Arrays and Strings CSCI 392 Week Three Declaring
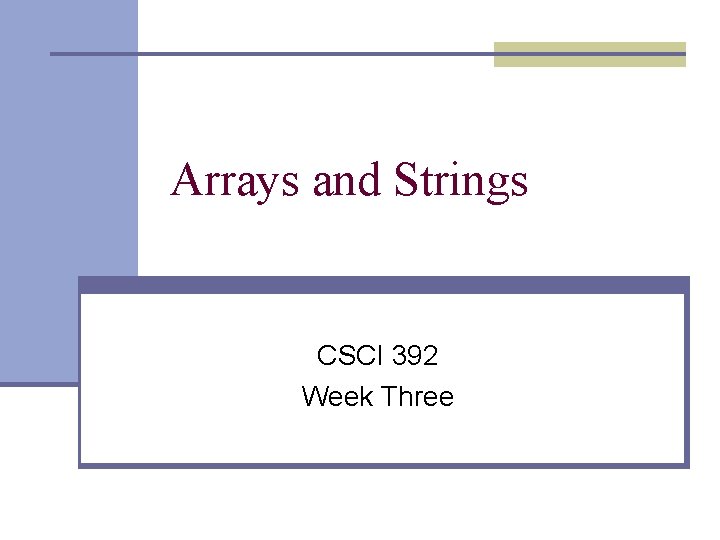
![Declaring an Array Variable Here are two version of the same declaration int[] intlist; Declaring an Array Variable Here are two version of the same declaration int[] intlist;](https://slidetodoc.com/presentation_image_h2/5be5fe29c5e7689d1c617ea2bf342a31/image-2.jpg)
![Creating Space for an Array Given the declaration int[] myarray; You must also tell Creating Space for an Array Given the declaration int[] myarray; You must also tell](https://slidetodoc.com/presentation_image_h2/5be5fe29c5e7689d1c617ea2bf342a31/image-3.jpg)
![Initializing Array Values Version One int[] one_to_four = new int[] {1, 2, 3, 4}; Initializing Array Values Version One int[] one_to_four = new int[] {1, 2, 3, 4};](https://slidetodoc.com/presentation_image_h2/5be5fe29c5e7689d1c617ea2bf342a31/image-4.jpg)
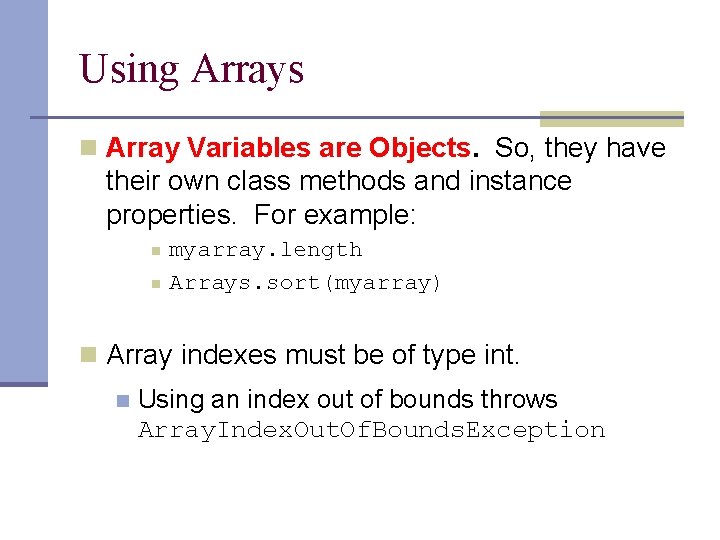
![Copying Arrays n Shallow Copy int[] original = {1, 2, 3, 4} int[] copy Copying Arrays n Shallow Copy int[] original = {1, 2, 3, 4} int[] copy](https://slidetodoc.com/presentation_image_h2/5be5fe29c5e7689d1c617ea2bf342a31/image-6.jpg)
![class array_test_1 { static void main(String[] args) { char[] msg = {'B', 'o', 'b'}; class array_test_1 { static void main(String[] args) { char[] msg = {'B', 'o', 'b'};](https://slidetodoc.com/presentation_image_h2/5be5fe29c5e7689d1c617ea2bf342a31/image-7.jpg)
![class array_test_2 { static void main(String[] args) { char[] msg = {'B', 'o', 'b'}; class array_test_2 { static void main(String[] args) { char[] msg = {'B', 'o', 'b'};](https://slidetodoc.com/presentation_image_h2/5be5fe29c5e7689d1c617ea2bf342a31/image-8.jpg)
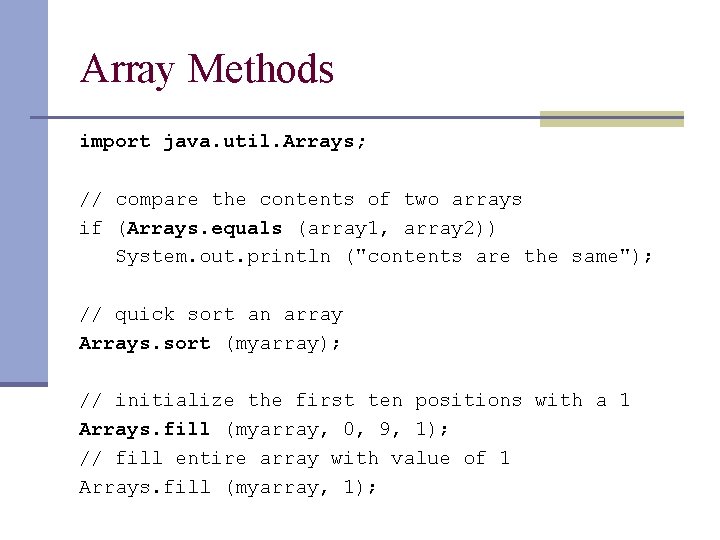
![char[] != String The "+" operator is overloaded for the String class, so this char[] != String The "+" operator is overloaded for the String class, so this](https://slidetodoc.com/presentation_image_h2/5be5fe29c5e7689d1c617ea2bf342a31/image-10.jpg)
![char[] != String This code yields a compiler error. class string_test_2 { static void char[] != String This code yields a compiler error. class string_test_2 { static void](https://slidetodoc.com/presentation_image_h2/5be5fe29c5e7689d1c617ea2bf342a31/image-11.jpg)
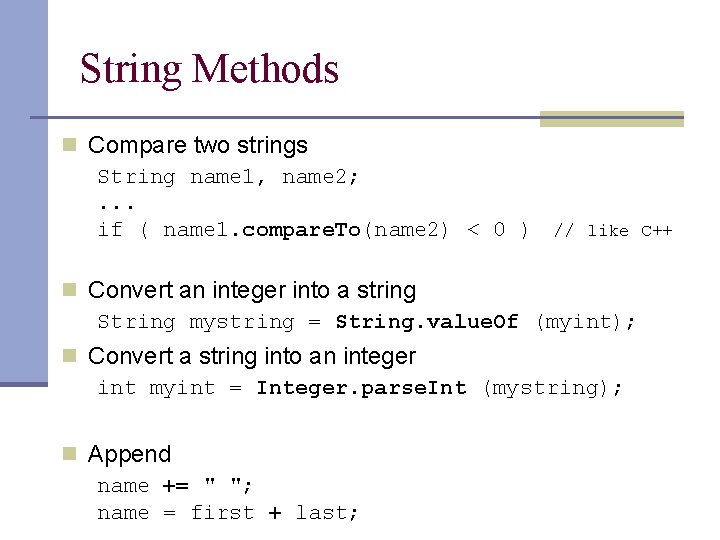
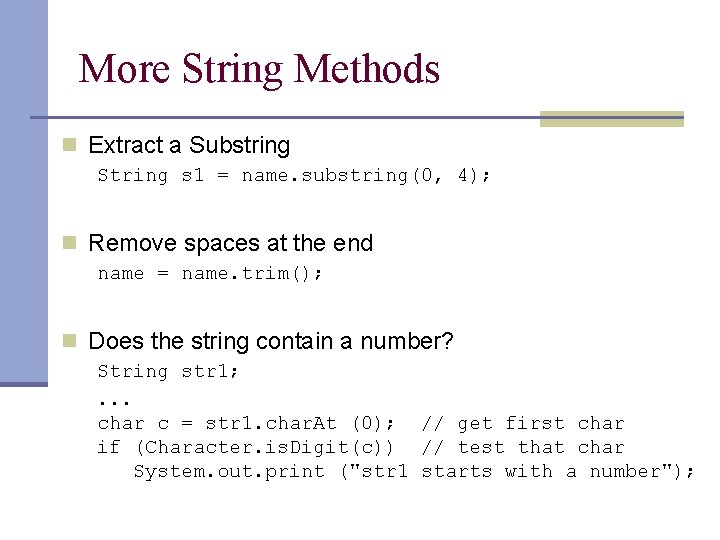
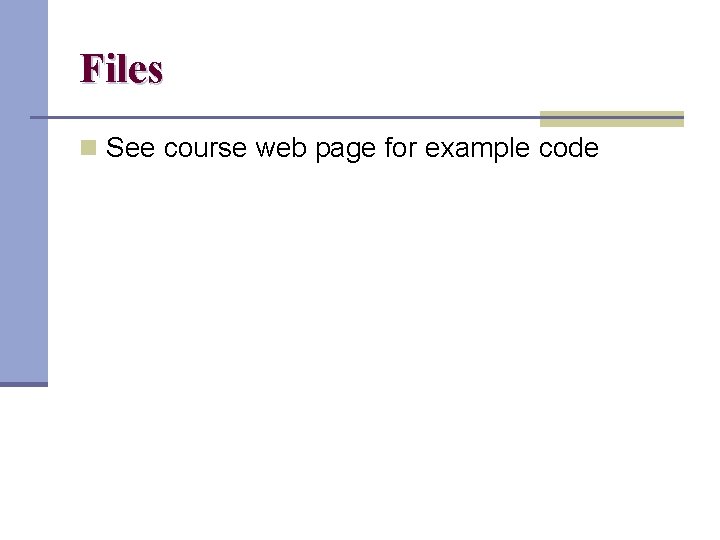
- Slides: 14
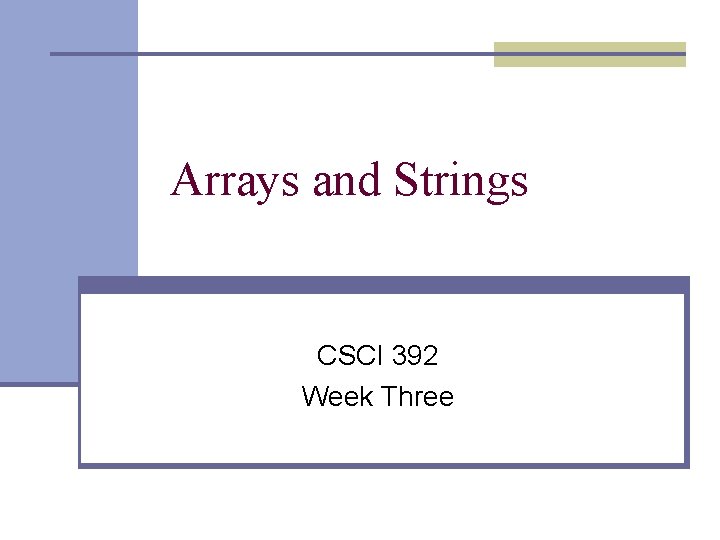
Arrays and Strings CSCI 392 Week Three
![Declaring an Array Variable Here are two version of the same declaration int intlist Declaring an Array Variable Here are two version of the same declaration int[] intlist;](https://slidetodoc.com/presentation_image_h2/5be5fe29c5e7689d1c617ea2bf342a31/image-2.jpg)
Declaring an Array Variable Here are two version of the same declaration int[] intlist; intlist[]; Declaring a two-dimensional array int[][] twodimarray; int twodimarray[][];
![Creating Space for an Array Given the declaration int myarray You must also tell Creating Space for an Array Given the declaration int[] myarray; You must also tell](https://slidetodoc.com/presentation_image_h2/5be5fe29c5e7689d1c617ea2bf342a31/image-3.jpg)
Creating Space for an Array Given the declaration int[] myarray; You must also tell the VM to create space myarray = new int[100]; You can do both steps in one line int[] myarray = new int[100]; You can also wait until runtime to decide how much space to use int[] myarray = new int[compute. Size()];
![Initializing Array Values Version One int onetofour new int 1 2 3 4 Initializing Array Values Version One int[] one_to_four = new int[] {1, 2, 3, 4};](https://slidetodoc.com/presentation_image_h2/5be5fe29c5e7689d1c617ea2bf342a31/image-4.jpg)
Initializing Array Values Version One int[] one_to_four = new int[] {1, 2, 3, 4}; Version Two int[] one_to_four = {1, 2, 3, 4}; An Array of Strings String[] names = {"Bob", "Joe", "Sue", "Fred"}; Computing Initial Values at Runtime int[] examscores = {grade. Exam(1), grade. Exam(2)};
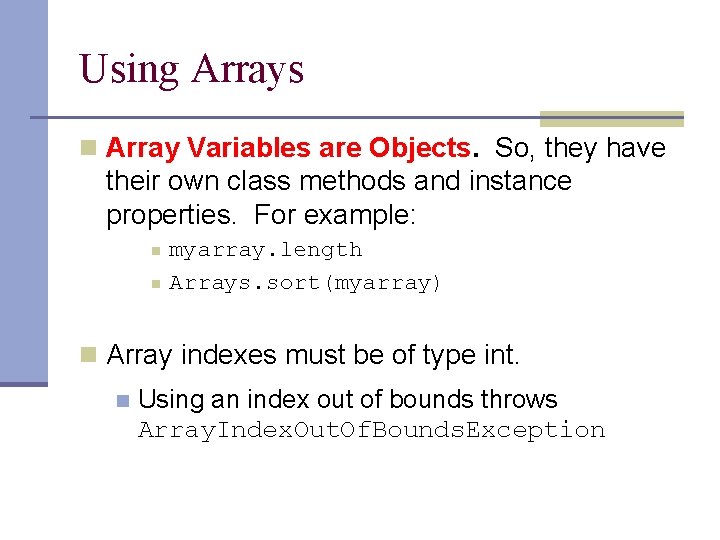
Using Arrays n Array Variables are Objects. So, they have their own class methods and instance properties. For example: n n myarray. length Arrays. sort(myarray) n Array indexes must be of type int. n Using an index out of bounds throws Array. Index. Out. Of. Bounds. Exception
![Copying Arrays n Shallow Copy int original 1 2 3 4 int copy Copying Arrays n Shallow Copy int[] original = {1, 2, 3, 4} int[] copy](https://slidetodoc.com/presentation_image_h2/5be5fe29c5e7689d1c617ea2bf342a31/image-6.jpg)
Copying Arrays n Shallow Copy int[] original = {1, 2, 3, 4} int[] copy = (int[]) original. clone(); n Better copy method System. arraycopy (original, 0, copy, 0, original. length);
![class arraytest1 static void mainString args char msg B o b class array_test_1 { static void main(String[] args) { char[] msg = {'B', 'o', 'b'};](https://slidetodoc.com/presentation_image_h2/5be5fe29c5e7689d1c617ea2bf342a31/image-7.jpg)
class array_test_1 { static void main(String[] args) { char[] msg = {'B', 'o', 'b'}; char[] copy 1 = msg; char[] copy 2 = (char[]) msg. clone(); copy 1[0] = 'b'; copy 2[2] = 'p'; System. out. print ("msg = "); System. out. println(msg); System. out. print ("copy 1 = "); System. out. println(copy 1); System. out. print ("copy 2 = "); System. out. println(copy 2); } } msg = bob copy 1 = bob copy 2 = Bop
![class arraytest2 static void mainString args char msg B o b class array_test_2 { static void main(String[] args) { char[] msg = {'B', 'o', 'b'};](https://slidetodoc.com/presentation_image_h2/5be5fe29c5e7689d1c617ea2bf342a31/image-8.jpg)
class array_test_2 { static void main(String[] args) { char[] msg = {'B', 'o', 'b'}; char[] copy 1 = msg; char[] copy 2 = (char[]) msg. clone(); if (msg == copy 1) System. out. println ("msg equals copy 1"); else System. out. println ("msg not equals copy 1"); if (copy 1 == copy 2) System. out. println ("copy 1 equals copy 2"); else System. out. println ("copy 1 not equals copy 2"); } } msg equals copy 1 not equals copy 2
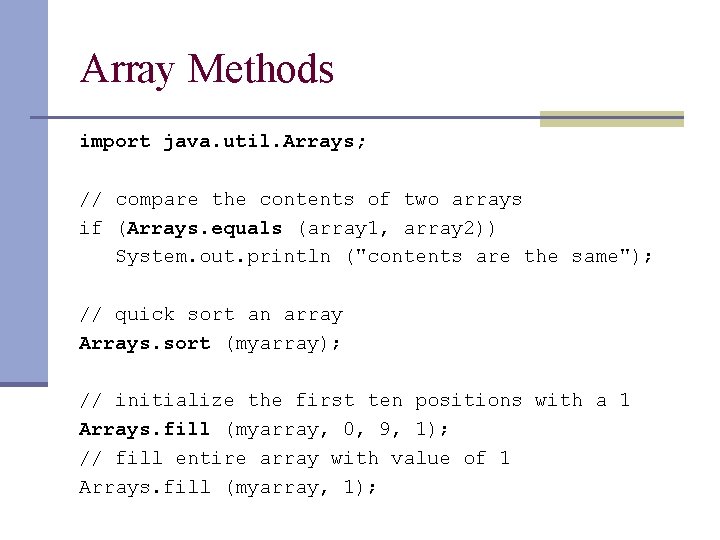
Array Methods import java. util. Arrays; // compare the contents of two arrays if (Arrays. equals (array 1, array 2)) System. out. println ("contents are the same"); // quick sort an array Arrays. sort (myarray); // initialize the first ten positions with a 1 Arrays. fill (myarray, 0, 9, 1); // fill entire array with value of 1 Arrays. fill (myarray, 1);
![char String The operator is overloaded for the String class so this char[] != String The "+" operator is overloaded for the String class, so this](https://slidetodoc.com/presentation_image_h2/5be5fe29c5e7689d1c617ea2bf342a31/image-10.jpg)
char[] != String The "+" operator is overloaded for the String class, so this code does not run correctly. class string_test_1 { static void main(String[] args) { char[] msg = {'B', 'o', 'b'}; System. out. println ("msg = " + msg); } } Correct Version System. out. println("msg = " + String. valueof(msg);
![char String This code yields a compiler error class stringtest2 static void char[] != String This code yields a compiler error. class string_test_2 { static void](https://slidetodoc.com/presentation_image_h2/5be5fe29c5e7689d1c617ea2bf342a31/image-11.jpg)
char[] != String This code yields a compiler error. class string_test_2 { static void main(String[] args) { String myvar = "bob"; myvar[0] = 'B'; // error: myvar is not an array } }
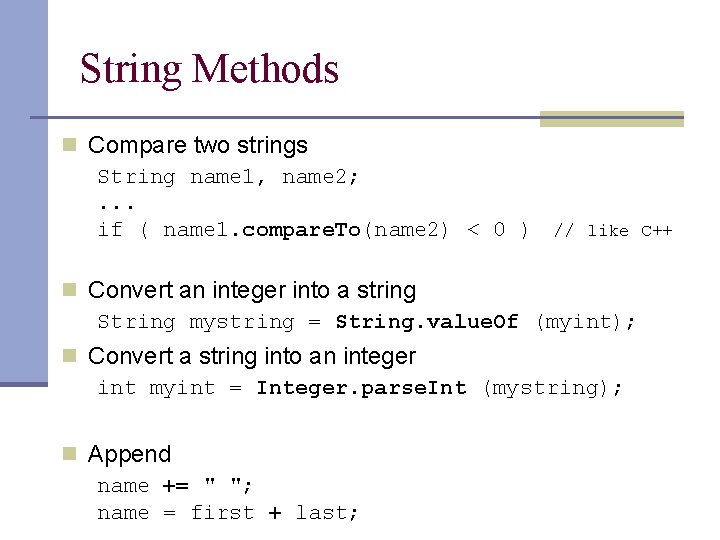
String Methods n Compare two strings String name 1, name 2; . . . if ( name 1. compare. To(name 2) < 0 ) // like C++ n Convert an integer into a string String mystring = String. value. Of (myint); n Convert a string into an integer int myint = Integer. parse. Int (mystring); n Append name += " "; name = first + last;
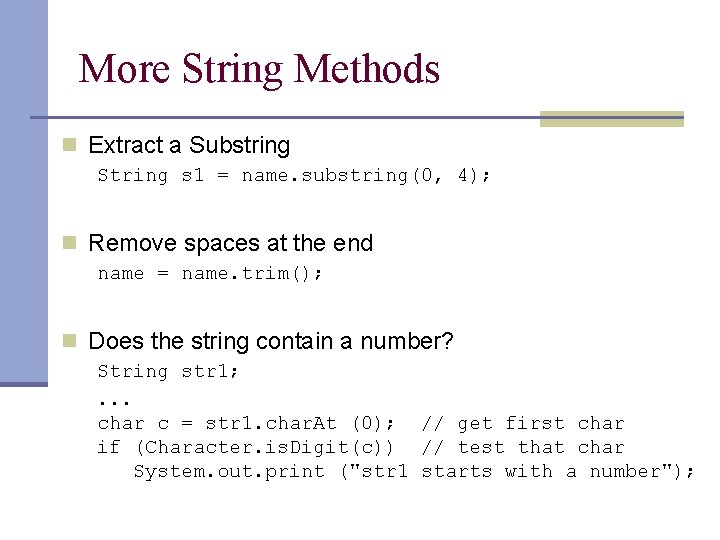
More String Methods n Extract a Substring String s 1 = name. substring(0, 4); n Remove spaces at the end name = name. trim(); n Does the string contain a number? String str 1; . . . char c = str 1. char. At (0); // get first char if (Character. is. Digit(c)) // test that char System. out. print ("str 1 starts with a number");
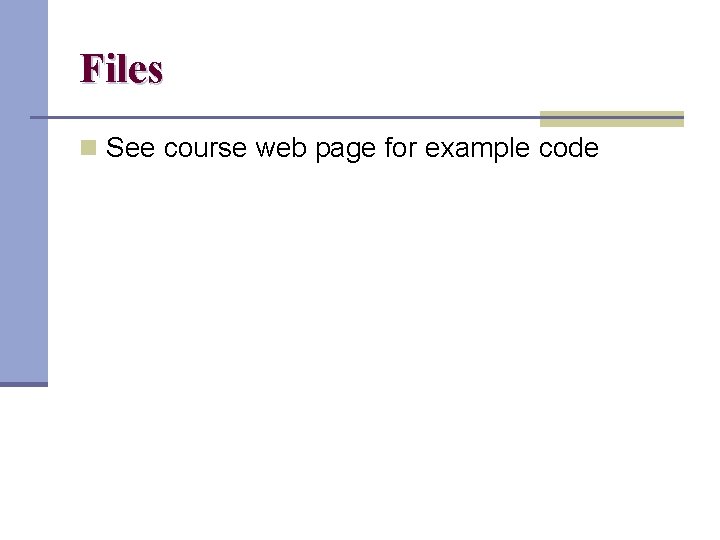
Files n See course web page for example code
Three masses are connected by strings
Wyłącz czynnik przed pierwiastek z 392
Kj 392
The passionate pupil declaring love
Chapter 5 lesson 4 declaring independence
Chapter 4 section 2 declaring independence
Declaring intent lol
Declaring the end from the beginning
Declaring intent lol
Sing to the king
Week by week plans for documenting children's development
Dynamic arrays and amortized analysis
Searching and sorting arrays in c++
What are the advantages of arrays?
Elastic strings and springs