Java Unit 9 Arrays Declaring and Processing Arrays
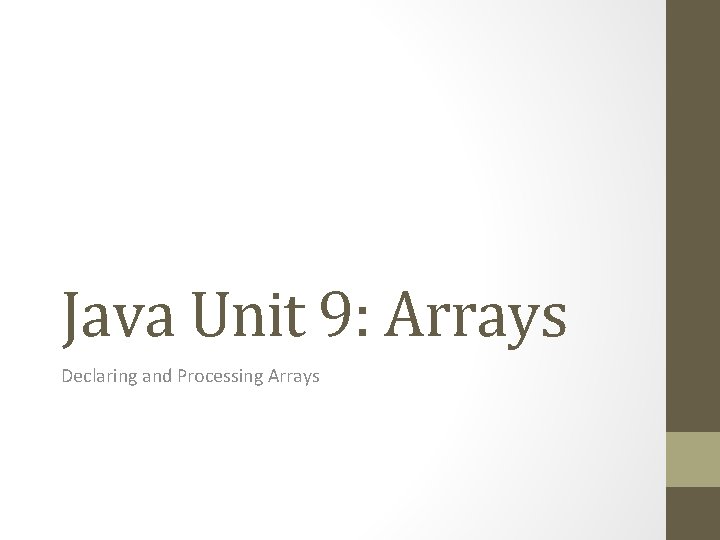
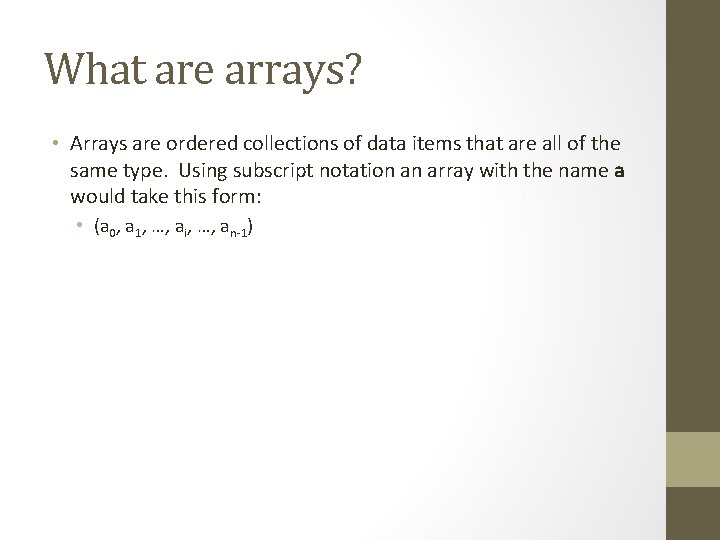
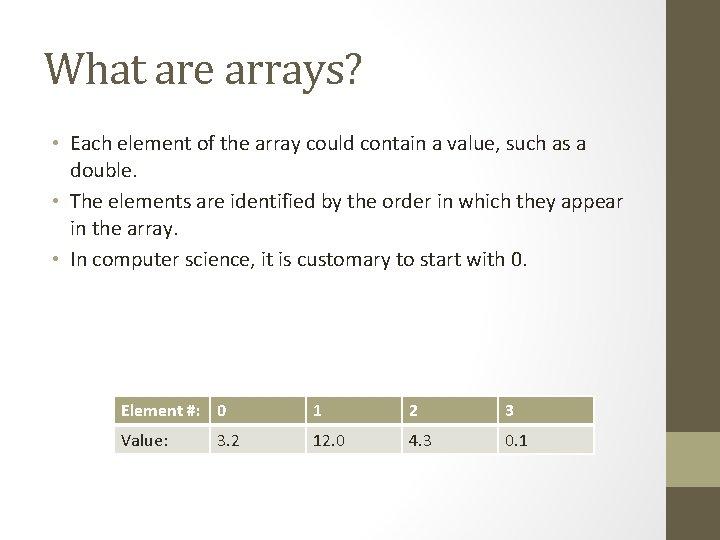
![Constructing arrays • Arrays in Java are objects, constructed like this: • double[] my. Constructing arrays • Arrays in Java are objects, constructed like this: • double[] my.](https://slidetodoc.com/presentation_image_h2/7e24197b7e2cb50d0c3c6cf826775d24/image-4.jpg)
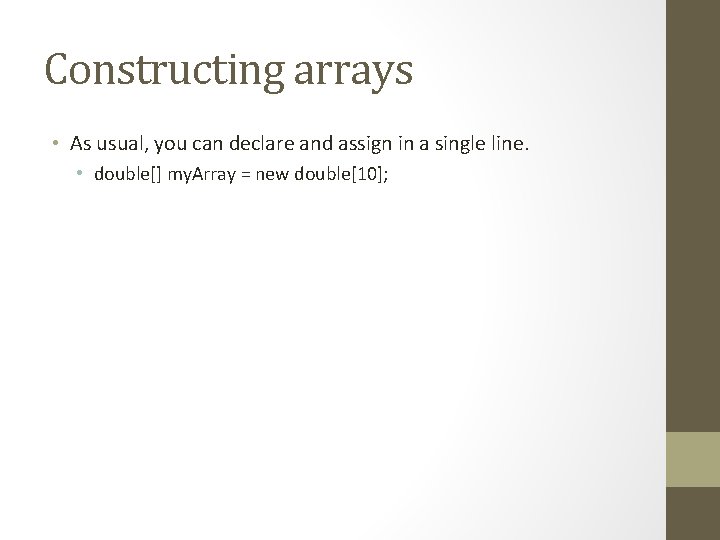
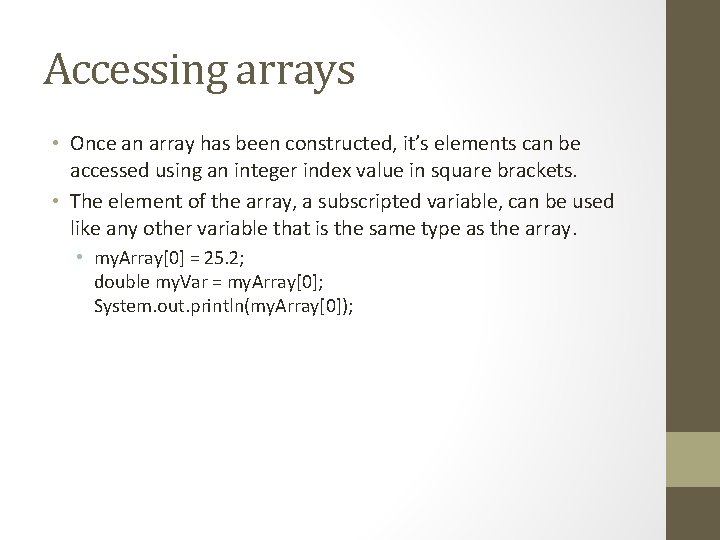
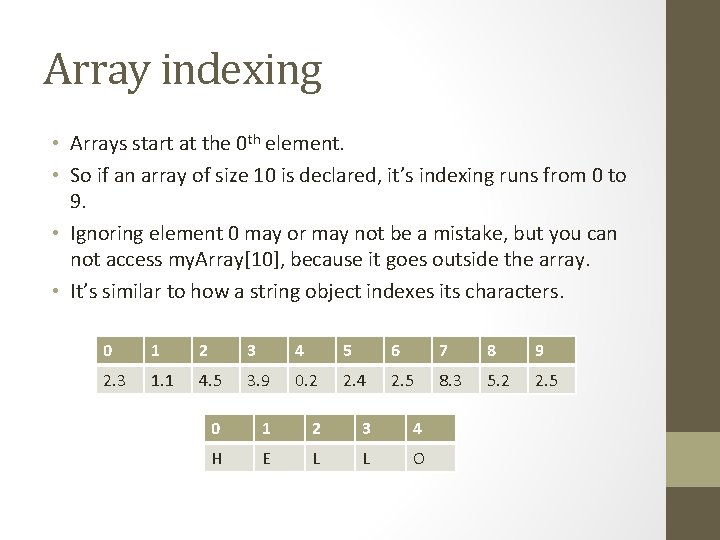
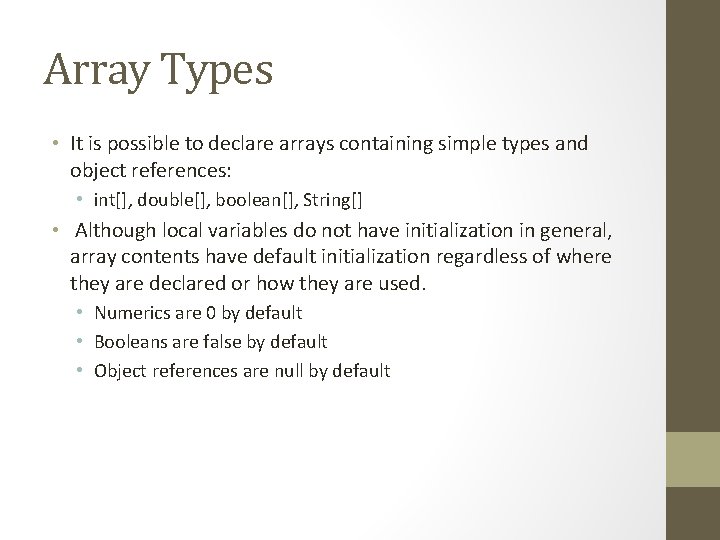
![main(String[] args) • Consider this line of code that appears in every program: • main(String[] args) • Consider this line of code that appears in every program: •](https://slidetodoc.com/presentation_image_h2/7e24197b7e2cb50d0c3c6cf826775d24/image-9.jpg)
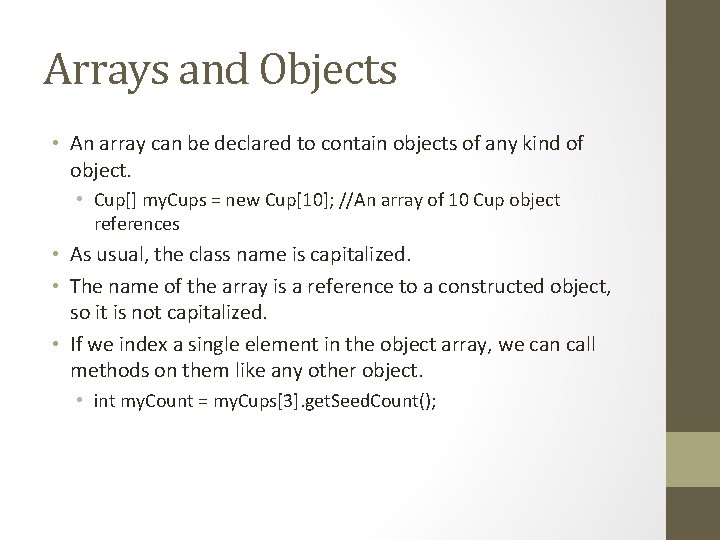
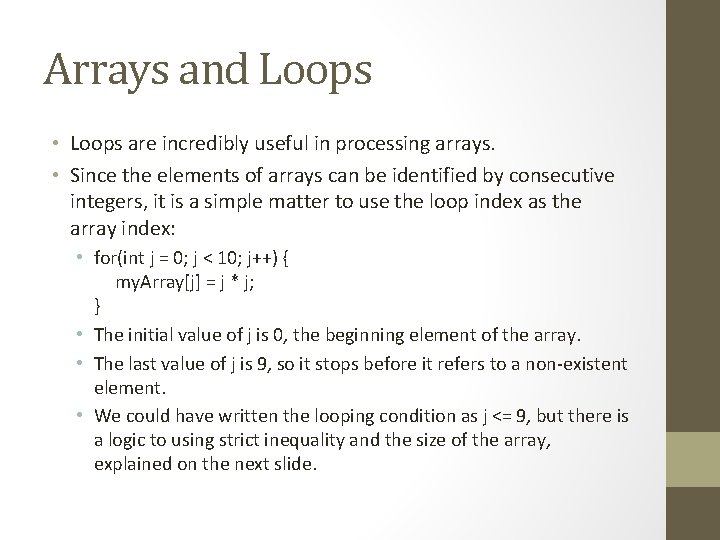
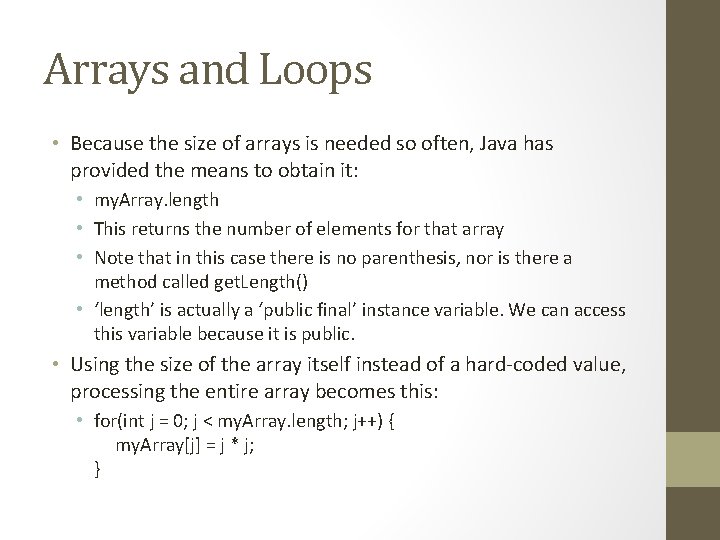
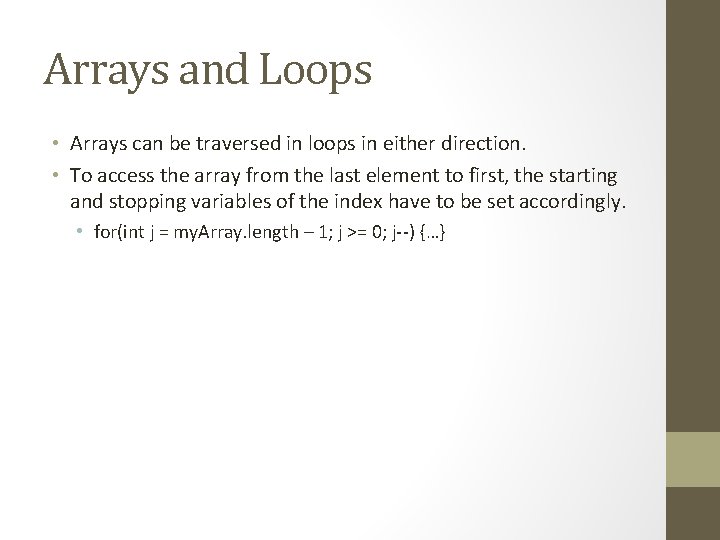
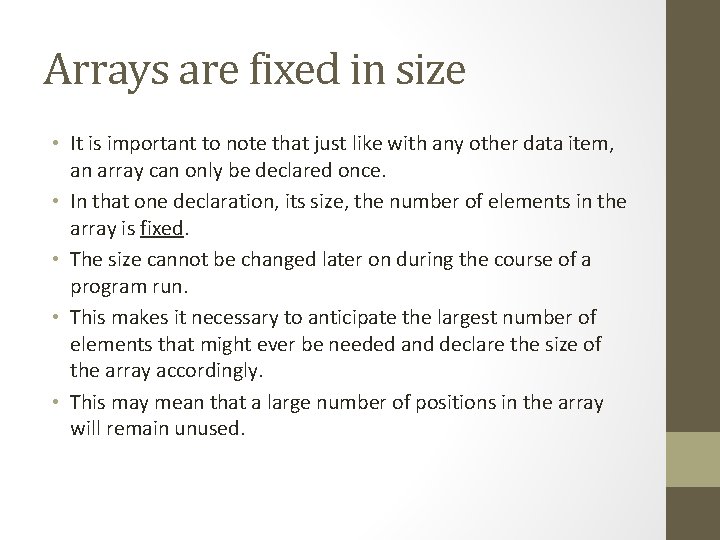
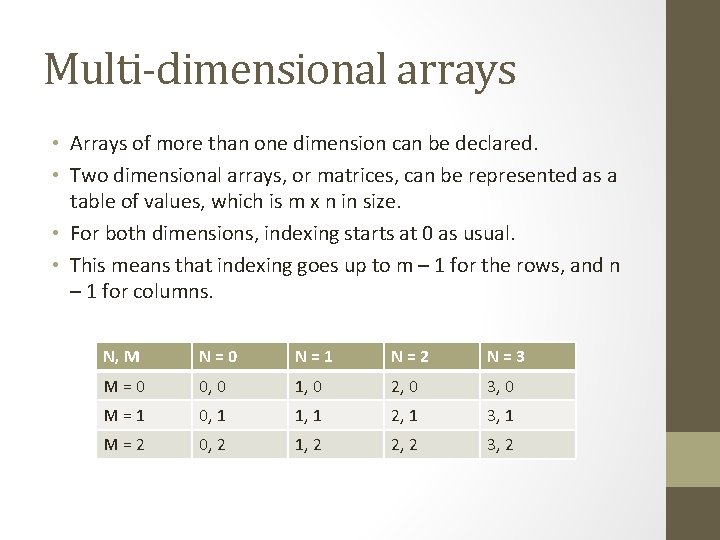
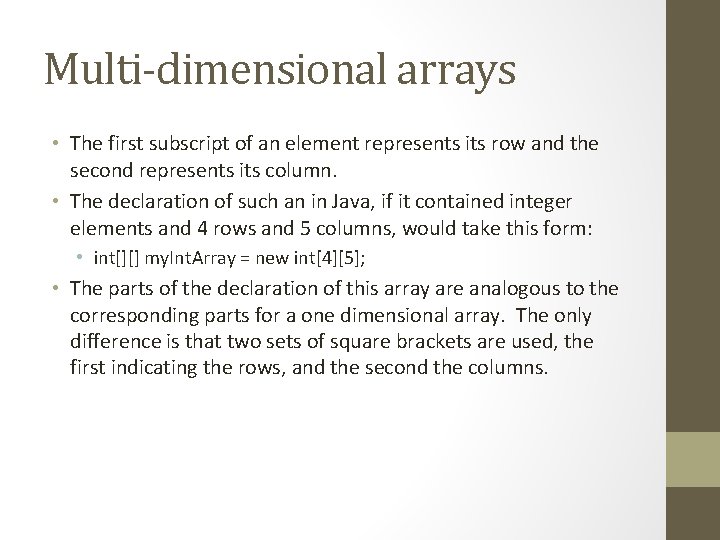
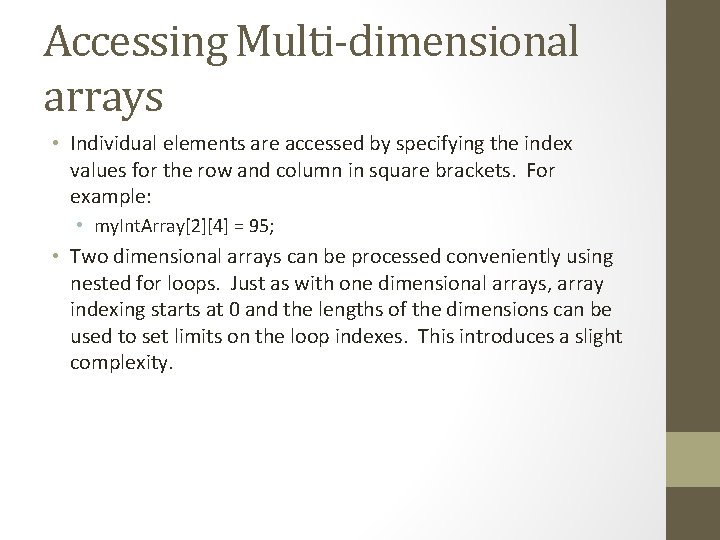
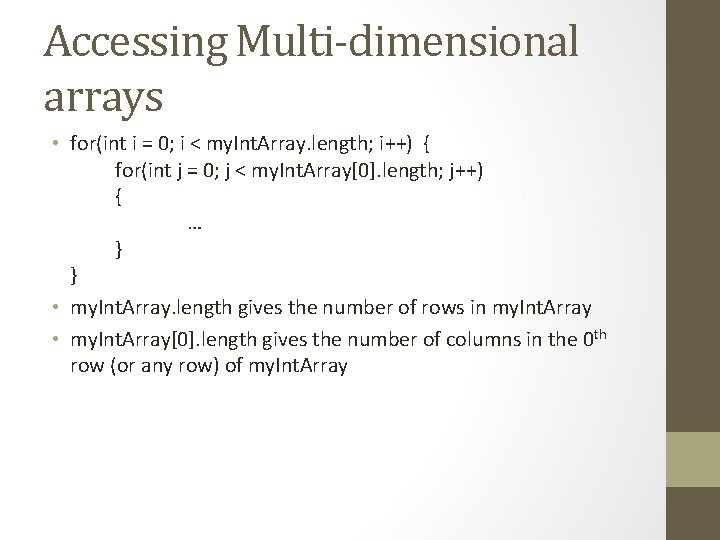
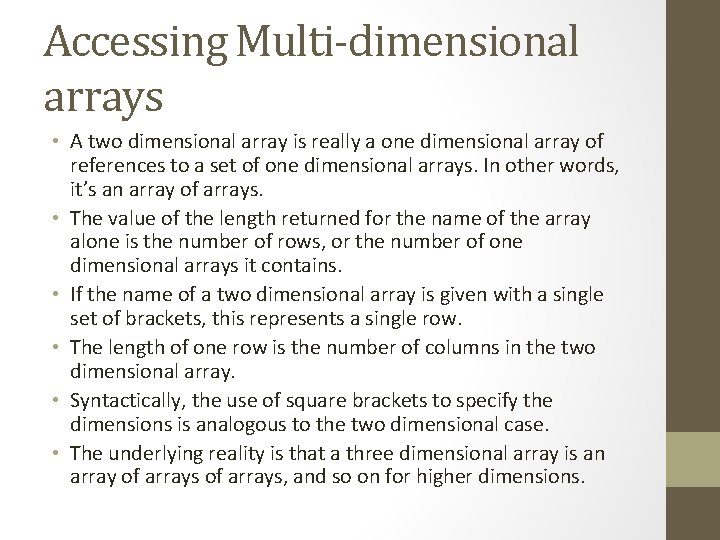
- Slides: 19
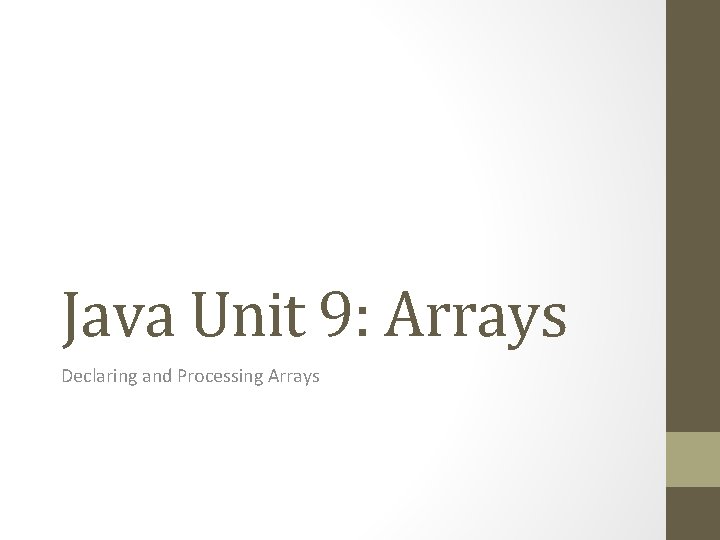
Java Unit 9: Arrays Declaring and Processing Arrays
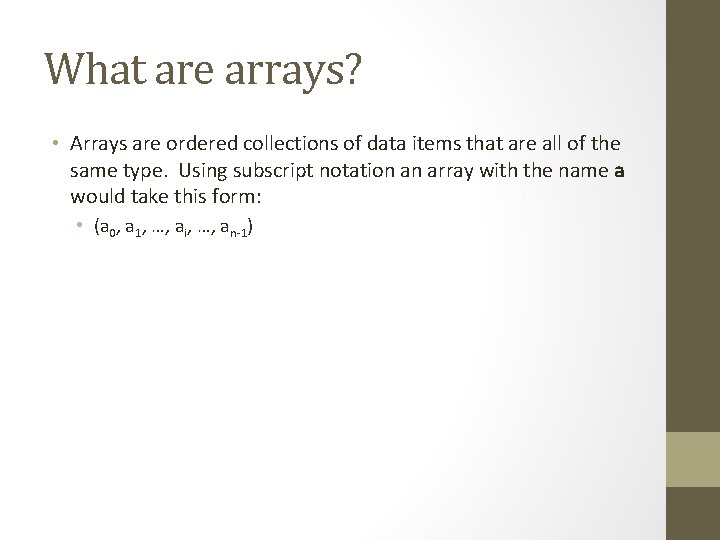
What are arrays? • Arrays are ordered collections of data items that are all of the same type. Using subscript notation an array with the name a would take this form: • (a 0, a 1, …, ai, …, an-1)
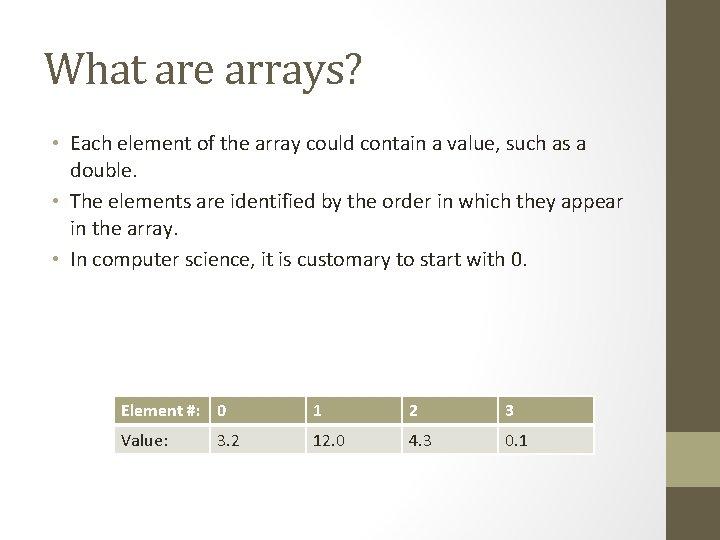
What are arrays? • Each element of the array could contain a value, such as a double. • The elements are identified by the order in which they appear in the array. • In computer science, it is customary to start with 0. Element #: 0 1 2 3 Value: 12. 0 4. 3 0. 1 3. 2
![Constructing arrays Arrays in Java are objects constructed like this double my Constructing arrays • Arrays in Java are objects, constructed like this: • double[] my.](https://slidetodoc.com/presentation_image_h2/7e24197b7e2cb50d0c3c6cf826775d24/image-4.jpg)
Constructing arrays • Arrays in Java are objects, constructed like this: • double[] my. Array; my. Array = new double[10]; • In this declaration, ‘double’ is the type of value that all of the elements in the array will be. • The brackets after ‘double’ indicates that this variable is an array. • In the assignment, we use the keyword new, followed by the type with brackets. In the brackets, the number of elements in the array is defined. In this case, it’s 10. • You could also say the array has a size of 10.
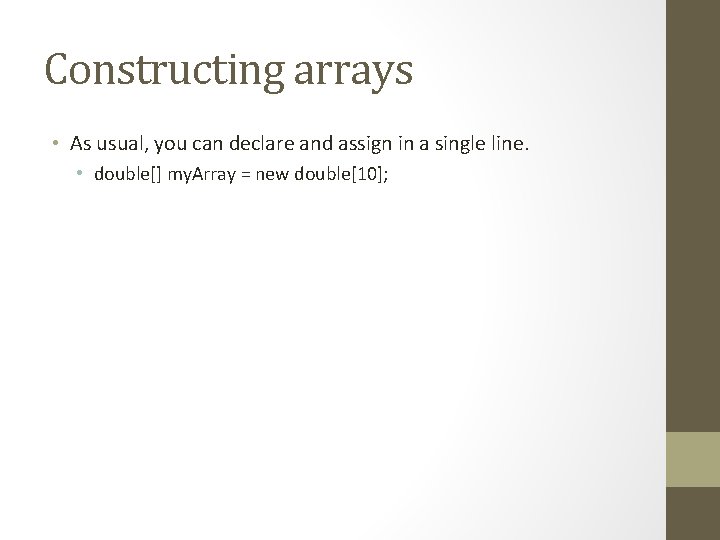
Constructing arrays • As usual, you can declare and assign in a single line. • double[] my. Array = new double[10];
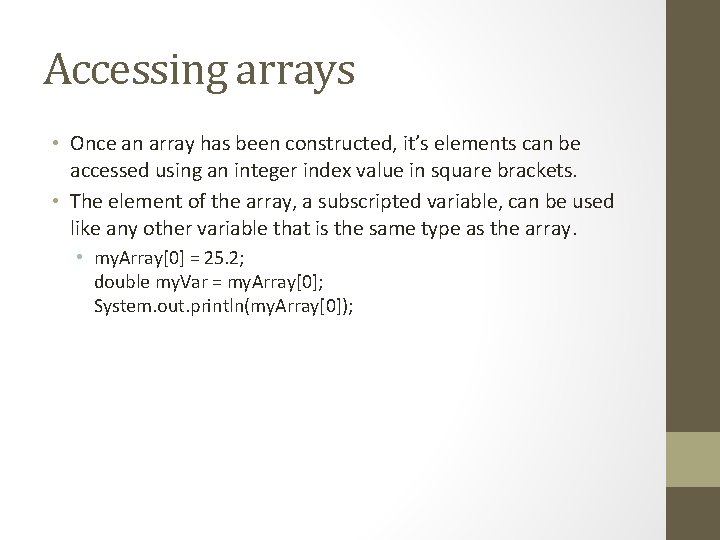
Accessing arrays • Once an array has been constructed, it’s elements can be accessed using an integer index value in square brackets. • The element of the array, a subscripted variable, can be used like any other variable that is the same type as the array. • my. Array[0] = 25. 2; double my. Var = my. Array[0]; System. out. println(my. Array[0]);
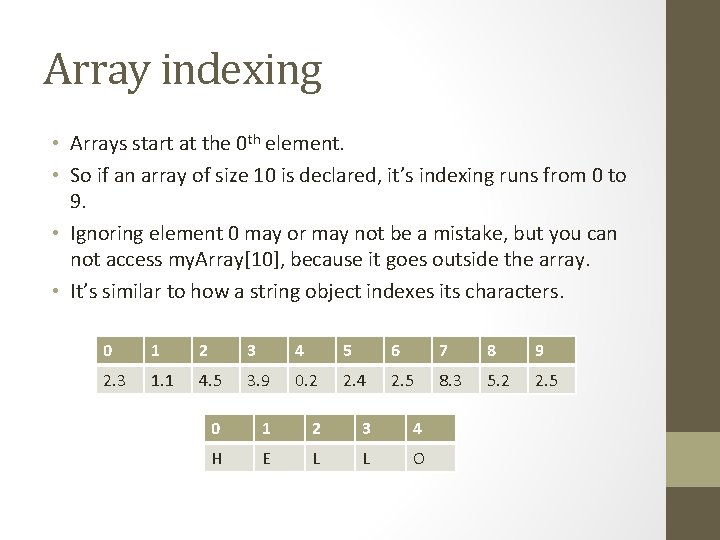
Array indexing • Arrays start at the 0 th element. • So if an array of size 10 is declared, it’s indexing runs from 0 to 9. • Ignoring element 0 may or may not be a mistake, but you can not access my. Array[10], because it goes outside the array. • It’s similar to how a string object indexes its characters. 0 1 2 3 4 5 6 7 8 9 2. 3 1. 1 4. 5 3. 9 0. 2 2. 4 2. 5 8. 3 5. 2 2. 5 0 1 2 3 4 H E L L O
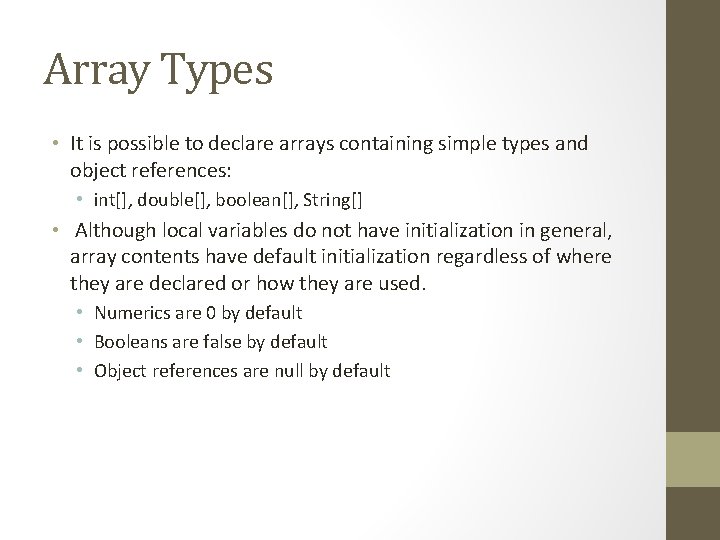
Array Types • It is possible to declare arrays containing simple types and object references: • int[], double[], boolean[], String[] • Although local variables do not have initialization in general, array contents have default initialization regardless of where they are declared or how they are used. • Numerics are 0 by default • Booleans are false by default • Object references are null by default
![mainString args Consider this line of code that appears in every program main(String[] args) • Consider this line of code that appears in every program: •](https://slidetodoc.com/presentation_image_h2/7e24197b7e2cb50d0c3c6cf826775d24/image-9.jpg)
main(String[] args) • Consider this line of code that appears in every program: • public static void main(String[] args) • We can see that it takes an array of Strings as a parameter. • If this program is run from the command line, multiple command line arguments can be entered on the same line that executes the program, with each argument separated by a string: • java My. Java. class my. Arg 1 my. Arg 2 my. Arg 3 • The command line arguments would be stored inside an array, and passed into the main() method as ‘args’.
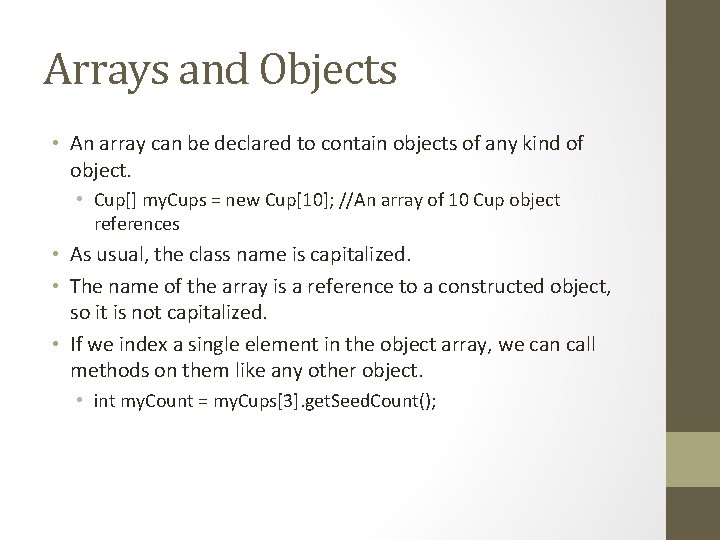
Arrays and Objects • An array can be declared to contain objects of any kind of object. • Cup[] my. Cups = new Cup[10]; //An array of 10 Cup object references • As usual, the class name is capitalized. • The name of the array is a reference to a constructed object, so it is not capitalized. • If we index a single element in the object array, we can call methods on them like any other object. • int my. Count = my. Cups[3]. get. Seed. Count();
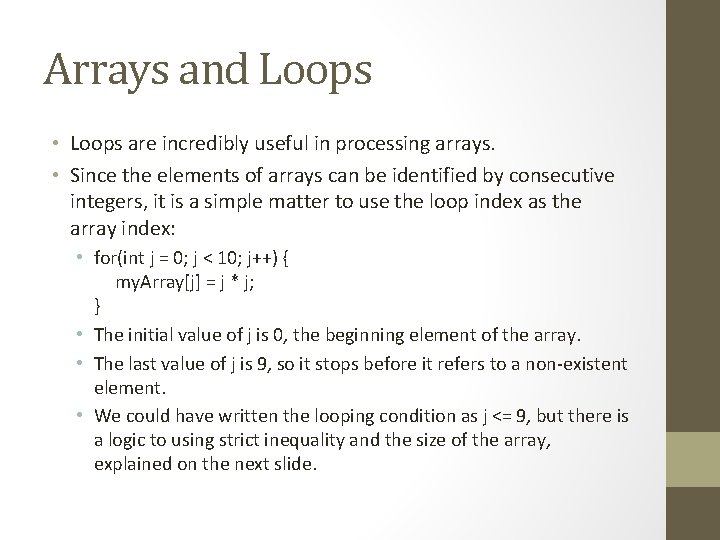
Arrays and Loops • Loops are incredibly useful in processing arrays. • Since the elements of arrays can be identified by consecutive integers, it is a simple matter to use the loop index as the array index: • for(int j = 0; j < 10; j++) { my. Array[j] = j * j; } • The initial value of j is 0, the beginning element of the array. • The last value of j is 9, so it stops before it refers to a non-existent element. • We could have written the looping condition as j <= 9, but there is a logic to using strict inequality and the size of the array, explained on the next slide.
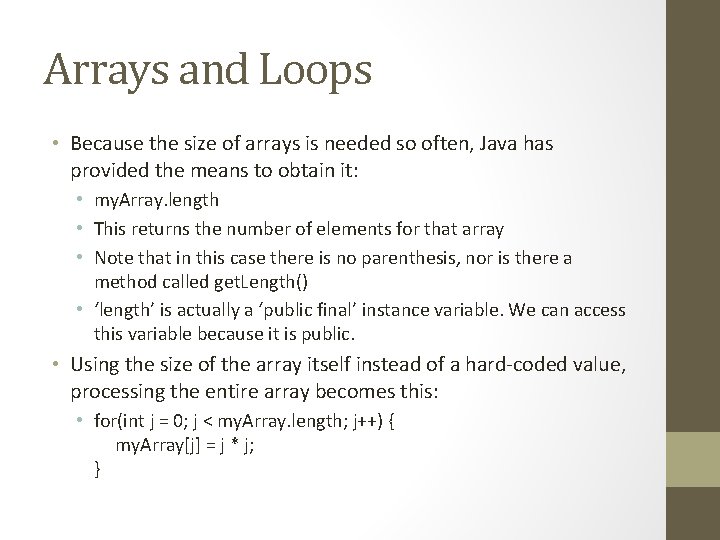
Arrays and Loops • Because the size of arrays is needed so often, Java has provided the means to obtain it: • my. Array. length • This returns the number of elements for that array • Note that in this case there is no parenthesis, nor is there a method called get. Length() • ‘length’ is actually a ‘public final’ instance variable. We can access this variable because it is public. • Using the size of the array itself instead of a hard-coded value, processing the entire array becomes this: • for(int j = 0; j < my. Array. length; j++) { my. Array[j] = j * j; }
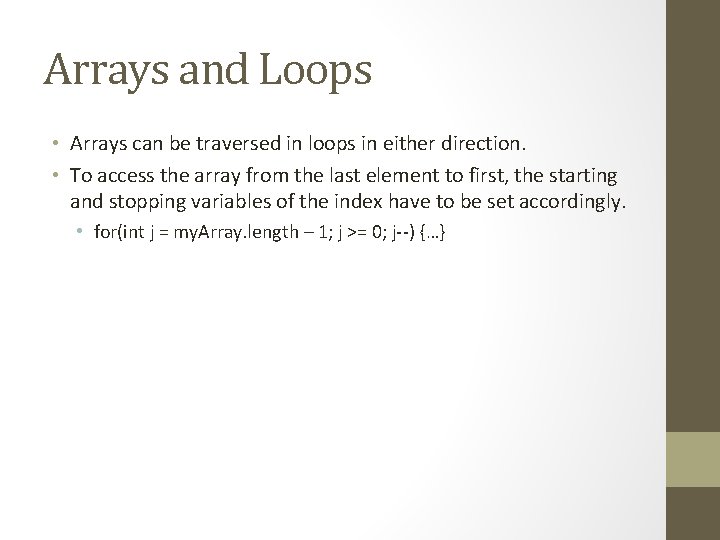
Arrays and Loops • Arrays can be traversed in loops in either direction. • To access the array from the last element to first, the starting and stopping variables of the index have to be set accordingly. • for(int j = my. Array. length – 1; j >= 0; j--) {…}
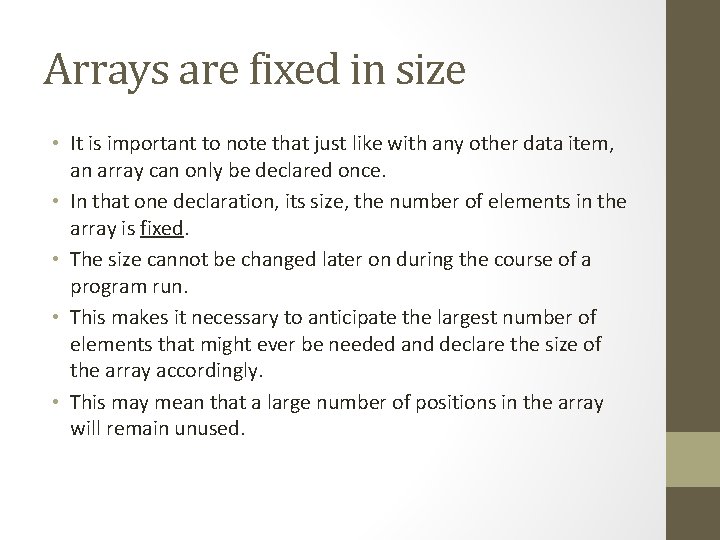
Arrays are fixed in size • It is important to note that just like with any other data item, an array can only be declared once. • In that one declaration, its size, the number of elements in the array is fixed. • The size cannot be changed later on during the course of a program run. • This makes it necessary to anticipate the largest number of elements that might ever be needed and declare the size of the array accordingly. • This may mean that a large number of positions in the array will remain unused.
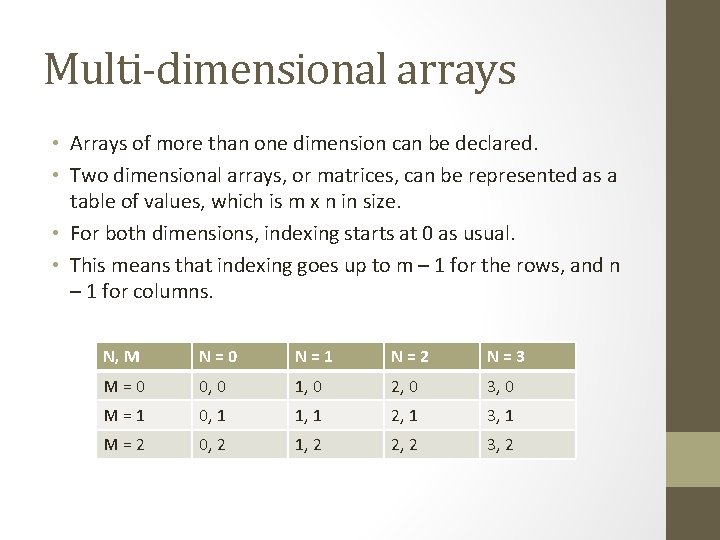
Multi-dimensional arrays • Arrays of more than one dimension can be declared. • Two dimensional arrays, or matrices, can be represented as a table of values, which is m x n in size. • For both dimensions, indexing starts at 0 as usual. • This means that indexing goes up to m – 1 for the rows, and n – 1 for columns. N, M N=0 N=1 N=2 N=3 M=0 0, 0 1, 0 2, 0 3, 0 M=1 0, 1 1, 1 2, 1 3, 1 M=2 0, 2 1, 2 2, 2 3, 2
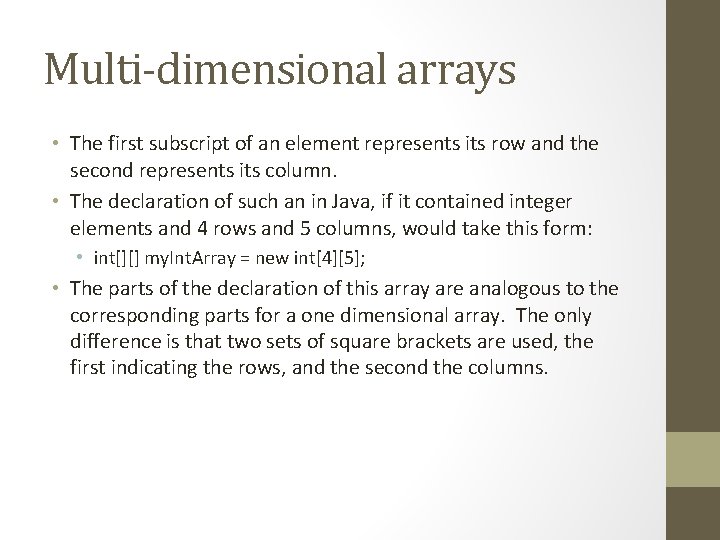
Multi-dimensional arrays • The first subscript of an element represents its row and the second represents its column. • The declaration of such an in Java, if it contained integer elements and 4 rows and 5 columns, would take this form: • int[][] my. Int. Array = new int[4][5]; • The parts of the declaration of this array are analogous to the corresponding parts for a one dimensional array. The only difference is that two sets of square brackets are used, the first indicating the rows, and the second the columns.
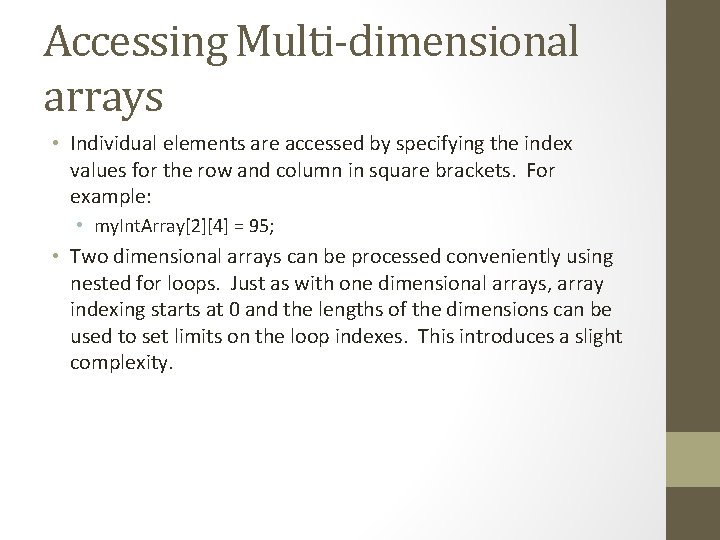
Accessing Multi-dimensional arrays • Individual elements are accessed by specifying the index values for the row and column in square brackets. For example: • my. Int. Array[2][4] = 95; • Two dimensional arrays can be processed conveniently using nested for loops. Just as with one dimensional arrays, array indexing starts at 0 and the lengths of the dimensions can be used to set limits on the loop indexes. This introduces a slight complexity.
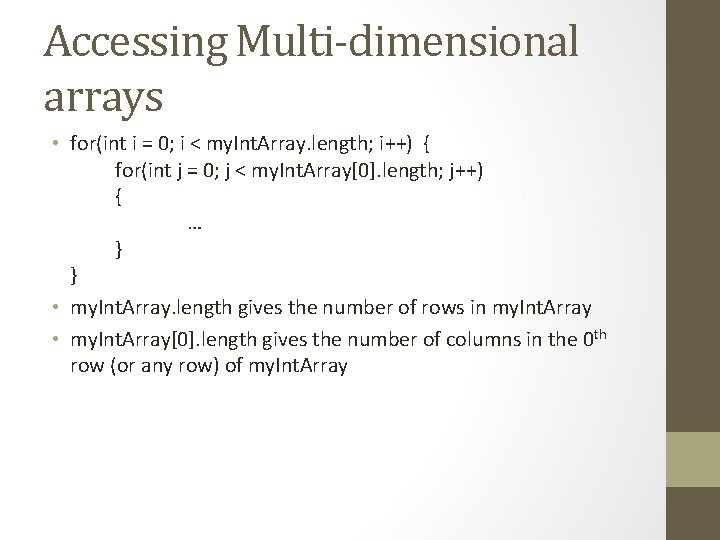
Accessing Multi-dimensional arrays • for(int i = 0; i < my. Int. Array. length; i++) { for(int j = 0; j < my. Int. Array[0]. length; j++) { … } } • my. Int. Array. length gives the number of rows in my. Int. Array • my. Int. Array[0]. length gives the number of columns in the 0 th row (or any row) of my. Int. Array
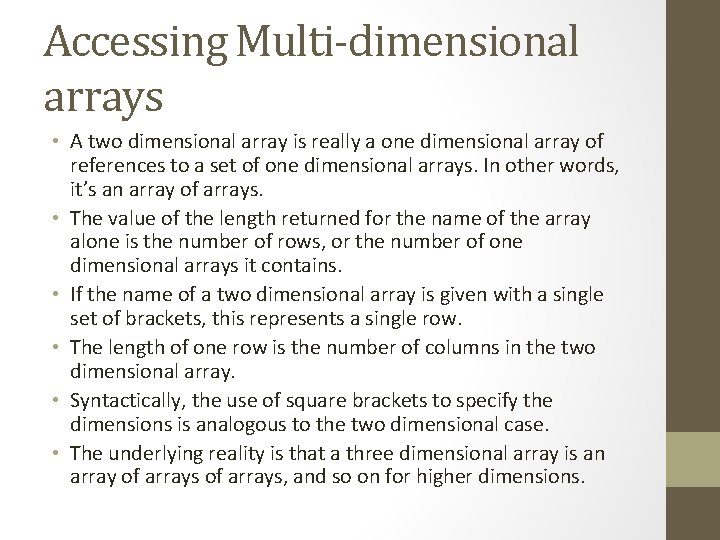
Accessing Multi-dimensional arrays • A two dimensional array is really a one dimensional array of references to a set of one dimensional arrays. In other words, it’s an array of arrays. • The value of the length returned for the name of the array alone is the number of rows, or the number of one dimensional arrays it contains. • If the name of a two dimensional array is given with a single set of brackets, this represents a single row. • The length of one row is the number of columns in the two dimensional array. • Syntactically, the use of square brackets to specify the dimensions is analogous to the two dimensional case. • The underlying reality is that a three dimensional array is an array of arrays, and so on for higher dimensions.
Parallel array java
Arreglos unidimensionales java
Arreglos bidimensionales en java
How to write a free verse poem
Declaration of independence sections
Chapter 4 section 2 declaring independence
Lol declaring intent
Declaring the end from the beginning
Declaring intent lol
Sing to the king
Neighborhood processing
Primary processing
Batch processing vs interactive processing
Dynamic arrays and amortized analysis
Searching and sorting arrays in c++
Advantages of array
Unit 6 review questions
Bottom up and top down processing
Gloria suarez
Bottom up processing