Chapter VIII Image Texturing 3 D Graphics for
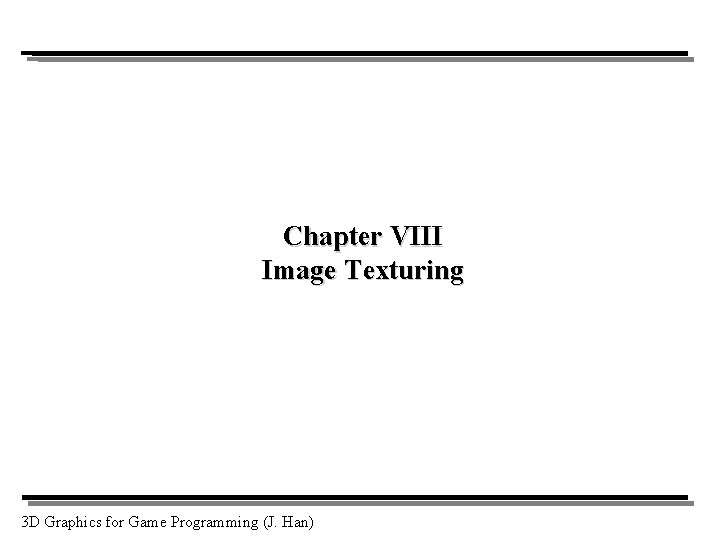
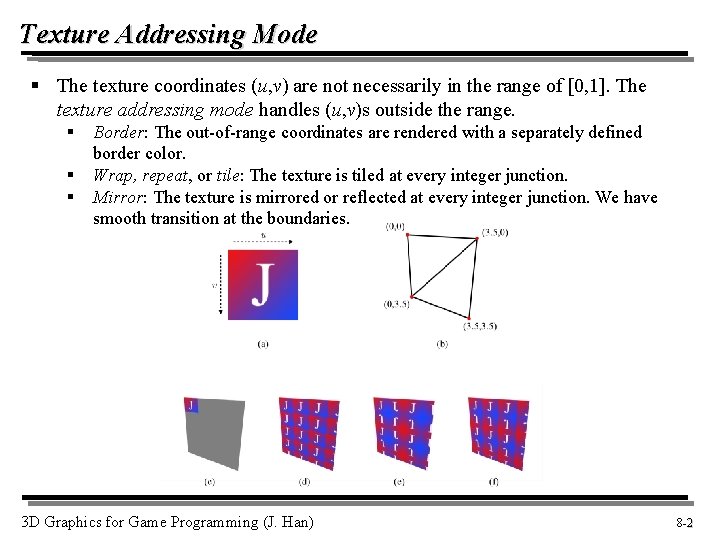
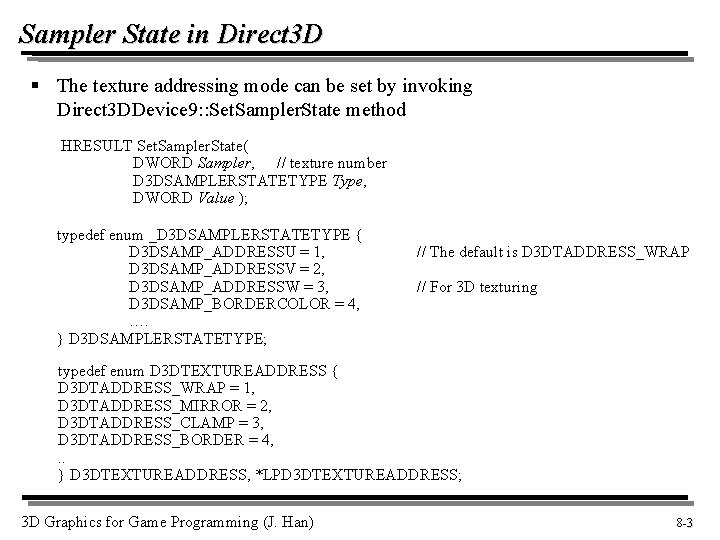
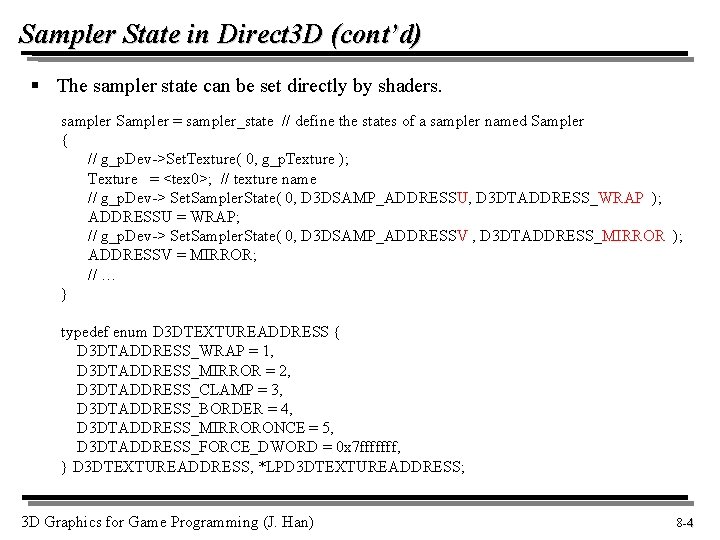
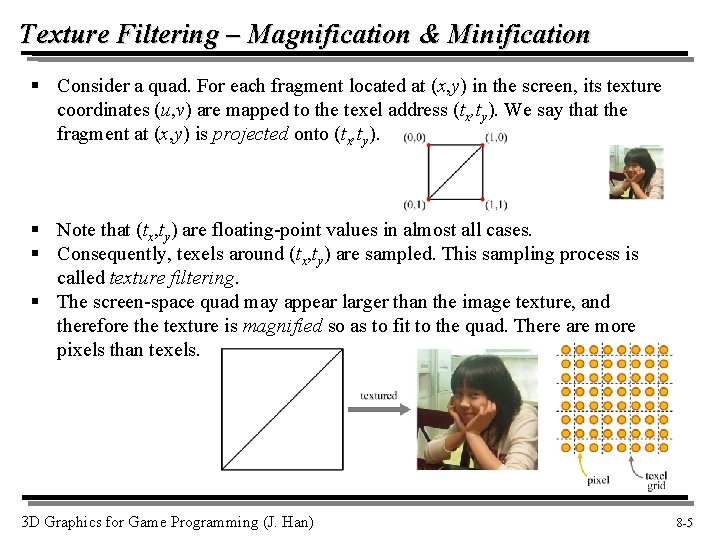
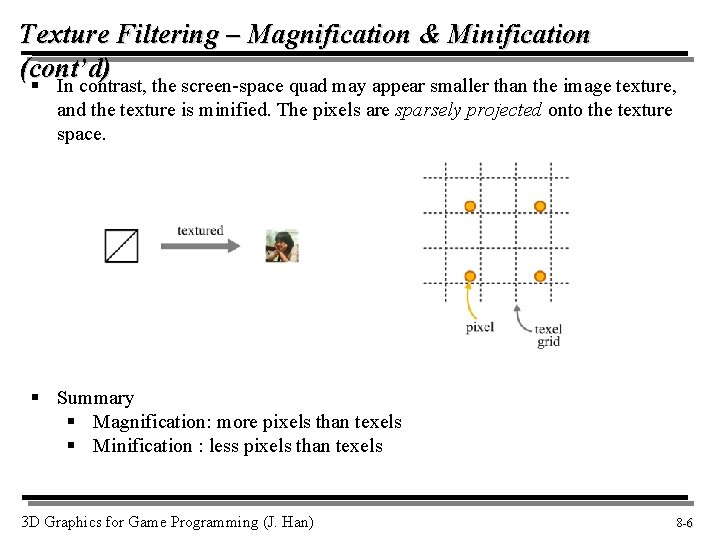
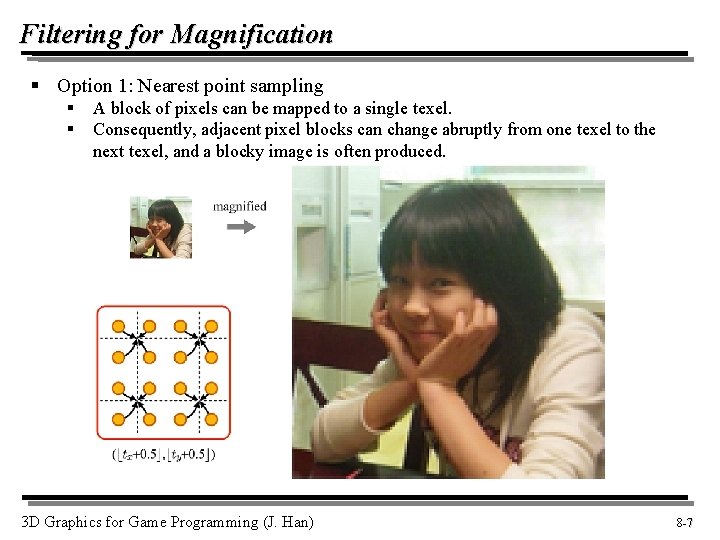
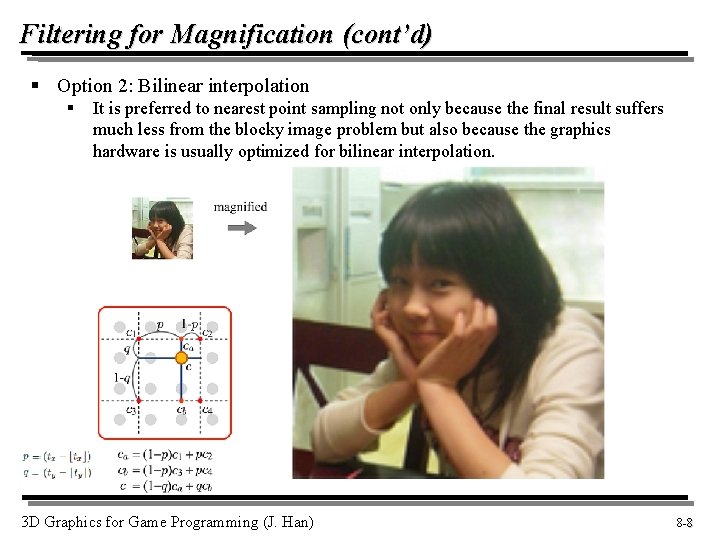
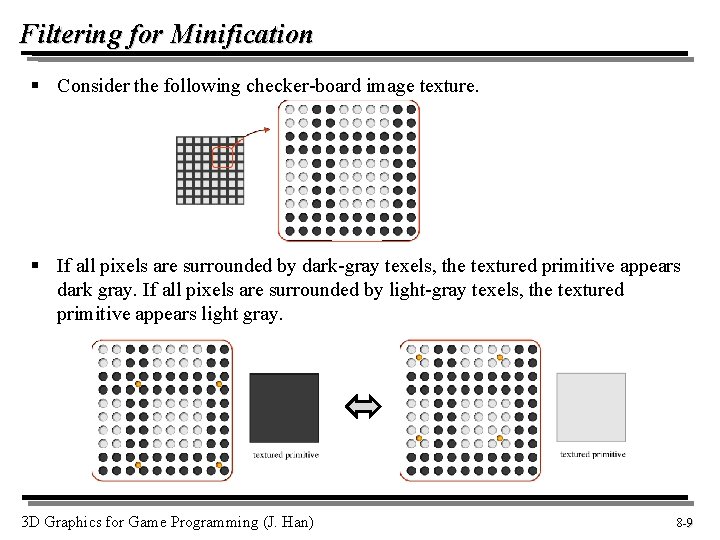
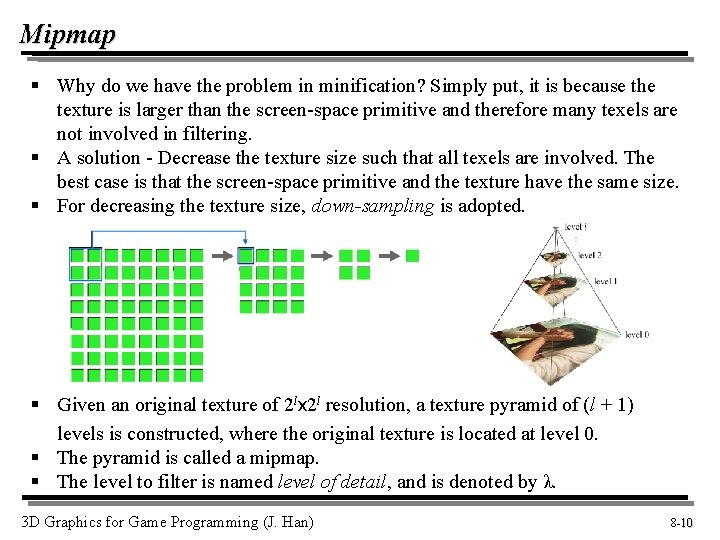
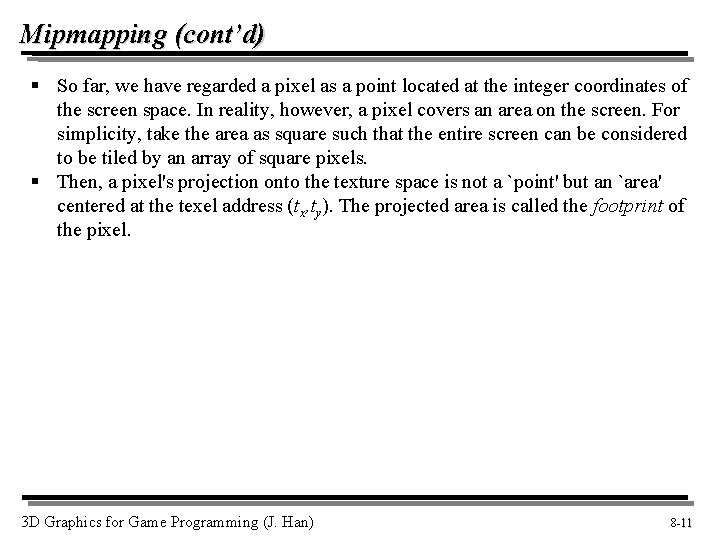
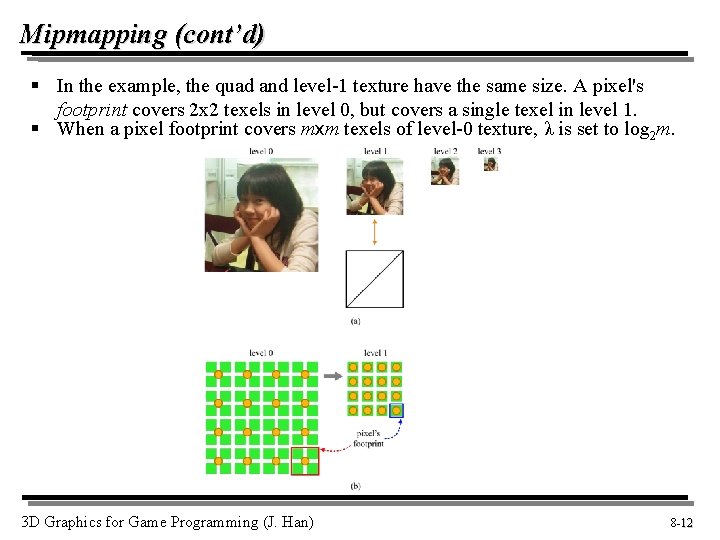
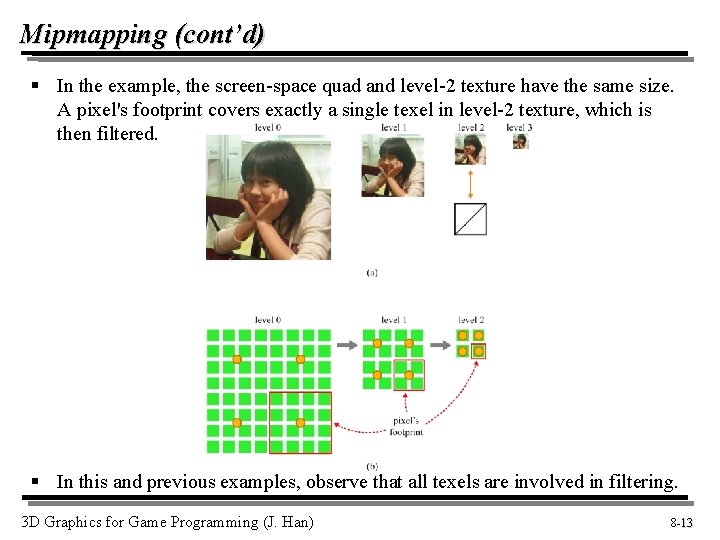
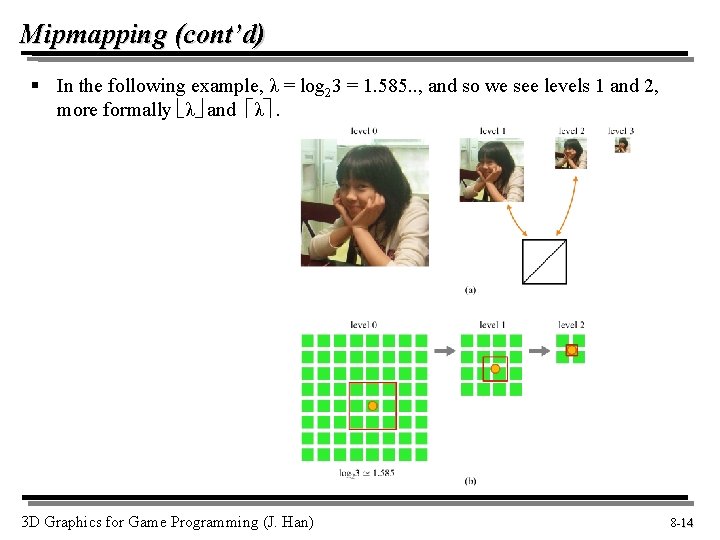
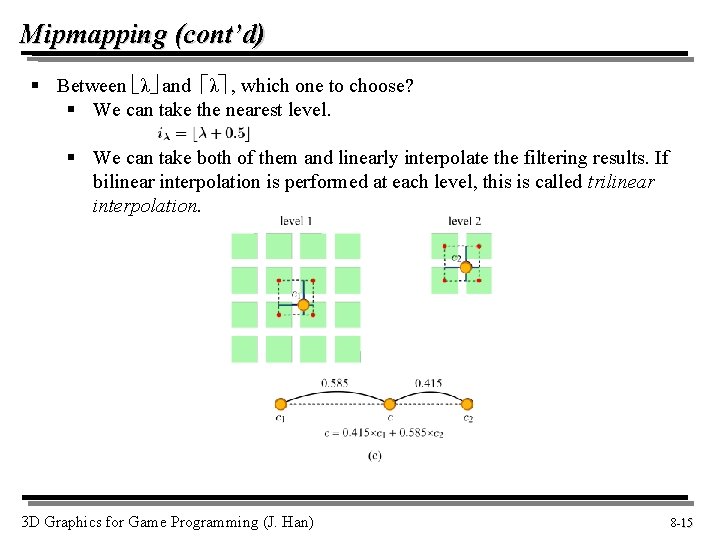
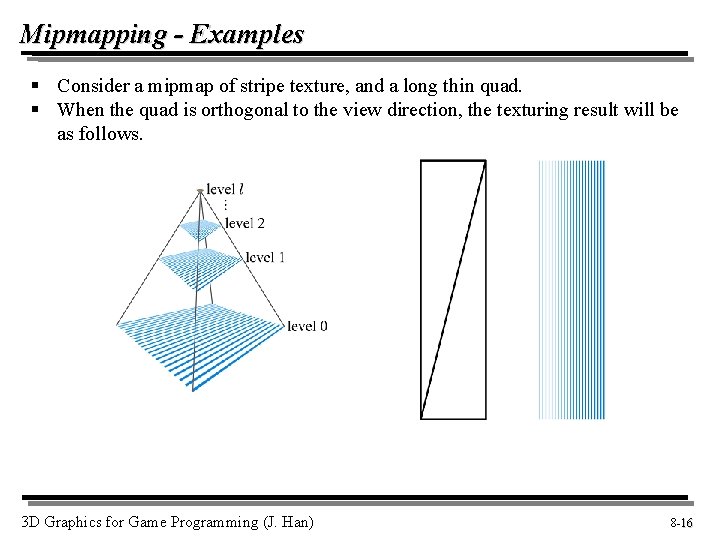
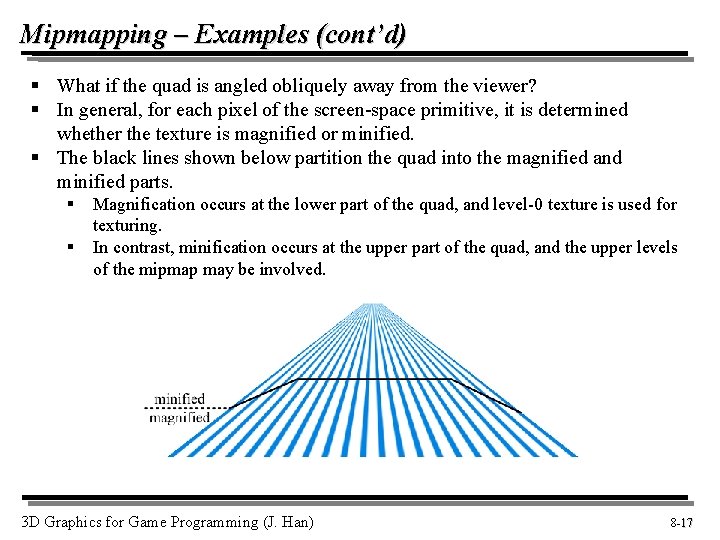
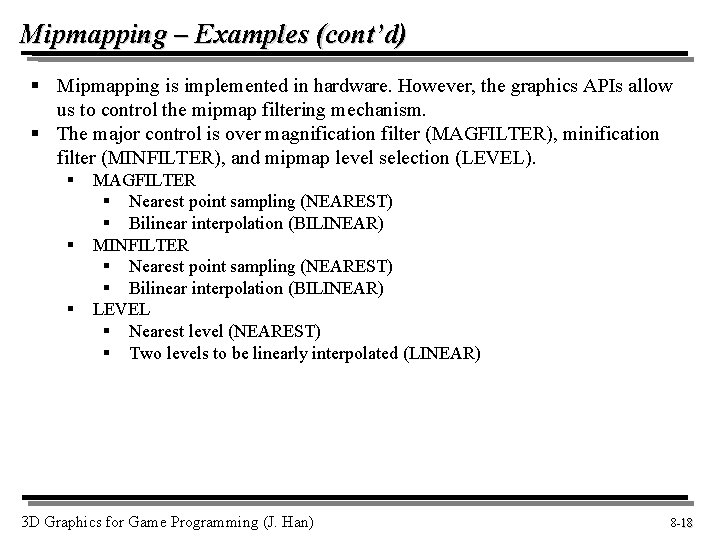
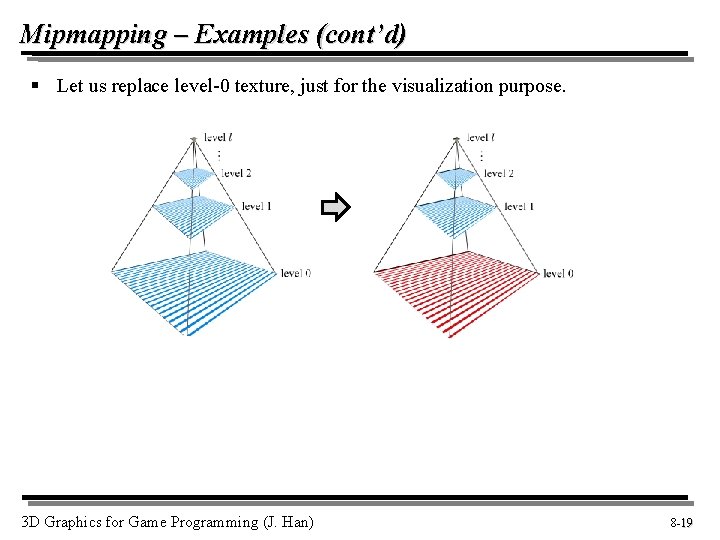
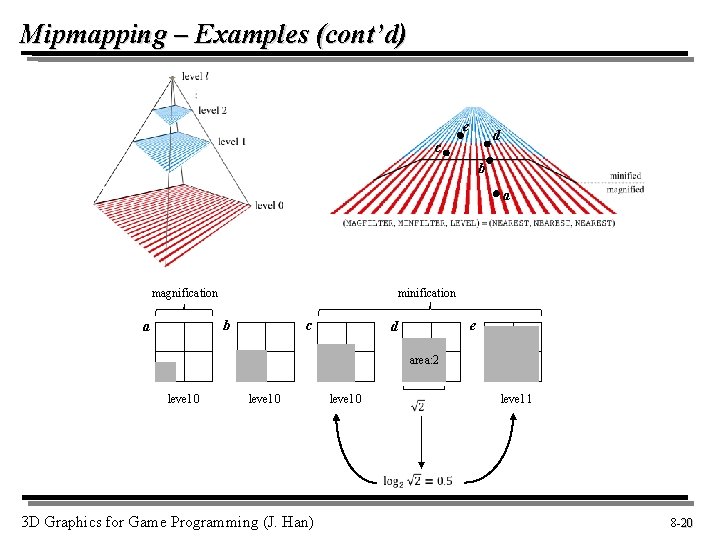
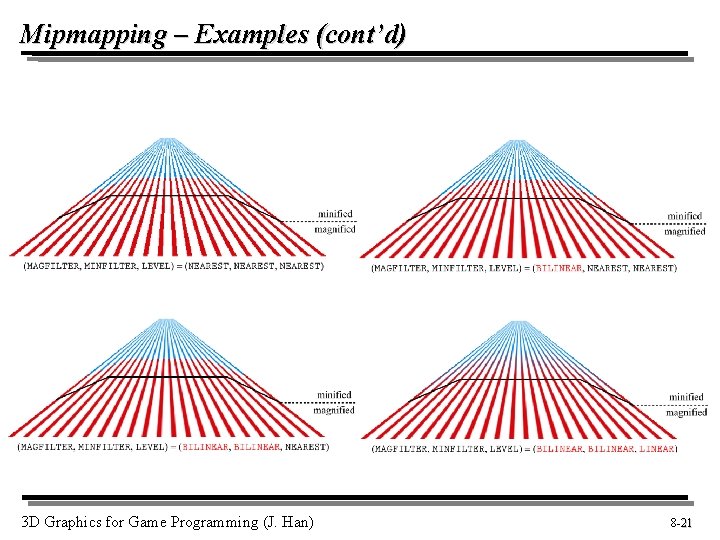
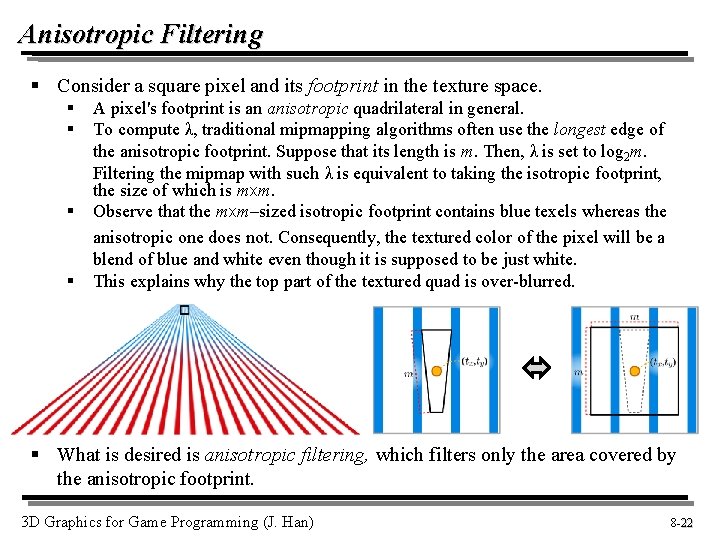
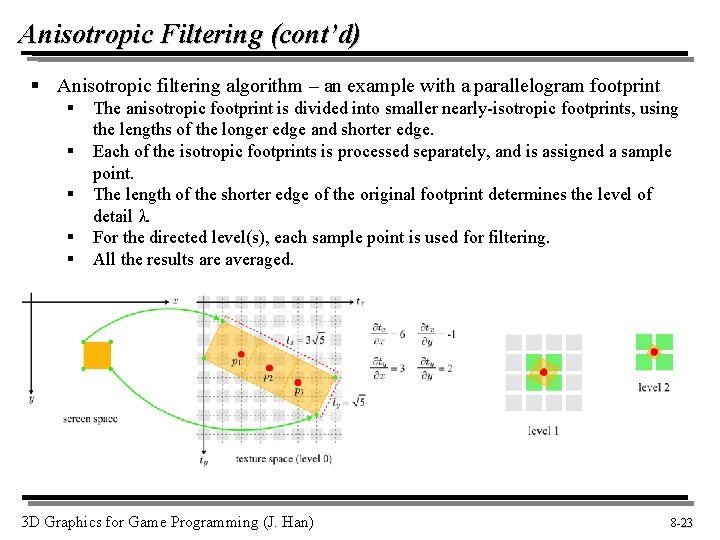
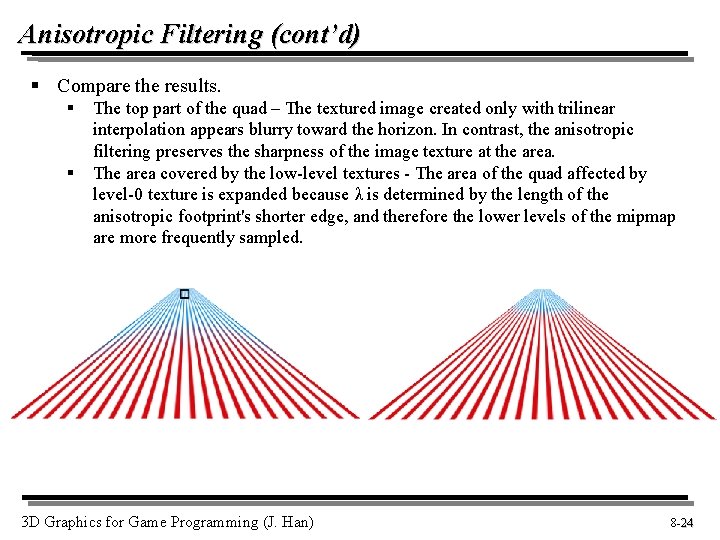
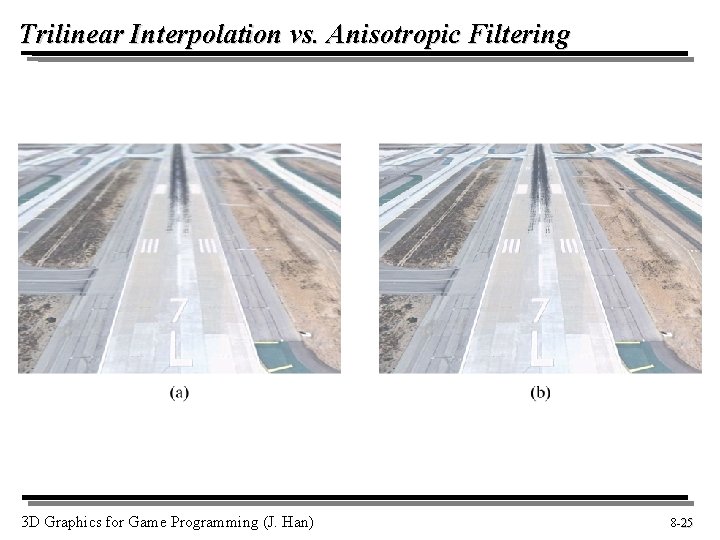
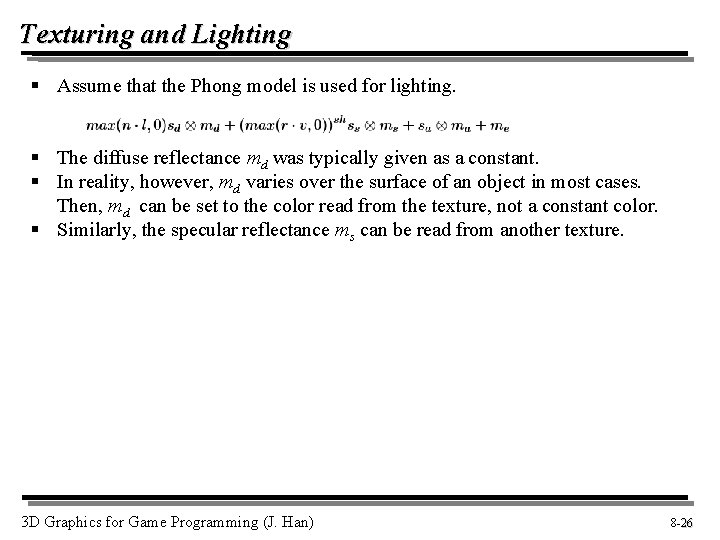
- Slides: 26
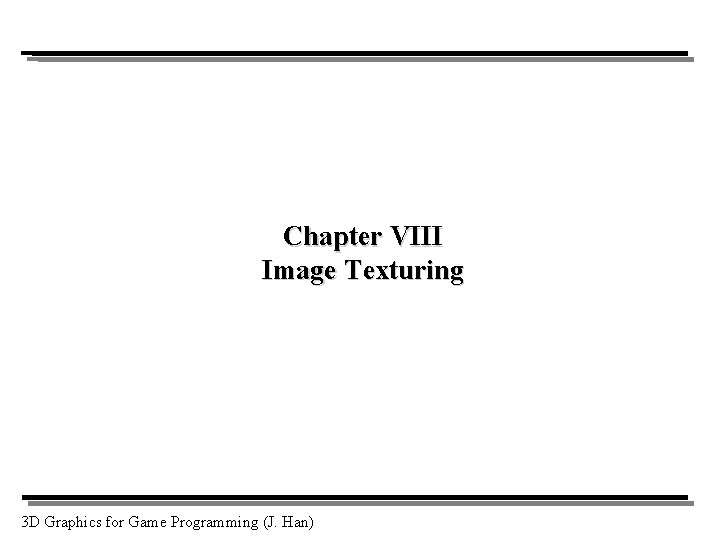
Chapter VIII Image Texturing 3 D Graphics for Game Programming (J. Han)
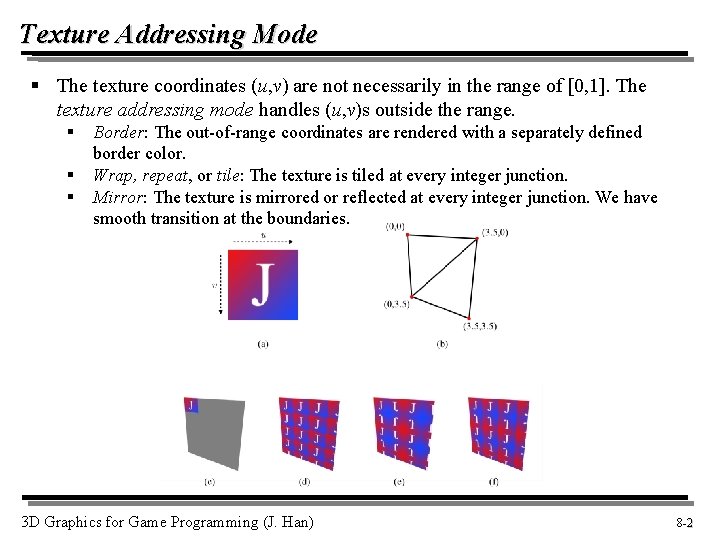
Texture Addressing Mode § The texture coordinates (u, v) are not necessarily in the range of [0, 1]. The texture addressing mode handles (u, v)s outside the range. § § § Border: The out-of-range coordinates are rendered with a separately defined border color. Wrap, repeat, or tile: The texture is tiled at every integer junction. Mirror: The texture is mirrored or reflected at every integer junction. We have smooth transition at the boundaries. 3 D Graphics for Game Programming (J. Han) 8 -2 2
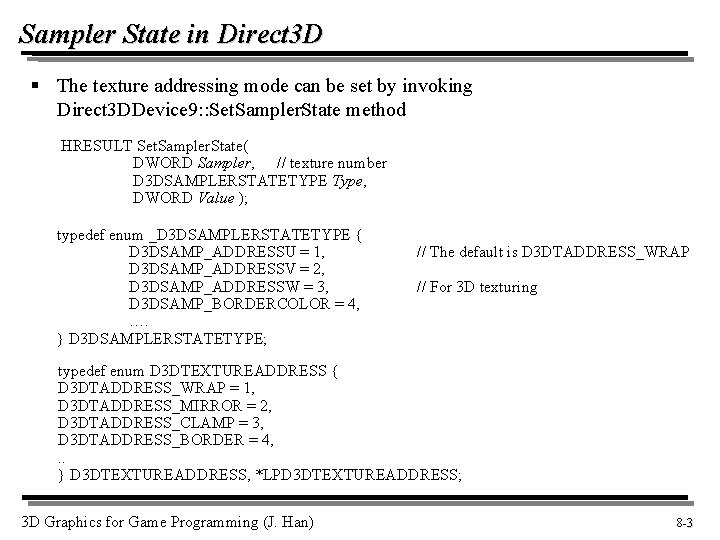
Sampler State in Direct 3 D § The texture addressing mode can be set by invoking Direct 3 DDevice 9: : Set. Sampler. State method HRESULT Set. Sampler. State( DWORD Sampler, // texture number D 3 DSAMPLERSTATETYPE Type, DWORD Value ); typedef enum _D 3 DSAMPLERSTATETYPE { D 3 DSAMP_ADDRESSU = 1, D 3 DSAMP_ADDRESSV = 2, D 3 DSAMP_ADDRESSW = 3, D 3 DSAMP_BORDERCOLOR = 4, …. } D 3 DSAMPLERSTATETYPE; // The default is D 3 DTADDRESS_WRAP // For 3 D texturing typedef enum D 3 DTEXTUREADDRESS { D 3 DTADDRESS_WRAP = 1, D 3 DTADDRESS_MIRROR = 2, D 3 DTADDRESS_CLAMP = 3, D 3 DTADDRESS_BORDER = 4, . . } D 3 DTEXTUREADDRESS, *LPD 3 DTEXTUREADDRESS; 3 D Graphics for Game Programming (J. Han) 8 -3 3
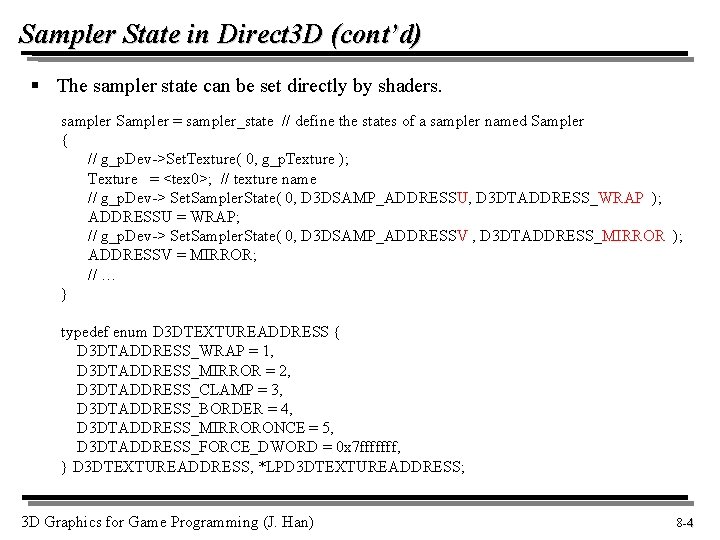
Sampler State in Direct 3 D (cont’d) § The sampler state can be set directly by shaders. sampler Sampler = sampler_state // define the states of a sampler named Sampler { // g_p. Dev->Set. Texture( 0, g_p. Texture ); Texture = <tex 0>; // texture name // g_p. Dev-> Set. Sampler. State( 0, D 3 DSAMP_ADDRESSU, D 3 DTADDRESS_WRAP ); ADDRESSU = WRAP; // g_p. Dev-> Set. Sampler. State( 0, D 3 DSAMP_ADDRESSV , D 3 DTADDRESS_MIRROR ); ADDRESSV = MIRROR; // … } typedef enum D 3 DTEXTUREADDRESS { D 3 DTADDRESS_WRAP = 1, D 3 DTADDRESS_MIRROR = 2, D 3 DTADDRESS_CLAMP = 3, D 3 DTADDRESS_BORDER = 4, D 3 DTADDRESS_MIRRORONCE = 5, D 3 DTADDRESS_FORCE_DWORD = 0 x 7 fffffff, } D 3 DTEXTUREADDRESS, *LPD 3 DTEXTUREADDRESS; 3 D Graphics for Game Programming (J. Han) 8 -4 4
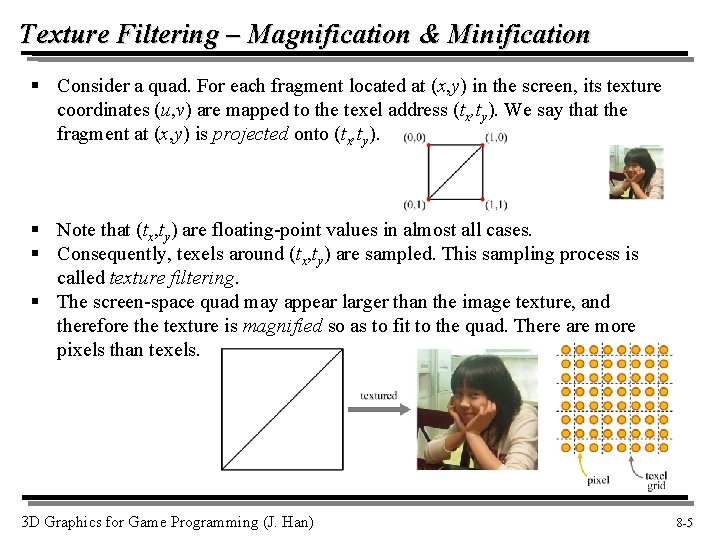
Texture Filtering – Magnification & Minification § Consider a quad. For each fragment located at (x, y) in the screen, its texture coordinates (u, v) are mapped to the texel address (tx, ty). We say that the fragment at (x, y) is projected onto (tx, ty). § Note that (tx, ty) are floating-point values in almost all cases. § Consequently, texels around (tx, ty) are sampled. This sampling process is called texture filtering. § The screen-space quad may appear larger than the image texture, and therefore the texture is magnified so as to fit to the quad. There are more pixels than texels. 3 D Graphics for Game Programming (J. Han) 8 -5 5
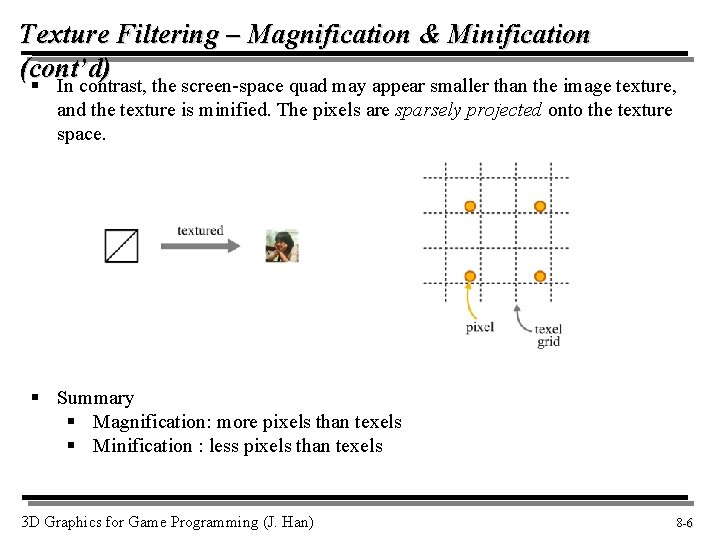
Texture Filtering – Magnification & Minification (cont’d) § In contrast, the screen-space quad may appear smaller than the image texture, and the texture is minified. The pixels are sparsely projected onto the texture space. § Summary § Magnification: more pixels than texels § Minification : less pixels than texels 3 D Graphics for Game Programming (J. Han) 8 -6 6
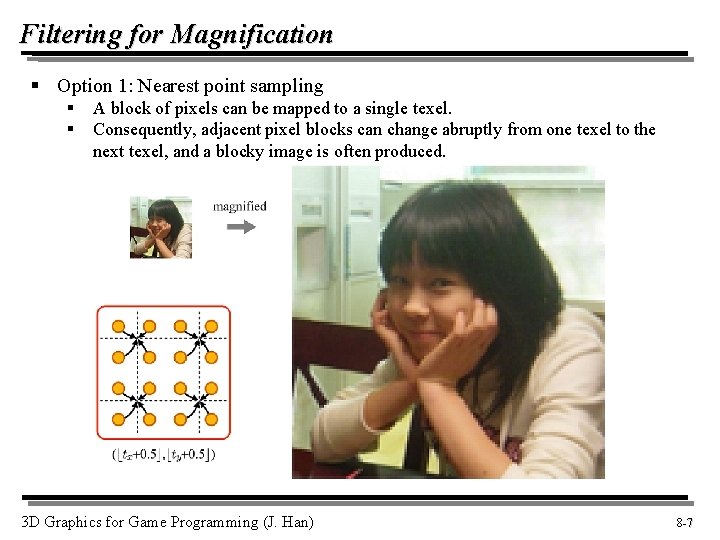
Filtering for Magnification § Option 1: Nearest point sampling § § A block of pixels can be mapped to a single texel. Consequently, adjacent pixel blocks can change abruptly from one texel to the next texel, and a blocky image is often produced. 3 D Graphics for Game Programming (J. Han) 8 -7 7
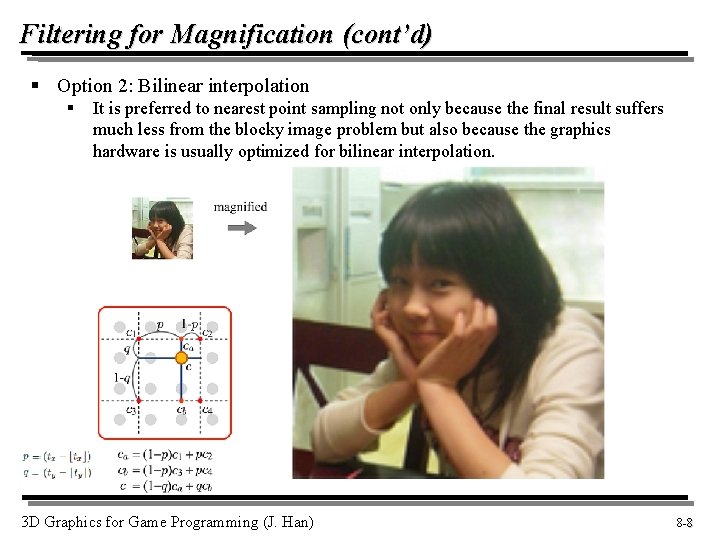
Filtering for Magnification (cont’d) § Option 2: Bilinear interpolation § It is preferred to nearest point sampling not only because the final result suffers much less from the blocky image problem but also because the graphics hardware is usually optimized for bilinear interpolation. 3 D Graphics for Game Programming (J. Han) 8 -8 8
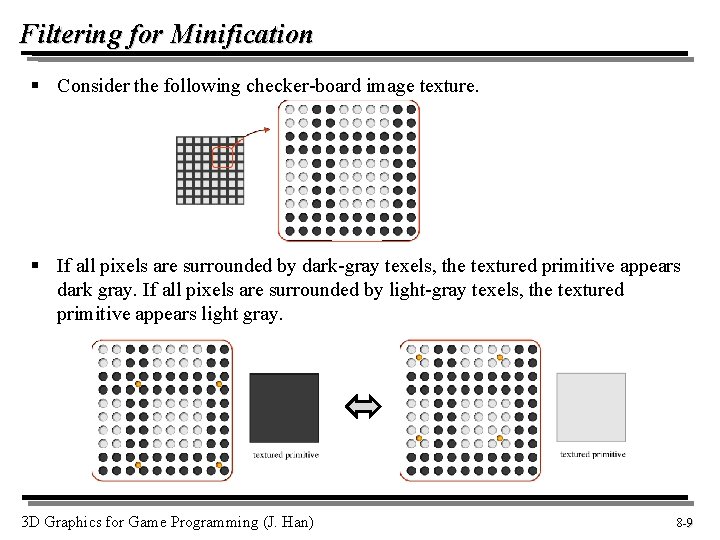
Filtering for Minification § Consider the following checker-board image texture. § If all pixels are surrounded by dark-gray texels, the textured primitive appears dark gray. If all pixels are surrounded by light-gray texels, the textured primitive appears light gray. 3 D Graphics for Game Programming (J. Han) 8 -9 9
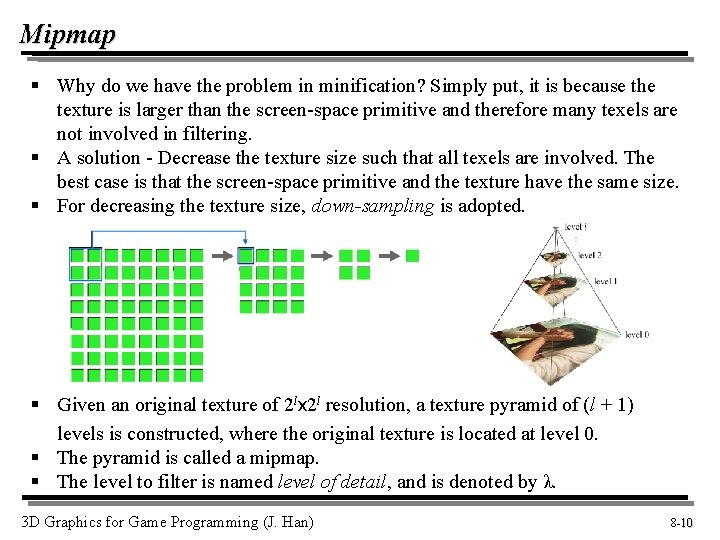
Mipmap § Why do we have the problem in minification? Simply put, it is because the texture is larger than the screen-space primitive and therefore many texels are not involved in filtering. § A solution - Decrease the texture size such that all texels are involved. The best case is that the screen-space primitive and the texture have the same size. § For decreasing the texture size, down-sampling is adopted. § Given an original texture of 2 lx 2 l resolution, a texture pyramid of (l + 1) levels is constructed, where the original texture is located at level 0. § The pyramid is called a mipmap. § The level to filter is named level of detail, and is denoted by λ. 3 D Graphics for Game Programming (J. Han) 8 -10 10
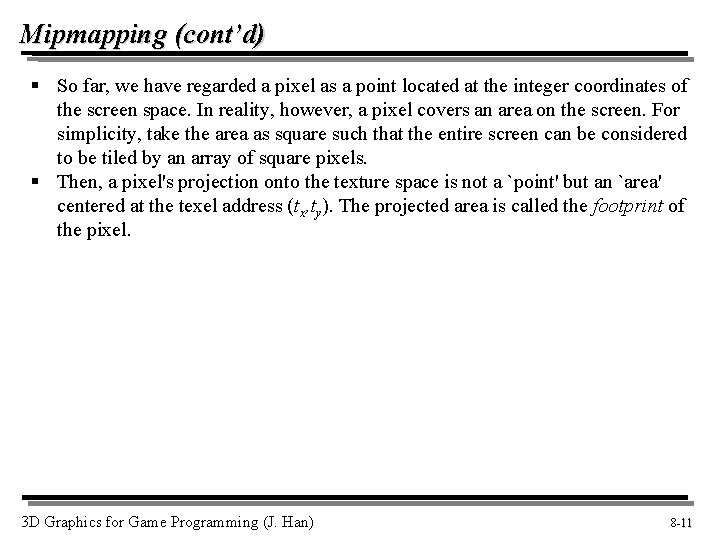
Mipmapping (cont’d) § So far, we have regarded a pixel as a point located at the integer coordinates of the screen space. In reality, however, a pixel covers an area on the screen. For simplicity, take the area as square such that the entire screen can be considered to be tiled by an array of square pixels. § Then, a pixel's projection onto the texture space is not a `point' but an `area' centered at the texel address (tx, ty). The projected area is called the footprint of the pixel. 3 D Graphics for Game Programming (J. Han) 8 -11 11
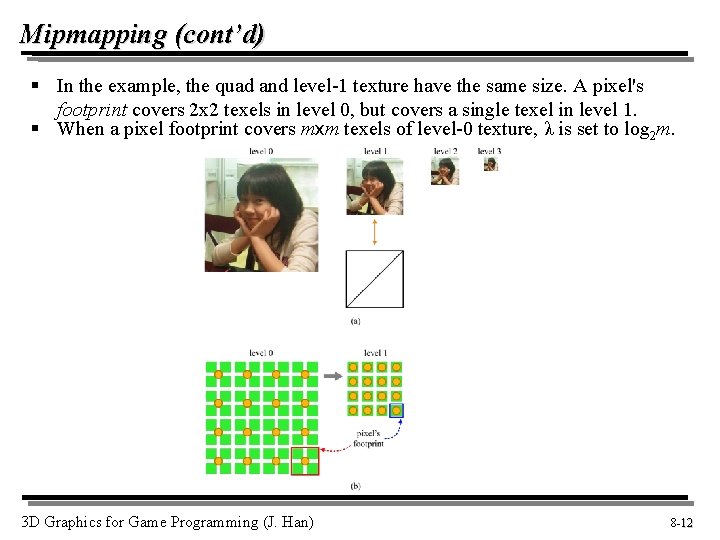
Mipmapping (cont’d) § In the example, the quad and level-1 texture have the same size. A pixel's footprint covers 2 x 2 texels in level 0, but covers a single texel in level 1. § When a pixel footprint covers mxm texels of level-0 texture, λ is set to log 2 m. 3 D Graphics for Game Programming (J. Han) 8 -12 12
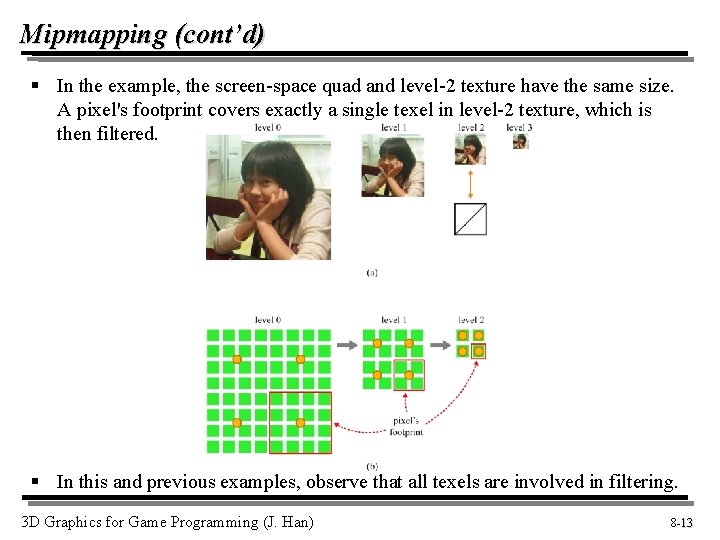
Mipmapping (cont’d) § In the example, the screen-space quad and level-2 texture have the same size. A pixel's footprint covers exactly a single texel in level-2 texture, which is then filtered. § In this and previous examples, observe that all texels are involved in filtering. 3 D Graphics for Game Programming (J. Han) 8 -13 13
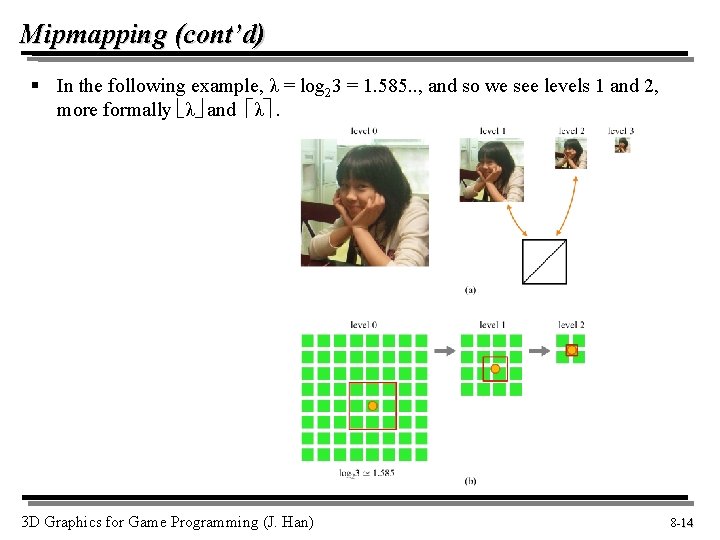
Mipmapping (cont’d) § In the following example, λ = log 23 = 1. 585. . , and so we see levels 1 and 2, more formally λ and λ . 3 D Graphics for Game Programming (J. Han) 8 -14 14
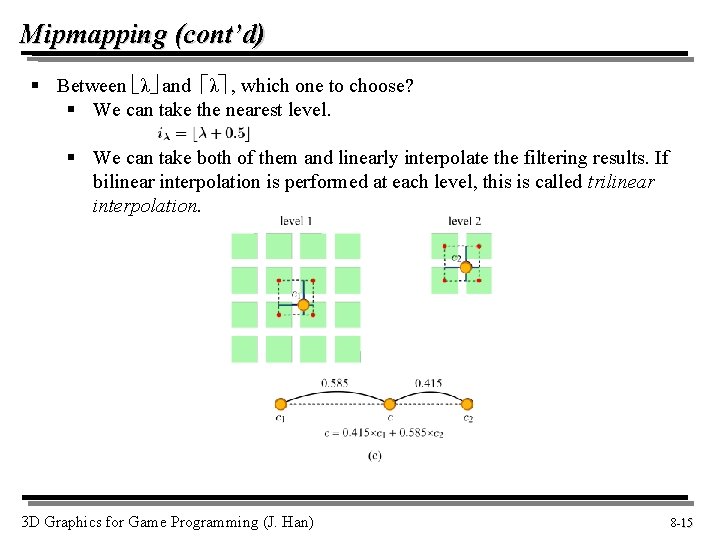
Mipmapping (cont’d) § Between λ and λ , which one to choose? § We can take the nearest level. § We can take both of them and linearly interpolate the filtering results. If bilinear interpolation is performed at each level, this is called trilinear interpolation. 3 D Graphics for Game Programming (J. Han) 8 -15 15
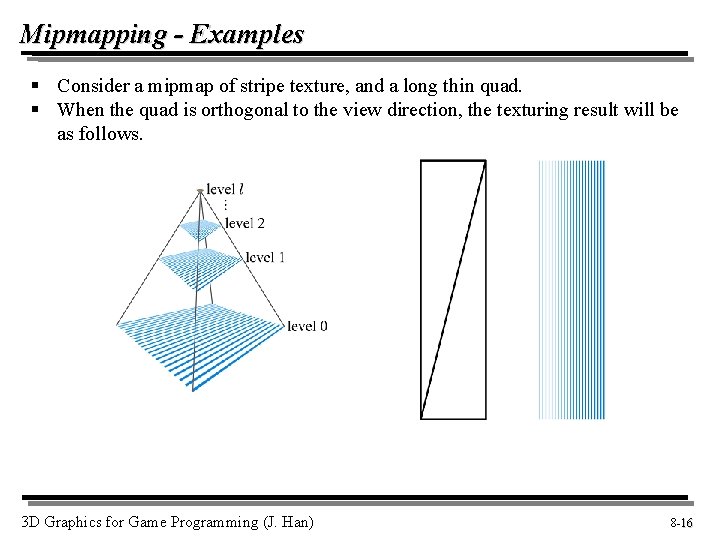
Mipmapping - Examples § Consider a mipmap of stripe texture, and a long thin quad. § When the quad is orthogonal to the view direction, the texturing result will be as follows. 3 D Graphics for Game Programming (J. Han) 8 -16 16
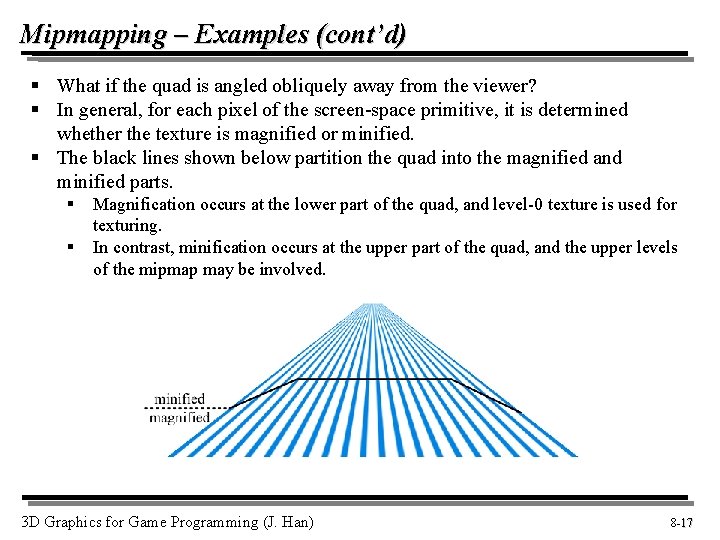
Mipmapping – Examples (cont’d) § What if the quad is angled obliquely away from the viewer? § In general, for each pixel of the screen-space primitive, it is determined whether the texture is magnified or minified. § The black lines shown below partition the quad into the magnified and minified parts. § § Magnification occurs at the lower part of the quad, and level-0 texture is used for texturing. In contrast, minification occurs at the upper part of the quad, and the upper levels of the mipmap may be involved. 3 D Graphics for Game Programming (J. Han) 8 -17 17
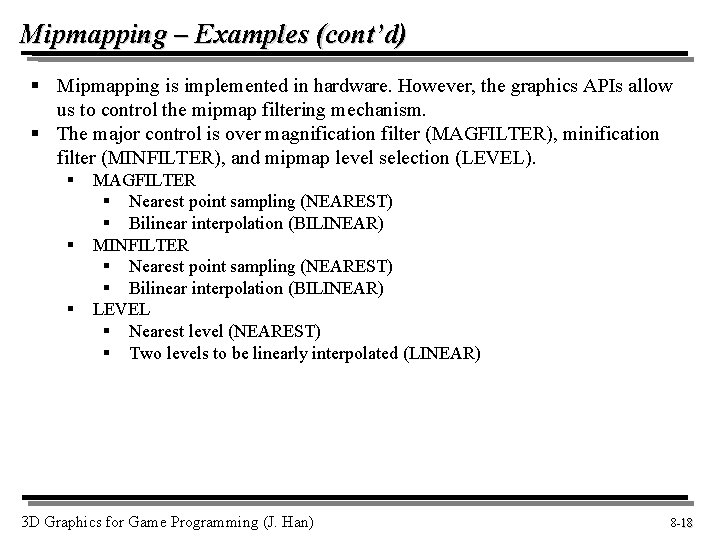
Mipmapping – Examples (cont’d) § Mipmapping is implemented in hardware. However, the graphics APIs allow us to control the mipmap filtering mechanism. § The major control is over magnification filter (MAGFILTER), minification filter (MINFILTER), and mipmap level selection (LEVEL). § § § MAGFILTER § Nearest point sampling (NEAREST) § Bilinear interpolation (BILINEAR) MINFILTER § Nearest point sampling (NEAREST) § Bilinear interpolation (BILINEAR) LEVEL § Nearest level (NEAREST) § Two levels to be linearly interpolated (LINEAR) 3 D Graphics for Game Programming (J. Han) 8 -18 18
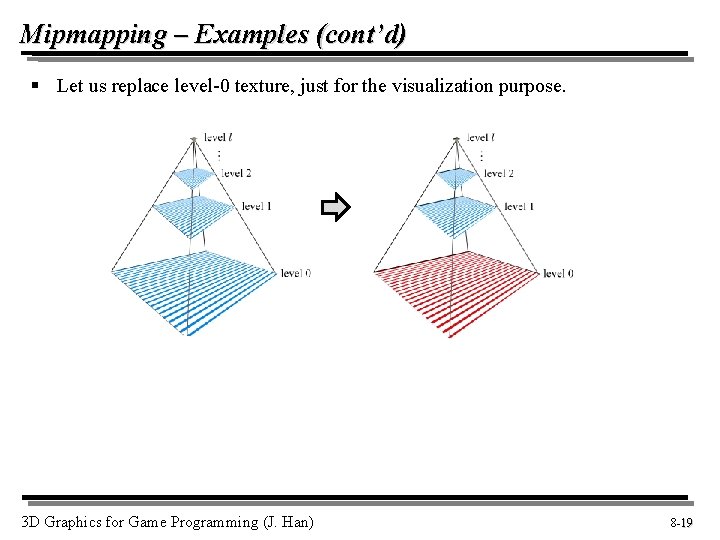
Mipmapping – Examples (cont’d) § Let us replace level-0 texture, just for the visualization purpose. 3 D Graphics for Game Programming (J. Han) 8 -19 19
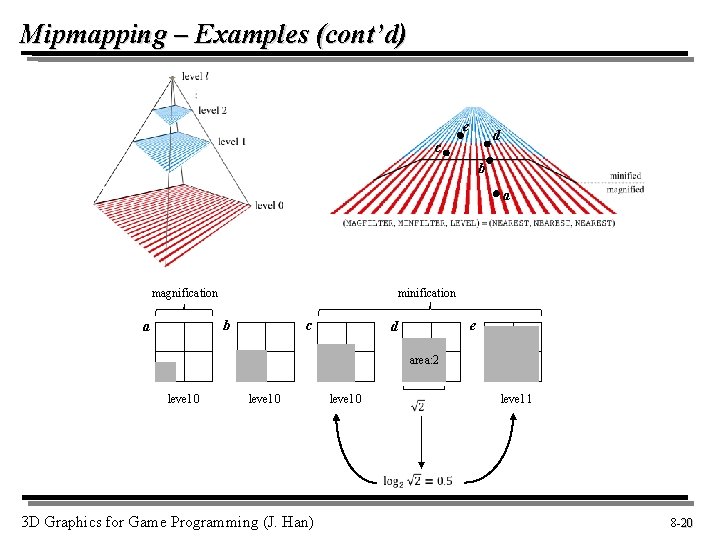
Mipmapping – Examples (cont’d) e d c b a magnification minification c b a e d area: 2 level 0 level 1 3 D Graphics for Game Programming (J. Han) 8 -20 20
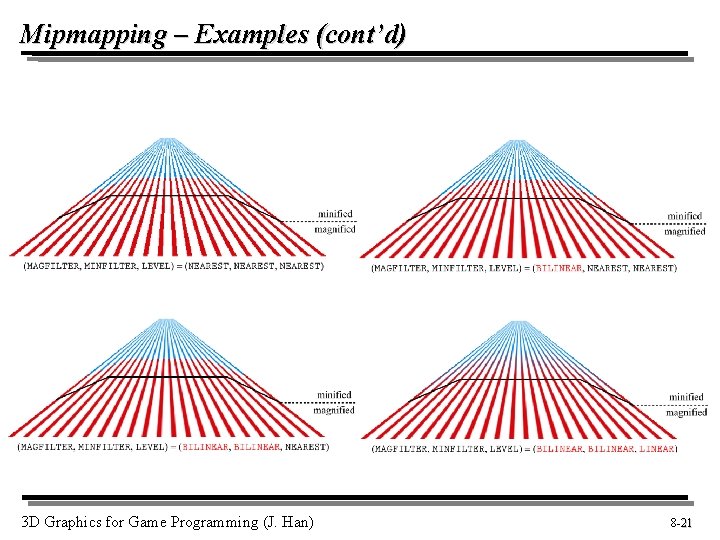
Mipmapping – Examples (cont’d) 3 D Graphics for Game Programming (J. Han) 8 -21 21
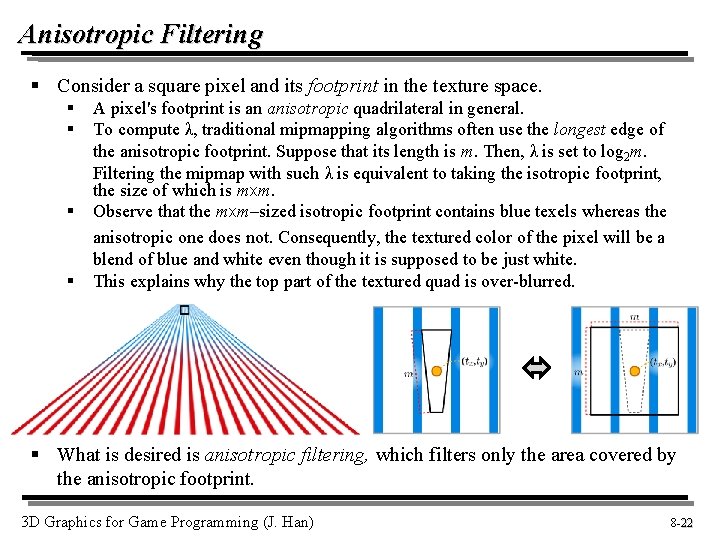
Anisotropic Filtering § Consider a square pixel and its footprint in the texture space. § § A pixel's footprint is an anisotropic quadrilateral in general. To compute λ, traditional mipmapping algorithms often use the longest edge of the anisotropic footprint. Suppose that its length is m. Then, λ is set to log 2 m. Filtering the mipmap with such λ is equivalent to taking the isotropic footprint, the size of which is mxm. Observe that the mxm–sized isotropic footprint contains blue texels whereas the anisotropic one does not. Consequently, the textured color of the pixel will be a blend of blue and white even though it is supposed to be just white. This explains why the top part of the textured quad is over-blurred. § What is desired is anisotropic filtering, which filters only the area covered by the anisotropic footprint. 3 D Graphics for Game Programming (J. Han) 8 -22 22
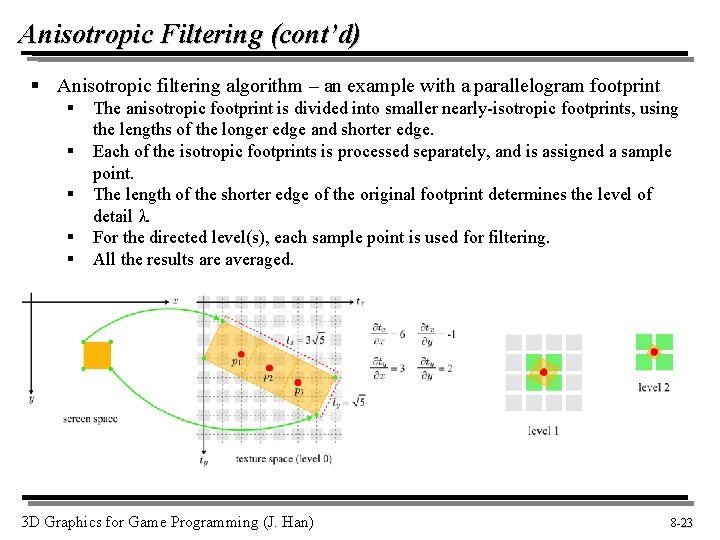
Anisotropic Filtering (cont’d) § Anisotropic filtering algorithm – an example with a parallelogram footprint § § § The anisotropic footprint is divided into smaller nearly-isotropic footprints, using the lengths of the longer edge and shorter edge. Each of the isotropic footprints is processed separately, and is assigned a sample point. The length of the shorter edge of the original footprint determines the level of detail λ. For the directed level(s), each sample point is used for filtering. All the results are averaged. 3 D Graphics for Game Programming (J. Han) 8 -23 23
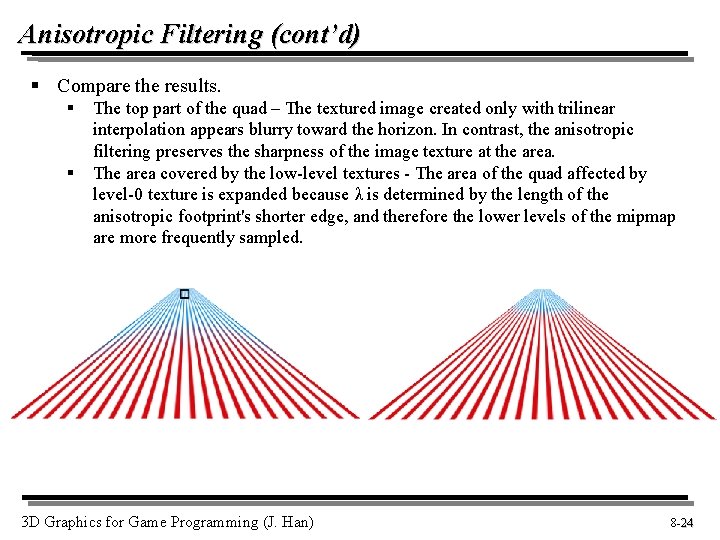
Anisotropic Filtering (cont’d) § Compare the results. § § The top part of the quad – The textured image created only with trilinear interpolation appears blurry toward the horizon. In contrast, the anisotropic filtering preserves the sharpness of the image texture at the area. The area covered by the low-level textures - The area of the quad affected by level-0 texture is expanded because λ is determined by the length of the anisotropic footprint's shorter edge, and therefore the lower levels of the mipmap are more frequently sampled. 3 D Graphics for Game Programming (J. Han) 8 -24 24
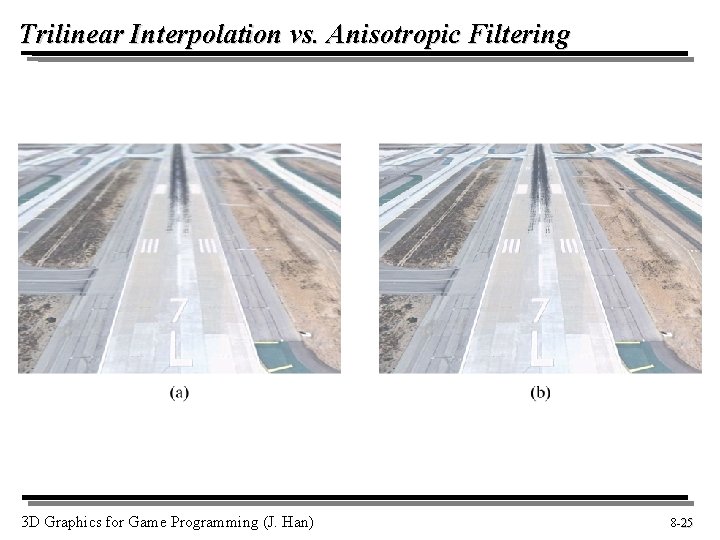
Trilinear Interpolation vs. Anisotropic Filtering 3 D Graphics for Game Programming (J. Han) 8 -25 25
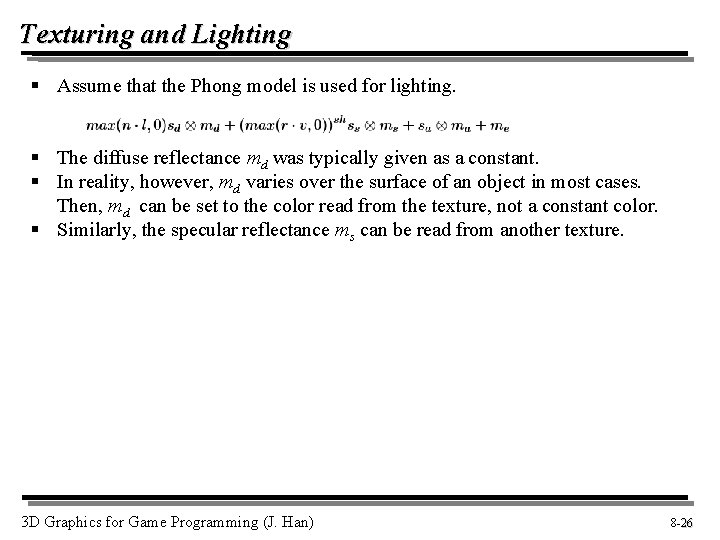
Texturing and Lighting § Assume that the Phong model is used for lighting. § The diffuse reflectance md was typically given as a constant. § In reality, however, md varies over the surface of an object in most cases. Then, md can be set to the color read from the texture, not a constant color. § Similarly, the specular reflectance ms can be read from another texture. 3 D Graphics for Game Programming (J. Han) 8 -26 26
Texturing
Standards governing the use of simulators diatur dalam
Graphics monitors and workstations in computer graphics
3d viewing devices in computer graphics ppt
Részei
Pembuatan unsur neon
King henry vii family tree
Donde se encuentra el nervio vago
Henry viii wives executed
French royal lineage
Mapa mental formação dos estados nacionais
Cn 8 test
Article viii the teachers and learners explanation
Reforma luterana consequências
Henry viii great matter
King henry viii banquet menu
Grupo viii a de la tabla periodica
Domingo 8 del tiempo ordinario ciclo c
Domingo xviii del tiempo ordinario ciclo c
Henry viii definition ap world history
Roma siglo viii a.c
Viii encuentro nacional de catalogadores
Tr.corticonuclearis
Tokoh merkantilisme
Csric viii
When did henry divorce catherine of aragon
The six wives of henry viii