1 Java Applications Consist of a class definition
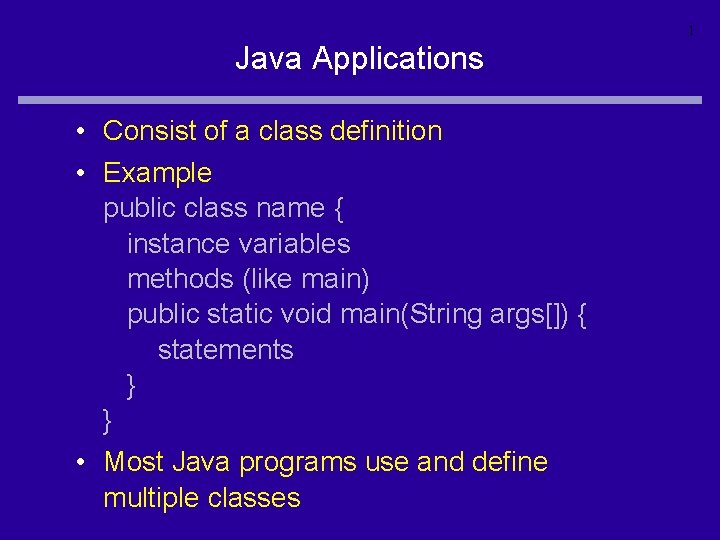
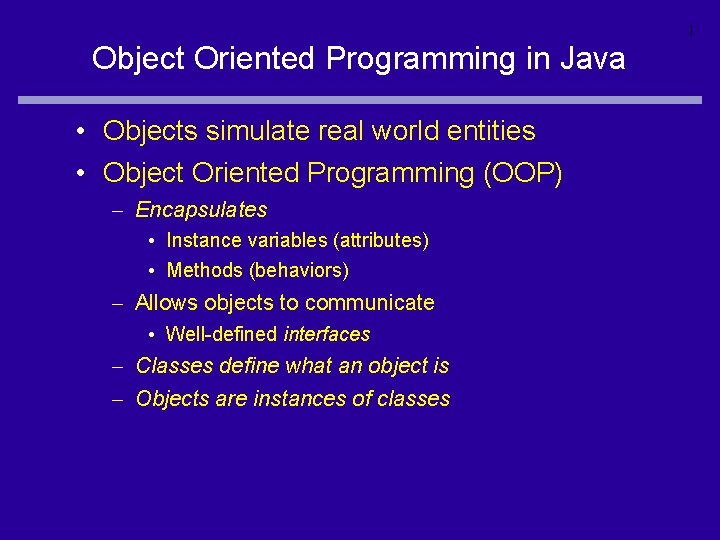
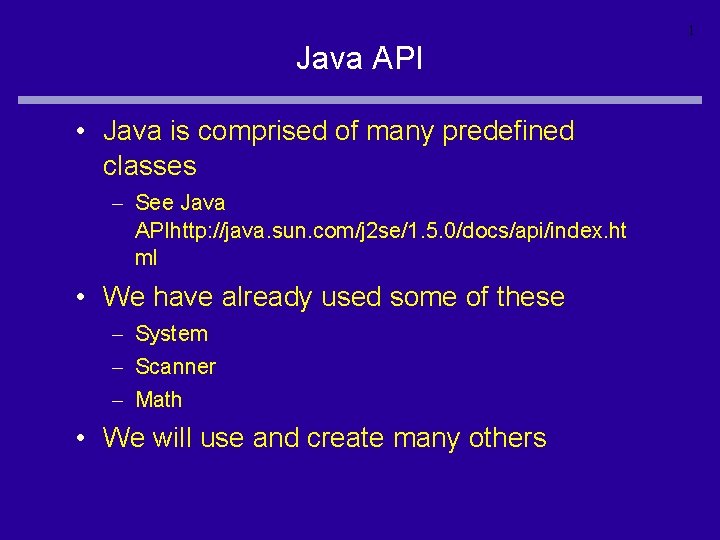
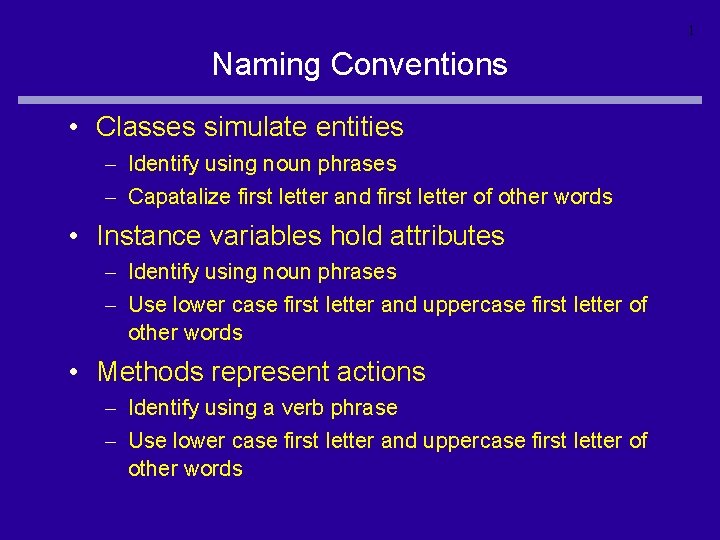
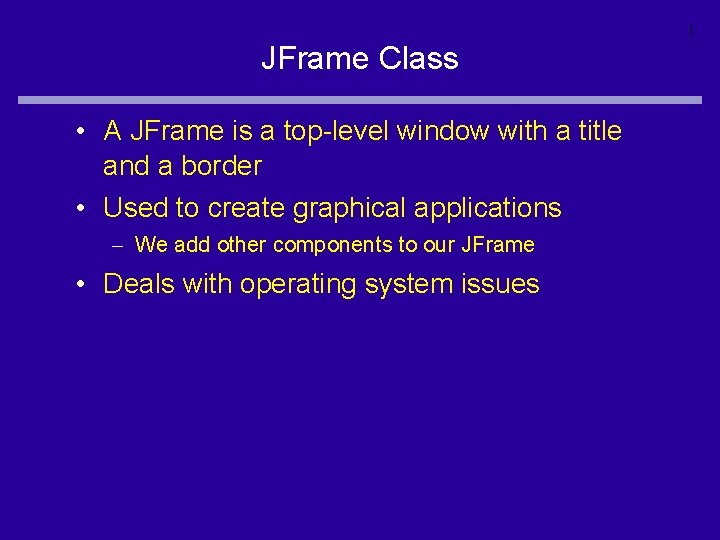
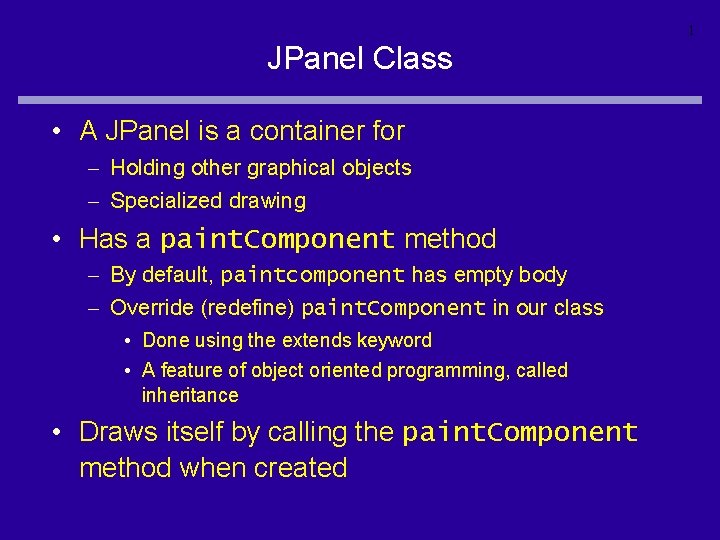
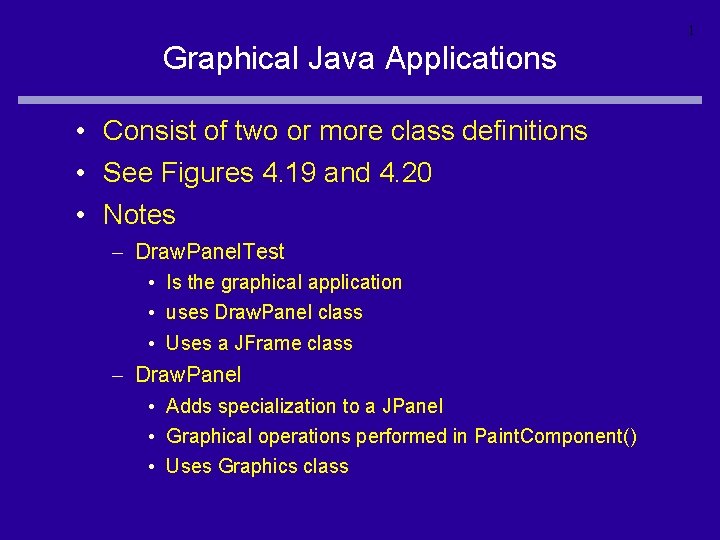
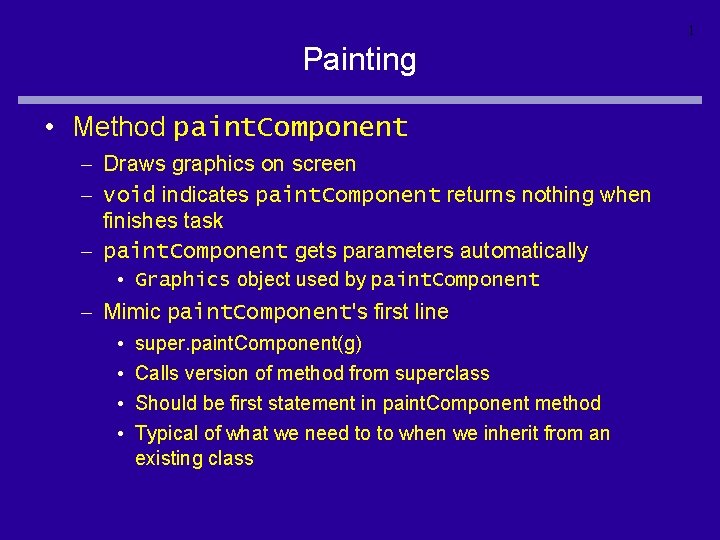
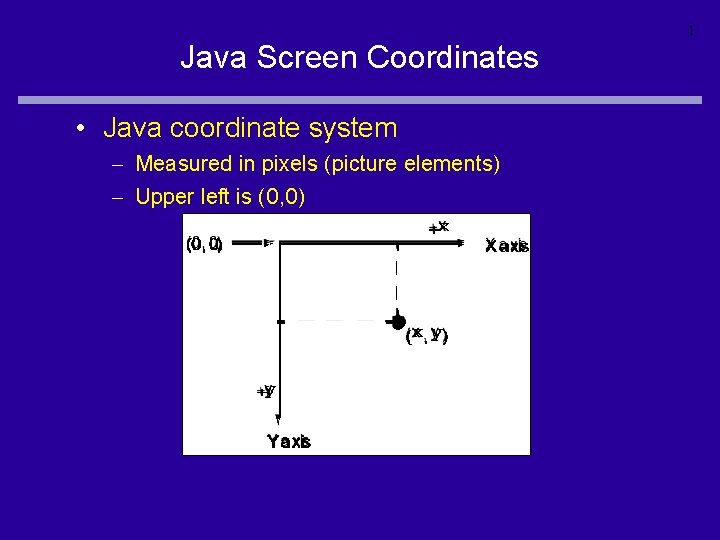
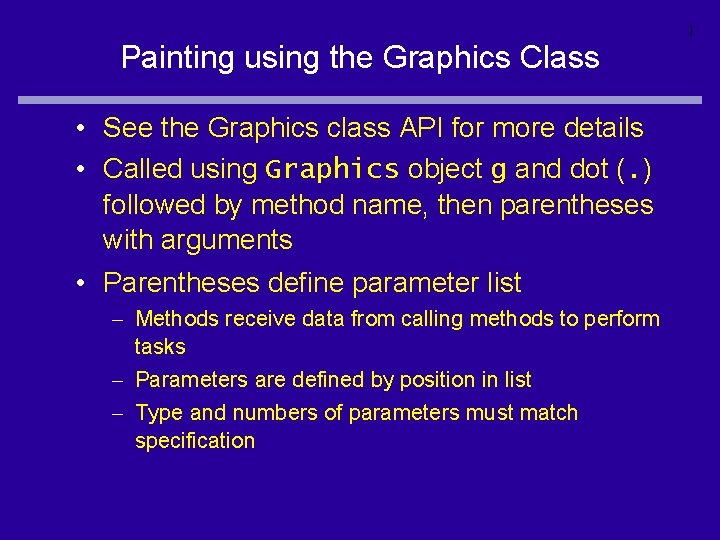
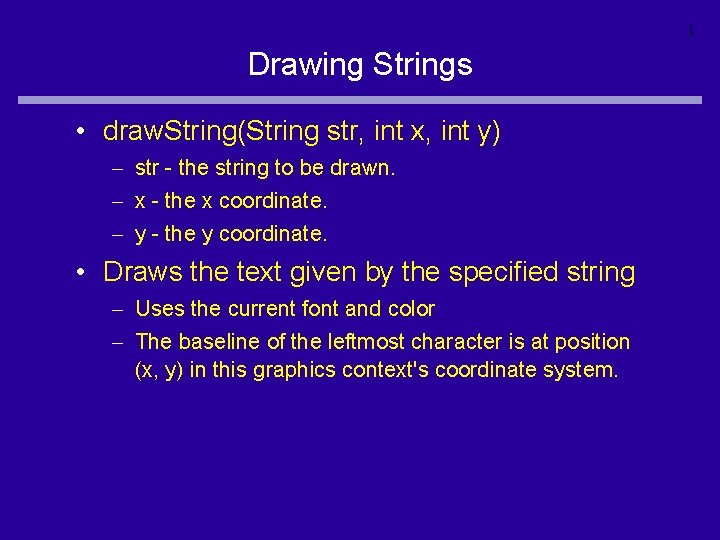
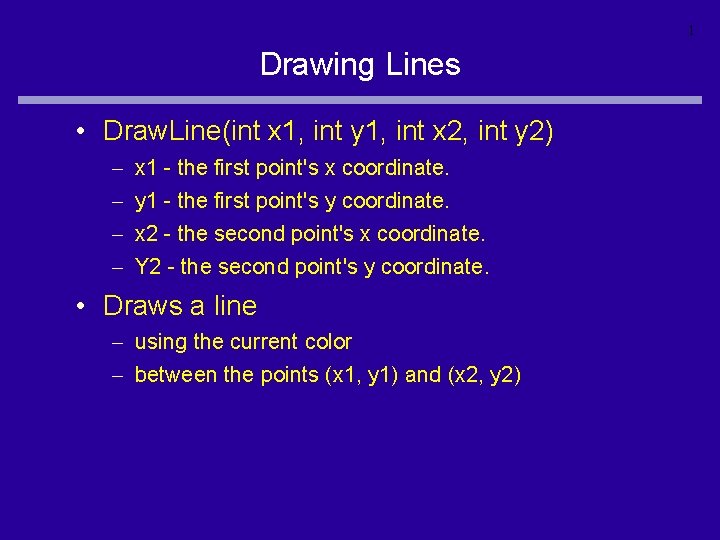
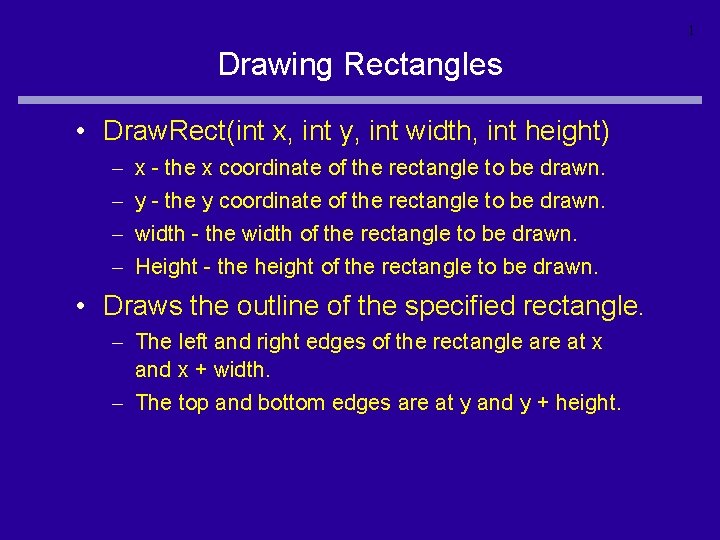
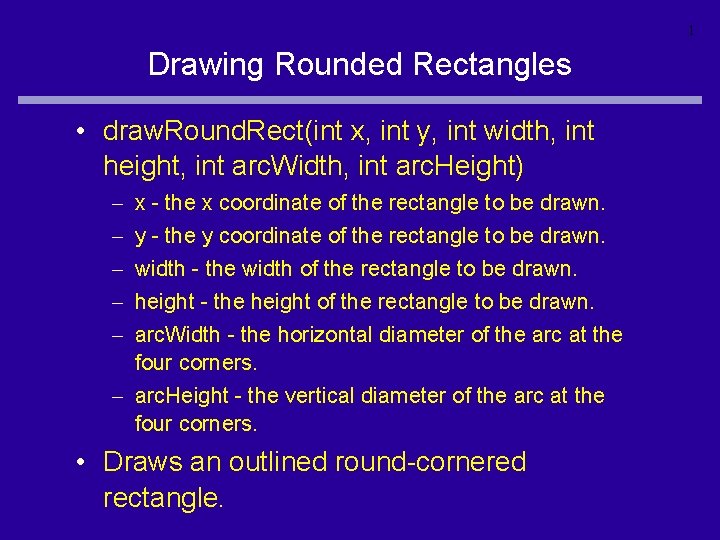
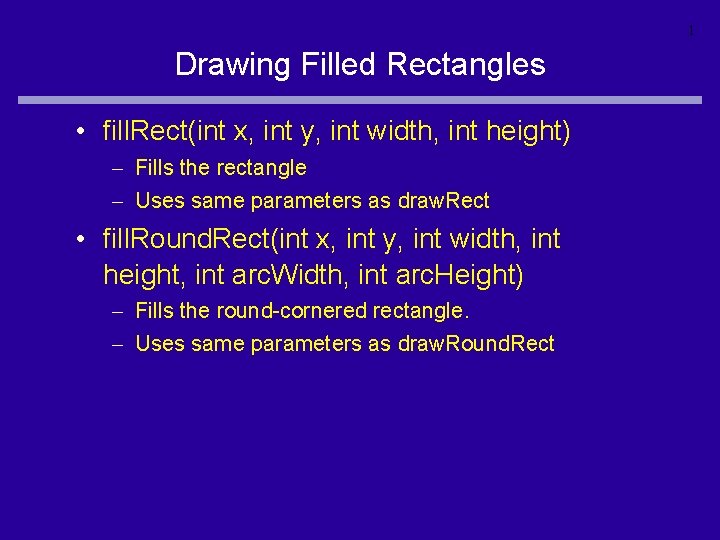
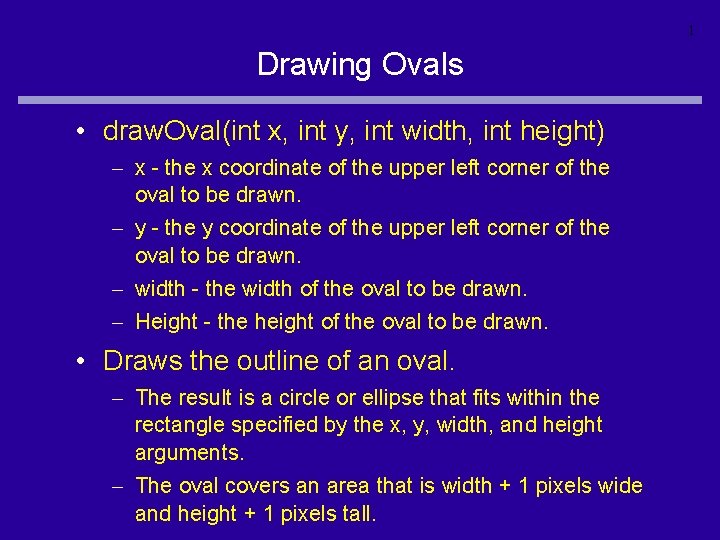
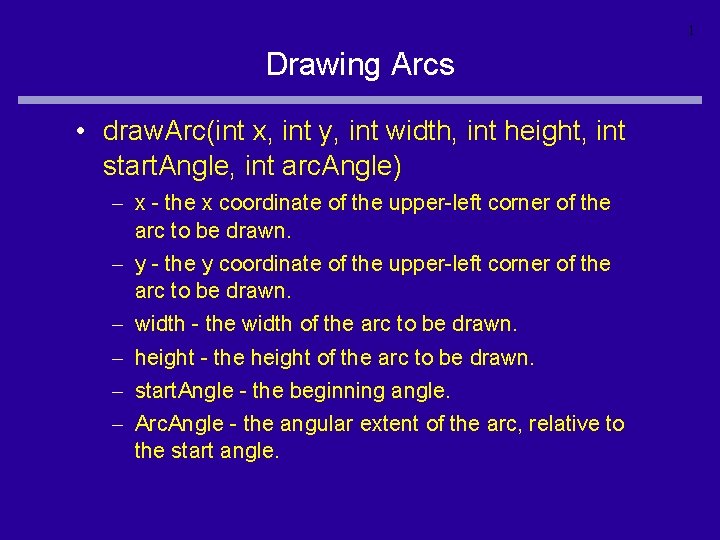
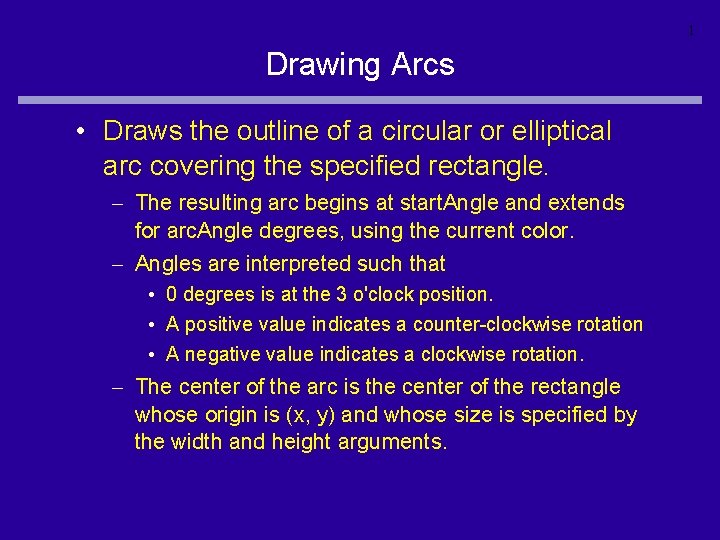
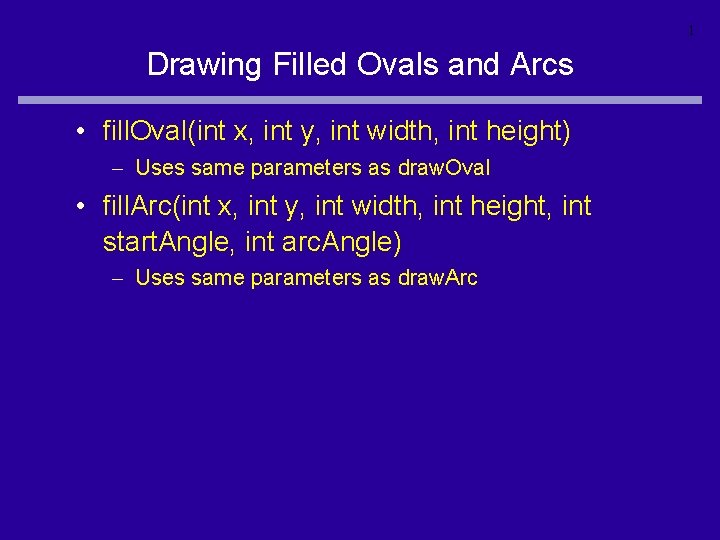
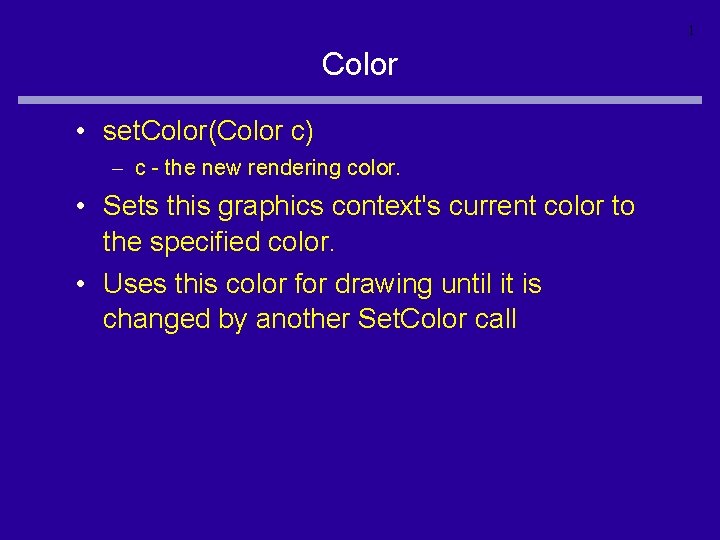
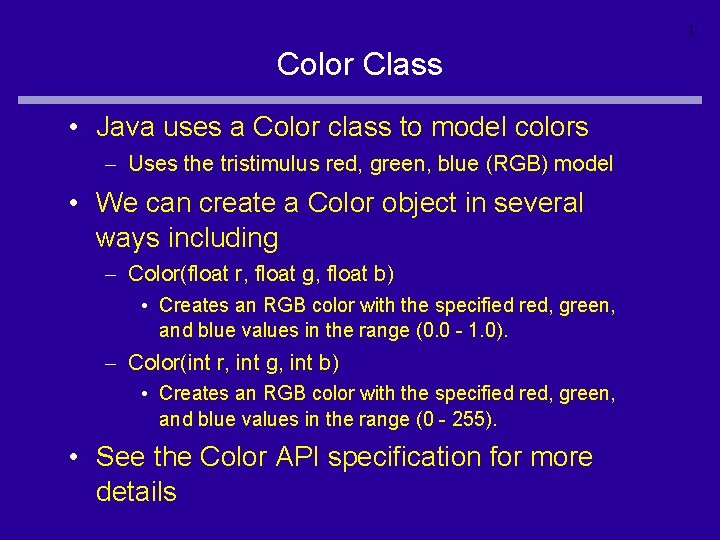
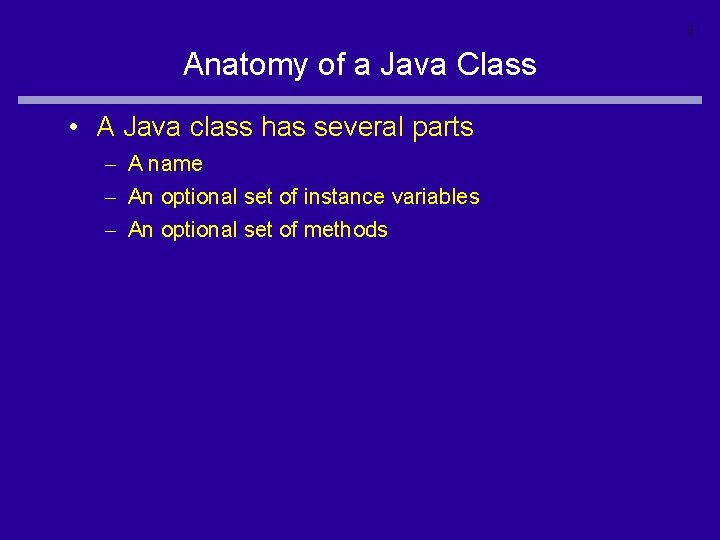
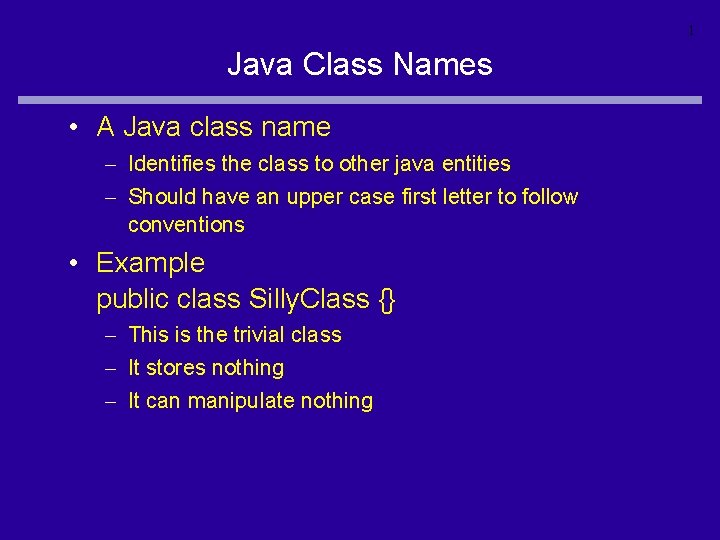
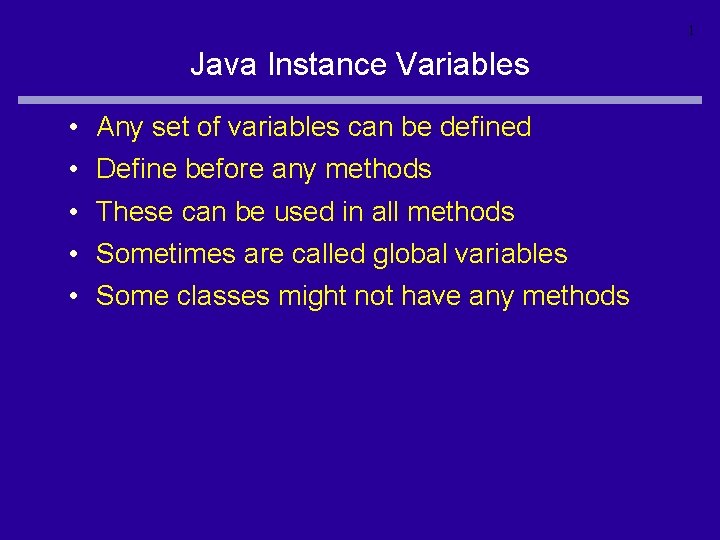
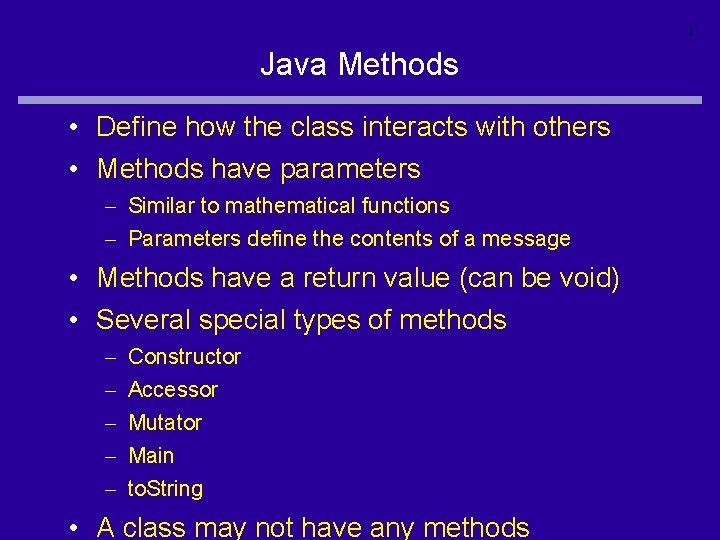
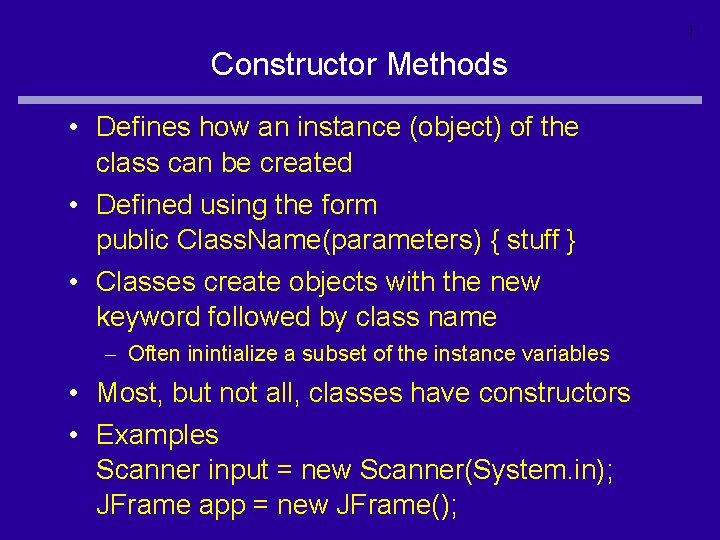
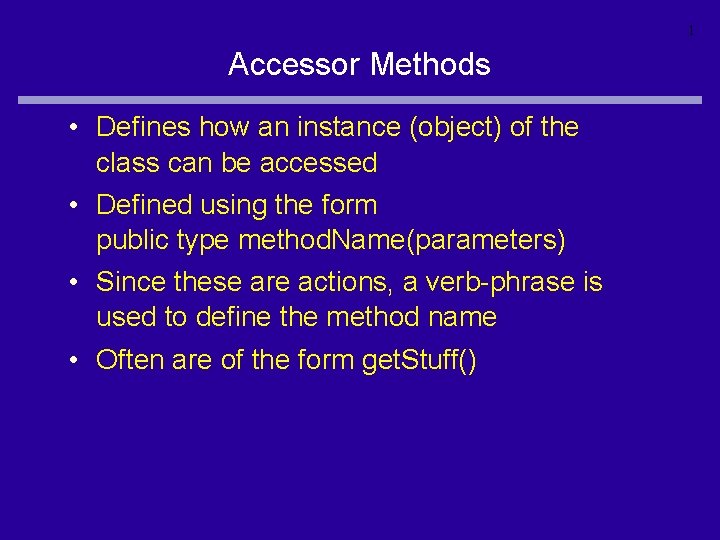
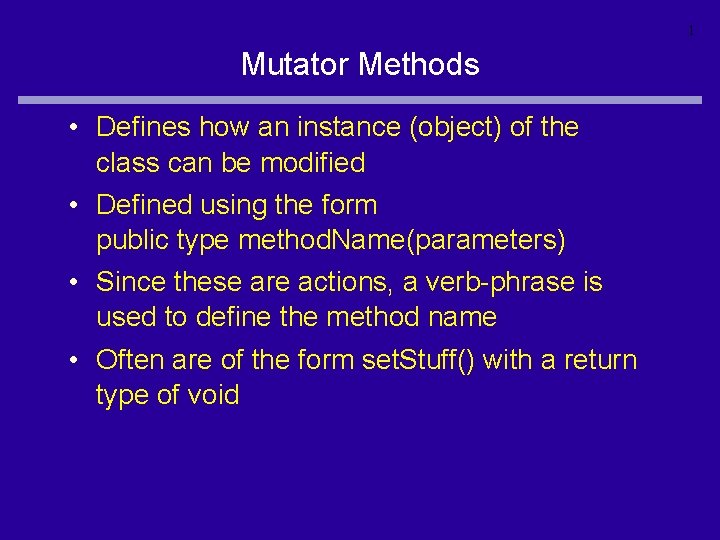
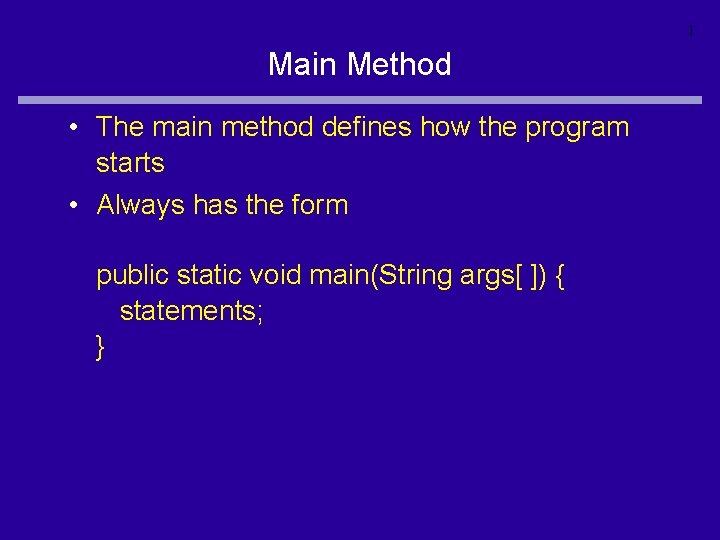
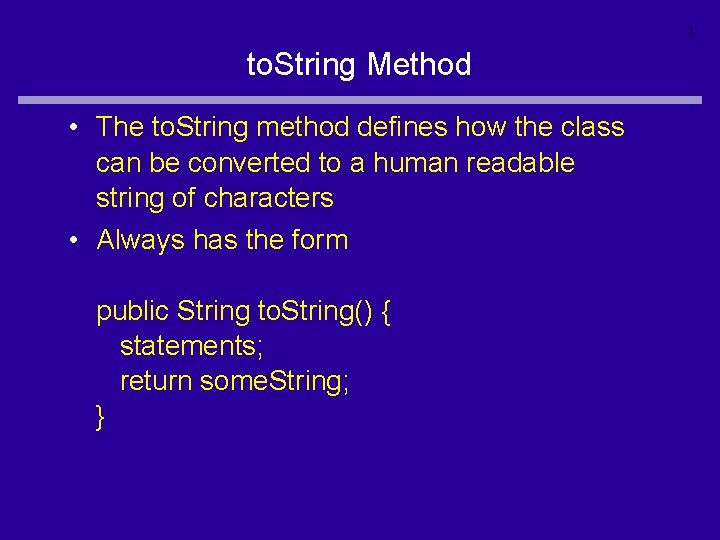
- Slides: 30
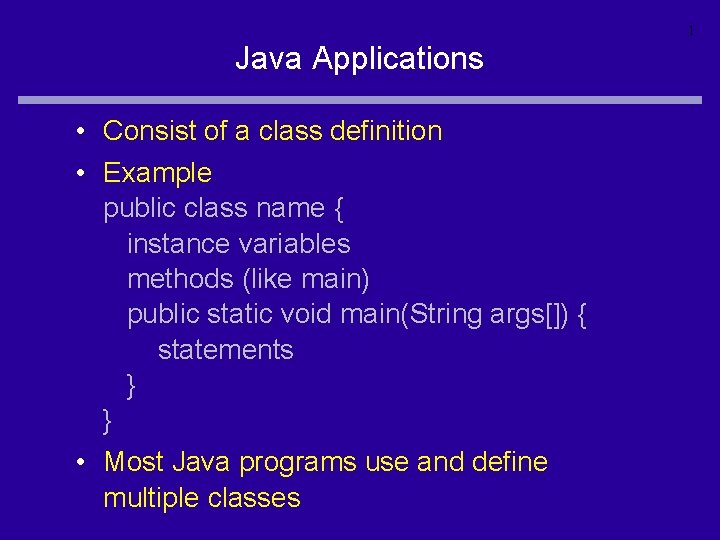
1 Java Applications • Consist of a class definition • Example public class name { instance variables methods (like main) public static void main(String args[]) { statements } } • Most Java programs use and define multiple classes
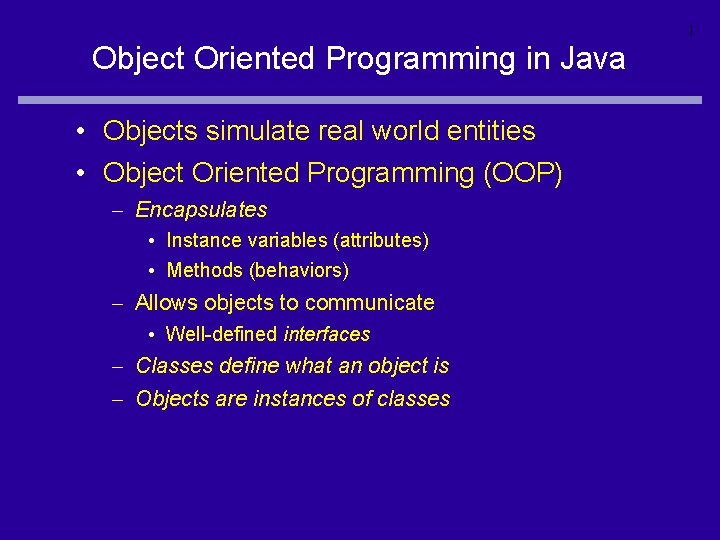
1 Object Oriented Programming in Java • Objects simulate real world entities • Object Oriented Programming (OOP) – Encapsulates • Instance variables (attributes) • Methods (behaviors) – Allows objects to communicate • Well-defined interfaces – Classes define what an object is – Objects are instances of classes
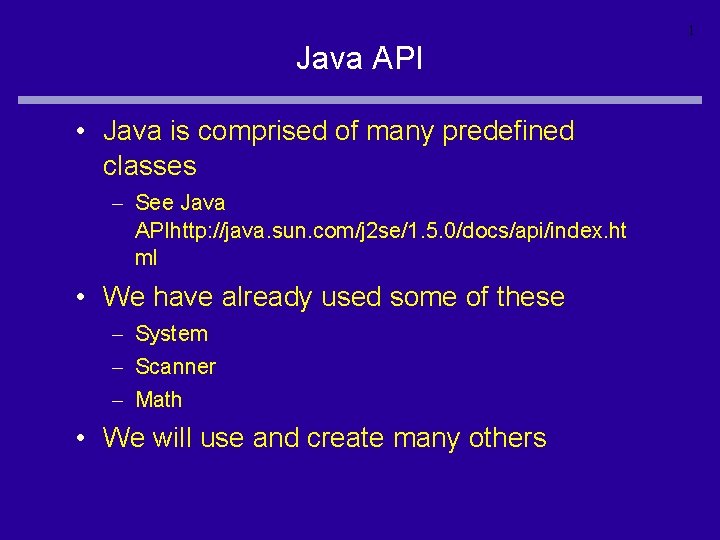
1 Java API • Java is comprised of many predefined classes – See Java APIhttp: //java. sun. com/j 2 se/1. 5. 0/docs/api/index. ht ml • We have already used some of these – System – Scanner – Math • We will use and create many others
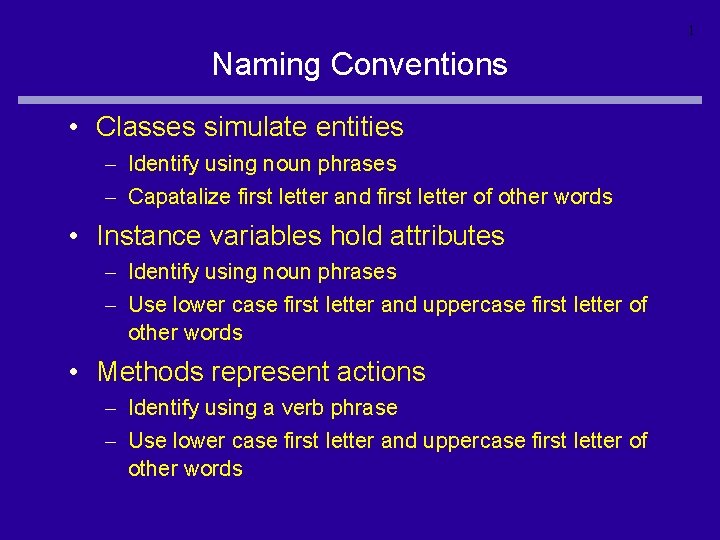
1 Naming Conventions • Classes simulate entities – Identify using noun phrases – Capatalize first letter and first letter of other words • Instance variables hold attributes – Identify using noun phrases – Use lower case first letter and uppercase first letter of other words • Methods represent actions – Identify using a verb phrase – Use lower case first letter and uppercase first letter of other words
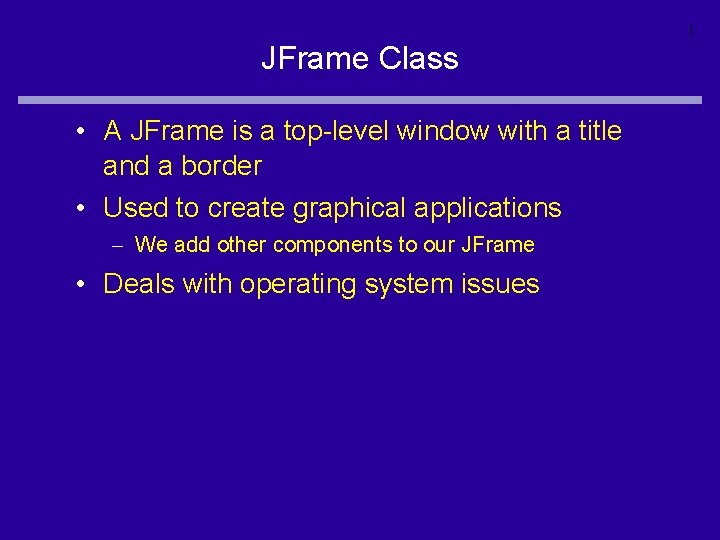
1 JFrame Class • A JFrame is a top-level window with a title and a border • Used to create graphical applications – We add other components to our JFrame • Deals with operating system issues
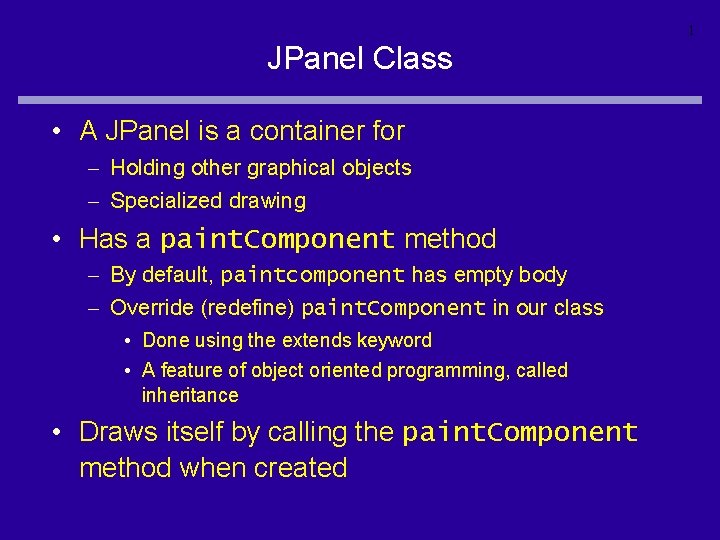
1 JPanel Class • A JPanel is a container for – Holding other graphical objects – Specialized drawing • Has a paint. Component method – By default, paintcomponent has empty body – Override (redefine) paint. Component in our class • Done using the extends keyword • A feature of object oriented programming, called inheritance • Draws itself by calling the paint. Component method when created
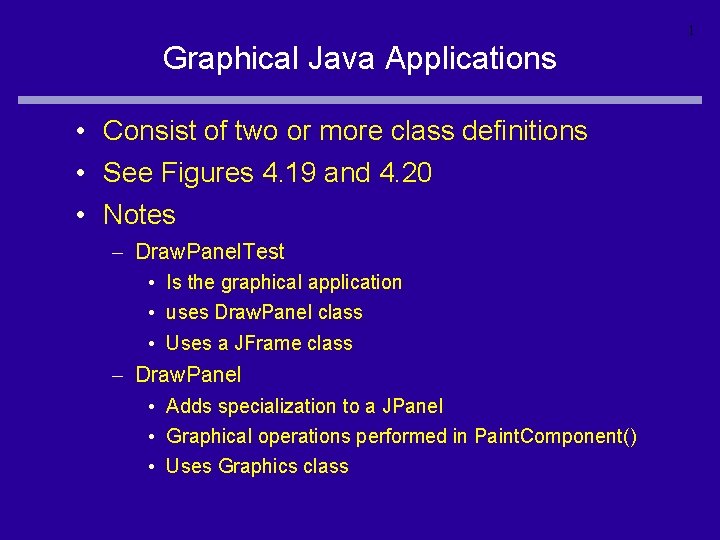
1 Graphical Java Applications • Consist of two or more class definitions • See Figures 4. 19 and 4. 20 • Notes – Draw. Panel. Test • Is the graphical application • uses Draw. Panel class • Uses a JFrame class – Draw. Panel • Adds specialization to a JPanel • Graphical operations performed in Paint. Component() • Uses Graphics class
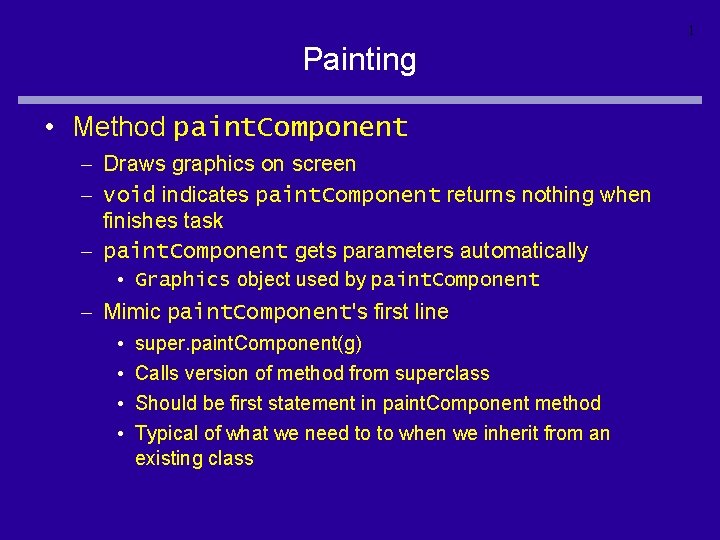
1 Painting • Method paint. Component – Draws graphics on screen – void indicates paint. Component returns nothing when finishes task – paint. Component gets parameters automatically • Graphics object used by paint. Component – Mimic paint. Component's first line • • super. paint. Component(g) Calls version of method from superclass Should be first statement in paint. Component method Typical of what we need to to when we inherit from an existing class
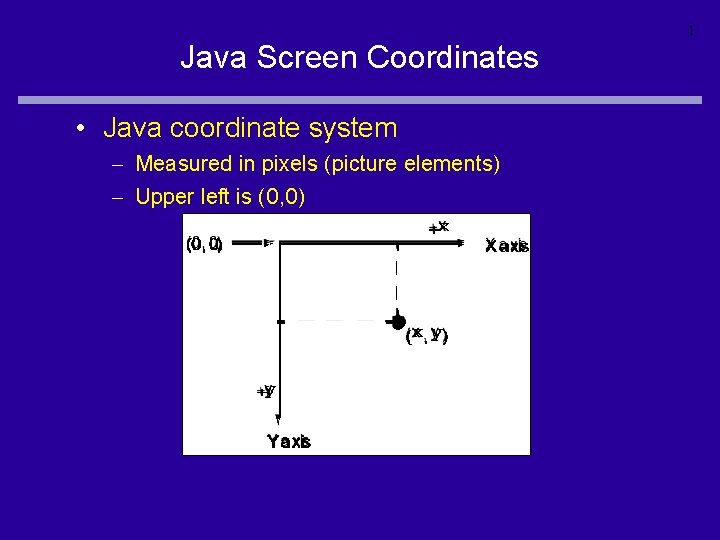
1 Java Screen Coordinates • Java coordinate system – Measured in pixels (picture elements) – Upper left is (0, 0)
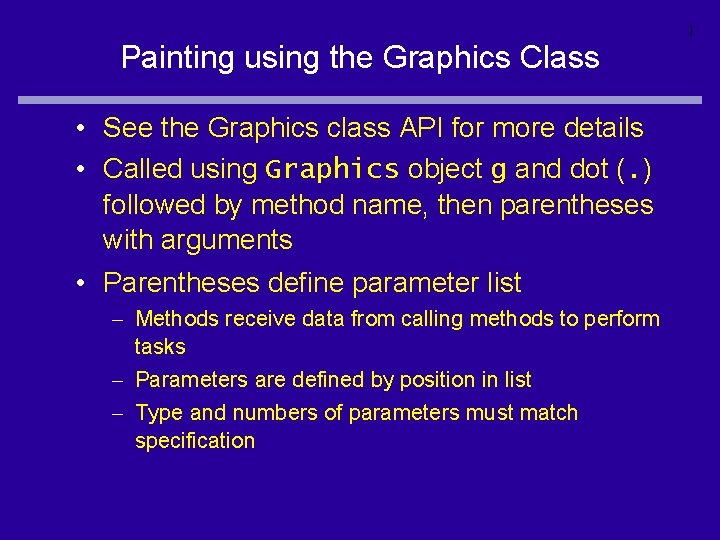
1 Painting using the Graphics Class • See the Graphics class API for more details • Called using Graphics object g and dot (. ) followed by method name, then parentheses with arguments • Parentheses define parameter list – Methods receive data from calling methods to perform tasks – Parameters are defined by position in list – Type and numbers of parameters must match specification
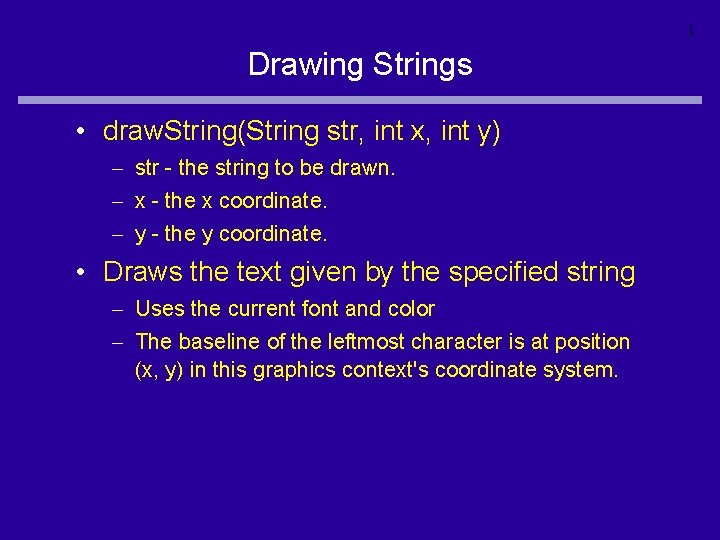
1 Drawing Strings • draw. String(String str, int x, int y) – str - the string to be drawn. – x - the x coordinate. – y - the y coordinate. • Draws the text given by the specified string – Uses the current font and color – The baseline of the leftmost character is at position (x, y) in this graphics context's coordinate system.
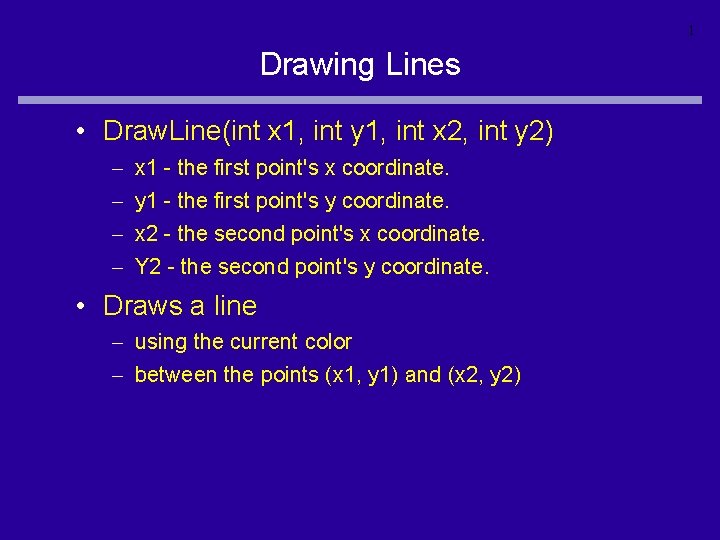
1 Drawing Lines • Draw. Line(int x 1, int y 1, int x 2, int y 2) – – x 1 - the first point's x coordinate. y 1 - the first point's y coordinate. x 2 - the second point's x coordinate. Y 2 - the second point's y coordinate. • Draws a line – using the current color – between the points (x 1, y 1) and (x 2, y 2)
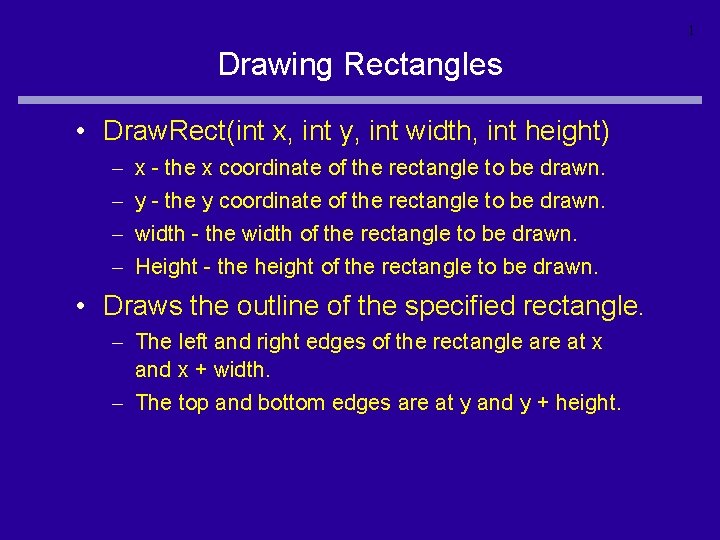
1 Drawing Rectangles • Draw. Rect(int x, int y, int width, int height) – – x - the x coordinate of the rectangle to be drawn. y - the y coordinate of the rectangle to be drawn. width - the width of the rectangle to be drawn. Height - the height of the rectangle to be drawn. • Draws the outline of the specified rectangle. – The left and right edges of the rectangle are at x and x + width. – The top and bottom edges are at y and y + height.
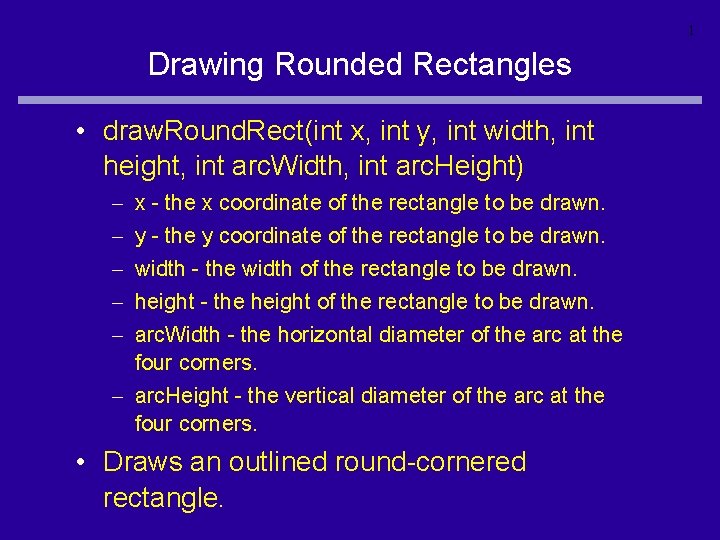
1 Drawing Rounded Rectangles • draw. Round. Rect(int x, int y, int width, int height, int arc. Width, int arc. Height) – – – x - the x coordinate of the rectangle to be drawn. y - the y coordinate of the rectangle to be drawn. width - the width of the rectangle to be drawn. height - the height of the rectangle to be drawn. arc. Width - the horizontal diameter of the arc at the four corners. – arc. Height - the vertical diameter of the arc at the four corners. • Draws an outlined round-cornered rectangle.
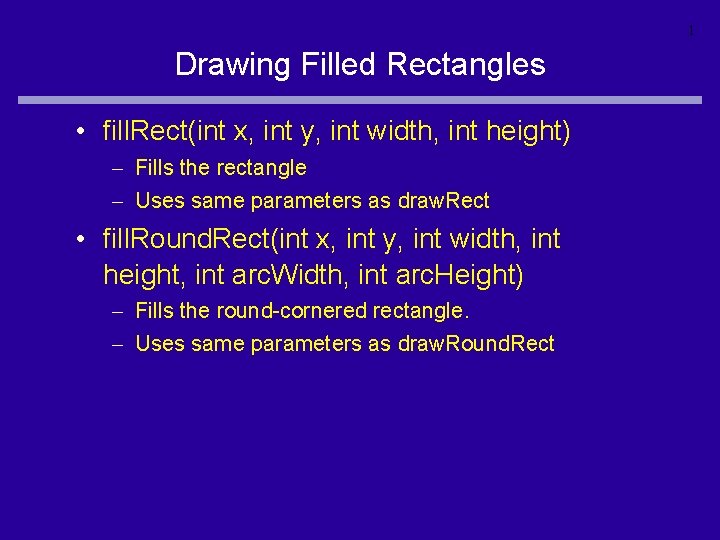
1 Drawing Filled Rectangles • fill. Rect(int x, int y, int width, int height) – Fills the rectangle – Uses same parameters as draw. Rect • fill. Round. Rect(int x, int y, int width, int height, int arc. Width, int arc. Height) – Fills the round-cornered rectangle. – Uses same parameters as draw. Round. Rect
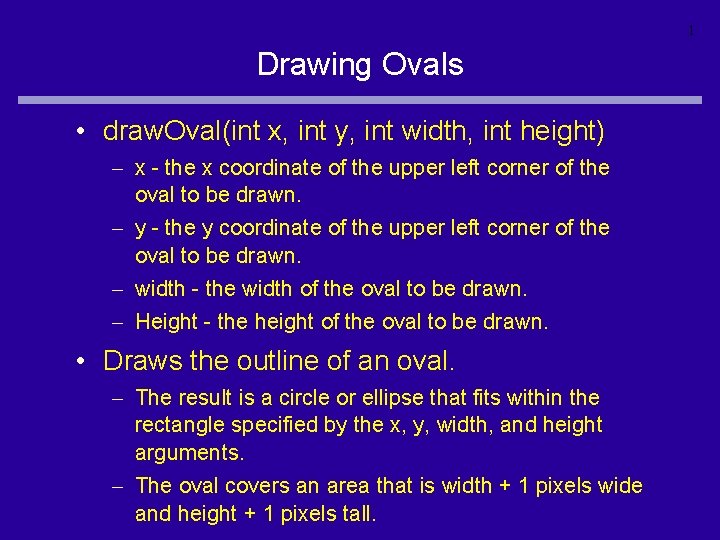
1 Drawing Ovals • draw. Oval(int x, int y, int width, int height) – x - the x coordinate of the upper left corner of the oval to be drawn. – y - the y coordinate of the upper left corner of the oval to be drawn. – width - the width of the oval to be drawn. – Height - the height of the oval to be drawn. • Draws the outline of an oval. – The result is a circle or ellipse that fits within the rectangle specified by the x, y, width, and height arguments. – The oval covers an area that is width + 1 pixels wide and height + 1 pixels tall.
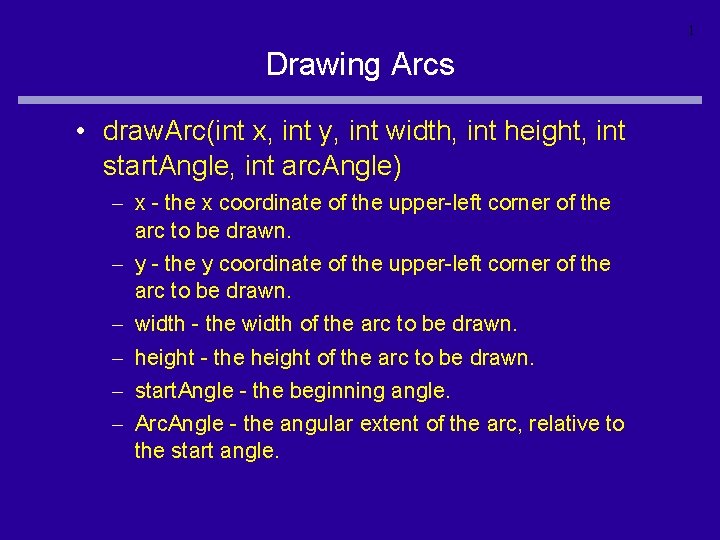
1 Drawing Arcs • draw. Arc(int x, int y, int width, int height, int start. Angle, int arc. Angle) – x - the x coordinate of the upper-left corner of the arc to be drawn. – y - the y coordinate of the upper-left corner of the arc to be drawn. – width - the width of the arc to be drawn. – height - the height of the arc to be drawn. – start. Angle - the beginning angle. – Arc. Angle - the angular extent of the arc, relative to the start angle.
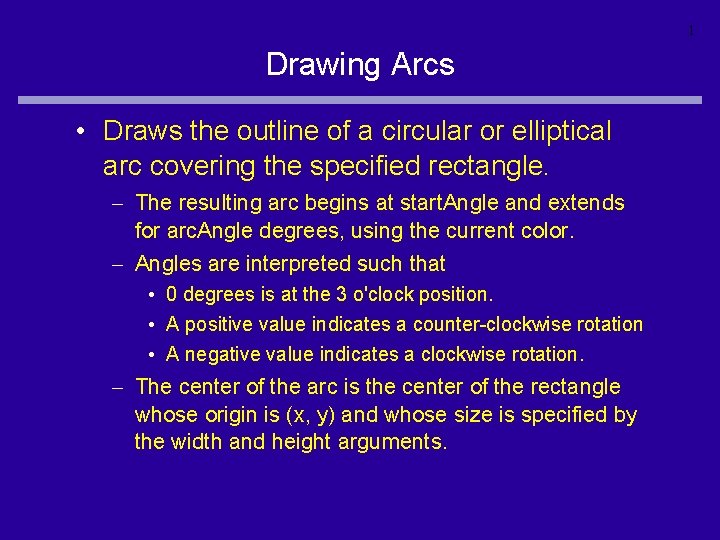
1 Drawing Arcs • Draws the outline of a circular or elliptical arc covering the specified rectangle. – The resulting arc begins at start. Angle and extends for arc. Angle degrees, using the current color. – Angles are interpreted such that • 0 degrees is at the 3 o'clock position. • A positive value indicates a counter-clockwise rotation • A negative value indicates a clockwise rotation. – The center of the arc is the center of the rectangle whose origin is (x, y) and whose size is specified by the width and height arguments.
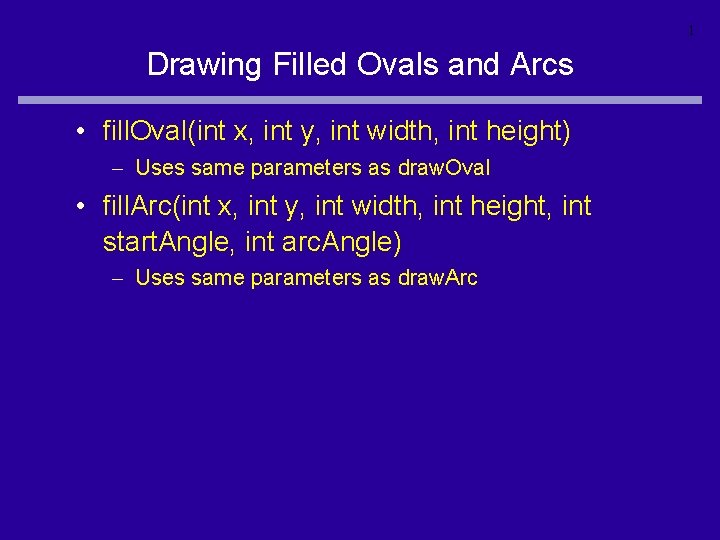
1 Drawing Filled Ovals and Arcs • fill. Oval(int x, int y, int width, int height) – Uses same parameters as draw. Oval • fill. Arc(int x, int y, int width, int height, int start. Angle, int arc. Angle) – Uses same parameters as draw. Arc
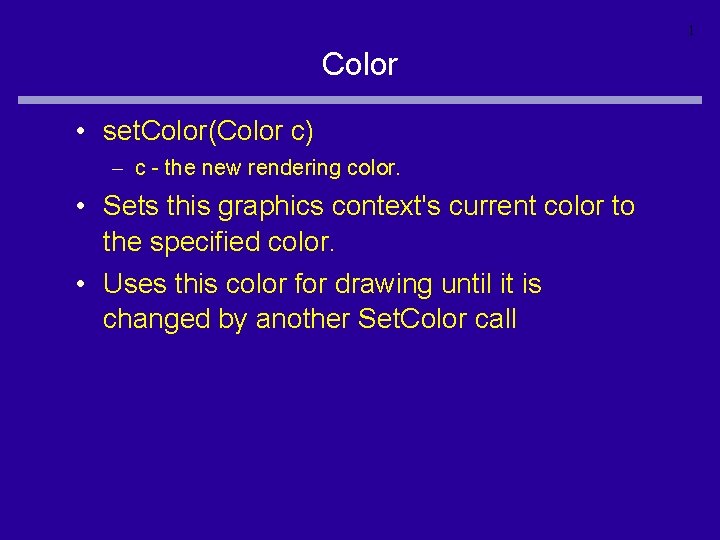
1 Color • set. Color(Color c) – c - the new rendering color. • Sets this graphics context's current color to the specified color. • Uses this color for drawing until it is changed by another Set. Color call
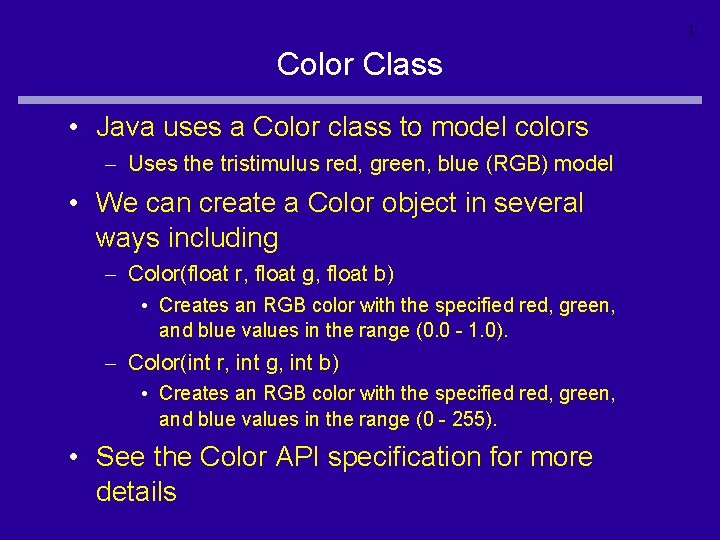
1 Color Class • Java uses a Color class to model colors – Uses the tristimulus red, green, blue (RGB) model • We can create a Color object in several ways including – Color(float r, float g, float b) • Creates an RGB color with the specified red, green, and blue values in the range (0. 0 - 1. 0). – Color(int r, int g, int b) • Creates an RGB color with the specified red, green, and blue values in the range (0 - 255). • See the Color API specification for more details
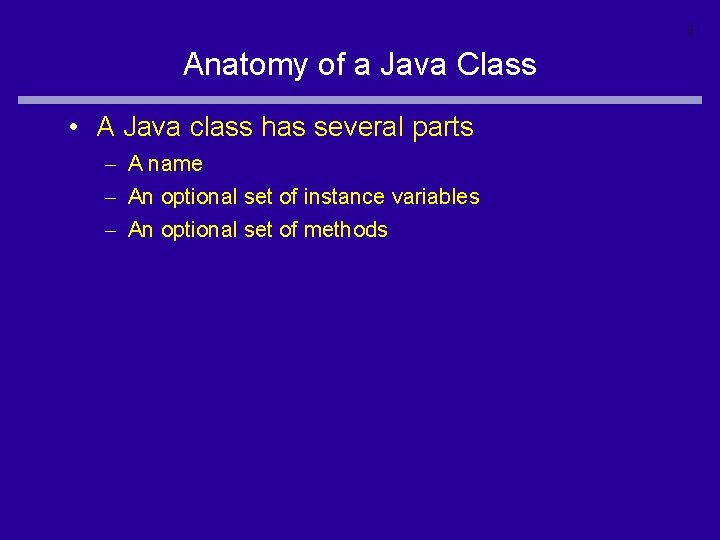
1 Anatomy of a Java Class • A Java class has several parts – A name – An optional set of instance variables – An optional set of methods
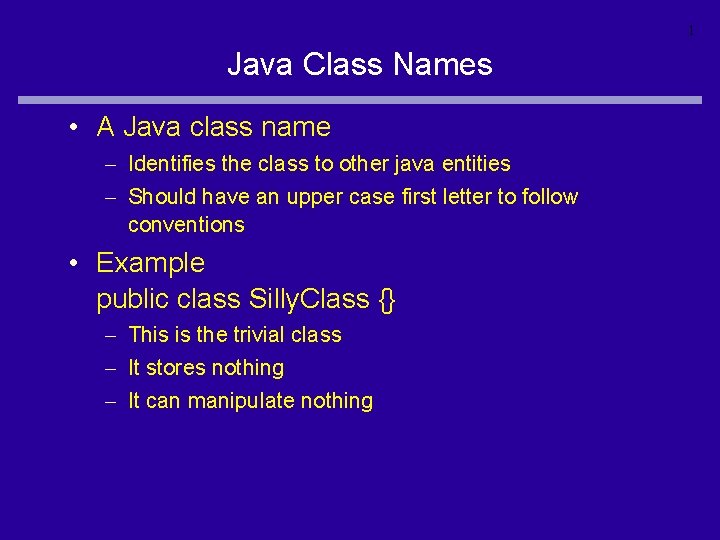
1 Java Class Names • A Java class name – Identifies the class to other java entities – Should have an upper case first letter to follow conventions • Example public class Silly. Class {} – This is the trivial class – It stores nothing – It can manipulate nothing
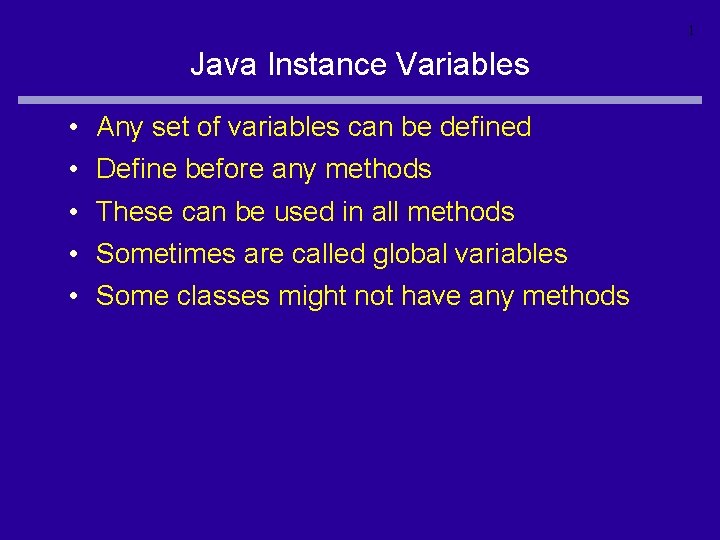
1 Java Instance Variables • • • Any set of variables can be defined Define before any methods These can be used in all methods Sometimes are called global variables Some classes might not have any methods
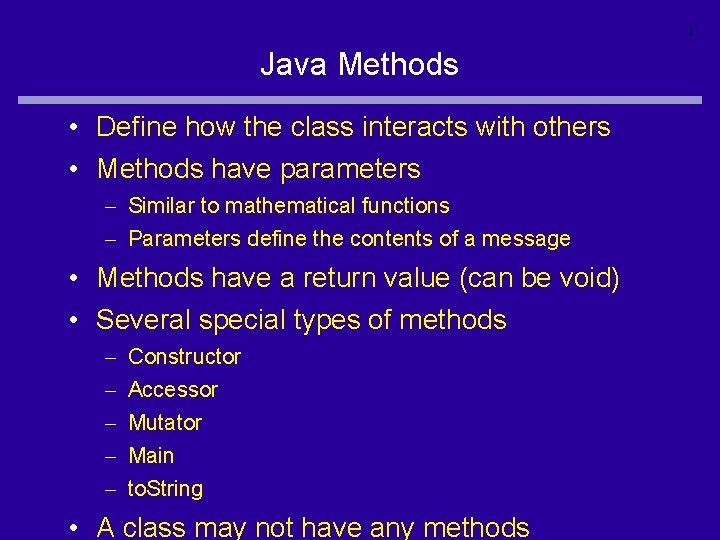
1 Java Methods • Define how the class interacts with others • Methods have parameters – Similar to mathematical functions – Parameters define the contents of a message • Methods have a return value (can be void) • Several special types of methods – – – Constructor Accessor Mutator Main to. String • A class may not have any methods
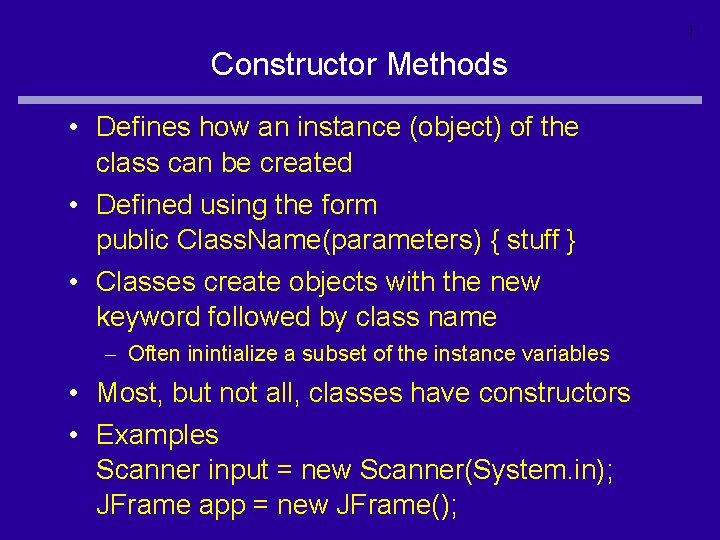
1 Constructor Methods • Defines how an instance (object) of the class can be created • Defined using the form public Class. Name(parameters) { stuff } • Classes create objects with the new keyword followed by class name – Often inintialize a subset of the instance variables • Most, but not all, classes have constructors • Examples Scanner input = new Scanner(System. in); JFrame app = new JFrame();
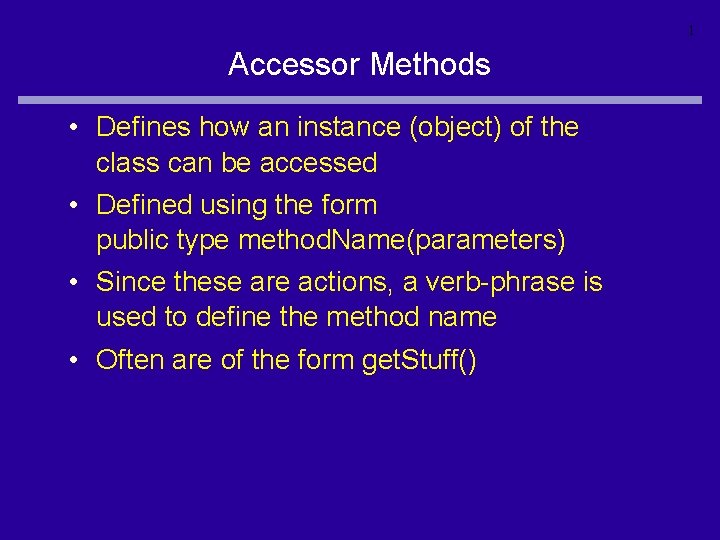
1 Accessor Methods • Defines how an instance (object) of the class can be accessed • Defined using the form public type method. Name(parameters) • Since these are actions, a verb-phrase is used to define the method name • Often are of the form get. Stuff()
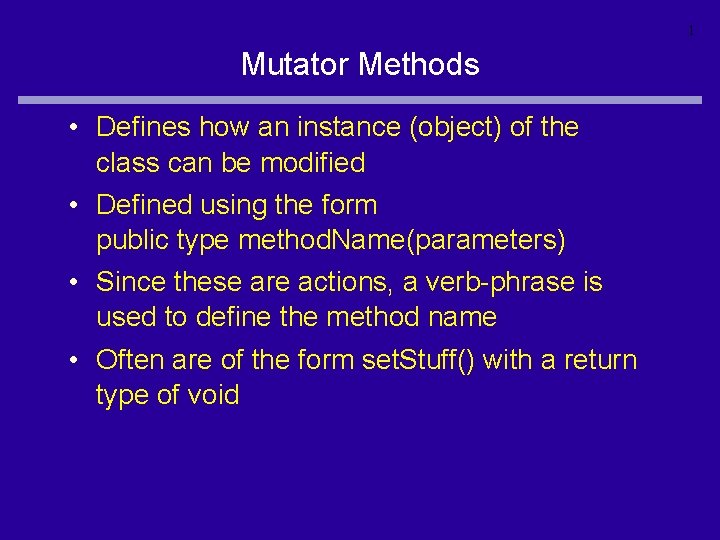
1 Mutator Methods • Defines how an instance (object) of the class can be modified • Defined using the form public type method. Name(parameters) • Since these are actions, a verb-phrase is used to define the method name • Often are of the form set. Stuff() with a return type of void
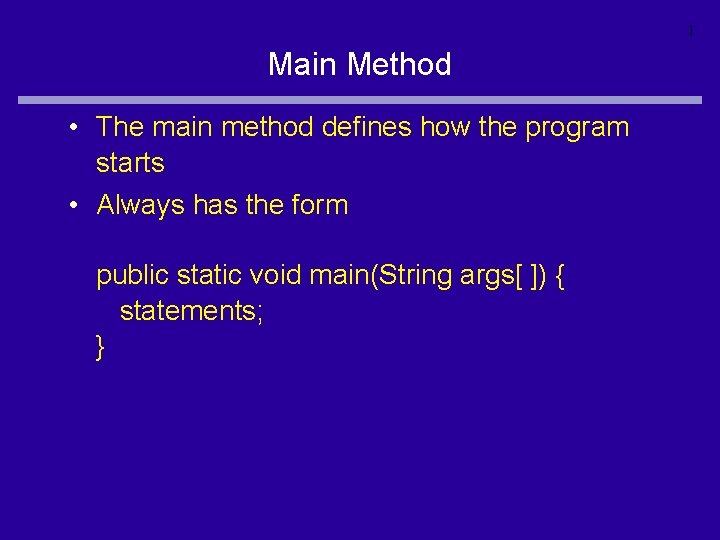
1 Main Method • The main method defines how the program starts • Always has the form public static void main(String args[ ]) { statements; }
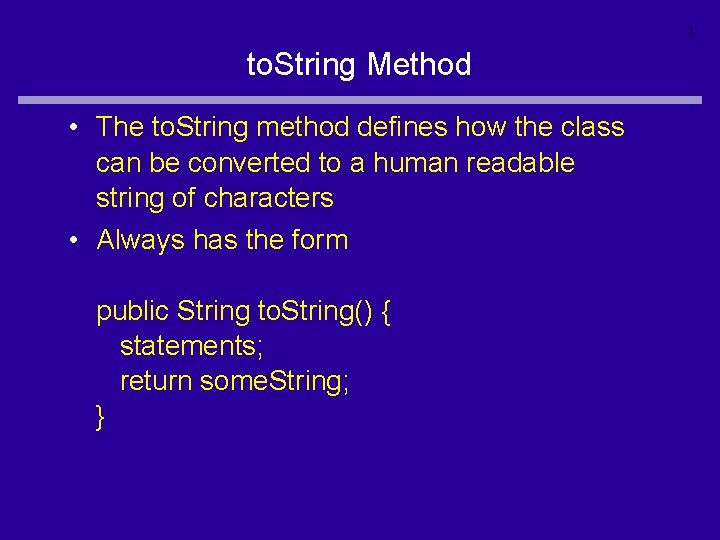
1 to. String Method • The to. String method defines how the class can be converted to a human readable string of characters • Always has the form public String to. String() { statements; return some. String; }