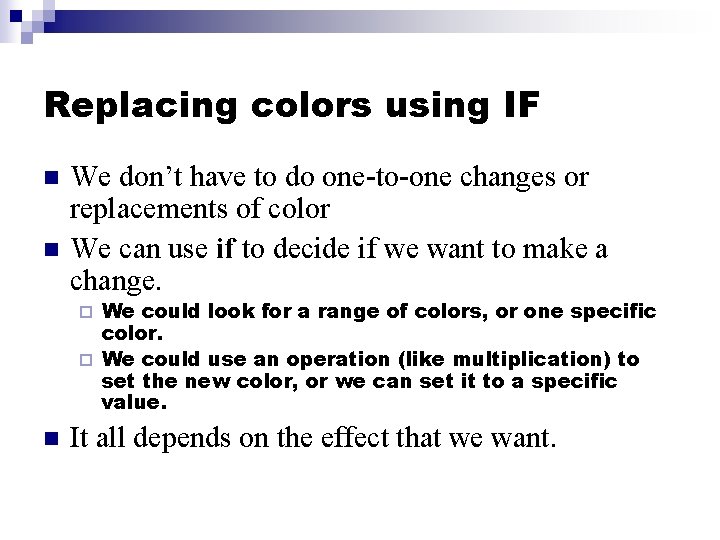
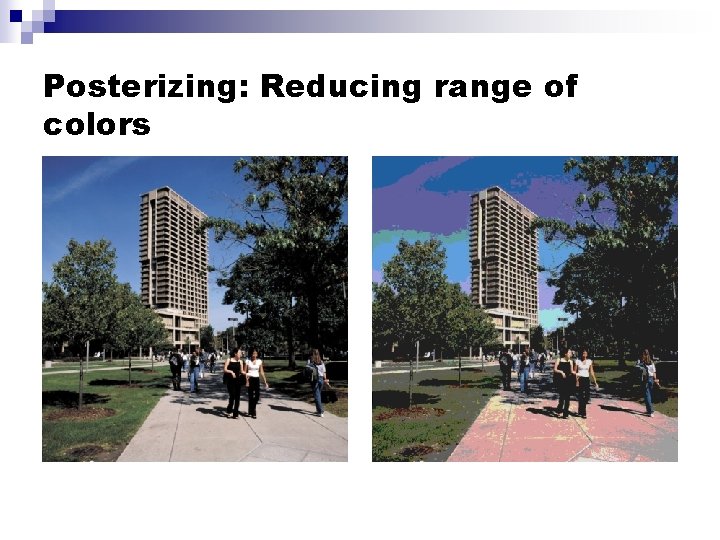
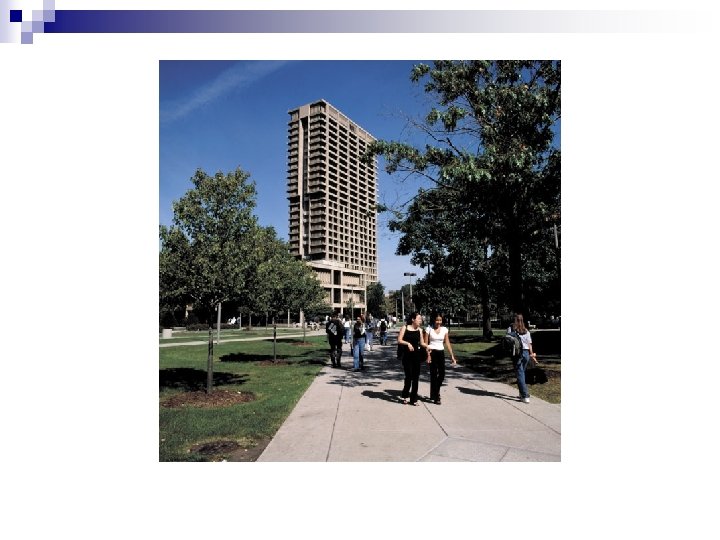
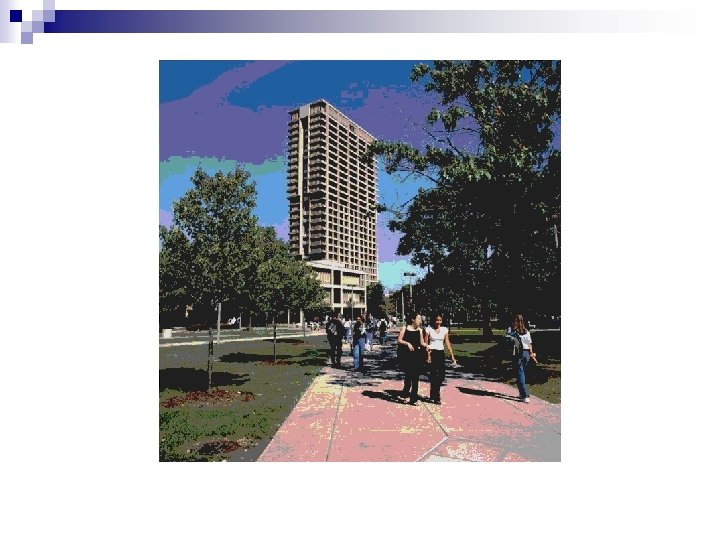
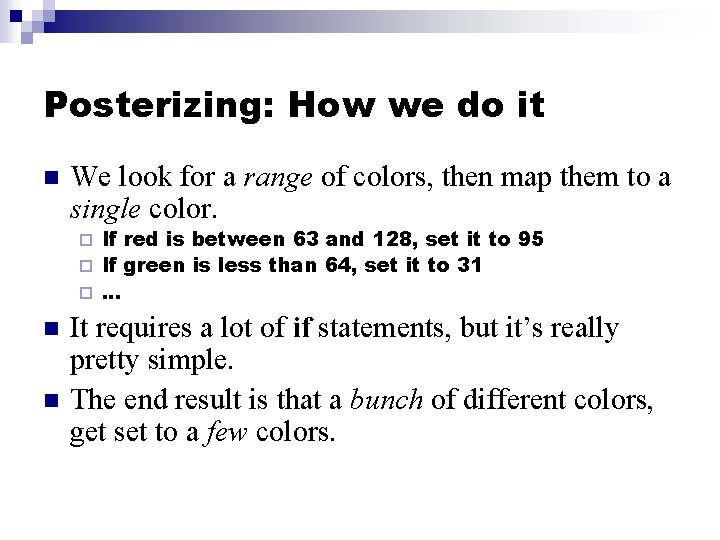
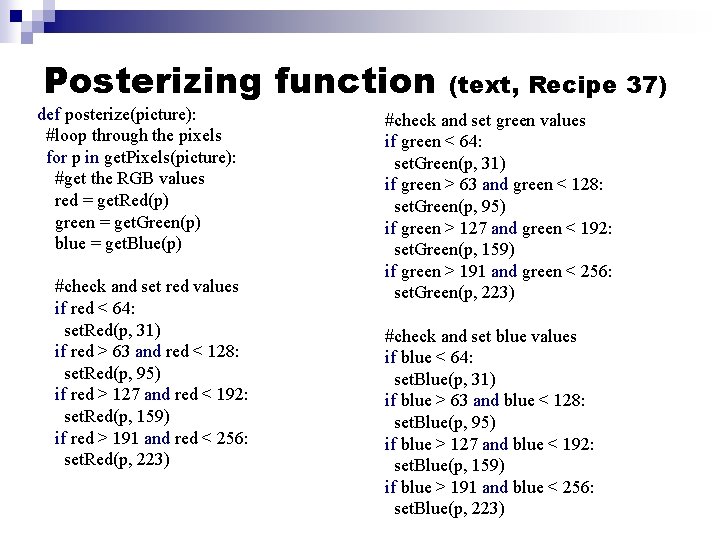
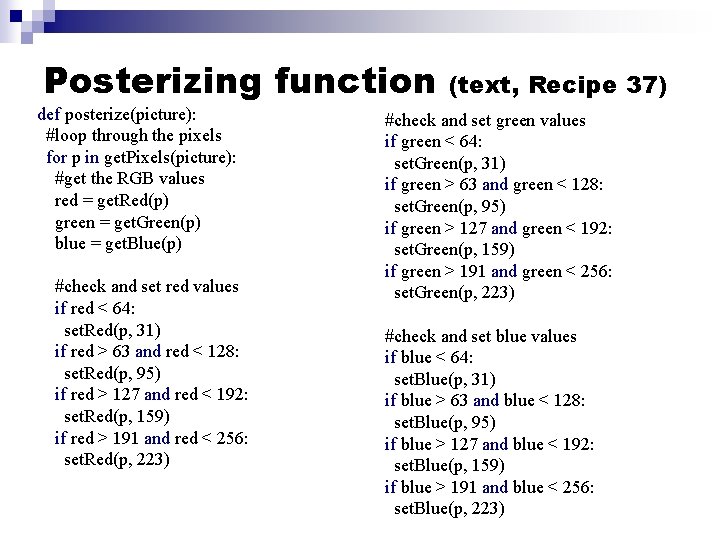
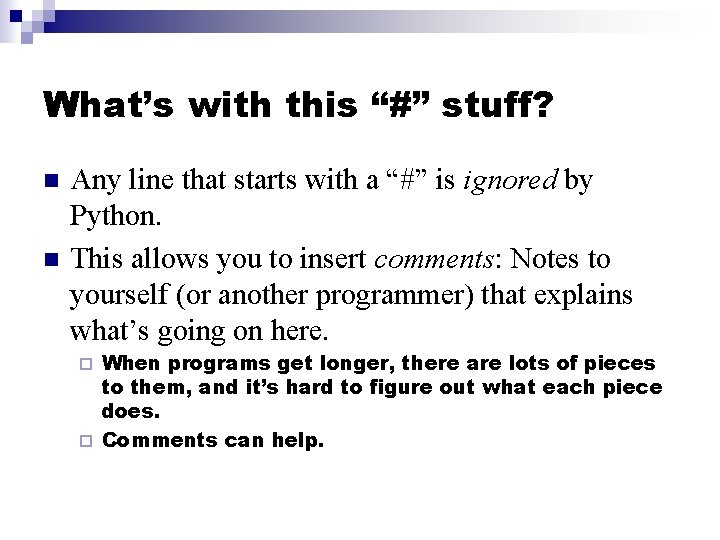
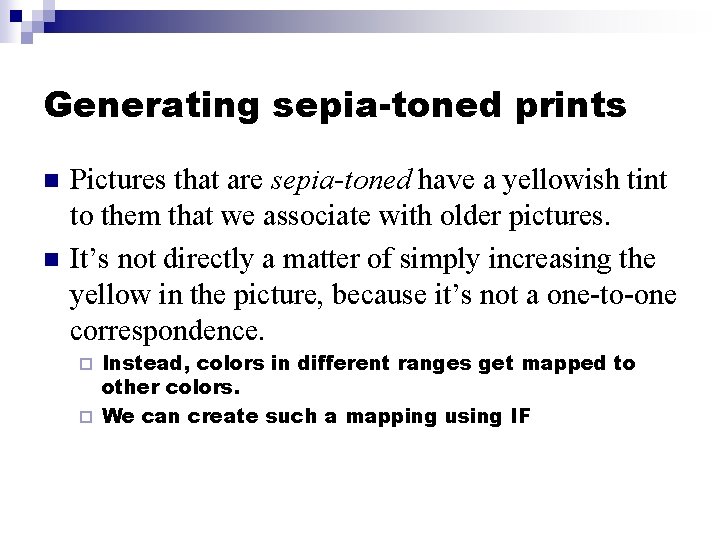
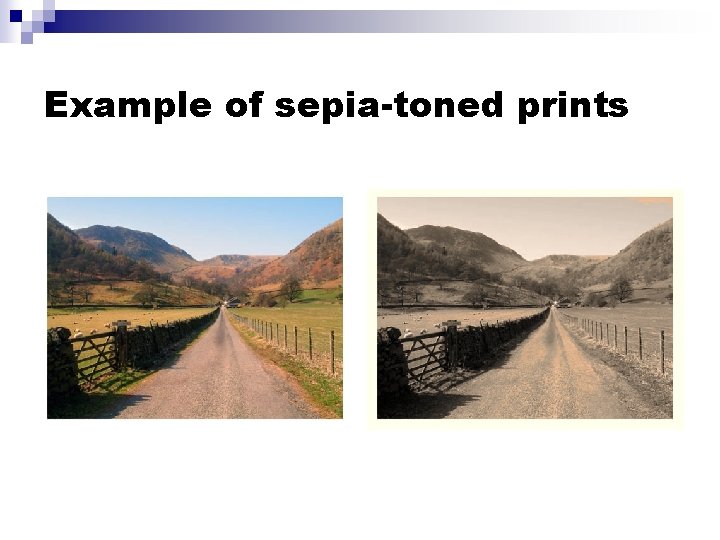
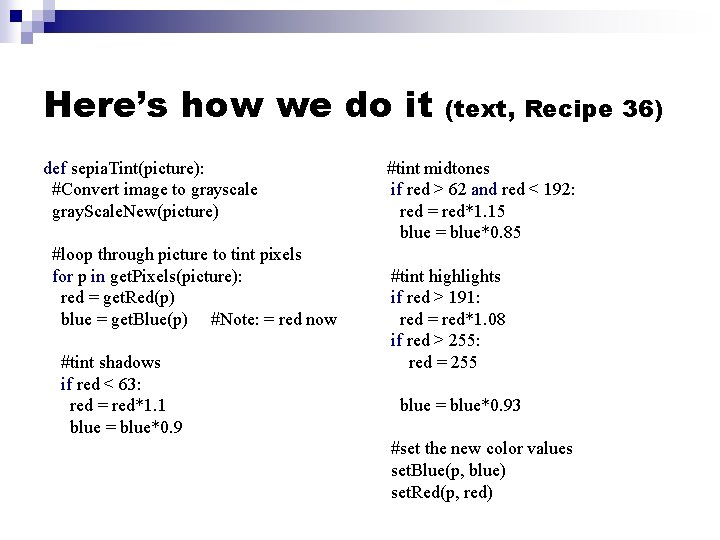
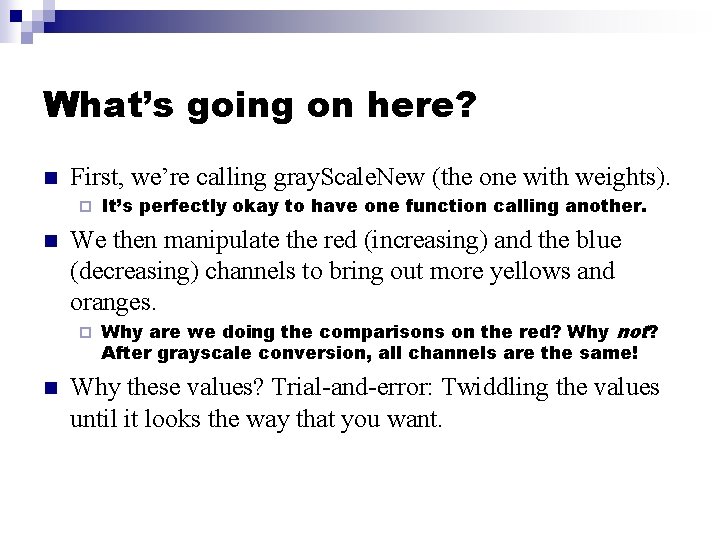
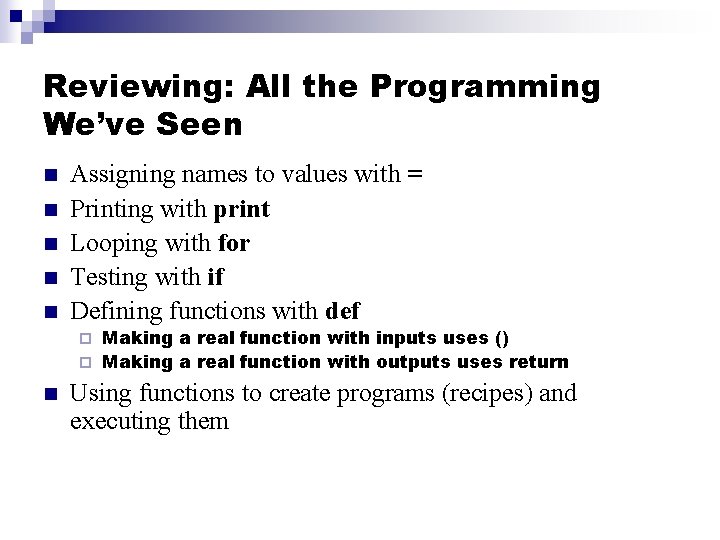
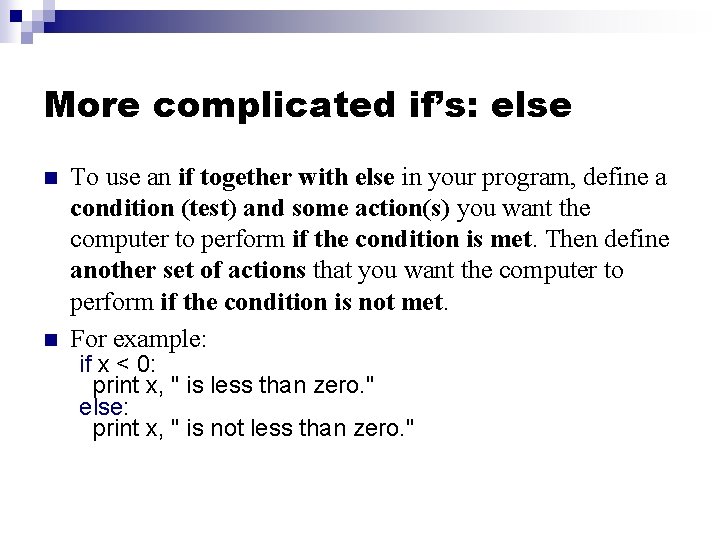
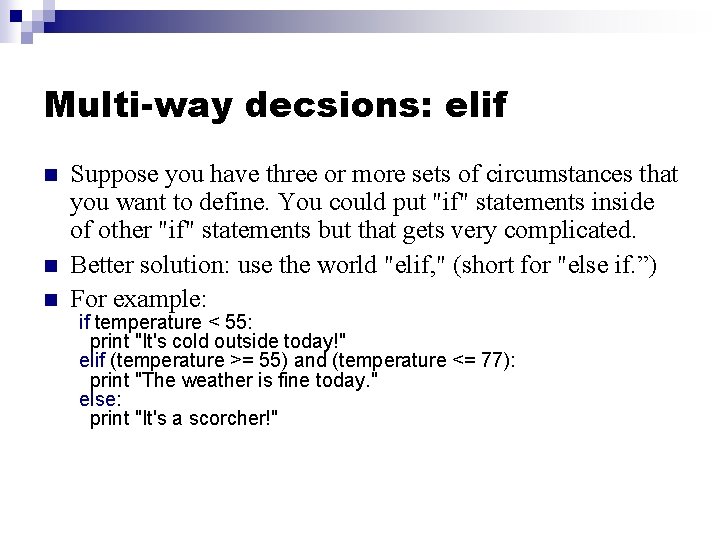
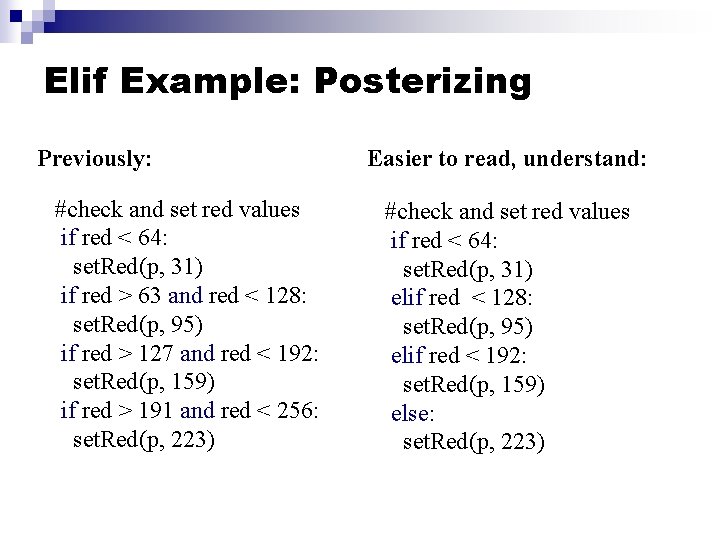
- Slides: 26
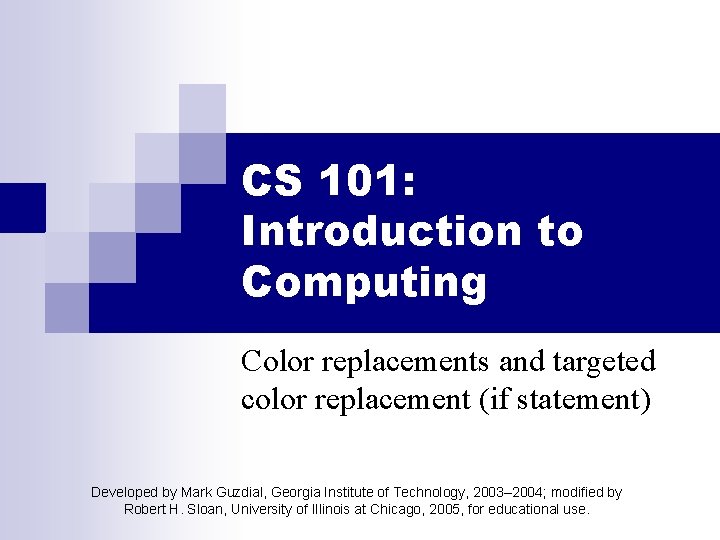
CS 101: Introduction to Computing Color replacements and targeted color replacement (if statement) Developed by Mark Guzdial, Georgia Institute of Technology, 2003– 2004; modified by Robert H. Sloan, University of Illinois at Chicago, 2005, for educational use.
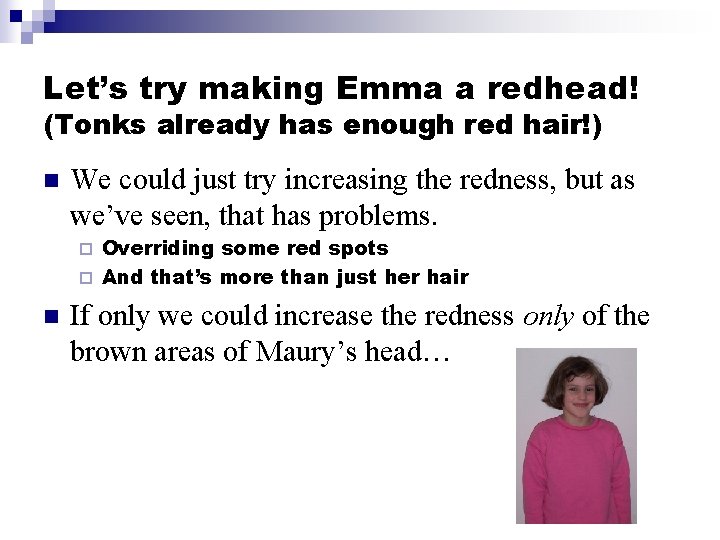
Let’s try making Emma a redhead! (Tonks already has enough red hair!) n We could just try increasing the redness, but as we’ve seen, that has problems. Overriding some red spots ¨ And that’s more than just her hair ¨ n If only we could increase the redness only of the brown areas of Maury’s head…
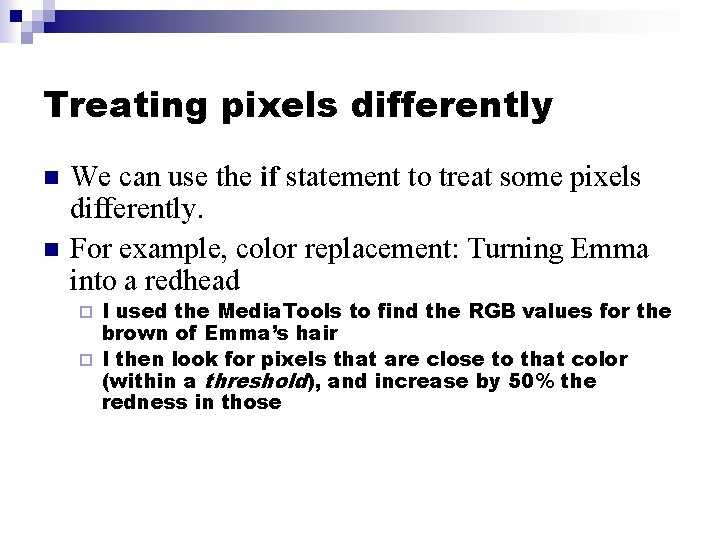
Treating pixels differently n n We can use the if statement to treat some pixels differently. For example, color replacement: Turning Emma into a redhead I used the Media. Tools to find the RGB values for the brown of Emma’s hair ¨ I then look for pixels that are close to that color (within a threshold), and increase by 50% the redness in those ¨
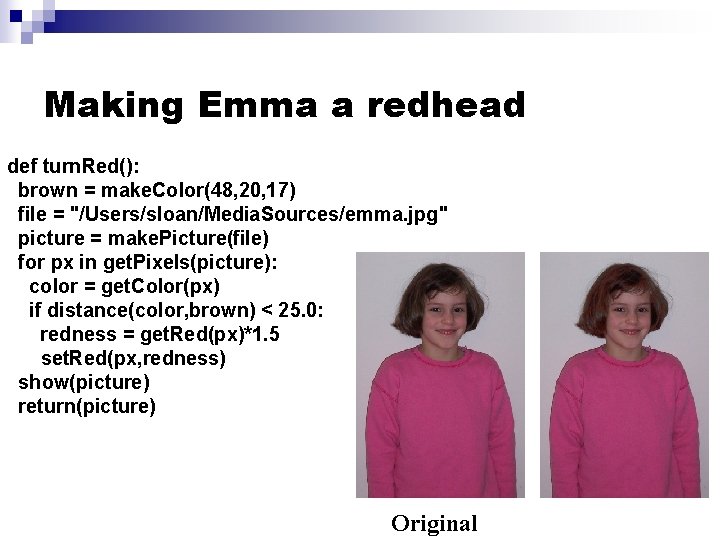
Making Emma a redhead def turn. Red(): brown = make. Color(48, 20, 17) file = "/Users/sloan/Media. Sources/emma. jpg" picture = make. Picture(file) for px in get. Pixels(picture): color = get. Color(px) if distance(color, brown) < 25. 0: redness = get. Red(px)*1. 5 set. Red(px, redness) show(picture) return(picture) Original
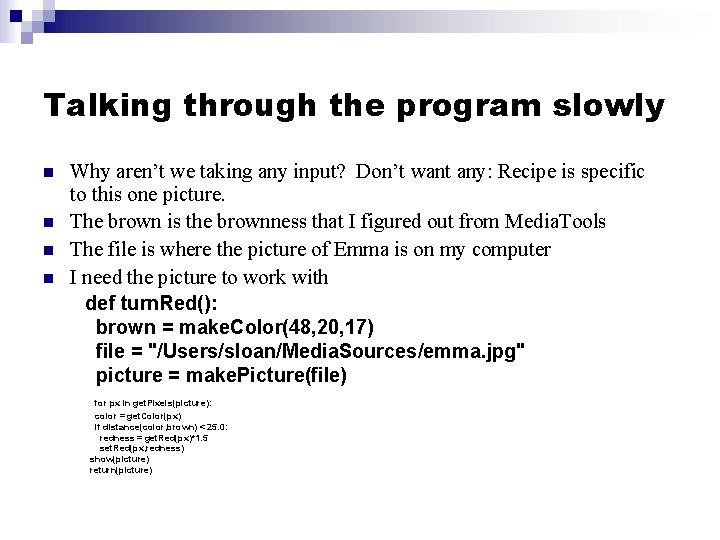
Talking through the program slowly n n Why aren’t we taking any input? Don’t want any: Recipe is specific to this one picture. The brown is the brownness that I figured out from Media. Tools The file is where the picture of Emma is on my computer I need the picture to work with def turn. Red(): brown = make. Color(48, 20, 17) file = "/Users/sloan/Media. Sources/emma. jpg" picture = make. Picture(file) for px in get. Pixels(picture): color = get. Color(px) if distance(color, brown) < 25. 0: redness = get. Red(px)*1. 5 set. Red(px, redness) show(picture) return(picture)
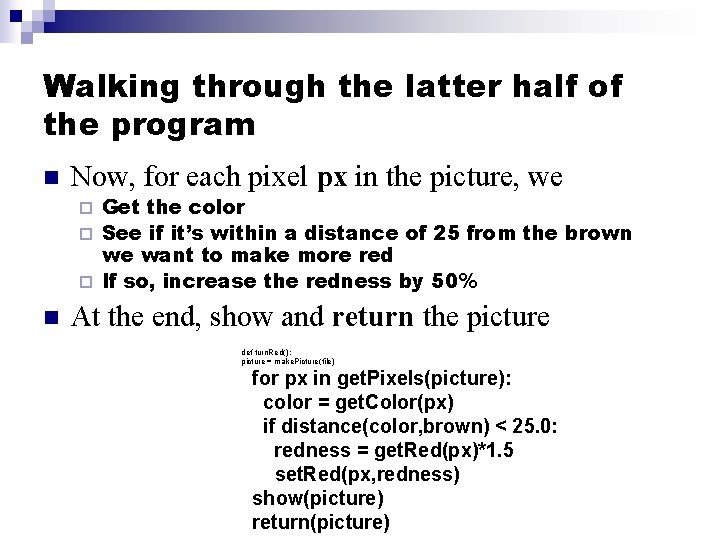
Walking through the latter half of the program n Now, for each pixel px in the picture, we Get the color ¨ See if it’s within a distance of 25 from the brown we want to make more red ¨ If so, increase the redness by 50% ¨ n At the end, show and return the picture def turn. Red(): picture = make. Picture(file) for px in get. Pixels(picture): color = get. Color(px) if distance(color, brown) < 25. 0: redness = get. Red(px)*1. 5 set. Red(px, redness) show(picture) return(picture)
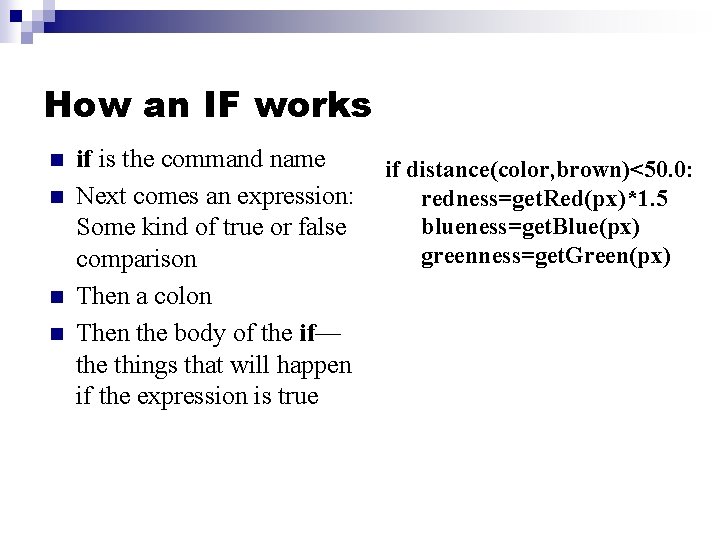
How an IF works n n if is the command name Next comes an expression: Some kind of true or false comparison Then a colon Then the body of the if— the things that will happen if the expression is true if distance(color, brown)<50. 0: redness=get. Red(px)*1. 5 blueness=get. Blue(px) greenness=get. Green(px)
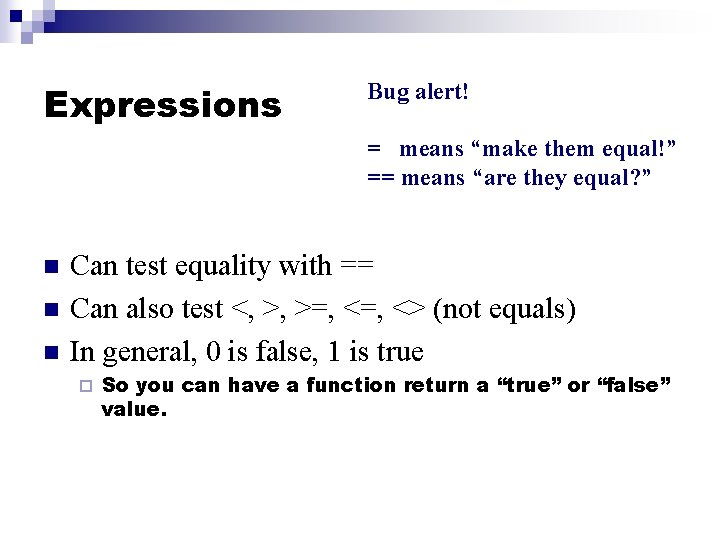
Expressions Bug alert! = means “make them equal!” == means “are they equal? ” n n n Can test equality with == Can also test <, >, >=, <> (not equals) In general, 0 is false, 1 is true ¨ So you can have a function return a “true” or “false” value.
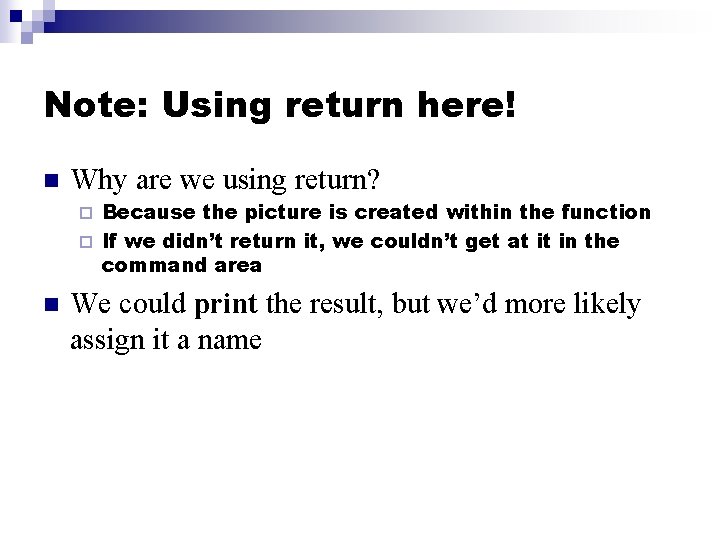
Note: Using return here! n Why are we using return? Because the picture is created within the function ¨ If we didn’t return it, we couldn’t get at it in the command area ¨ n We could print the result, but we’d more likely assign it a name
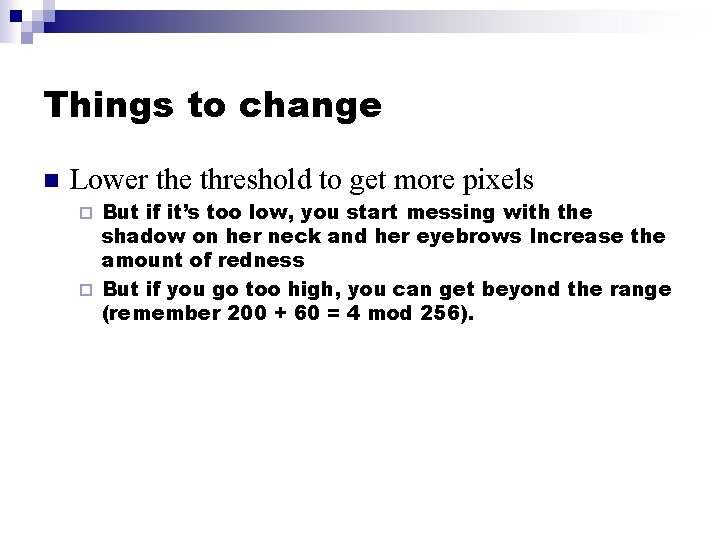
Things to change n Lower the threshold to get more pixels But if it’s too low, you start messing with the shadow on her neck and her eyebrows Increase the amount of redness ¨ But if you go too high, you can get beyond the range (remember 200 + 60 = 4 mod 256). ¨
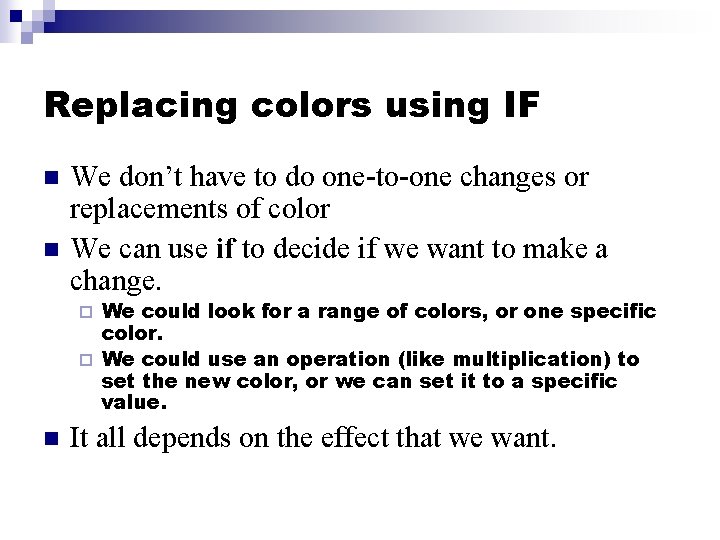
Replacing colors using IF n n We don’t have to do one-to-one changes or replacements of color We can use if to decide if we want to make a change. We could look for a range of colors, or one specific color. ¨ We could use an operation (like multiplication) to set the new color, or we can set it to a specific value. ¨ n It all depends on the effect that we want.
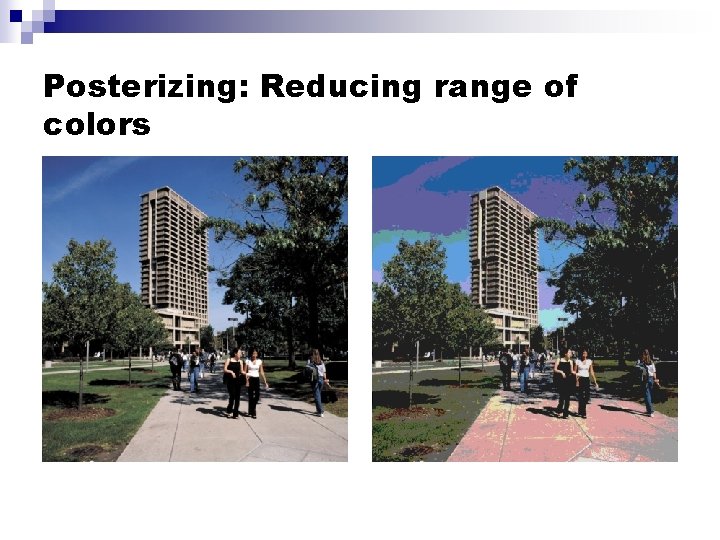
Posterizing: Reducing range of colors
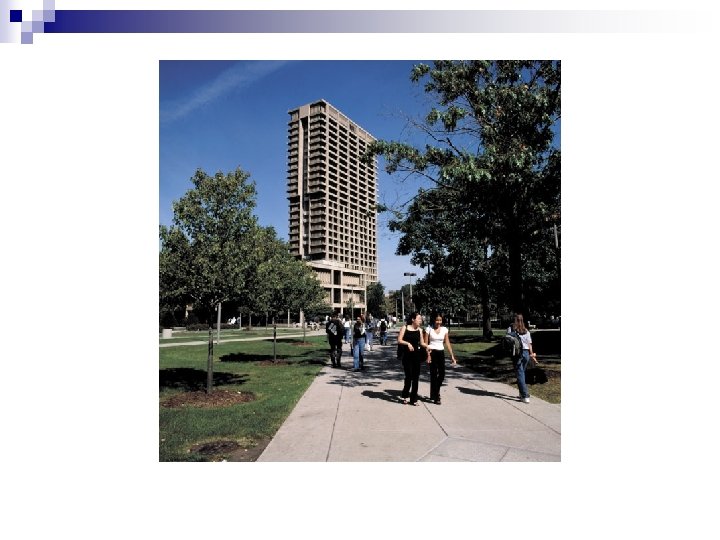
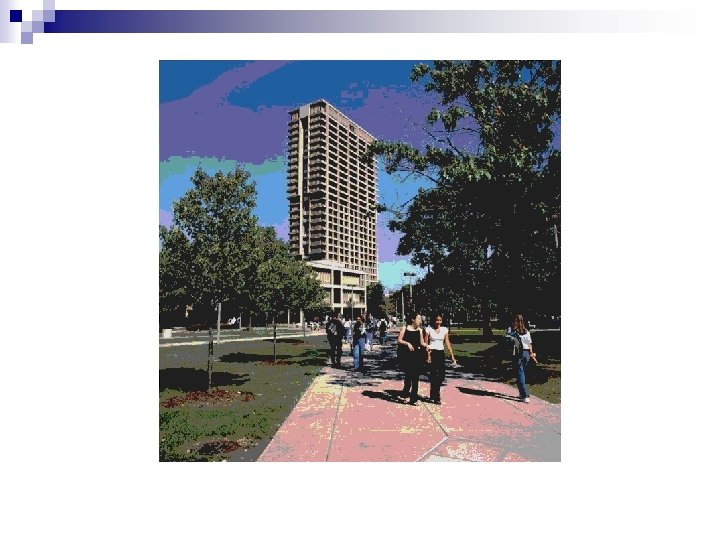
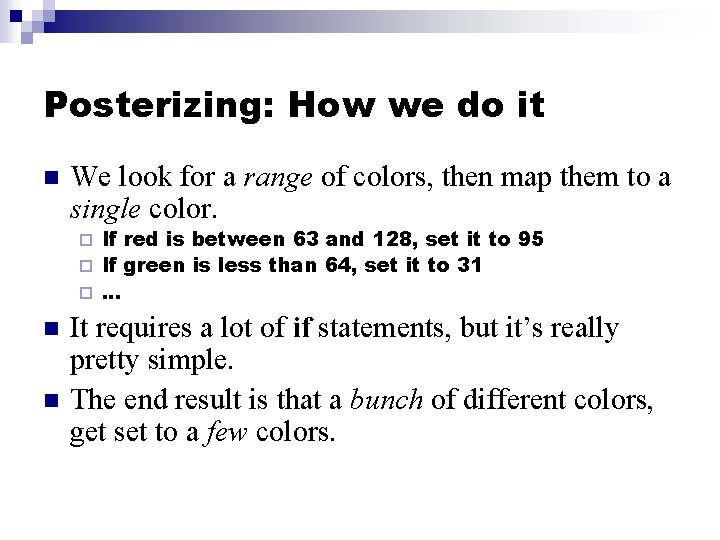
Posterizing: How we do it n We look for a range of colors, then map them to a single color. If red is between 63 and 128, set it to 95 ¨ If green is less than 64, set it to 31 ¨. . . ¨ n n It requires a lot of if statements, but it’s really pretty simple. The end result is that a bunch of different colors, get set to a few colors.
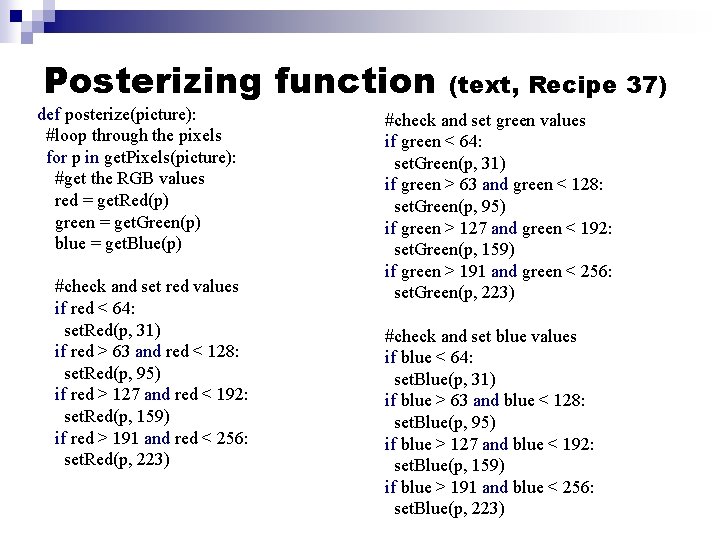
Posterizing function def posterize(picture): #loop through the pixels for p in get. Pixels(picture): #get the RGB values red = get. Red(p) green = get. Green(p) blue = get. Blue(p) #check and set red values if red < 64: set. Red(p, 31) if red > 63 and red < 128: set. Red(p, 95) if red > 127 and red < 192: set. Red(p, 159) if red > 191 and red < 256: set. Red(p, 223) (text, Recipe 37) #check and set green values if green < 64: set. Green(p, 31) if green > 63 and green < 128: set. Green(p, 95) if green > 127 and green < 192: set. Green(p, 159) if green > 191 and green < 256: set. Green(p, 223) #check and set blue values if blue < 64: set. Blue(p, 31) if blue > 63 and blue < 128: set. Blue(p, 95) if blue > 127 and blue < 192: set. Blue(p, 159) if blue > 191 and blue < 256: set. Blue(p, 223)
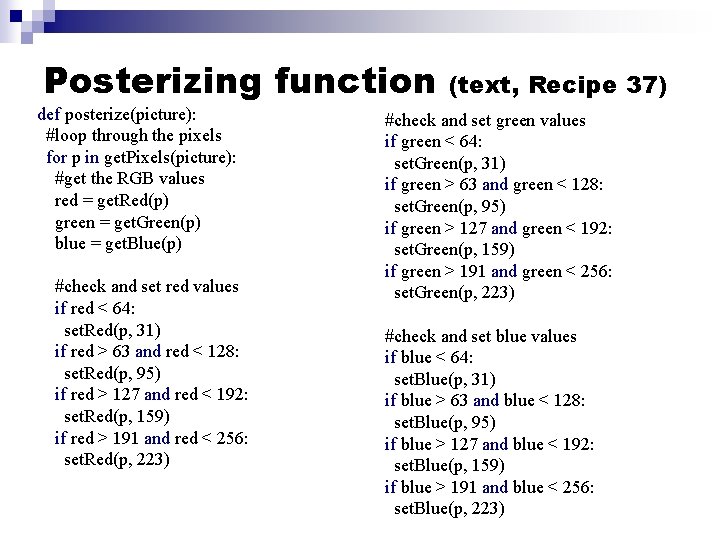
Posterizing function def posterize(picture): #loop through the pixels for p in get. Pixels(picture): #get the RGB values red = get. Red(p) green = get. Green(p) blue = get. Blue(p) #check and set red values if red < 64: set. Red(p, 31) if red > 63 and red < 128: set. Red(p, 95) if red > 127 and red < 192: set. Red(p, 159) if red > 191 and red < 256: set. Red(p, 223) (text, Recipe 37) #check and set green values if green < 64: set. Green(p, 31) if green > 63 and green < 128: set. Green(p, 95) if green > 127 and green < 192: set. Green(p, 159) if green > 191 and green < 256: set. Green(p, 223) #check and set blue values if blue < 64: set. Blue(p, 31) if blue > 63 and blue < 128: set. Blue(p, 95) if blue > 127 and blue < 192: set. Blue(p, 159) if blue > 191 and blue < 256: set. Blue(p, 223)
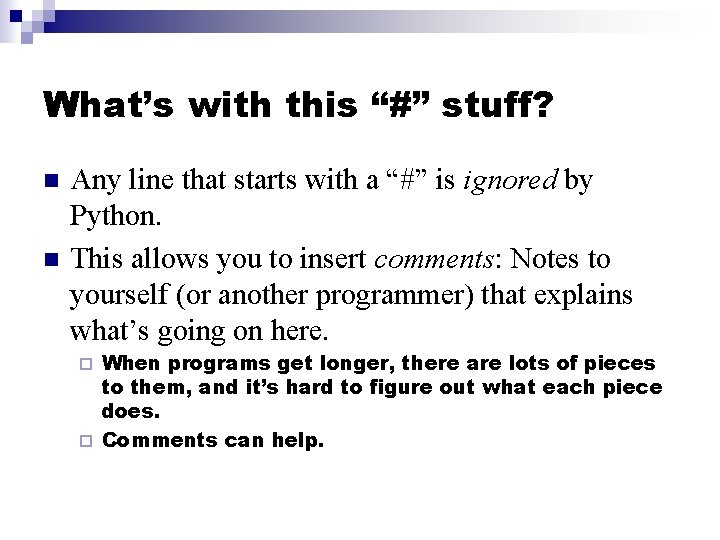
What’s with this “#” stuff? n n Any line that starts with a “#” is ignored by Python. This allows you to insert comments: Notes to yourself (or another programmer) that explains what’s going on here. When programs get longer, there are lots of pieces to them, and it’s hard to figure out what each piece does. ¨ Comments can help. ¨
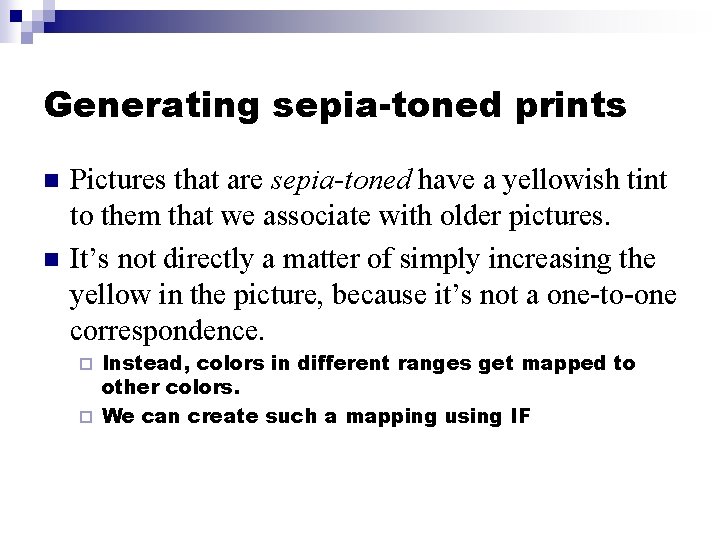
Generating sepia-toned prints n n Pictures that are sepia-toned have a yellowish tint to them that we associate with older pictures. It’s not directly a matter of simply increasing the yellow in the picture, because it’s not a one-to-one correspondence. Instead, colors in different ranges get mapped to other colors. ¨ We can create such a mapping using IF ¨
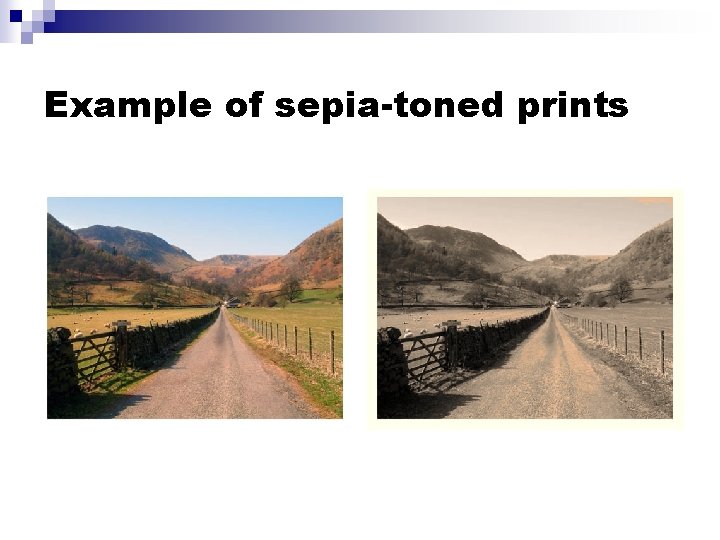
Example of sepia-toned prints
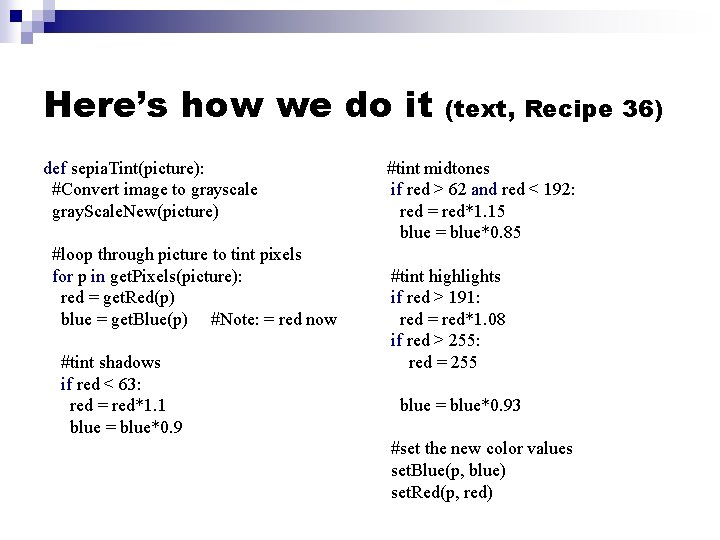
Here’s how we do it def sepia. Tint(picture): #Convert image to grayscale gray. Scale. New(picture) #loop through picture to tint pixels for p in get. Pixels(picture): red = get. Red(p) blue = get. Blue(p) #Note: = red now #tint shadows if red < 63: red = red*1. 1 blue = blue*0. 9 (text, Recipe 36) #tint midtones if red > 62 and red < 192: red = red*1. 15 blue = blue*0. 85 #tint highlights if red > 191: red = red*1. 08 if red > 255: red = 255 blue = blue*0. 93 #set the new color values set. Blue(p, blue) set. Red(p, red)
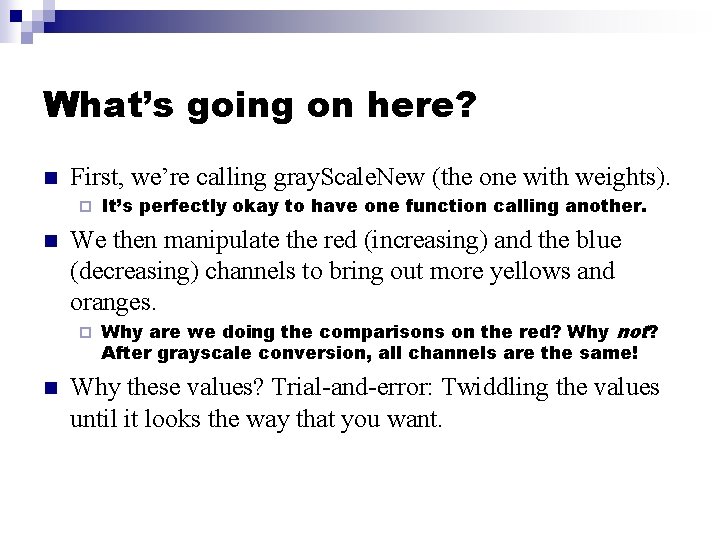
What’s going on here? n First, we’re calling gray. Scale. New (the one with weights). ¨ n We then manipulate the red (increasing) and the blue (decreasing) channels to bring out more yellows and oranges. ¨ n It’s perfectly okay to have one function calling another. Why are we doing the comparisons on the red? Why not? After grayscale conversion, all channels are the same! Why these values? Trial-and-error: Twiddling the values until it looks the way that you want.
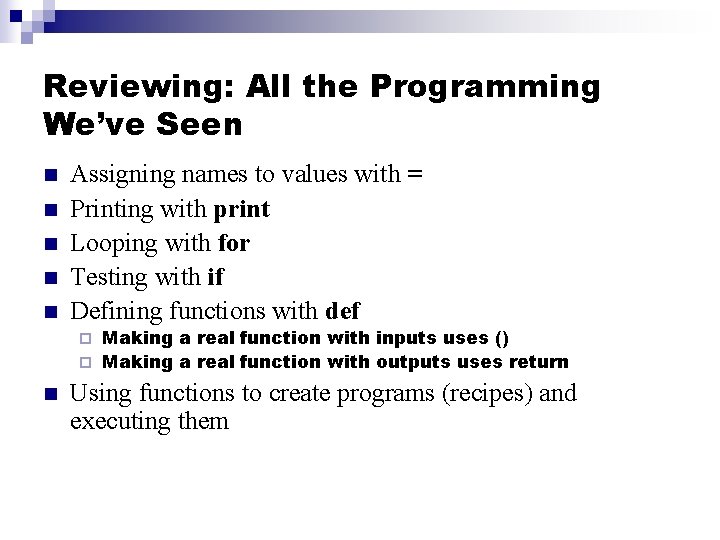
Reviewing: All the Programming We’ve Seen n n Assigning names to values with = Printing with print Looping with for Testing with if Defining functions with def Making a real function with inputs uses () ¨ Making a real function with outputs uses return ¨ n Using functions to create programs (recipes) and executing them
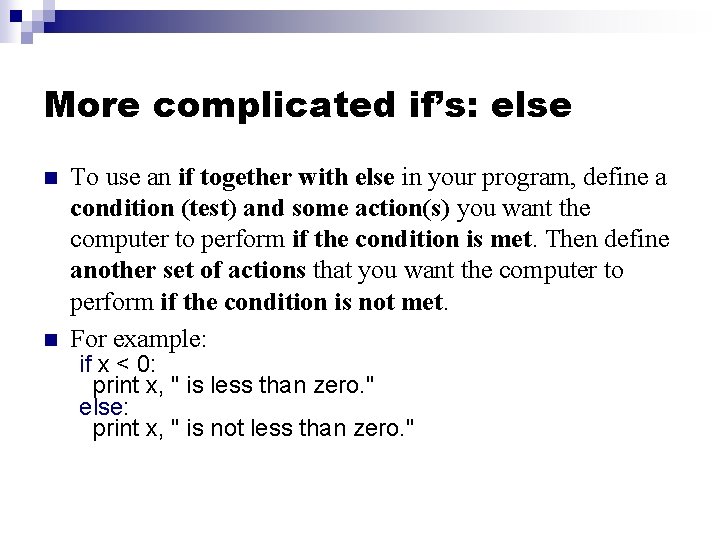
More complicated if’s: else n n To use an if together with else in your program, define a condition (test) and some action(s) you want the computer to perform if the condition is met. Then define another set of actions that you want the computer to perform if the condition is not met. For example: if x < 0: print x, " is less than zero. " else: print x, " is not less than zero. "
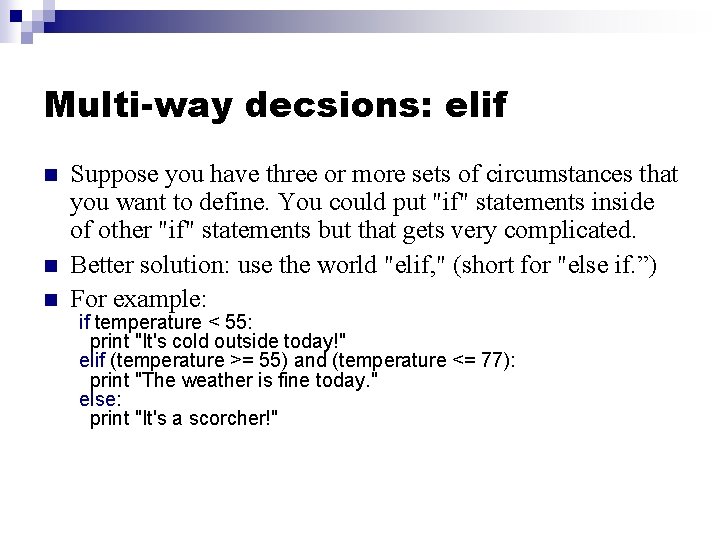
Multi-way decsions: elif n n n Suppose you have three or more sets of circumstances that you want to define. You could put "if" statements inside of other "if" statements but that gets very complicated. Better solution: use the world "elif, " (short for "else if. ”) For example: if temperature < 55: print "It's cold outside today!" elif (temperature >= 55) and (temperature <= 77): print "The weather is fine today. " else: print "It's a scorcher!"
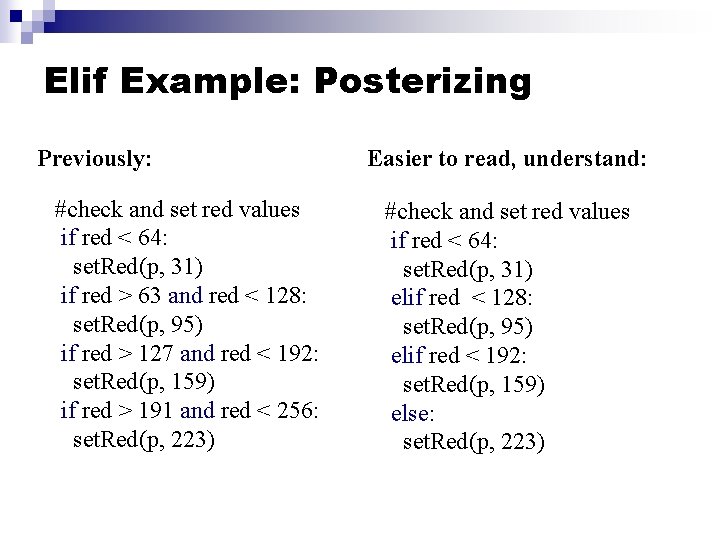
Elif Example: Posterizing Previously: #check and set red values if red < 64: set. Red(p, 31) if red > 63 and red < 128: set. Red(p, 95) if red > 127 and red < 192: set. Red(p, 159) if red > 191 and red < 256: set. Red(p, 223) Easier to read, understand: #check and set red values if red < 64: set. Red(p, 31) elif red < 128: set. Red(p, 95) elif red < 192: set. Red(p, 159) else: set. Red(p, 223)
Three biotic factors
It 101 - introduction to computing
It 101 - introduction to computing
It 101 introduction to computing
Conventional computing and intelligent computing
101 computing network
101 computing network design
101 computing
101computing
Io subsystem
101computing
Level system hair
Color theory light
Post-lesson assessment: l 101 lesson 1
Salesforce 101: introduction to salesforce kurs
Qi 101: introduction to health care improvement
Regarder introduction to cloud computing
Introduction to parallel computing ananth grama
Introduction to evolutionary computing
Introduction to mapreduce in cloud computing
Introduction to ubiquitous computing
Mobility of bits and bytes
Introduction to distributed computing
Introduction to cloud computing
Introduction to grid computing
Grid computing introduction
Introduction to cloud computing