Chapter7 part 3 Arrays TwoDimensional Arrays The Array
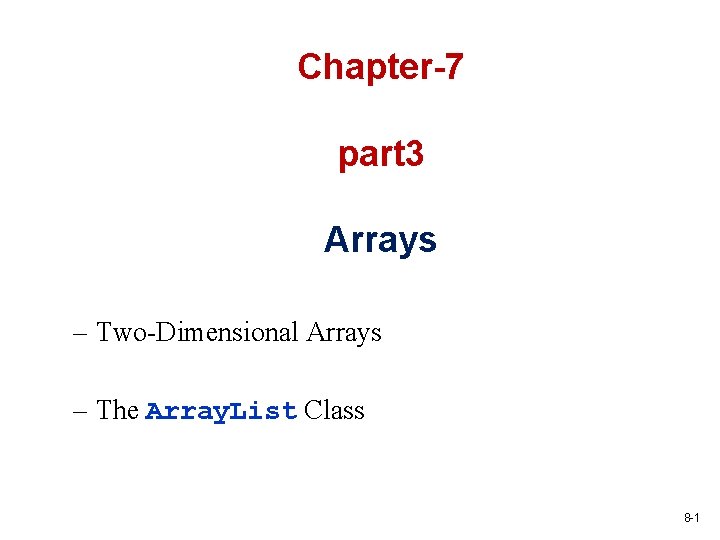
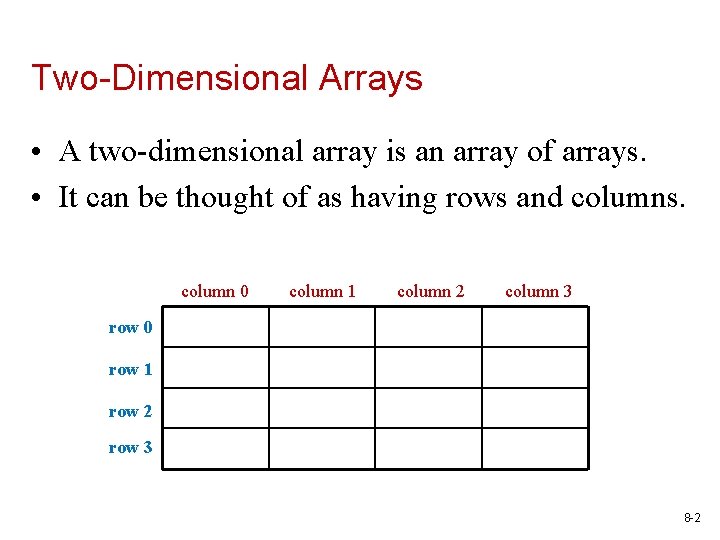
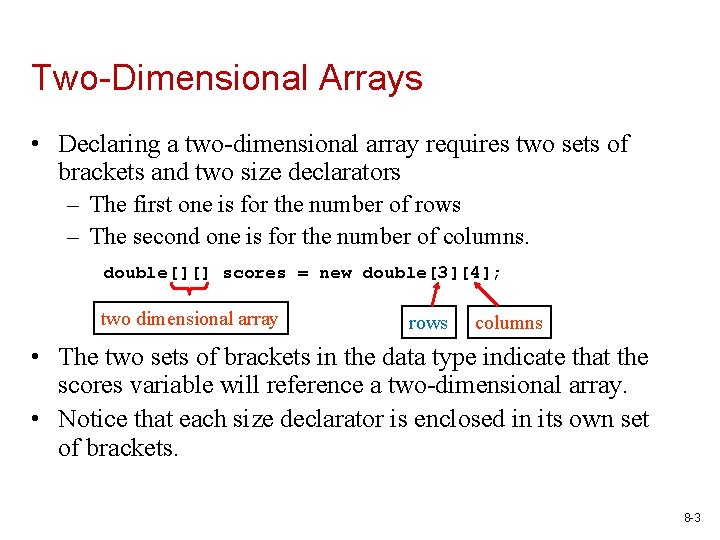
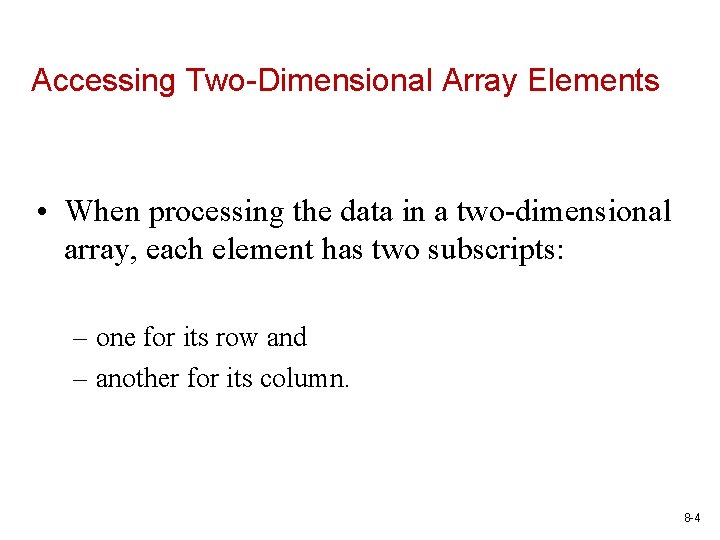
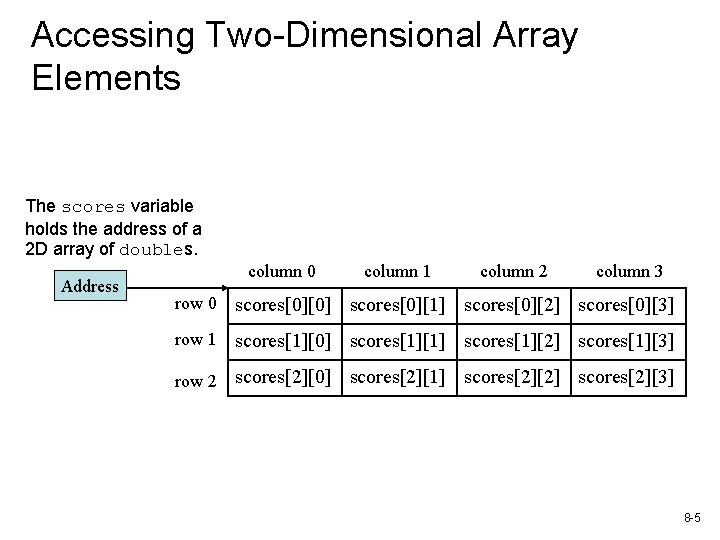
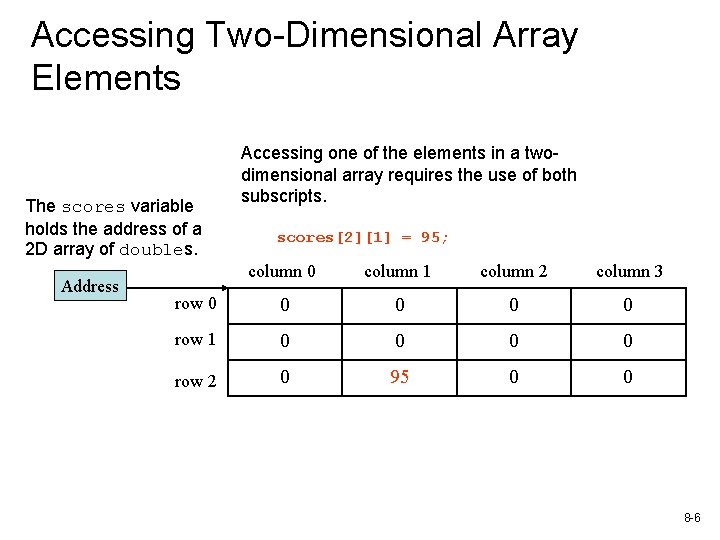
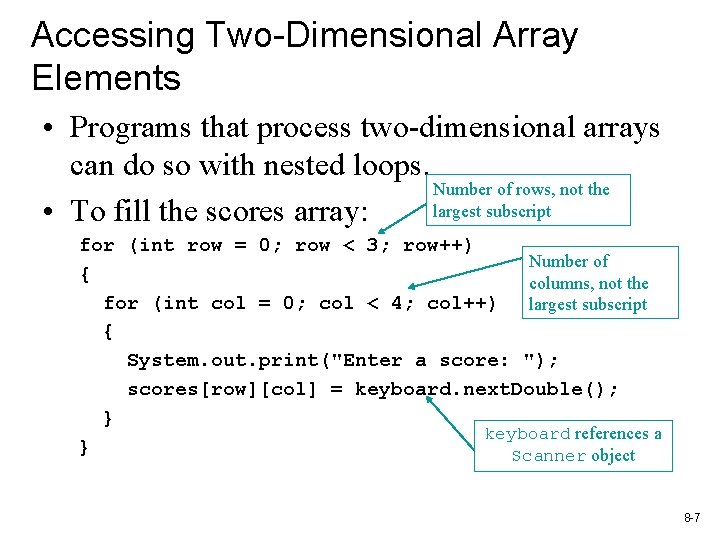
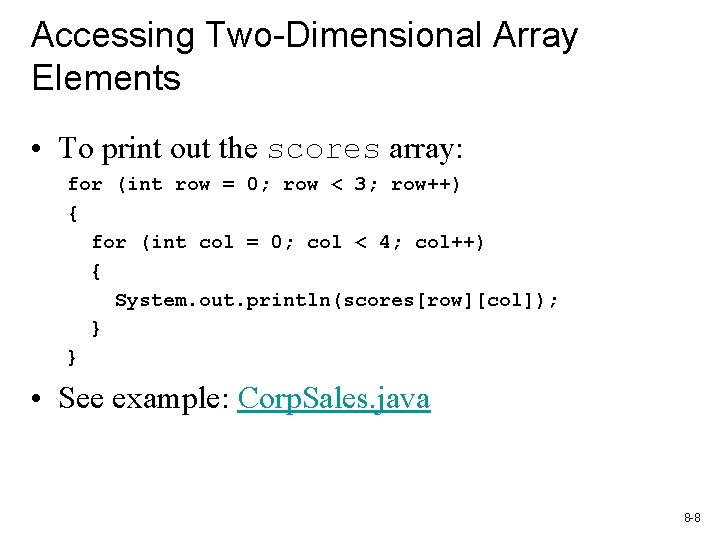
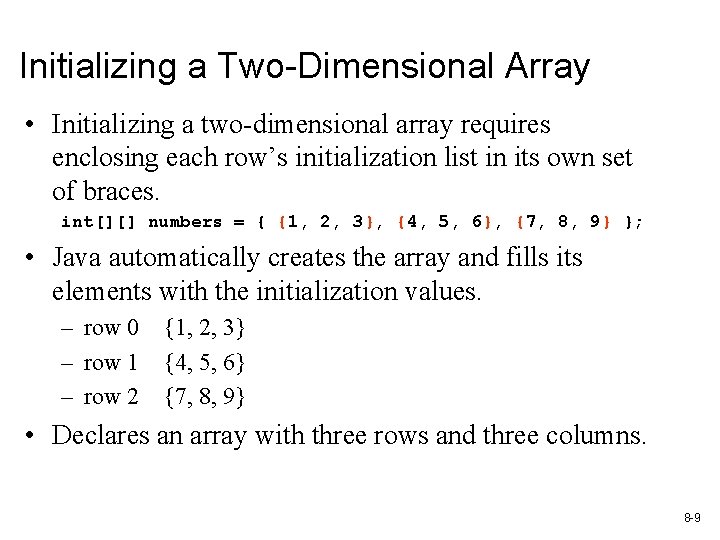
![Initializing a Two-Dimensional Array int[][] numbers = {{1, 2, 3}, {4, 5, 6}, {7, Initializing a Two-Dimensional Array int[][] numbers = {{1, 2, 3}, {4, 5, 6}, {7,](https://slidetodoc.com/presentation_image_h/0884650d2ff138e24ad545ce2b0266bf/image-10.jpg)
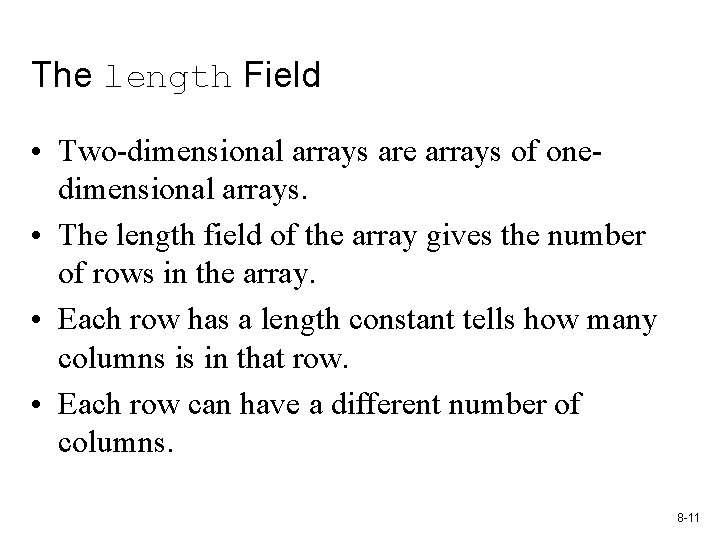
![The length Field • To access the length fields of the array: int[][] numbers The length Field • To access the length fields of the array: int[][] numbers](https://slidetodoc.com/presentation_image_h/0884650d2ff138e24ad545ce2b0266bf/image-12.jpg)
![Summing The Elements of a Two. Dimensional Array int[][] numbers = { { 1, Summing The Elements of a Two. Dimensional Array int[][] numbers = { { 1,](https://slidetodoc.com/presentation_image_h/0884650d2ff138e24ad545ce2b0266bf/image-13.jpg)
![Summing The Rows of a Two. Dimensional Array int[][] numbers = {{ 1, 2, Summing The Rows of a Two. Dimensional Array int[][] numbers = {{ 1, 2,](https://slidetodoc.com/presentation_image_h/0884650d2ff138e24ad545ce2b0266bf/image-14.jpg)
![Summing The Columns of a Two. Dimensional Array int[][] numbers = {{1, 2, 3, Summing The Columns of a Two. Dimensional Array int[][] numbers = {{1, 2, 3,](https://slidetodoc.com/presentation_image_h/0884650d2ff138e24ad545ce2b0266bf/image-15.jpg)
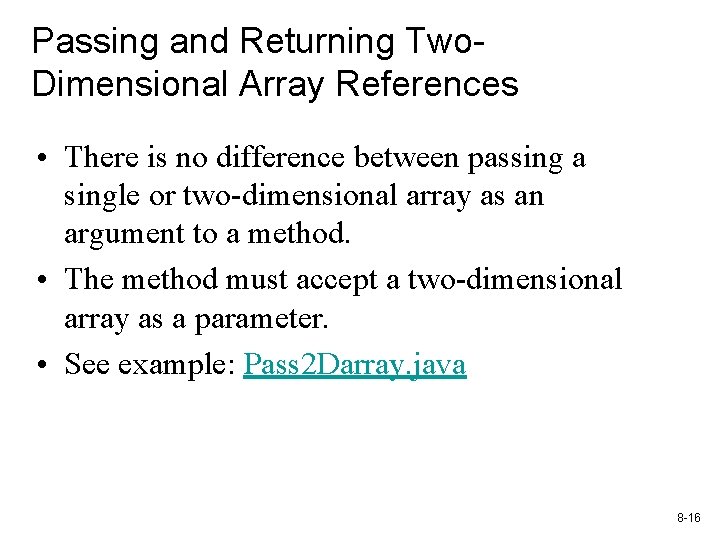
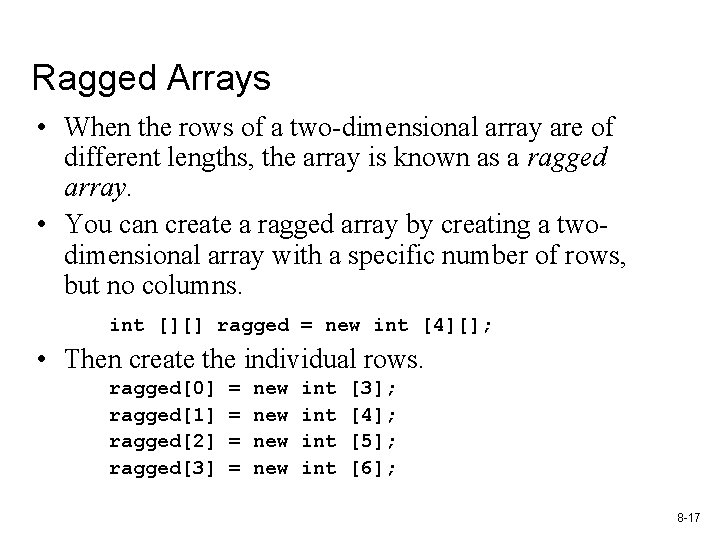
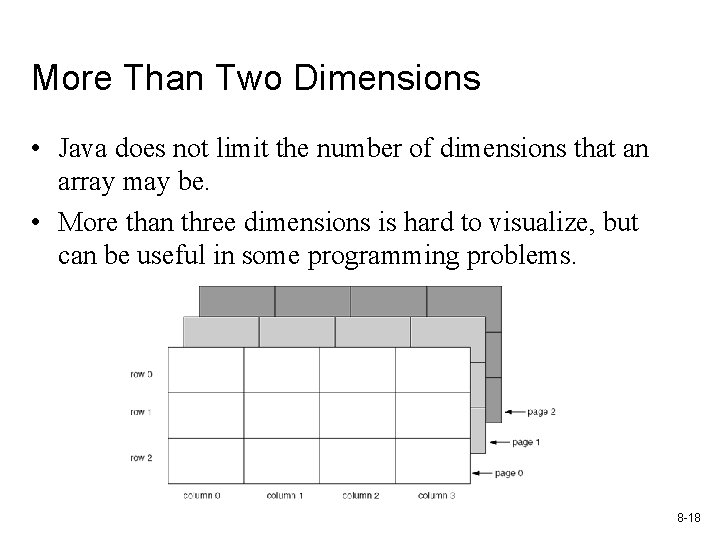
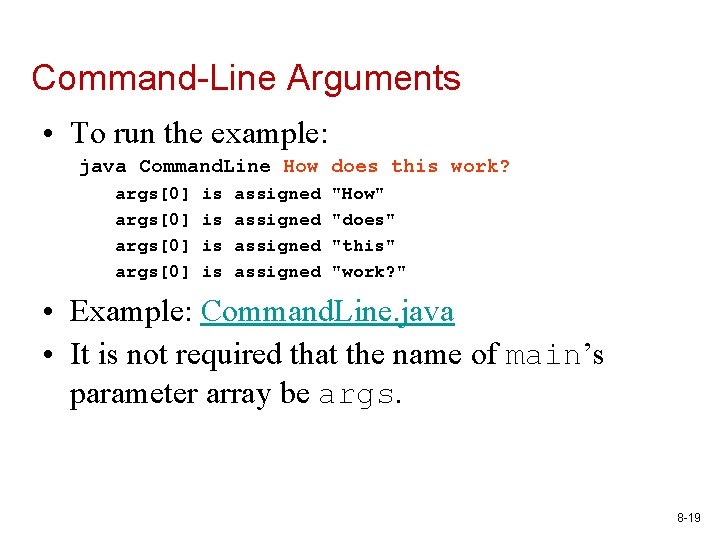
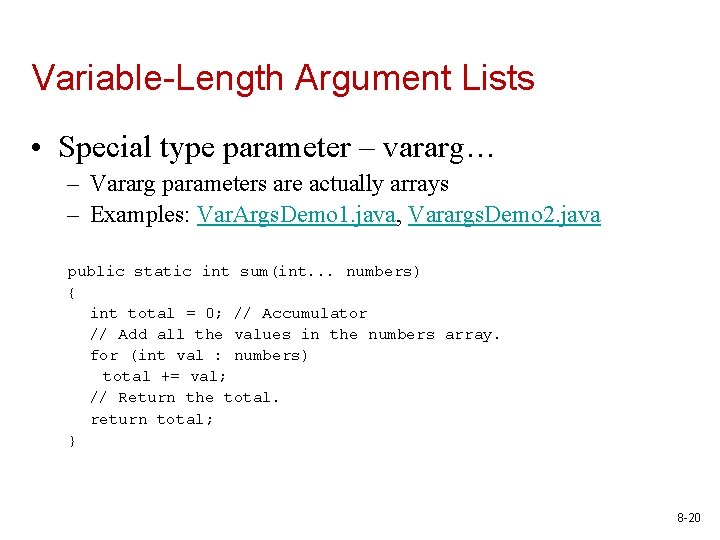
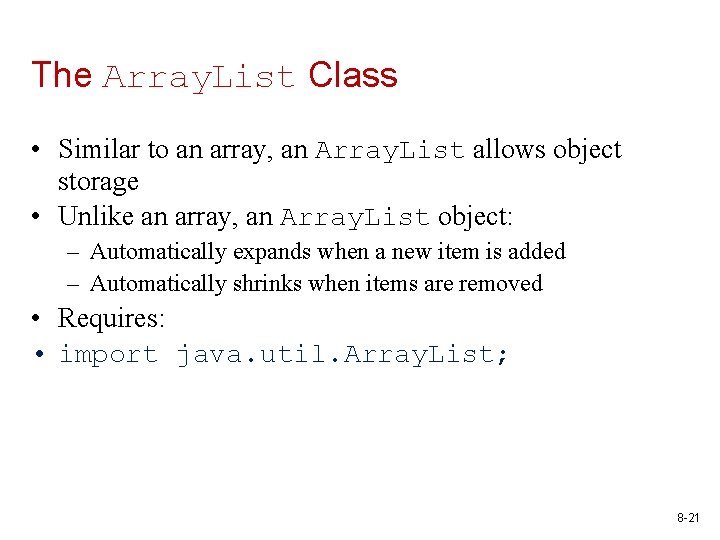
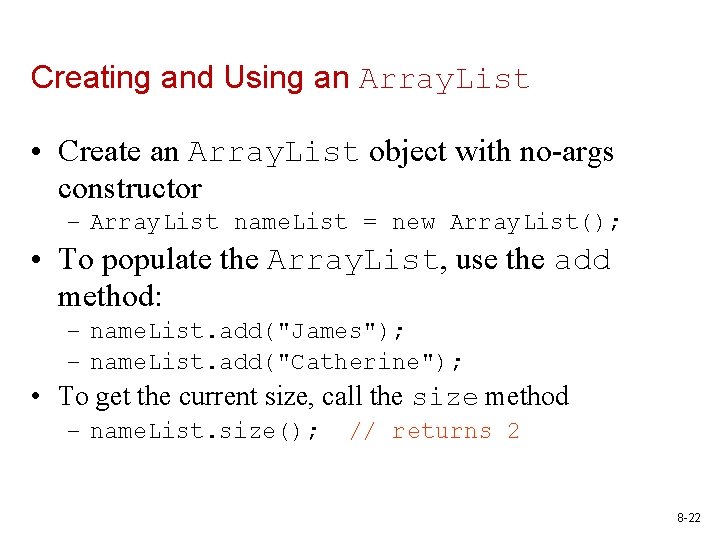
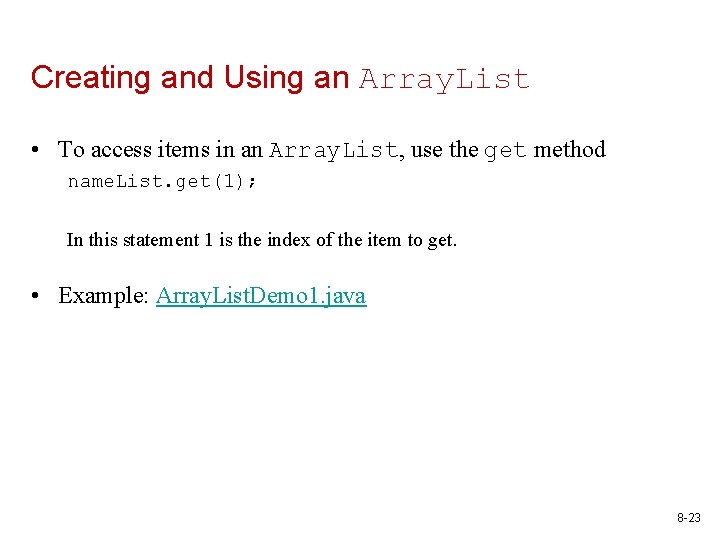
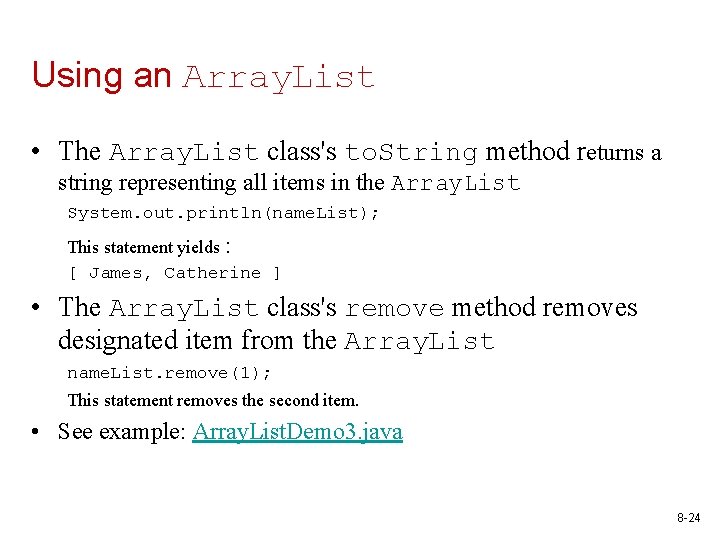
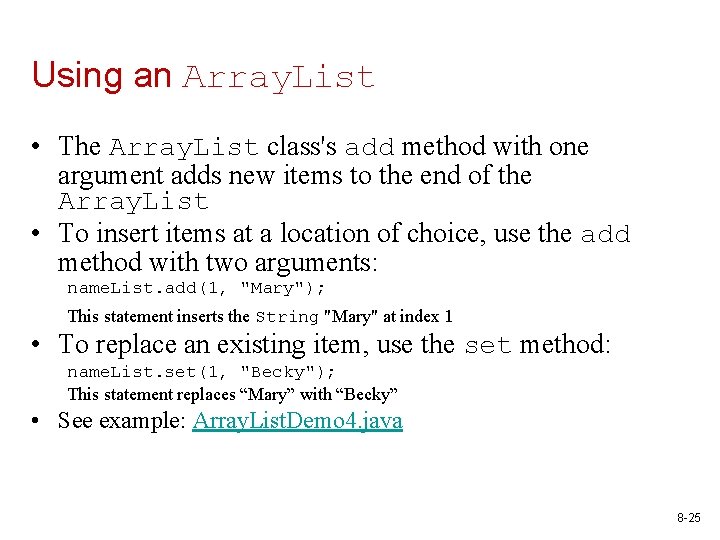
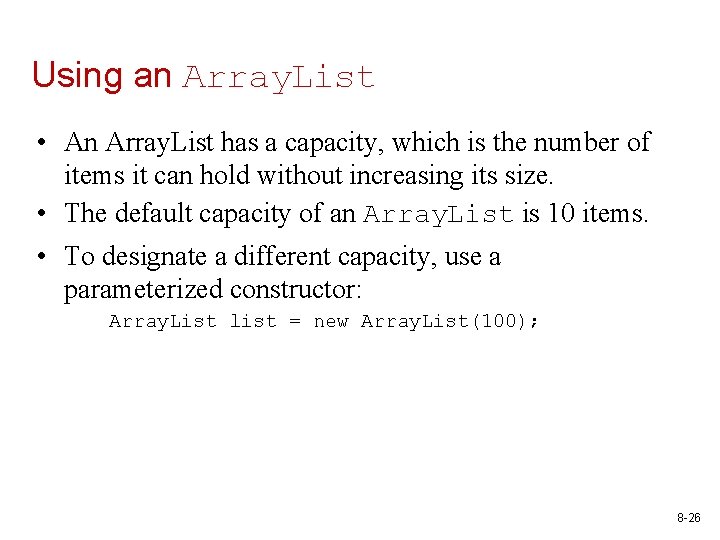
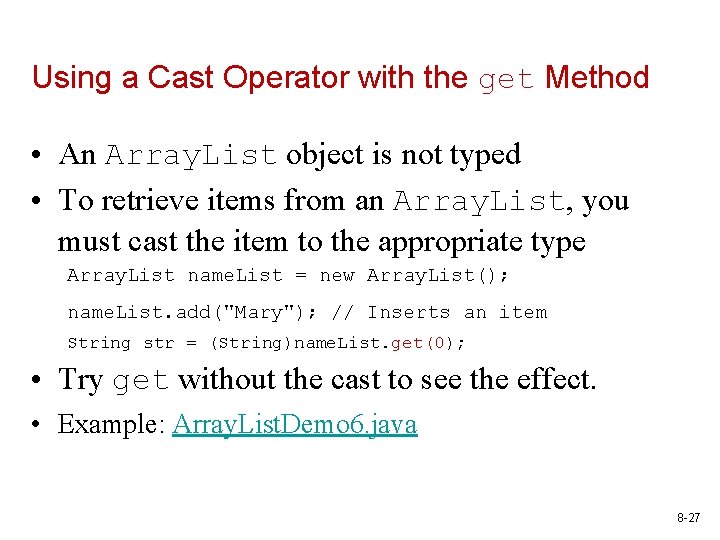
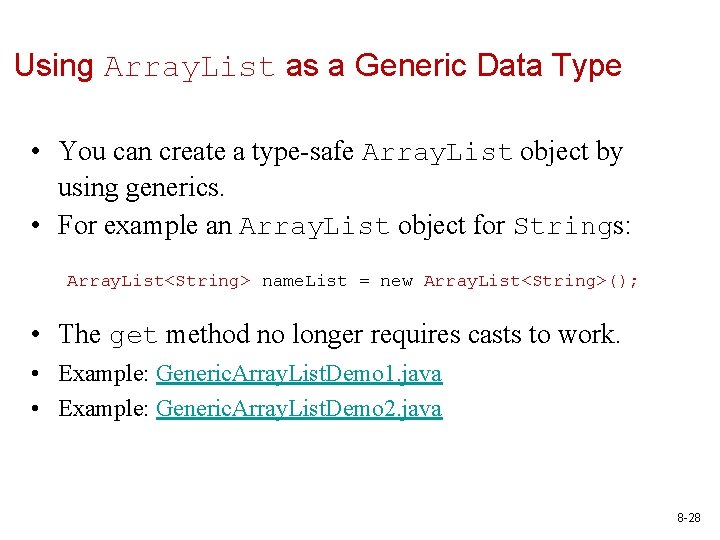
- Slides: 28
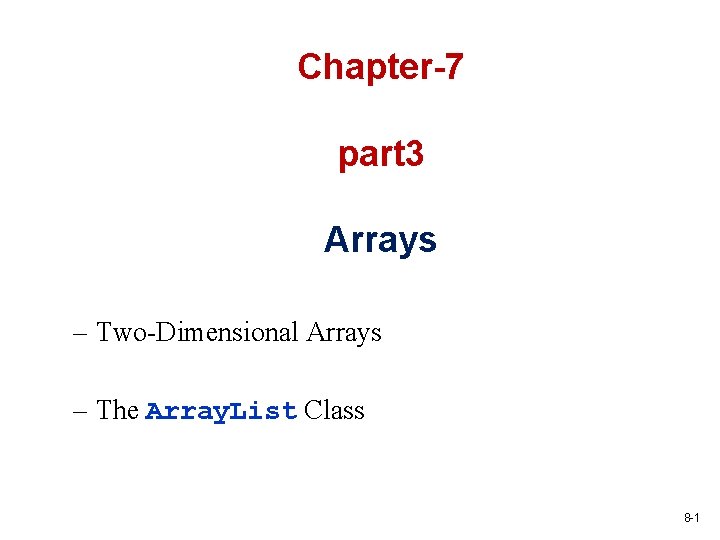
Chapter-7 part 3 Arrays – Two-Dimensional Arrays – The Array. List Class 8 -1
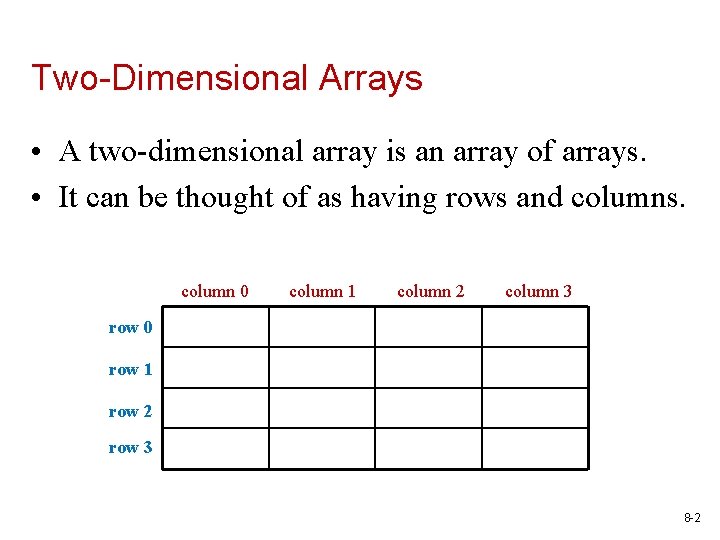
Two-Dimensional Arrays • A two-dimensional array is an array of arrays. • It can be thought of as having rows and columns. column 0 column 1 column 2 column 3 row 0 row 1 row 2 row 3 8 -2
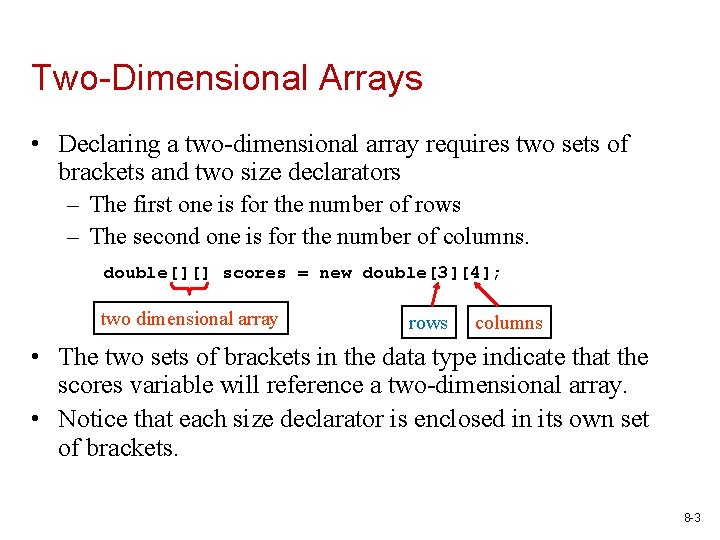
Two-Dimensional Arrays • Declaring a two-dimensional array requires two sets of brackets and two size declarators – The first one is for the number of rows – The second one is for the number of columns. double[][] scores = new double[3][4]; two dimensional array rows columns • The two sets of brackets in the data type indicate that the scores variable will reference a two-dimensional array. • Notice that each size declarator is enclosed in its own set of brackets. 8 -3
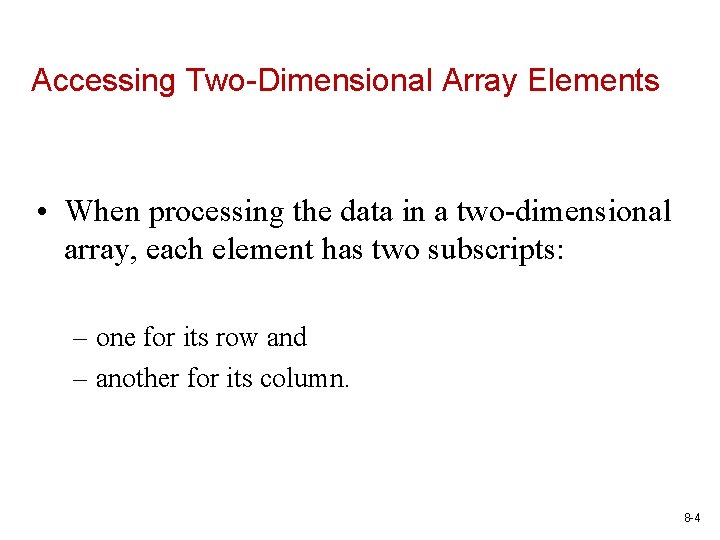
Accessing Two-Dimensional Array Elements • When processing the data in a two-dimensional array, each element has two subscripts: – one for its row and – another for its column. 8 -4
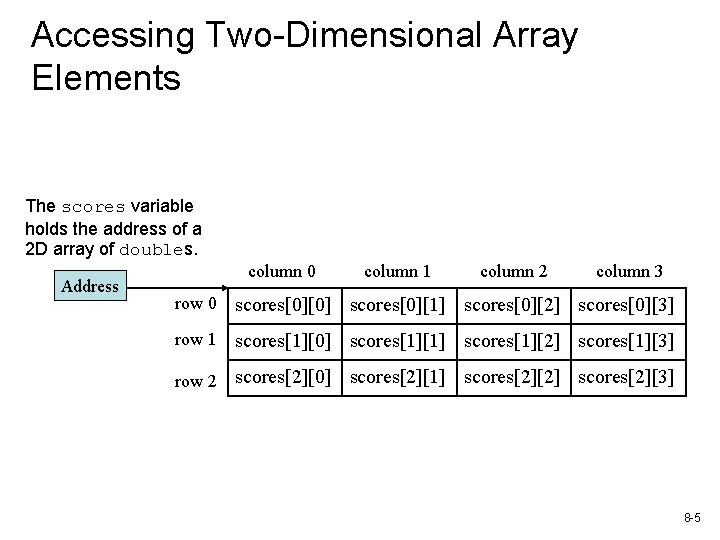
Accessing Two-Dimensional Array Elements The scores variable holds the address of a 2 D array of doubles. Address column 0 column 1 column 2 column 3 row 0 scores[0][0] scores[0][1] scores[0][2] scores[0][3] row 1 scores[1][0] scores[1][1] scores[1][2] scores[1][3] row 2 scores[2][0] scores[2][1] scores[2][2] scores[2][3] 8 -5
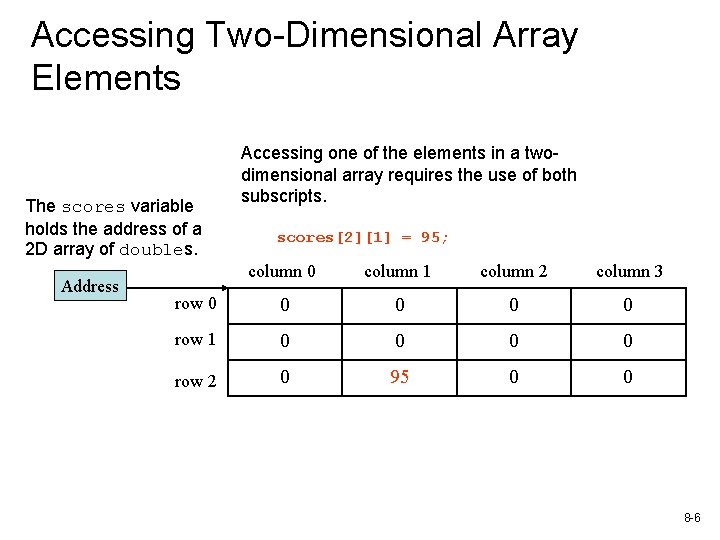
Accessing Two-Dimensional Array Elements The scores variable holds the address of a 2 D array of doubles. Address Accessing one of the elements in a twodimensional array requires the use of both subscripts. scores[2][1] = 95; column 0 column 1 column 2 column 3 row 0 0 0 row 1 0 0 row 2 0 95 0 0 8 -6
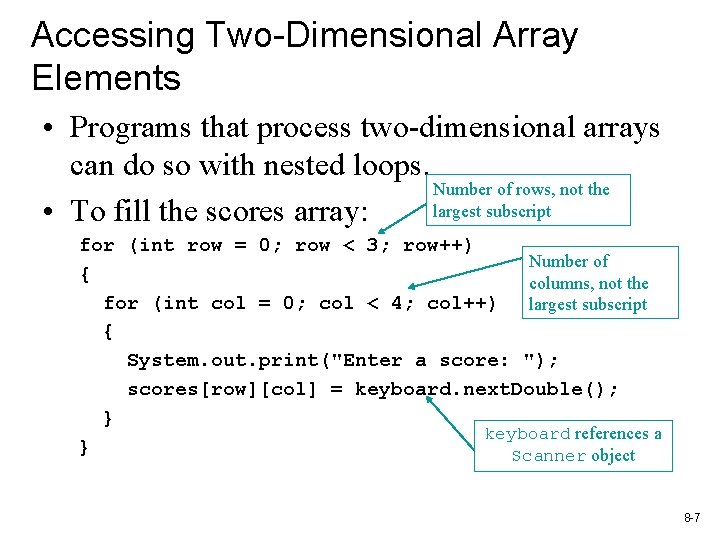
Accessing Two-Dimensional Array Elements • Programs that process two-dimensional arrays can do so with nested loops. Number of rows, not the largest subscript • To fill the scores array: for (int row = 0; row < 3; row++) Number of { columns, not the for (int col = 0; col < 4; col++) largest subscript { System. out. print("Enter a score: "); scores[row][col] = keyboard. next. Double(); } keyboard references a } Scanner object 8 -7
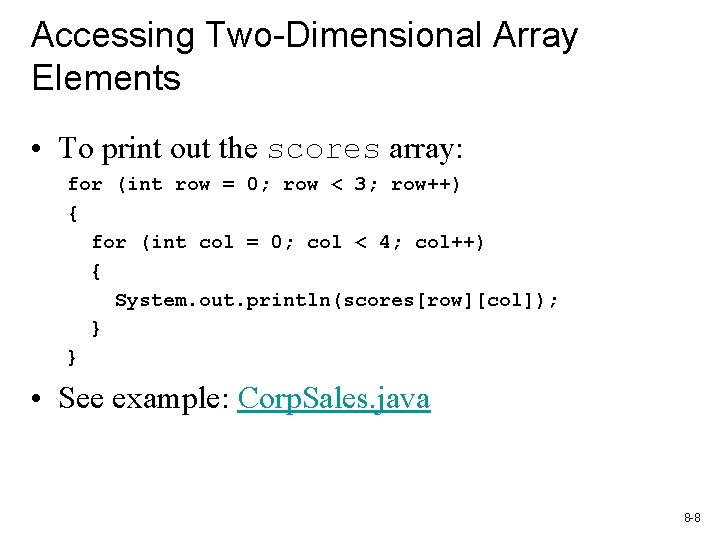
Accessing Two-Dimensional Array Elements • To print out the scores array: for (int row = 0; row < 3; row++) { for (int col = 0; col < 4; col++) { System. out. println(scores[row][col]); } } • See example: Corp. Sales. java 8 -8
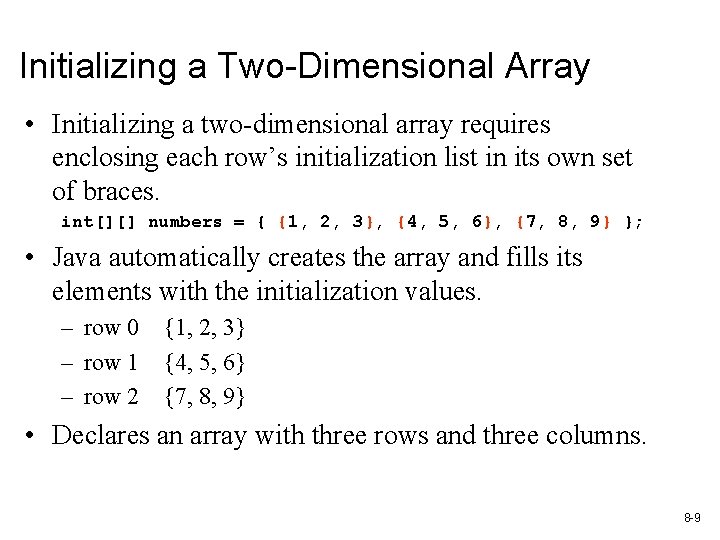
Initializing a Two-Dimensional Array • Initializing a two-dimensional array requires enclosing each row’s initialization list in its own set of braces. int[][] numbers = { {1, 2, 3}, {4, 5, 6}, {7, 8, 9} }; • Java automatically creates the array and fills its elements with the initialization values. – row 0 {1, 2, 3} – row 1 {4, 5, 6} – row 2 {7, 8, 9} • Declares an array with three rows and three columns. 8 -9
![Initializing a TwoDimensional Array int numbers 1 2 3 4 5 6 7 Initializing a Two-Dimensional Array int[][] numbers = {{1, 2, 3}, {4, 5, 6}, {7,](https://slidetodoc.com/presentation_image_h/0884650d2ff138e24ad545ce2b0266bf/image-10.jpg)
Initializing a Two-Dimensional Array int[][] numbers = {{1, 2, 3}, {4, 5, 6}, {7, 8, 9}}; The numbers variable holds the address of a 2 D array of int values. Address produces: column 0 column 1 column 2 row 0 1 2 3 row 1 4 5 6 row 2 7 8 9 8 -10
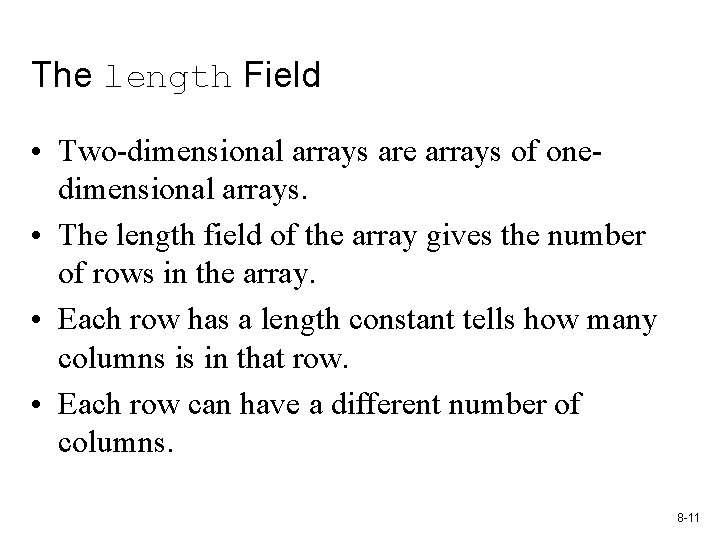
The length Field • Two-dimensional arrays are arrays of onedimensional arrays. • The length field of the array gives the number of rows in the array. • Each row has a length constant tells how many columns is in that row. • Each row can have a different number of columns. 8 -11
![The length Field To access the length fields of the array int numbers The length Field • To access the length fields of the array: int[][] numbers](https://slidetodoc.com/presentation_image_h/0884650d2ff138e24ad545ce2b0266bf/image-12.jpg)
The length Field • To access the length fields of the array: int[][] numbers = { { 1, 2, 3, 4 }, { 5, 6, 7 }, { 9, 10, 11, 12 } }; for (int row = 0; row < numbers. length; row++) { for (int col = 0; col < numbers[row]. length; col++) System. out. println(numbers[row][col]); } Number of rows Number of columns in this row. • See example: Lengths. java The array can have variable length rows. 8 -12
![Summing The Elements of a Two Dimensional Array int numbers 1 Summing The Elements of a Two. Dimensional Array int[][] numbers = { { 1,](https://slidetodoc.com/presentation_image_h/0884650d2ff138e24ad545ce2b0266bf/image-13.jpg)
Summing The Elements of a Two. Dimensional Array int[][] numbers = { { 1, 2, 3, 4 }, {5, 6, 7, 8}, {9, 10, 11, 12} }; int total; total = 0; for (int row = 0; row < numbers. length; row++) { for (int col = 0; col < numbers[row]. length; col++) total += numbers[row][col]; } System. out. println("The total is " + total); 8 -13
![Summing The Rows of a Two Dimensional Array int numbers 1 2 Summing The Rows of a Two. Dimensional Array int[][] numbers = {{ 1, 2,](https://slidetodoc.com/presentation_image_h/0884650d2ff138e24ad545ce2b0266bf/image-14.jpg)
Summing The Rows of a Two. Dimensional Array int[][] numbers = {{ 1, 2, 3, 4}, {5, 6, 7, 8}, {9, 10, 11, 12}}; int total; for (int row = 0; row < numbers. length; row++) { total = 0; for (int col = 0; col < numbers[row]. length; col++) total += numbers[row][col]; System. out. println("Total of row " + row + " is " + total); } 8 -14
![Summing The Columns of a Two Dimensional Array int numbers 1 2 3 Summing The Columns of a Two. Dimensional Array int[][] numbers = {{1, 2, 3,](https://slidetodoc.com/presentation_image_h/0884650d2ff138e24ad545ce2b0266bf/image-15.jpg)
Summing The Columns of a Two. Dimensional Array int[][] numbers = {{1, 2, 3, 4}, {5, 6, 7, 8}, {9, 10, 11, 12}}; int total; for (int col = 0; col < numbers[0]. length; col++) { total = 0; for (int row = 0; row < numbers. length; row++) total += numbers[row][col]; System. out. println("Total of column " + col + " is " + total); } 8 -15
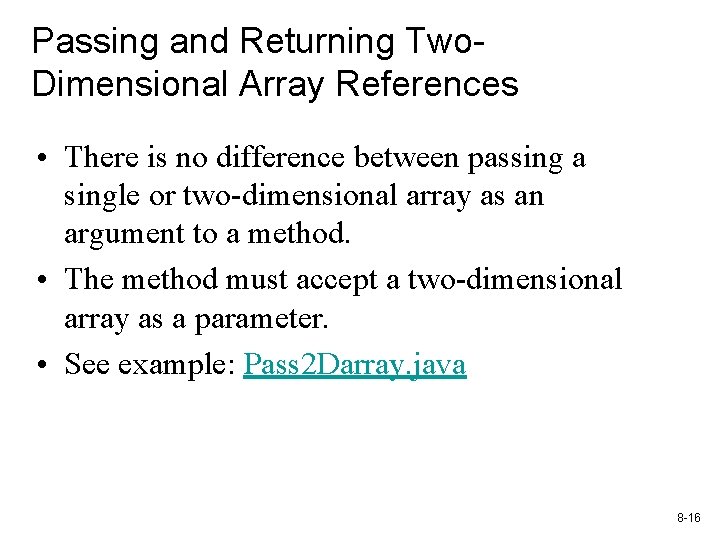
Passing and Returning Two. Dimensional Array References • There is no difference between passing a single or two-dimensional array as an argument to a method. • The method must accept a two-dimensional array as a parameter. • See example: Pass 2 Darray. java 8 -16
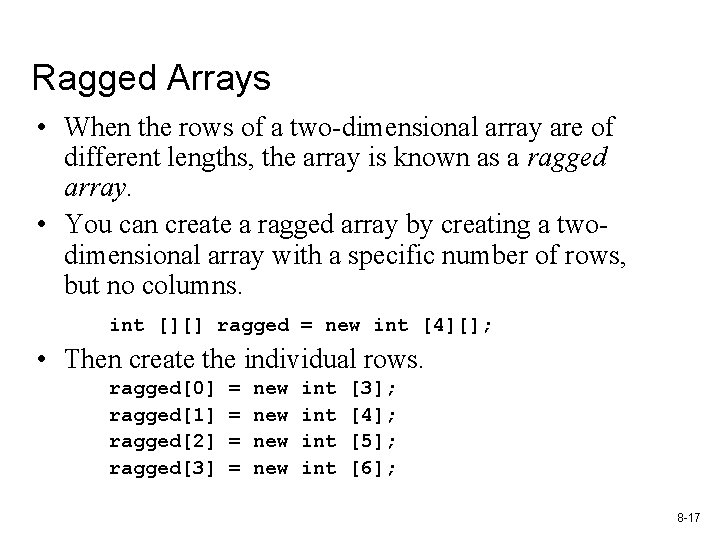
Ragged Arrays • When the rows of a two-dimensional array are of different lengths, the array is known as a ragged array. • You can create a ragged array by creating a twodimensional array with a specific number of rows, but no columns. int [][] ragged = new int [4][]; • Then create the individual rows. ragged[0] ragged[1] ragged[2] ragged[3] = = new new int int [3]; [4]; [5]; [6]; 8 -17
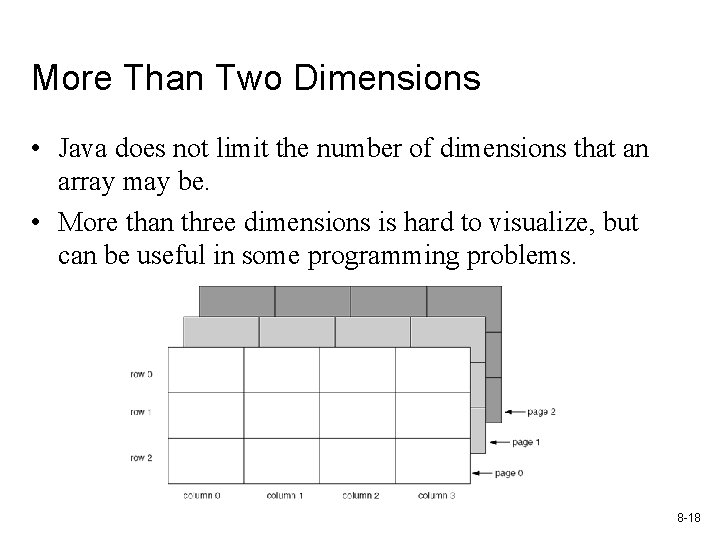
More Than Two Dimensions • Java does not limit the number of dimensions that an array may be. • More than three dimensions is hard to visualize, but can be useful in some programming problems. 8 -18
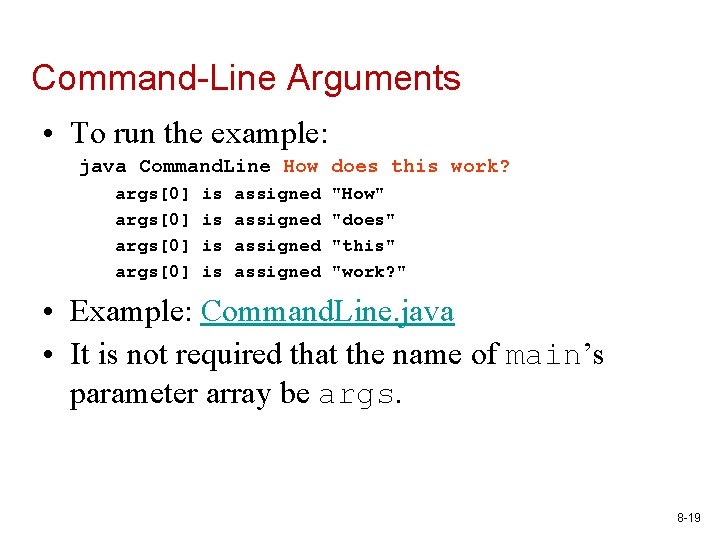
Command-Line Arguments • To run the example: java Command. Line How does this work? args[0] is is assigned "How" "does" "this" "work? " • Example: Command. Line. java • It is not required that the name of main’s parameter array be args. 8 -19
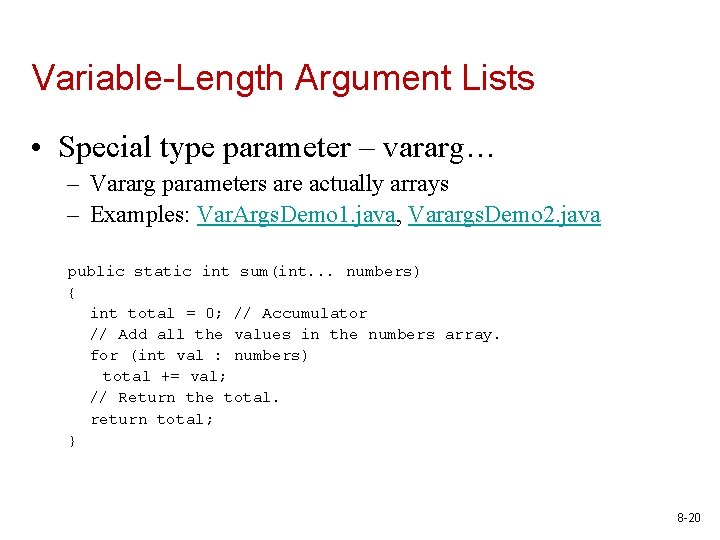
Variable-Length Argument Lists • Special type parameter – vararg… – Vararg parameters are actually arrays – Examples: Var. Args. Demo 1. java, Varargs. Demo 2. java public static int sum(int. . . numbers) { int total = 0; // Accumulator // Add all the values in the numbers array. for (int val : numbers) total += val; // Return the total. return total; } 8 -20
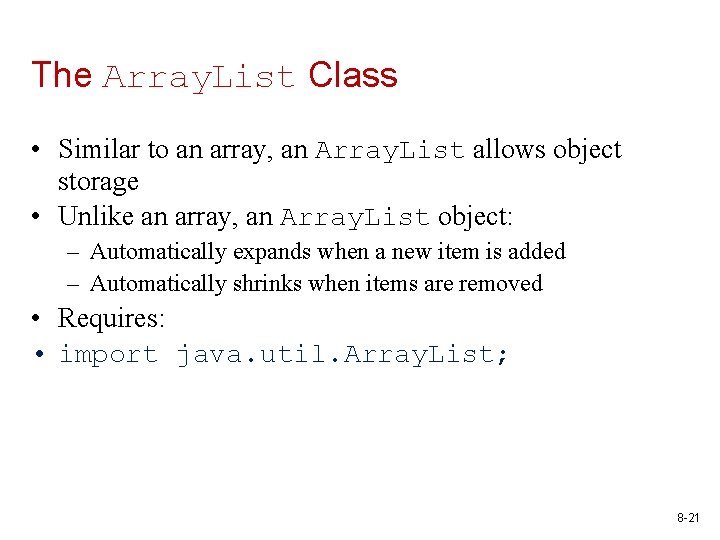
The Array. List Class • Similar to an array, an Array. List allows object storage • Unlike an array, an Array. List object: – Automatically expands when a new item is added – Automatically shrinks when items are removed • Requires: • import java. util. Array. List; 8 -21
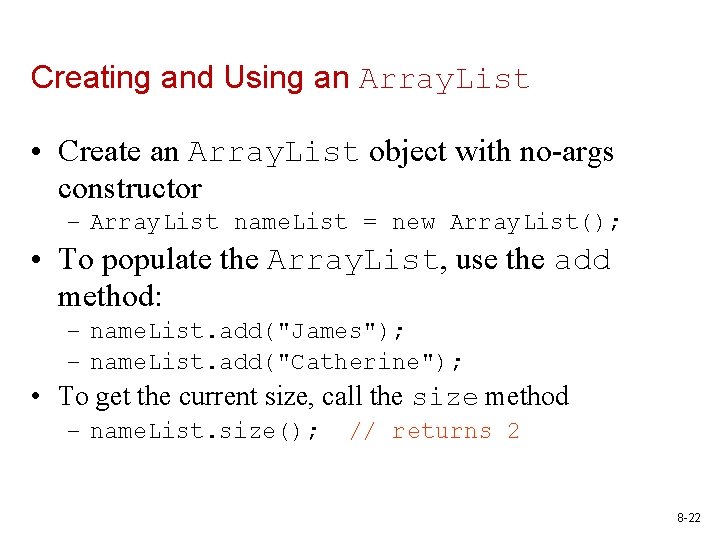
Creating and Using an Array. List • Create an Array. List object with no-args constructor – Array. List name. List = new Array. List(); • To populate the Array. List, use the add method: – name. List. add("James"); – name. List. add("Catherine"); • To get the current size, call the size method – name. List. size(); // returns 2 8 -22
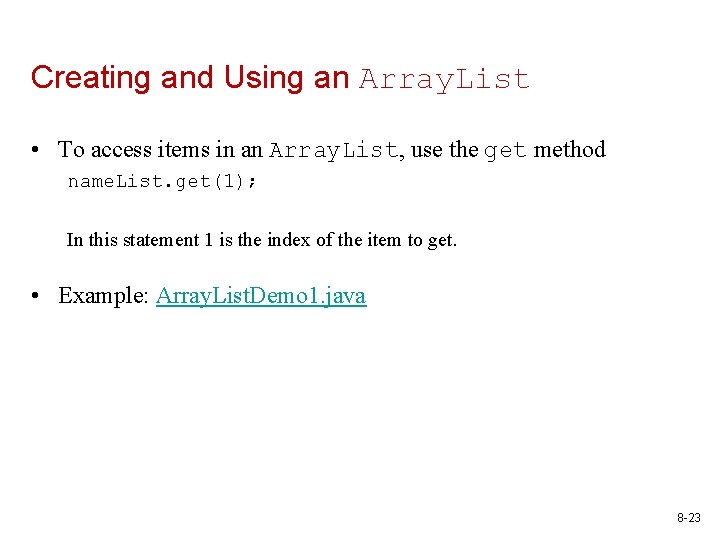
Creating and Using an Array. List • To access items in an Array. List, use the get method name. List. get(1); In this statement 1 is the index of the item to get. • Example: Array. List. Demo 1. java 8 -23
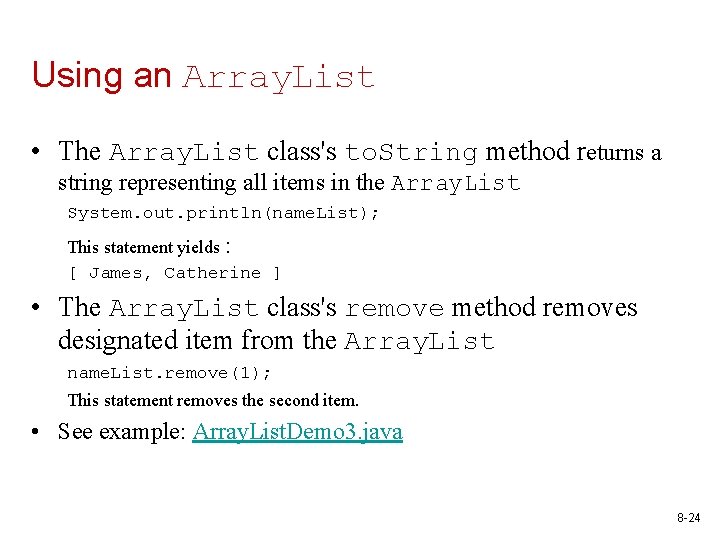
Using an Array. List • The Array. List class's to. String method returns a string representing all items in the Array. List System. out. println(name. List); This statement yields : [ James, Catherine ] • The Array. List class's remove method removes designated item from the Array. List name. List. remove(1); This statement removes the second item. • See example: Array. List. Demo 3. java 8 -24
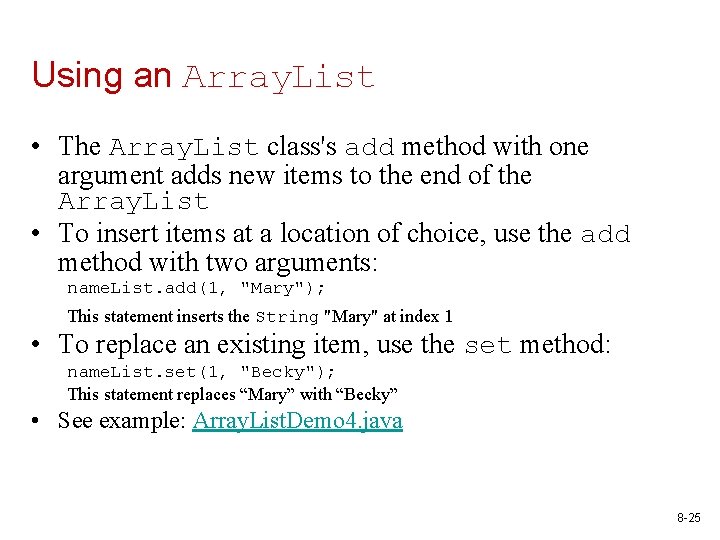
Using an Array. List • The Array. List class's add method with one argument adds new items to the end of the Array. List • To insert items at a location of choice, use the add method with two arguments: name. List. add(1, "Mary"); This statement inserts the String "Mary" at index 1 • To replace an existing item, use the set method: name. List. set(1, "Becky"); This statement replaces “Mary” with “Becky” • See example: Array. List. Demo 4. java 8 -25
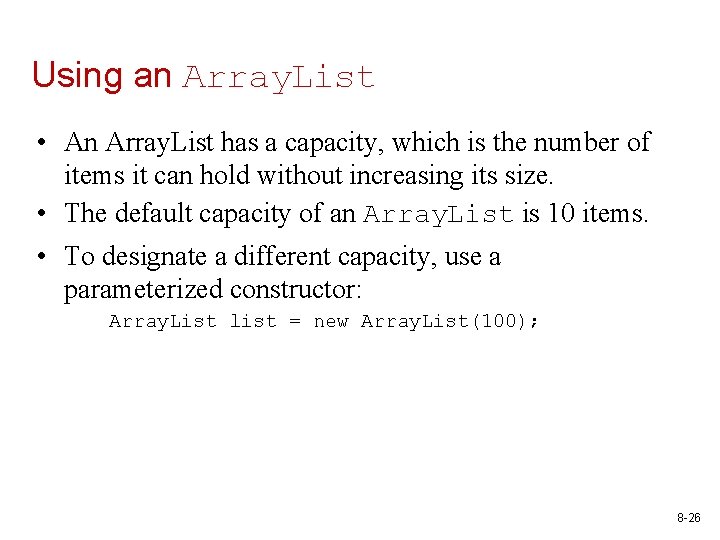
Using an Array. List • An Array. List has a capacity, which is the number of items it can hold without increasing its size. • The default capacity of an Array. List is 10 items. • To designate a different capacity, use a parameterized constructor: Array. List list = new Array. List(100); 8 -26
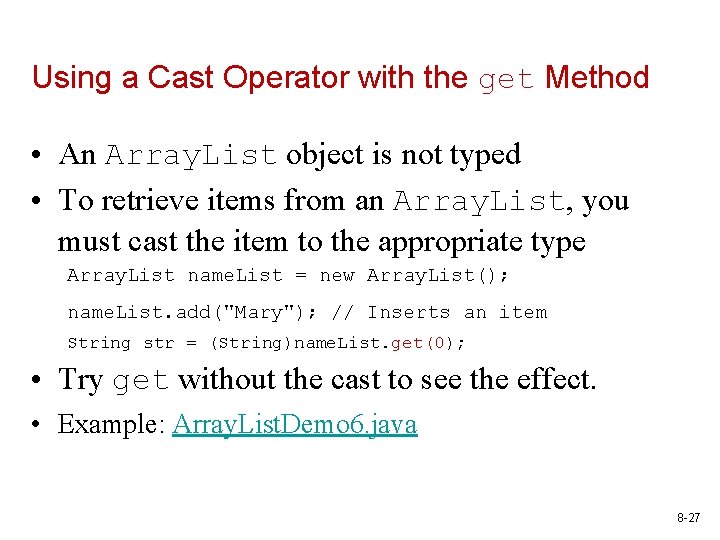
Using a Cast Operator with the get Method • An Array. List object is not typed • To retrieve items from an Array. List, you must cast the item to the appropriate type Array. List name. List = new Array. List(); name. List. add("Mary"); // Inserts an item String str = (String)name. List. get(0); • Try get without the cast to see the effect. • Example: Array. List. Demo 6. java 8 -27
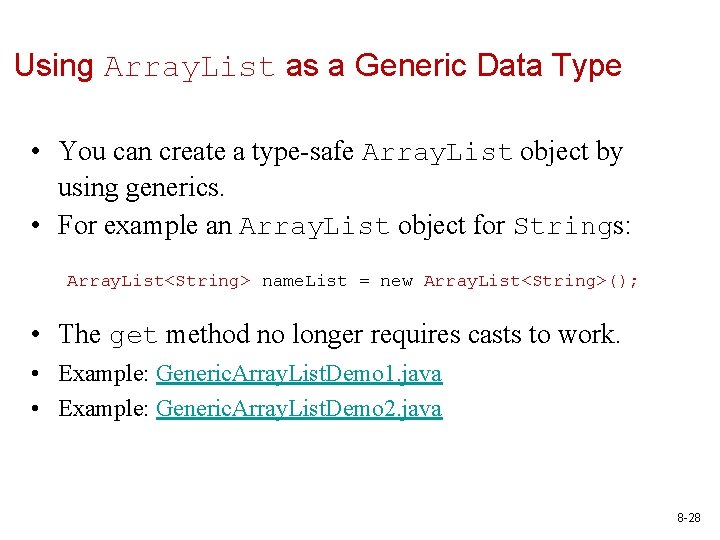
Using Array. List as a Generic Data Type • You can create a type-safe Array. List object by using generics. • For example an Array. List object for Strings: Array. List<String> name. List = new Array. List<String>(); • The get method no longer requires casts to work. • Example: Generic. Array. List. Demo 1. java • Example: Generic. Array. List. Demo 2. java 8 -28
Array of arrays c++
Jagged array
Array yang sangat banyak elemen nol-nya
Associative array vs indexed array
Contoh program array 3 dimensi python
Pin grid array and land grid array
Comparison between broadside array and endfire array
Photovoltaic array maximum power point tracking array
Array dimensi banyak
Larik
Array in mips
Parallel arrays in data structure
Partially filled array
Computer science arrays
Dynamic arrays and amortized analysis
Why do we need arrays?
Day 3: arrays
Advantages and disadvantages of arrays in data structure
Polynomial representation using array in c
Microled arrays
Redundancy array of independent disk
Ragged array
Searching and sorting arrays in c++
Que es un arreglo unidimensional en java
Basics of raid
How many arrays in 24
Creating arrays matlab
Arrays in arm assembly
Are vectors dynamic arrays