Chapter 14 Advanced C Topics Outline 14 1
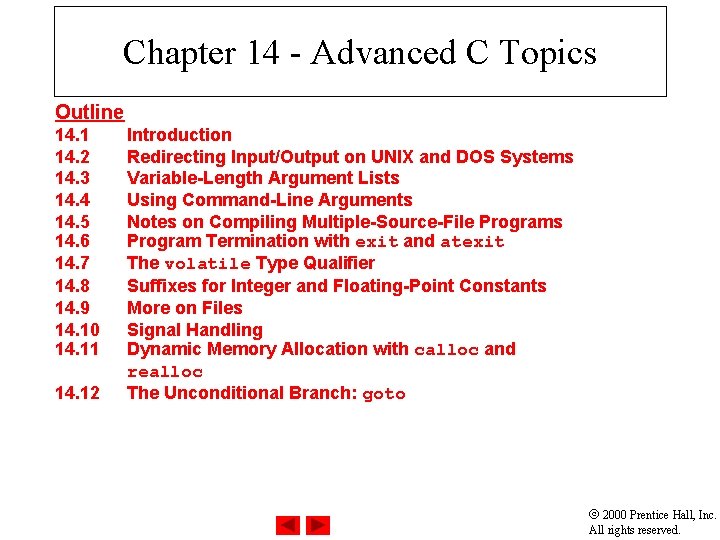
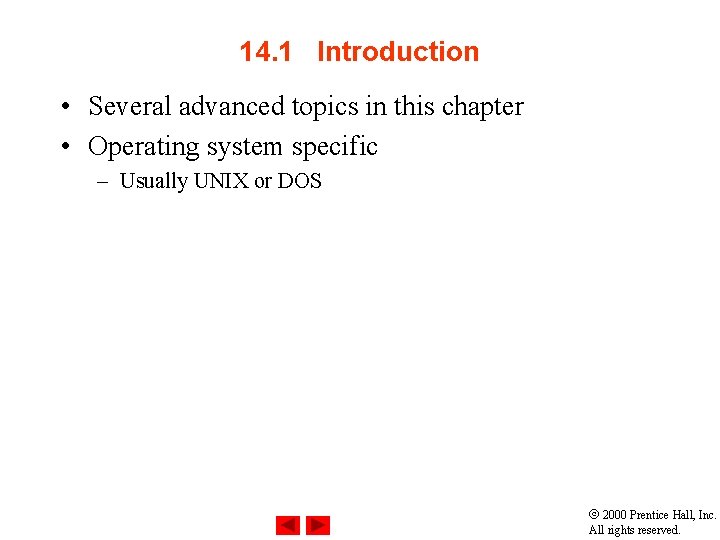
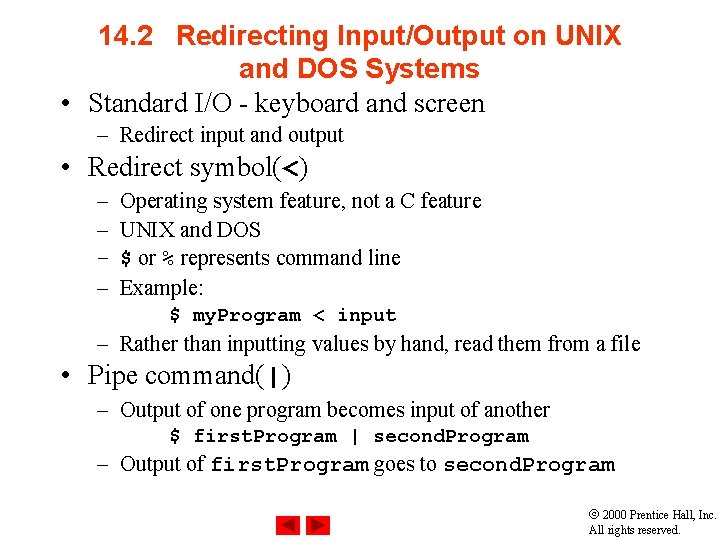
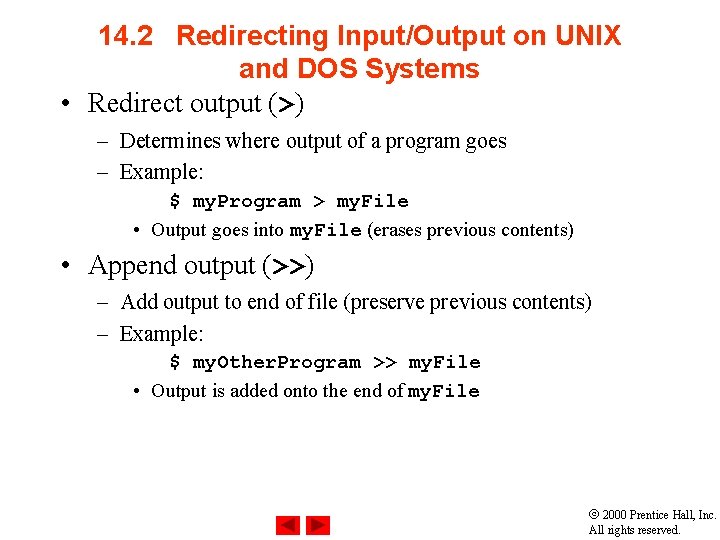
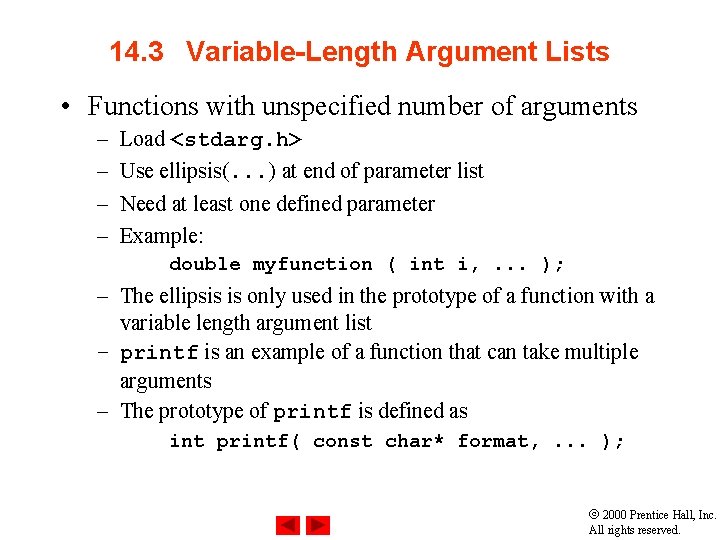
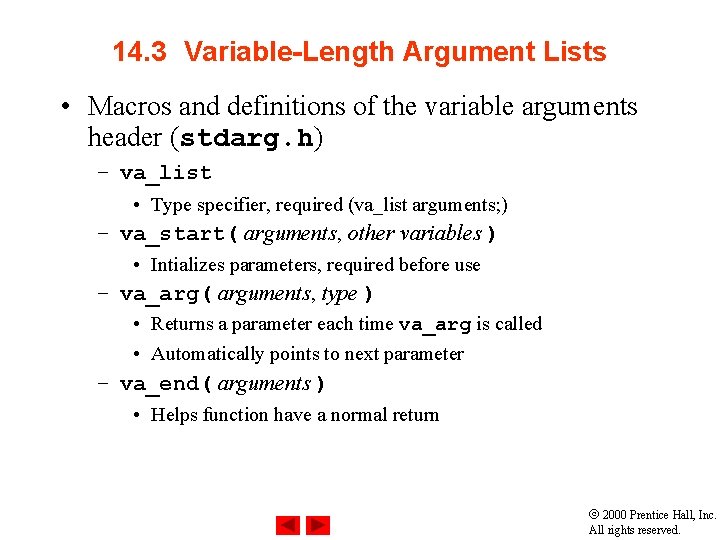
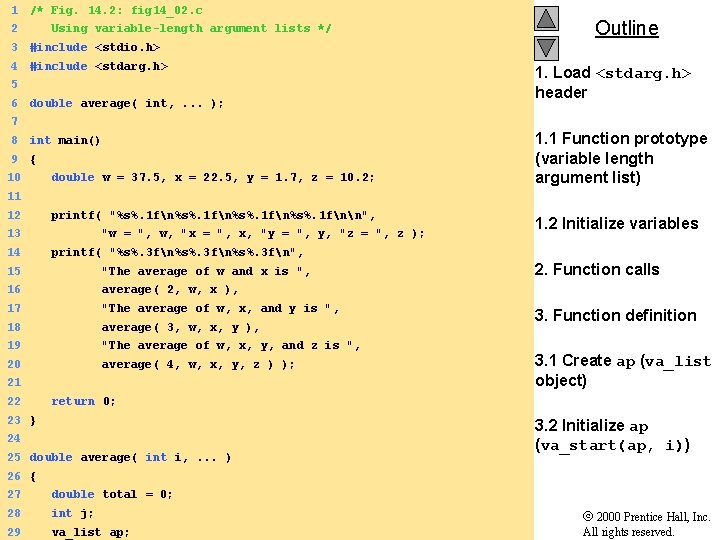
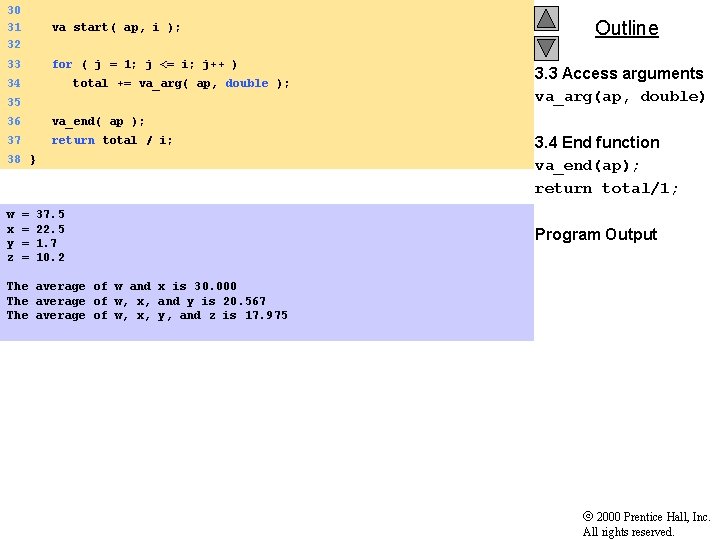
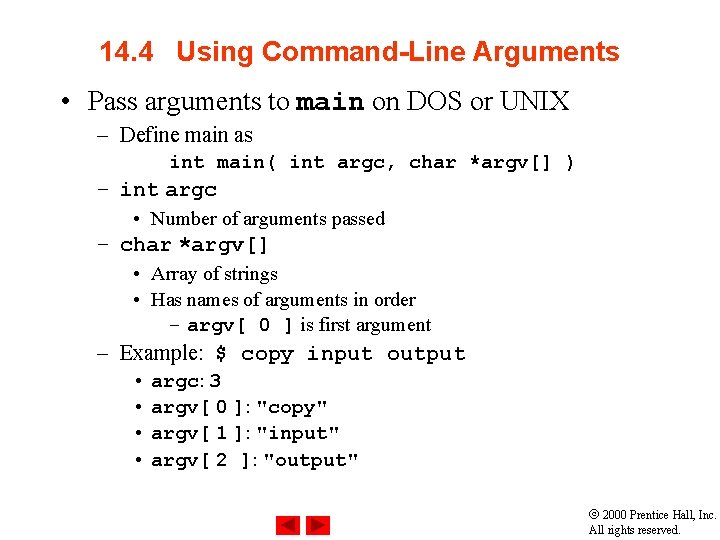
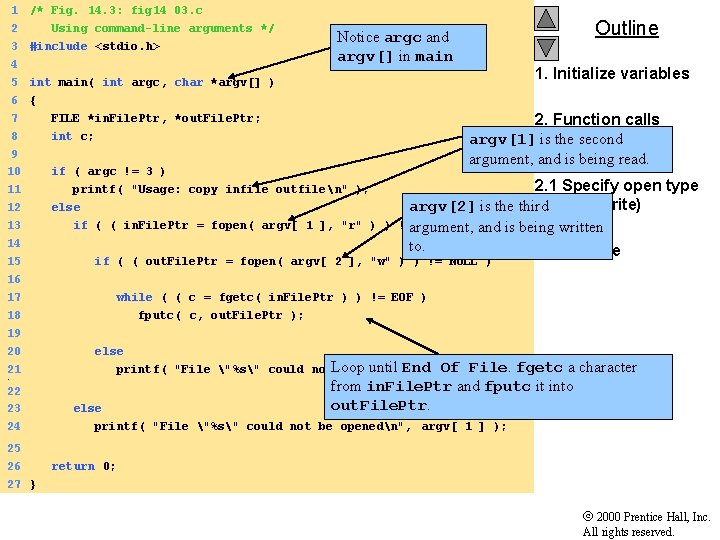
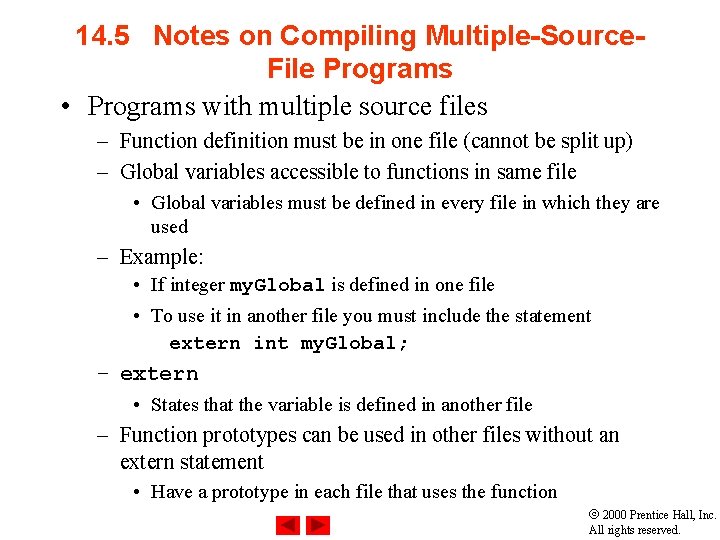
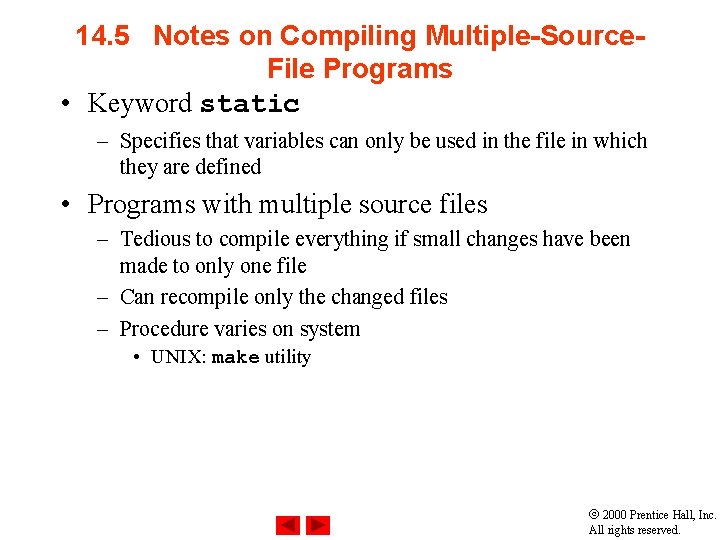
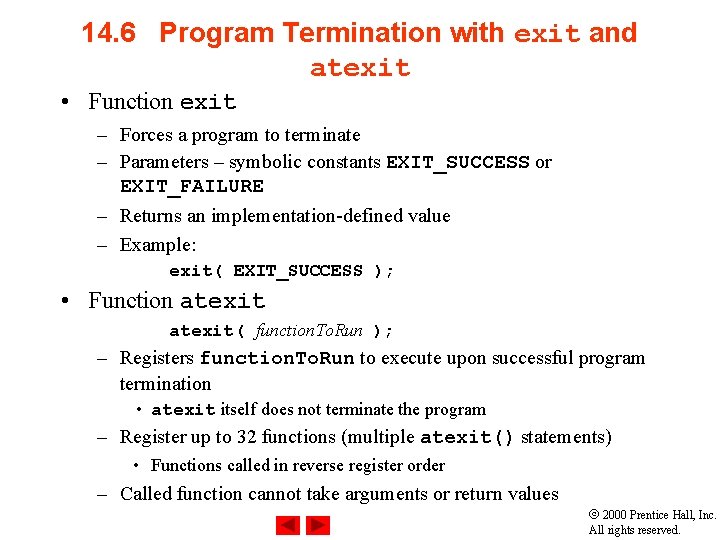
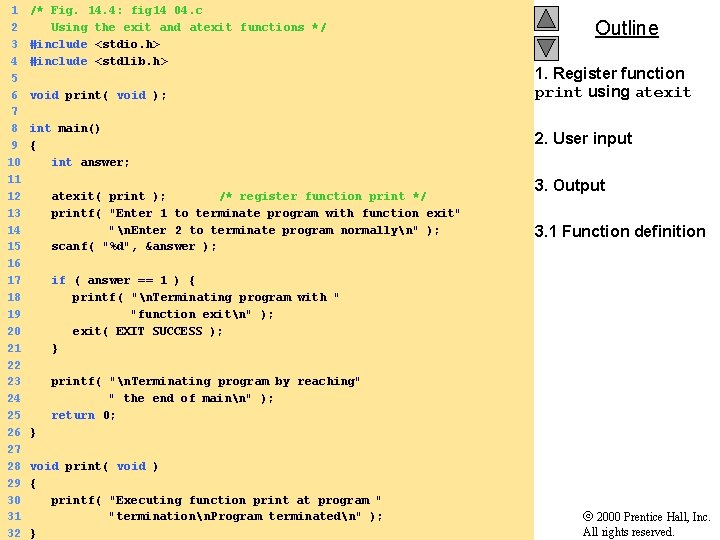
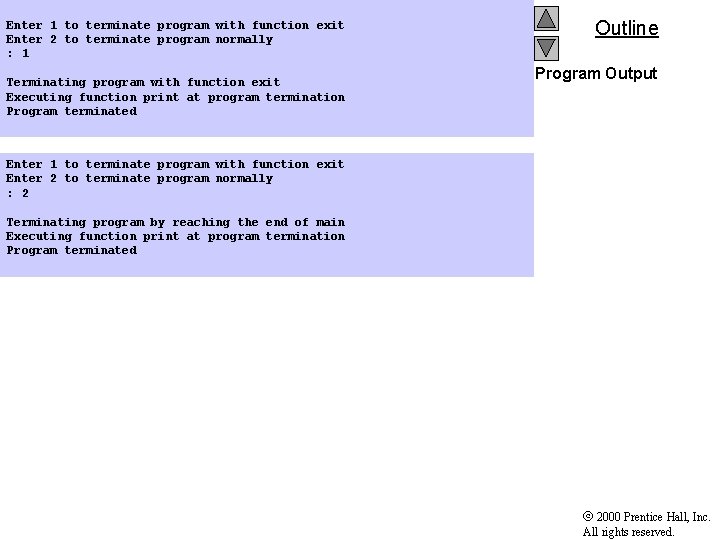
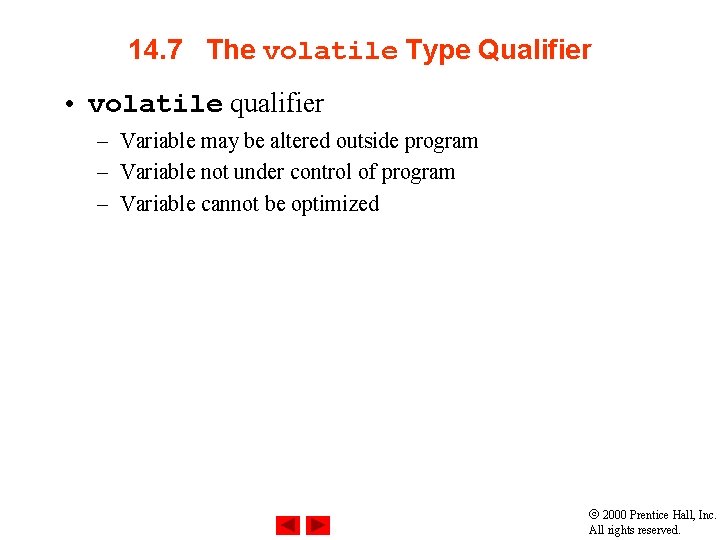
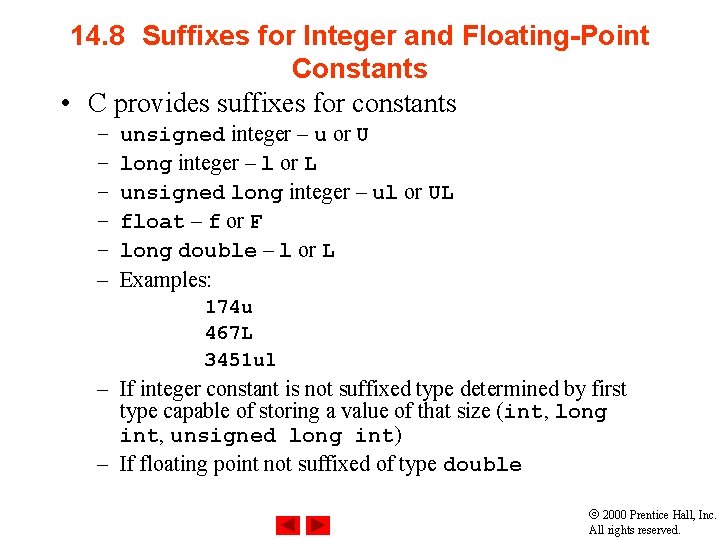
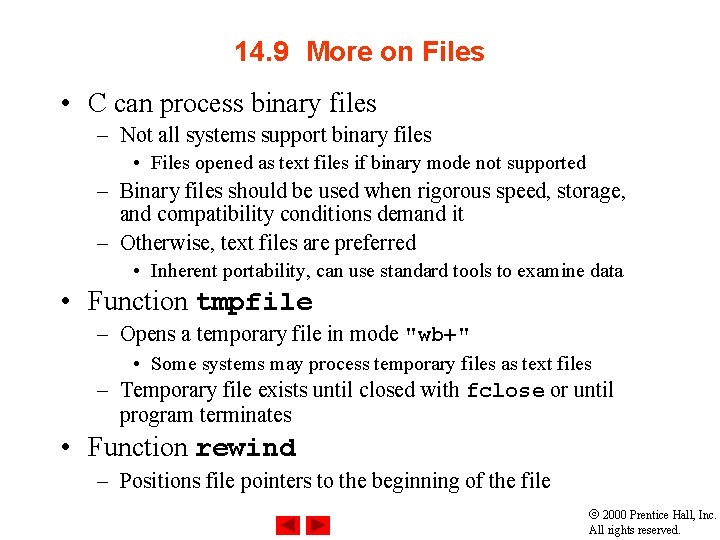
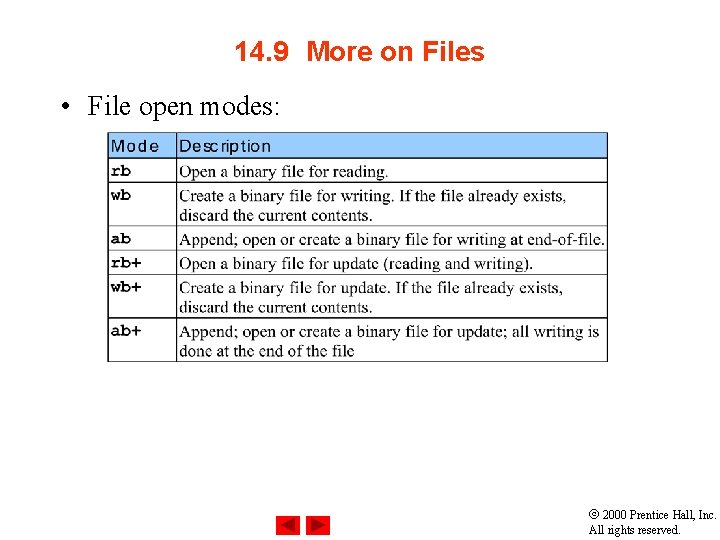
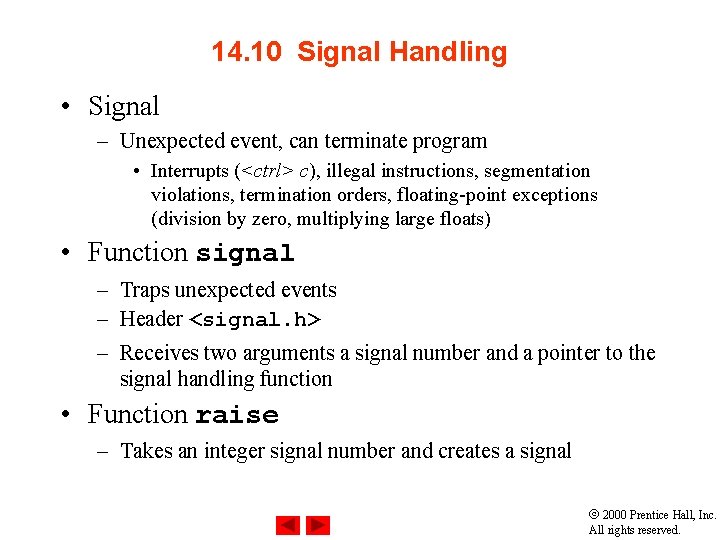
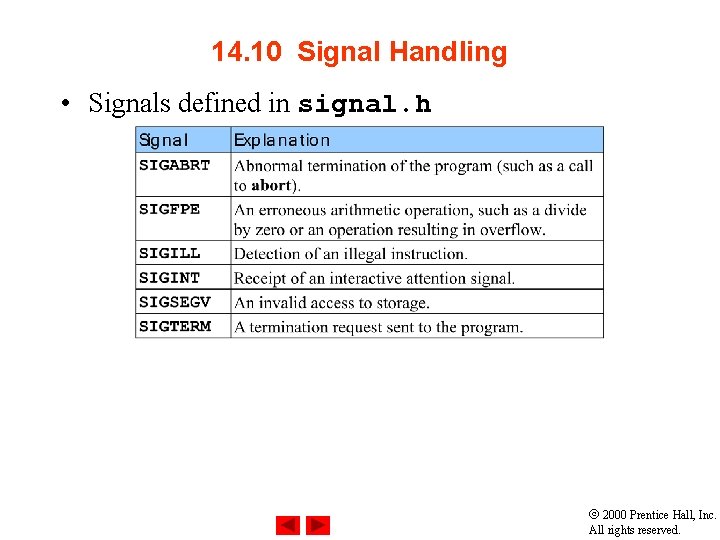
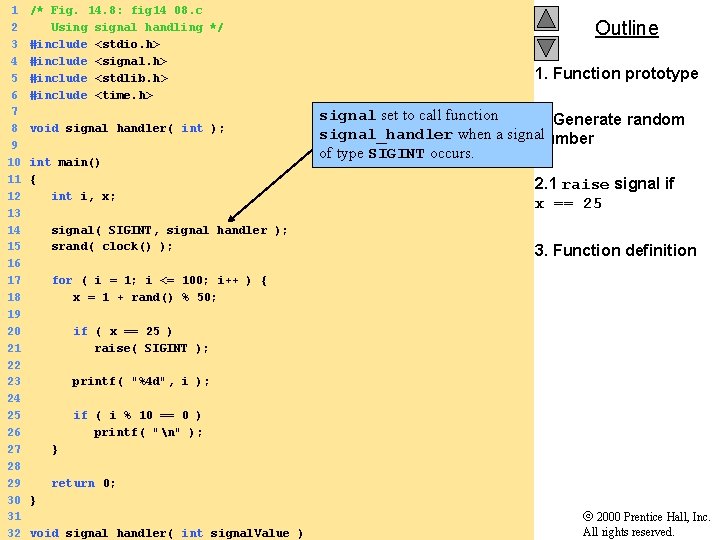
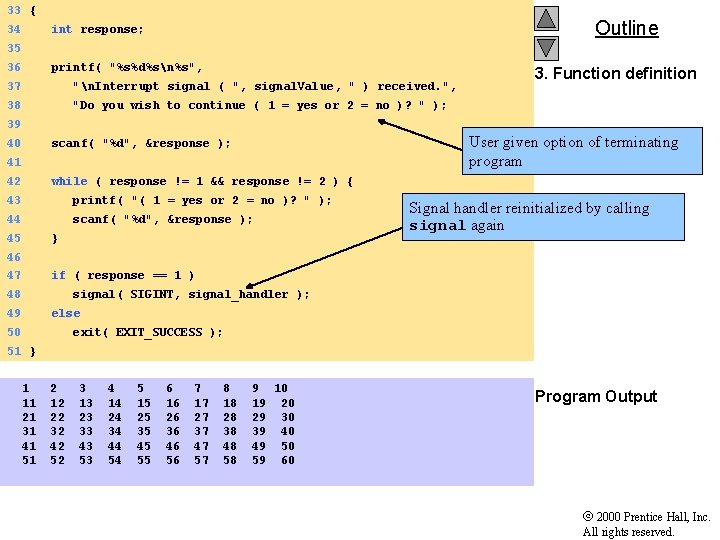
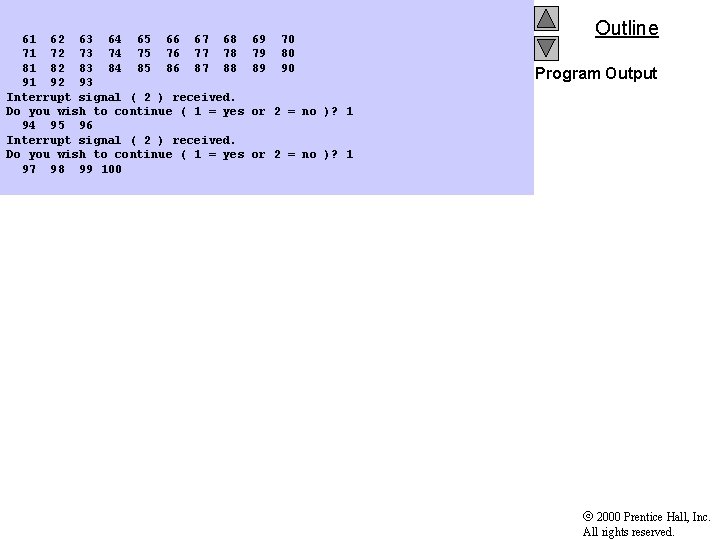
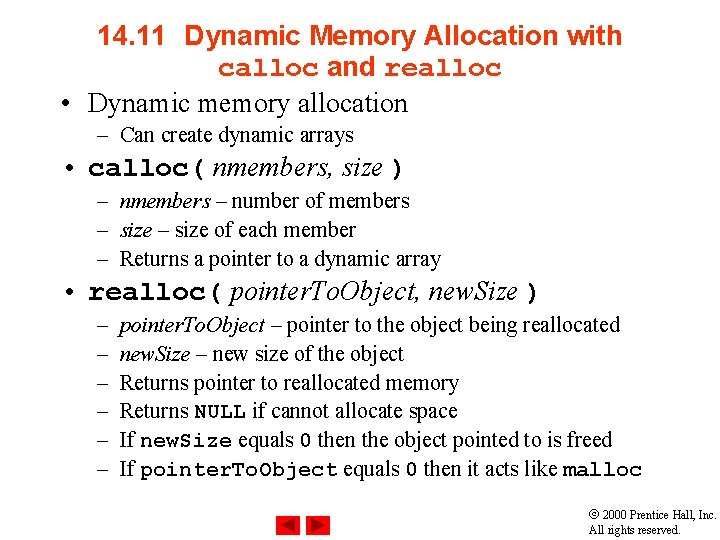
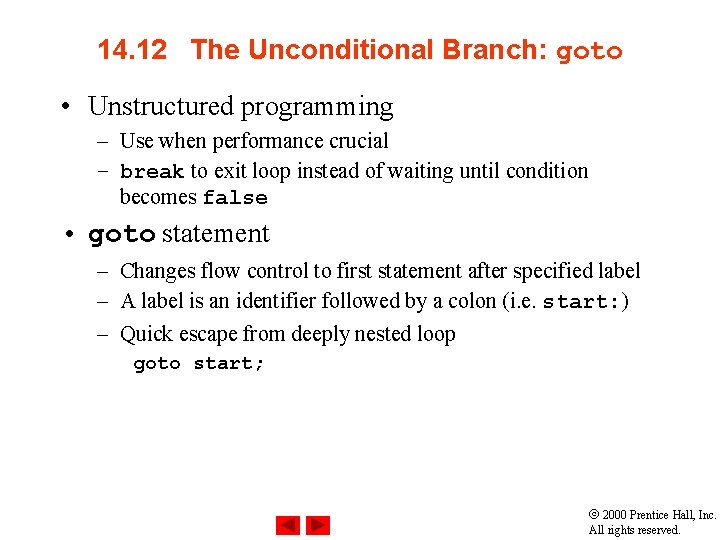
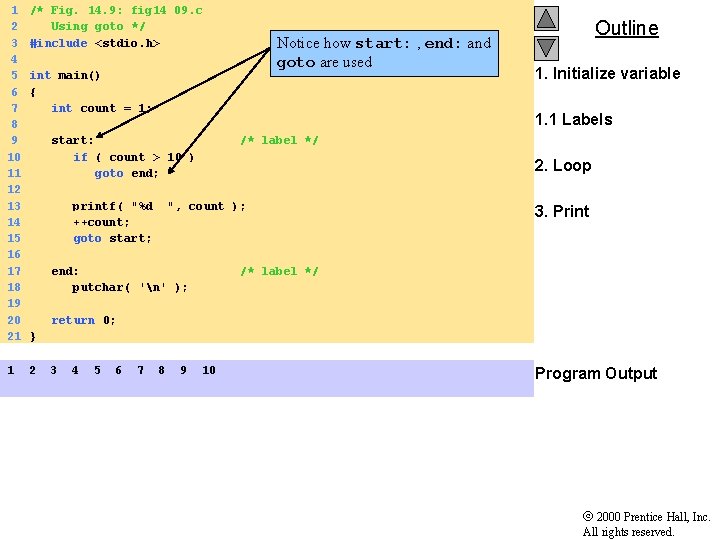
- Slides: 27
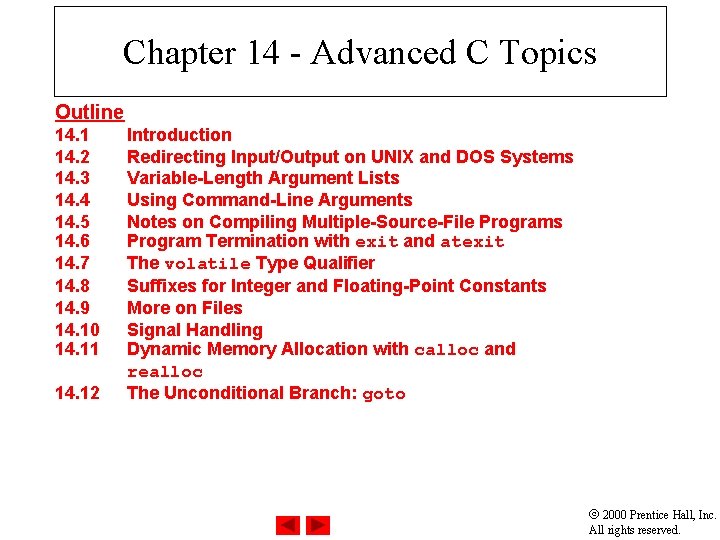
Chapter 14 - Advanced C Topics Outline 14. 1 14. 2 14. 3 14. 4 14. 5 14. 6 14. 7 14. 8 14. 9 14. 10 14. 11 14. 12 Introduction Redirecting Input/Output on UNIX and DOS Systems Variable-Length Argument Lists Using Command-Line Arguments Notes on Compiling Multiple-Source-File Programs Program Termination with exit and atexit The volatile Type Qualifier Suffixes for Integer and Floating-Point Constants More on Files Signal Handling Dynamic Memory Allocation with calloc and realloc The Unconditional Branch: goto 2000 Prentice Hall, Inc. All rights reserved.
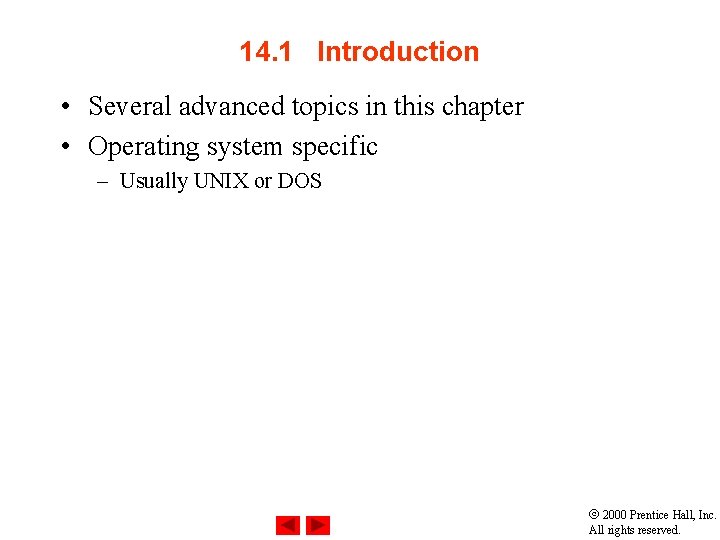
14. 1 Introduction • Several advanced topics in this chapter • Operating system specific – Usually UNIX or DOS 2000 Prentice Hall, Inc. All rights reserved.
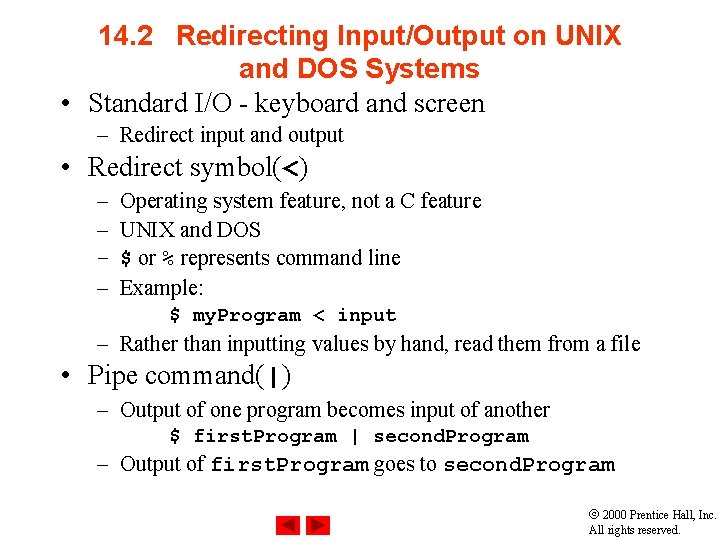
14. 2 Redirecting Input/Output on UNIX and DOS Systems • Standard I/O - keyboard and screen – Redirect input and output • Redirect symbol(<) – – Operating system feature, not a C feature UNIX and DOS $ or % represents command line Example: $ my. Program < input – Rather than inputting values by hand, read them from a file • Pipe command(|) – Output of one program becomes input of another $ first. Program | second. Program – Output of first. Program goes to second. Program 2000 Prentice Hall, Inc. All rights reserved.
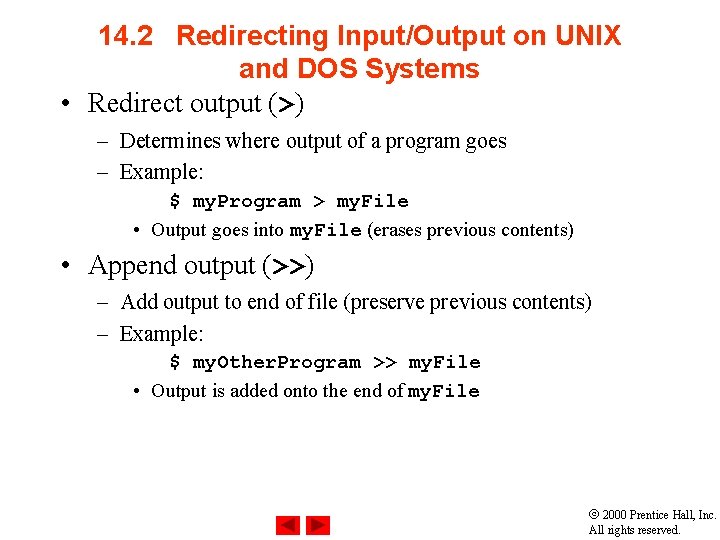
14. 2 Redirecting Input/Output on UNIX and DOS Systems • Redirect output (>) – Determines where output of a program goes – Example: $ my. Program > my. File • Output goes into my. File (erases previous contents) • Append output (>>) – Add output to end of file (preserve previous contents) – Example: $ my. Other. Program >> my. File • Output is added onto the end of my. File 2000 Prentice Hall, Inc. All rights reserved.
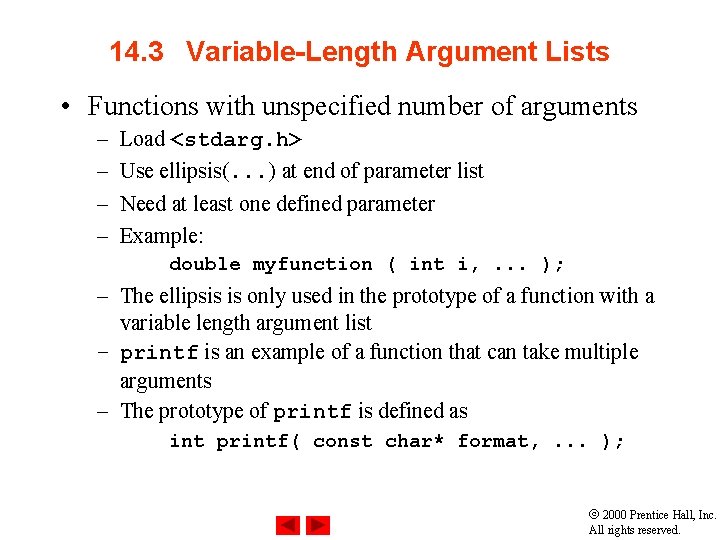
14. 3 Variable-Length Argument Lists • Functions with unspecified number of arguments – – Load <stdarg. h> Use ellipsis(. . . ) at end of parameter list Need at least one defined parameter Example: double myfunction ( int i, . . . ); – The ellipsis is only used in the prototype of a function with a variable length argument list – printf is an example of a function that can take multiple arguments – The prototype of printf is defined as int printf( const char* format, . . . ); 2000 Prentice Hall, Inc. All rights reserved.
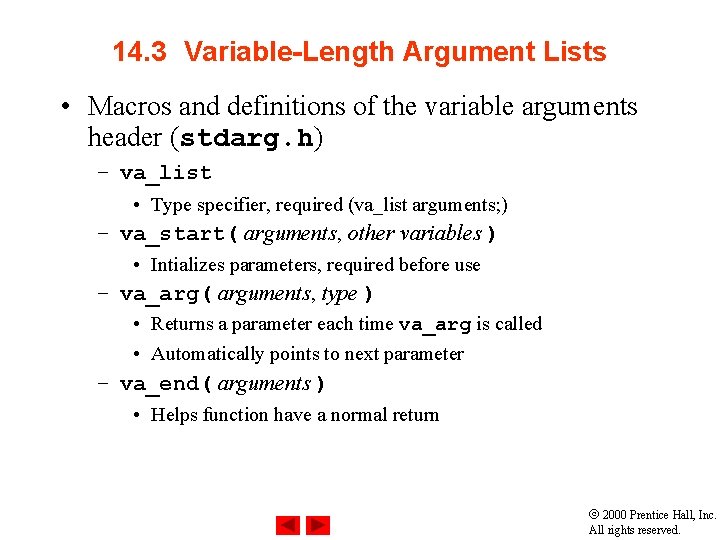
14. 3 Variable-Length Argument Lists • Macros and definitions of the variable arguments header (stdarg. h) – va_list • Type specifier, required (va_list arguments; ) – va_start( arguments, other variables ) • Intializes parameters, required before use – va_arg( arguments, type ) • Returns a parameter each time va_arg is called • Automatically points to next parameter – va_end( arguments ) • Helps function have a normal return 2000 Prentice Hall, Inc. All rights reserved.
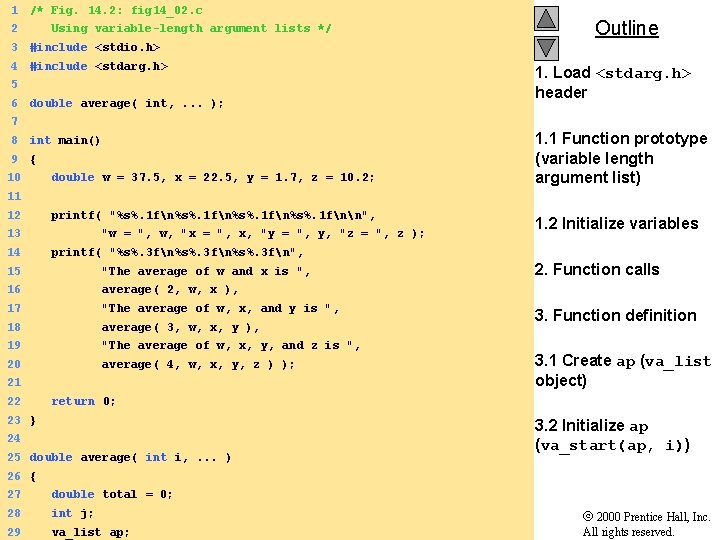
1 /* Fig. 14. 2: fig 14_02. c 2 Using variable-length argument lists */ 3 #include <stdio. h> 4 #include <stdarg. h> 5 6 double average( int, . . . ); Outline 1. Load <stdarg. h> header 7 8 int main() 9 { 10 double w = 37. 5, x = 22. 5, y = 1. 7, z = 10. 2; 1. 1 Function prototype (variable length argument list) 11 12 printf( "%s%. 1 fn%s%. 1 fnn", 13 14 "w = ", w, "x = ", x, "y = ", y, "z = ", z ); printf( "%s%. 3 fn%s%. 3 fn", 15 "The average of w and x is ", 16 average( 2, w, x ), 17 "The average of w, x, and y is ", 18 average( 3, w, x, y ), 19 "The average of w, x, y, and z is ", 20 average( 4, w, x, y, z ) ); 21 22 1. 2 Initialize variables 2. Function calls 3. Function definition 3. 1 Create ap (va_list object) return 0; 23 } 24 25 double average( int i, . . . ) 3. 2 Initialize ap (va_start(ap, i)) 26 { 27 double total = 0; 28 int j; 2000 Prentice Hall, Inc. 29 va_list ap; All rights reserved.
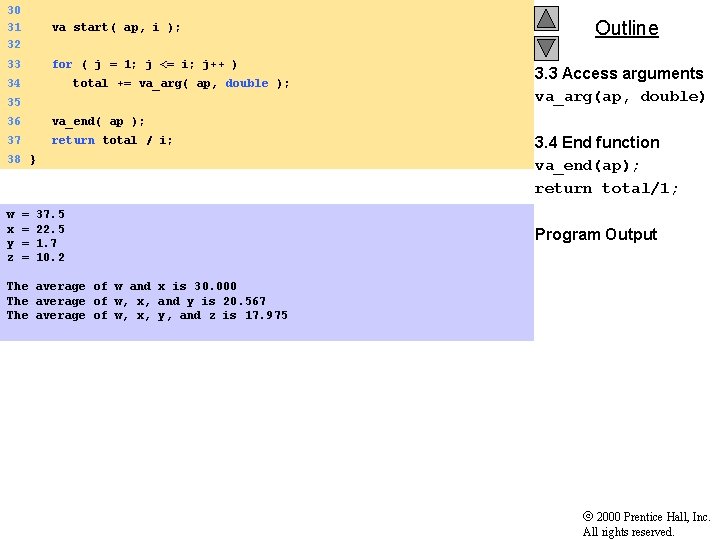
30 31 32 va_start( ap, i ); 33 for ( j = 1; j <= i; j++ ) 34 total += va_arg( ap, double ); 35 36 va_end( ap ); 37 return total / i; Outline 3. 3 Access arguments va_arg(ap, double) 38 } 3. 4 End function va_end(ap); return total/1; w x y z Program Output = = 37. 5 22. 5 1. 7 10. 2 The average of w and x is 30. 000 The average of w, x, and y is 20. 567 The average of w, x, y, and z is 17. 975 2000 Prentice Hall, Inc. All rights reserved.
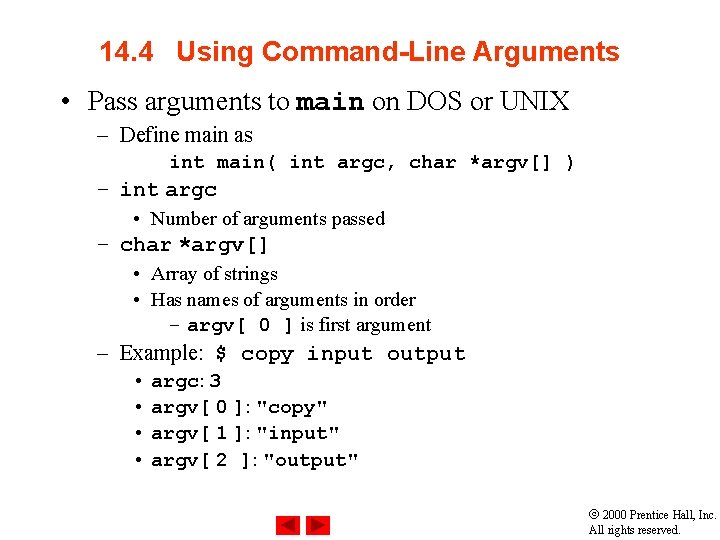
14. 4 Using Command-Line Arguments • Pass arguments to main on DOS or UNIX – Define main as int main( int argc, char *argv[] ) – int argc • Number of arguments passed – char *argv[] • Array of strings • Has names of arguments in order – argv[ 0 ] is first argument – Example: $ copy input output • argc: 3 • argv[ 0 ]: "copy" • argv[ 1 ]: "input" • argv[ 2 ]: "output" 2000 Prentice Hall, Inc. All rights reserved.
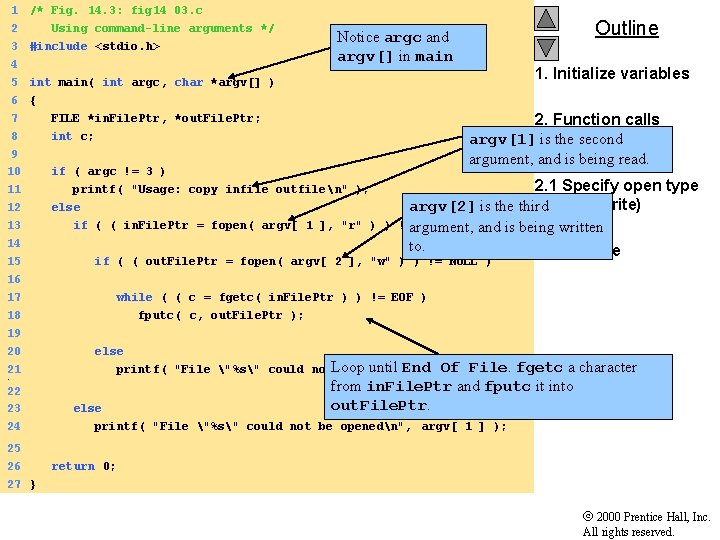
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 ); 22 23 24 /* Fig. 14. 3: fig 14_03. c Using command-line arguments */ #include <stdio. h> int main( int argc, char *argv[] ) { FILE *in. File. Ptr, *out. File. Ptr; int c; 25 26 27 } Outline Notice argc and argv[] in main 1. Initialize variables 2. Function calls argv[1](fopen) is the second argument, and is being read. if ( argc != 3 ) 2. 1 Specify open printf( "Usage: copy infile outfilen" ); (read or write) argv[2] is the third else if ( ( in. File. Ptr = fopen( argv[ 1 ], "r" ) ) !=argument, NULL ) and is being written to. if ( ( out. File. Ptr = fopen( argv[ 2 ], "w" ) ) != NULL ) type 3. Copy file while ( ( c = fgetc( in. File. Ptr ) ) != EOF ) fputc( c, out. File. Ptr ); else until End Of File. printf( "File "%s" could not. Loop be openedn", argv[ 2 ] fgetc a character from in. File. Ptr and fputc it into out. File. Ptr. else printf( "File "%s" could not be openedn", argv[ 1 ] ); return 0; 2000 Prentice Hall, Inc. All rights reserved.
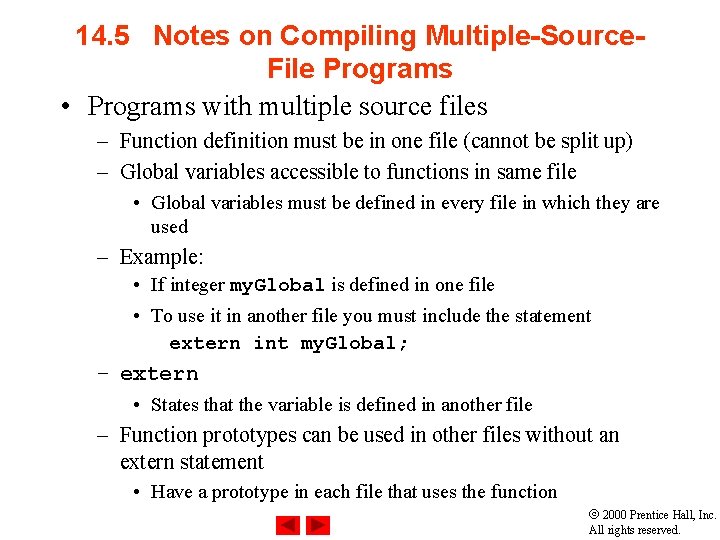
14. 5 Notes on Compiling Multiple-Source. File Programs • Programs with multiple source files – Function definition must be in one file (cannot be split up) – Global variables accessible to functions in same file • Global variables must be defined in every file in which they are used – Example: • If integer my. Global is defined in one file • To use it in another file you must include the statement extern int my. Global; – extern • States that the variable is defined in another file – Function prototypes can be used in other files without an extern statement • Have a prototype in each file that uses the function 2000 Prentice Hall, Inc. All rights reserved.
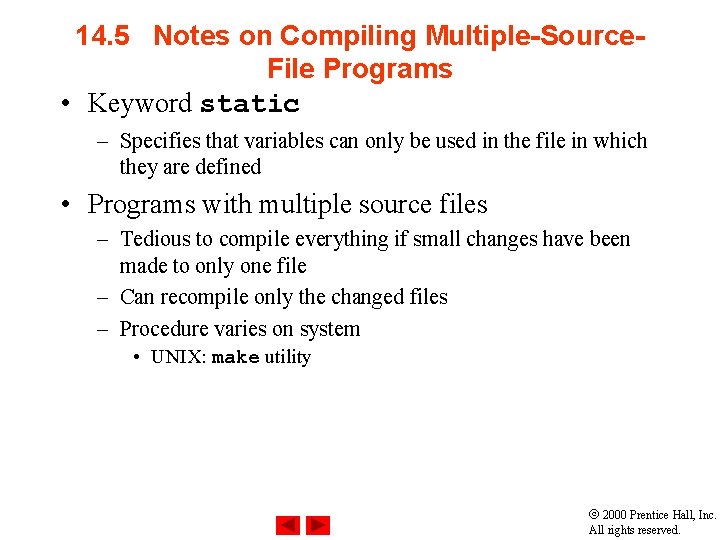
14. 5 Notes on Compiling Multiple-Source. File Programs • Keyword static – Specifies that variables can only be used in the file in which they are defined • Programs with multiple source files – Tedious to compile everything if small changes have been made to only one file – Can recompile only the changed files – Procedure varies on system • UNIX: make utility 2000 Prentice Hall, Inc. All rights reserved.
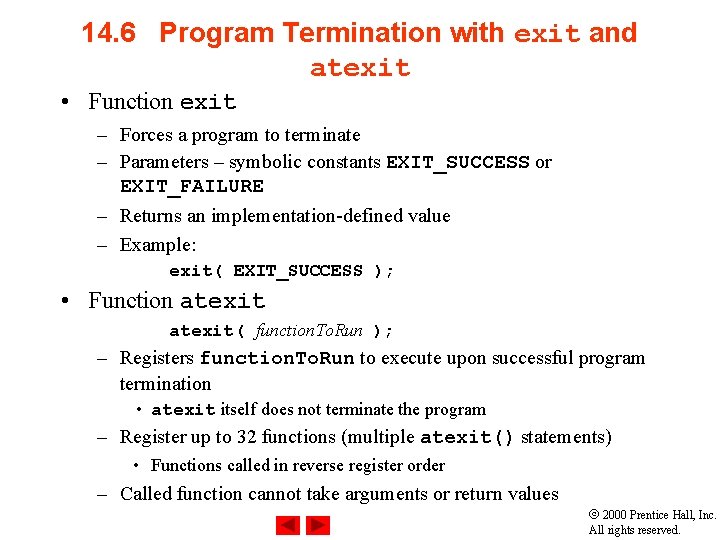
14. 6 Program Termination with exit and atexit • Function exit – Forces a program to terminate – Parameters – symbolic constants EXIT_SUCCESS or EXIT_FAILURE – Returns an implementation-defined value – Example: exit( EXIT_SUCCESS ); • Function atexit( function. To. Run ); – Registers function. To. Run to execute upon successful program termination • atexit itself does not terminate the program – Register up to 32 functions (multiple atexit() statements) • Functions called in reverse register order – Called function cannot take arguments or return values 2000 Prentice Hall, Inc. All rights reserved.
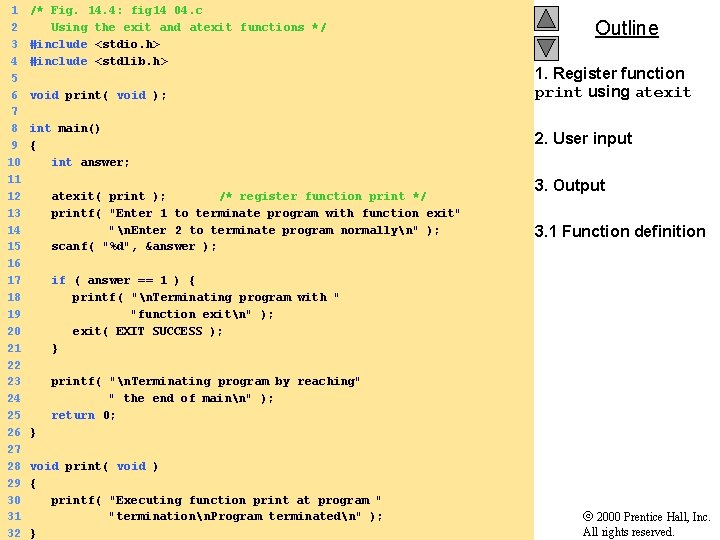
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 /* Fig. 14. 4: fig 14_04. c Using the exit and atexit functions */ #include <stdio. h> #include <stdlib. h> void print( void ); int main() { int answer; atexit( print ); /* register function print */ printf( "Enter 1 to terminate program with function exit" "n. Enter 2 to terminate program normallyn" ); scanf( "%d", &answer ); Outline 1. Register function print using atexit 2. User input 3. Output 3. 1 Function definition if ( answer == 1 ) { printf( "n. Terminating program with " "function exitn" ); exit( EXIT_SUCCESS ); } printf( "n. Terminating program by reaching" " the end of mainn" ); return 0; } void print( void ) { printf( "Executing function print at program " "termination n. Program terminatedn" ); } 2000 Prentice Hall, Inc. All rights reserved.
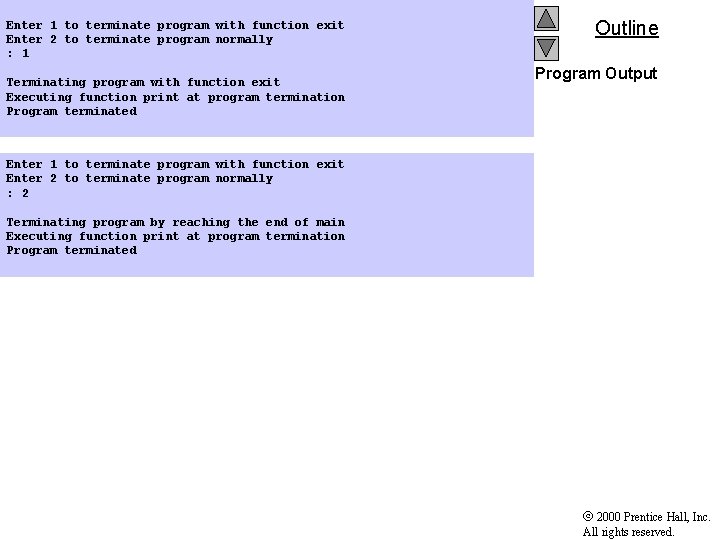
Enter 1 to terminate program with function exit Enter 2 to terminate program normally : 1 Terminating program with function exit Executing function print at program termination Program terminated Outline Program Output Enter 1 to terminate program with function exit Enter 2 to terminate program normally : 2 Terminating program by reaching the end of main Executing function print at program termination Program terminated 2000 Prentice Hall, Inc. All rights reserved.
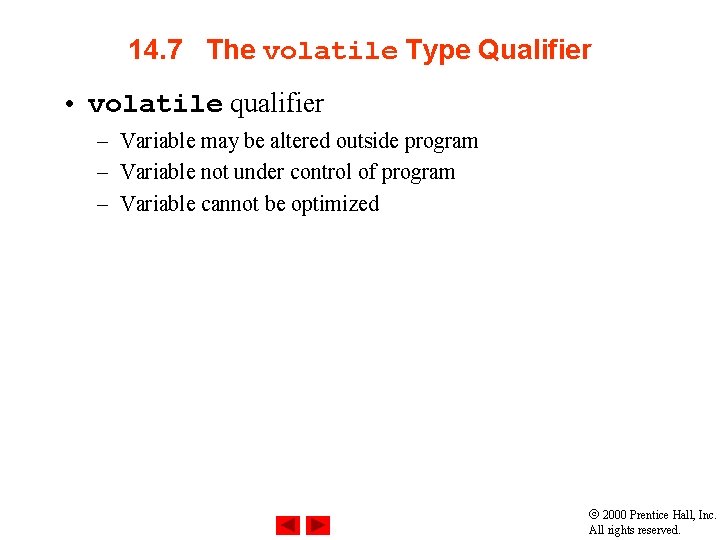
14. 7 The volatile Type Qualifier • volatile qualifier – Variable may be altered outside program – Variable not under control of program – Variable cannot be optimized 2000 Prentice Hall, Inc. All rights reserved.
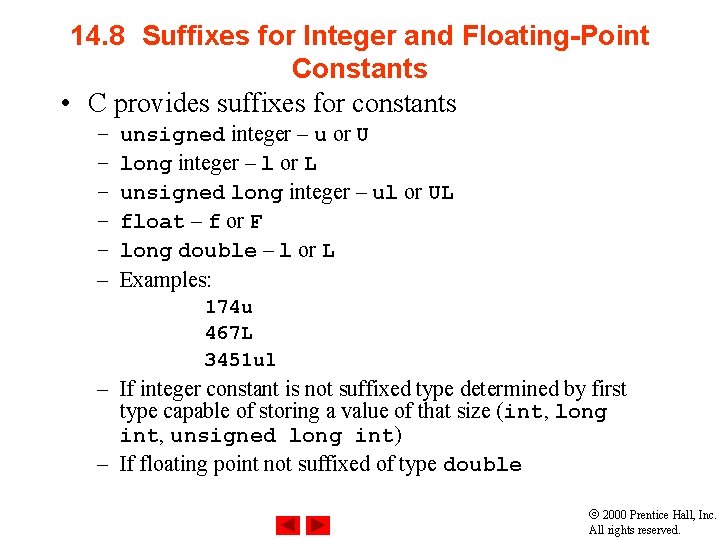
14. 8 Suffixes for Integer and Floating-Point Constants • C provides suffixes for constants – – – unsigned integer – u or U long integer – l or L unsigned long integer – ul or UL float – f or F long double – l or L Examples: 174 u 467 L 3451 ul – If integer constant is not suffixed type determined by first type capable of storing a value of that size (int, long int, unsigned long int) – If floating point not suffixed of type double 2000 Prentice Hall, Inc. All rights reserved.
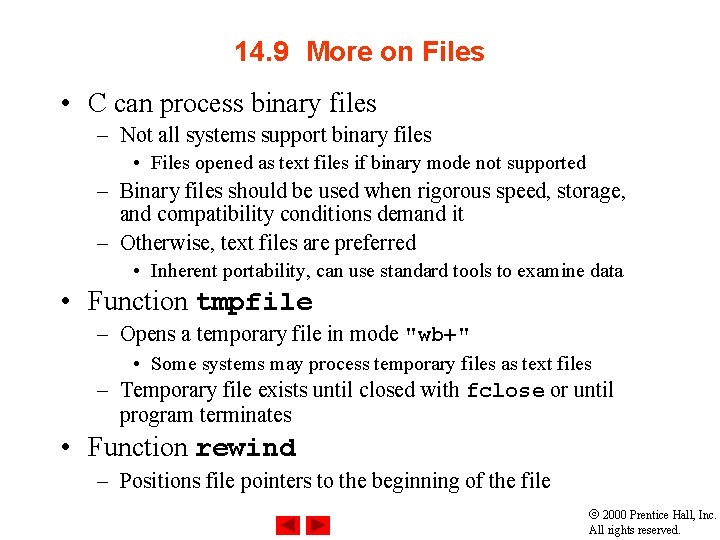
14. 9 More on Files • C can process binary files – Not all systems support binary files • Files opened as text files if binary mode not supported – Binary files should be used when rigorous speed, storage, and compatibility conditions demand it – Otherwise, text files are preferred • Inherent portability, can use standard tools to examine data • Function tmpfile – Opens a temporary file in mode "wb+" • Some systems may process temporary files as text files – Temporary file exists until closed with fclose or until program terminates • Function rewind – Positions file pointers to the beginning of the file 2000 Prentice Hall, Inc. All rights reserved.
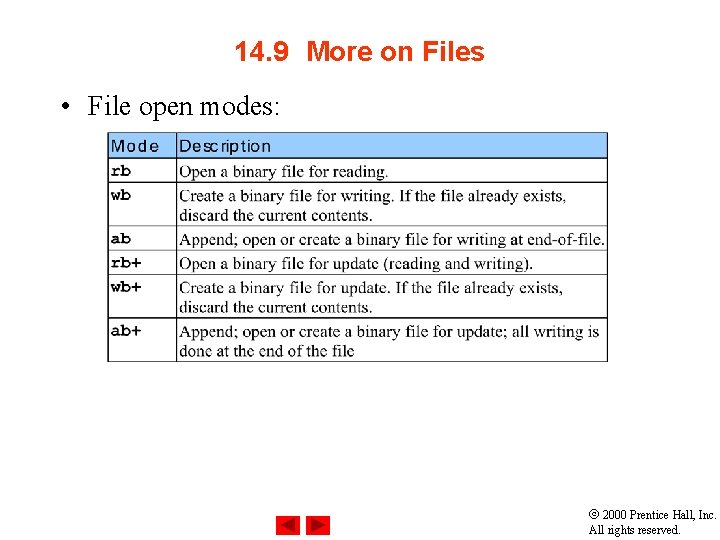
14. 9 More on Files • File open modes: 2000 Prentice Hall, Inc. All rights reserved.
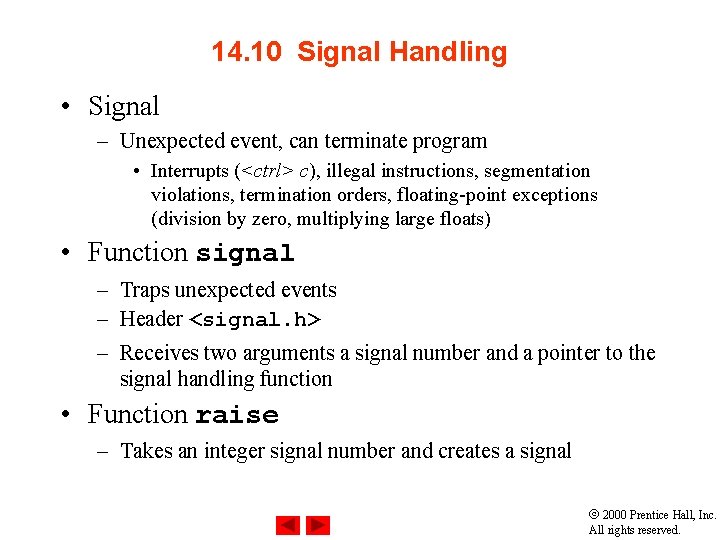
14. 10 Signal Handling • Signal – Unexpected event, can terminate program • Interrupts (<ctrl> c), illegal instructions, segmentation violations, termination orders, floating-point exceptions (division by zero, multiplying large floats) • Function signal – Traps unexpected events – Header <signal. h> – Receives two arguments a signal number and a pointer to the signal handling function • Function raise – Takes an integer signal number and creates a signal 2000 Prentice Hall, Inc. All rights reserved.
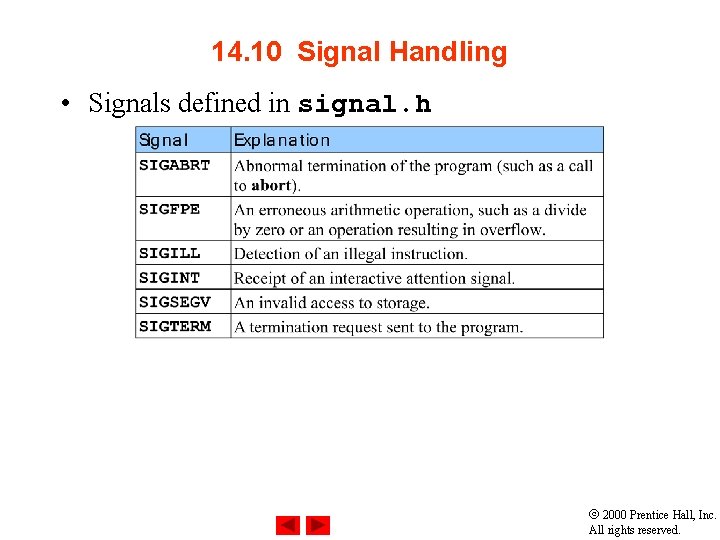
14. 10 Signal Handling • Signals defined in signal. h 2000 Prentice Hall, Inc. All rights reserved.
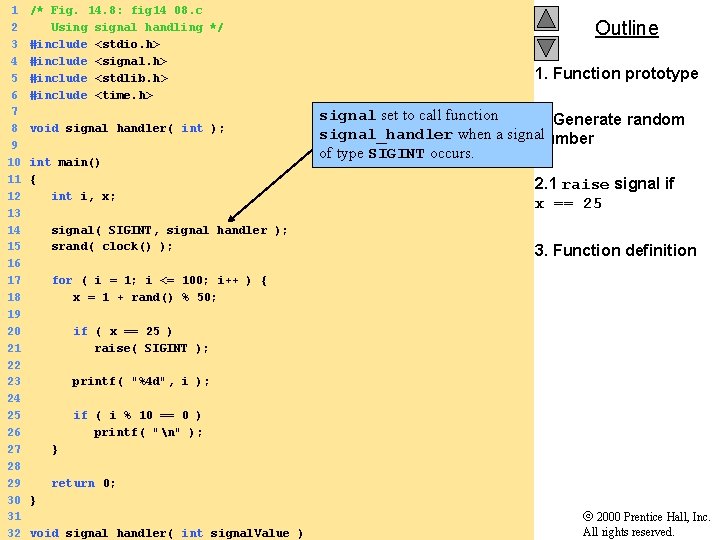
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 /* Fig. 14. 8: fig 14_08. c Using signal handling */ #include <stdio. h> #include <signal. h> #include <stdlib. h> #include <time. h> void signal_handler( int ); int main() { int i, x; signal( SIGINT, signal_handler ); srand( clock() ); Outline 1. Function prototype signal set to call function 2. Generate random signal_handler when a signal number of type SIGINT occurs. 2. 1 raise signal if x == 25 3. Function definition for ( i = 1; i <= 100; i++ ) { x = 1 + rand() % 50; if ( x == 25 ) raise( SIGINT ); printf( "%4 d", i ); if ( i % 10 == 0 ) printf( "n" ); } return 0; } 2000 Prentice Hall, Inc. void signal_handler( int signal. Value ) All rights reserved.
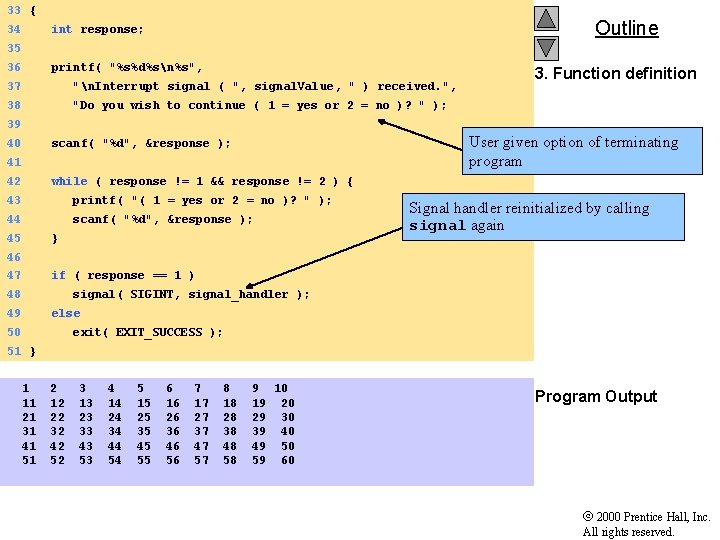
33 { 34 Outline int response; 35 36 printf( "%s%d%sn%s", 37 "n. Interrupt signal ( ", signal. Value, " ) received. ", 38 "Do you wish to continue ( 1 = yes or 2 = no )? " ); 3. Function definition 39 40 User given option of terminating program scanf( "%d", &response ); 41 42 while ( response != 1 && response != 2 ) { 43 printf( "( 1 = yes or 2 = no )? " ); 44 scanf( "%d", &response ); 45 } Signal handler reinitialized by calling signal again 46 47 if ( response == 1 ) 48 49 signal( SIGINT, signal_handler ); else 50 exit( EXIT_SUCCESS ); 51 } 1 11 21 31 41 51 2 12 22 32 42 52 3 13 23 33 43 53 4 14 24 34 44 54 5 15 25 35 45 55 6 16 26 36 46 56 7 17 27 37 47 57 8 18 28 38 48 58 9 10 19 20 29 30 39 40 49 50 59 60 Program Output 2000 Prentice Hall, Inc. All rights reserved.
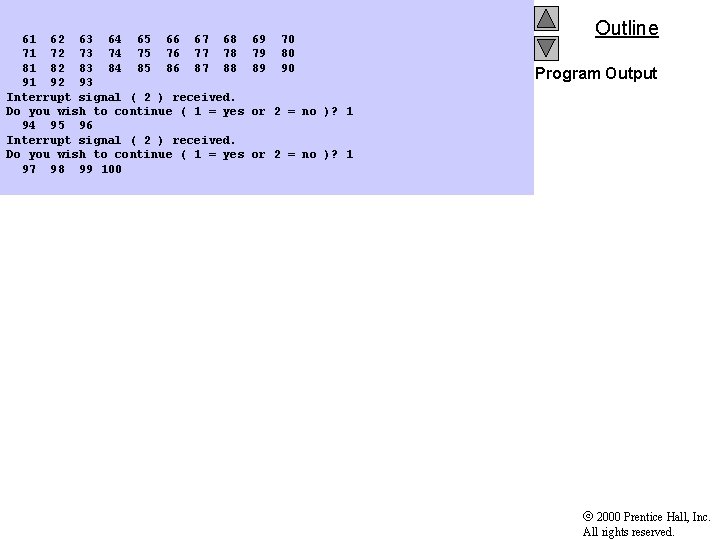
61 62 63 64 65 66 67 68 71 72 73 74 75 76 77 78 81 82 83 84 85 86 87 88 91 92 93 Interrupt signal ( 2 ) received. Do you wish to continue ( 1 = yes 94 95 96 Interrupt signal ( 2 ) received. Do you wish to continue ( 1 = yes 97 98 99 100 69 79 89 70 80 90 Outline Program Output or 2 = no )? 1 2000 Prentice Hall, Inc. All rights reserved.
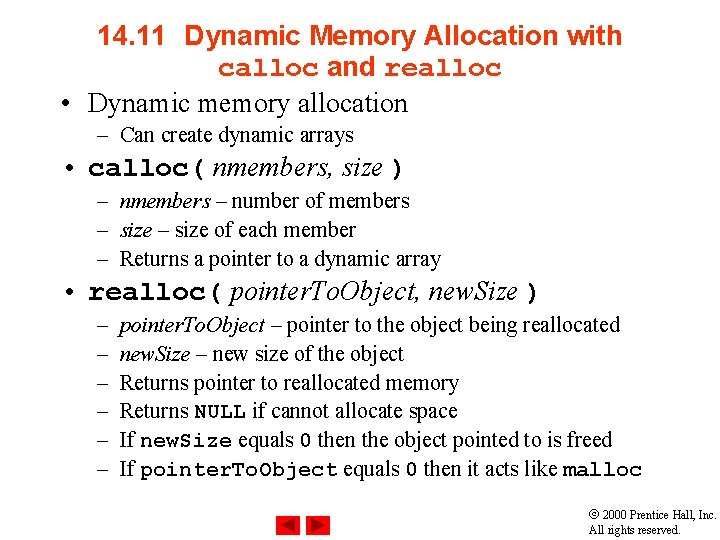
14. 11 Dynamic Memory Allocation with calloc and realloc • Dynamic memory allocation – Can create dynamic arrays • calloc( nmembers, size ) – nmembers – number of members – size of each member – Returns a pointer to a dynamic array • realloc( pointer. To. Object, new. Size ) – – – pointer. To. Object – pointer to the object being reallocated new. Size – new size of the object Returns pointer to reallocated memory Returns NULL if cannot allocate space If new. Size equals 0 then the object pointed to is freed If pointer. To. Object equals 0 then it acts like malloc 2000 Prentice Hall, Inc. All rights reserved.
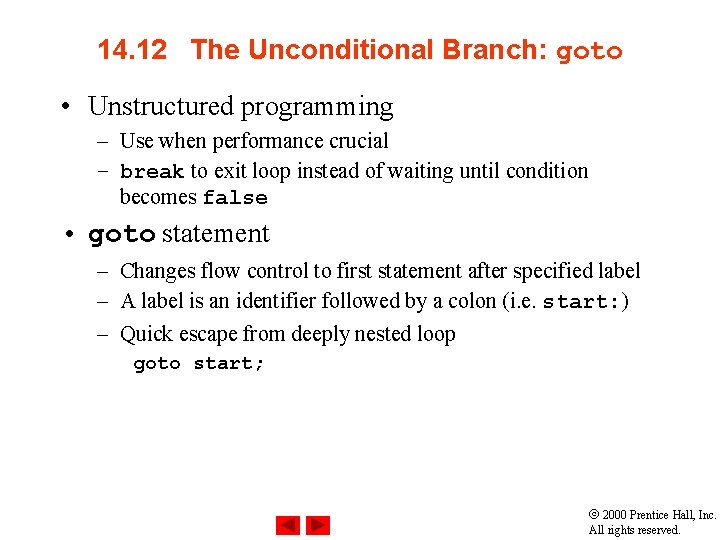
14. 12 The Unconditional Branch: goto • Unstructured programming – Use when performance crucial – break to exit loop instead of waiting until condition becomes false • goto statement – Changes flow control to first statement after specified label – A label is an identifier followed by a colon (i. e. start: ) – Quick escape from deeply nested loop goto start; 2000 Prentice Hall, Inc. All rights reserved.
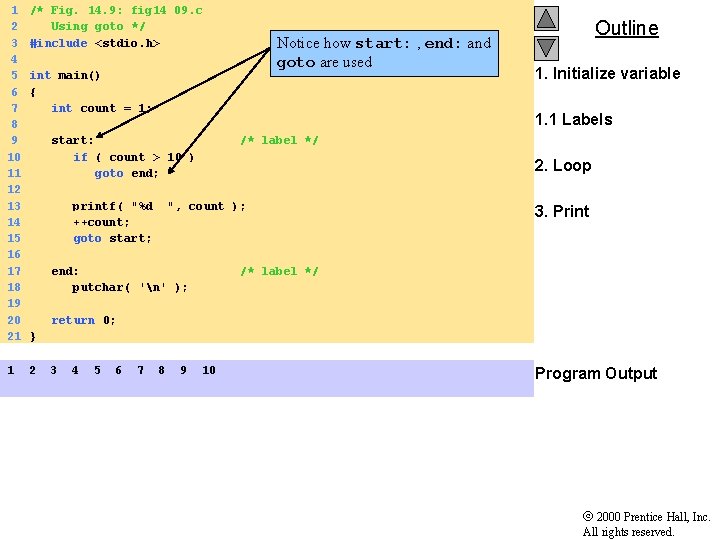
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 /* Fig. 14. 9: fig 14_09. c Using goto */ #include <stdio. h> 1 2 Notice how start: , end: and goto are used int main() { int count = 1; Outline 1. Initialize variable 1. 1 Labels start: if ( count > 10 ) goto end; printf( "%d ++count; goto start; /* label */ 2. Loop ", count ); end: putchar( 'n' ); 3. Print /* label */ return 0; } 3 4 5 6 7 8 9 10 Program Output 2000 Prentice Hall, Inc. All rights reserved.