Arrays ARRAYS 2 D ARRAY APPLICATIONS DYNAMIC ARRAYS
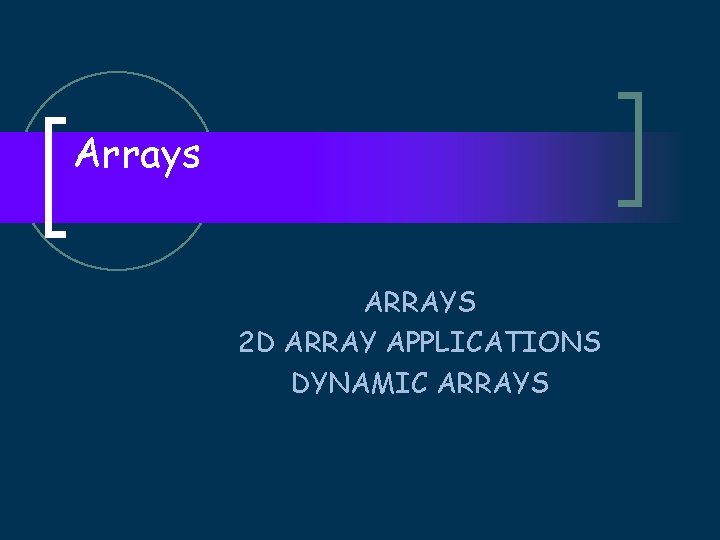
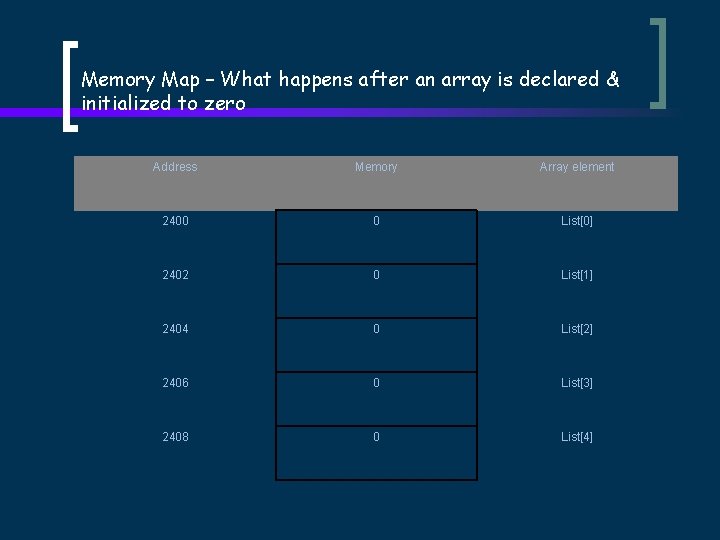
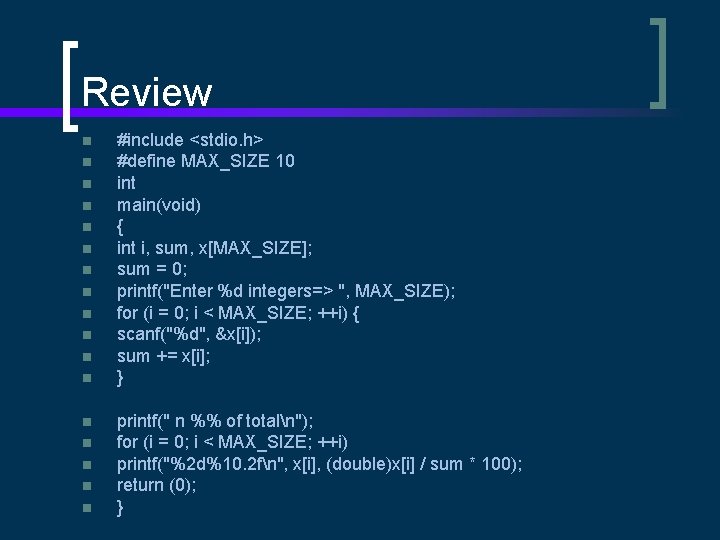
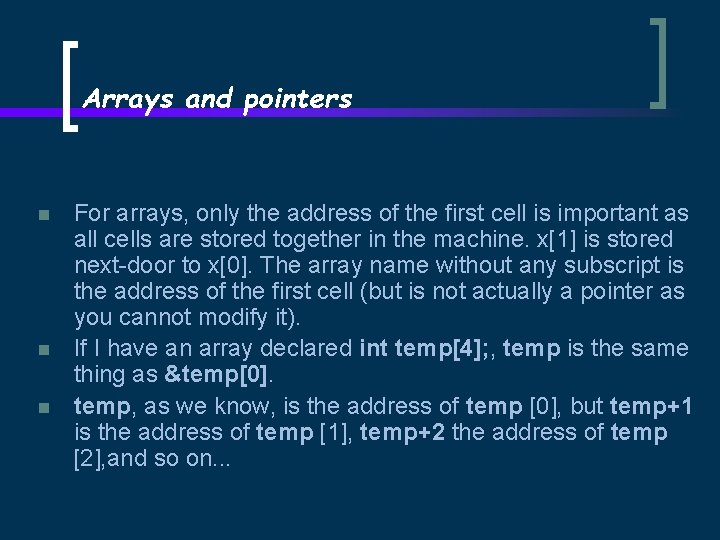
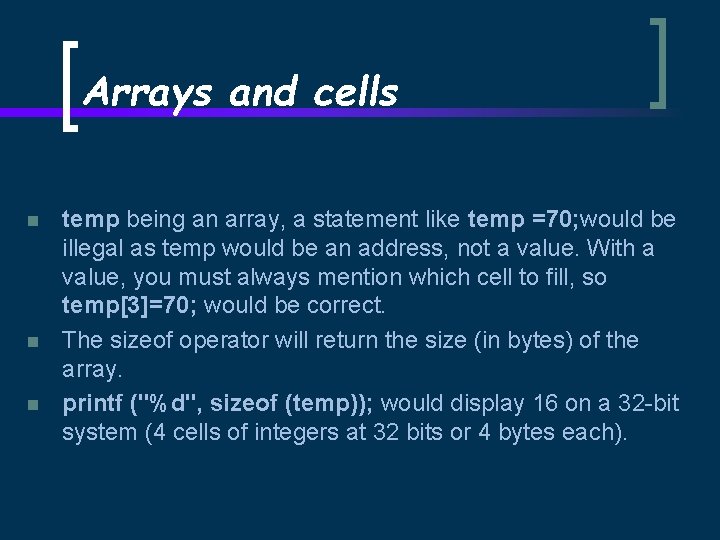
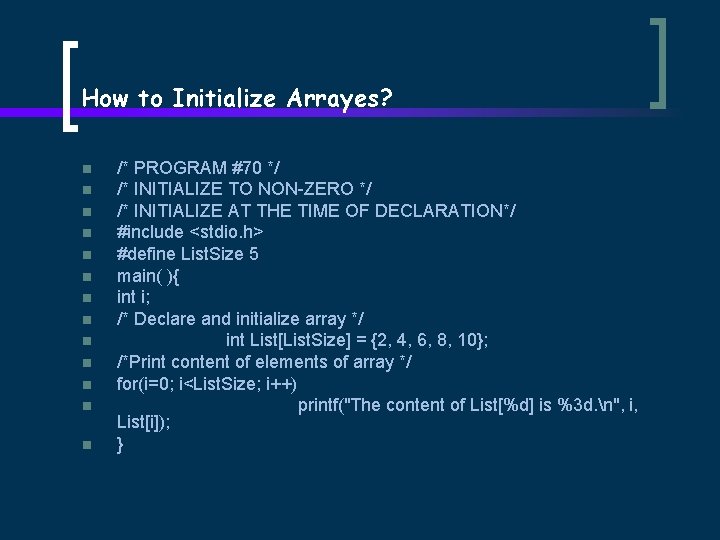
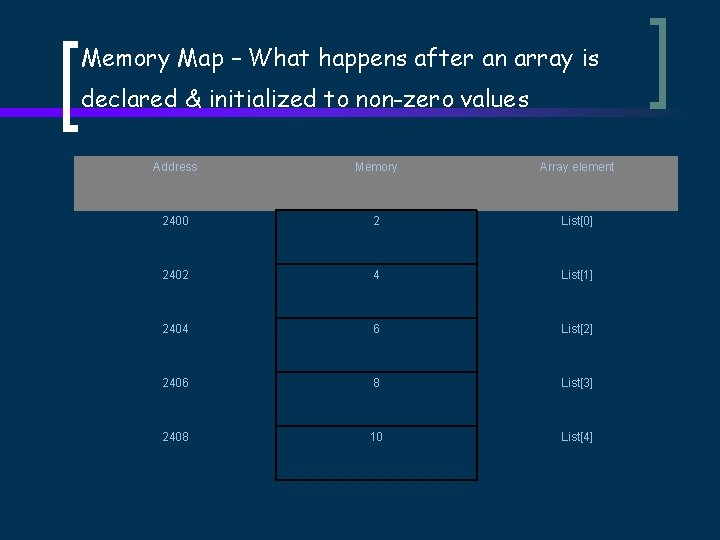
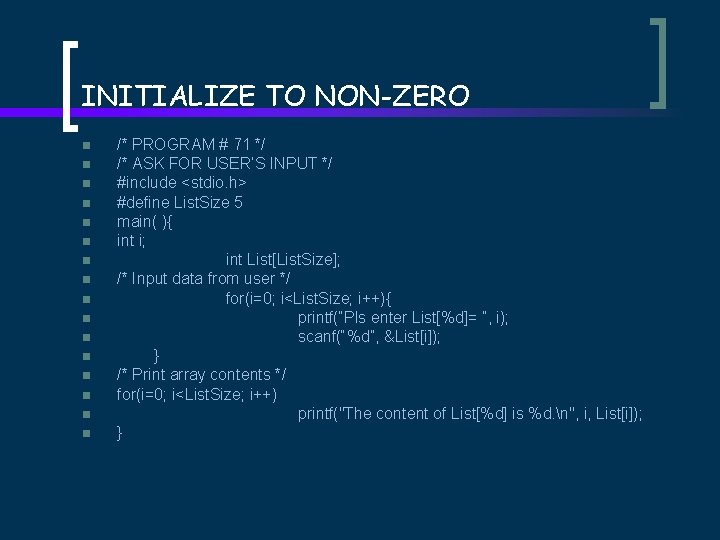
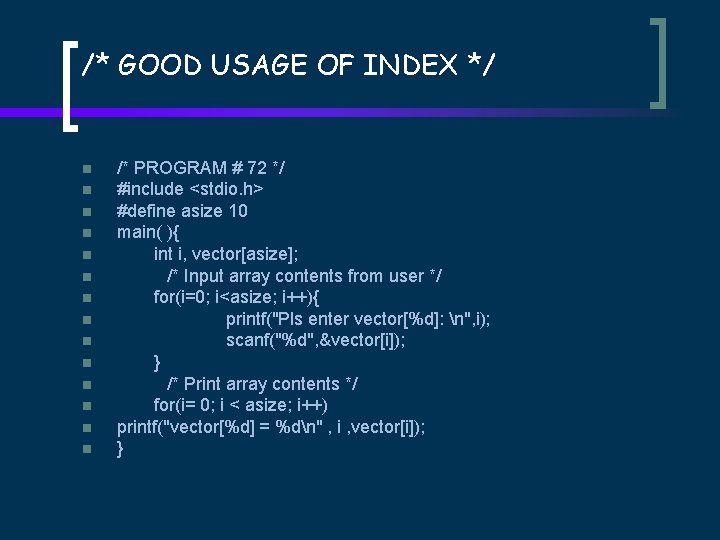
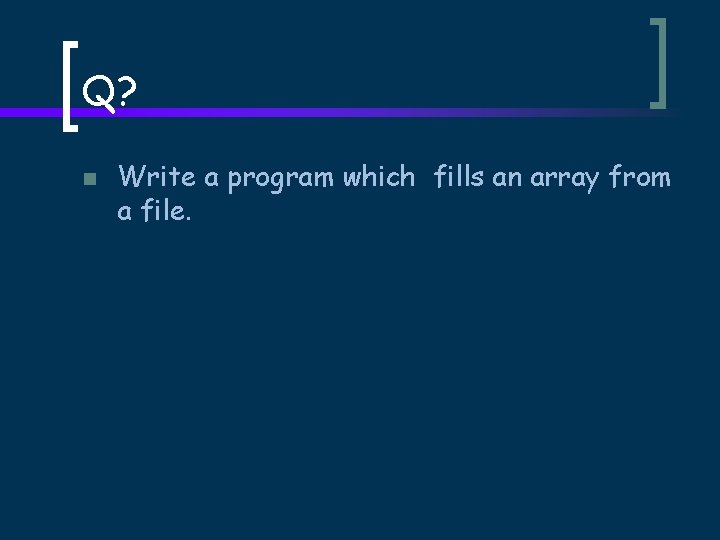
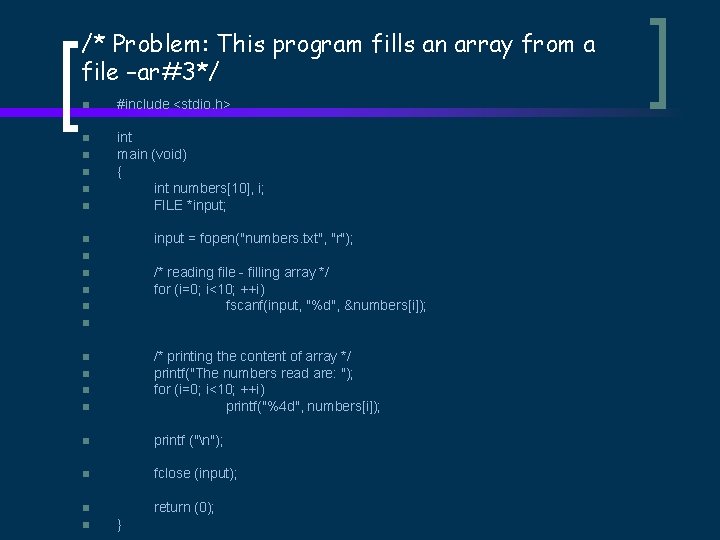
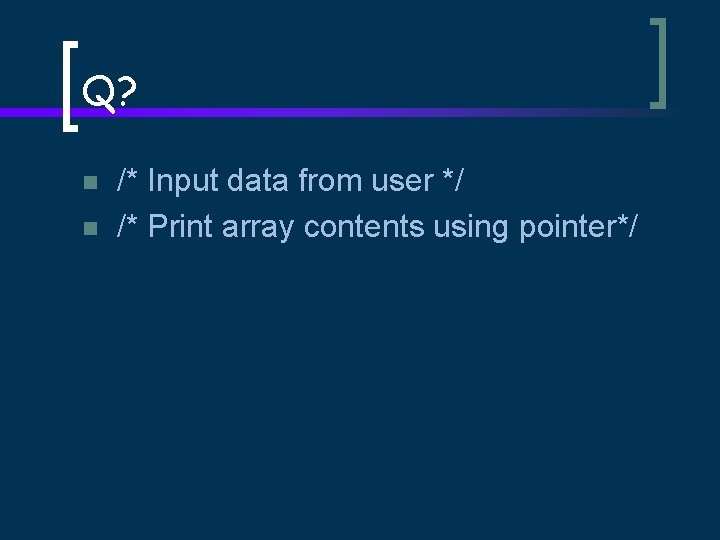
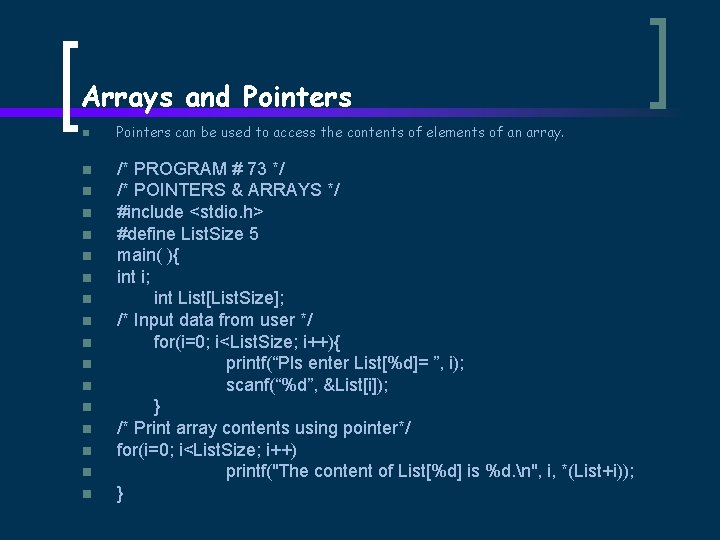
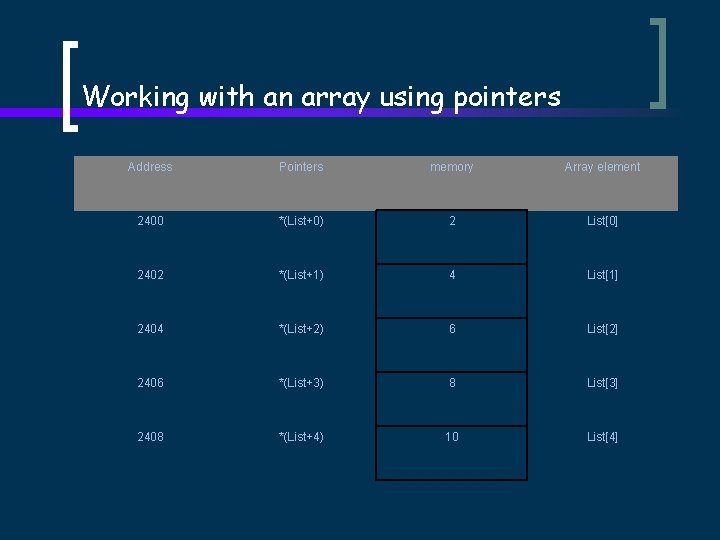
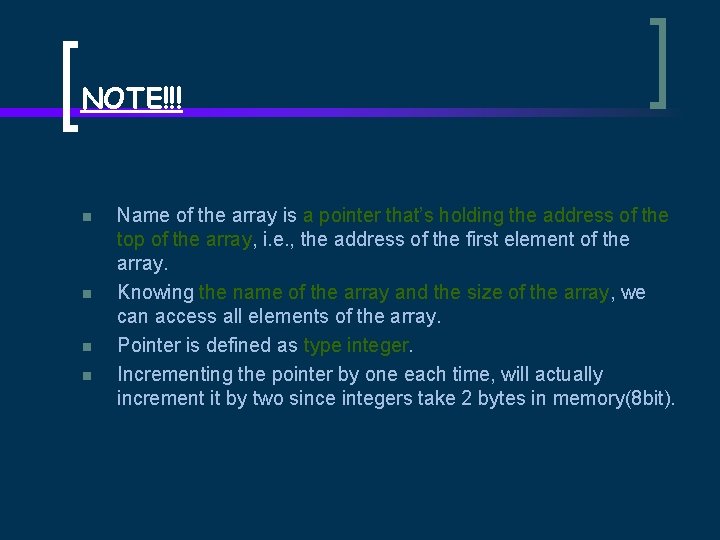
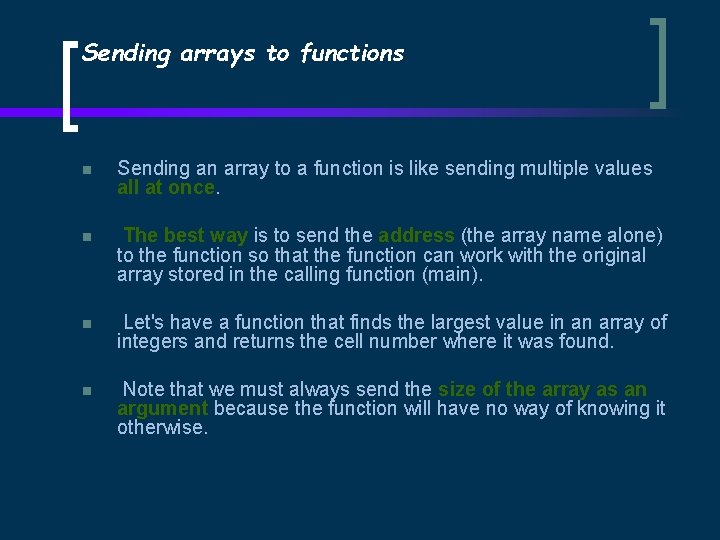
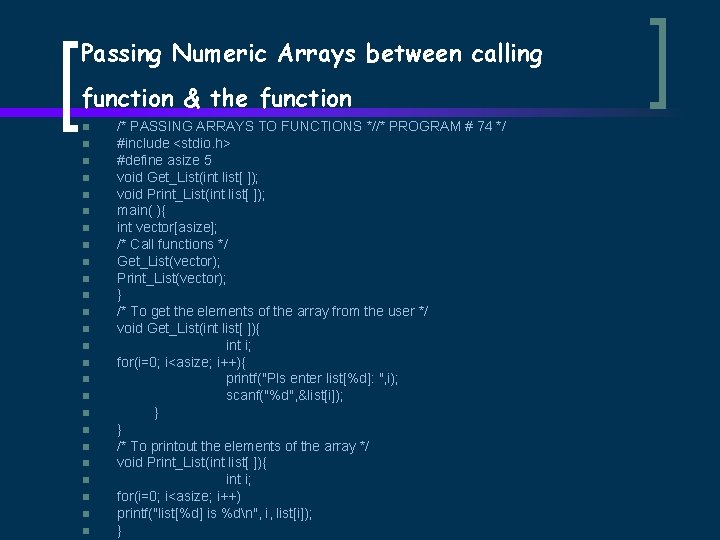
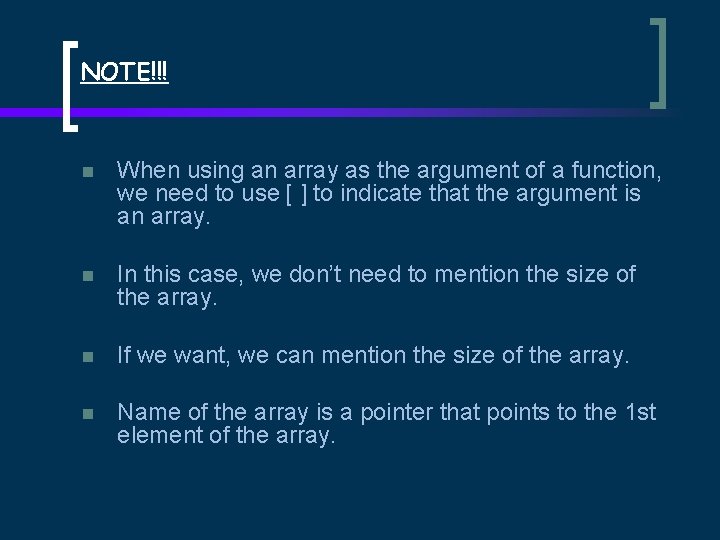
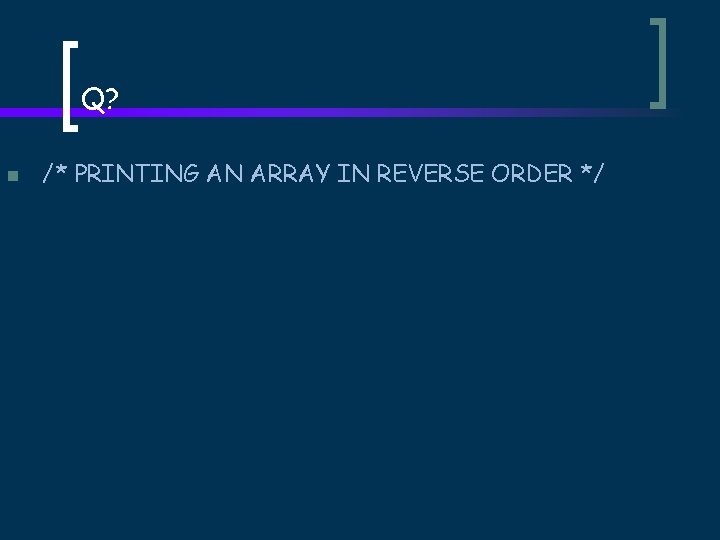
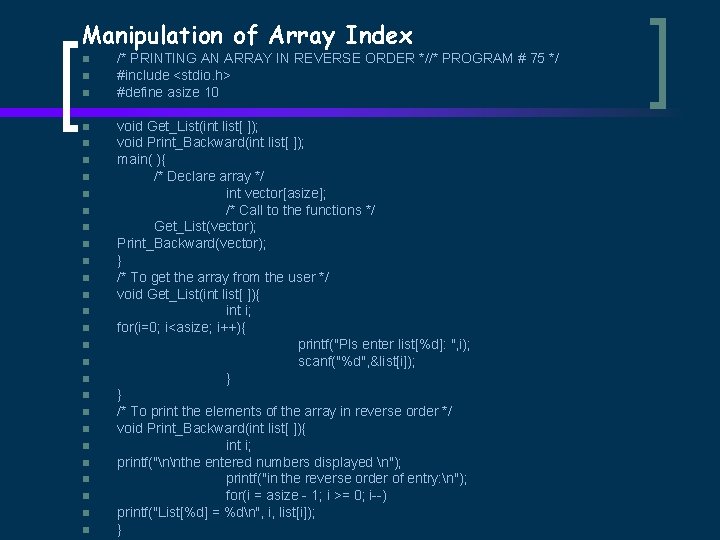
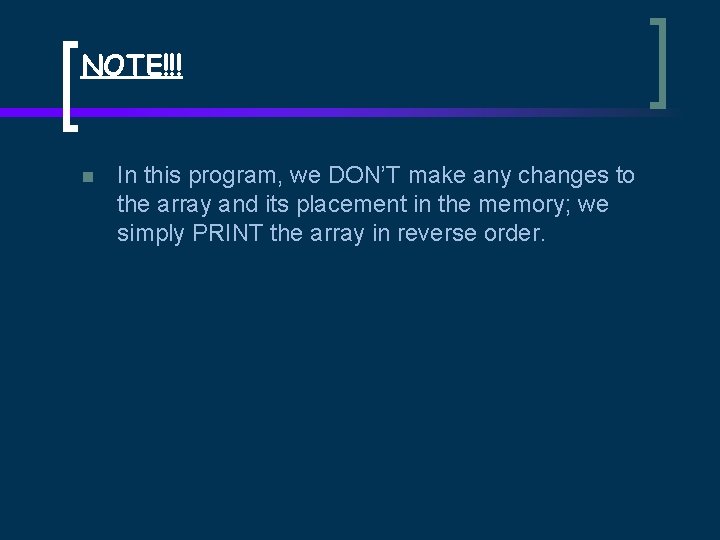
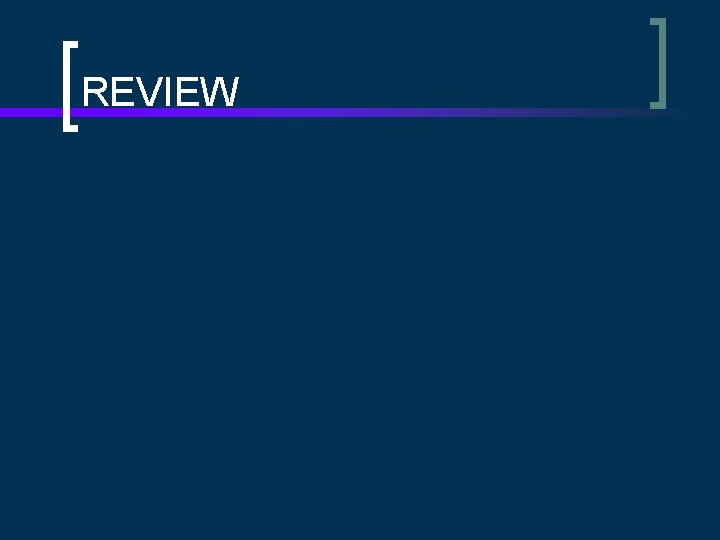
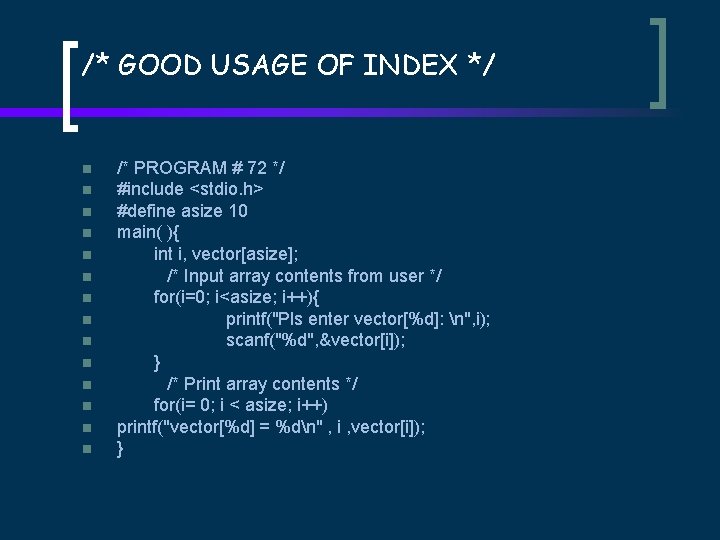
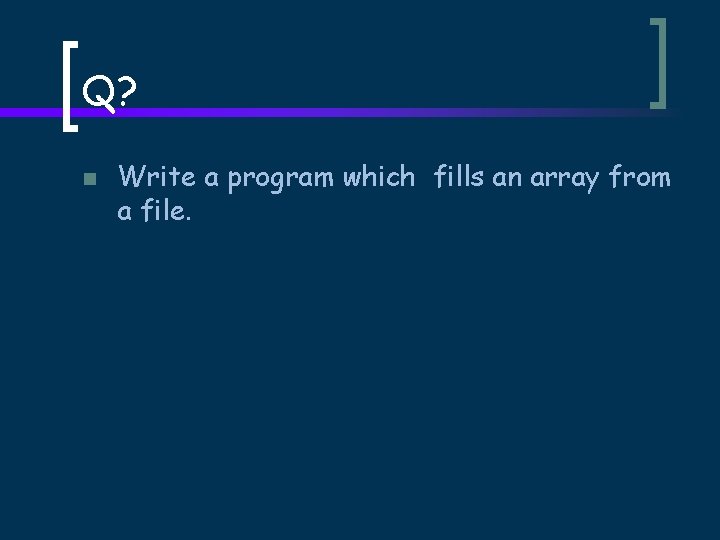
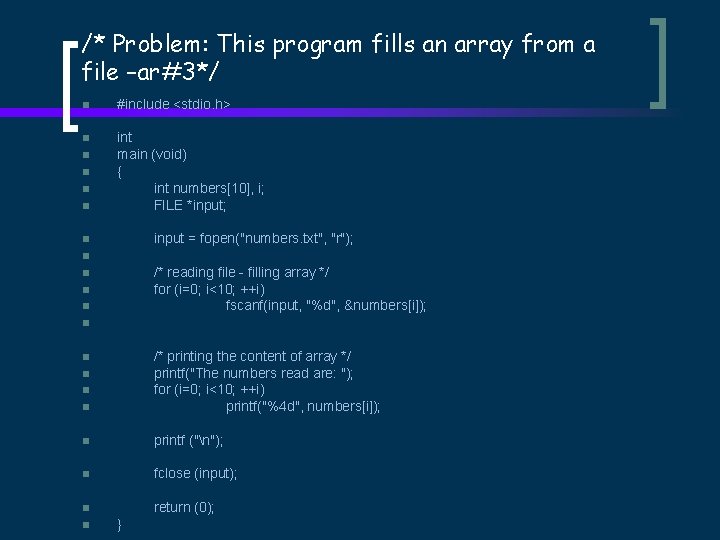
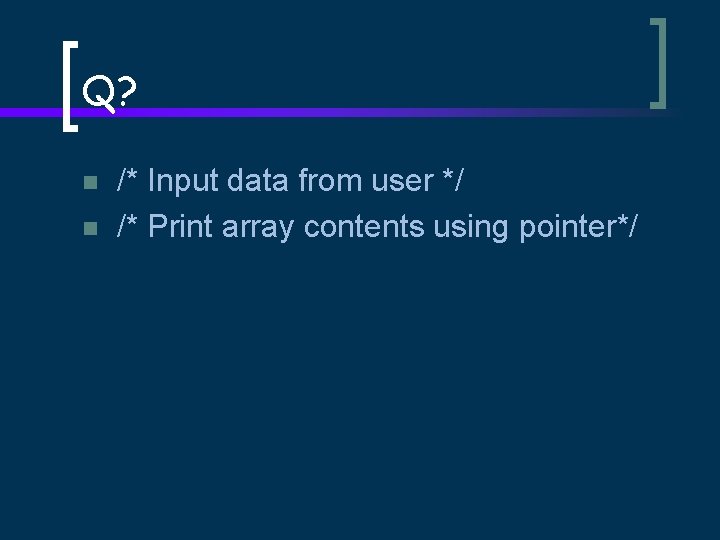
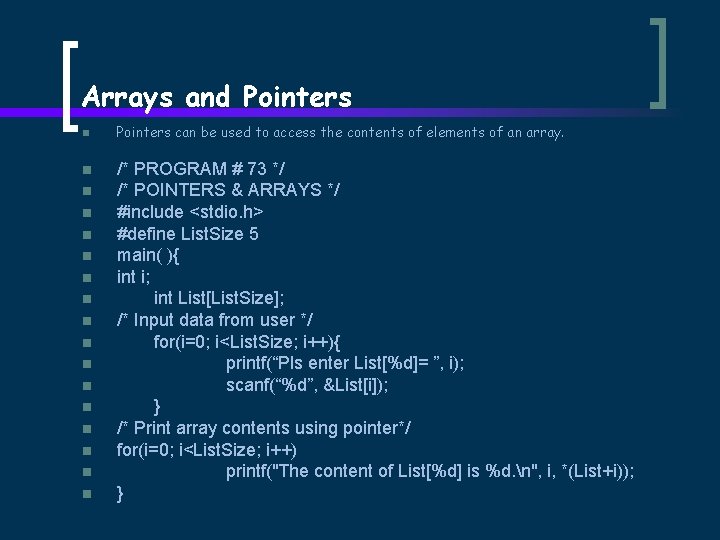
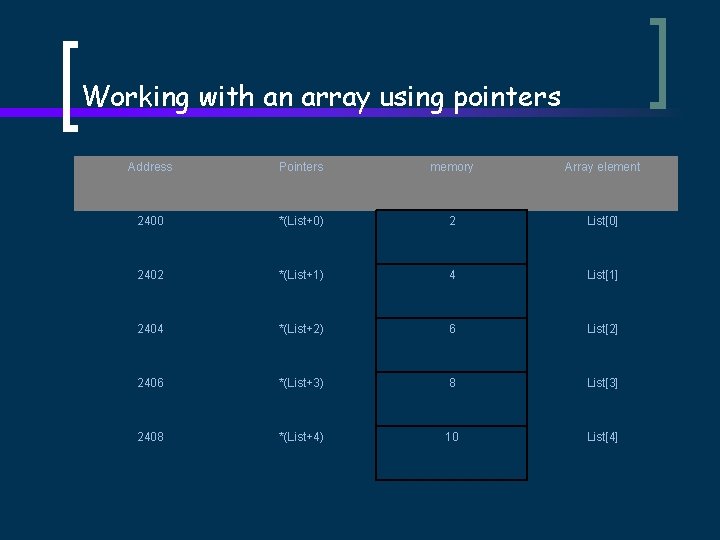
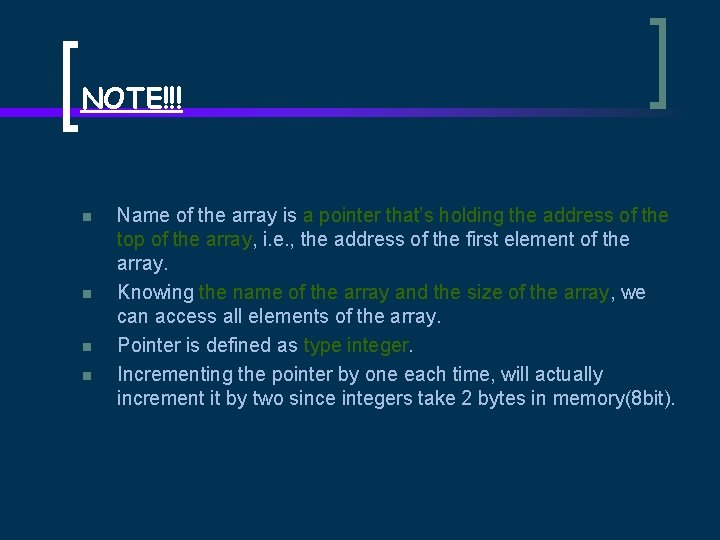
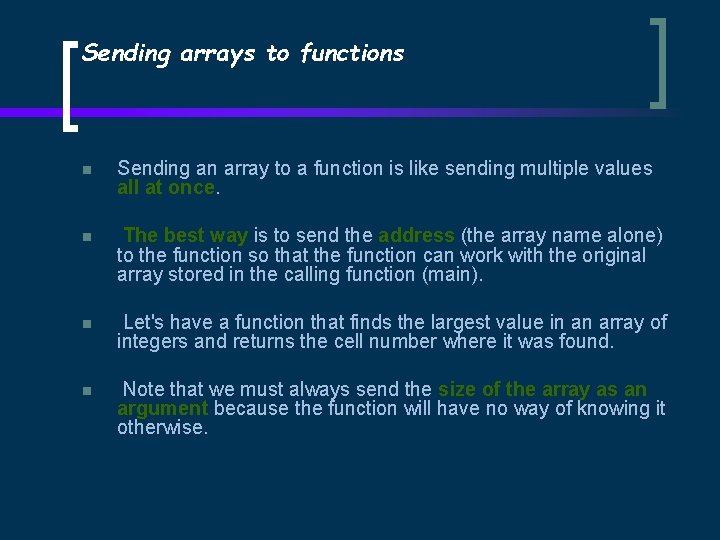
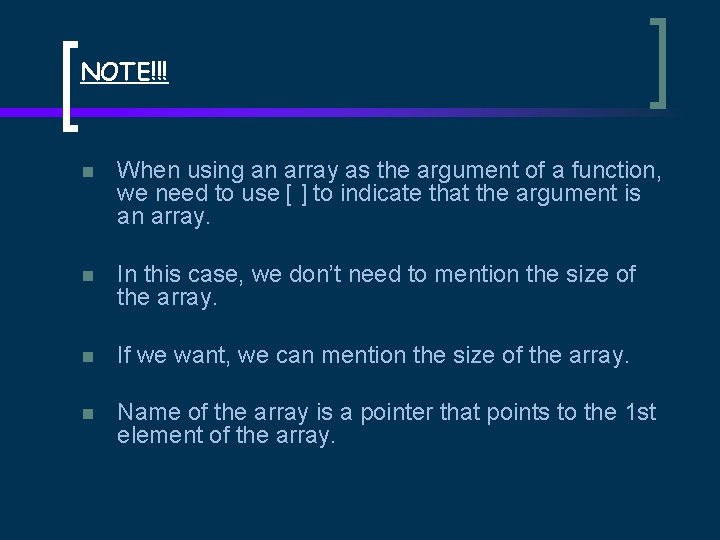
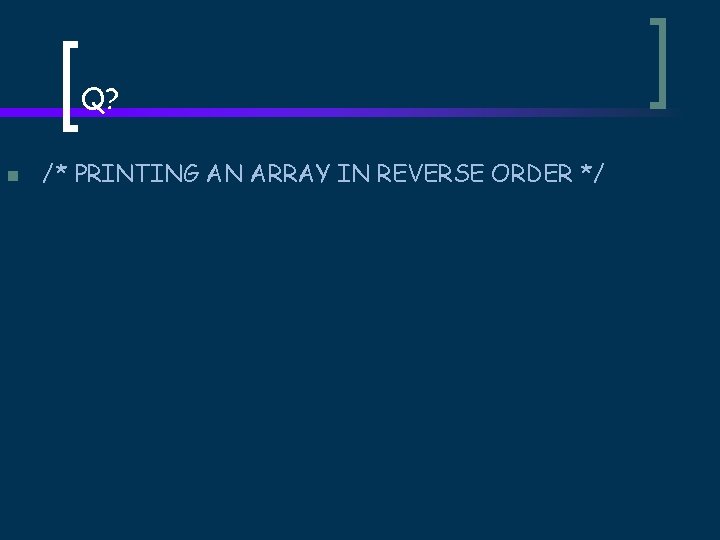
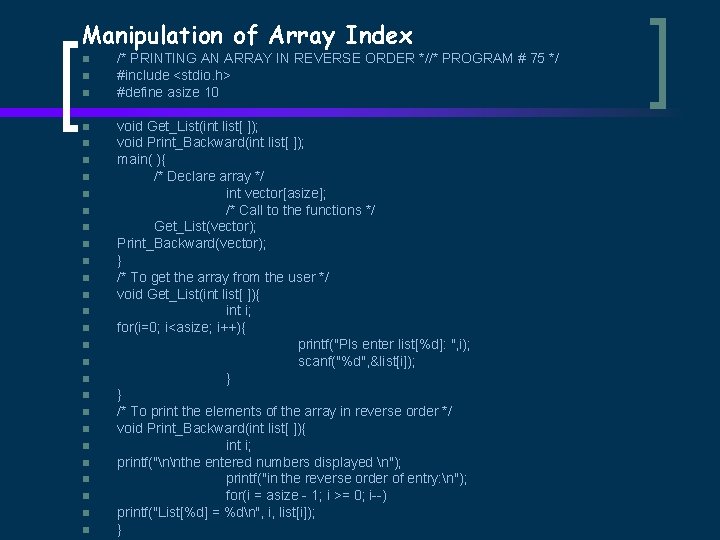
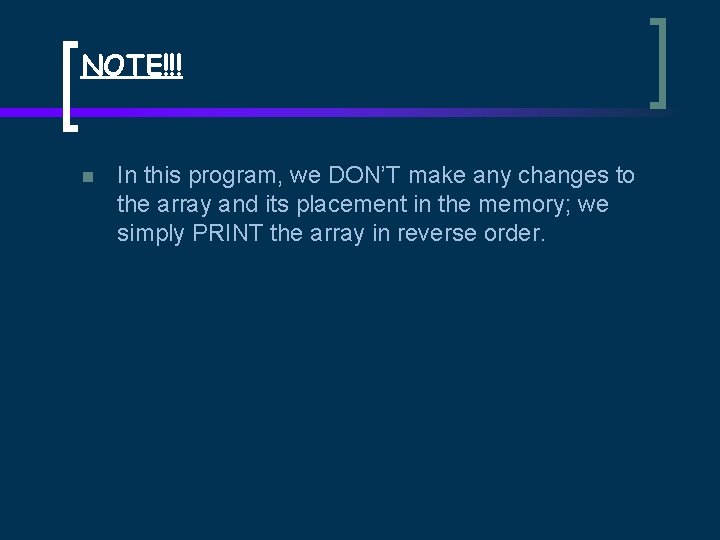
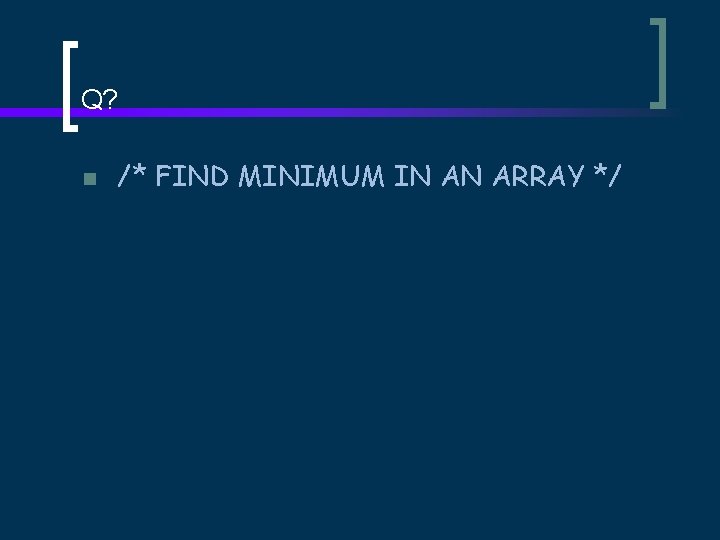
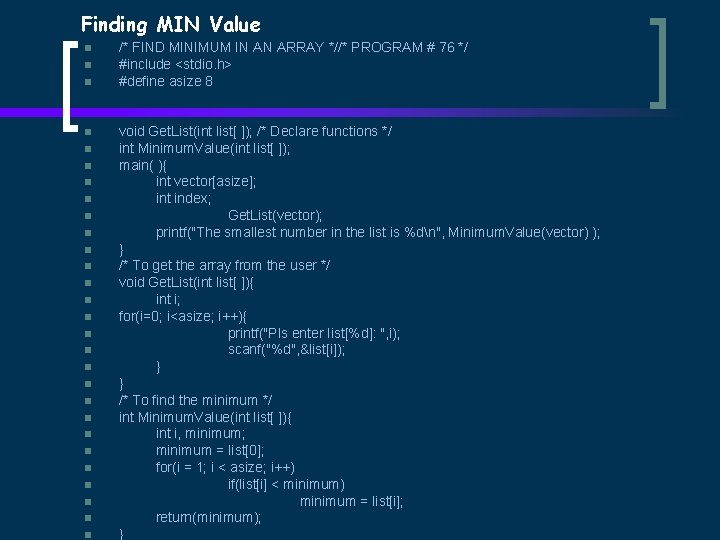
- Slides: 36
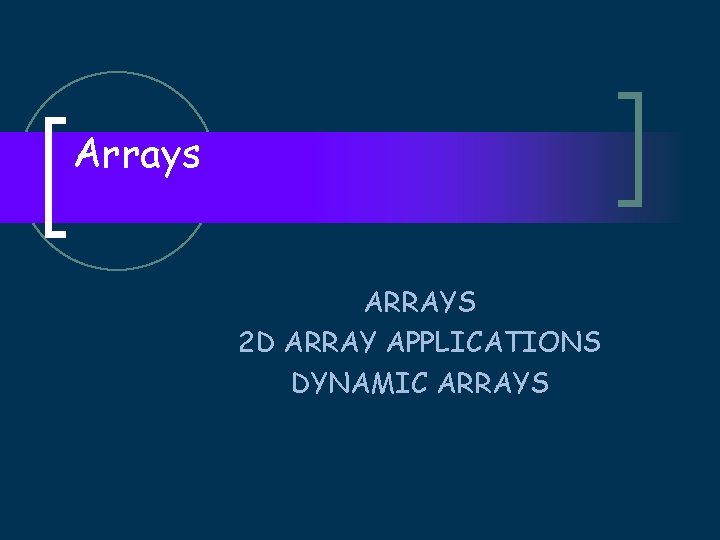
Arrays ARRAYS 2 D ARRAY APPLICATIONS DYNAMIC ARRAYS
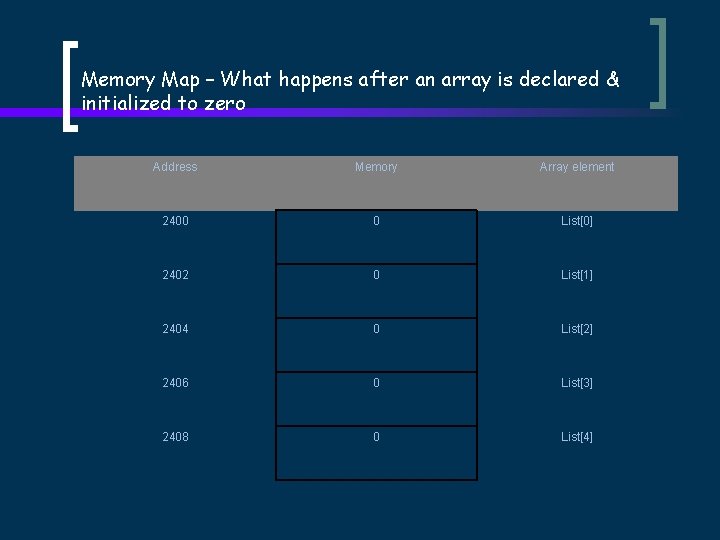
Memory Map – What happens after an array is declared & initialized to zero Address Memory Array element 2400 0 List[0] 2402 0 List[1] 2404 0 List[2] 2406 0 List[3] 2408 0 List[4]
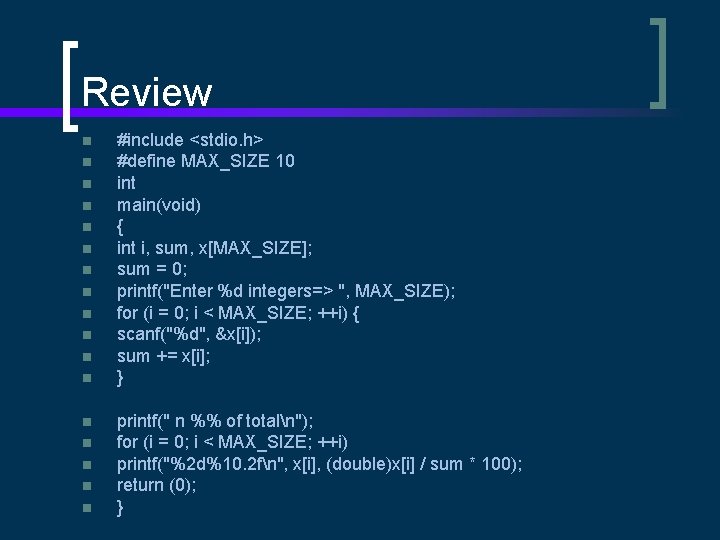
Review n n n n n #include <stdio. h> #define MAX_SIZE 10 int main(void) { int i, sum, x[MAX_SIZE]; sum = 0; printf("Enter %d integers=> ", MAX_SIZE); for (i = 0; i < MAX_SIZE; ++i) { scanf("%d", &x[i]); sum += x[i]; } printf(" n %% of totaln"); for (i = 0; i < MAX_SIZE; ++i) printf("%2 d%10. 2 fn", x[i], (double)x[i] / sum * 100); return (0); }
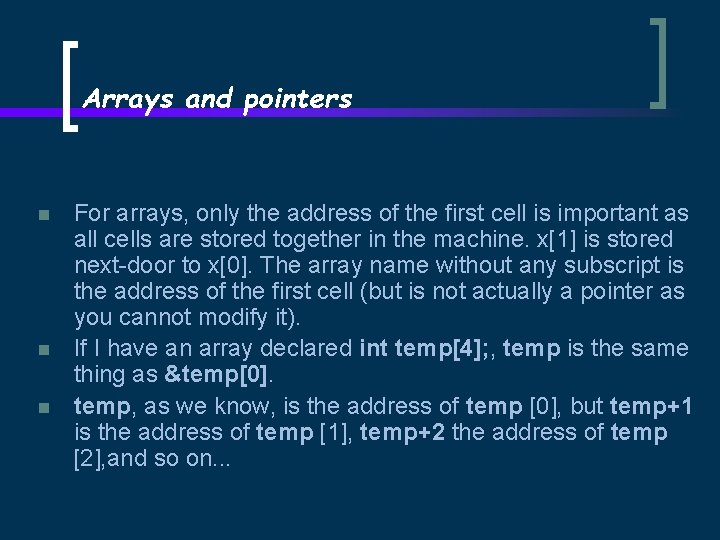
Arrays and pointers n n n For arrays, only the address of the first cell is important as all cells are stored together in the machine. x[1] is stored next-door to x[0]. The array name without any subscript is the address of the first cell (but is not actually a pointer as you cannot modify it). If I have an array declared int temp[4]; , temp is the same thing as &temp[0]. temp, as we know, is the address of temp [0], but temp+1 is the address of temp [1], temp+2 the address of temp [2], and so on. . .
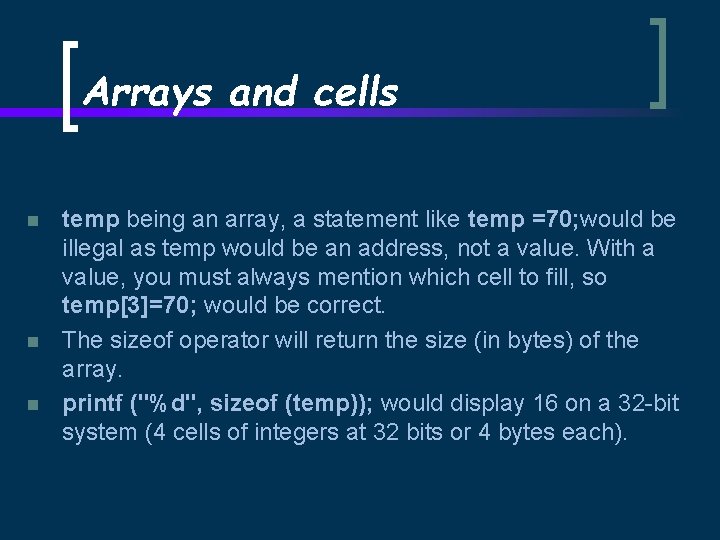
Arrays and cells n n n temp being an array, a statement like temp =70; would be illegal as temp would be an address, not a value. With a value, you must always mention which cell to fill, so temp[3]=70; would be correct. The sizeof operator will return the size (in bytes) of the array. printf ("%d", sizeof (temp)); would display 16 on a 32 -bit system (4 cells of integers at 32 bits or 4 bytes each).
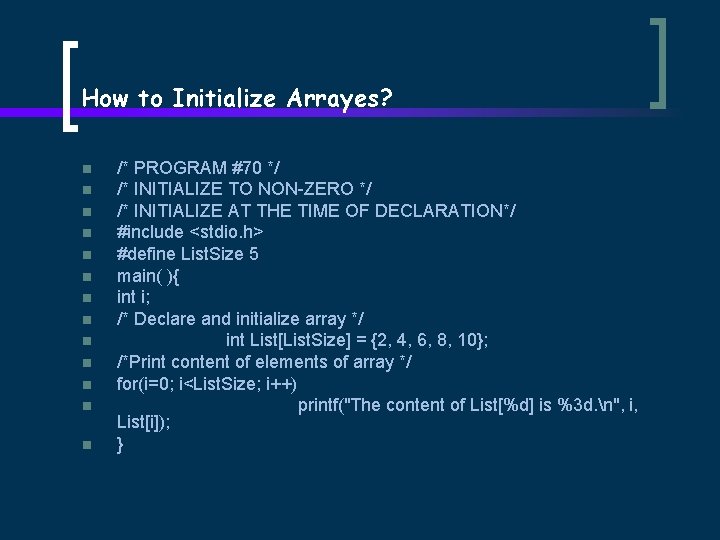
How to Initialize Arrayes? n n n n /* PROGRAM #70 */ /* INITIALIZE TO NON-ZERO */ /* INITIALIZE AT THE TIME OF DECLARATION*/ #include <stdio. h> #define List. Size 5 main( ){ int i; /* Declare and initialize array */ int List[List. Size] = {2, 4, 6, 8, 10}; /*Print content of elements of array */ for(i=0; i<List. Size; i++) printf("The content of List[%d] is %3 d. n", i, List[i]); }
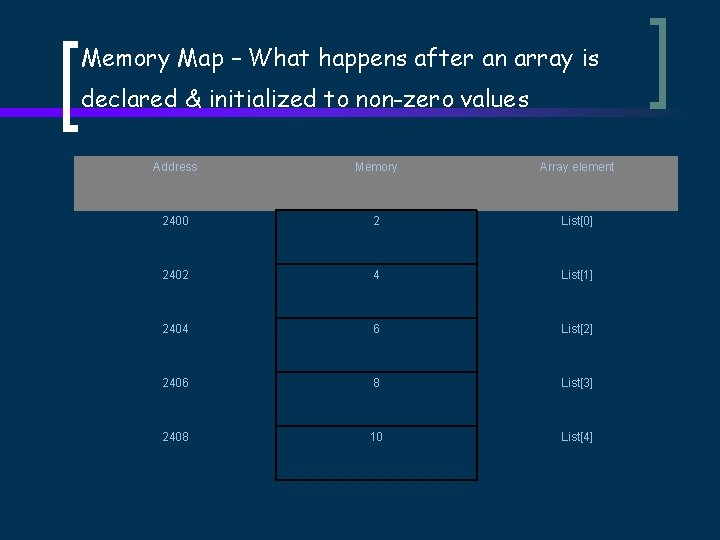
Memory Map – What happens after an array is declared & initialized to non-zero values Address Memory Array element 2400 2 List[0] 2402 4 List[1] 2404 6 List[2] 2406 8 List[3] 2408 10 List[4]
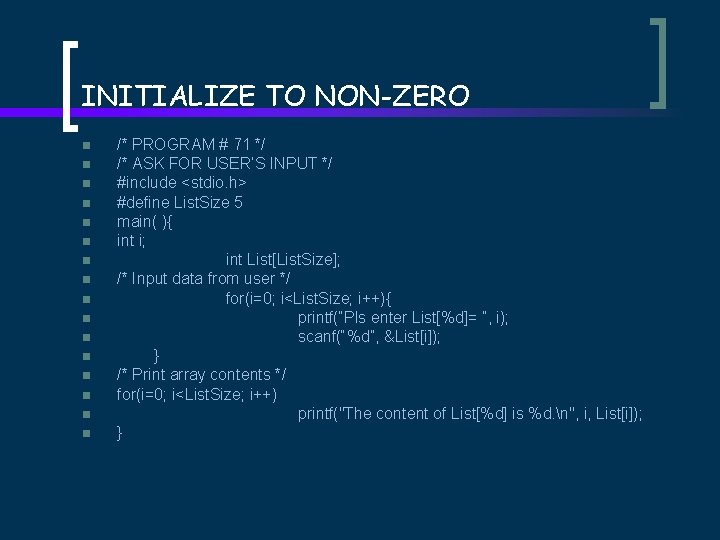
INITIALIZE TO NON-ZERO n n n n /* PROGRAM # 71 */ /* ASK FOR USER’S INPUT */ #include <stdio. h> #define List. Size 5 main( ){ int i; int List[List. Size]; /* Input data from user */ for(i=0; i<List. Size; i++){ printf(“Pls enter List[%d]= ”, i); scanf(“%d”, &List[i]); } /* Print array contents */ for(i=0; i<List. Size; i++) printf("The content of List[%d] is %d. n", i, List[i]); }
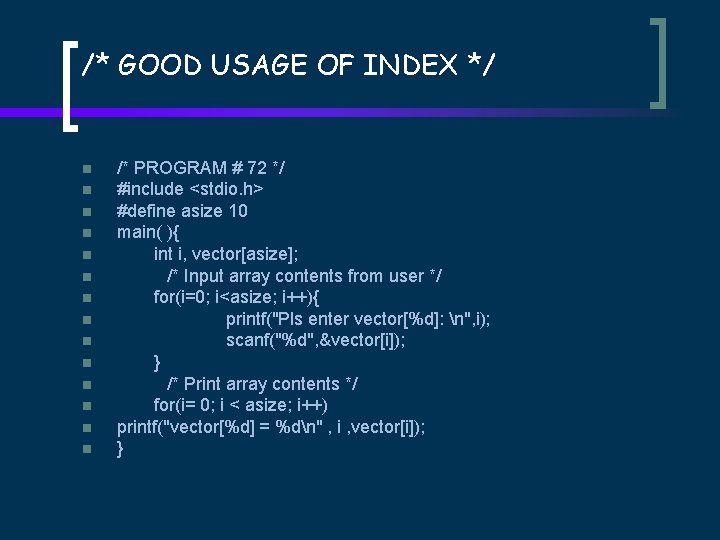
/* GOOD USAGE OF INDEX */ n n n n /* PROGRAM # 72 */ #include <stdio. h> #define asize 10 main( ){ int i, vector[asize]; /* Input array contents from user */ for(i=0; i<asize; i++){ printf("Pls enter vector[%d]: n", i); scanf("%d", &vector[i]); } /* Print array contents */ for(i= 0; i < asize; i++) printf("vector[%d] = %dn" , i , vector[i]); }
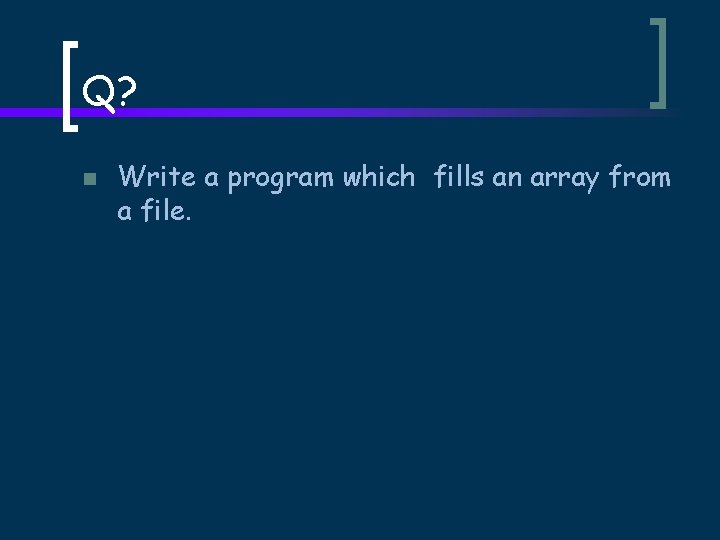
Q? n Write a program which fills an array from a file.
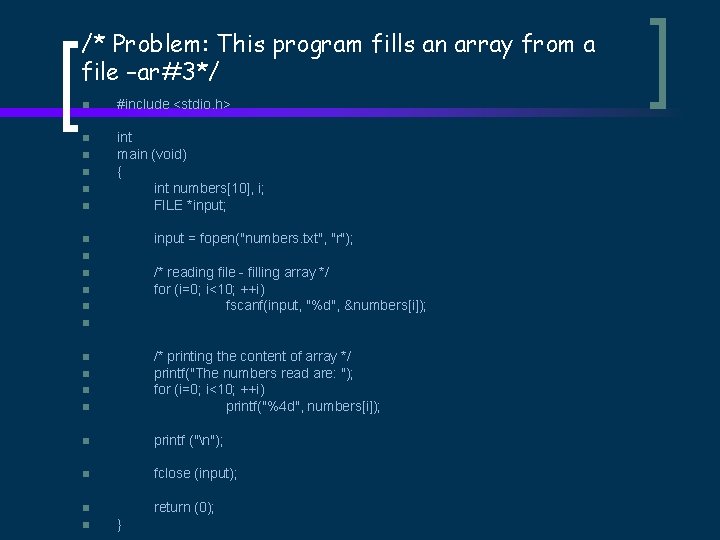
/* Problem: This program fills an array from a file –ar#3*/ n #include <stdio. h> n int main (void) { int numbers[10], i; FILE *input; n n input = fopen("numbers. txt", "r"); n n /* reading file - filling array */ for (i=0; i<10; ++i) fscanf(input, "%d", &numbers[i]); n n n /* printing the content of array */ printf("The numbers read are: "); for (i=0; i<10; ++i) printf("%4 d", numbers[i]); n printf ("n"); n fclose (input); n return (0); n n }
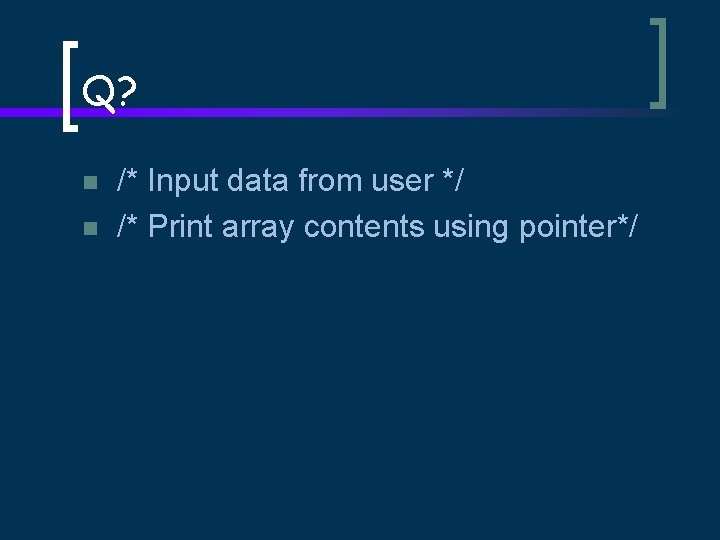
Q? n n /* Input data from user */ /* Print array contents using pointer*/
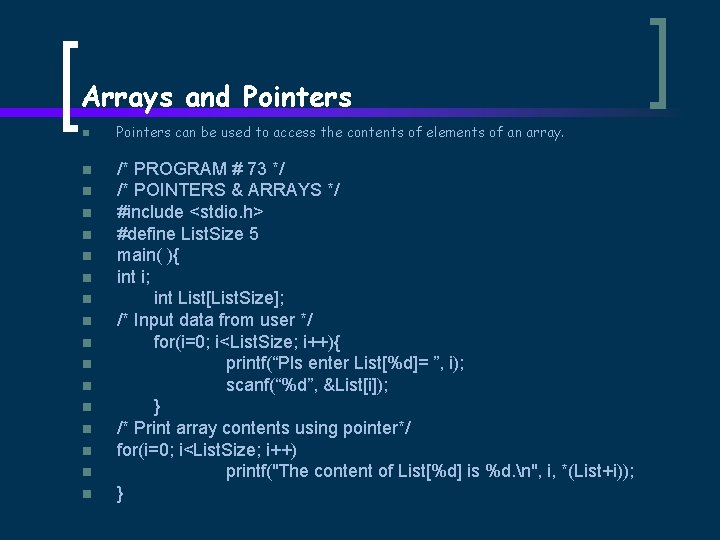
Arrays and Pointers n n n n n Pointers can be used to access the contents of elements of an array. /* PROGRAM # 73 */ /* POINTERS & ARRAYS */ #include <stdio. h> #define List. Size 5 main( ){ int i; int List[List. Size]; /* Input data from user */ for(i=0; i<List. Size; i++){ printf(“Pls enter List[%d]= ”, i); scanf(“%d”, &List[i]); } /* Print array contents using pointer*/ for(i=0; i<List. Size; i++) printf("The content of List[%d] is %d. n", i, *(List+i)); }
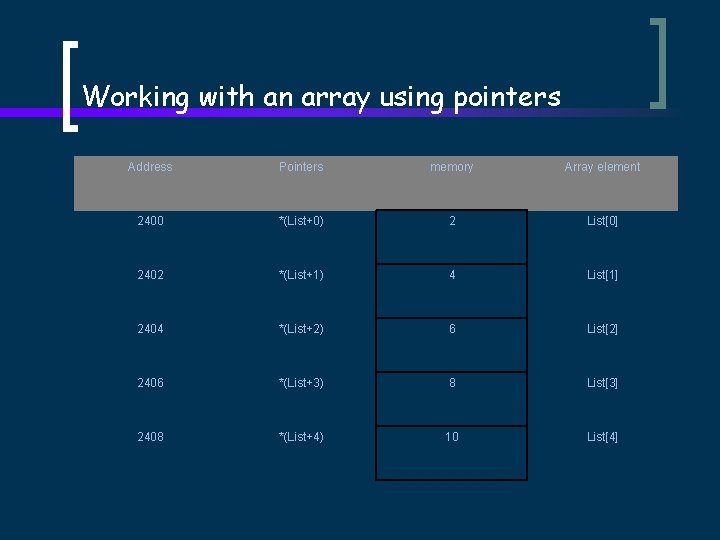
Working with an array using pointers Address Pointers memory Array element 2400 *(List+0) 2 List[0] 2402 *(List+1) 4 List[1] 2404 *(List+2) 6 List[2] 2406 *(List+3) 8 List[3] 2408 *(List+4) 10 List[4]
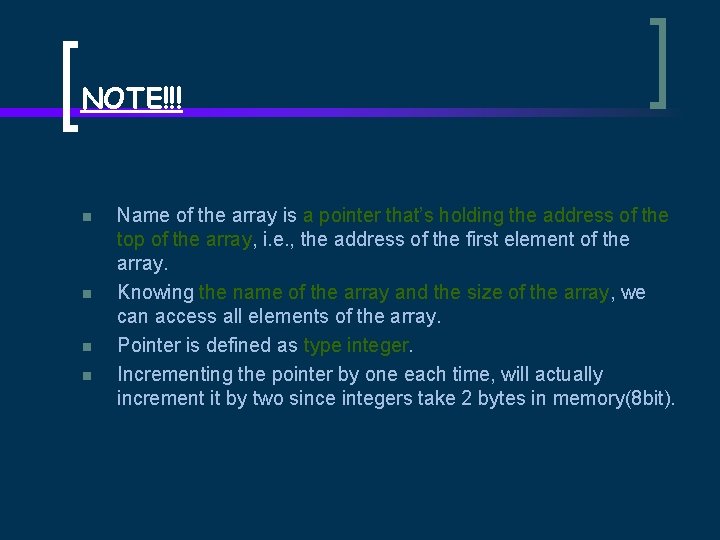
NOTE!!! n n Name of the array is a pointer that’s holding the address of the top of the array, i. e. , the address of the first element of the array. Knowing the name of the array and the size of the array, we can access all elements of the array. Pointer is defined as type integer. Incrementing the pointer by one each time, will actually increment it by two since integers take 2 bytes in memory(8 bit).
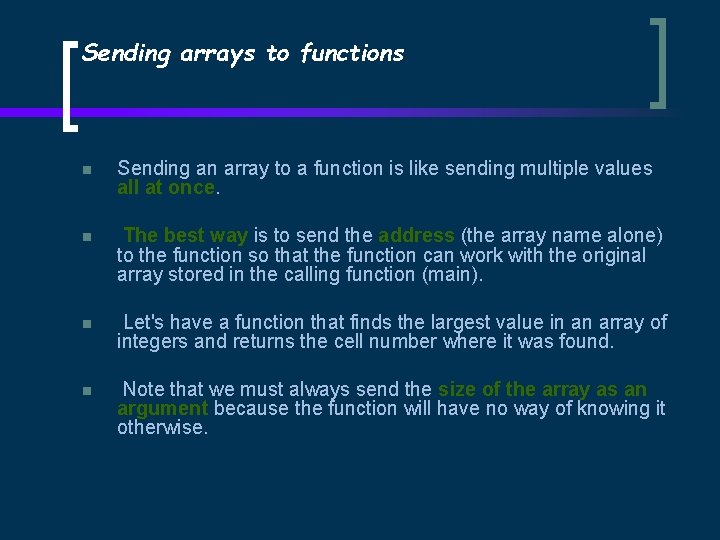
Sending arrays to functions n Sending an array to a function is like sending multiple values all at once. n The best way is to send the address (the array name alone) to the function so that the function can work with the original array stored in the calling function (main). n Let's have a function that finds the largest value in an array of integers and returns the cell number where it was found. n Note that we must always send the size of the array as an argument because the function will have no way of knowing it otherwise.
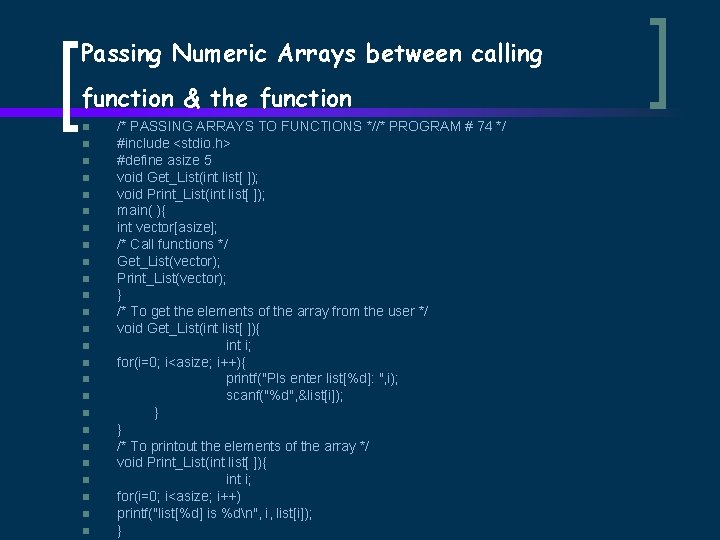
Passing Numeric Arrays between calling function & the function n n n n n n /* PASSING ARRAYS TO FUNCTIONS *//* PROGRAM # 74 */ #include <stdio. h> #define asize 5 void Get_List(int list[ ]); void Print_List(int list[ ]); main( ){ int vector[asize]; /* Call functions */ Get_List(vector); Print_List(vector); } /* To get the elements of the array from the user */ void Get_List(int list[ ]){ int i; for(i=0; i<asize; i++){ printf("Pls enter list[%d]: ", i); scanf("%d", &list[i]); } } /* To printout the elements of the array */ void Print_List(int list[ ]){ int i; for(i=0; i<asize; i++) printf("list[%d] is %dn", i, list[i]); }
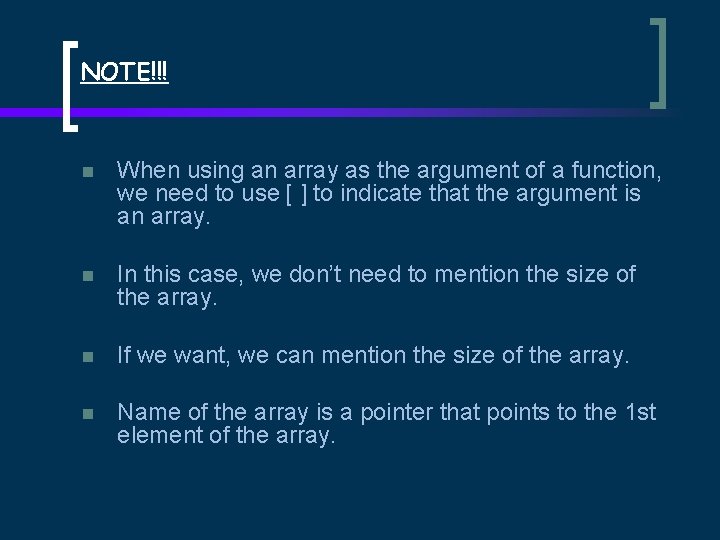
NOTE!!! n When using an array as the argument of a function, we need to use [ ] to indicate that the argument is an array. n In this case, we don’t need to mention the size of the array. n If we want, we can mention the size of the array. n Name of the array is a pointer that points to the 1 st element of the array.
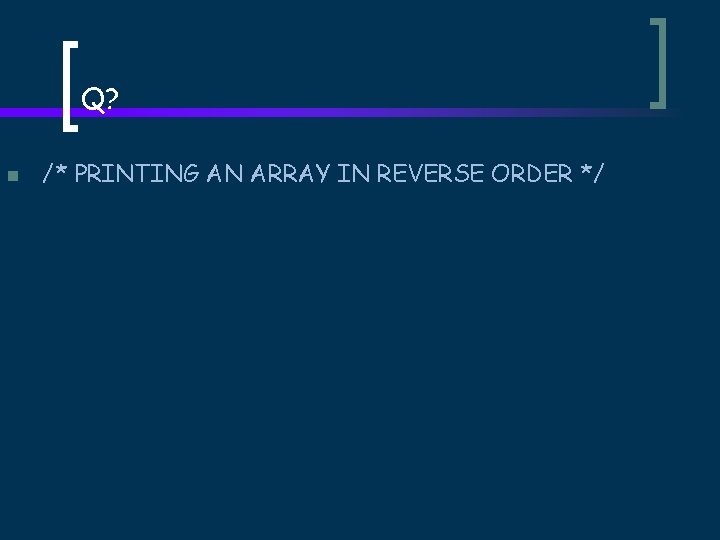
Q? n /* PRINTING AN ARRAY IN REVERSE ORDER */
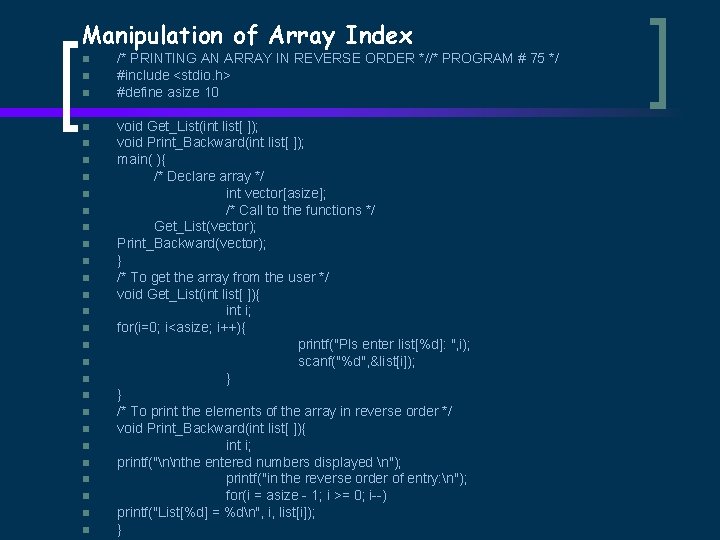
Manipulation of Array Index n n n n n n n /* PRINTING AN ARRAY IN REVERSE ORDER *//* PROGRAM # 75 */ #include <stdio. h> #define asize 10 void Get_List(int list[ ]); void Print_Backward(int list[ ]); main( ){ /* Declare array */ int vector[asize]; /* Call to the functions */ Get_List(vector); Print_Backward(vector); } /* To get the array from the user */ void Get_List(int list[ ]){ int i; for(i=0; i<asize; i++){ printf("Pls enter list[%d]: ", i); scanf("%d", &list[i]); } } /* To print the elements of the array in reverse order */ void Print_Backward(int list[ ]){ int i; printf("nnthe entered numbers displayed n"); printf("in the reverse order of entry: n"); for(i = asize - 1; i >= 0; i--) printf("List[%d] = %dn", i, list[i]); }
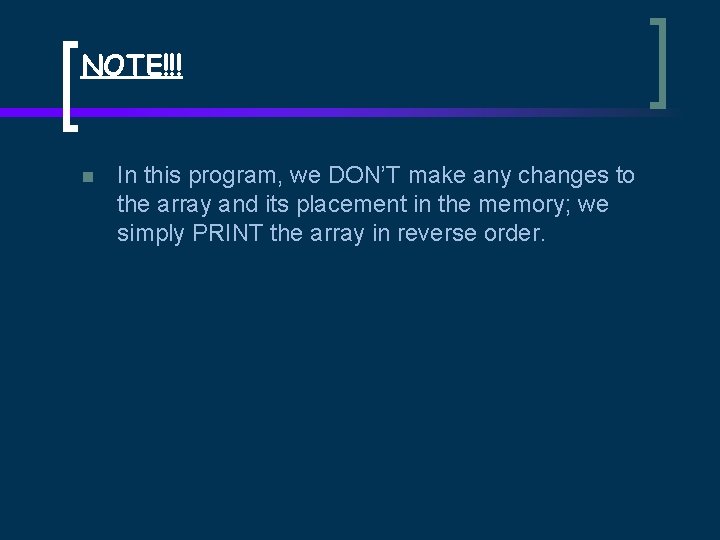
NOTE!!! n In this program, we DON’T make any changes to the array and its placement in the memory; we simply PRINT the array in reverse order.
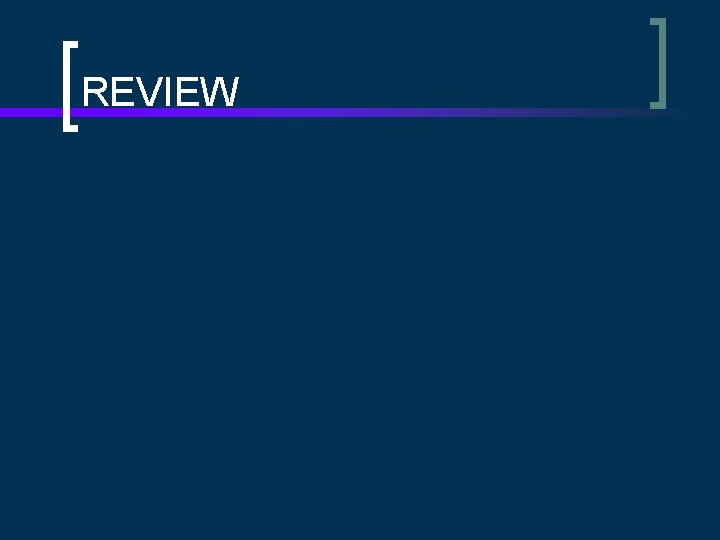
REVIEW
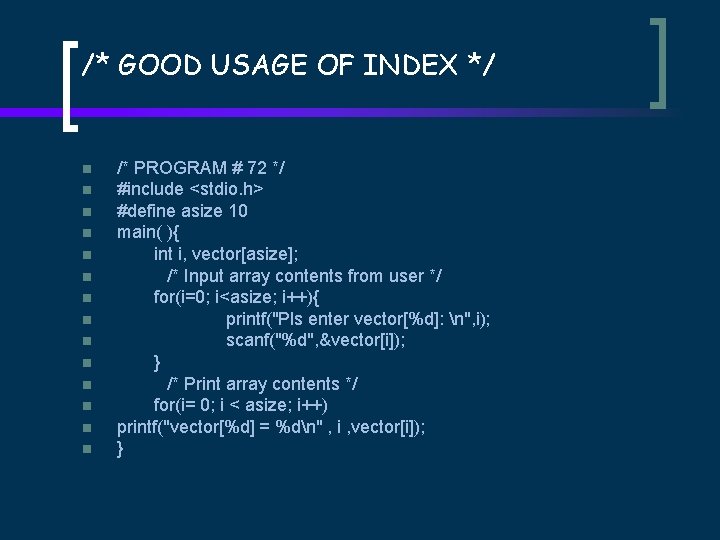
/* GOOD USAGE OF INDEX */ n n n n /* PROGRAM # 72 */ #include <stdio. h> #define asize 10 main( ){ int i, vector[asize]; /* Input array contents from user */ for(i=0; i<asize; i++){ printf("Pls enter vector[%d]: n", i); scanf("%d", &vector[i]); } /* Print array contents */ for(i= 0; i < asize; i++) printf("vector[%d] = %dn" , i , vector[i]); }
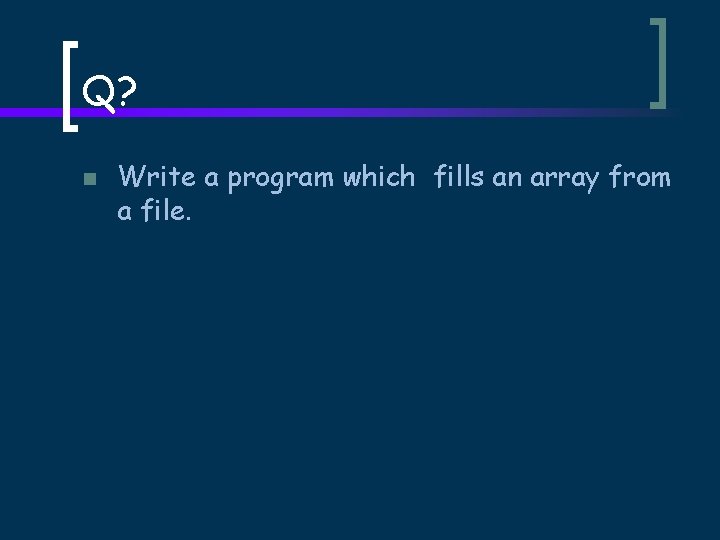
Q? n Write a program which fills an array from a file.
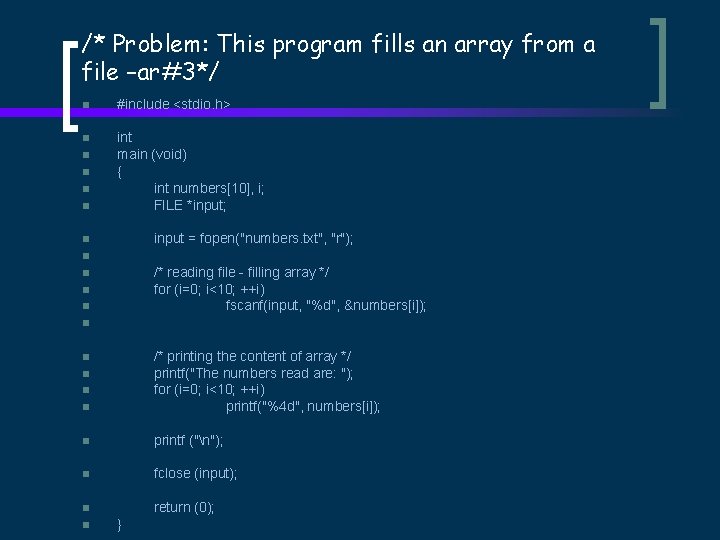
/* Problem: This program fills an array from a file –ar#3*/ n #include <stdio. h> n int main (void) { int numbers[10], i; FILE *input; n n input = fopen("numbers. txt", "r"); n n /* reading file - filling array */ for (i=0; i<10; ++i) fscanf(input, "%d", &numbers[i]); n n n /* printing the content of array */ printf("The numbers read are: "); for (i=0; i<10; ++i) printf("%4 d", numbers[i]); n printf ("n"); n fclose (input); n return (0); n n }
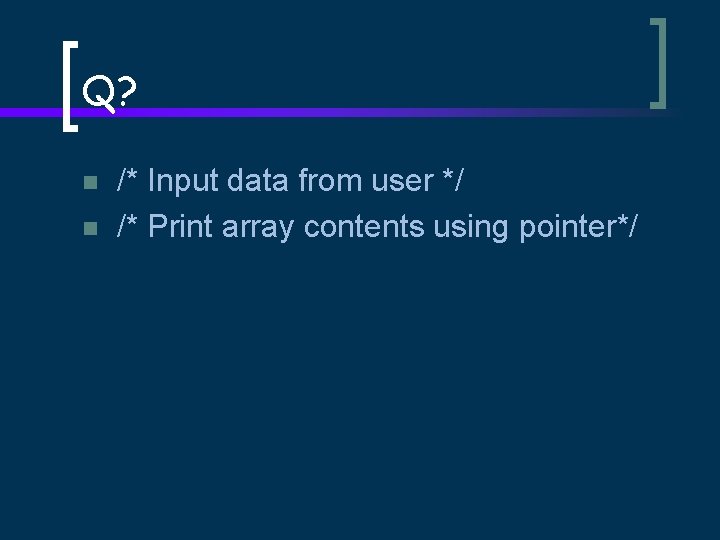
Q? n n /* Input data from user */ /* Print array contents using pointer*/
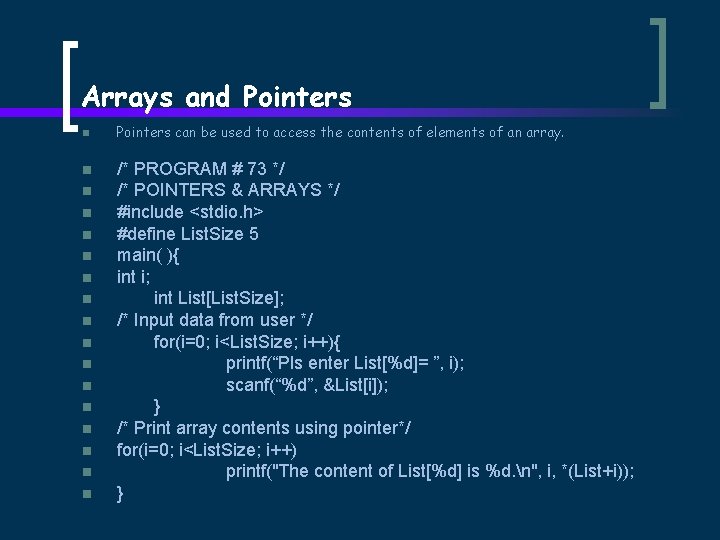
Arrays and Pointers n n n n n Pointers can be used to access the contents of elements of an array. /* PROGRAM # 73 */ /* POINTERS & ARRAYS */ #include <stdio. h> #define List. Size 5 main( ){ int i; int List[List. Size]; /* Input data from user */ for(i=0; i<List. Size; i++){ printf(“Pls enter List[%d]= ”, i); scanf(“%d”, &List[i]); } /* Print array contents using pointer*/ for(i=0; i<List. Size; i++) printf("The content of List[%d] is %d. n", i, *(List+i)); }
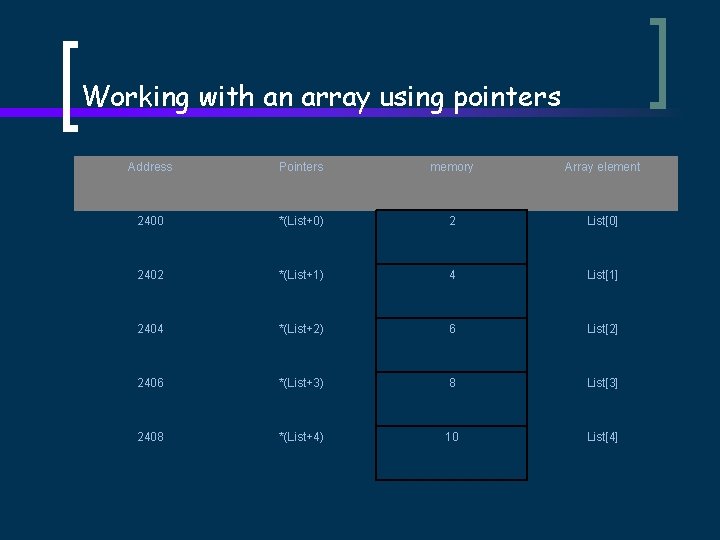
Working with an array using pointers Address Pointers memory Array element 2400 *(List+0) 2 List[0] 2402 *(List+1) 4 List[1] 2404 *(List+2) 6 List[2] 2406 *(List+3) 8 List[3] 2408 *(List+4) 10 List[4]
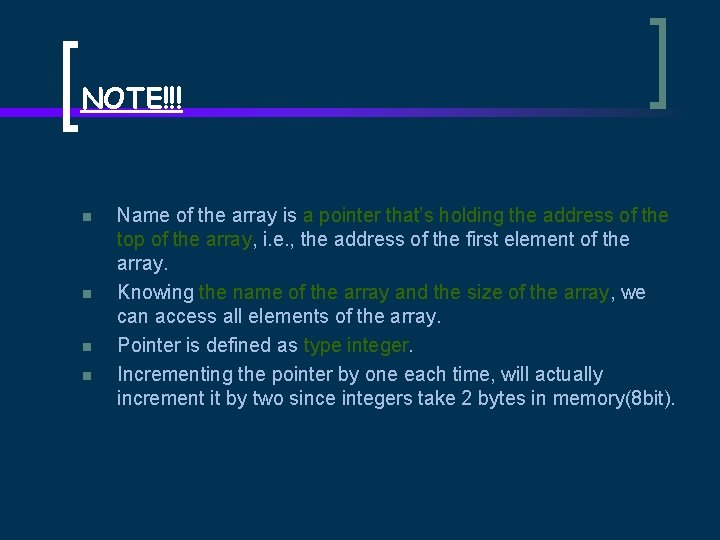
NOTE!!! n n Name of the array is a pointer that’s holding the address of the top of the array, i. e. , the address of the first element of the array. Knowing the name of the array and the size of the array, we can access all elements of the array. Pointer is defined as type integer. Incrementing the pointer by one each time, will actually increment it by two since integers take 2 bytes in memory(8 bit).
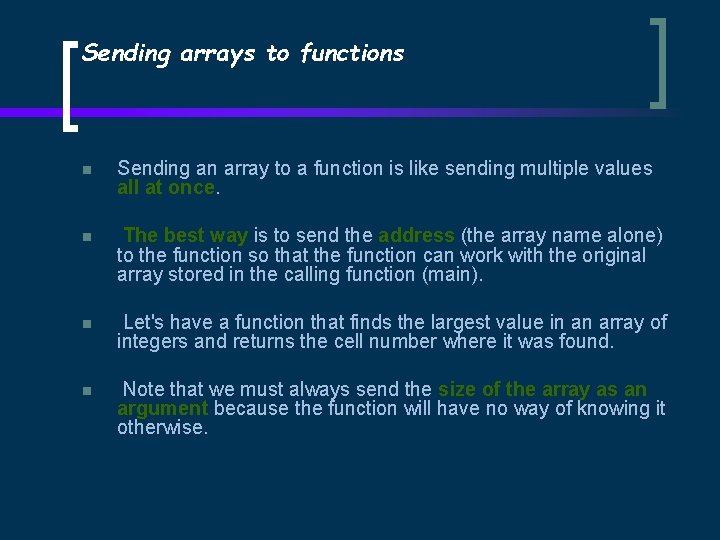
Sending arrays to functions n Sending an array to a function is like sending multiple values all at once. n The best way is to send the address (the array name alone) to the function so that the function can work with the original array stored in the calling function (main). n Let's have a function that finds the largest value in an array of integers and returns the cell number where it was found. n Note that we must always send the size of the array as an argument because the function will have no way of knowing it otherwise.
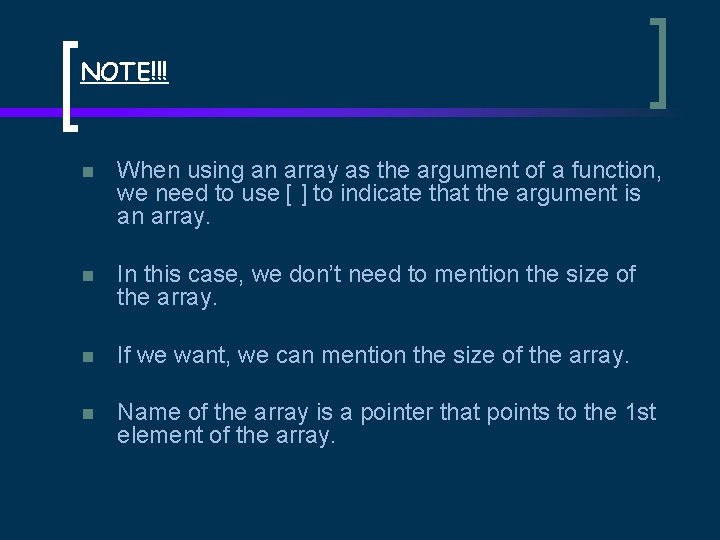
NOTE!!! n When using an array as the argument of a function, we need to use [ ] to indicate that the argument is an array. n In this case, we don’t need to mention the size of the array. n If we want, we can mention the size of the array. n Name of the array is a pointer that points to the 1 st element of the array.
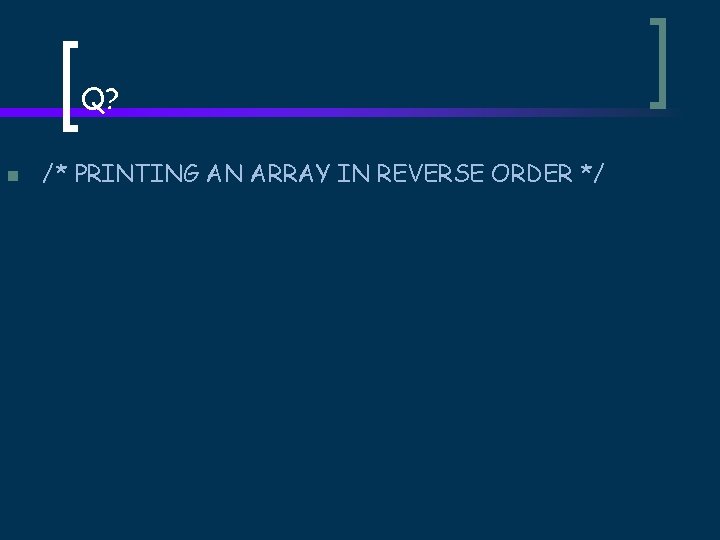
Q? n /* PRINTING AN ARRAY IN REVERSE ORDER */
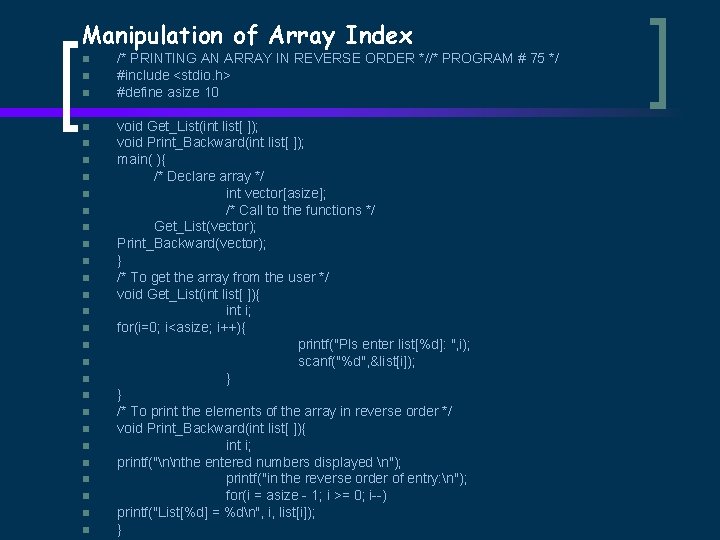
Manipulation of Array Index n n n n n n n /* PRINTING AN ARRAY IN REVERSE ORDER *//* PROGRAM # 75 */ #include <stdio. h> #define asize 10 void Get_List(int list[ ]); void Print_Backward(int list[ ]); main( ){ /* Declare array */ int vector[asize]; /* Call to the functions */ Get_List(vector); Print_Backward(vector); } /* To get the array from the user */ void Get_List(int list[ ]){ int i; for(i=0; i<asize; i++){ printf("Pls enter list[%d]: ", i); scanf("%d", &list[i]); } } /* To print the elements of the array in reverse order */ void Print_Backward(int list[ ]){ int i; printf("nnthe entered numbers displayed n"); printf("in the reverse order of entry: n"); for(i = asize - 1; i >= 0; i--) printf("List[%d] = %dn", i, list[i]); }
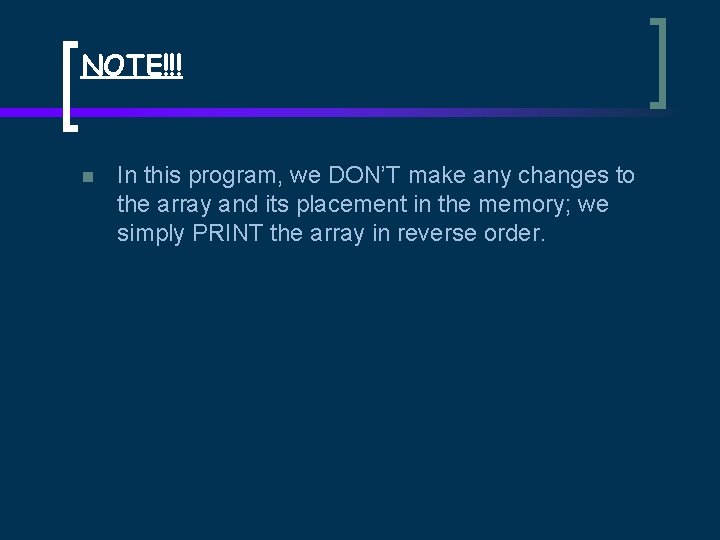
NOTE!!! n In this program, we DON’T make any changes to the array and its placement in the memory; we simply PRINT the array in reverse order.
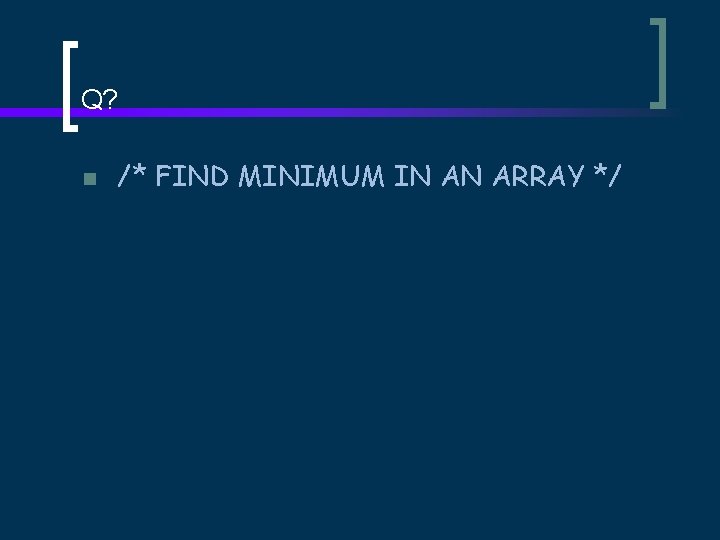
Q? n /* FIND MINIMUM IN AN ARRAY */
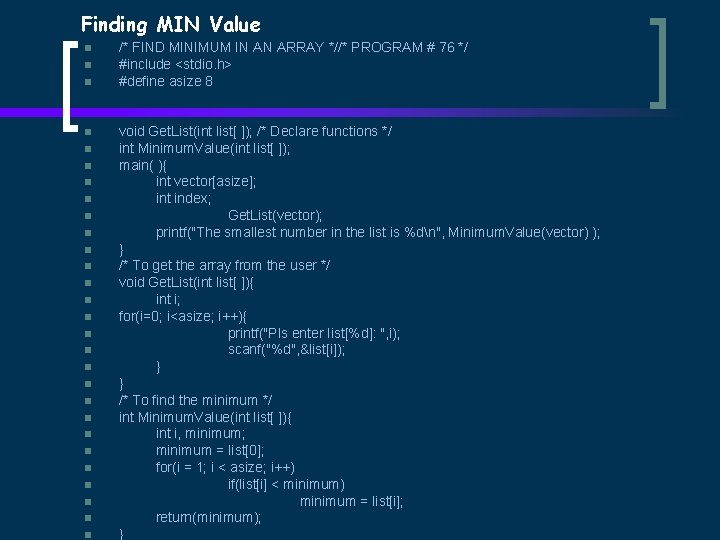
Finding MIN Value n n n n n n n /* FIND MINIMUM IN AN ARRAY *//* PROGRAM # 76 */ #include <stdio. h> #define asize 8 void Get. List(int list[ ]); /* Declare functions */ int Minimum. Value(int list[ ]); main( ){ int vector[asize]; int index; Get. List(vector); printf("The smallest number in the list is %dn", Minimum. Value(vector) ); } /* To get the array from the user */ void Get. List(int list[ ]){ int i; for(i=0; i<asize; i++){ printf("Pls enter list[%d]: ", i); scanf("%d", &list[i]); } } /* To find the minimum */ int Minimum. Value(int list[ ]){ int i, minimum; minimum = list[0]; for(i = 1; i < asize; i++) if(list[i] < minimum) minimum = list[i]; return(minimum); }