Advanced Programming in Java Peyman Dodangeh Sharif University
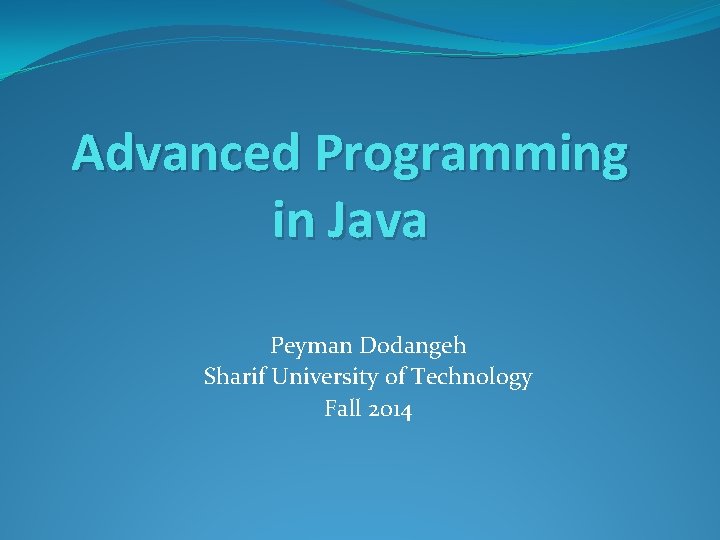
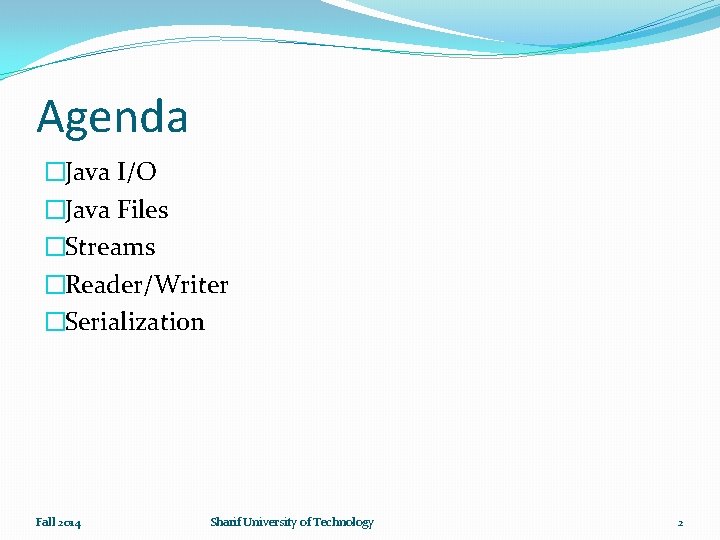
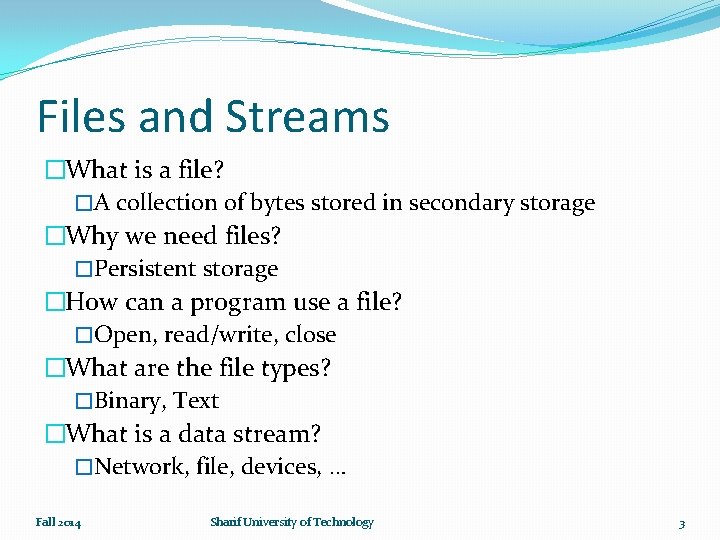
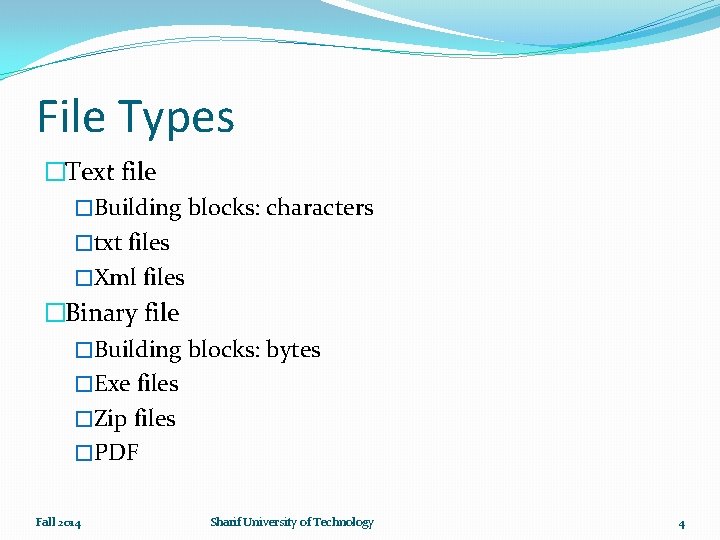
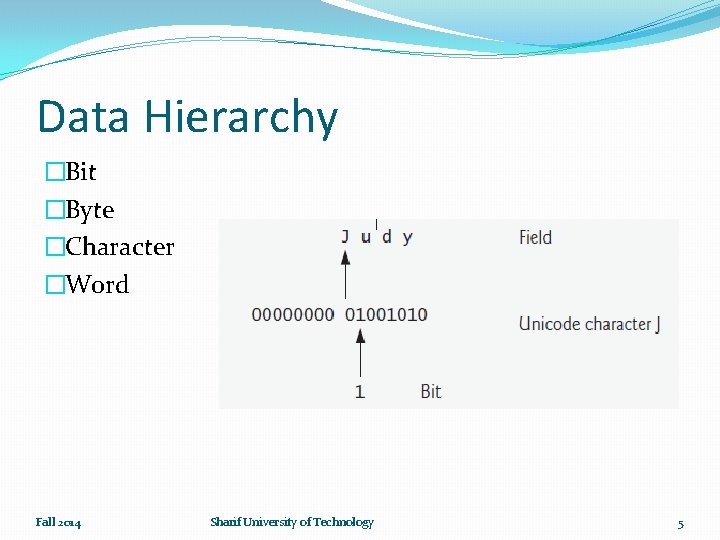
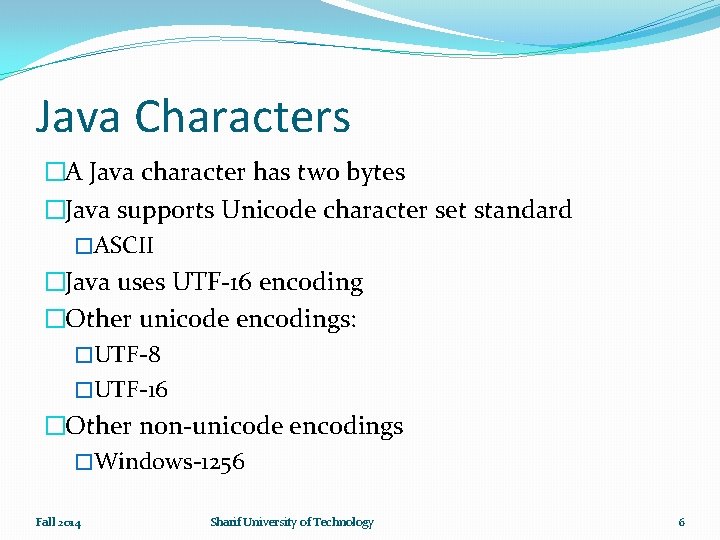
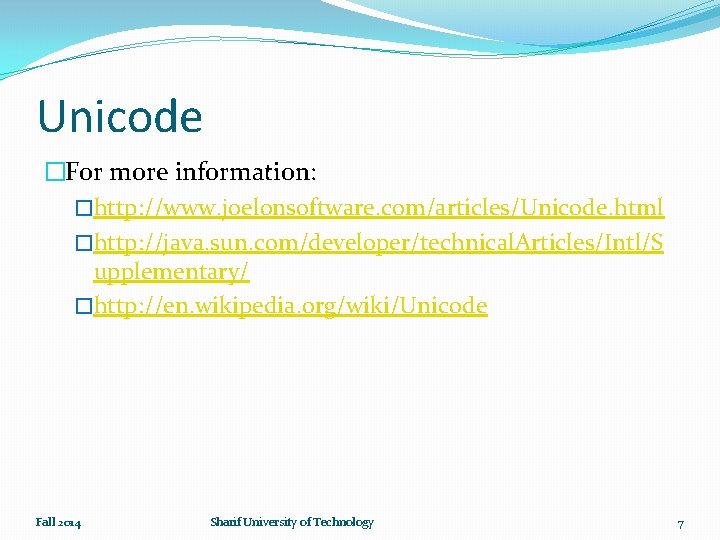
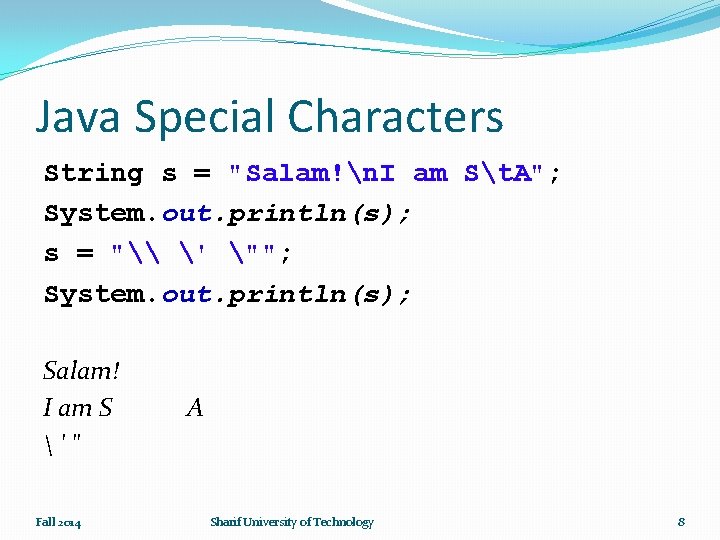
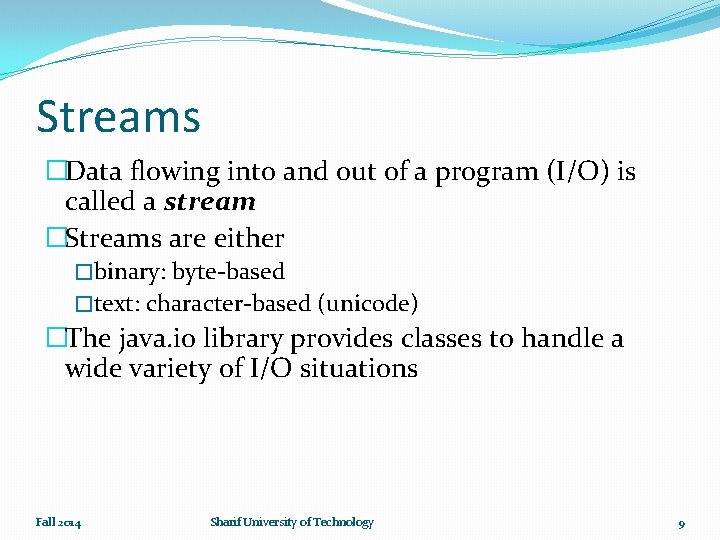
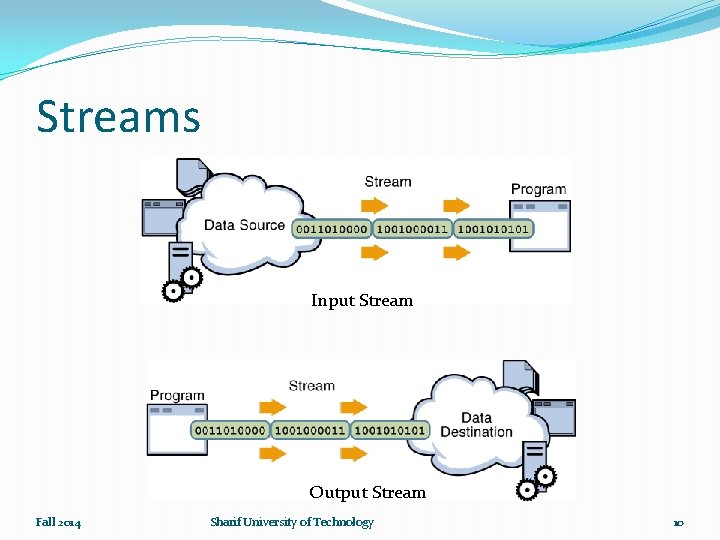
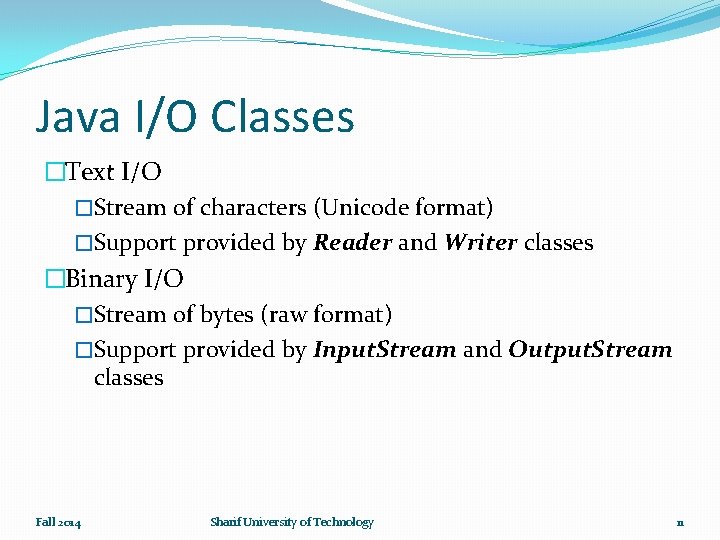
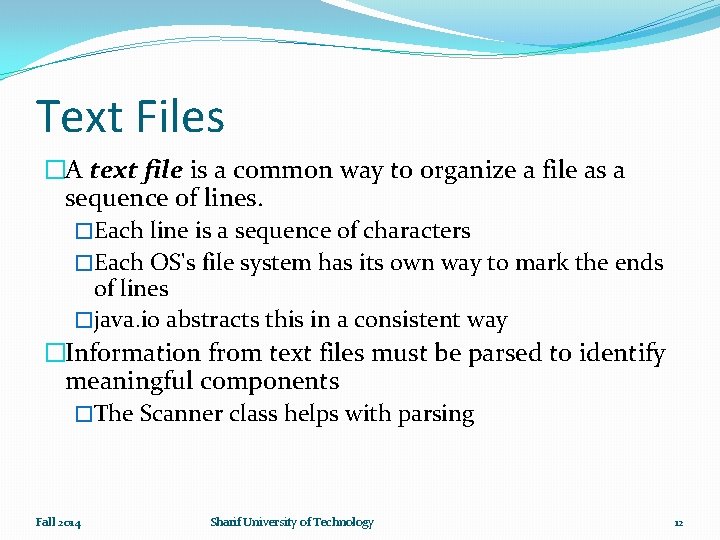
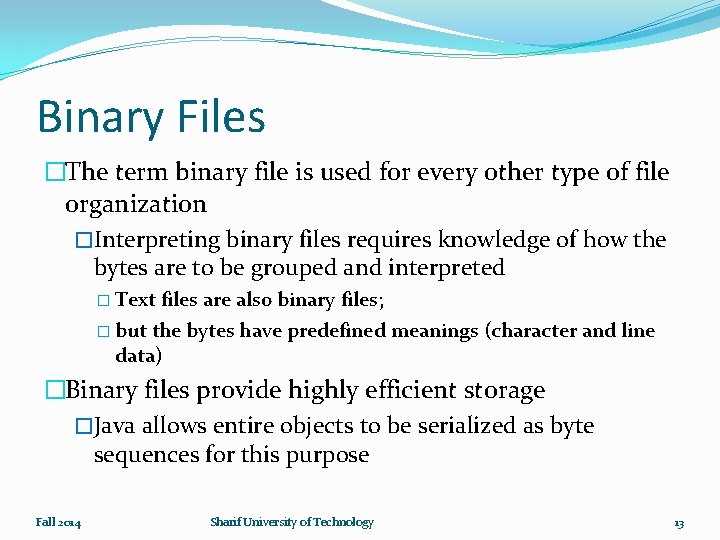
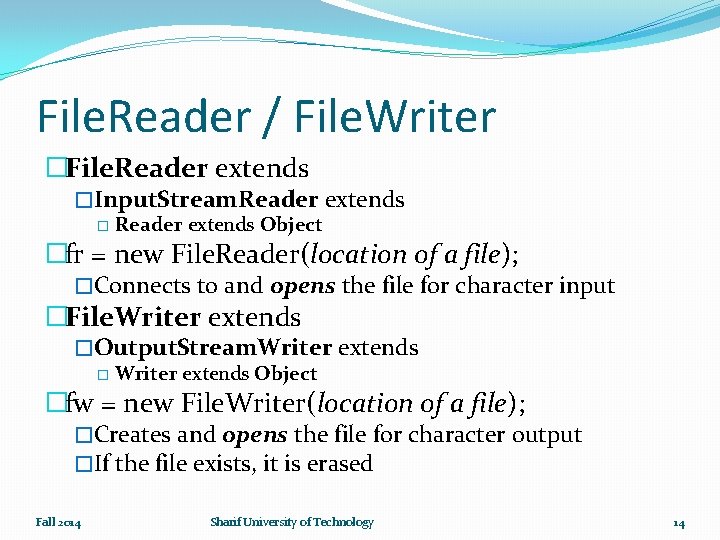
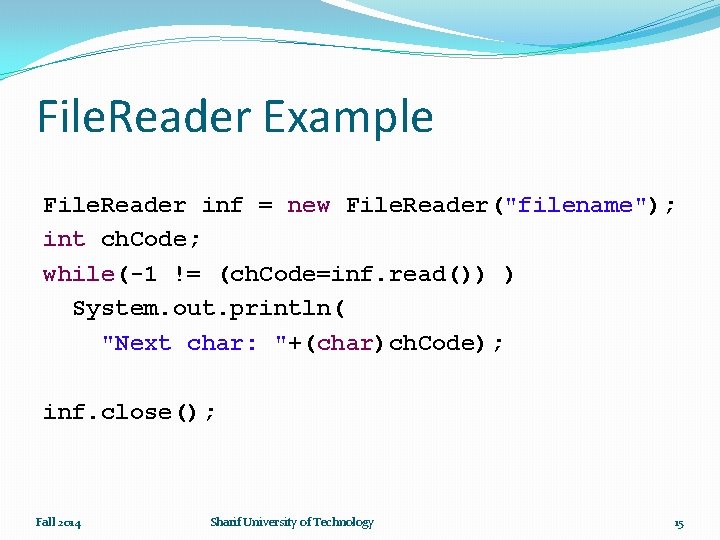
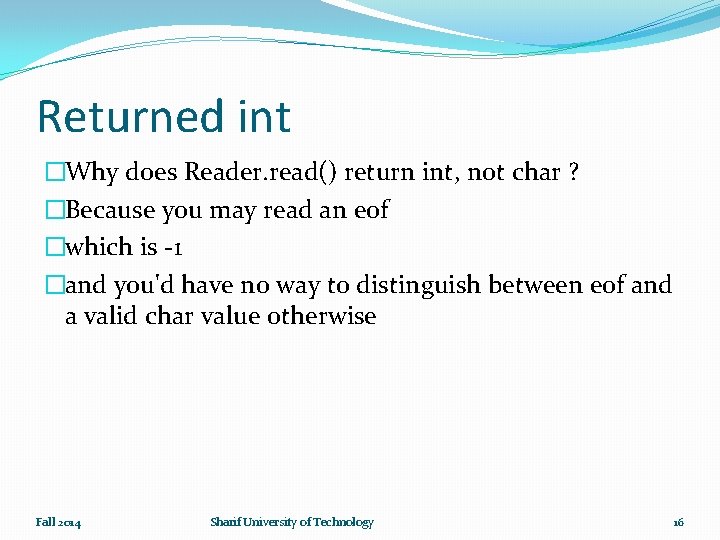
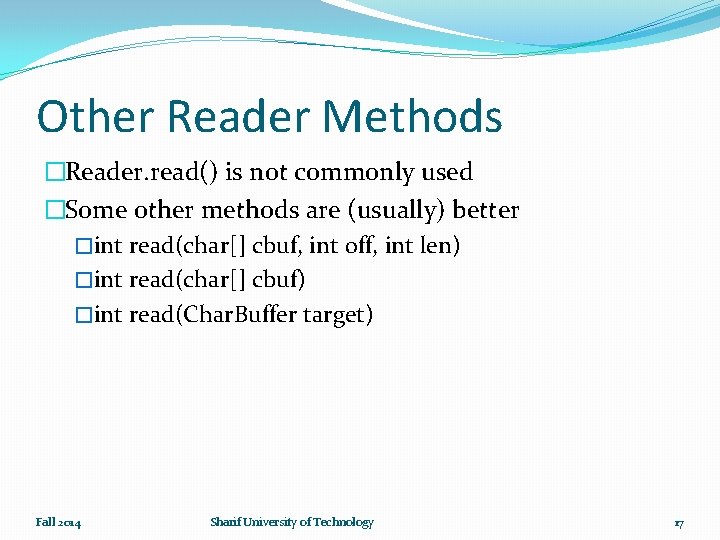
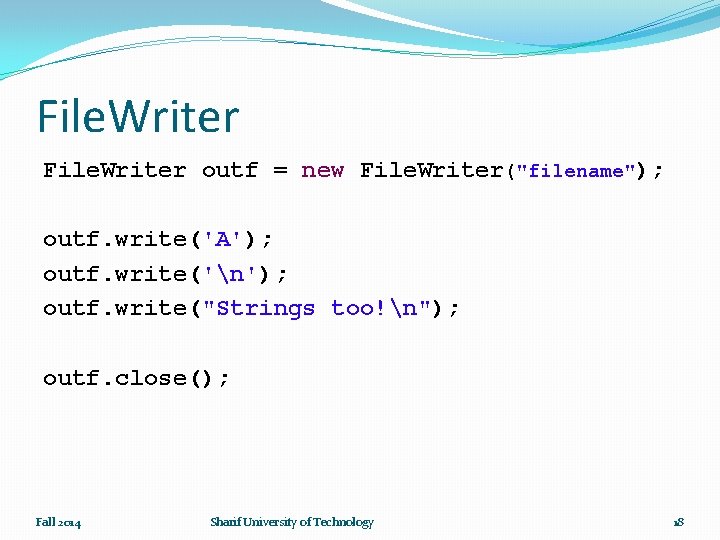
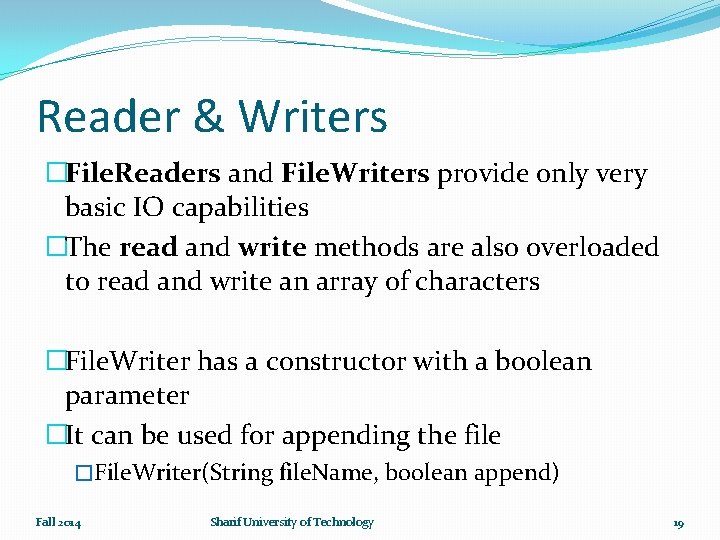
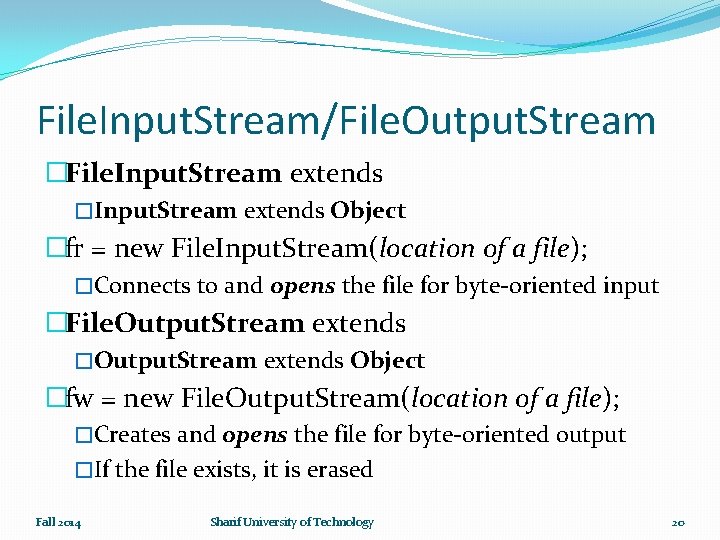
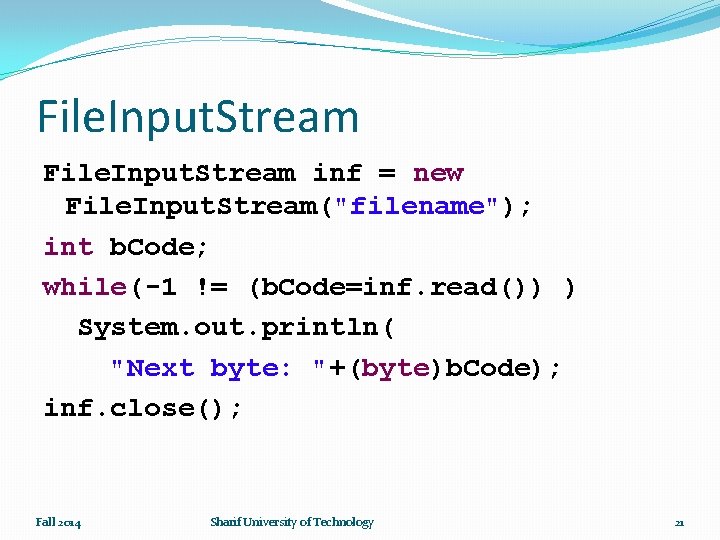
![�Some other Input. Stream methods: �int read(byte b[]) �int read(byte b[], int off, int �Some other Input. Stream methods: �int read(byte b[]) �int read(byte b[], int off, int](https://slidetodoc.com/presentation_image_h2/06fd402e600ddfc1303be333dc25e602/image-22.jpg)
![File. Output. Stream outf = new File. Output. Stream("filename"); byte[] out = {52, 99, File. Output. Stream outf = new File. Output. Stream("filename"); byte[] out = {52, 99,](https://slidetodoc.com/presentation_image_h2/06fd402e600ddfc1303be333dc25e602/image-23.jpg)
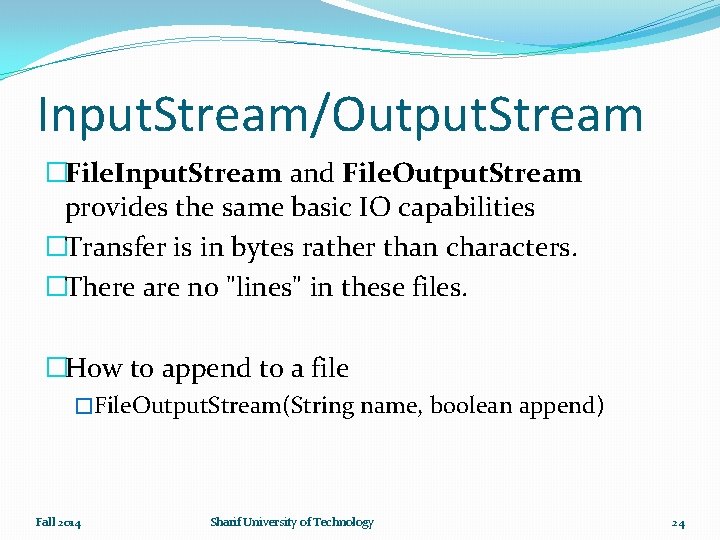
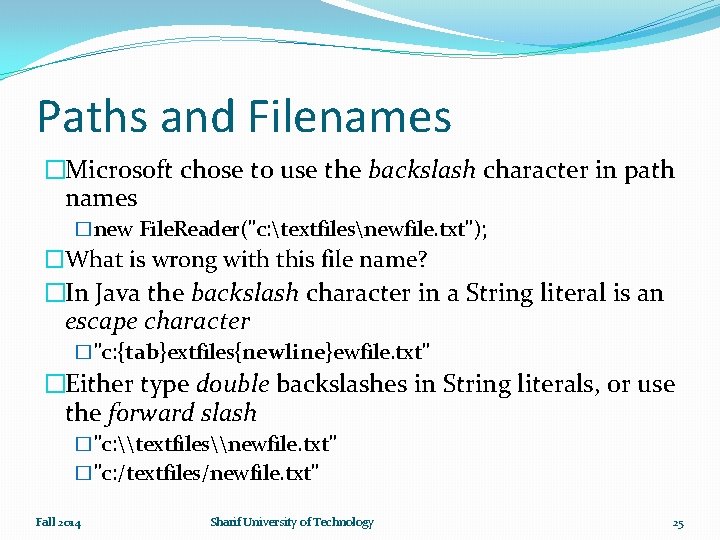
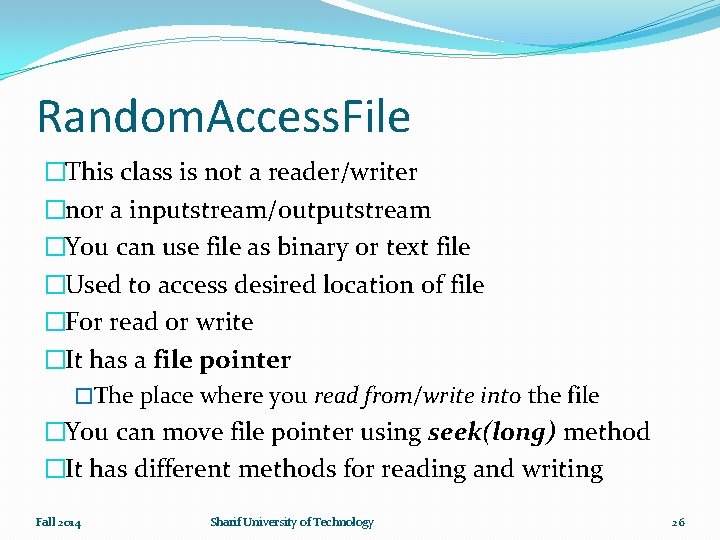
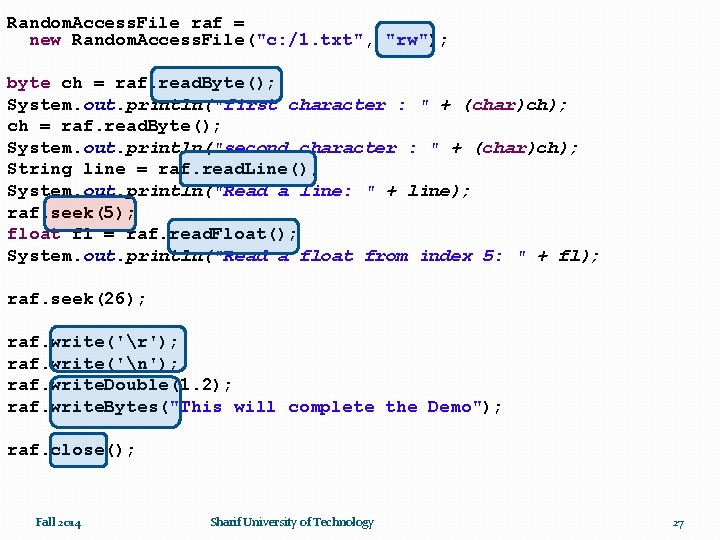
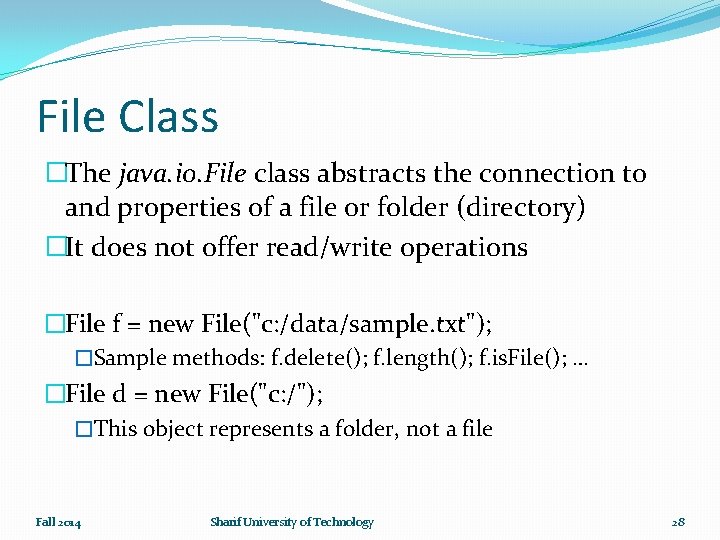
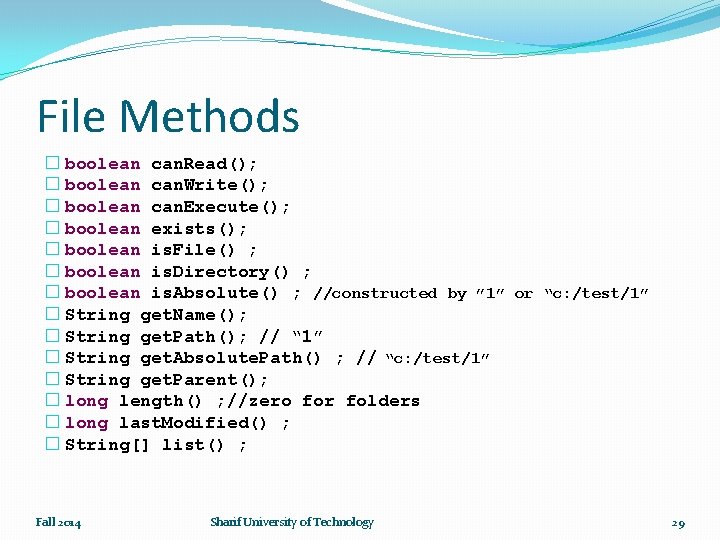
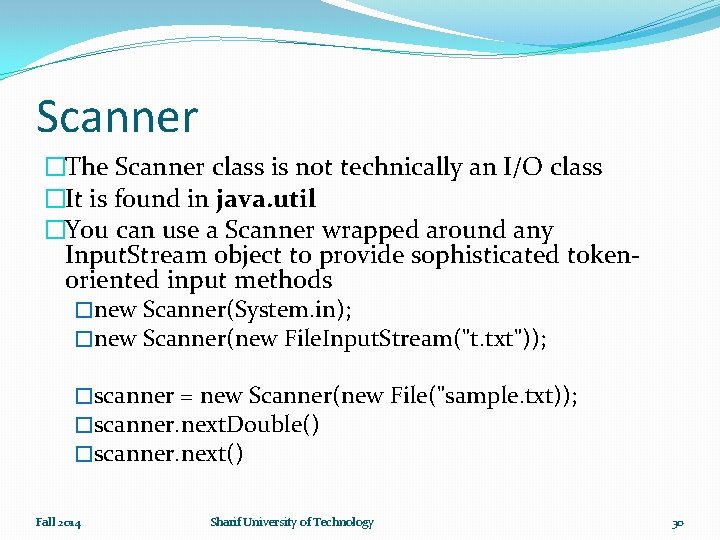
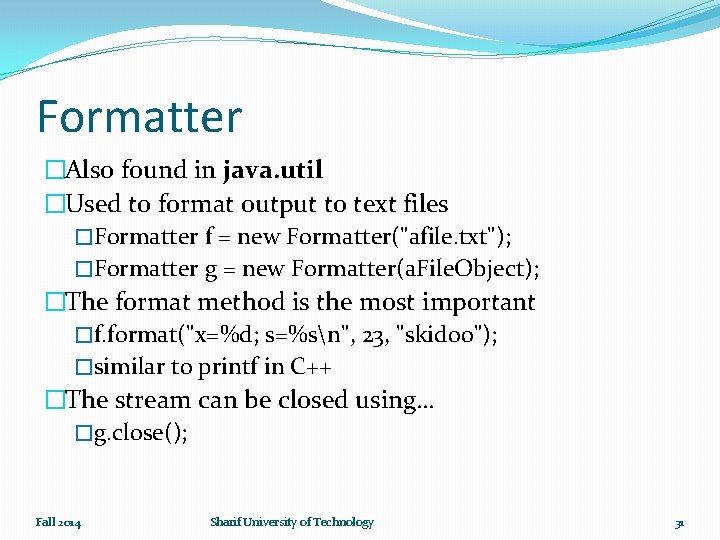
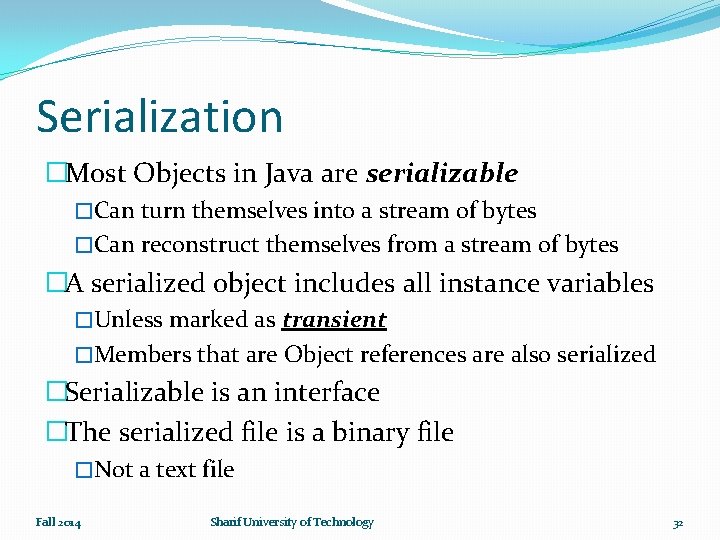
![public class Student implements Serializable{ private String name; private String student. ID; private double[] public class Student implements Serializable{ private String name; private String student. ID; private double[]](https://slidetodoc.com/presentation_image_h2/06fd402e600ddfc1303be333dc25e602/image-33.jpg)
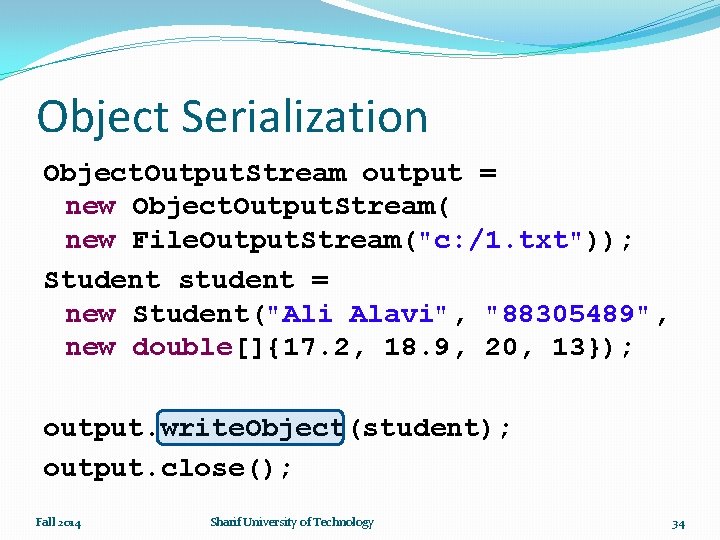
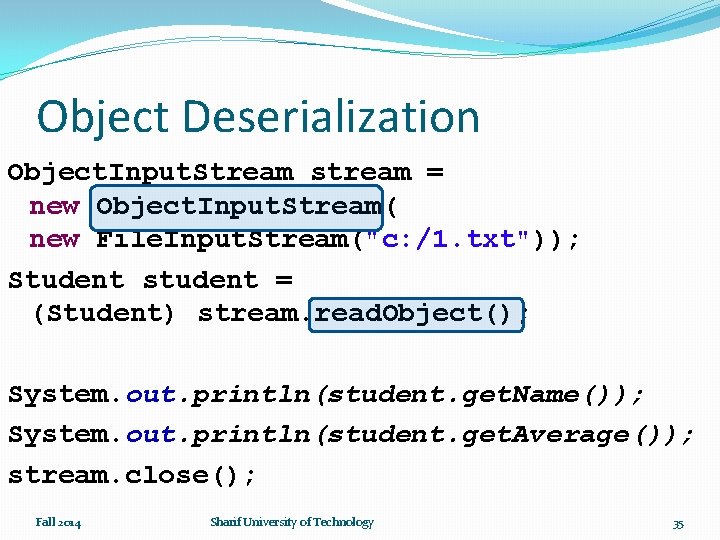
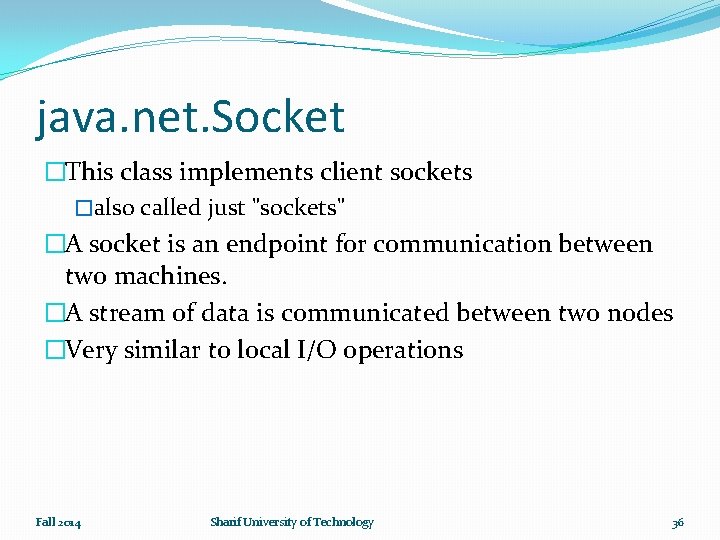
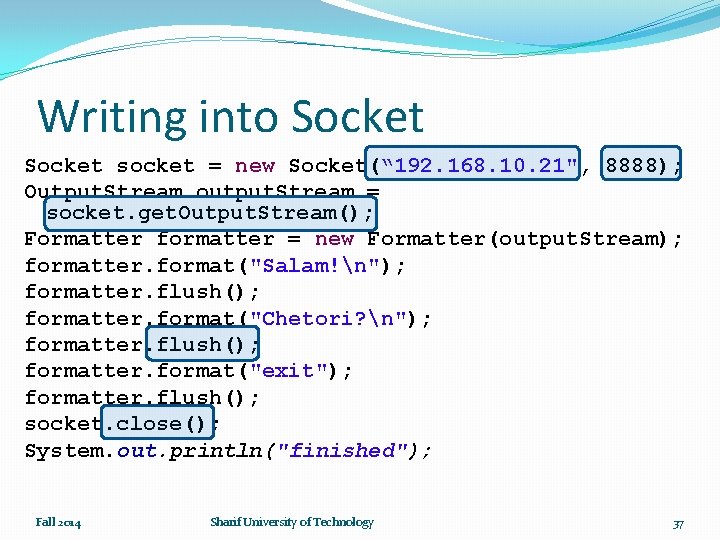
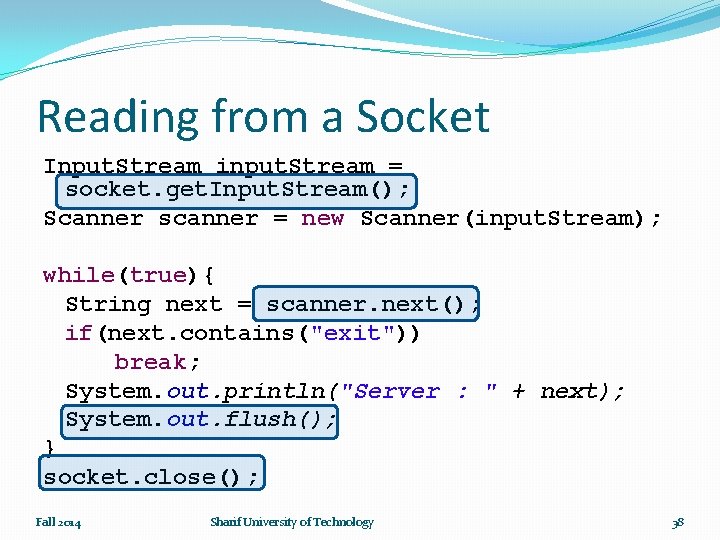
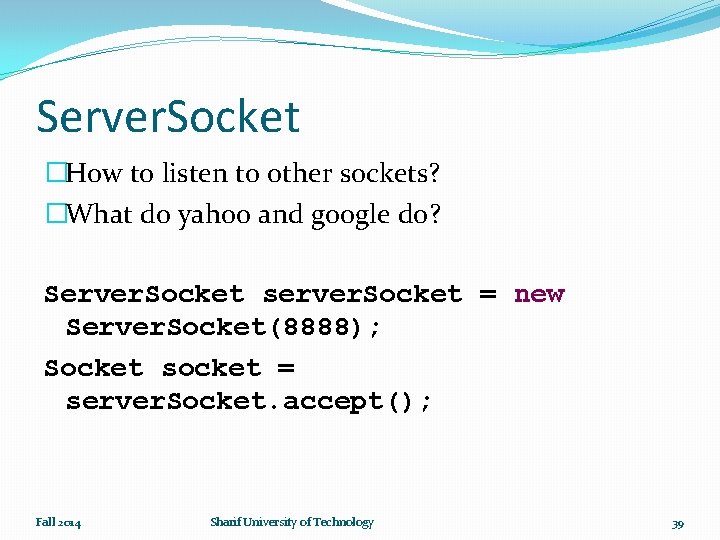
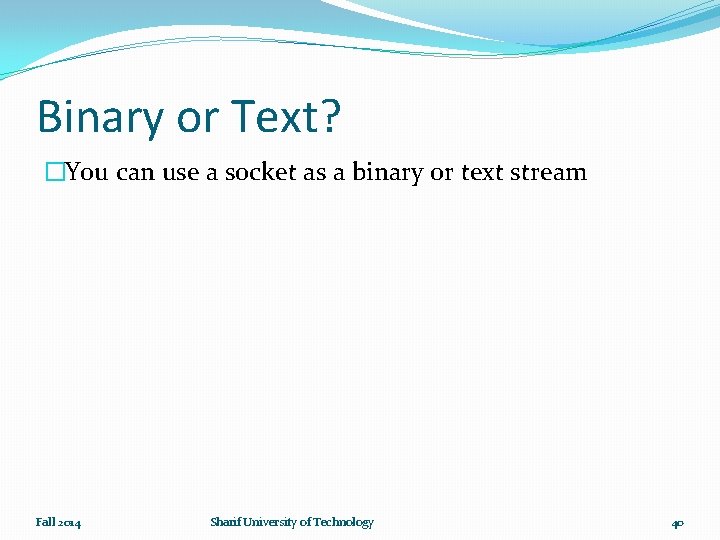
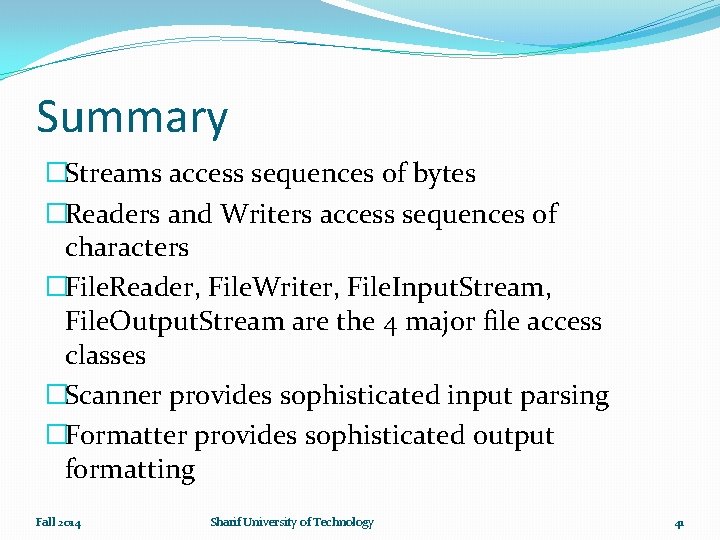
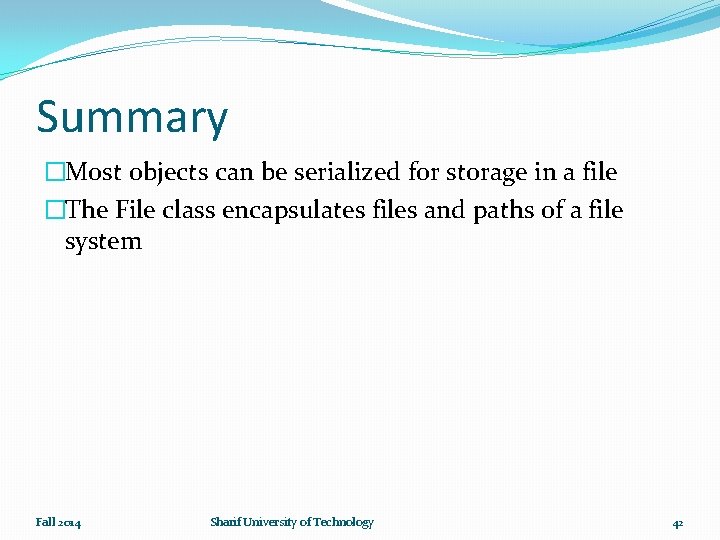
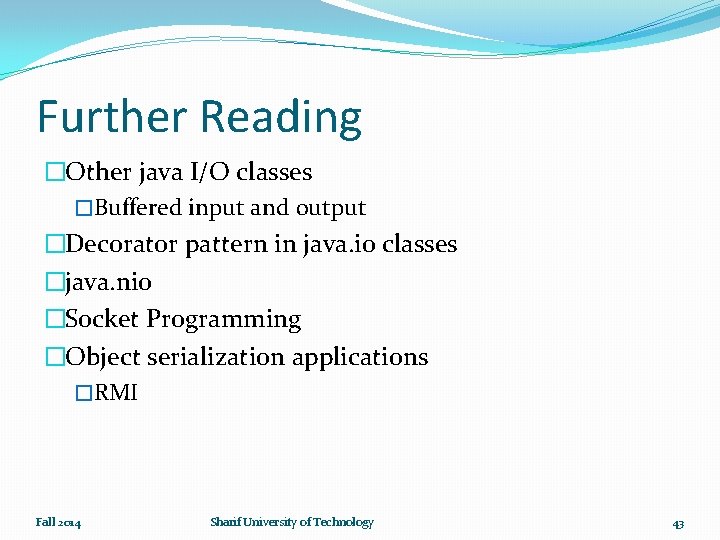
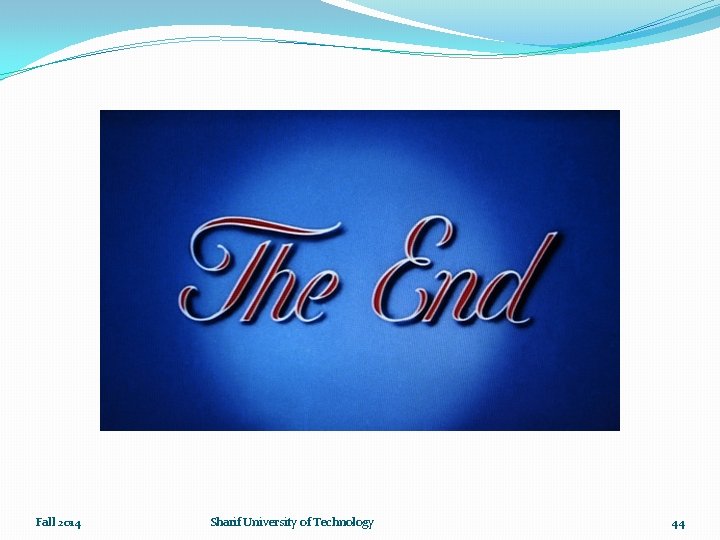
- Slides: 44
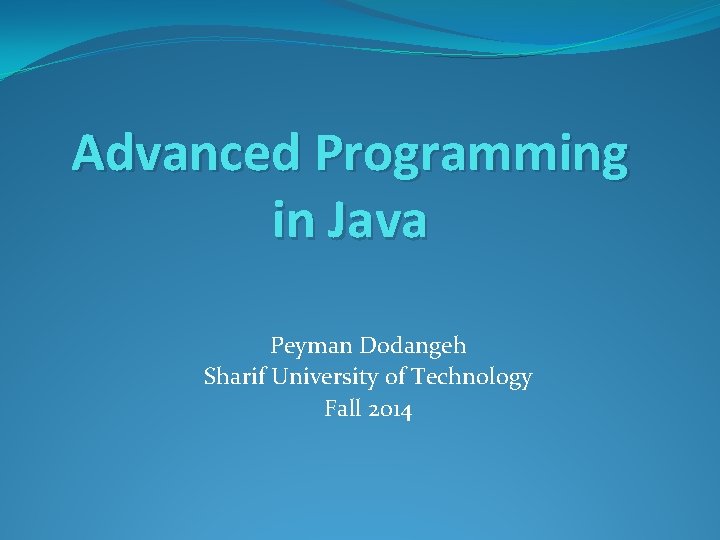
Advanced Programming in Java Peyman Dodangeh Sharif University of Technology Fall 2014
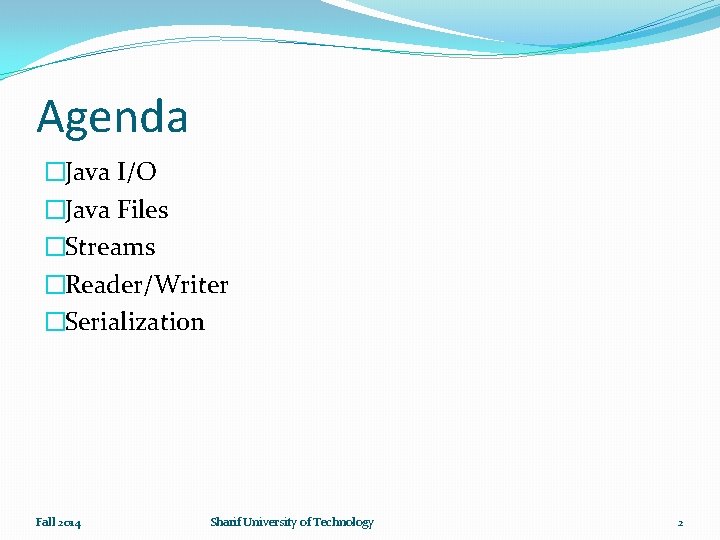
Agenda �Java I/O �Java Files �Streams �Reader/Writer �Serialization Fall 2014 Sharif University of Technology 2
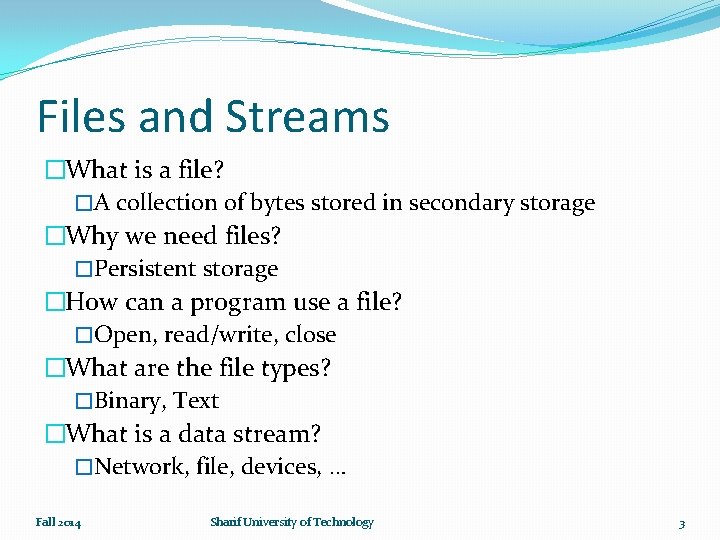
Files and Streams �What is a file? �A collection of bytes stored in secondary storage �Why we need files? �Persistent storage �How can a program use a file? �Open, read/write, close �What are the file types? �Binary, Text �What is a data stream? �Network, file, devices, … Fall 2014 Sharif University of Technology 3
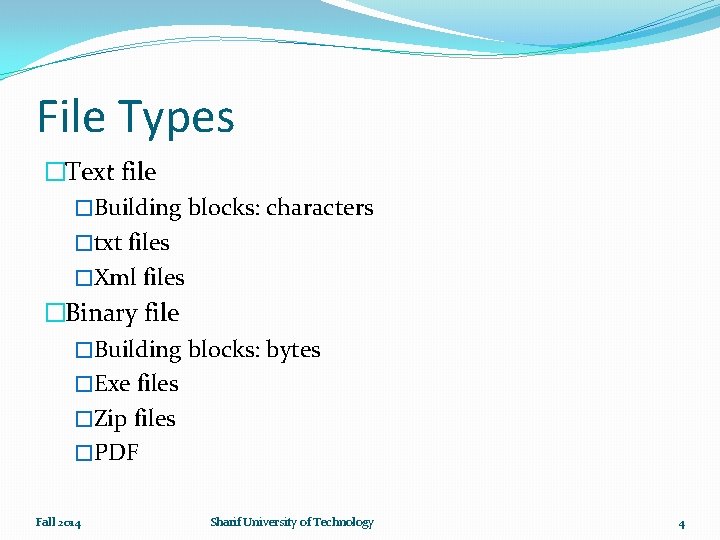
File Types �Text file �Building blocks: characters �txt files �Xml files �Binary file �Building blocks: bytes �Exe files �Zip files �PDF Fall 2014 Sharif University of Technology 4
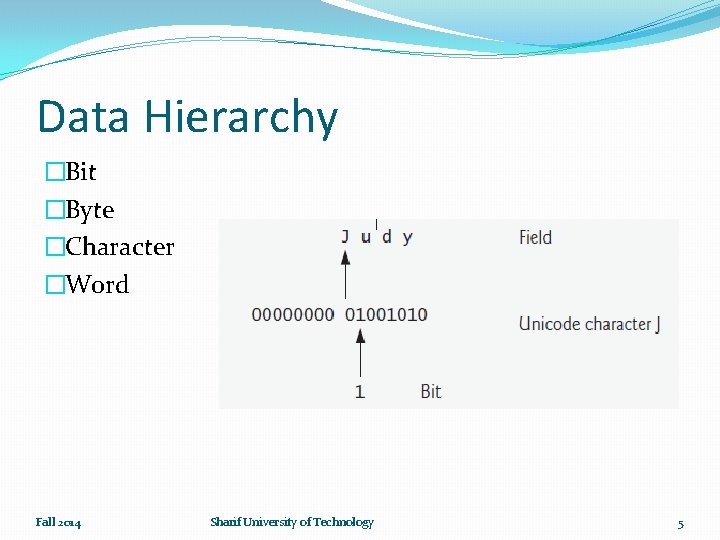
Data Hierarchy �Bit �Byte �Character �Word Fall 2014 Sharif University of Technology 5
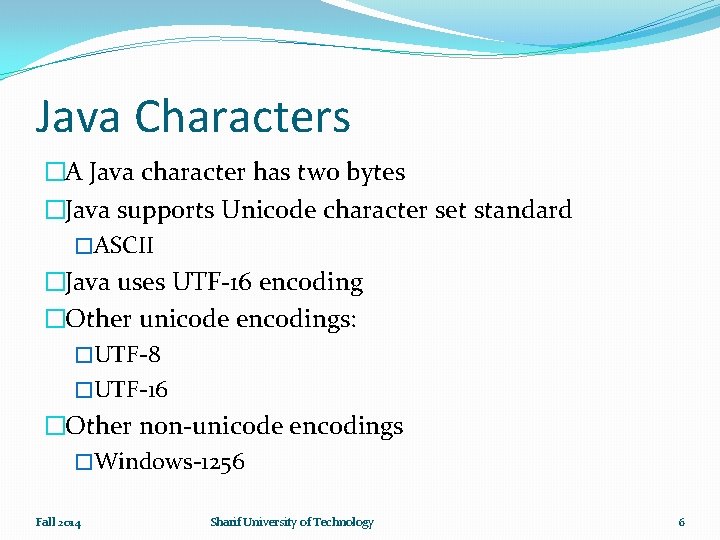
Java Characters �A Java character has two bytes �Java supports Unicode character set standard �ASCII �Java uses UTF-16 encoding �Other unicode encodings: �UTF-8 �UTF-16 �Other non-unicode encodings �Windows-1256 Fall 2014 Sharif University of Technology 6
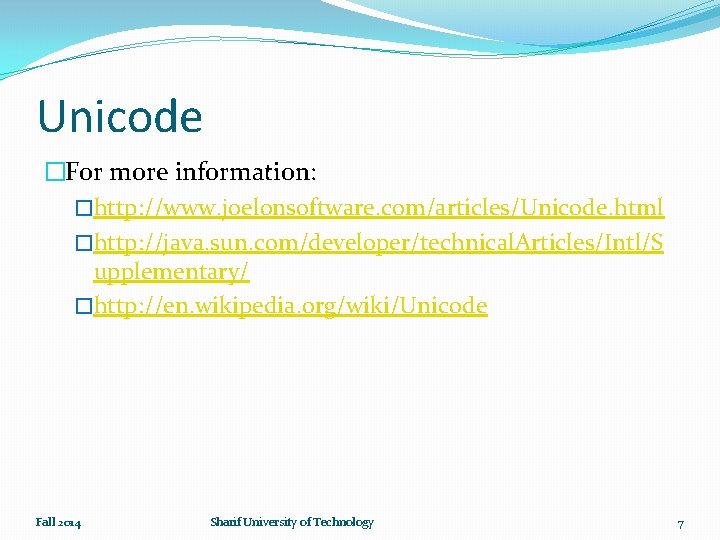
Unicode �For more information: �http: //www. joelonsoftware. com/articles/Unicode. html �http: //java. sun. com/developer/technical. Articles/Intl/S upplementary/ �http: //en. wikipedia. org/wiki/Unicode Fall 2014 Sharif University of Technology 7
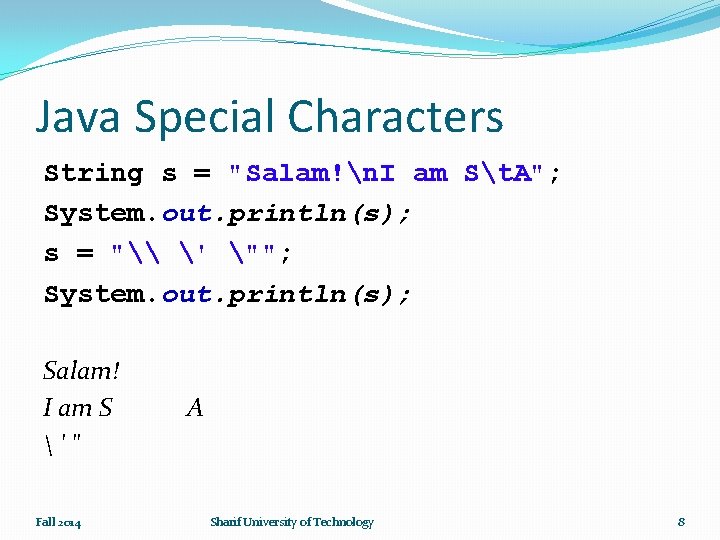
Java Special Characters String s = "Salam!n. I am St. A"; System. out. println(s); s = "\ ' ""; System. out. println(s); Salam! I am S '" Fall 2014 A Sharif University of Technology 8
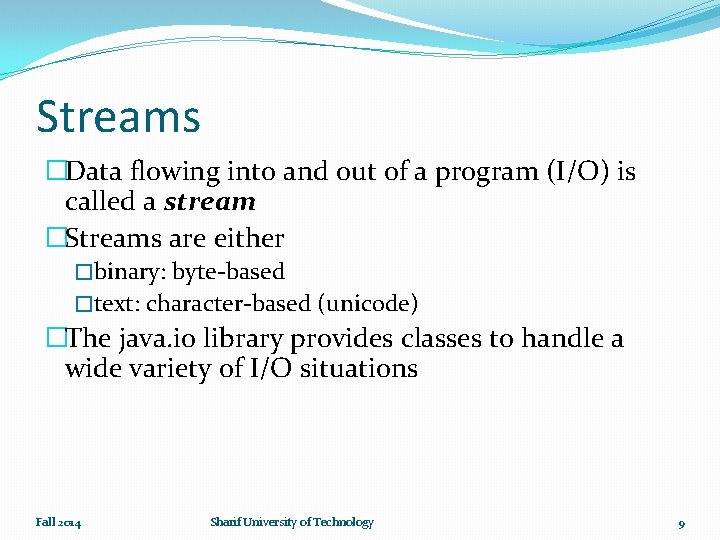
Streams �Data flowing into and out of a program (I/O) is called a stream �Streams are either �binary: byte-based �text: character-based (unicode) �The java. io library provides classes to handle a wide variety of I/O situations Fall 2014 Sharif University of Technology 9
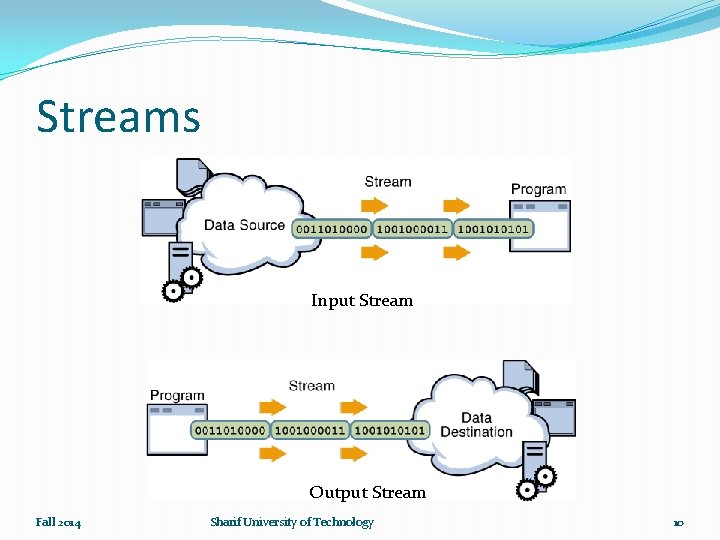
Streams Input Stream Output Stream Fall 2014 Sharif University of Technology 10
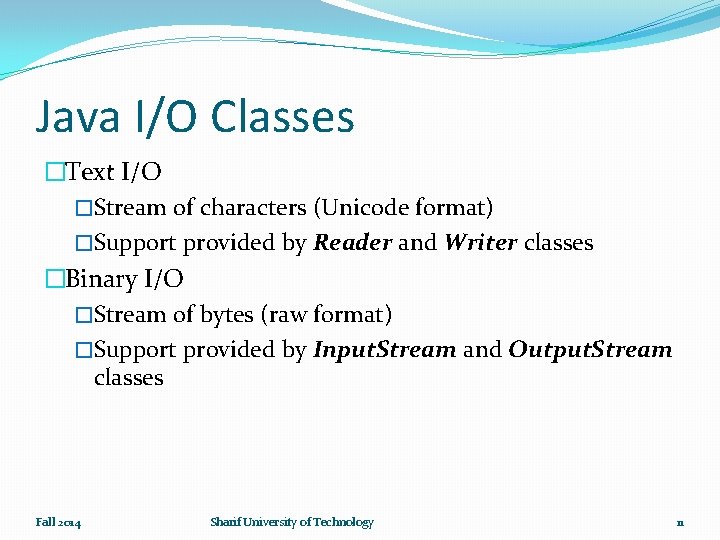
Java I/O Classes �Text I/O �Stream of characters (Unicode format) �Support provided by Reader and Writer classes �Binary I/O �Stream of bytes (raw format) �Support provided by Input. Stream and Output. Stream classes Fall 2014 Sharif University of Technology 11
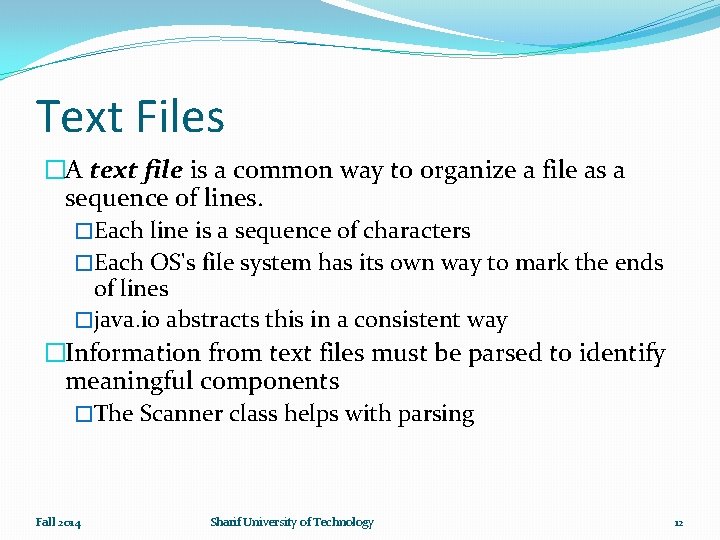
Text Files �A text file is a common way to organize a file as a sequence of lines. �Each line is a sequence of characters �Each OS's file system has its own way to mark the ends of lines �java. io abstracts this in a consistent way �Information from text files must be parsed to identify meaningful components �The Scanner class helps with parsing Fall 2014 Sharif University of Technology 12
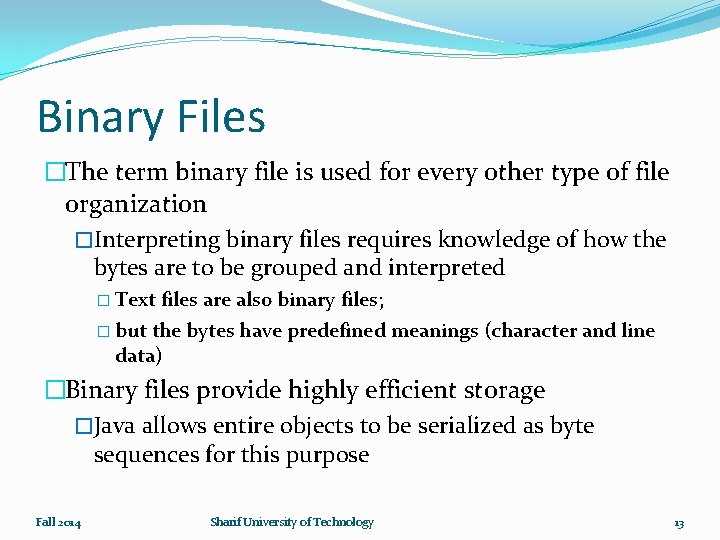
Binary Files �The term binary file is used for every other type of file organization �Interpreting binary files requires knowledge of how the bytes are to be grouped and interpreted � Text files are also binary files; � but the bytes have predefined meanings (character and line data) �Binary files provide highly efficient storage �Java allows entire objects to be serialized as byte sequences for this purpose Fall 2014 Sharif University of Technology 13
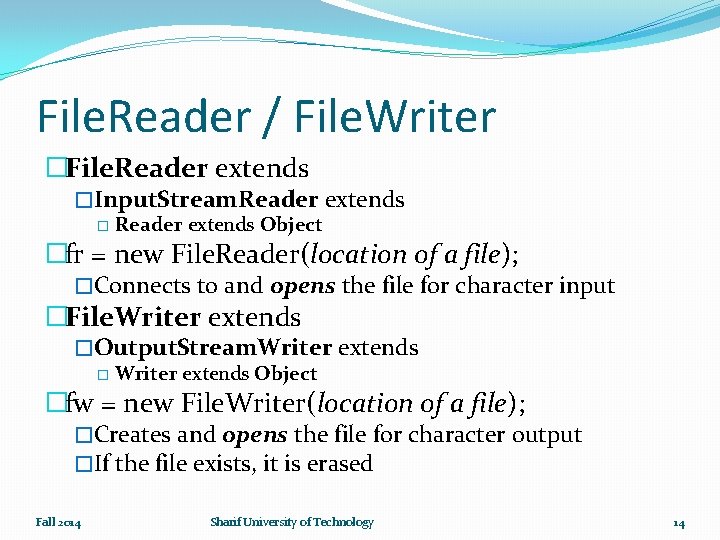
File. Reader / File. Writer �File. Reader extends �Input. Stream. Reader extends � Reader extends Object �fr = new File. Reader(location of a file); �Connects to and opens the file for character input �File. Writer extends �Output. Stream. Writer extends � Writer extends Object �fw = new File. Writer(location of a file); �Creates and opens the file for character output �If the file exists, it is erased Fall 2014 Sharif University of Technology 14
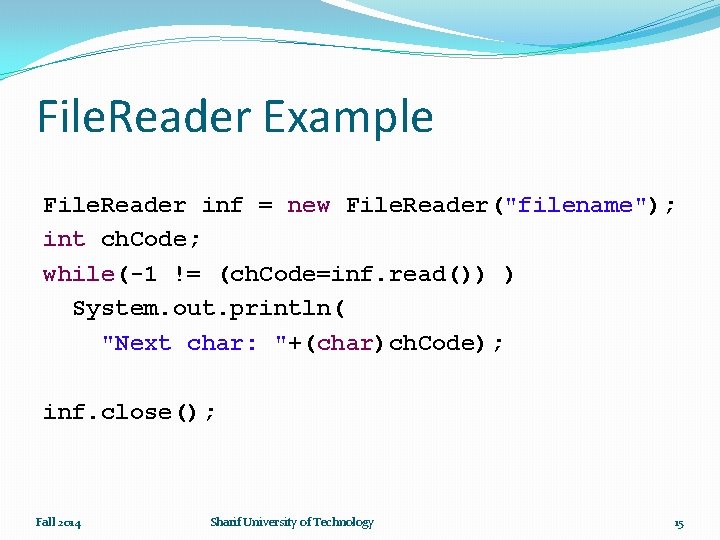
File. Reader Example File. Reader inf = new File. Reader("filename"); int ch. Code; while(-1 != (ch. Code=inf. read()) ) System. out. println( "Next char: "+(char)ch. Code); inf. close(); Fall 2014 Sharif University of Technology 15
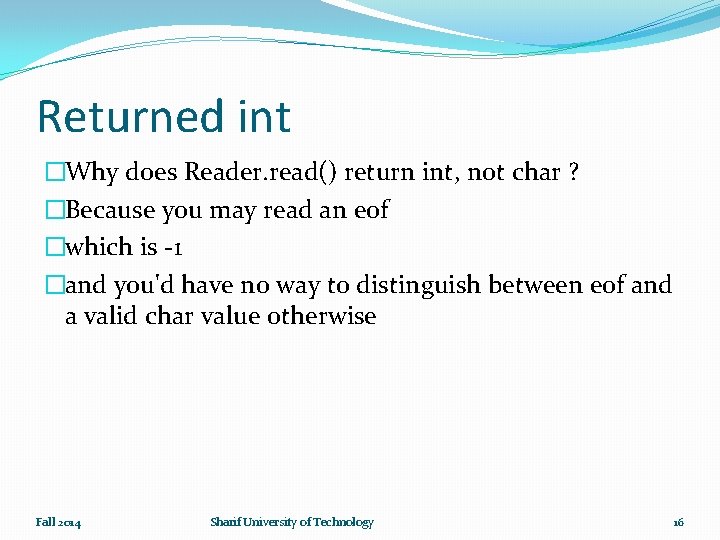
Returned int �Why does Reader. read() return int, not char ? �Because you may read an eof �which is -1 �and you'd have no way to distinguish between eof and a valid char value otherwise Fall 2014 Sharif University of Technology 16
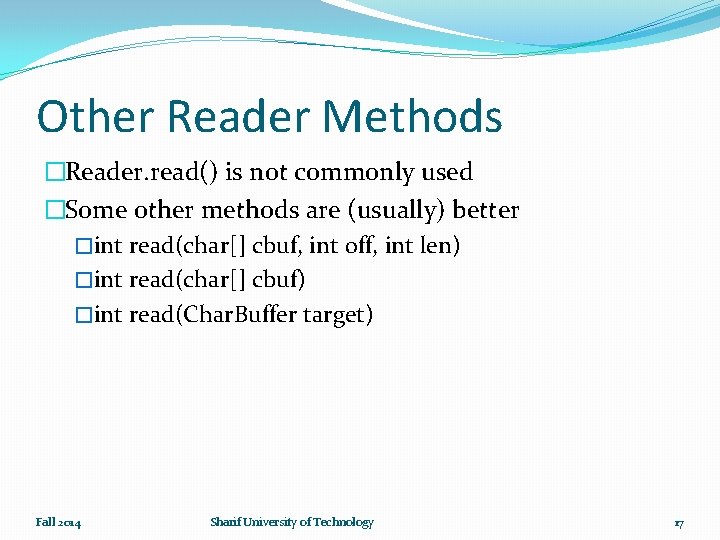
Other Reader Methods �Reader. read() is not commonly used �Some other methods are (usually) better �int read(char[] cbuf, int off, int len) �int read(char[] cbuf) �int read(Char. Buffer target) Fall 2014 Sharif University of Technology 17
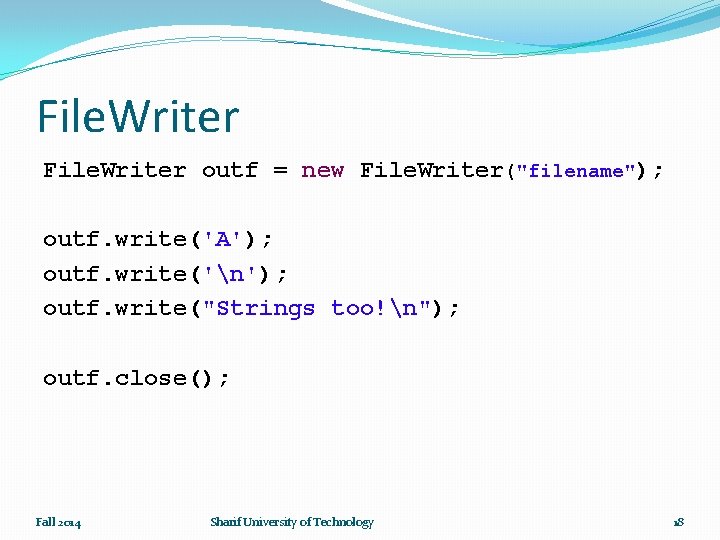
File. Writer outf = new File. Writer("filename"); outf. write('A'); outf. write('n'); outf. write("Strings too!n"); outf. close(); Fall 2014 Sharif University of Technology 18
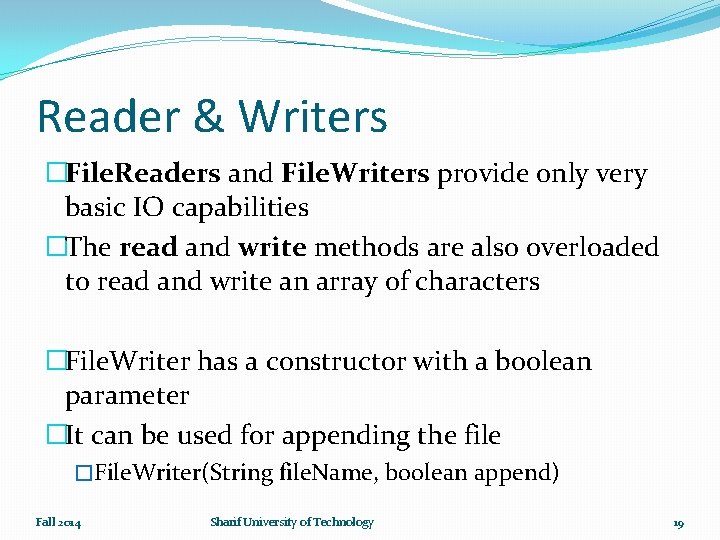
Reader & Writers �File. Readers and File. Writers provide only very basic IO capabilities �The read and write methods are also overloaded to read and write an array of characters �File. Writer has a constructor with a boolean parameter �It can be used for appending the file �File. Writer(String file. Name, boolean append) Fall 2014 Sharif University of Technology 19
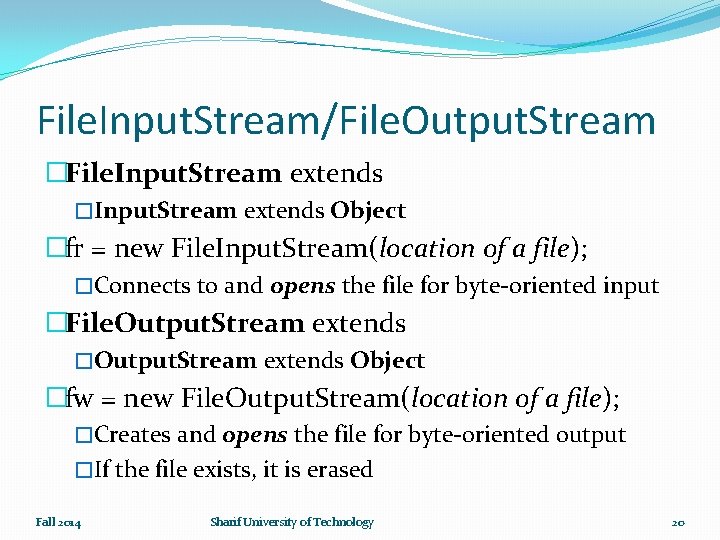
File. Input. Stream/File. Output. Stream �File. Input. Stream extends �Input. Stream extends Object �fr = new File. Input. Stream(location of a file); �Connects to and opens the file for byte-oriented input �File. Output. Stream extends �Output. Stream extends Object �fw = new File. Output. Stream(location of a file); �Creates and opens the file for byte-oriented output �If the file exists, it is erased Fall 2014 Sharif University of Technology 20
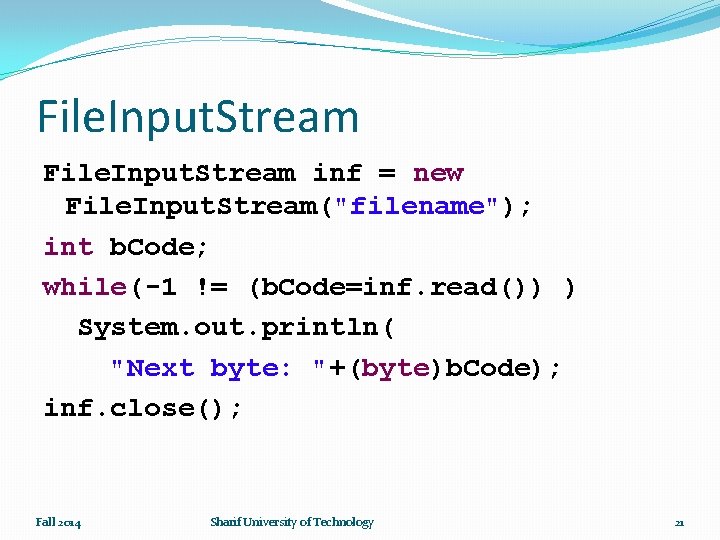
File. Input. Stream inf = new File. Input. Stream("filename"); int b. Code; while(-1 != (b. Code=inf. read()) ) System. out. println( "Next byte: "+(byte)b. Code); inf. close(); Fall 2014 Sharif University of Technology 21
![Some other Input Stream methods int readbyte b int readbyte b int off int �Some other Input. Stream methods: �int read(byte b[]) �int read(byte b[], int off, int](https://slidetodoc.com/presentation_image_h2/06fd402e600ddfc1303be333dc25e602/image-22.jpg)
�Some other Input. Stream methods: �int read(byte b[]) �int read(byte b[], int off, int len) Fall 2014 Sharif University of Technology 22
![File Output Stream outf new File Output Streamfilename byte out 52 99 File. Output. Stream outf = new File. Output. Stream("filename"); byte[] out = {52, 99,](https://slidetodoc.com/presentation_image_h2/06fd402e600ddfc1303be333dc25e602/image-23.jpg)
File. Output. Stream outf = new File. Output. Stream("filename"); byte[] out = {52, 99, 13, 10}; outf. write(out); outf. close(); Fall 2014 Sharif University of Technology 23
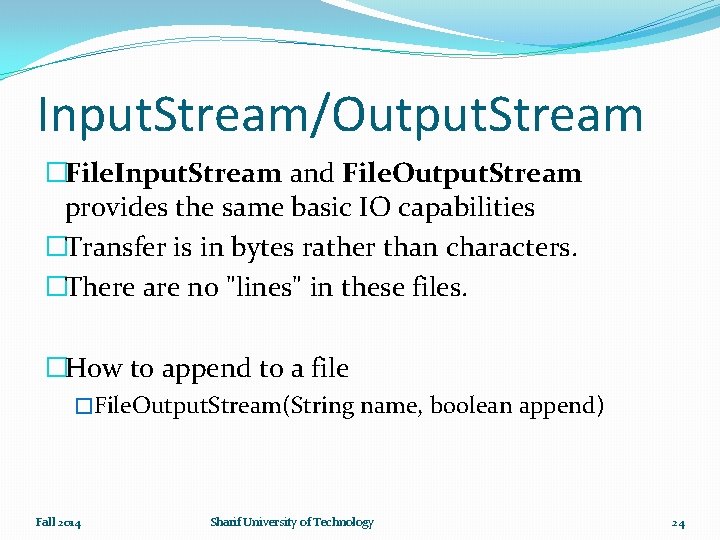
Input. Stream/Output. Stream �File. Input. Stream and File. Output. Stream provides the same basic IO capabilities �Transfer is in bytes rather than characters. �There are no "lines" in these files. �How to append to a file �File. Output. Stream(String name, boolean append) Fall 2014 Sharif University of Technology 24
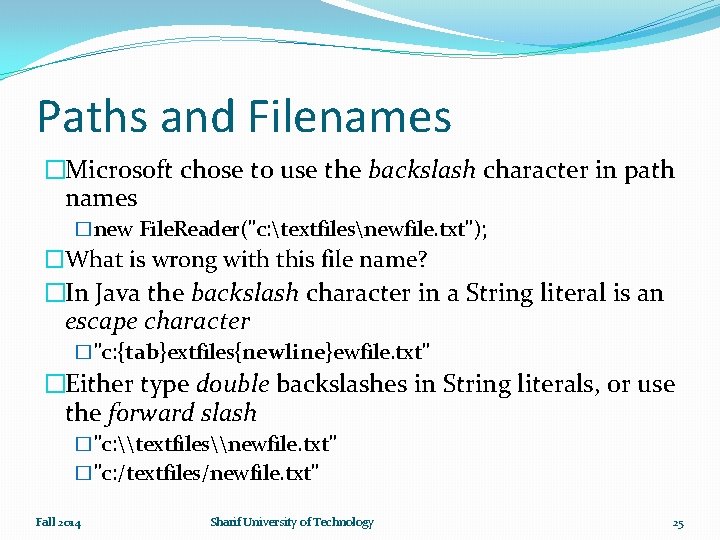
Paths and Filenames �Microsoft chose to use the backslash character in path names �new File. Reader("c: textfilesnewfile. txt"); �What is wrong with this file name? �In Java the backslash character in a String literal is an escape character �"c: {tab}extfiles{newline}ewfile. txt" �Either type double backslashes in String literals, or use the forward slash �"c: \textfiles\newfile. txt" �"c: /textfiles/newfile. txt" Fall 2014 Sharif University of Technology 25
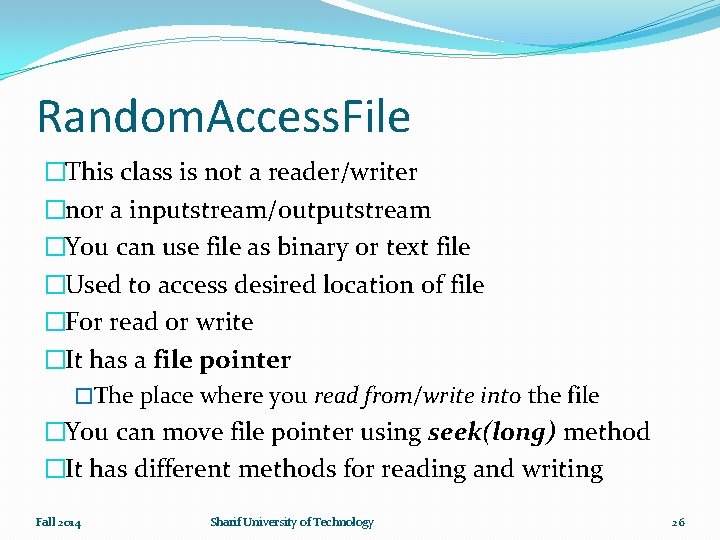
Random. Access. File �This class is not a reader/writer �nor a inputstream/outputstream �You can use file as binary or text file �Used to access desired location of file �For read or write �It has a file pointer �The place where you read from/write into the file �You can move file pointer using seek(long) method �It has different methods for reading and writing Fall 2014 Sharif University of Technology 26
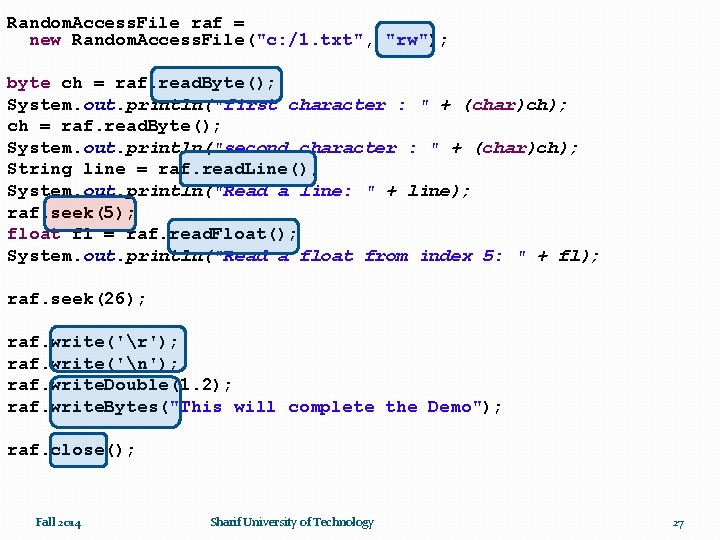
Random. Access. File raf = new Random. Access. File("c: /1. txt", "rw"); byte ch = raf. read. Byte(); System. out. println("first character : " + (char)ch); ch = raf. read. Byte(); System. out. println("second character : " + (char)ch); String line = raf. read. Line(); System. out. println("Read a line: " + line); raf. seek(5); float fl = raf. read. Float(); System. out. println("Read a float from index 5: " + fl); raf. seek(26); raf. write('r'); raf. write('n'); raf. write. Double(1. 2); raf. write. Bytes("This will complete the Demo"); raf. close(); Fall 2014 Sharif University of Technology 27
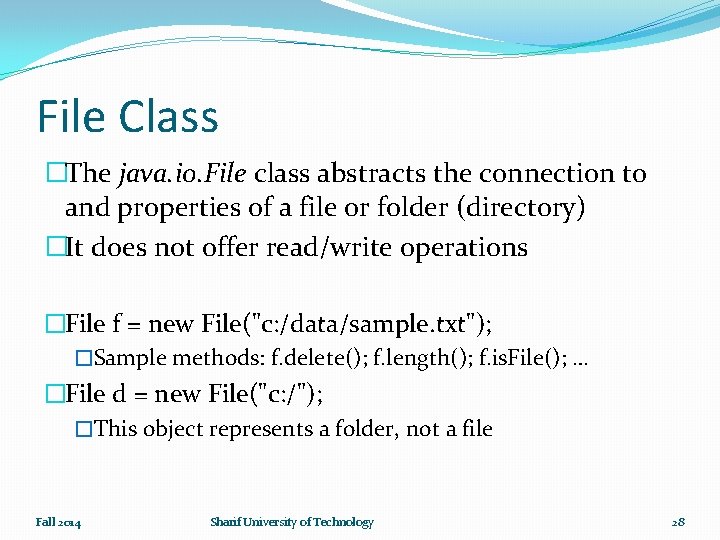
File Class �The java. io. File class abstracts the connection to and properties of a file or folder (directory) �It does not offer read/write operations �File f = new File("c: /data/sample. txt"); �Sample methods: f. delete(); f. length(); f. is. File(); … �File d = new File("c: /"); �This object represents a folder, not a file Fall 2014 Sharif University of Technology 28
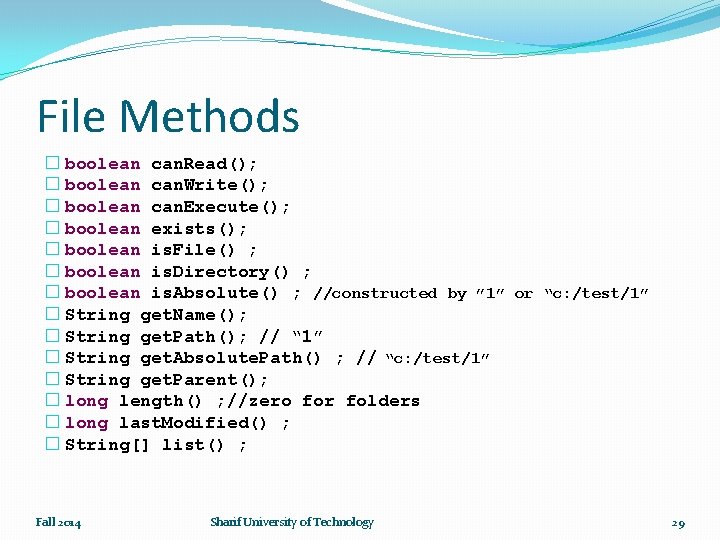
File Methods � boolean can. Read(); � boolean can. Write(); � boolean can. Execute(); � boolean exists(); � boolean is. File() ; � boolean is. Directory() ; � boolean is. Absolute() ; //constructed by ” 1” or “c: /test/1” � String get. Name(); � String get. Path(); // “ 1” � String get. Absolute. Path() ; // “c: /test/1” � String get. Parent(); � long length() ; //zero for folders � long last. Modified() ; � String[] list() ; Fall 2014 Sharif University of Technology 29
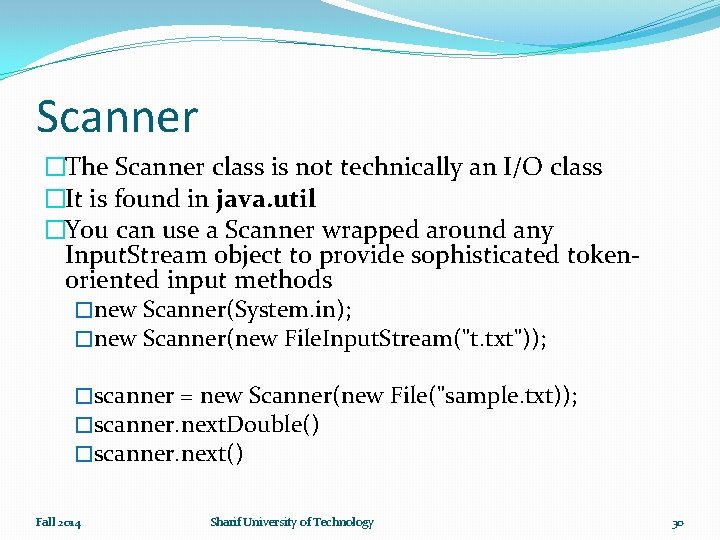
Scanner �The Scanner class is not technically an I/O class �It is found in java. util �You can use a Scanner wrapped around any Input. Stream object to provide sophisticated tokenoriented input methods �new Scanner(System. in); �new Scanner(new File. Input. Stream("t. txt")); �scanner = new Scanner(new File("sample. txt)); �scanner. next. Double() �scanner. next() Fall 2014 Sharif University of Technology 30
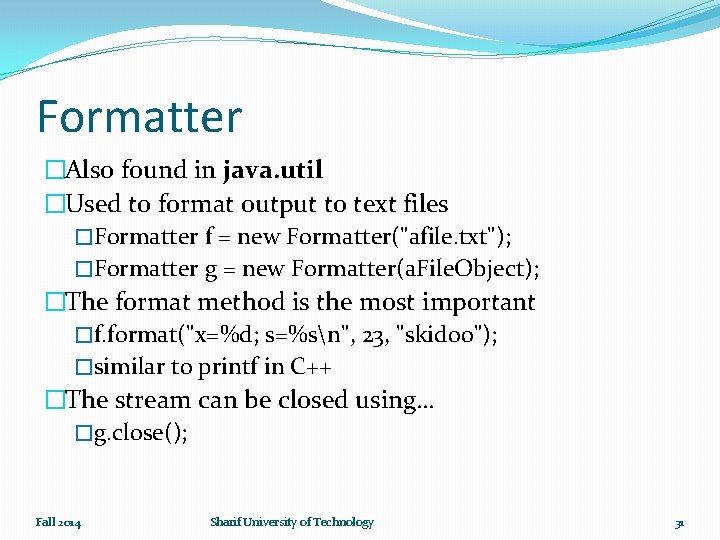
Formatter �Also found in java. util �Used to format output to text files �Formatter f = new Formatter("afile. txt"); �Formatter g = new Formatter(a. File. Object); �The format method is the most important �f. format("x=%d; s=%sn", 23, "skidoo"); �similar to printf in C++ �The stream can be closed using… �g. close(); Fall 2014 Sharif University of Technology 31
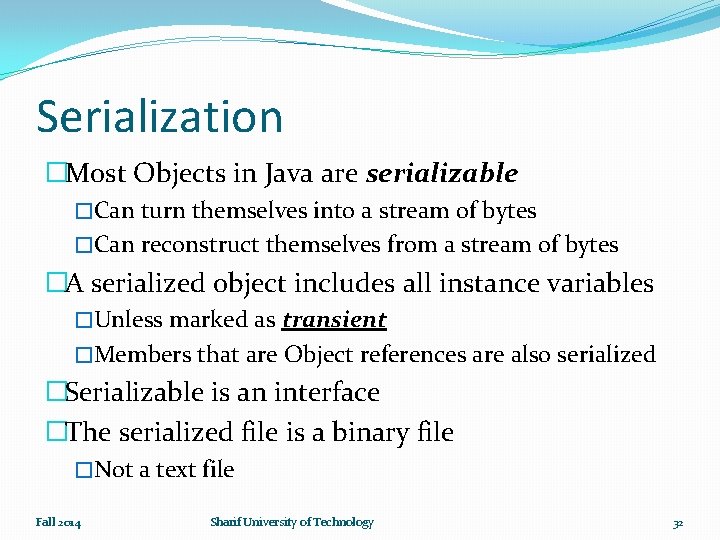
Serialization �Most Objects in Java are serializable �Can turn themselves into a stream of bytes �Can reconstruct themselves from a stream of bytes �A serialized object includes all instance variables �Unless marked as transient �Members that are Object references are also serialized �Serializable is an interface �The serialized file is a binary file �Not a text file Fall 2014 Sharif University of Technology 32
![public class Student implements Serializable private String name private String student ID private double public class Student implements Serializable{ private String name; private String student. ID; private double[]](https://slidetodoc.com/presentation_image_h2/06fd402e600ddfc1303be333dc25e602/image-33.jpg)
public class Student implements Serializable{ private String name; private String student. ID; private double[] grades ; private transient double average = 17. 27; public Student(String name, String student. ID, double[] grades) { this. name = name; this. student. ID = student. ID; this. grades = grades; } public double get. Average() { double sum = 0; if(grades==null) return -1; for (double grade : grades) { sum+=grade; } return sum/grades. length; } //setters and getters for name, student. ID and grades } Fall 2014 Sharif University of Technology 33
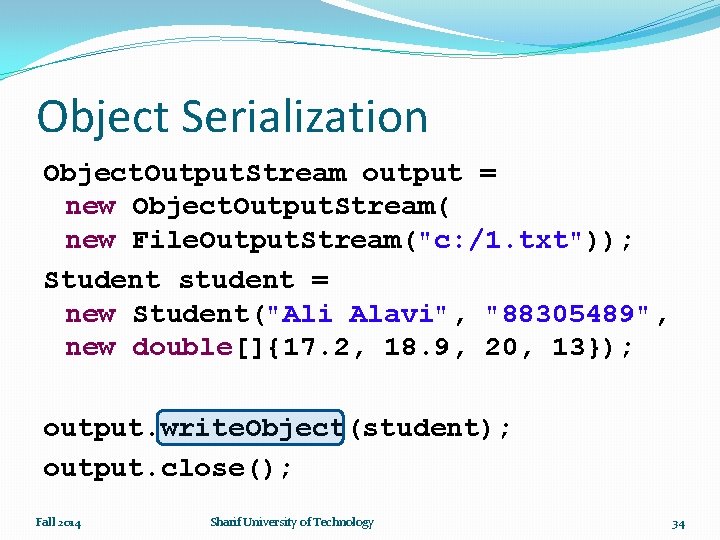
Object Serialization Object. Output. Stream output = new Object. Output. Stream( new File. Output. Stream("c: /1. txt")); Student student = new Student("Ali Alavi", "88305489", new double[]{17. 2, 18. 9, 20, 13}); output. write. Object(student); output. close(); Fall 2014 Sharif University of Technology 34
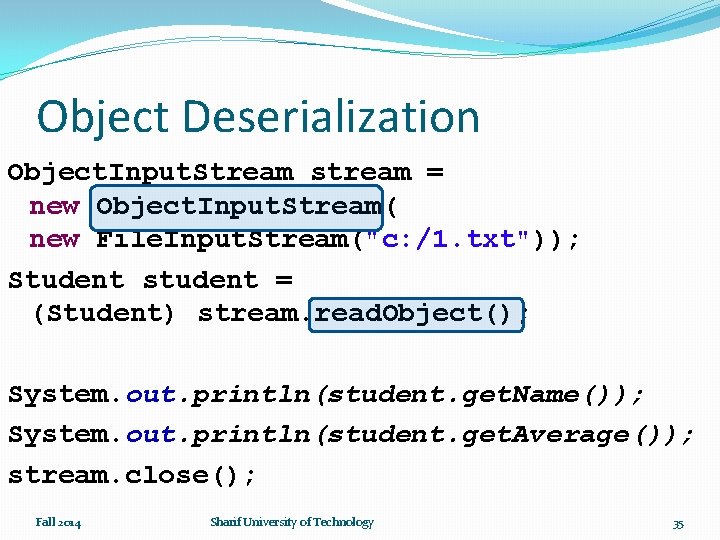
Object Deserialization Object. Input. Stream stream = new Object. Input. Stream( new File. Input. Stream("c: /1. txt")); Student student = (Student) stream. read. Object(); System. out. println(student. get. Name()); System. out. println(student. get. Average()); stream. close(); Fall 2014 Sharif University of Technology 35
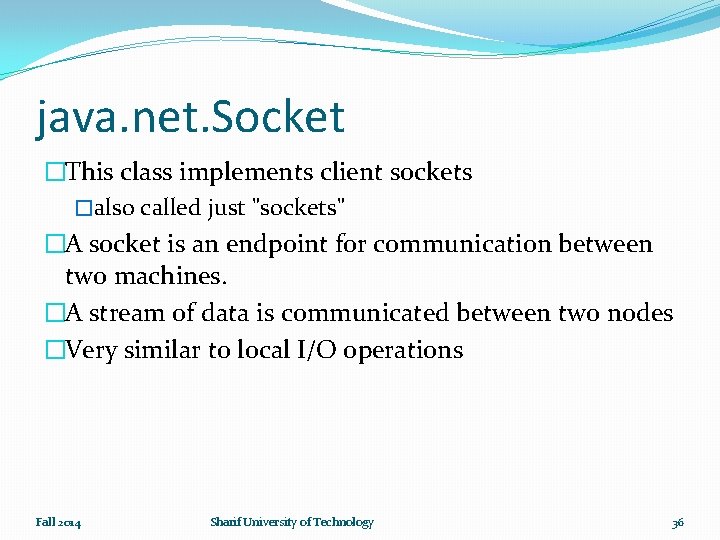
java. net. Socket �This class implements client sockets �also called just "sockets" �A socket is an endpoint for communication between two machines. �A stream of data is communicated between two nodes �Very similar to local I/O operations Fall 2014 Sharif University of Technology 36
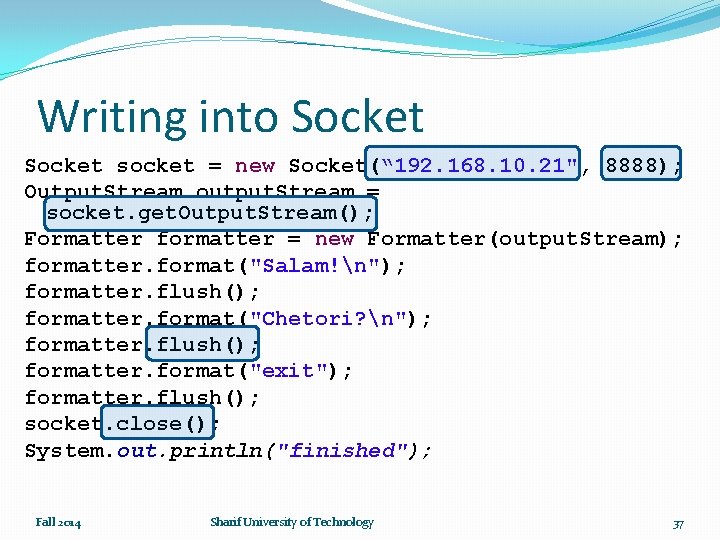
Writing into Socket socket = new Socket(“ 192. 168. 10. 21", 8888); Output. Stream output. Stream = socket. get. Output. Stream(); Formatter formatter = new Formatter(output. Stream); formatter. format("Salam!n"); formatter. flush(); formatter. format("Chetori? n"); formatter. flush(); formatter. format("exit"); formatter. flush(); socket. close(); System. out. println("finished"); Fall 2014 Sharif University of Technology 37
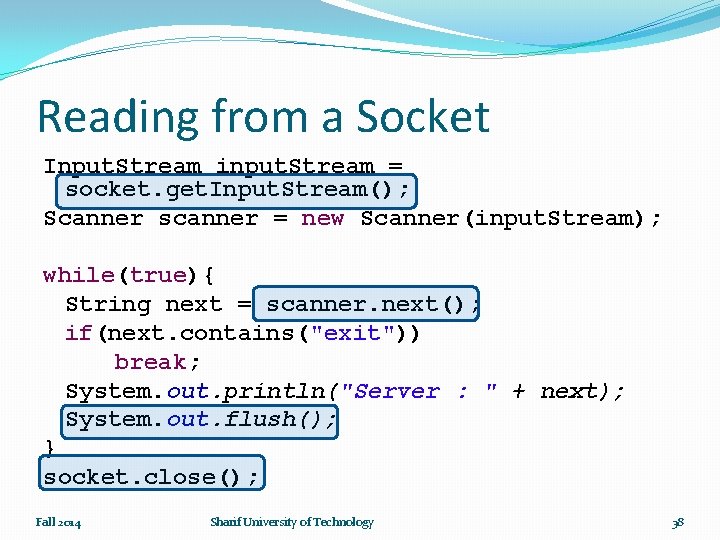
Reading from a Socket Input. Stream input. Stream = socket. get. Input. Stream(); Scanner scanner = new Scanner(input. Stream); while(true){ String next = scanner. next(); if(next. contains("exit")) break; System. out. println("Server : " + next); System. out. flush(); } socket. close(); Fall 2014 Sharif University of Technology 38
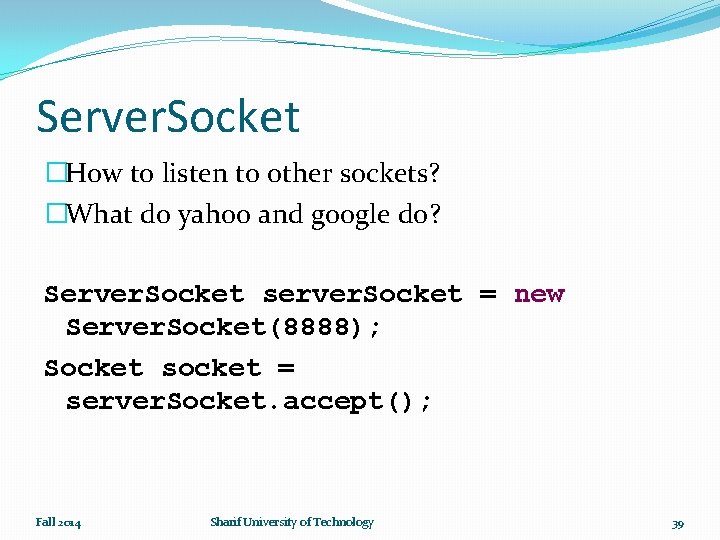
Server. Socket �How to listen to other sockets? �What do yahoo and google do? Server. Socket server. Socket = new Server. Socket(8888); Socket socket = server. Socket. accept(); Fall 2014 Sharif University of Technology 39
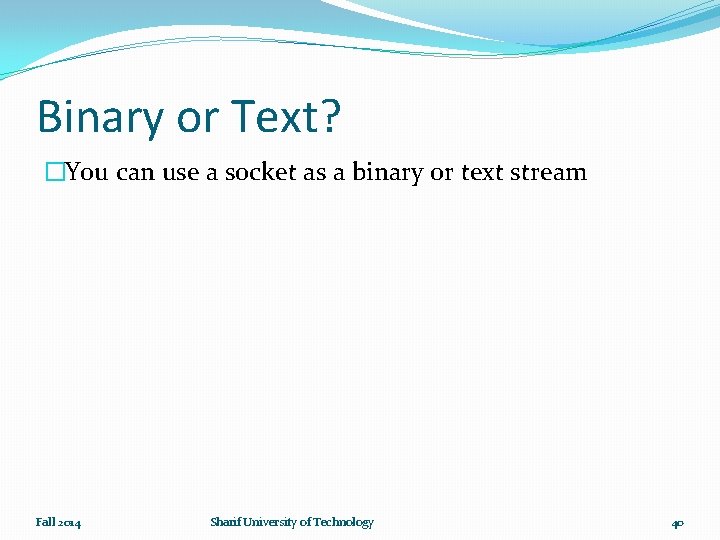
Binary or Text? �You can use a socket as a binary or text stream Fall 2014 Sharif University of Technology 40
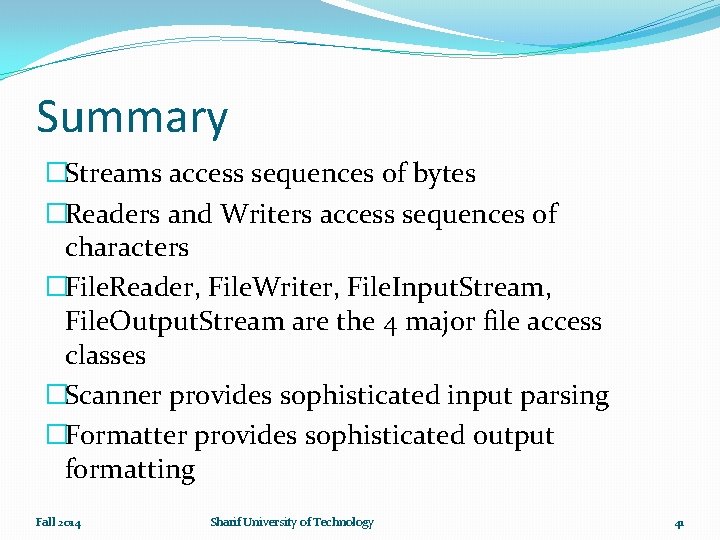
Summary �Streams access sequences of bytes �Readers and Writers access sequences of characters �File. Reader, File. Writer, File. Input. Stream, File. Output. Stream are the 4 major file access classes �Scanner provides sophisticated input parsing �Formatter provides sophisticated output formatting Fall 2014 Sharif University of Technology 41
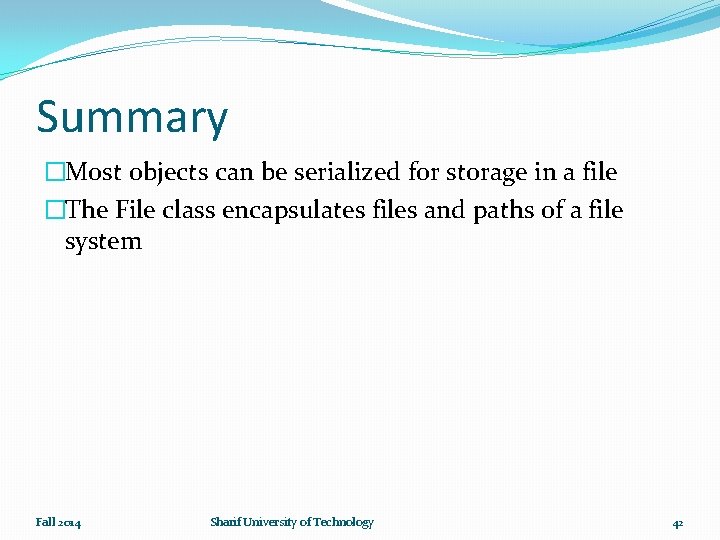
Summary �Most objects can be serialized for storage in a file �The File class encapsulates files and paths of a file system Fall 2014 Sharif University of Technology 42
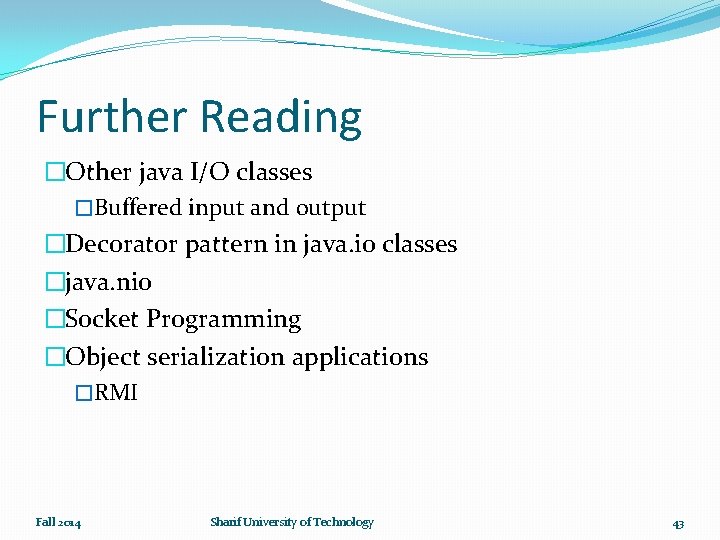
Further Reading �Other java I/O classes �Buffered input and output �Decorator pattern in java. io classes �java. nio �Socket Programming �Object serialization applications �RMI Fall 2014 Sharif University of Technology 43
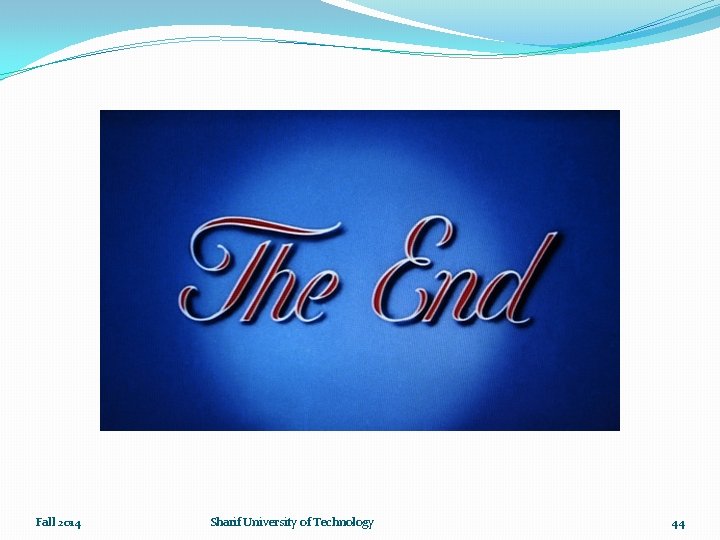
Fall 2014 Sharif University of Technology 44
Advanced programming in java
Drpeyman.ir
Amin kheradmand
Dr. alireza peyman
Peyman kazemian
Pgnna
Alireza yalda
Sharif university of technology
Muhammad nawaz shareef university of agriculture
Advanced dynamic programming
Advanced internet programming
What is ltorg in system programming
Assembly language programming
Advanced data structures in java
Java advanced exercises
Perbedaan linear programming dan integer programming
Greedy programming vs dynamic programming
System programming vs application programming
Integer programming vs linear programming
Programing adalah
Zaman kite runner
Ce.sharif
Leila sharif
Trove
Edu.sharif.edu
My.edu.sharif
Biodata sharif masahor
Peristiwa penentangan sharif masahor
Cw.sharif.eud
Sharif
Sebab sebab penentangan mat salleh
Sharif taha
Behjat sharif
Lenticular nucleus function
Ayan sharif
Andy field
Pico finer
Application
Parallel programming in java
Problem solving
Event-driven programming in java
Elementary programming in java
Java asynchronous programming
Java structured programming
Importance of java programming