class Person private String name private int age
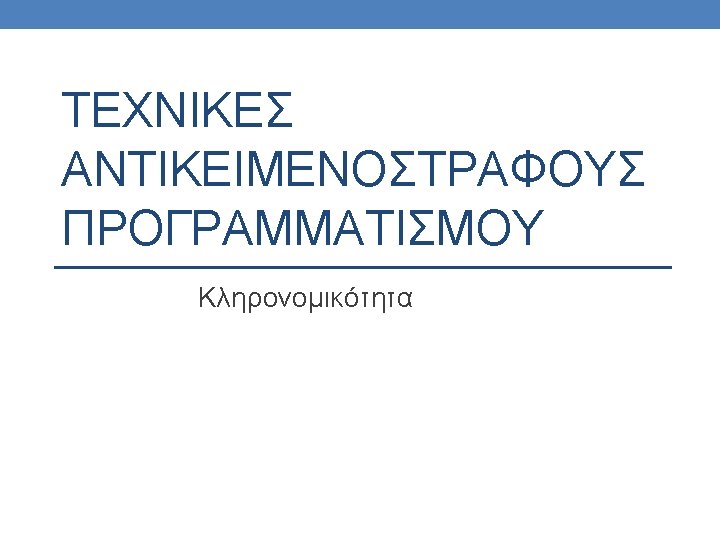
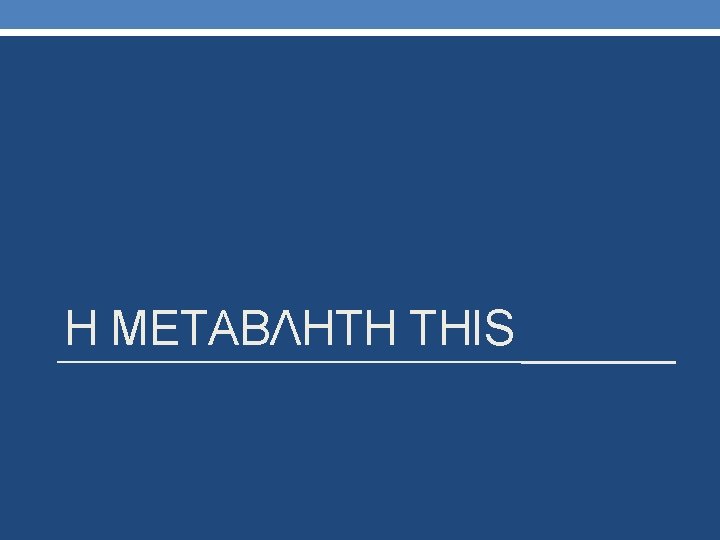
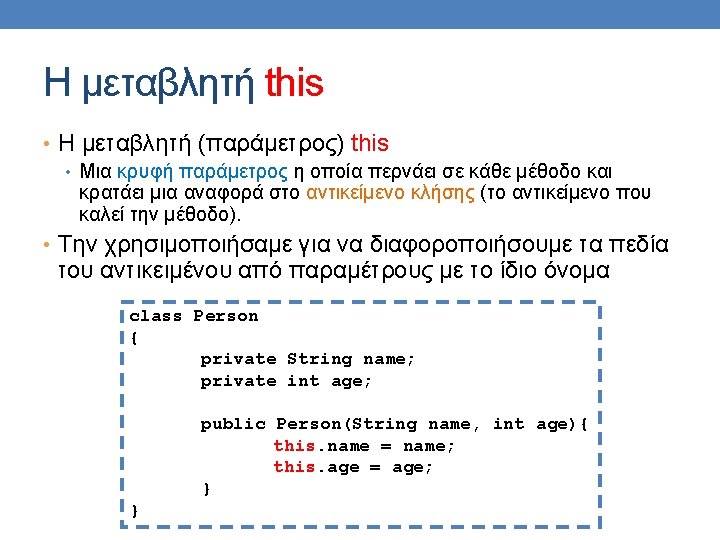
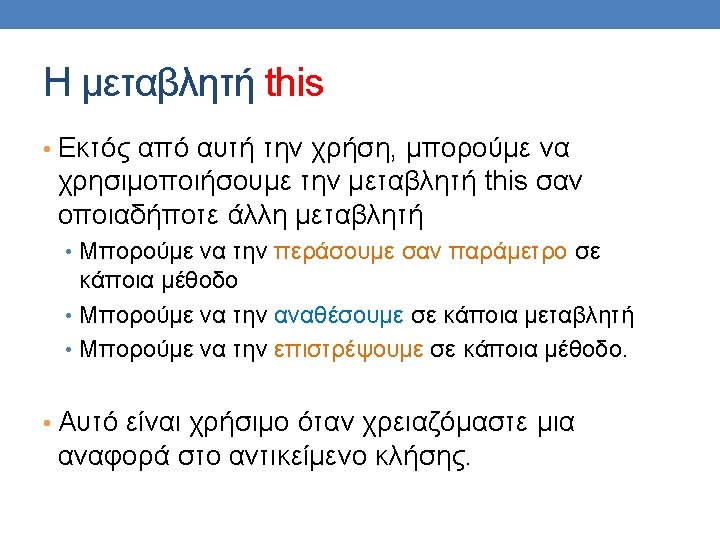
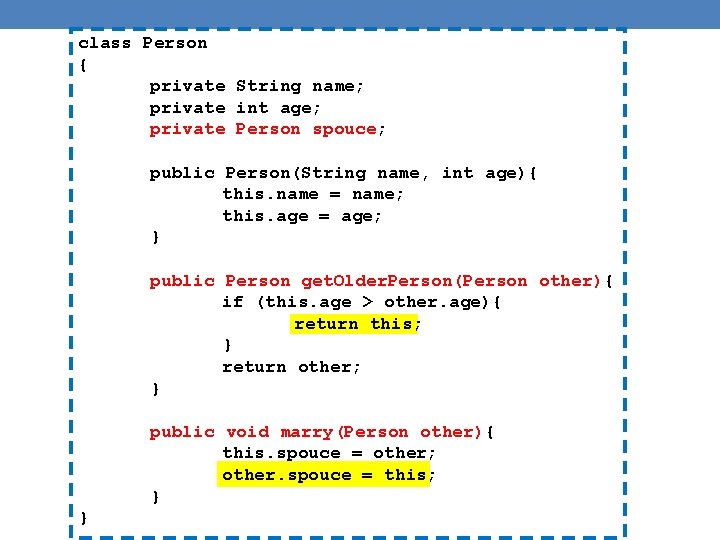
![class Test { public static void main(String[] args){ Person alice = new Person("Alice", 30); class Test { public static void main(String[] args){ Person alice = new Person("Alice", 30);](https://slidetodoc.com/presentation_image_h2/54025790b7c56deab685e5849b85eea8/image-6.jpg)
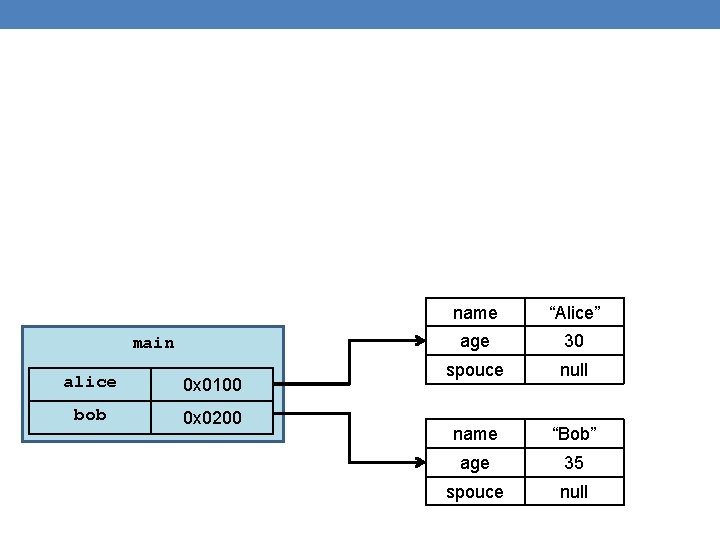
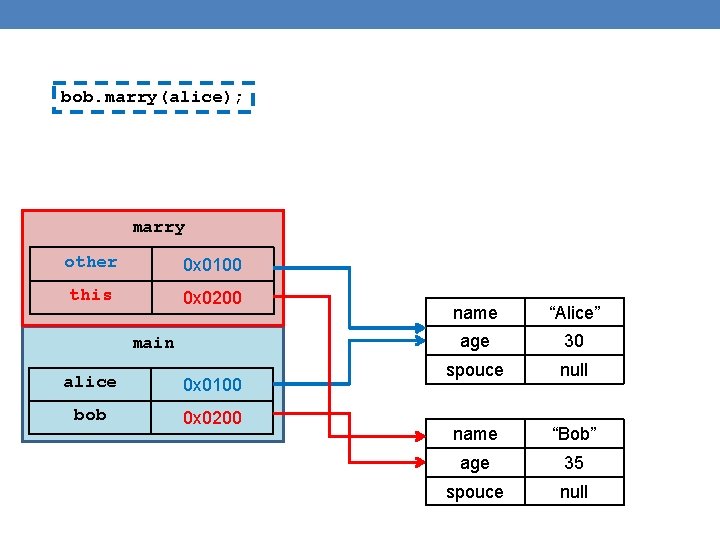
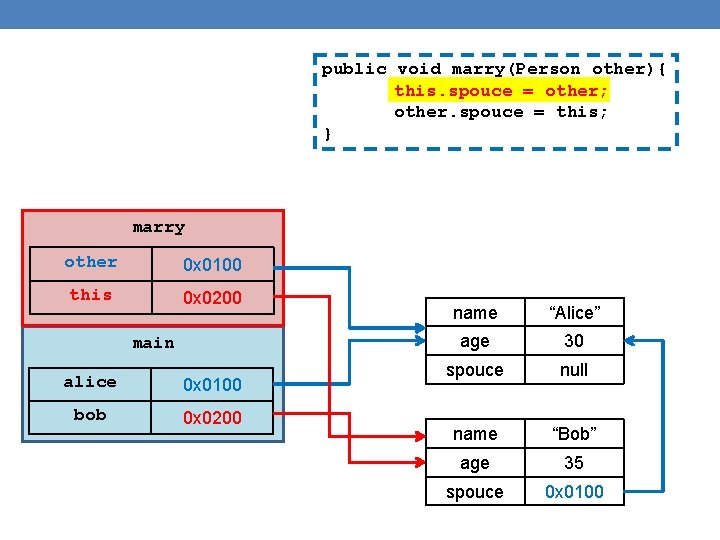
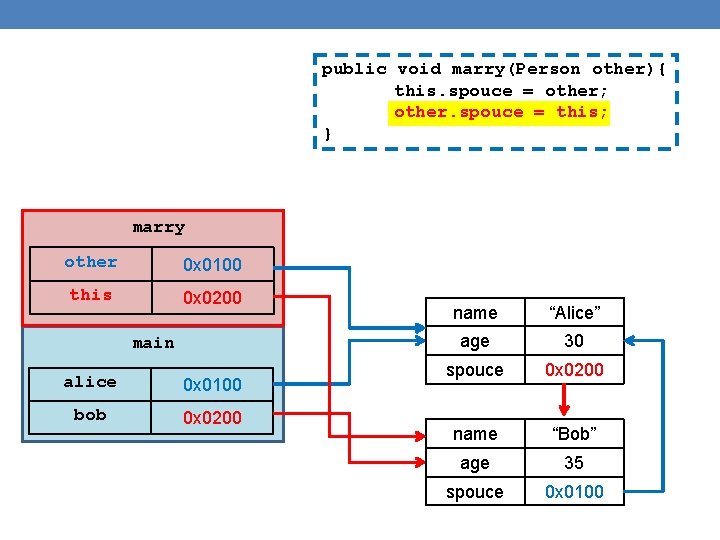
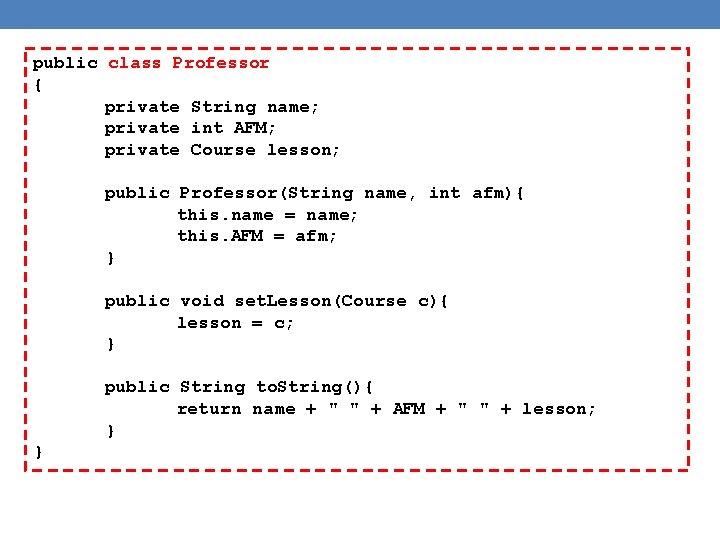
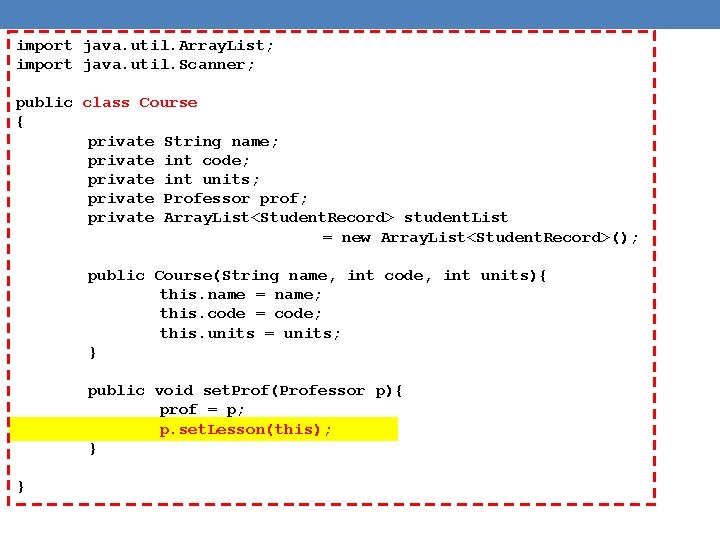
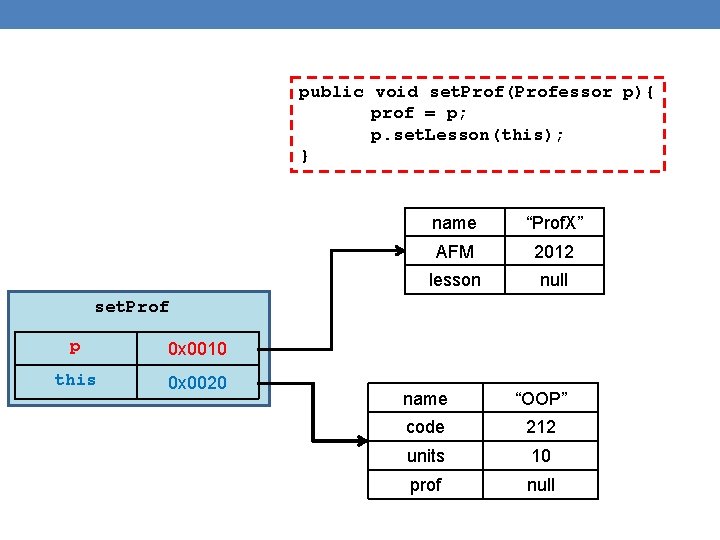
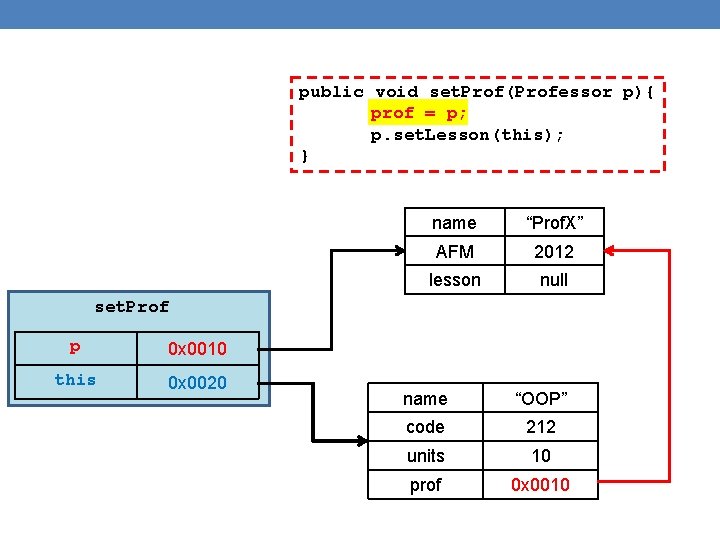
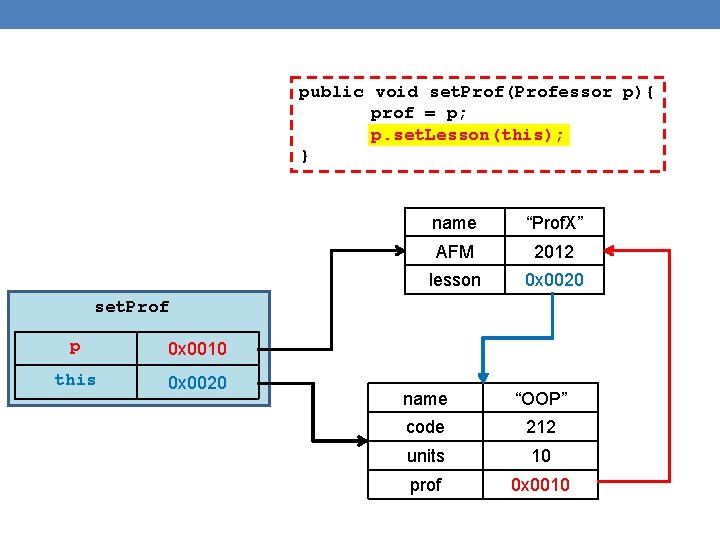
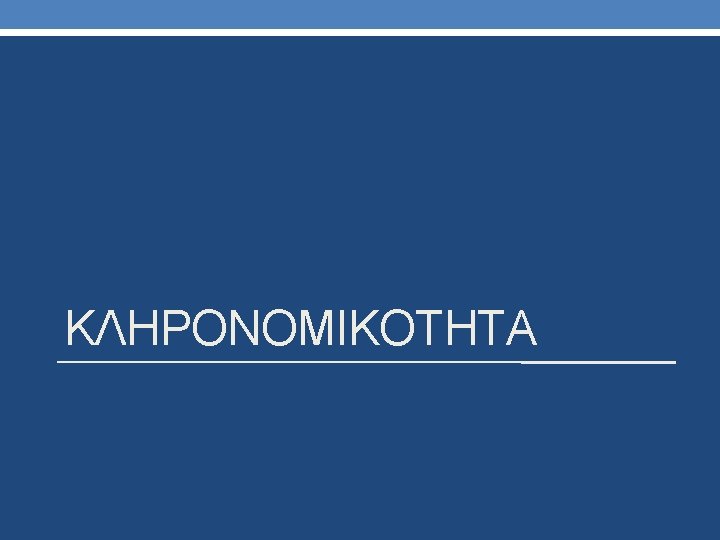
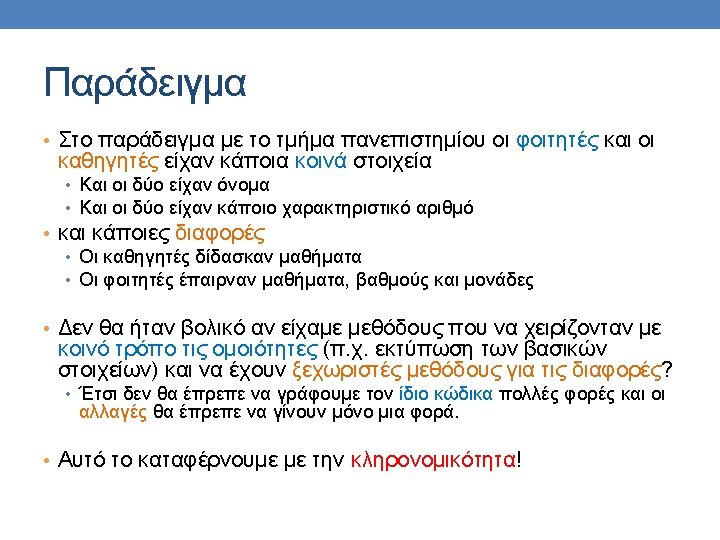
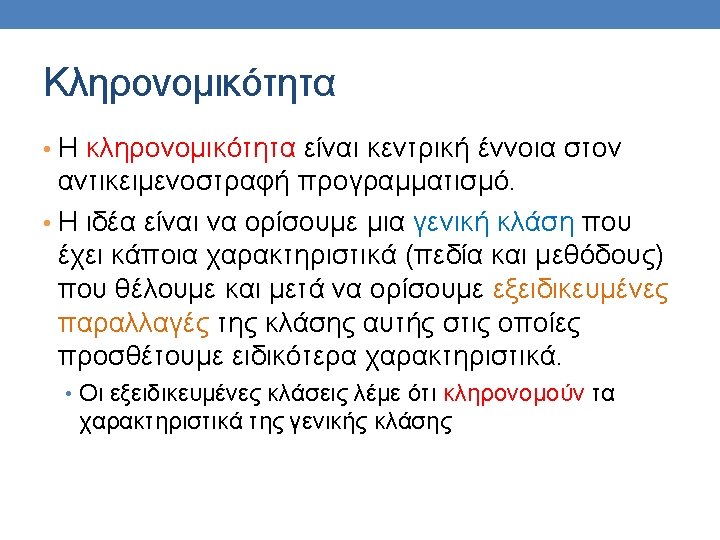
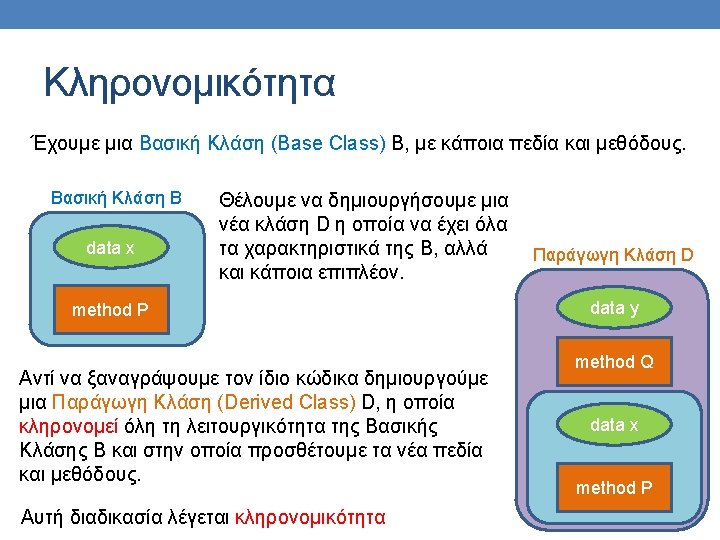
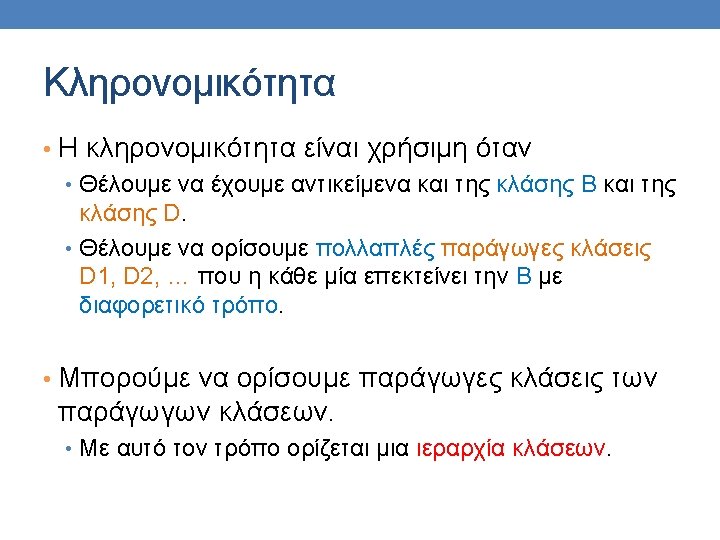
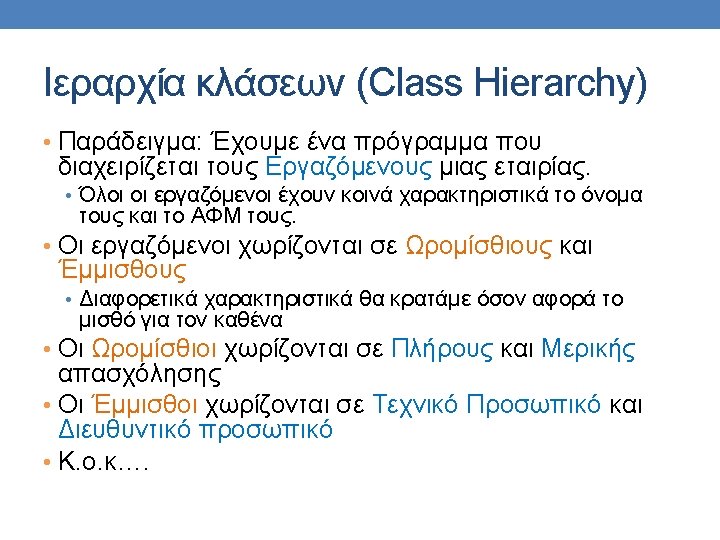
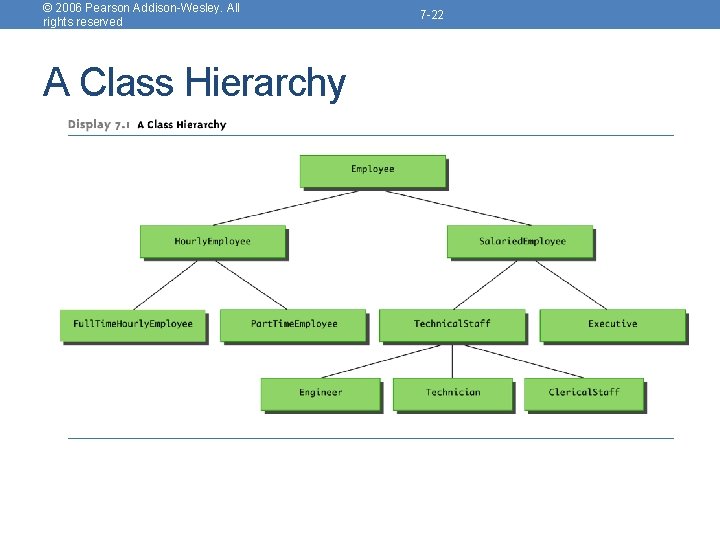
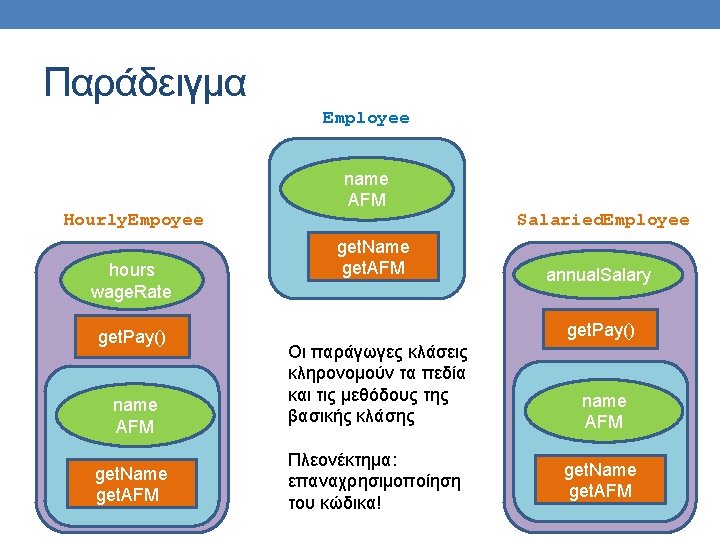
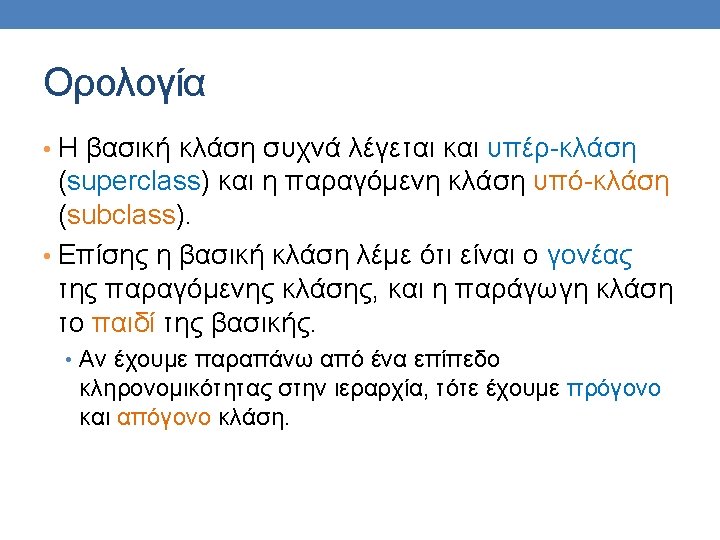
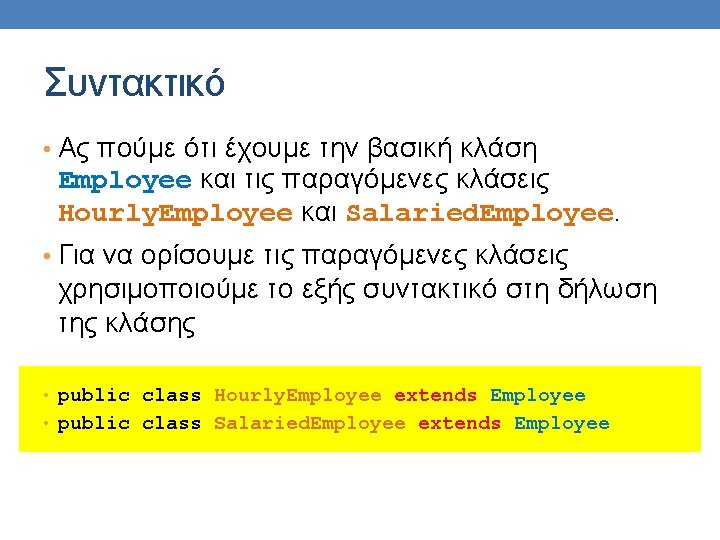
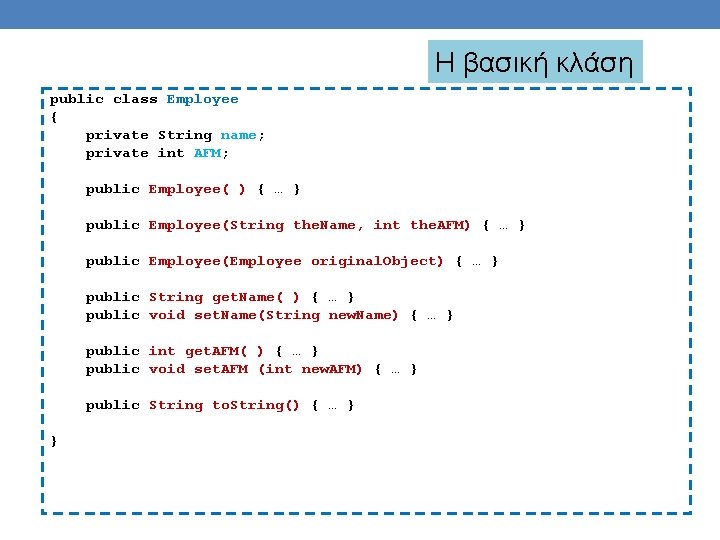
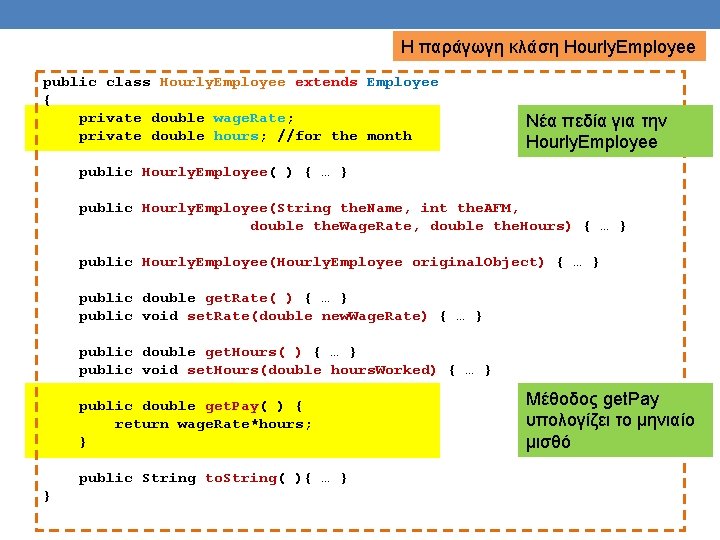
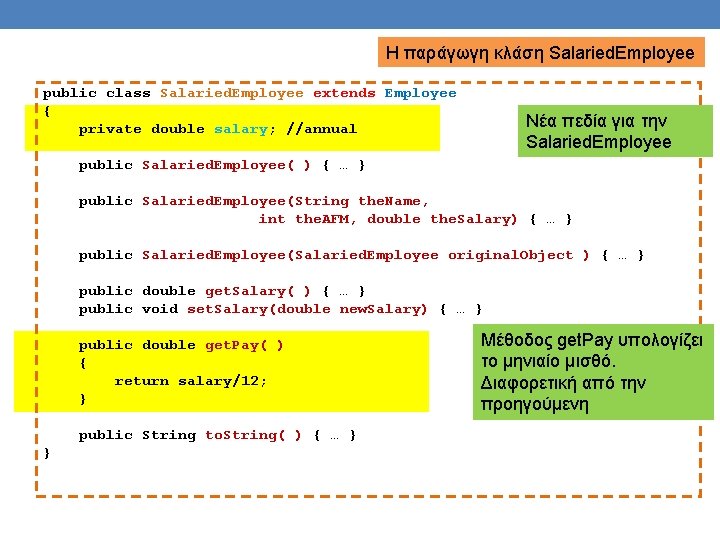
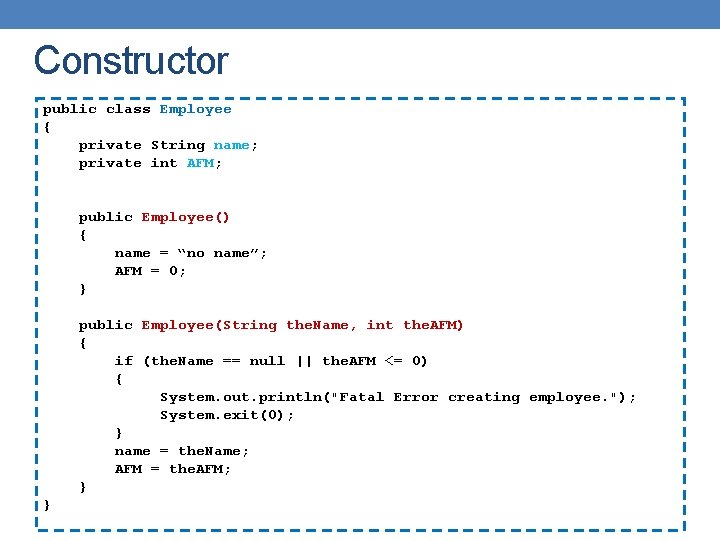
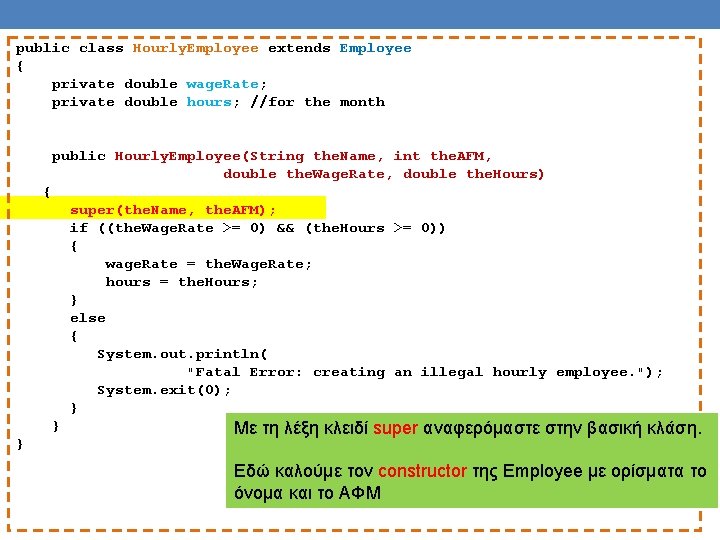
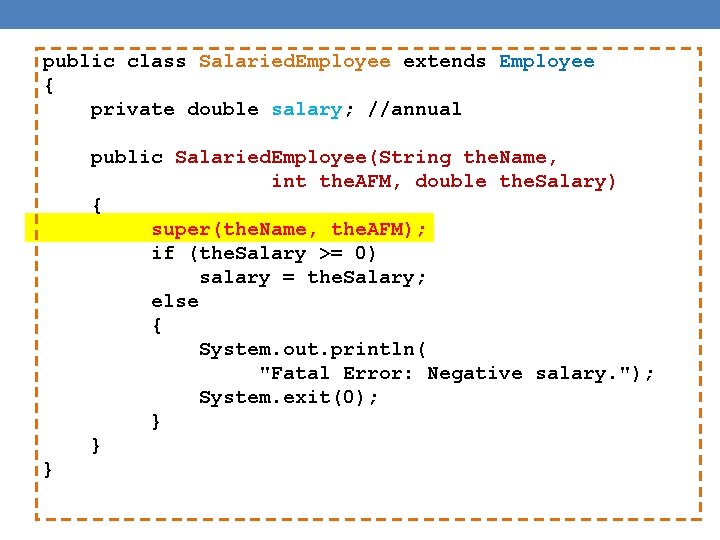
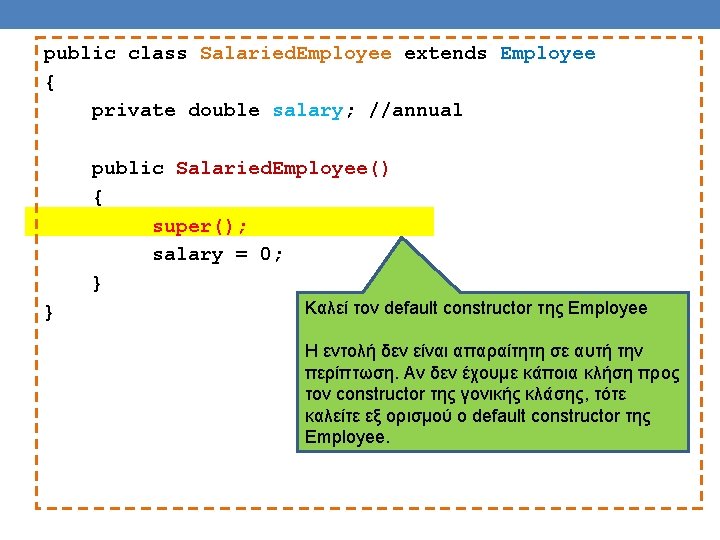
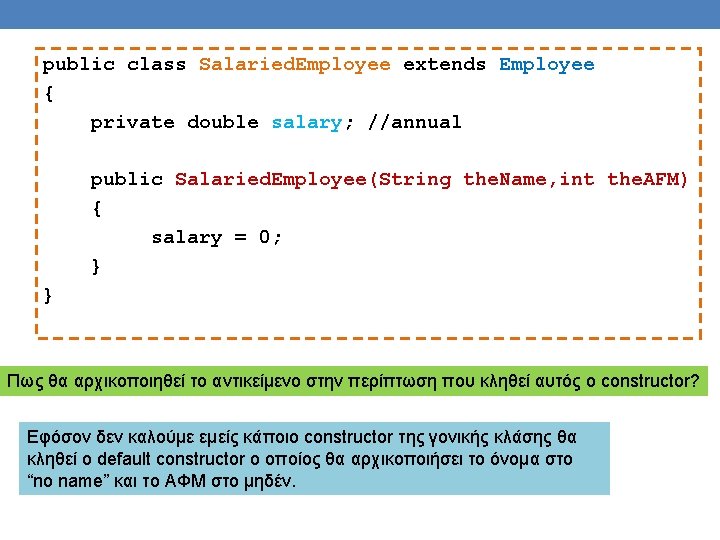
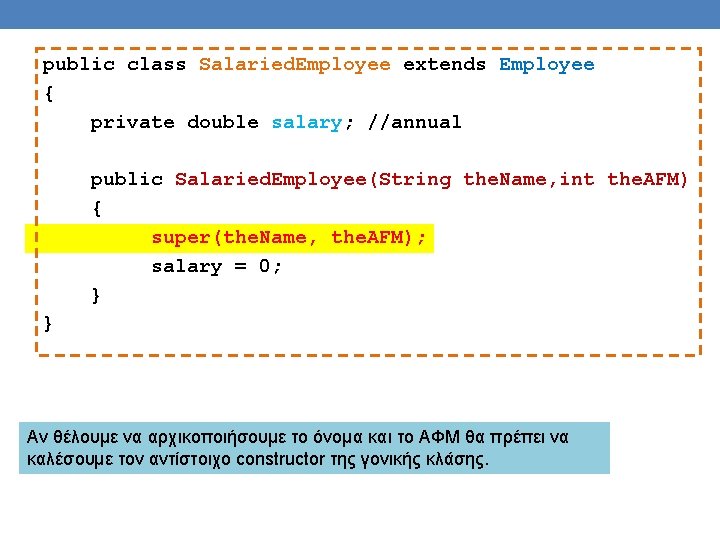
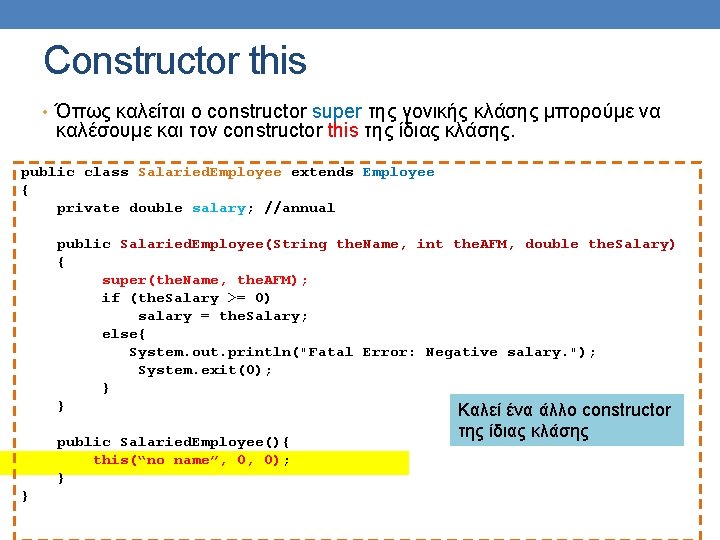
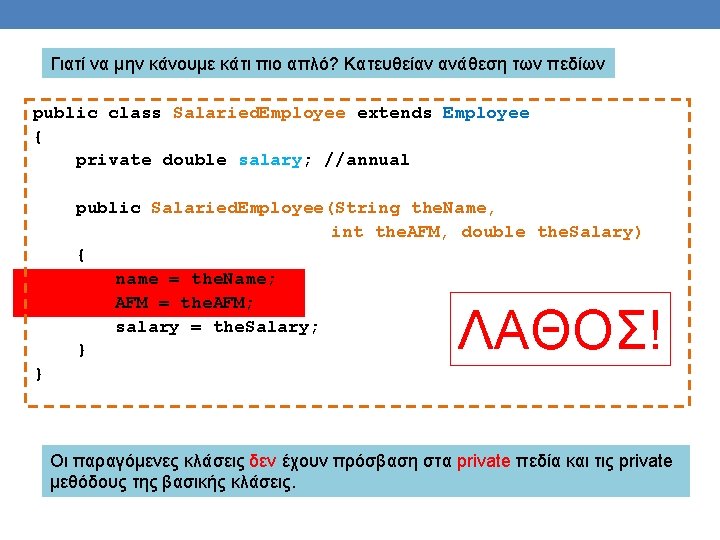
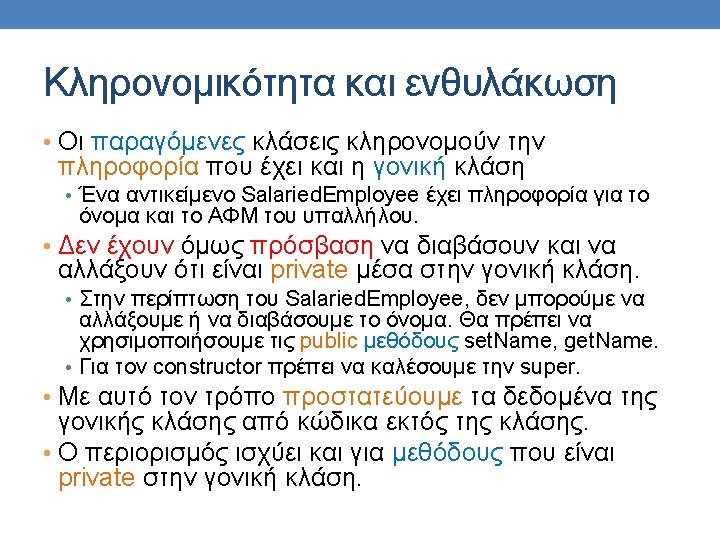
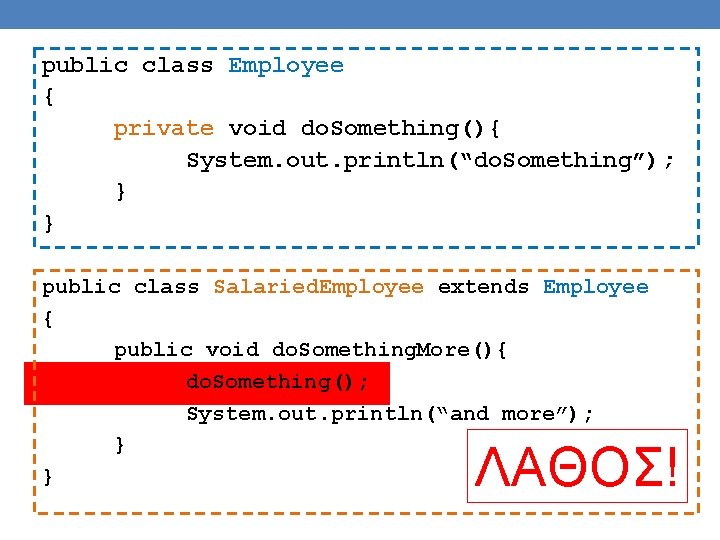
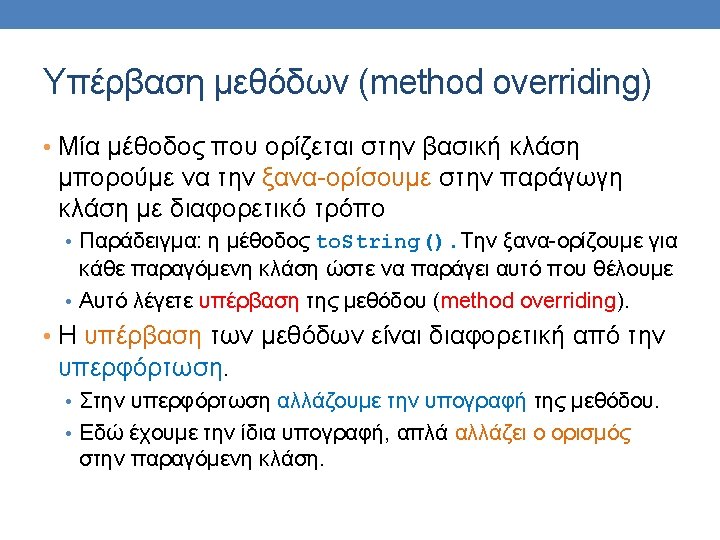
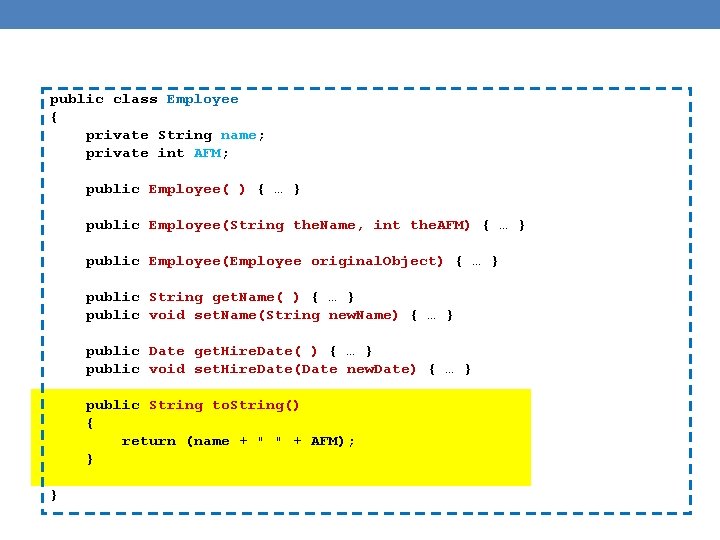
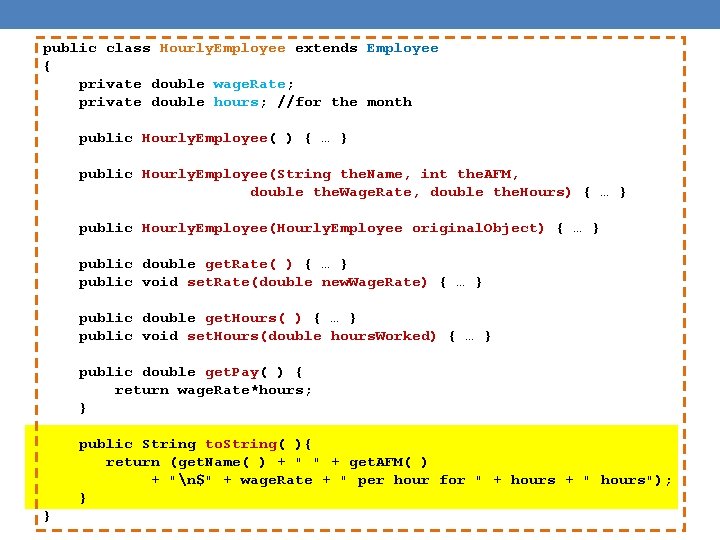
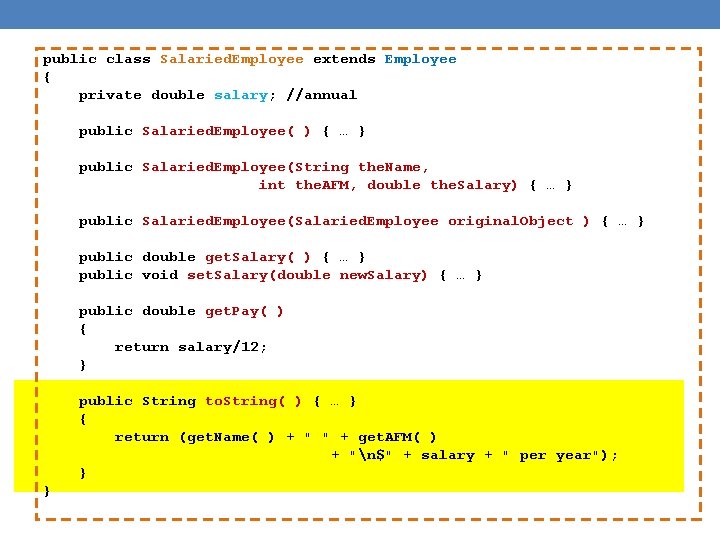
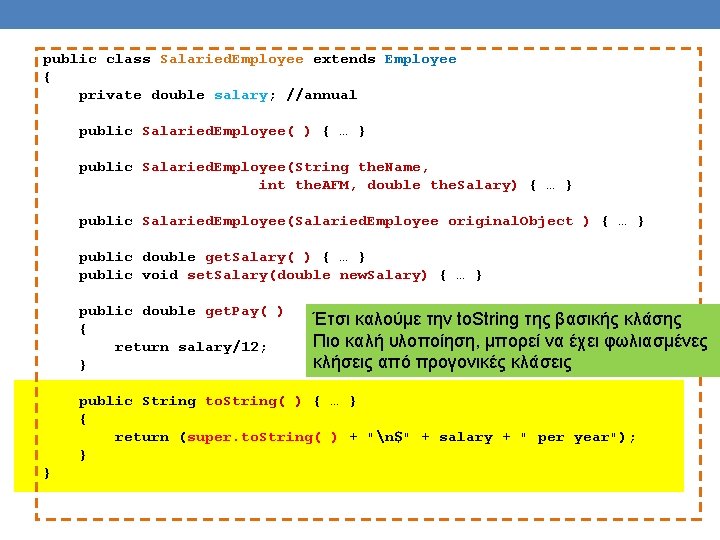
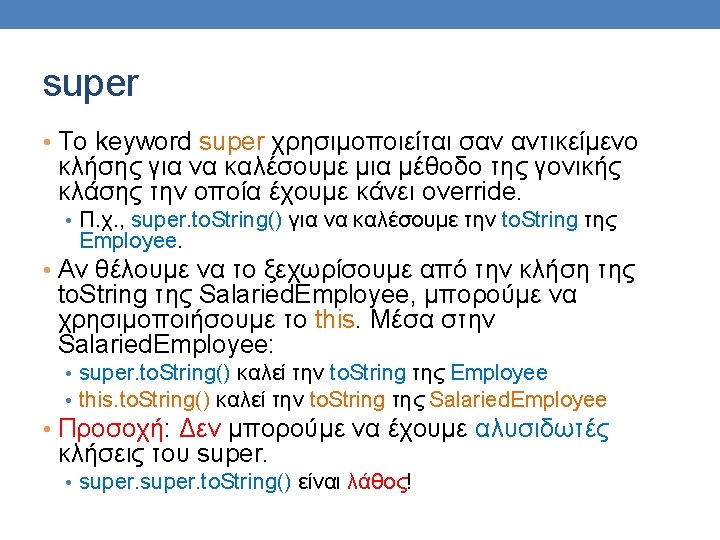
![Παράδειγμα χρήσης public class Inheritance. Demo { public static void main(String[] args) { Hourly. Παράδειγμα χρήσης public class Inheritance. Demo { public static void main(String[] args) { Hourly.](https://slidetodoc.com/presentation_image_h2/54025790b7c56deab685e5849b85eea8/image-45.jpg)
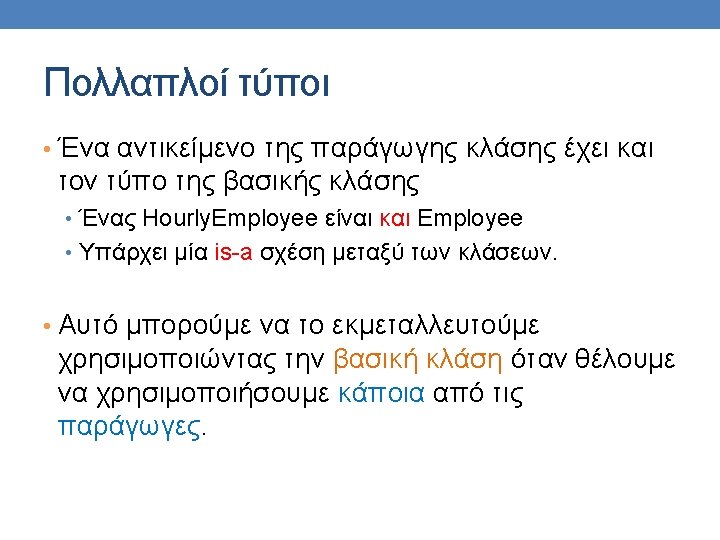
![public class Is. ADemo { public static void main(String[] args) { Salaried. Employee joe public class Is. ADemo { public static void main(String[] args) { Salaried. Employee joe](https://slidetodoc.com/presentation_image_h2/54025790b7c56deab685e5849b85eea8/image-47.jpg)
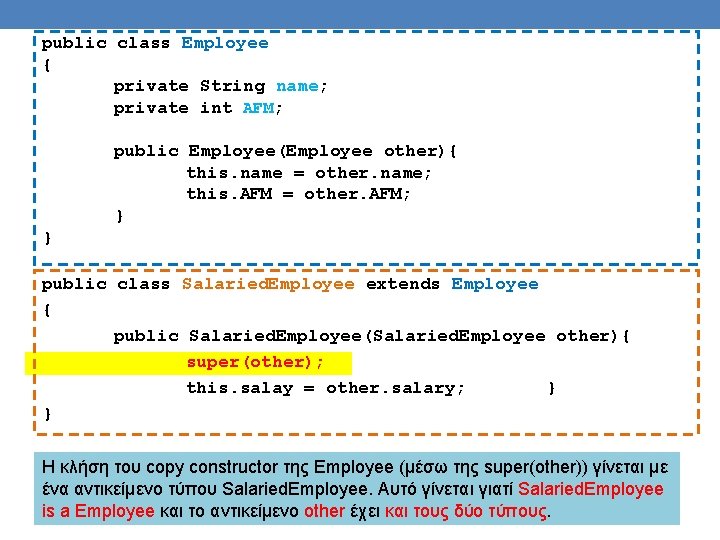
![public class Is. ADemo { public static void main(String[] args) { Salaried. Employee joe public class Is. ADemo { public static void main(String[] args) { Salaried. Employee joe](https://slidetodoc.com/presentation_image_h2/54025790b7c56deab685e5849b85eea8/image-49.jpg)
- Slides: 49
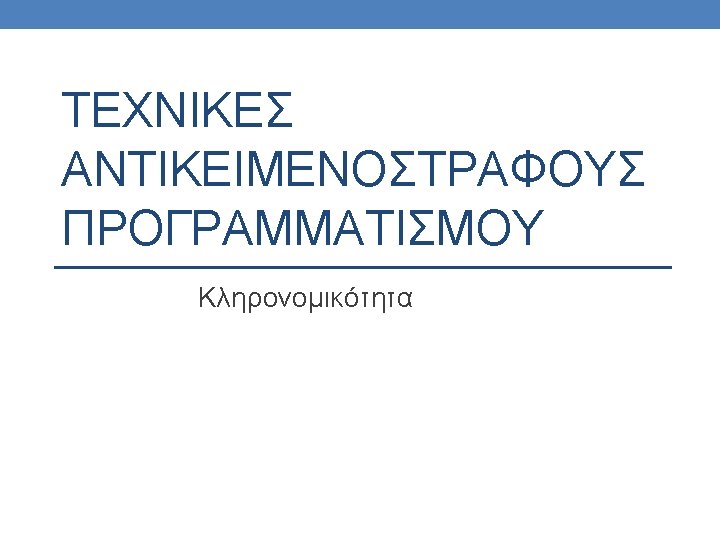
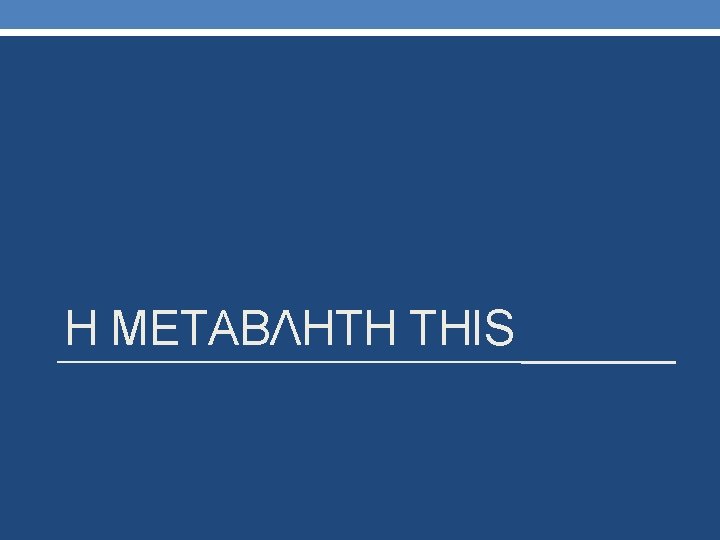
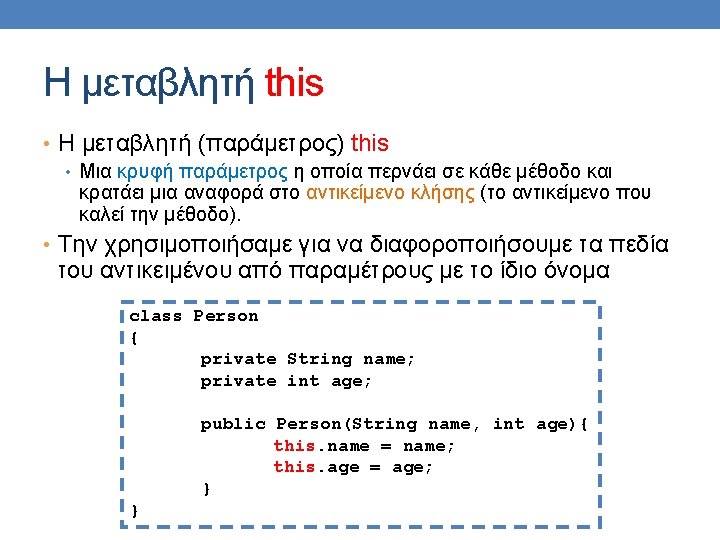
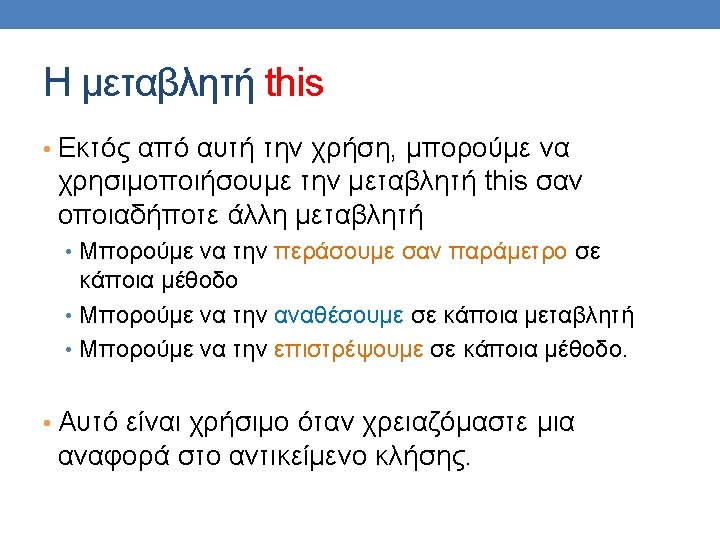
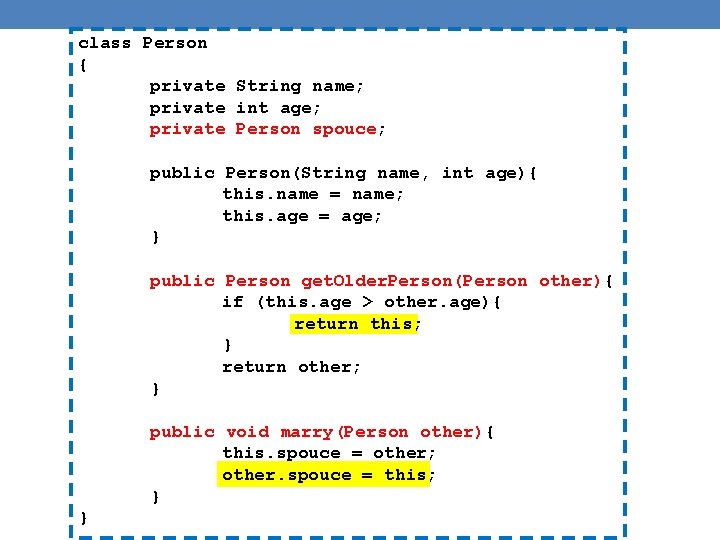
class Person { private String name; private int age; private Person spouce; public Person(String name, int age){ this. name = name; this. age = age; } public Person get. Older. Person(Person other){ if (this. age > other. age){ return this; } return other; } public void marry(Person other){ this. spouce = other; other. spouce = this; } }
![class Test public static void mainString args Person alice new PersonAlice 30 class Test { public static void main(String[] args){ Person alice = new Person("Alice", 30);](https://slidetodoc.com/presentation_image_h2/54025790b7c56deab685e5849b85eea8/image-6.jpg)
class Test { public static void main(String[] args){ Person alice = new Person("Alice", 30); Person bob = new Person("Bob", 35); Person older = bob. get. Older. Person(alice); bob. marry(alice); }
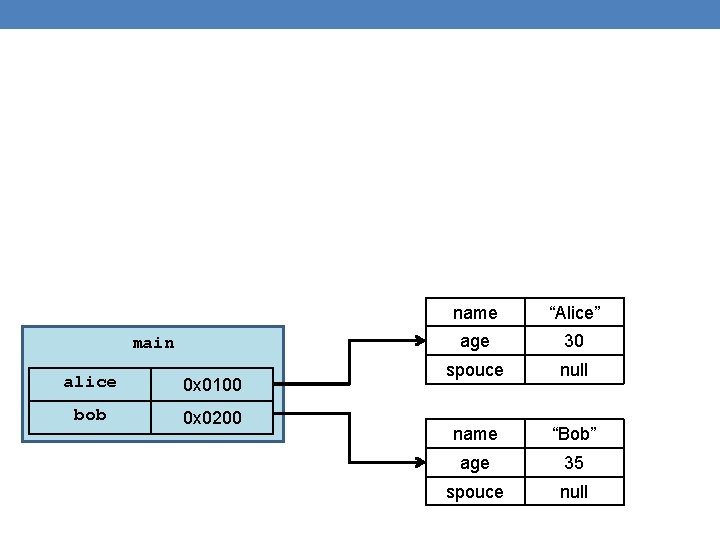
main alice 0 x 0100 bob 0 x 0200 name “Alice” age 30 spouce null name “Bob” age 35 spouce null
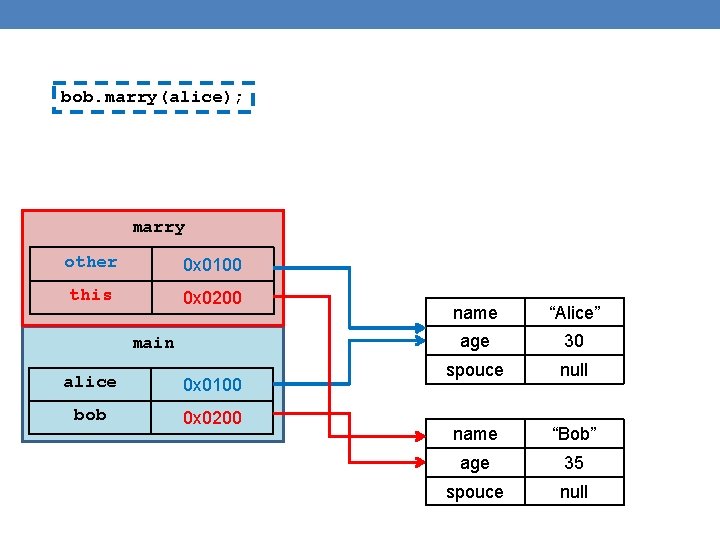
bob. marry(alice); marry other 0 x 0100 this 0 x 0200 main alice 0 x 0100 bob 0 x 0200 name “Alice” age 30 spouce null name “Bob” age 35 spouce null
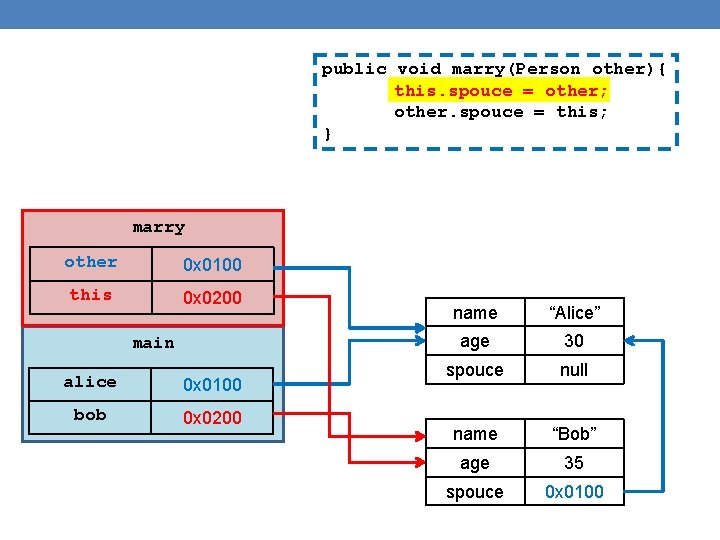
public void marry(Person other){ this. spouce = other; other. spouce = this; } marry other 0 x 0100 this 0 x 0200 main alice 0 x 0100 bob 0 x 0200 name “Alice” age 30 spouce null name “Bob” age 35 spouce 0 x 0100
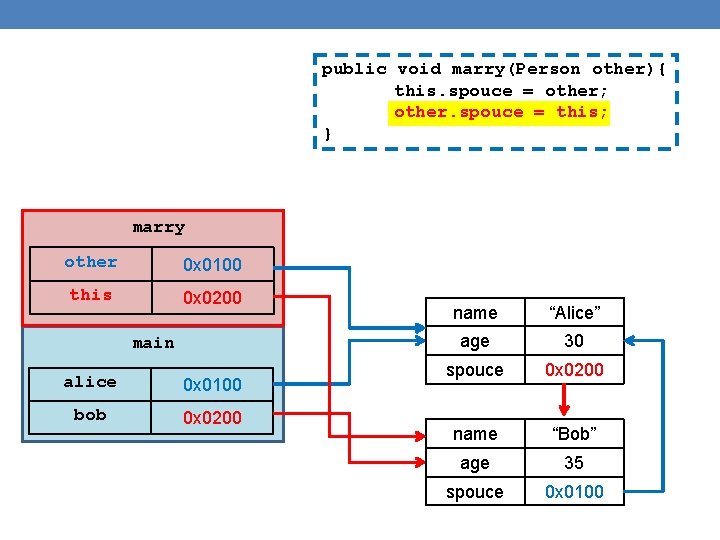
public void marry(Person other){ this. spouce = other; other. spouce = this; } marry other 0 x 0100 this 0 x 0200 main alice 0 x 0100 bob 0 x 0200 name “Alice” age 30 spouce 0 x 0200 name “Bob” age 35 spouce 0 x 0100
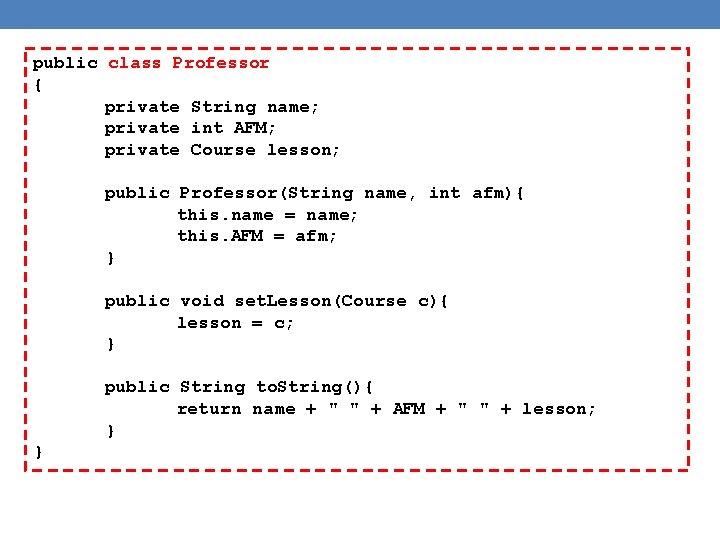
public class Professor { private String name; private int AFM; private Course lesson; public Professor(String name, int afm){ this. name = name; this. AFM = afm; } public void set. Lesson(Course c){ lesson = c; } public String to. String(){ return name + " " + AFM + " " + lesson; } }
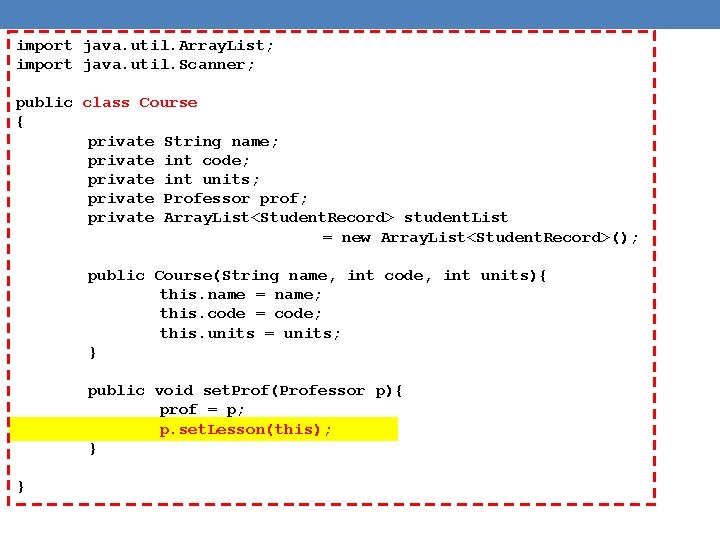
import java. util. Array. List; import java. util. Scanner; public class Course { private String name; private int code; private int units; private Professor prof; private Array. List<Student. Record> student. List = new Array. List<Student. Record>(); public Course(String name, int code, int units){ this. name = name; this. code = code; this. units = units; } public void set. Prof(Professor p){ prof = p; p. set. Lesson(this); } }
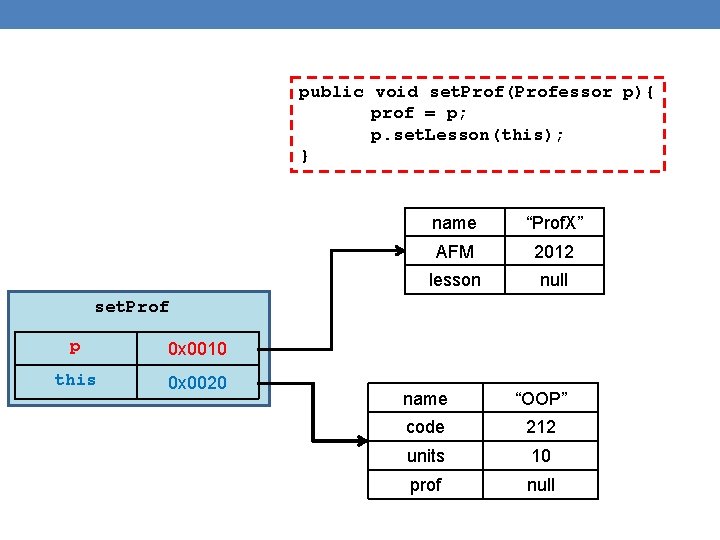
public void set. Prof(Professor p){ prof = p; p. set. Lesson(this); } name “Prof. X” AFM 2012 lesson null set. Prof p 0 x 0010 this 0 x 0020 name “OOP” code 212 units 10 prof null
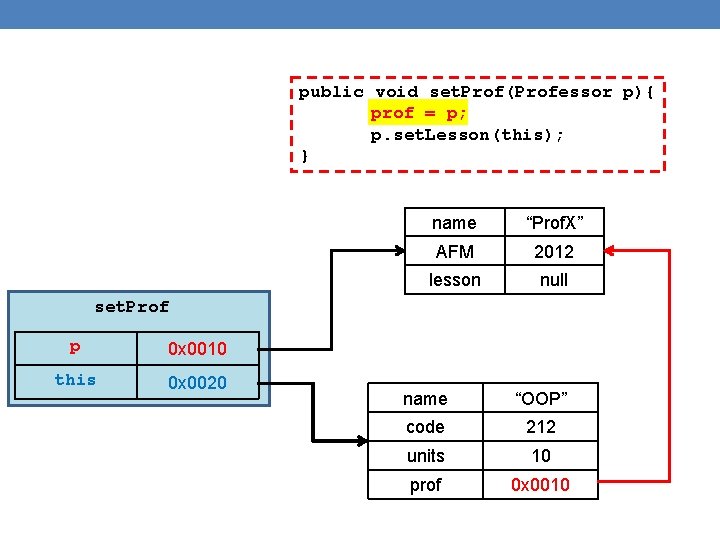
public void set. Prof(Professor p){ prof = p; p. set. Lesson(this); } name “Prof. X” AFM 2012 lesson null set. Prof p 0 x 0010 this 0 x 0020 name “OOP” code 212 units 10 prof 0 x 0010
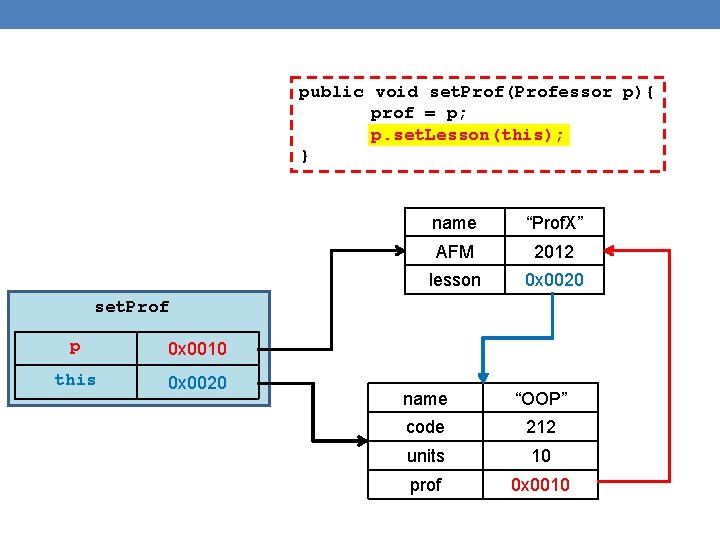
public void set. Prof(Professor p){ prof = p; p. set. Lesson(this); } name “Prof. X” AFM 2012 lesson 0 x 0020 set. Prof p 0 x 0010 this 0 x 0020 name “OOP” code 212 units 10 prof 0 x 0010
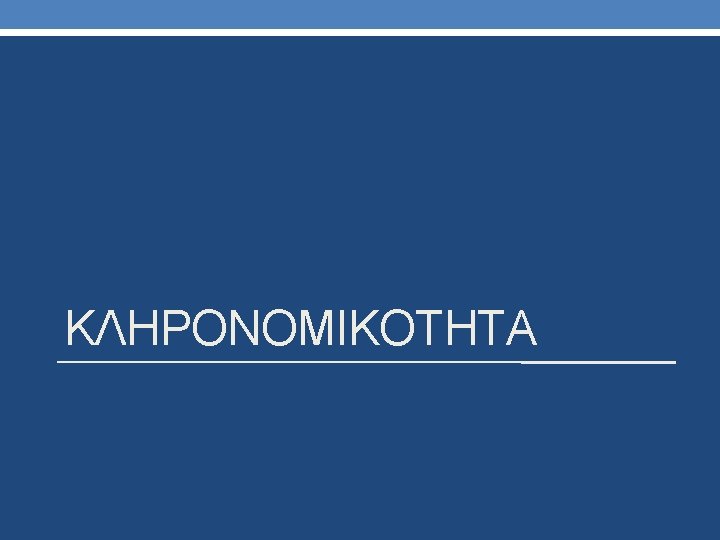
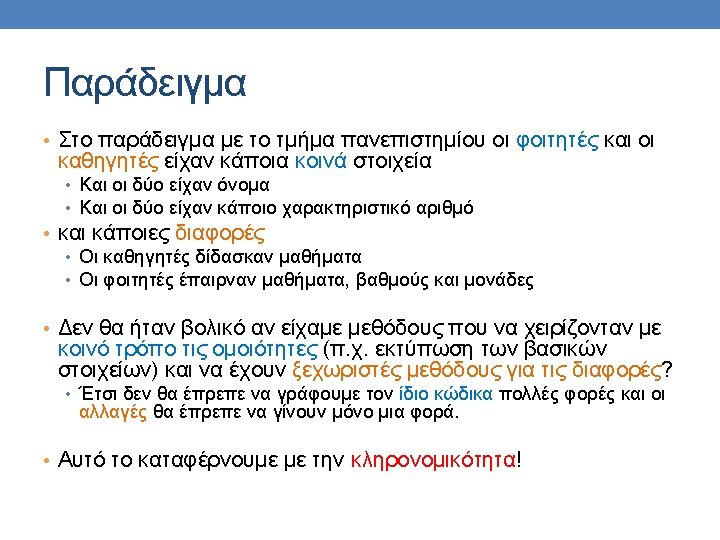
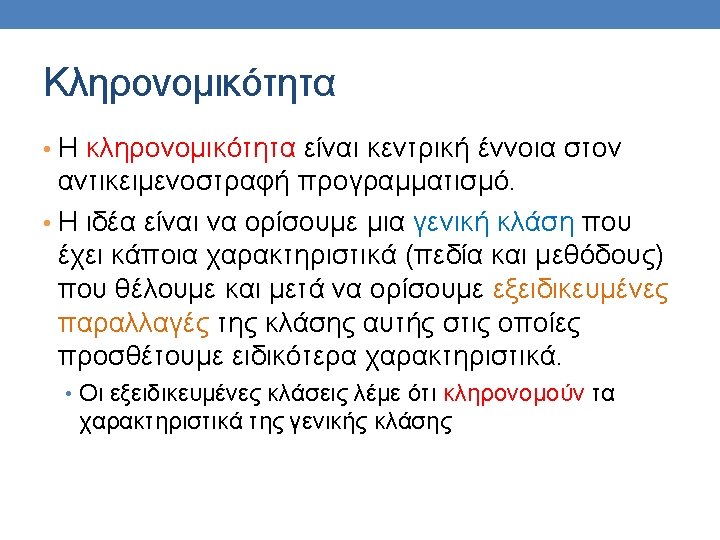
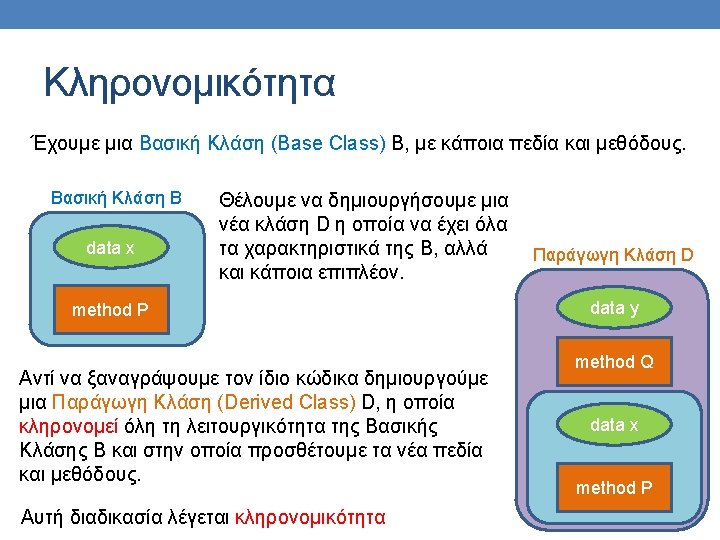
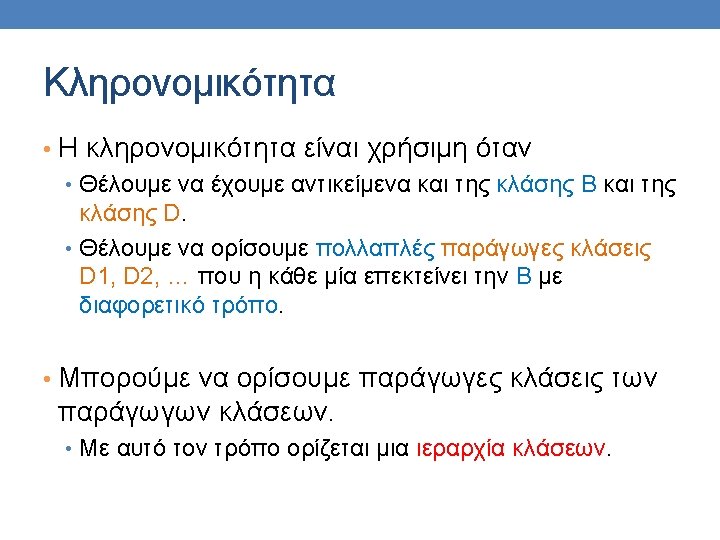
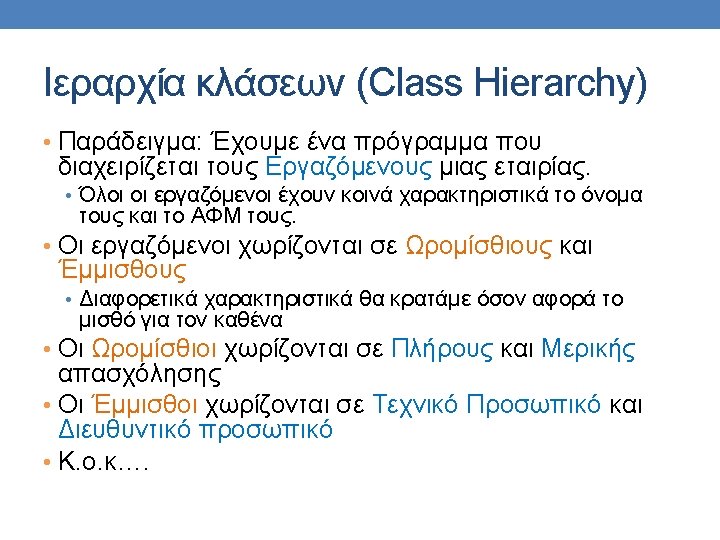
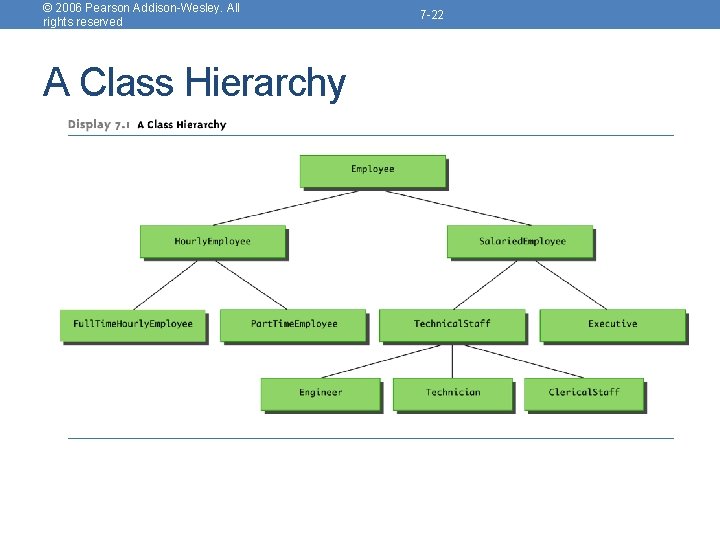
© 2006 Pearson Addison-Wesley. All rights reserved A Class Hierarchy 7 -22
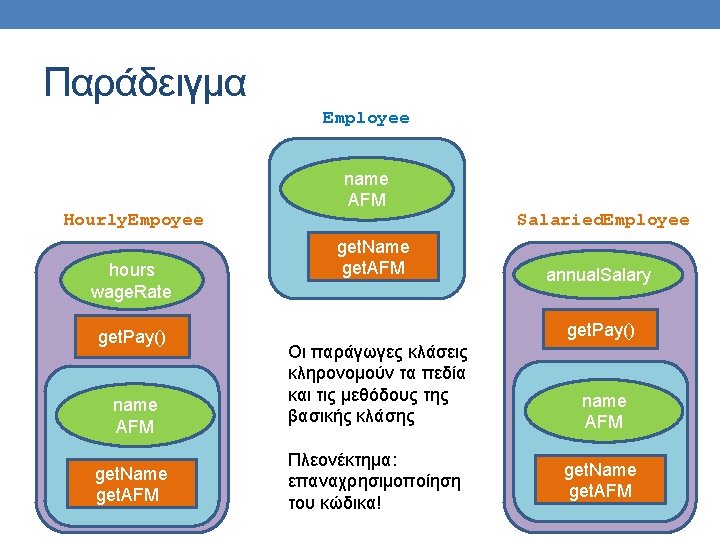
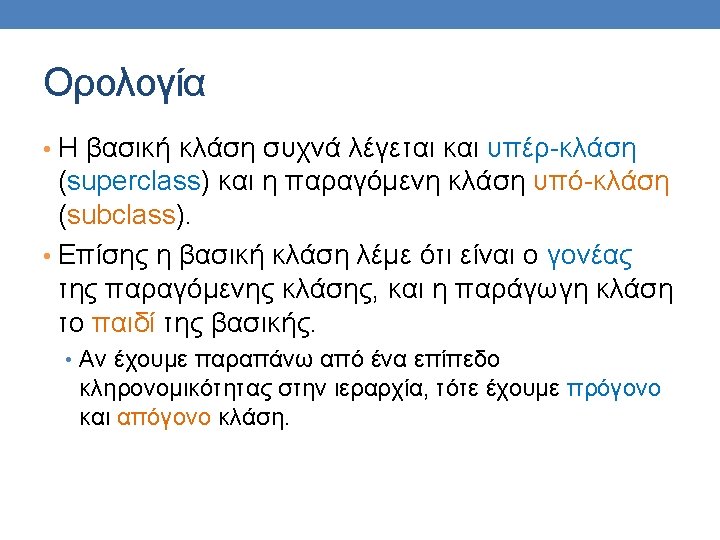
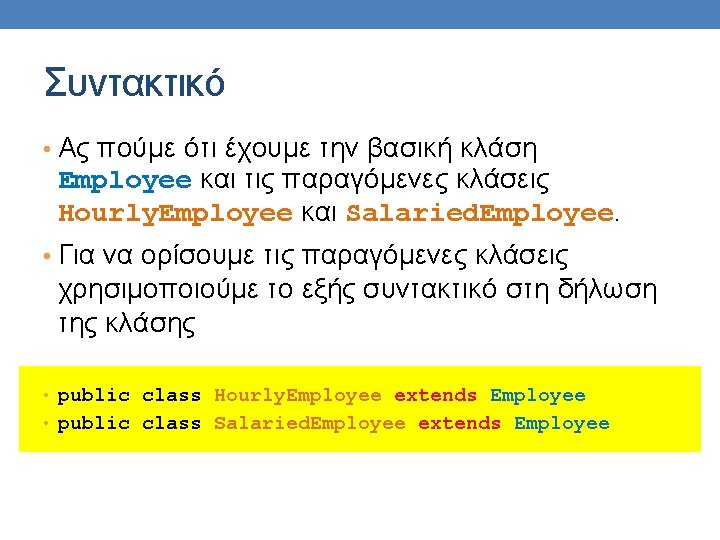
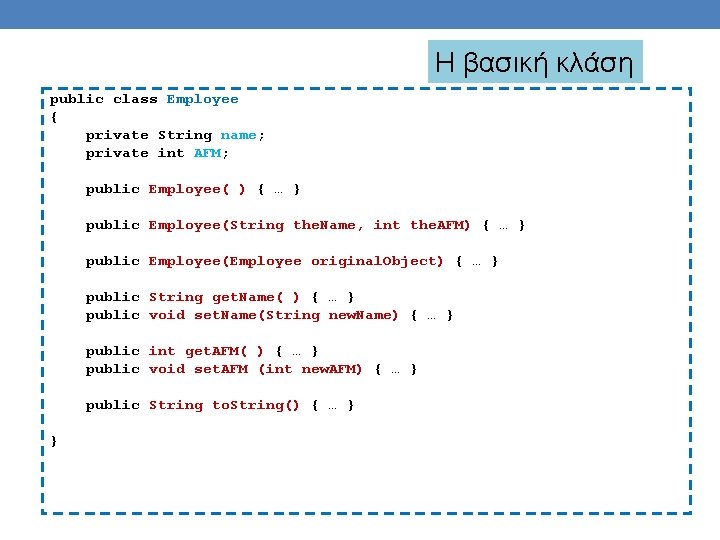
Η βασική κλάση public class Employee { private String name; private int AFM; public Employee( ) { … } public Employee(String the. Name, int the. AFM) { … } public Employee(Employee original. Object) { … } public String get. Name( ) { … } public void set. Name(String new. Name) { … } public int get. AFM( ) { … } public void set. AFM (int new. AFM) { … } public String to. String() { … } }
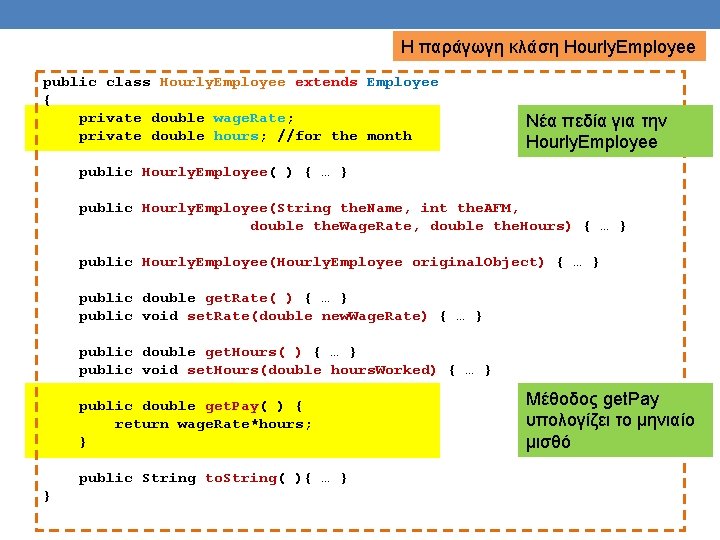
Η παράγωγη κλάση Hourly. Employee public class Hourly. Employee extends Employee { private double wage. Rate; private double hours; //for the month Νέα πεδία για την Hourly. Employee public Hourly. Employee( ) { … } public Hourly. Employee(String the. Name, int the. AFM, double the. Wage. Rate, double the. Hours) { … } public Hourly. Employee(Hourly. Employee original. Object) { … } public double get. Rate( ) { … } public void set. Rate(double new. Wage. Rate) { … } public double get. Hours( ) { … } public void set. Hours(double hours. Worked) { … } public double get. Pay( ) { return wage. Rate*hours; } public String to. String( ){ … } } Μέθοδος get. Pay υπολογίζει το μηνιαίο μισθό
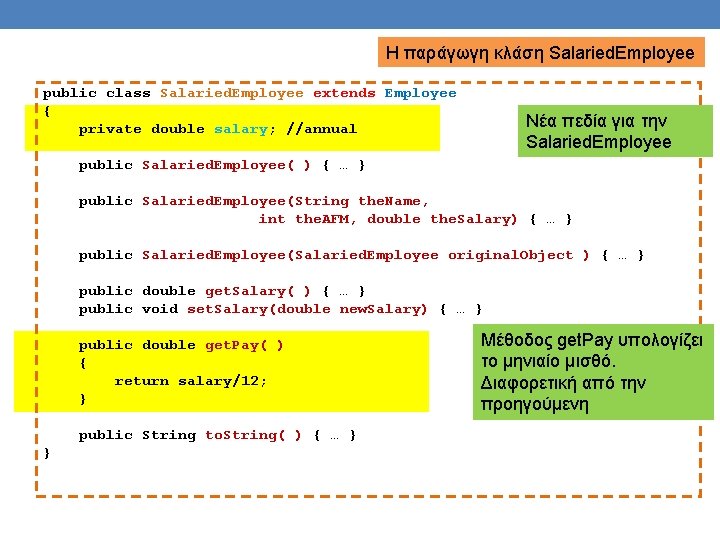
Η παράγωγη κλάση Salaried. Employee public class Salaried. Employee extends Employee { private double salary; //annual Νέα πεδία για την Salaried. Employee public Salaried. Employee( ) { … } public Salaried. Employee(String the. Name, int the. AFM, double the. Salary) { … } public Salaried. Employee(Salaried. Employee original. Object ) { … } public double get. Salary( ) { … } public void set. Salary(double new. Salary) { … } public double get. Pay( ) { return salary/12; } public String to. String( ) { … } } Μέθοδος get. Pay υπολογίζει το μηνιαίο μισθό. Διαφορετική από την προηγούμενη
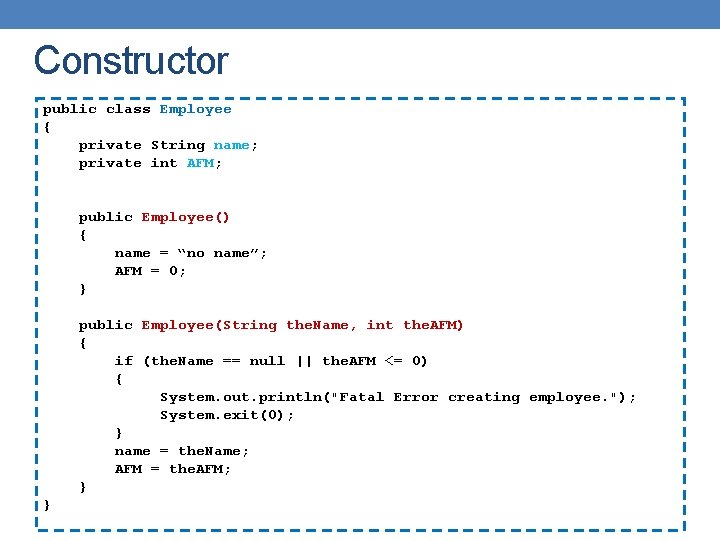
Constructor public class Employee { private String name; private int AFM; public Employee() { name = “no name”; AFM = 0; } public Employee(String the. Name, int the. AFM) { if (the. Name == null || the. AFM <= 0) { System. out. println("Fatal Error creating employee. "); System. exit(0); } name = the. Name; AFM = the. AFM; } }
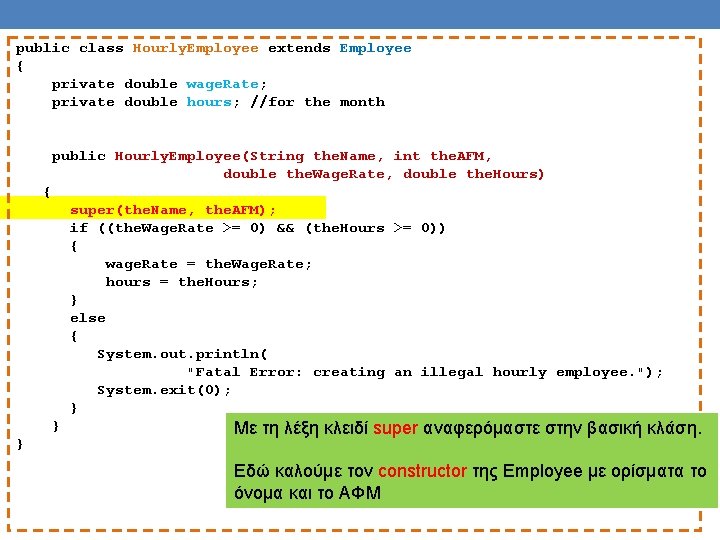
public class Hourly. Employee extends Employee { private double wage. Rate; private double hours; //for the month public Hourly. Employee(String the. Name, int the. AFM, double the. Wage. Rate, double the. Hours) { super(the. Name, the. AFM); if ((the. Wage. Rate >= 0) && (the. Hours >= 0)) { wage. Rate = the. Wage. Rate; hours = the. Hours; } else { System. out. println( "Fatal Error: creating an illegal hourly employee. "); System. exit(0); } } } Με τη λέξη κλειδί super αναφερόμαστε στην βασική κλάση. Εδώ καλούμε τον constructor της Employee με ορίσματα το όνομα και το ΑΦΜ
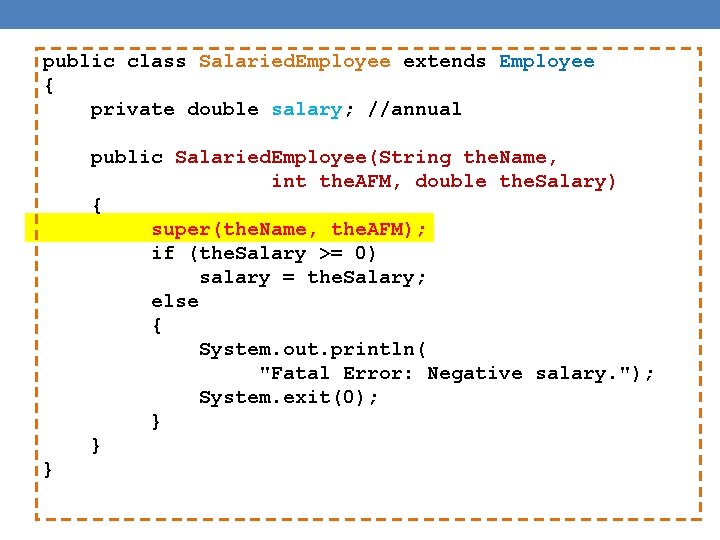
public class Salaried. Employee extends Employee { private double salary; //annual public Salaried. Employee(String the. Name, int the. AFM, double the. Salary) { super(the. Name, the. AFM); if (the. Salary >= 0) salary = the. Salary; else { System. out. println( "Fatal Error: Negative salary. "); System. exit(0); } } }
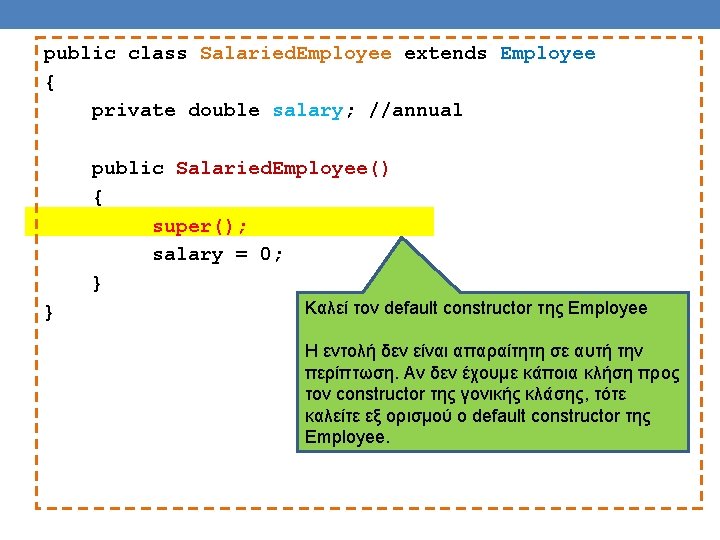
public class Salaried. Employee extends Employee { private double salary; //annual public Salaried. Employee() { super(); salary = 0; } } Καλεί τον default constructor της Employee Η εντολή δεν είναι απαραίτητη σε αυτή την περίπτωση. Αν δεν έχουμε κάποια κλήση προς τον constructor της γονικής κλάσης, τότε καλείτε εξ ορισμού ο default constructor της Employee.
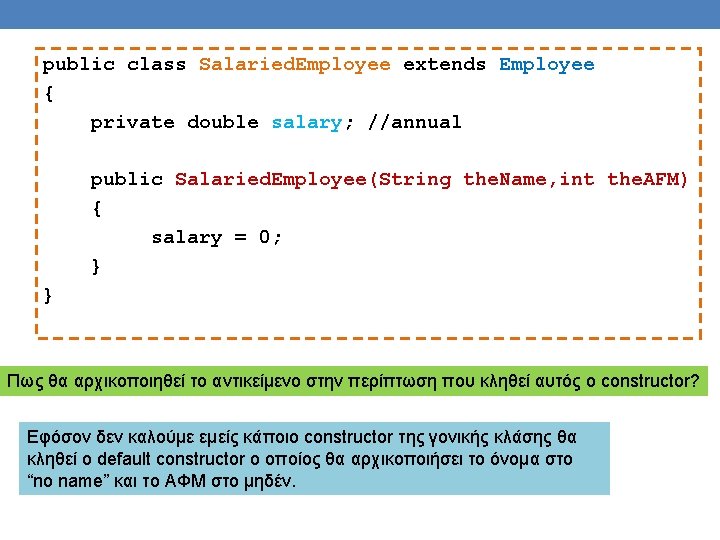
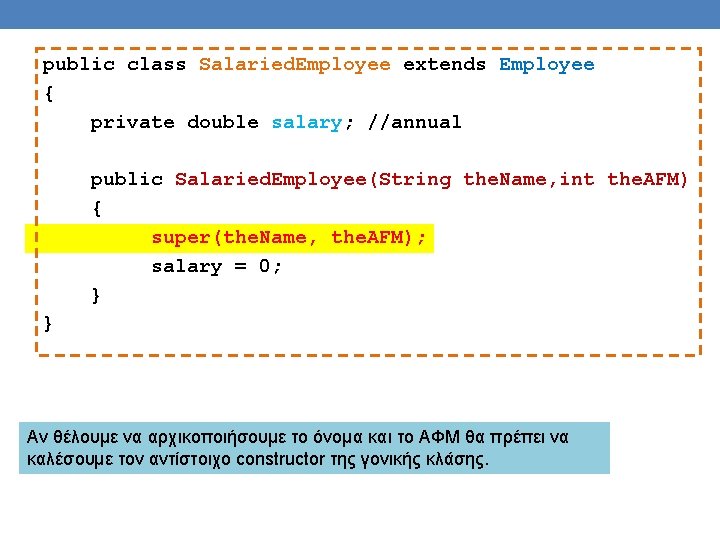
public class Salaried. Employee extends Employee { private double salary; //annual public Salaried. Employee(String the. Name, int the. AFM) { super(the. Name, the. AFM); salary = 0; } } Αν θέλουμε να αρχικοποιήσουμε το όνομα και το ΑΦΜ θα πρέπει να καλέσουμε τον αντίστοιχο constructor της γονικής κλάσης.
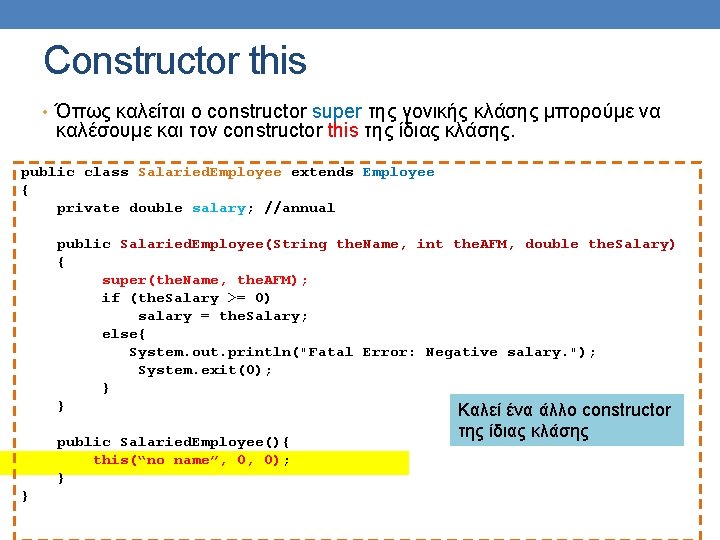
Constructor this • Όπως καλείται ο constructor super της γονικής κλάσης μπορούμε να καλέσουμε και τον constructor this της ίδιας κλάσης. public class Salaried. Employee extends Employee { private double salary; //annual public Salaried. Employee(String the. Name, int the. AFM, double the. Salary) { super(the. Name, the. AFM); if (the. Salary >= 0) salary = the. Salary; else{ System. out. println("Fatal Error: Negative salary. "); System. exit(0); } } Καλεί ένα άλλο constructor public Salaried. Employee(){ this(“no name”, 0, 0); } } της ίδιας κλάσης
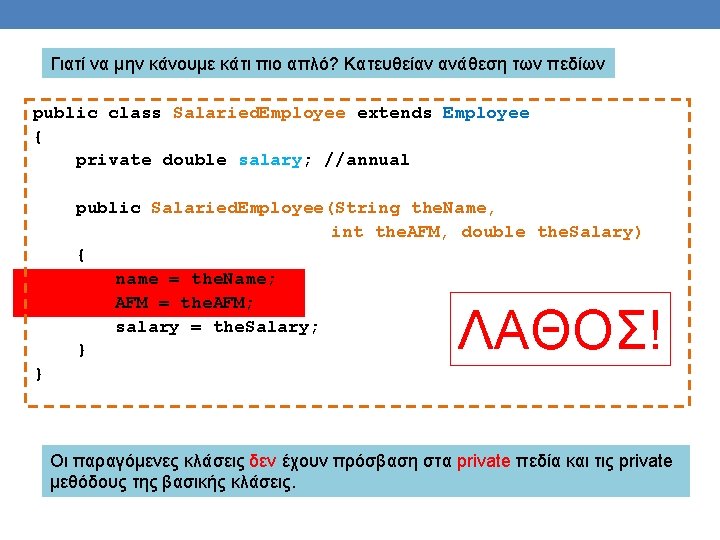
Γιατί να μην κάνουμε κάτι πιο απλό? Κατευθείαν ανάθεση των πεδίων public class Salaried. Employee extends Employee { private double salary; //annual public Salaried. Employee(String the. Name, int the. AFM, double the. Salary) { name = the. Name; AFM = the. AFM; salary = the. Salary; } ΛΑΘΟΣ! } Οι παραγόμενες κλάσεις δεν έχουν πρόσβαση στα private πεδία και τις private μεθόδους της βασικής κλάσεις.
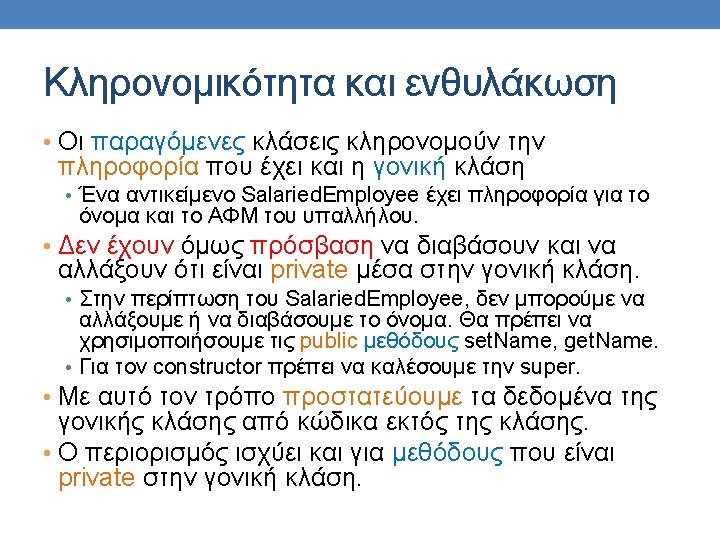
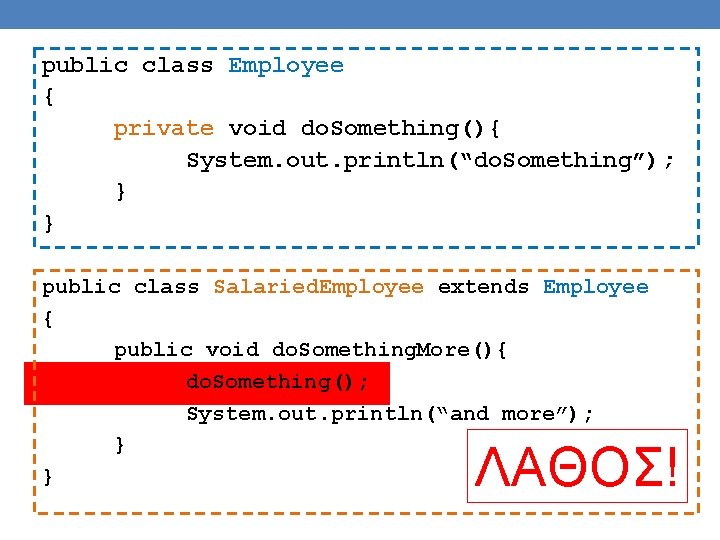
public class Employee { private void do. Something(){ System. out. println(“do. Something”); } } public class Salaried. Employee extends Employee { public void do. Something. More(){ do. Something(); System. out. println(“and more”); } } ΛΑΘΟΣ!
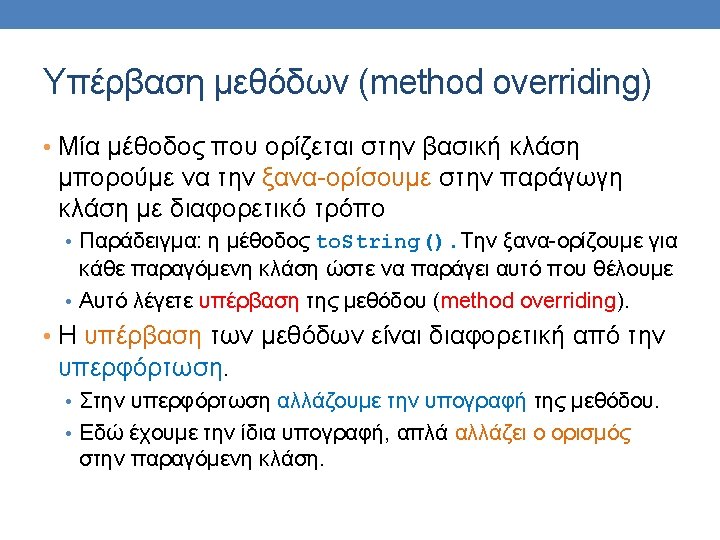
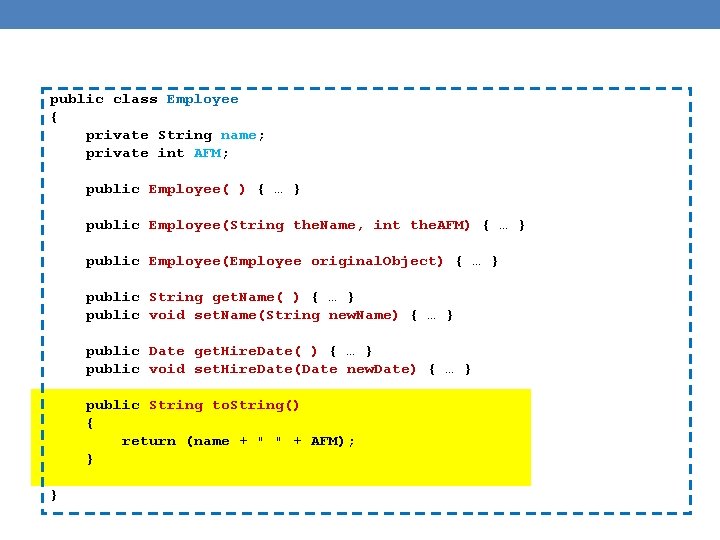
public class Employee { private String name; private int AFM; public Employee( ) { … } public Employee(String the. Name, int the. AFM) { … } public Employee(Employee original. Object) { … } public String get. Name( ) { … } public void set. Name(String new. Name) { … } public Date get. Hire. Date( ) { … } public void set. Hire. Date(Date new. Date) { … } public String to. String() { return (name + " " + AFM); } }
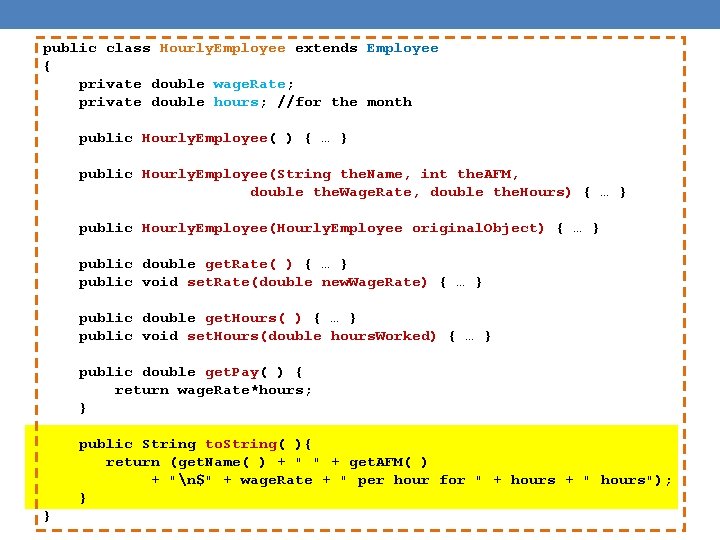
public class Hourly. Employee extends Employee { private double wage. Rate; private double hours; //for the month public Hourly. Employee( ) { … } public Hourly. Employee(String the. Name, int the. AFM, double the. Wage. Rate, double the. Hours) { … } public Hourly. Employee(Hourly. Employee original. Object) { … } public double get. Rate( ) { … } public void set. Rate(double new. Wage. Rate) { … } public double get. Hours( ) { … } public void set. Hours(double hours. Worked) { … } public double get. Pay( ) { return wage. Rate*hours; } public String to. String( ){ return (get. Name( ) + " " + get. AFM( ) + "n$" + wage. Rate + " per hour for " + hours + " hours"); } }
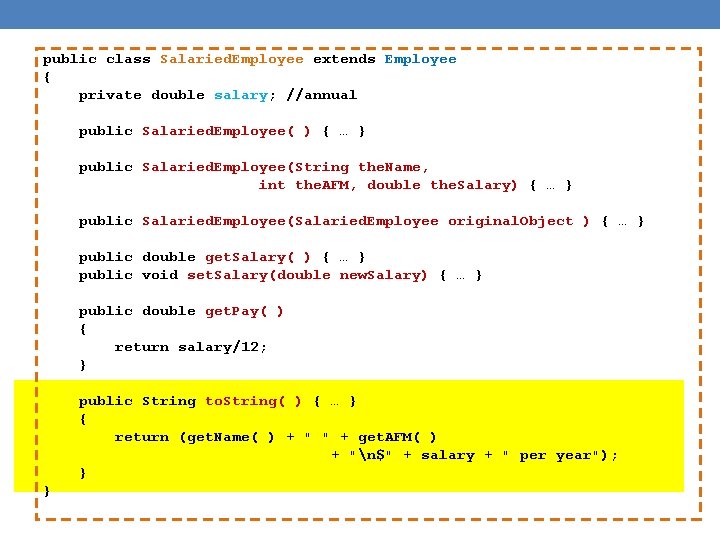
public class Salaried. Employee extends Employee { private double salary; //annual public Salaried. Employee( ) { … } public Salaried. Employee(String the. Name, int the. AFM, double the. Salary) { … } public Salaried. Employee(Salaried. Employee original. Object ) { … } public double get. Salary( ) { … } public void set. Salary(double new. Salary) { … } public double get. Pay( ) { return salary/12; } public String to. String( ) { … } { return (get. Name( ) + " " + get. AFM( ) + "n$" + salary + " per year"); } }
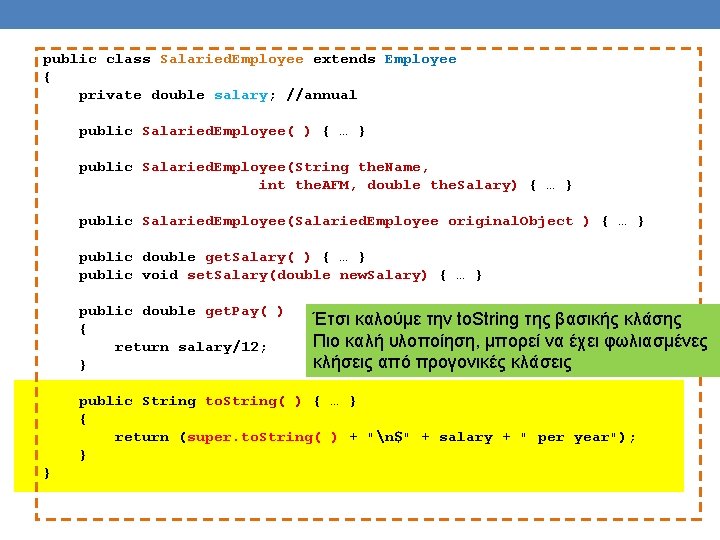
public class Salaried. Employee extends Employee { private double salary; //annual public Salaried. Employee( ) { … } public Salaried. Employee(String the. Name, int the. AFM, double the. Salary) { … } public Salaried. Employee(Salaried. Employee original. Object ) { … } public double get. Salary( ) { … } public void set. Salary(double new. Salary) { … } public double get. Pay( ) { return salary/12; } Έτσι καλούμε την to. String της βασικής κλάσης Πιο καλή υλοποίηση, μπορεί να έχει φωλιασμένες κλήσεις από προγονικές κλάσεις public String to. String( ) { … } { return (super. to. String( ) + "n$" + salary + " per year"); } }
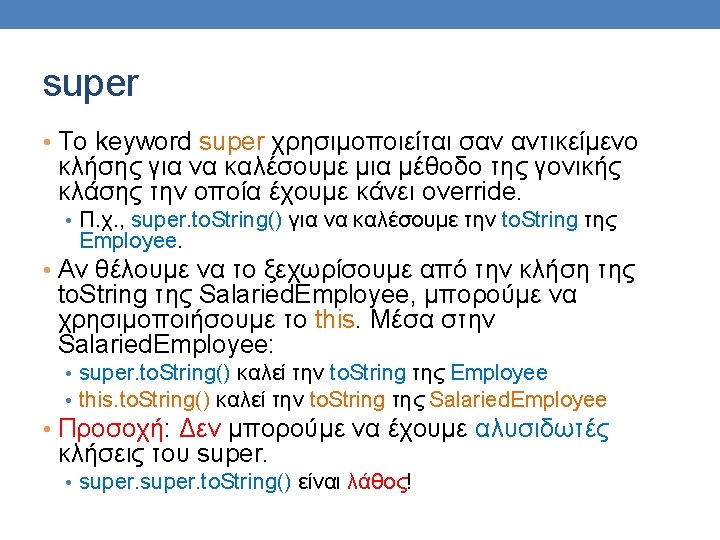
![Παράδειγμα χρήσης public class Inheritance Demo public static void mainString args Hourly Παράδειγμα χρήσης public class Inheritance. Demo { public static void main(String[] args) { Hourly.](https://slidetodoc.com/presentation_image_h2/54025790b7c56deab685e5849b85eea8/image-45.jpg)
Παράδειγμα χρήσης public class Inheritance. Demo { public static void main(String[] args) { Hourly. Employee joe = new Hourly. Employee("Joe Worker", 100, 50. 50, 160); System. out. println("joe's longer name is " + joe. get. Name( )); System. out. println("Changing joe's name to Josephine. "); joe. set. Name("Josephine"); Καλεί τις μεθόδους της Employee System. out. println("joe's record is as follows: "); System. out. println(joe); } } Καλεί την μέθοδο to. Strong της Hourly. Employee
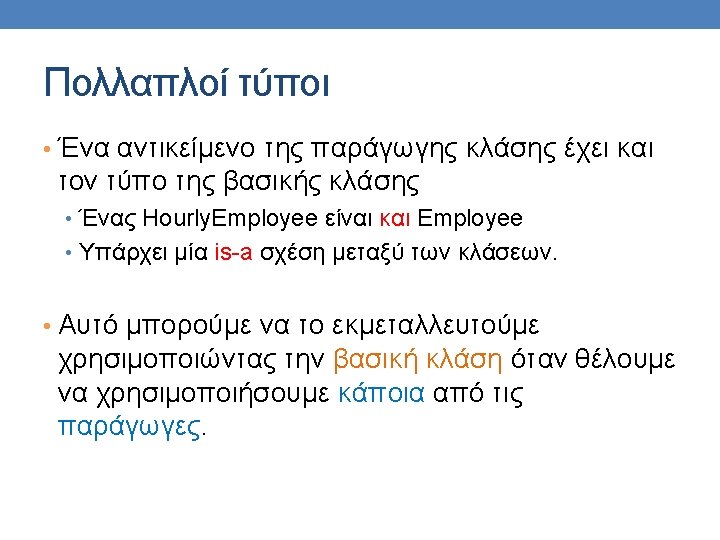
![public class Is ADemo public static void mainString args Salaried Employee joe public class Is. ADemo { public static void main(String[] args) { Salaried. Employee joe](https://slidetodoc.com/presentation_image_h2/54025790b7c56deab685e5849b85eea8/image-47.jpg)
public class Is. ADemo { public static void main(String[] args) { Salaried. Employee joe = new Salaried. Employee("Josephine", 100000); Hourly. Employee sam = new Hourly. Employee("Sam", 200, 50. 50, 40); System. out. println("joe's longer name is " + joe. get. Name( )); System. out. println("show. Employee(joe): "); show. Employee(joe); System. out. println("show. Employee(sam): "); show. Employee(sam); } Μπορούμε να καλέσουμε τη μέθοδο και με Hourly. Employee και με Salaried. Employee public static void show. Employee(Employee employee. Object) { System. out. println(employee. Object. get. Name( )); System. out. println(employee. Object. get. AFM( )); } }
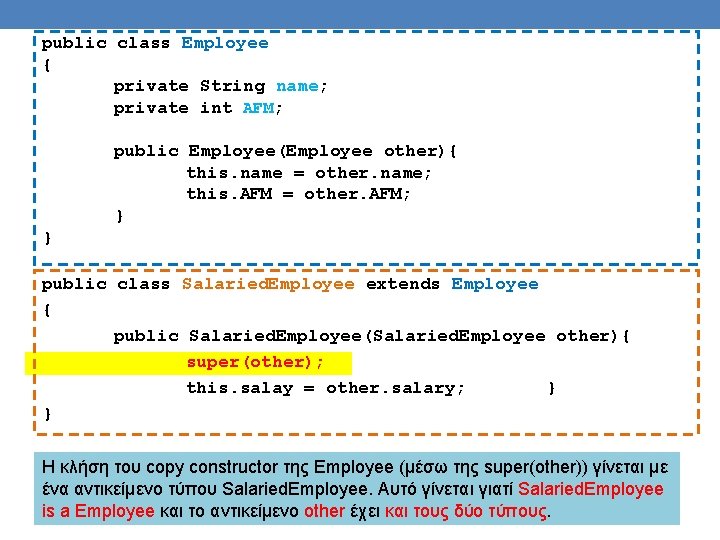
public class Employee { private String name; private int AFM; public Employee(Employee other){ this. name = other. name; this. AFM = other. AFM; } } public class Salaried. Employee extends Employee { public Salaried. Employee(Salaried. Employee other){ super(other); this. salay = other. salary; } } Η κλήση του copy constructor της Employee (μέσω της super(other)) γίνεται με ένα αντικείμενο τύπου Salaried. Employee. Αυτό γίνεται γιατί Salaried. Employee is a Employee και το αντικείμενο other έχει και τους δύο τύπους.
![public class Is ADemo public static void mainString args Salaried Employee joe public class Is. ADemo { public static void main(String[] args) { Salaried. Employee joe](https://slidetodoc.com/presentation_image_h2/54025790b7c56deab685e5849b85eea8/image-49.jpg)
public class Is. ADemo { public static void main(String[] args) { Salaried. Employee joe = new Salaried. Employee("Josephine", 100000); Hourly. Employee sam = new Hourly. Employee("Sam", 200, 50. 50, 40); System. out. println("joe's longer name is " + joe. get. Name( )); System. out. println("show. Employee(joe) invoked: "); show. Employee(joe); System. out. println("show. Employee(sam) invoked: "); show. Employee(sam); } public static void show. Employee(Employee employee. Object) { System. out. println(employee. Object); } } Θα καλέσει την to. String που αντιστοιχεί στο αντικείμενο που περάσαμε ως παράμετρο και όχι την to. String της Employee.
Private string
Class person string name int age
Int sum(int a int n) int sum=0 i
Inheritance calculator
Public class person
Private string name;
Private string java
Int max(int x int y)
Public void drawsquare(int x, int y, int len)
Public int divide(int a int b)
Const name void
Licenseid=string&content=string&/paramsxml=string
Public class employee
Public class vehicle private string name
Public class animal private string name
Public class employee private string name
Class maths student student1 class student string name
Public class person private name
Public class telephone { private string number
Public class student
Private string name
Private string[]
Iron age bronze age stone age timeline
Iron age bronze age stone age timeline
2. person singular
Thrid person narrator
Person person = new person()
Is the word that a pronoun
Interface myinterface int foo(int x)
Int main int argc char argv
Nnxn com
7팩토리얼
Arduino constant int
Int main() int num=4
Voidswap
Void
Int max int min c++
Int main int argc char argv
String value of int
Temario de java
Intmain
Const int size =18 string *tbl2
Java new string
A { private int x; public a( val) { x = val; } }
Class bucket int capacity bucket()
Private int
Private int
Name class teacher date
Name teachers name class date
Name date class