Chapter 7 Strings F Processing strings using the
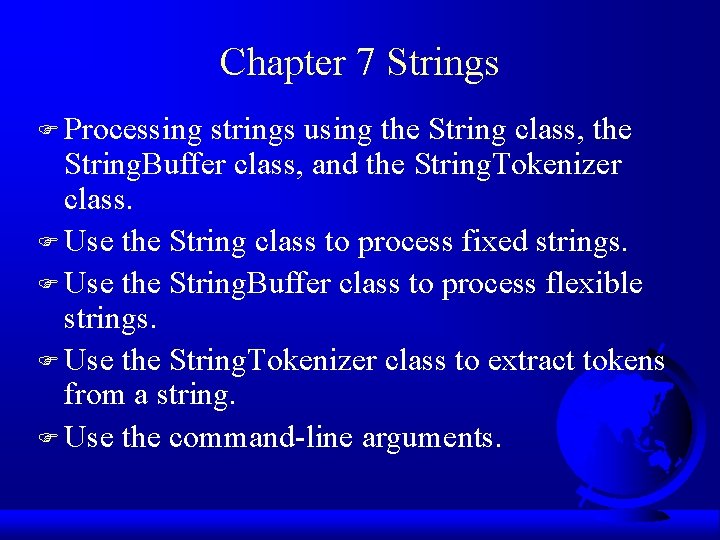
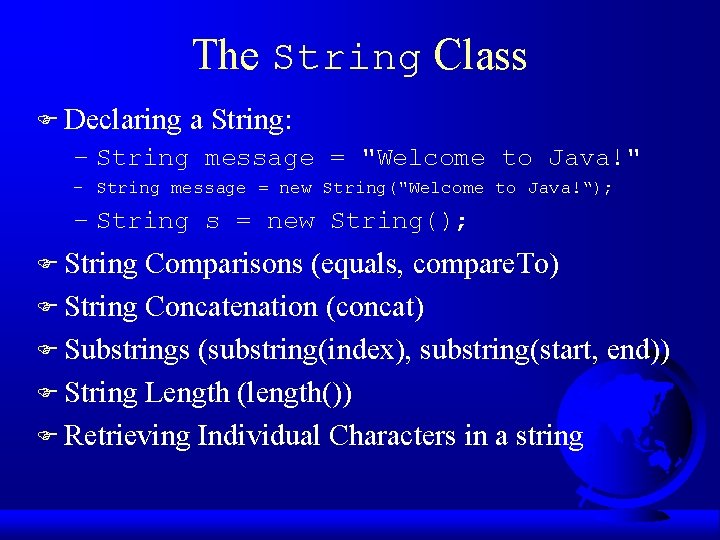
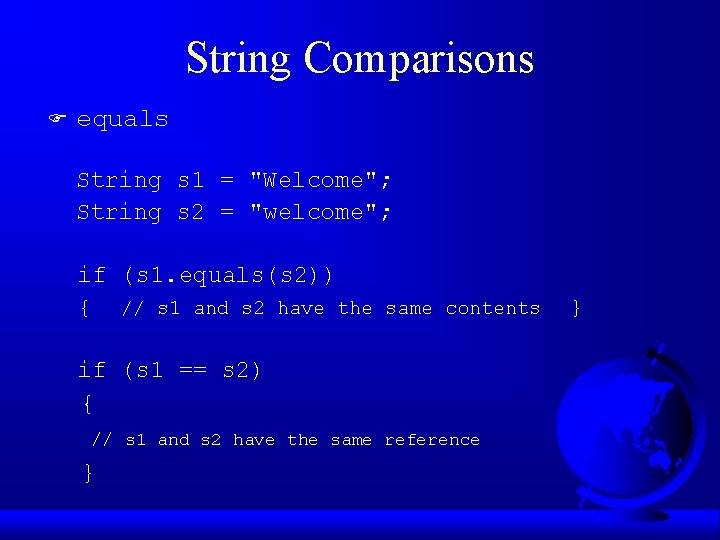
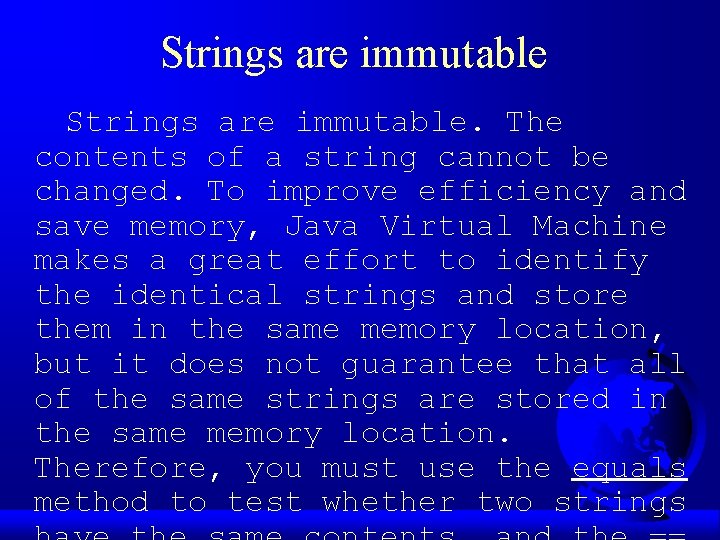
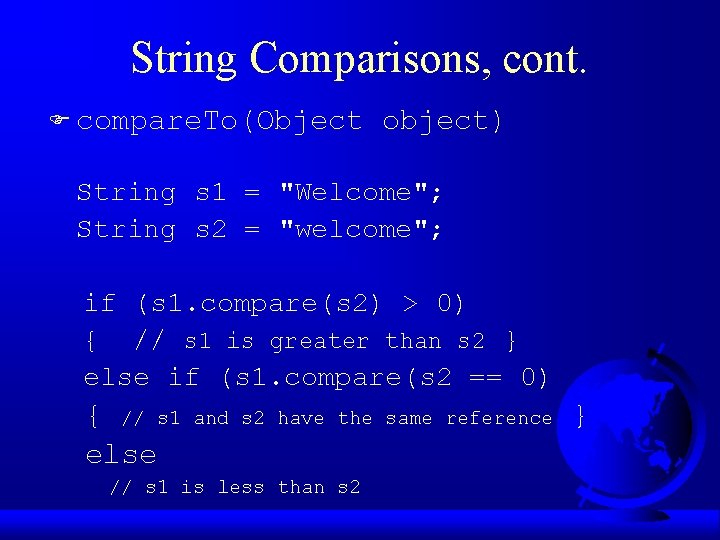
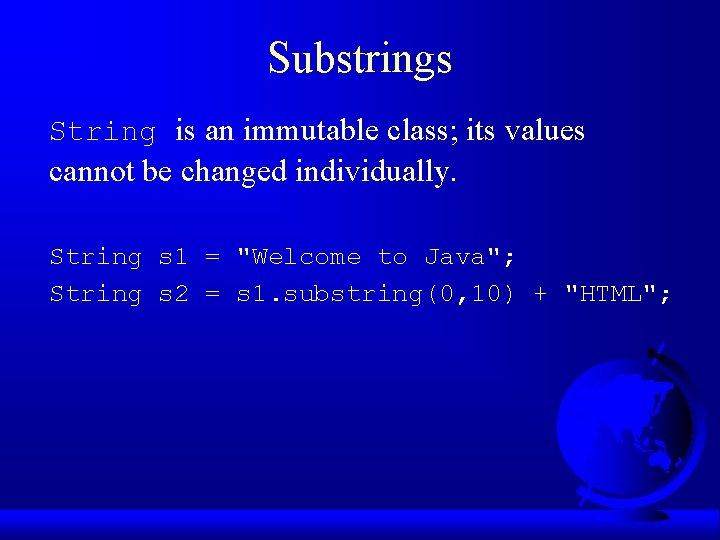
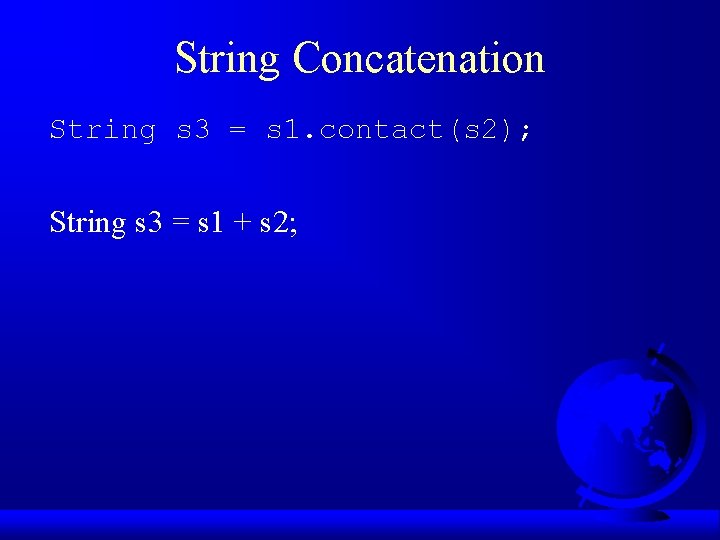
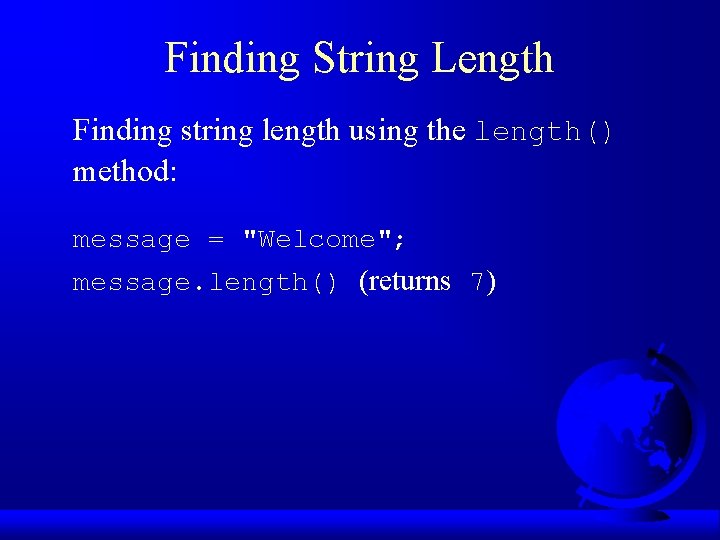
![Retrieving Individual Characters in a String F Do not use message[0] F Use message. Retrieving Individual Characters in a String F Do not use message[0] F Use message.](https://slidetodoc.com/presentation_image_h2/063cc76cb985ec44cb387df23ba0e951/image-9.jpg)
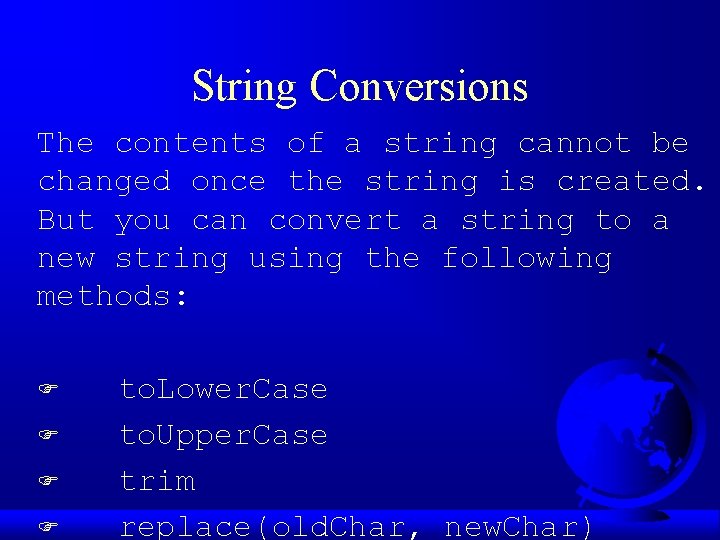
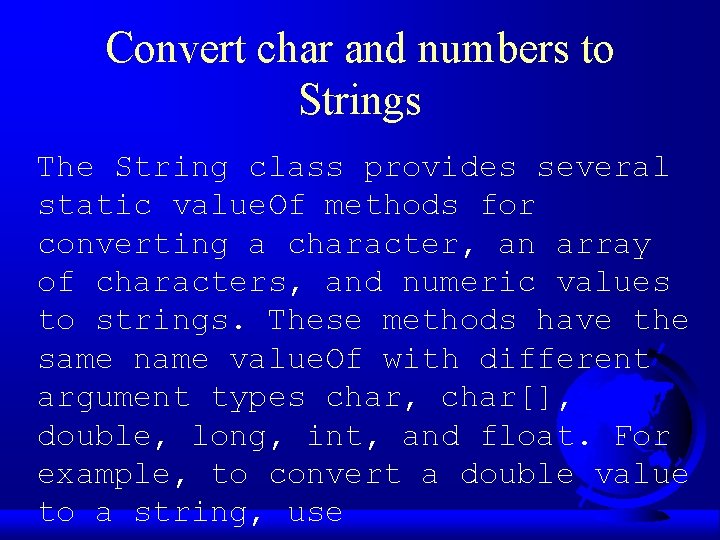
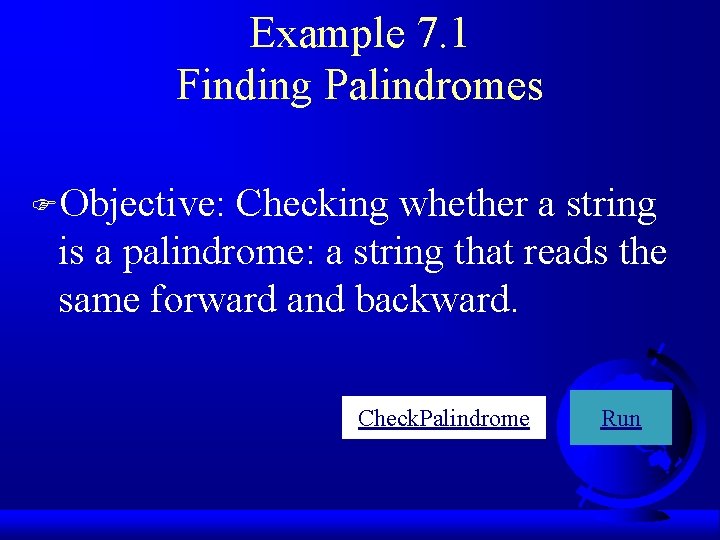
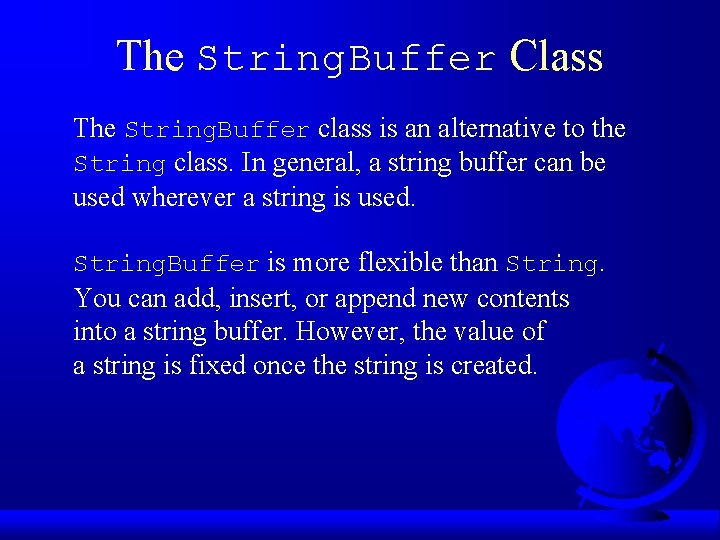
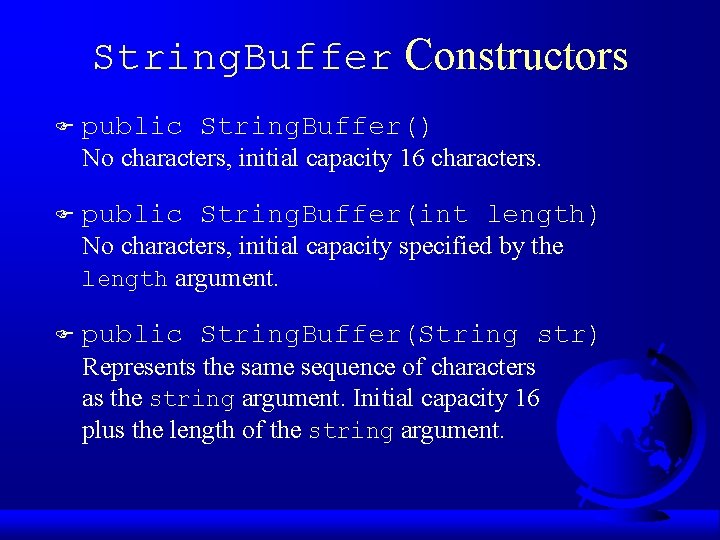
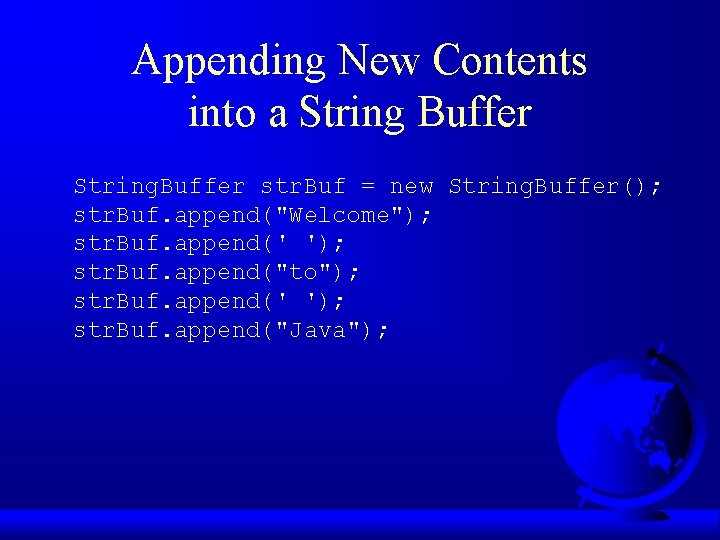
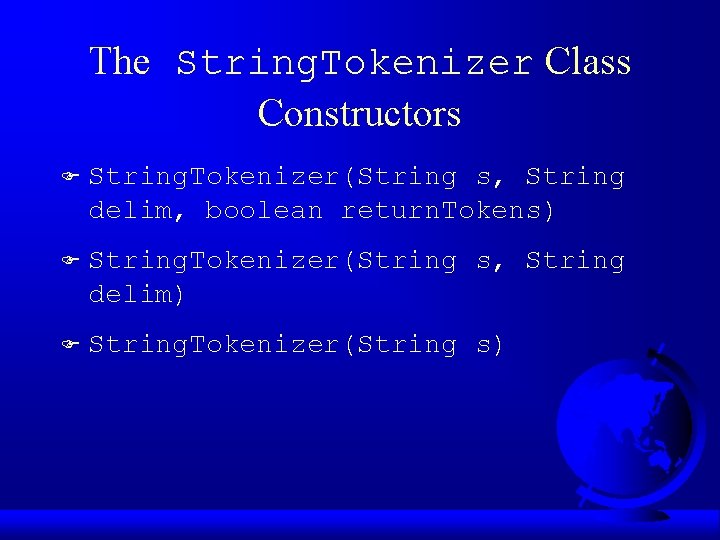
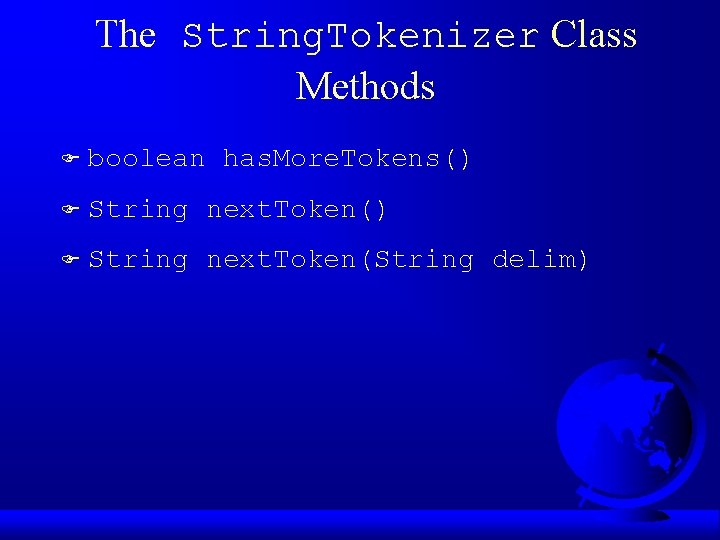
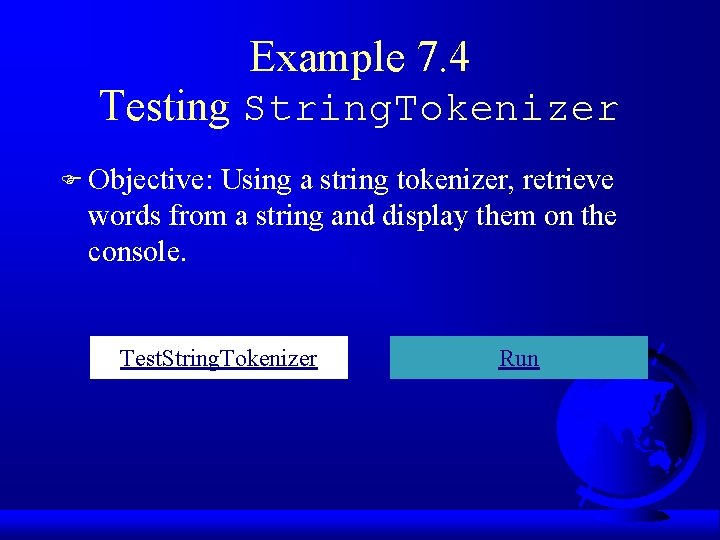
![Command-Line Parameters class Test. Main { public static void main(String[] args) {. . . Command-Line Parameters class Test. Main { public static void main(String[] args) {. . .](https://slidetodoc.com/presentation_image_h2/063cc76cb985ec44cb387df23ba0e951/image-19.jpg)
![Processing Command-Line Parameters In the main method, get the arguments from args[0], args[1], . Processing Command-Line Parameters In the main method, get the arguments from args[0], args[1], .](https://slidetodoc.com/presentation_image_h2/063cc76cb985ec44cb387df23ba0e951/image-20.jpg)
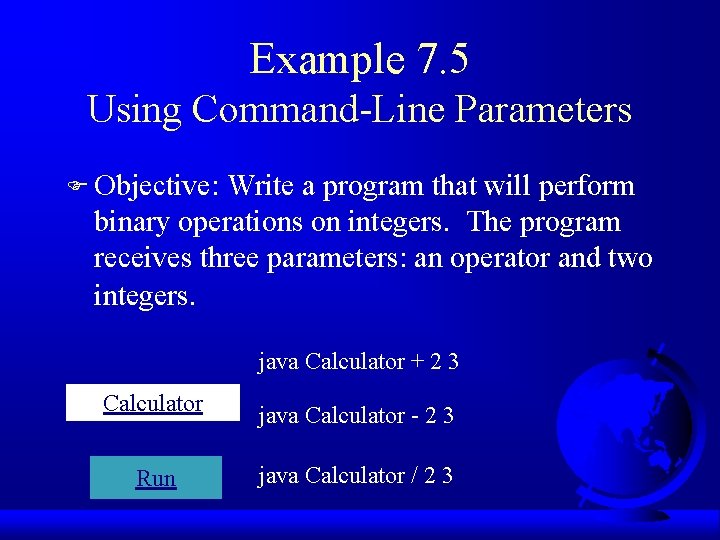
- Slides: 21
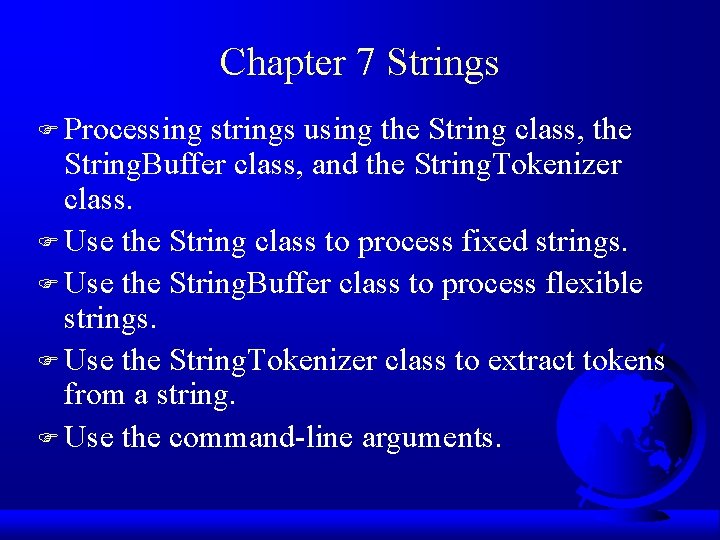
Chapter 7 Strings F Processing strings using the String class, the String. Buffer class, and the String. Tokenizer class. F Use the String class to process fixed strings. F Use the String. Buffer class to process flexible strings. F Use the String. Tokenizer class to extract tokens from a string. F Use the command-line arguments.
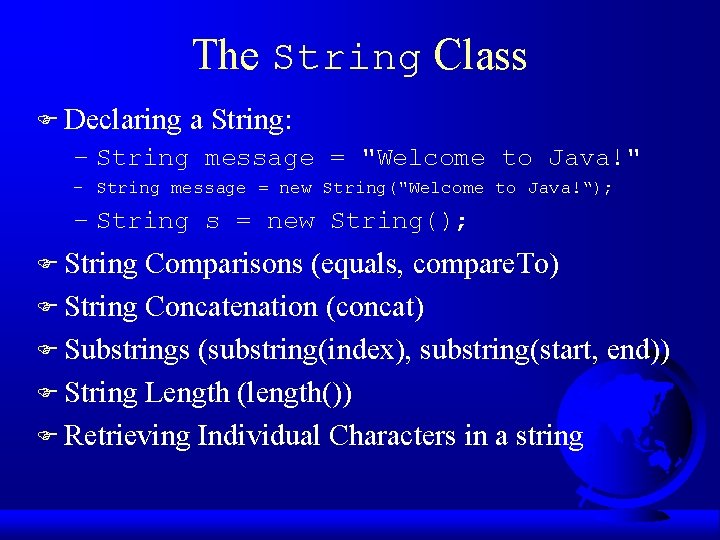
The String Class F Declaring a String: – String message = "Welcome to Java!" – String message = new String("Welcome to Java!“); – String s = new String(); F String Comparisons (equals, compare. To) F String Concatenation (concat) F Substrings (substring(index), substring(start, end)) F String Length (length()) F Retrieving Individual Characters in a string
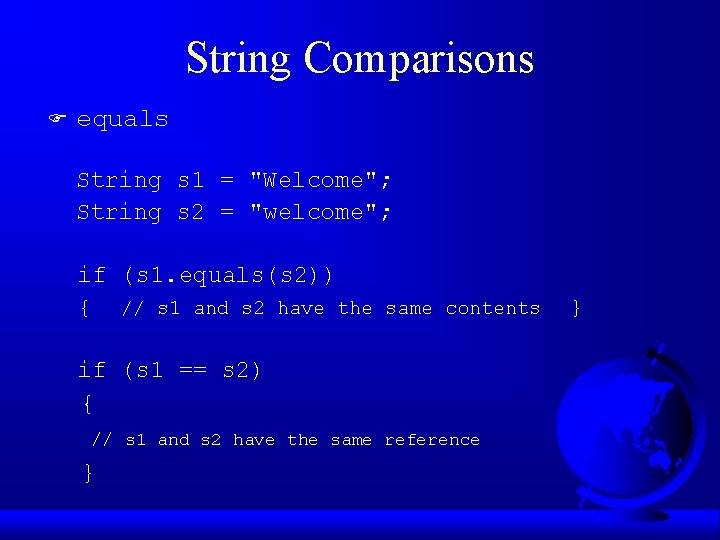
String Comparisons F equals String s 1 = "Welcome"; String s 2 = "welcome"; if (s 1. equals(s 2)) { // s 1 and s 2 have the same contents if (s 1 == s 2) { // s 1 and s 2 have the same reference } }
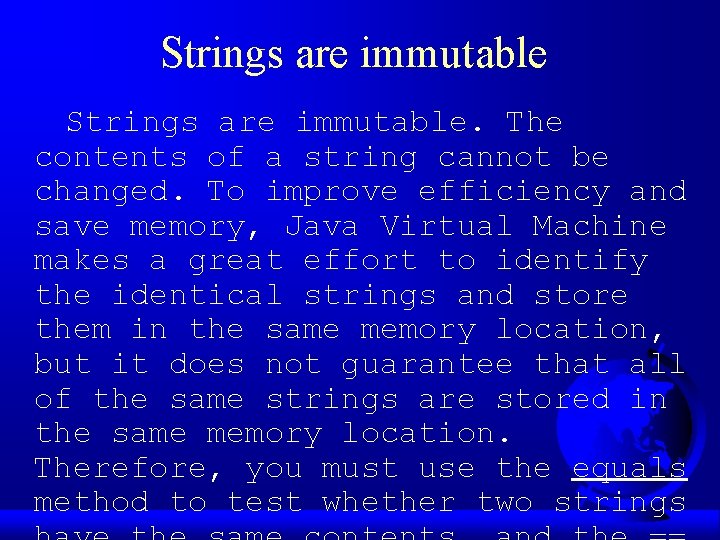
Strings are immutable. The contents of a string cannot be changed. To improve efficiency and save memory, Java Virtual Machine makes a great effort to identify the identical strings and store them in the same memory location, but it does not guarantee that all of the same strings are stored in the same memory location. Therefore, you must use the equals method to test whether two strings
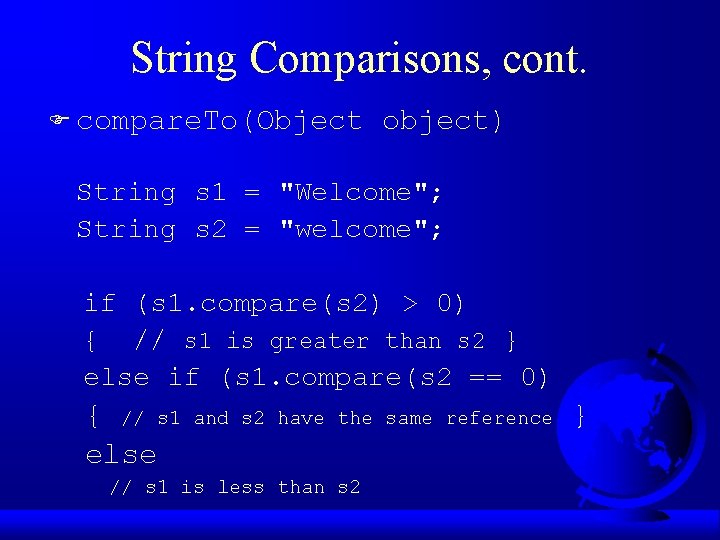
String Comparisons, cont. F compare. To(Object object) String s 1 = "Welcome"; String s 2 = "welcome"; if (s 1. compare(s 2) > 0) { // s 1 is greater than s 2 } else if (s 1. compare(s 2 == 0) { // s 1 else and s 2 have the same reference // s 1 is less than s 2 }
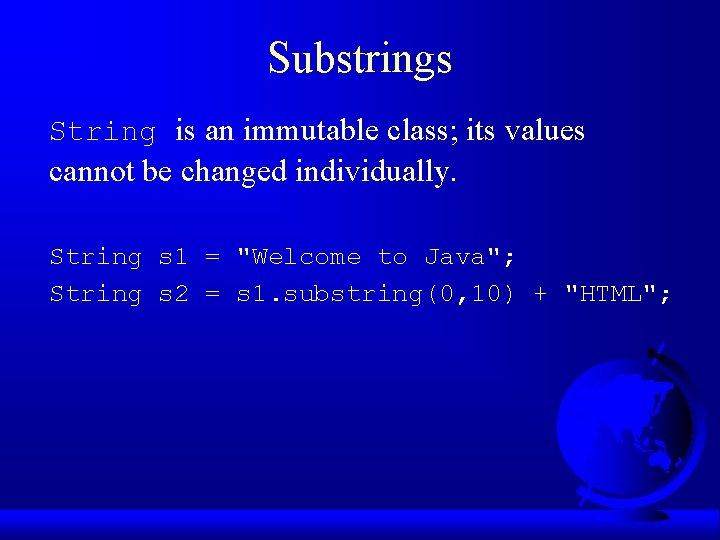
Substrings String is an immutable class; its values cannot be changed individually. String s 1 = "Welcome to Java"; String s 2 = s 1. substring(0, 10) + "HTML";
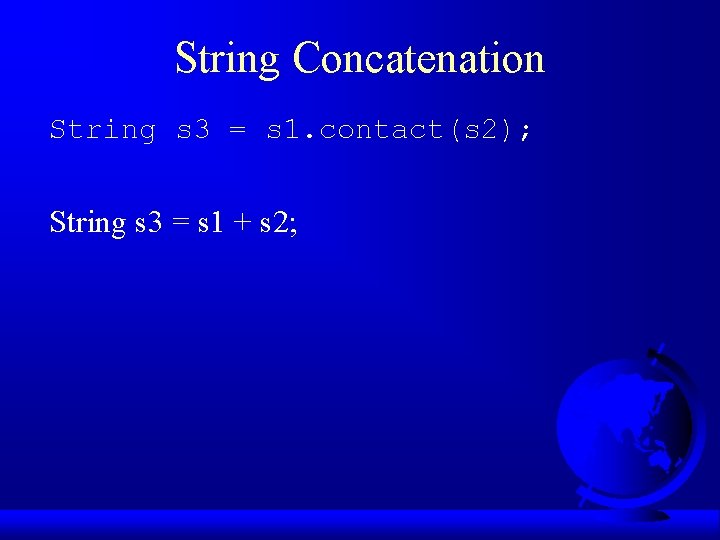
String Concatenation String s 3 = s 1. contact(s 2); String s 3 = s 1 + s 2;
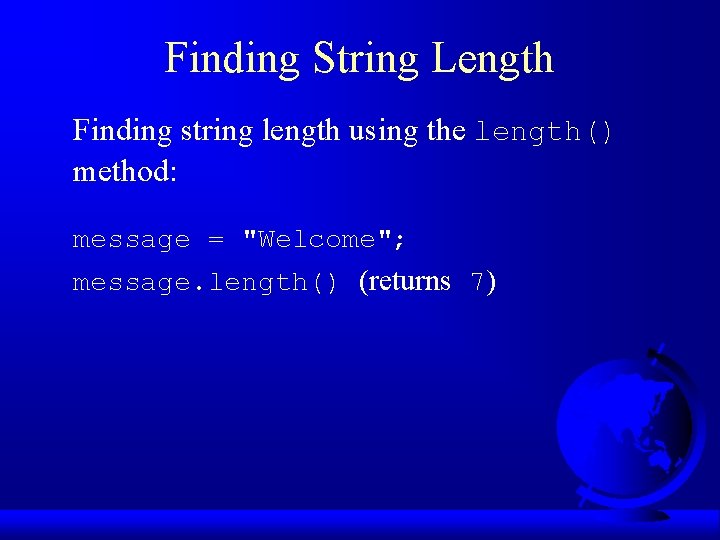
Finding String Length Finding string length using the length() method: message = "Welcome"; message. length() (returns 7)
![Retrieving Individual Characters in a String F Do not use message0 F Use message Retrieving Individual Characters in a String F Do not use message[0] F Use message.](https://slidetodoc.com/presentation_image_h2/063cc76cb985ec44cb387df23ba0e951/image-9.jpg)
Retrieving Individual Characters in a String F Do not use message[0] F Use message. char. At(index) F Index starts from 0
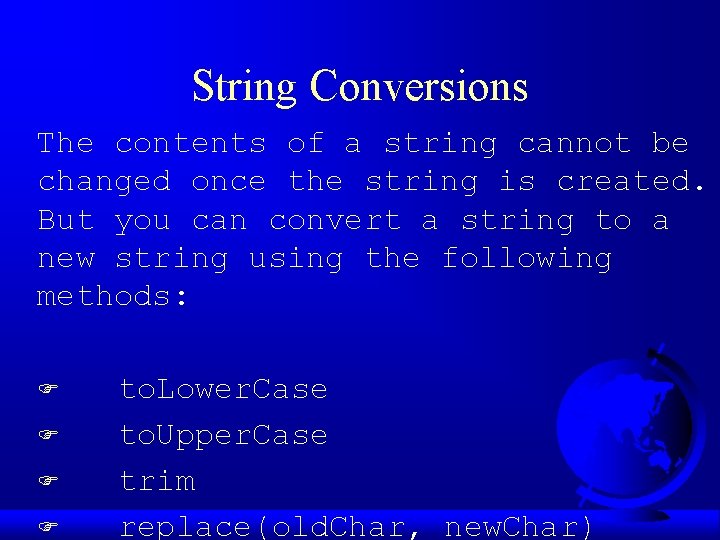
String Conversions The contents of a string cannot be changed once the string is created. But you can convert a string to a new string using the following methods: F F to. Lower. Case to. Upper. Case trim replace(old. Char, new. Char)
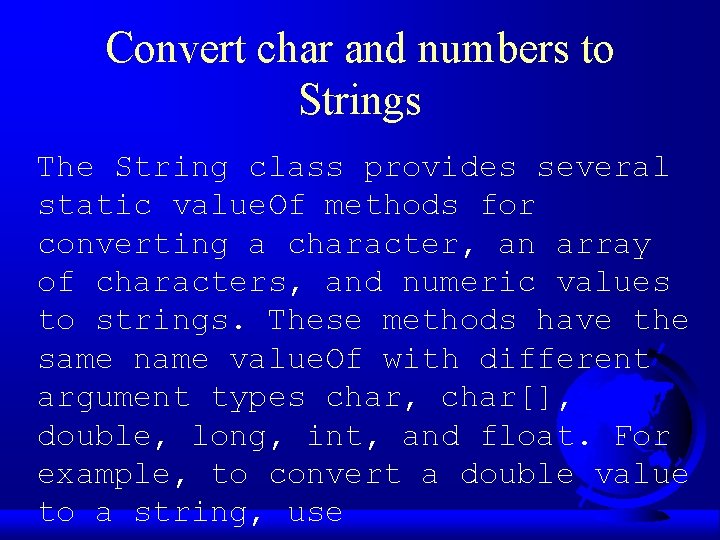
Convert char and numbers to Strings The String class provides several static value. Of methods for converting a character, an array of characters, and numeric values to strings. These methods have the same name value. Of with different argument types char, char[], double, long, int, and float. For example, to convert a double value to a string, use
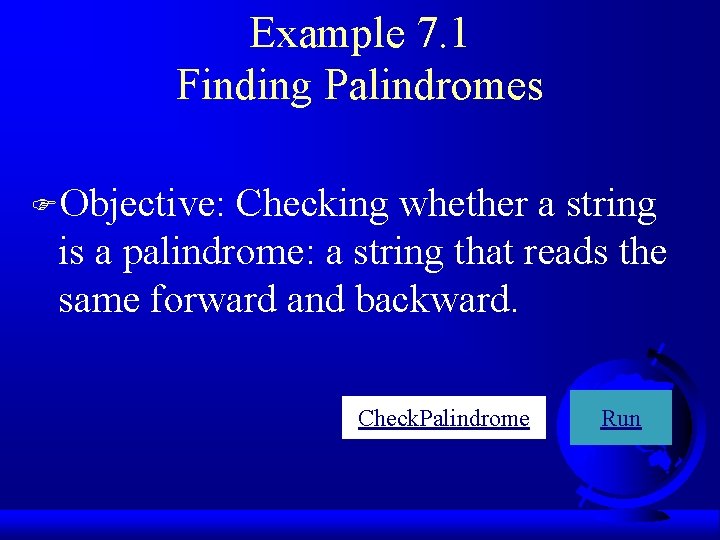
Example 7. 1 Finding Palindromes FObjective: Checking whether a string is a palindrome: a string that reads the same forward and backward. Check. Palindrome Run
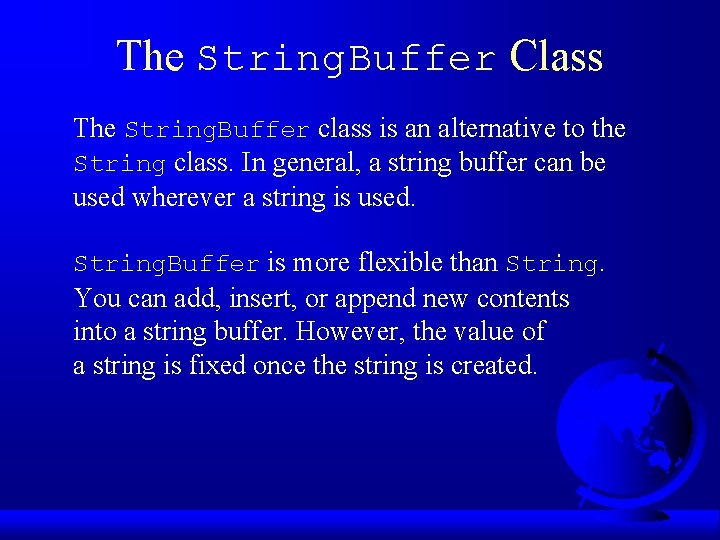
The String. Buffer Class The String. Buffer class is an alternative to the String class. In general, a string buffer can be used wherever a string is used. String. Buffer is more flexible than String. You can add, insert, or append new contents into a string buffer. However, the value of a string is fixed once the string is created.
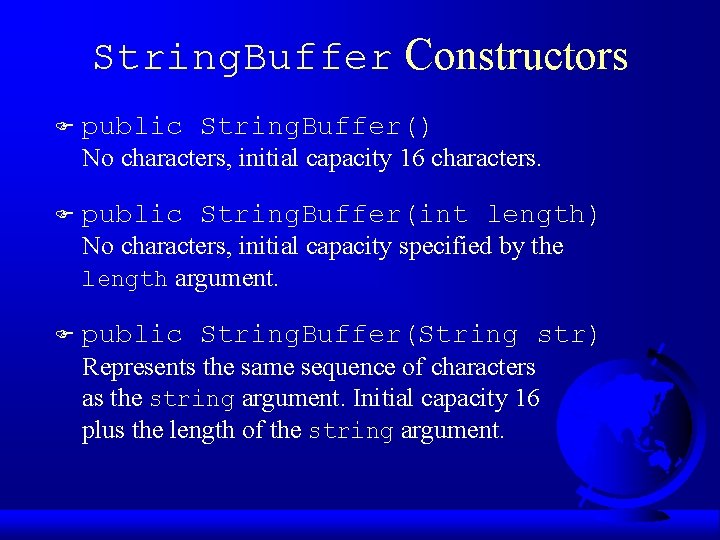
String. Buffer Constructors F public String. Buffer() No characters, initial capacity 16 characters. F public String. Buffer(int length) No characters, initial capacity specified by the length argument. F public String. Buffer(String str) Represents the same sequence of characters as the string argument. Initial capacity 16 plus the length of the string argument.
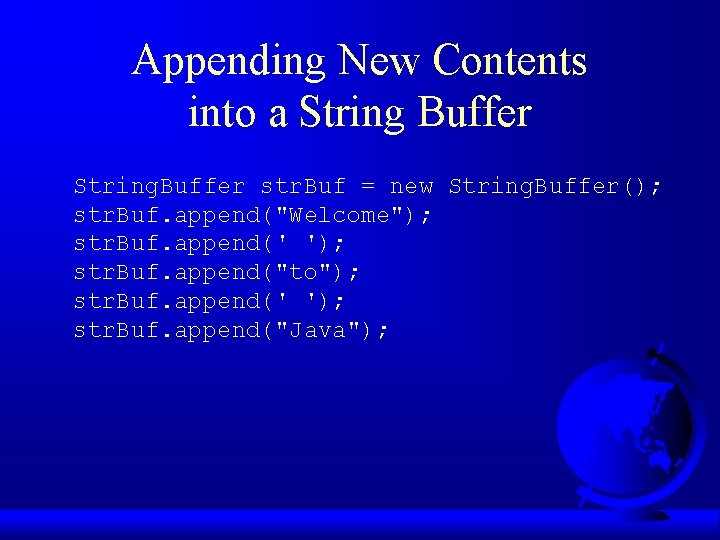
Appending New Contents into a String Buffer String. Buffer str. Buf = new String. Buffer(); str. Buf. append("Welcome"); str. Buf. append(' '); str. Buf. append("to"); str. Buf. append(' '); str. Buf. append("Java");
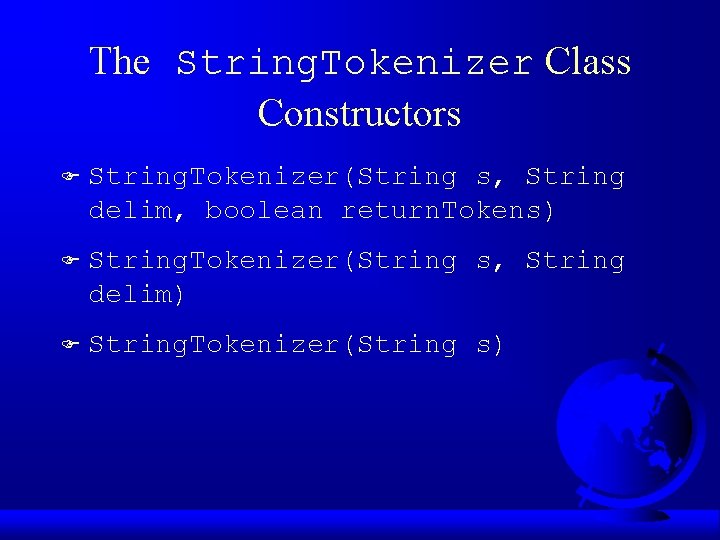
The String. Tokenizer Class Constructors F String. Tokenizer(String s, String delim, boolean return. Tokens) F String. Tokenizer(String s, String delim) F String. Tokenizer(String s)
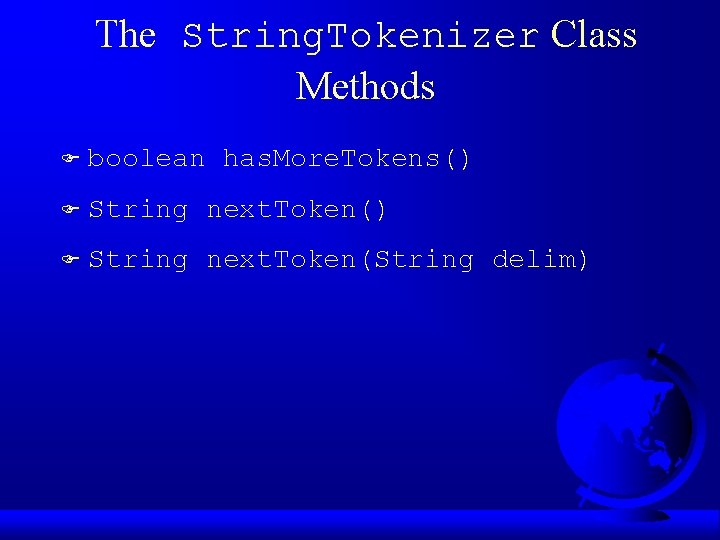
The String. Tokenizer Class Methods F boolean has. More. Tokens() F String next. Token(String delim)
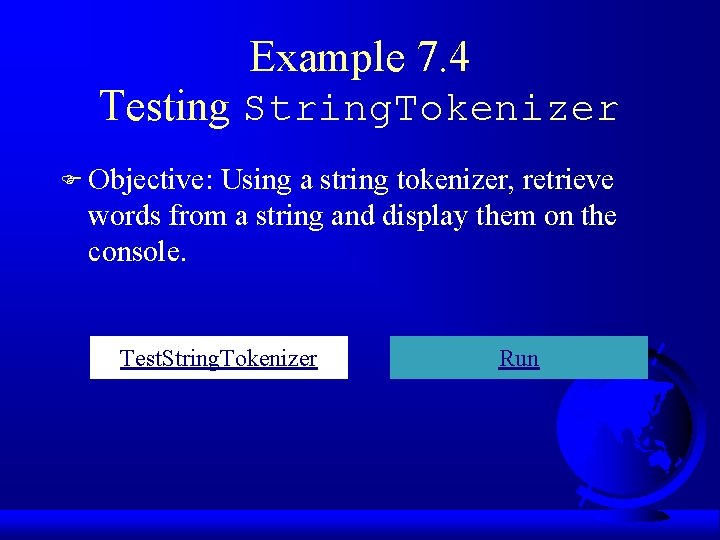
Example 7. 4 Testing String. Tokenizer F Objective: Using a string tokenizer, retrieve words from a string and display them on the console. Test. String. Tokenizer Run
![CommandLine Parameters class Test Main public static void mainString args Command-Line Parameters class Test. Main { public static void main(String[] args) {. . .](https://slidetodoc.com/presentation_image_h2/063cc76cb985ec44cb387df23ba0e951/image-19.jpg)
Command-Line Parameters class Test. Main { public static void main(String[] args) {. . . } } java Test. Main arg 0 arg 1 arg 2. . . argn
![Processing CommandLine Parameters In the main method get the arguments from args0 args1 Processing Command-Line Parameters In the main method, get the arguments from args[0], args[1], .](https://slidetodoc.com/presentation_image_h2/063cc76cb985ec44cb387df23ba0e951/image-20.jpg)
Processing Command-Line Parameters In the main method, get the arguments from args[0], args[1], . . . , args[n], which corresponds to arg 0, arg 1, . . . , argn in the command line.
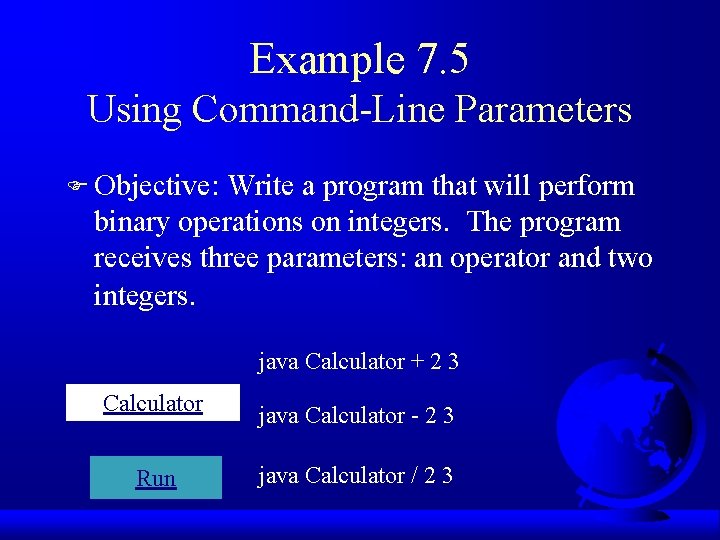
Example 7. 5 Using Command-Line Parameters F Objective: Write a program that will perform binary operations on integers. The program receives three parameters: an operator and two integers. java Calculator + 2 3 Calculator Run java Calculator - 2 3 java Calculator / 2 3
Bottom up and top down processing
Gloria suarez
Bottom up and top down processing
Point processing operations
What is secondary food processing
What is point processing in digital image processing
Histogram processing in digital image processing
Parallel processing vs concurrent processing
A generalization of unsharp masking is
پردازش تصویر
Thinning and thickening in image processing example
Top down vs bottom up psychology
Interactive processing
Hát kết hợp bộ gõ cơ thể
Bổ thể
Tỉ lệ cơ thể trẻ em
Chó sói
Tư thế worm breton
Bài hát chúa yêu trần thế alleluia
Các môn thể thao bắt đầu bằng tiếng đua
Thế nào là hệ số cao nhất