COMP 171 Data Structures and Algorithms Tutorial 4
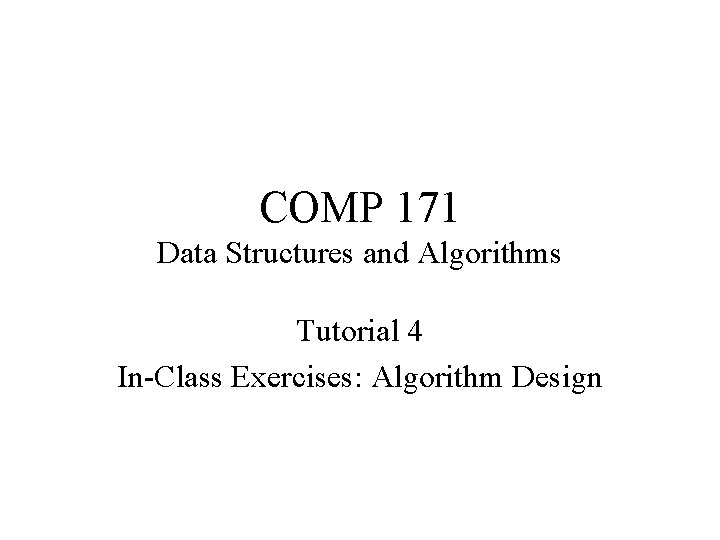
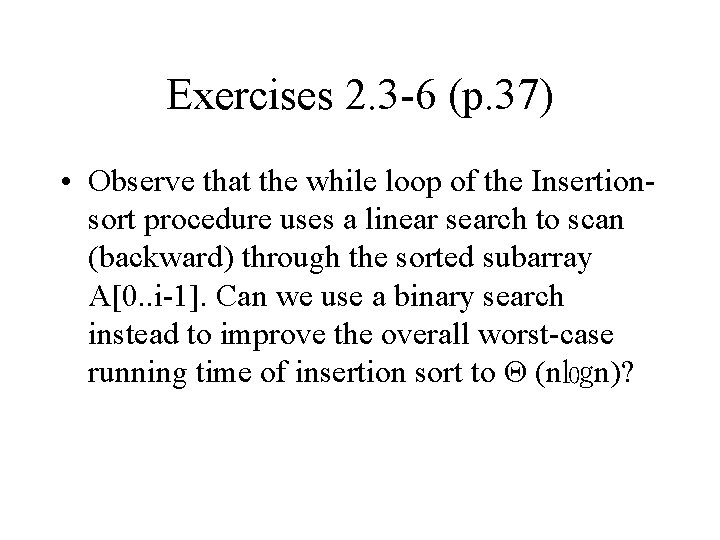
![Insertion. Sort(A) for i ← 1 to size(A)-1 key ← A[i] j ← i Insertion. Sort(A) for i ← 1 to size(A)-1 key ← A[i] j ← i](https://slidetodoc.com/presentation_image_h2/a2976dbd6bcf089e1905f048830984e5/image-3.jpg)
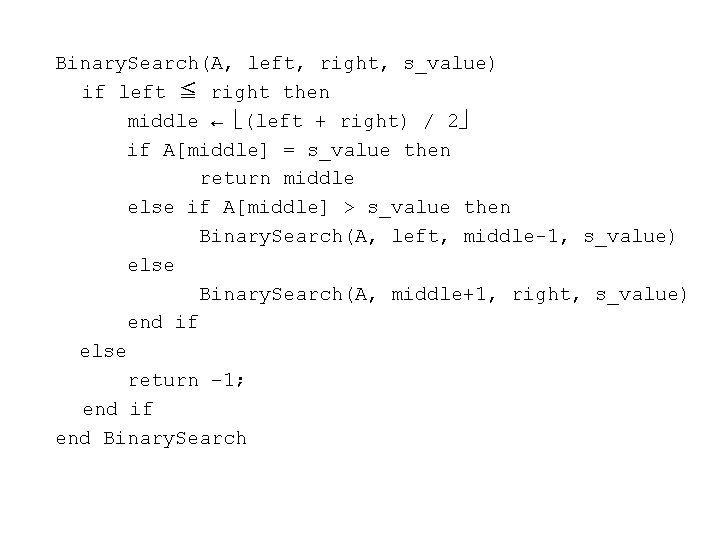
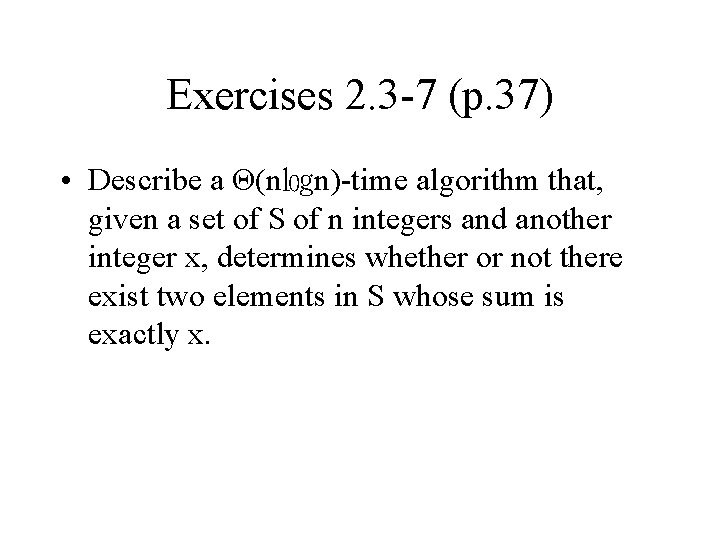
![Exercises 2 -4 (p. 39) • Let A[1. . n] be an array of Exercises 2 -4 (p. 39) • Let A[1. . n] be an array of](https://slidetodoc.com/presentation_image_h2/a2976dbd6bcf089e1905f048830984e5/image-6.jpg)
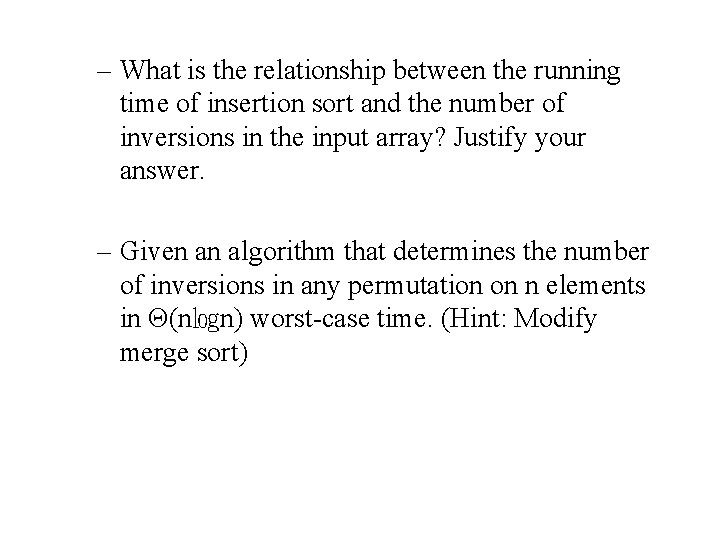
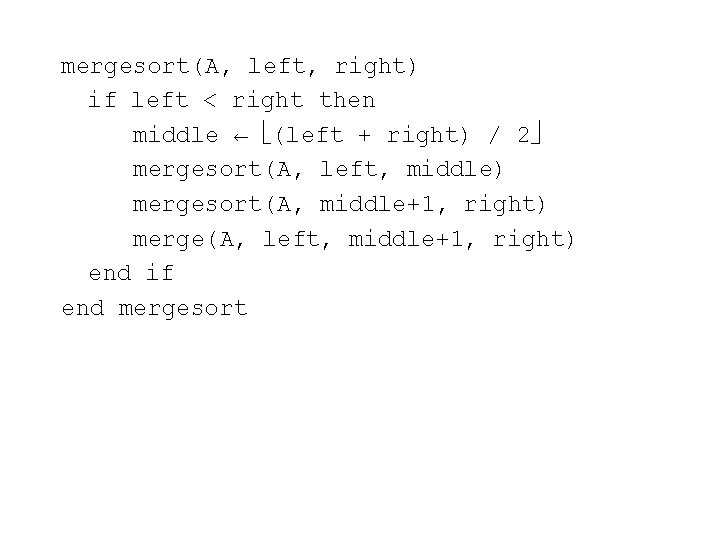
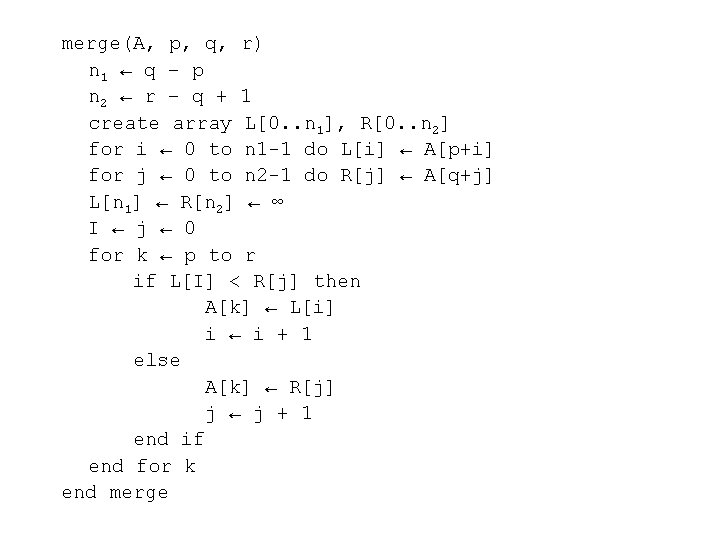
- Slides: 9
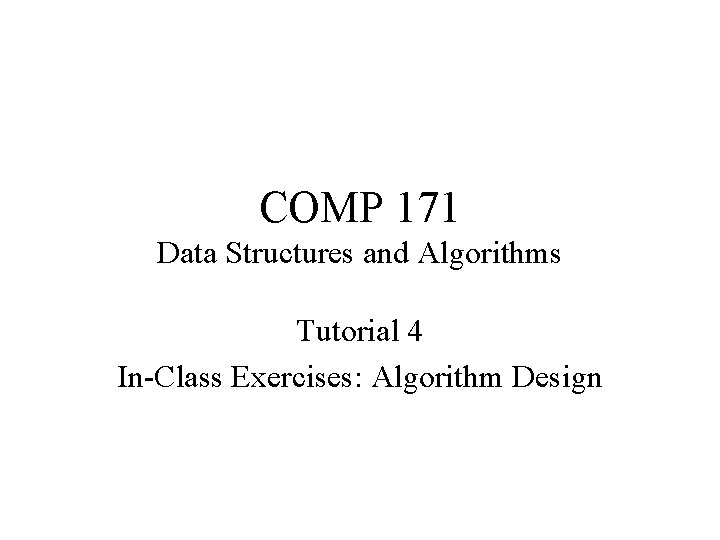
COMP 171 Data Structures and Algorithms Tutorial 4 In-Class Exercises: Algorithm Design
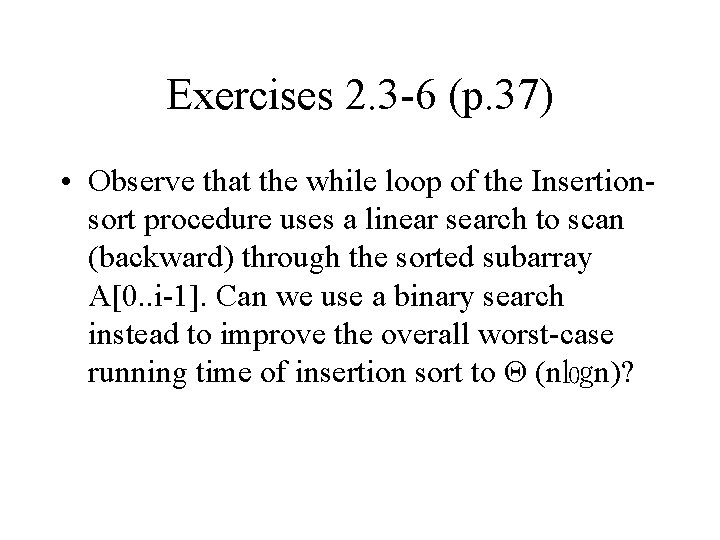
Exercises 2. 3 -6 (p. 37) • Observe that the while loop of the Insertionsort procedure uses a linear search to scan (backward) through the sorted subarray A[0. . i-1]. Can we use a binary search instead to improve the overall worst-case running time of insertion sort to Θ (n㏒n)?
![Insertion SortA for i 1 to sizeA1 key Ai j i Insertion. Sort(A) for i ← 1 to size(A)-1 key ← A[i] j ← i](https://slidetodoc.com/presentation_image_h2/a2976dbd6bcf089e1905f048830984e5/image-3.jpg)
Insertion. Sort(A) for i ← 1 to size(A)-1 key ← A[i] j ← i – 1 while j ≧ 0 and A[j] > key A[j + 1] ← A[j] j ← j – 1 end while A[j + 1] ← key end for i end Insertion. Sort
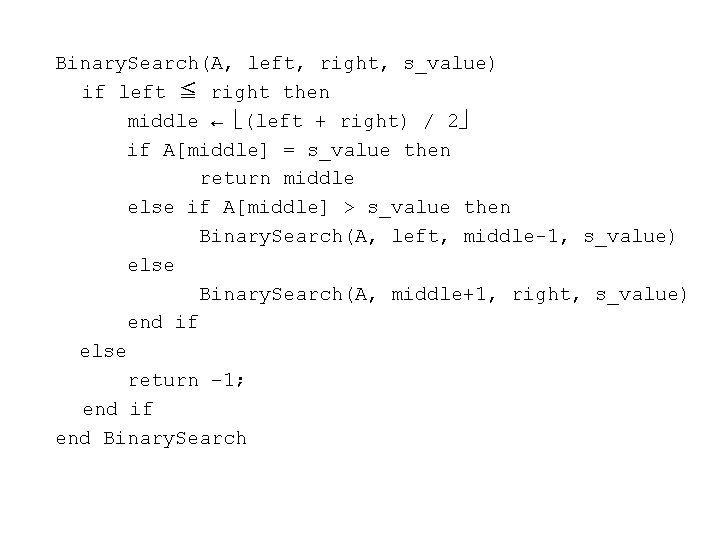
Binary. Search(A, left, right, s_value) if left ≦ right then middle ← (left + right) / 2 if A[middle] = s_value then return middle else if A[middle] > s_value then Binary. Search(A, left, middle-1, s_value) else Binary. Search(A, middle+1, right, s_value) end if else return – 1; end if end Binary. Search
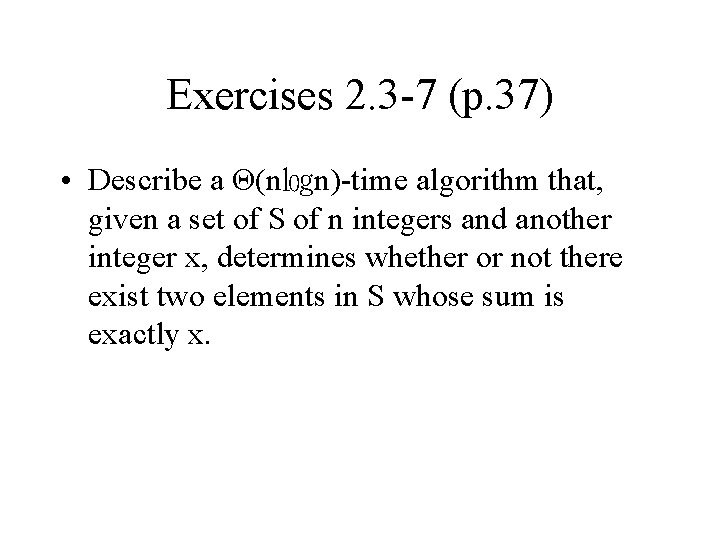
Exercises 2. 3 -7 (p. 37) • Describe a Θ(n㏒n)-time algorithm that, given a set of S of n integers and another integer x, determines whether or not there exist two elements in S whose sum is exactly x.
![Exercises 2 4 p 39 Let A1 n be an array of Exercises 2 -4 (p. 39) • Let A[1. . n] be an array of](https://slidetodoc.com/presentation_image_h2/a2976dbd6bcf089e1905f048830984e5/image-6.jpg)
Exercises 2 -4 (p. 39) • Let A[1. . n] be an array of n distinct numbers. If i < j and A[i] > A[j], then the pair (i, j) is called an inversion of A. – List the five inversions of the array {2, 3, 8, 6, 1} – What array with elements from the set {1, 2, …, n} has the most inversions? How many does it have?
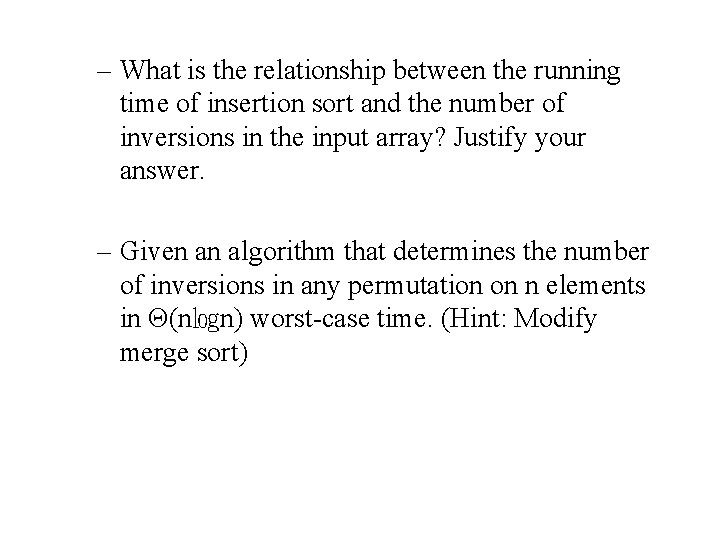
– What is the relationship between the running time of insertion sort and the number of inversions in the input array? Justify your answer. – Given an algorithm that determines the number of inversions in any permutation on n elements in Θ(n㏒n) worst-case time. (Hint: Modify merge sort)
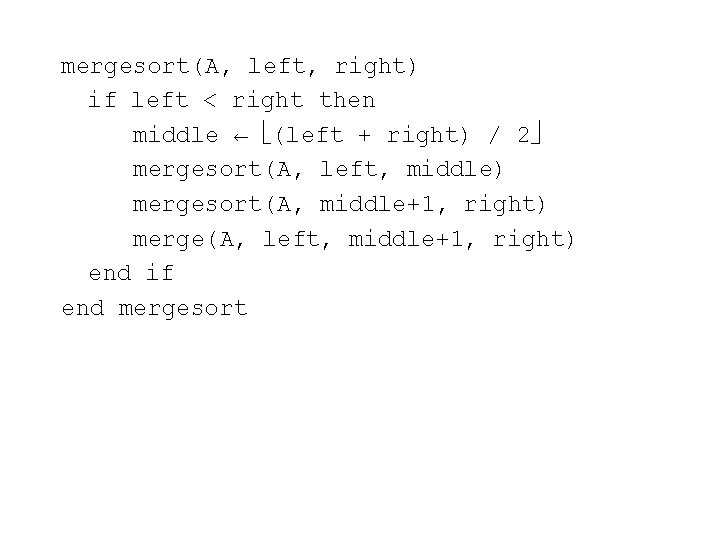
mergesort(A, left, right) if left < right then middle ← (left + right) / 2 mergesort(A, left, middle) mergesort(A, middle+1, right) merge(A, left, middle+1, right) end if end mergesort
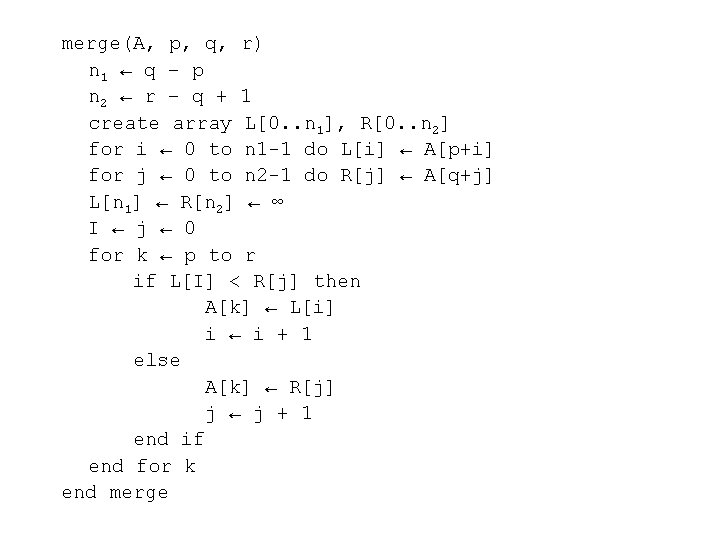
merge(A, p, q, r) n 1 ← q – p n 2 ← r – q + 1 create array L[0. . n 1], R[0. . n 2] for i ← 0 to n 1 -1 do L[i] ← A[p+i] for j ← 0 to n 2 -1 do R[j] ← A[q+j] L[n 1] ← R[n 2] ← ∞ I ← j ← 0 for k ← p to r if L[I] < R[j] then A[k] ← L[i] i ← i + 1 else A[k] ← R[j] j ← j + 1 end if end for k end merge
Data structures and algorithms tutorial
Ajit diwan
Cos 423 princeton
Information retrieval data structures and algorithms
Data structures and algorithms bits pilani
Data structures and algorithms iit bombay
Data structures and algorithms
Data structures and algorithms
Ian munro waterloo
Information retrieval data structures and algorithms