Numeric literals and named constants Numeric literals Numeric
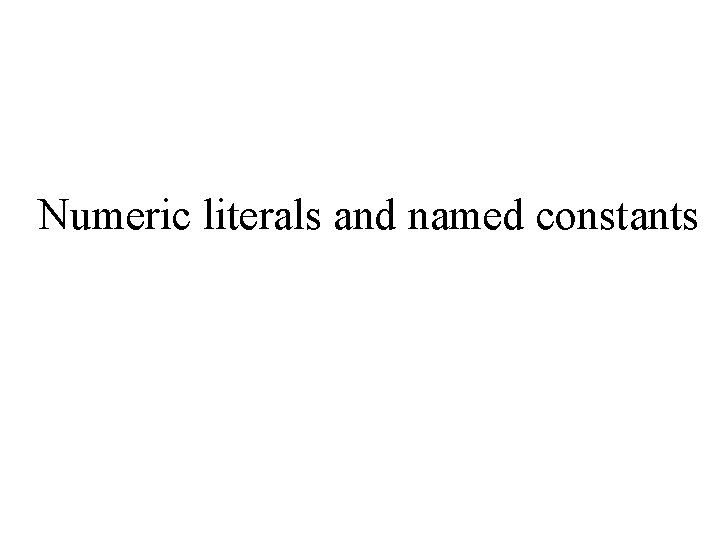
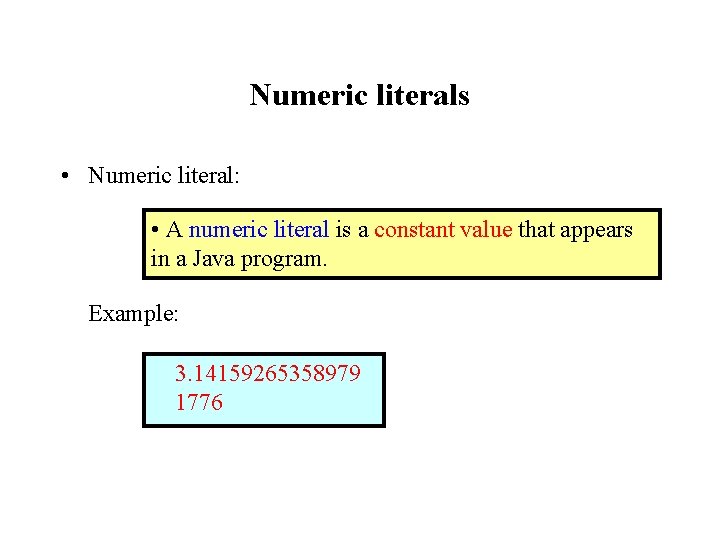
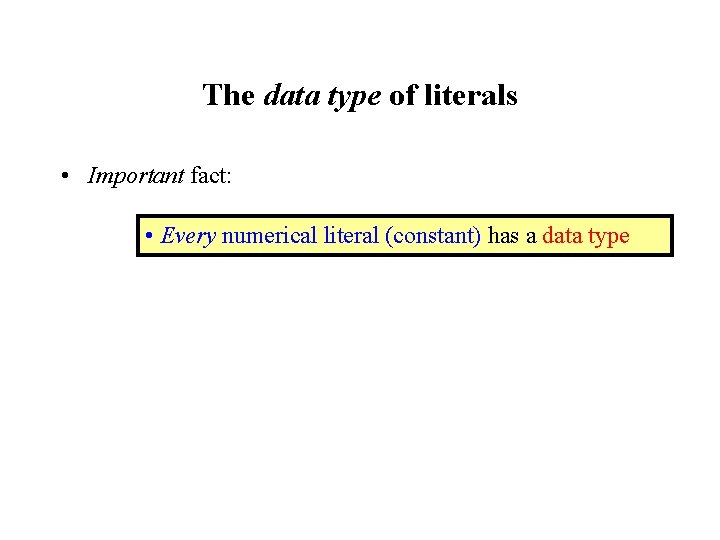
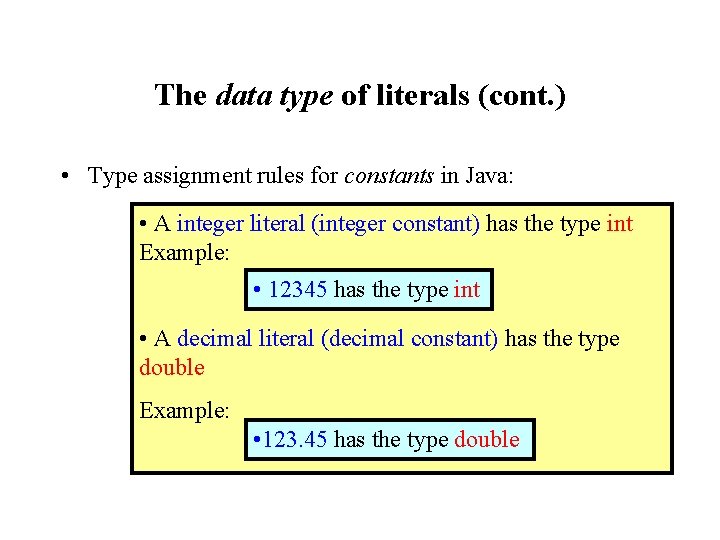
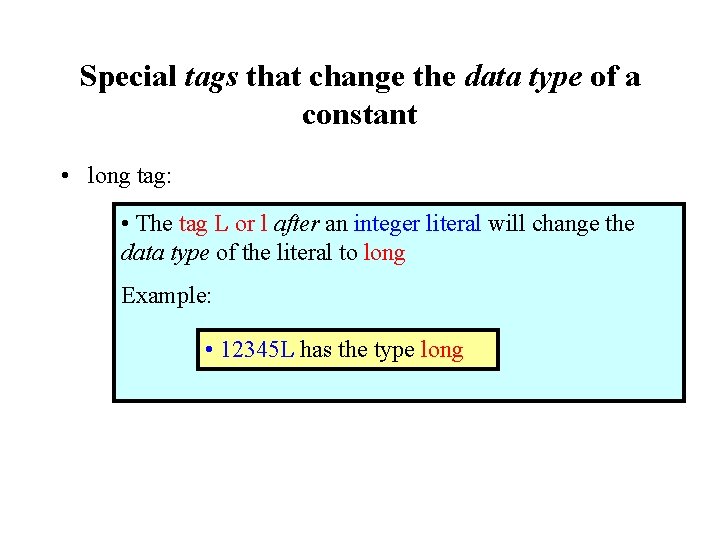
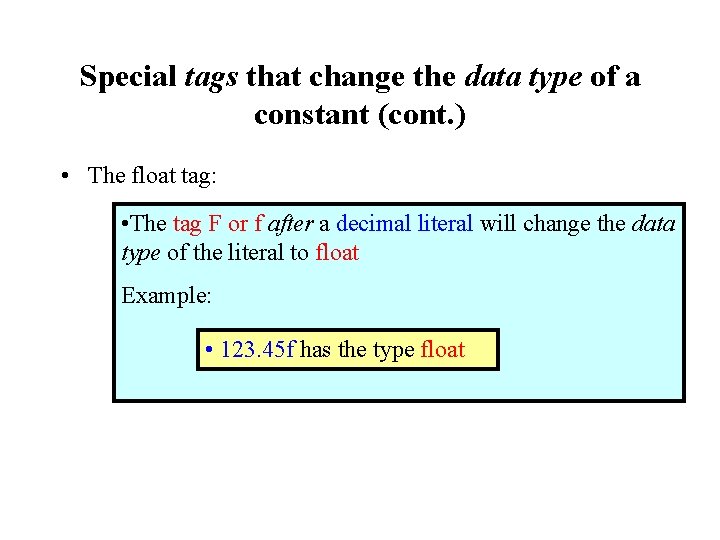
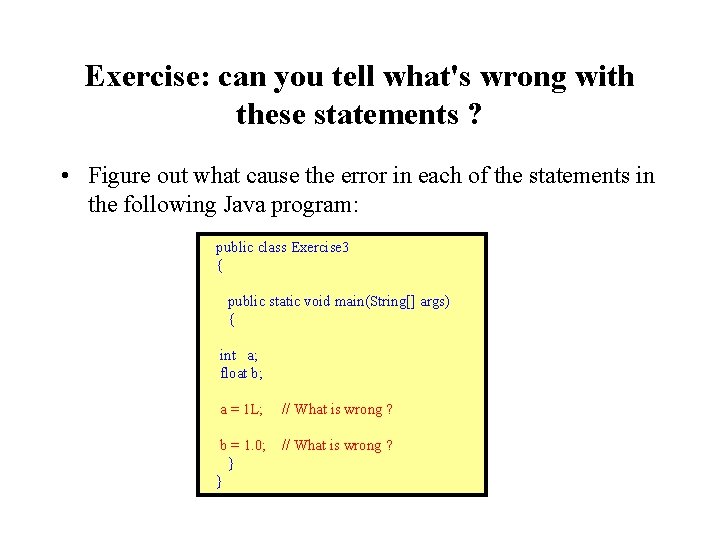
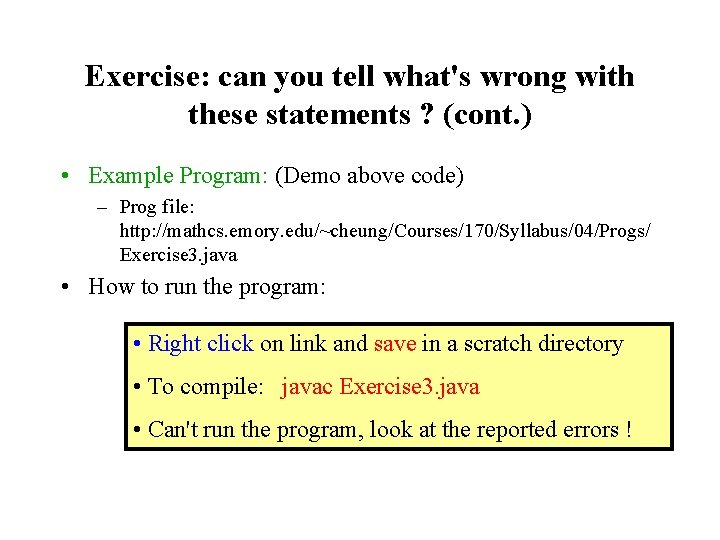
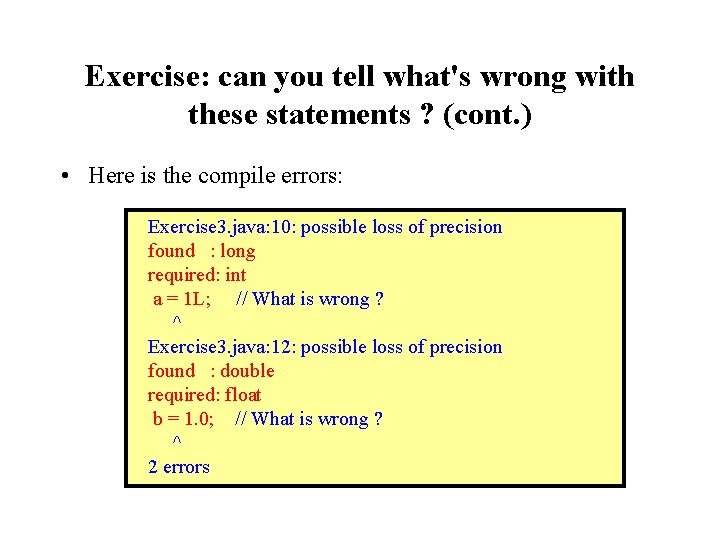
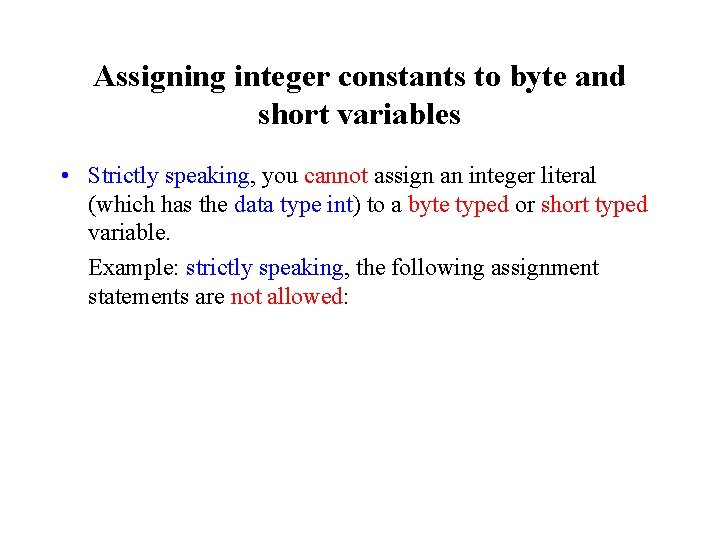
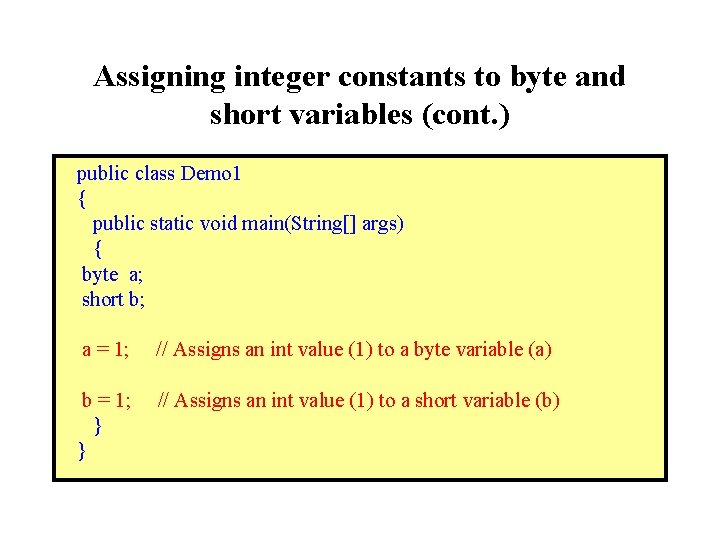
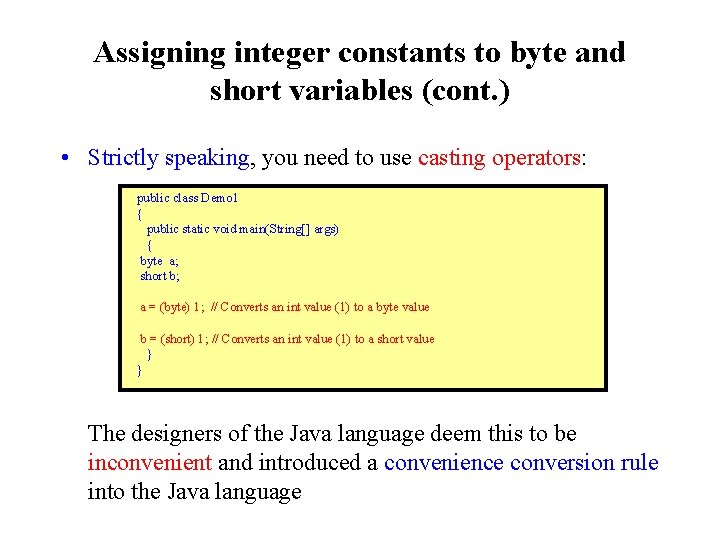
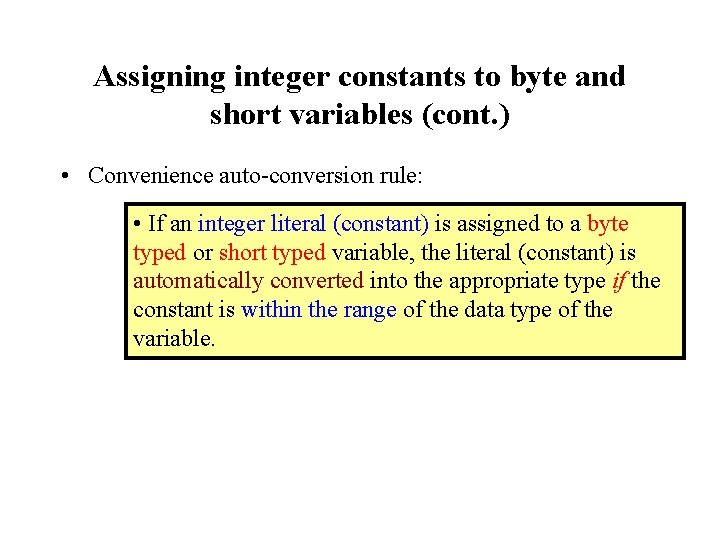
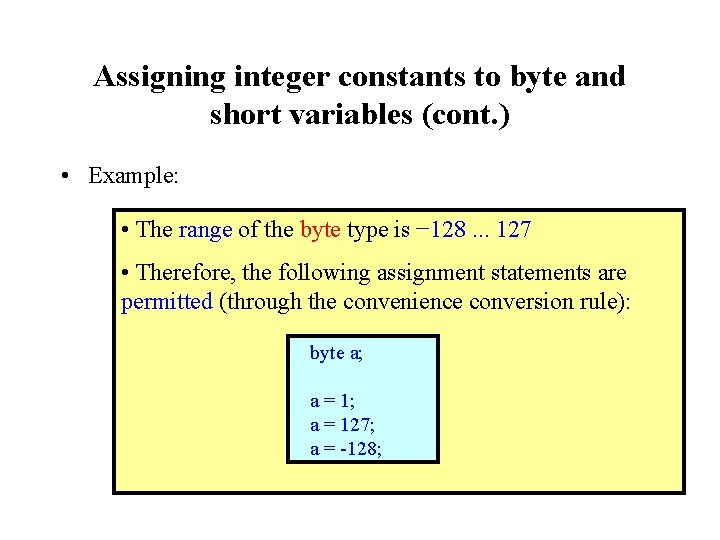
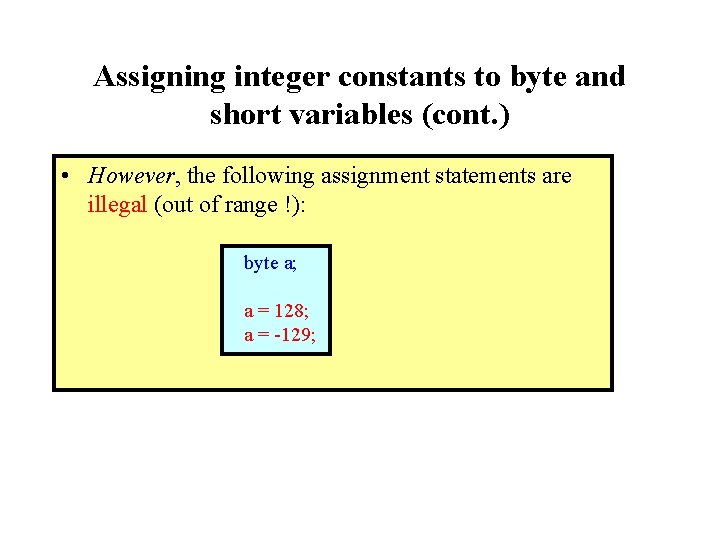
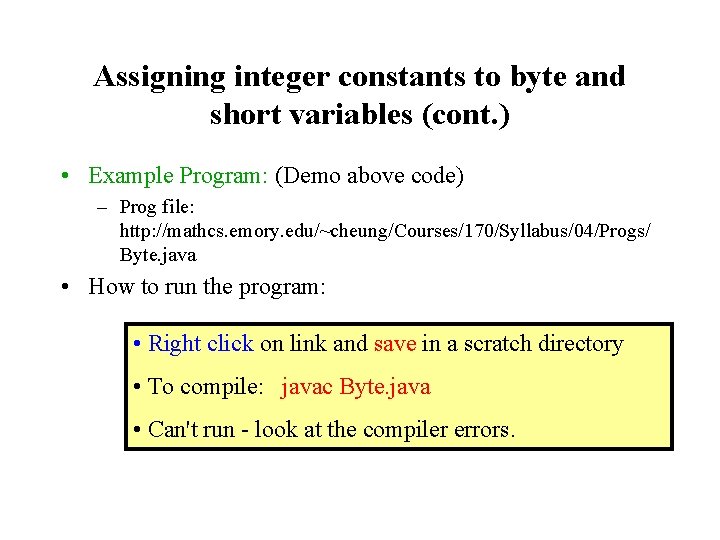
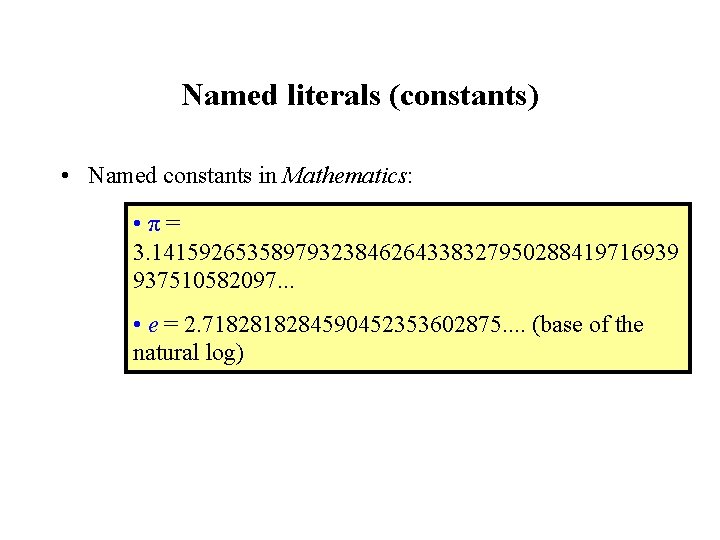
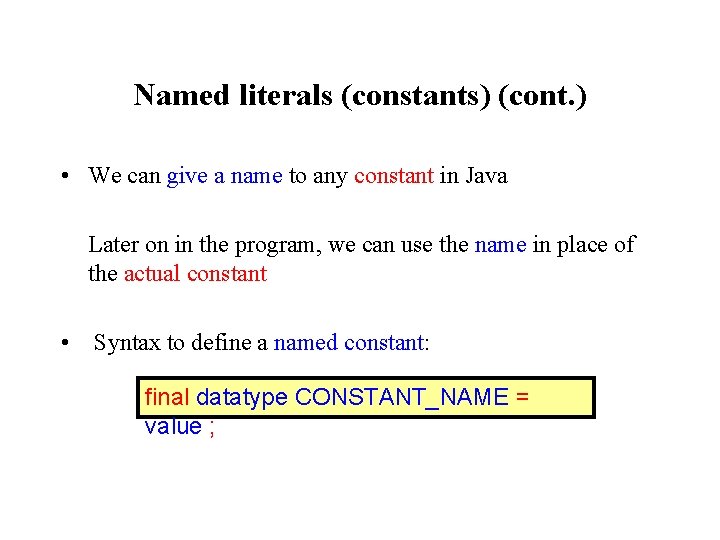
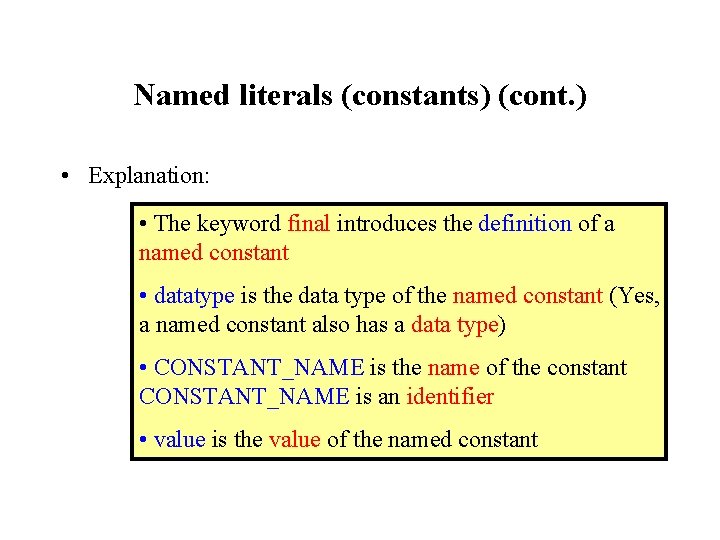
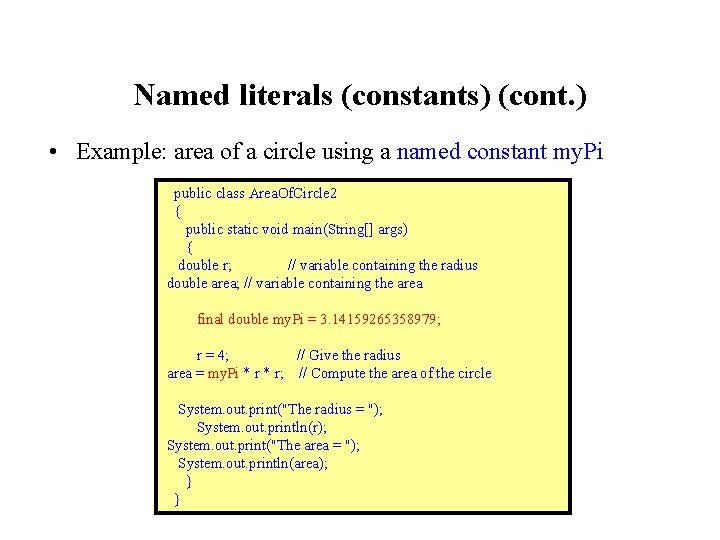
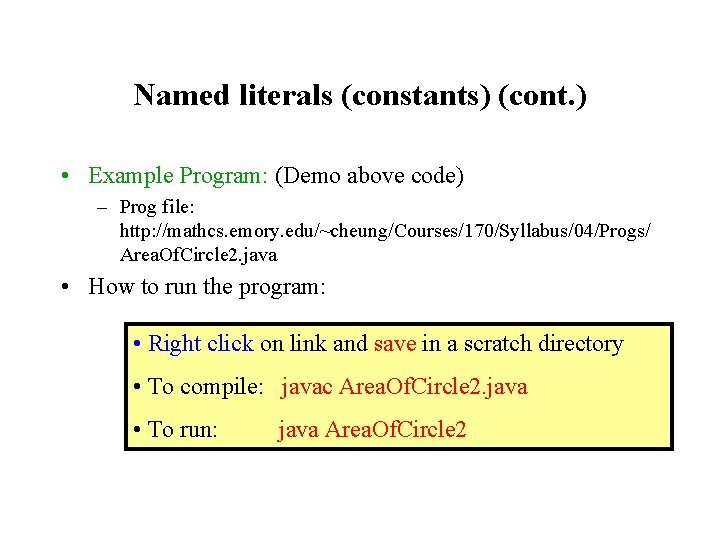
- Slides: 21
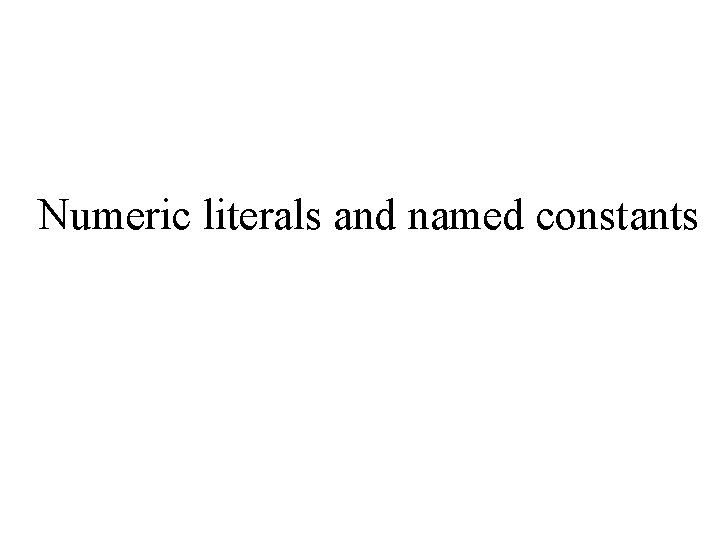
Numeric literals and named constants
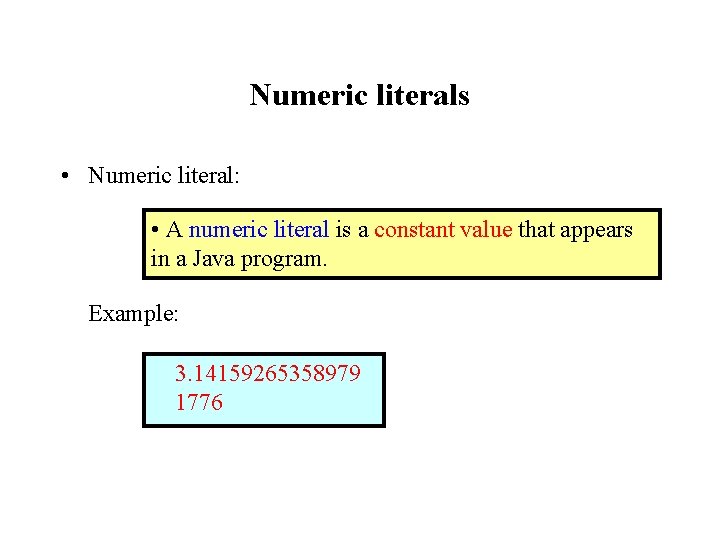
Numeric literals • Numeric literal: • A numeric literal is a constant value that appears in a Java program. Example: 3. 14159265358979 1776
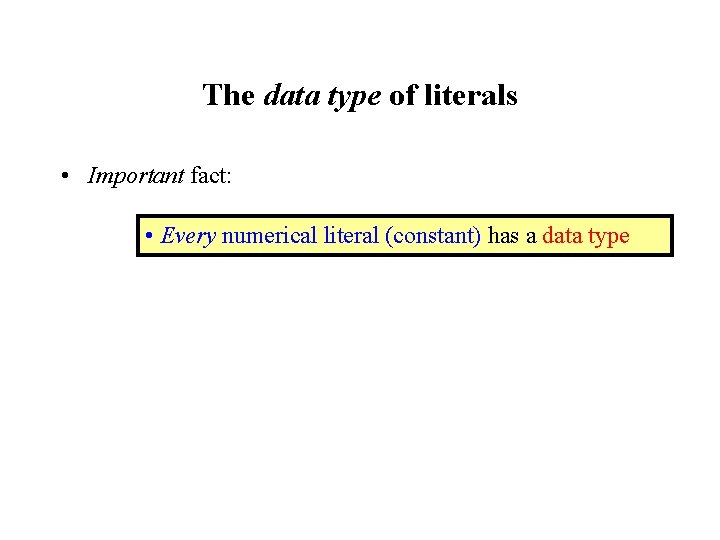
The data type of literals • Important fact: • Every numerical literal (constant) has a data type
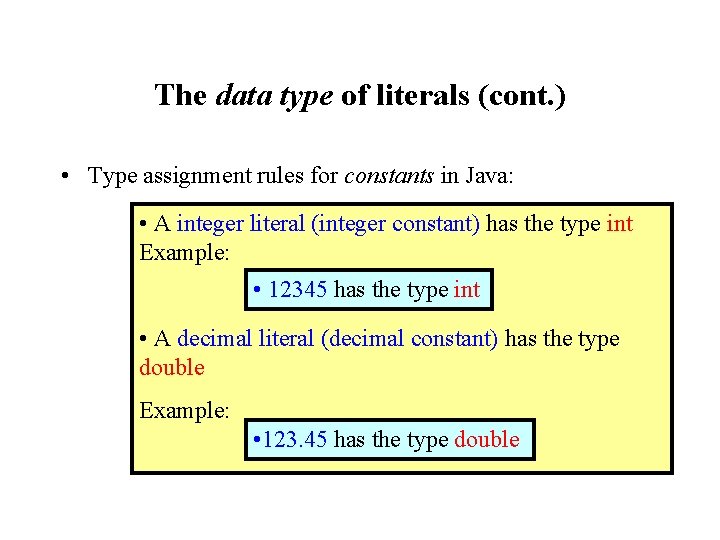
The data type of literals (cont. ) • Type assignment rules for constants in Java: • A integer literal (integer constant) has the type int Example: • 12345 has the type int • A decimal literal (decimal constant) has the type double Example: • 123. 45 has the type double
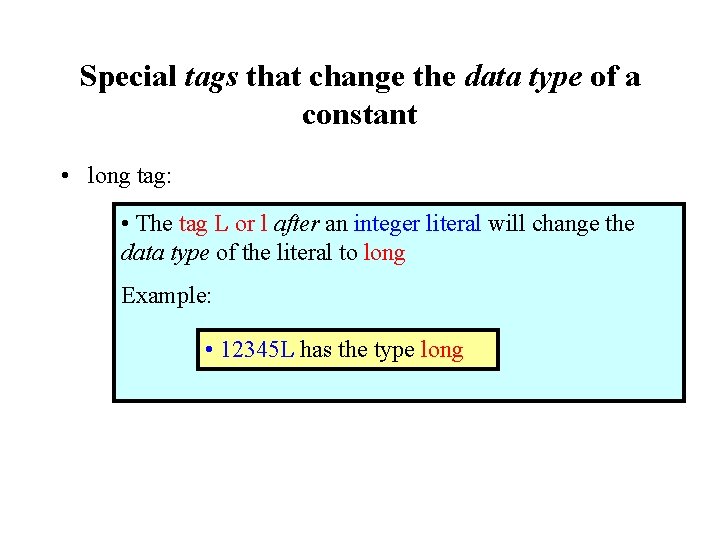
Special tags that change the data type of a constant • long tag: • The tag L or l after an integer literal will change the data type of the literal to long Example: • 12345 L has the type long
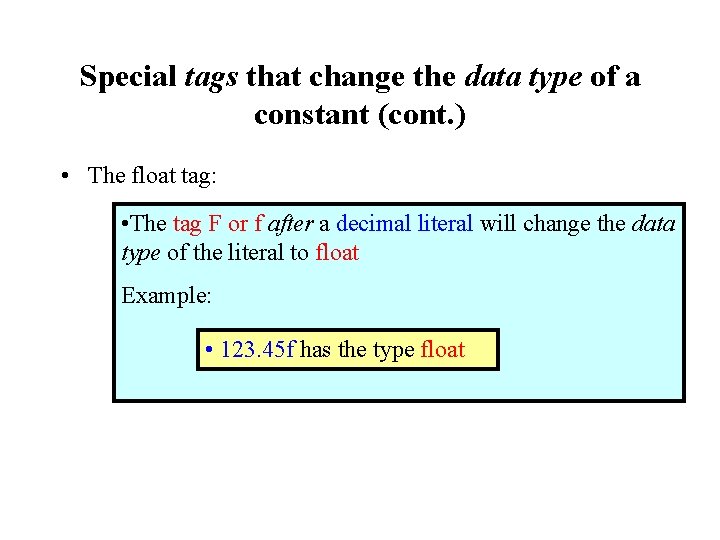
Special tags that change the data type of a constant (cont. ) • The float tag: • The tag F or f after a decimal literal will change the data type of the literal to float Example: • 123. 45 f has the type float
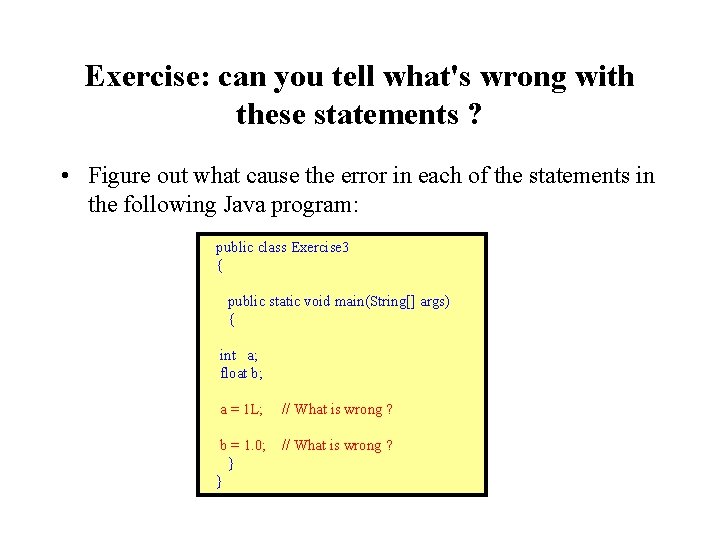
Exercise: can you tell what's wrong with these statements ? • Figure out what cause the error in each of the statements in the following Java program: public class Exercise 3 { public static void main(String[] args) { int a; float b; a = 1 L; // What is wrong ? b = 1. 0; // What is wrong ? } }
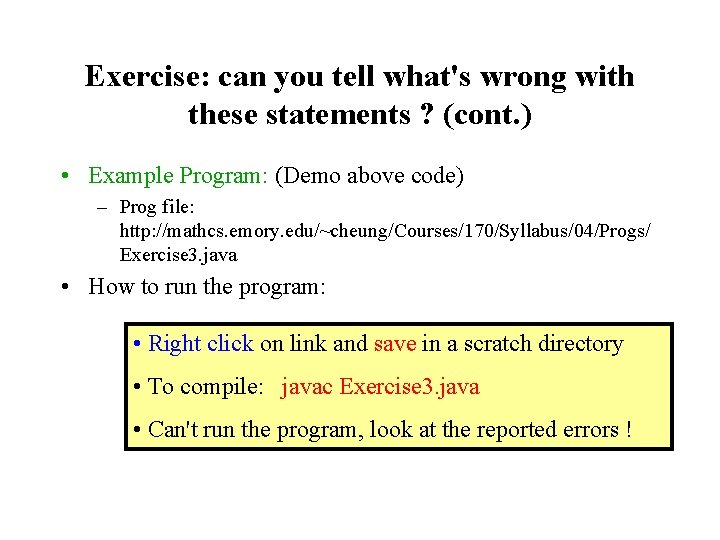
Exercise: can you tell what's wrong with these statements ? (cont. ) • Example Program: (Demo above code) – Prog file: http: //mathcs. emory. edu/~cheung/Courses/170/Syllabus/04/Progs/ Exercise 3. java • How to run the program: • Right click on link and save in a scratch directory • To compile: javac Exercise 3. java • Can't run the program, look at the reported errors !
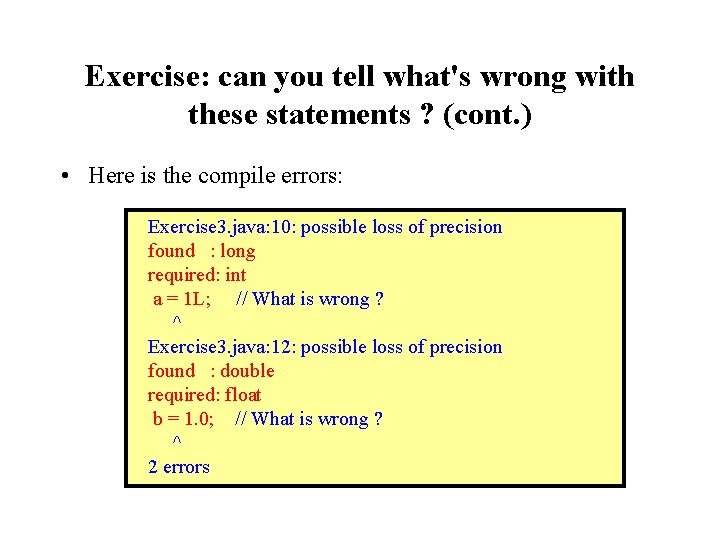
Exercise: can you tell what's wrong with these statements ? (cont. ) • Here is the compile errors: Exercise 3. java: 10: possible loss of precision found : long required: int a = 1 L; // What is wrong ? ^ Exercise 3. java: 12: possible loss of precision found : double required: float b = 1. 0; // What is wrong ? ^ 2 errors
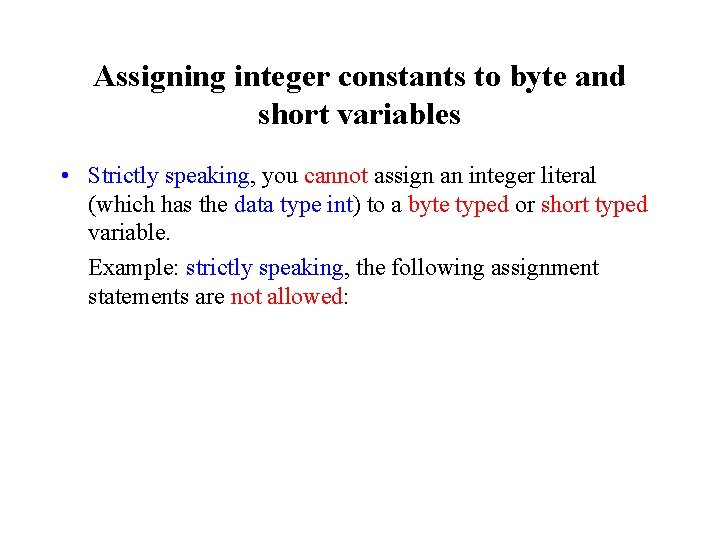
Assigning integer constants to byte and short variables • Strictly speaking, you cannot assign an integer literal (which has the data type int) to a byte typed or short typed variable. Example: strictly speaking, the following assignment statements are not allowed:
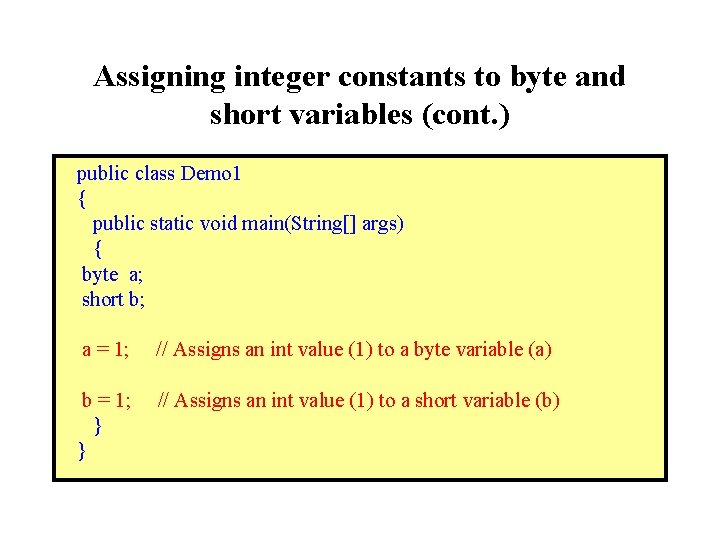
Assigning integer constants to byte and short variables (cont. ) public class Demo 1 { public static void main(String[] args) { byte a; short b; a = 1; // Assigns an int value (1) to a byte variable (a) b = 1; // Assigns an int value (1) to a short variable (b) } }
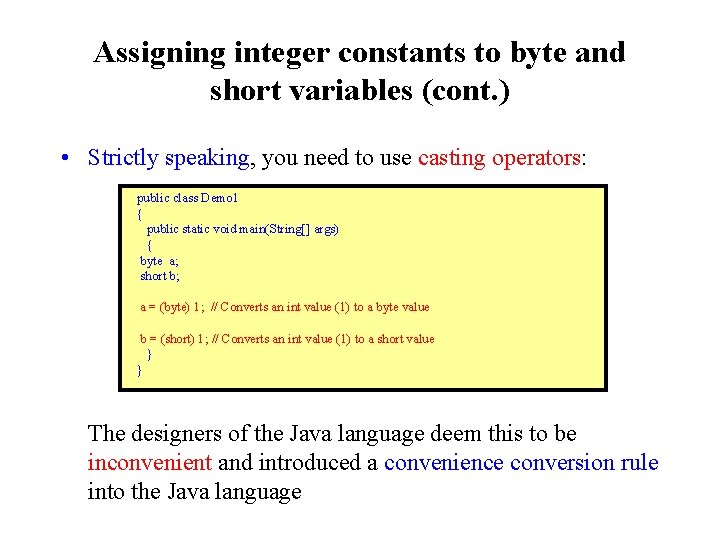
Assigning integer constants to byte and short variables (cont. ) • Strictly speaking, you need to use casting operators: public class Demo 1 { public static void main(String[] args) { byte a; short b; a = (byte) 1; // Converts an int value (1) to a byte value b = (short) 1; // Converts an int value (1) to a short value } } The designers of the Java language deem this to be inconvenient and introduced a convenience conversion rule into the Java language
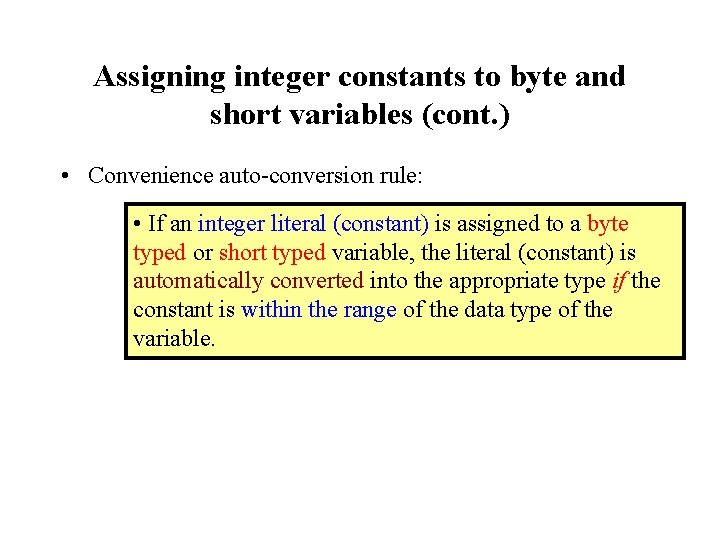
Assigning integer constants to byte and short variables (cont. ) • Convenience auto-conversion rule: • If an integer literal (constant) is assigned to a byte typed or short typed variable, the literal (constant) is automatically converted into the appropriate type if the constant is within the range of the data type of the variable.
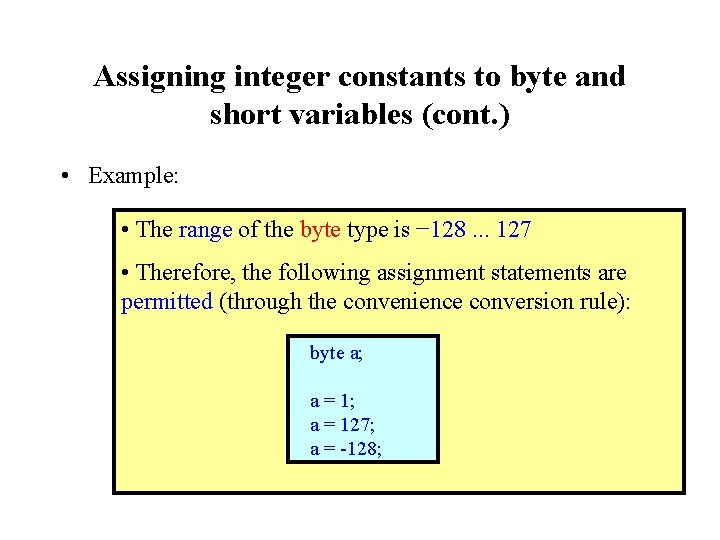
Assigning integer constants to byte and short variables (cont. ) • Example: • The range of the byte type is − 128. . . 127 • Therefore, the following assignment statements are permitted (through the convenience conversion rule): byte a; a = 127; a = -128;
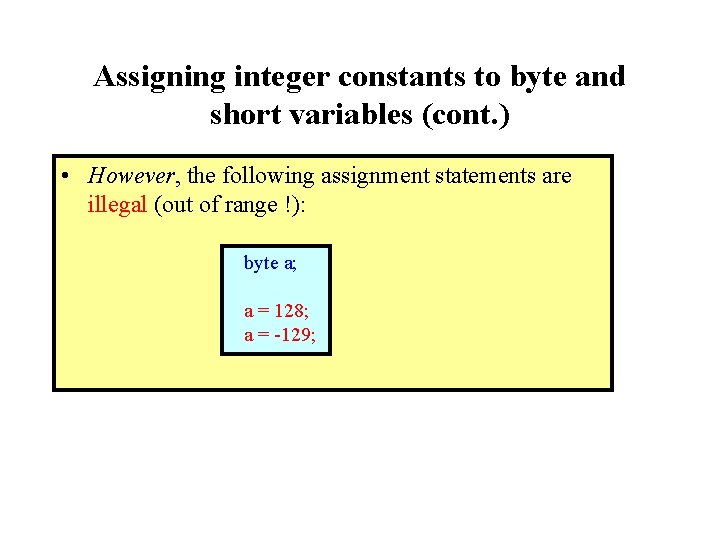
Assigning integer constants to byte and short variables (cont. ) • However, the following assignment statements are illegal (out of range !): byte a; a = 128; a = -129;
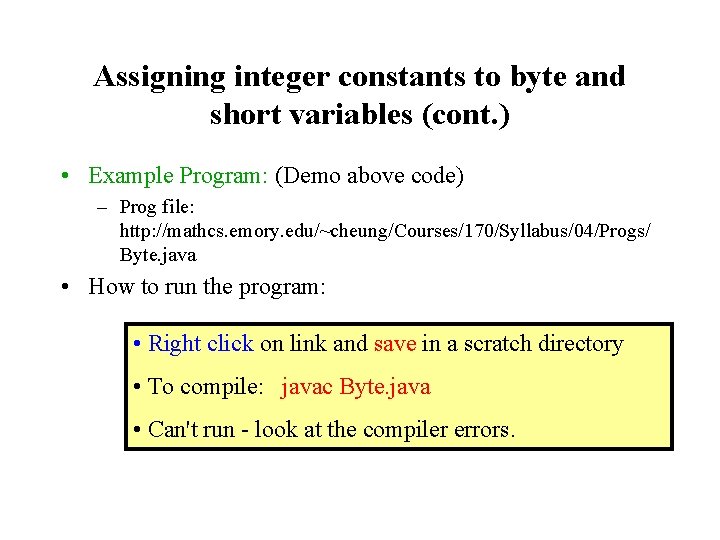
Assigning integer constants to byte and short variables (cont. ) • Example Program: (Demo above code) – Prog file: http: //mathcs. emory. edu/~cheung/Courses/170/Syllabus/04/Progs/ Byte. java • How to run the program: • Right click on link and save in a scratch directory • To compile: javac Byte. java • Can't run - look at the compiler errors.
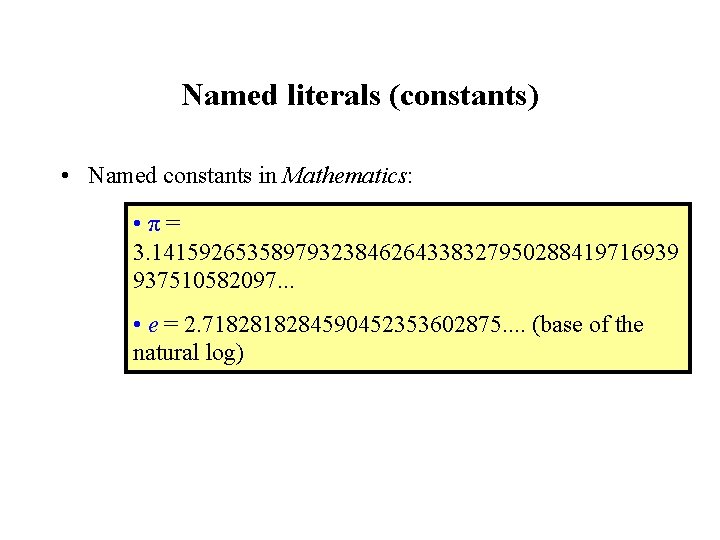
Named literals (constants) • Named constants in Mathematics: • π = 3. 14159265358979323846264338327950288419716939 937510582097. . . • e = 2. 718284590452353602875. . (base of the natural log)
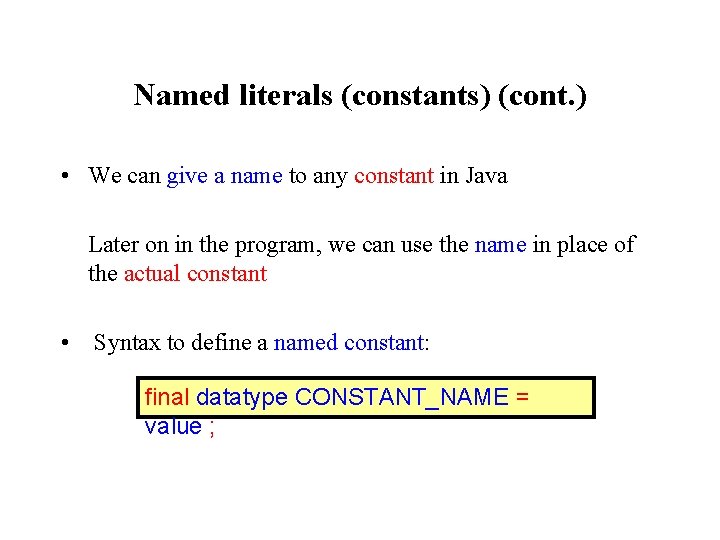
Named literals (constants) (cont. ) • We can give a name to any constant in Java Later on in the program, we can use the name in place of the actual constant • Syntax to define a named constant: final datatype CONSTANT_NAME = value ;
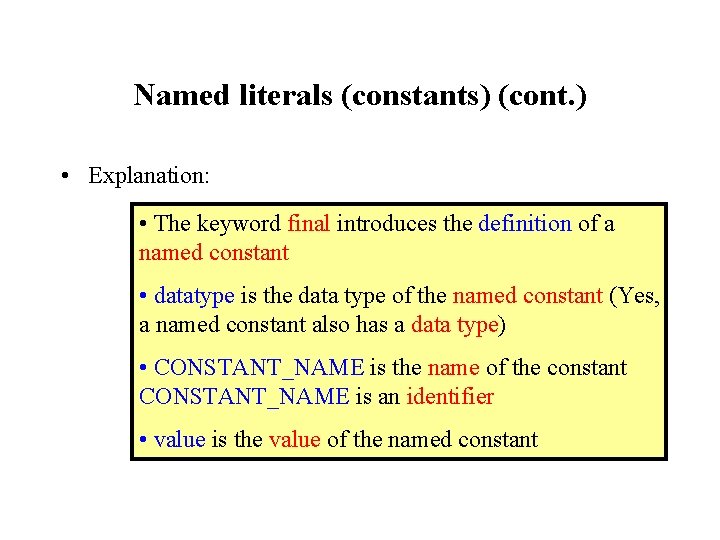
Named literals (constants) (cont. ) • Explanation: • The keyword final introduces the definition of a named constant • datatype is the data type of the named constant (Yes, a named constant also has a data type) • CONSTANT_NAME is the name of the constant CONSTANT_NAME is an identifier • value is the value of the named constant
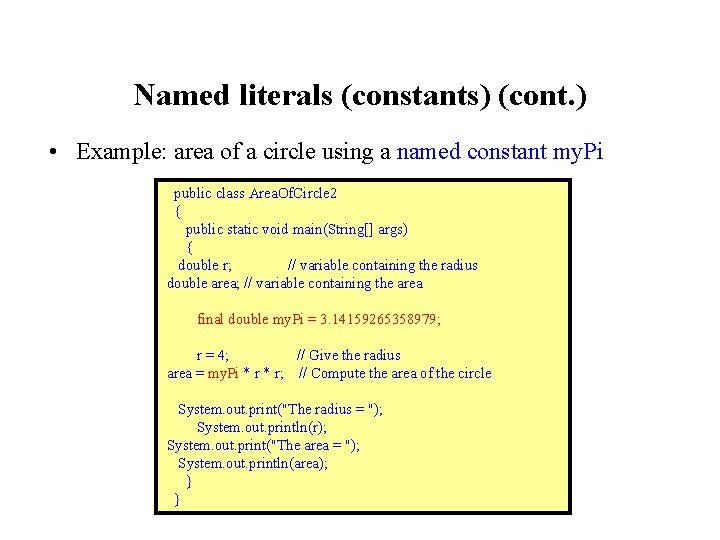
Named literals (constants) (cont. ) • Example: area of a circle using a named constant my. Pi public class Area. Of. Circle 2 { public static void main(String[] args) { double r; // variable containing the radius double area; // variable containing the area final double my. Pi = 3. 14159265358979; r = 4; // Give the radius area = my. Pi * r; // Compute the area of the circle System. out. print("The radius = "); System. out. println(r); System. out. print("The area = "); System. out. println(area); } }
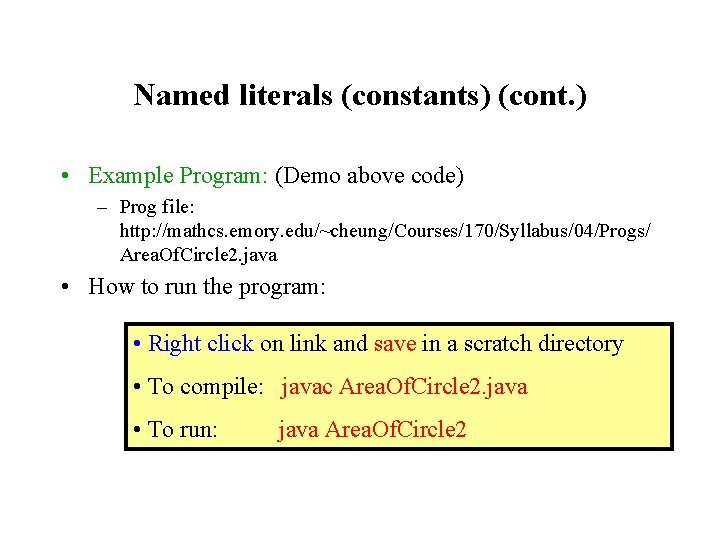
Named literals (constants) (cont. ) • Example Program: (Demo above code) – Prog file: http: //mathcs. emory. edu/~cheung/Courses/170/Syllabus/04/Progs/ Area. Of. Circle 2. java • How to run the program: • Right click on link and save in a scratch directory • To compile: javac Area. Of. Circle 2. java • To run: java Area. Of. Circle 2