Chapter Thirteen Pointers 1 Pointers A pointer is
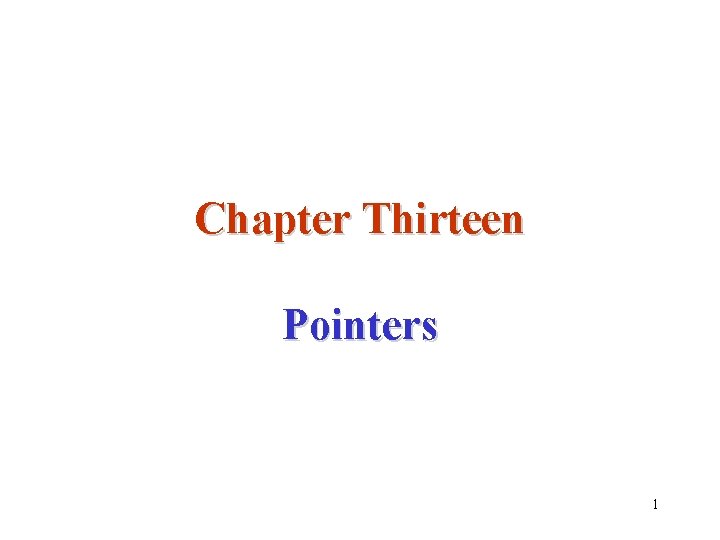
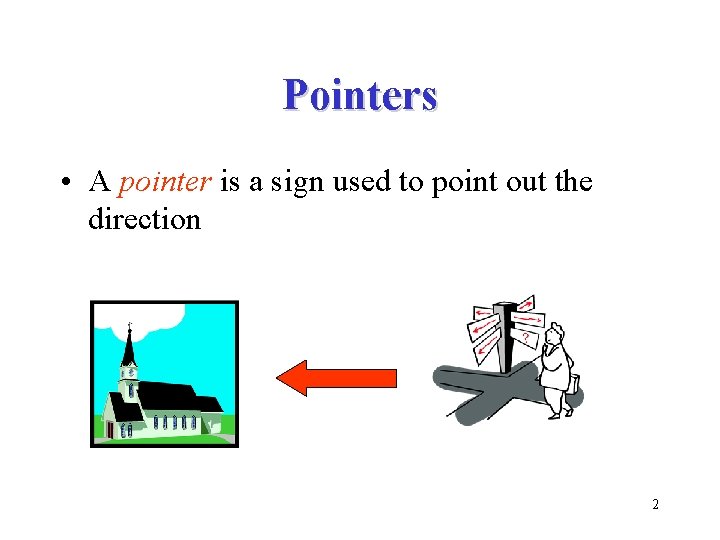
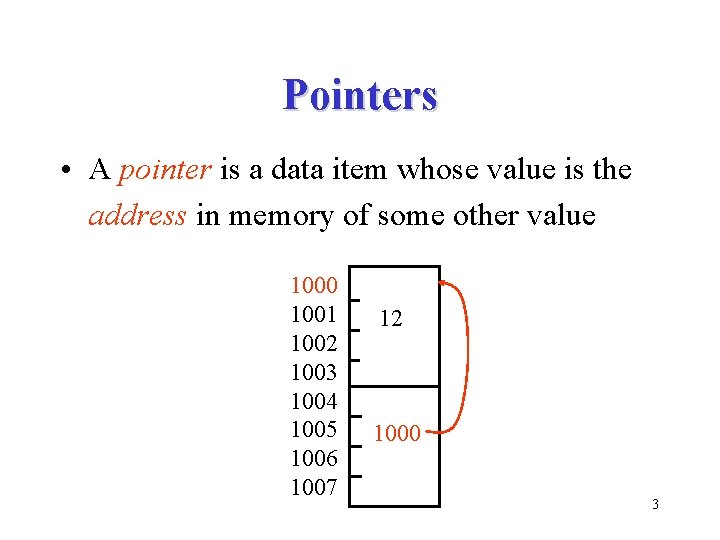
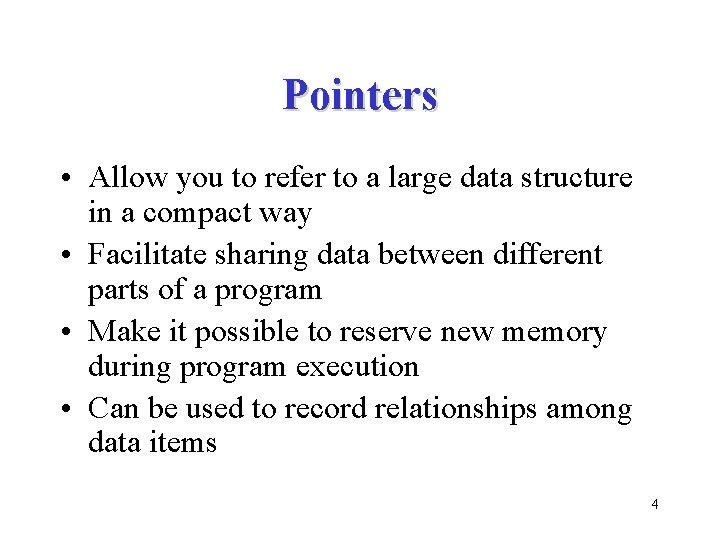
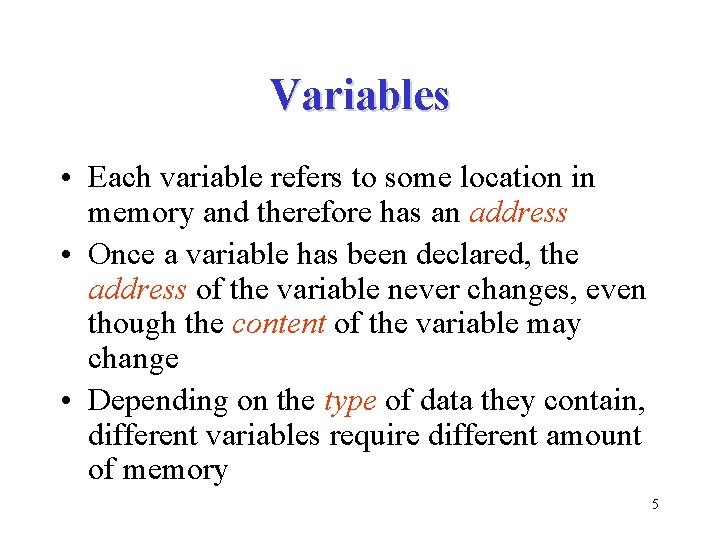
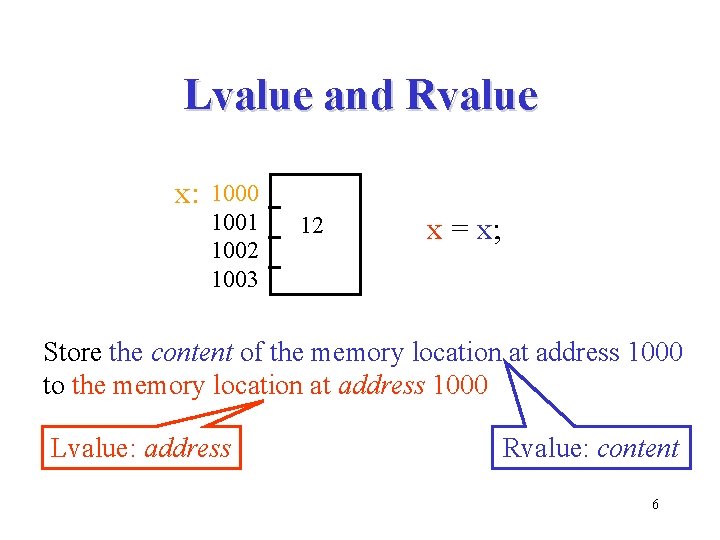
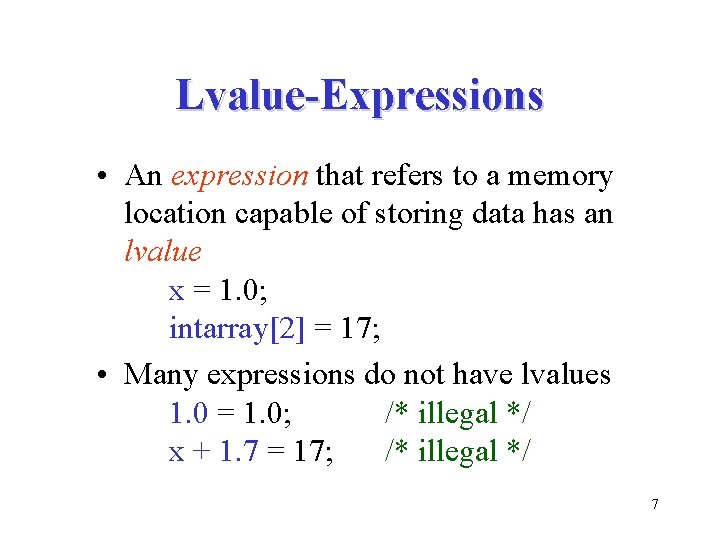
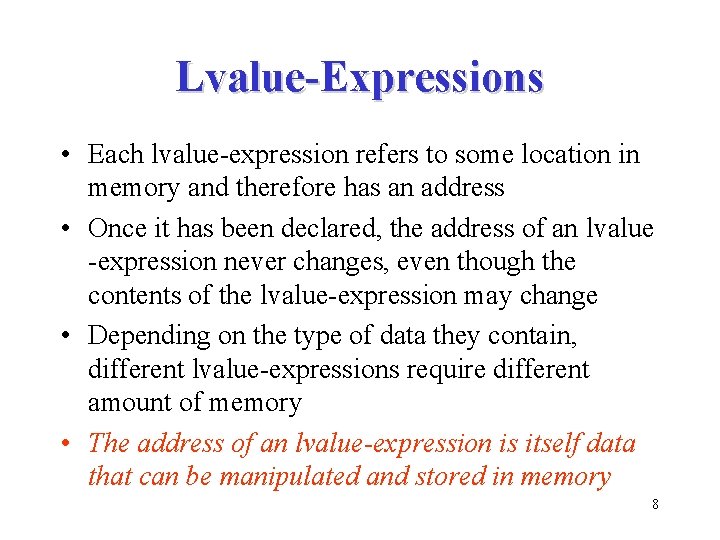
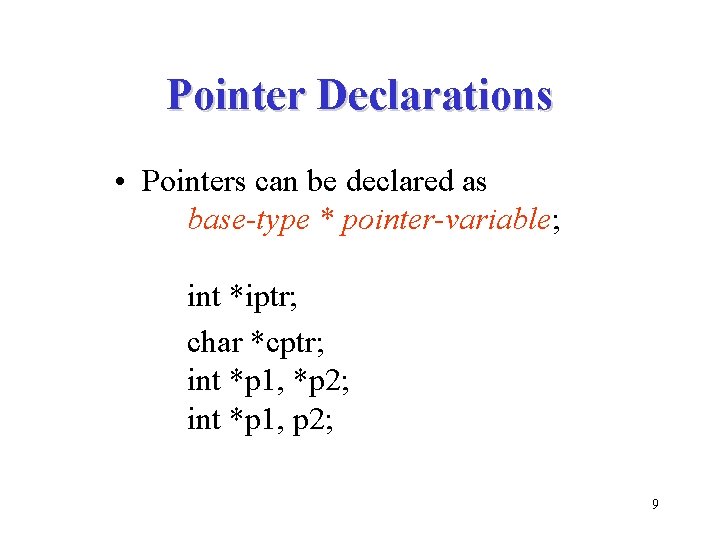
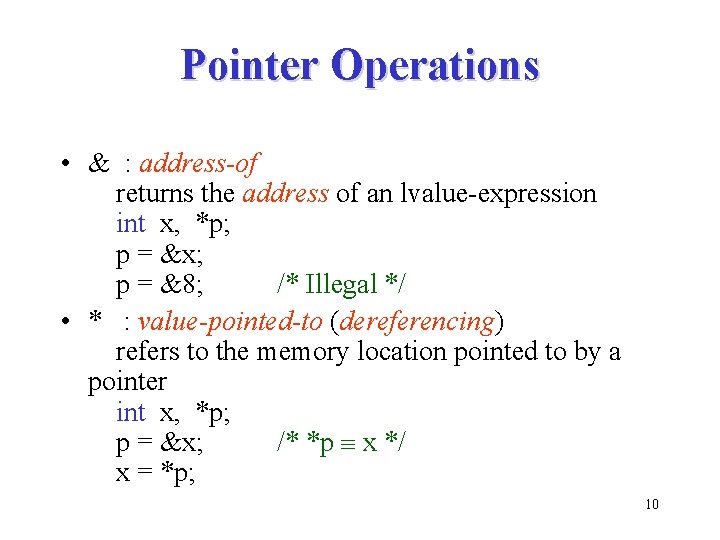
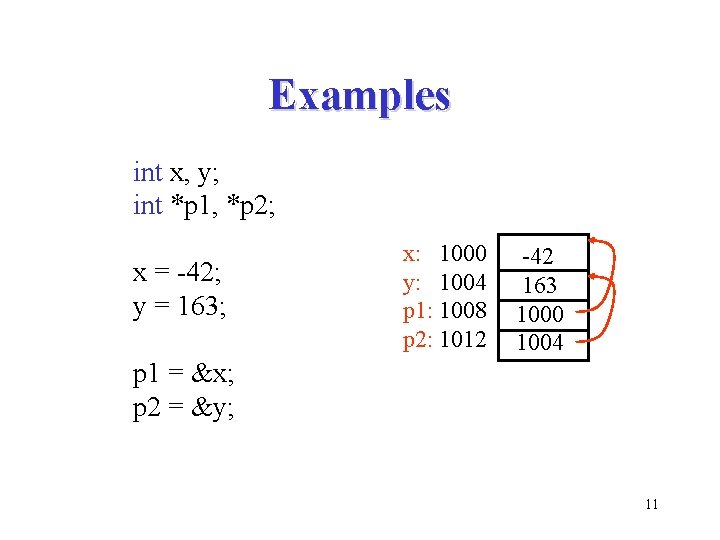
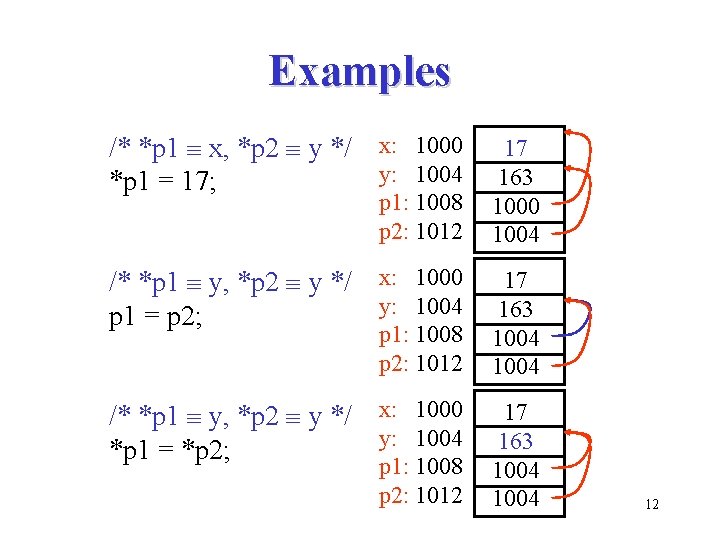
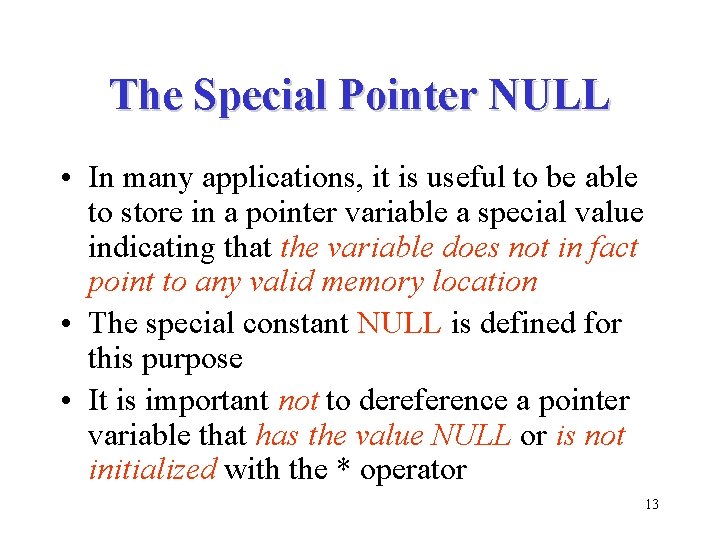
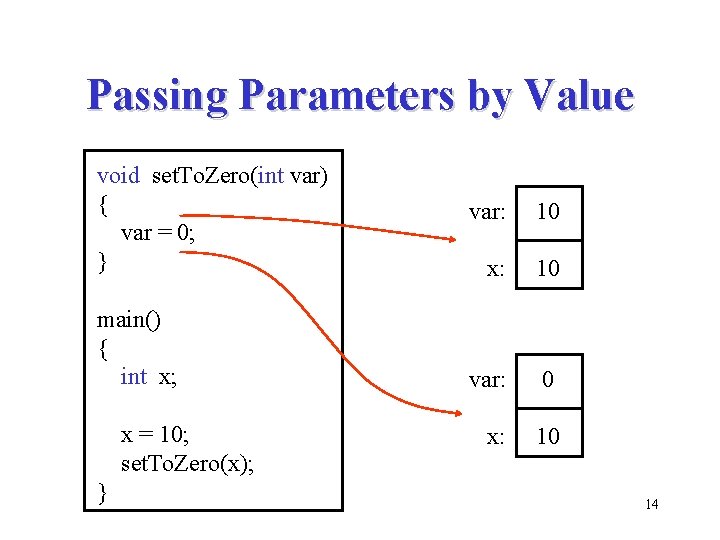
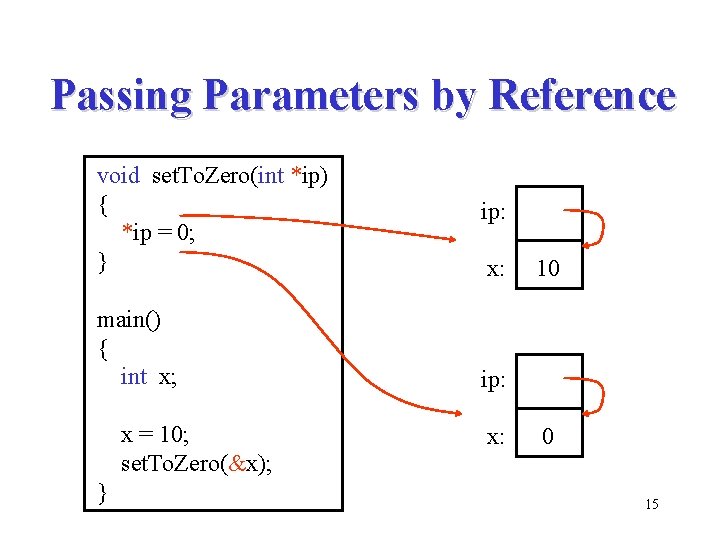
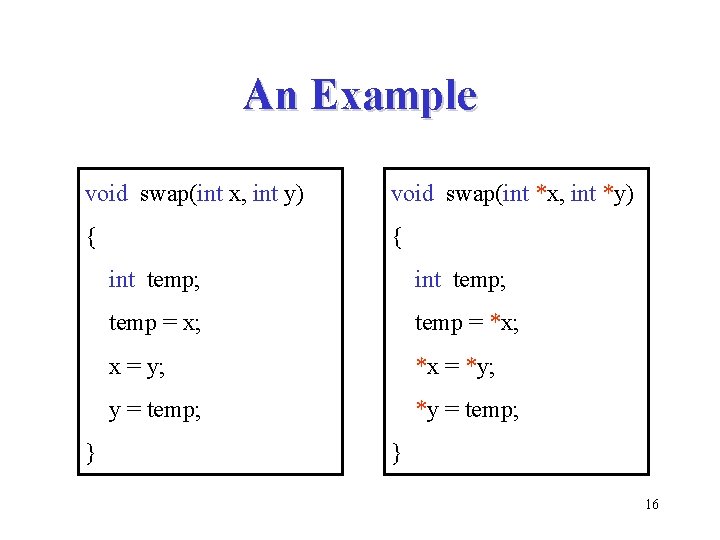
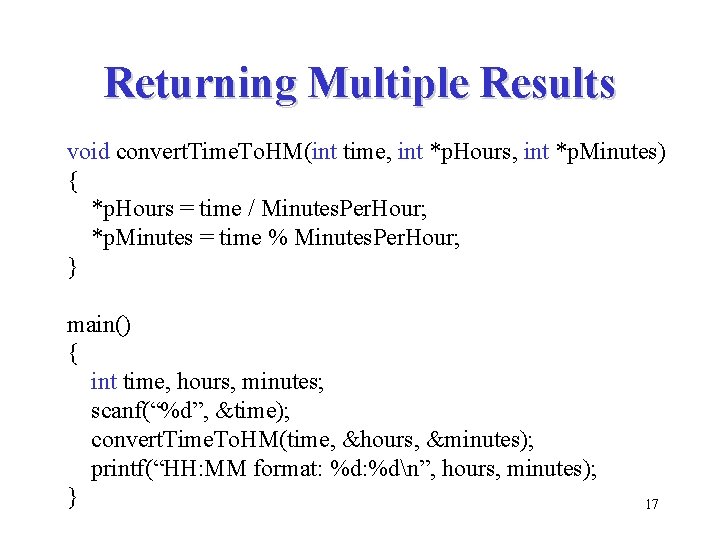
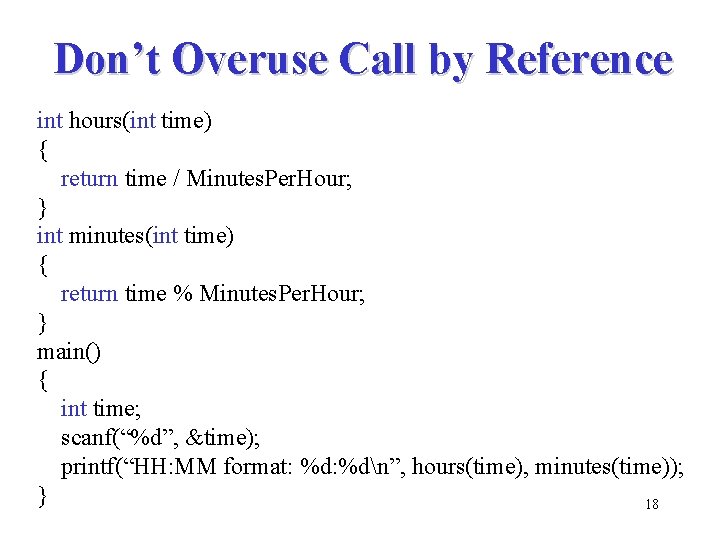
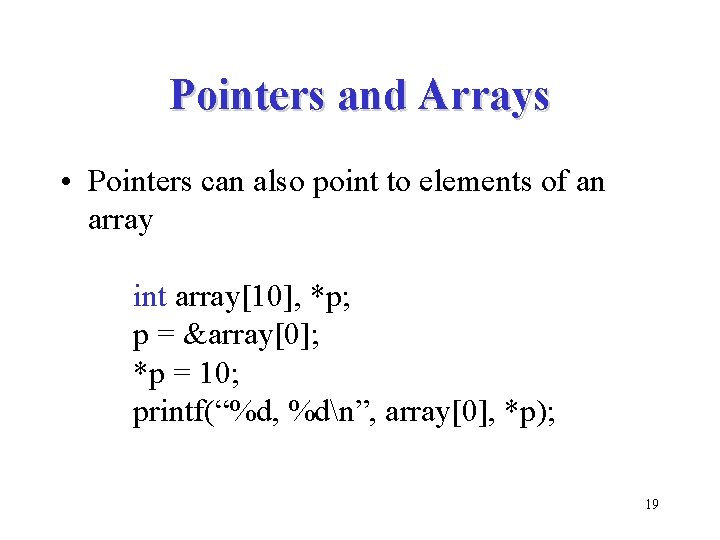
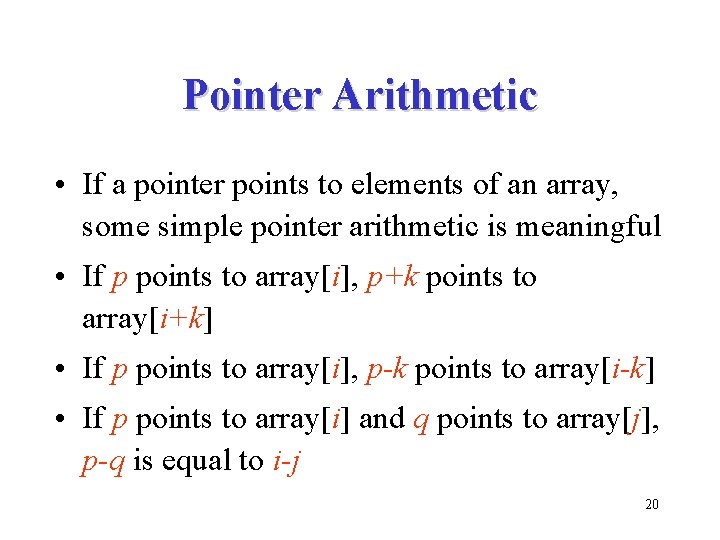
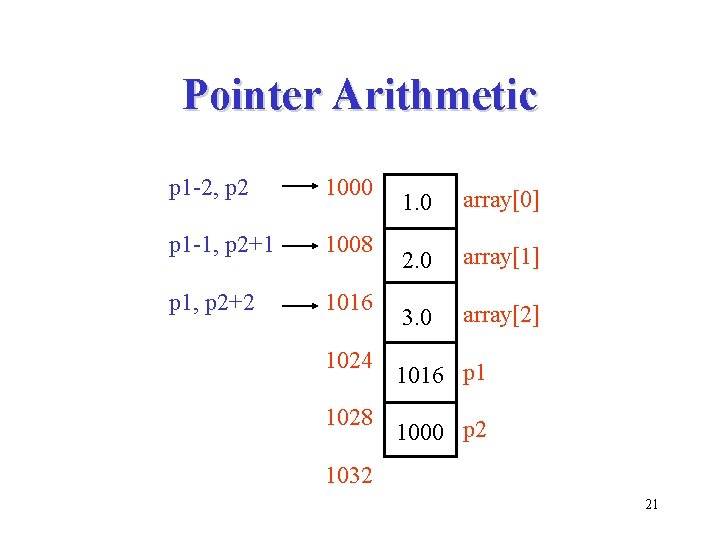
![An Example main() { int i, sum, array[10]; main() { int i, sum, array[10], An Example main() { int i, sum, array[10]; main() { int i, sum, array[10],](https://slidetodoc.com/presentation_image_h2/a16c59dcb891c47fc658eee3c31662a3/image-22.jpg)
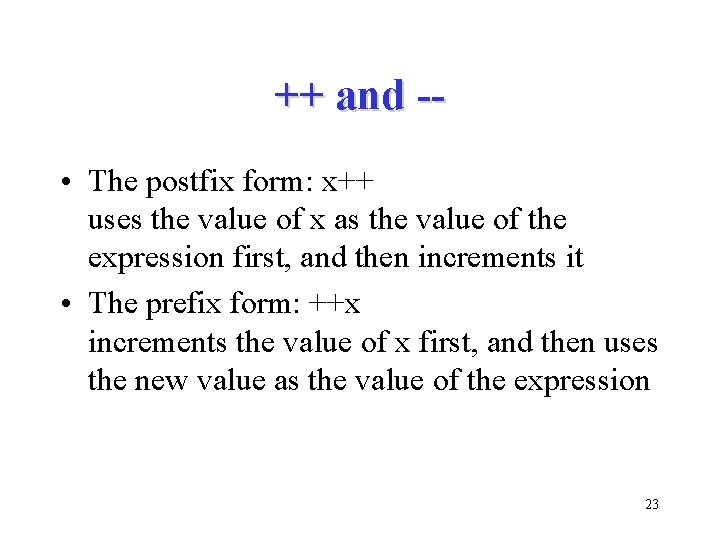
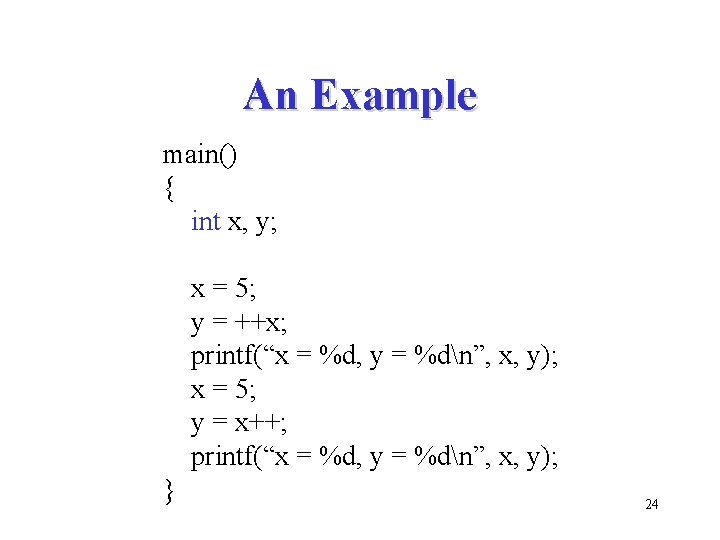
![An Example for (i = 0; i < n; i++) arr[i] = 0; for An Example for (i = 0; i < n; i++) arr[i] = 0; for](https://slidetodoc.com/presentation_image_h2/a16c59dcb891c47fc658eee3c31662a3/image-25.jpg)
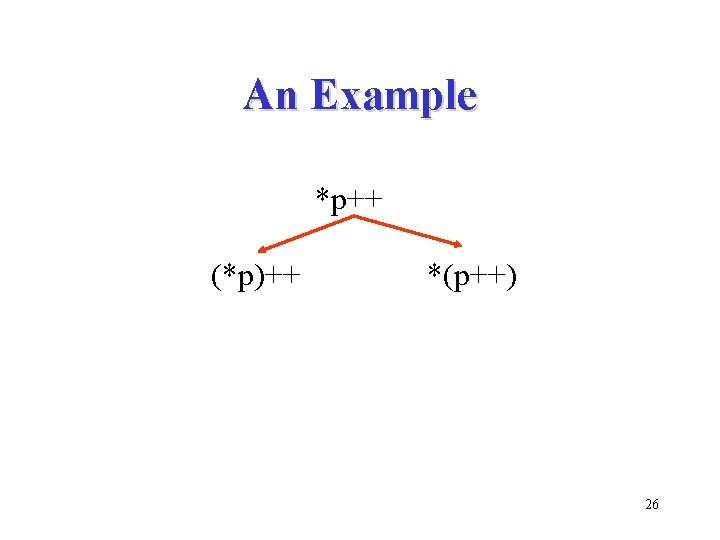
![An Example main() { int i, sum, array[10], *p; main() { int i, sum, An Example main() { int i, sum, array[10], *p; main() { int i, sum,](https://slidetodoc.com/presentation_image_h2/a16c59dcb891c47fc658eee3c31662a3/image-27.jpg)
![An Example int add(int array[], int size) int add(int *array, int size) { { An Example int add(int array[], int size) int add(int *array, int size) { {](https://slidetodoc.com/presentation_image_h2/a16c59dcb891c47fc658eee3c31662a3/image-28.jpg)
![An Example main() 40 bytes { int i, sum, array[10]; for (i = 0; An Example main() 40 bytes { int i, sum, array[10]; for (i = 0;](https://slidetodoc.com/presentation_image_h2/a16c59dcb891c47fc658eee3c31662a3/image-29.jpg)
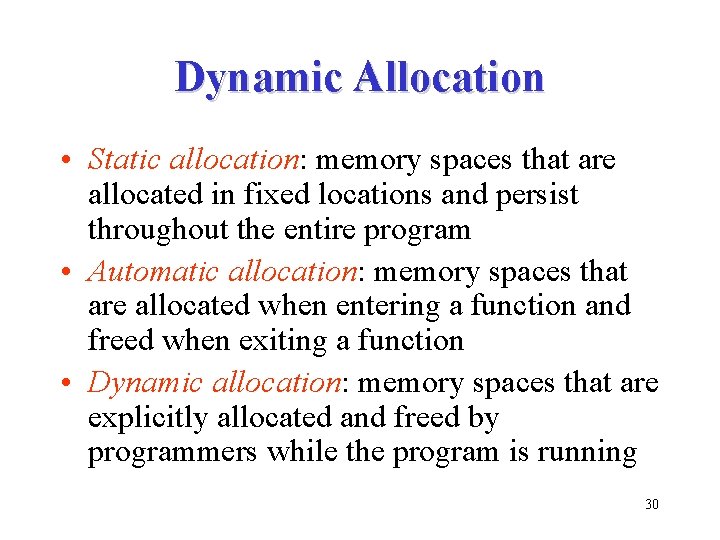
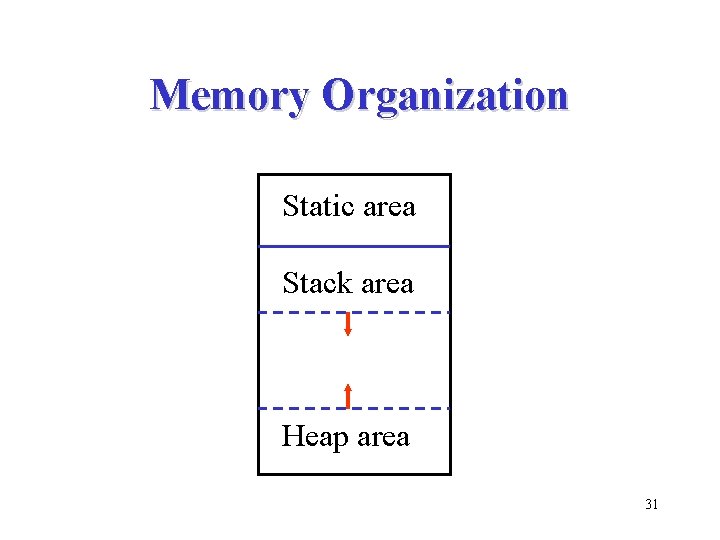
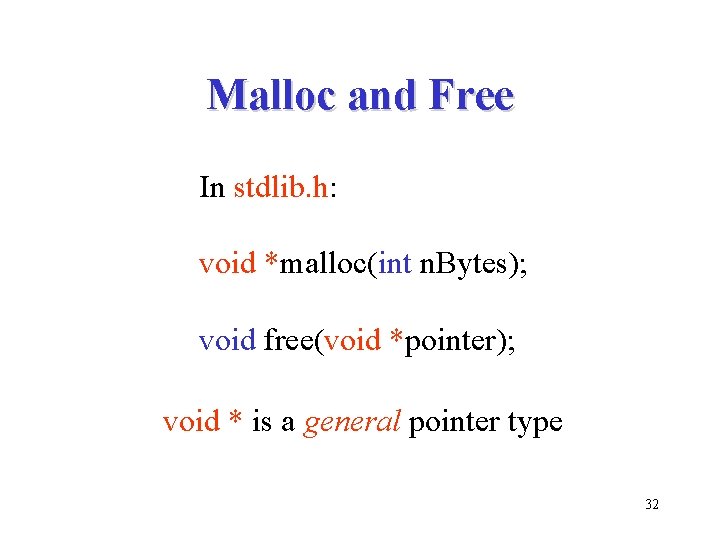
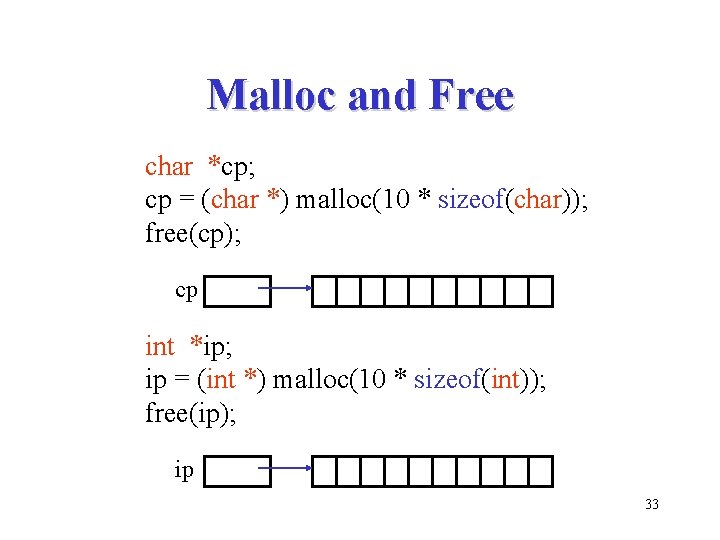
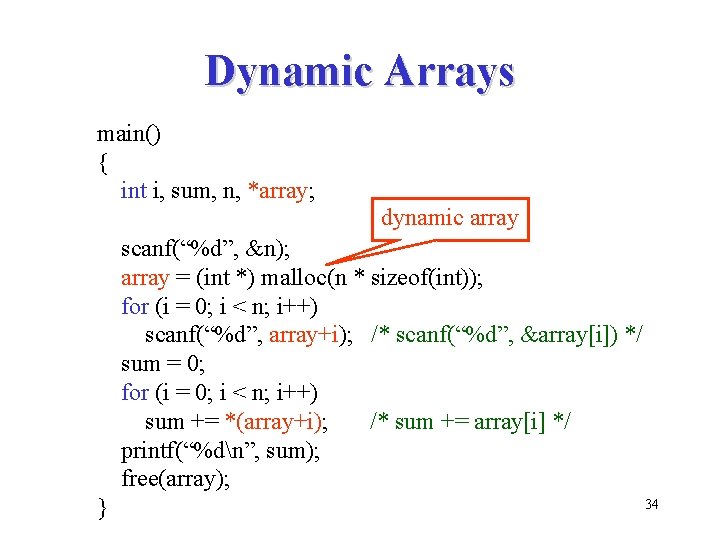
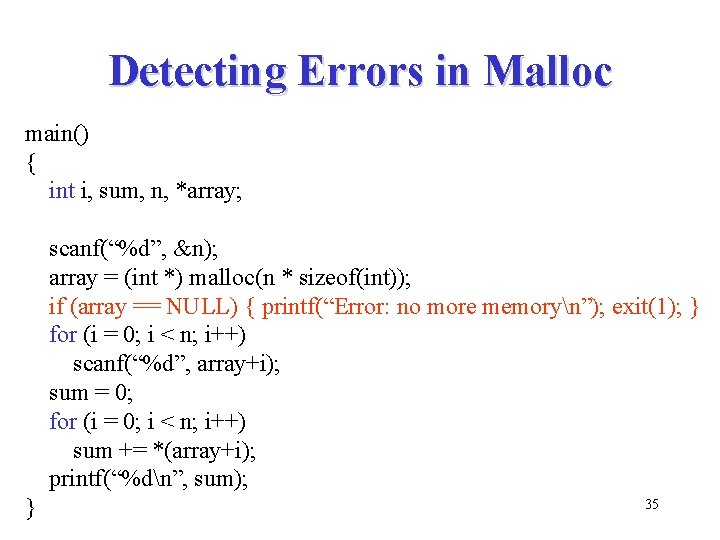
- Slides: 35
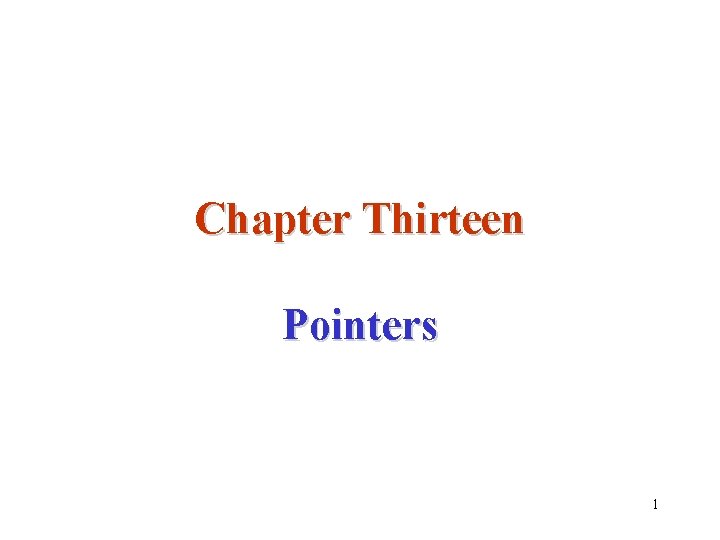
Chapter Thirteen Pointers 1
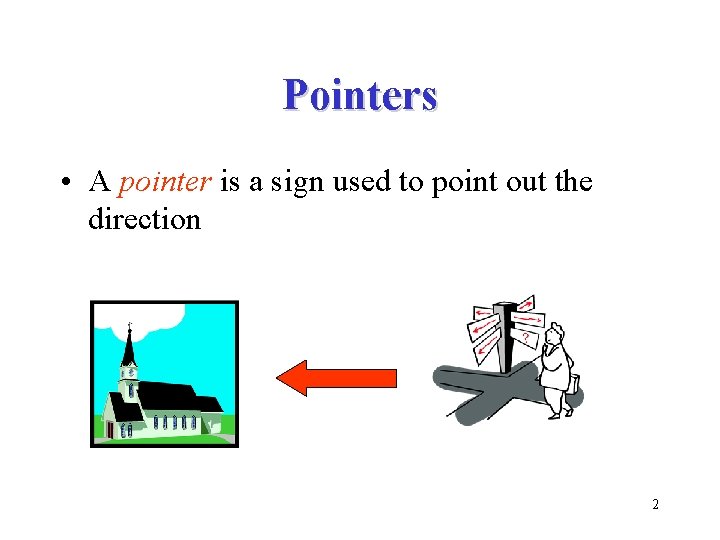
Pointers • A pointer is a sign used to point out the direction 2
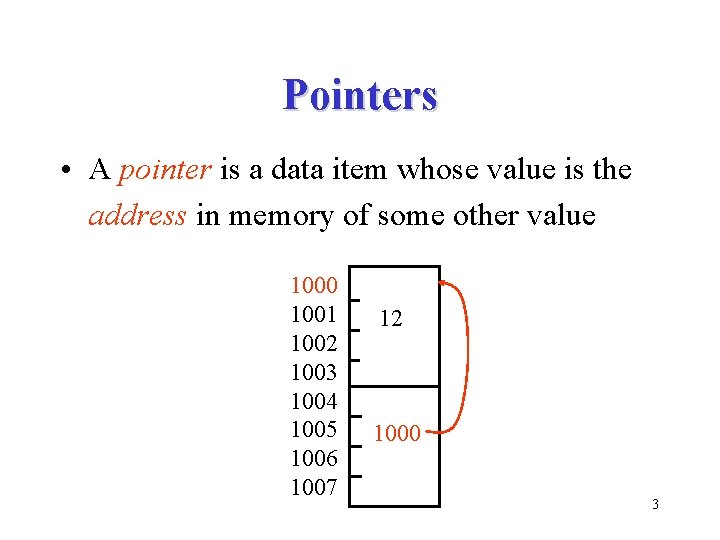
Pointers • A pointer is a data item whose value is the address in memory of some other value 1000 1001 1002 1003 1004 1005 1006 1007 12 1000 3
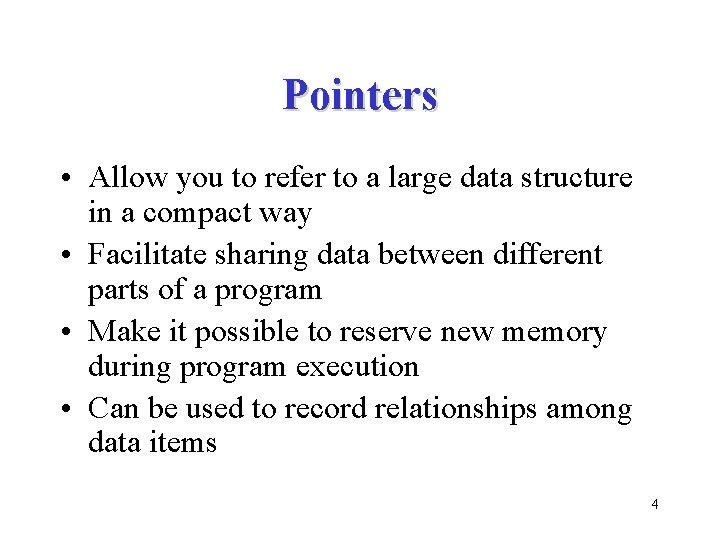
Pointers • Allow you to refer to a large data structure in a compact way • Facilitate sharing data between different parts of a program • Make it possible to reserve new memory during program execution • Can be used to record relationships among data items 4
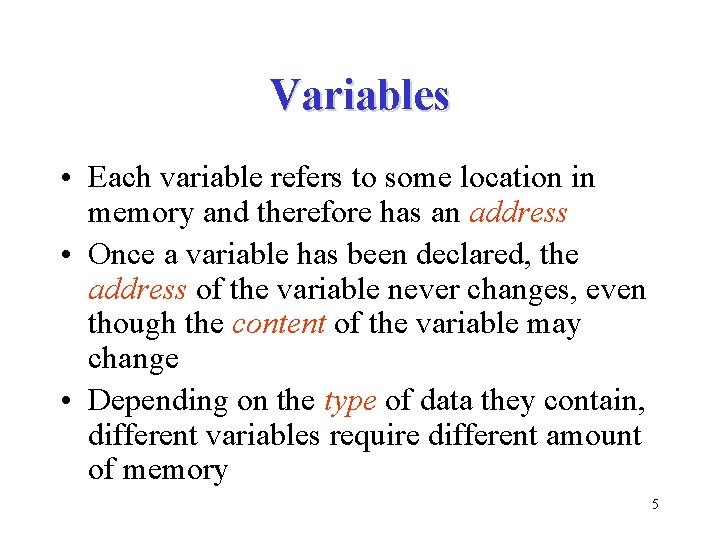
Variables • Each variable refers to some location in memory and therefore has an address • Once a variable has been declared, the address of the variable never changes, even though the content of the variable may change • Depending on the type of data they contain, different variables require different amount of memory 5
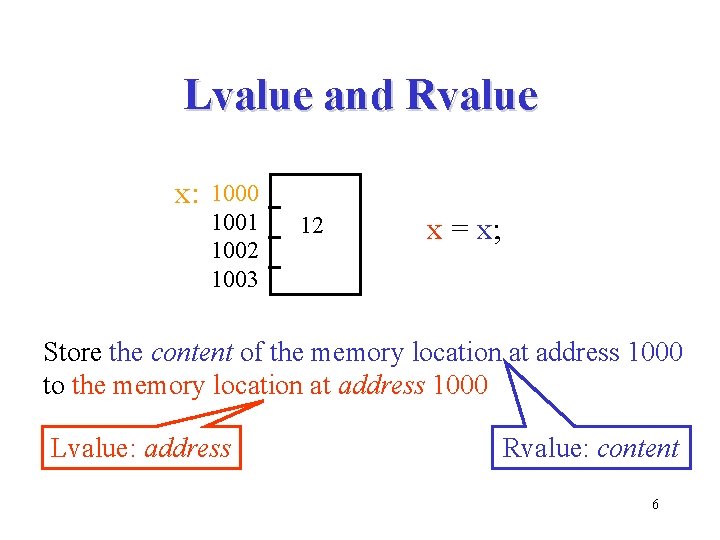
Lvalue and Rvalue x: 1000 1001 1002 1003 12 x = x; Store the content of the memory location at address 1000 to the memory location at address 1000 Lvalue: address Rvalue: content 6
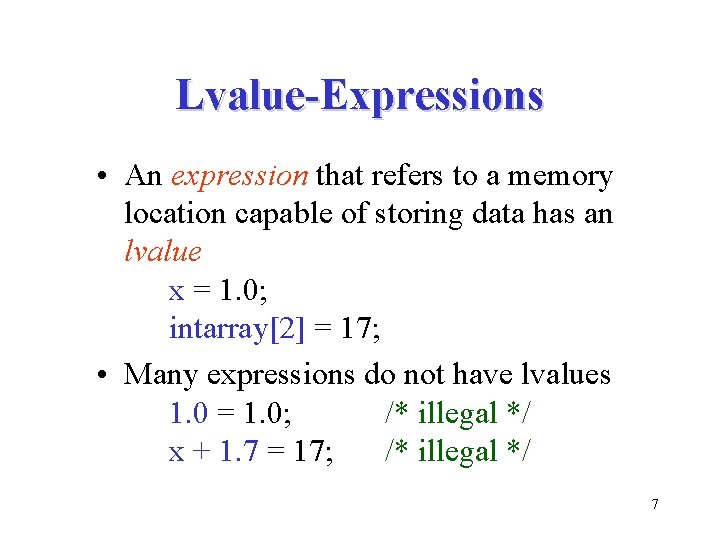
Lvalue-Expressions • An expression that refers to a memory location capable of storing data has an lvalue x = 1. 0; intarray[2] = 17; • Many expressions do not have lvalues 1. 0 = 1. 0; /* illegal */ x + 1. 7 = 17; /* illegal */ 7
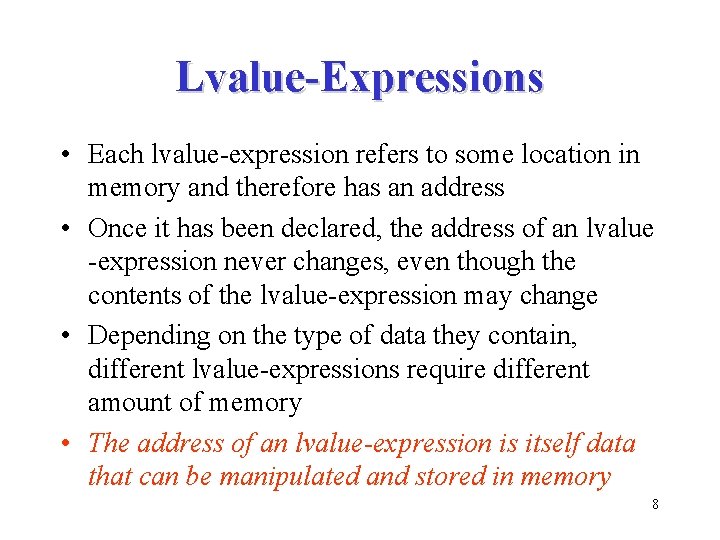
Lvalue-Expressions • Each lvalue-expression refers to some location in memory and therefore has an address • Once it has been declared, the address of an lvalue -expression never changes, even though the contents of the lvalue-expression may change • Depending on the type of data they contain, different lvalue-expressions require different amount of memory • The address of an lvalue-expression is itself data that can be manipulated and stored in memory 8
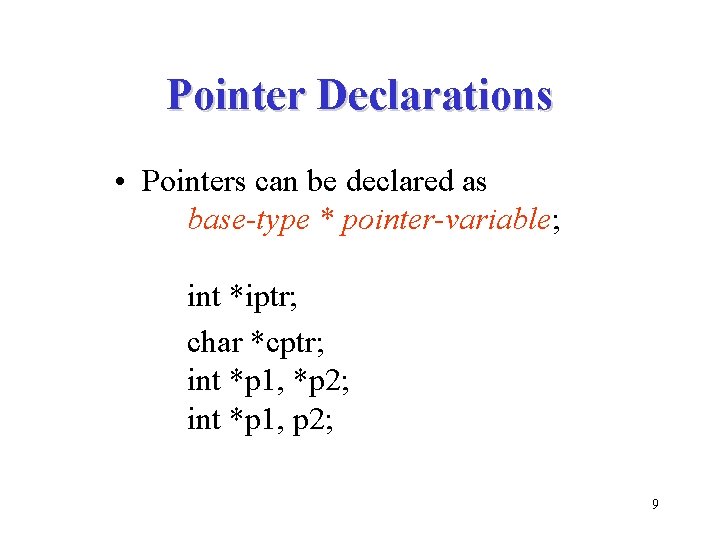
Pointer Declarations • Pointers can be declared as base-type * pointer-variable; int *iptr; char *cptr; int *p 1, *p 2; int *p 1, p 2; 9
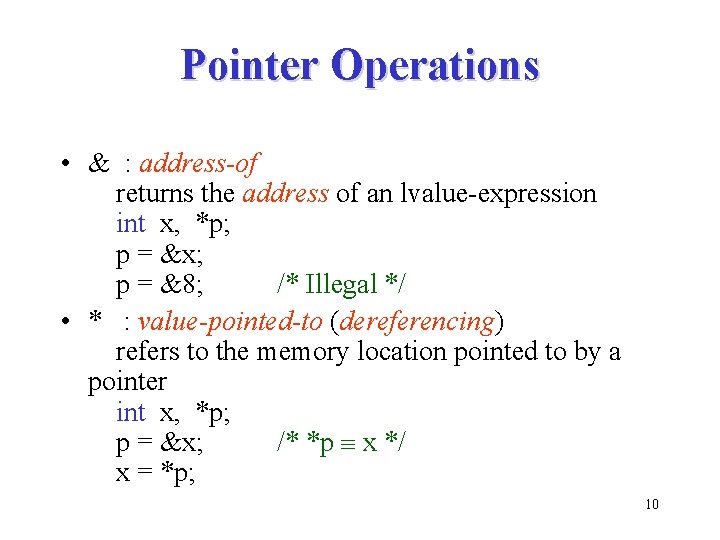
Pointer Operations • & : address-of returns the address of an lvalue-expression int x, *p; p = &x; p = &8; /* Illegal */ • * : value-pointed-to (dereferencing) refers to the memory location pointed to by a pointer int x, *p; p = &x; /* *p x */ x = *p; 10
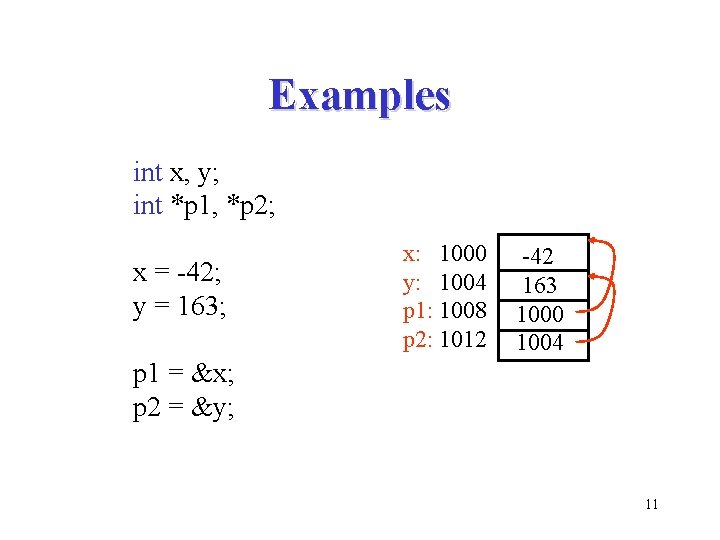
Examples int x, y; int *p 1, *p 2; x = -42; y = 163; x: 1000 y: 1004 p 1: 1008 p 2: 1012 -42 163 1000 1004 p 1 = &x; p 2 = &y; 11
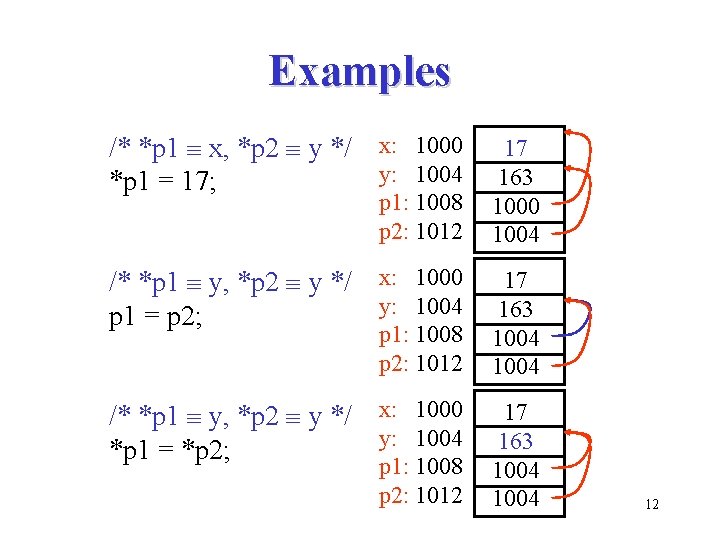
Examples /* *p 1 x, *p 2 y */ x: 1000 y: 1004 *p 1 = 17; 17 163 1000 1004 /* *p 1 y, *p 2 y */ x: 1000 y: 1004 p 1 = p 2; 17 163 1004 /* *p 1 y, *p 2 y */ x: 1000 y: 1004 *p 1 = *p 2; 17 163 1004 p 1: 1008 p 2: 1012 12
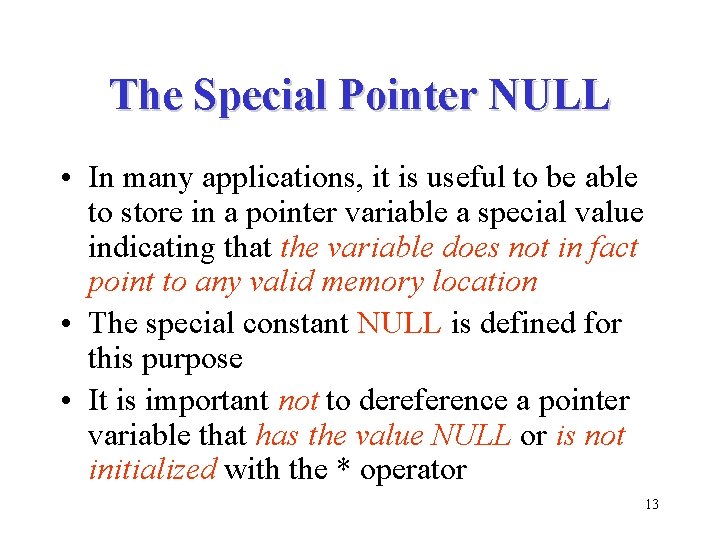
The Special Pointer NULL • In many applications, it is useful to be able to store in a pointer variable a special value indicating that the variable does not in fact point to any valid memory location • The special constant NULL is defined for this purpose • It is important not to dereference a pointer variable that has the value NULL or is not initialized with the * operator 13
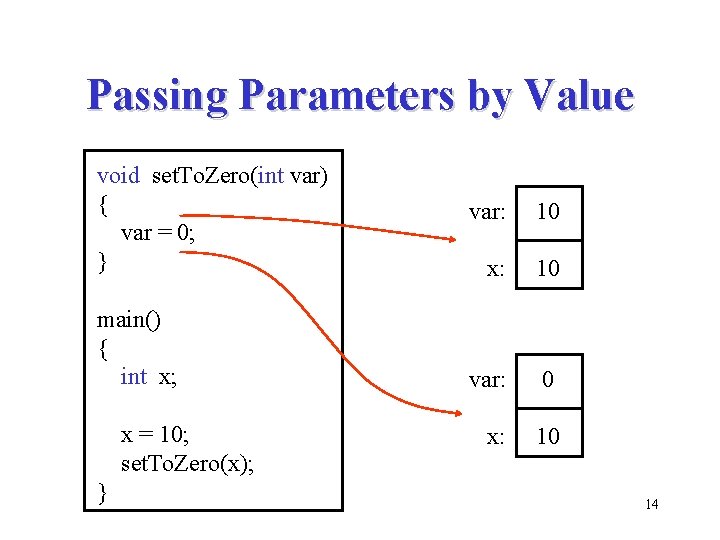
Passing Parameters by Value void set. To. Zero(int var) { var = 0; } main() { int x; x = 10; set. To. Zero(x); } var: 10 x: 10 var: 0 x: 10 14
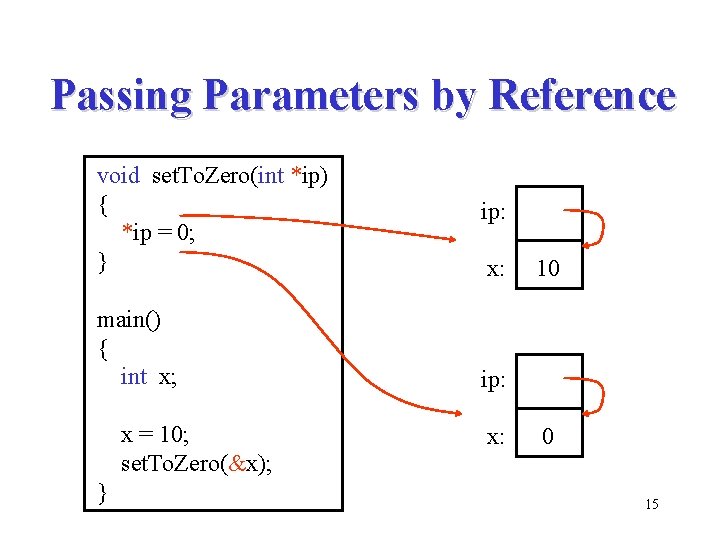
Passing Parameters by Reference void set. To. Zero(int *ip) { *ip = 0; } main() { int x; x = 10; set. To. Zero(&x); } ip: x: 10 ip: x: 0 15
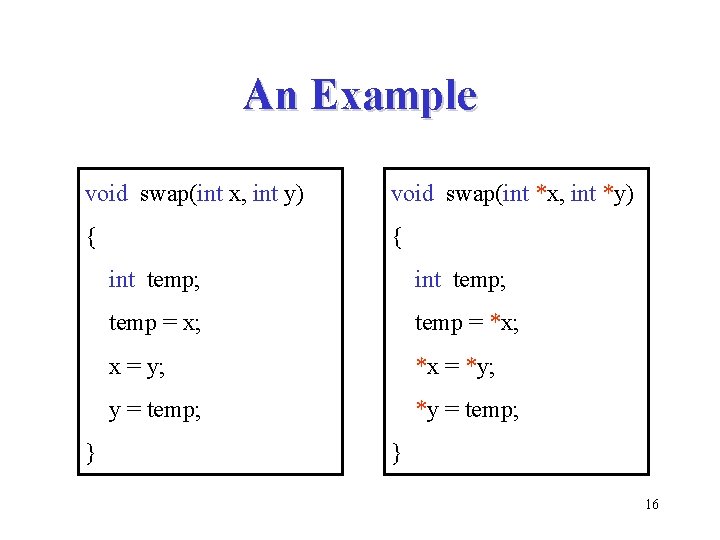
An Example void swap(int x, int y) void swap(int *x, int *y) { { } int temp; temp = x; temp = *x; x = y; *x = *y; y = temp; *y = temp; } 16
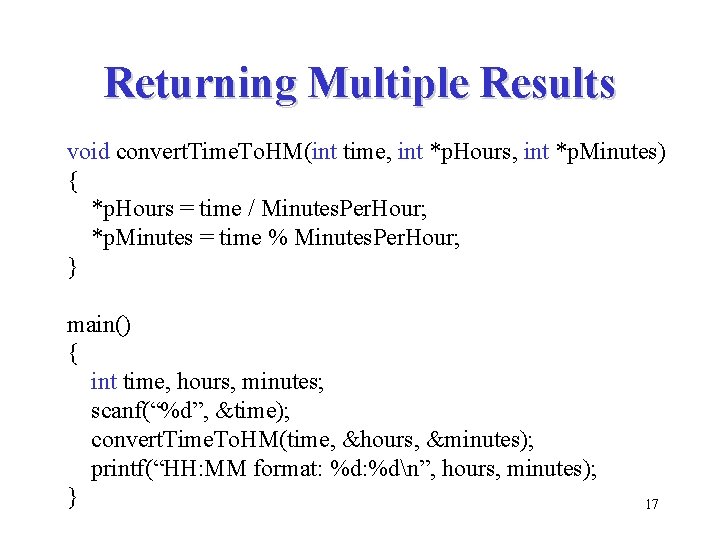
Returning Multiple Results void convert. Time. To. HM(int time, int *p. Hours, int *p. Minutes) { *p. Hours = time / Minutes. Per. Hour; *p. Minutes = time % Minutes. Per. Hour; } main() { int time, hours, minutes; scanf(“%d”, &time); convert. Time. To. HM(time, &hours, &minutes); printf(“HH: MM format: %dn”, hours, minutes); } 17
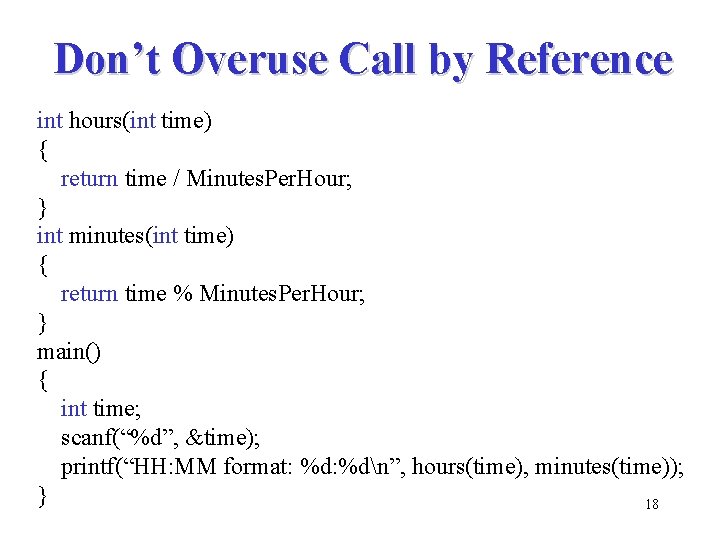
Don’t Overuse Call by Reference int hours(int time) { return time / Minutes. Per. Hour; } int minutes(int time) { return time % Minutes. Per. Hour; } main() { int time; scanf(“%d”, &time); printf(“HH: MM format: %dn”, hours(time), minutes(time)); } 18
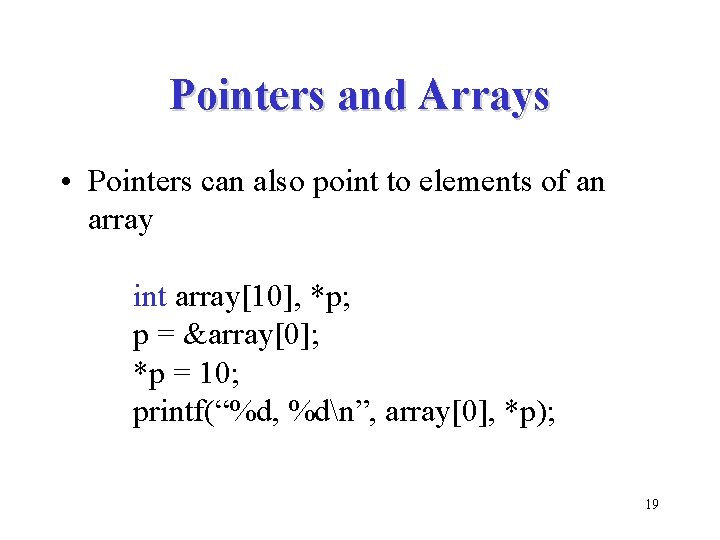
Pointers and Arrays • Pointers can also point to elements of an array int array[10], *p; p = &array[0]; *p = 10; printf(“%d, %dn”, array[0], *p); 19
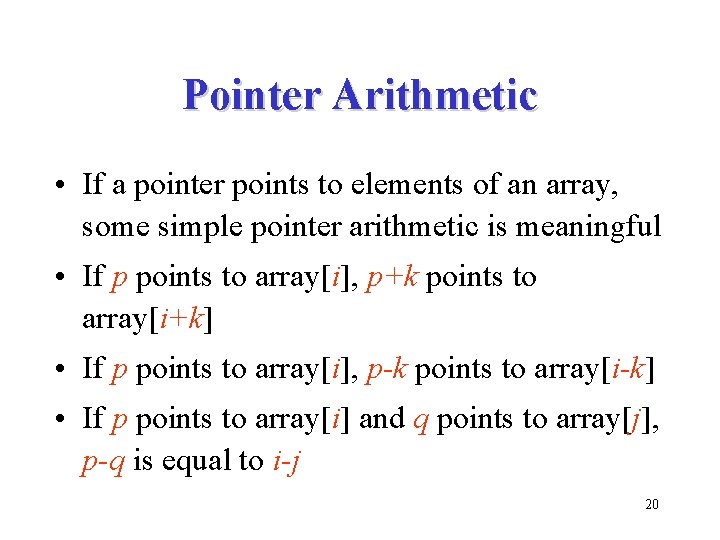
Pointer Arithmetic • If a pointer points to elements of an array, some simple pointer arithmetic is meaningful • If p points to array[i], p+k points to array[i+k] • If p points to array[i], p-k points to array[i-k] • If p points to array[i] and q points to array[j], p-q is equal to i-j 20
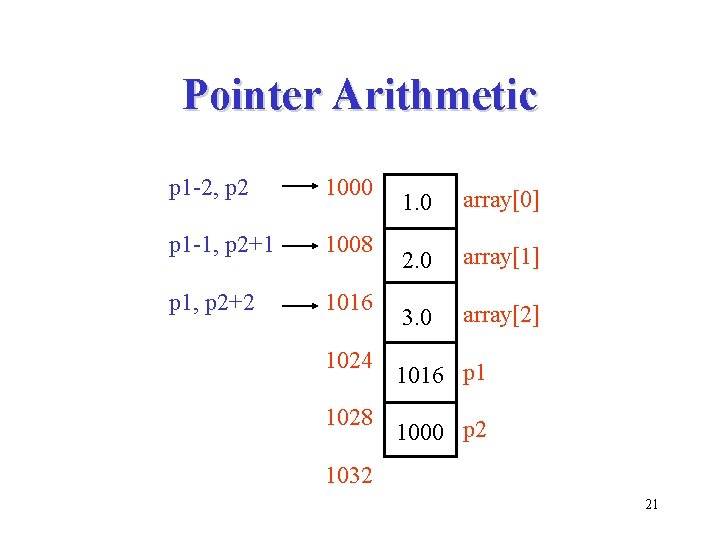
Pointer Arithmetic p 1 -2, p 2 1000 p 1 -1, p 2+1 1008 p 1, p 2+2 1016 1. 0 array[0] 2. 0 array[1] 3. 0 array[2] 1024 1016 p 1 1028 1000 p 2 1032 21
![An Example main int i sum array10 main int i sum array10 An Example main() { int i, sum, array[10]; main() { int i, sum, array[10],](https://slidetodoc.com/presentation_image_h2/a16c59dcb891c47fc658eee3c31662a3/image-22.jpg)
An Example main() { int i, sum, array[10]; main() { int i, sum, array[10], *p; for (i = 0; i < 10; i++) { scanf(“%d”, &array[i]); } sum = 0; for (i = 0; i < 10; i++) { sum += array[i]; } } for (i = 0; i < 10; i++) { scanf(“%d”, &array[i]); } sum = 0; for (p = &array[0]; p <= &array[9]; p++) { sum += *p; } } 22
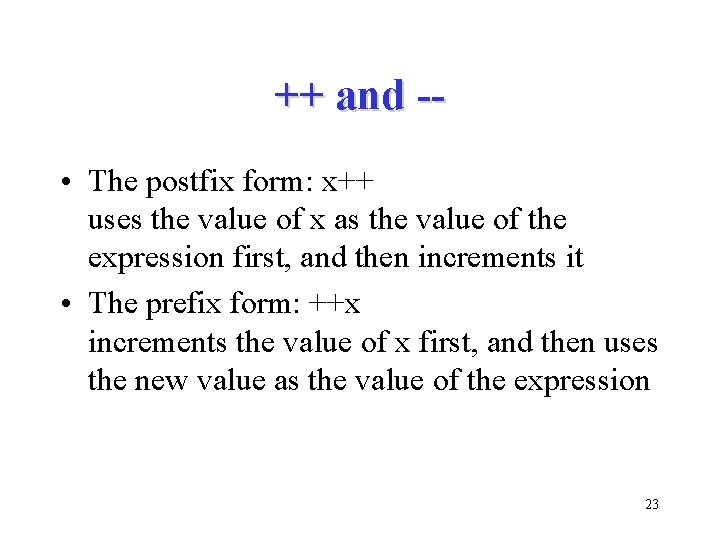
++ and - • The postfix form: x++ uses the value of x as the value of the expression first, and then increments it • The prefix form: ++x increments the value of x first, and then uses the new value as the value of the expression 23
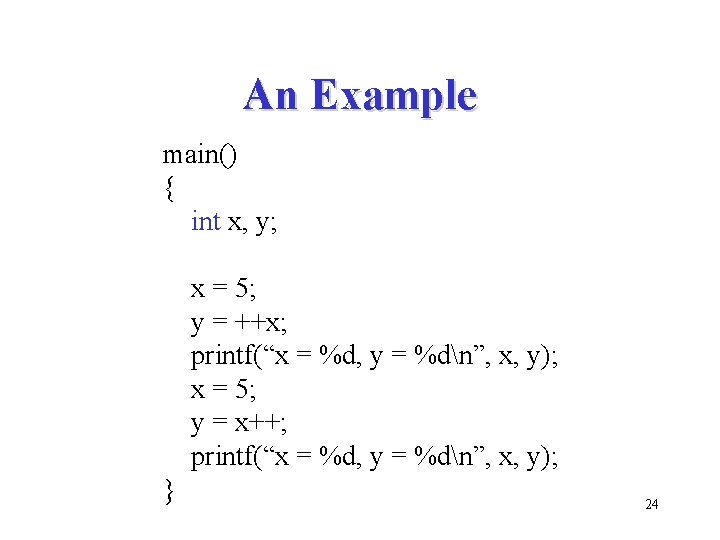
An Example main() { int x, y; x = 5; y = ++x; printf(“x = %d, y = %dn”, x, y); x = 5; y = x++; printf(“x = %d, y = %dn”, x, y); } 24
![An Example for i 0 i n i arri 0 for An Example for (i = 0; i < n; i++) arr[i] = 0; for](https://slidetodoc.com/presentation_image_h2/a16c59dcb891c47fc658eee3c31662a3/image-25.jpg)
An Example for (i = 0; i < n; i++) arr[i] = 0; for (i = 0; i < n; ) arr[i++] = 0; for (i = 0; i < n; ) arr[i] = i++; 25
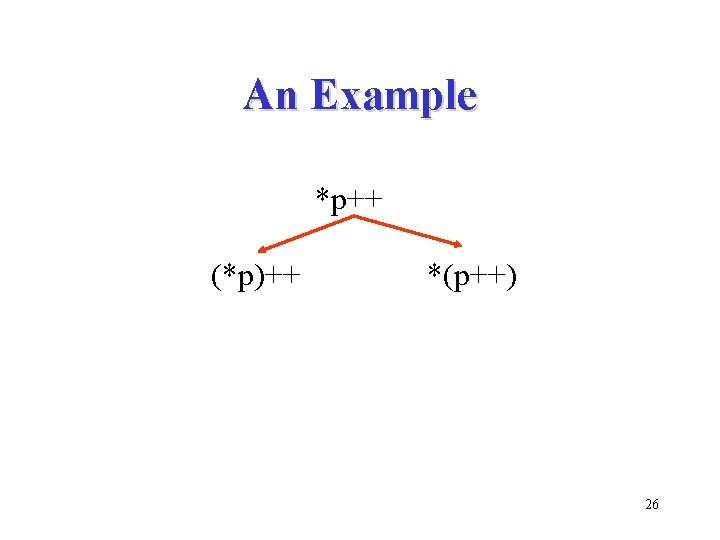
An Example *p++ (*p)++ *(p++) 26
![An Example main int i sum array10 p main int i sum An Example main() { int i, sum, array[10], *p; main() { int i, sum,](https://slidetodoc.com/presentation_image_h2/a16c59dcb891c47fc658eee3c31662a3/image-27.jpg)
An Example main() { int i, sum, array[10], *p; main() { int i, sum, array[10]; for (i = 0; i < 10; i++) { scanf(“%d”, &array[i]); } sum = 0; for (p = array; p <= &array[9]; ) { sum += *p++; } } for (i = 0; i < 10; i++) { scanf(“%d”, array+i); } sum = 0; for (i = 0; i < 10; i++) { sum += *(array+i); } } 27
![An Example int addint array int size int addint array int size An Example int add(int array[], int size) int add(int *array, int size) { {](https://slidetodoc.com/presentation_image_h2/a16c59dcb891c47fc658eee3c31662a3/image-28.jpg)
An Example int add(int array[], int size) int add(int *array, int size) { { int i, sum; sum = 0; for (i = 0; i < size; i++) sum += array[i]; sum += *(array+i); return sum; } } main() { int s, n[SIZE]; s = add(n, SIZE); } 28
![An Example main 40 bytes int i sum array10 for i 0 An Example main() 40 bytes { int i, sum, array[10]; for (i = 0;](https://slidetodoc.com/presentation_image_h2/a16c59dcb891c47fc658eee3c31662a3/image-29.jpg)
An Example main() 40 bytes { int i, sum, array[10]; for (i = 0; i < 10; i++) { scanf(“%d”, &array[i]); } sum = 0; for (i = 0; i < 10; i++) { sum += array[i]; } printf(“%dn”, sum); } main() 4 bytes { int i, sum, *array; for (i = 0; i < 10; i++) { scanf(“%d”, array+i); } error sum = 0; for (i = 0; i < 10; i++) { sum += *(array+i); } error printf(“%dn”, sum); } 29
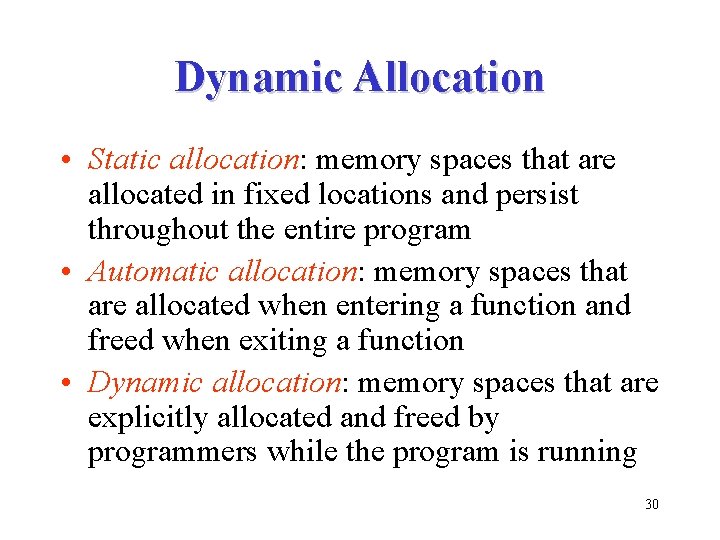
Dynamic Allocation • Static allocation: memory spaces that are allocated in fixed locations and persist throughout the entire program • Automatic allocation: memory spaces that are allocated when entering a function and freed when exiting a function • Dynamic allocation: memory spaces that are explicitly allocated and freed by programmers while the program is running 30
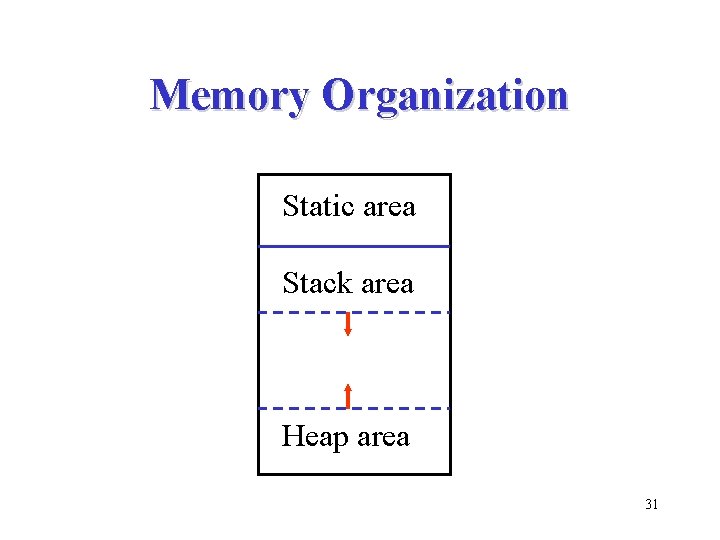
Memory Organization Static area Stack area Heap area 31
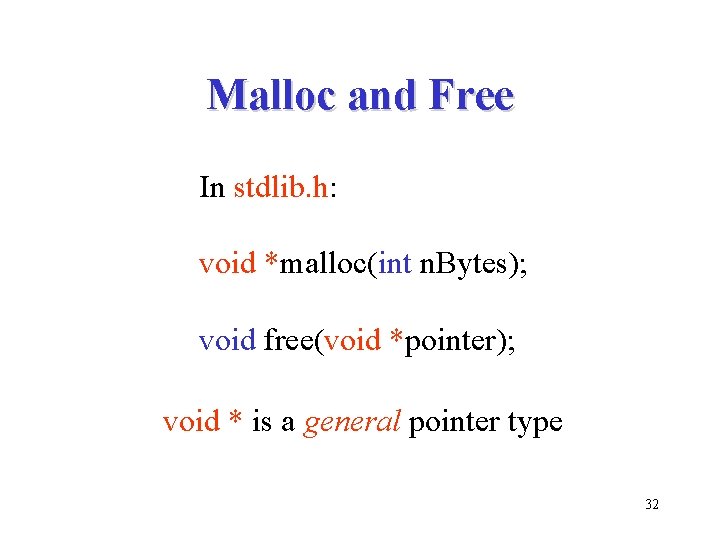
Malloc and Free In stdlib. h: void *malloc(int n. Bytes); void free(void *pointer); void * is a general pointer type 32
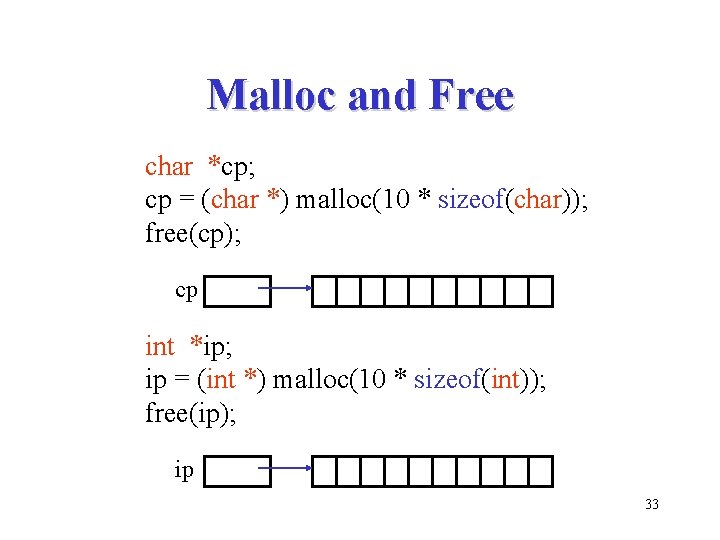
Malloc and Free char *cp; cp = (char *) malloc(10 * sizeof(char)); free(cp); cp int *ip; ip = (int *) malloc(10 * sizeof(int)); free(ip); ip 33
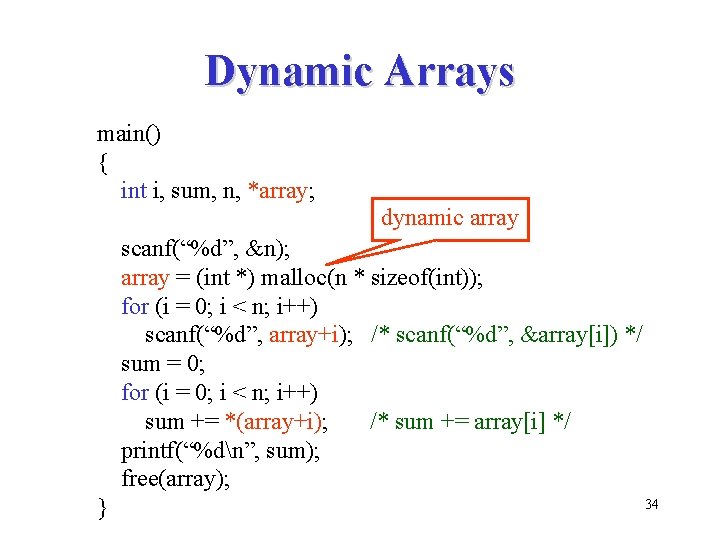
Dynamic Arrays main() { int i, sum, n, *array; dynamic array scanf(“%d”, &n); array = (int *) malloc(n * sizeof(int)); for (i = 0; i < n; i++) scanf(“%d”, array+i); /* scanf(“%d”, &array[i]) */ sum = 0; for (i = 0; i < n; i++) sum += *(array+i); /* sum += array[i] */ printf(“%dn”, sum); free(array); } 34
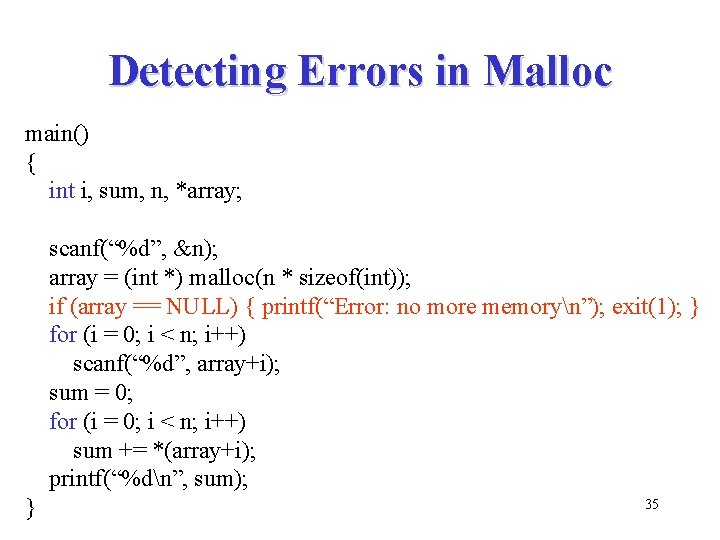
Detecting Errors in Malloc main() { int i, sum, n, *array; scanf(“%d”, &n); array = (int *) malloc(n * sizeof(int)); if (array == NULL) { printf(“Error: no more memoryn”); exit(1); } for (i = 0; i < n; i++) scanf(“%d”, array+i); sum = 0; for (i = 0; i < n; i++) sum += *(array+i); printf(“%dn”, sum); } 35
Constant pointer and pointer to constant
What is pointer to pointer in c
Constant pointer and pointer to constant
Constant pointer and pointer to constant
Constant pointer and pointer to constant
Pointer expressions and pointer arithmetic in c
Pointer pointer
Pointer pointer
Thirteen colonies
Eleven twelve thirteen fourteen fifteen
Thirteen ways of seeing nature in la summary
Sdbsweb
Freddy the thirteen
13 colonies quizlet
How many dogs do you see answer
Fifty nifty united states
Colonial regions chart
Brainpop regions of the 13 colonies
Thirteen apostles
New concept english 3
Caedb
Exmple
C array of pointers to structs
Scale factor of pointer in c
Which is a good idea for using skip pointers
Positional index information retrieval
Which is a good idea for using skip pointers
Explicit pointers
Pointers and strings
What are pointers in java
Function of inter
Swizzling glsl
Shuffle left algorithm
Hazard pointers
12 pointers
I-need-a-few-pointers-98hpou5