Announcements Assignment 2 is posted due Wed Oct
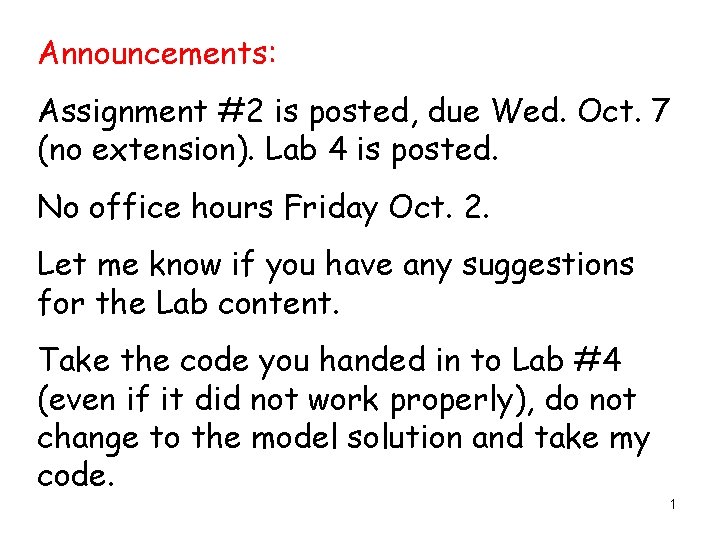
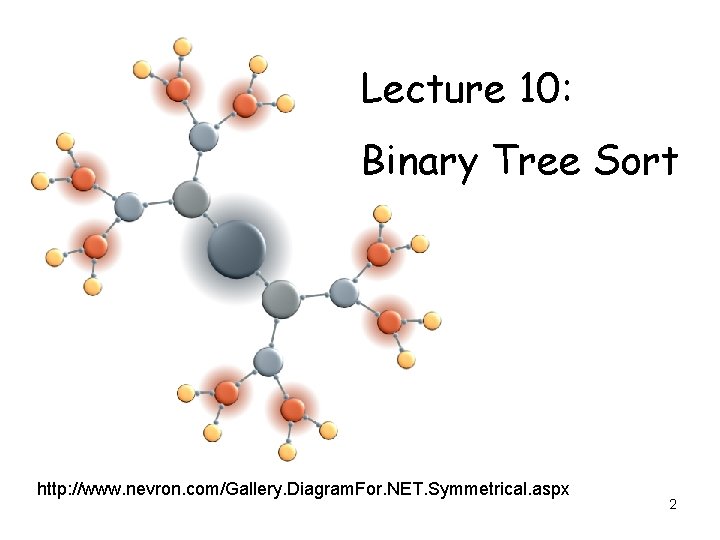
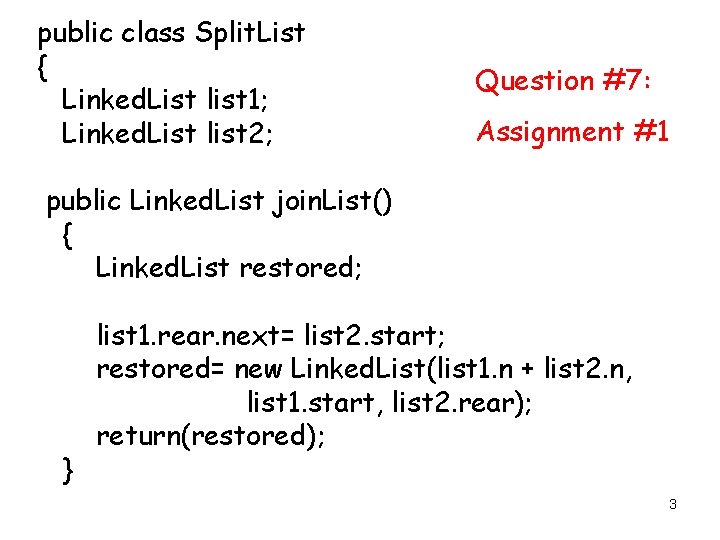
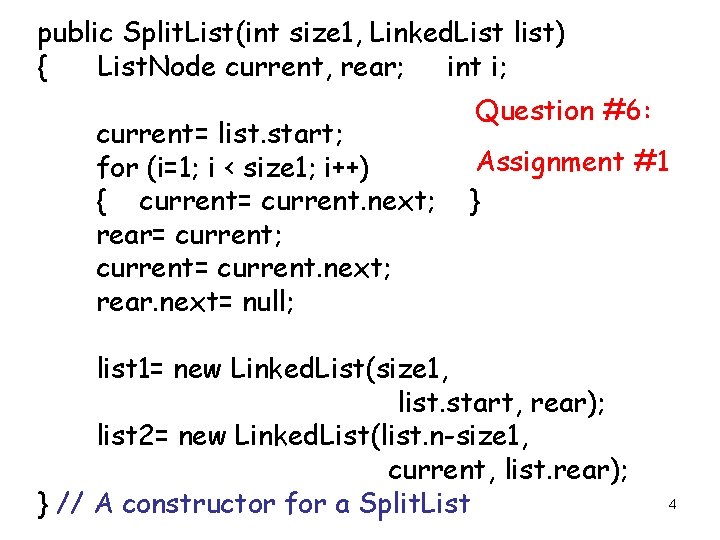
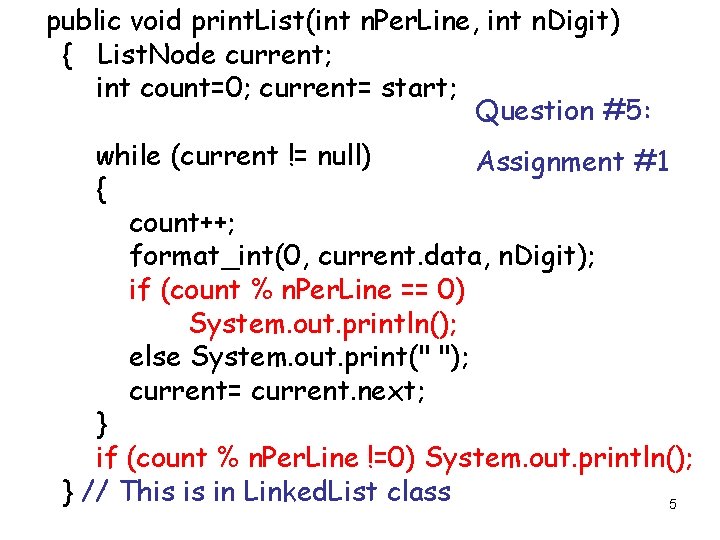
![public static void main(String args[]) Main: { int i; Linked. List list; Split. List public static void main(String args[]) Main: { int i; Linked. List list; Split. List](https://slidetodoc.com/presentation_image_h2/0d56b0b5d273fdbab378df38f39bdbf1/image-6.jpg)
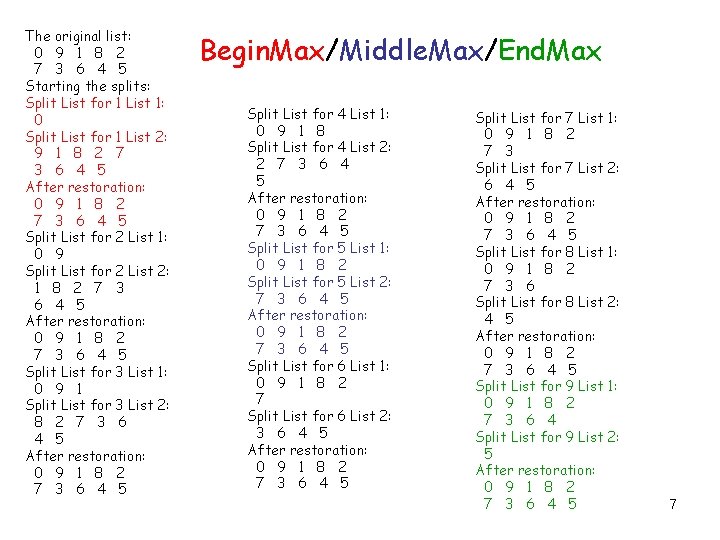
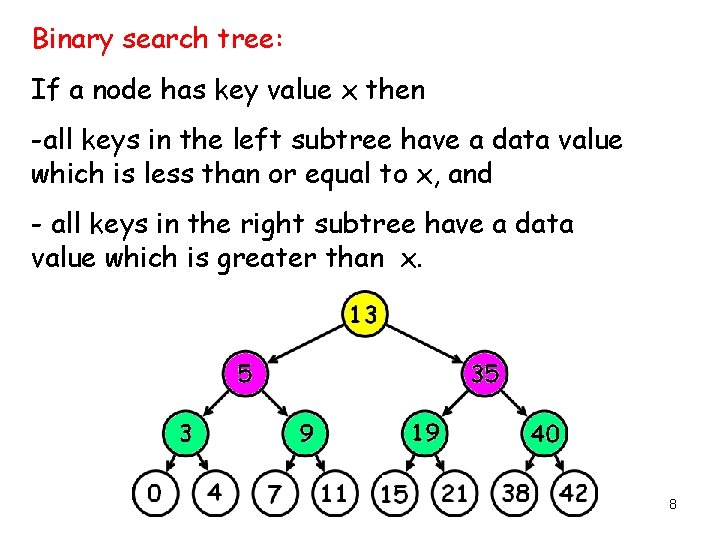
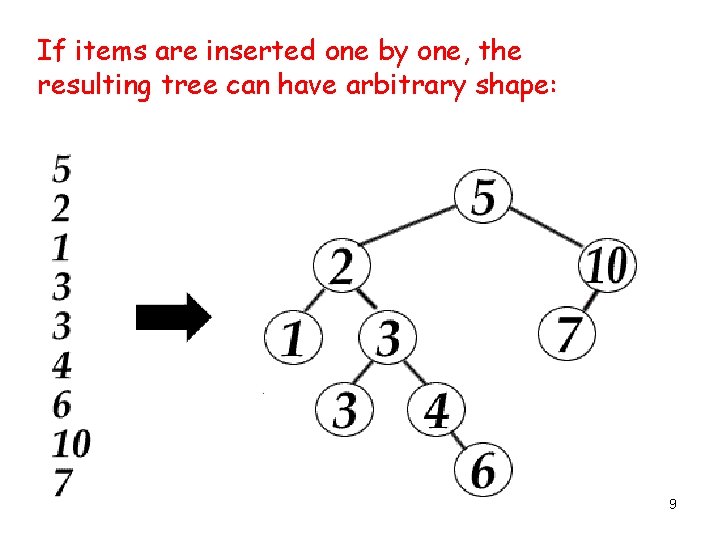
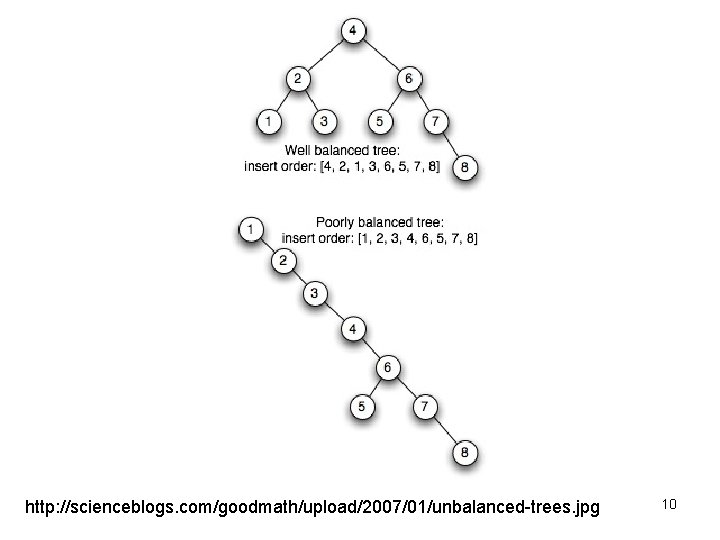
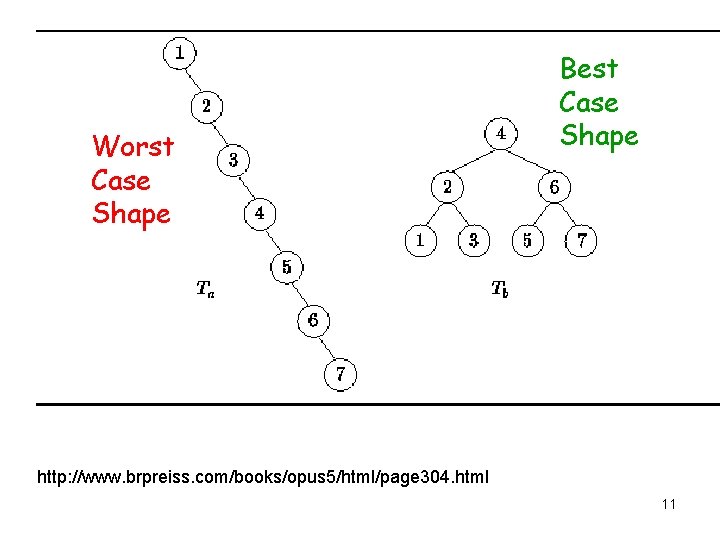
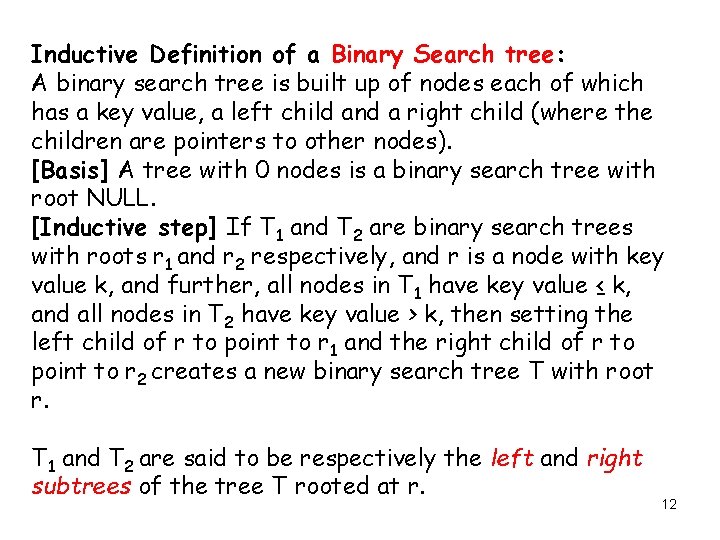
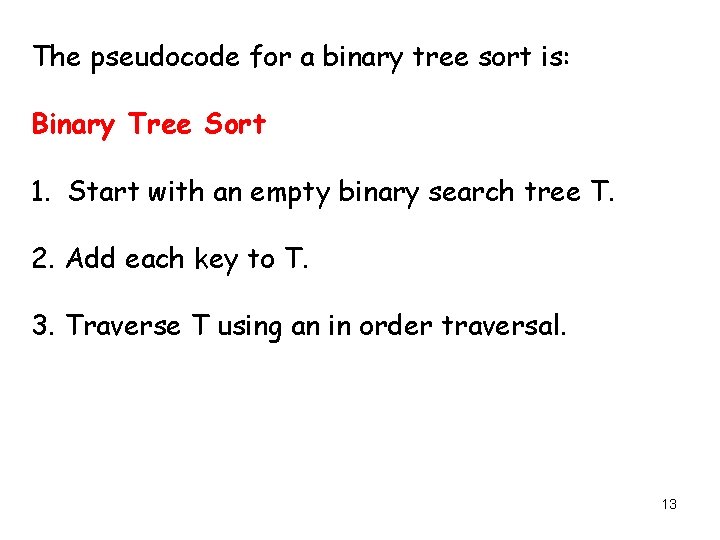
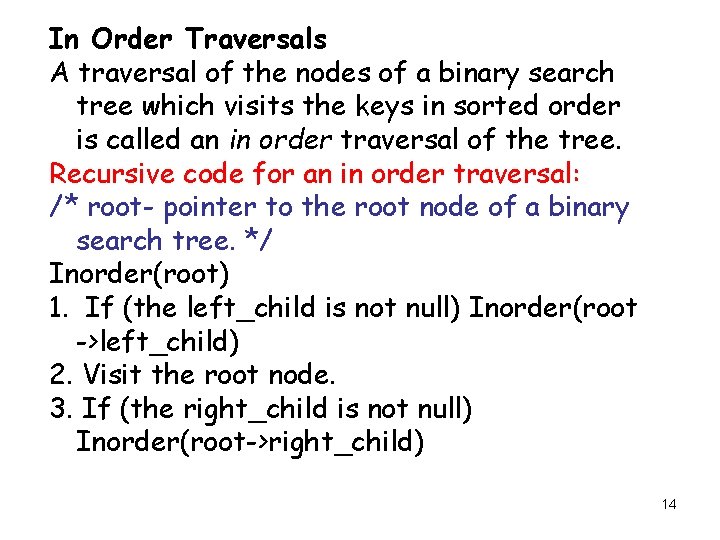
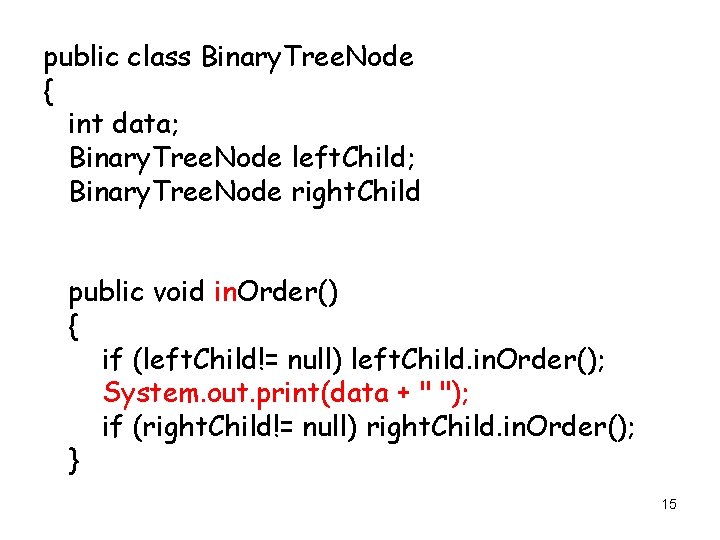
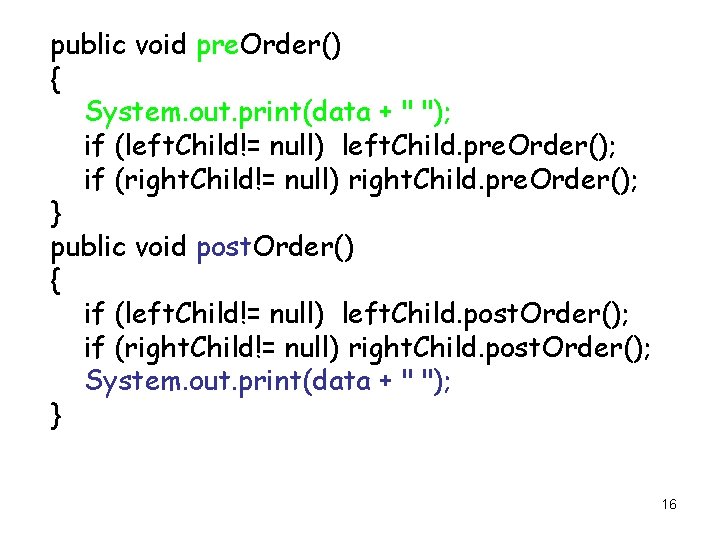
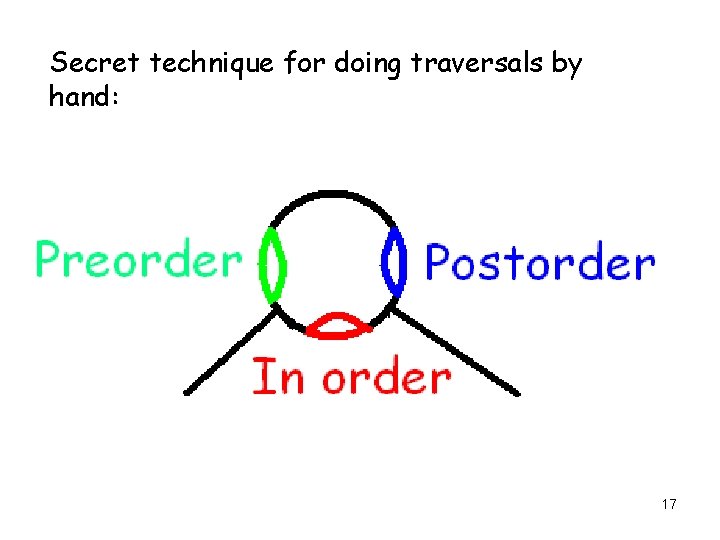
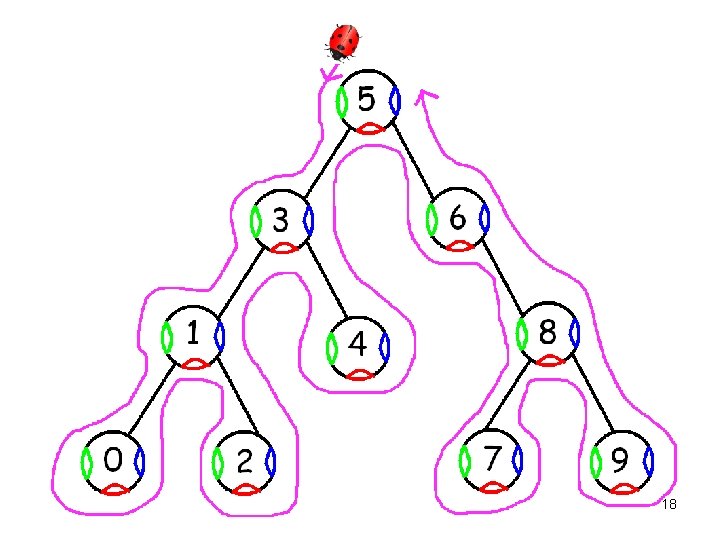
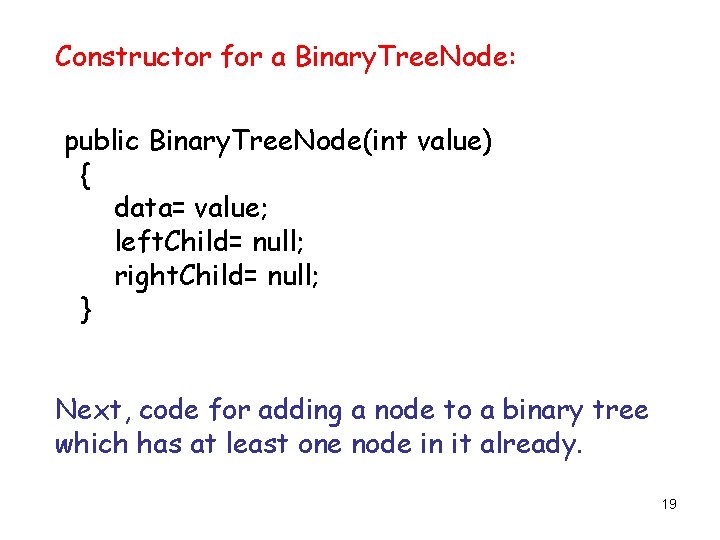
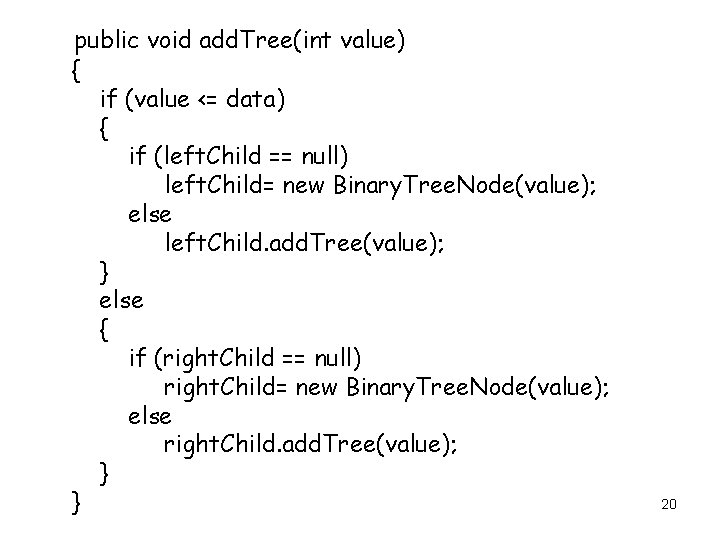
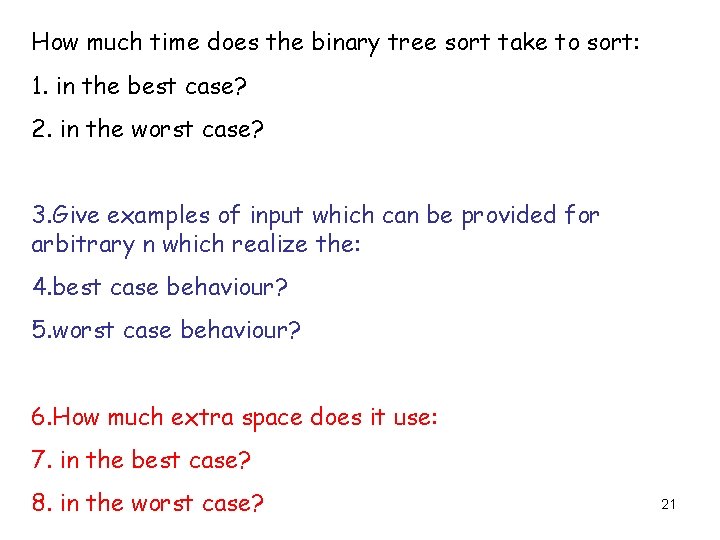
- Slides: 21
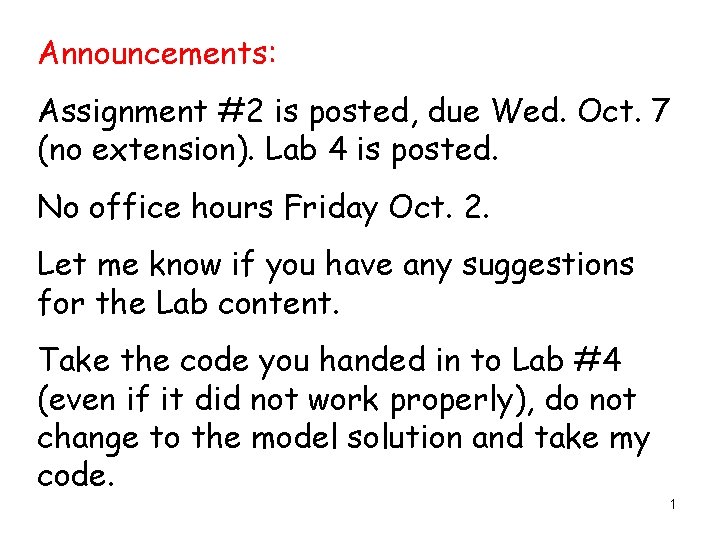
Announcements: Assignment #2 is posted, due Wed. Oct. 7 (no extension). Lab 4 is posted. No office hours Friday Oct. 2. Let me know if you have any suggestions for the Lab content. Take the code you handed in to Lab #4 (even if it did not work properly), do not change to the model solution and take my code. 1
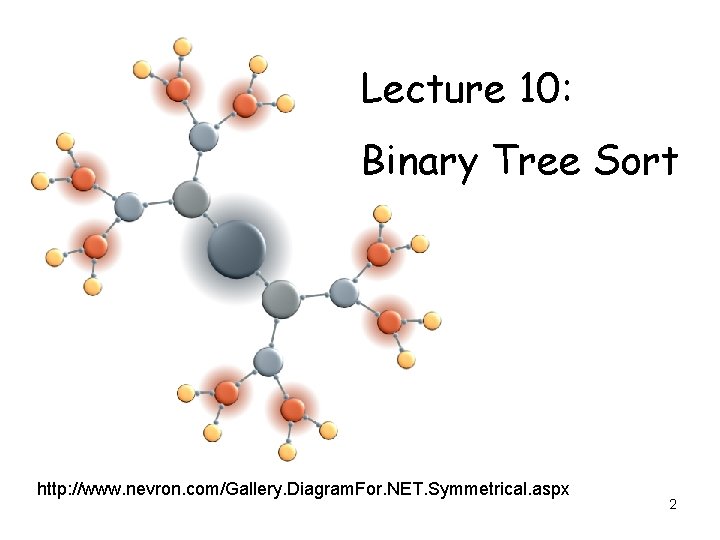
Lecture 10: Binary Tree Sort http: //www. nevron. com/Gallery. Diagram. For. NET. Symmetrical. aspx 2
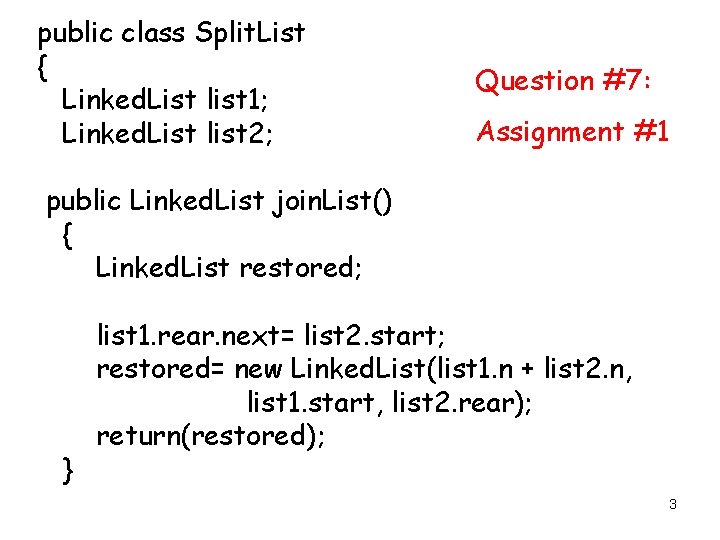
public class Split. List { Linked. List list 1; Linked. List list 2; Question #7: Assignment #1 public Linked. List join. List() { Linked. List restored; } list 1. rear. next= list 2. start; restored= new Linked. List(list 1. n + list 2. n, list 1. start, list 2. rear); return(restored); 3
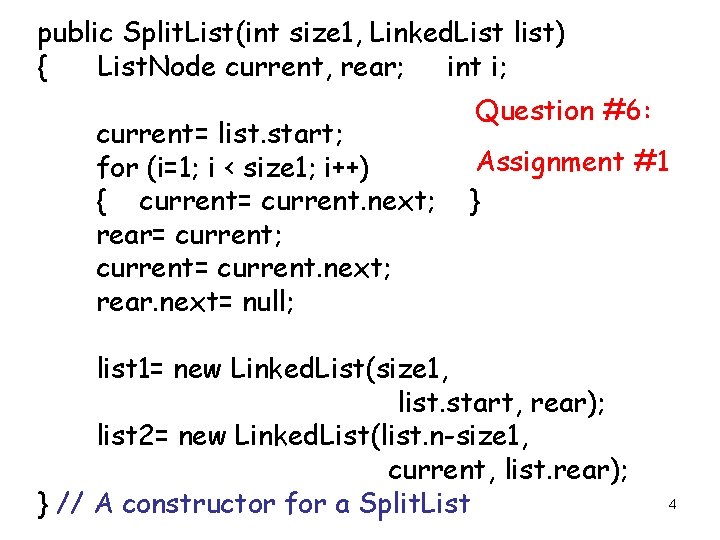
public Split. List(int size 1, Linked. List list) { List. Node current, rear; int i; current= list. start; for (i=1; i < size 1; i++) { current= current. next; rear= current; current= current. next; rear. next= null; Question #6: Assignment #1 } list 1= new Linked. List(size 1, list. start, rear); list 2= new Linked. List(list. n-size 1, current, list. rear); } // A constructor for a Split. List 4
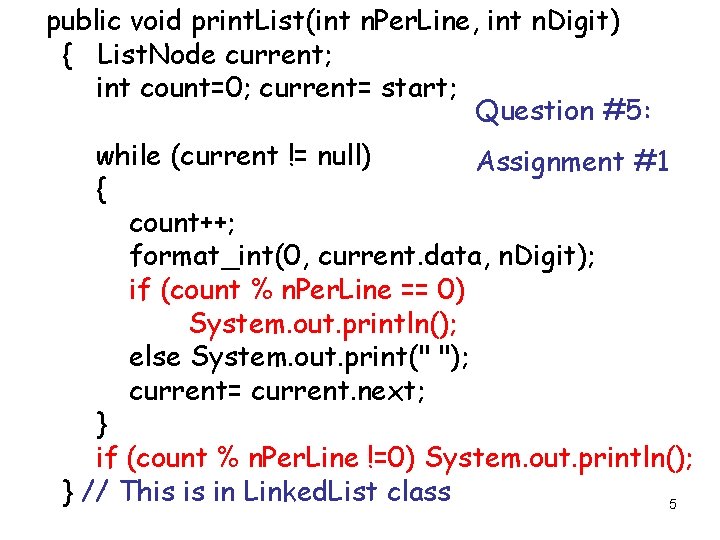
public void print. List(int n. Per. Line, int n. Digit) { List. Node current; int count=0; current= start; Question #5: while (current != null) Assignment #1 { count++; format_int(0, current. data, n. Digit); if (count % n. Per. Line == 0) System. out. println(); else System. out. print(" "); current= current. next; } if (count % n. Per. Line !=0) System. out. println(); } // This is in Linked. List class 5
![public static void mainString args Main int i Linked List list Split List public static void main(String args[]) Main: { int i; Linked. List list; Split. List](https://slidetodoc.com/presentation_image_h2/0d56b0b5d273fdbab378df38f39bdbf1/image-6.jpg)
public static void main(String args[]) Main: { int i; Linked. List list; Split. List split; Scanner in = new Scanner(System. in); Assignment list= new Linked. List(); while (list. read. Rear(in)) { System. out. println("The original list: "); list. print. List(5, 3); System. out. println("Starting the splits: "); for (i=1; i < list. n; i++) { split= new Split. List(i, list); System. out. println("Split List for " + i + " List 1: "); split. list 1. print. List(5, 3); System. out. println("Split List for " + i + " List 2: "); split. list 2. print. List(5, 3); list= split. join. List(); System. out. println("After restoration: "); list. print. List(5, 3); } } } #1 6
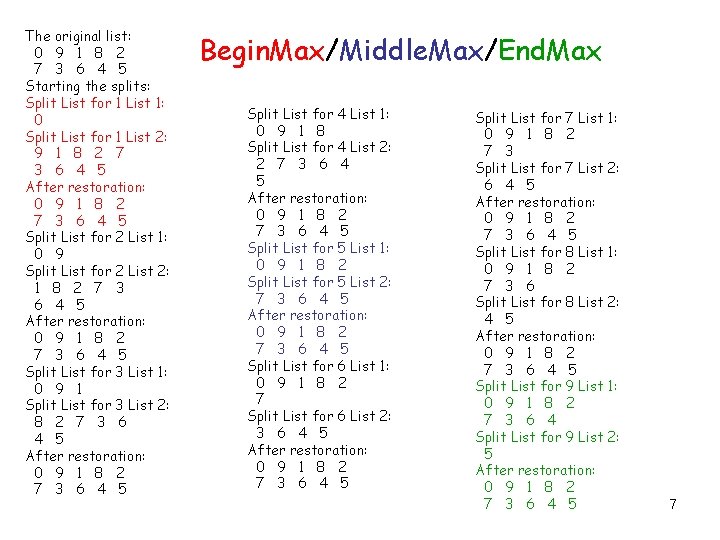
The original list: 0 9 1 8 2 7 3 6 4 5 Starting the splits: Split List for 1 List 1: 0 Split List for 1 List 2: 9 1 8 2 7 3 6 4 5 After restoration: 0 9 1 8 2 7 3 6 4 5 Split List for 2 List 1: 0 9 Split List for 2 List 2: 1 8 2 7 3 6 4 5 After restoration: 0 9 1 8 2 7 3 6 4 5 Split List for 3 List 1: 0 9 1 Split List for 3 List 2: 8 2 7 3 6 4 5 After restoration: 0 9 1 8 2 7 3 6 4 5 Begin. Max/Middle. Max/End. Max Split List for 4 List 1: 0 9 1 8 Split List for 4 List 2: 2 7 3 6 4 5 After restoration: 0 9 1 8 2 7 3 6 4 5 Split List for 5 List 1: 0 9 1 8 2 Split List for 5 List 2: 7 3 6 4 5 After restoration: 0 9 1 8 2 7 3 6 4 5 Split List for 6 List 1: 0 9 1 8 2 7 Split List for 6 List 2: 3 6 4 5 After restoration: 0 9 1 8 2 7 3 6 4 5 Split List for 7 List 1: 0 9 1 8 2 7 3 Split List for 7 List 2: 6 4 5 After restoration: 0 9 1 8 2 7 3 6 4 5 Split List for 8 List 1: 0 9 1 8 2 7 3 6 Split List for 8 List 2: 4 5 After restoration: 0 9 1 8 2 7 3 6 4 5 Split List for 9 List 1: 0 9 1 8 2 7 3 6 4 Split List for 9 List 2: 5 After restoration: 0 9 1 8 2 7 3 6 4 5 7
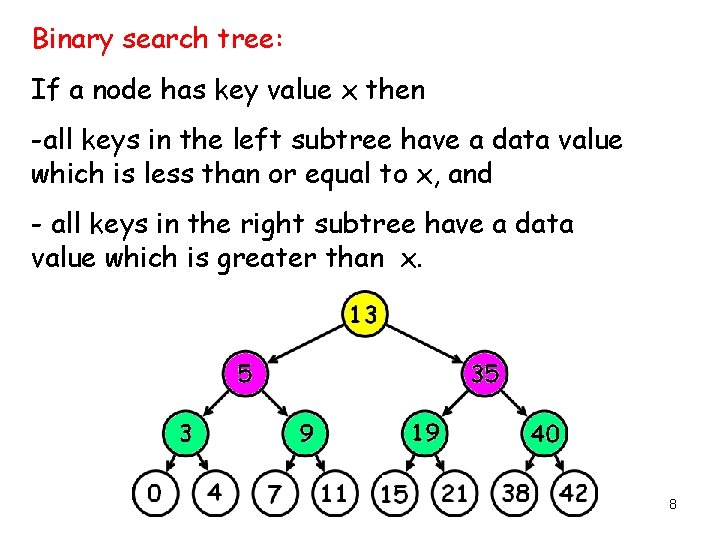
Binary search tree: If a node has key value x then -all keys in the left subtree have a data value which is less than or equal to x, and - all keys in the right subtree have a data value which is greater than x. 8
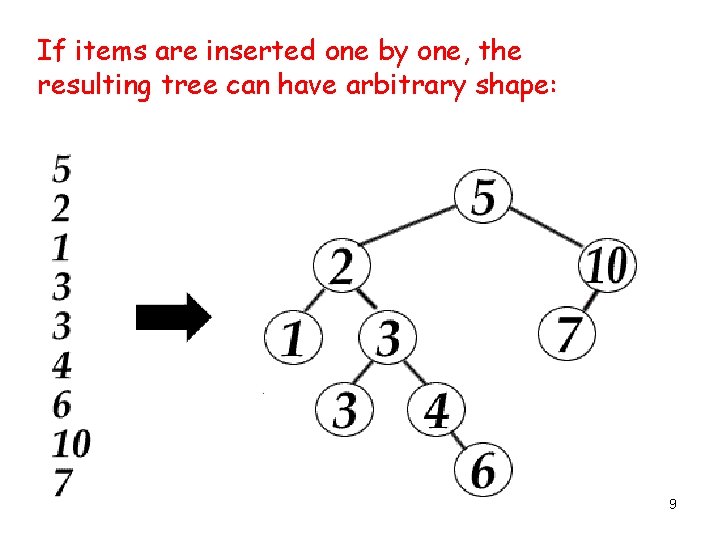
If items are inserted one by one, the resulting tree can have arbitrary shape: 9
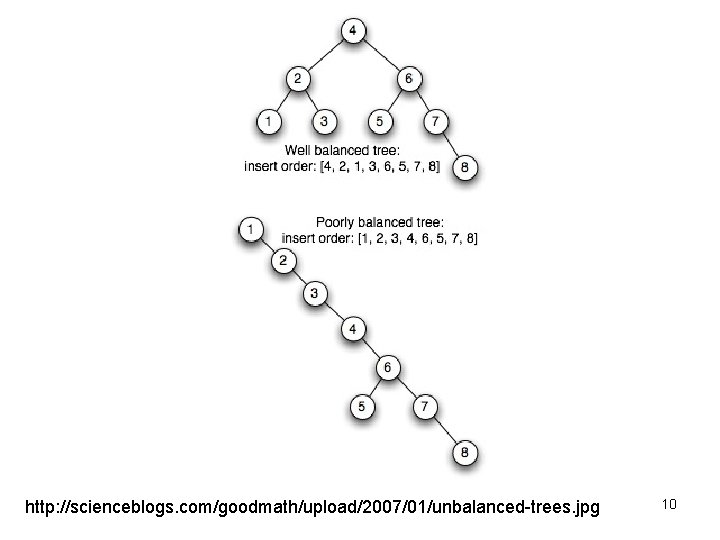
http: //scienceblogs. com/goodmath/upload/2007/01/unbalanced-trees. jpg 10
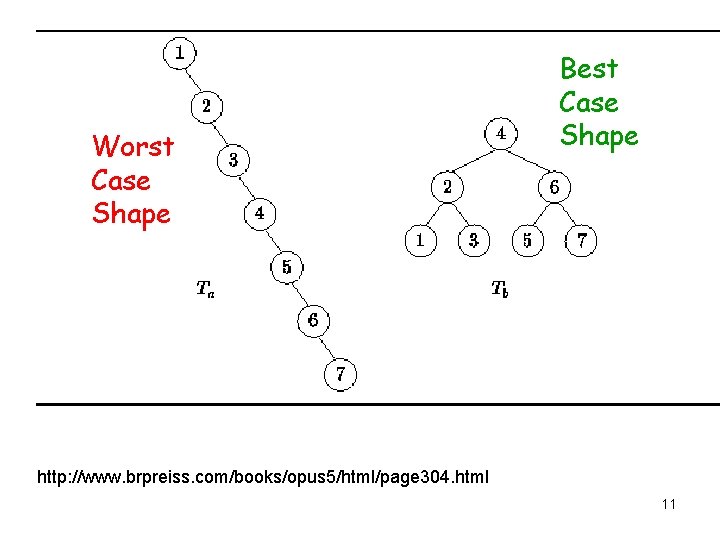
Worst Case Shape Best Case Shape http: //www. brpreiss. com/books/opus 5/html/page 304. html 11
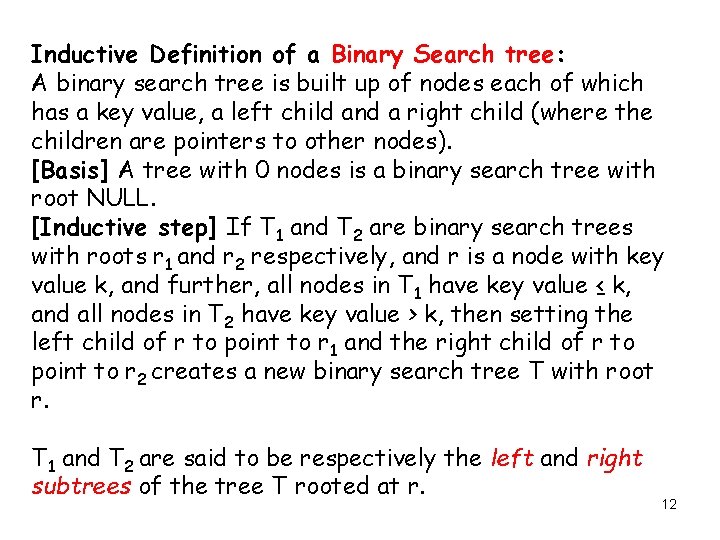
Inductive Definition of a Binary Search tree: A binary search tree is built up of nodes each of which has a key value, a left child and a right child (where the children are pointers to other nodes). [Basis] A tree with 0 nodes is a binary search tree with root NULL. [Inductive step] If T 1 and T 2 are binary search trees with roots r 1 and r 2 respectively, and r is a node with key value k, and further, all nodes in T 1 have key value ≤ k, and all nodes in T 2 have key value > k, then setting the left child of r to point to r 1 and the right child of r to point to r 2 creates a new binary search tree T with root r. T 1 and T 2 are said to be respectively the left and right subtrees of the tree T rooted at r. 12
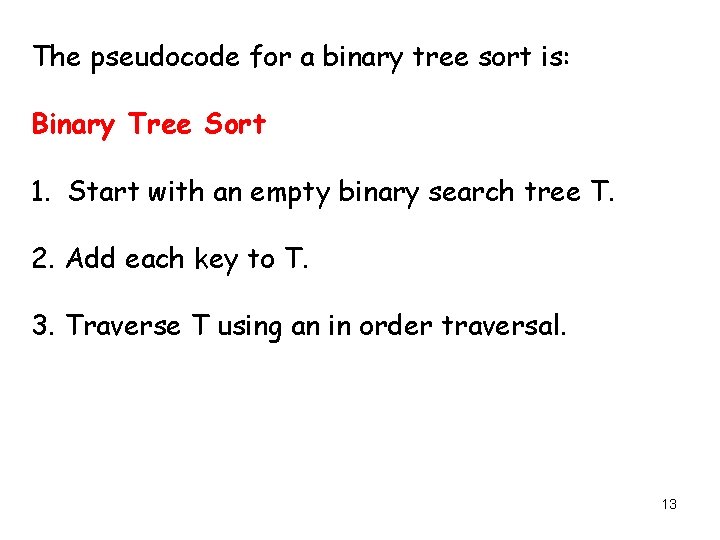
The pseudocode for a binary tree sort is: Binary Tree Sort 1. Start with an empty binary search tree T. 2. Add each key to T. 3. Traverse T using an in order traversal. 13
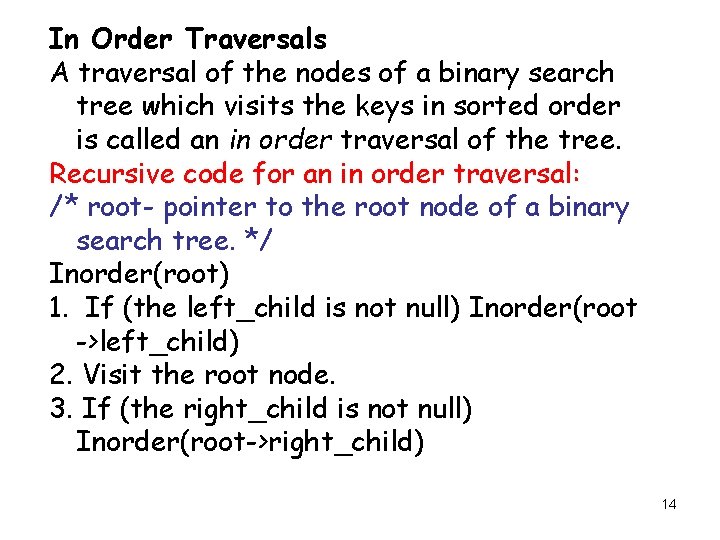
In Order Traversals A traversal of the nodes of a binary search tree which visits the keys in sorted order is called an in order traversal of the tree. Recursive code for an in order traversal: /* root- pointer to the root node of a binary search tree. */ Inorder(root) 1. If (the left_child is not null) Inorder(root ->left_child) 2. Visit the root node. 3. If (the right_child is not null) Inorder(root->right_child) 14
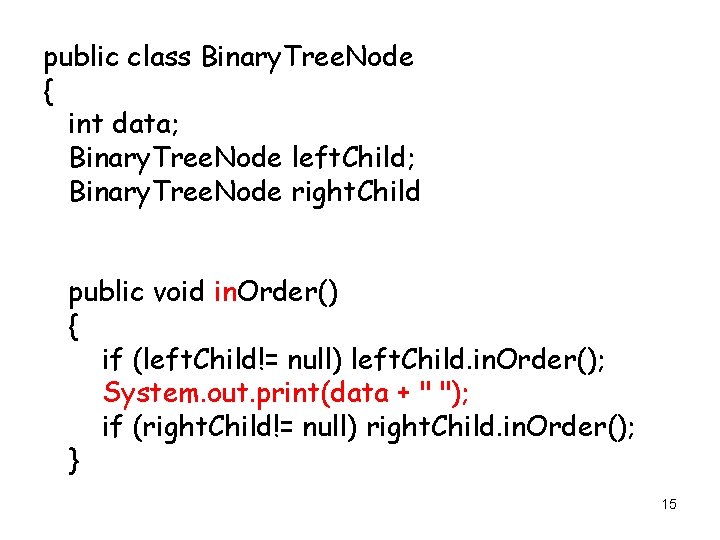
public class Binary. Tree. Node { int data; Binary. Tree. Node left. Child; Binary. Tree. Node right. Child public void in. Order() { if (left. Child!= null) left. Child. in. Order(); System. out. print(data + " "); if (right. Child!= null) right. Child. in. Order(); } 15
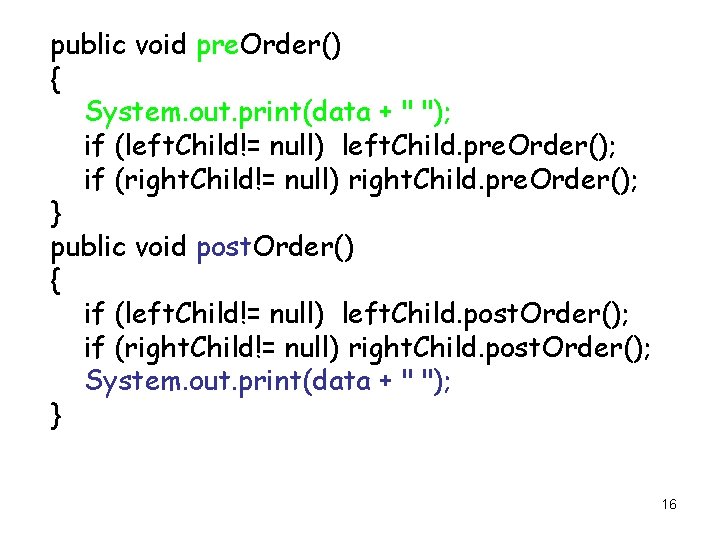
public void pre. Order() { System. out. print(data + " "); if (left. Child!= null) left. Child. pre. Order(); if (right. Child!= null) right. Child. pre. Order(); } public void post. Order() { if (left. Child!= null) left. Child. post. Order(); if (right. Child!= null) right. Child. post. Order(); System. out. print(data + " "); } 16
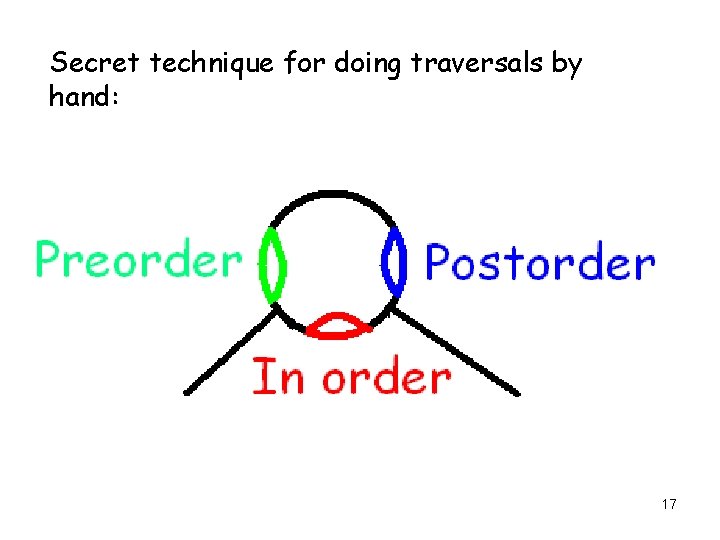
Secret technique for doing traversals by hand: 17
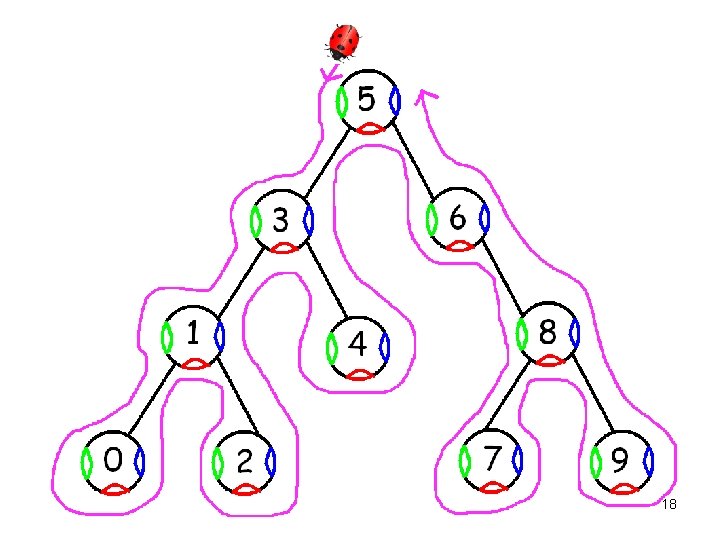
18
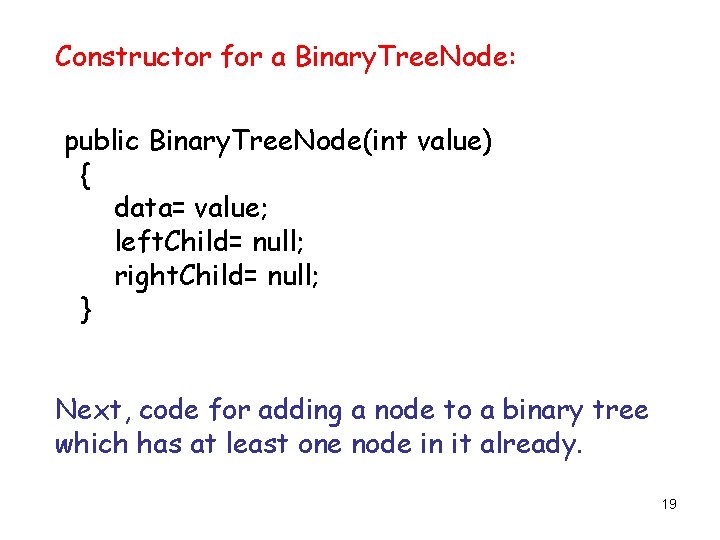
Constructor for a Binary. Tree. Node: public Binary. Tree. Node(int value) { data= value; left. Child= null; right. Child= null; } Next, code for adding a node to a binary tree which has at least one node in it already. 19
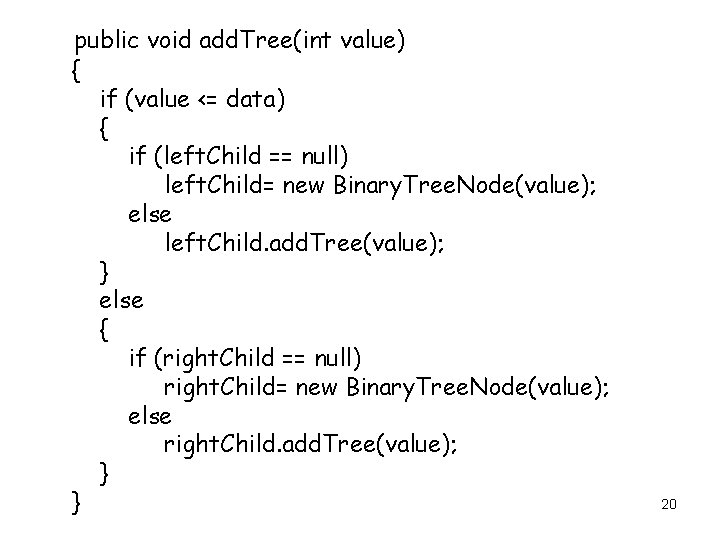
public void add. Tree(int value) { if (value <= data) { if (left. Child == null) left. Child= new Binary. Tree. Node(value); else left. Child. add. Tree(value); } else { if (right. Child == null) right. Child= new Binary. Tree. Node(value); else right. Child. add. Tree(value); } } 20
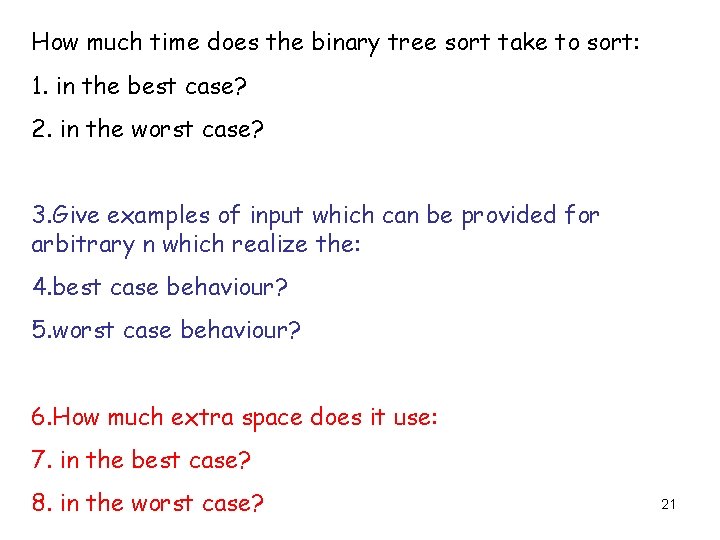
How much time does the binary tree sort take to sort: 1. in the best case? 2. in the worst case? 3. Give examples of input which can be provided for arbitrary n which realize the: 4. best case behaviour? 5. worst case behaviour? 6. How much extra space does it use: 7. in the best case? 8. in the worst case? 21
Pvu announcements
Kayl announcements
R/announcements!
General announcements
Church announcements
Fahrenheit 451 burning bright summary
Assignment due today
Blood supply of liver
Assignment due today
Assignment due today
Procedural due process vs substantive due process
Slidetodoc
Due piccole sfere identiche sono sospese a due punti
Le diagonali del parallelogramma sono perpendicolari
Office operations
Posted
Look mom europe
Errors discovered after an entry is posted
Post the journal’s special amount column totals.
The only completely reliable backflow prevention
Signage posted at a handwashing station must include
Jhlt. 2019 oct; 38(10): 1015-1066