Twodimensional arrays Some languages Fortran Pascal support twodimensional
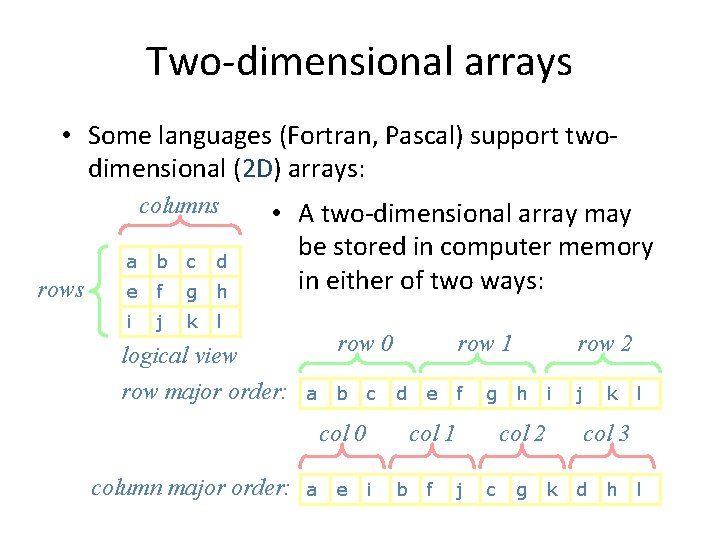
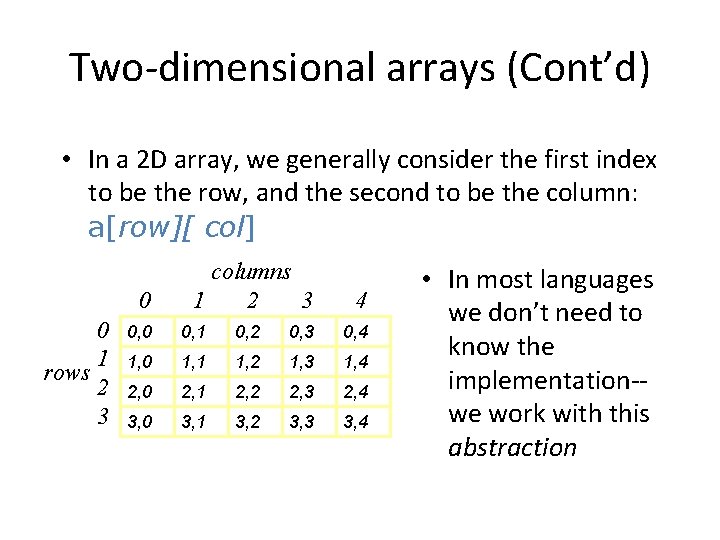
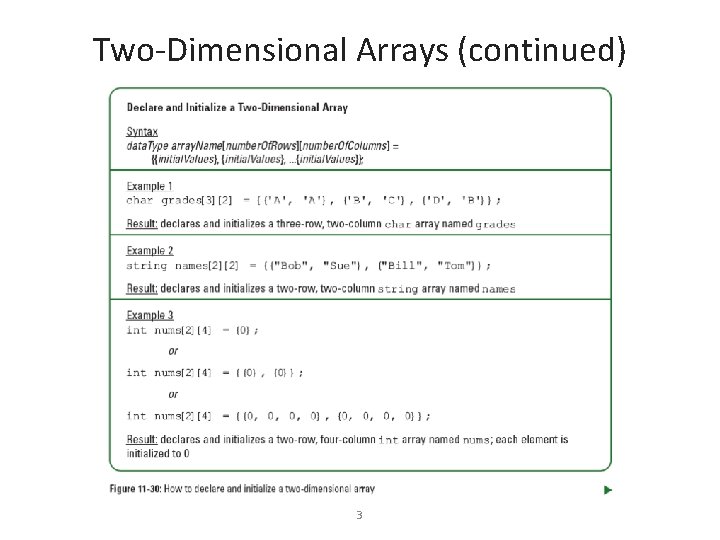
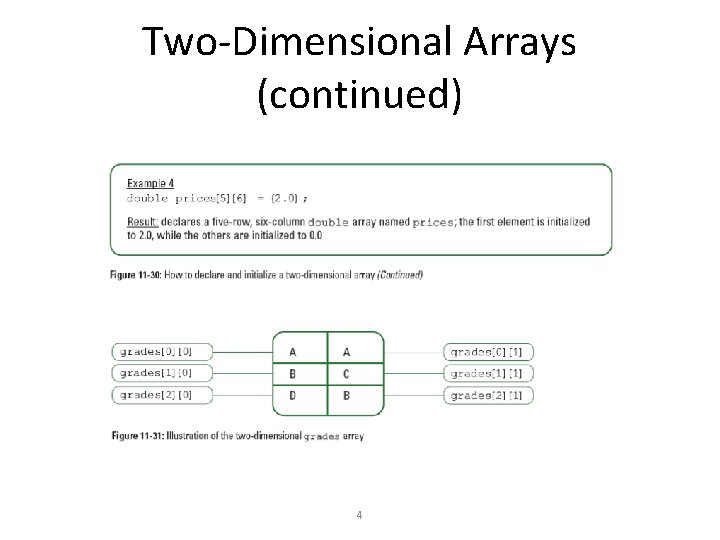
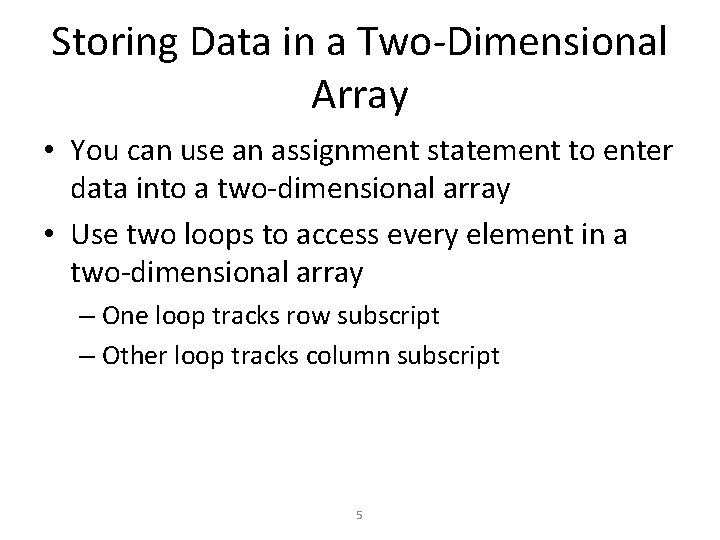
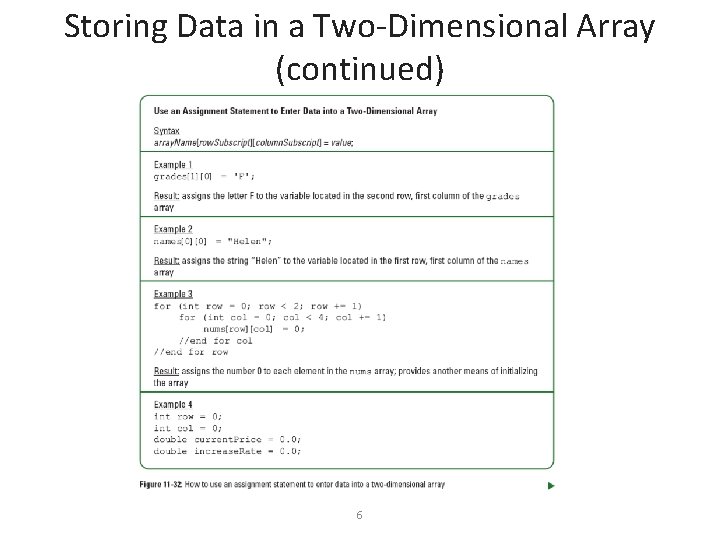
![Example for two dimensional array #include<iostream. h> void main( ) { int array[2][3] = Example for two dimensional array #include<iostream. h> void main( ) { int array[2][3] =](https://slidetodoc.com/presentation_image_h2/22d5ae2a83c02abfea472735a030799e/image-7.jpg)
![Example(2) #include<iostream. h> void main( ) { int b [5][5]; int i, j; for Example(2) #include<iostream. h> void main( ) { int b [5][5]; int i, j; for](https://slidetodoc.com/presentation_image_h2/22d5ae2a83c02abfea472735a030799e/image-8.jpg)
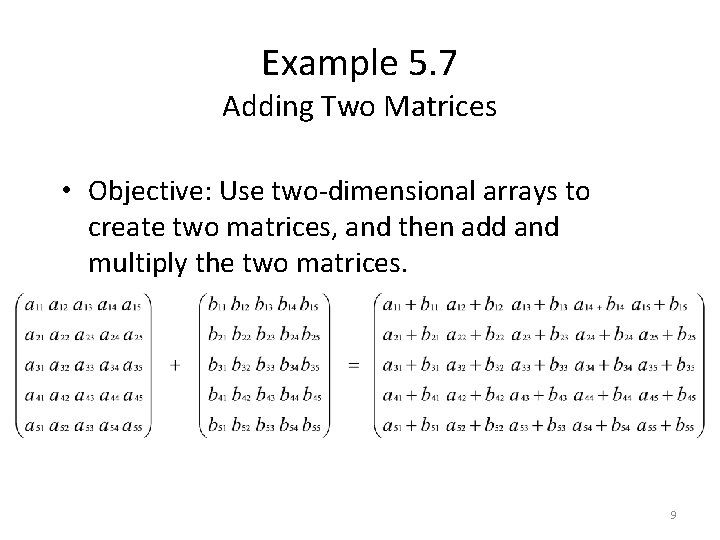
![Example #include<iostream. h> void main( ) { int i, j; int b [5][5]; int Example #include<iostream. h> void main( ) { int i, j; int b [5][5]; int](https://slidetodoc.com/presentation_image_h2/22d5ae2a83c02abfea472735a030799e/image-10.jpg)
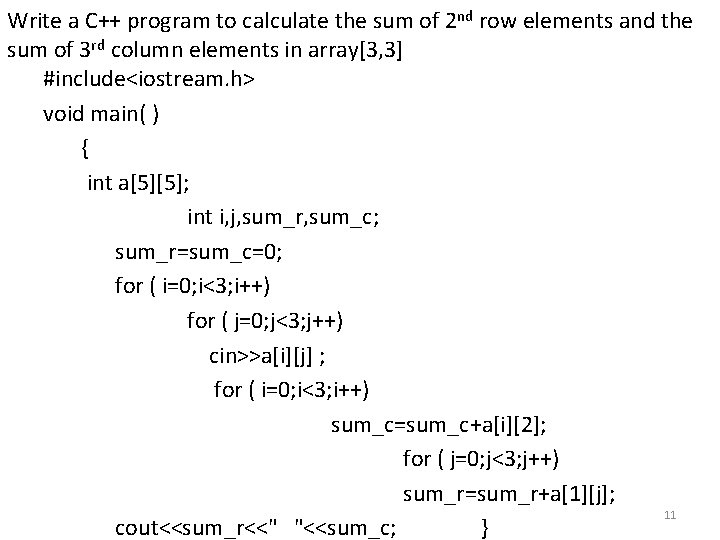
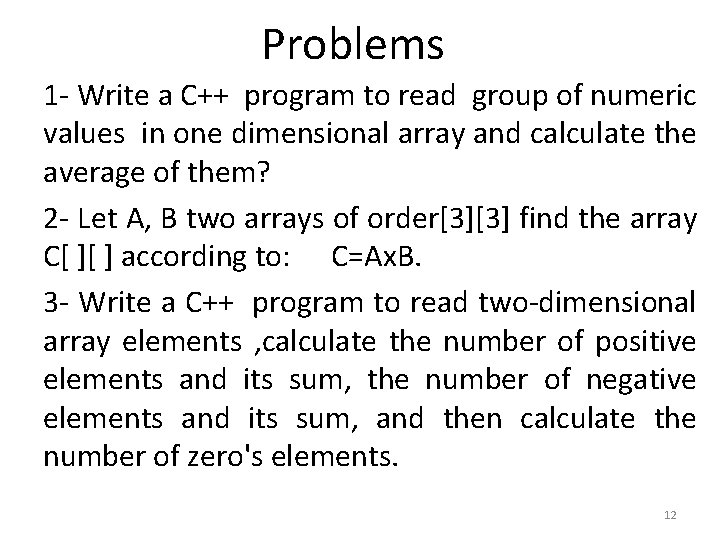
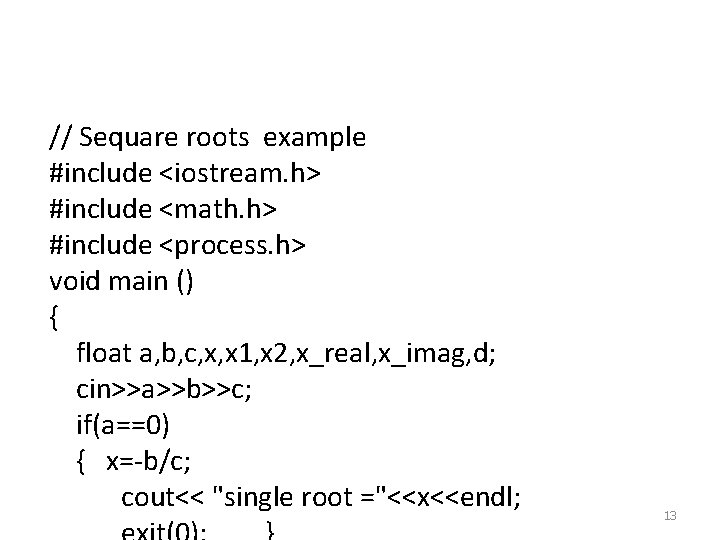
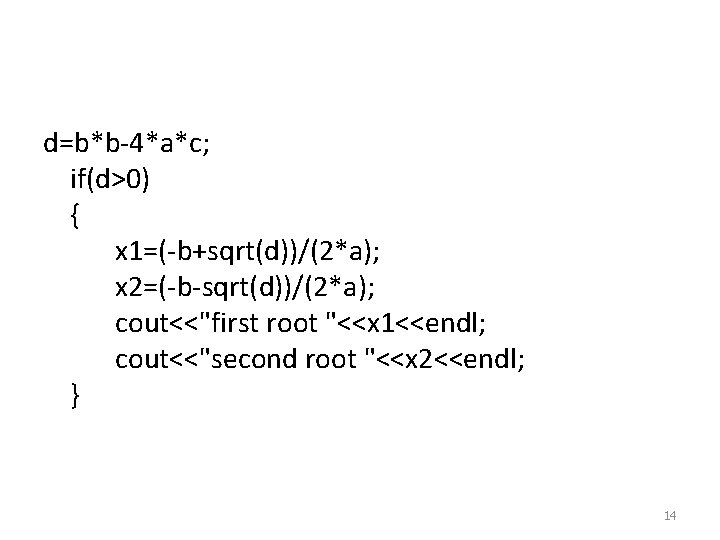
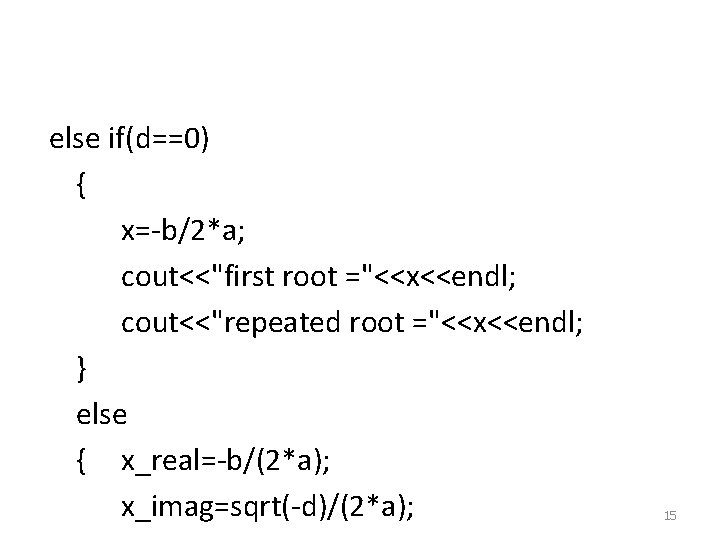
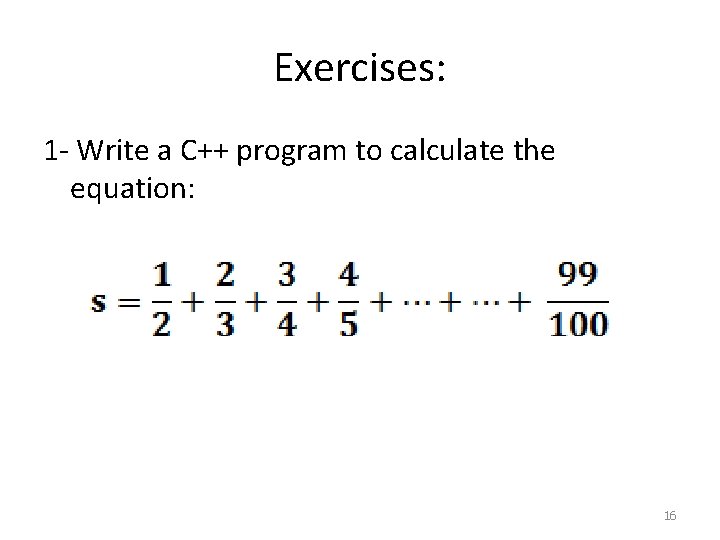
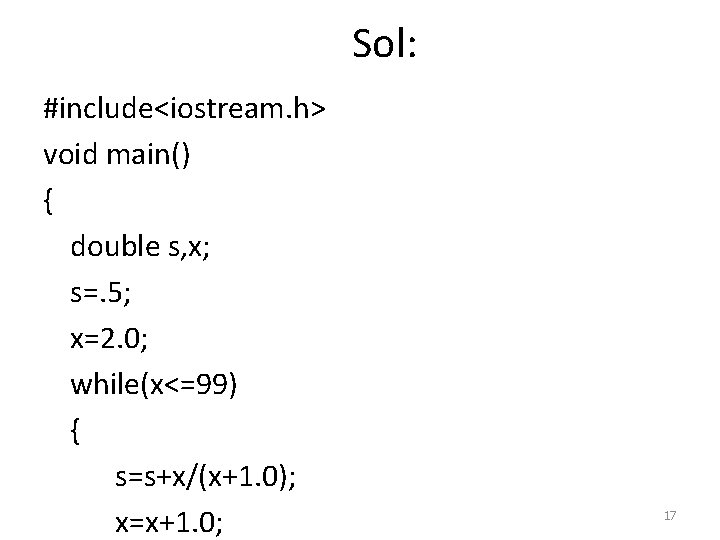
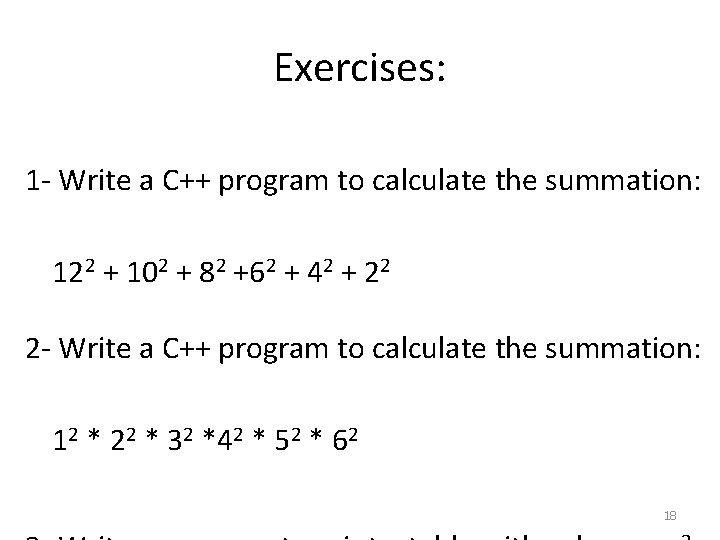
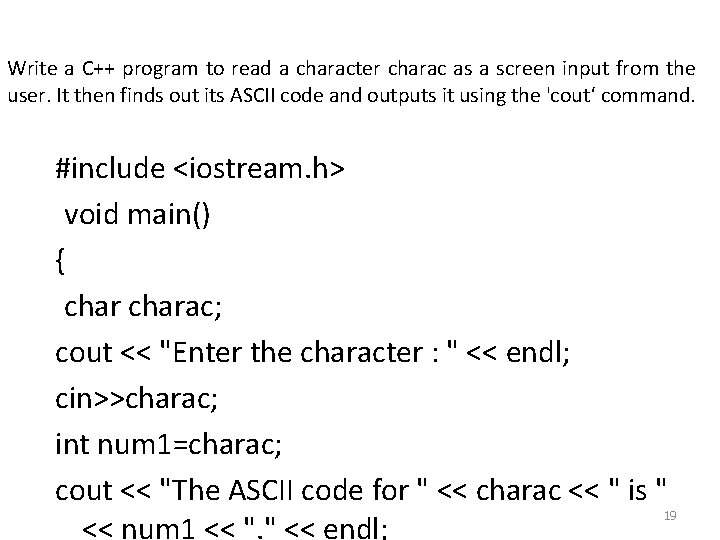
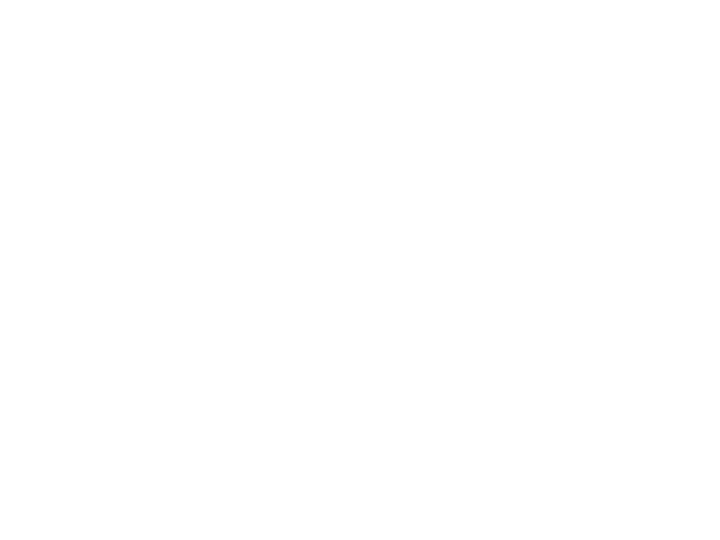
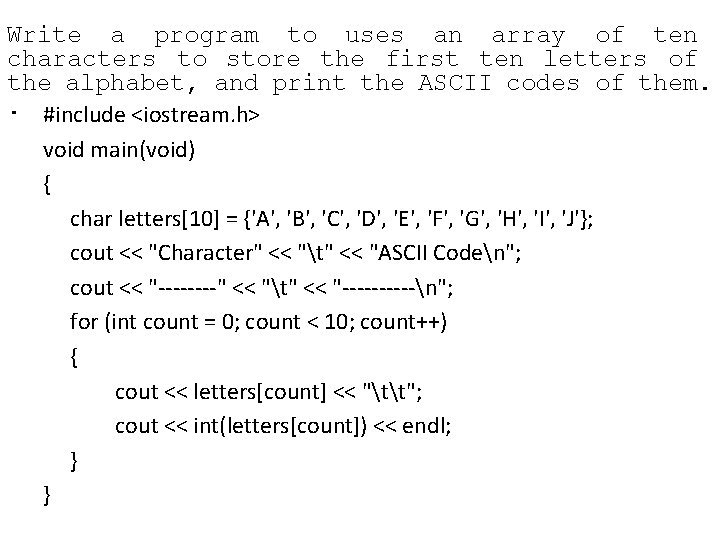
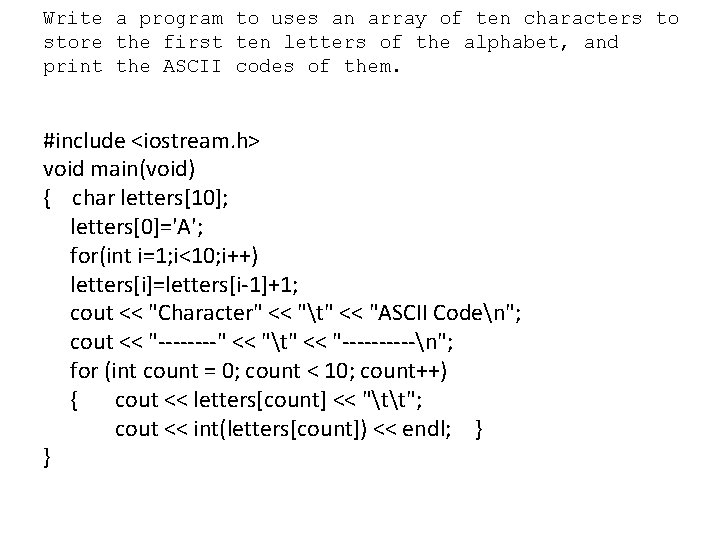
- Slides: 22
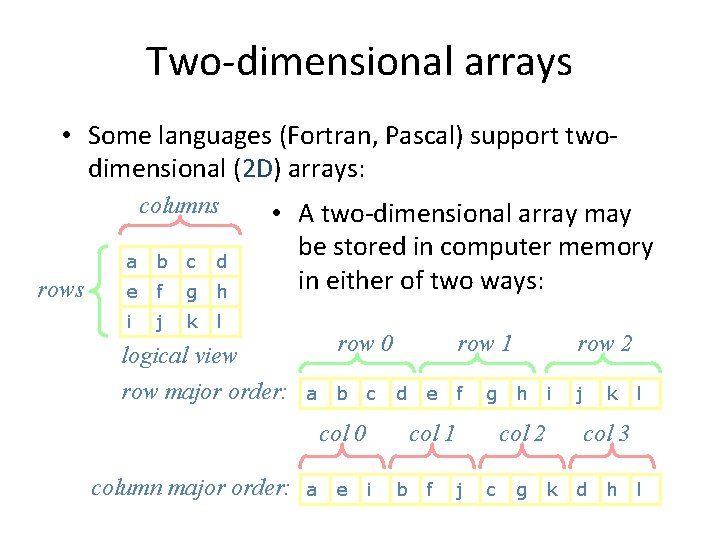
Two-dimensional arrays • Some languages (Fortran, Pascal) support twodimensional (2 D) arrays: columns rows a b c d e f g h i j k l • A two-dimensional array may be stored in computer memory in either of two ways: logical view row major order: row 0 a b c row 1 d col 0 column major order: a e e f g col 1 i b f row 2 h i col 2 j c g j k l col 3 k d h l
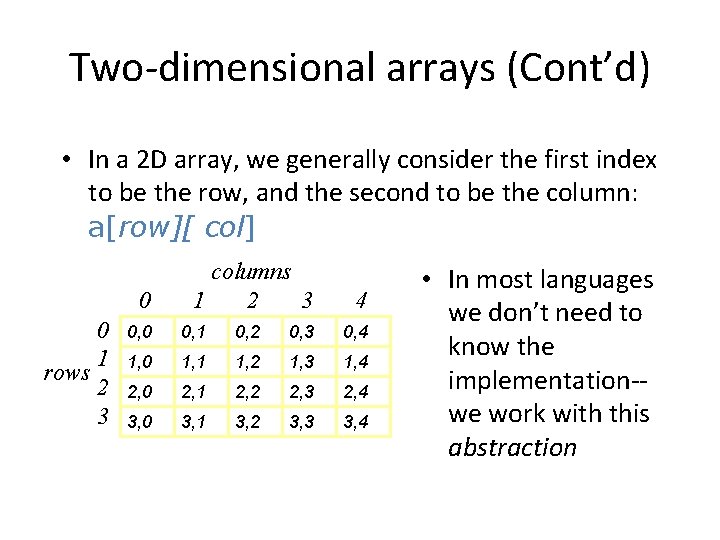
Two-dimensional arrays (Cont’d) • In a 2 D array, we generally consider the first index to be the row, and the second to be the column: a[row][ col] 0 0 1 rows 2 3 columns 1 2 3 4 0, 0 0, 1 0, 2 0, 3 0, 4 1, 0 1, 1 1, 2 1, 3 1, 4 2, 0 2, 1 2, 2 2, 3 2, 4 3, 0 3, 1 3, 2 3, 3 3, 4 • In most languages we don’t need to know the implementation-we work with this abstraction
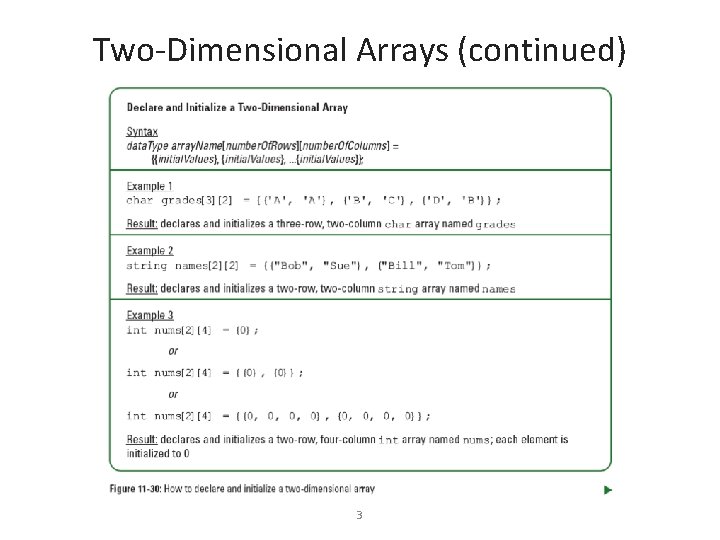
Two-Dimensional Arrays (continued) 3
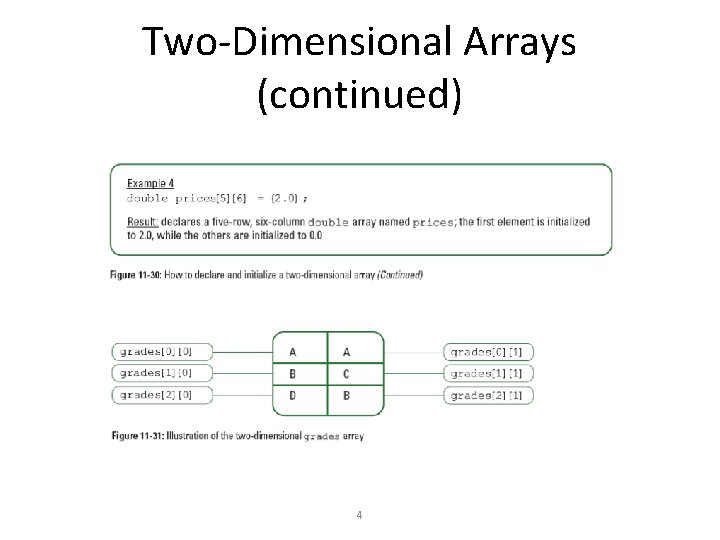
Two-Dimensional Arrays (continued) 4
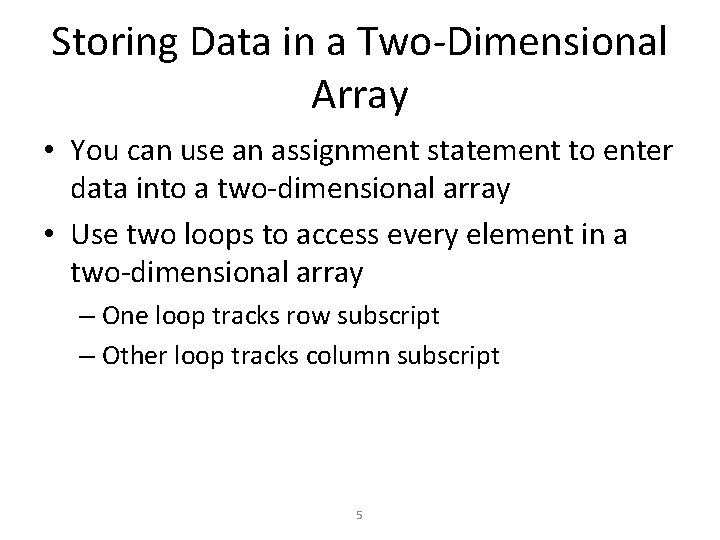
Storing Data in a Two-Dimensional Array • You can use an assignment statement to enter data into a two-dimensional array • Use two loops to access every element in a two-dimensional array – One loop tracks row subscript – Other loop tracks column subscript 5
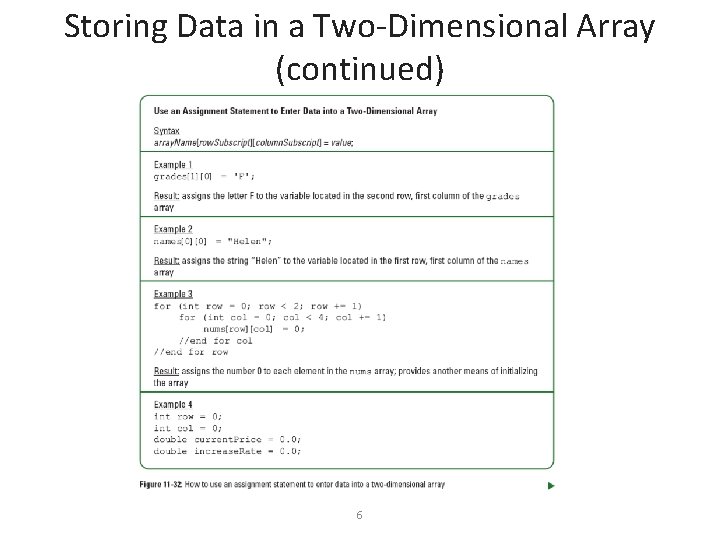
Storing Data in a Two-Dimensional Array (continued) 6
![Example for two dimensional array includeiostream h void main int array23 Example for two dimensional array #include<iostream. h> void main( ) { int array[2][3] =](https://slidetodoc.com/presentation_image_h2/22d5ae2a83c02abfea472735a030799e/image-7.jpg)
Example for two dimensional array #include<iostream. h> void main( ) { int array[2][3] = { {1, 2, 3}, {4, 5, 6} }; int i, j; for (i = 0; i < 2; i++) { for (j = 0; j < 3; j++) cout<<(array[i][j] << " "); } }
![Example2 includeiostream h void main int b 55 int i j for Example(2) #include<iostream. h> void main( ) { int b [5][5]; int i, j; for](https://slidetodoc.com/presentation_image_h2/22d5ae2a83c02abfea472735a030799e/image-8.jpg)
Example(2) #include<iostream. h> void main( ) { int b [5][5]; int i, j; for ( i=0; i<3; i++) for ( j=0; j<3; j++) cin>>b[i][j]; for ( i=0; i<3; i++) { for ( j=0; j<3; j++) cout<<b[i][j]<<" "; cout<<endl; } }
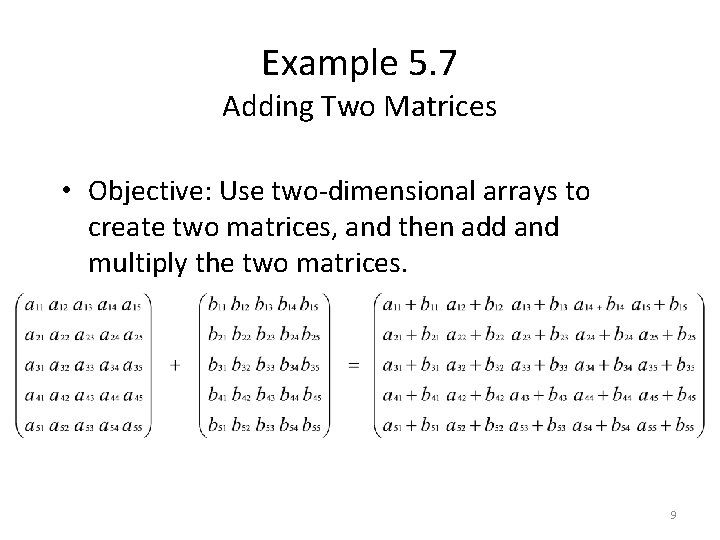
Example 5. 7 Adding Two Matrices • Objective: Use two-dimensional arrays to create two matrices, and then add and multiply the two matrices. 9
![Example includeiostream h void main int i j int b 55 int Example #include<iostream. h> void main( ) { int i, j; int b [5][5]; int](https://slidetodoc.com/presentation_image_h2/22d5ae2a83c02abfea472735a030799e/image-10.jpg)
Example #include<iostream. h> void main( ) { int i, j; int b [5][5]; int a [5][5]; int c [5][5]; for ( i=0; i<3; i++) for ( j=0; j<3; j++) { cin>>a[i][j]; cin>>b[i][j]; c[i][j]=a[i][j]+b[i][j]; } for ( i=0; i<3; i++) { for ( j=0; j<3; j++) cout<<c[i][j]<<" "; cout<<endl; } }
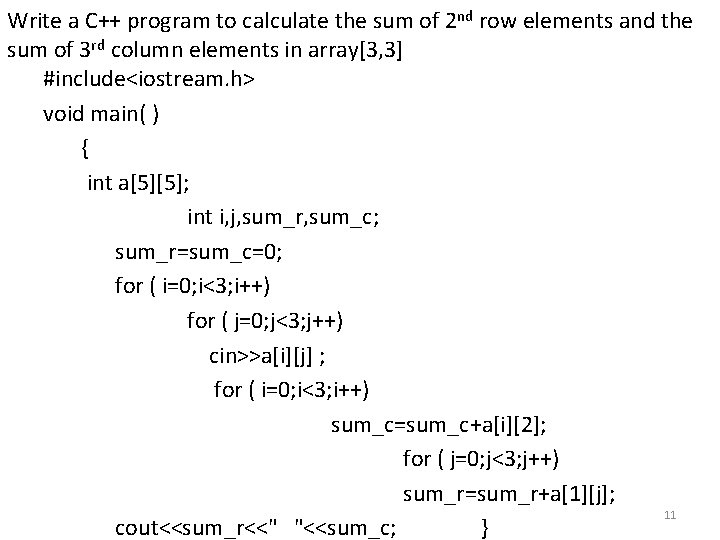
Write a C++ program to calculate the sum of 2 nd row elements and the sum of 3 rd column elements in array[3, 3] #include<iostream. h> void main( ) { int a[5][5]; int i, j, sum_r, sum_c; sum_r=sum_c=0; for ( i=0; i<3; i++) for ( j=0; j<3; j++) cin>>a[i][j] ; for ( i=0; i<3; i++) sum_c=sum_c+a[i][2]; for ( j=0; j<3; j++) sum_r=sum_r+a[1][j]; 11 cout<<sum_r<<" "<<sum_c; }
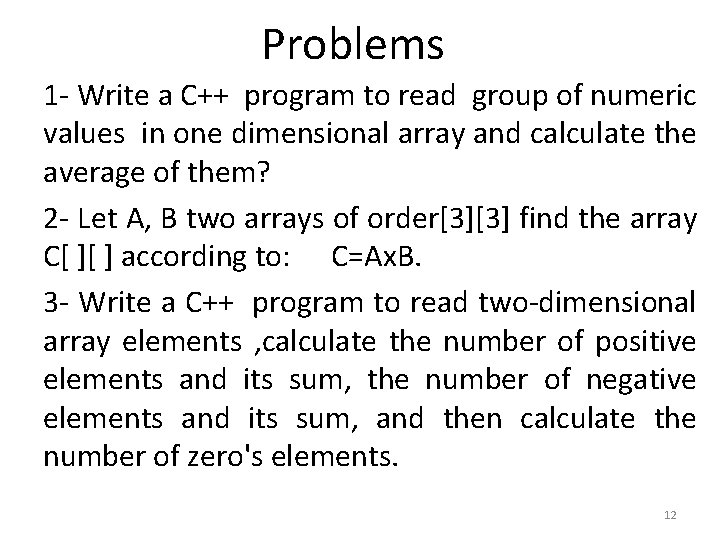
Problems 1 - Write a C++ program to read group of numeric values in one dimensional array and calculate the average of them? 2 - Let A, B two arrays of order[3][3] find the array C[ ][ ] according to: C=Ax. B. 3 - Write a C++ program to read two-dimensional array elements , calculate the number of positive elements and its sum, the number of negative elements and its sum, and then calculate the number of zero's elements. 12
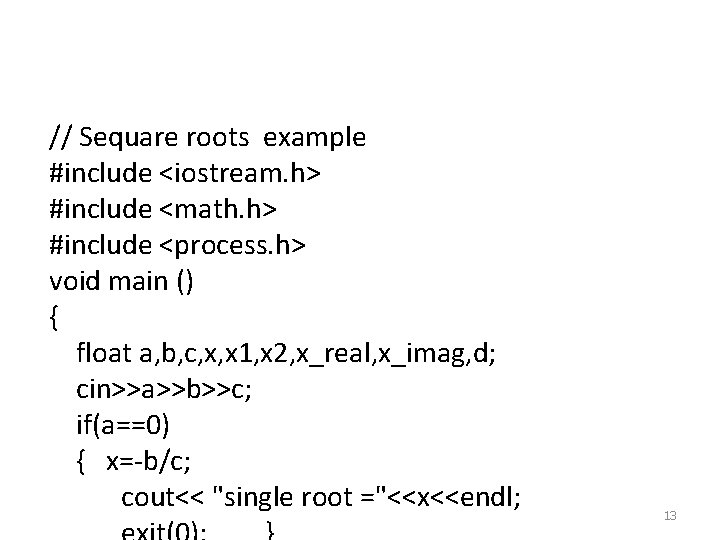
// Sequare roots example #include <iostream. h> #include <math. h> #include <process. h> void main () { float a, b, c, x, x 1, x 2, x_real, x_imag, d; cin>>a>>b>>c; if(a==0) { x=-b/c; cout<< "single root ="<<x<<endl; 13
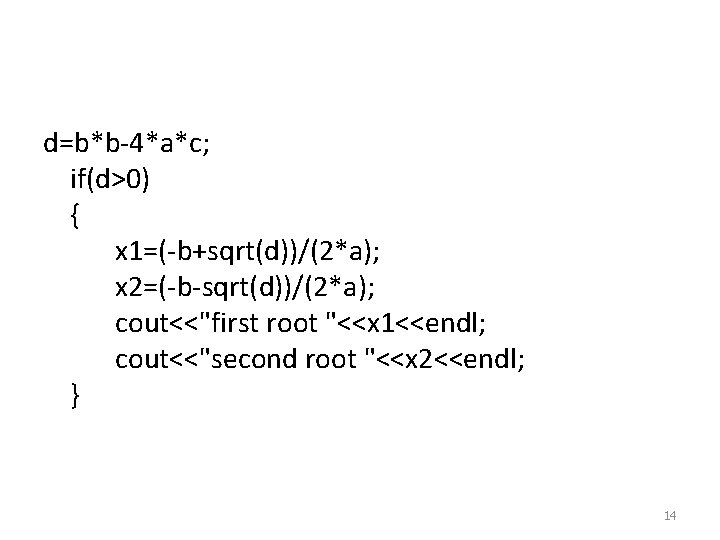
d=b*b-4*a*c; if(d>0) { x 1=(-b+sqrt(d))/(2*a); x 2=(-b-sqrt(d))/(2*a); cout<<"first root "<<x 1<<endl; cout<<"second root "<<x 2<<endl; } 14
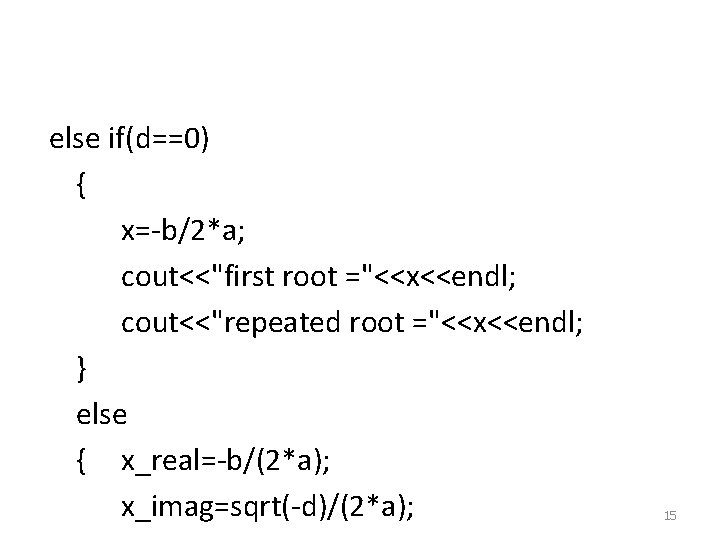
else if(d==0) { x=-b/2*a; cout<<"first root ="<<x<<endl; cout<<"repeated root ="<<x<<endl; } else { x_real=-b/(2*a); x_imag=sqrt(-d)/(2*a); 15
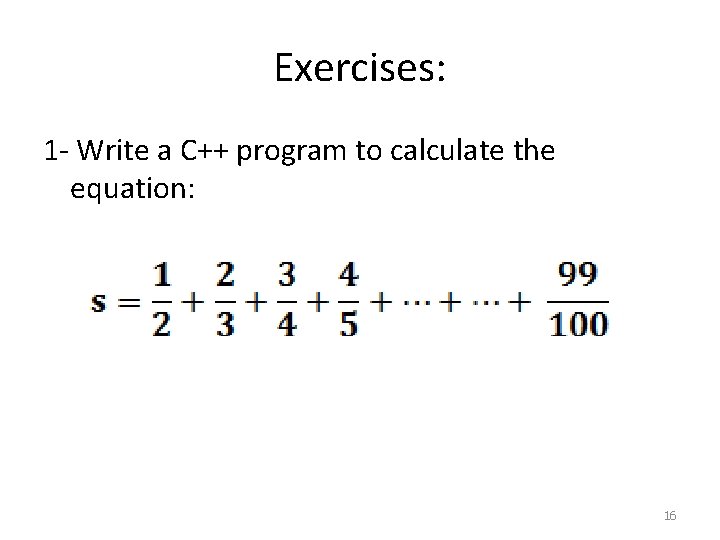
Exercises: 1 - Write a C++ program to calculate the equation: 16
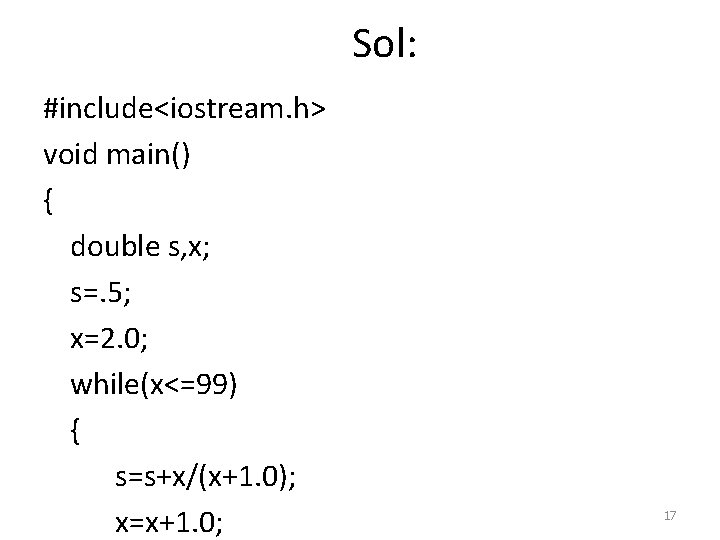
Sol: #include<iostream. h> void main() { double s, x; s=. 5; x=2. 0; while(x<=99) { s=s+x/(x+1. 0); x=x+1. 0; 17
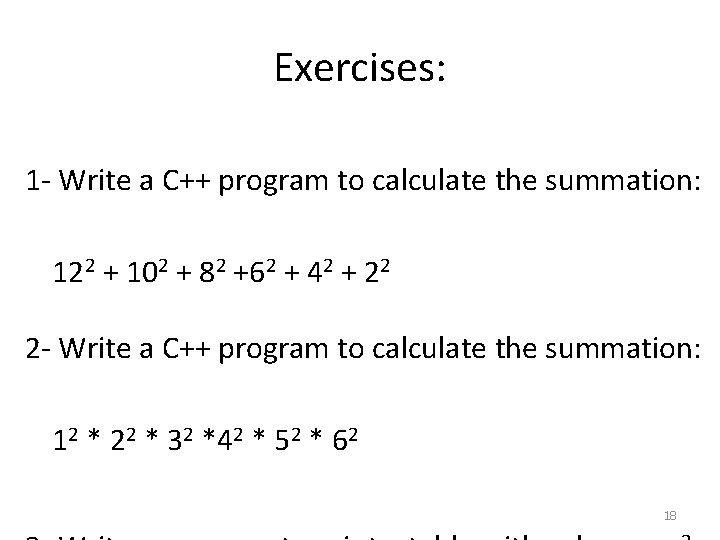
Exercises: 1 - Write a C++ program to calculate the summation: 122 + 102 + 82 +62 + 42 + 22 2 - Write a C++ program to calculate the summation: 12 * 22 * 32 *42 * 52 * 62 18
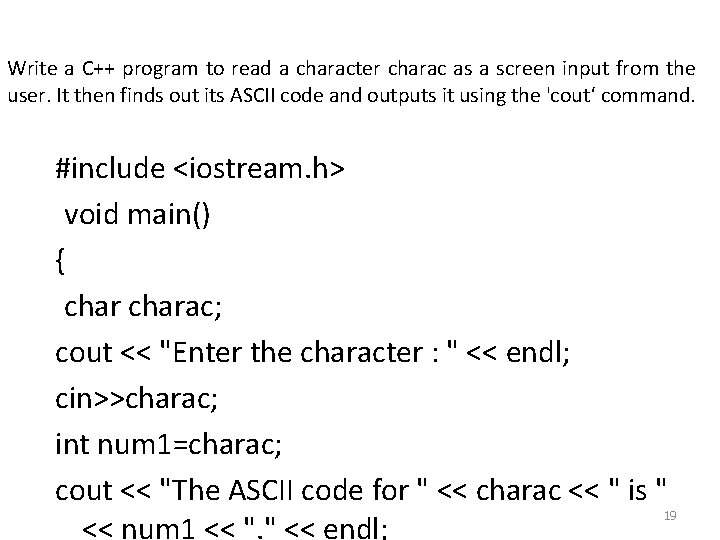
Write a C++ program to read a character charac as a screen input from the user. It then finds out its ASCII code and outputs it using the 'cout‘ command. #include <iostream. h> void main() { charac; cout << "Enter the character : " << endl; cin>>charac; int num 1=charac; cout << "The ASCII code for " << charac << " is " << num 1 << ". " << endl; 19
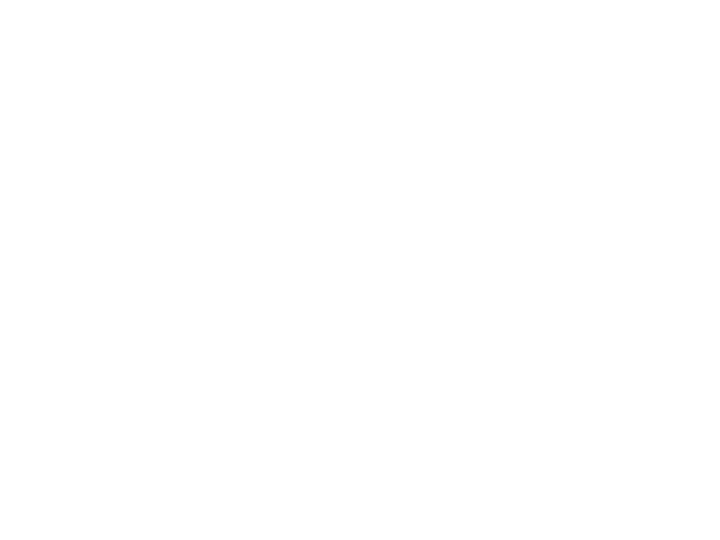
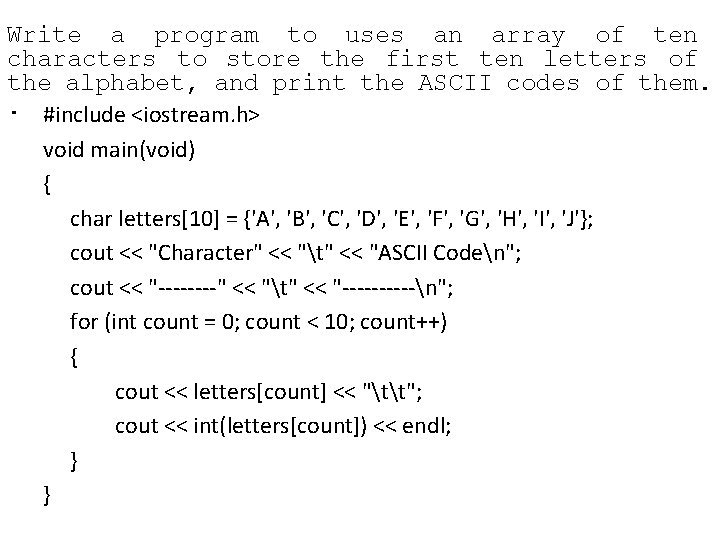
Write a program to uses an array of ten characters to store the first ten letters of the alphabet, and print the ASCII codes of them. . #include <iostream. h> void main(void) { char letters[10] = {'A', 'B', 'C', 'D', 'E', 'F', 'G', 'H', 'I', 'J'}; cout << "Character" << "t" << "ASCII Coden"; cout << "----" << "t" << "-----n"; for (int count = 0; count < 10; count++) { cout << letters[count] << "tt"; cout << int(letters[count]) << endl; } }
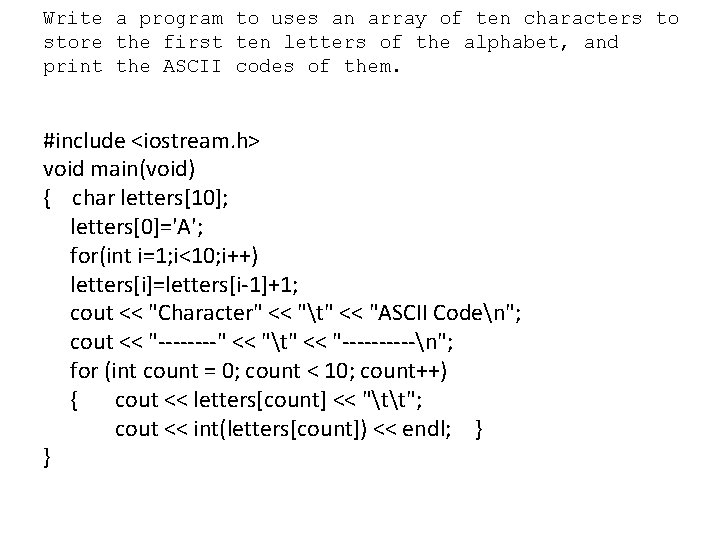
Write a program to uses an array of ten characters to store the first ten letters of the alphabet, and print the ASCII codes of them. #include <iostream. h> void main(void) { char letters[10]; letters[0]='A'; for(int i=1; i<10; i++) letters[i]=letters[i-1]+1; cout << "Character" << "t" << "ASCII Coden"; cout << "----" << "t" << "-----n"; for (int count = 0; count < 10; count++) { cout << letters[count] << "tt"; cout << int(letters[count]) << endl; } }
Arrays in pascal
Cobol pascal
Side language
Day 3: arrays
Arreglo java
Advantages of static memory allocation
Declare array in mips
Parallel array java
Global arrays in c
Strings in assembly language
Redundant arrays of independent disks
Parallel arrays
Python find index of max
Microled arrays
Arreglo java
Array of arrays c++
Creating arrays matlab
Computer science arrays
Python list of arrays
Why do we need arrays?
Raid redundant array of inexpensive disks
Advantages and disadvantages of array
Arrays mips