Advanced C Topics Chapter 8 CS 308 Chapter
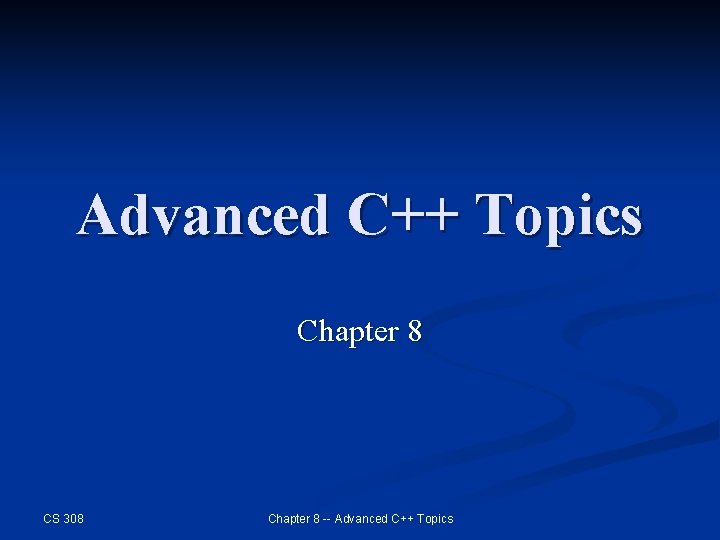
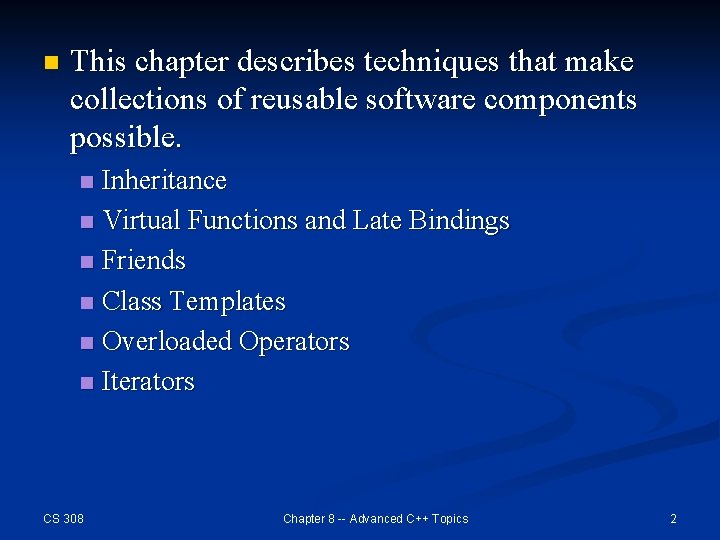
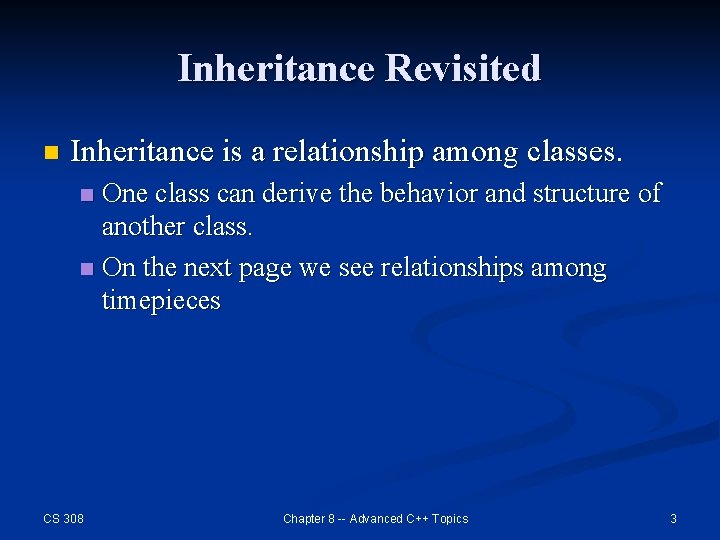
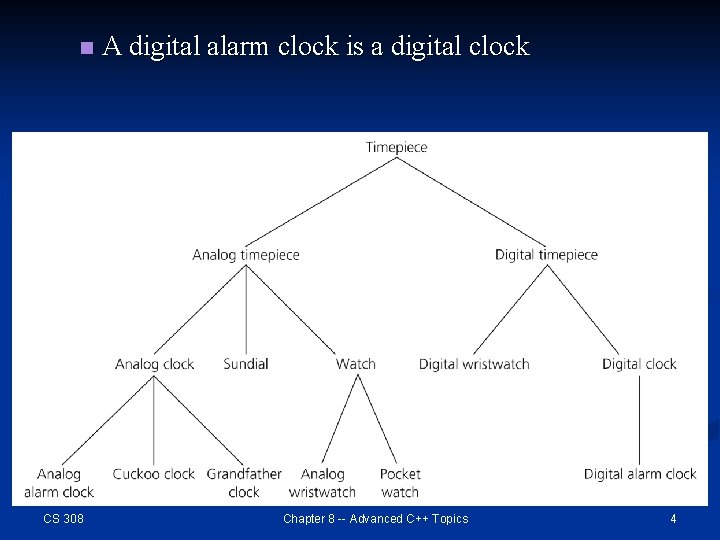
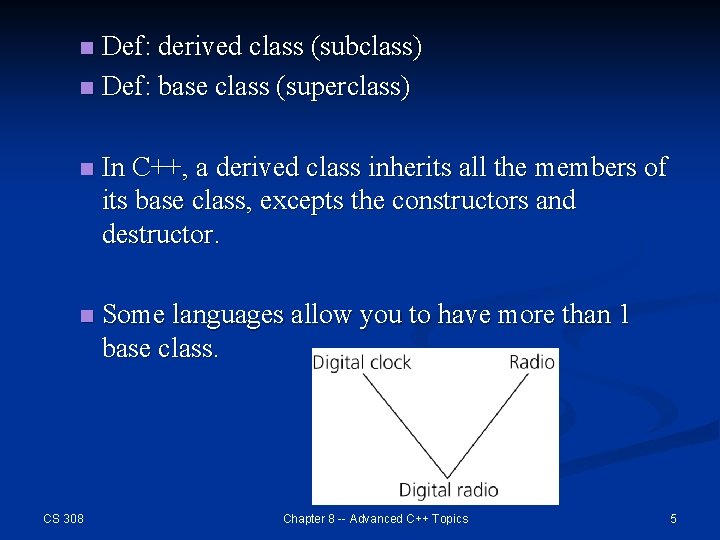
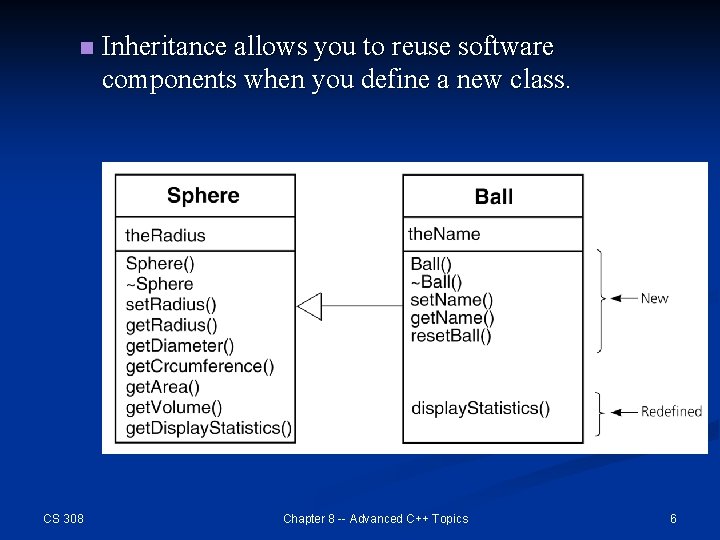
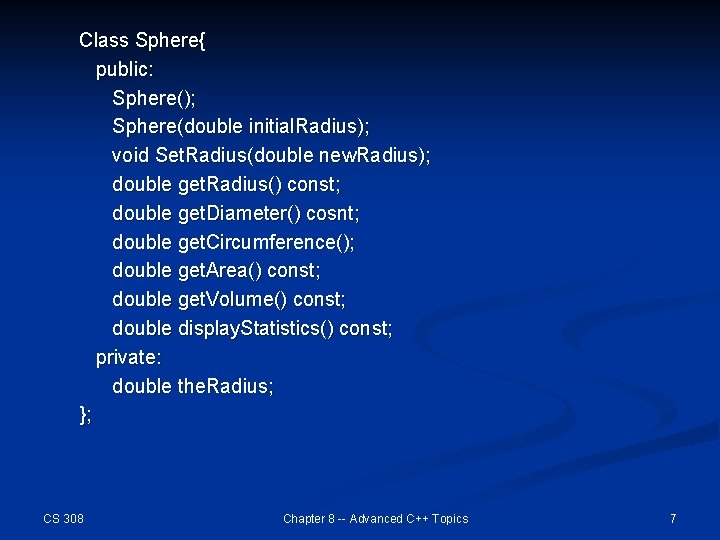
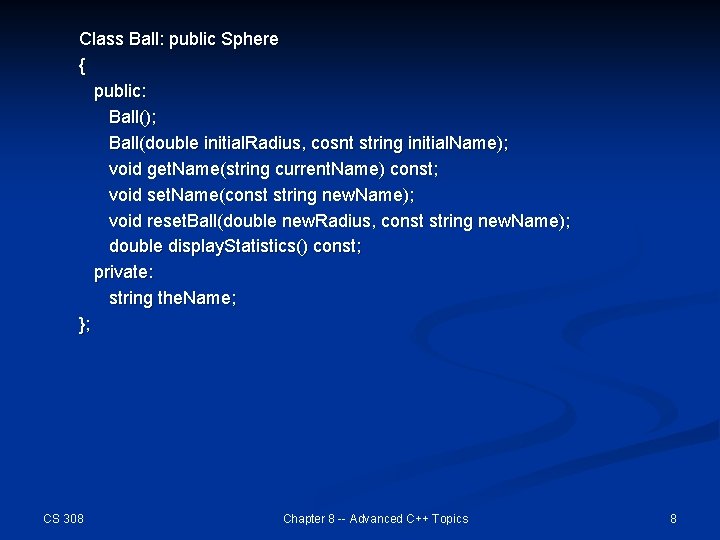
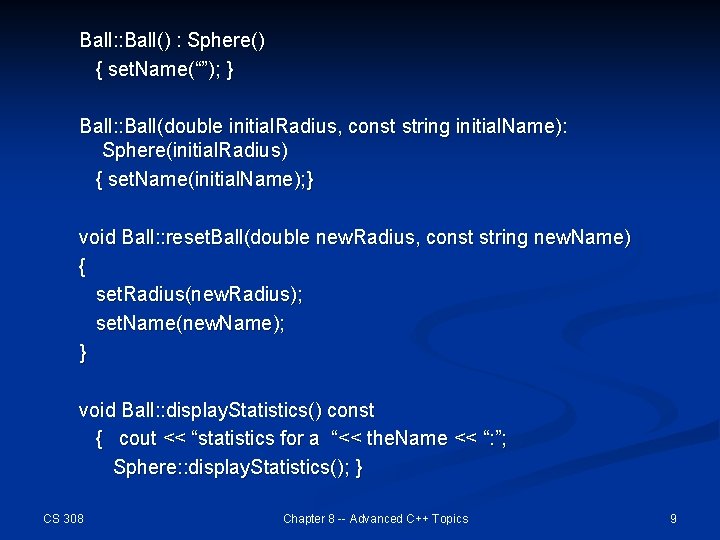
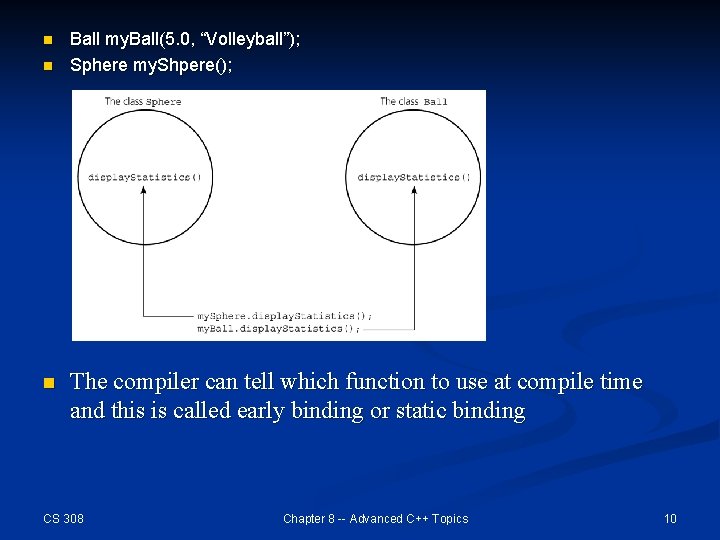
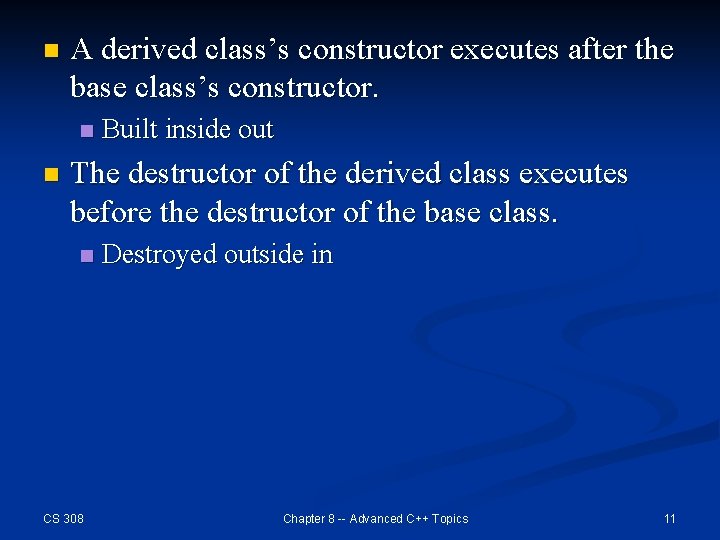
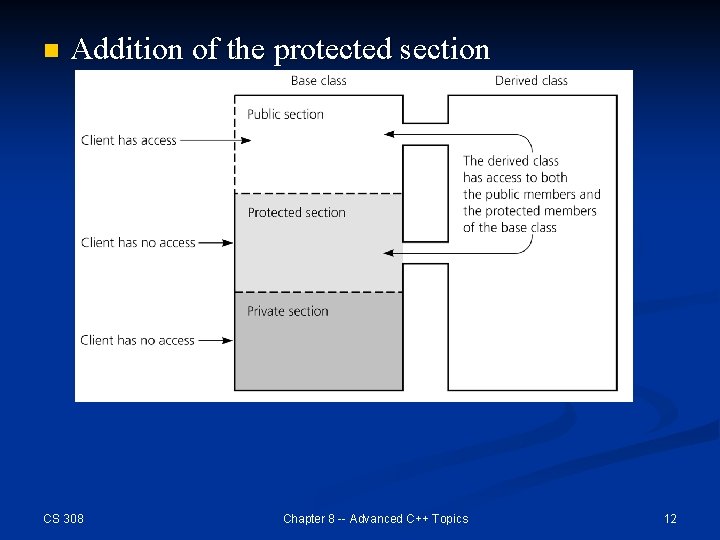
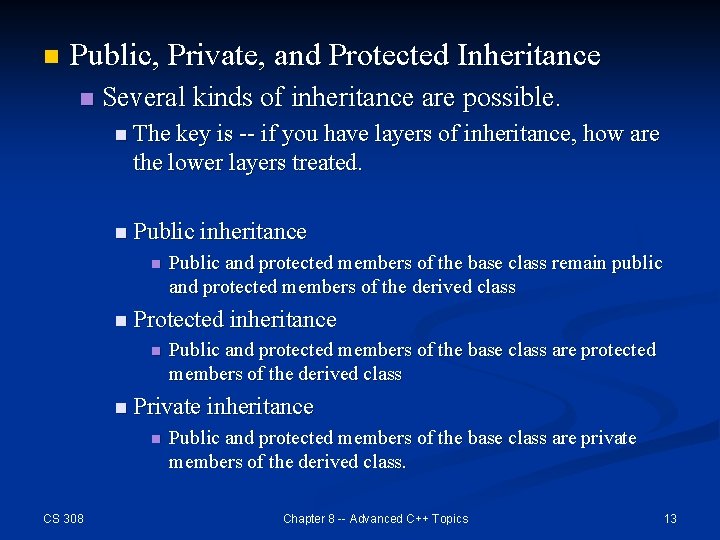
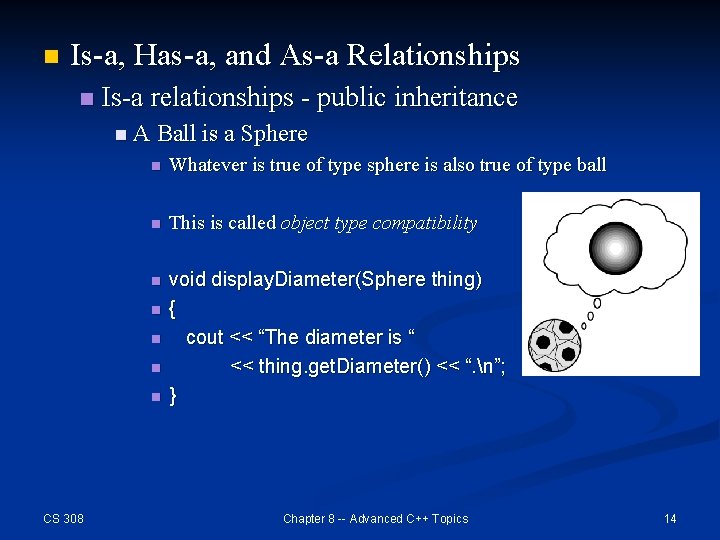
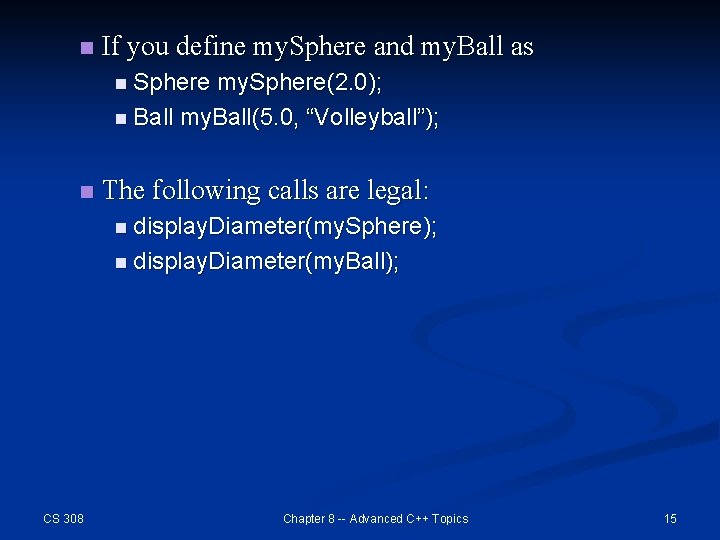
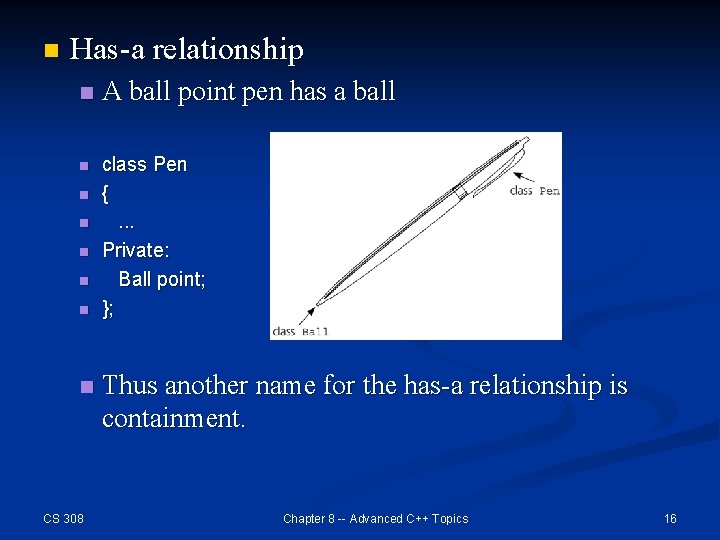
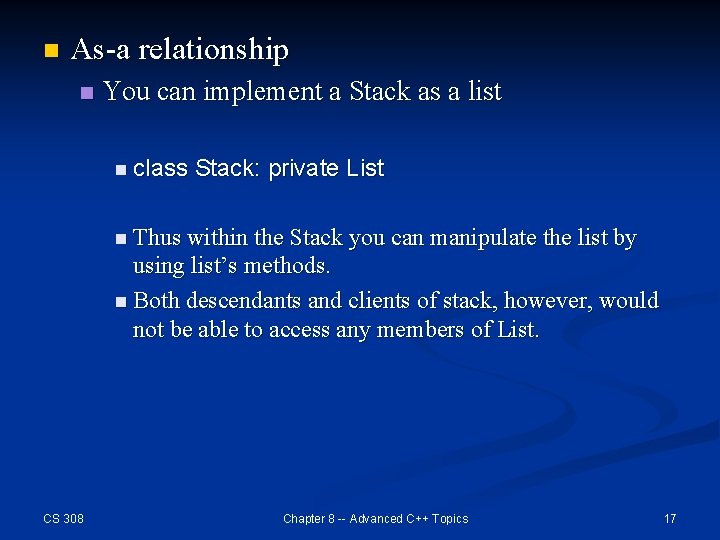
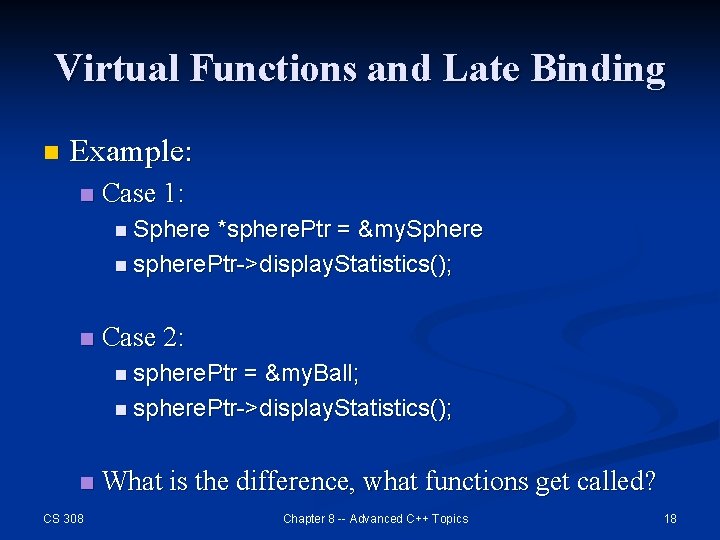
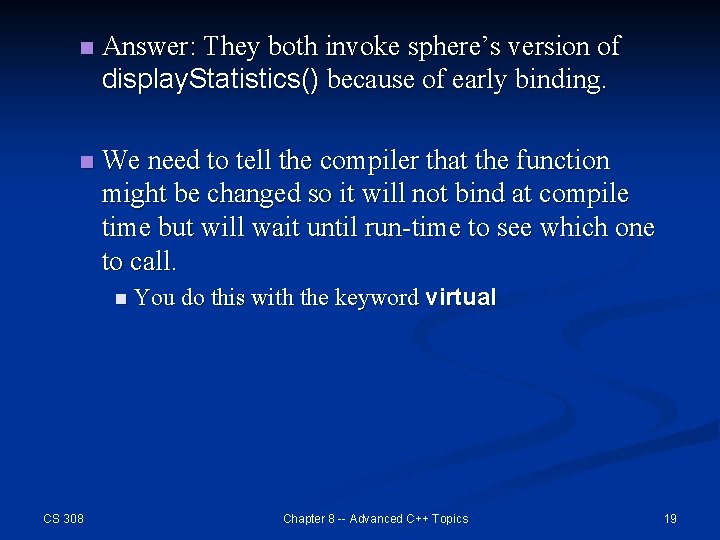
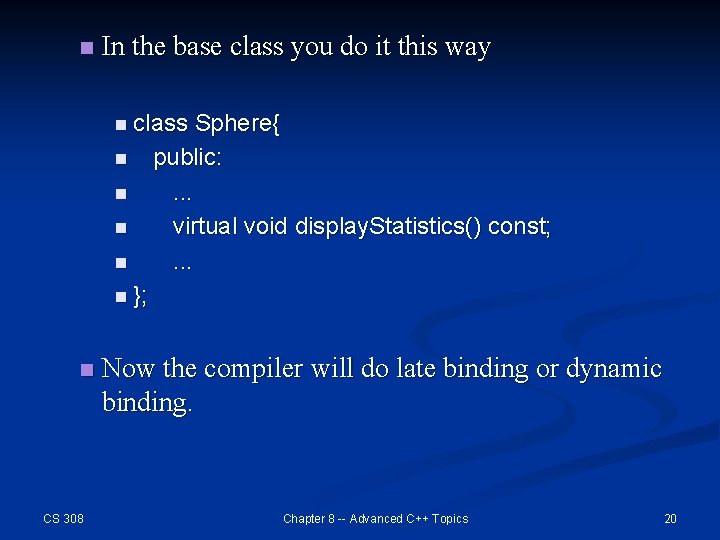
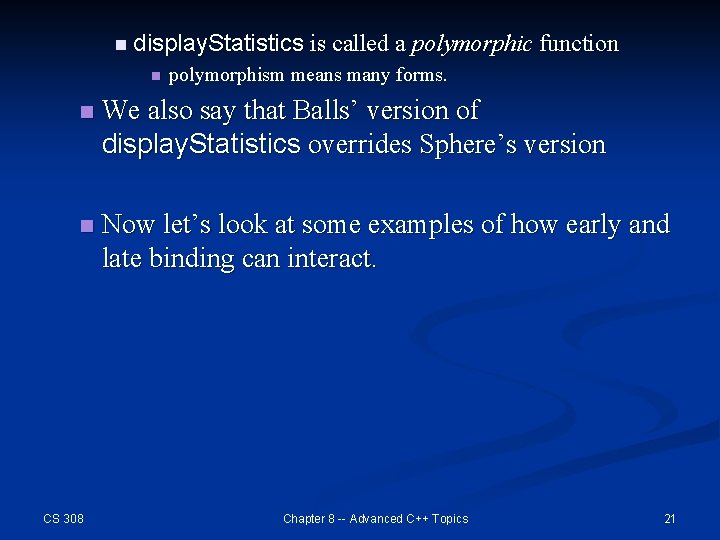
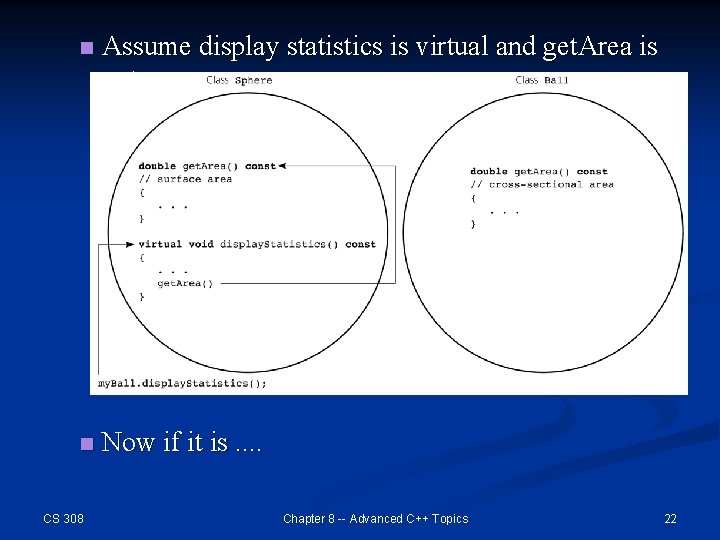
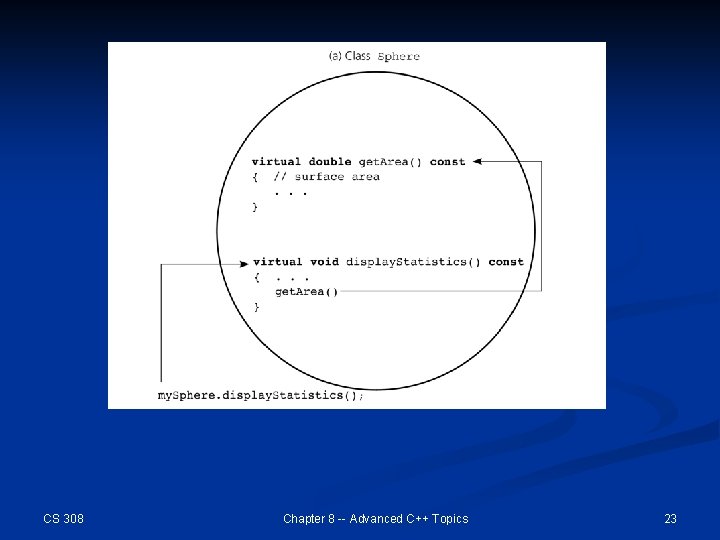
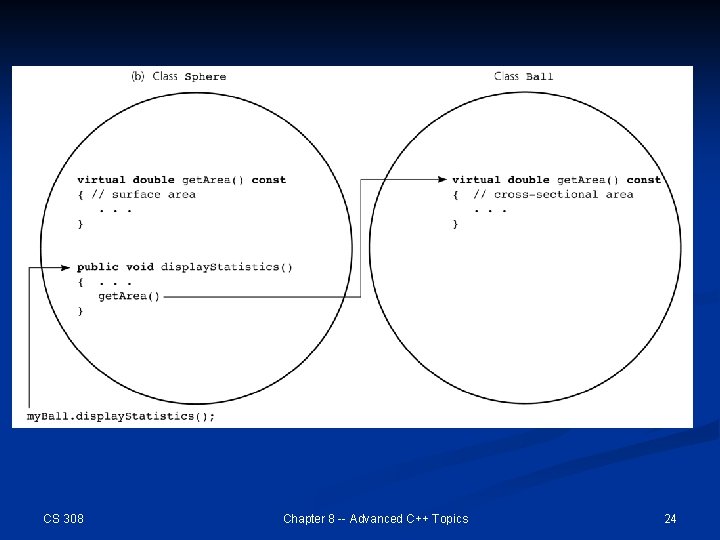
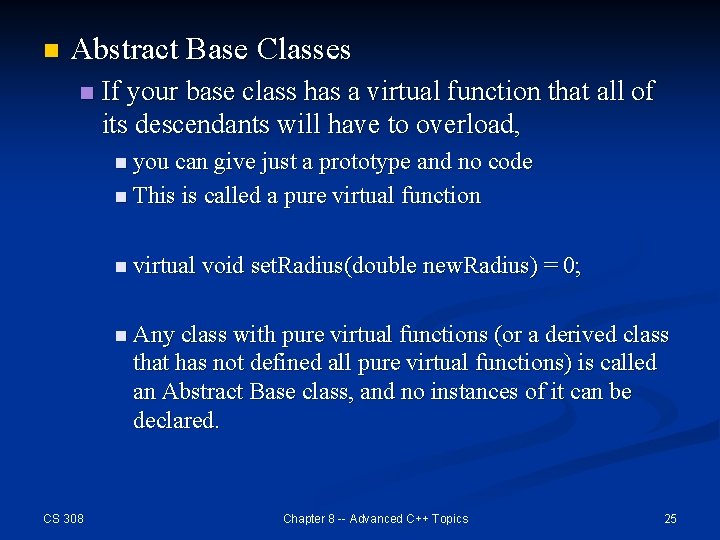
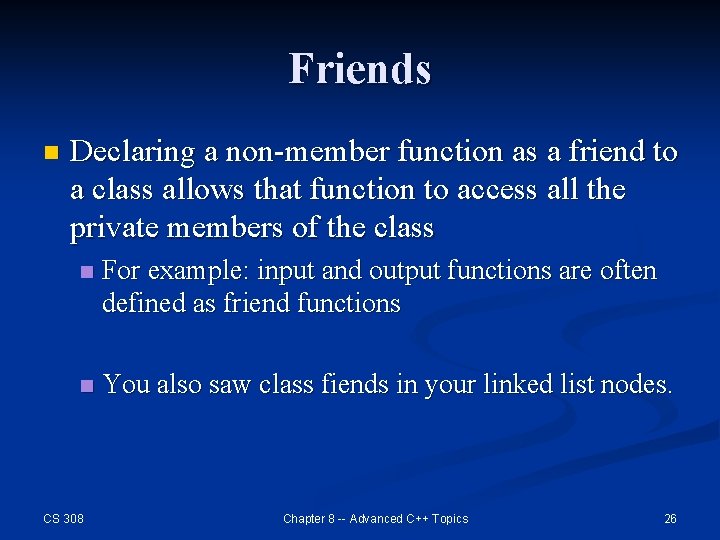
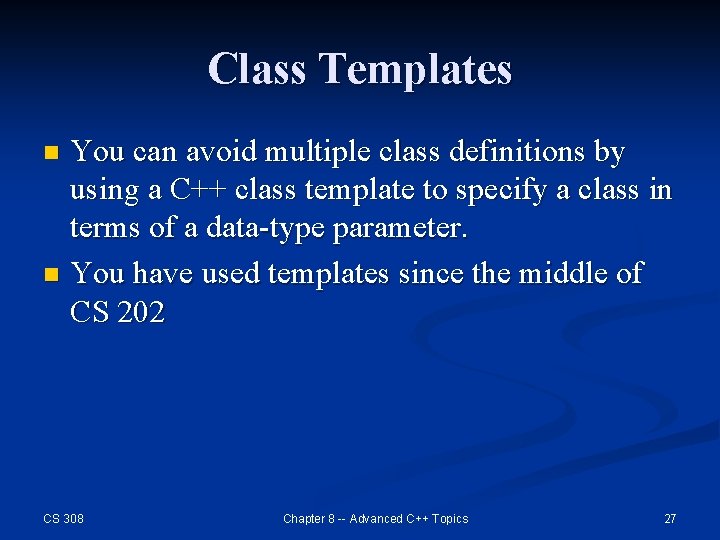
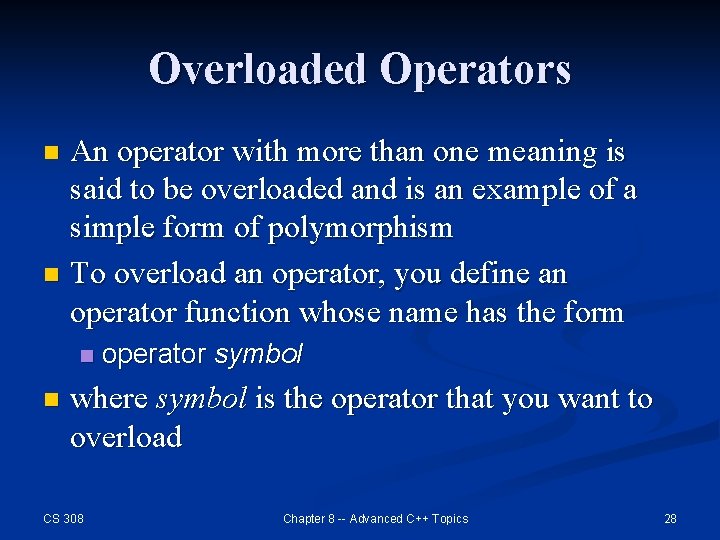
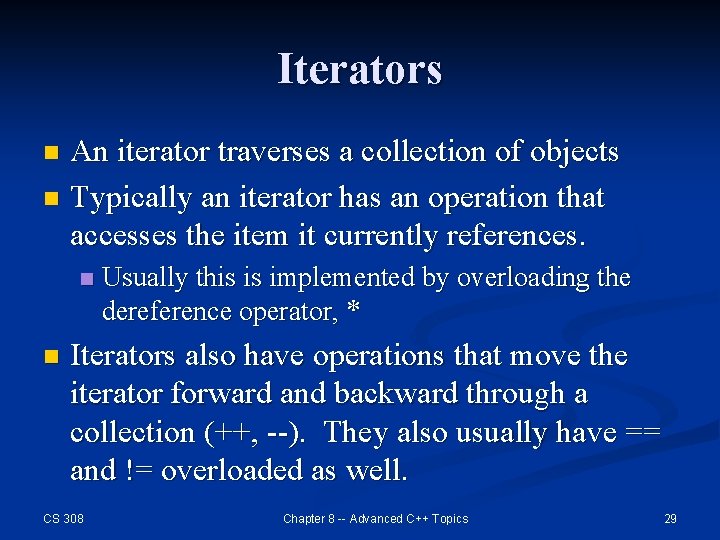
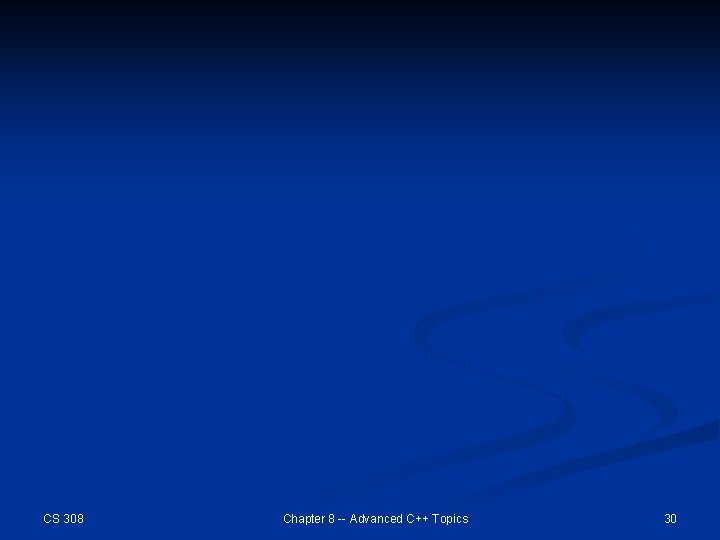
- Slides: 30
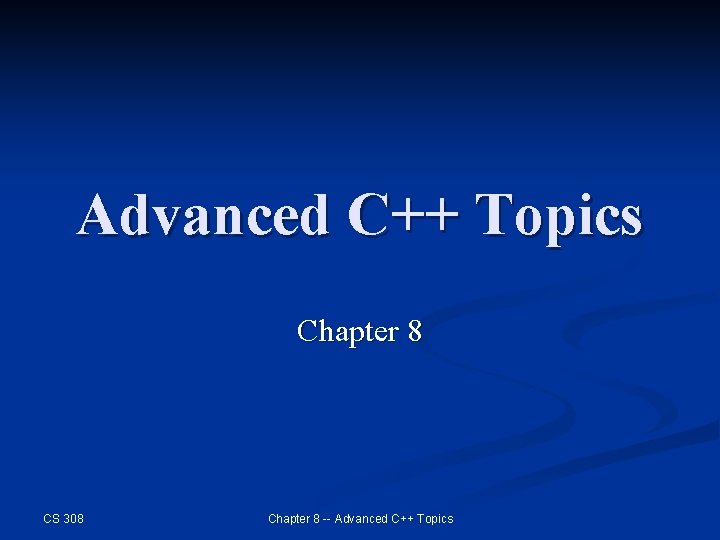
Advanced C++ Topics Chapter 8 CS 308 Chapter 8 -- Advanced C++ Topics
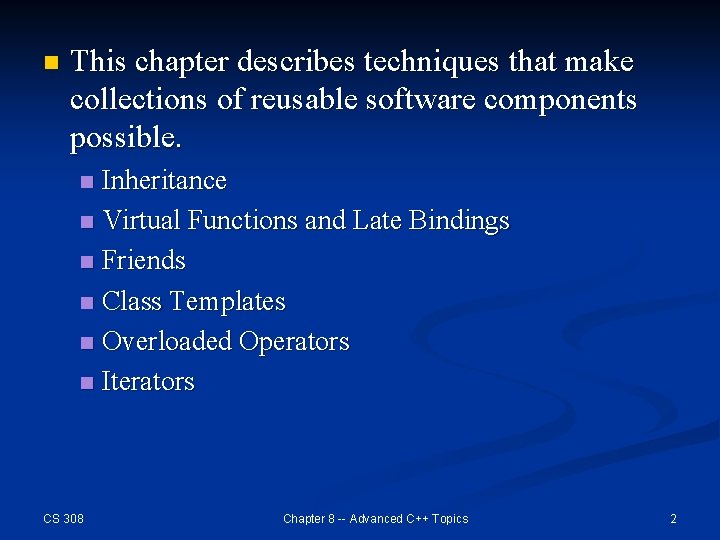
n This chapter describes techniques that make collections of reusable software components possible. Inheritance n Virtual Functions and Late Bindings n Friends n Class Templates n Overloaded Operators n Iterators n CS 308 Chapter 8 -- Advanced C++ Topics 2
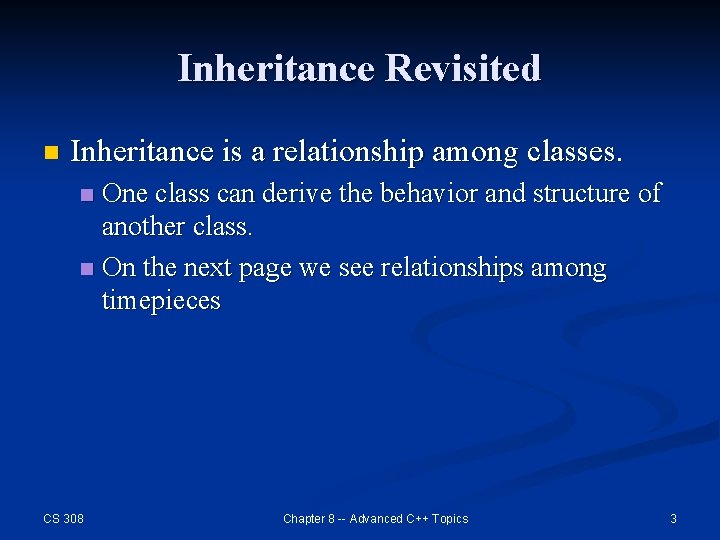
Inheritance Revisited n Inheritance is a relationship among classes. One class can derive the behavior and structure of another class. n On the next page we see relationships among timepieces n CS 308 Chapter 8 -- Advanced C++ Topics 3
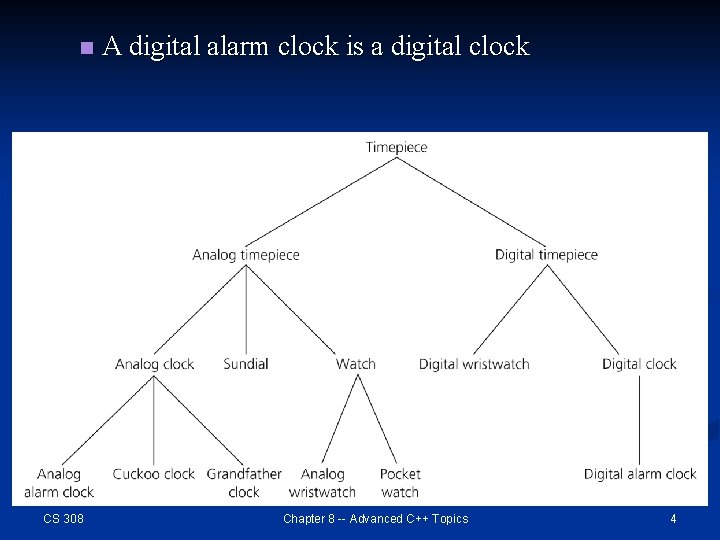
n CS 308 A digital alarm clock is a digital clock Chapter 8 -- Advanced C++ Topics 4
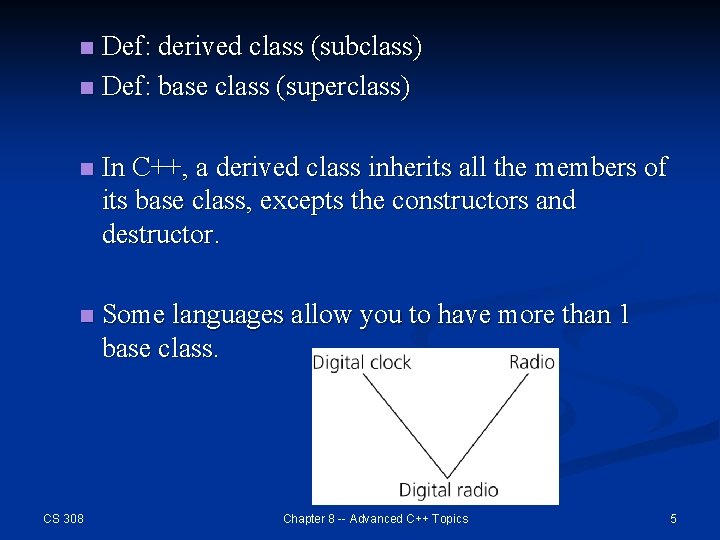
Def: derived class (subclass) n Def: base class (superclass) n n In C++, a derived class inherits all the members of its base class, excepts the constructors and destructor. n Some languages allow you to have more than 1 base class. CS 308 Chapter 8 -- Advanced C++ Topics 5
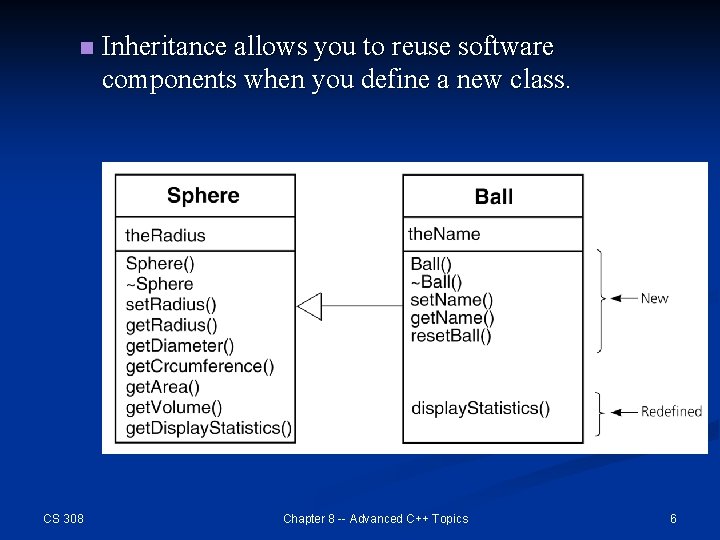
n CS 308 Inheritance allows you to reuse software components when you define a new class. Chapter 8 -- Advanced C++ Topics 6
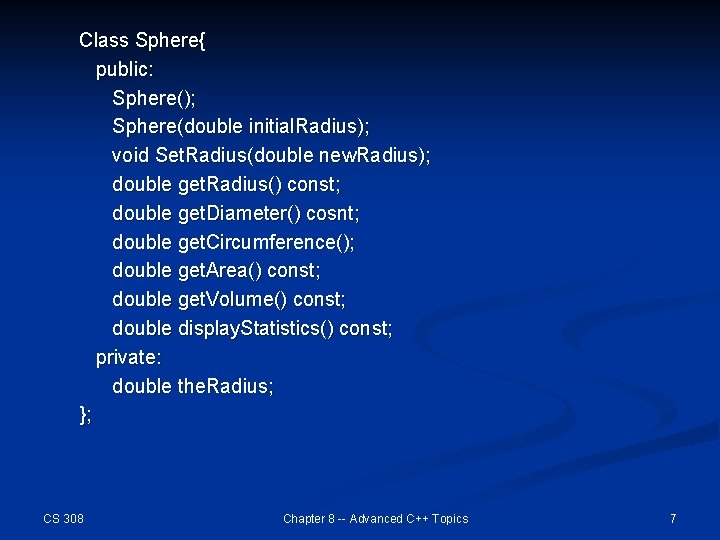
Class Sphere{ public: Sphere(); Sphere(double initial. Radius); void Set. Radius(double new. Radius); double get. Radius() const; double get. Diameter() cosnt; double get. Circumference(); double get. Area() const; double get. Volume() const; double display. Statistics() const; private: double the. Radius; }; CS 308 Chapter 8 -- Advanced C++ Topics 7
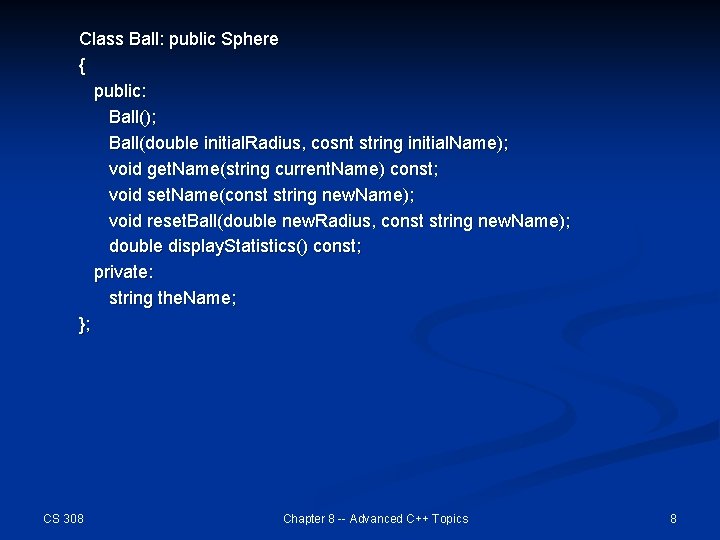
Class Ball: public Sphere { public: Ball(); Ball(double initial. Radius, cosnt string initial. Name); void get. Name(string current. Name) const; void set. Name(const string new. Name); void reset. Ball(double new. Radius, const string new. Name); double display. Statistics() const; private: string the. Name; }; CS 308 Chapter 8 -- Advanced C++ Topics 8
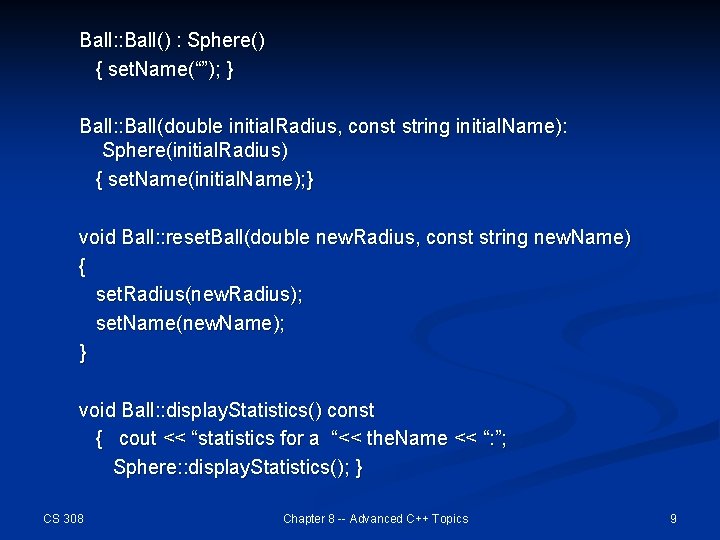
Ball: : Ball() : Sphere() { set. Name(“”); } Ball: : Ball(double initial. Radius, const string initial. Name): Sphere(initial. Radius) { set. Name(initial. Name); } void Ball: : reset. Ball(double new. Radius, const string new. Name) { set. Radius(new. Radius); set. Name(new. Name); } void Ball: : display. Statistics() const { cout << “statistics for a “<< the. Name << “: ”; Sphere: : display. Statistics(); } CS 308 Chapter 8 -- Advanced C++ Topics 9
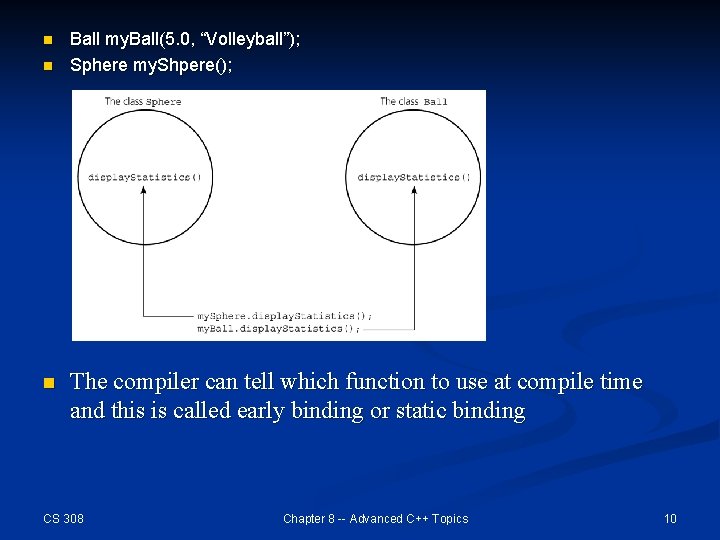
n n n Ball my. Ball(5. 0, “Volleyball”); Sphere my. Shpere(); The compiler can tell which function to use at compile time and this is called early binding or static binding CS 308 Chapter 8 -- Advanced C++ Topics 10
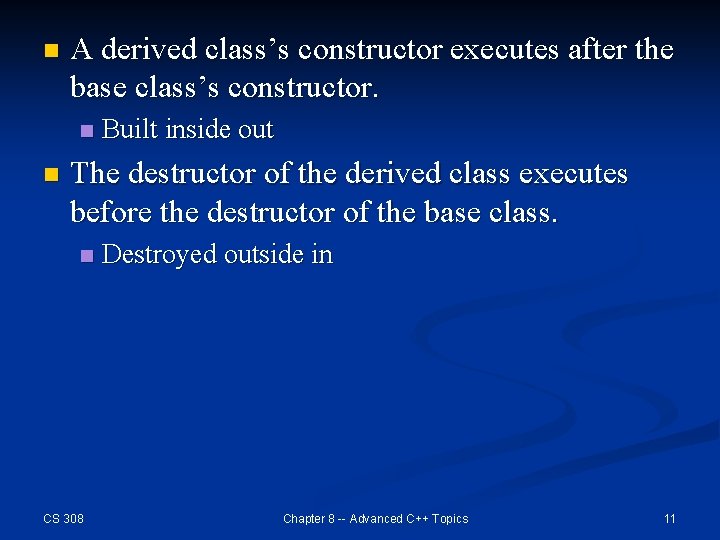
n A derived class’s constructor executes after the base class’s constructor. n n Built inside out The destructor of the derived class executes before the destructor of the base class. n CS 308 Destroyed outside in Chapter 8 -- Advanced C++ Topics 11
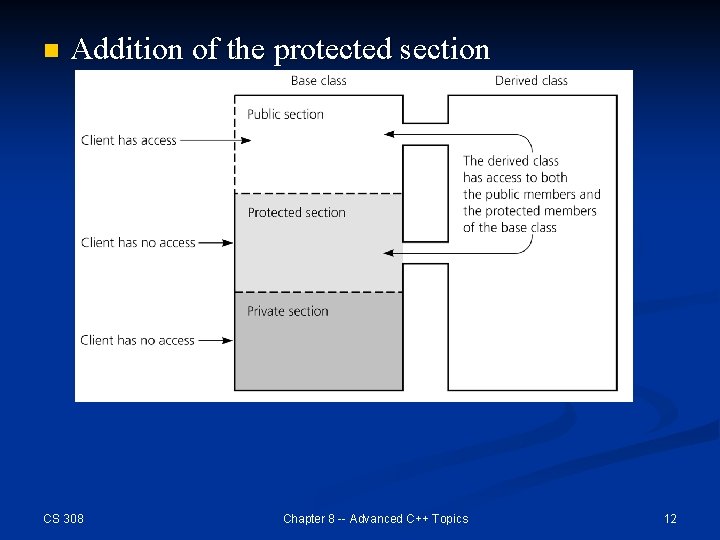
n Addition of the protected section CS 308 Chapter 8 -- Advanced C++ Topics 12
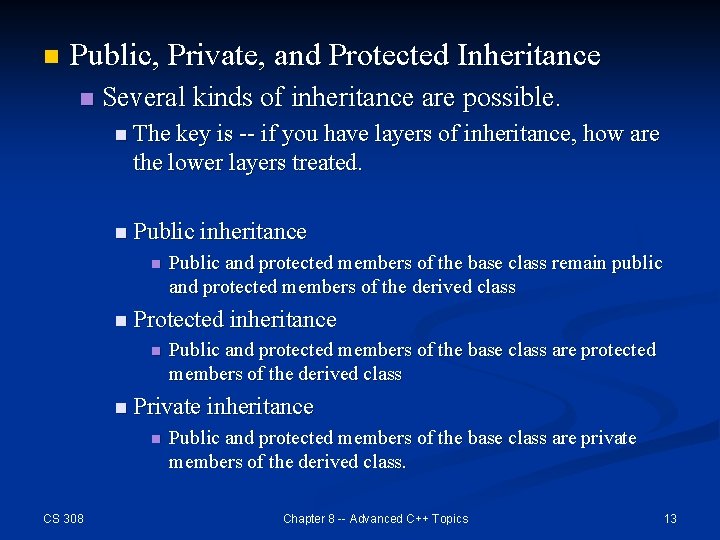
n Public, Private, and Protected Inheritance n Several kinds of inheritance are possible. n The key is -- if you have layers of inheritance, how are the lower layers treated. n Public inheritance n Public and protected members of the base class remain public and protected members of the derived class n Protected inheritance n Public and protected members of the base class are protected members of the derived class n Private inheritance n CS 308 Public and protected members of the base class are private members of the derived class. Chapter 8 -- Advanced C++ Topics 13
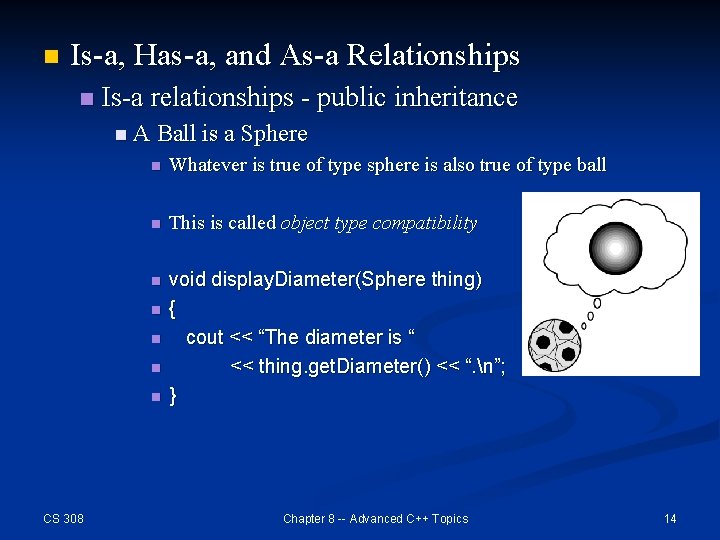
n Is-a, Has-a, and As-a Relationships n Is-a relationships - public inheritance n A Ball is a Sphere n Whatever is true of type sphere is also true of type ball n This is called object type compatibility n void display. Diameter(Sphere thing) { cout << “The diameter is “ << thing. get. Diameter() << “. n”; } n n CS 308 Chapter 8 -- Advanced C++ Topics 14
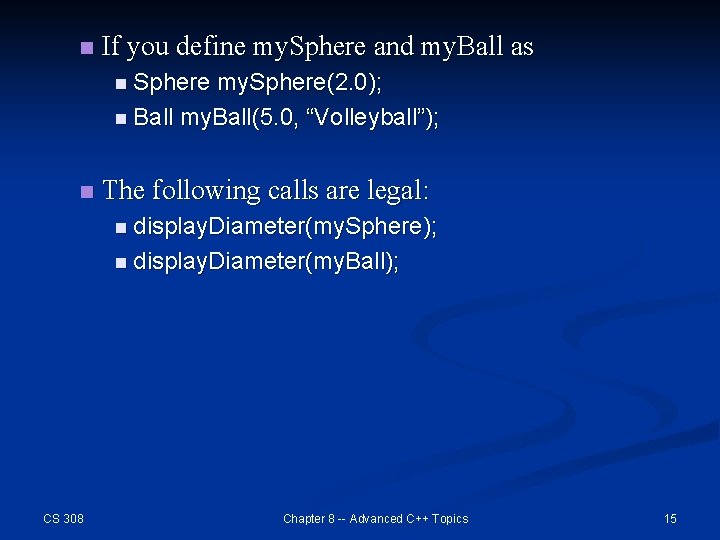
n If you define my. Sphere and my. Ball as n Sphere my. Sphere(2. 0); n Ball my. Ball(5. 0, “Volleyball”); n The following calls are legal: n display. Diameter(my. Sphere); n display. Diameter(my. Ball); CS 308 Chapter 8 -- Advanced C++ Topics 15
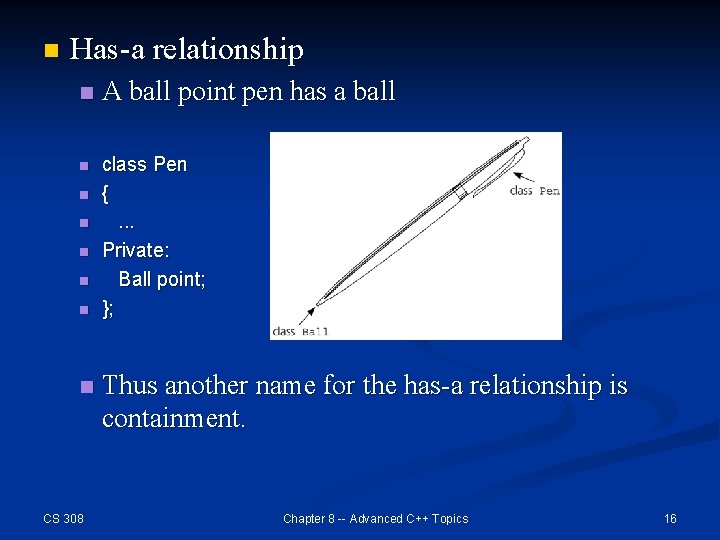
n Has-a relationship n A ball point pen has a ball n class Pen {. . . Private: Ball point; }; n n n CS 308 Thus another name for the has-a relationship is containment. Chapter 8 -- Advanced C++ Topics 16
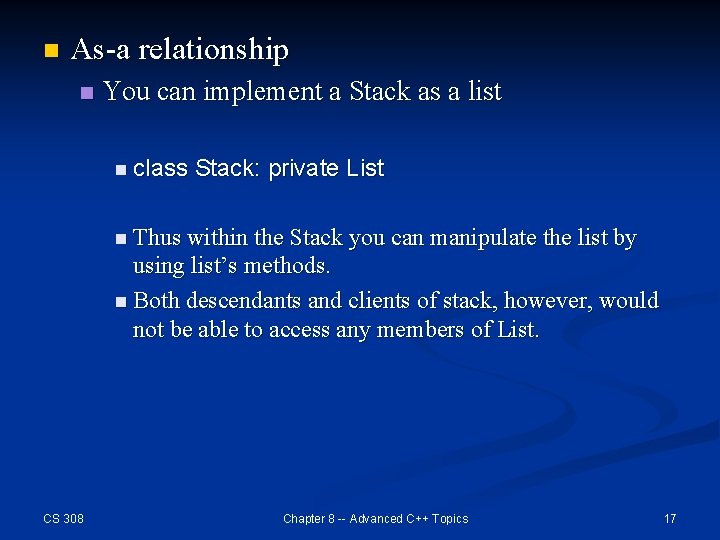
n As-a relationship n You can implement a Stack as a list n class Stack: private List n Thus within the Stack you can manipulate the list by using list’s methods. n Both descendants and clients of stack, however, would not be able to access any members of List. CS 308 Chapter 8 -- Advanced C++ Topics 17
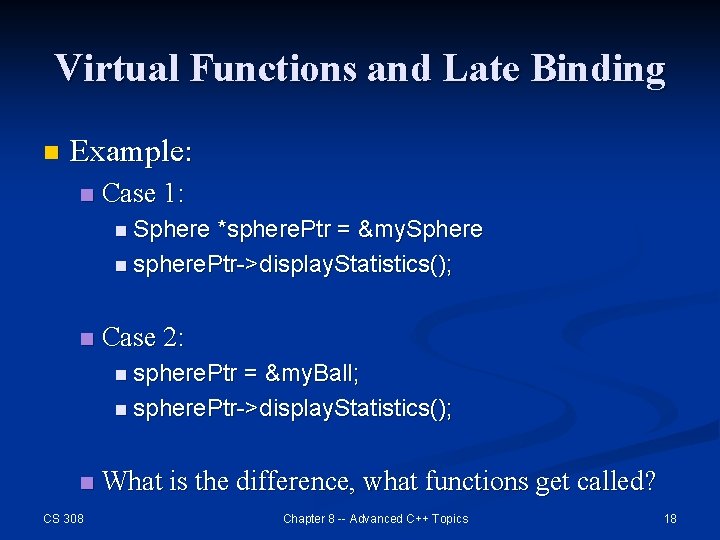
Virtual Functions and Late Binding n Example: n Case 1: n Sphere *sphere. Ptr = &my. Sphere n sphere. Ptr->display. Statistics(); n Case 2: n sphere. Ptr = &my. Ball; n sphere. Ptr->display. Statistics(); n CS 308 What is the difference, what functions get called? Chapter 8 -- Advanced C++ Topics 18
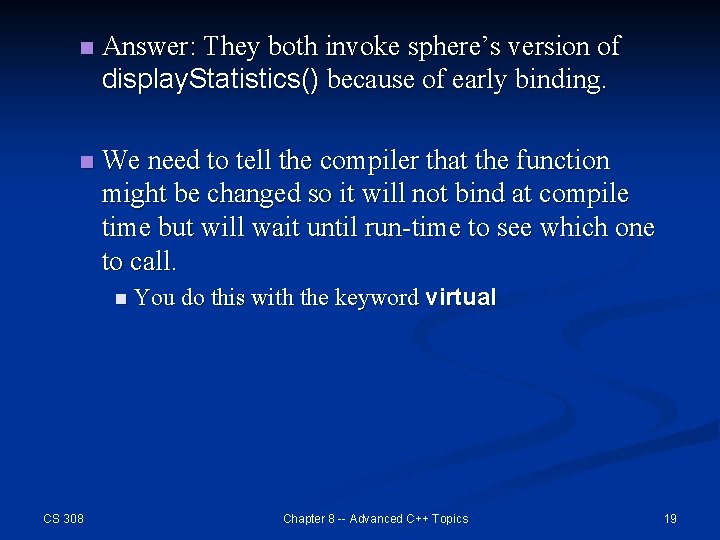
n Answer: They both invoke sphere’s version of display. Statistics() because of early binding. n We need to tell the compiler that the function might be changed so it will not bind at compile time but will wait until run-time to see which one to call. n You do this with the keyword virtual CS 308 Chapter 8 -- Advanced C++ Topics 19
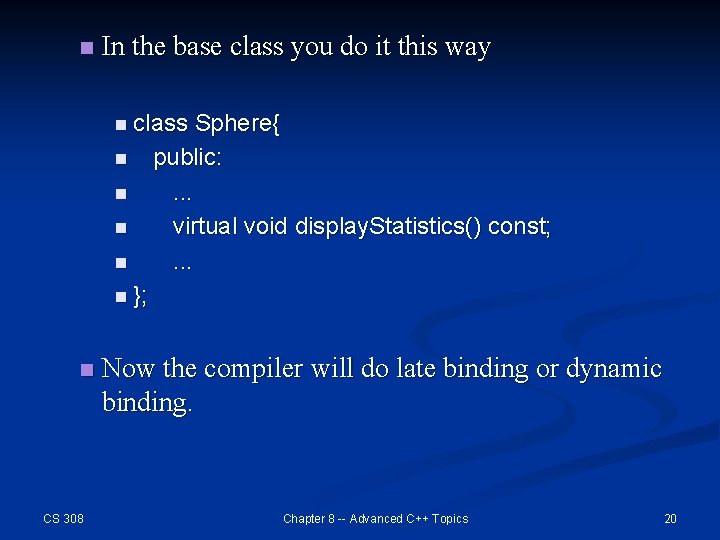
n In the base class you do it this way n class n n Sphere{ public: . . . virtual void display. Statistics() const; . . . n }; n CS 308 Now the compiler will do late binding or dynamic binding. Chapter 8 -- Advanced C++ Topics 20
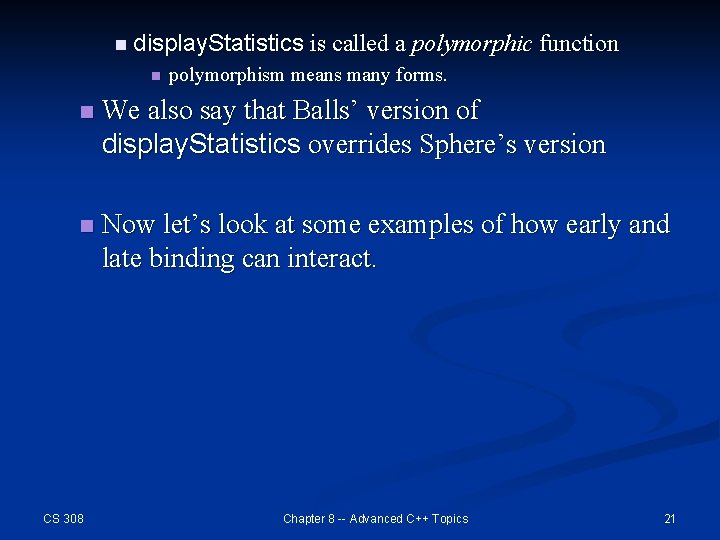
n display. Statistics is called a polymorphic function n polymorphism means many forms. n We also say that Balls’ version of display. Statistics overrides Sphere’s version n Now let’s look at some examples of how early and late binding can interact. CS 308 Chapter 8 -- Advanced C++ Topics 21
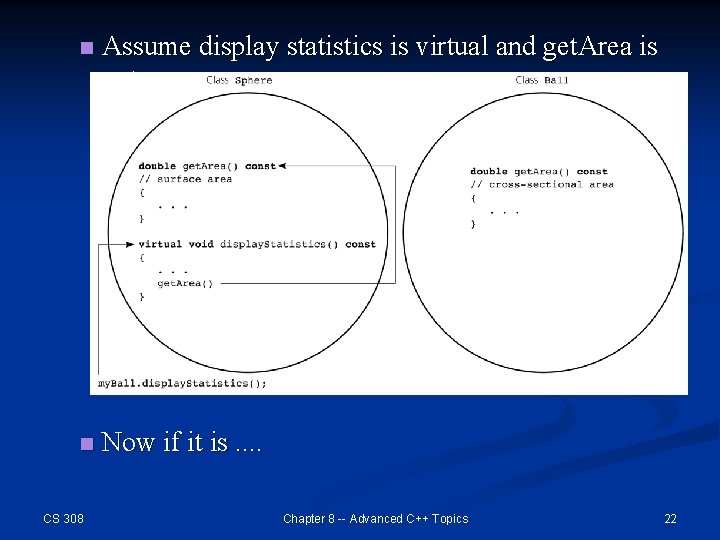
n Assume display statistics is virtual and get. Area is not. n Now if it is. . CS 308 Chapter 8 -- Advanced C++ Topics 22
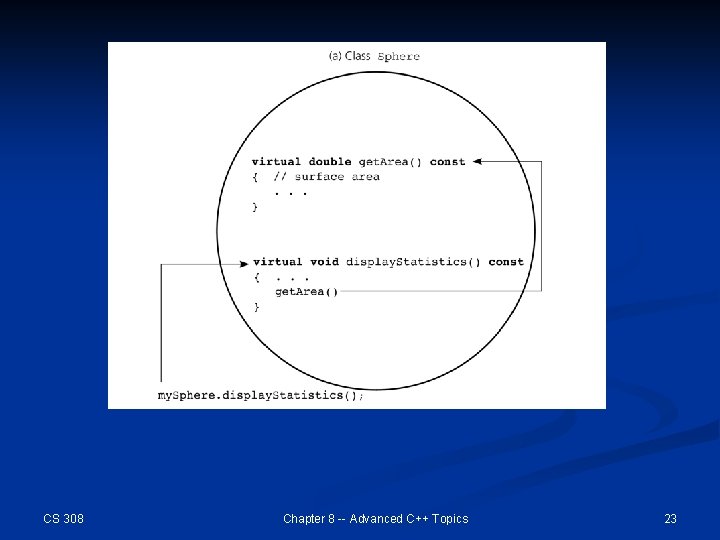
CS 308 Chapter 8 -- Advanced C++ Topics 23
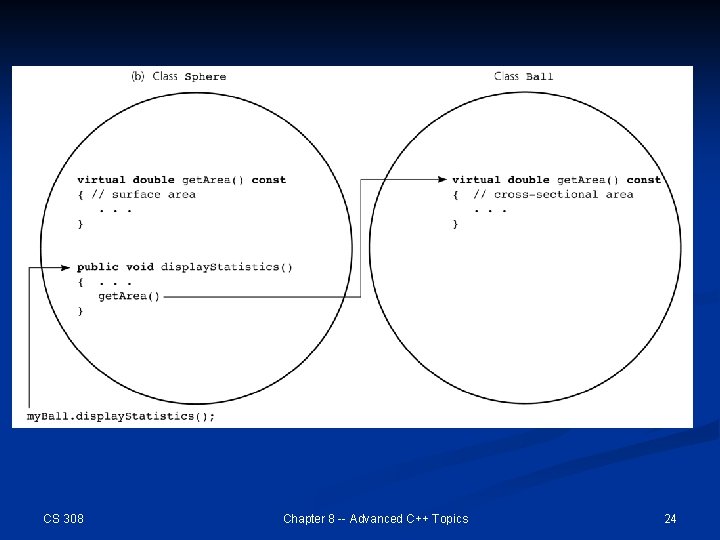
CS 308 Chapter 8 -- Advanced C++ Topics 24
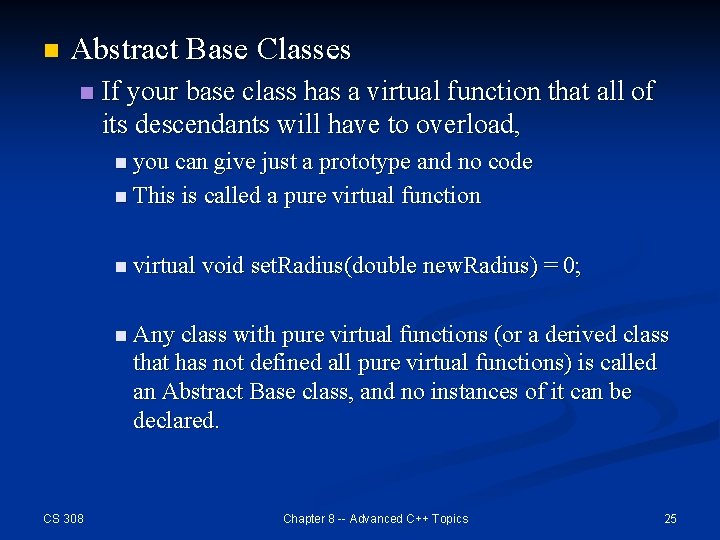
n Abstract Base Classes n If your base class has a virtual function that all of its descendants will have to overload, n you can give just a prototype and no code n This is called a pure virtual function n virtual void set. Radius(double new. Radius) = 0; n Any class with pure virtual functions (or a derived class that has not defined all pure virtual functions) is called an Abstract Base class, and no instances of it can be declared. CS 308 Chapter 8 -- Advanced C++ Topics 25
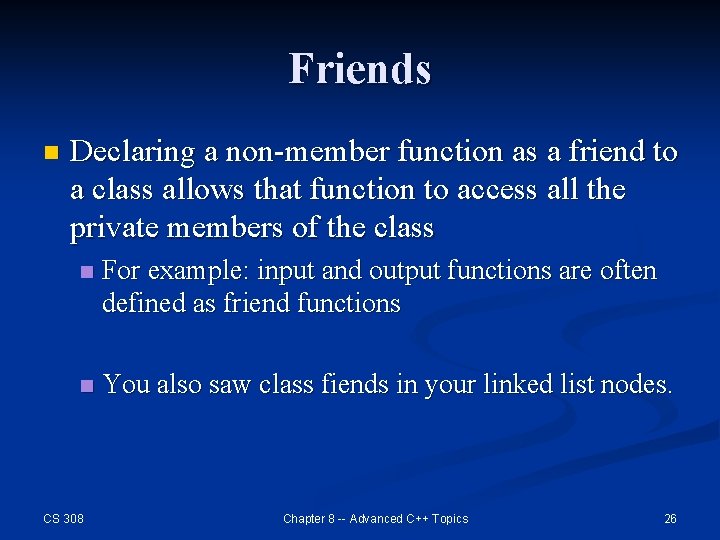
Friends n Declaring a non-member function as a friend to a class allows that function to access all the private members of the class n For example: input and output functions are often defined as friend functions n You also saw class fiends in your linked list nodes. CS 308 Chapter 8 -- Advanced C++ Topics 26
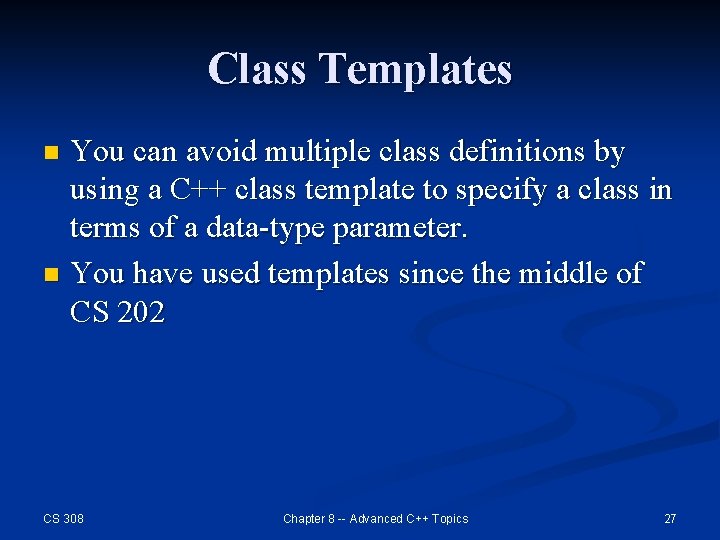
Class Templates You can avoid multiple class definitions by using a C++ class template to specify a class in terms of a data-type parameter. n You have used templates since the middle of CS 202 n CS 308 Chapter 8 -- Advanced C++ Topics 27
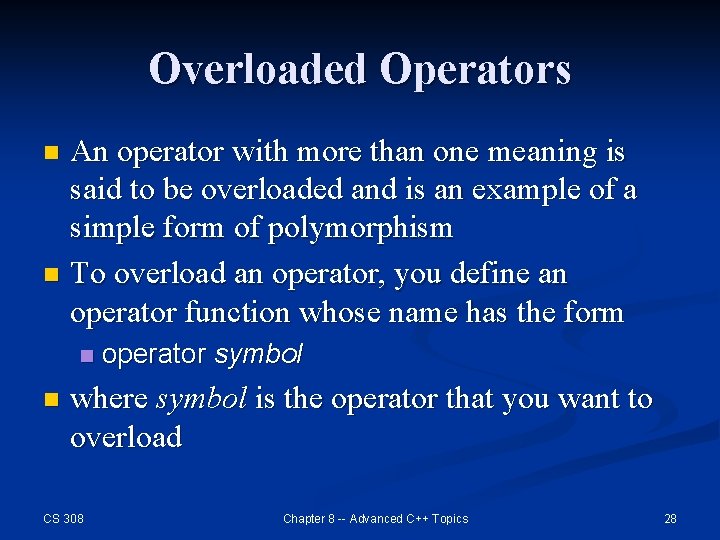
Overloaded Operators An operator with more than one meaning is said to be overloaded and is an example of a simple form of polymorphism n To overload an operator, you define an operator function whose name has the form n n n operator symbol where symbol is the operator that you want to overload CS 308 Chapter 8 -- Advanced C++ Topics 28
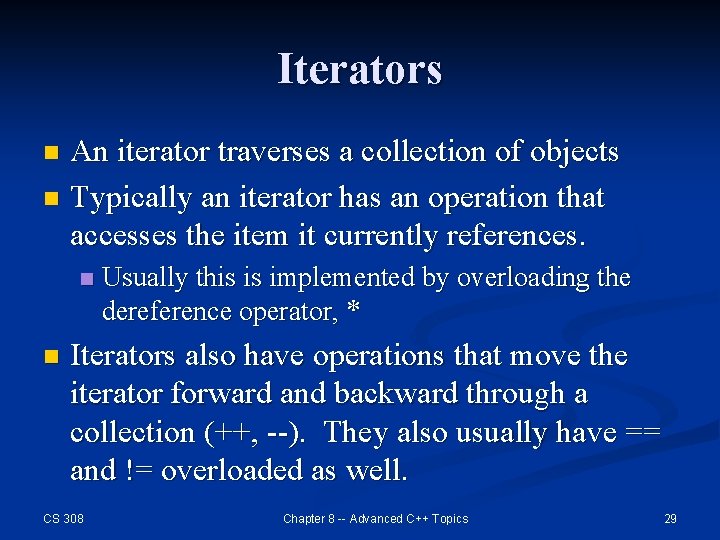
Iterators An iterator traverses a collection of objects n Typically an iterator has an operation that accesses the item it currently references. n n n Usually this is implemented by overloading the dereference operator, * Iterators also have operations that move the iterator forward and backward through a collection (++, --). They also usually have == and != overloaded as well. CS 308 Chapter 8 -- Advanced C++ Topics 29
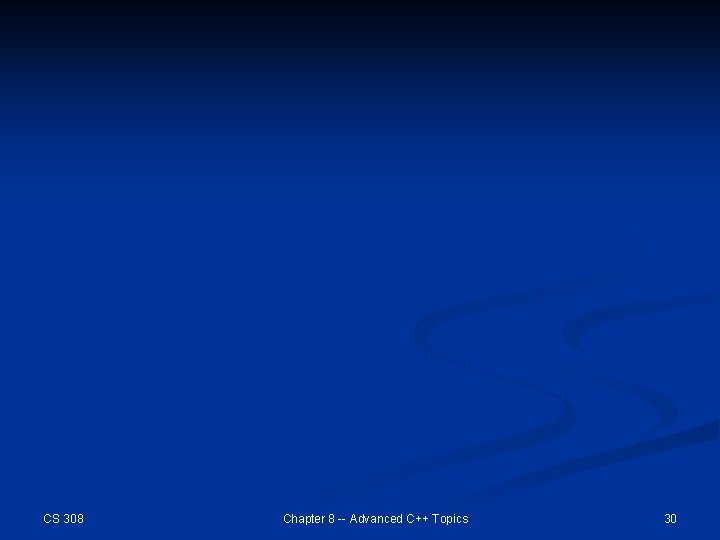
CS 308 Chapter 8 -- Advanced C++ Topics 30
Cs 527
Angular guard naming convention
Advanced topics in angular
Advanced c topics
Advanced topics in web development
Android advanced topics
Advanced topics in computer science
The present progressive tense (p. 308)
Elaun makan dan elaun harian
Fw 308
Palsmodesmata
Cmpt 308
Akta tadika
Ona tili 5-sinf 326-mashq
Bot 308
Udi dahan
308
Sec 308
Red hartebeest shot placement
2cfr200.308
Chapter 9 topics in analytic geometry
Chapter 6 advanced shielded metal arc welding
Pseudocde
Advanced evolution chapter 4
Advanced evolution chapter 8
Advanced accounting chapter 1
School magazine topics
Good essay topics for grade 9
Thematic essay conclusion
Behavioural training topics
Skill 15 listen for untrue conditions