COMP 171 Data Structures and Algorithms Tutorial 1
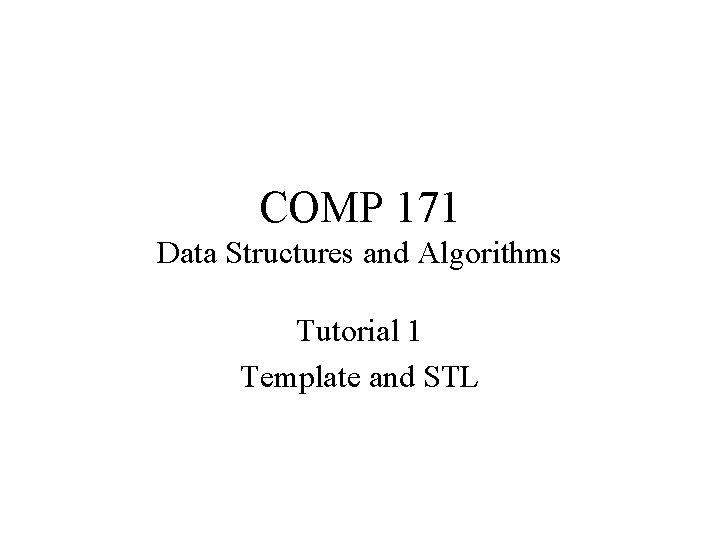
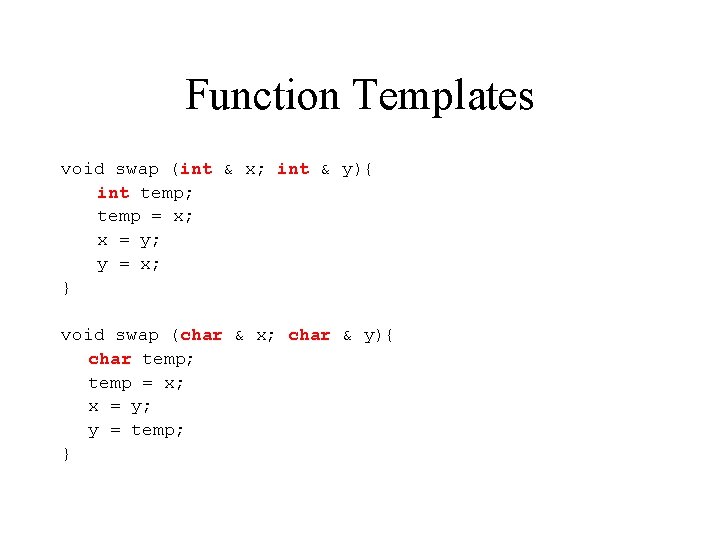
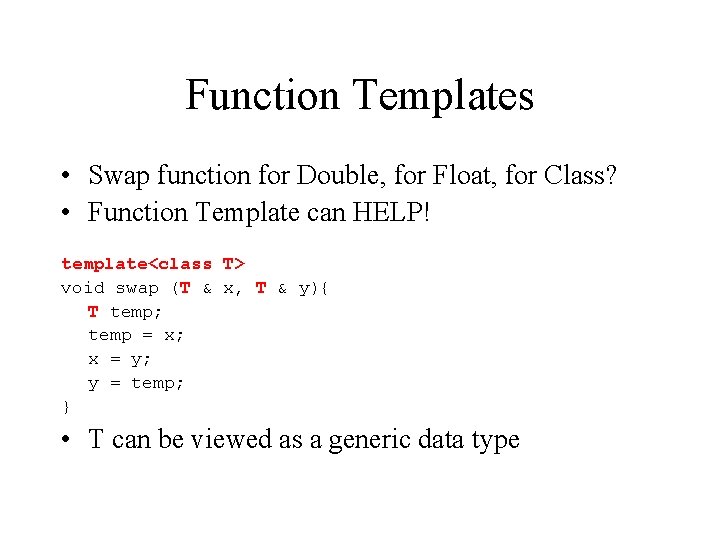
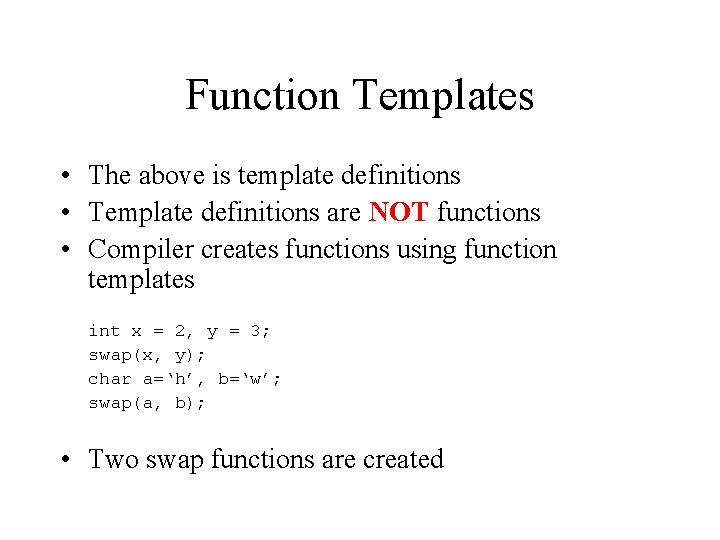
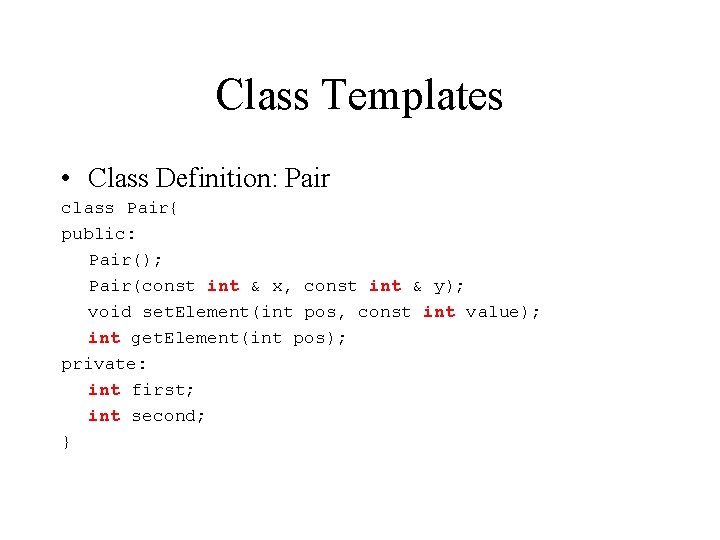
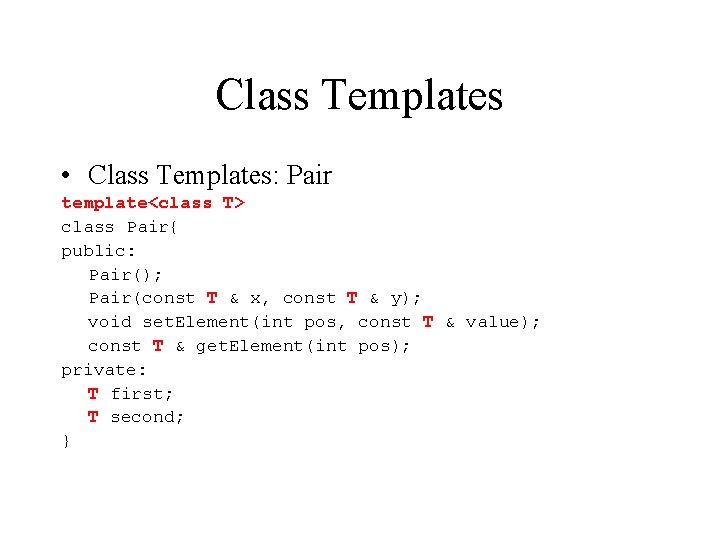
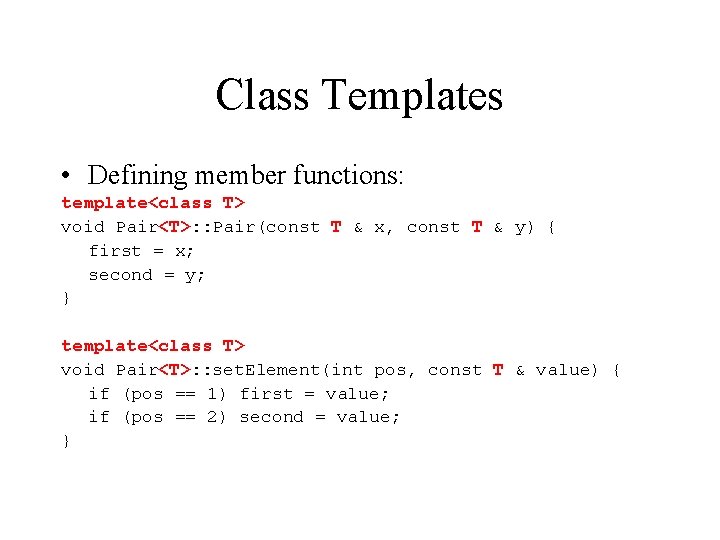
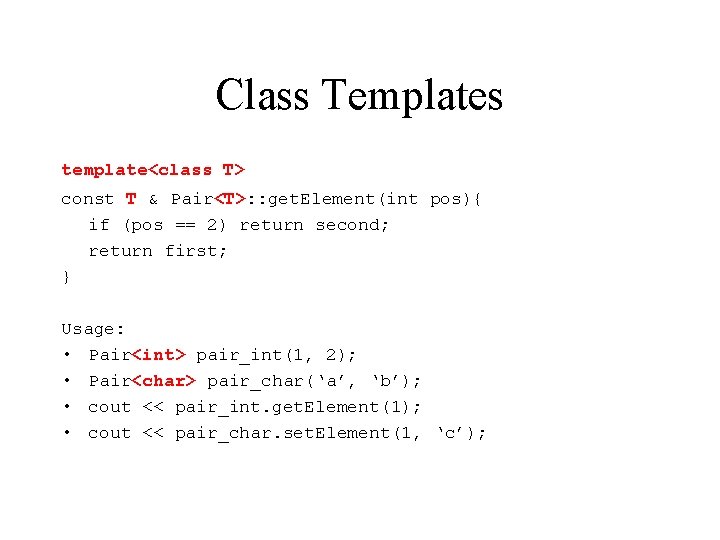
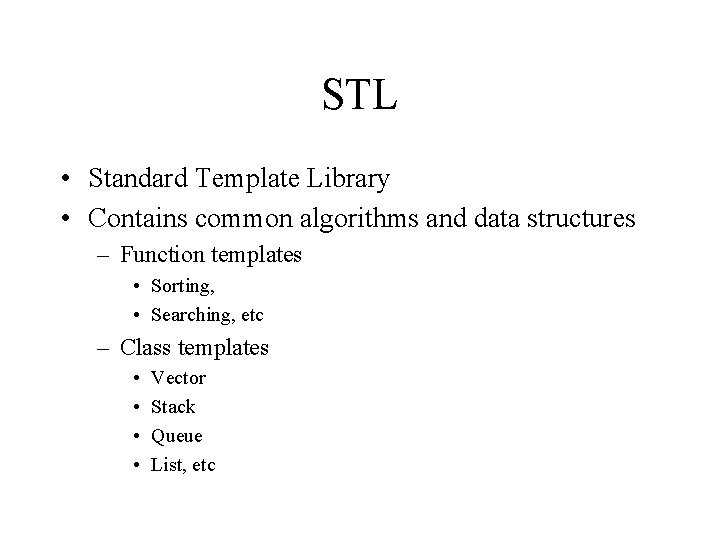
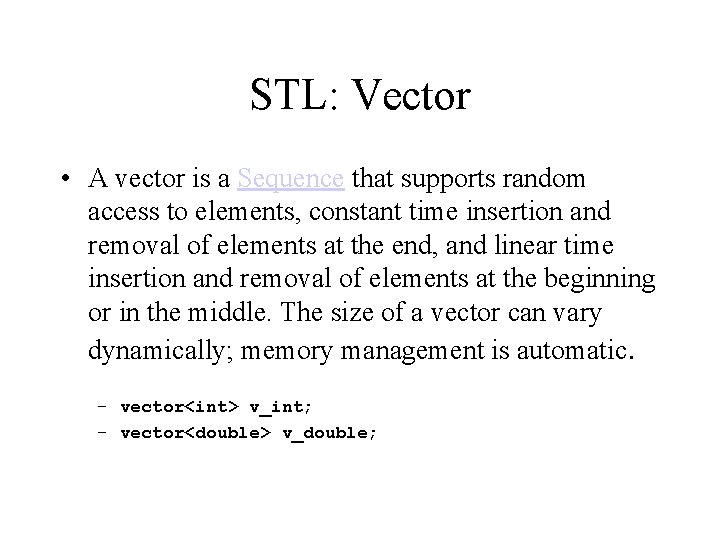
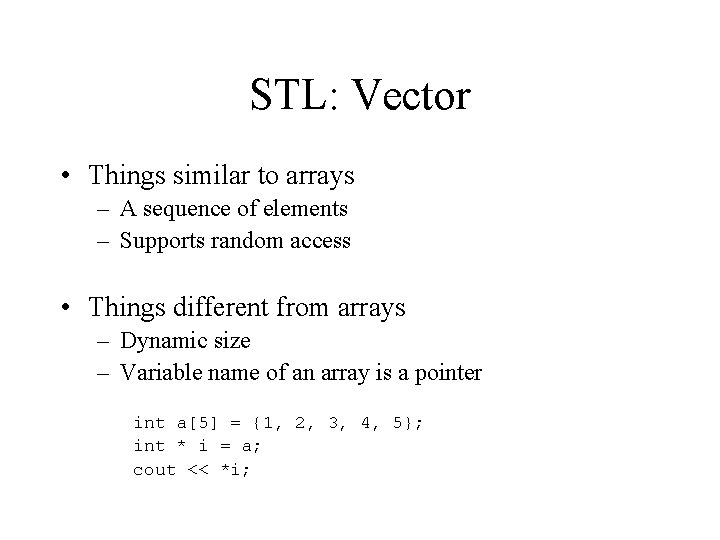
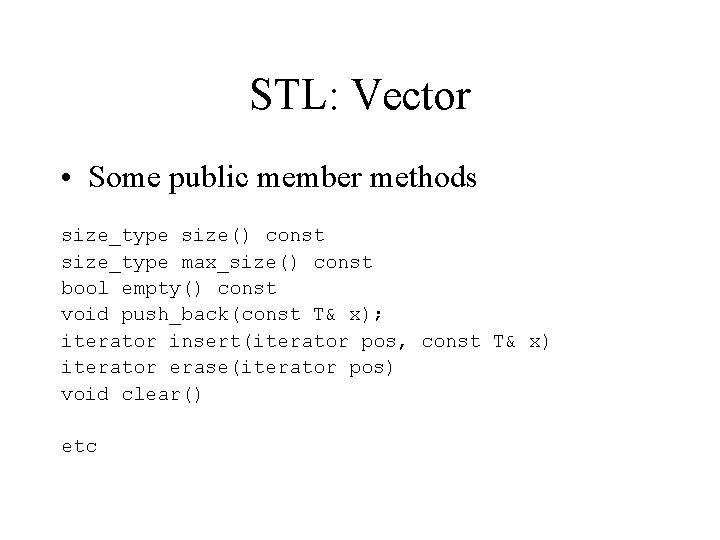
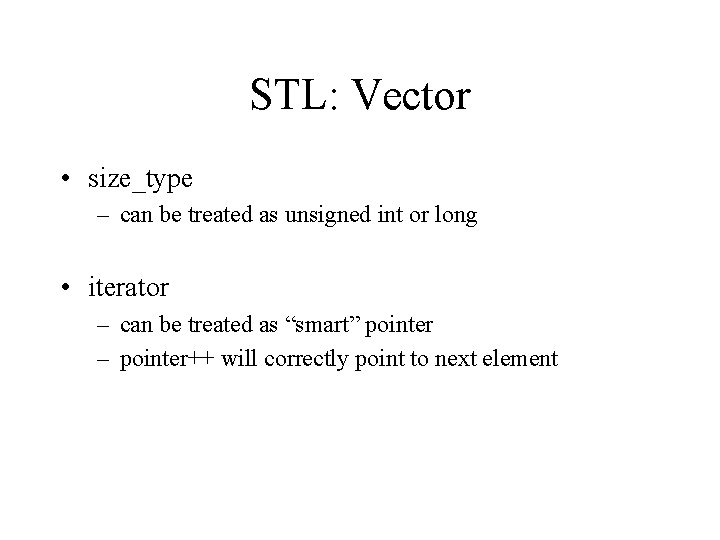
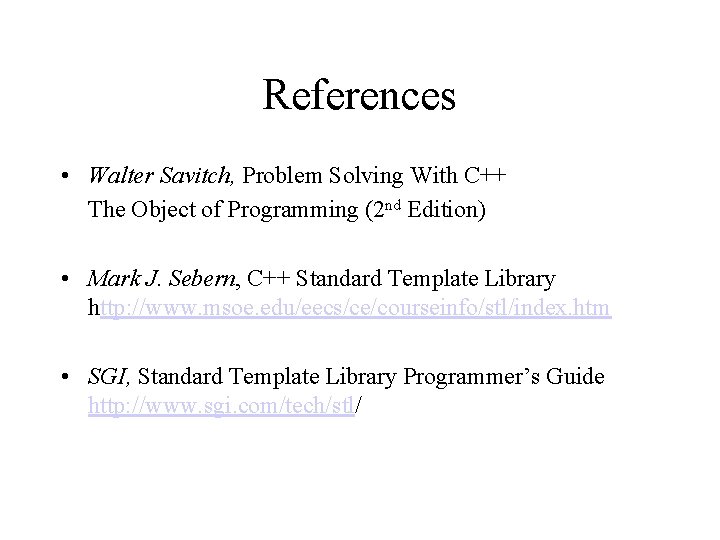
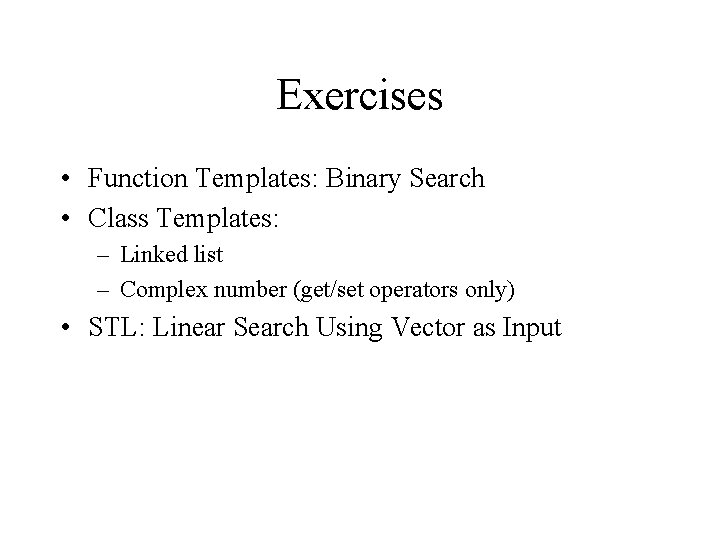
- Slides: 15
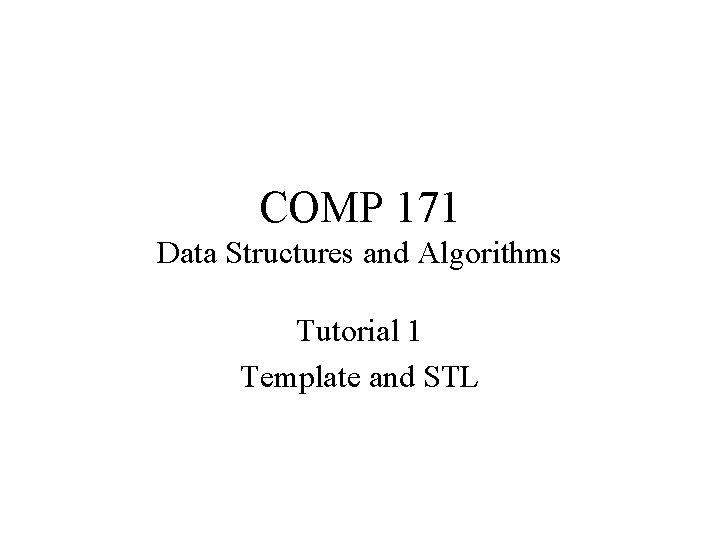
COMP 171 Data Structures and Algorithms Tutorial 1 Template and STL
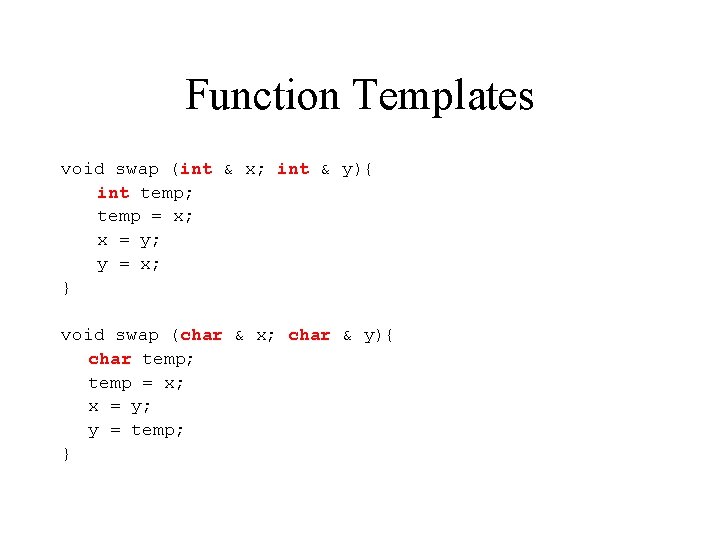
Function Templates void swap (int & x; int & y){ int temp; temp = x; x = y; y = x; } void swap (char & x; char & y){ char temp; temp = x; x = y; y = temp; }
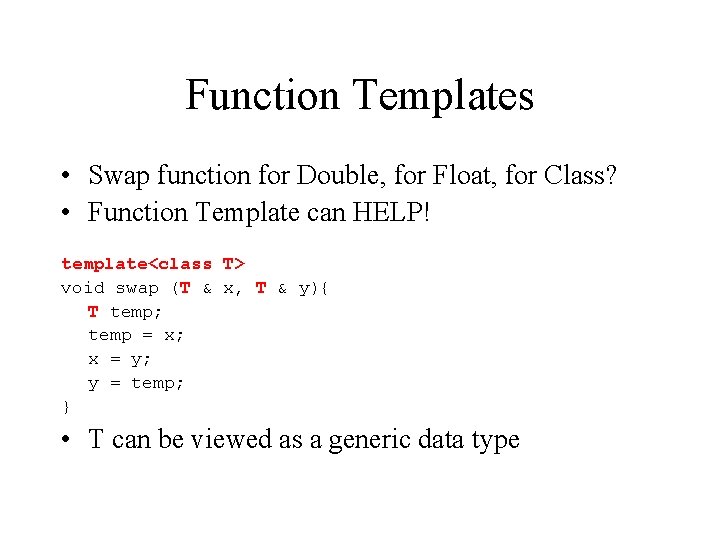
Function Templates • Swap function for Double, for Float, for Class? • Function Template can HELP! template<class T> void swap (T & x, T & y){ T temp; temp = x; x = y; y = temp; } • T can be viewed as a generic data type
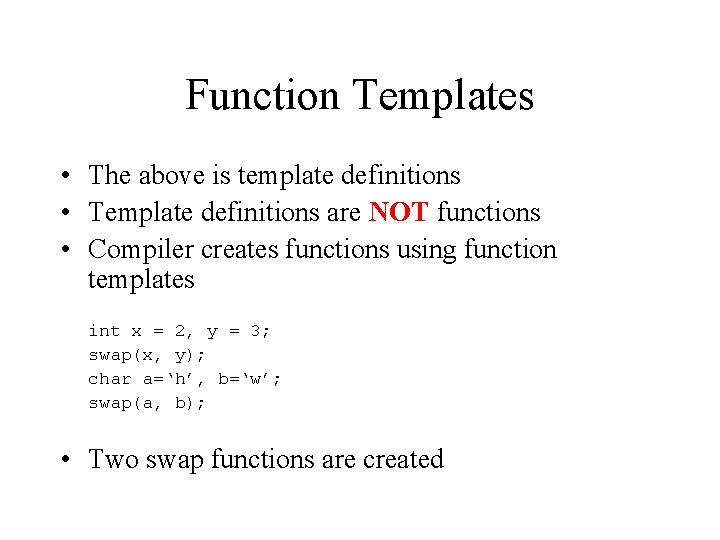
Function Templates • The above is template definitions • Template definitions are NOT functions • Compiler creates functions using function templates int x = 2, y = 3; swap(x, y); char a=‘h’, b=‘w’; swap(a, b); • Two swap functions are created
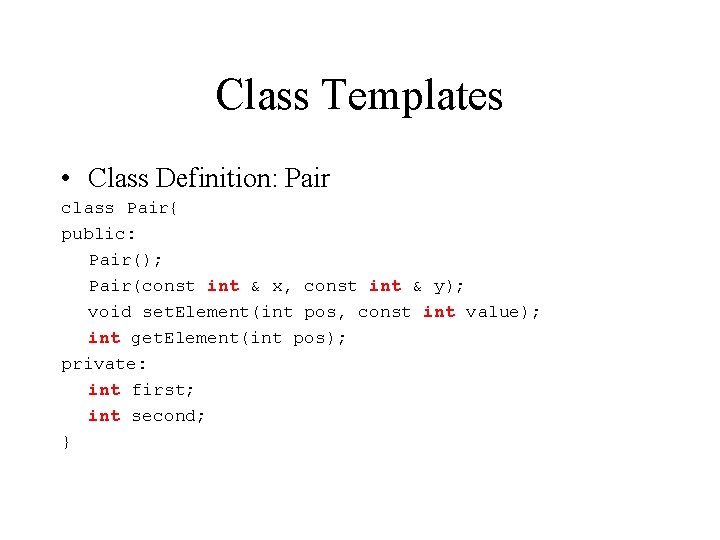
Class Templates • Class Definition: Pair class Pair{ public: Pair(); Pair(const int & x, const int & y); void set. Element(int pos, const int value); int get. Element(int pos); private: int first; int second; }
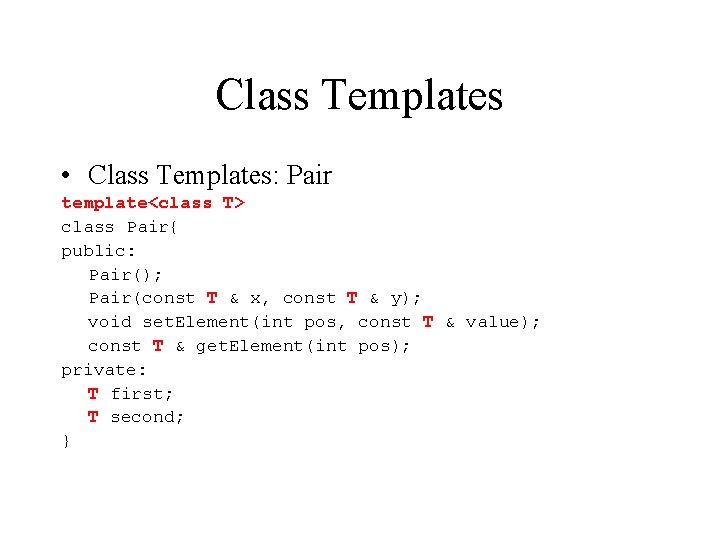
Class Templates • Class Templates: Pair template<class T> class Pair{ public: Pair(); Pair(const T & x, const T & y); void set. Element(int pos, const T & value); const T & get. Element(int pos); private: T first; T second; }
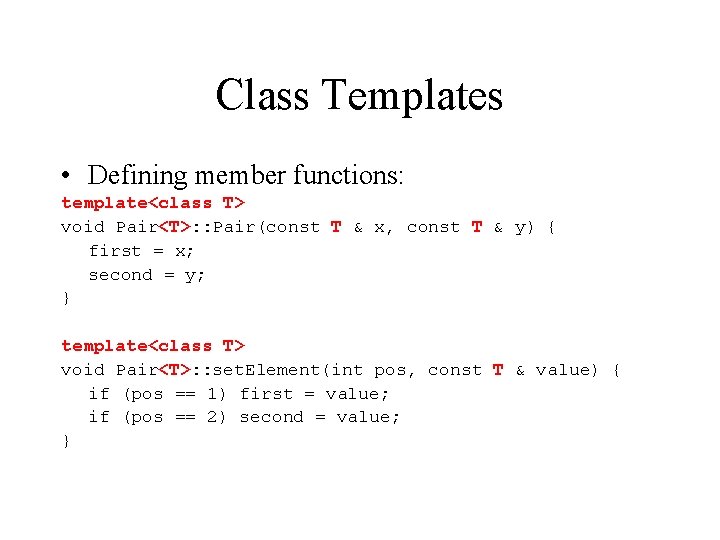
Class Templates • Defining member functions: template<class T> void Pair<T>: : Pair(const T & x, const T & y) { first = x; second = y; } template<class T> void Pair<T>: : set. Element(int pos, const T & value) { if (pos == 1) first = value; if (pos == 2) second = value; }
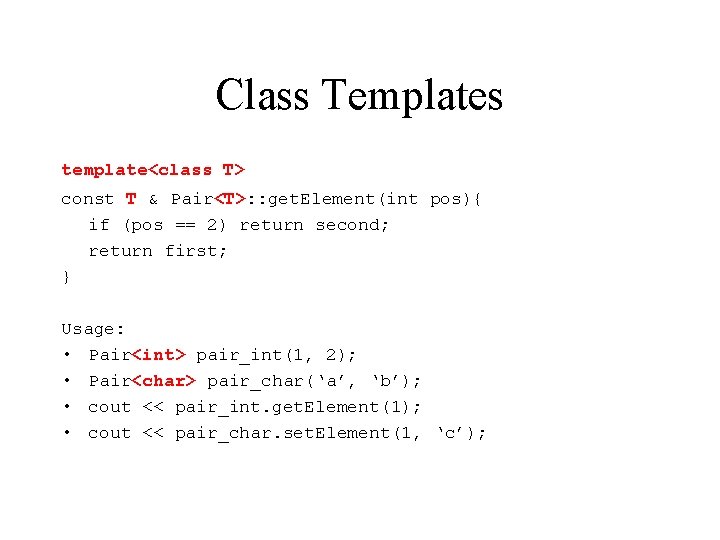
Class Templates template<class T> const T & Pair<T>: : get. Element(int pos){ if (pos == 2) return second; return first; } Usage: • Pair<int> pair_int(1, 2); • Pair<char> pair_char(‘a’, ‘b’); • cout << pair_int. get. Element(1); • cout << pair_char. set. Element(1, ‘c’);
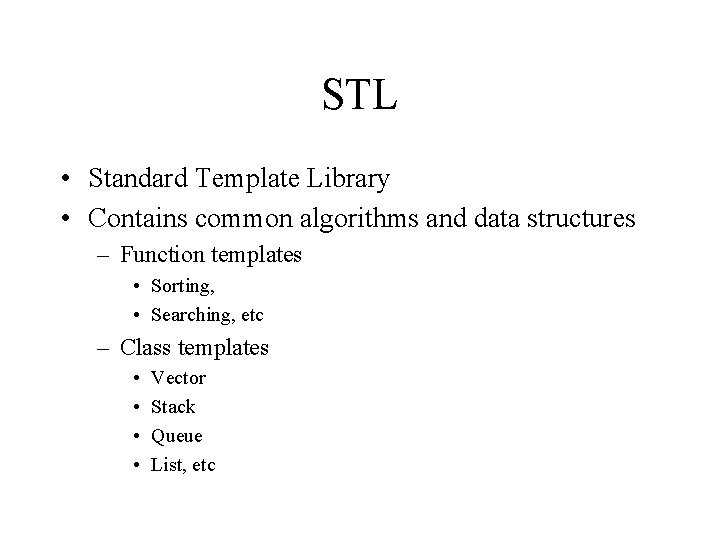
STL • Standard Template Library • Contains common algorithms and data structures – Function templates • Sorting, • Searching, etc – Class templates • • Vector Stack Queue List, etc
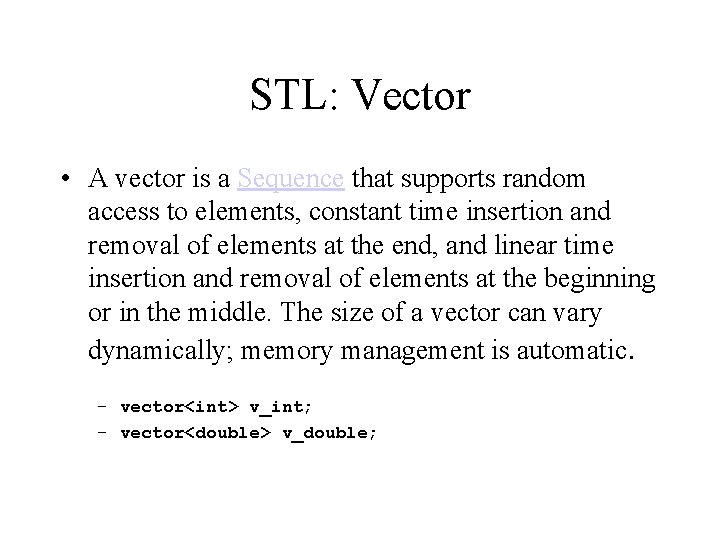
STL: Vector • A vector is a Sequence that supports random access to elements, constant time insertion and removal of elements at the end, and linear time insertion and removal of elements at the beginning or in the middle. The size of a vector can vary dynamically; memory management is automatic. – vector<int> v_int; – vector<double> v_double;
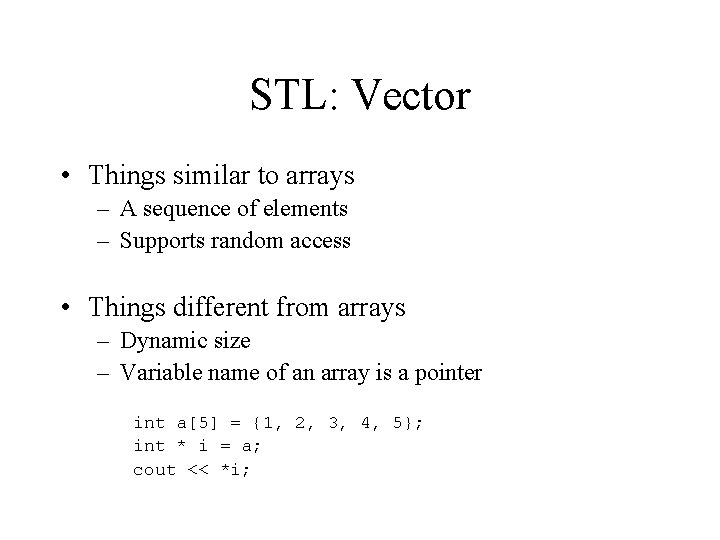
STL: Vector • Things similar to arrays – A sequence of elements – Supports random access • Things different from arrays – Dynamic size – Variable name of an array is a pointer int a[5] = {1, 2, 3, 4, 5}; int * i = a; cout << *i;
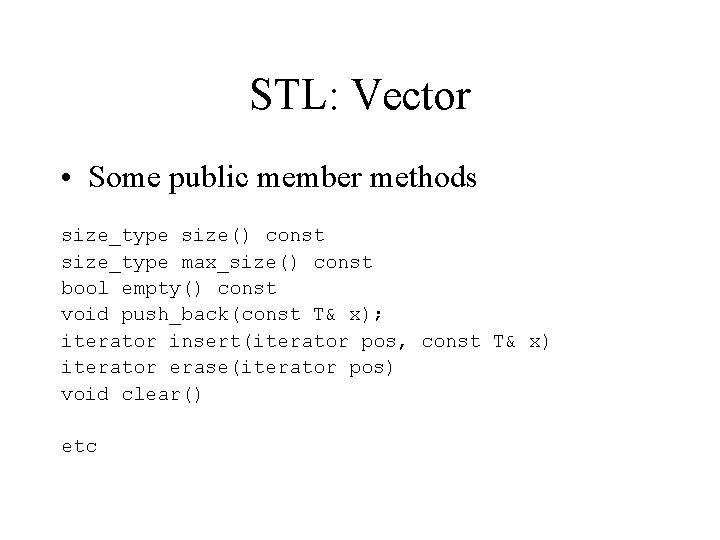
STL: Vector • Some public member methods size_type size() const size_type max_size() const bool empty() const void push_back(const T& x); iterator insert(iterator pos, const T& x) iterator erase(iterator pos) void clear() etc
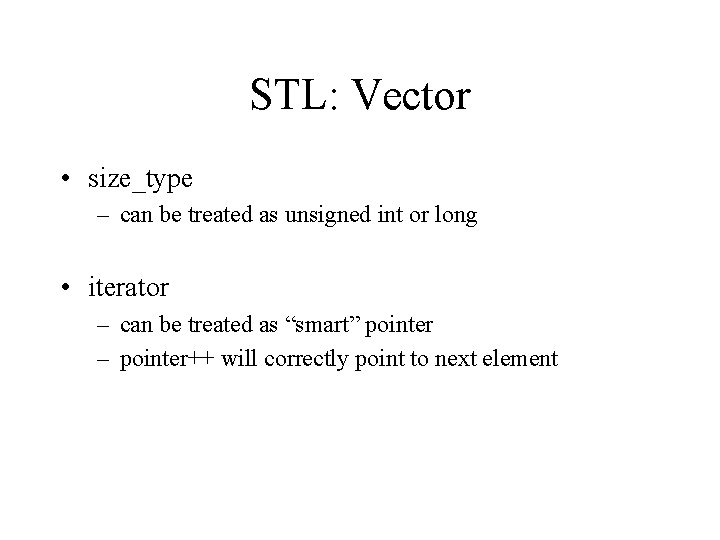
STL: Vector • size_type – can be treated as unsigned int or long • iterator – can be treated as “smart” pointer – pointer++ will correctly point to next element
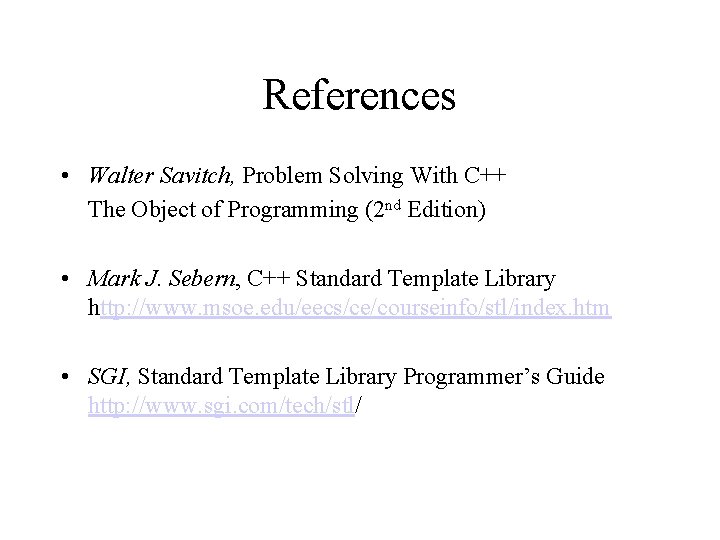
References • Walter Savitch, Problem Solving With C++ The Object of Programming (2 nd Edition) • Mark J. Sebern, C++ Standard Template Library http: //www. msoe. edu/eecs/ce/courseinfo/stl/index. htm • SGI, Standard Template Library Programmer’s Guide http: //www. sgi. com/tech/stl/
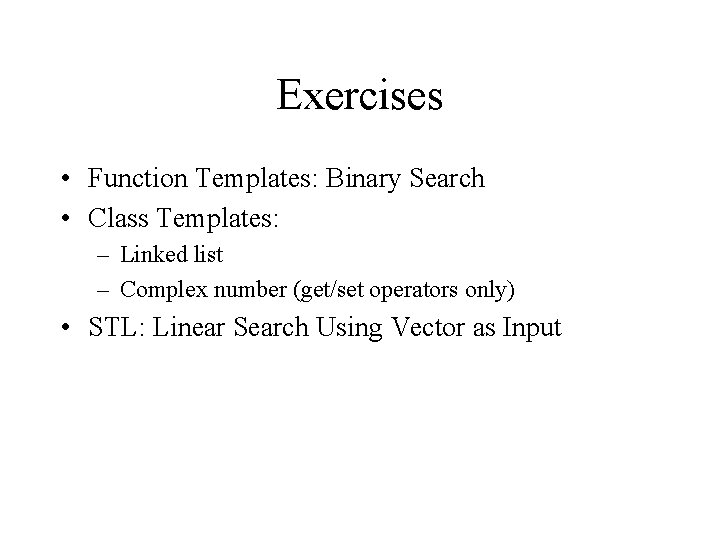
Exercises • Function Templates: Binary Search • Class Templates: – Linked list – Complex number (get/set operators only) • STL: Linear Search Using Vector as Input
Data structures and algorithms tutorial
Ajit diwan iit bombay
Princeton data structures and algorithms
Information retrieval data structures and algorithms
Data structures and algorithms bits pilani
Data structures and algorithms iit bombay
Data structures and algorithms
Data structures and algorithms
Ian munro waterloo
Signature file structure in information retrieval system
Data structures and algorithms
Algorithms + data structures = programs
Pg 171
Lei 171 de 2007
Nosotros salir
Nonlinear pricing strategies