Chapter 17 2 Templates and Exceptions DaleWeems 1
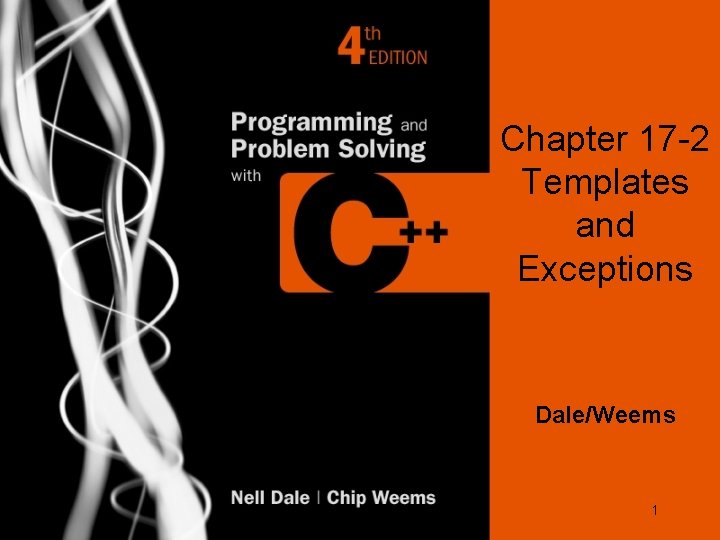
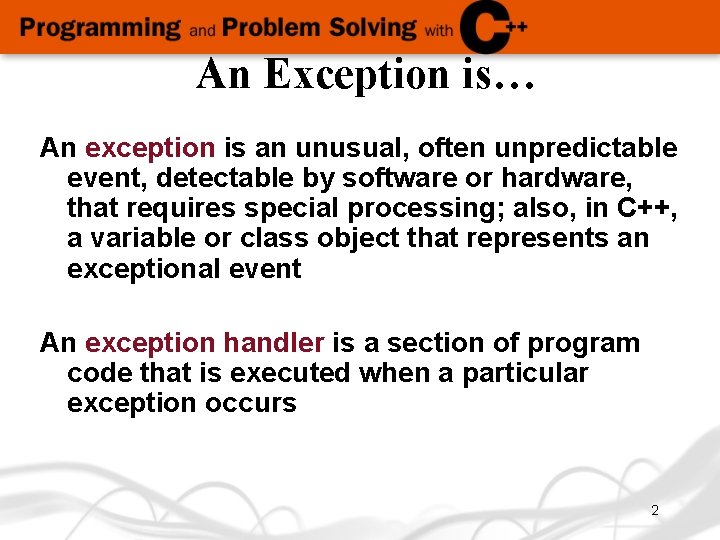
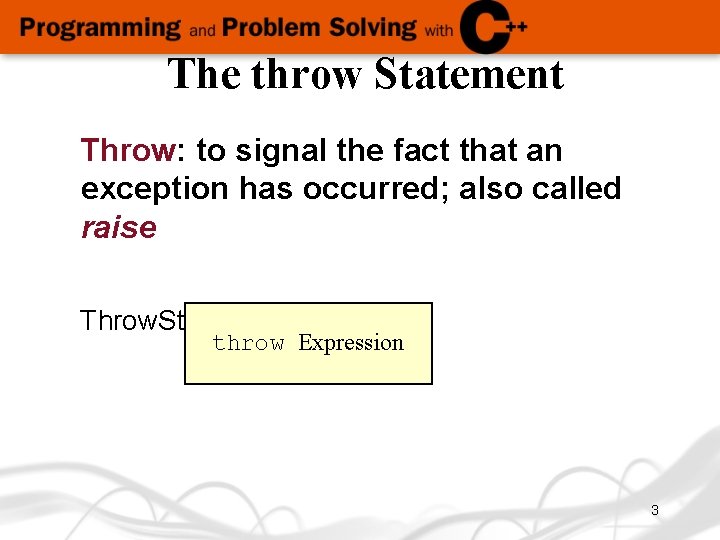
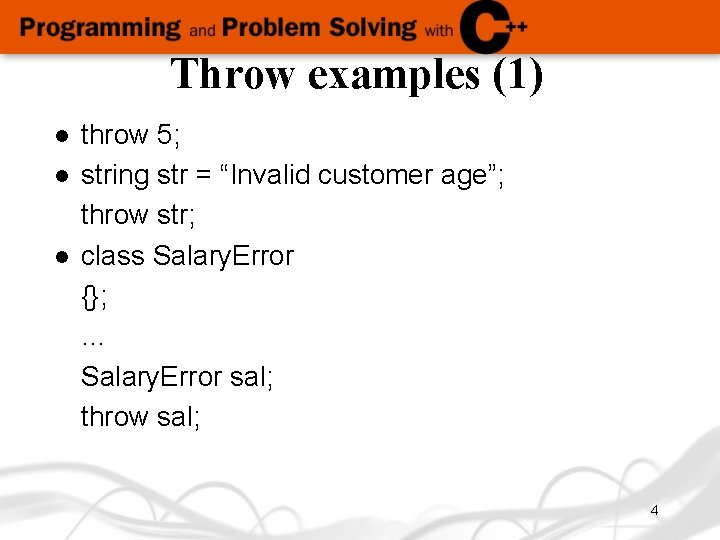
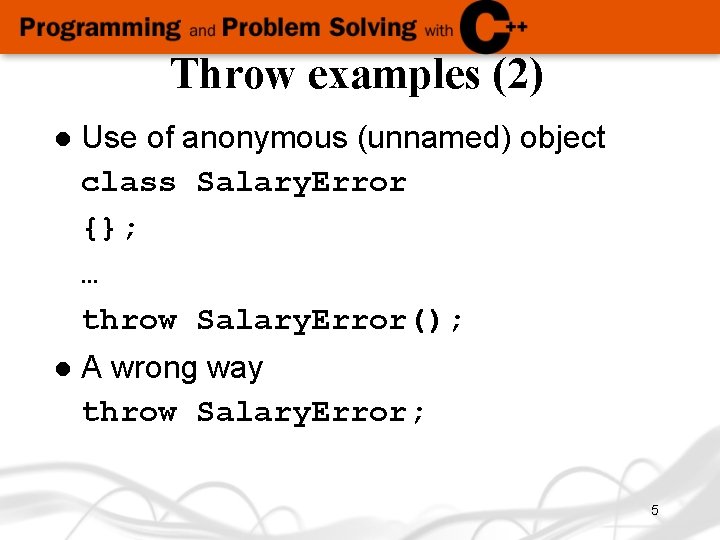
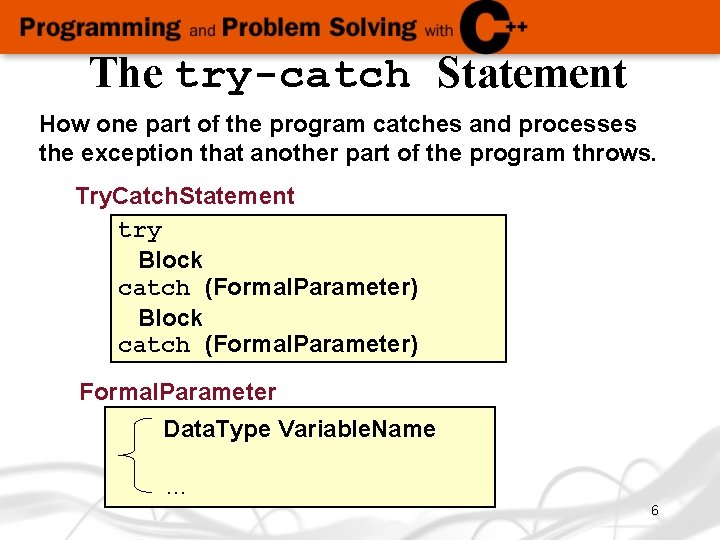
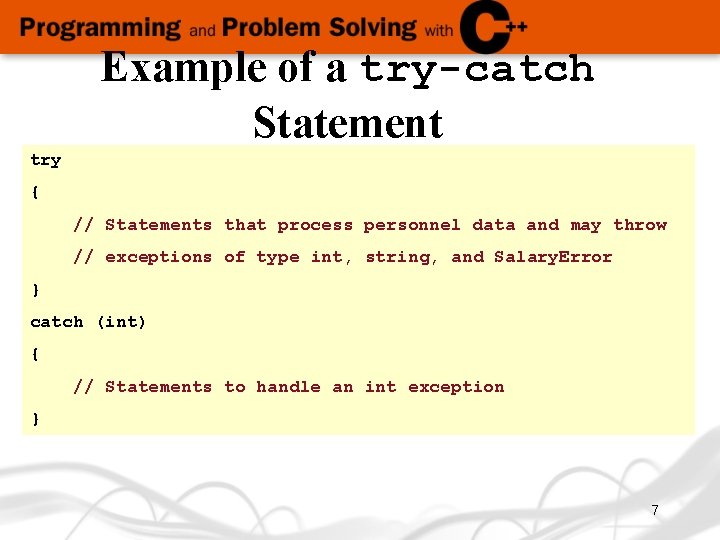
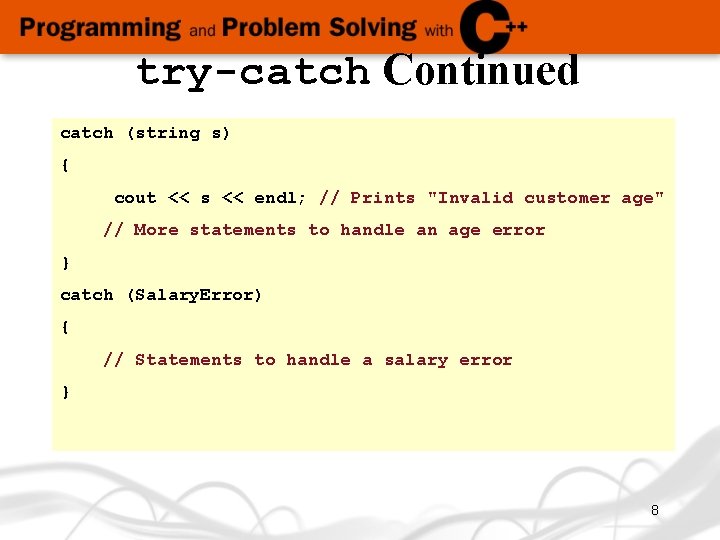
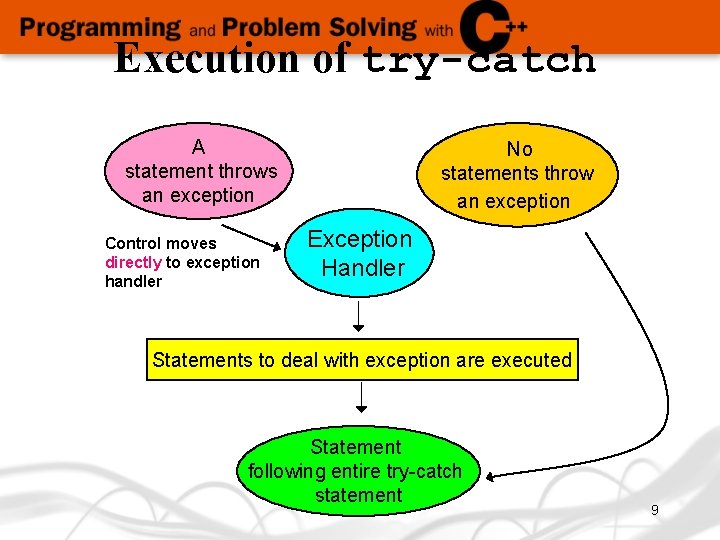
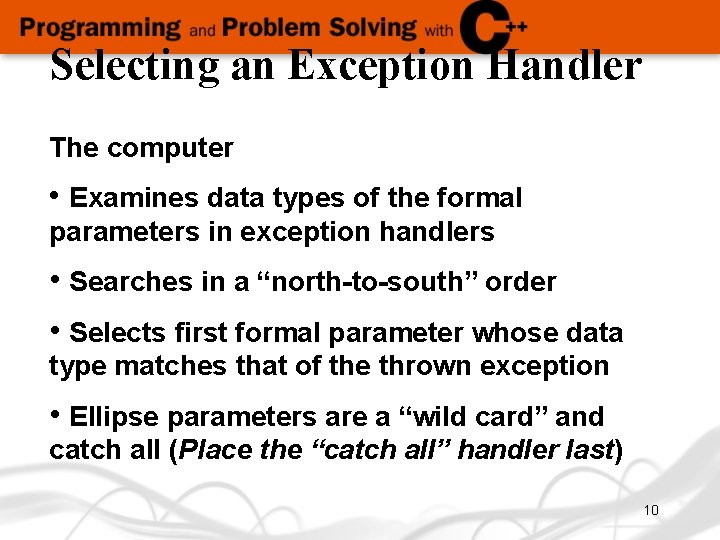
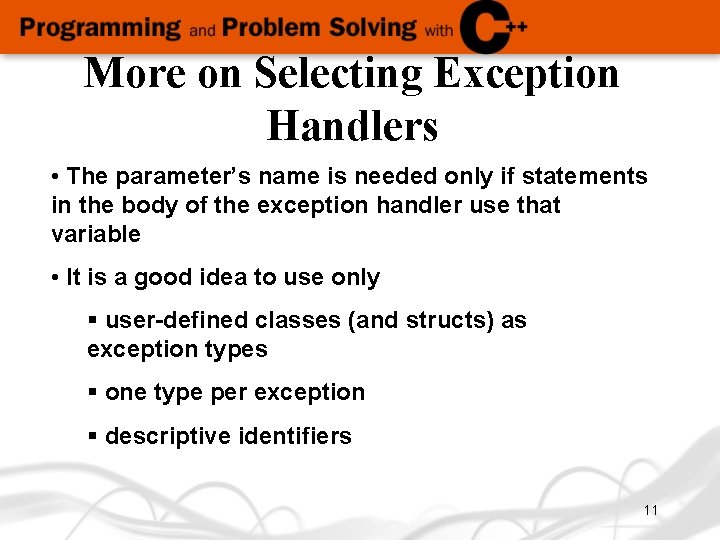
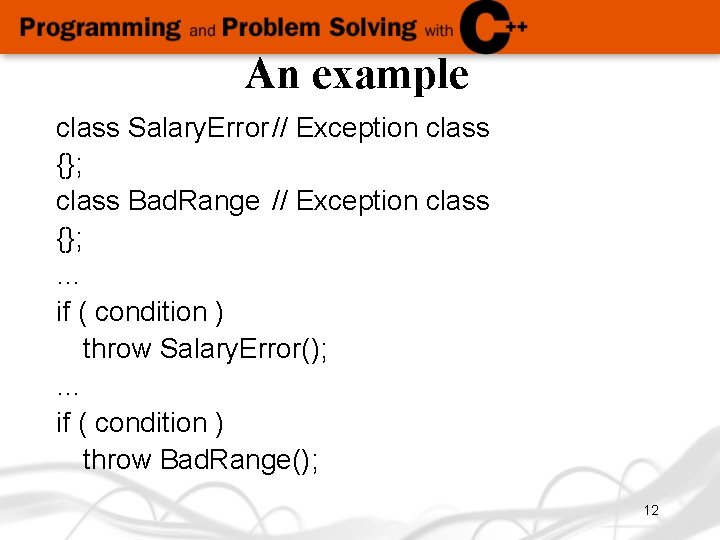
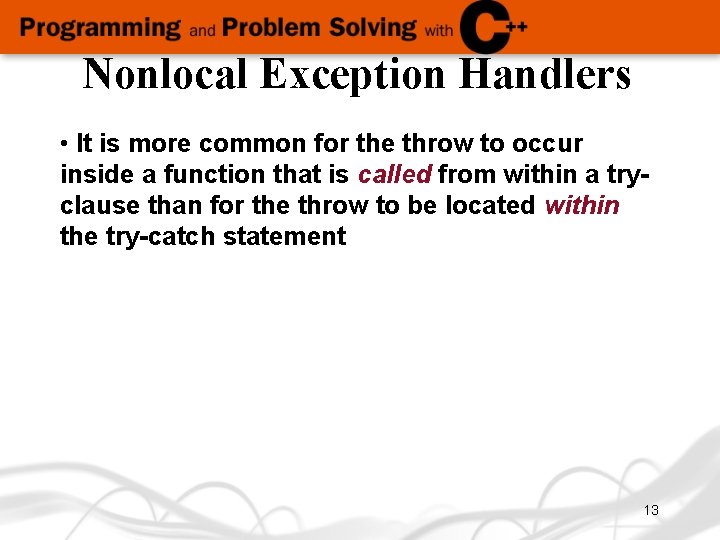
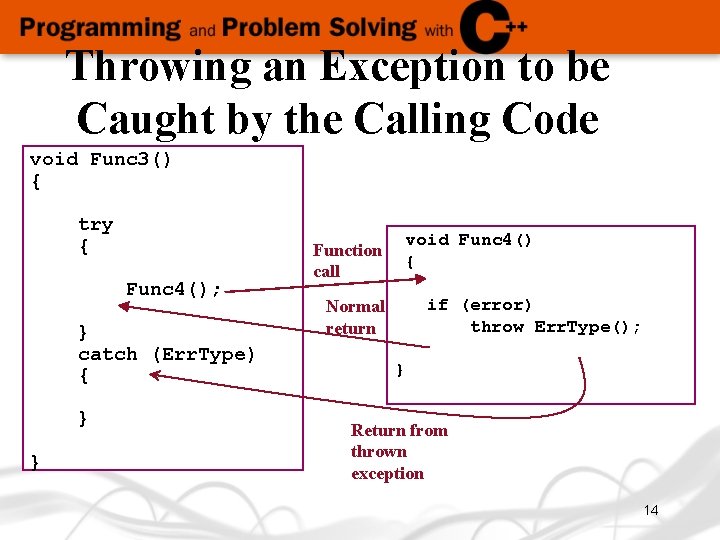
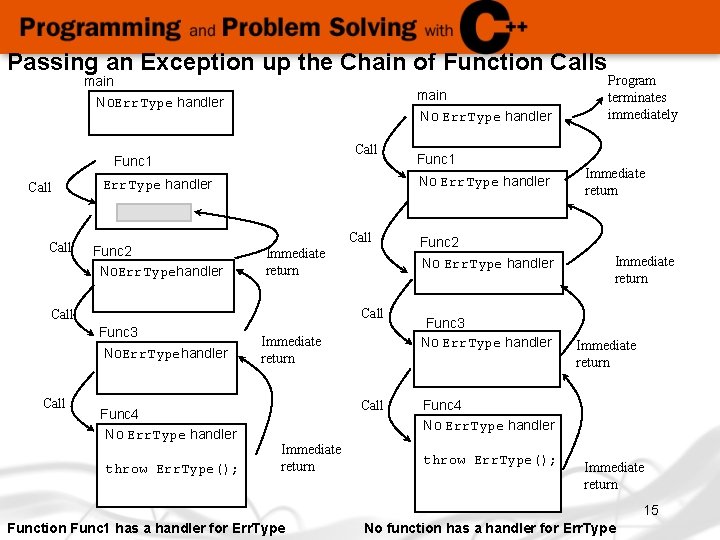
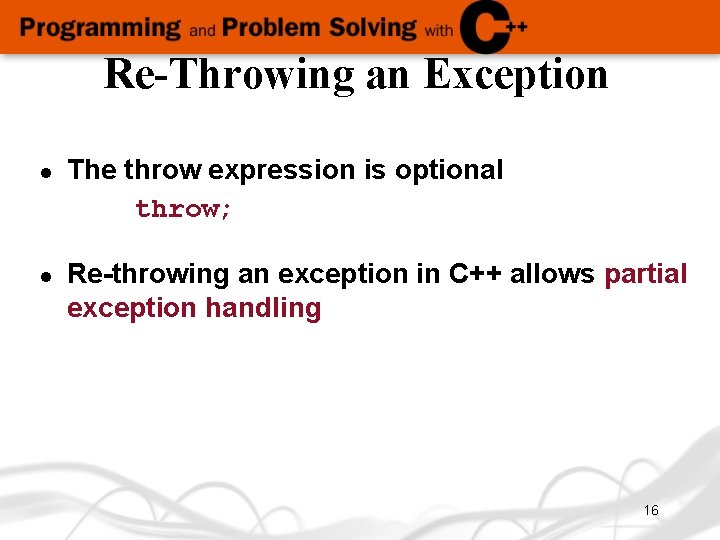
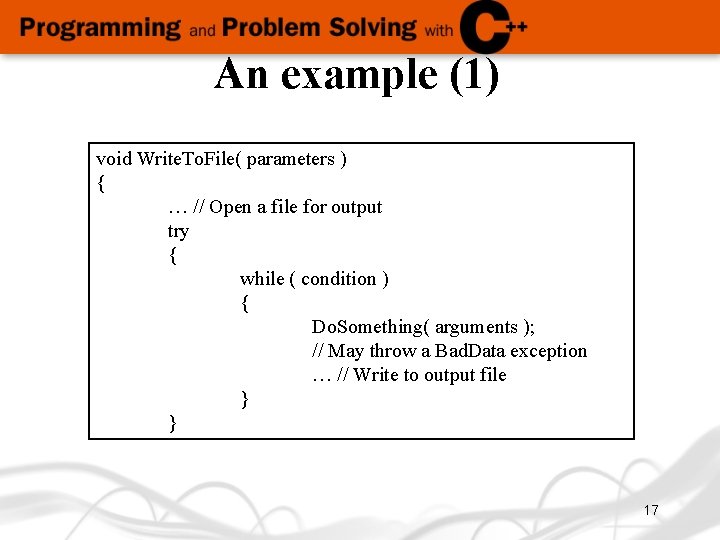
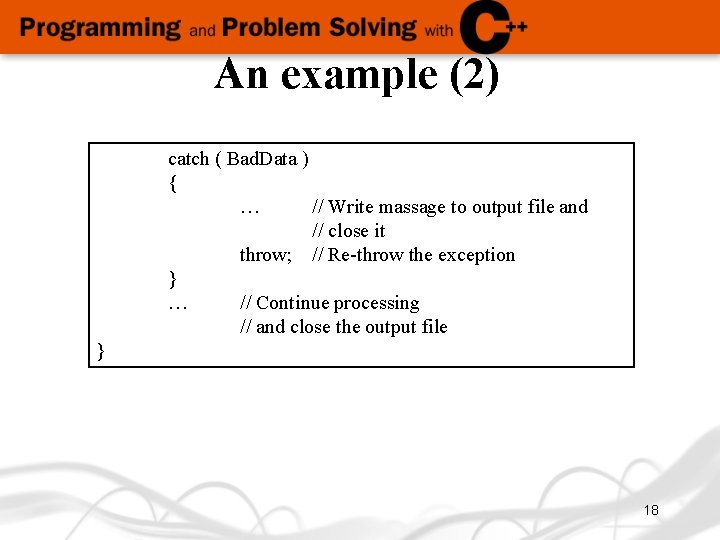
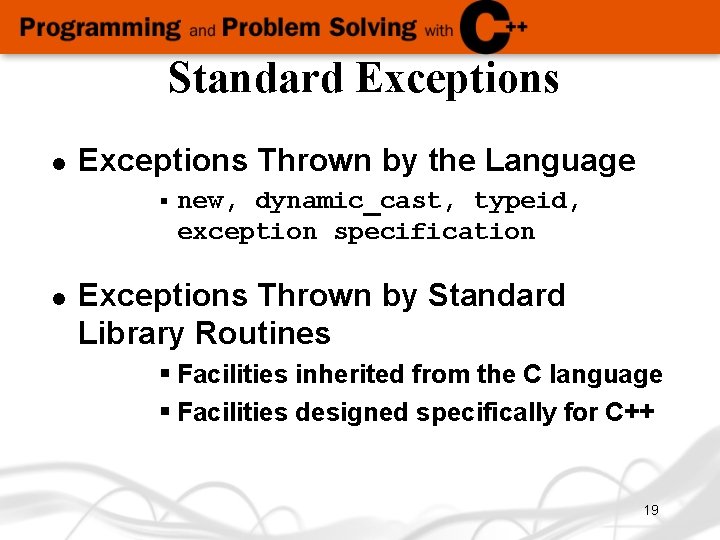
![Exception thrown by new float* arr; try { arr = new float[50000]; } catch Exception thrown by new float* arr; try { arr = new float[50000]; } catch](https://slidetodoc.com/presentation_image/bb8a0fe88f7fbd7f8f5ad54e22bce0fd/image-20.jpg)
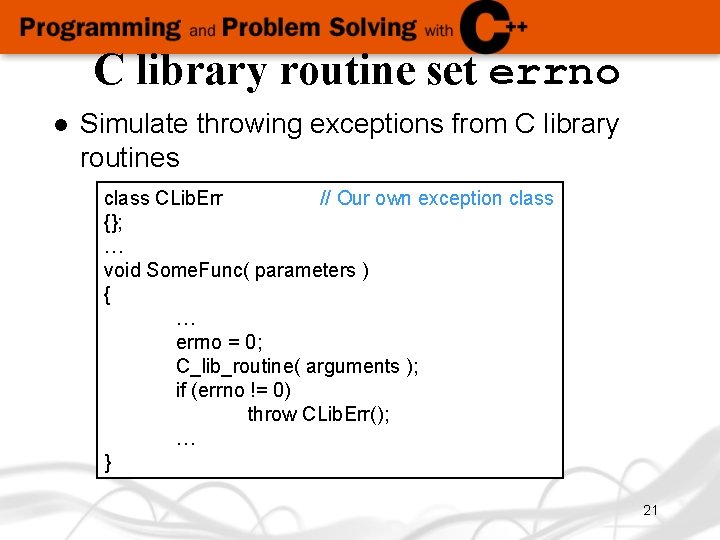
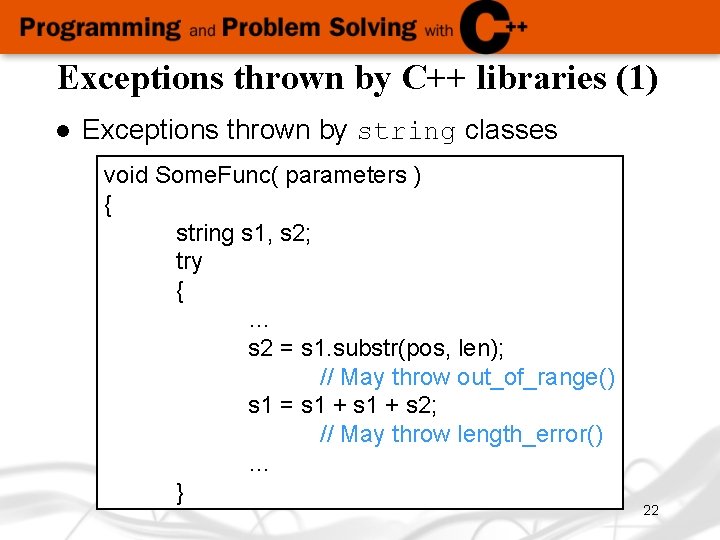
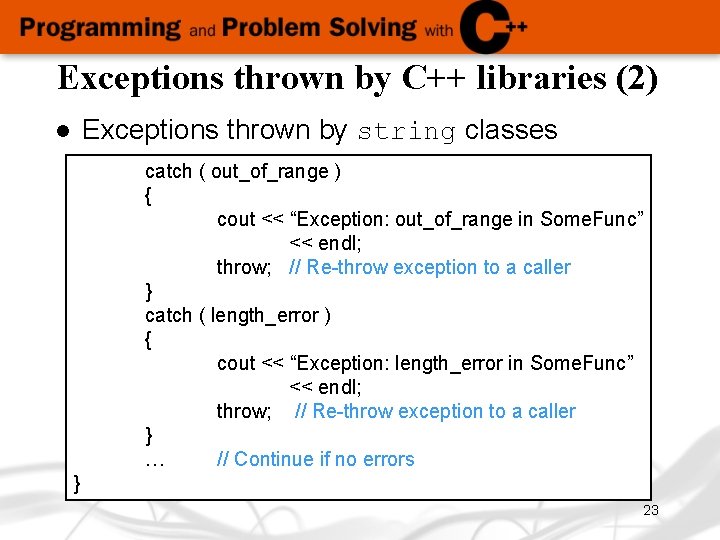
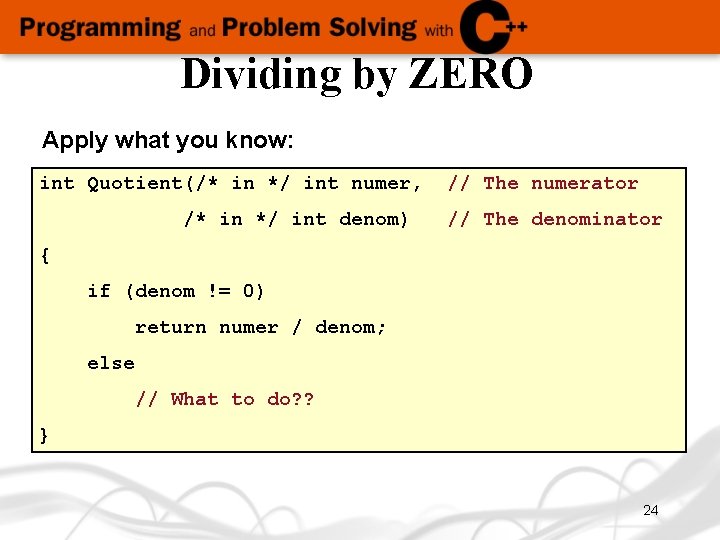
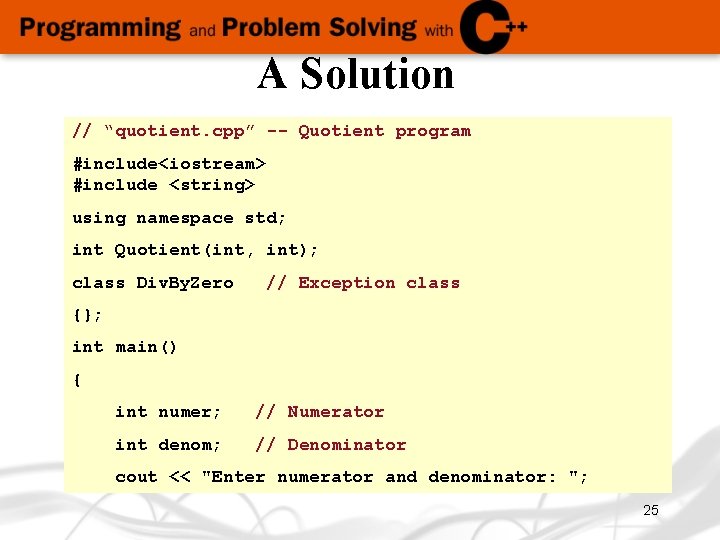
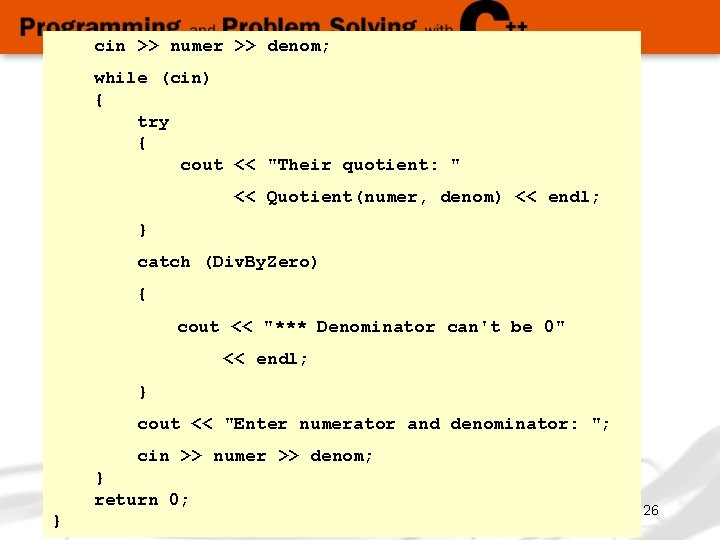
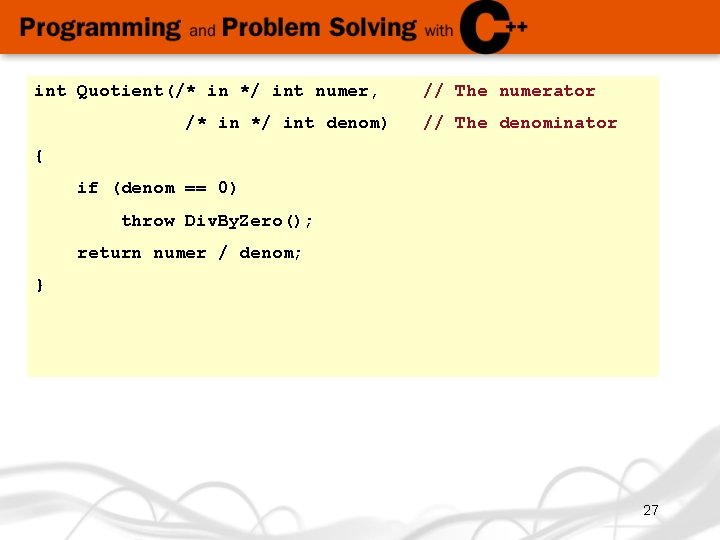
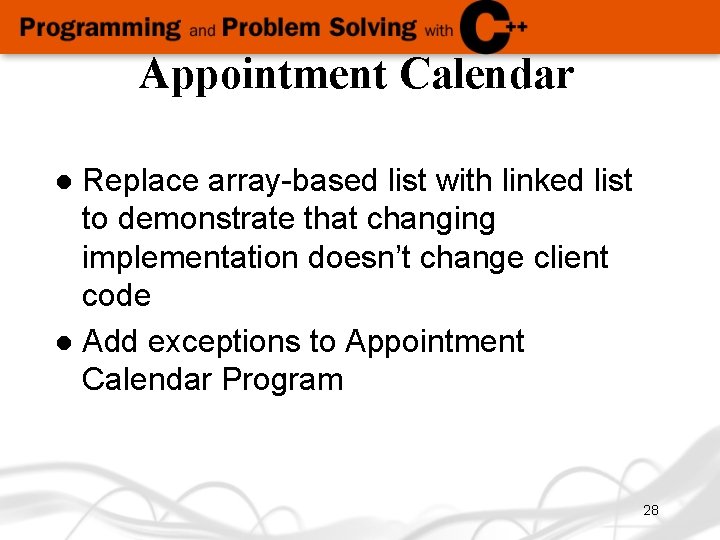
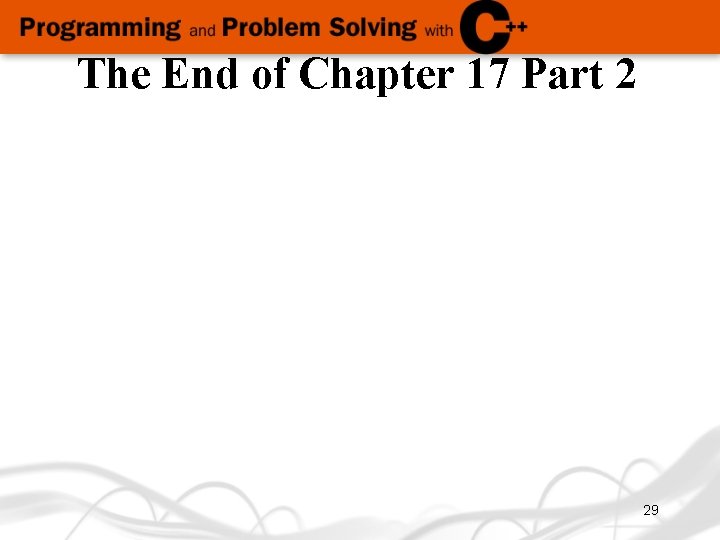
- Slides: 29
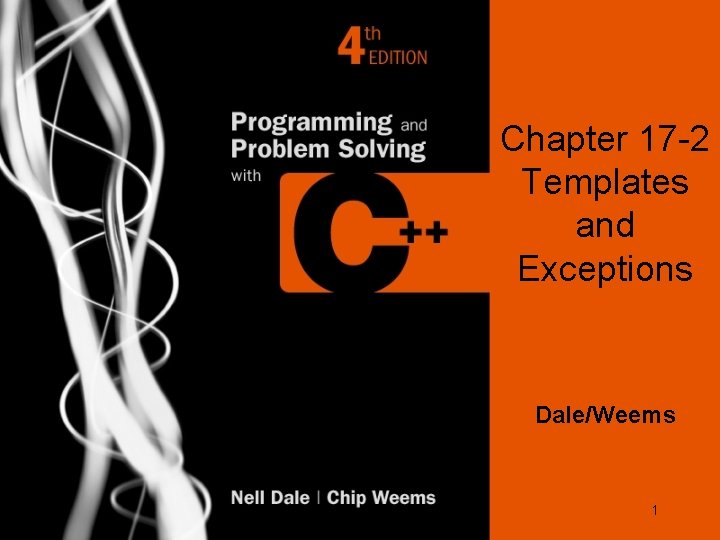
Chapter 17 -2 Templates and Exceptions Dale/Weems 1
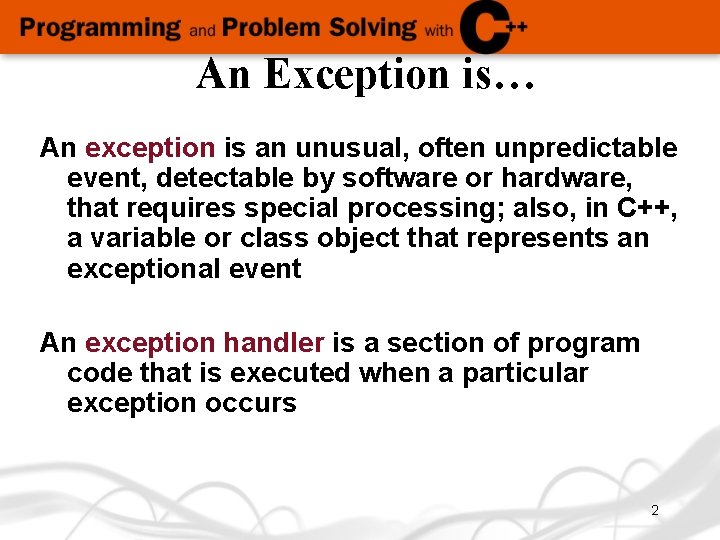
An Exception is… An exception is an unusual, often unpredictable event, detectable by software or hardware, that requires special processing; also, in C++, a variable or class object that represents an exceptional event An exception handler is a section of program code that is executed when a particular exception occurs 2
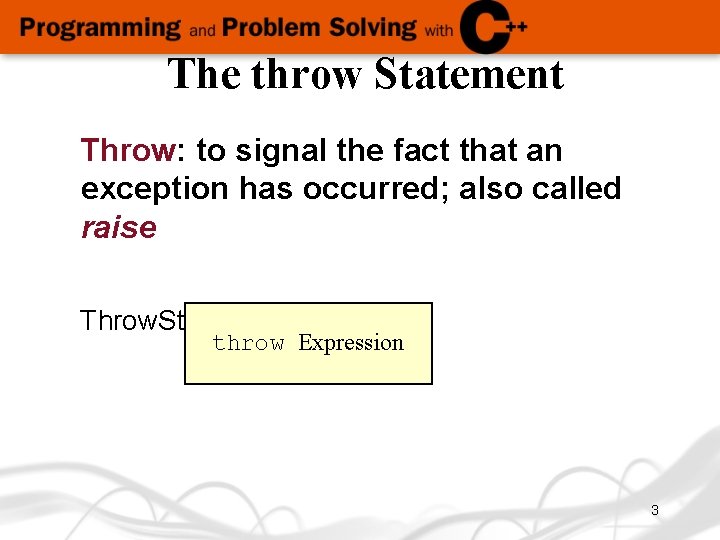
The throw Statement Throw: to signal the fact that an exception has occurred; also called raise Throw. Statement throw Expression 3
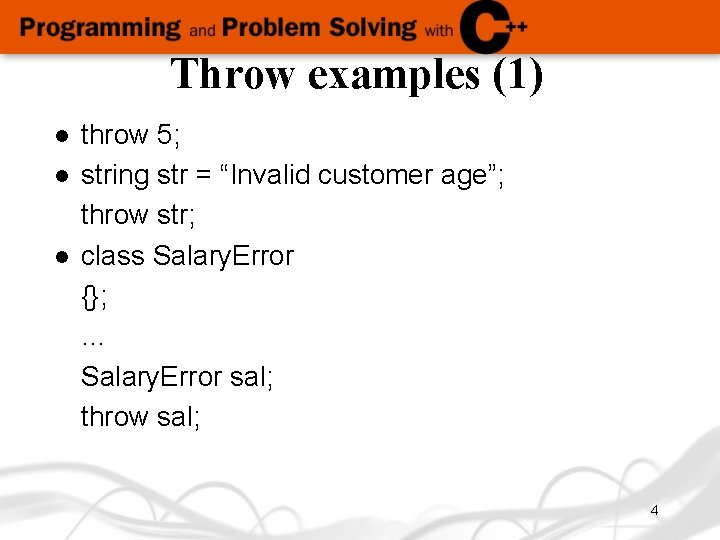
Throw examples (1) l l l throw 5; string str = “Invalid customer age”; throw str; class Salary. Error {}; … Salary. Error sal; throw sal; 4
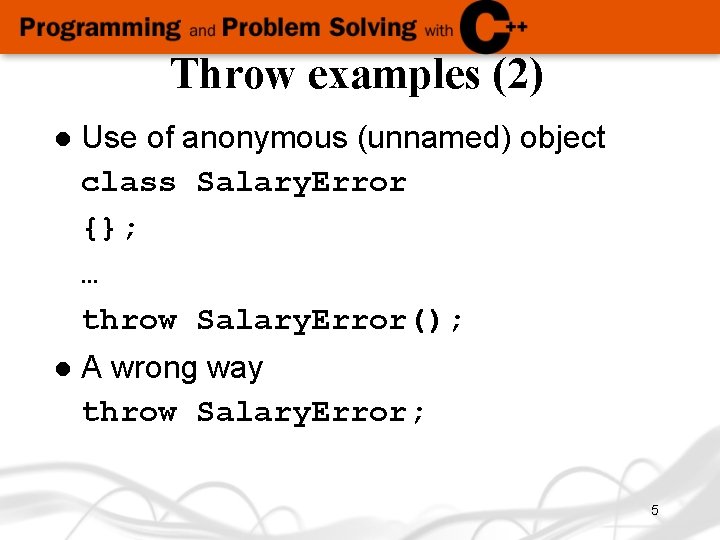
Throw examples (2) l Use of anonymous (unnamed) object class Salary. Error {}; … throw Salary. Error(); l A wrong way throw Salary. Error; 5
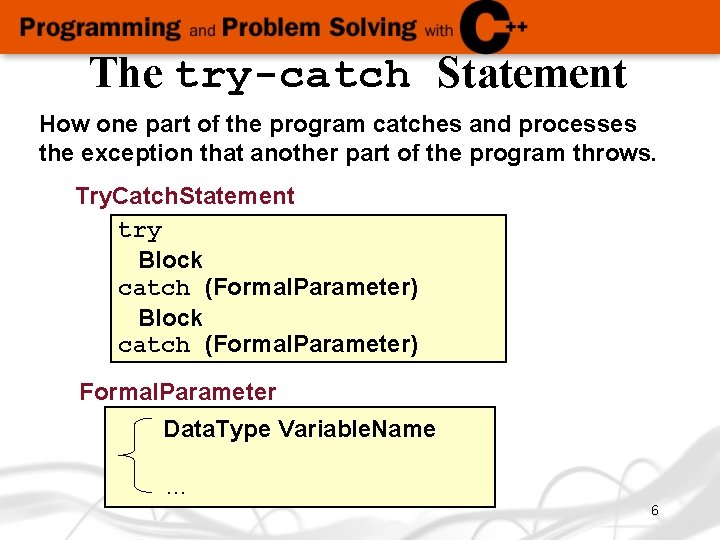
The try-catch Statement How one part of the program catches and processes the exception that another part of the program throws. Try. Catch. Statement try Block catch (Formal. Parameter) Formal. Parameter Data. Type Variable. Name … 6
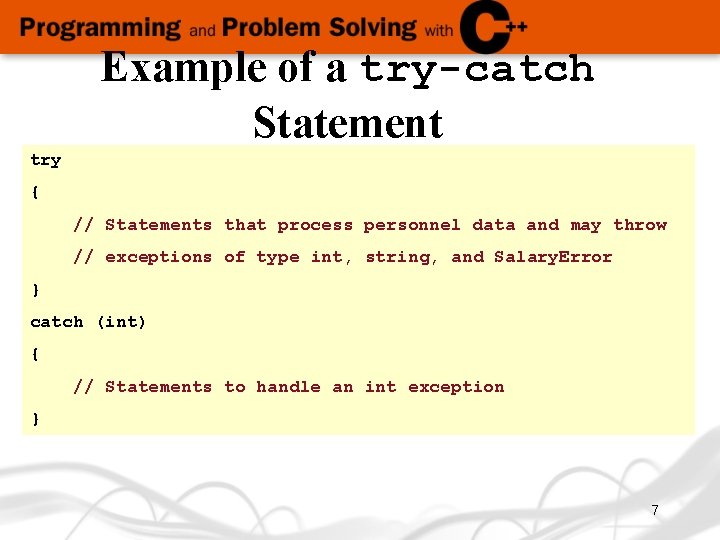
Example of a try-catch Statement try { // Statements that process personnel data and may throw // exceptions of type int, string, and Salary. Error } catch (int) { // Statements to handle an int exception } 7
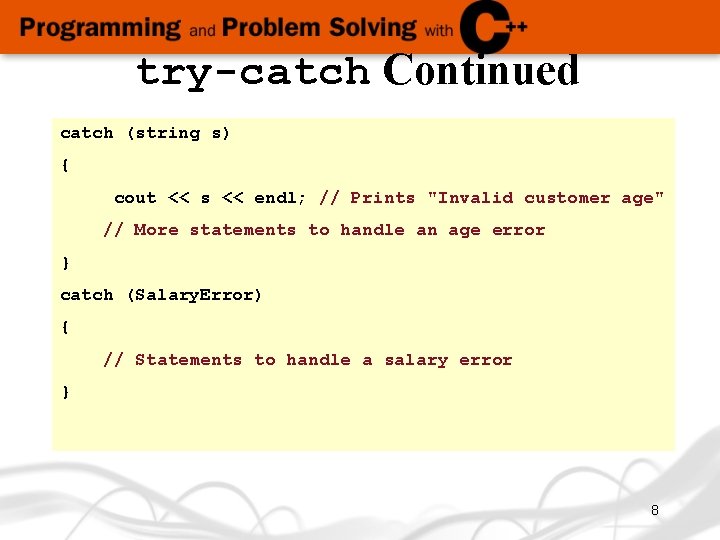
try-catch Continued catch (string s) { cout << s << endl; // Prints "Invalid customer age" // More statements to handle an age error } catch (Salary. Error) { // Statements to handle a salary error } 8
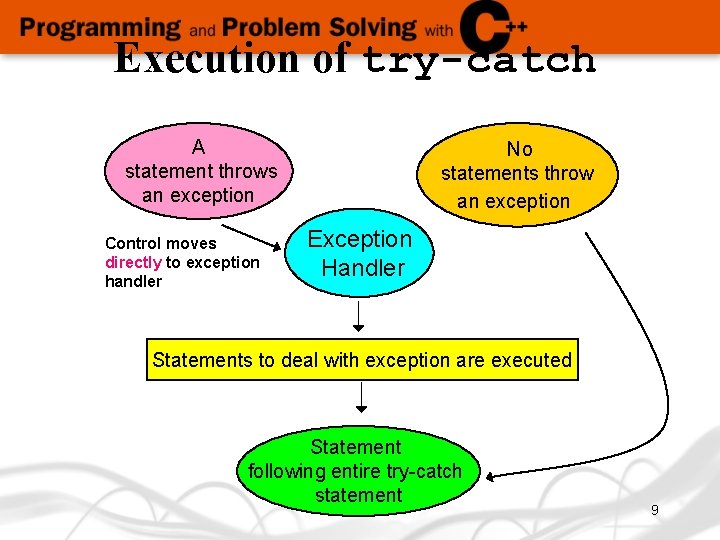
Execution of try-catch A statement throws an exception Control moves directly to exception handler No statements throw an exception Exception Handler Statements to deal with exception are executed Statement following entire try-catch statement 9
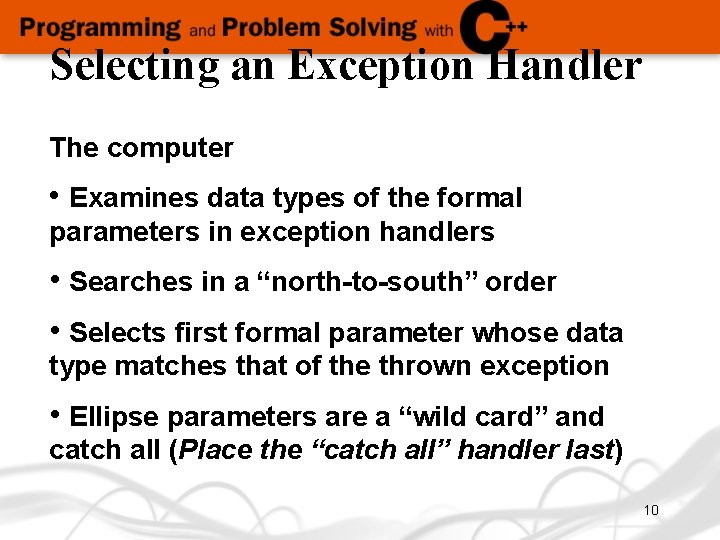
Selecting an Exception Handler The computer • Examines data types of the formal parameters in exception handlers • Searches in a “north-to-south” order • Selects first formal parameter whose data type matches that of the thrown exception • Ellipse parameters are a “wild card” and catch all (Place the “catch all” handler last) 10
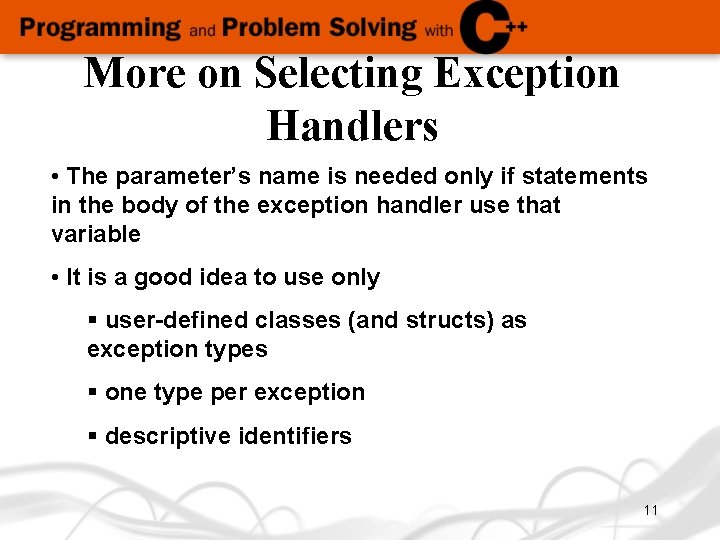
More on Selecting Exception Handlers • The parameter’s name is needed only if statements in the body of the exception handler use that variable • It is a good idea to use only § user-defined classes (and structs) as exception types § one type per exception § descriptive identifiers 11
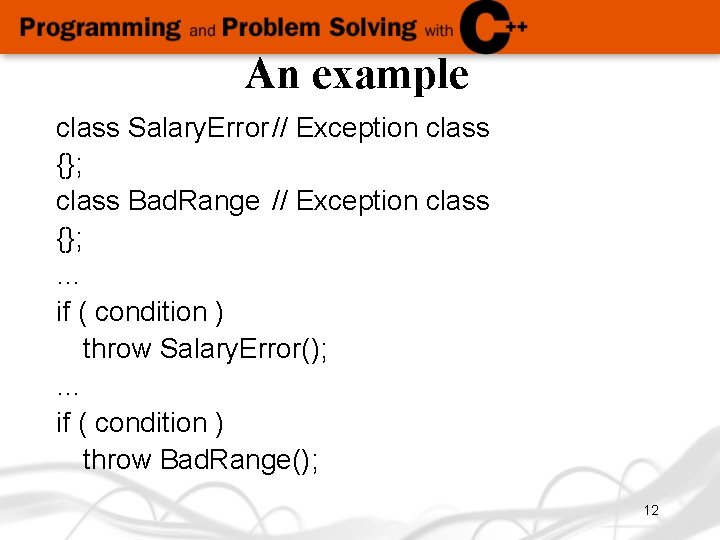
An example class Salary. Error // Exception class {}; class Bad. Range // Exception class {}; … if ( condition ) throw Salary. Error(); … if ( condition ) throw Bad. Range(); 12
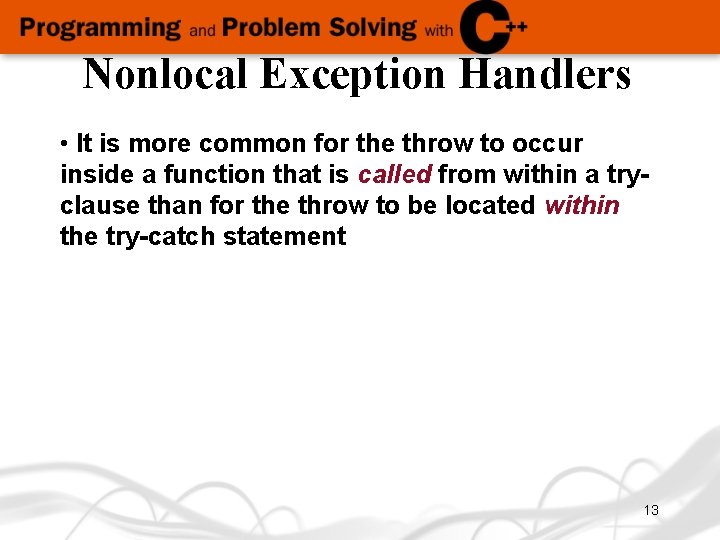
Nonlocal Exception Handlers • It is more common for the throw to occur inside a function that is called from within a tryclause than for the throw to be located within the try-catch statement 13
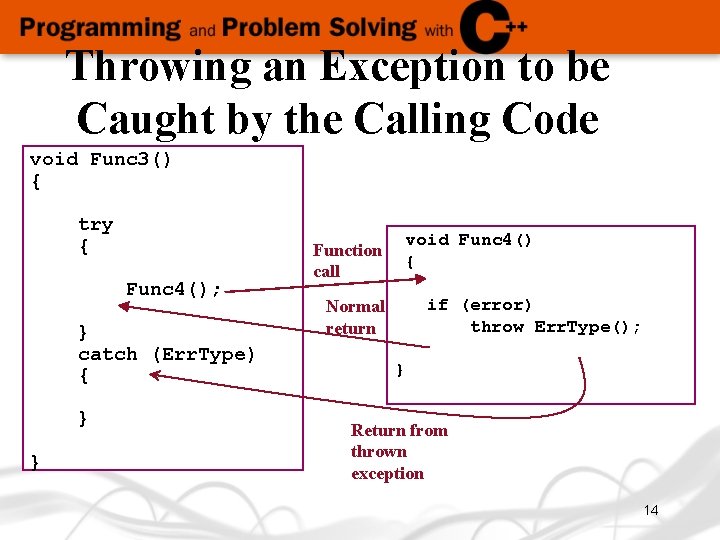
Throwing an Exception to be Caught by the Calling Code void Func 3() { try { Func 4(); } catch (Err. Type) { } } void Func 4() { Function call if (error) throw Err. Type(); Normal return } Return from thrown exception 14
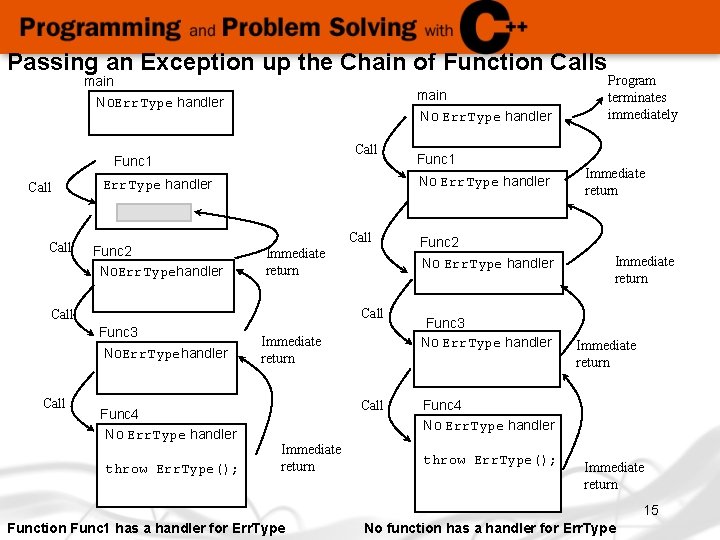
Passing an Exception up the Chain of Function Calls main No. Err. Type handler main No Err. Type handler Call Func 1 Call No Err. Type handler Func 2 No. Err. Typehandler Call Immediate return Call Func 4 No Err. Type handler throw Err. Type(); Immediate return Call Immediate return Func 2 No Err. Type handler Call Func 3 No. Err. Typehandler Func 1 Program terminates immediately Func 3 No Err. Type handler Immediate return Func 4 No Err. Type handler throw Err. Type(); Immediate return 15 Function Func 1 has a handler for Err. Type No function has a handler for Err. Type
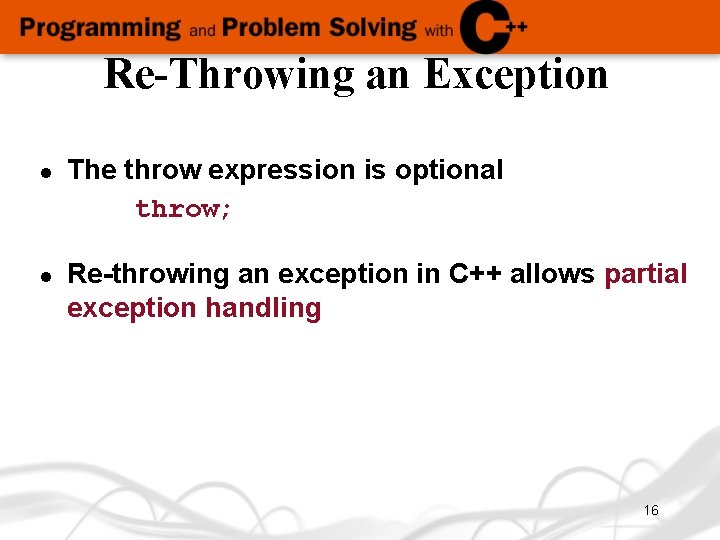
Re-Throwing an Exception l l The throw expression is optional throw; Re-throwing an exception in C++ allows partial exception handling 16
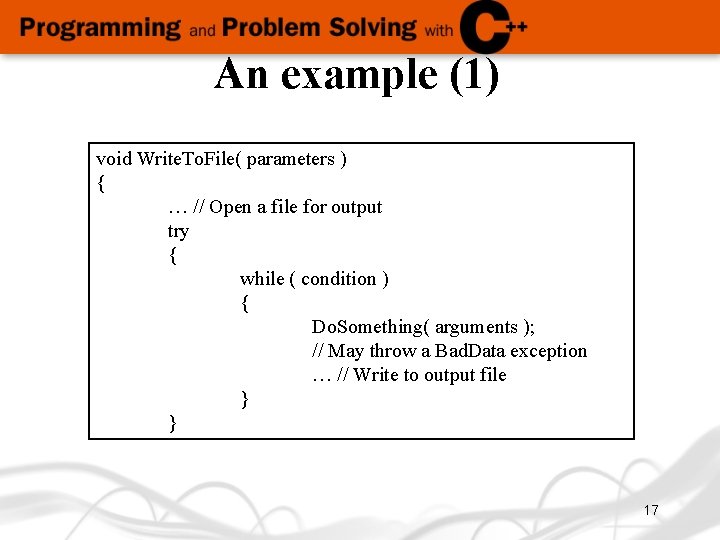
An example (1) void Write. To. File( parameters ) { … // Open a file for output try { while ( condition ) { Do. Something( arguments ); // May throw a Bad. Data exception … // Write to output file } } 17
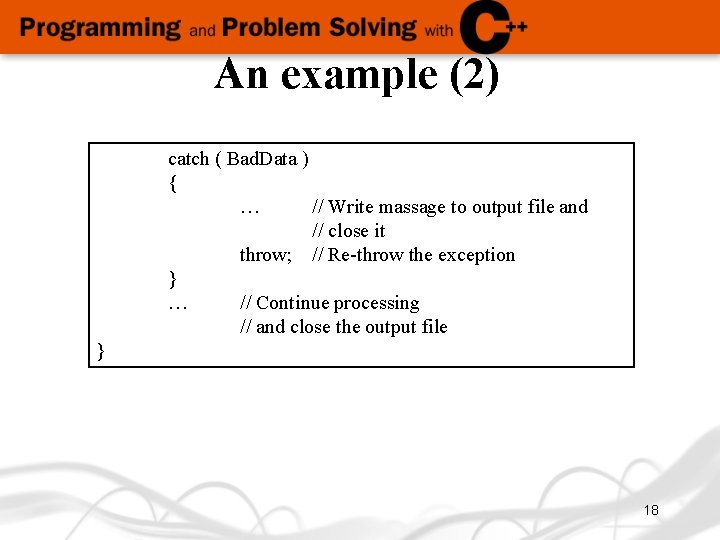
An example (2) catch ( Bad. Data ) { … // Write massage to output file and // close it throw; // Re-throw the exception } … // Continue processing // and close the output file } 18
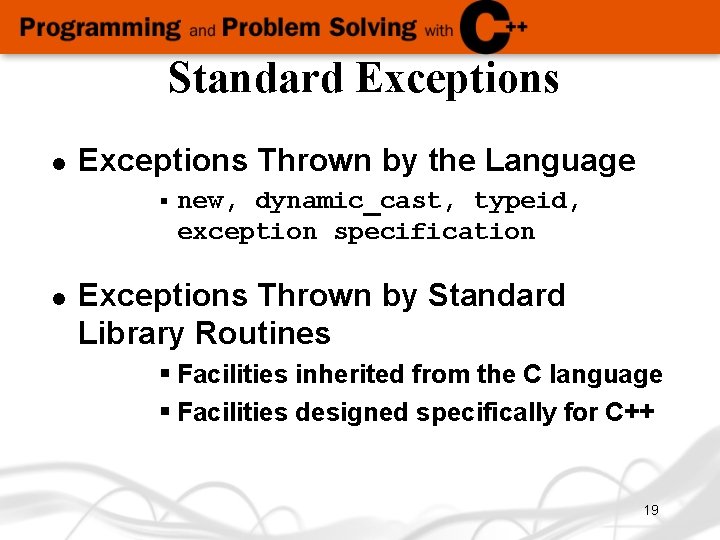
Standard Exceptions l Exceptions Thrown by the Language § l new, dynamic_cast, typeid, exception specification Exceptions Thrown by Standard Library Routines § Facilities inherited from the C language § Facilities designed specifically for C++ 19
![Exception thrown by new float arr try arr new float50000 catch Exception thrown by new float* arr; try { arr = new float[50000]; } catch](https://slidetodoc.com/presentation_image/bb8a0fe88f7fbd7f8f5ad54e22bce0fd/image-20.jpg)
Exception thrown by new float* arr; try { arr = new float[50000]; } catch ( bad_alloc ) { cout << “*** Out of memory. Can’t allocate array. ” << endl; return 1; // Terminate the program } … // Continue. Allocation succeeded 20
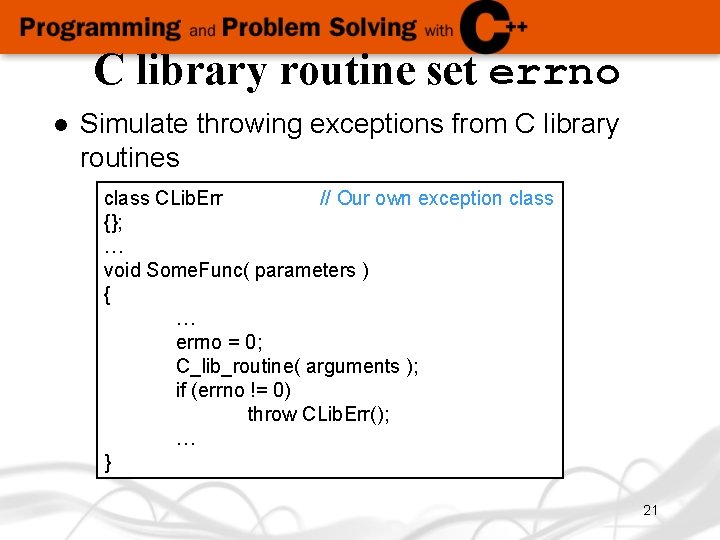
C library routine set errno l Simulate throwing exceptions from C library routines class CLib. Err // Our own exception class {}; … void Some. Func( parameters ) { … errno = 0; C_lib_routine( arguments ); if (errno != 0) throw CLib. Err(); … } 21
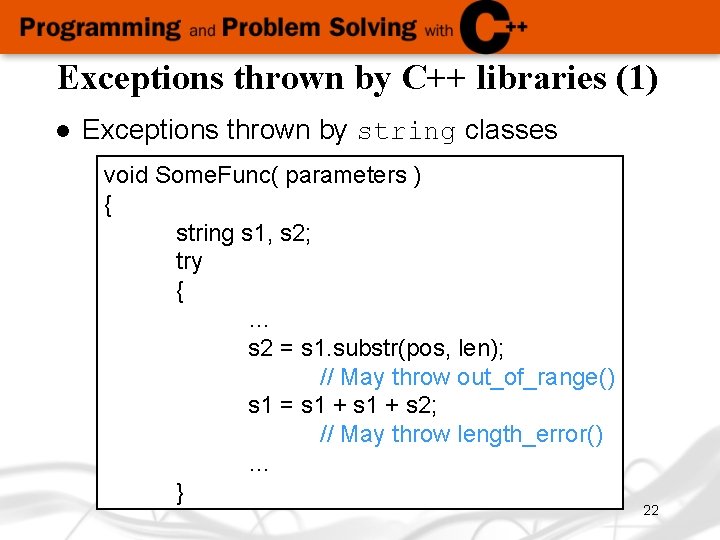
Exceptions thrown by C++ libraries (1) l Exceptions thrown by string classes void Some. Func( parameters ) { string s 1, s 2; try { … s 2 = s 1. substr(pos, len); // May throw out_of_range() s 1 = s 1 + s 2; // May throw length_error() … } 22
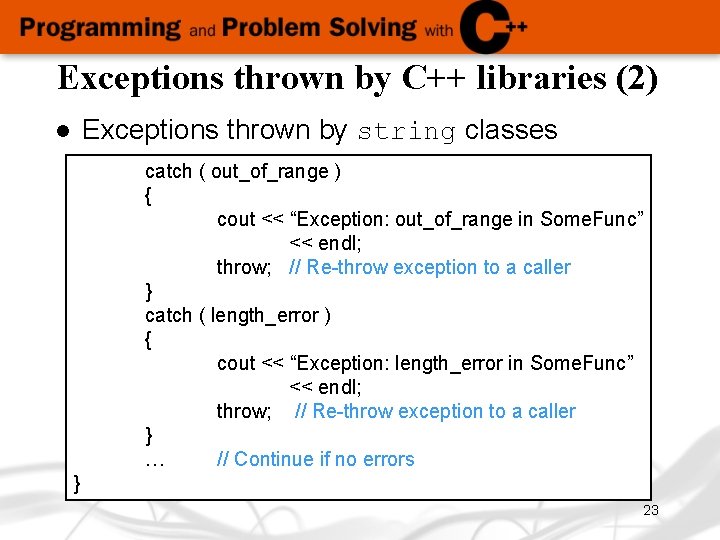
Exceptions thrown by C++ libraries (2) Exceptions thrown by string classes l catch ( out_of_range ) { cout << “Exception: out_of_range in Some. Func” << endl; throw; // Re-throw exception to a caller } catch ( length_error ) { cout << “Exception: length_error in Some. Func” << endl; throw; // Re-throw exception to a caller } … // Continue if no errors } 23
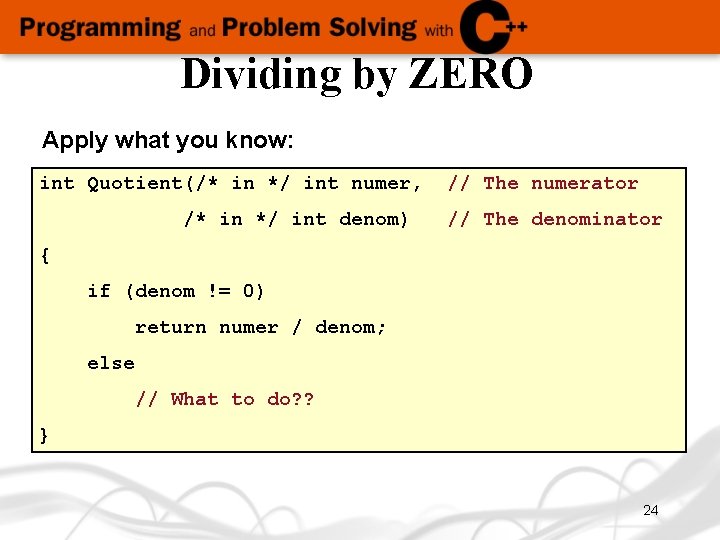
Dividing by ZERO Apply what you know: int Quotient(/* in */ int numer, /* in */ int denom) // The numerator // The denominator { if (denom != 0) return numer / denom; else // What to do? ? } 24
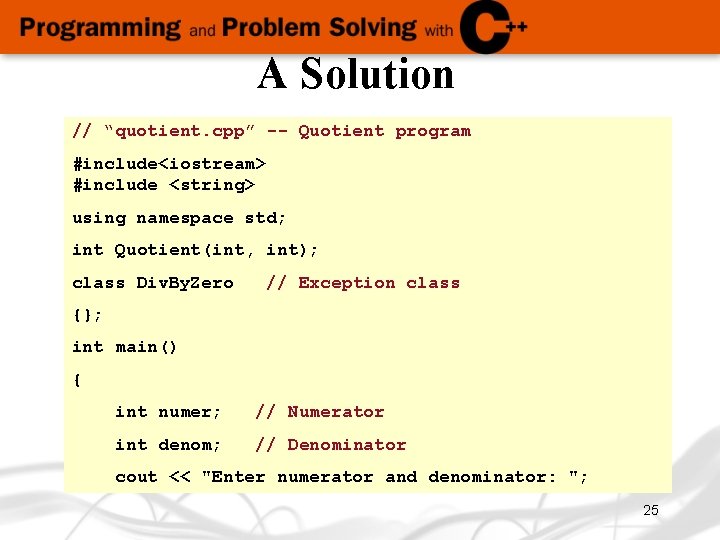
A Solution // “quotient. cpp” -- Quotient program #include<iostream> #include <string> using namespace std; int Quotient(int, int); class Div. By. Zero // Exception class {}; int main() { int numer; // Numerator int denom; // Denominator cout << "Enter numerator and denominator: "; 25
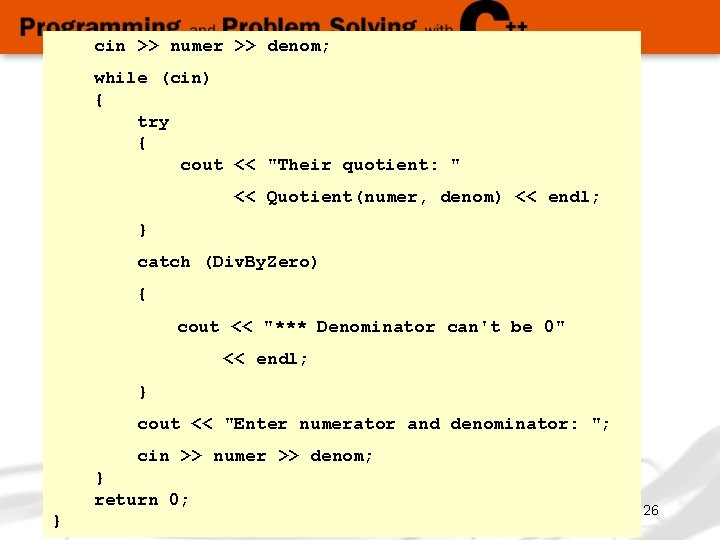
cin >> numer >> denom; while (cin) { try { cout << "Their quotient: " << Quotient(numer, denom) << endl; } catch (Div. By. Zero) { cout << "*** Denominator can't be 0" << endl; } cout << "Enter numerator and denominator: "; cin >> numer >> denom; } return 0; } 26
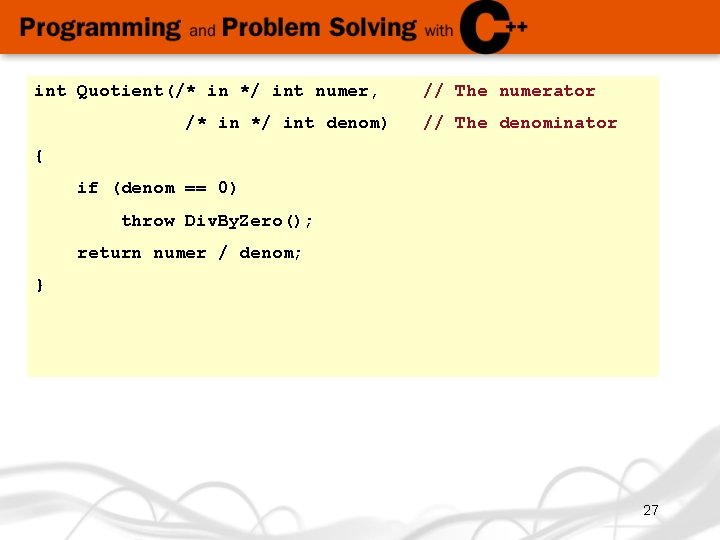
int Quotient(/* in */ int numer, /* in */ int denom) // The numerator // The denominator { if (denom == 0) throw Div. By. Zero(); return numer / denom; } 27
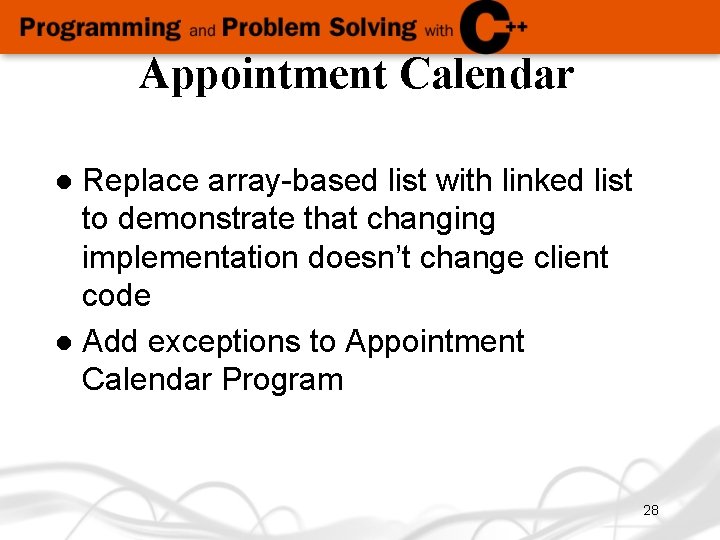
Appointment Calendar Replace array-based list with linked list to demonstrate that changing implementation doesn’t change client code l Add exceptions to Appointment Calendar Program l 28
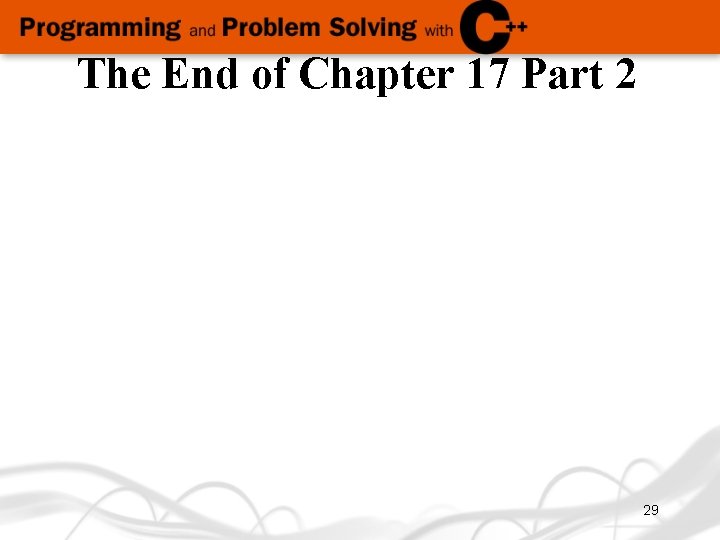
The End of Chapter 17 Part 2 29
Thoughtful comparative and superlative
Non predefined exceptions in oracle
Badly comparative superlative
Comparatives and superlatives exceptions
Spl exceptions
Robust programs
Stark law exceptions
Present continuous questions
Php exception handling
Coccus
Koch's postulates exceptions
Scope of microbiology
Stark law exceptions
What is first ionization energy
Octet exceptions
What is microbiology
It rolls downhill
Multiple alleles
Different types of exceptions in c++
Java exceptions list
Question tag short form
Paragraph in present continuous tense
Invoice exceptions
Hybridization exceptions
Pad see ew pronunciation
Imparfait exceptions
Imparfait exceptions
Exception to mendel's laws
Cvc rule
Berry compliance requirements