POINTERS Pointers A pointer type is one in
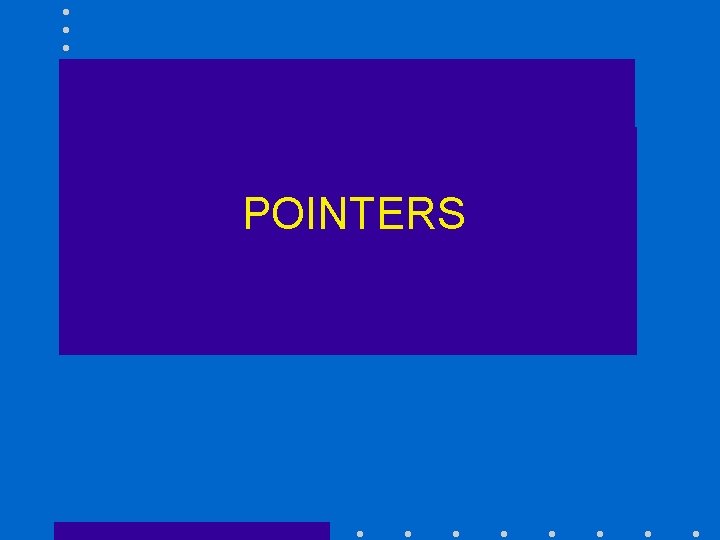
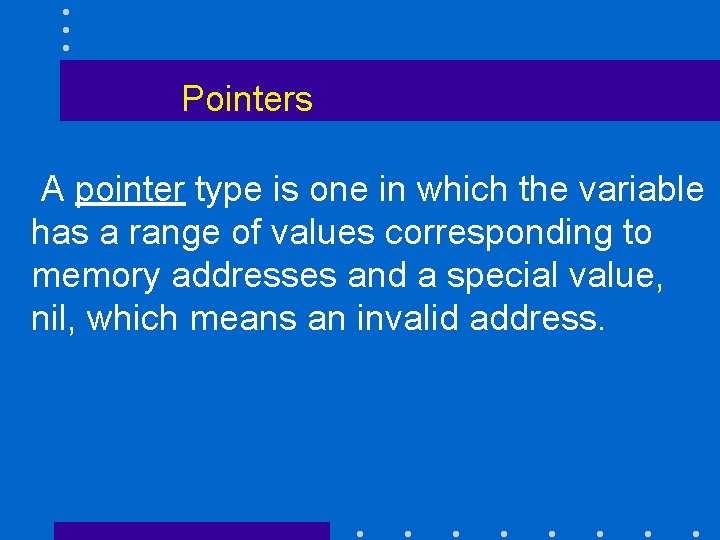
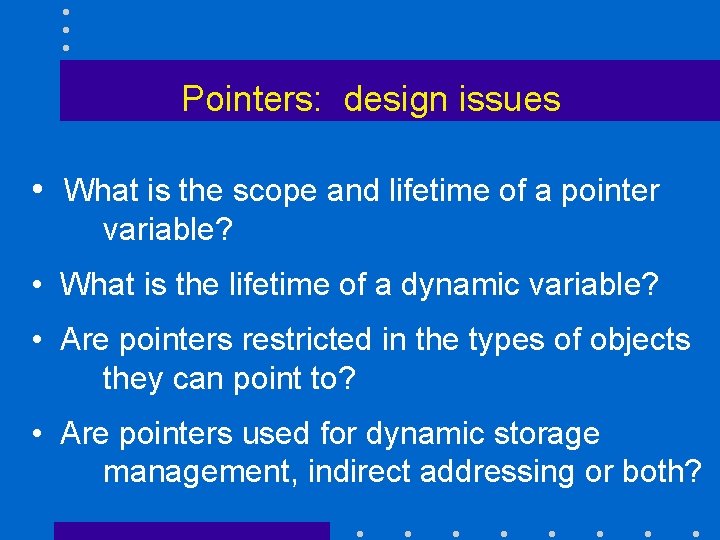
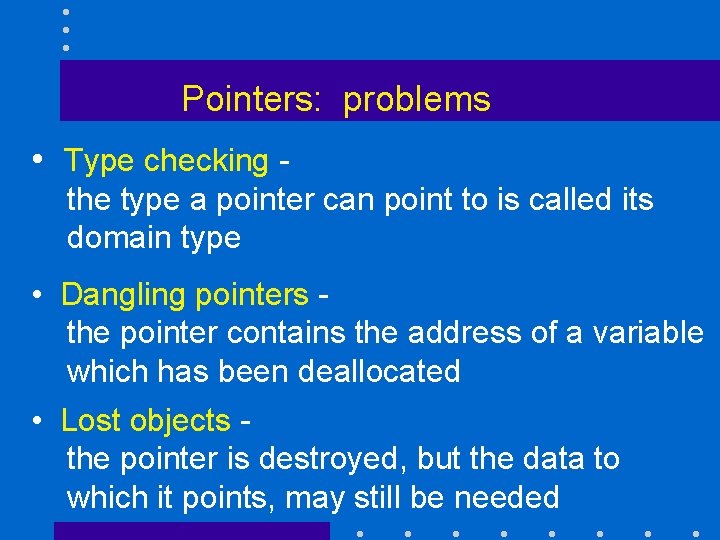
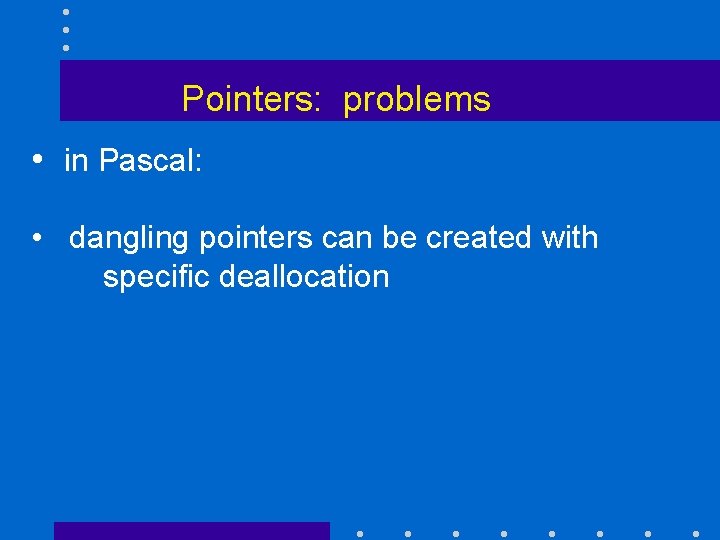
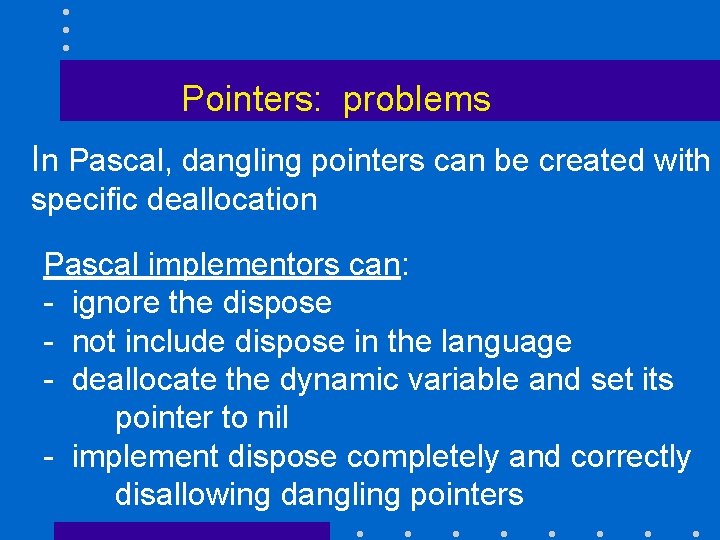
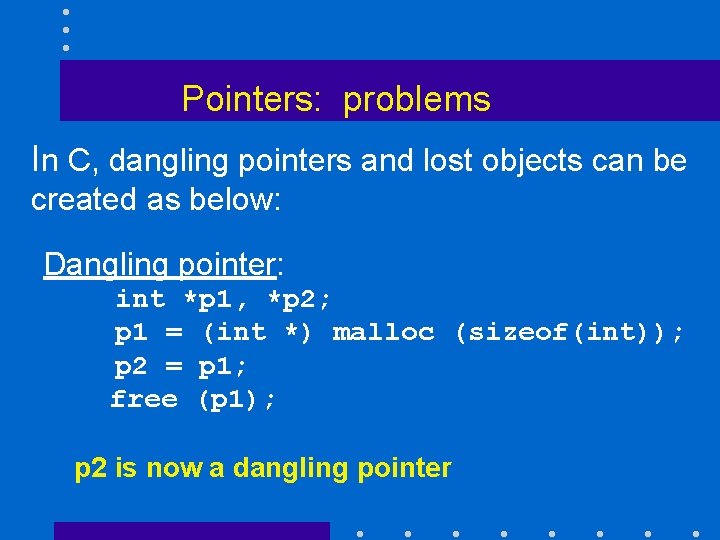
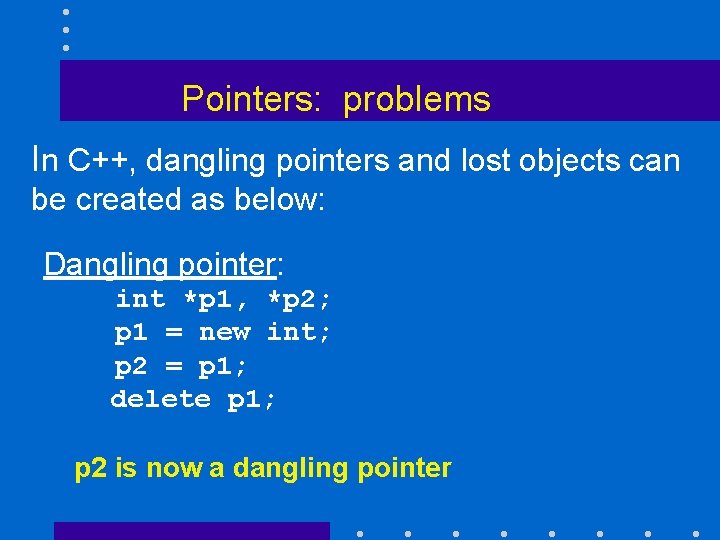
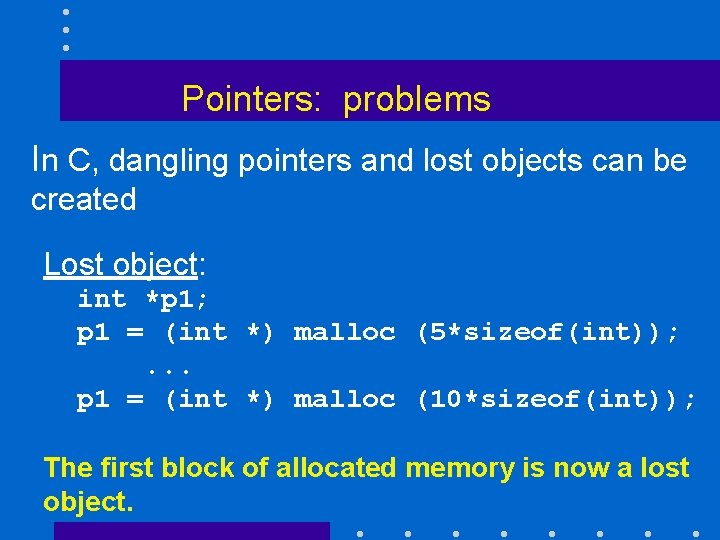
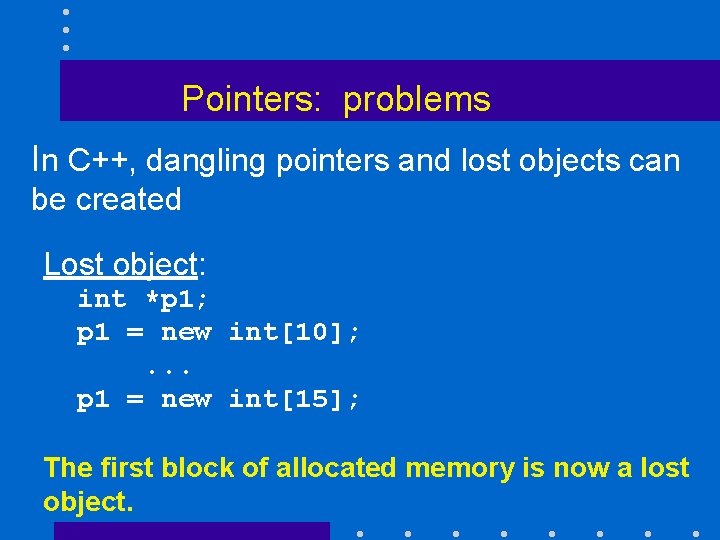
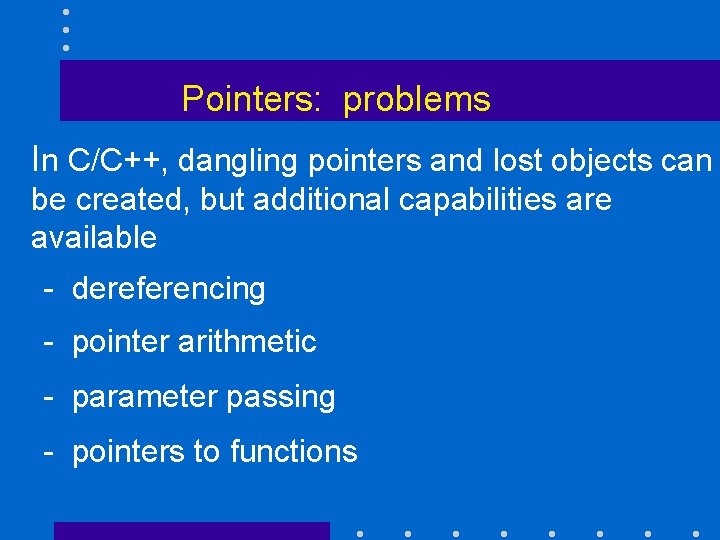
- Slides: 11
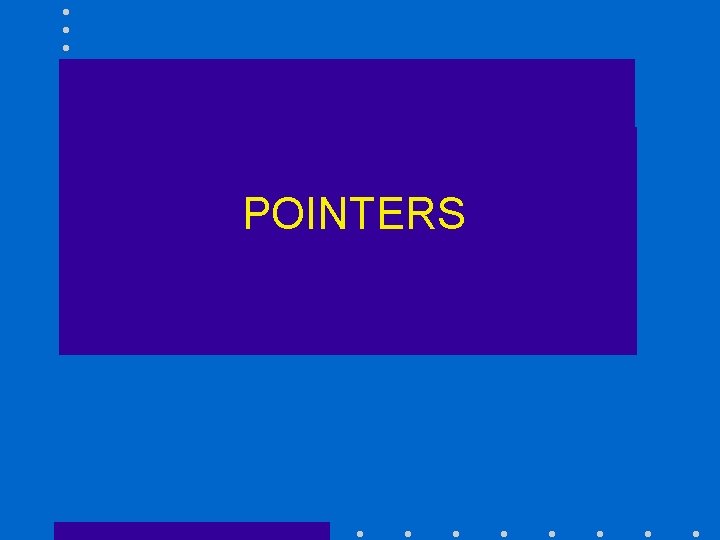
POINTERS
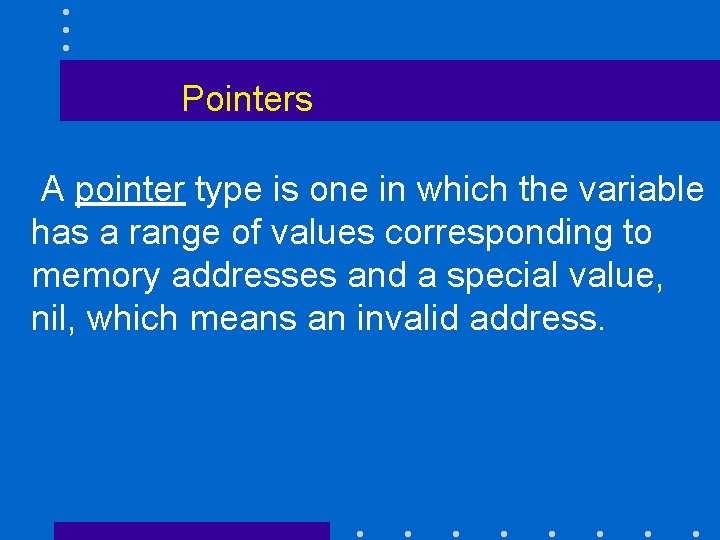
Pointers A pointer type is one in which the variable has a range of values corresponding to memory addresses and a special value, nil, which means an invalid address.
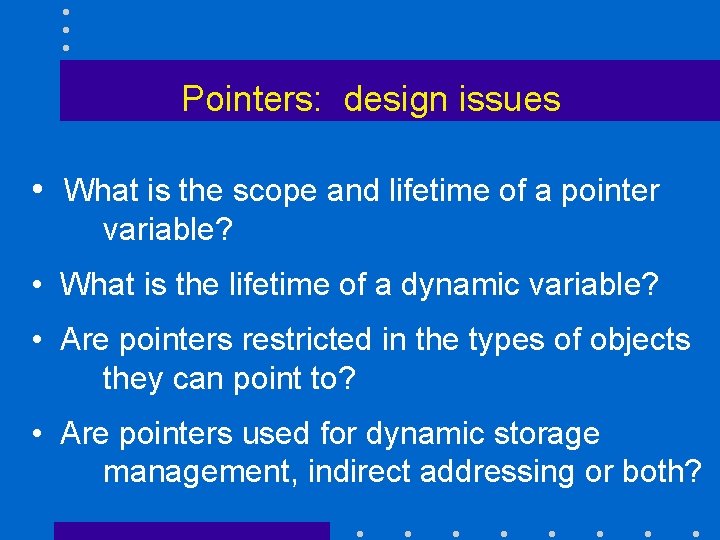
Pointers: design issues • What is the scope and lifetime of a pointer variable? • What is the lifetime of a dynamic variable? • Are pointers restricted in the types of objects they can point to? • Are pointers used for dynamic storage management, indirect addressing or both?
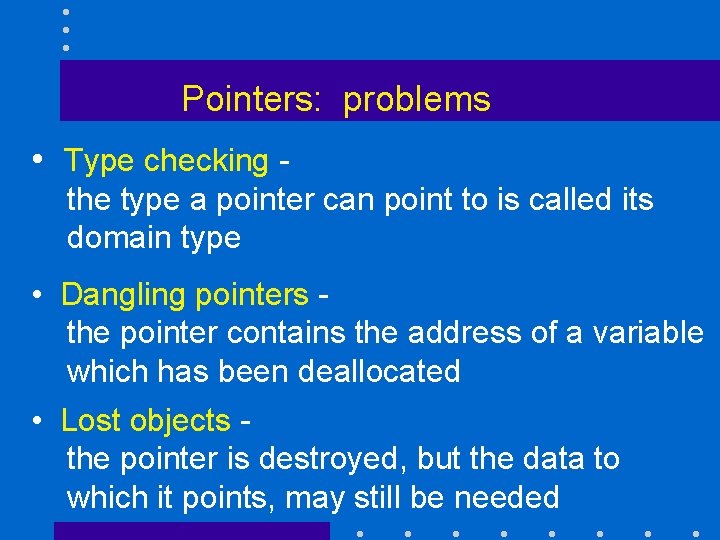
Pointers: problems • Type checking the type a pointer can point to is called its domain type • Dangling pointers the pointer contains the address of a variable which has been deallocated • Lost objects the pointer is destroyed, but the data to which it points, may still be needed
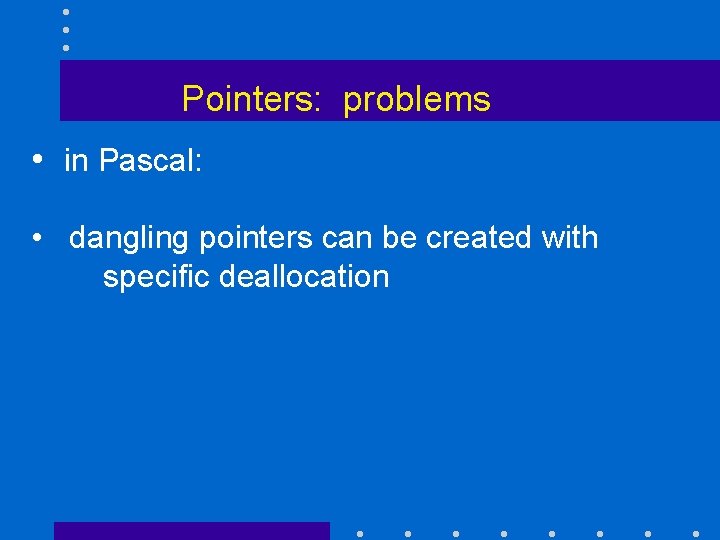
Pointers: problems • in Pascal: • dangling pointers can be created with specific deallocation
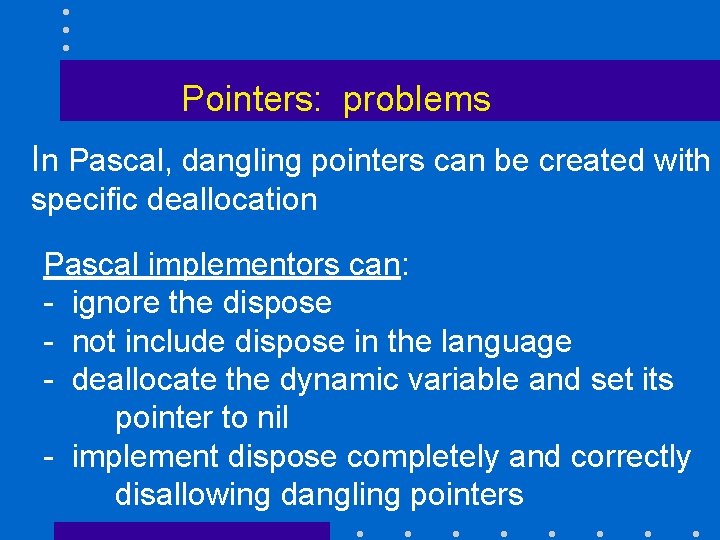
Pointers: problems In Pascal, dangling pointers can be created with specific deallocation Pascal implementors can: - ignore the dispose - not include dispose in the language - deallocate the dynamic variable and set its pointer to nil - implement dispose completely and correctly disallowing dangling pointers
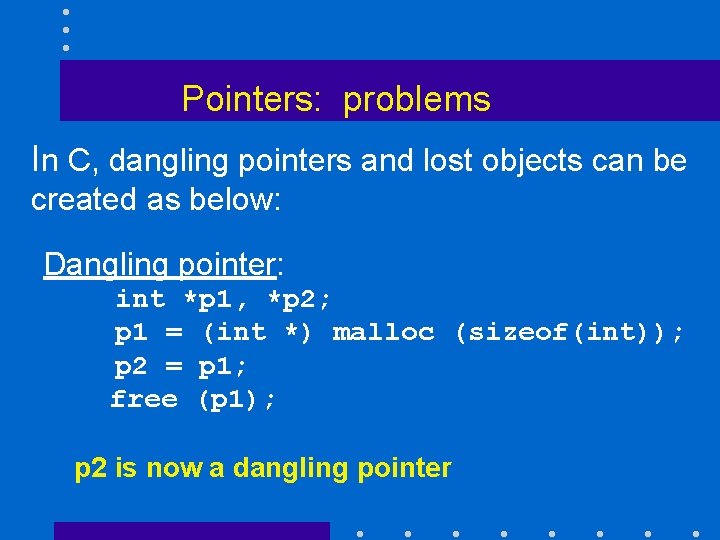
Pointers: problems In C, dangling pointers and lost objects can be created as below: Dangling pointer: int *p 1, *p 2; p 1 = (int *) malloc (sizeof(int)); p 2 = p 1; free (p 1); p 2 is now a dangling pointer
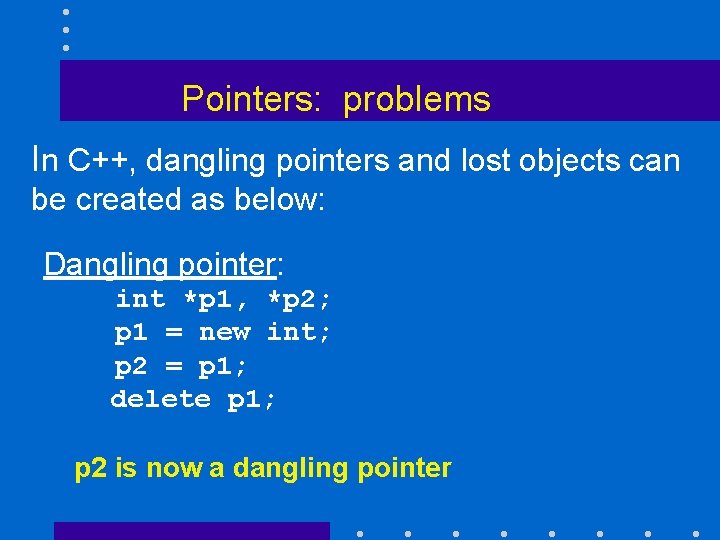
Pointers: problems In C++, dangling pointers and lost objects can be created as below: Dangling pointer: int *p 1, *p 2; p 1 = new int; p 2 = p 1; delete p 1; p 2 is now a dangling pointer
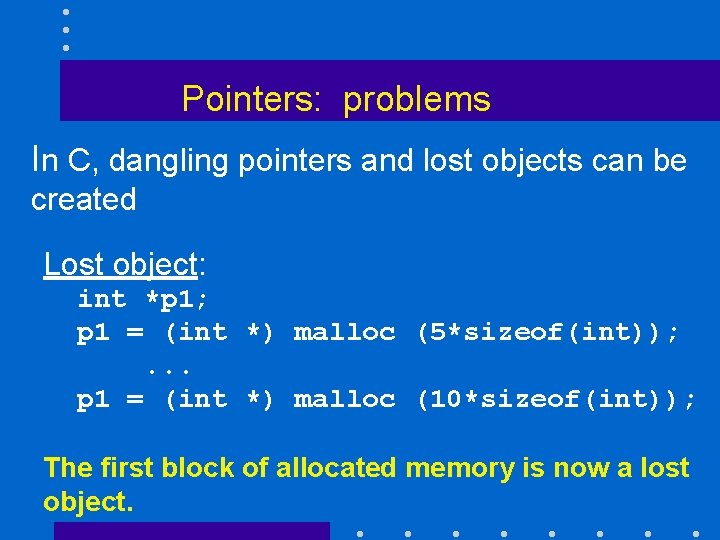
Pointers: problems In C, dangling pointers and lost objects can be created Lost object: int *p 1; p 1 = (int *) malloc (5*sizeof(int)); . . . p 1 = (int *) malloc (10*sizeof(int)); The first block of allocated memory is now a lost object.
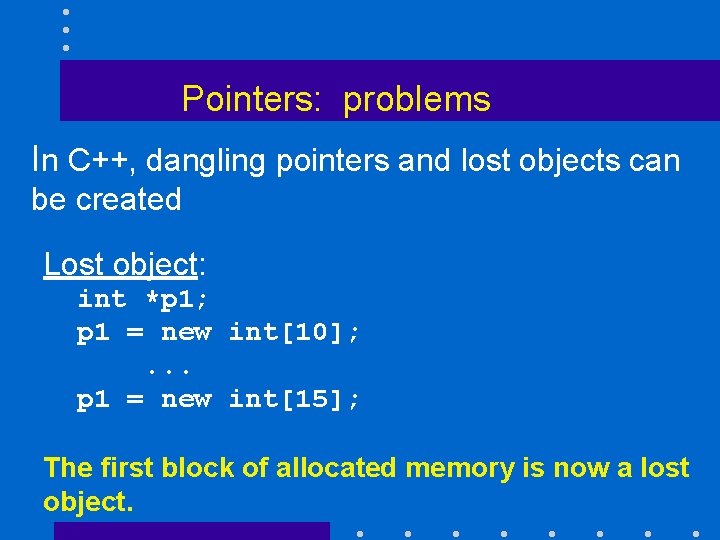
Pointers: problems In C++, dangling pointers and lost objects can be created Lost object: int *p 1; p 1 = new int[10]; . . . p 1 = new int[15]; The first block of allocated memory is now a lost object.
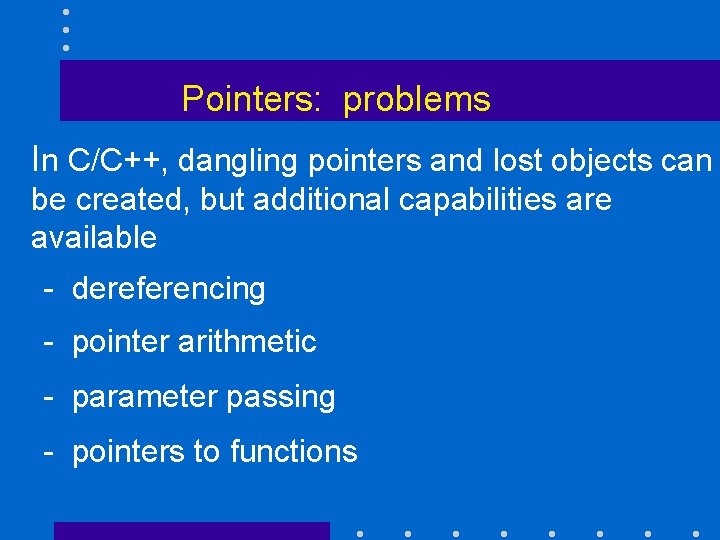
Pointers: problems In C/C++, dangling pointers and lost objects can be created, but additional capabilities are available - dereferencing - pointer arithmetic - parameter passing - pointers to functions
Constant pointer and pointer to constant
What is pointer to pointer in c
9 pointers
Constant to pointer in c
Display the address of intval using cout and intptr.
Pointer expressions and pointer arithmetic
Pointer pointer
Pointer pointer
Pointeris
One full revolution of the pointer on the dial equals:
Pointer and one dimensional array
Rock cycle