class Car private long engine Num private int
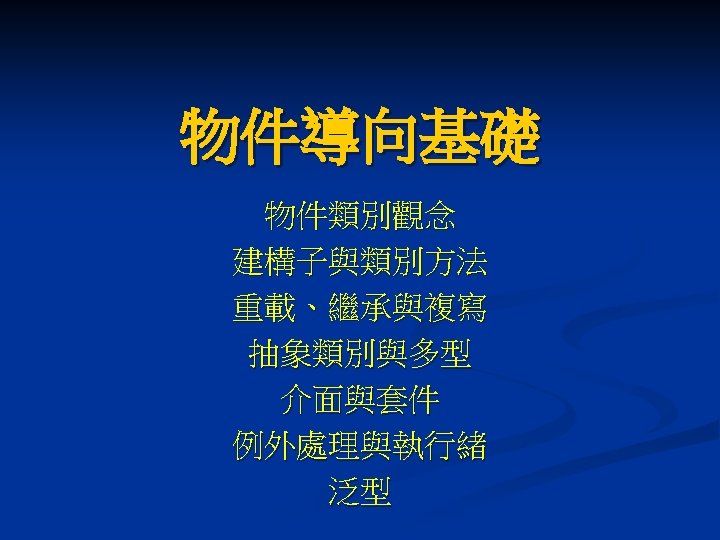
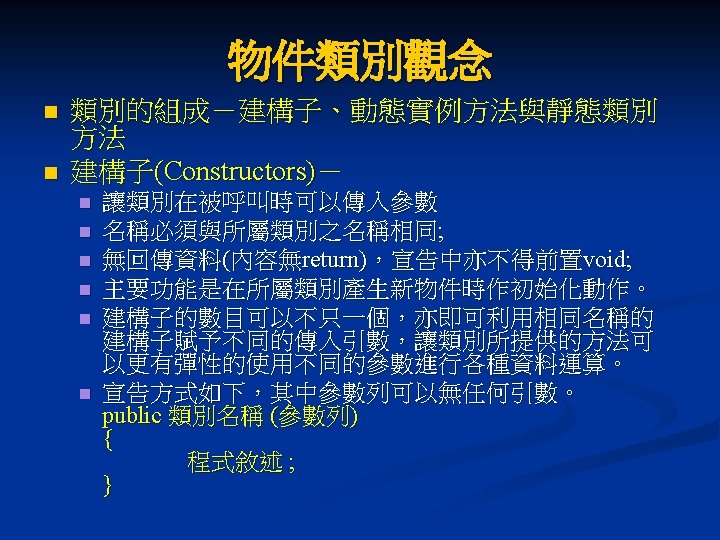
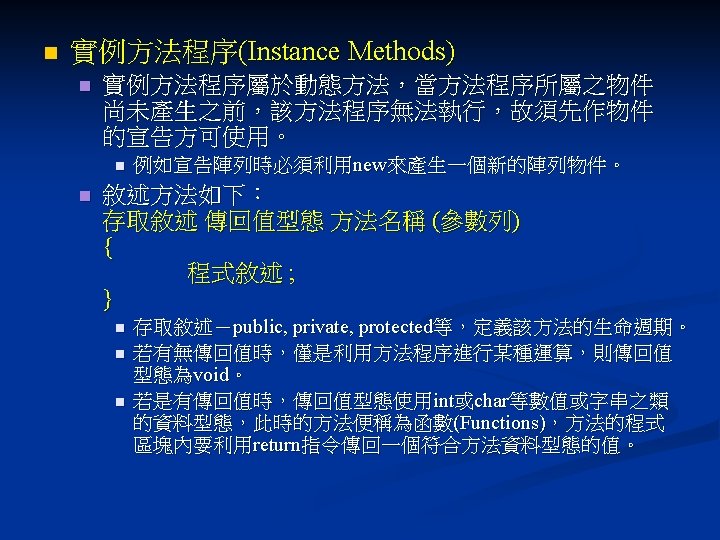
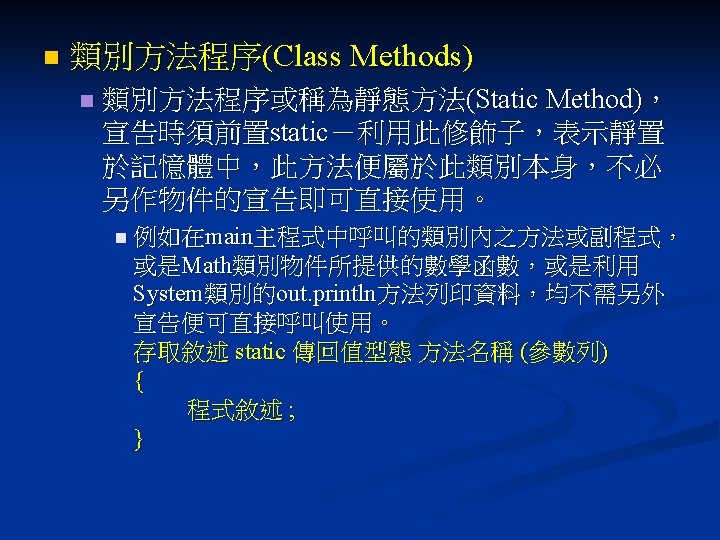
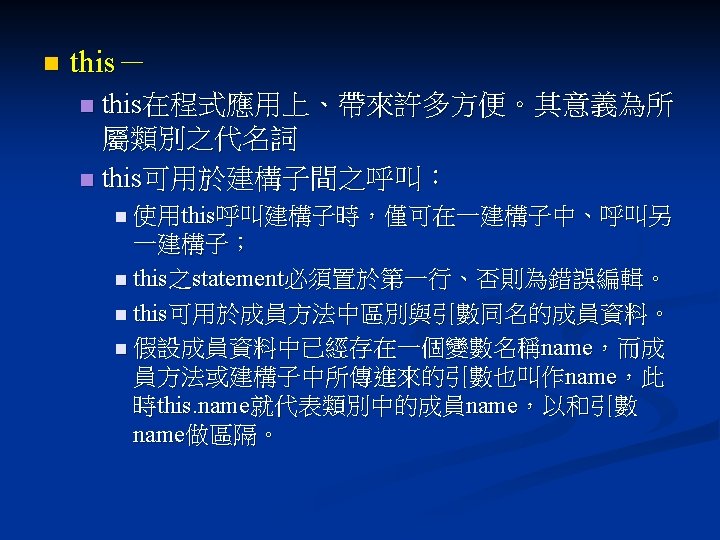
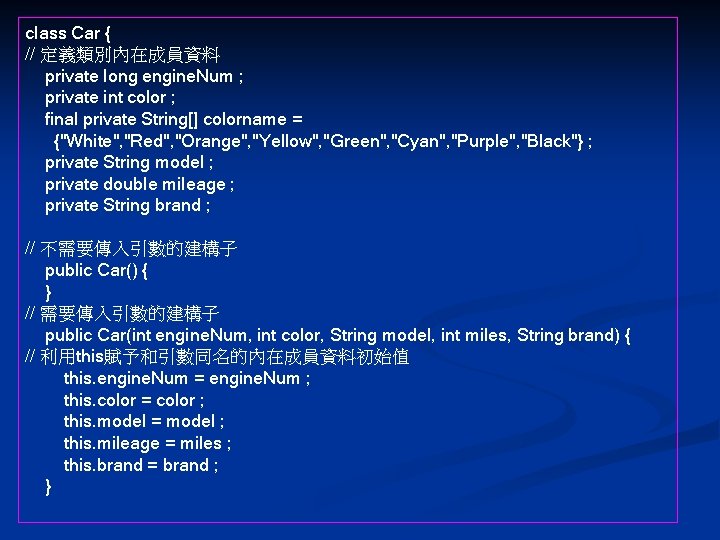
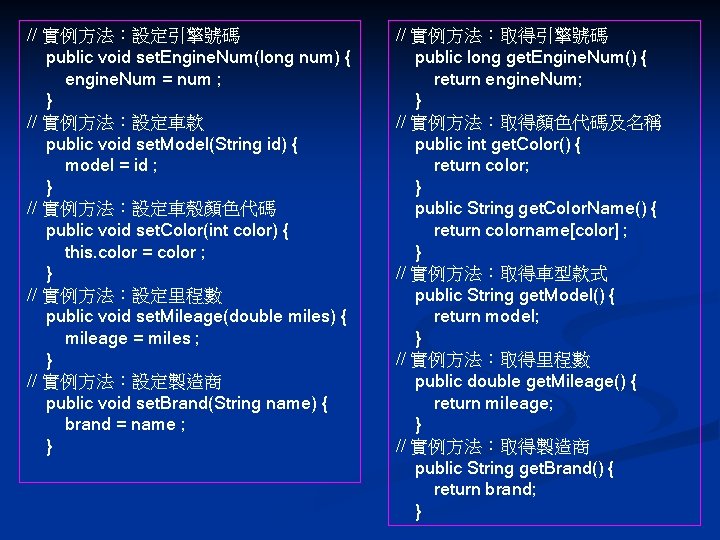
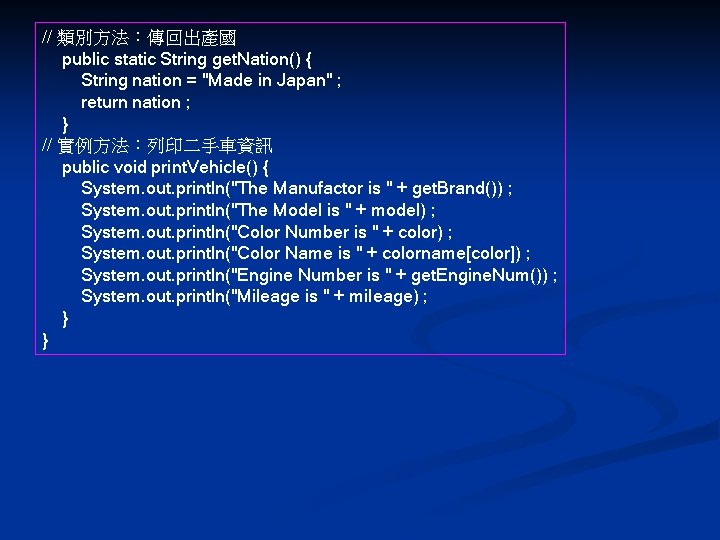
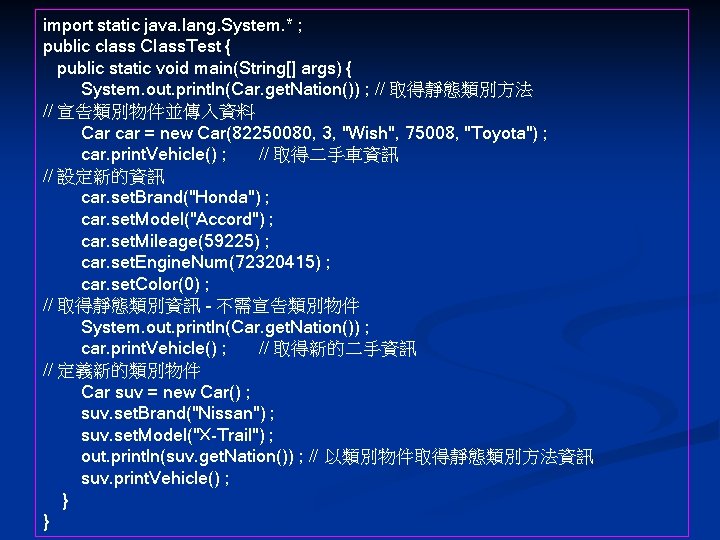
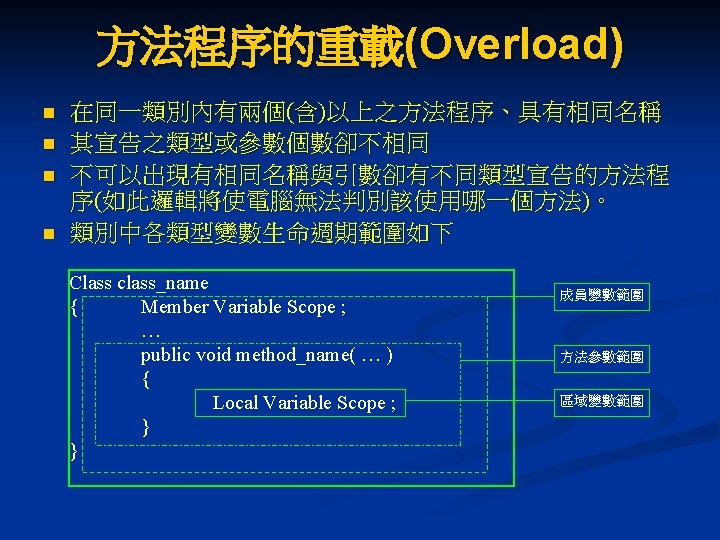
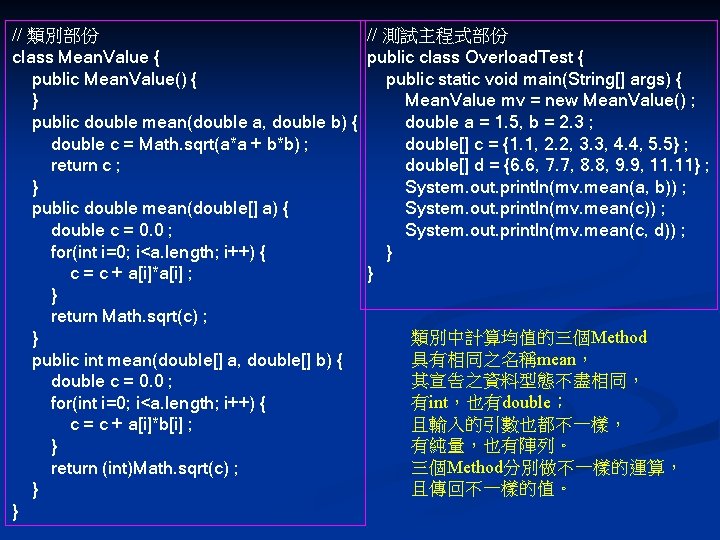
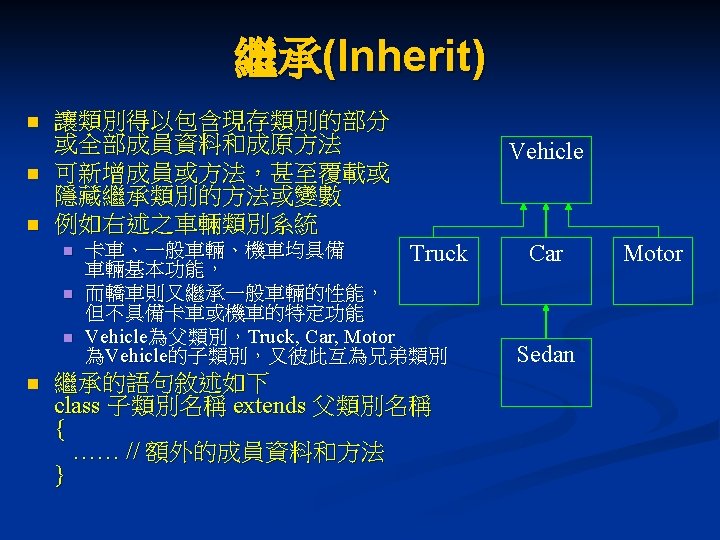
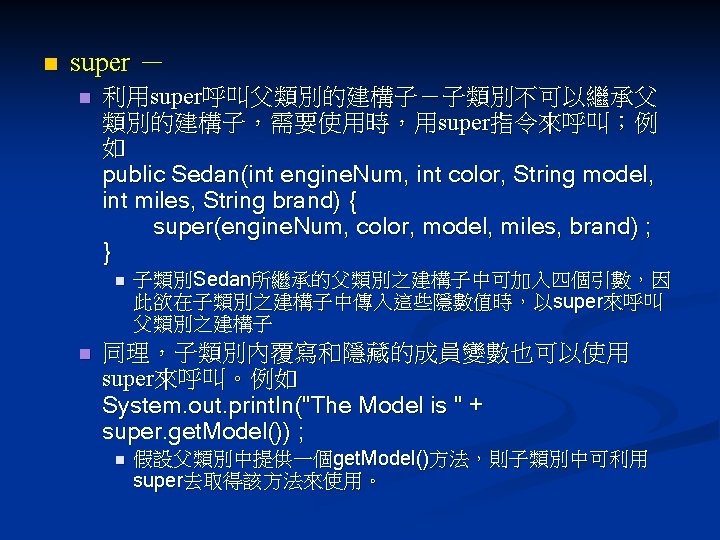
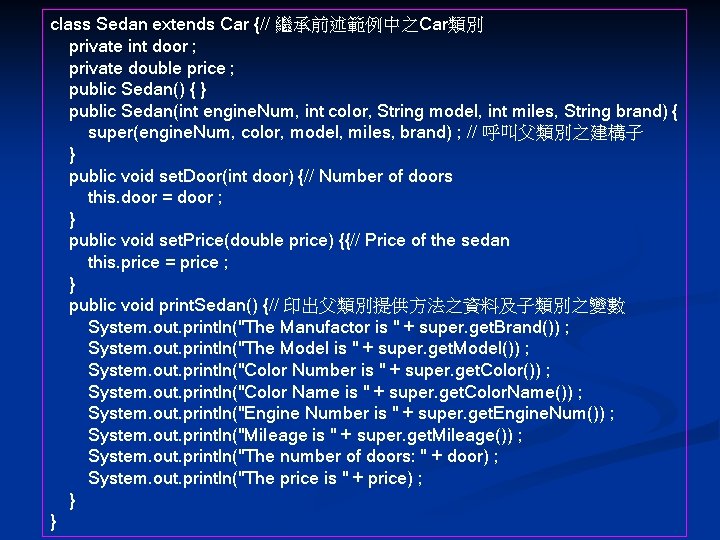
![public class Inherit. Test { public Inherit. Test() { } public static void main(String[] public class Inherit. Test { public Inherit. Test() { } public static void main(String[]](https://slidetodoc.com/presentation_image/2ea38baa6be45d6d840cd6c77bc8b5cd/image-15.jpg)
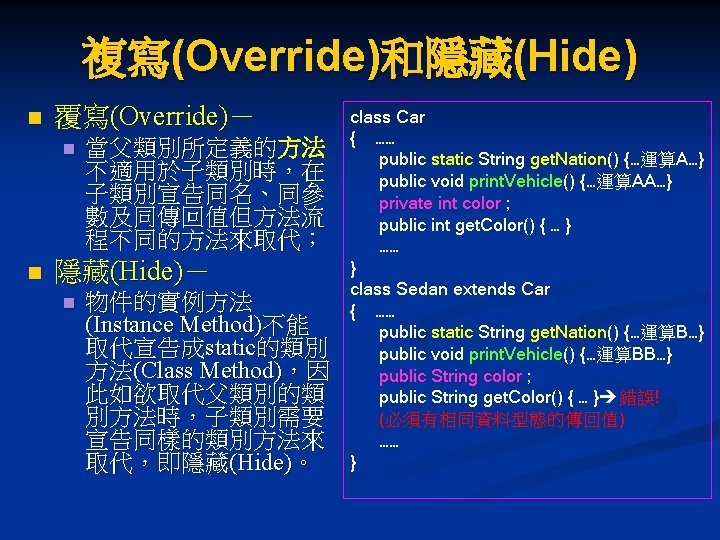
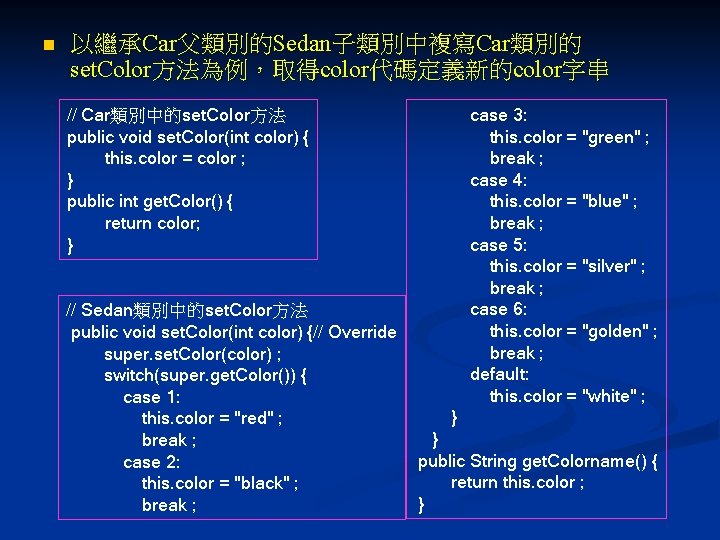
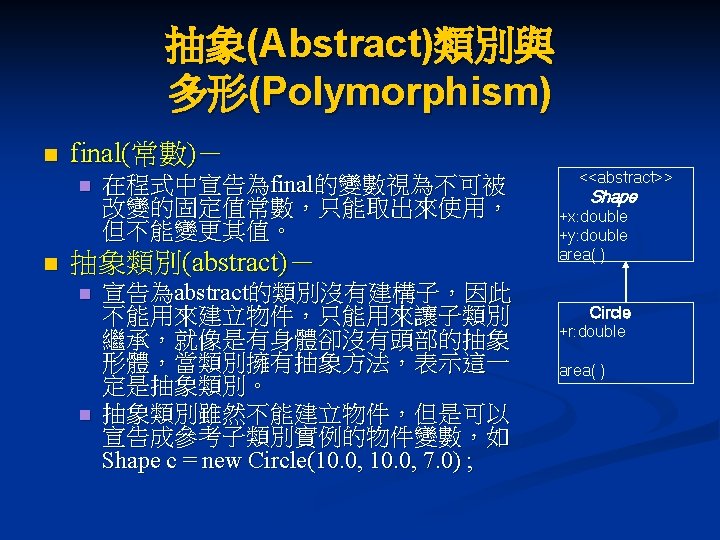
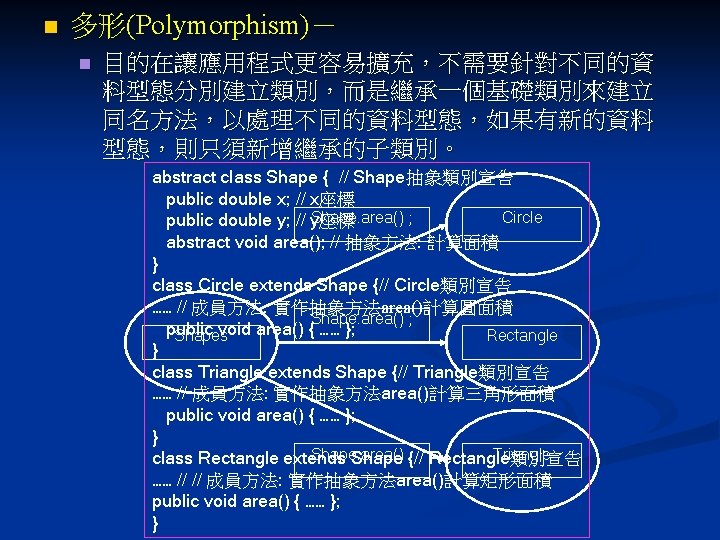
![public class Polymorphism. Test { public Polymorphism. Test() { } public static void main(String[] public class Polymorphism. Test { public Polymorphism. Test() { } public static void main(String[]](https://slidetodoc.com/presentation_image/2ea38baa6be45d6d840cd6c77bc8b5cd/image-20.jpg)
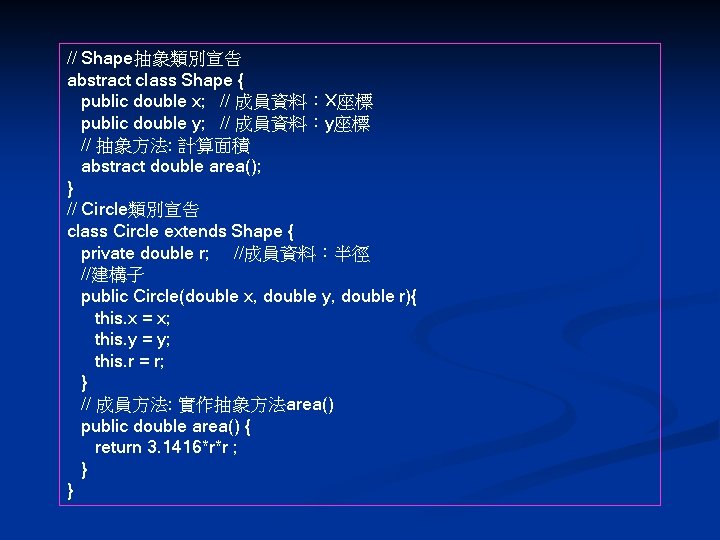
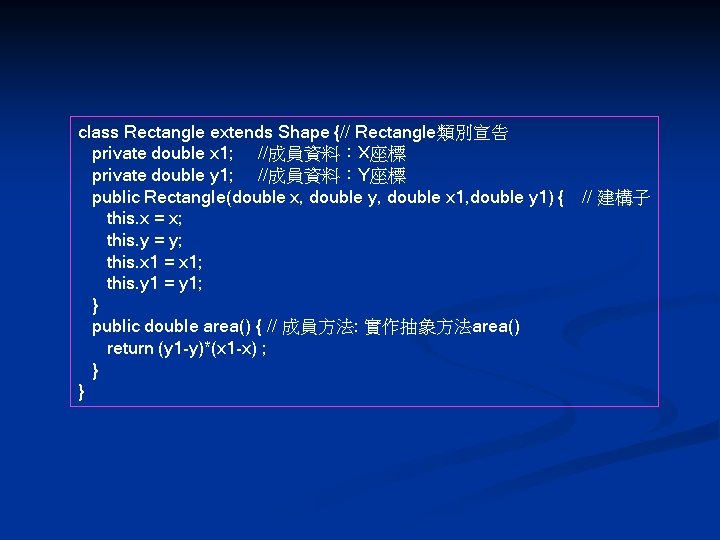
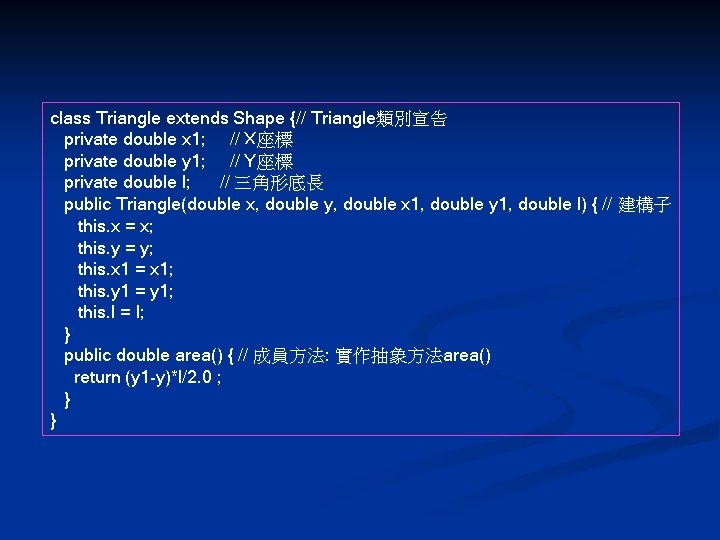
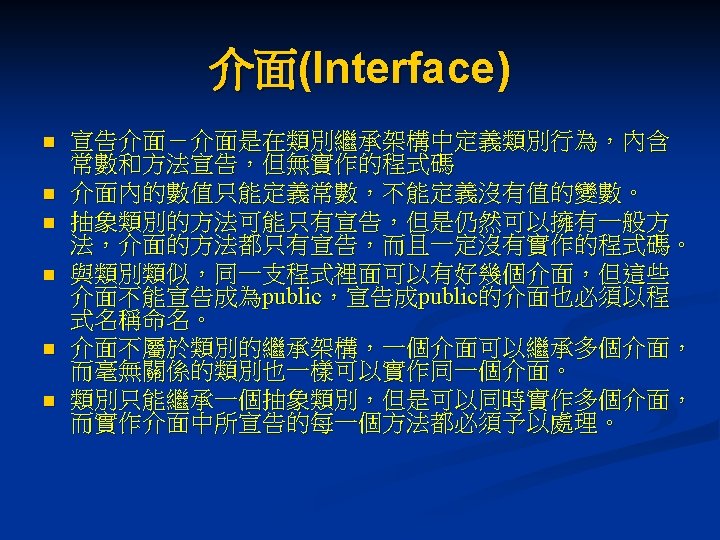
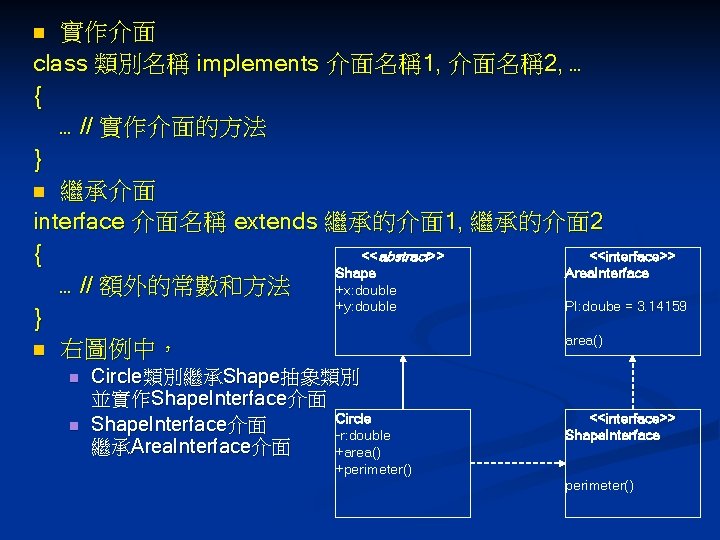
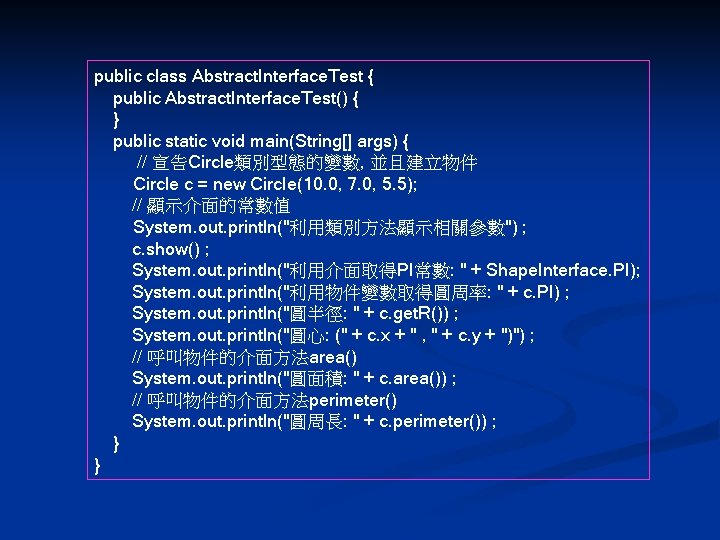
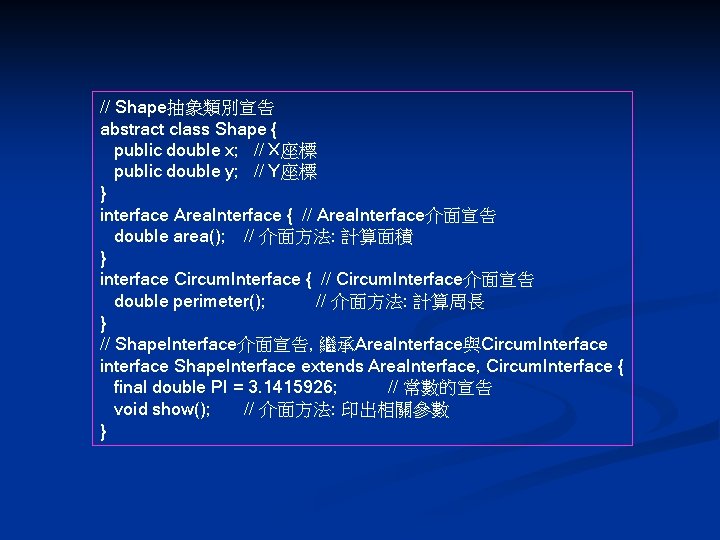
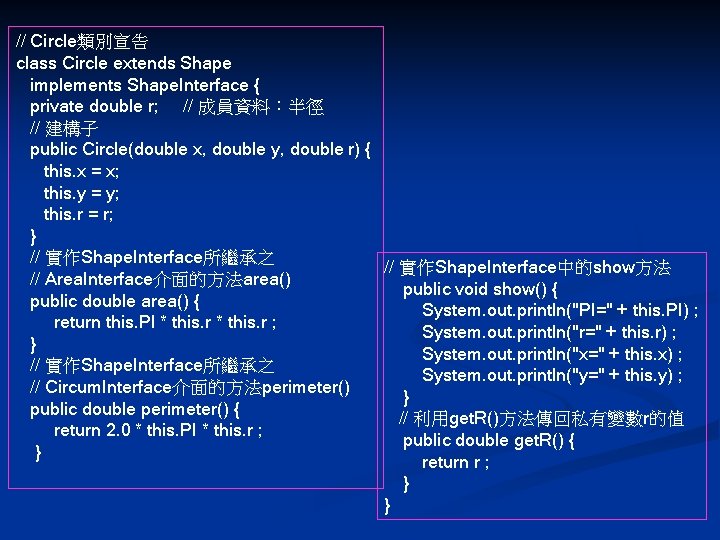
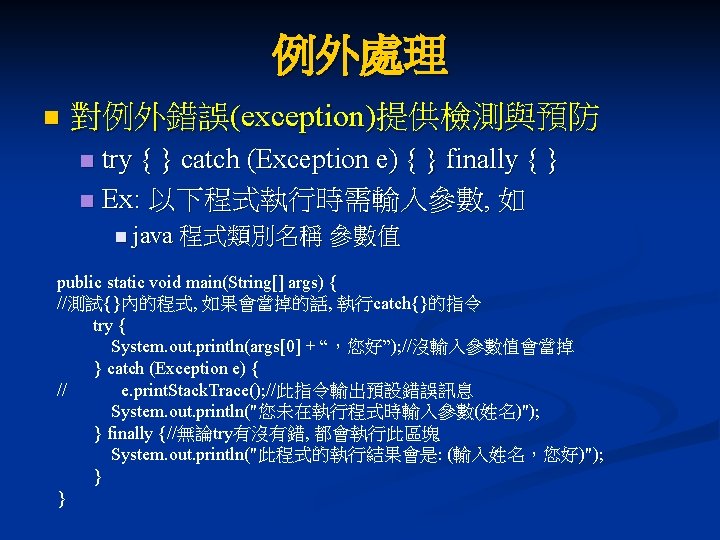
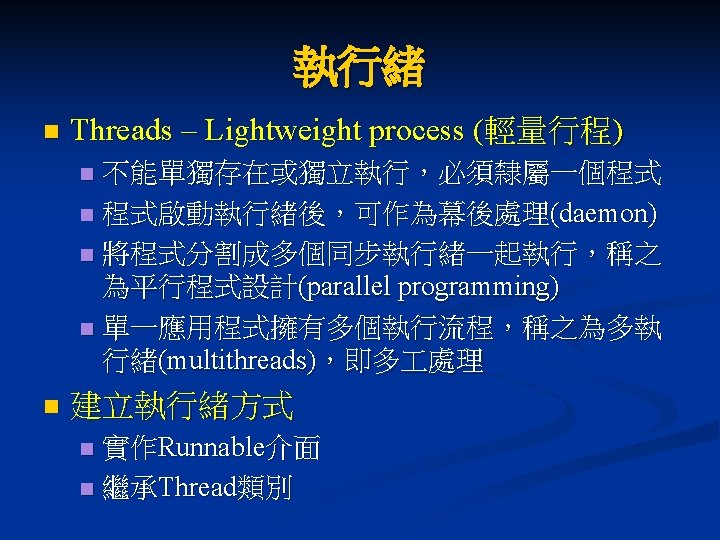
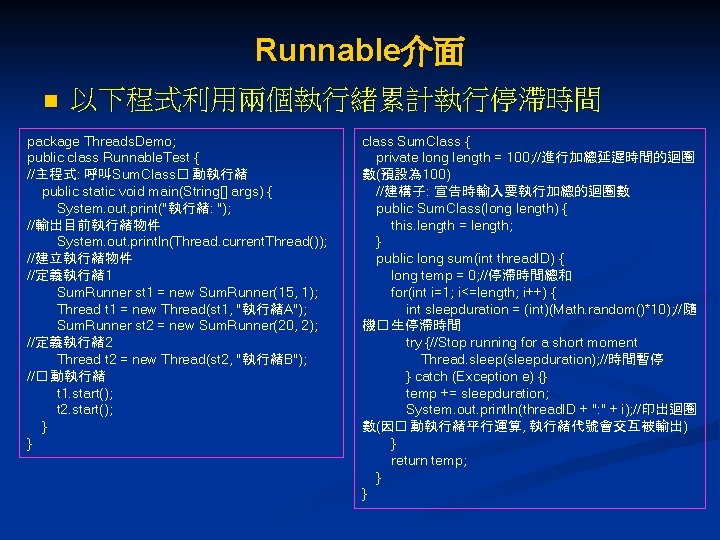
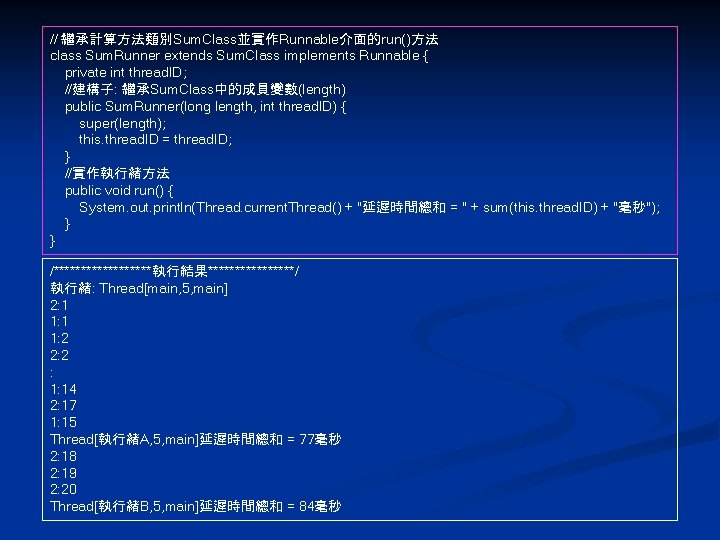
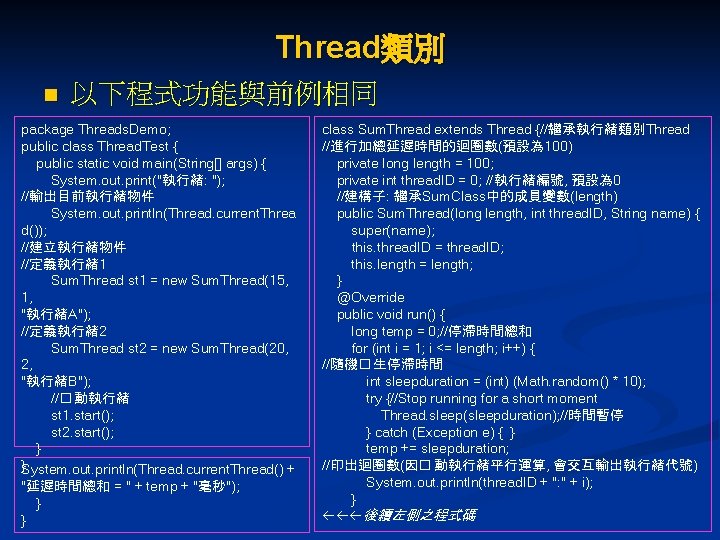
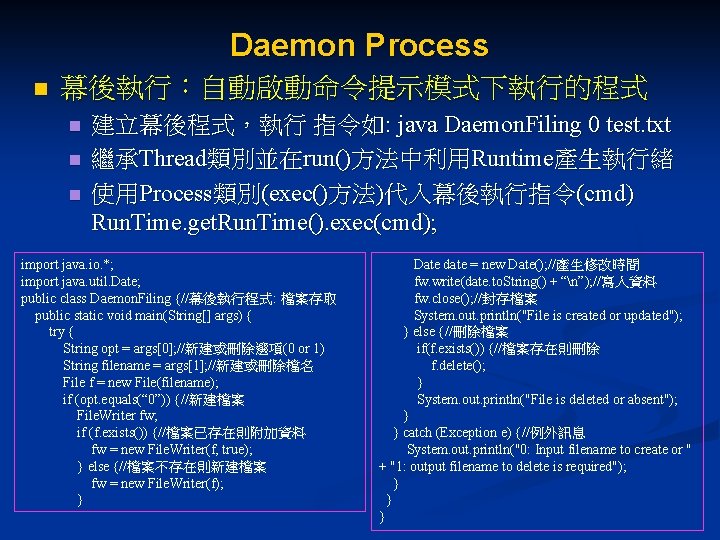
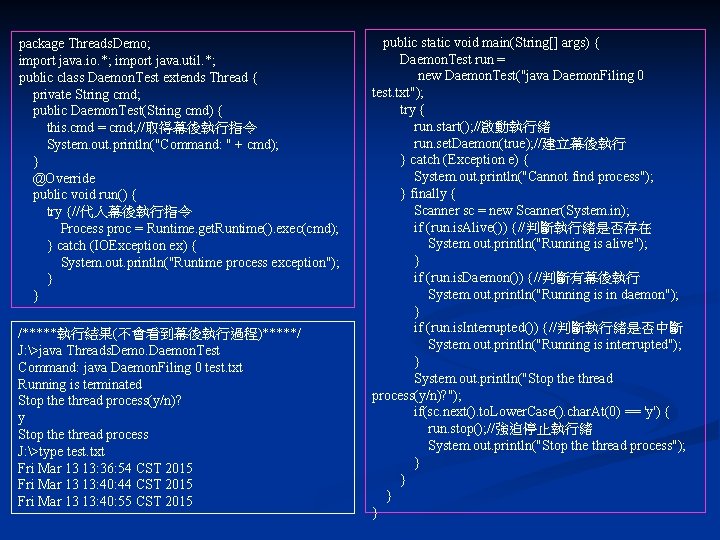
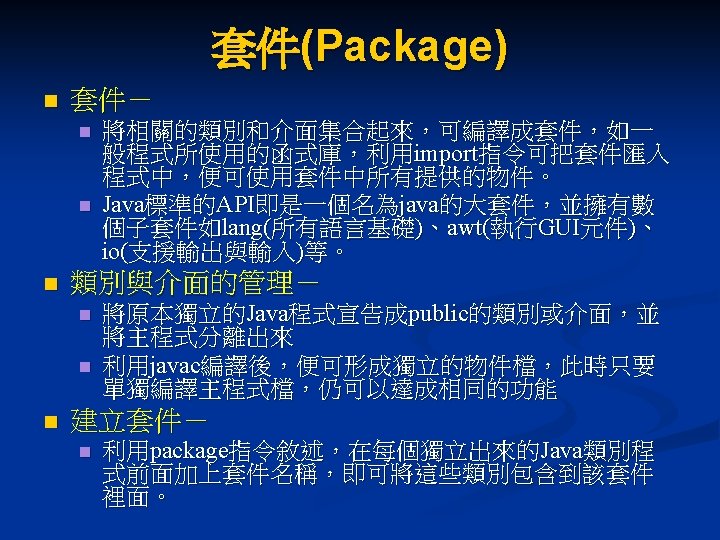
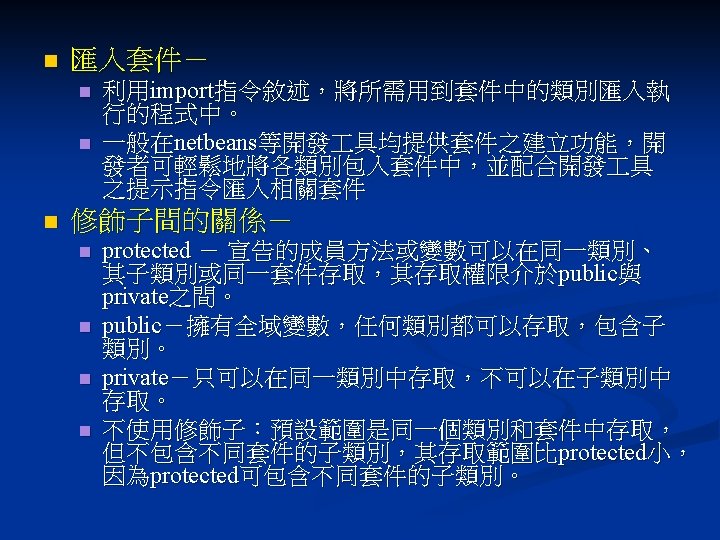
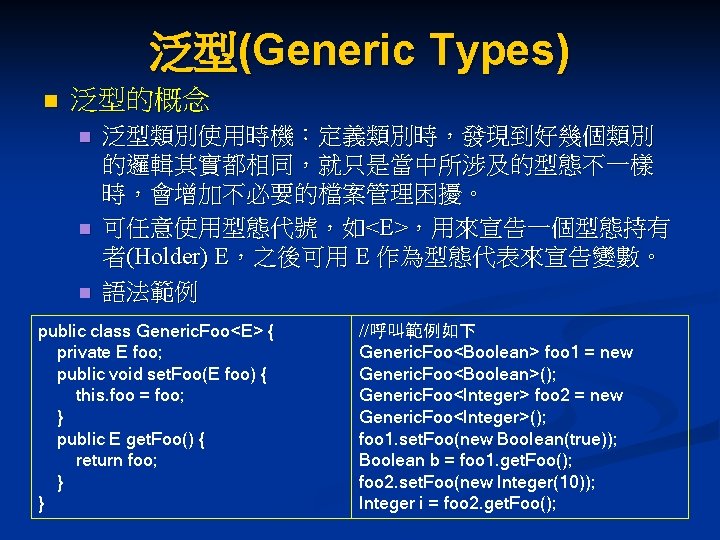
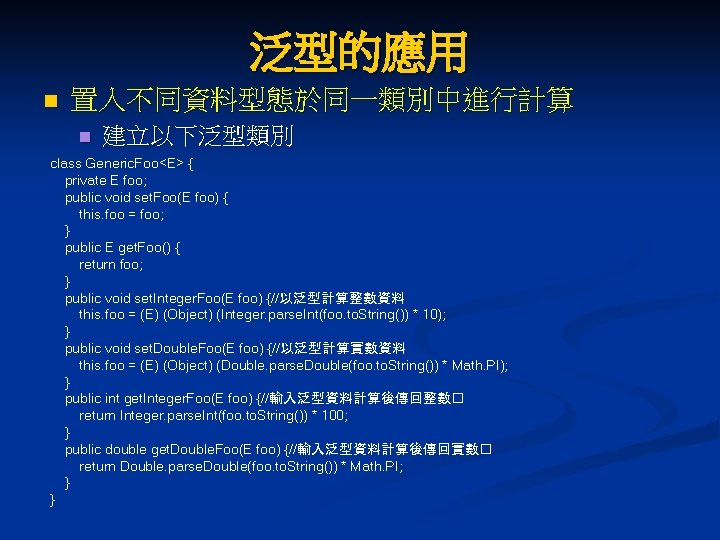
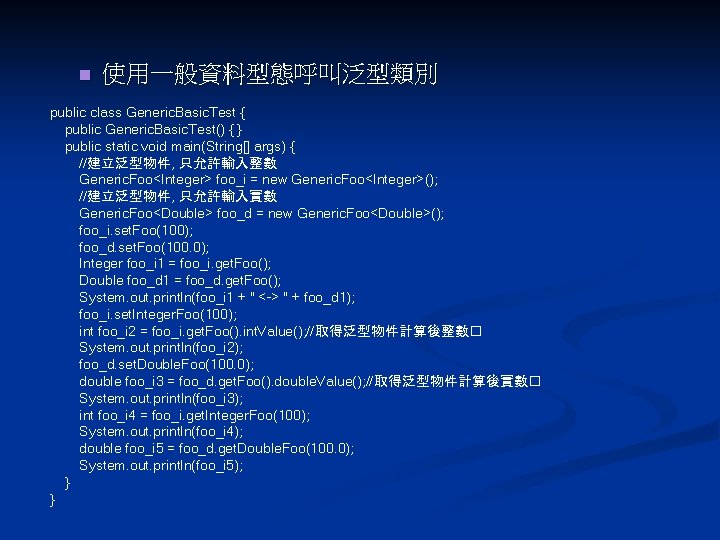
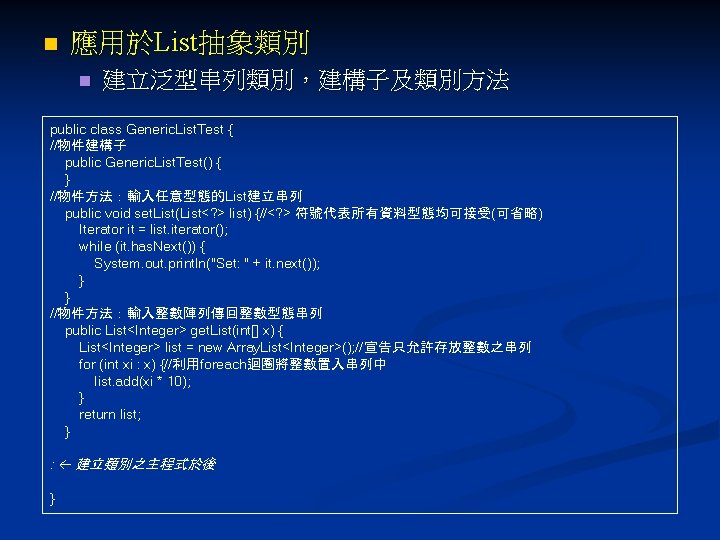
![n 建立主程式如下 //物件主程式 public static void main(String[] args) { //宣告List抽象類別,只允許輸入字串String資料 //抽象類別必須透過實體類別實體化,在此例如Array. List, Linked. List, n 建立主程式如下 //物件主程式 public static void main(String[] args) { //宣告List抽象類別,只允許輸入字串String資料 //抽象類別必須透過實體類別實體化,在此例如Array. List, Linked. List,](https://slidetodoc.com/presentation_image/2ea38baa6be45d6d840cd6c77bc8b5cd/image-42.jpg)
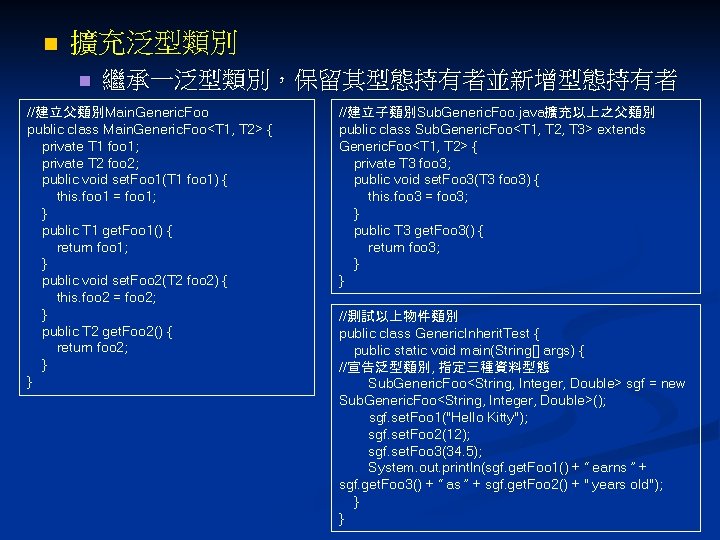
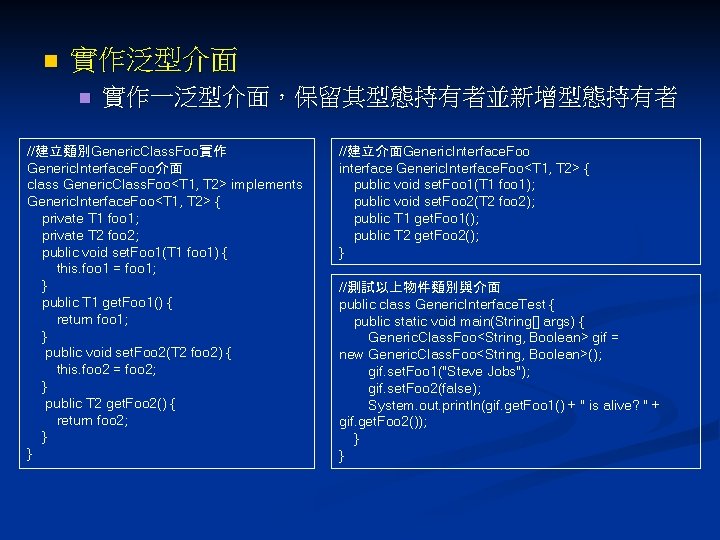
- Slides: 44
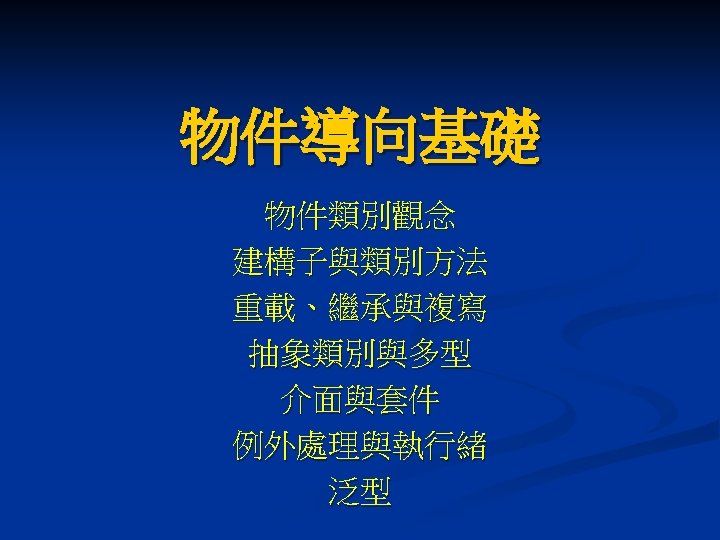
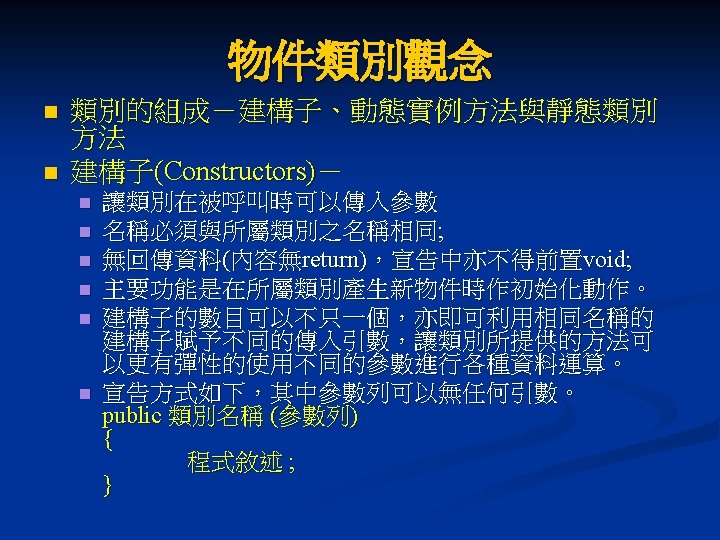
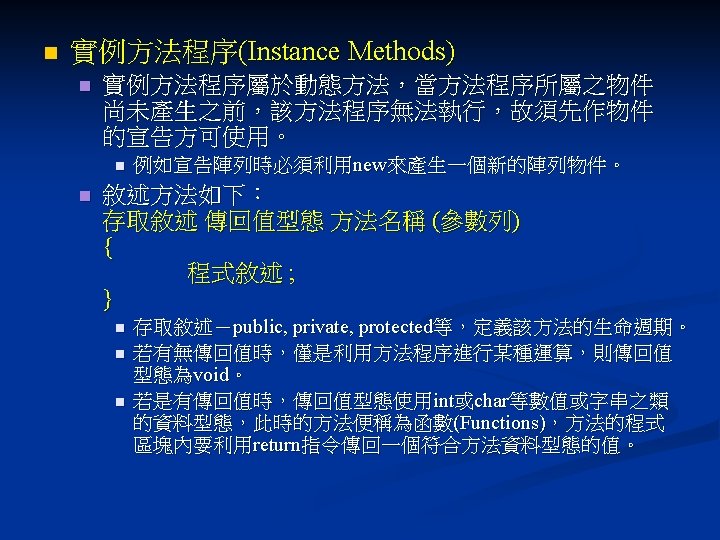
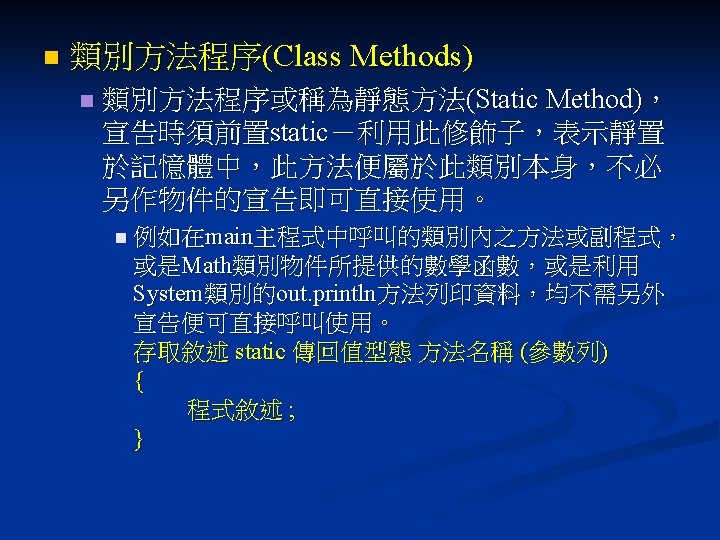
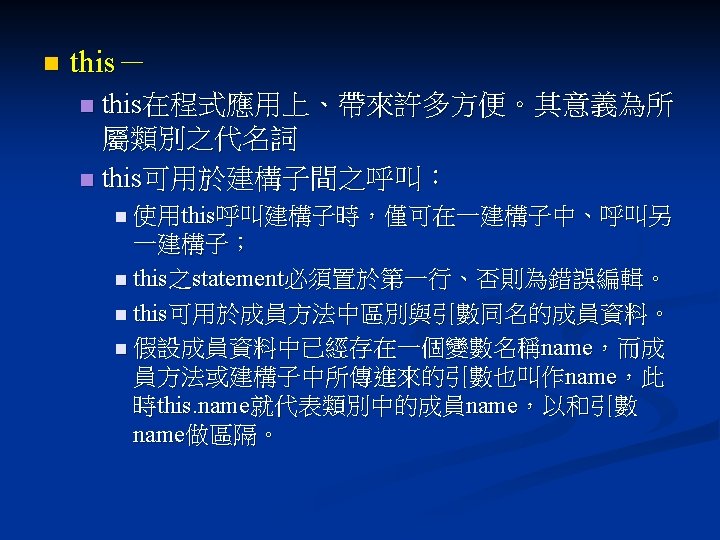
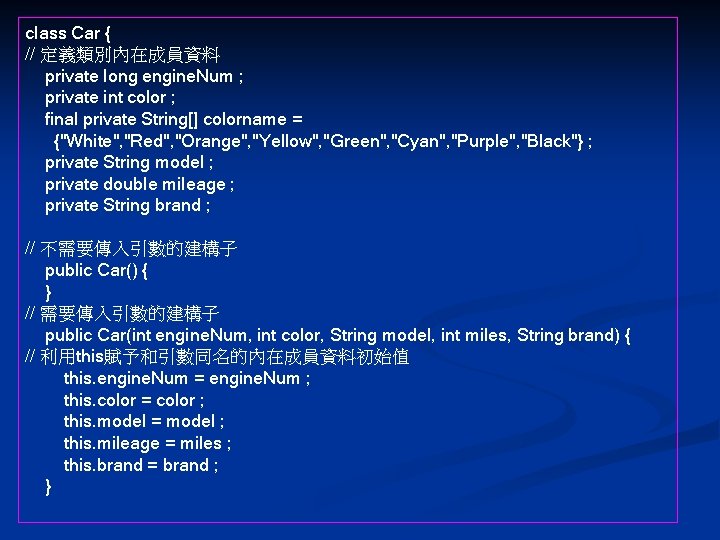
class Car { // 定義類別內在成員資料 private long engine. Num ; private int color ; final private String[] colorname = {"White", "Red", "Orange", "Yellow", "Green", "Cyan", "Purple", "Black"} ; private String model ; private double mileage ; private String brand ; // 不需要傳入引數的建構子 public Car() { } // 需要傳入引數的建構子 public Car(int engine. Num, int color, String model, int miles, String brand) { // 利用this賦予和引數同名的內在成員資料初始值 this. engine. Num = engine. Num ; this. color = color ; this. model = model ; this. mileage = miles ; this. brand = brand ; }
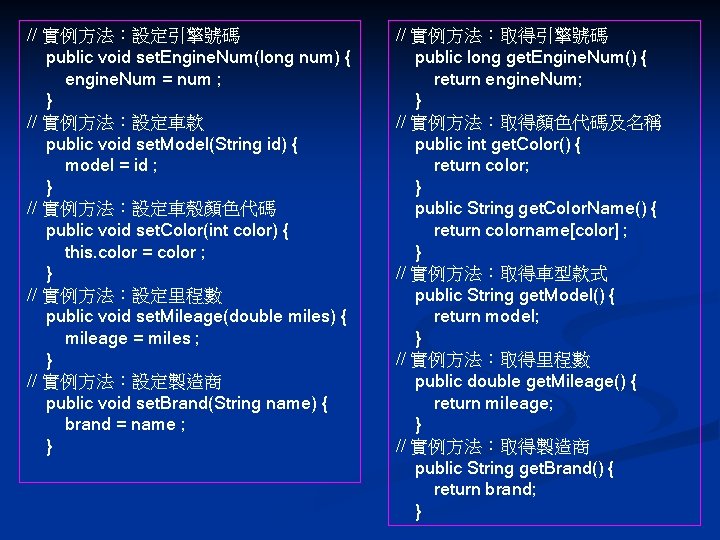
// 實例方法:設定引擎號碼 public void set. Engine. Num(long num) { engine. Num = num ; } // 實例方法:設定車款 public void set. Model(String id) { model = id ; } // 實例方法:設定車殼顏色代碼 public void set. Color(int color) { this. color = color ; } // 實例方法:設定里程數 public void set. Mileage(double miles) { mileage = miles ; } // 實例方法:設定製造商 public void set. Brand(String name) { brand = name ; } // 實例方法:取得引擎號碼 public long get. Engine. Num() { return engine. Num; } // 實例方法:取得顏色代碼及名稱 public int get. Color() { return color; } public String get. Color. Name() { return colorname[color] ; } // 實例方法:取得車型款式 public String get. Model() { return model; } // 實例方法:取得里程數 public double get. Mileage() { return mileage; } // 實例方法:取得製造商 public String get. Brand() { return brand; }
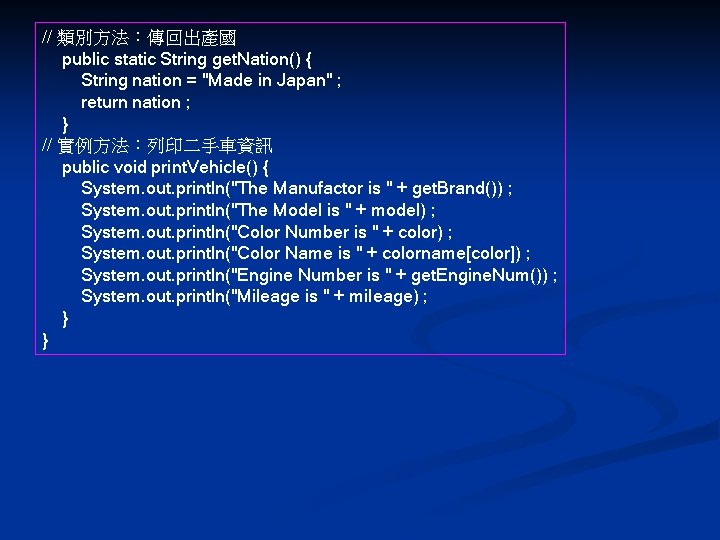
// 類別方法:傳回出產國 public static String get. Nation() { String nation = "Made in Japan" ; return nation ; } // 實例方法:列印二手車資訊 public void print. Vehicle() { System. out. println("The Manufactor is " + get. Brand()) ; System. out. println("The Model is " + model) ; System. out. println("Color Number is " + color) ; System. out. println("Color Name is " + colorname[color]) ; System. out. println("Engine Number is " + get. Engine. Num()) ; System. out. println("Mileage is " + mileage) ; } }
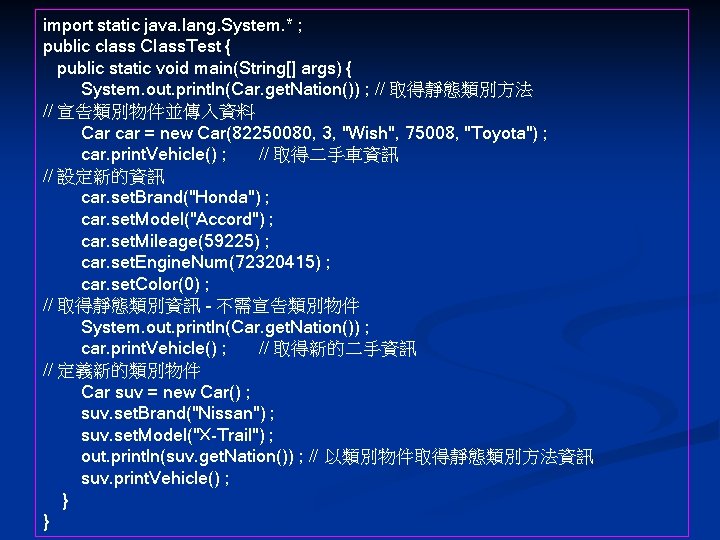
import static java. lang. System. * ; public class Class. Test { public static void main(String[] args) { System. out. println(Car. get. Nation()) ; // 取得靜態類別方法 // 宣告類別物件並傳入資料 Car car = new Car(82250080, 3, "Wish", 75008, "Toyota") ; car. print. Vehicle() ; // 取得二手車資訊 // 設定新的資訊 car. set. Brand("Honda") ; car. set. Model("Accord") ; car. set. Mileage(59225) ; car. set. Engine. Num(72320415) ; car. set. Color(0) ; // 取得靜態類別資訊 – 不需宣告類別物件 System. out. println(Car. get. Nation()) ; car. print. Vehicle() ; // 取得新的二手資訊 // 定義新的類別物件 Car suv = new Car() ; suv. set. Brand("Nissan") ; suv. set. Model("X-Trail") ; out. println(suv. get. Nation()) ; // 以類別物件取得靜態類別方法資訊 suv. print. Vehicle() ; } }
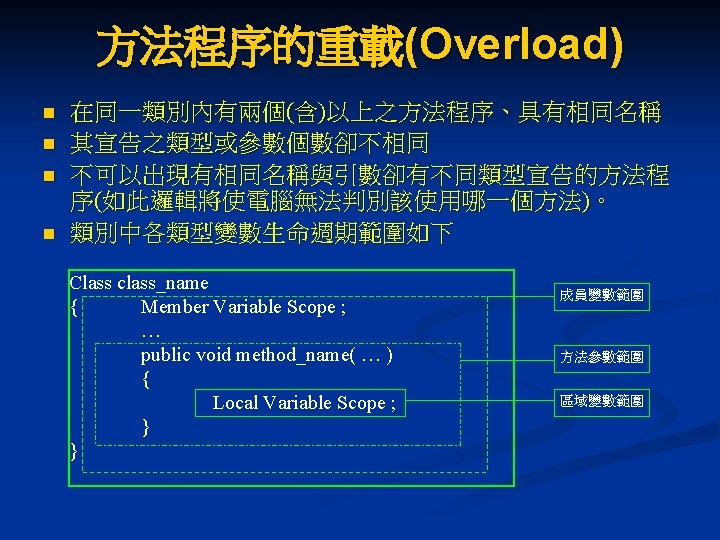
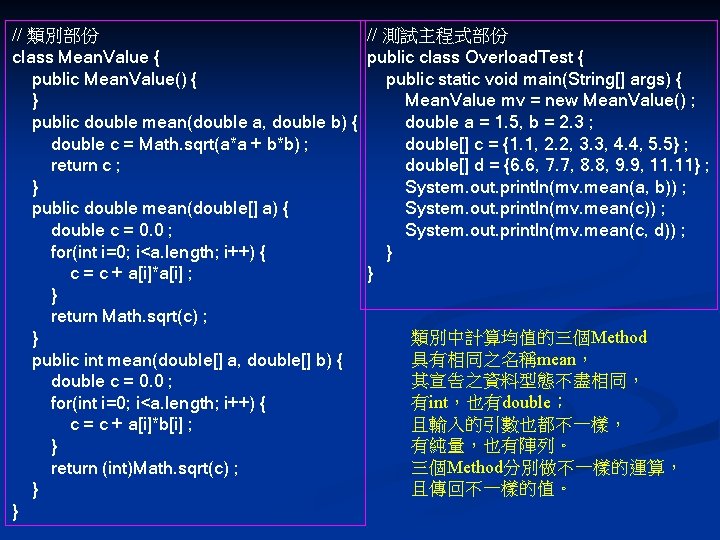
// 類別部份 // 測試主程式部份 class Mean. Value { public class Overload. Test { public Mean. Value() { public static void main(String[] args) { } Mean. Value mv = new Mean. Value() ; public double mean(double a, double b) { double a = 1. 5, b = 2. 3 ; double c = Math. sqrt(a*a + b*b) ; double[] c = {1. 1, 2. 2, 3. 3, 4. 4, 5. 5} ; return c ; double[] d = {6. 6, 7. 7, 8. 8, 9. 9, 11. 11} ; } System. out. println(mv. mean(a, b)) ; public double mean(double[] a) { System. out. println(mv. mean(c)) ; double c = 0. 0 ; System. out. println(mv. mean(c, d)) ; for(int i=0; i<a. length; i++) { } c = c + a[i]*a[i] ; } } return Math. sqrt(c) ; 類別中計算均值的三個Method } 具有相同之名稱mean, public int mean(double[] a, double[] b) { 其宣告之資料型態不盡相同, double c = 0. 0 ; 有int,也有double; for(int i=0; i<a. length; i++) { 且輸入的引數也都不一樣, c = c + a[i]*b[i] ; 有純量,也有陣列。 } 三個Method分別做不一樣的運算, return (int)Math. sqrt(c) ; 且傳回不一樣的值。 } }
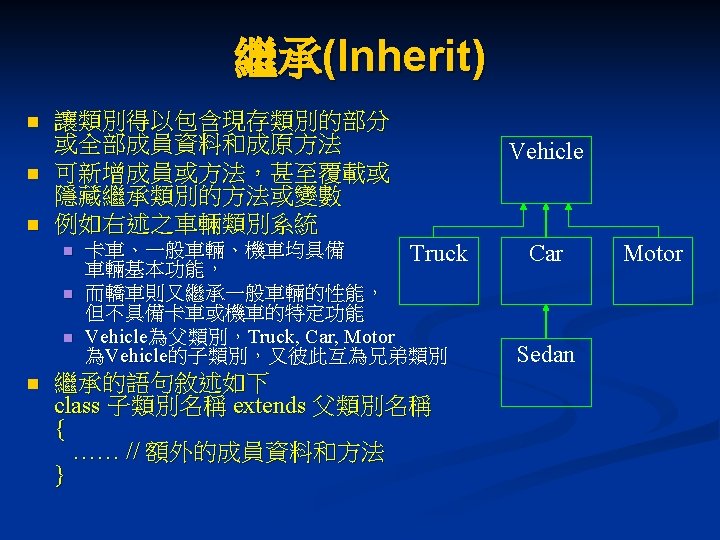
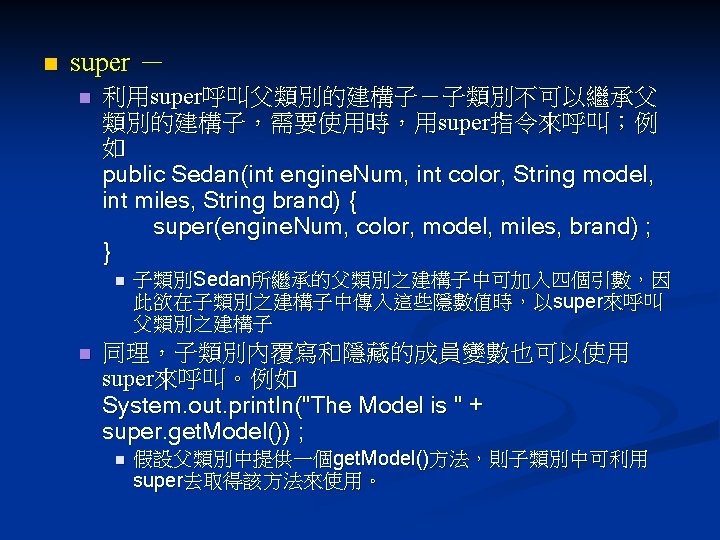
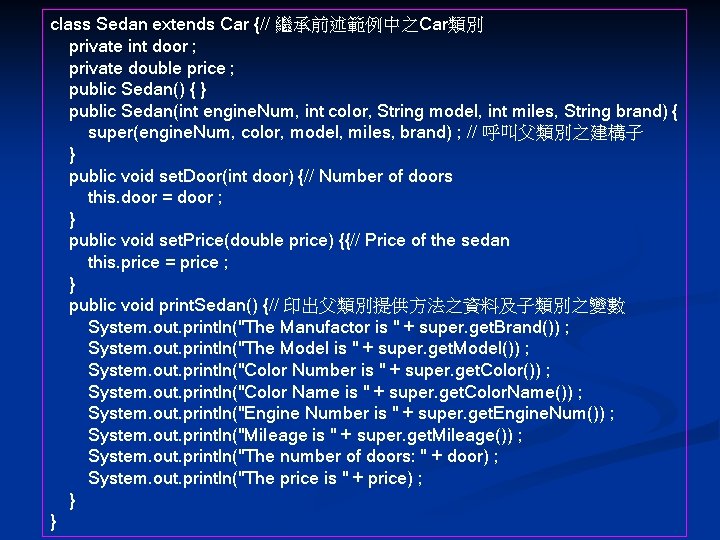
class Sedan extends Car {// 繼承前述範例中之Car類別 private int door ; private double price ; public Sedan() { } public Sedan(int engine. Num, int color, String model, int miles, String brand) { super(engine. Num, color, model, miles, brand) ; // 呼叫父類別之建構子 } public void set. Door(int door) {// Number of doors this. door = door ; } public void set. Price(double price) {{// Price of the sedan this. price = price ; } public void print. Sedan() {// 印出父類別提供方法之資料及子類別之變數 System. out. println("The Manufactor is " + super. get. Brand()) ; System. out. println("The Model is " + super. get. Model()) ; System. out. println("Color Number is " + super. get. Color()) ; System. out. println("Color Name is " + super. get. Color. Name()) ; System. out. println("Engine Number is " + super. get. Engine. Num()) ; System. out. println("Mileage is " + super. get. Mileage()) ; System. out. println("The number of doors: " + door) ; System. out. println("The price is " + price) ; } }
![public class Inherit Test public Inherit Test public static void mainString public class Inherit. Test { public Inherit. Test() { } public static void main(String[]](https://slidetodoc.com/presentation_image/2ea38baa6be45d6d840cd6c77bc8b5cd/image-15.jpg)
public class Inherit. Test { public Inherit. Test() { } public static void main(String[] args) { // TODO code application logic here // 取得父類別之靜態類別方法 System. out. println(Car. get. Nation()) ; // 宣告類別物件並傳入資料 Sedan car = new Sedan(82250080, 3, "Wish", 75008, "Toyota") ; car. print. Vehicle() ; // 利用子類別繼承父類別二手車資訊並印出 // 定義新的子類別物件 Sedan suv = new Sedan() ; suv. set. Brand(“Nissan”) ; // 輸入子類別所繼承之父類別變數資料 suv. set. Model("X-Trail") ; suv. set. Mileage(59225) ; suv. set. Engine. Num(72320415) ; suv. set. Color(0) ; System. out. println(suv. get. Nation()) ; // 取得父類別之靜態類別方法資訊 suv. set. Door(4) ; // 輸入子類別變數資料 suv. set. Price(75000) ; suv. print. Sedan() ; } }
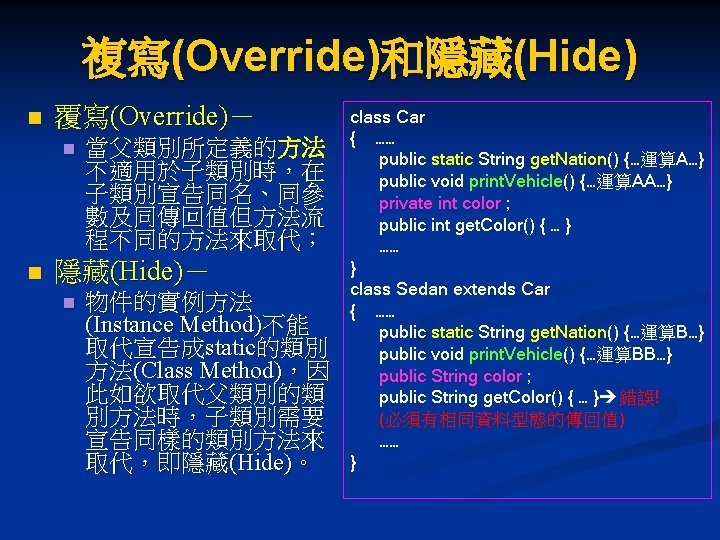
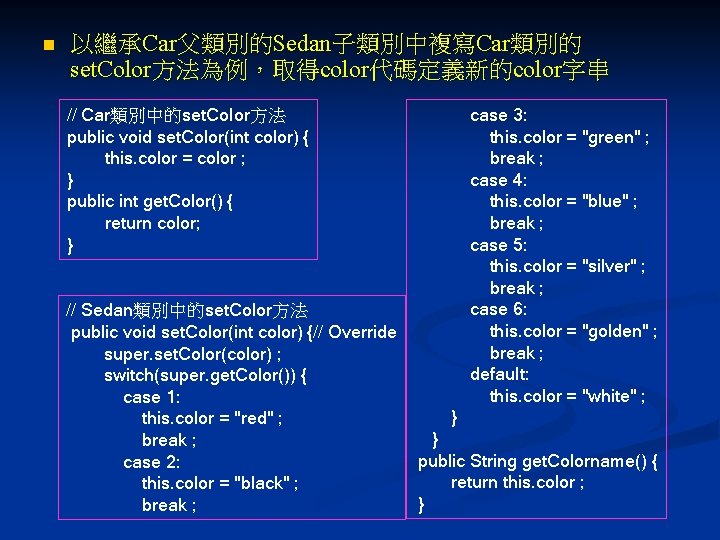
n 以繼承Car父類別的Sedan子類別中複寫Car類別的 set. Color方法為例,取得color代碼定義新的color字串 // Car類別中的set. Color方法 public void set. Color(int color) { this. color = color ; } public int get. Color() { return color; } // Sedan類別中的set. Color方法 public void set. Color(int color) {// Override super. set. Color(color) ; switch(super. get. Color()) { case 1: this. color = "red" ; break ; case 2: this. color = "black" ; break ; case 3: this. color = "green" ; break ; case 4: this. color = "blue" ; break ; case 5: this. color = "silver" ; break ; case 6: this. color = "golden" ; break ; default: this. color = "white" ; } } public String get. Colorname() { return this. color ; }
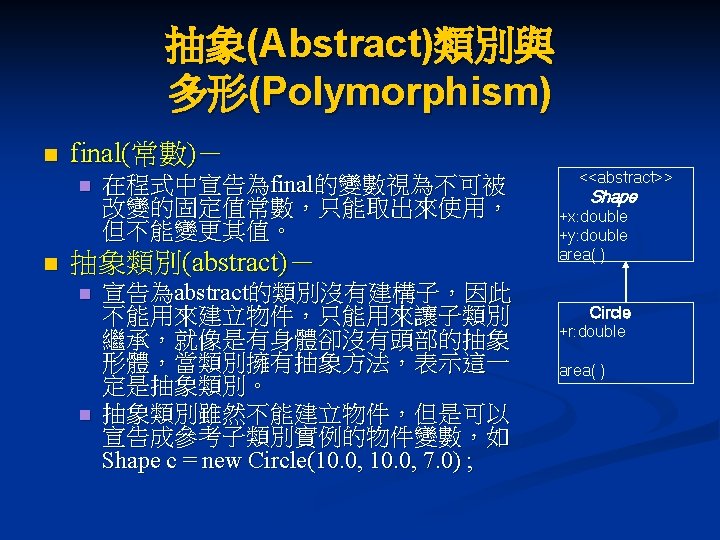
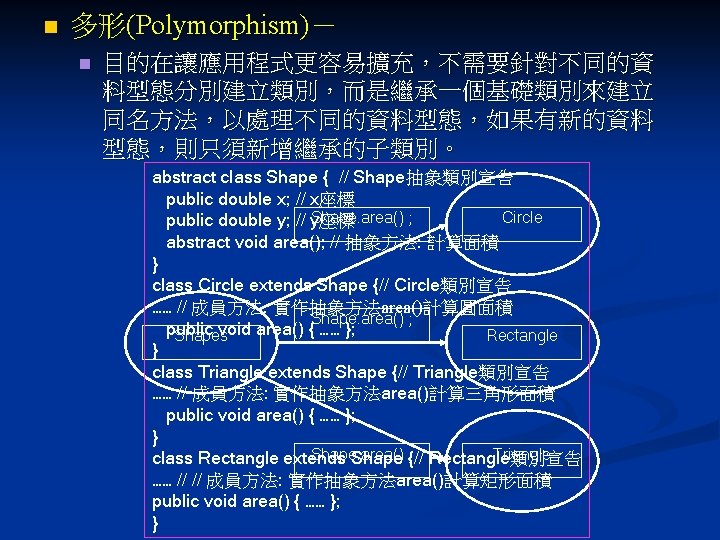
![public class Polymorphism Test public Polymorphism Test public static void mainString public class Polymorphism. Test { public Polymorphism. Test() { } public static void main(String[]](https://slidetodoc.com/presentation_image/2ea38baa6be45d6d840cd6c77bc8b5cd/image-20.jpg)
public class Polymorphism. Test { public Polymorphism. Test() { } public static void main(String[] args) { Shape s; // 抽象類別的物件變數 // 宣告類別型態的變數, 並且建立物件 Circle s 1 = new Circle(5. 0, 10. 0, 4. 0); Rectangle s 2 = new Rectangle(10. 0, 20. 0); Triangle s 3 = new Triangle(10. 0, 5. 0, 25. 0, 5. 0); // 呼叫抽象類型物件的抽象方法area() s = s 1; // 圓形 System. out. println("圓面積" + s. area()); // 相當於執行 Circle. area() ; s = s 2; // 長方形 System. out. println("長方形面積" + s. area()); // 相當於執行 Rectangle. area() s= s 3; // 三角形 System. out. println("三角形面積" + s. area()); // 相當於執行 Triangle. area() } }
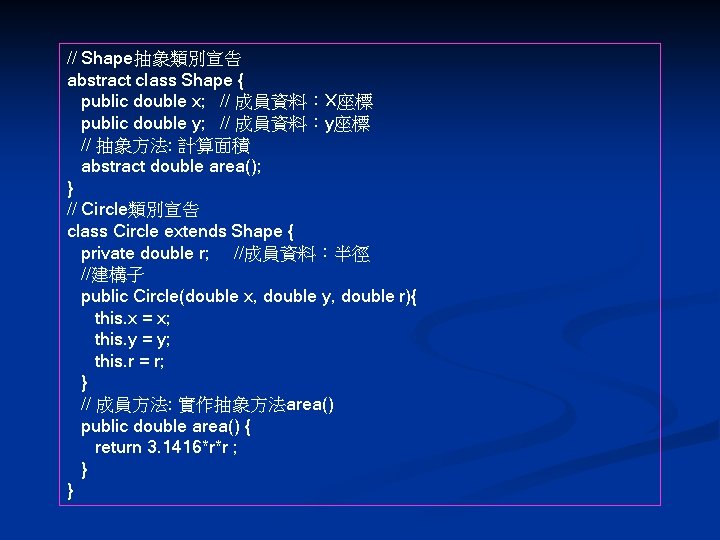
// Shape抽象類別宣告 abstract class Shape { public double x; // 成員資料:X座標 public double y; // 成員資料:y座標 // 抽象方法: 計算面積 abstract double area(); } // Circle類別宣告 class Circle extends Shape { private double r; //成員資料:半徑 //建構子 public Circle(double x, double y, double r){ this. x = x; this. y = y; this. r = r; } // 成員方法: 實作抽象方法area() public double area() { return 3. 1416*r*r ; } }
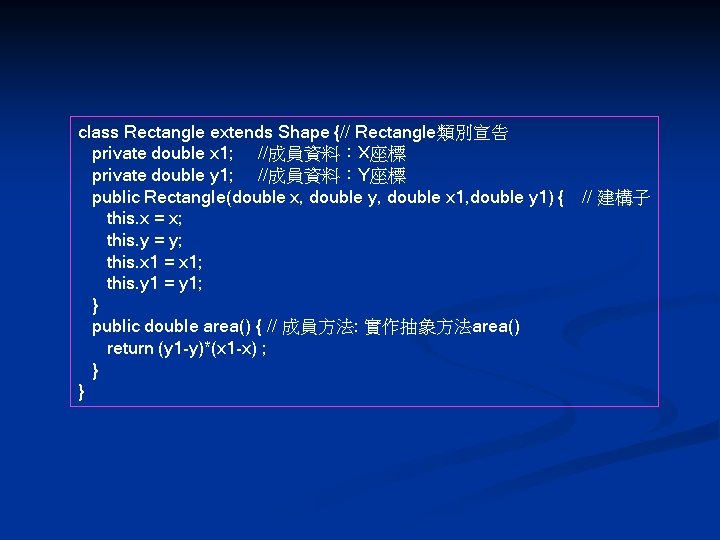
class Rectangle extends Shape {// Rectangle類別宣告 private double x 1; //成員資料:X座標 private double y 1; //成員資料:Y座標 public Rectangle(double x, double y, double x 1, double y 1) { // 建構子 this. x = x; this. y = y; this. x 1 = x 1; this. y 1 = y 1; } public double area() { // 成員方法: 實作抽象方法area() return (y 1 -y)*(x 1 -x) ; } }
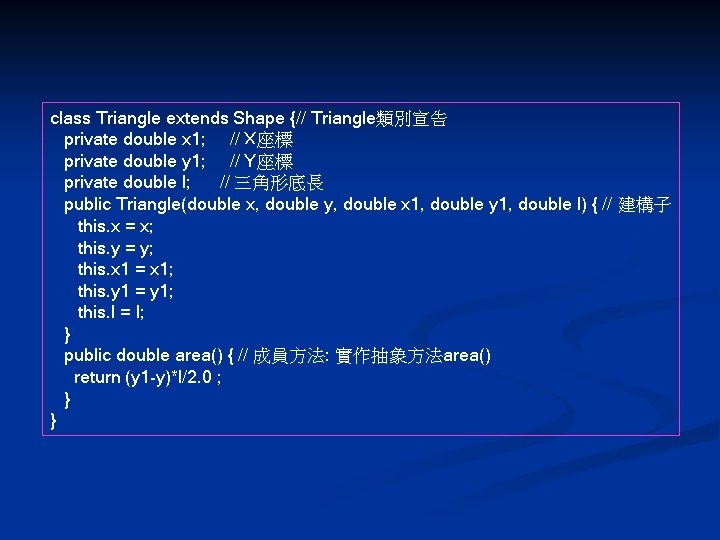
class Triangle extends Shape {// Triangle類別宣告 private double x 1; // X座標 private double y 1; // Y座標 private double l; // 三角形底長 public Triangle(double x, double y, double x 1, double y 1, double l) { // 建構子 this. x = x; this. y = y; this. x 1 = x 1; this. y 1 = y 1; this. l = l; } public double area() { // 成員方法: 實作抽象方法area() return (y 1 -y)*l/2. 0 ; } }
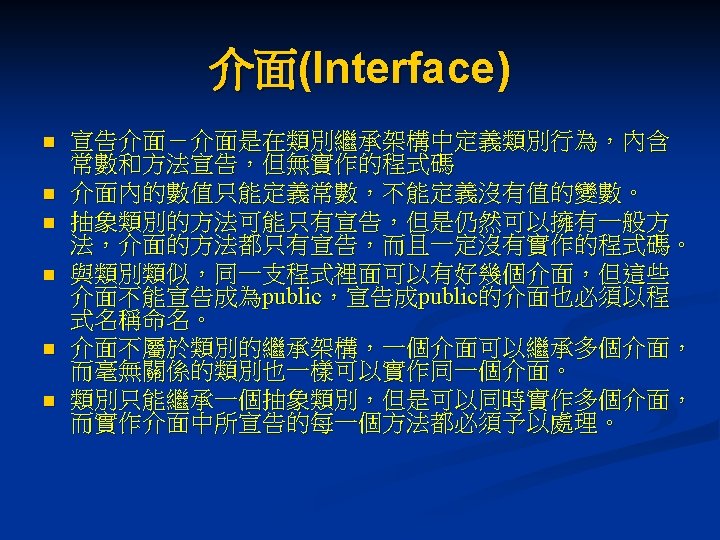
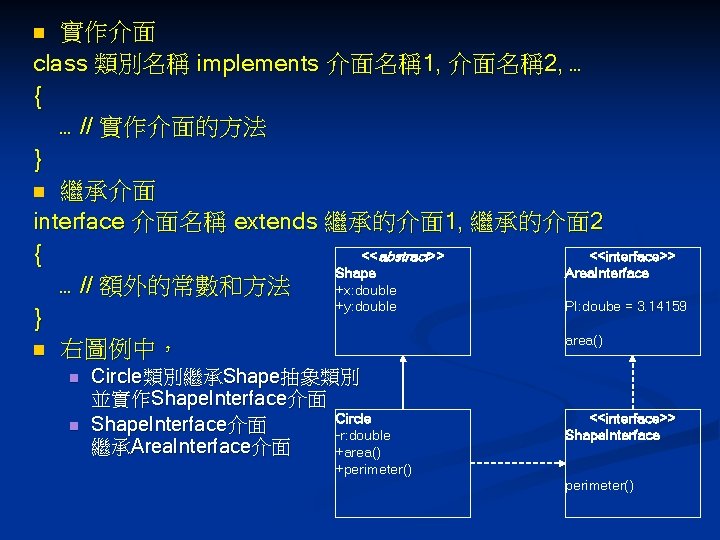
實作介面 class 類別名稱 implements 介面名稱 1, 介面名稱 2, … { … // 實作介面的方法 } n 繼承介面 interface 介面名稱 extends 繼承的介面 1, 繼承的介面 2 <<abstract>> <<interface>> { Shape Area. Interface … // 額外的常數和方法 +x: double +y: double PI: doube = 3. 14159 } area() n 右圖例中, n n n Circle類別繼承Shape抽象類別 並實作Shape. Interface介面 Circle Shape. Interface介面 -r: double 繼承Area. Interface介面 +area() <<interface>> Shape. Interface +perimeter()
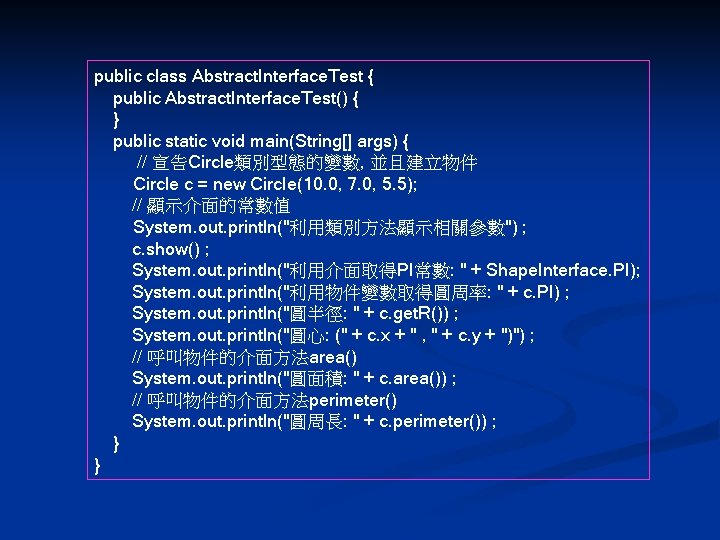
public class Abstract. Interface. Test { public Abstract. Interface. Test() { } public static void main(String[] args) { // 宣告Circle類別型態的變數, 並且建立物件 Circle c = new Circle(10. 0, 7. 0, 5. 5); // 顯示介面的常數值 System. out. println("利用類別方法顯示相關參數") ; c. show() ; System. out. println("利用介面取得PI常數: " + Shape. Interface. PI); System. out. println("利用物件變數取得圓周率: " + c. PI) ; System. out. println("圓半徑: " + c. get. R()) ; System. out. println("圓心: (" + c. x + " , " + c. y + ")") ; // 呼叫物件的介面方法area() System. out. println("圓面積: " + c. area()) ; // 呼叫物件的介面方法perimeter() System. out. println("圓周長: " + c. perimeter()) ; } }
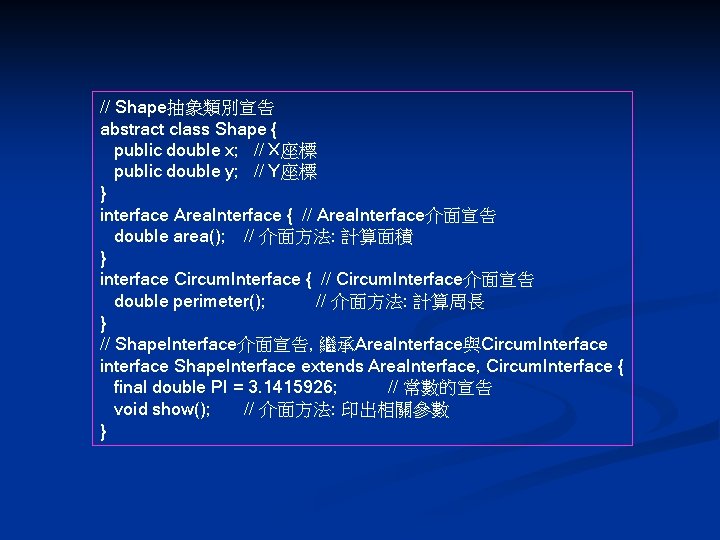
// Shape抽象類別宣告 abstract class Shape { public double x; // X座標 public double y; // Y座標 } interface Area. Interface { // Area. Interface介面宣告 double area(); // 介面方法: 計算面積 } interface Circum. Interface { // Circum. Interface介面宣告 double perimeter(); // 介面方法: 計算周長 } // Shape. Interface介面宣告, 繼承Area. Interface與Circum. Interface interface Shape. Interface extends Area. Interface, Circum. Interface { final double PI = 3. 1415926; // 常數的宣告 void show(); // 介面方法: 印出相關參數 }
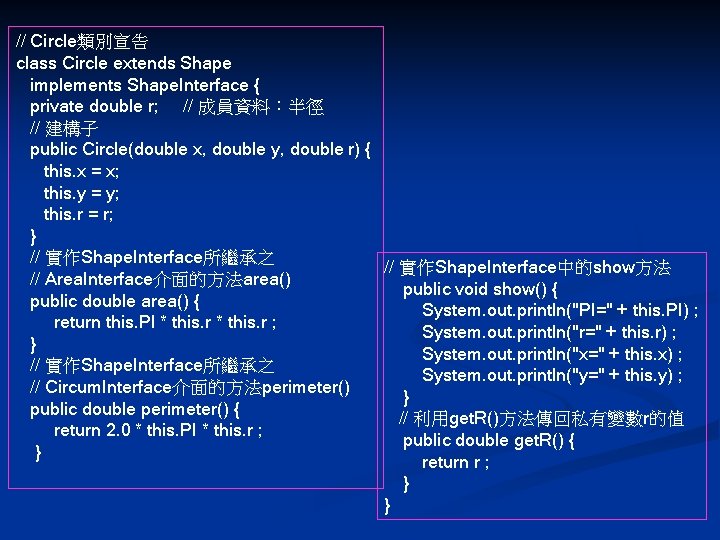
// Circle類別宣告 class Circle extends Shape implements Shape. Interface { private double r; // 成員資料:半徑 // 建構子 public Circle(double x, double y, double r) { this. x = x; this. y = y; this. r = r; } // 實作Shape. Interface所繼承之 // 實作Shape. Interface中的show方法 // Area. Interface介面的方法area() public void show() { public double area() { System. out. println("PI=" + this. PI) ; return this. PI * this. r ; System. out. println("r=" + this. r) ; } System. out. println("x=" + this. x) ; // 實作Shape. Interface所繼承之 System. out. println("y=" + this. y) ; // Circum. Interface介面的方法perimeter() } public double perimeter() { // 利用get. R()方法傳回私有變數r的值 return 2. 0 * this. PI * this. r ; public double get. R() { } return r ; } }
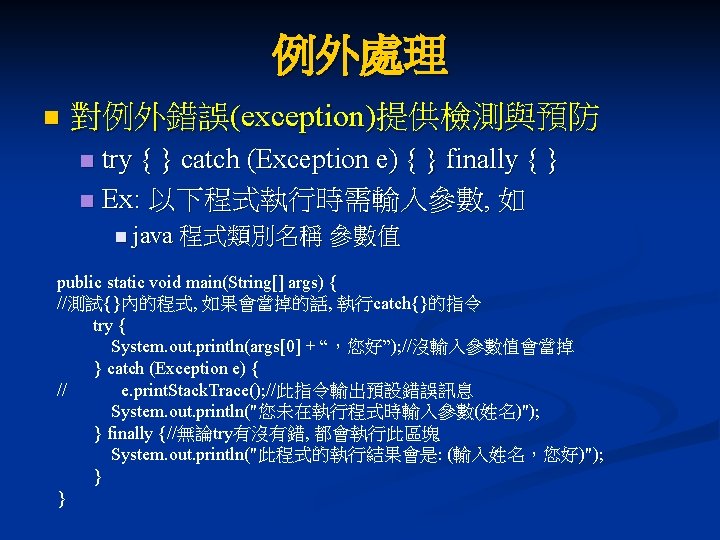
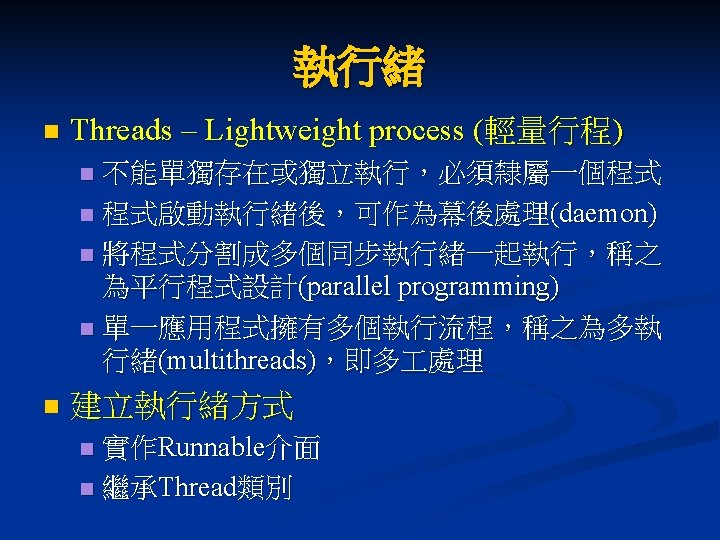
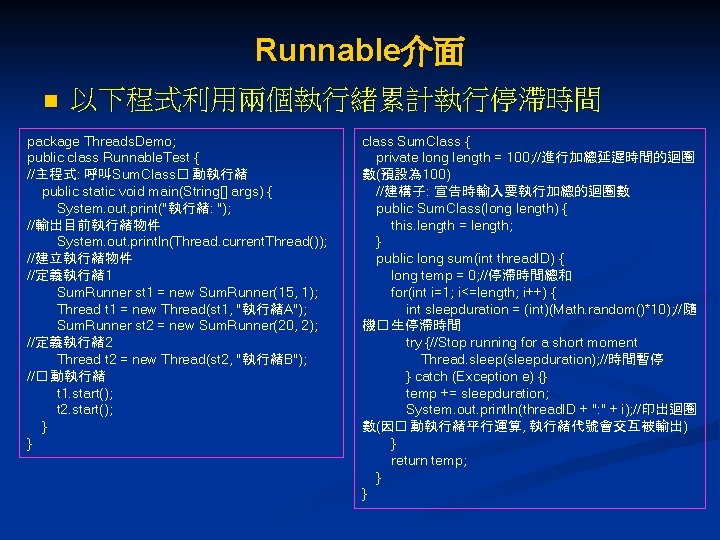
Runnable介面 n 以下程式利用兩個執行緒累計執行停滯時間 package Threads. Demo; public class Runnable. Test { //主程式: 呼叫Sum. Class� 動執行緒 public static void main(String[] args) { System. out. print("執行緒: "); //輸出目前執行緒物件 System. out. println(Thread. current. Thread()); //建立執行緒物件 //定義執行緒 1 Sum. Runner st 1 = new Sum. Runner(15, 1); Thread t 1 = new Thread(st 1, "執行緒A"); Sum. Runner st 2 = new Sum. Runner(20, 2); //定義執行緒 2 Thread t 2 = new Thread(st 2, "執行緒B"); //� 動執行緒 t 1. start(); t 2. start(); } } class Sum. Class { private long length = 100; //進行加總延遲時間的迴圈 數(預設為 100) //建構子: 宣告時輸入要執行加總的迴圈數 public Sum. Class(long length) { this. length = length; } public long sum(int thread. ID) { long temp = 0; //停滯時間總和 for(int i=1; i<=length; i++) { int sleepduration = (int)(Math. random()*10); //隨 機� 生停滯時間 try {//Stop running for a short moment Thread. sleep(sleepduration); //時間暫停 } catch (Exception e) {} temp += sleepduration; System. out. println(thread. ID + ": " + i); //印出迴圈 數(因� 動執行緒平行運算, 執行緒代號會交互被輸出) } return temp; } }
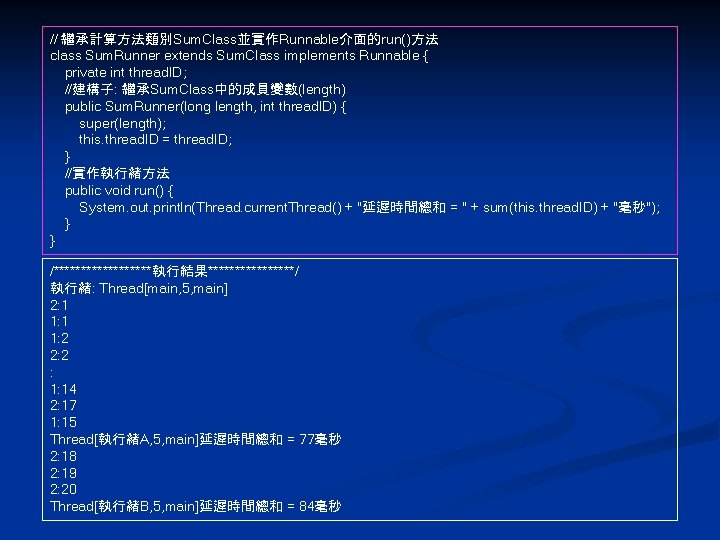
// 繼承計算方法類別Sum. Class並實作Runnable介面的run()方法 class Sum. Runner extends Sum. Class implements Runnable { private int thread. ID; //建構子: 繼承Sum. Class中的成員變數(length) public Sum. Runner(long length, int thread. ID) { super(length); this. thread. ID = thread. ID; } //實作執行緒方法 public void run() { System. out. println(Thread. current. Thread() + "延遲時間總和 = " + sum(this. thread. ID) + "毫秒"); } } /*********執行結果********/ 執行緒: Thread[main, 5, main] 2: 1 1: 2 2: 2 : 1: 14 2: 17 1: 15 Thread[執行緒A, 5, main]延遲時間總和 = 77毫秒 2: 18 2: 19 2: 20 Thread[執行緒B, 5, main]延遲時間總和 = 84毫秒
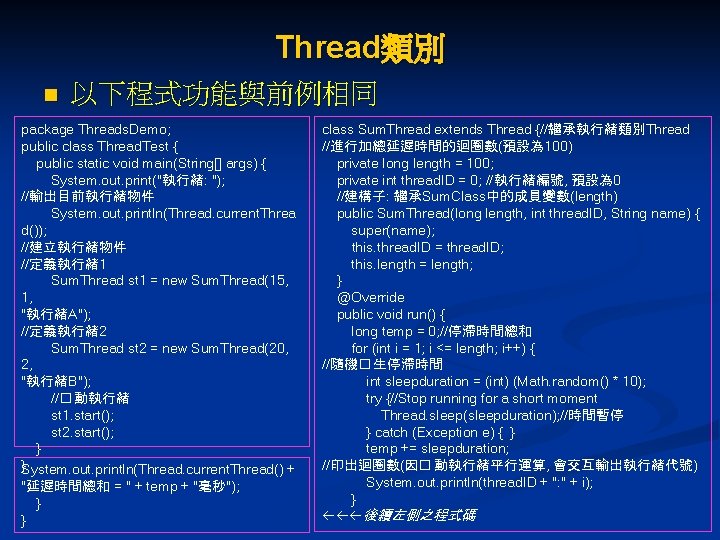
Thread類別 n 以下程式功能與前例相同 package Threads. Demo; public class Thread. Test { public static void main(String[] args) { System. out. print("執行緒: "); //輸出目前執行緒物件 System. out. println(Thread. current. Threa d()); //建立執行緒物件 //定義執行緒 1 Sum. Thread st 1 = new Sum. Thread(15, 1, "執行緒A"); //定義執行緒 2 Sum. Thread st 2 = new Sum. Thread(20, 2, "執行緒B"); //� 動執行緒 st 1. start(); st 2. start(); } }System. out. println(Thread. current. Thread() + "延遲時間總和 = " + temp + "毫秒"); } } class Sum. Thread extends Thread {//繼承執行緒類別Thread //進行加總延遲時間的迴圈數(預設為 100) private long length = 100; private int thread. ID = 0; //執行緒編號, 預設為 0 //建構子: 繼承Sum. Class中的成員變數(length) public Sum. Thread(long length, int thread. ID, String name) { super(name); this. thread. ID = thread. ID; this. length = length; } @Override public void run() { long temp = 0; //停滯時間總和 for (int i = 1; i <= length; i++) { //隨機� 生停滯時間 int sleepduration = (int) (Math. random() * 10); try {//Stop running for a short moment Thread. sleep(sleepduration); //時間暫停 } catch (Exception e) { } temp += sleepduration; //印出迴圈數(因� 動執行緒平行運算, 會交互輸出執行緒代號) System. out. println(thread. ID + ": " + i); } 後續左側之程式碼
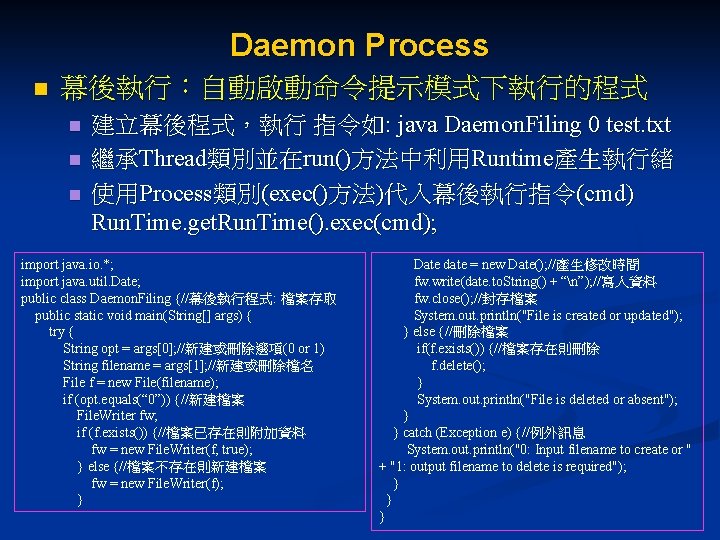
Daemon Process n 幕後執行:自動啟動命令提示模式下執行的程式 n n n 建立幕後程式,執行 指令如: java Daemon. Filing 0 test. txt 繼承Thread類別並在run()方法中利用Runtime產生執行緒 使用Process類別(exec()方法)代入幕後執行指令(cmd) Run. Time. get. Run. Time(). exec(cmd); import java. io. *; import java. util. Date; public class Daemon. Filing {//幕後執行程式: 檔案存取 public static void main(String[] args) { try { String opt = args[0]; //新建或刪除選項(0 or 1) String filename = args[1]; //新建或刪除檔名 File f = new File(filename); if (opt. equals(“ 0”)) {//新建檔案 File. Writer fw; if (f. exists()) {//檔案已存在則附加資料 fw = new File. Writer(f, true); } else {//檔案不存在則新建檔案 fw = new File. Writer(f); } Date date = new Date(); //產生修改時間 fw. write(date. to. String() + “n”); //寫入資料 fw. close(); //封存檔案 System. out. println("File is created or updated"); } else {//刪除檔案 if(f. exists()) {//檔案存在則刪除 f. delete(); } System. out. println("File is deleted or absent"); } } catch (Exception e) {//例外訊息 System. out. println("0: Input filename to create or " + "1: output filename to delete is required"); } } }
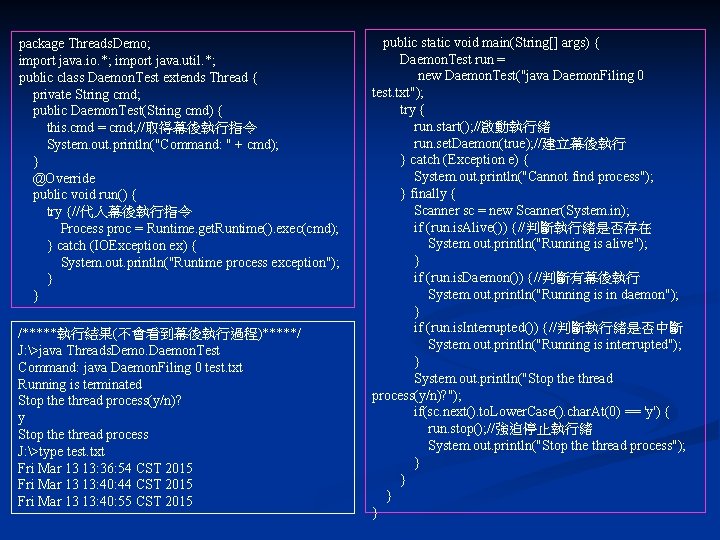
package Threads. Demo; import java. io. *; import java. util. *; public class Daemon. Test extends Thread { private String cmd; public Daemon. Test(String cmd) { this. cmd = cmd; //取得幕後執行指令 System. out. println("Command: " + cmd); } @Override public void run() { try {//代入幕後執行指令 Process proc = Runtime. get. Runtime(). exec(cmd); } catch (IOException ex) { System. out. println("Runtime process exception"); } } /*****執行結果(不會看到幕後執行過程)*****/ J: >java Threads. Demo. Daemon. Test Command: java Daemon. Filing 0 test. txt Running is terminated Stop the thread process(y/n)? y Stop the thread process J: >type test. txt Fri Mar 13 13: 36: 54 CST 2015 Fri Mar 13 13: 40: 44 CST 2015 Fri Mar 13 13: 40: 55 CST 2015 public static void main(String[] args) { Daemon. Test run = new Daemon. Test("java Daemon. Filing 0 test. txt"); try { run. start(); //啟動執行緒 run. set. Daemon(true); //建立幕後執行 } catch (Exception e) { System. out. println("Cannot find process"); } finally { Scanner sc = new Scanner(System. in); if (run. is. Alive()) {//判斷執行緒是否存在 System. out. println("Running is alive"); } if (run. is. Daemon()) {//判斷有幕後執行 System. out. println("Running is in daemon"); } if (run. is. Interrupted()) {//判斷執行緒是否中斷 System. out. println("Running is interrupted"); } System. out. println("Stop the thread process(y/n)? "); if(sc. next(). to. Lower. Case(). char. At(0) == 'y') { run. stop(); //強迫停止執行緒 System. out. println("Stop the thread process"); } }
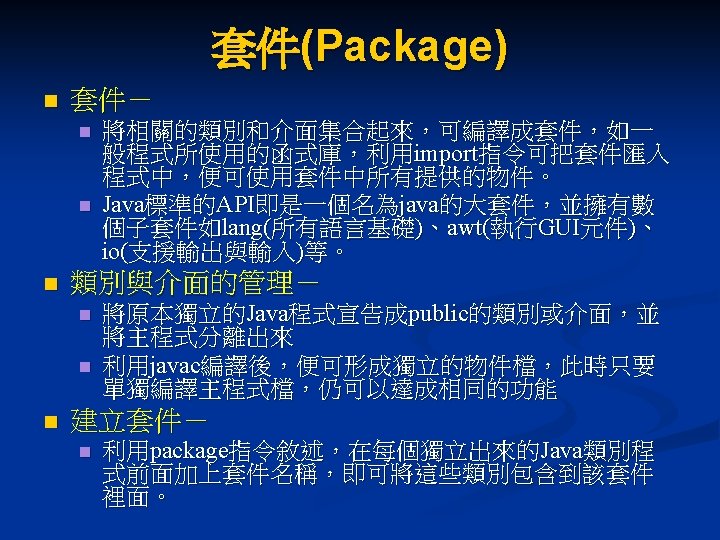
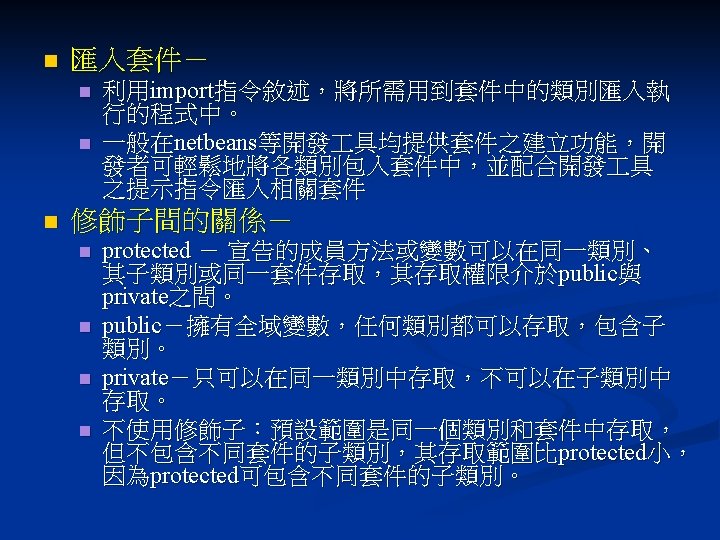
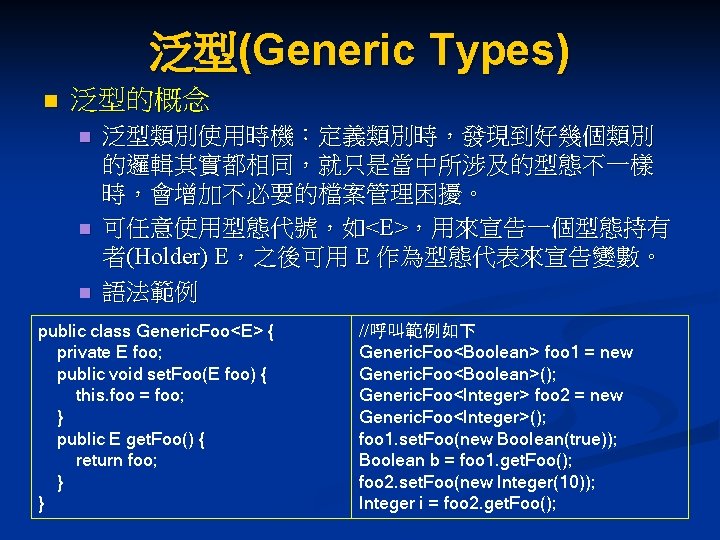
泛型(Generic Types) n 泛型的概念 n n n 泛型類別使用時機:定義類別時,發現到好幾個類別 的邏輯其實都相同,就只是當中所涉及的型態不一樣 時,會增加不必要的檔案管理困擾。 可任意使用型態代號,如<E>,用來宣告一個型態持有 者(Holder) E,之後可用 E 作為型態代表來宣告變數。 語法範例 public class Generic. Foo<E> { private E foo; public void set. Foo(E foo) { this. foo = foo; } public E get. Foo() { return foo; } } //呼叫範例如下 Generic. Foo<Boolean> foo 1 = new Generic. Foo<Boolean>(); Generic. Foo<Integer> foo 2 = new Generic. Foo<Integer>(); foo 1. set. Foo(new Boolean(true)); Boolean b = foo 1. get. Foo(); foo 2. set. Foo(new Integer(10)); Integer i = foo 2. get. Foo();
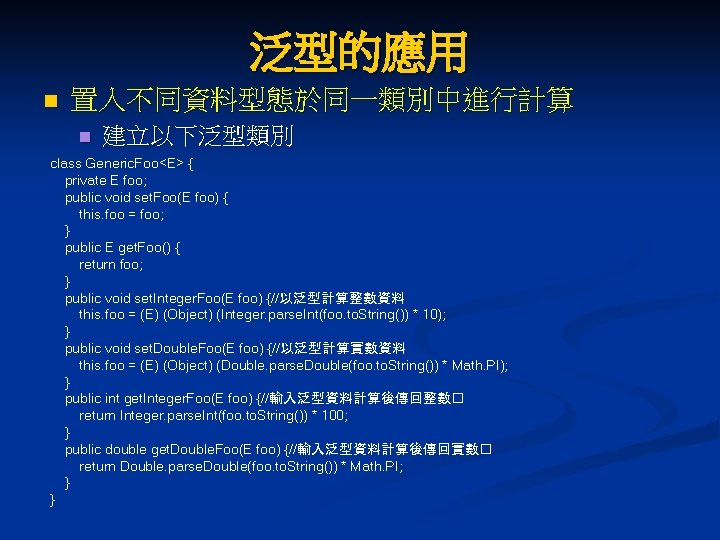
泛型的應用 n 置入不同資料型態於同一類別中進行計算 n 建立以下泛型類別 class Generic. Foo<E> { private E foo; public void set. Foo(E foo) { this. foo = foo; } public E get. Foo() { return foo; } public void set. Integer. Foo(E foo) {//以泛型計算整數資料 this. foo = (E) (Object) (Integer. parse. Int(foo. to. String()) * 10); } public void set. Double. Foo(E foo) {//以泛型計算實數資料 this. foo = (E) (Object) (Double. parse. Double(foo. to. String()) * Math. PI); } public int get. Integer. Foo(E foo) {//輸入泛型資料計算後傳回整數� return Integer. parse. Int(foo. to. String()) * 100; } public double get. Double. Foo(E foo) {//輸入泛型資料計算後傳回實數� return Double. parse. Double(foo. to. String()) * Math. PI; } }
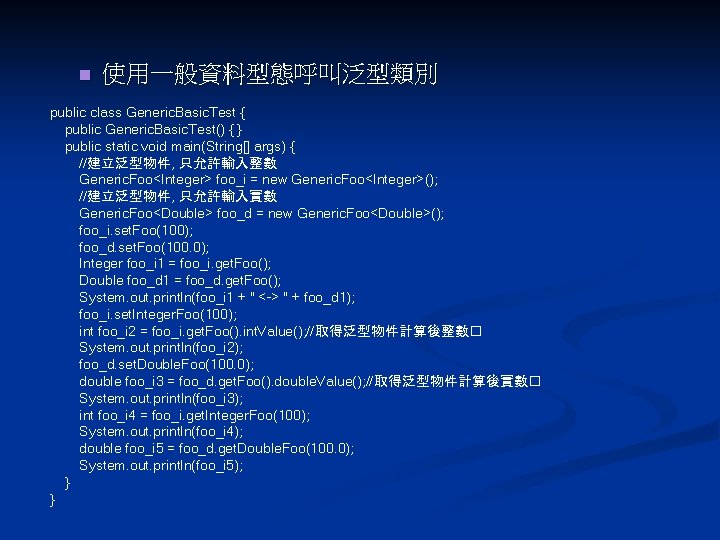
n 使用一般資料型態呼叫泛型類別 public class Generic. Basic. Test { public Generic. Basic. Test() { } public static void main(String[] args) { //建立泛型物件, 只允許輸入整數 Generic. Foo<Integer> foo_i = new Generic. Foo<Integer>(); //建立泛型物件, 只允許輸入實數 Generic. Foo<Double> foo_d = new Generic. Foo<Double>(); foo_i. set. Foo(100); foo_d. set. Foo(100. 0); Integer foo_i 1 = foo_i. get. Foo(); Double foo_d 1 = foo_d. get. Foo(); System. out. println(foo_i 1 + " <-> " + foo_d 1); foo_i. set. Integer. Foo(100); int foo_i 2 = foo_i. get. Foo(). int. Value(); //取得泛型物件計算後整數� System. out. println(foo_i 2); foo_d. set. Double. Foo(100. 0); double foo_i 3 = foo_d. get. Foo(). double. Value(); //取得泛型物件計算後實數� System. out. println(foo_i 3); int foo_i 4 = foo_i. get. Integer. Foo(100); System. out. println(foo_i 4); double foo_i 5 = foo_d. get. Double. Foo(100. 0); System. out. println(foo_i 5); } }
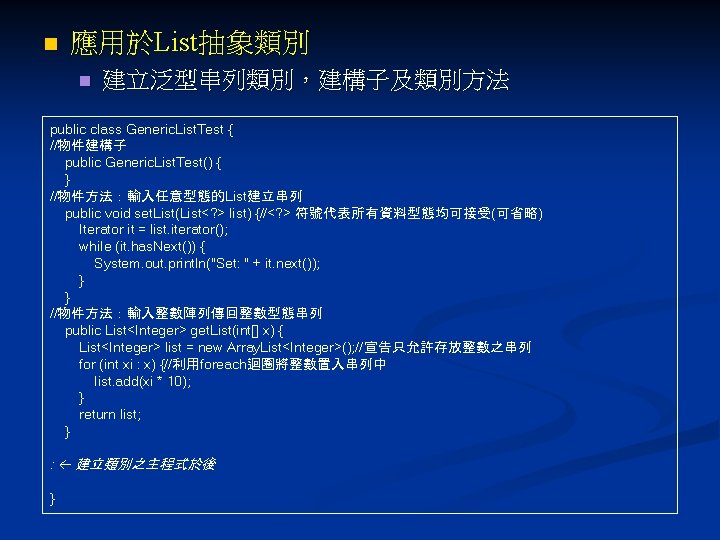
n 應用於List抽象類別 n 建立泛型串列類別,建構子及類別方法 public class Generic. List. Test { //物件建構子 public Generic. List. Test() { } //物件方法:輸入任意型態的List建立串列 public void set. List(List<? > list) {//<? > 符號代表所有資料型態均可接受(可省略) Iterator it = list. iterator(); while (it. has. Next()) { System. out. println("Set: " + it. next()); } } //物件方法:輸入整數陣列傳回整數型態串列 public List<Integer> get. List(int[] x) { List<Integer> list = new Array. List<Integer>(); //宣告只允許存放整數之串列 for (int xi : x) {//利用foreach迴圈將整數置入串列中 list. add(xi * 10); } return list; } : 建立類別之主程式於後 }
![n 建立主程式如下 物件主程式 public static void mainString args 宣告List抽象類別只允許輸入字串String資料 抽象類別必須透過實體類別實體化在此例如Array List Linked List n 建立主程式如下 //物件主程式 public static void main(String[] args) { //宣告List抽象類別,只允許輸入字串String資料 //抽象類別必須透過實體類別實體化,在此例如Array. List, Linked. List,](https://slidetodoc.com/presentation_image/2ea38baa6be45d6d840cd6c77bc8b5cd/image-42.jpg)
n 建立主程式如下 //物件主程式 public static void main(String[] args) { //宣告List抽象類別,只允許輸入字串String資料 //抽象類別必須透過實體類別實體化,在此例如Array. List, Linked. List, Vector等等 List<String> alist = new Array. List<String>(); alist. add("Snow"); alist. add(0, "Ball"); //將下一個字串放在前一個字串之前,字串依序後推一位 Iterator it = alist. iterator(); //宣告走訪串列物件 while (it. has. Next()) {//走訪串列 System. out. println(it. next()); //輸出走訪資料 } Generic. List. Test glt = new Generic. List. Test(); //宣告泛型串列物件 glt. set. List(alist); int[] xx = {1, 2, 3, 4, 5}; List blist = glt. get. List(xx); it = blist. iterator(); while (it. has. Next()) { System. out. println(it. next()); } }
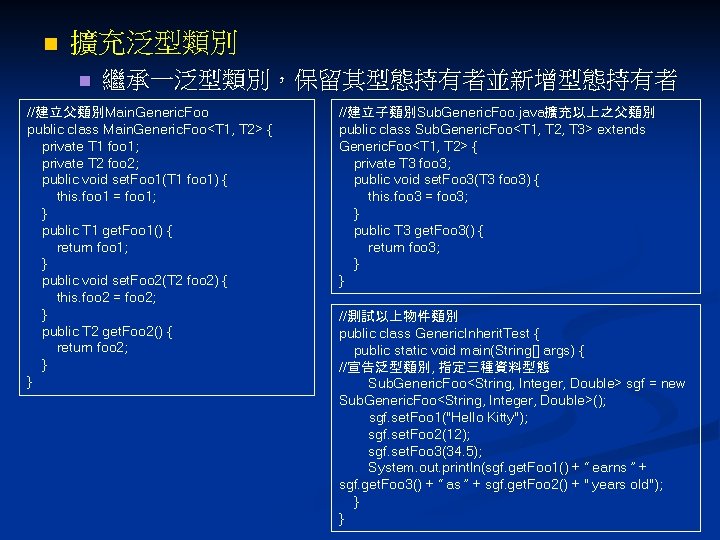
n 擴充泛型類別 n 繼承一泛型類別,保留其型態持有者並新增型態持有者 //建立父類別Main. Generic. Foo public class Main. Generic. Foo<T 1, T 2> { private T 1 foo 1; private T 2 foo 2; public void set. Foo 1(T 1 foo 1) { this. foo 1 = foo 1; } public T 1 get. Foo 1() { return foo 1; } public void set. Foo 2(T 2 foo 2) { this. foo 2 = foo 2; } public T 2 get. Foo 2() { return foo 2; } } //建立子類別Sub. Generic. Foo. java擴充以上之父類別 public class Sub. Generic. Foo<T 1, T 2, T 3> extends Generic. Foo<T 1, T 2> { private T 3 foo 3; public void set. Foo 3(T 3 foo 3) { this. foo 3 = foo 3; } public T 3 get. Foo 3() { return foo 3; } } //測試以上物件類別 public class Generic. Inherit. Test { public static void main(String[] args) { //宣告泛型類別, 指定三種資料型態 Sub. Generic. Foo<String, Integer, Double> sgf = new Sub. Generic. Foo<String, Integer, Double>(); sgf. set. Foo 1("Hello Kitty"); sgf. set. Foo 2(12); sgf. set. Foo 3(34. 5); System. out. println(sgf. get. Foo 1() + “ earns ” + sgf. get. Foo 3() + “ as ” + sgf. get. Foo 2() + " years old"); } }
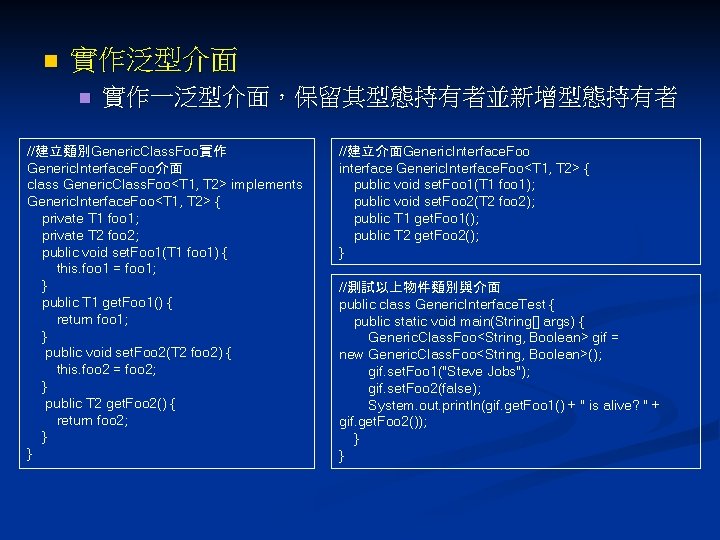
n 實作泛型介面 n 實作一泛型介面,保留其型態持有者並新增型態持有者 //建立類別Generic. Class. Foo實作 Generic. Interface. Foo介面 class Generic. Class. Foo<T 1, T 2> implements Generic. Interface. Foo<T 1, T 2> { private T 1 foo 1; private T 2 foo 2; public void set. Foo 1(T 1 foo 1) { this. foo 1 = foo 1; } public T 1 get. Foo 1() { return foo 1; } public void set. Foo 2(T 2 foo 2) { this. foo 2 = foo 2; } public T 2 get. Foo 2() { return foo 2; } } //建立介面Generic. Interface. Foo interface Generic. Interface. Foo<T 1, T 2> { public void set. Foo 1(T 1 foo 1); public void set. Foo 2(T 2 foo 2); public T 1 get. Foo 1(); public T 2 get. Foo 2(); } //測試以上物件類別與介面 public class Generic. Interface. Test { public static void main(String[] args) { Generic. Class. Foo<String, Boolean> gif = new Generic. Class. Foo<String, Boolean>(); gif. set. Foo 1("Steve Jobs"); gif. set. Foo 2(false); System. out. println(gif. get. Foo 1() + " is alive? " + gif. get. Foo 2()); } }
Sum0
Interface calculator public int add class test
Int main() int num=4
Int max(int x int y)
Public void drawsquare(int x, int y, int len)
Public int divide(int a int b)
Num 2
++
1 num
If car a passes car b, then car a must be ____.
Long long int c
Class person string name
Void func(int num)
Int num;
Int num
Int num
Int num
Int num
Int num
Int num
Tall + short h
Once upon a time there was a house with a man,
Interface myinterface int foo(int x)
Int main(int argc, char** argv)
#include stdio.h void main()
7팩토리얼
Const arduino
Void swap(int a int b)
Int f (int n)
Int b
Char argv
Internal combustion engine vs external combustion engine
Uwheeledvehiclemovementcomponent
Thecartech.com
A { private int x; public a( val) { x = val; } }
Private int
L
Private int
Car 2 car consortium
A 1000 kg car and a 2000 kg car is hoisted the same height
Unsigned int 크기
Unsigned int long
Short int
Byte short int long
Long char