Academic Misconduct InputOutput Conditionals Writing a Simple Algorithm
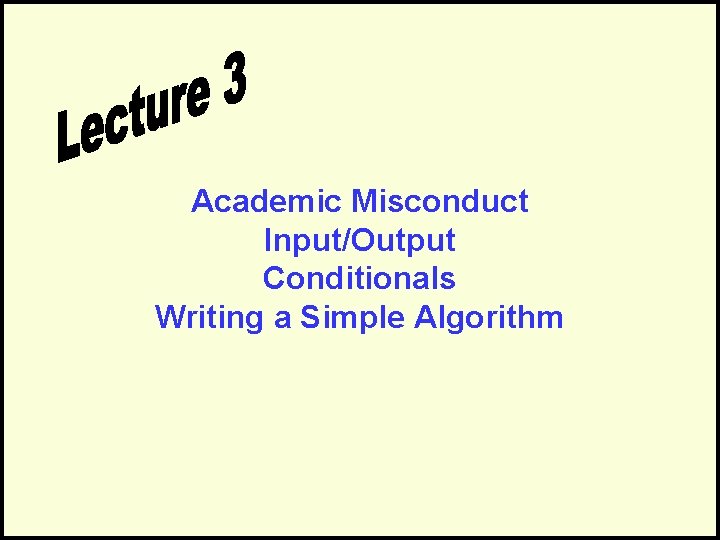
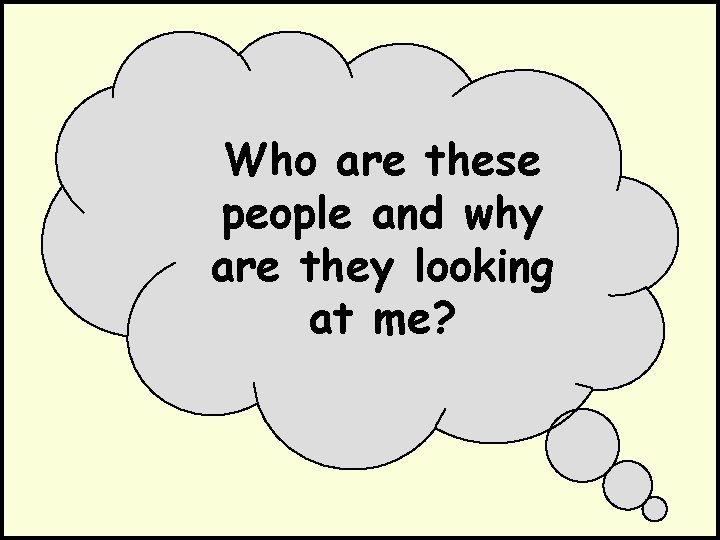
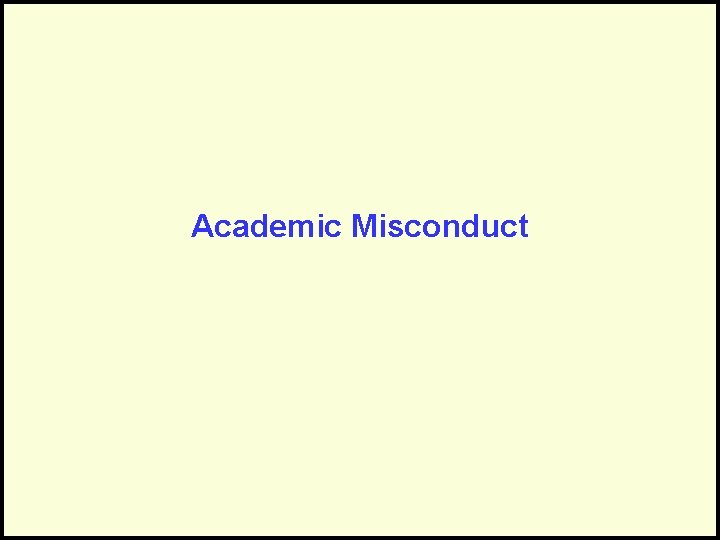
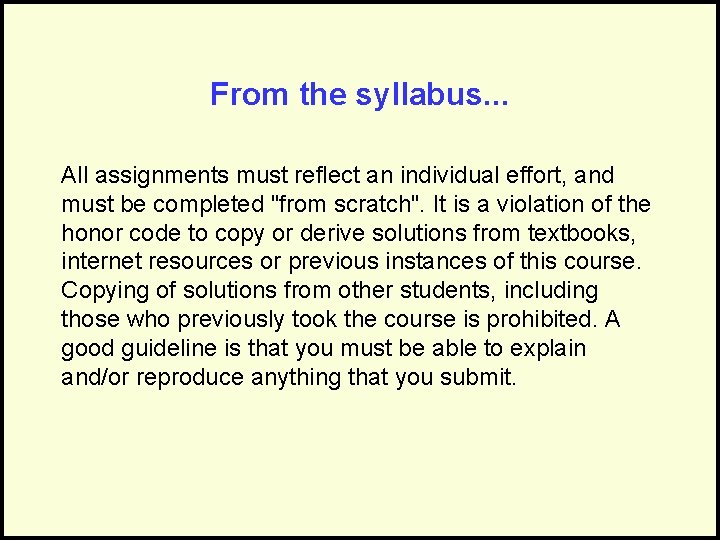
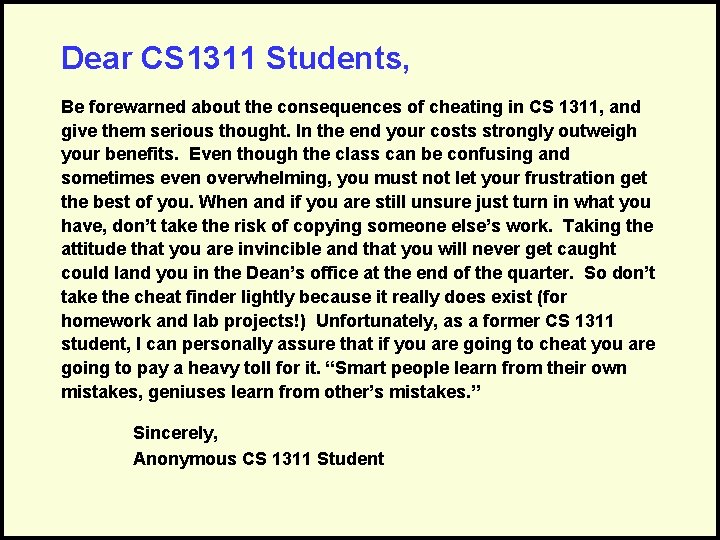
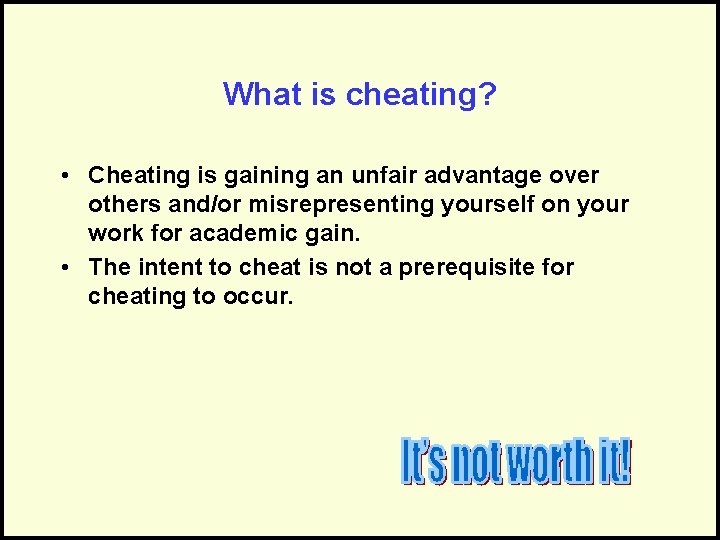
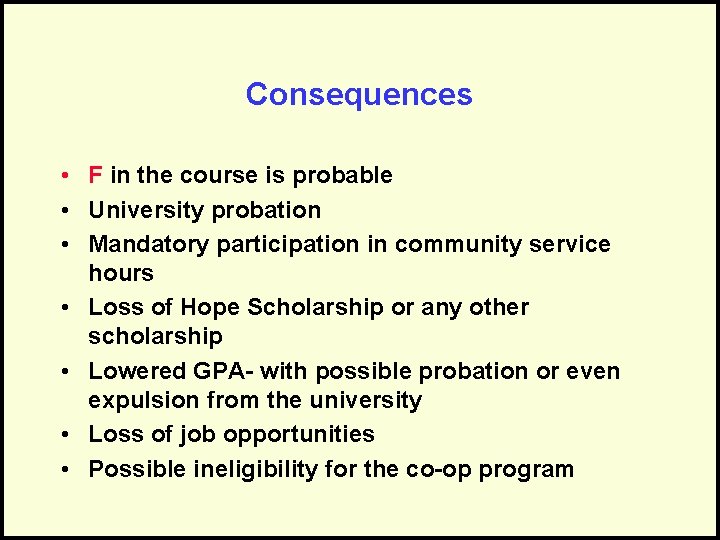
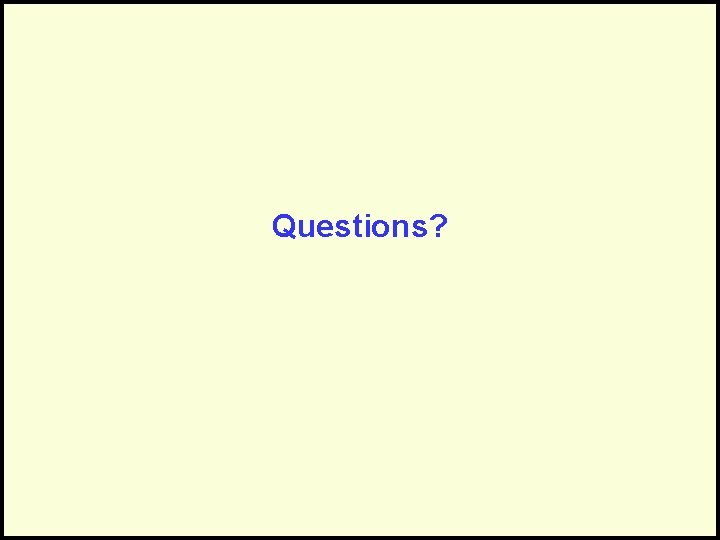
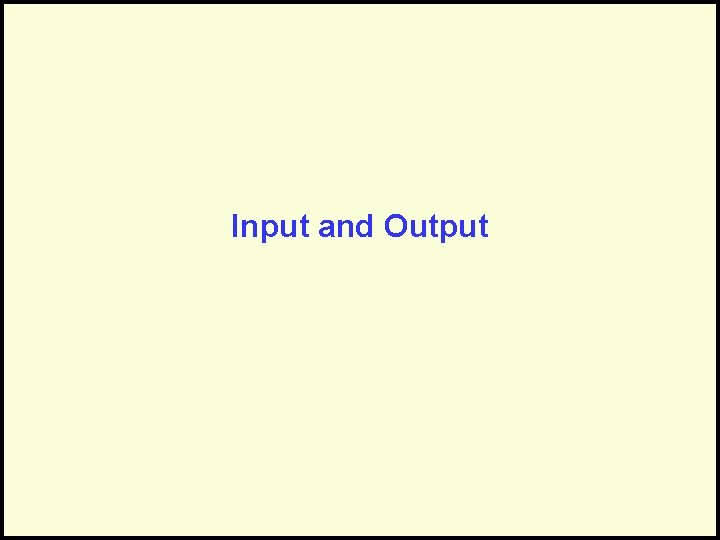
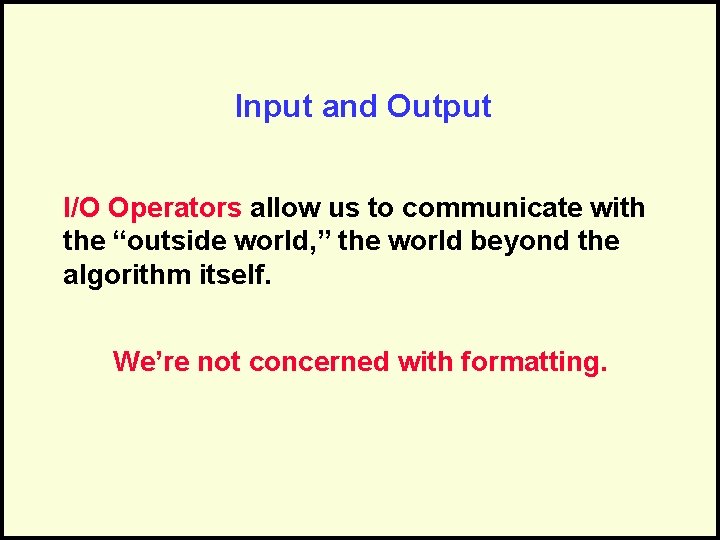
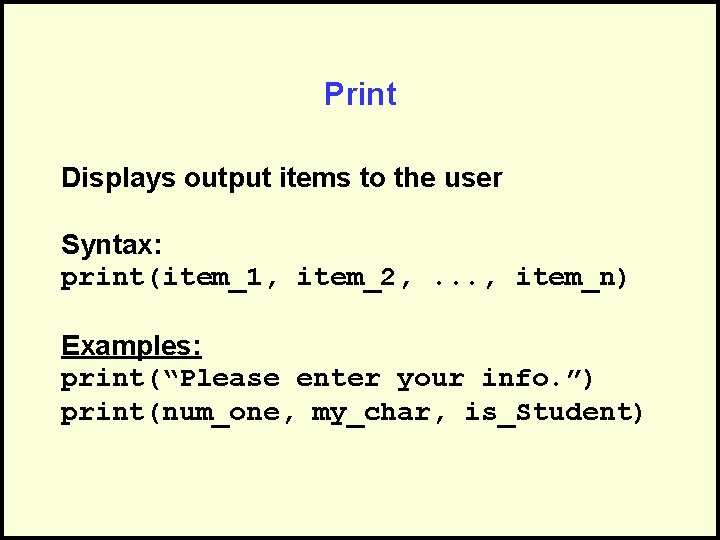
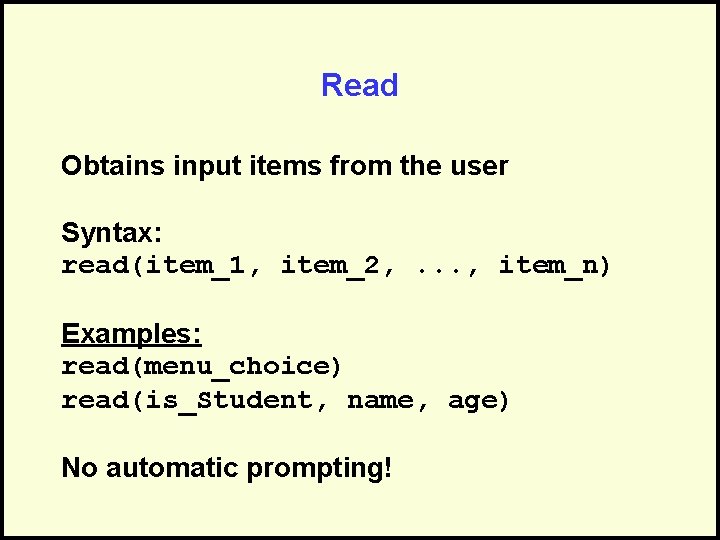
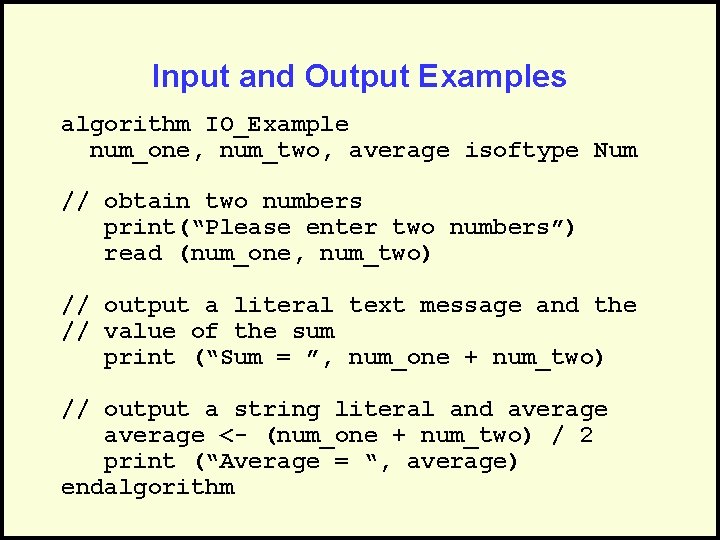
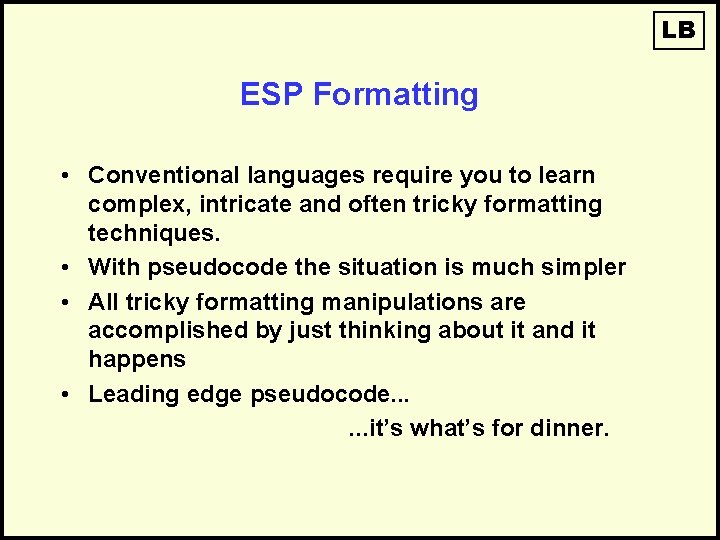
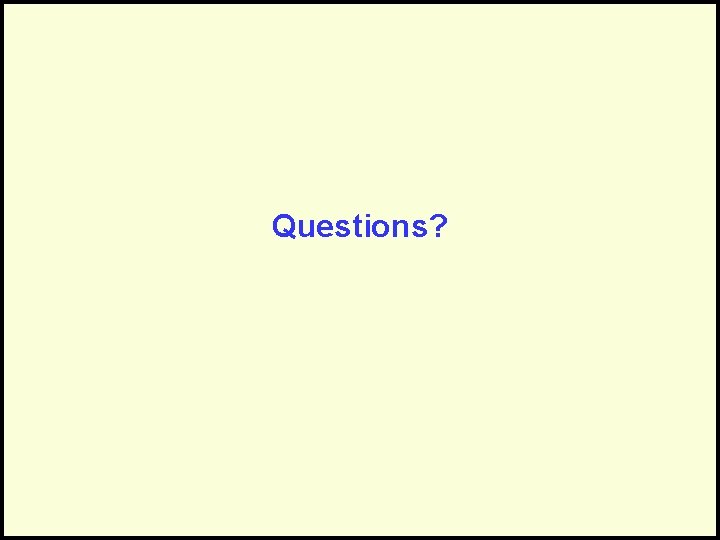
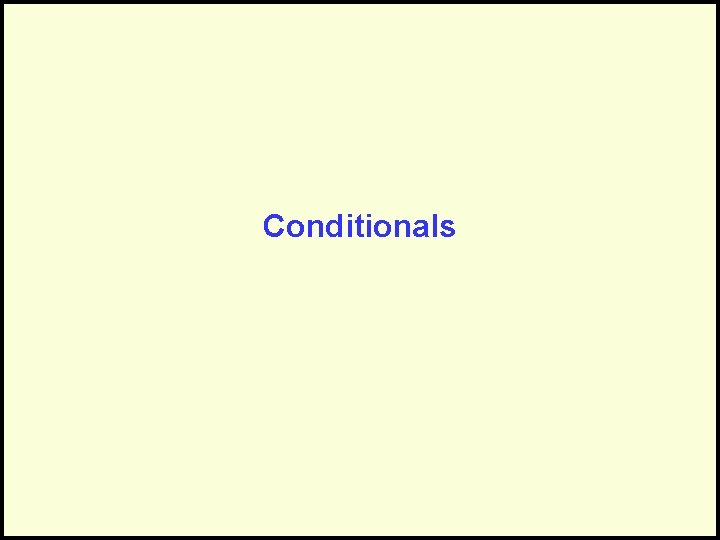
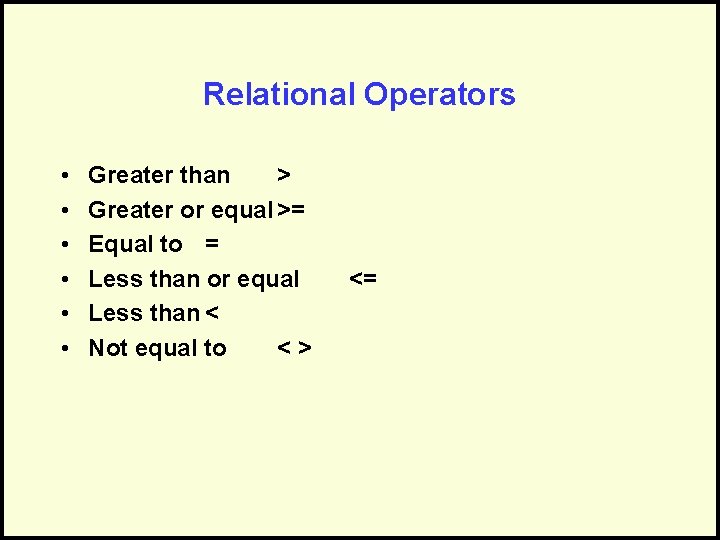
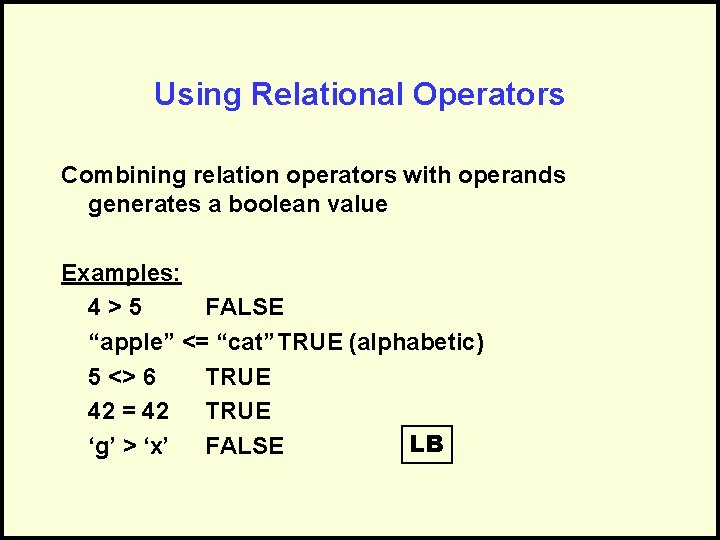
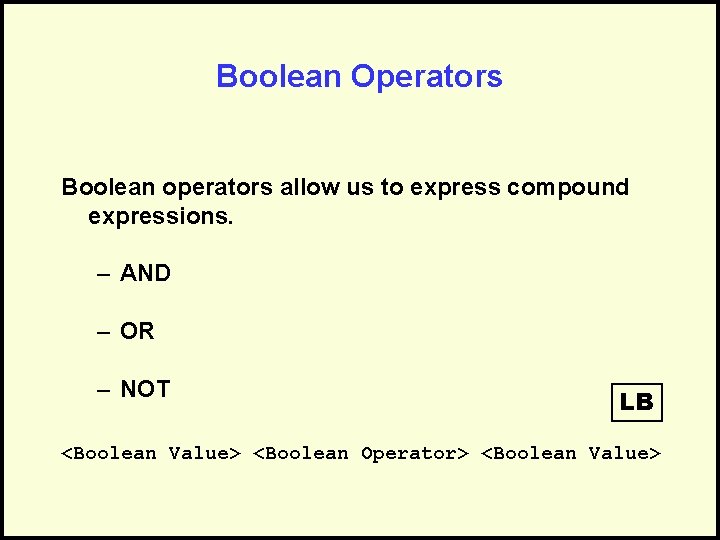
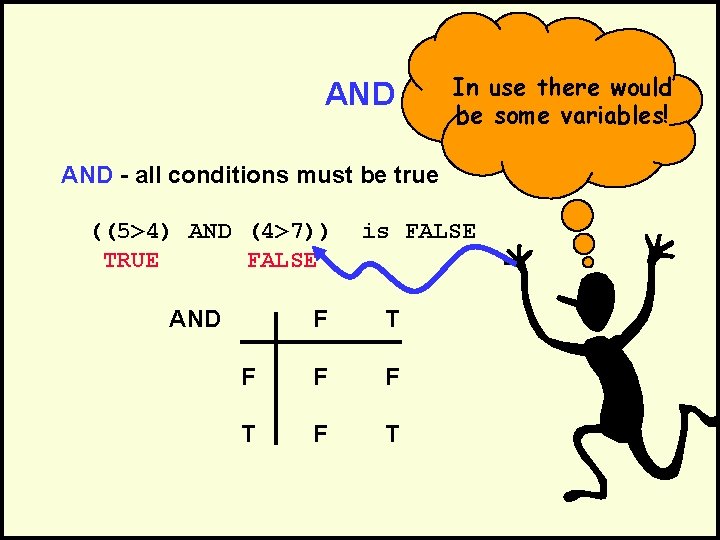
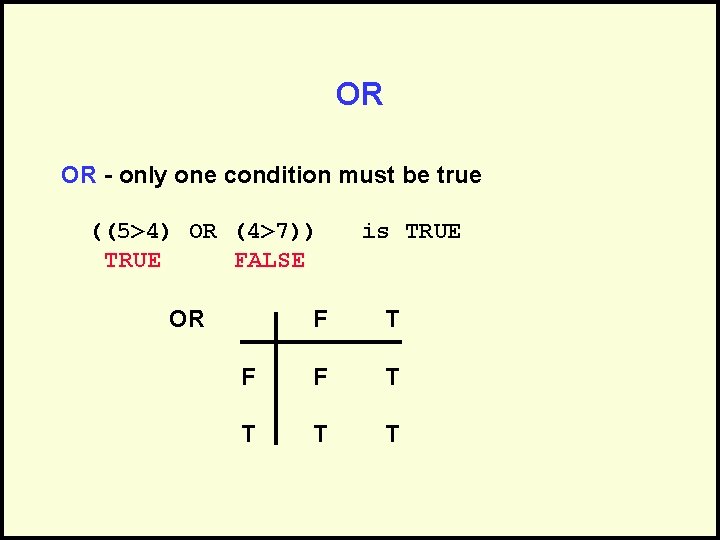
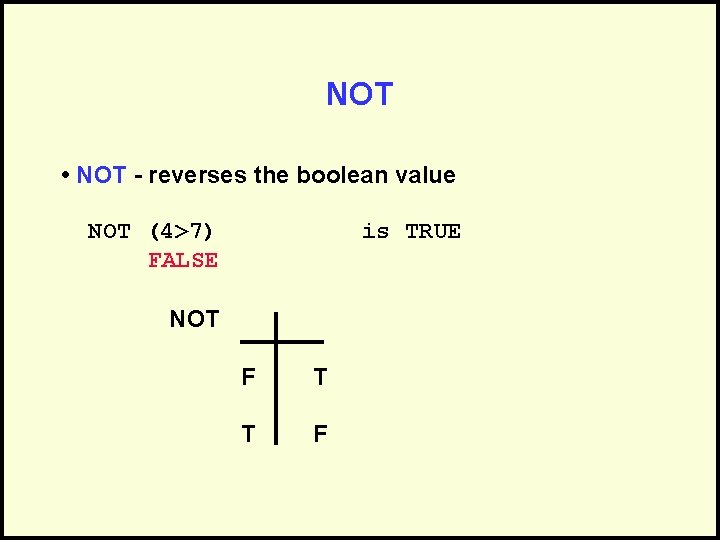
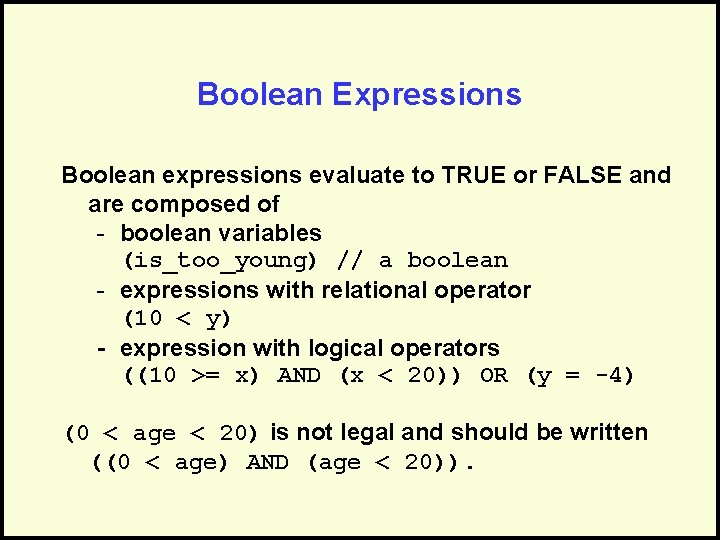
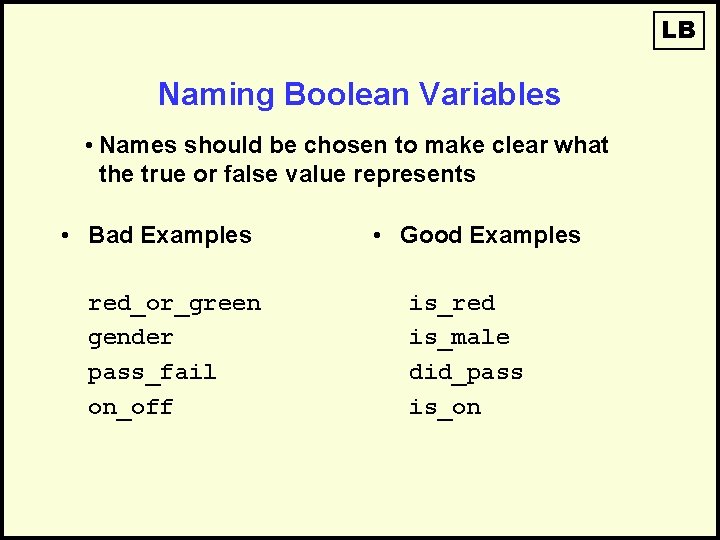
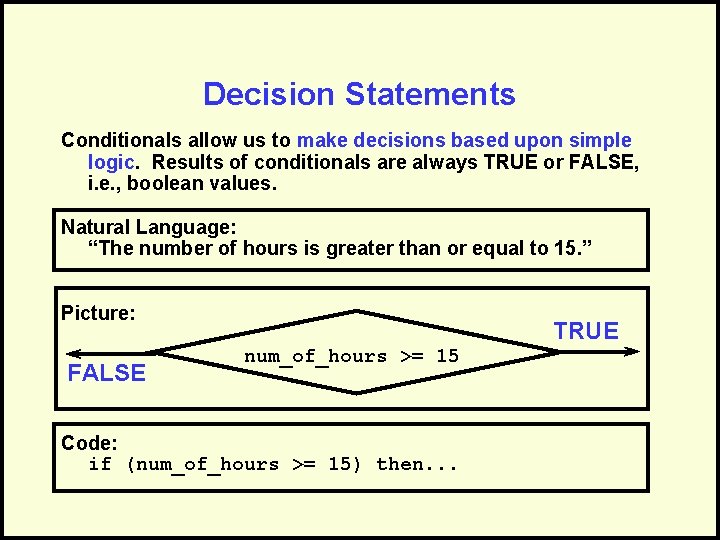
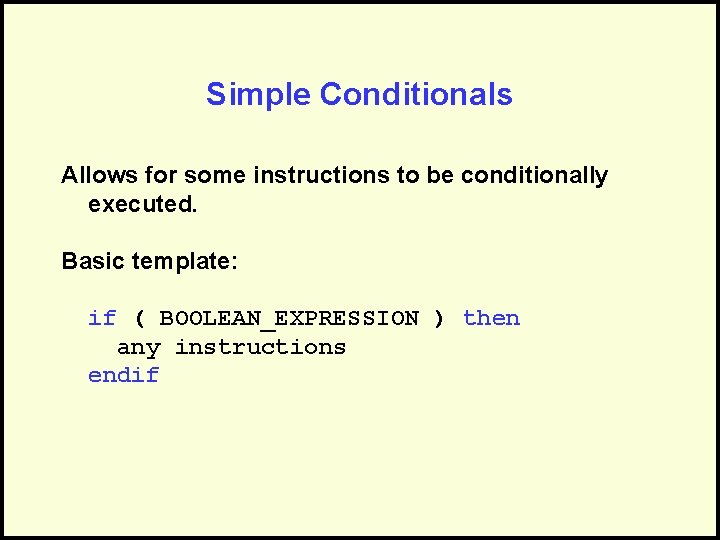
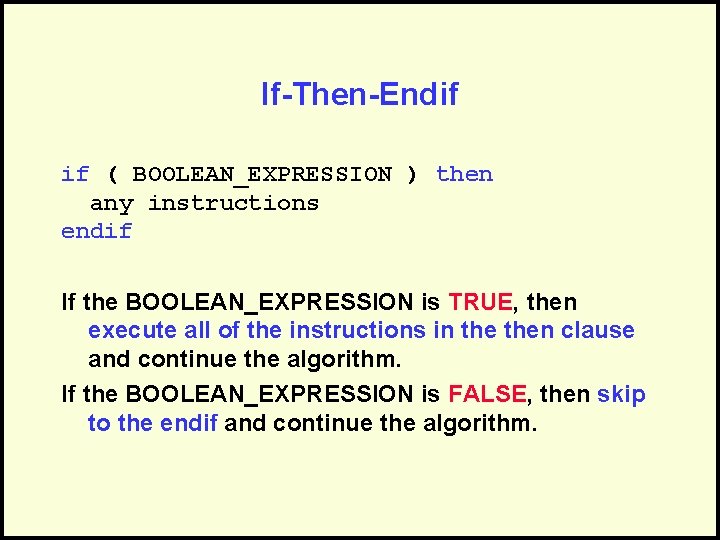
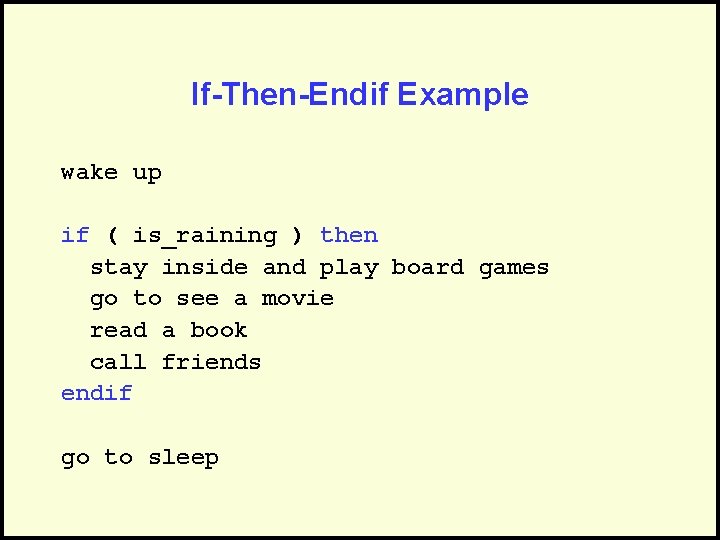
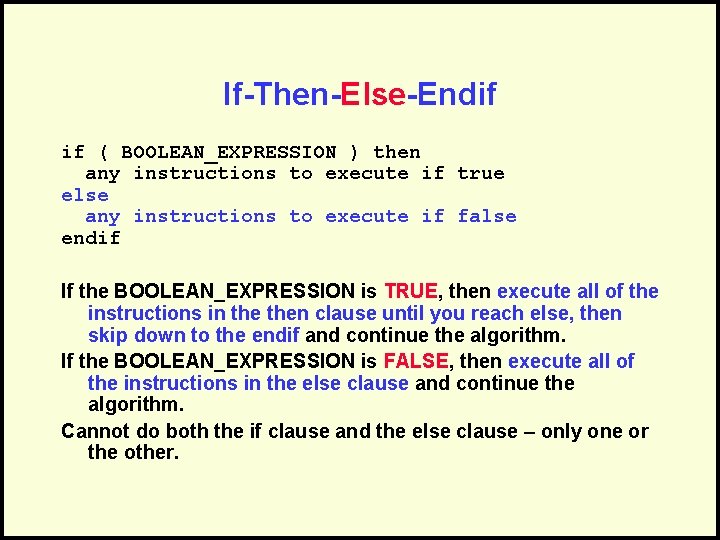
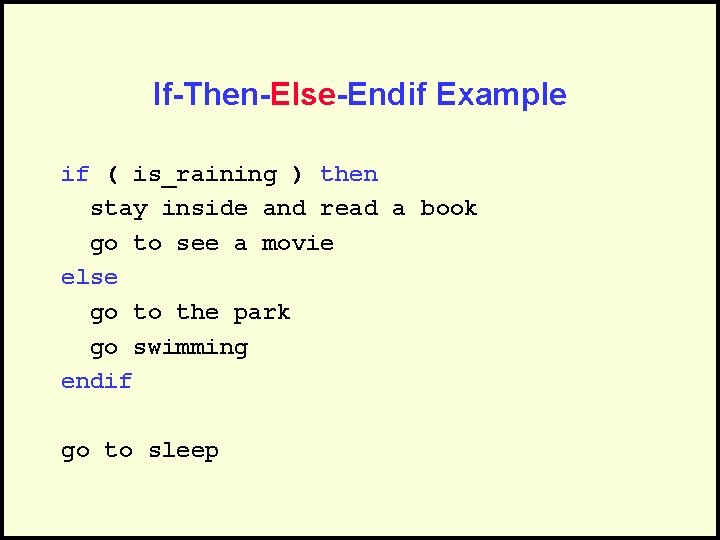
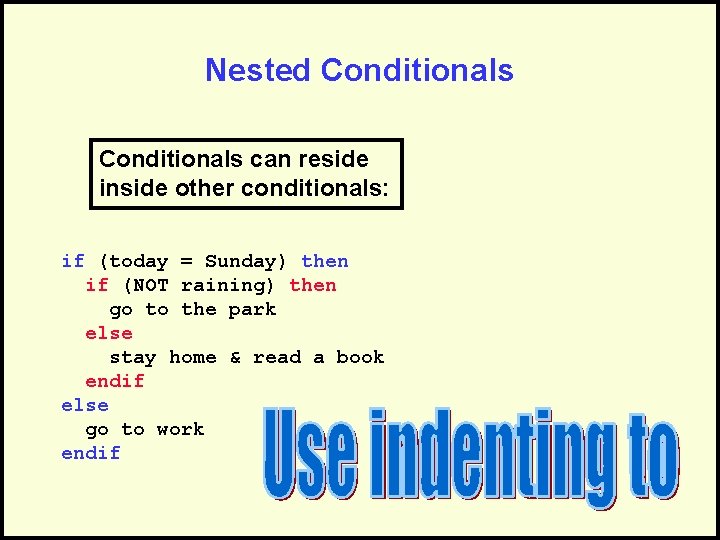
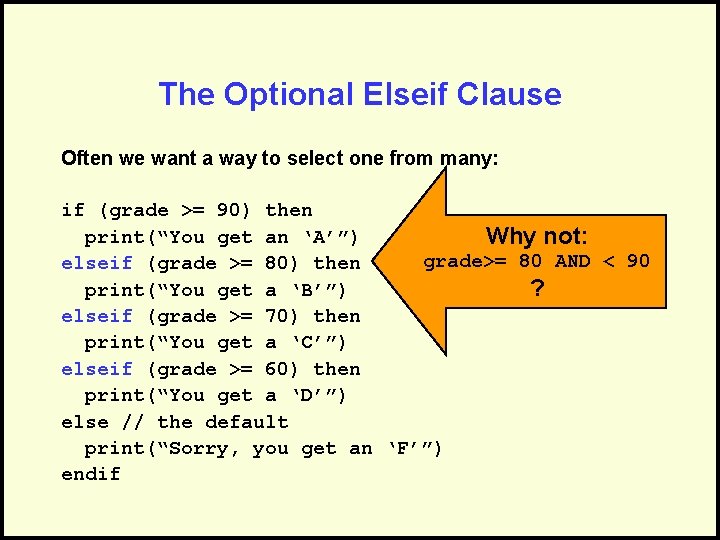
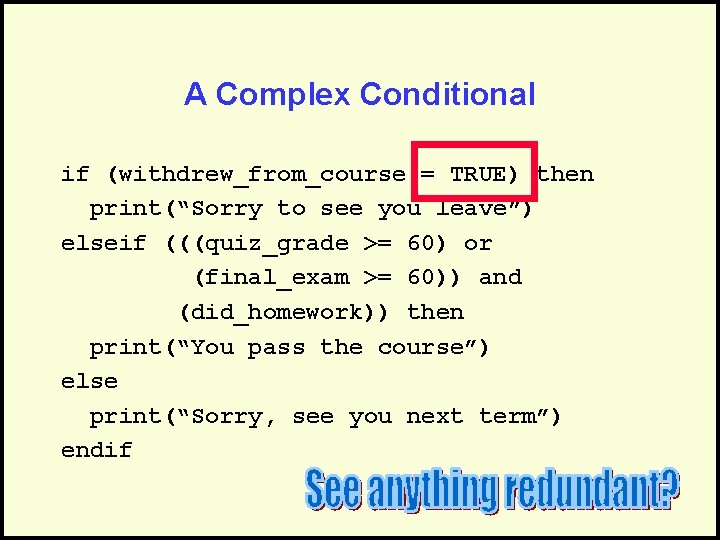
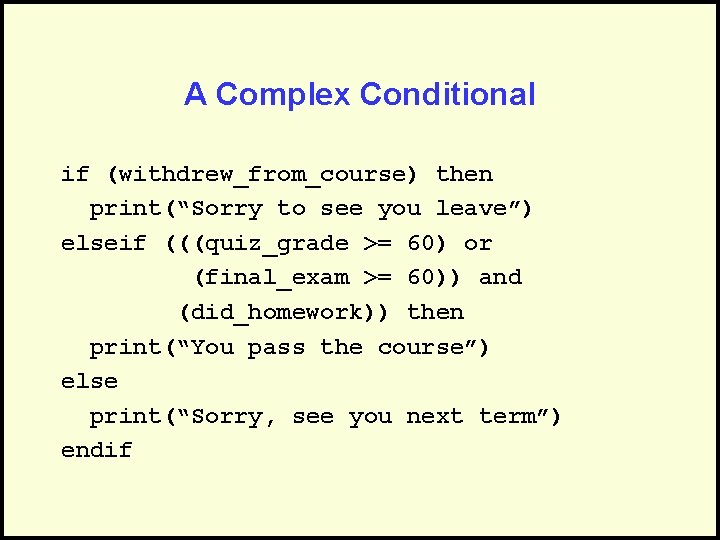
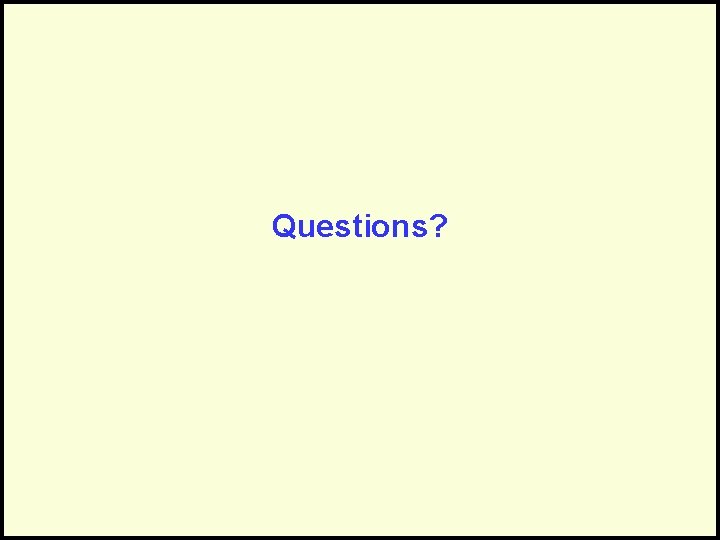
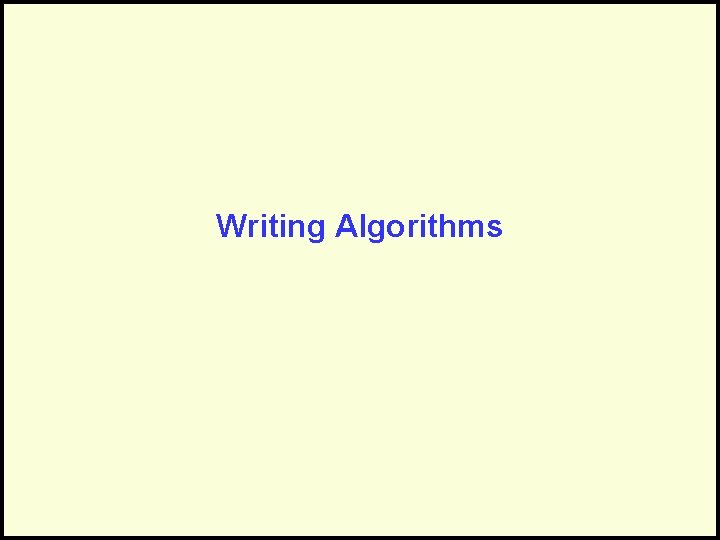
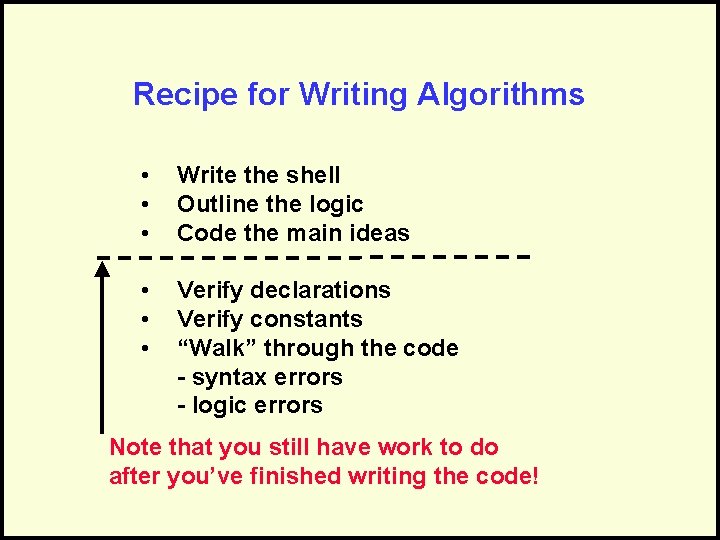
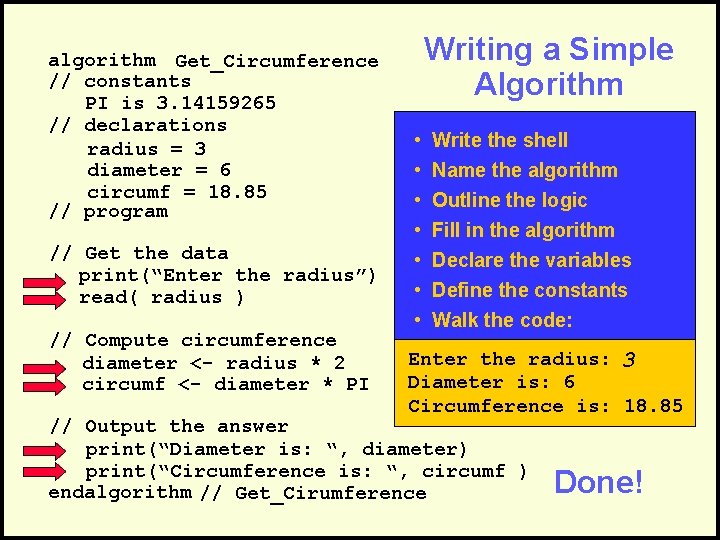
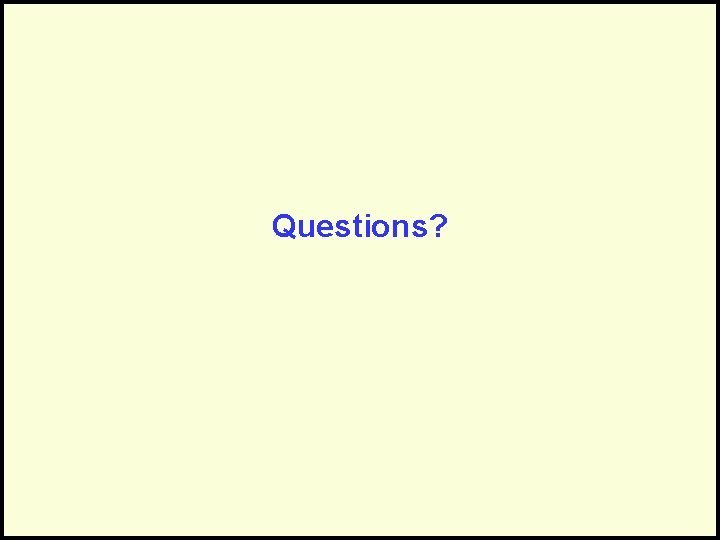
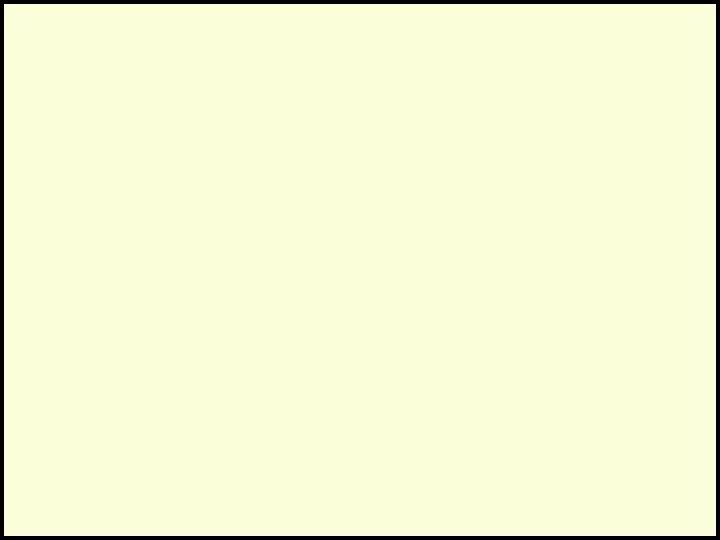
- Slides: 40
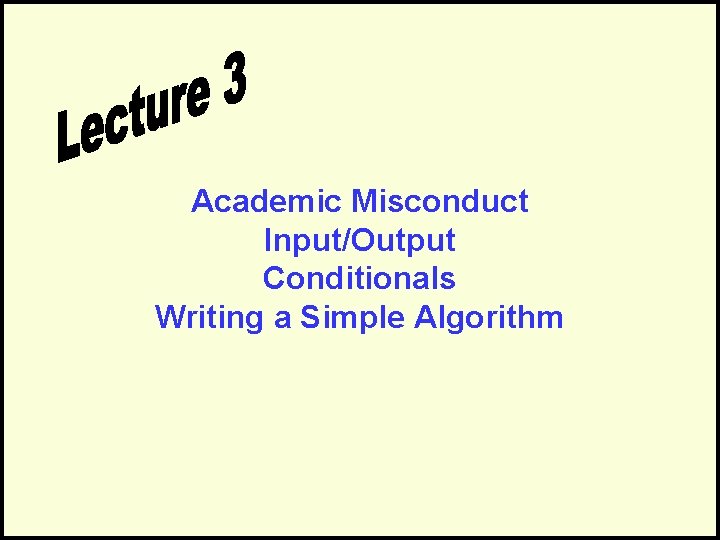
Academic Misconduct Input/Output Conditionals Writing a Simple Algorithm
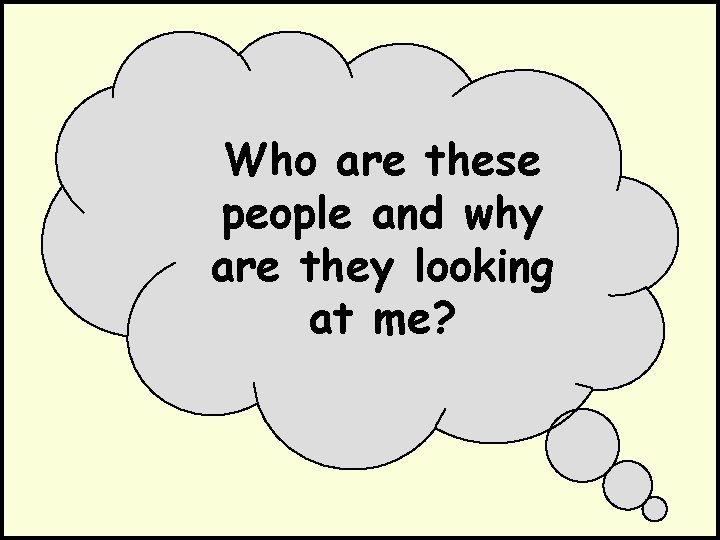
Who are these people and why are they looking at me?
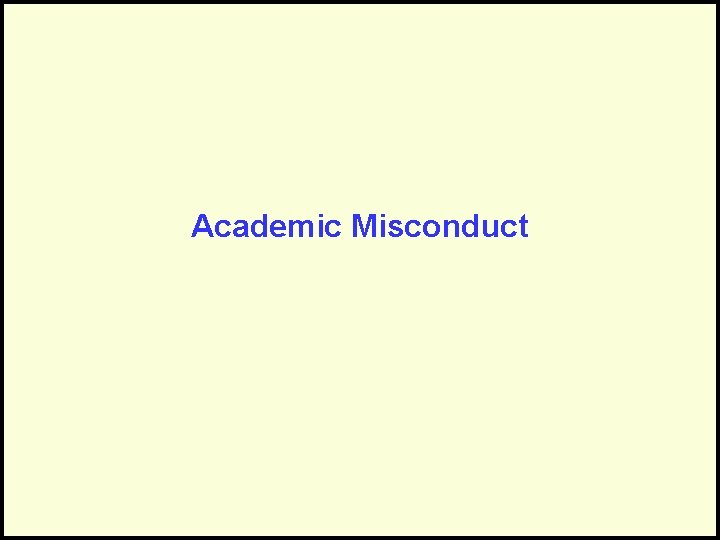
Academic Misconduct
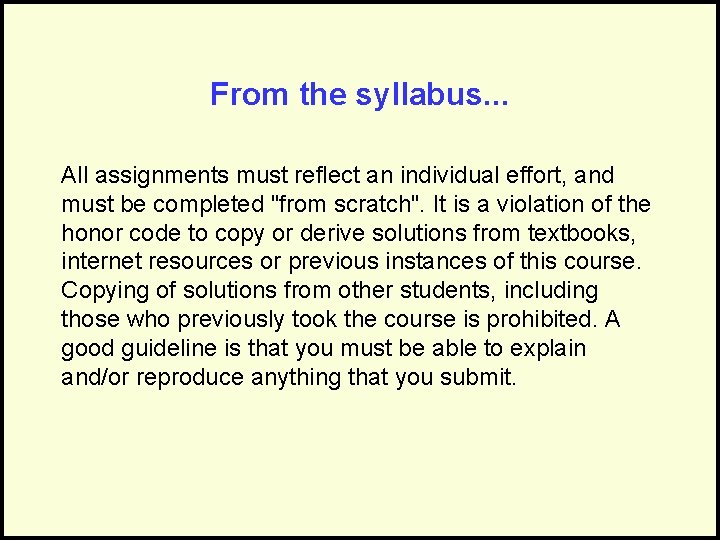
From the syllabus. . . All assignments must reflect an individual effort, and must be completed "from scratch". It is a violation of the honor code to copy or derive solutions from textbooks, internet resources or previous instances of this course. Copying of solutions from other students, including those who previously took the course is prohibited. A good guideline is that you must be able to explain and/or reproduce anything that you submit.
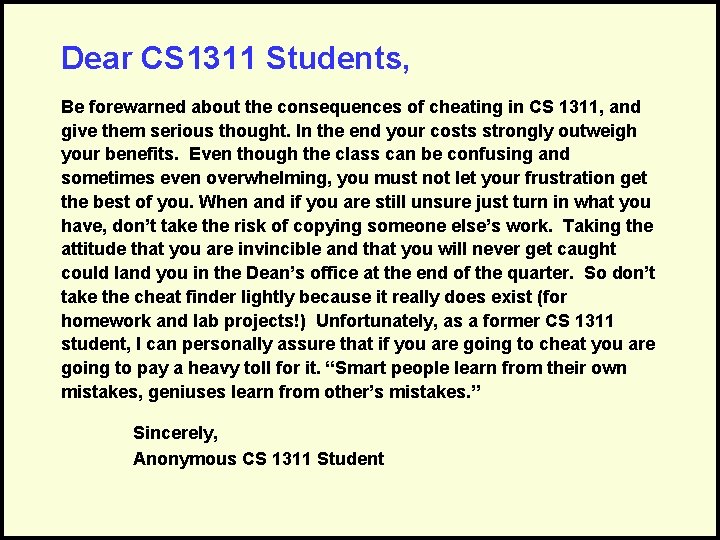
Dear CS 1311 Students, Be forewarned about the consequences of cheating in CS 1311, and give them serious thought. In the end your costs strongly outweigh your benefits. Even though the class can be confusing and sometimes even overwhelming, you must not let your frustration get the best of you. When and if you are still unsure just turn in what you have, don’t take the risk of copying someone else’s work. Taking the attitude that you are invincible and that you will never get caught could land you in the Dean’s office at the end of the quarter. So don’t take the cheat finder lightly because it really does exist (for homework and lab projects!) Unfortunately, as a former CS 1311 student, I can personally assure that if you are going to cheat you are going to pay a heavy toll for it. “Smart people learn from their own mistakes, geniuses learn from other’s mistakes. ” Sincerely, Anonymous CS 1311 Student
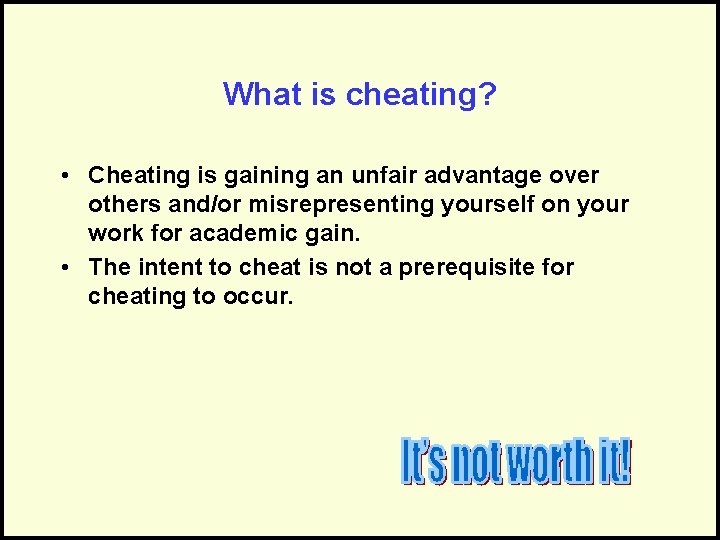
What is cheating? • Cheating is gaining an unfair advantage over others and/or misrepresenting yourself on your work for academic gain. • The intent to cheat is not a prerequisite for cheating to occur.
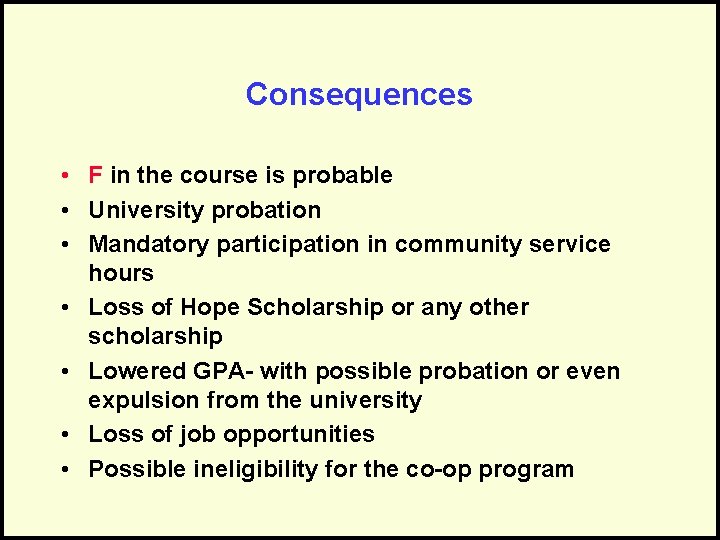
Consequences • F in the course is probable • University probation • Mandatory participation in community service hours • Loss of Hope Scholarship or any other scholarship • Lowered GPA- with possible probation or even expulsion from the university • Loss of job opportunities • Possible ineligibility for the co-op program
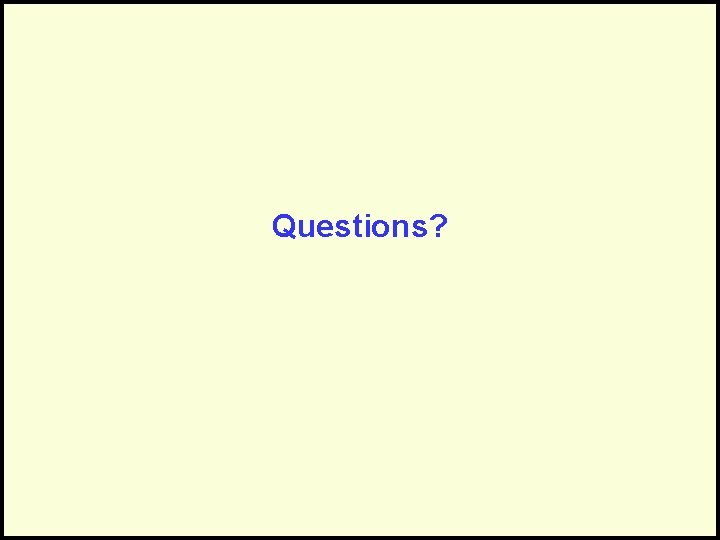
Questions?
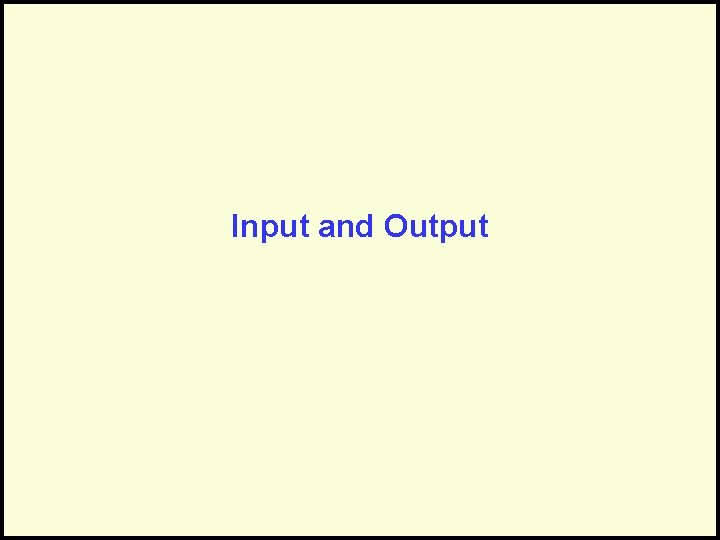
Input and Output
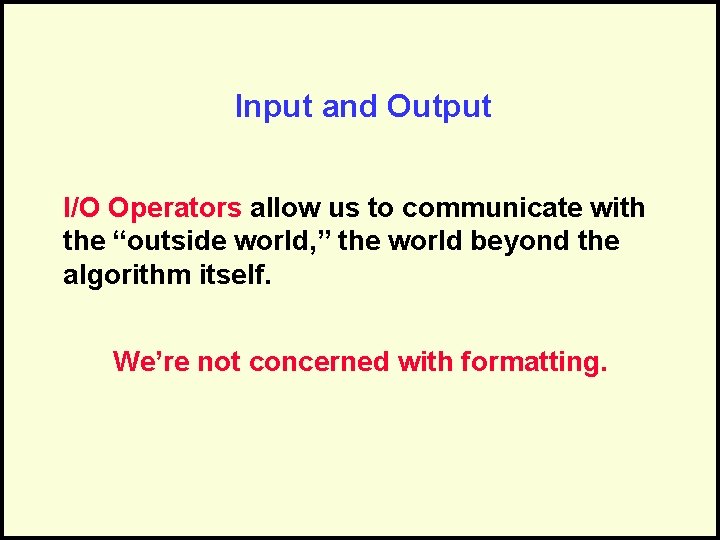
Input and Output I/O Operators allow us to communicate with the “outside world, ” the world beyond the algorithm itself. We’re not concerned with formatting.
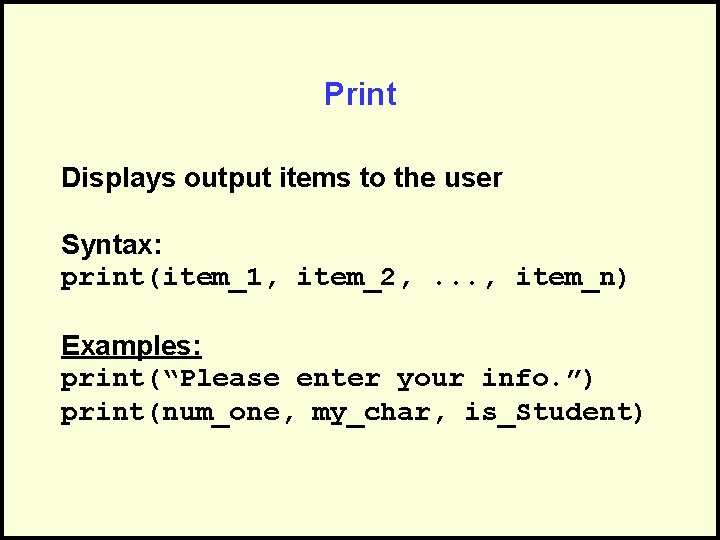
Print Displays output items to the user Syntax: print(item_1, item_2, . . . , item_n) Examples: print(“Please enter your info. ”) print(num_one, my_char, is_Student)
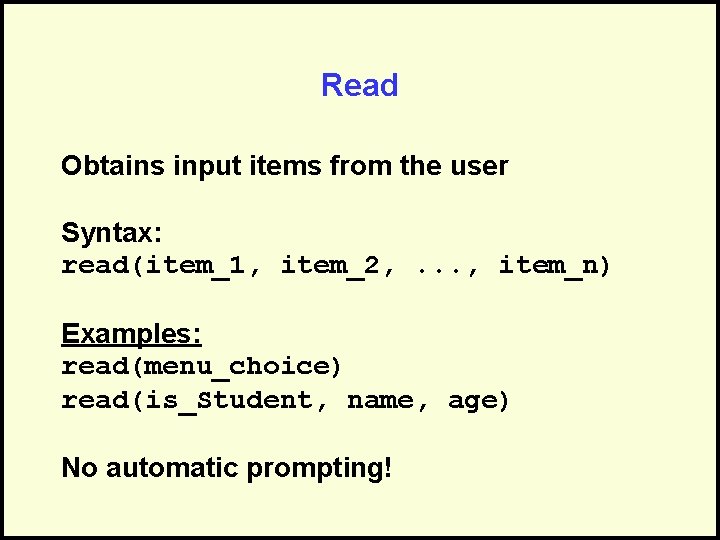
Read Obtains input items from the user Syntax: read(item_1, item_2, . . . , item_n) Examples: read(menu_choice) read(is_Student, name, age) No automatic prompting!
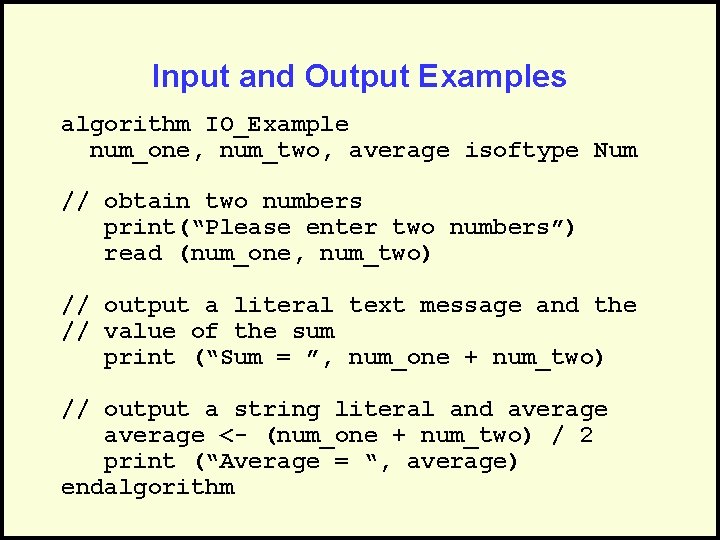
Input and Output Examples algorithm IO_Example num_one, num_two, average isoftype Num // obtain two numbers print(“Please enter two numbers”) read (num_one, num_two) // output a literal text message and the // value of the sum print (“Sum = ”, num_one + num_two) // output a string literal and average <- (num_one + num_two) / 2 print (“Average = “, average) endalgorithm
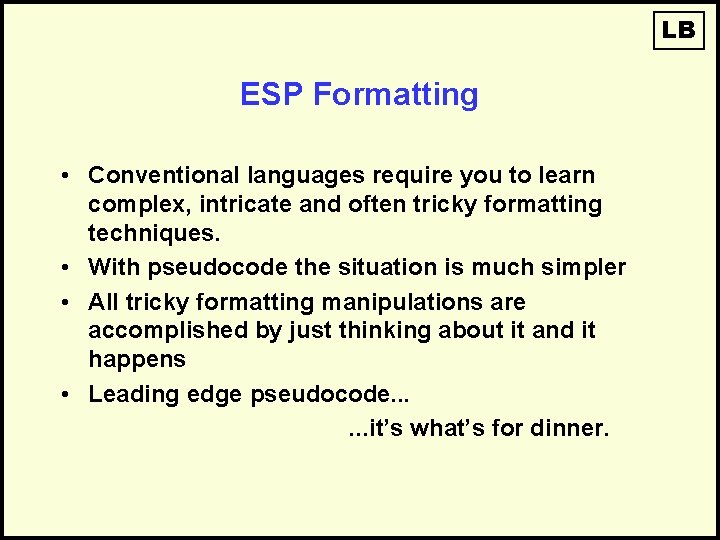
LB ESP Formatting • Conventional languages require you to learn complex, intricate and often tricky formatting techniques. • With pseudocode the situation is much simpler • All tricky formatting manipulations are accomplished by just thinking about it and it happens • Leading edge pseudocode. . . it’s what’s for dinner.
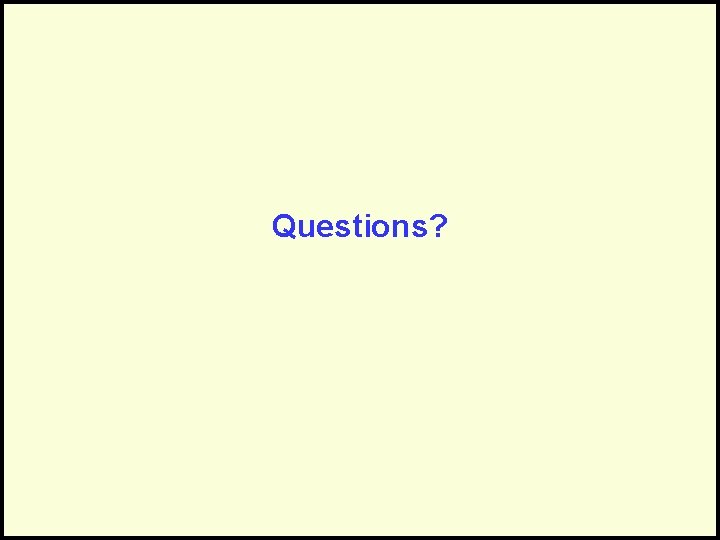
Questions?
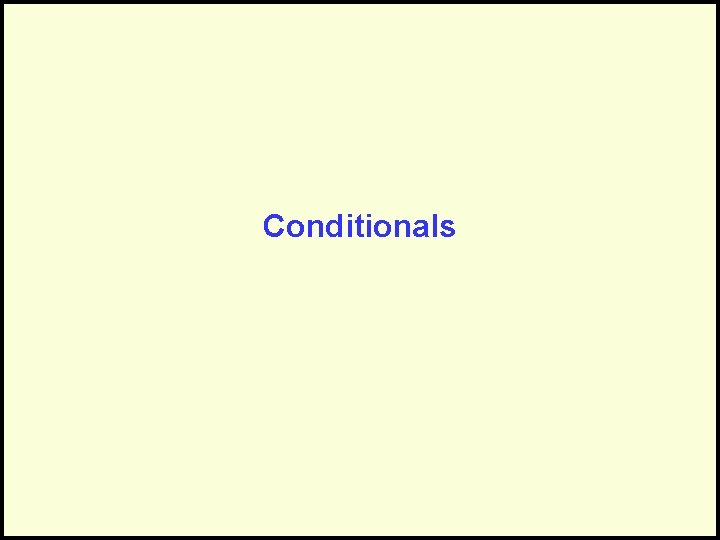
Conditionals
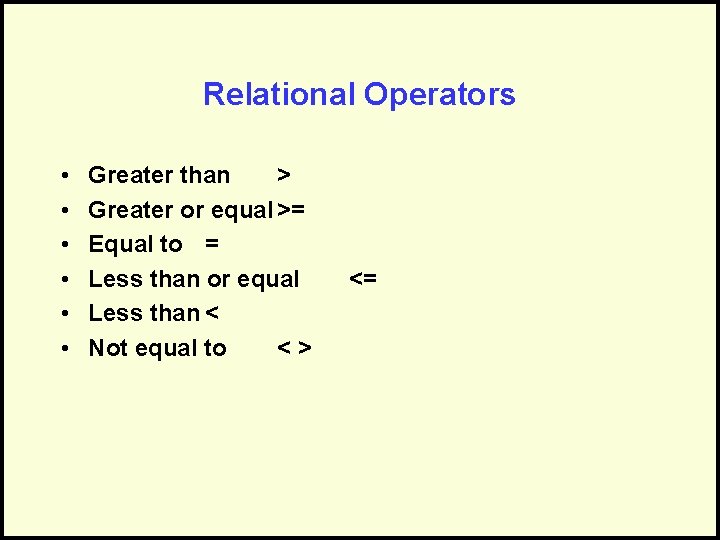
Relational Operators • • • Greater than > Greater or equal >= Equal to = Less than or equal Less than < Not equal to <> <=
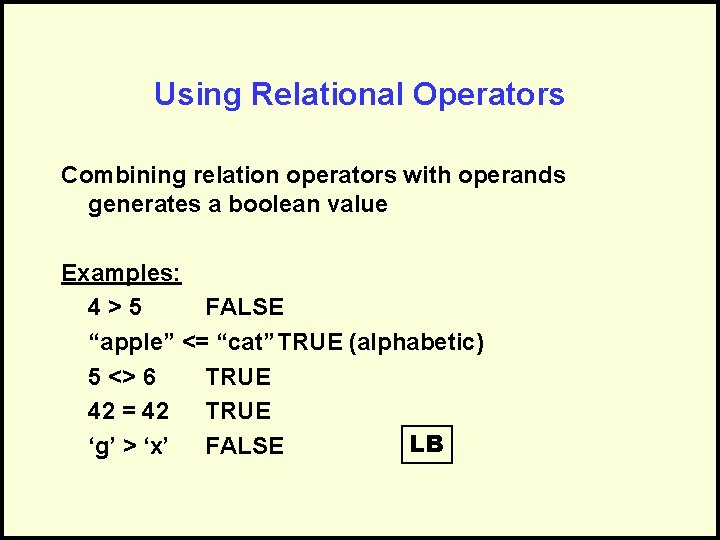
Using Relational Operators Combining relation operators with operands generates a boolean value Examples: 4>5 FALSE “apple” <= “cat” TRUE (alphabetic) 5 <> 6 TRUE 42 = 42 TRUE LB ‘g’ > ‘x’ FALSE
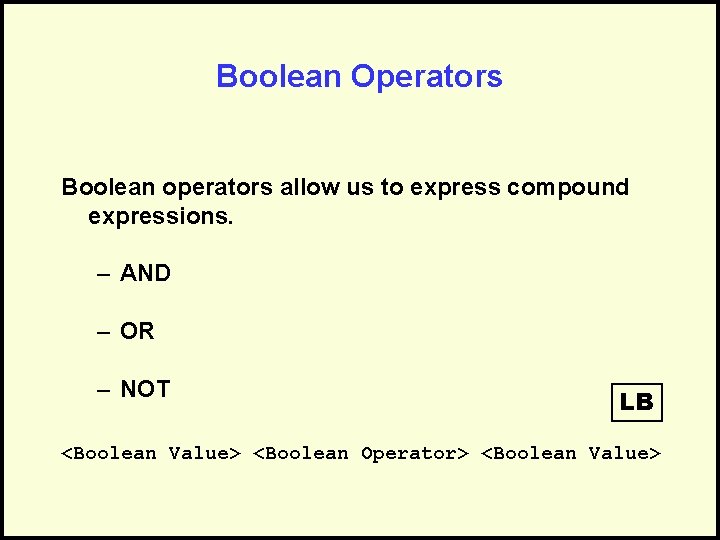
Boolean Operators Boolean operators allow us to express compound expressions. – AND – OR – NOT LB <Boolean Value> <Boolean Operator> <Boolean Value>
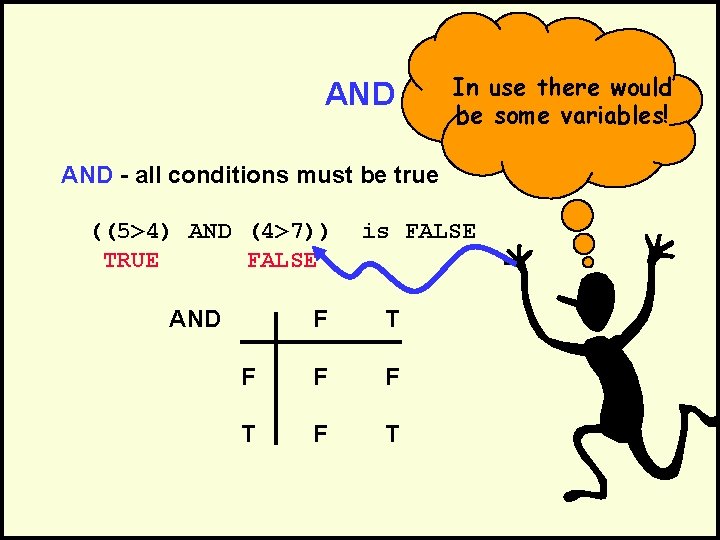
AND In use there would be some variables! AND - all conditions must be true ((5>4) AND (4>7)) TRUE FALSE AND is FALSE F T F F F T
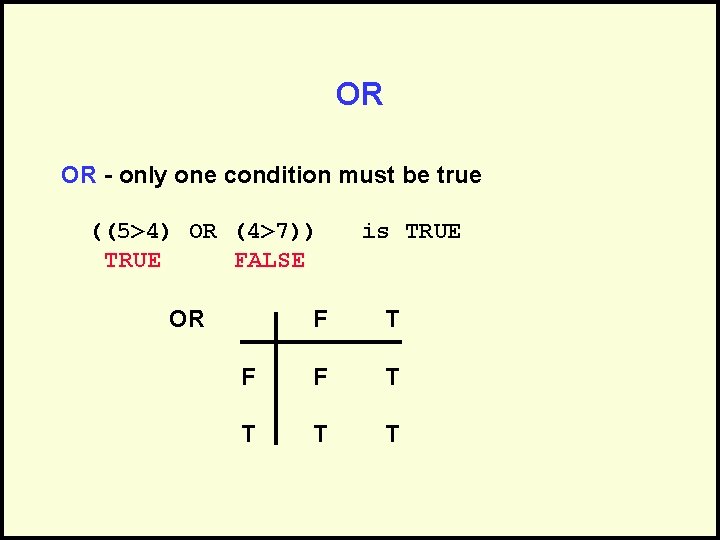
OR OR - only one condition must be true ((5>4) OR (4>7)) TRUE FALSE OR is TRUE F T F F T T
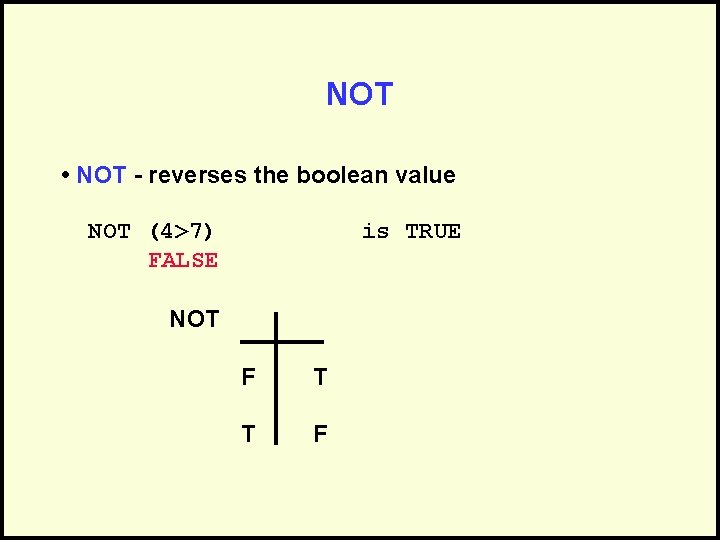
NOT • NOT - reverses the boolean value NOT (4>7) FALSE is TRUE NOT F T T F
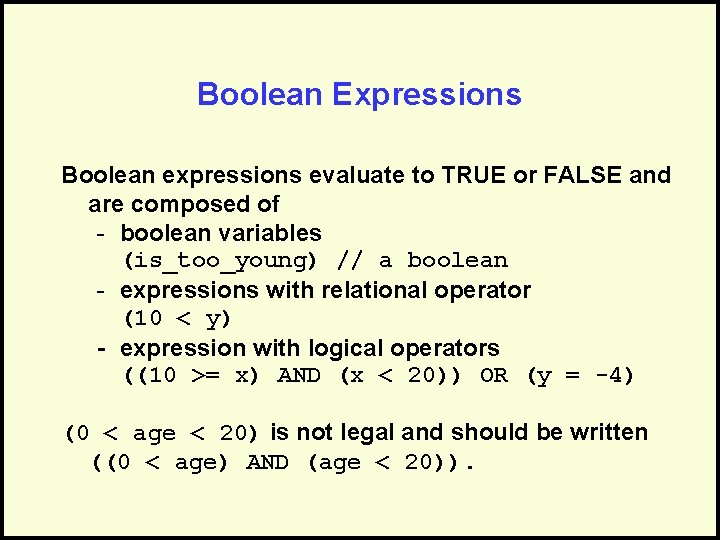
Boolean Expressions Boolean expressions evaluate to TRUE or FALSE and are composed of - boolean variables (is_too_young) // a boolean - expressions with relational operator (10 < y) - expression with logical operators ((10 >= x) AND (x < 20)) OR (y = -4) (0 < age < 20) is not legal and should be written ((0 < age) AND (age < 20)).
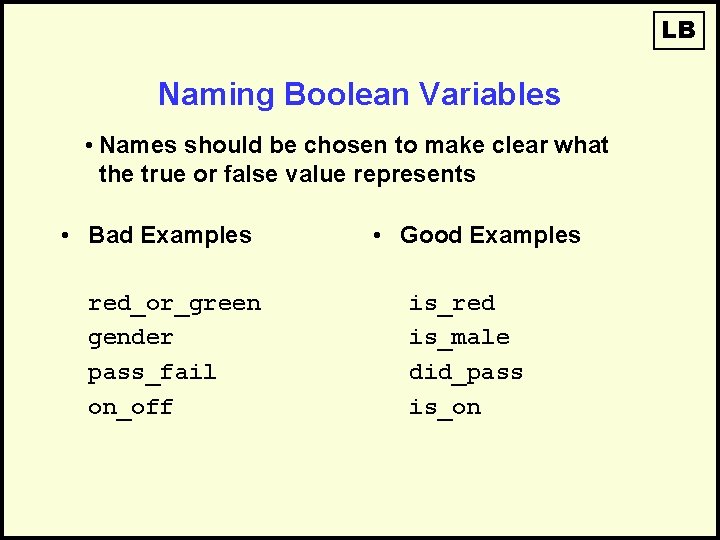
LB Naming Boolean Variables • Names should be chosen to make clear what the true or false value represents • Bad Examples red_or_green gender pass_fail on_off • Good Examples is_red is_male did_pass is_on
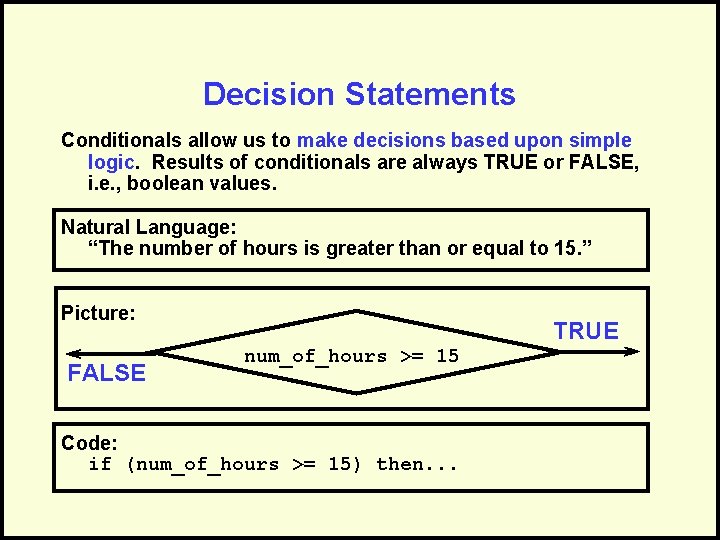
Decision Statements Conditionals allow us to make decisions based upon simple logic. Results of conditionals are always TRUE or FALSE, i. e. , boolean values. Natural Language: “The number of hours is greater than or equal to 15. ” Picture: FALSE TRUE num_of_hours >= 15 Code: if (num_of_hours >= 15) then. . .
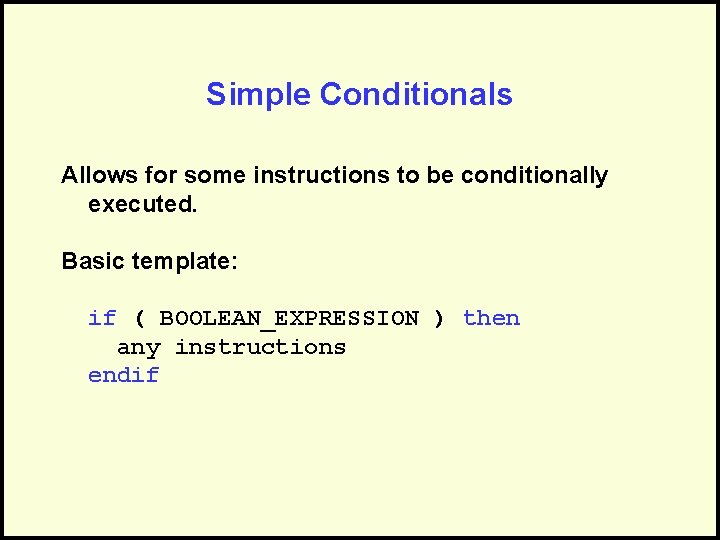
Simple Conditionals Allows for some instructions to be conditionally executed. Basic template: if ( BOOLEAN_EXPRESSION ) then any instructions endif
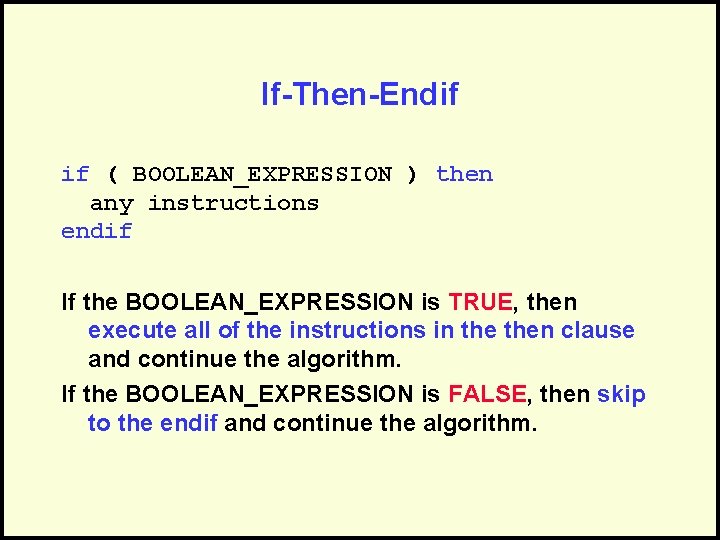
If-Then-Endif if ( BOOLEAN_EXPRESSION ) then any instructions endif If the BOOLEAN_EXPRESSION is TRUE, then execute all of the instructions in then clause and continue the algorithm. If the BOOLEAN_EXPRESSION is FALSE, then skip to the endif and continue the algorithm.
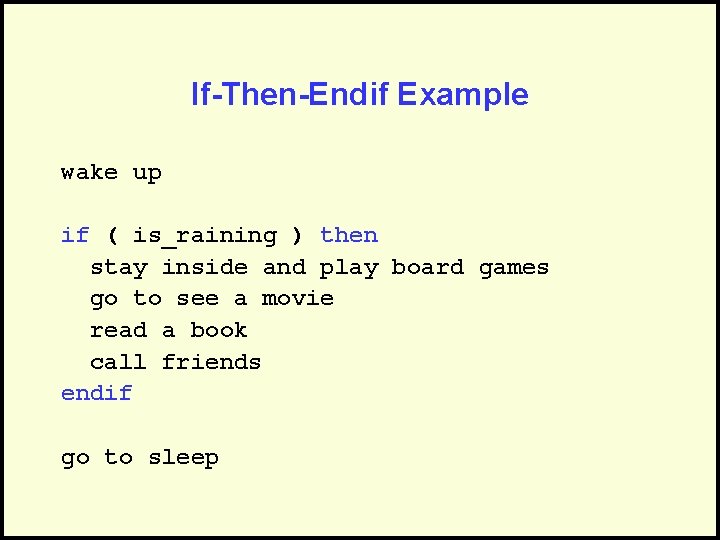
If-Then-Endif Example wake up if ( is_raining ) then stay inside and play board games go to see a movie read a book call friends endif go to sleep
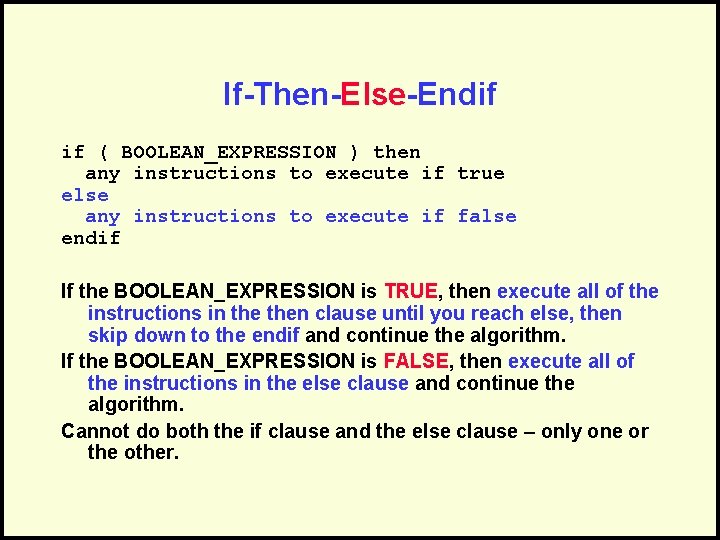
If-Then-Else-Endif if ( BOOLEAN_EXPRESSION ) then any instructions to execute if true else any instructions to execute if false endif If the BOOLEAN_EXPRESSION is TRUE, then execute all of the instructions in then clause until you reach else, then skip down to the endif and continue the algorithm. If the BOOLEAN_EXPRESSION is FALSE, then execute all of the instructions in the else clause and continue the algorithm. Cannot do both the if clause and the else clause – only one or the other.
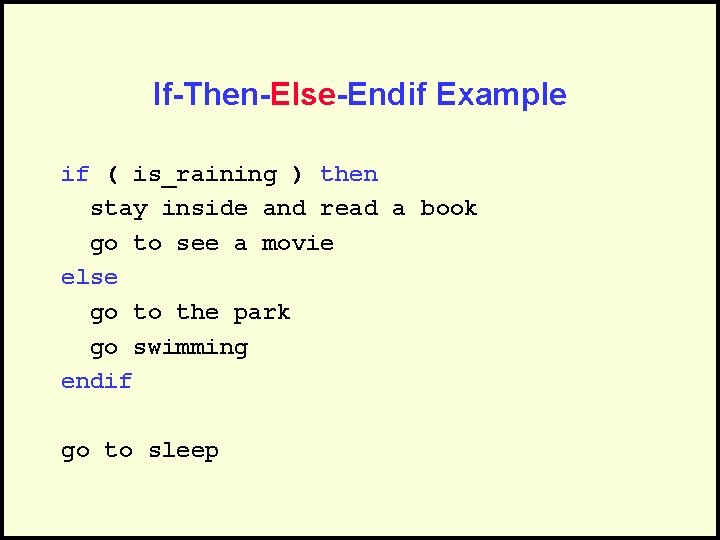
If-Then-Else-Endif Example if ( is_raining ) then stay inside and read a book go to see a movie else go to the park go swimming endif go to sleep
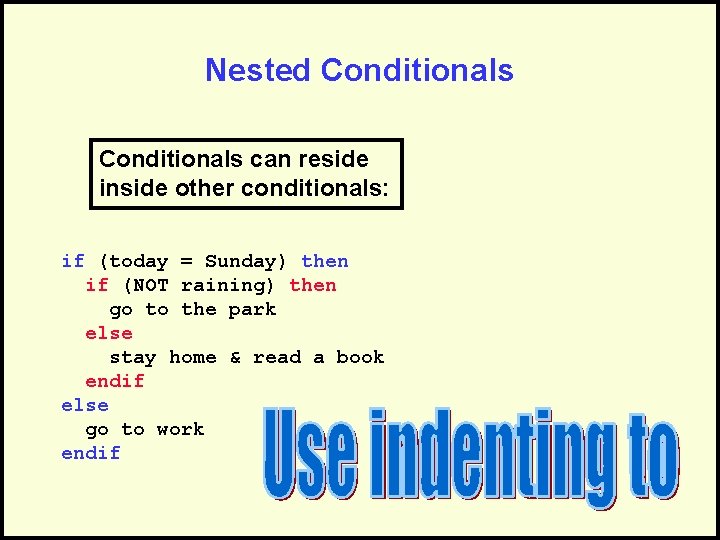
Nested Conditionals can reside inside other conditionals: if (today = Sunday) then if (NOT raining) then go to the park else stay home & read a book endif else go to work endif
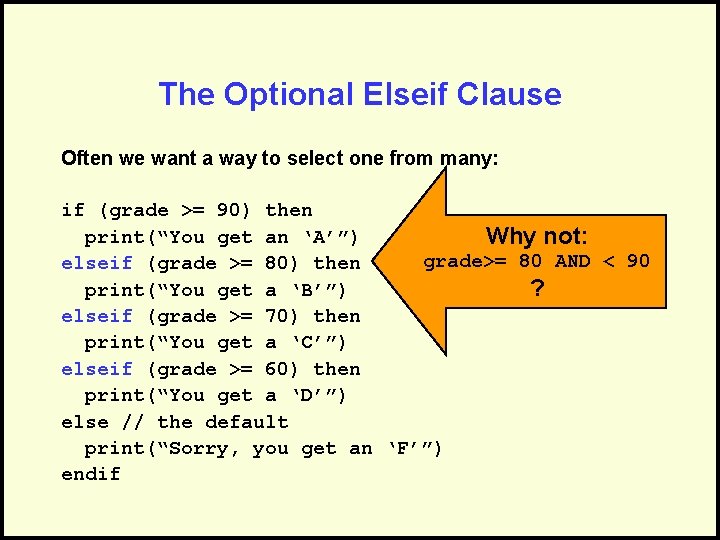
The Optional Elseif Clause Often we want a way to select one from many: if (grade >= 90) then print(“You get an ‘A’”) Why not: grade>= 80 AND < 90 elseif (grade >= 80) then print(“You get a ‘B’”) ? elseif (grade >= 70) then print(“You get a ‘C’”) elseif (grade >= 60) then print(“You get a ‘D’”) else // the default print(“Sorry, you get an ‘F’”) endif
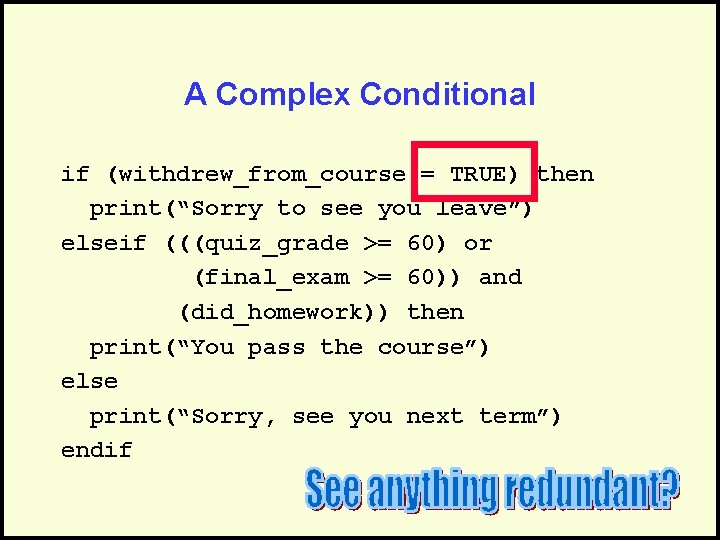
A Complex Conditional if (withdrew_from_course = TRUE) then print(“Sorry to see you leave”) elseif (((quiz_grade >= 60) or (final_exam >= 60)) and (did_homework)) then print(“You pass the course”) else print(“Sorry, see you next term”) endif
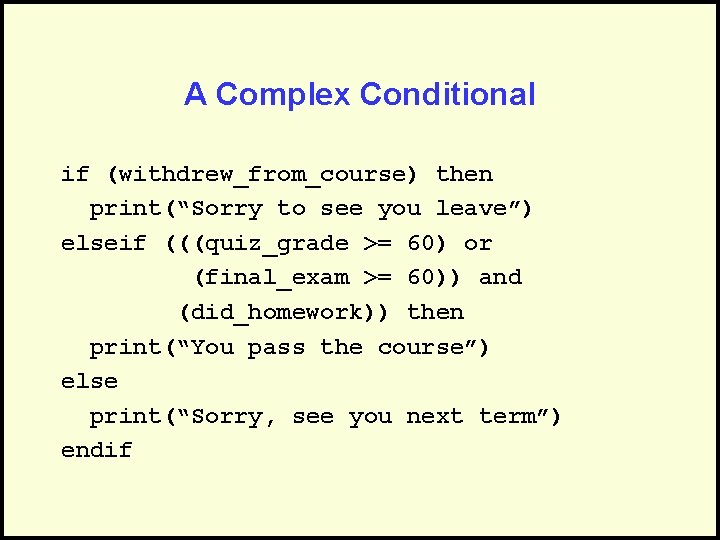
A Complex Conditional if (withdrew_from_course) then print(“Sorry to see you leave”) elseif (((quiz_grade >= 60) or (final_exam >= 60)) and (did_homework)) then print(“You pass the course”) else print(“Sorry, see you next term”) endif
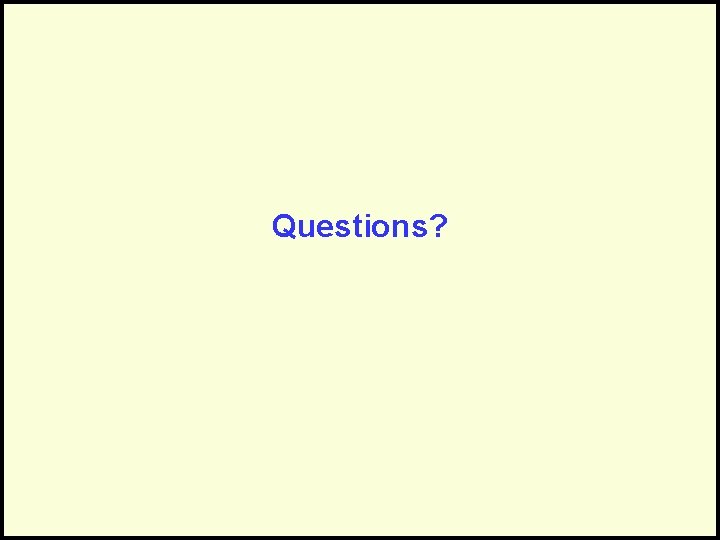
Questions?
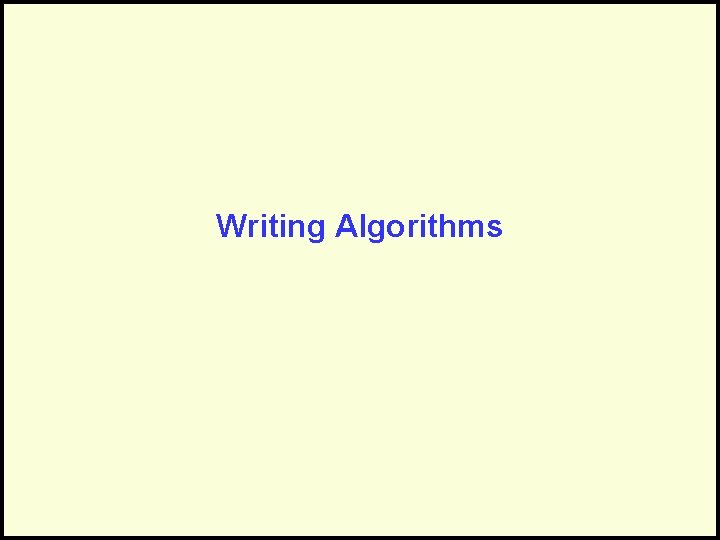
Writing Algorithms
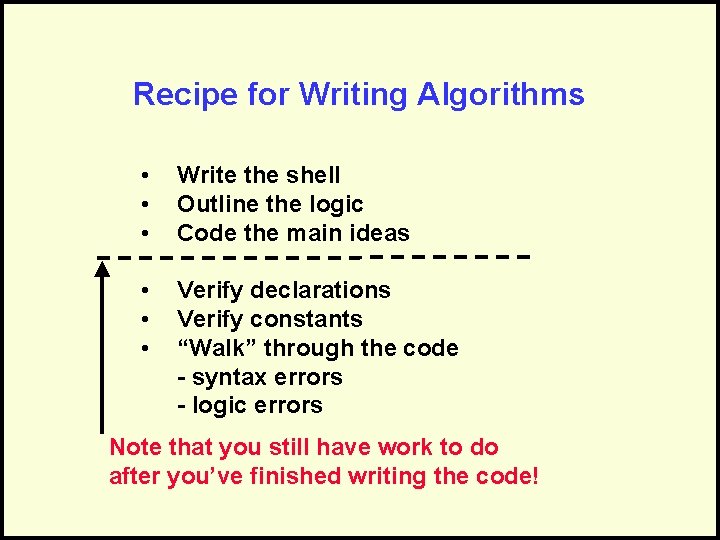
Recipe for Writing Algorithms • • • Write the shell Outline the logic Code the main ideas • • • Verify declarations Verify constants “Walk” through the code - syntax errors - logic errors Note that you still have work to do after you’ve finished writing the code!
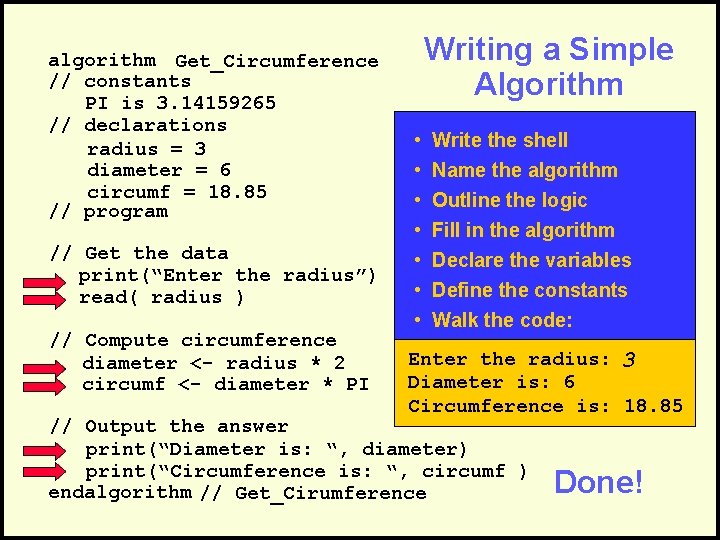
algorithm Get_Circumference // constants PI is 3. 14159265 // declarations radius = isoftype 3 Num = 6 diameter isoftype Num circumf isoftype = 18. 85 Num // program // Get the data print(“Enter the radius”) read( radius ) // Compute circumference diameter <- radius * 2 circumf <- diameter * PI Writing a Simple Algorithm • • Write the shell Name the algorithm Outline the logic Fill in the algorithm Declare the variables Define the constants Walk the code: Enter the radius: 3 Diameter is: 6 Circumference is: 18. 85 // Output the answer print(“Diameter is: “, diameter) print(“Circumference is: “, circumf ) endalgorithm // Get_Cirumference Done!
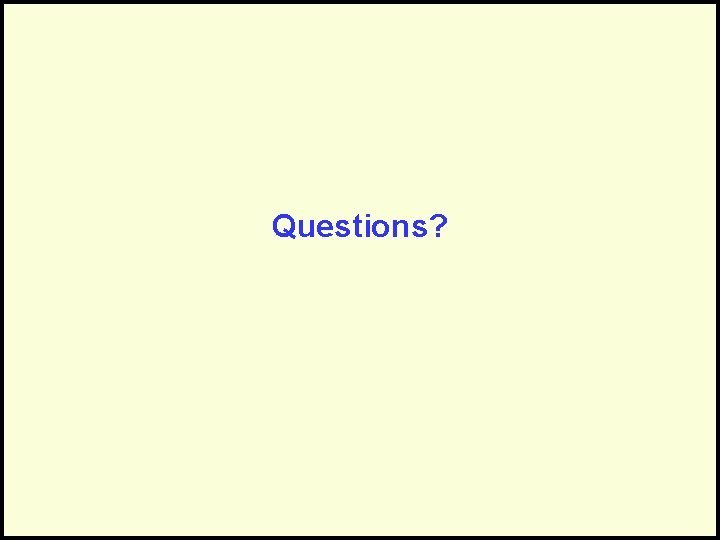
Questions?
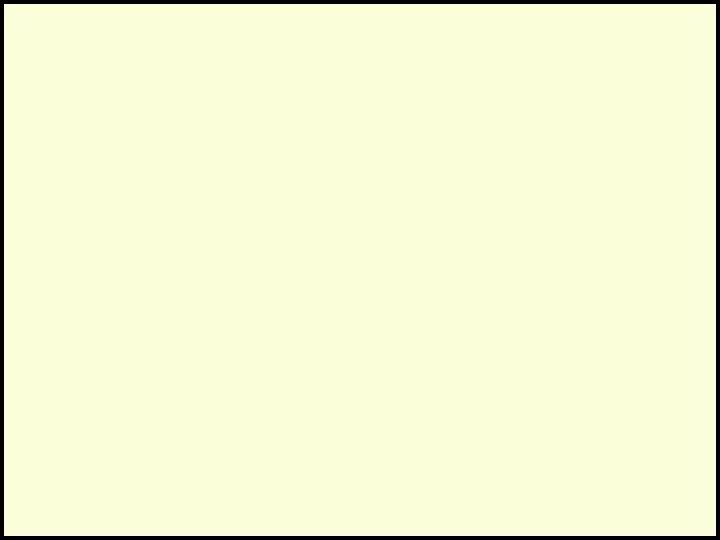