Control Conditionals Loops Comments Constants Conditionals The simple
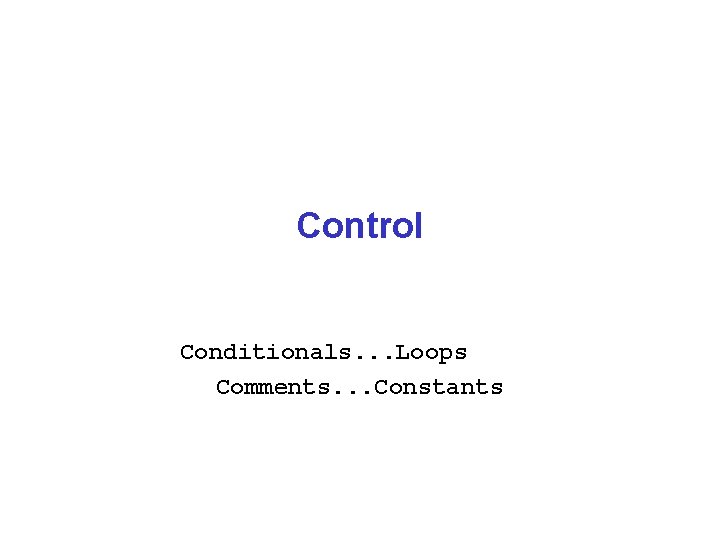
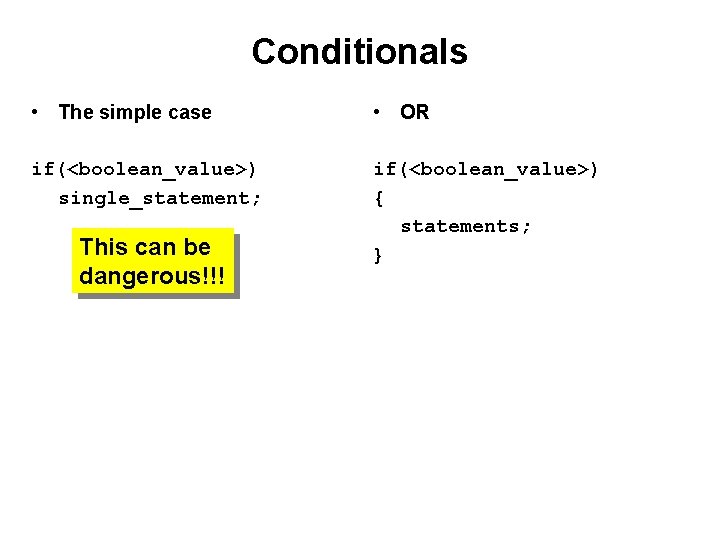
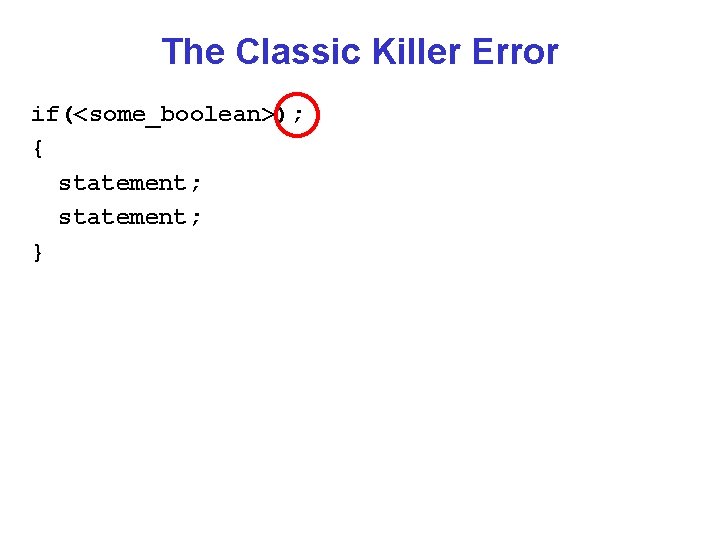
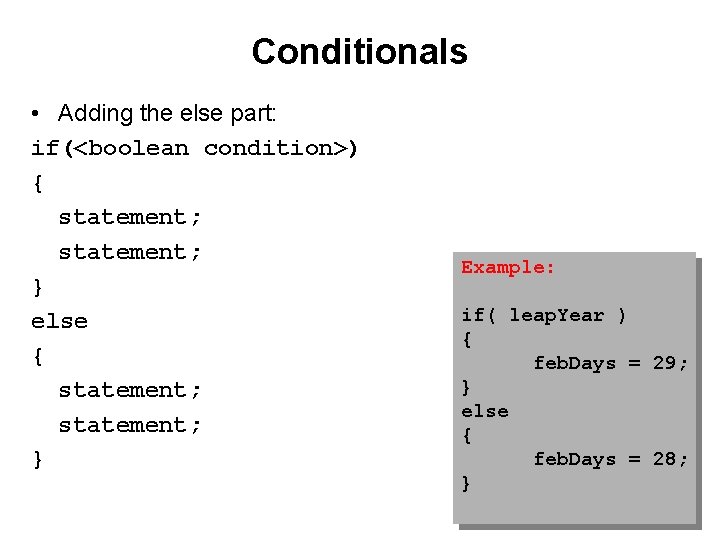
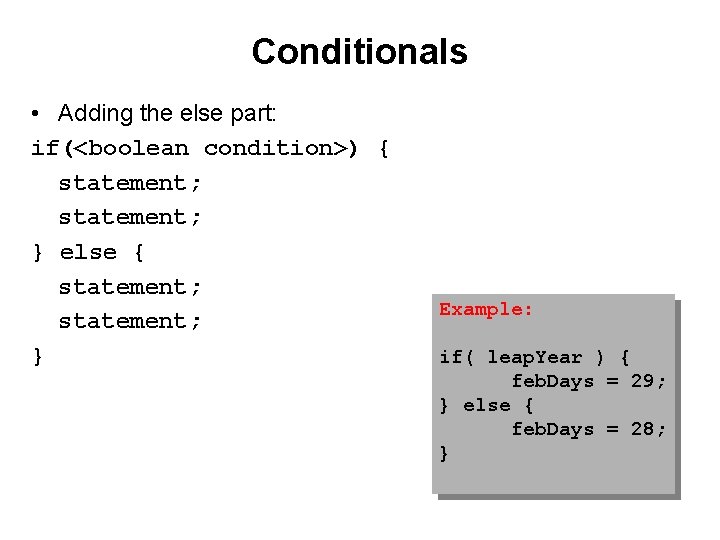
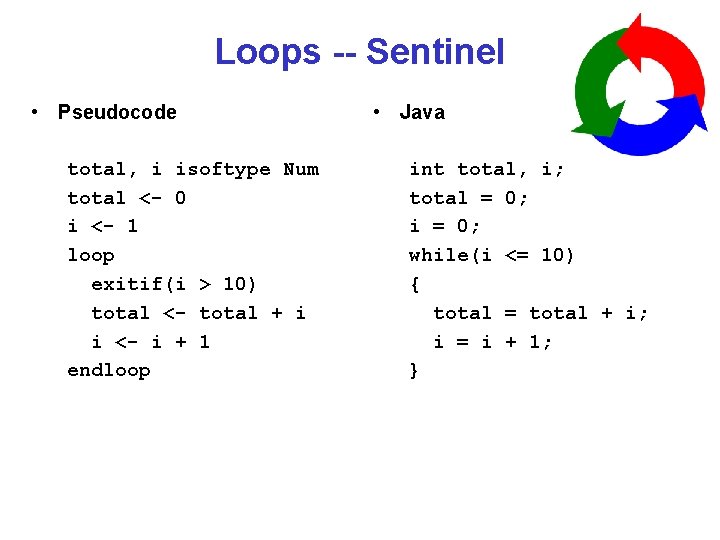
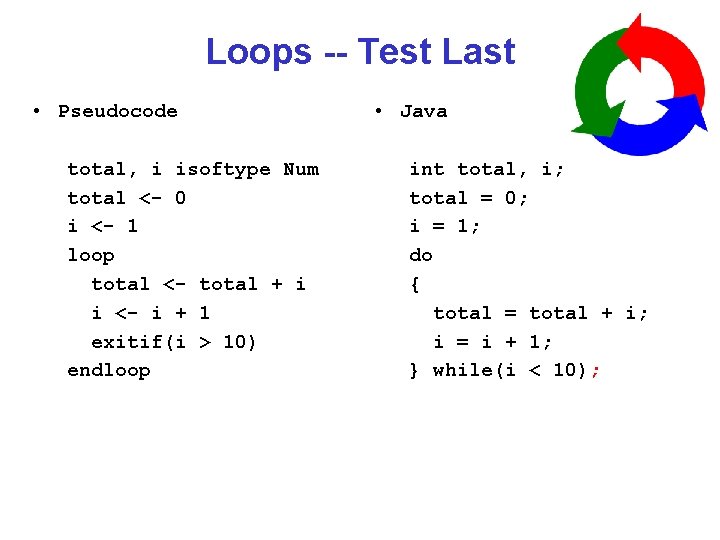
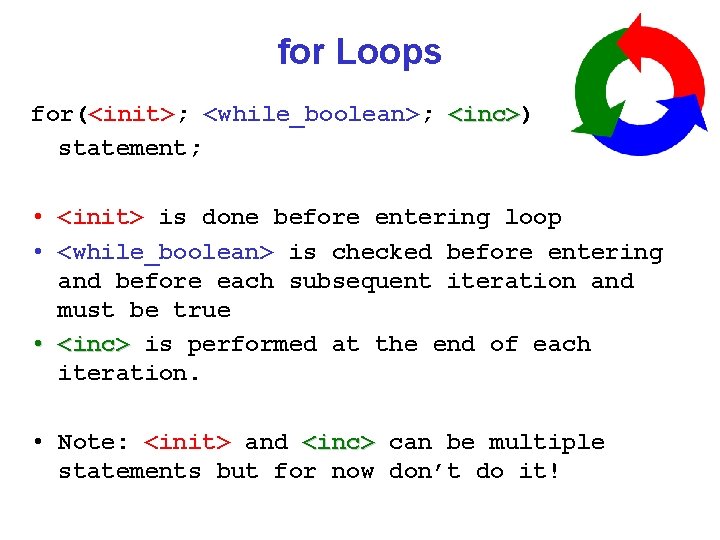
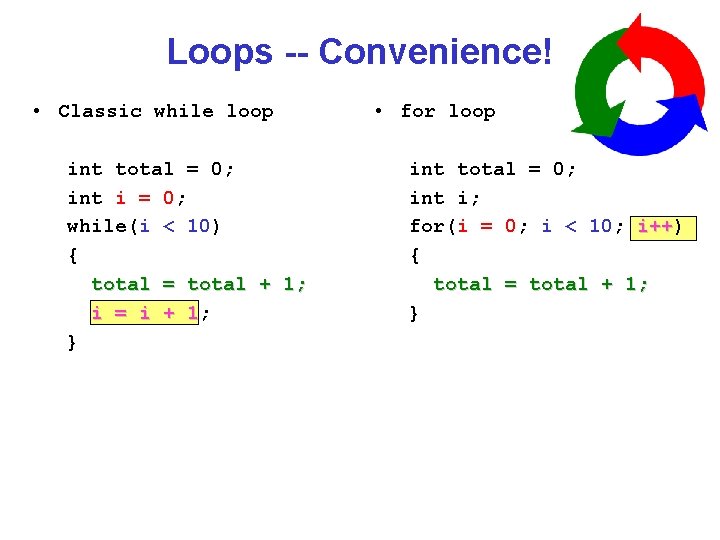
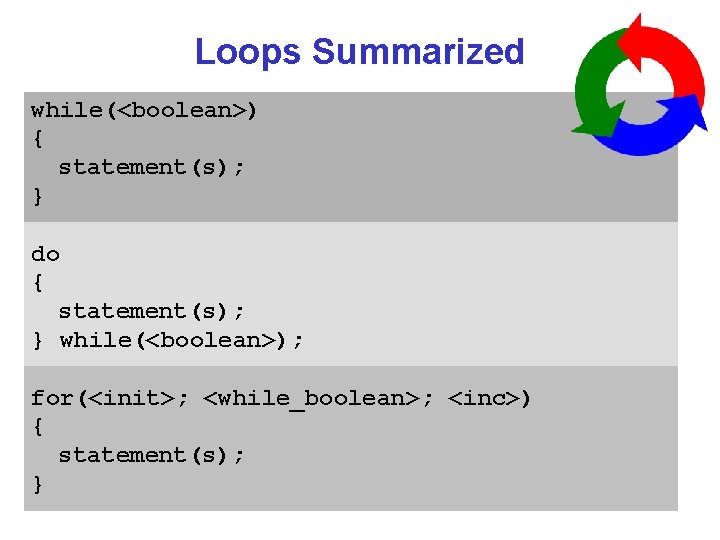
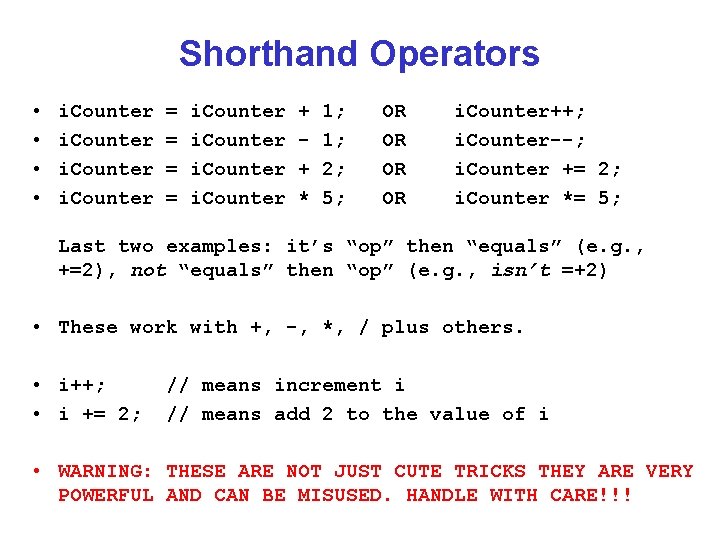
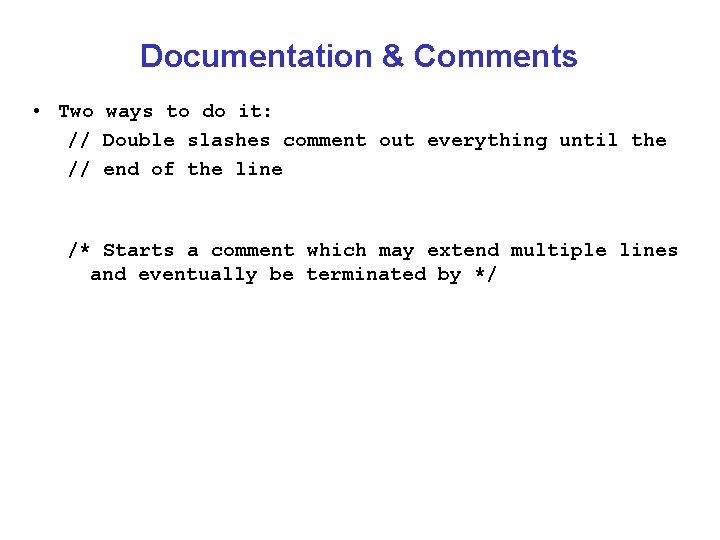
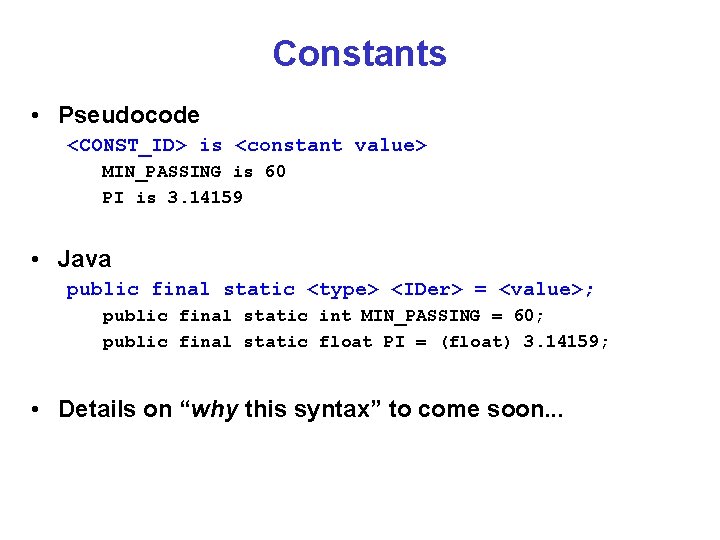
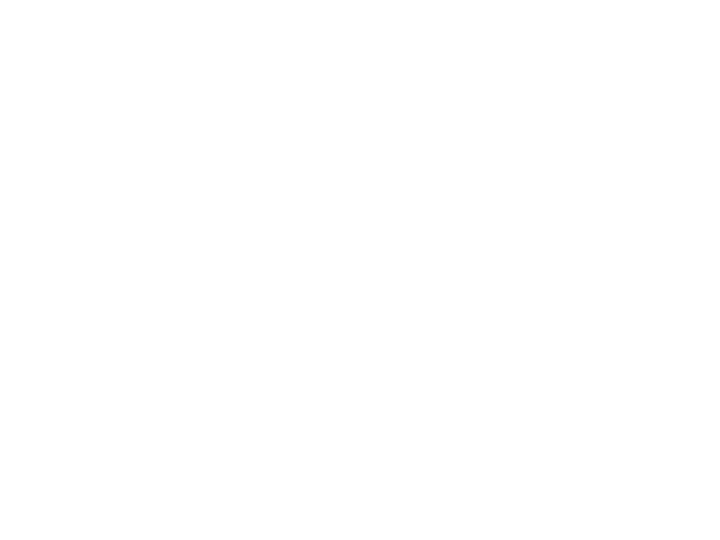
- Slides: 14
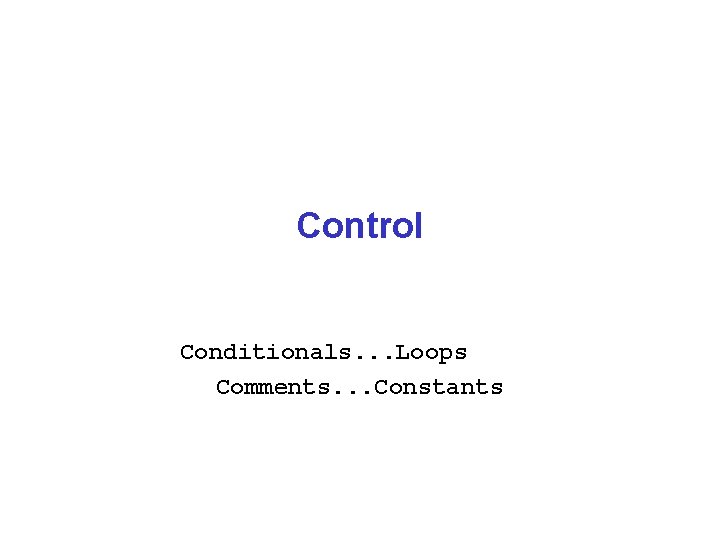
Control Conditionals. . . Loops Comments. . . Constants
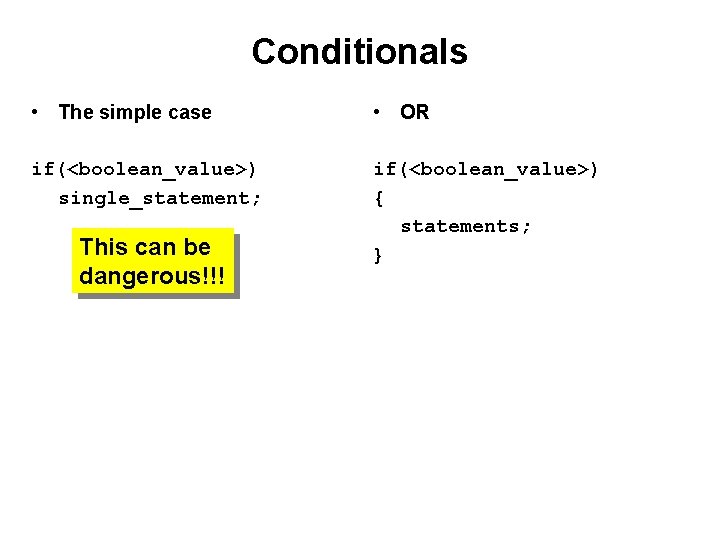
Conditionals • The simple case • OR if(<boolean_value>) single_statement; if(<boolean_value>) { statements; } This can be dangerous!!!
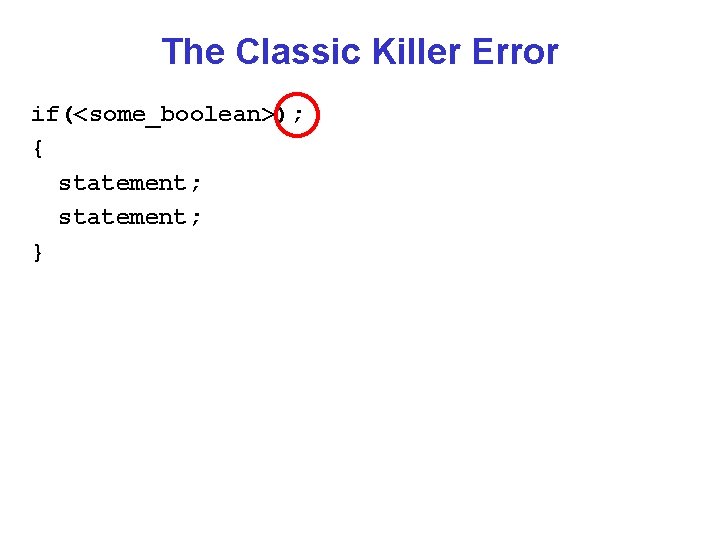
The Classic Killer Error if(<some_boolean>); { statement; }
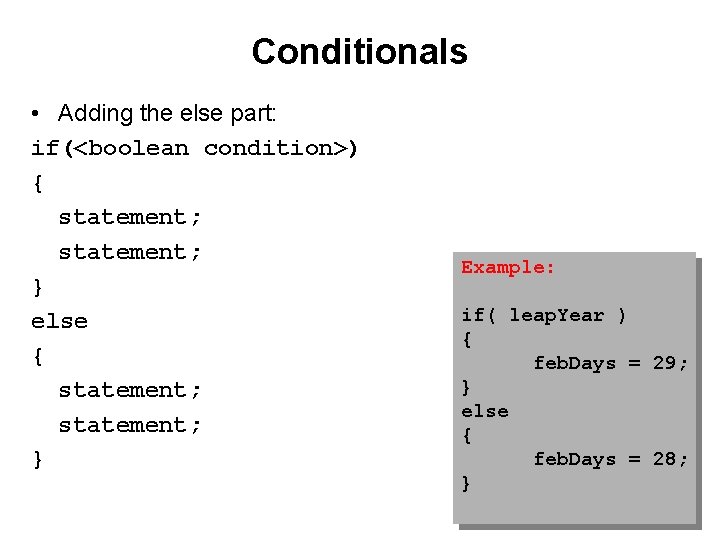
Conditionals • Adding the else part: if(<boolean condition>) { statement; } else { statement; } Example: if( leap. Year ) { feb. Days = 29; } else { feb. Days = 28; }
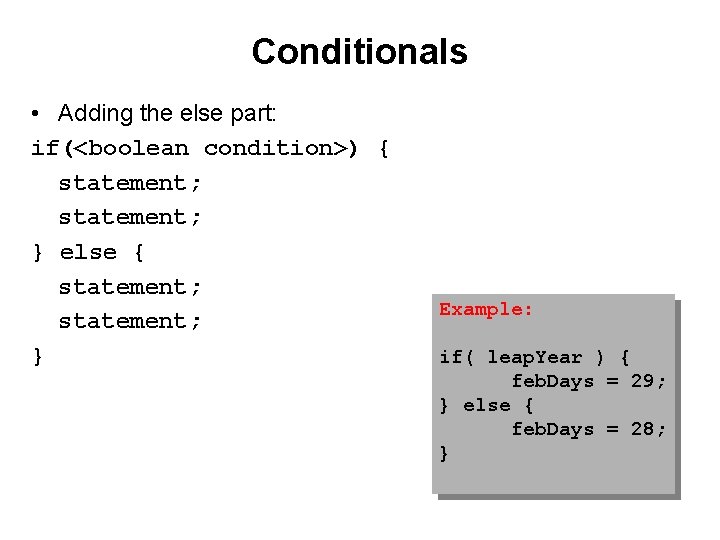
Conditionals • Adding the else part: if(<boolean condition>) { statement; } else { statement; } Example: if( leap. Year ) { feb. Days = 29; } else { feb. Days = 28; }
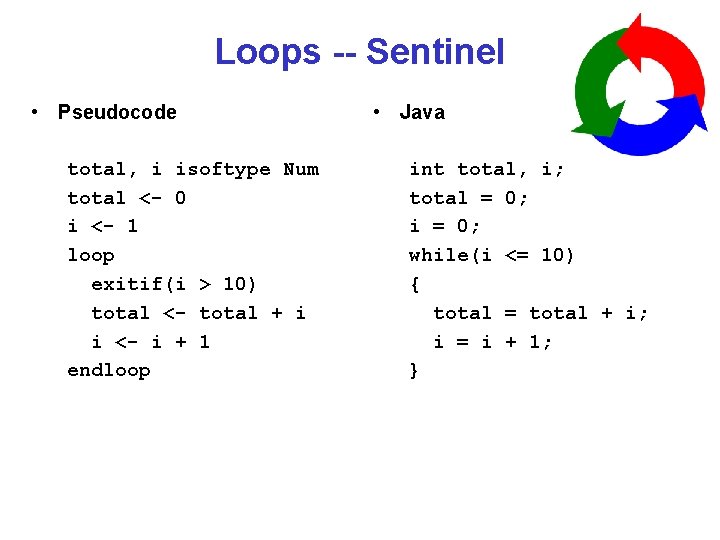
Loops -- Sentinel • Pseudocode total, i isoftype Num total <- 0 i <- 1 loop exitif(i > 10) total <- total + i i <- i + 1 endloop • Java int total, i; total = 0; i = 0; while(i <= 10) { total = total + i; i = i + 1; }
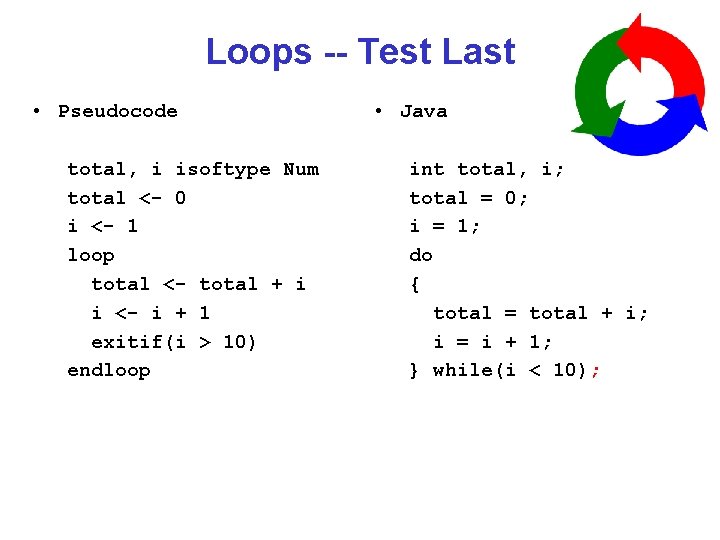
Loops -- Test Last • Pseudocode total, i isoftype Num total <- 0 i <- 1 loop total <- total + i i <- i + 1 exitif(i > 10) endloop • Java int total, i; total = 0; i = 1; do { total = total + i; i = i + 1; } while(i < 10);
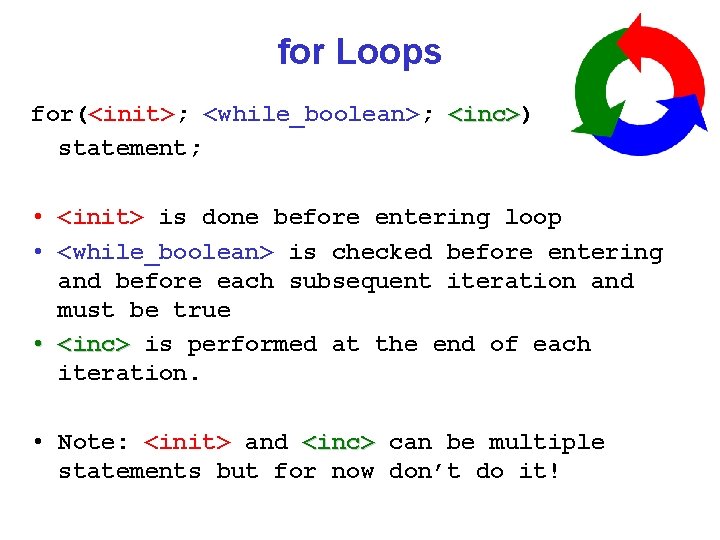
for Loops for(<init>; <while_boolean>; <inc>) statement; • <init> is done before entering loop • <while_boolean> is checked before entering and before each subsequent iteration and must be true • <inc> is performed at the end of each iteration. • Note: <init> and <inc> can be multiple statements but for now don’t do it!
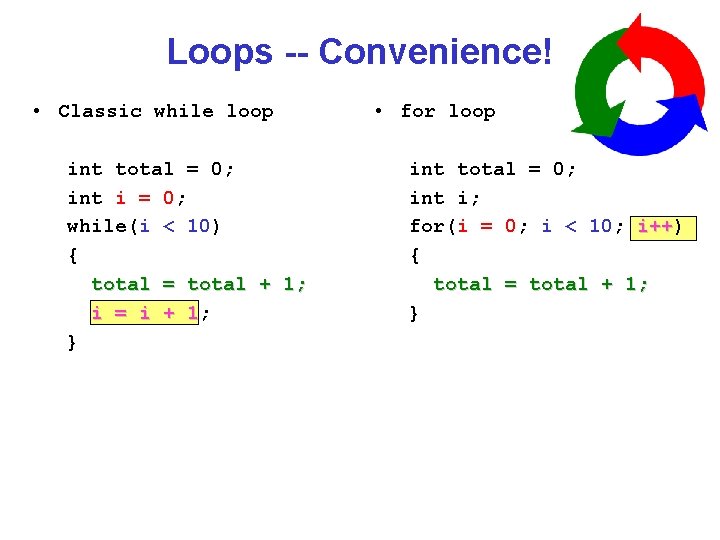
Loops -- Convenience! • Classic while loop int total = 0; int i = 0; while(i < 10) { total = total + 1; i = i + 1; 1 } • for loop int total = 0; int i; for(i = 0; i < 10; i++) i++ { total = total + 1; }
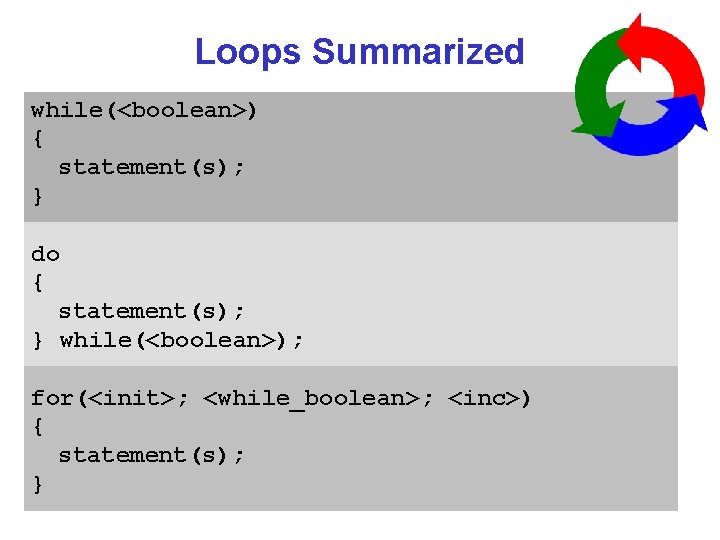
Loops Summarized while(<boolean>) { statement(s); } do { statement(s); } while(<boolean>); for(<init>; <while_boolean>; <inc>) { statement(s); }
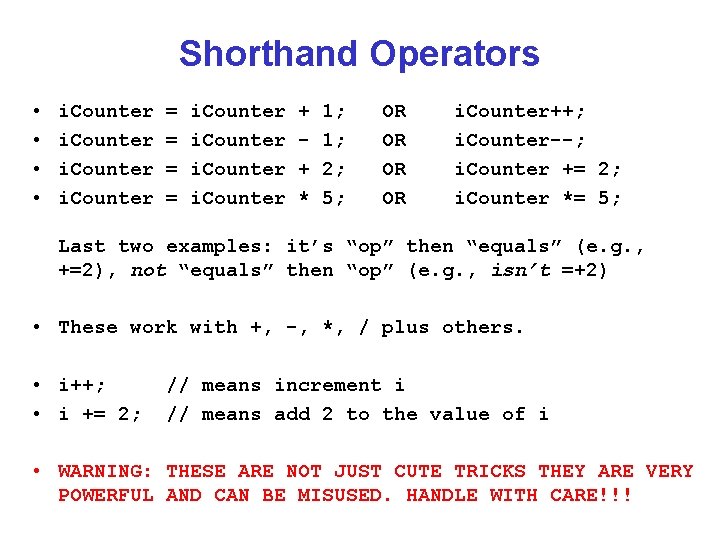
Shorthand Operators • • i. Counter = = i. Counter + + * 1; 1; 2; 5; OR OR i. Counter++; i. Counter--; i. Counter += 2; i. Counter *= 5; Last two examples: it’s “op” then “equals” (e. g. , +=2), not “equals” then “op” (e. g. , isn’t =+2) • These work with +, -, *, / plus others. • i++; • i += 2; // means increment i // means add 2 to the value of i • WARNING: THESE ARE NOT JUST CUTE TRICKS THEY ARE VERY POWERFUL AND CAN BE MISUSED. HANDLE WITH CARE!!!
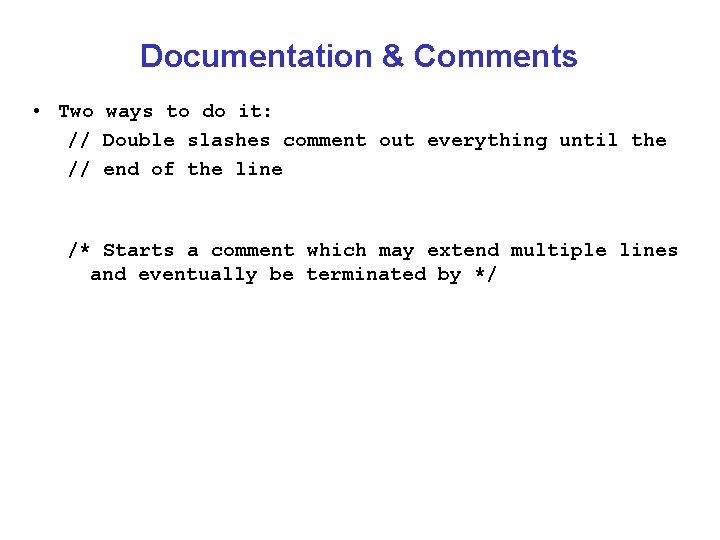
Documentation & Comments • Two ways to do it: // Double slashes comment out everything until the // end of the line /* Starts a comment which may extend multiple lines and eventually be terminated by */
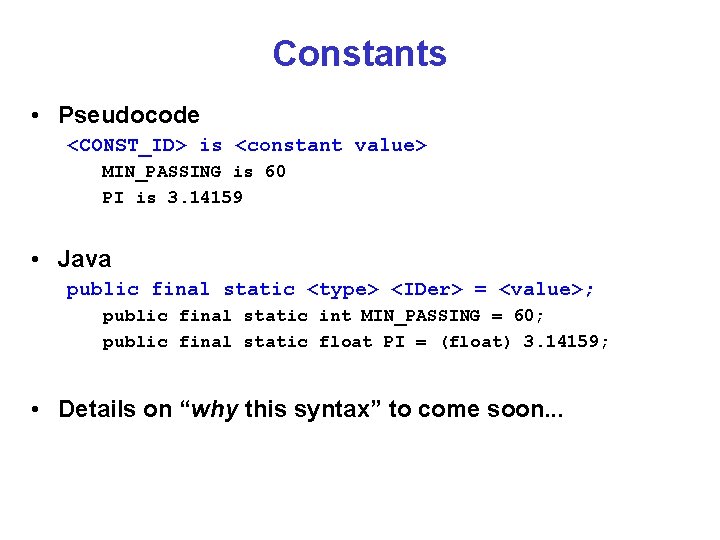
Constants • Pseudocode <CONST_ID> is <constant value> MIN_PASSING is 60 PI is 3. 14159 • Java public final static <type> <IDer> = <value>; public final static int MIN_PASSING = 60; public final static float PI = (float) 3. 14159; • Details on “why this syntax” to come soon. . .
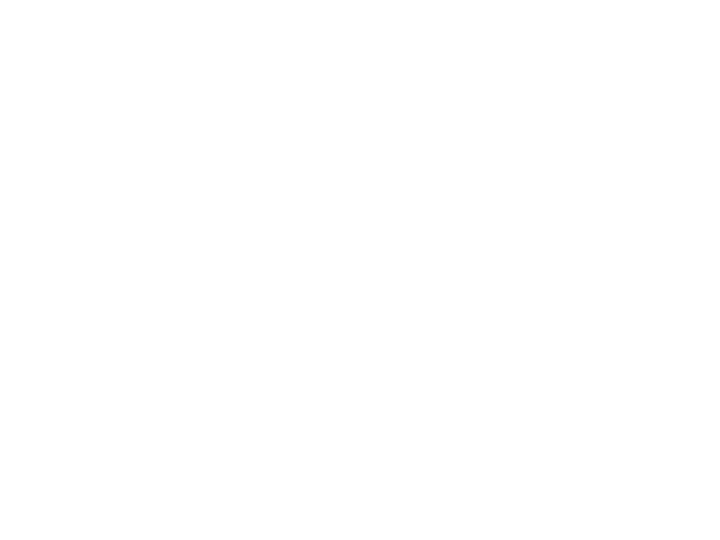
Error constant in control system
C chart six sigma
Non touching loop in control system
Const in c#
Constant integer
Named constants
Factors influencing chemical shift
Geminal coupling
J coupling constant
Geminal and vicinal coupling constants
Charles law constant
Crystal binding and elastic constants
The timing device in an automobile's intermittent
Definition of le chatelier's principle
Rc time constants