Computer Science 209 Graphics and GUIs Working with
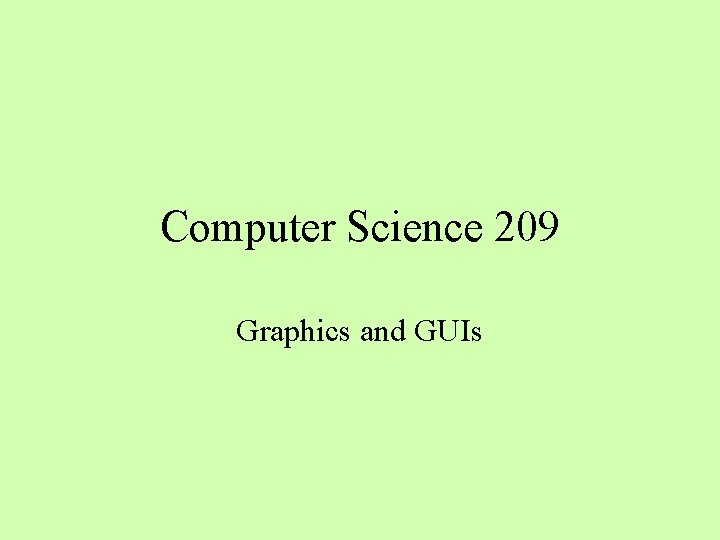
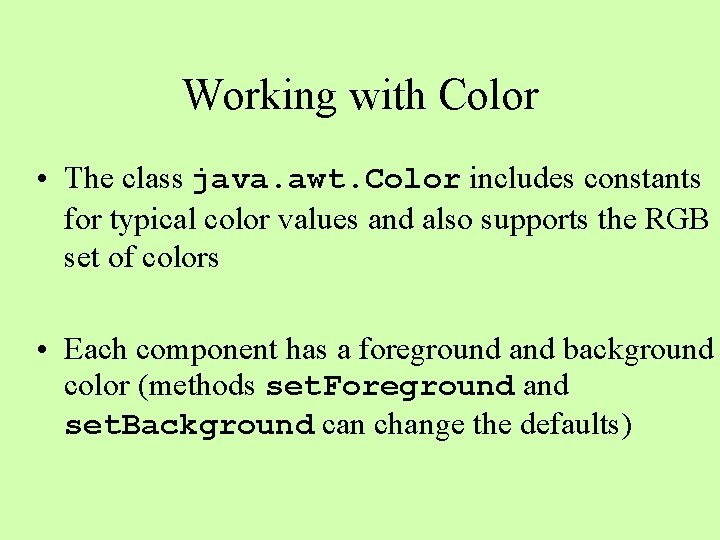
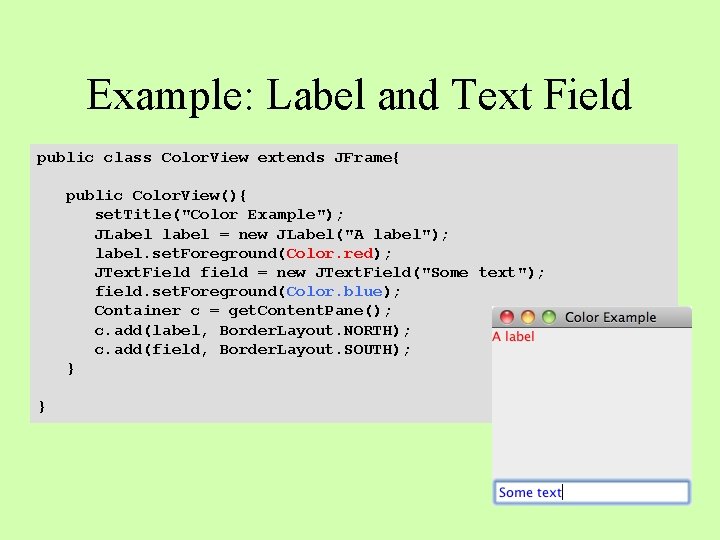
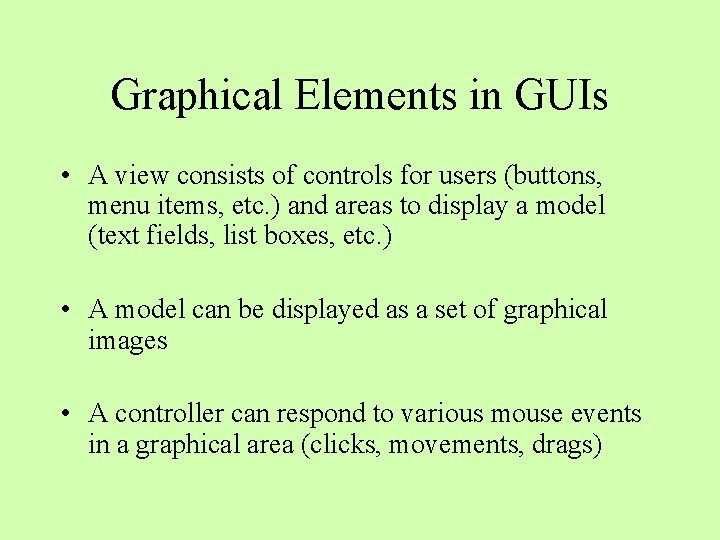
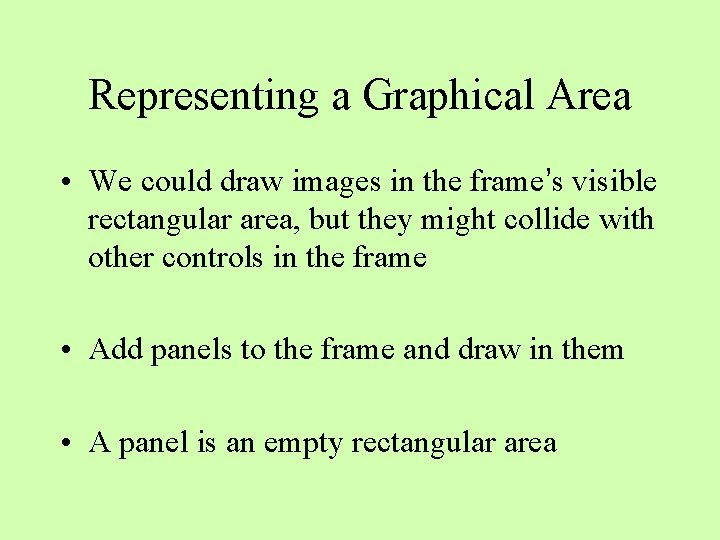
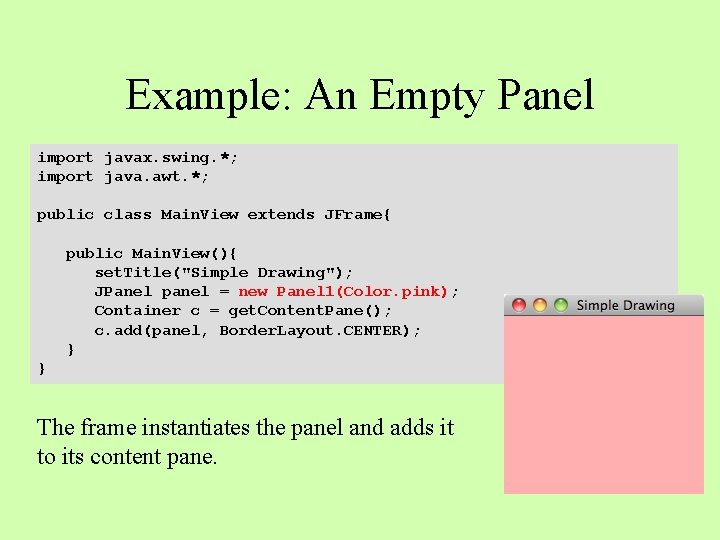
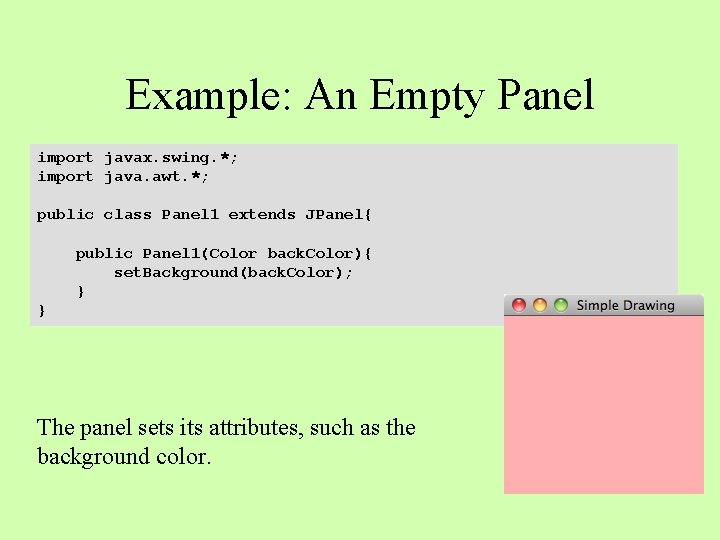
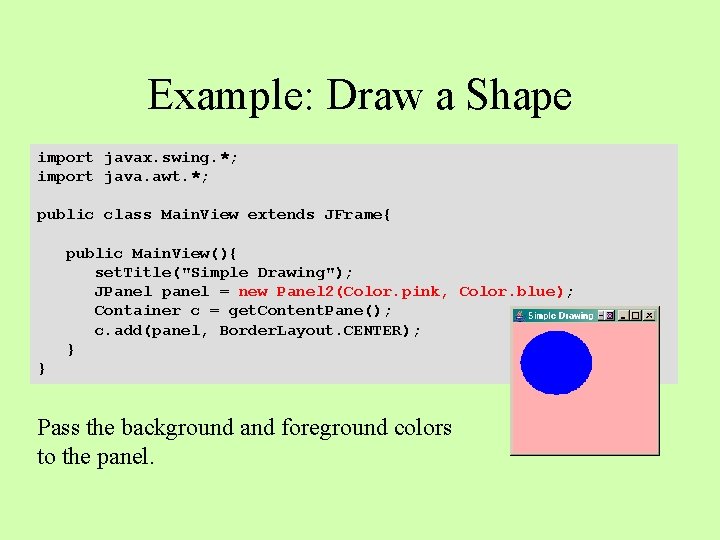
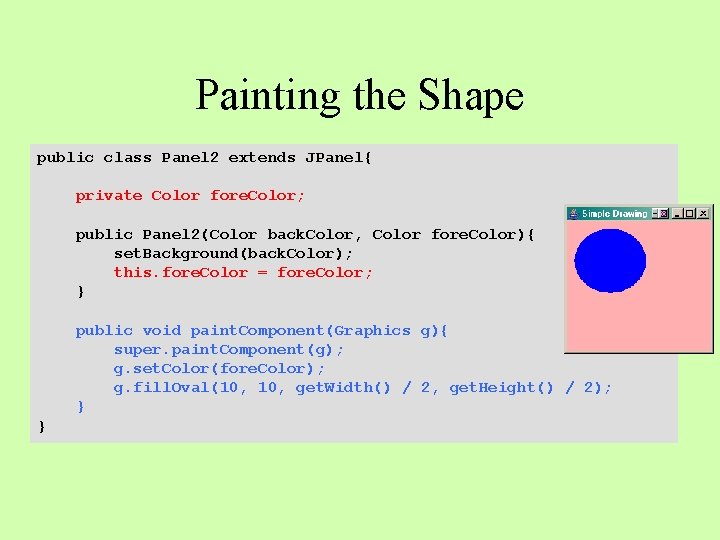
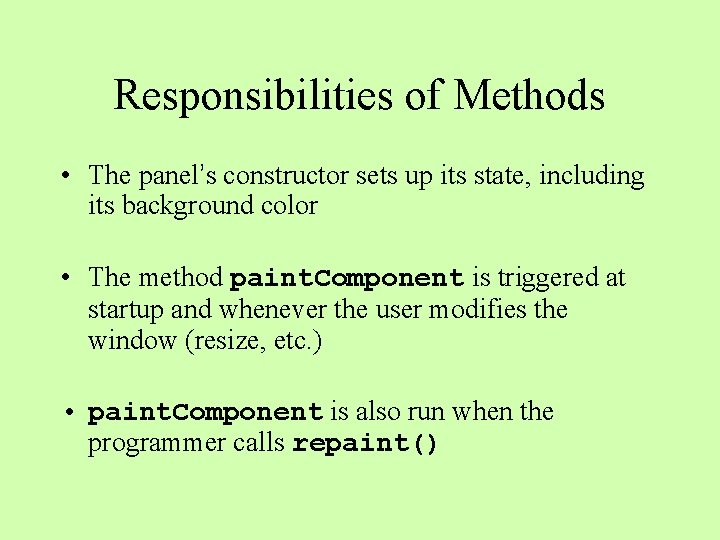
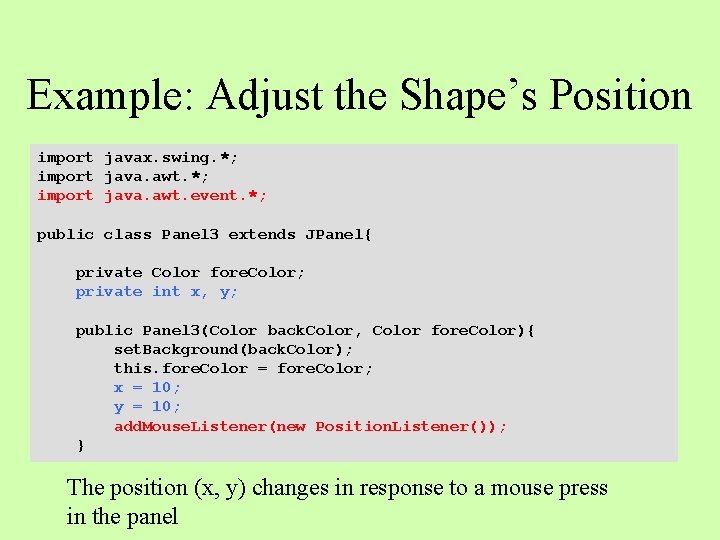
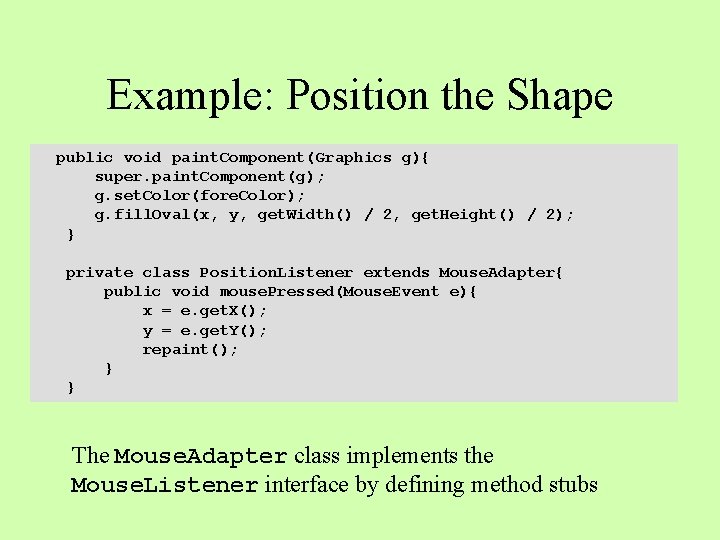
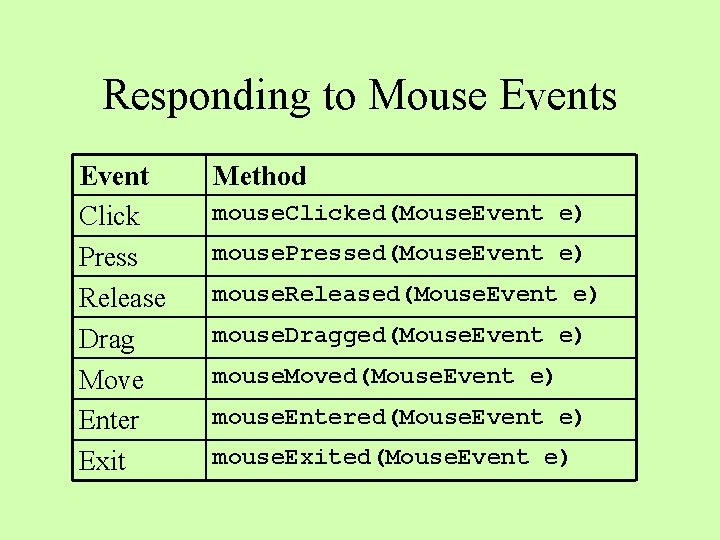
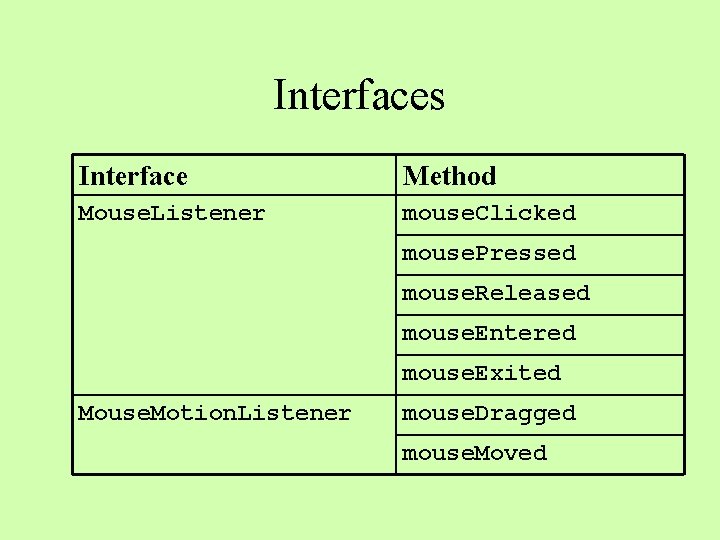
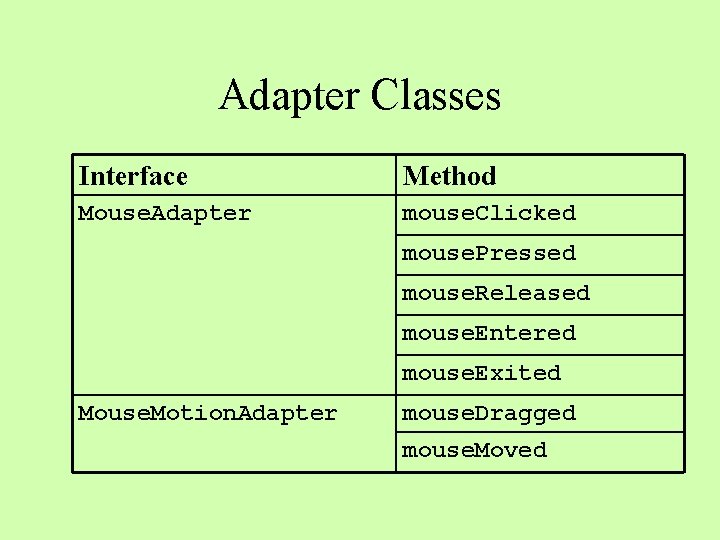
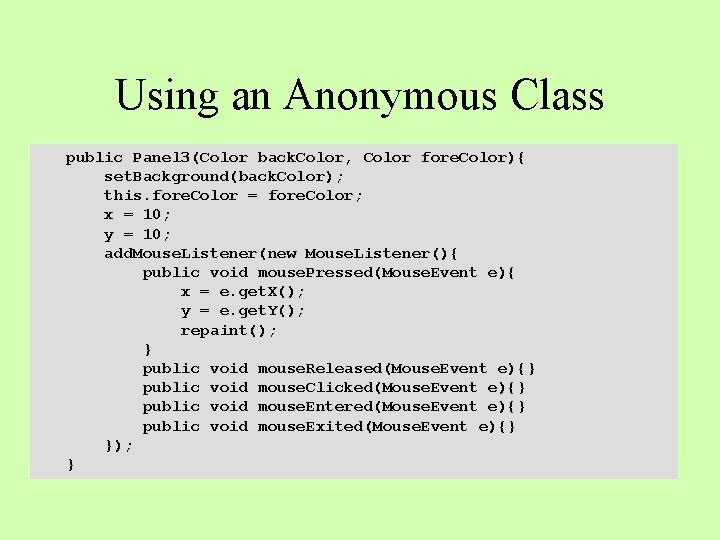
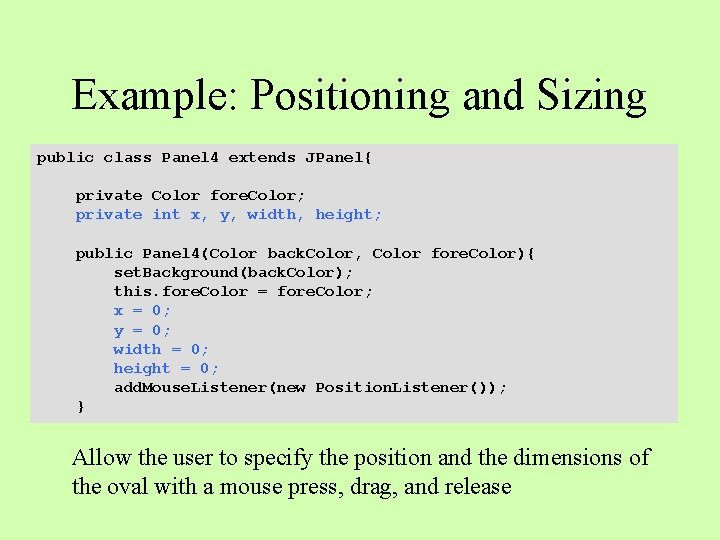
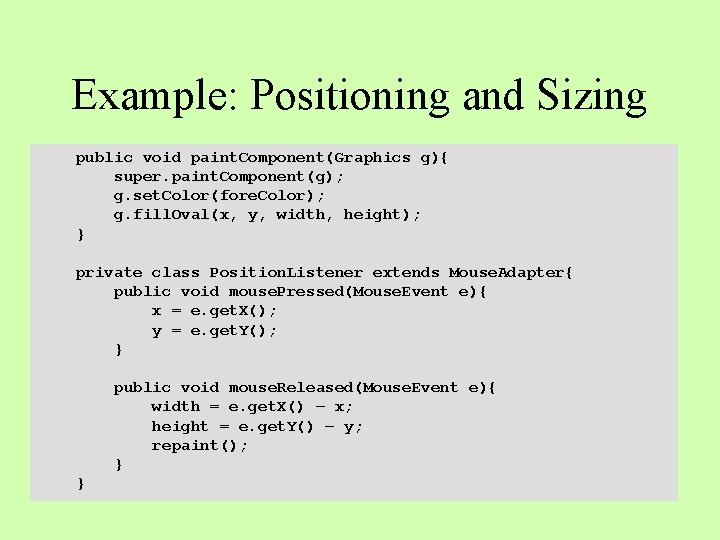
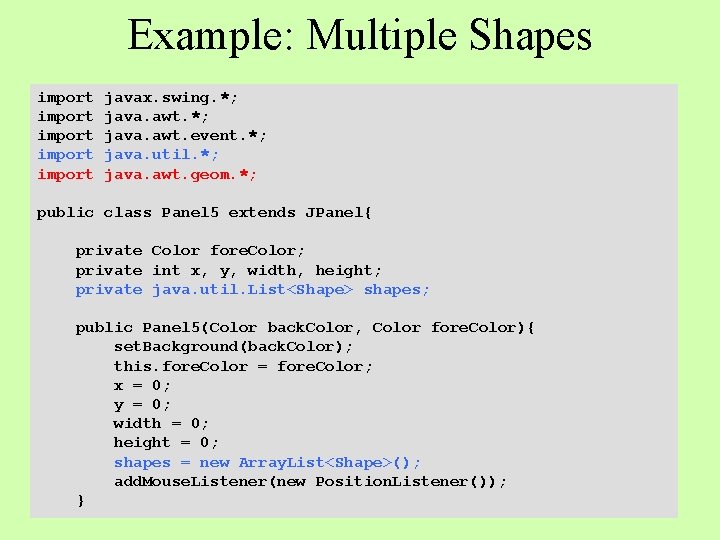
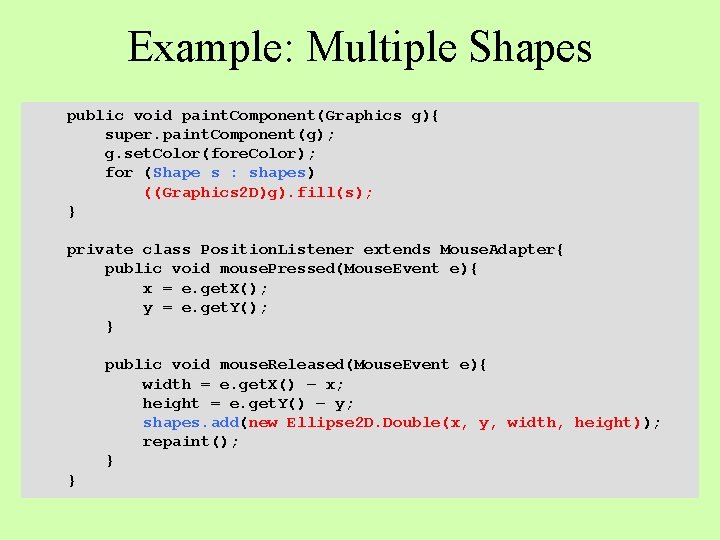
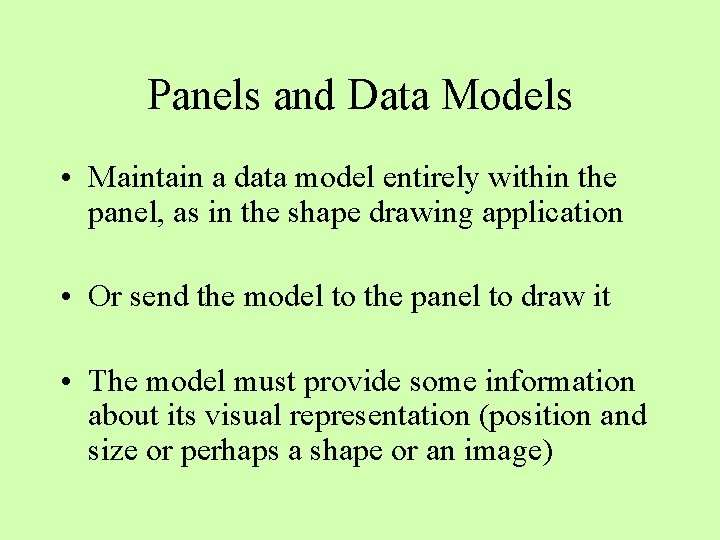
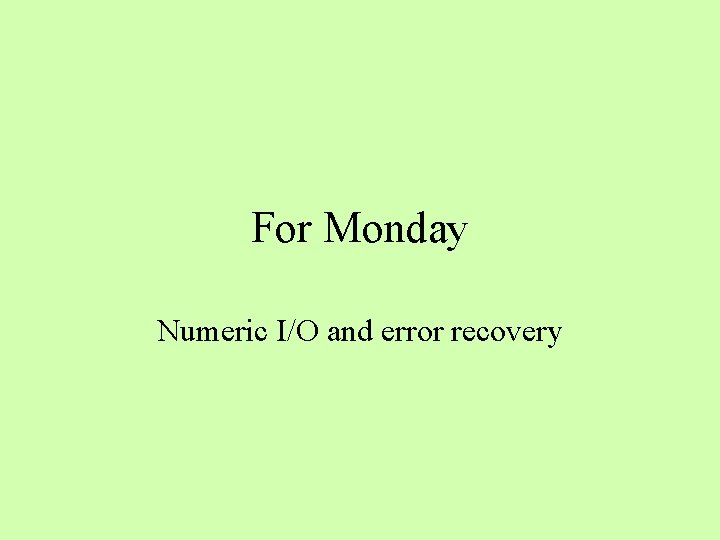
- Slides: 22
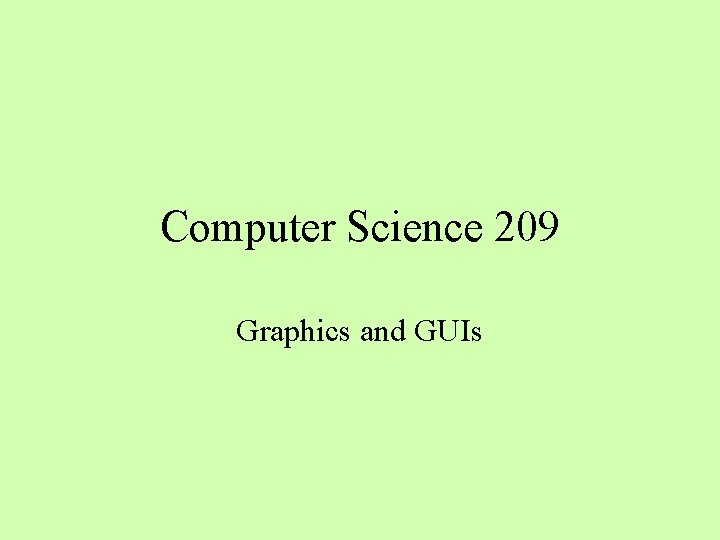
Computer Science 209 Graphics and GUIs
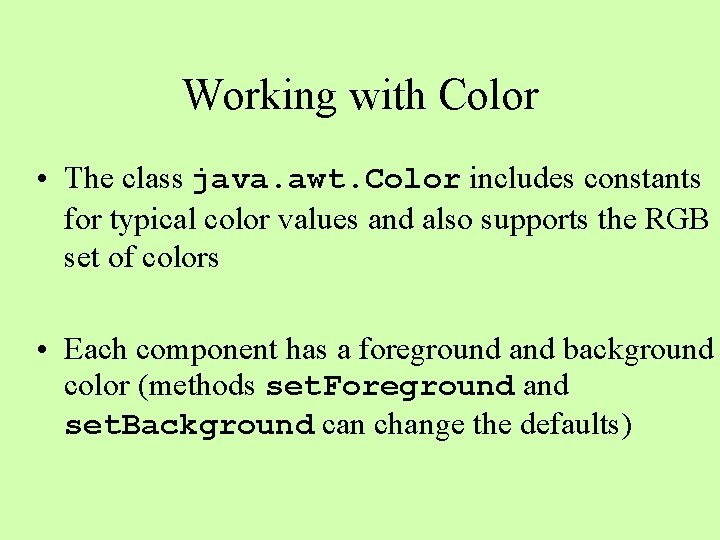
Working with Color • The class java. awt. Color includes constants for typical color values and also supports the RGB set of colors • Each component has a foreground and background color (methods set. Foreground and set. Background can change the defaults)
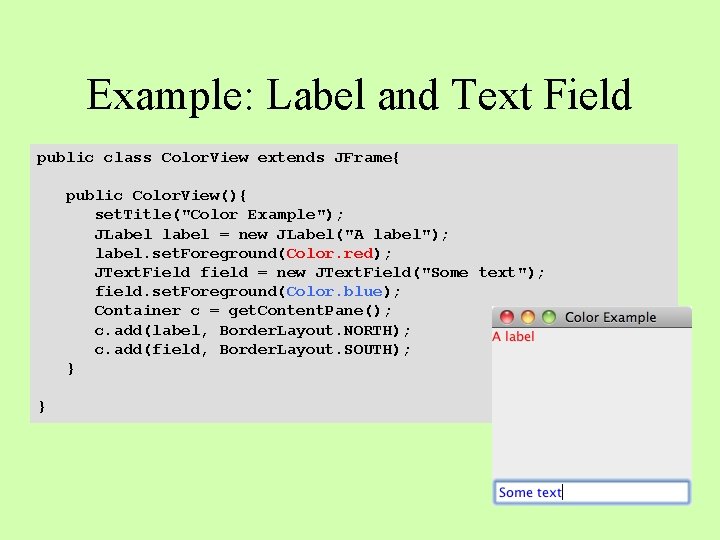
Example: Label and Text Field public class Color. View extends JFrame{ public Color. View(){ set. Title("Color Example"); JLabel label = new JLabel("A label"); label. set. Foreground(Color. red); JText. Field field = new JText. Field("Some text"); field. set. Foreground(Color. blue); Container c = get. Content. Pane(); c. add(label, Border. Layout. NORTH); c. add(field, Border. Layout. SOUTH); } }
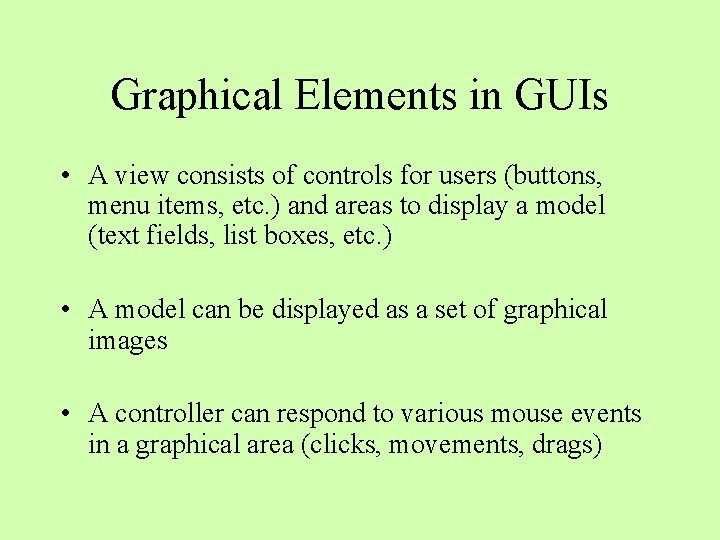
Graphical Elements in GUIs • A view consists of controls for users (buttons, menu items, etc. ) and areas to display a model (text fields, list boxes, etc. ) • A model can be displayed as a set of graphical images • A controller can respond to various mouse events in a graphical area (clicks, movements, drags)
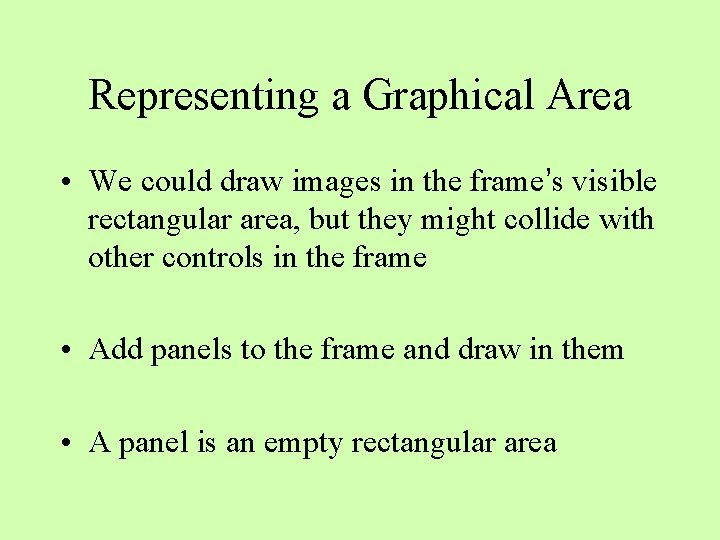
Representing a Graphical Area • We could draw images in the frame’s visible rectangular area, but they might collide with other controls in the frame • Add panels to the frame and draw in them • A panel is an empty rectangular area
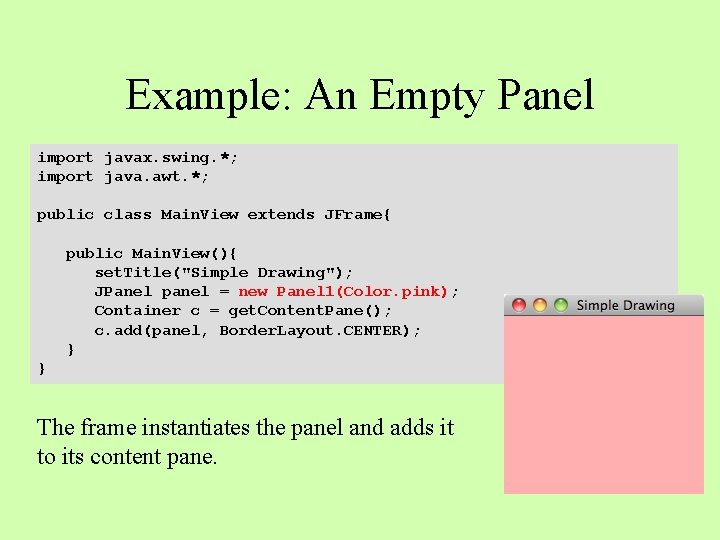
Example: An Empty Panel import javax. swing. *; import java. awt. *; public class Main. View extends JFrame{ public Main. View(){ set. Title("Simple Drawing"); JPanel panel = new Panel 1(Color. pink); Container c = get. Content. Pane(); c. add(panel, Border. Layout. CENTER); } } The frame instantiates the panel and adds it to its content pane.
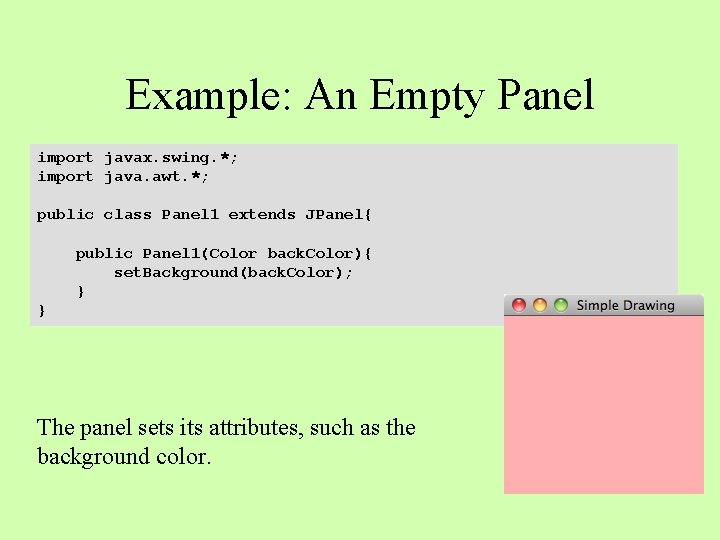
Example: An Empty Panel import javax. swing. *; import java. awt. *; public class Panel 1 extends JPanel{ public Panel 1(Color back. Color){ set. Background(back. Color); } } The panel sets its attributes, such as the background color.
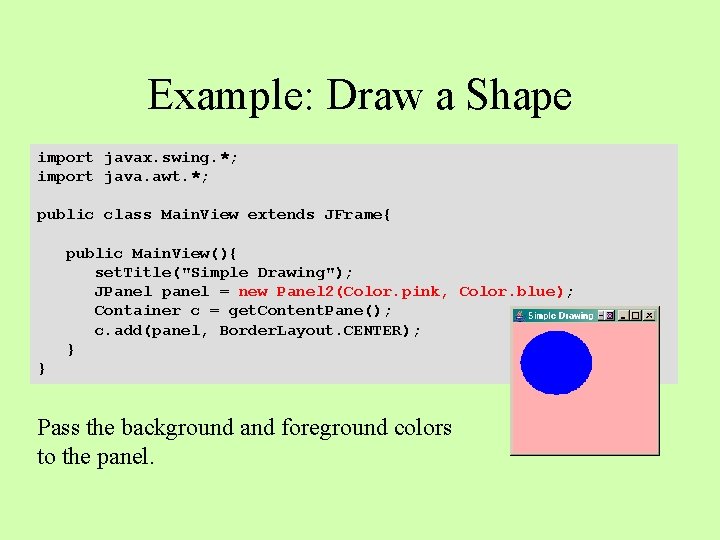
Example: Draw a Shape import javax. swing. *; import java. awt. *; public class Main. View extends JFrame{ public Main. View(){ set. Title("Simple Drawing"); JPanel panel = new Panel 2(Color. pink, Color. blue); Container c = get. Content. Pane(); c. add(panel, Border. Layout. CENTER); } } Pass the background and foreground colors to the panel.
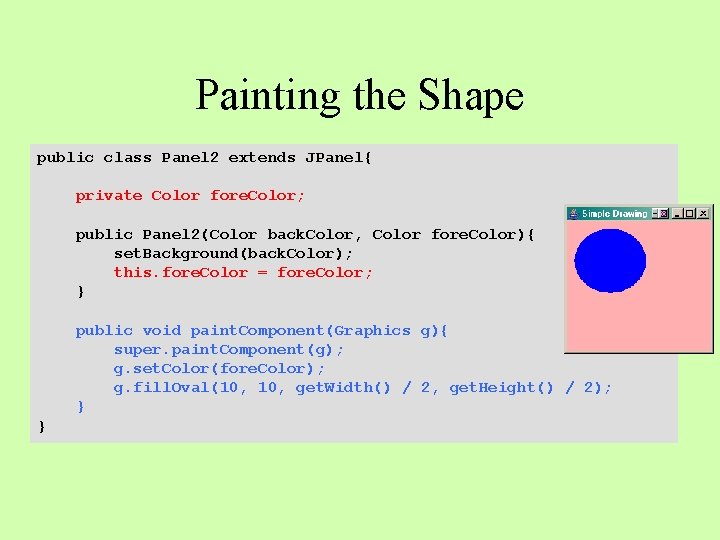
Painting the Shape public class Panel 2 extends JPanel{ private Color fore. Color; public Panel 2(Color back. Color, Color fore. Color){ set. Background(back. Color); this. fore. Color = fore. Color; } public void paint. Component(Graphics g){ super. paint. Component(g); g. set. Color(fore. Color); g. fill. Oval(10, get. Width() / 2, get. Height() / 2); } }
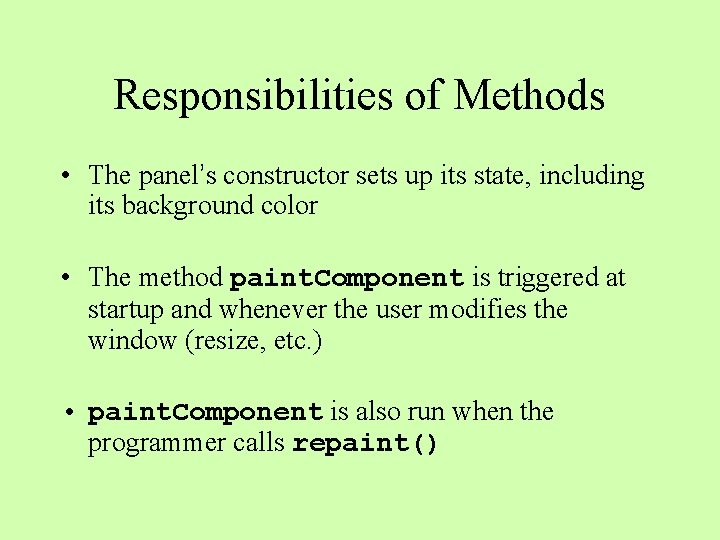
Responsibilities of Methods • The panel’s constructor sets up its state, including its background color • The method paint. Component is triggered at startup and whenever the user modifies the window (resize, etc. ) • paint. Component is also run when the programmer calls repaint()
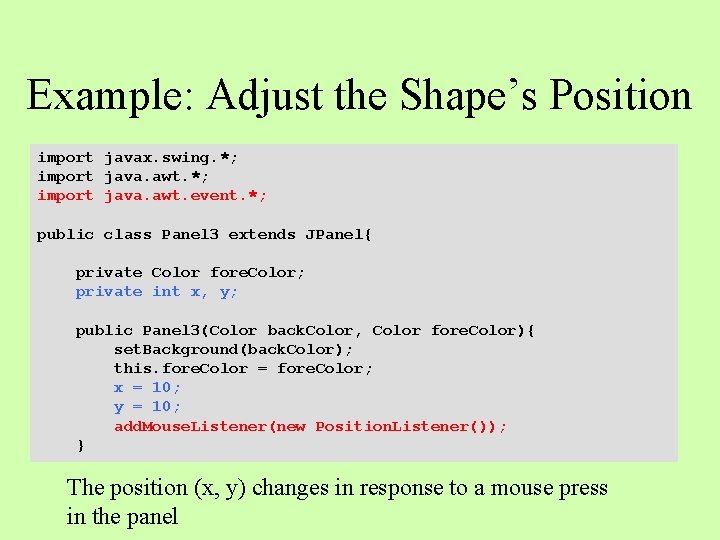
Example: Adjust the Shape’s Position import javax. swing. *; import java. awt. event. *; public class Panel 3 extends JPanel{ private Color fore. Color; private int x, y; public Panel 3(Color back. Color, Color fore. Color){ set. Background(back. Color); this. fore. Color = fore. Color; x = 10; y = 10; add. Mouse. Listener(new Position. Listener()); } The position (x, y) changes in response to a mouse press in the panel
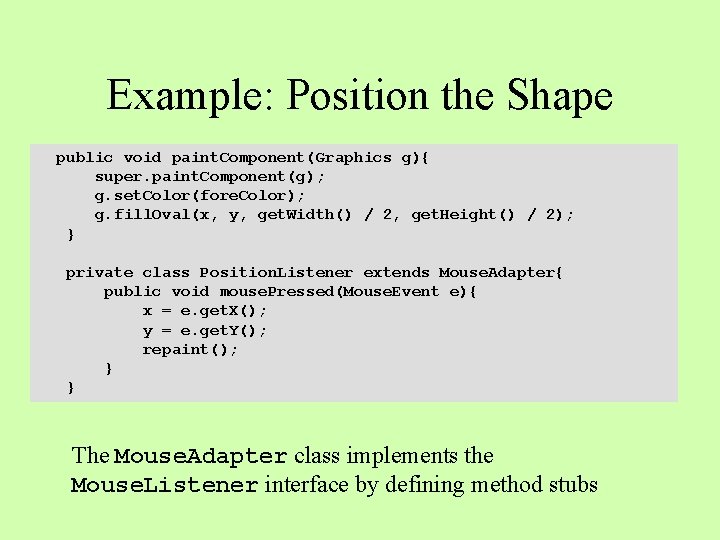
Example: Position the Shape public void paint. Component(Graphics g){ super. paint. Component(g); g. set. Color(fore. Color); g. fill. Oval(x, y, get. Width() / 2, get. Height() / 2); } private class Position. Listener extends Mouse. Adapter{ public void mouse. Pressed(Mouse. Event e){ x = e. get. X(); y = e. get. Y(); repaint(); } } The Mouse. Adapter class implements the Mouse. Listener interface by defining method stubs
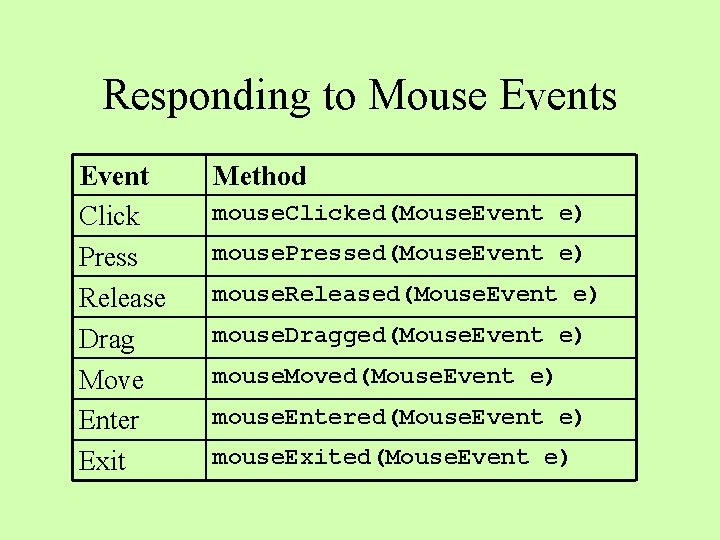
Responding to Mouse Events Event Click Press Release Drag Move Enter Exit Method mouse. Clicked(Mouse. Event e) mouse. Pressed(Mouse. Event e) mouse. Released(Mouse. Event e) mouse. Dragged(Mouse. Event e) mouse. Moved(Mouse. Event e) mouse. Entered(Mouse. Event e) mouse. Exited(Mouse. Event e)
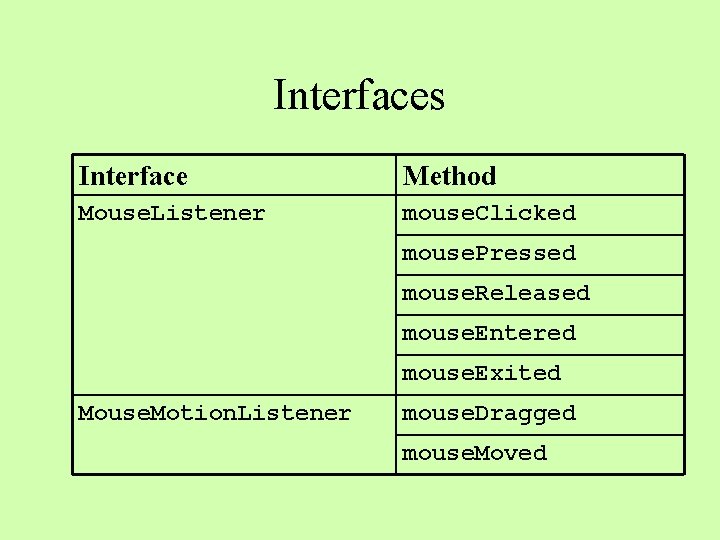
Interfaces Interface Method Mouse. Listener mouse. Clicked mouse. Pressed mouse. Released mouse. Entered mouse. Exited Mouse. Motion. Listener mouse. Dragged mouse. Moved
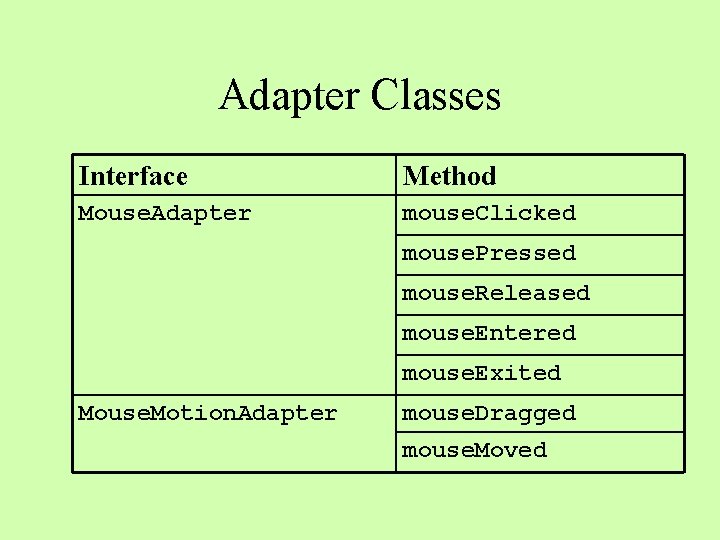
Adapter Classes Interface Method Mouse. Adapter mouse. Clicked mouse. Pressed mouse. Released mouse. Entered mouse. Exited Mouse. Motion. Adapter mouse. Dragged mouse. Moved
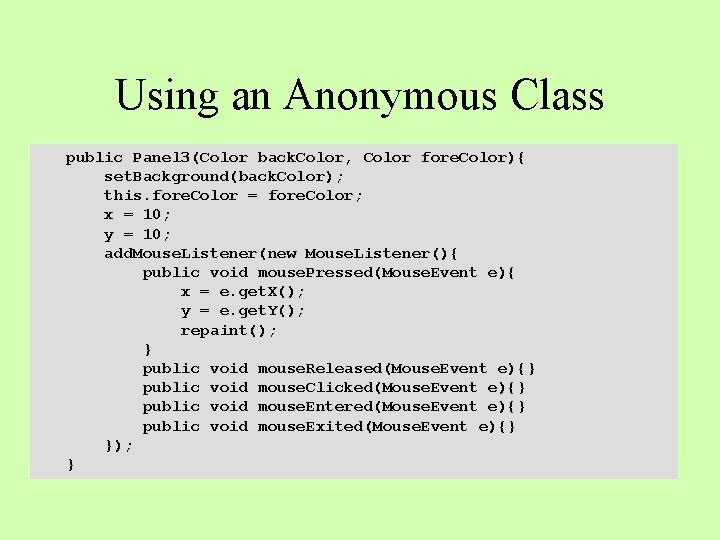
Using an Anonymous Class public Panel 3(Color back. Color, Color fore. Color){ set. Background(back. Color); this. fore. Color = fore. Color; x = 10; y = 10; add. Mouse. Listener(new Mouse. Listener(){ public void mouse. Pressed(Mouse. Event e){ x = e. get. X(); y = e. get. Y(); repaint(); } public void mouse. Released(Mouse. Event e){} public void mouse. Clicked(Mouse. Event e){} public void mouse. Entered(Mouse. Event e){} public void mouse. Exited(Mouse. Event e){} }); }
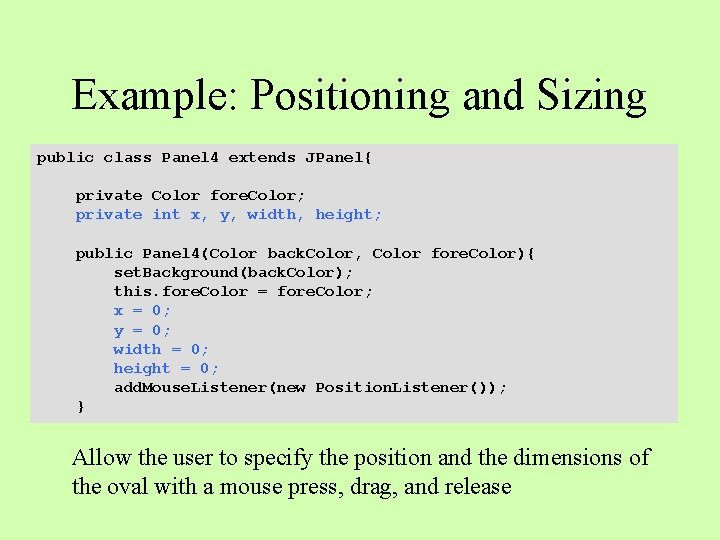
Example: Positioning and Sizing public class Panel 4 extends JPanel{ private Color fore. Color; private int x, y, width, height; public Panel 4(Color back. Color, Color fore. Color){ set. Background(back. Color); this. fore. Color = fore. Color; x = 0; y = 0; width = 0; height = 0; add. Mouse. Listener(new Position. Listener()); } Allow the user to specify the position and the dimensions of the oval with a mouse press, drag, and release
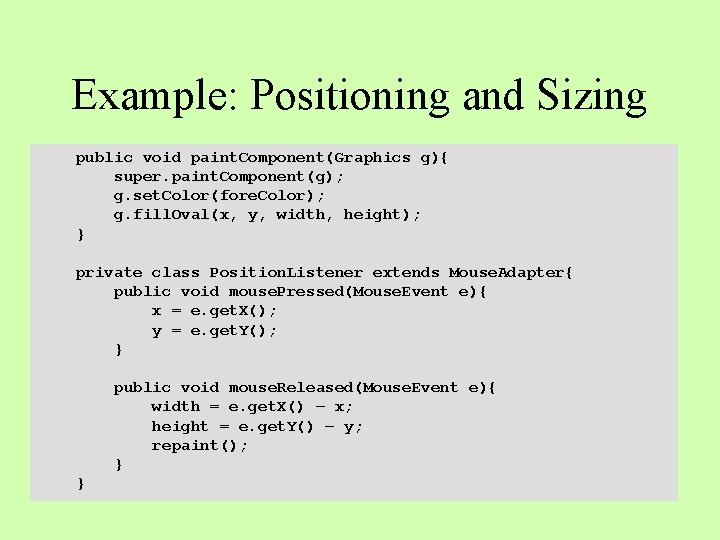
Example: Positioning and Sizing public void paint. Component(Graphics g){ super. paint. Component(g); g. set. Color(fore. Color); g. fill. Oval(x, y, width, height); } private class Position. Listener extends Mouse. Adapter{ public void mouse. Pressed(Mouse. Event e){ x = e. get. X(); y = e. get. Y(); } public void mouse. Released(Mouse. Event e){ width = e. get. X() – x; height = e. get. Y() – y; repaint(); } }
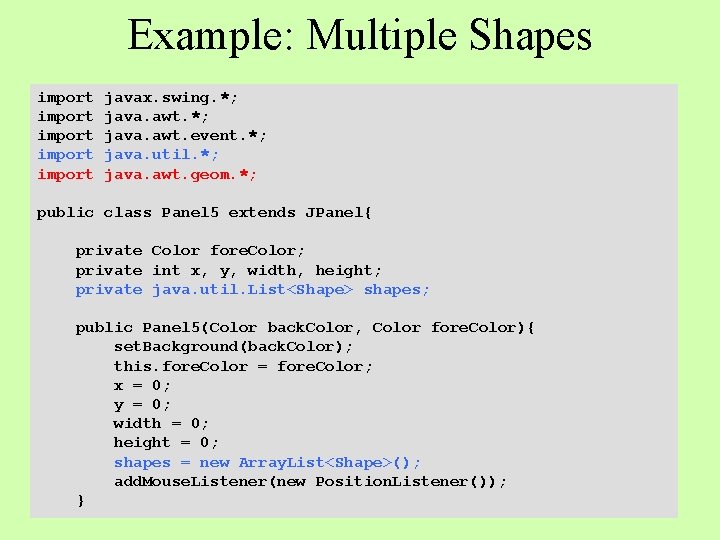
Example: Multiple Shapes import import javax. swing. *; java. awt. event. *; java. util. *; java. awt. geom. *; public class Panel 5 extends JPanel{ private Color fore. Color; private int x, y, width, height; private java. util. List<Shape> shapes; public Panel 5(Color back. Color, Color fore. Color){ set. Background(back. Color); this. fore. Color = fore. Color; x = 0; y = 0; width = 0; height = 0; shapes = new Array. List<Shape>(); add. Mouse. Listener(new Position. Listener()); }
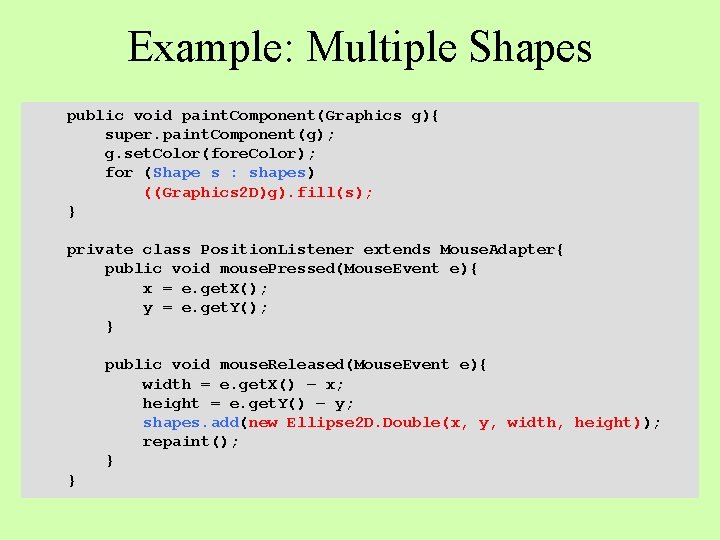
Example: Multiple Shapes public void paint. Component(Graphics g){ super. paint. Component(g); g. set. Color(fore. Color); for (Shape s : shapes) ((Graphics 2 D)g). fill(s); } private class Position. Listener extends Mouse. Adapter{ public void mouse. Pressed(Mouse. Event e){ x = e. get. X(); y = e. get. Y(); } public void mouse. Released(Mouse. Event e){ width = e. get. X() – x; height = e. get. Y() – y; shapes. add(new Ellipse 2 D. Double(x, y, width, height)); repaint(); } }
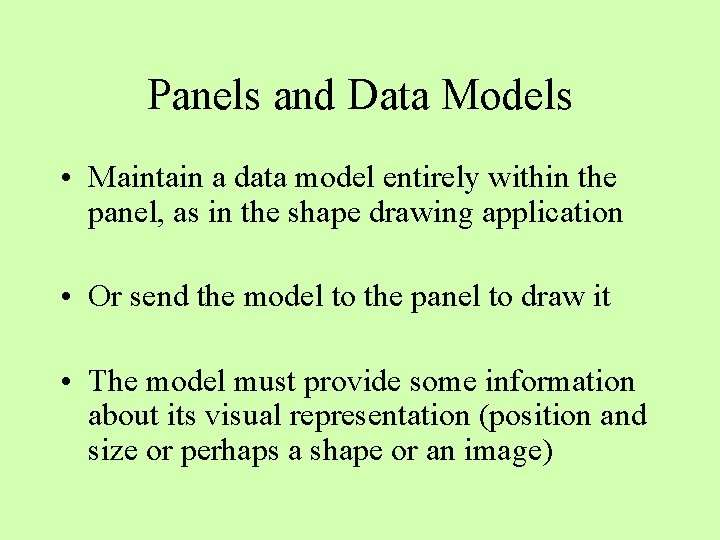
Panels and Data Models • Maintain a data model entirely within the panel, as in the shape drawing application • Or send the model to the panel to draw it • The model must provide some information about its visual representation (position and size or perhaps a shape or an image)
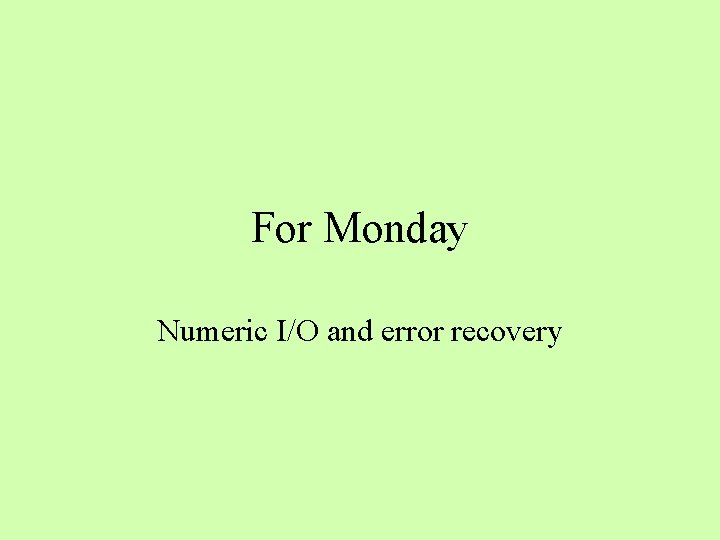
For Monday Numeric I/O and error recovery
Graphics and guis with matlab
Graphics monitors and workstations in computer graphics
Gui vs web user interface
Dot matrix display ppt
In my heart there rings a melody
735 ilcs 5/13-209
Lesson 2 add integers page 209 answers
Ece 209
Presente activo
2 half lives
Ece 209
Hot working and cold working difference
Hot metal forming
Machining operations
Proses pengerjaan panas
Hard work vs smart work
Science is my favorite subject because
In two dimensional viewing we have?
Line drawing algorithm in computer graphics with example
Points and lines in computer graphics ppt
What is window and viewport in computer graphics
Reflection and shearing in computer graphics
Mathematical foundations of computer graphics and vision