CS 225 Java Review Java Applications A java
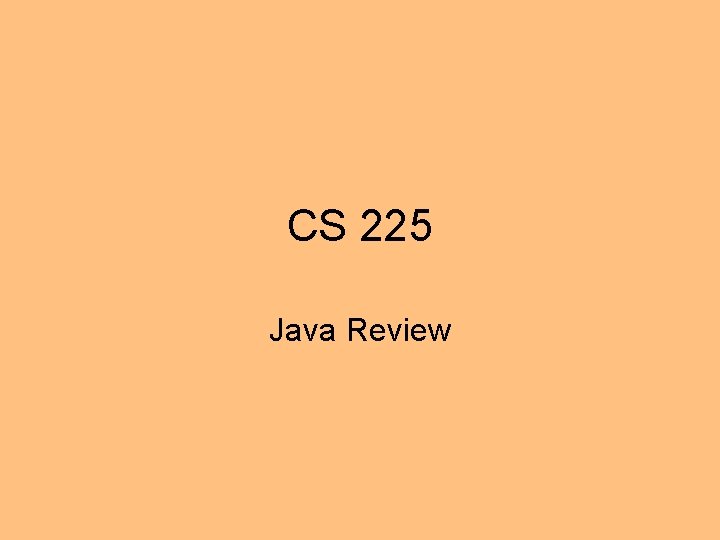
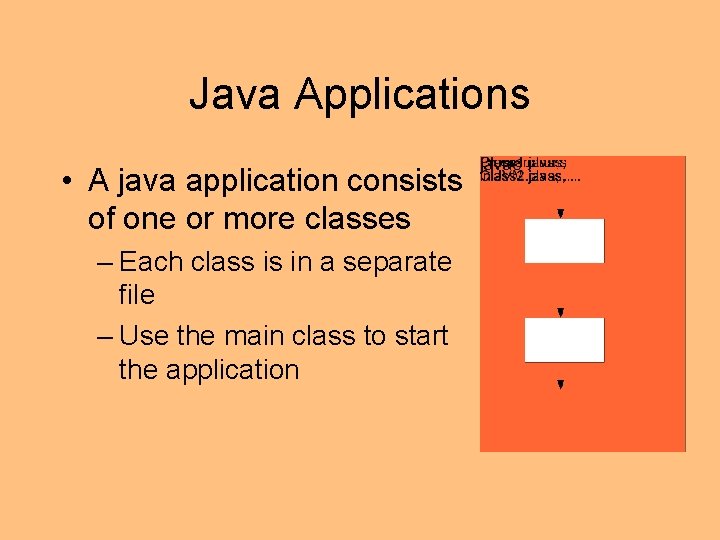
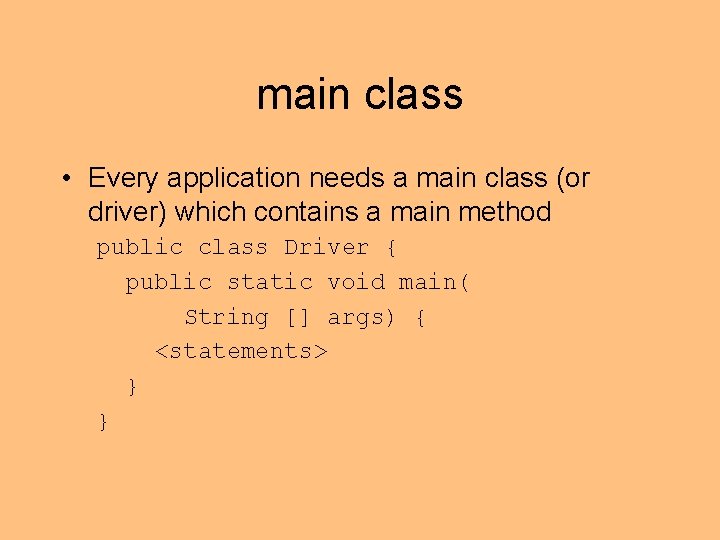
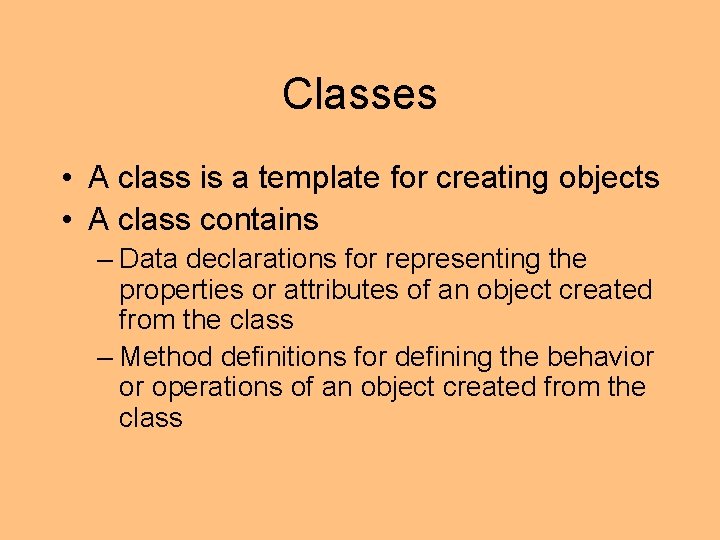
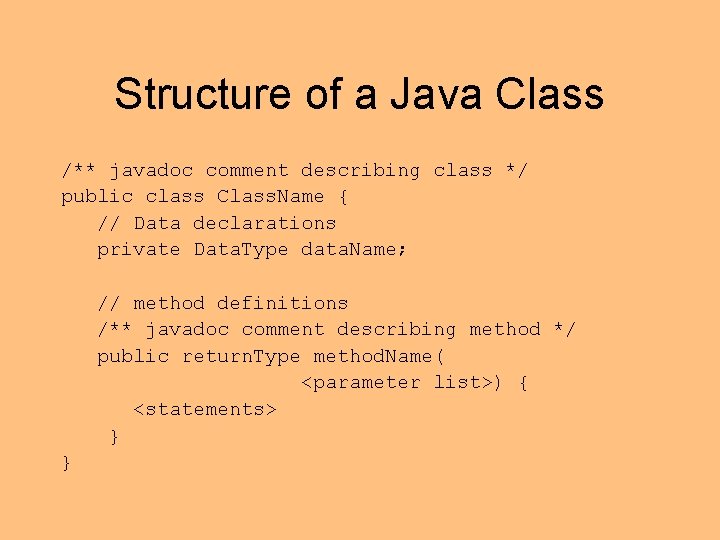
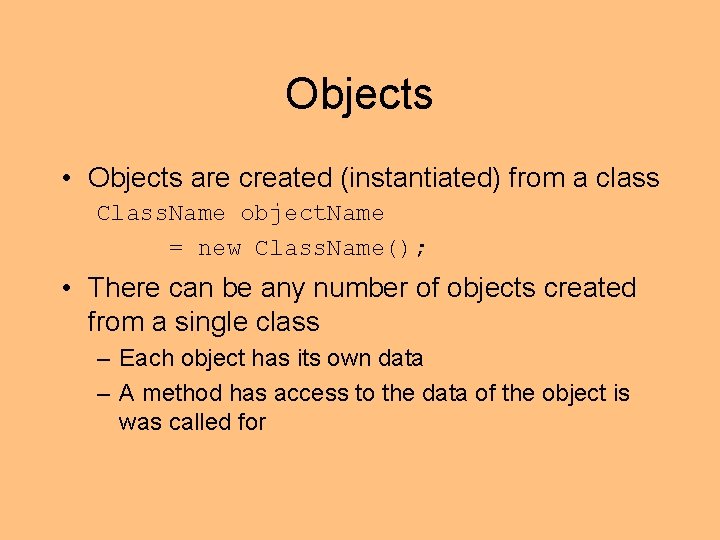
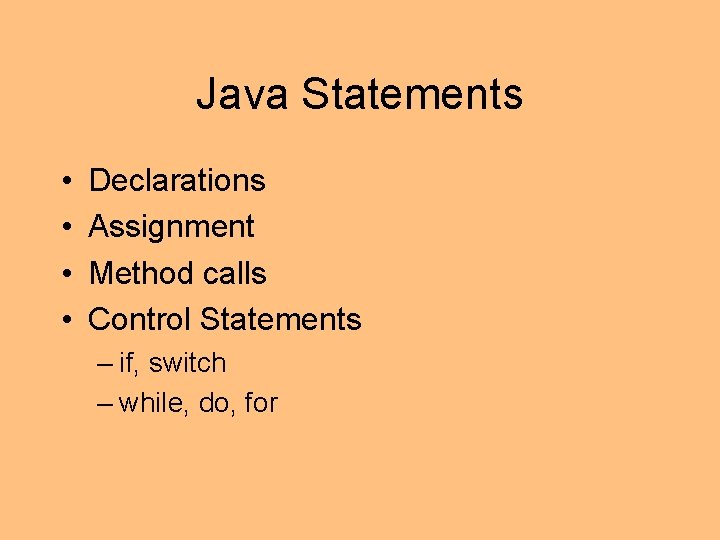
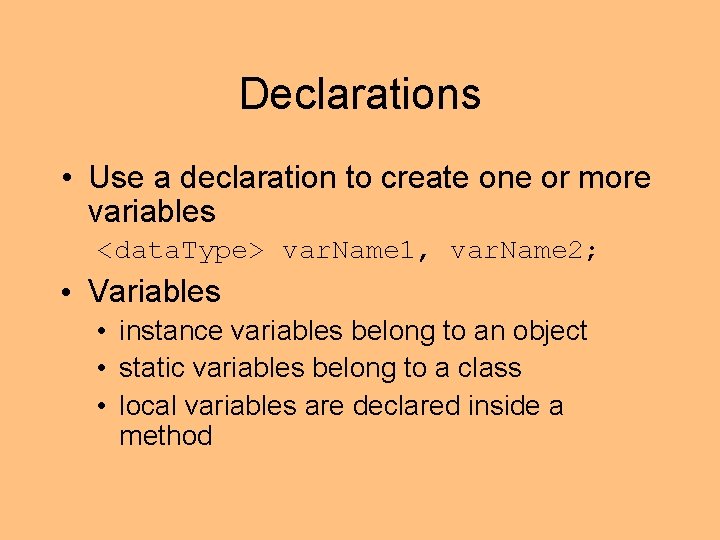
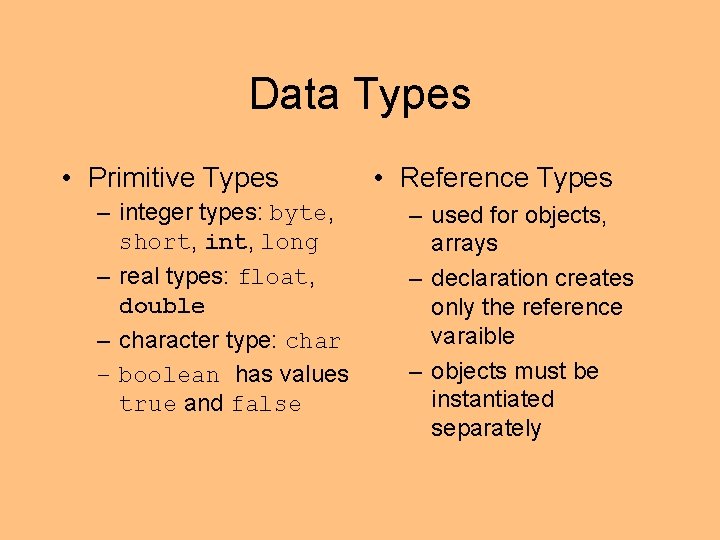
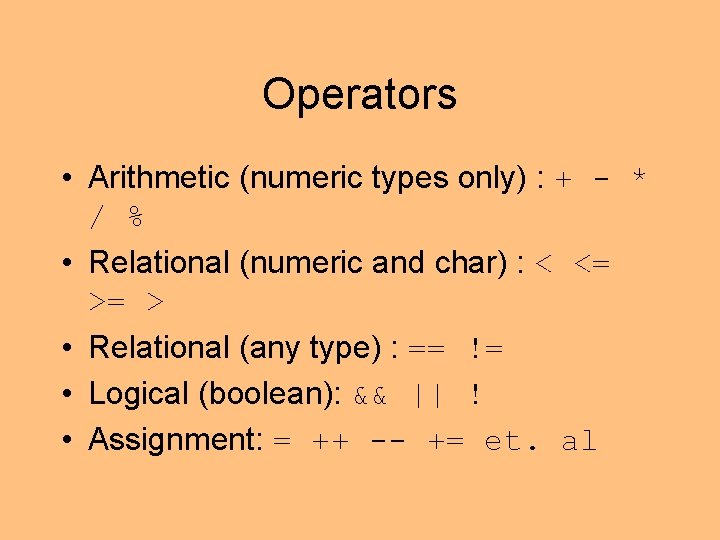
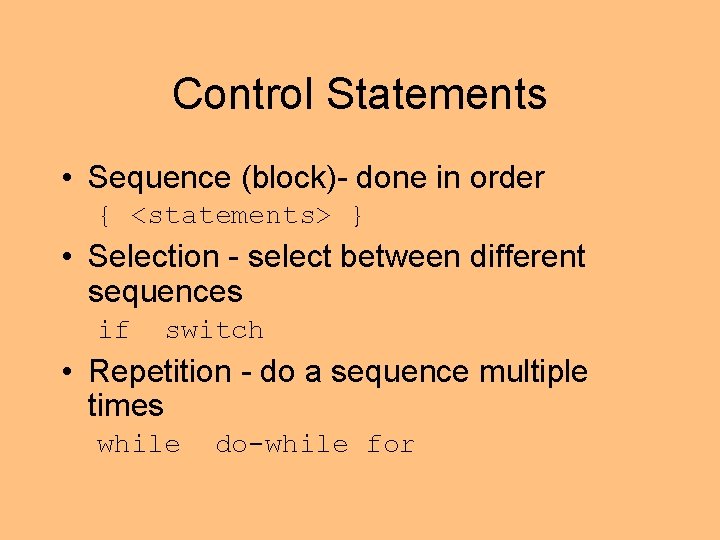
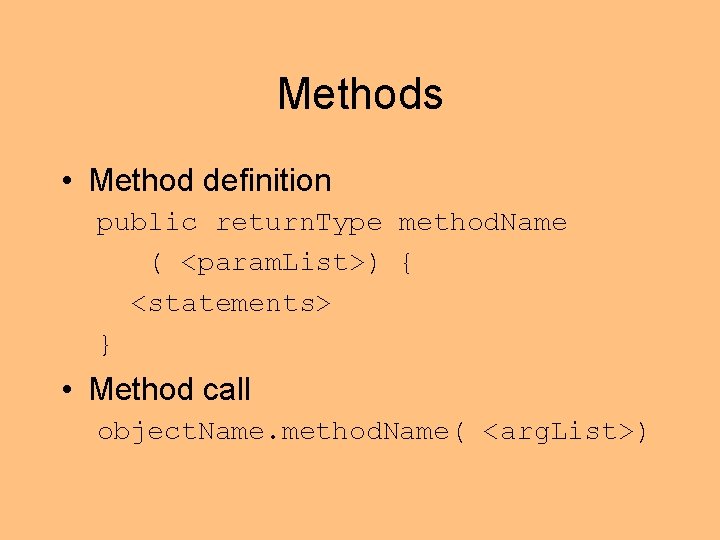
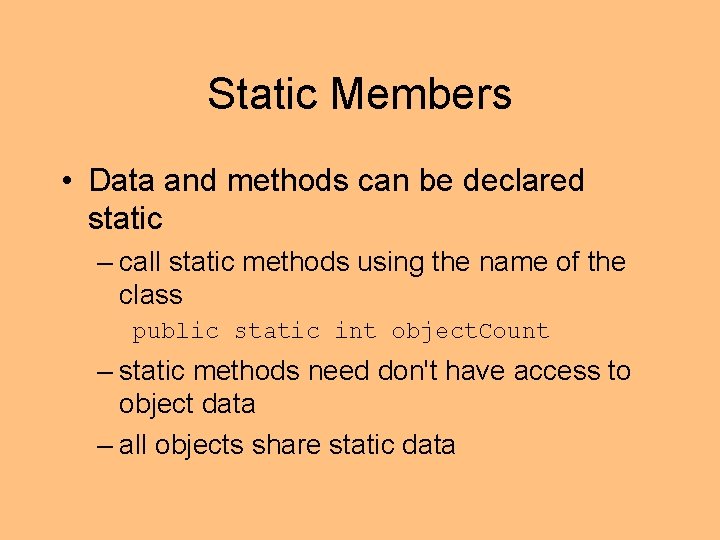
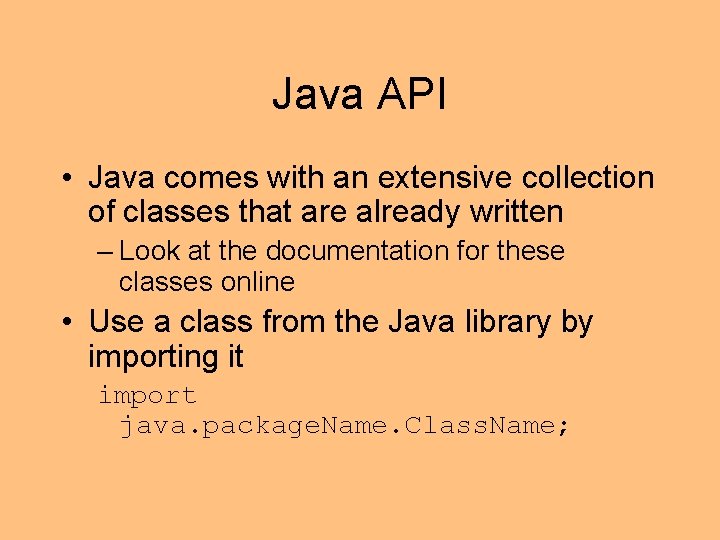
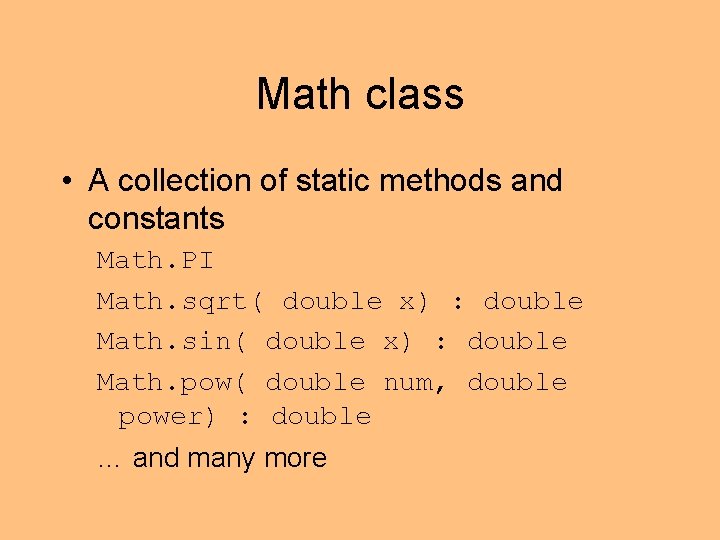
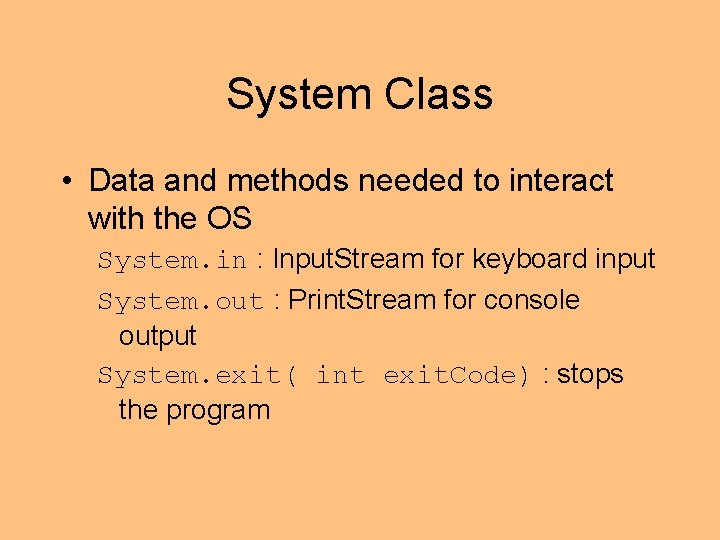
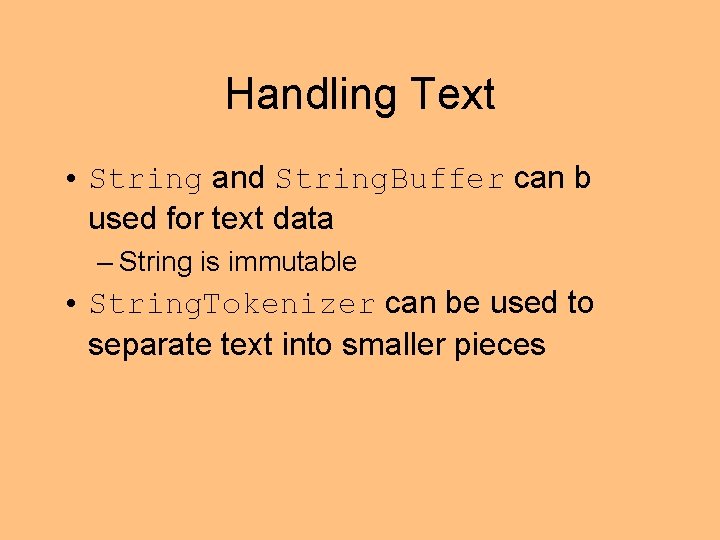
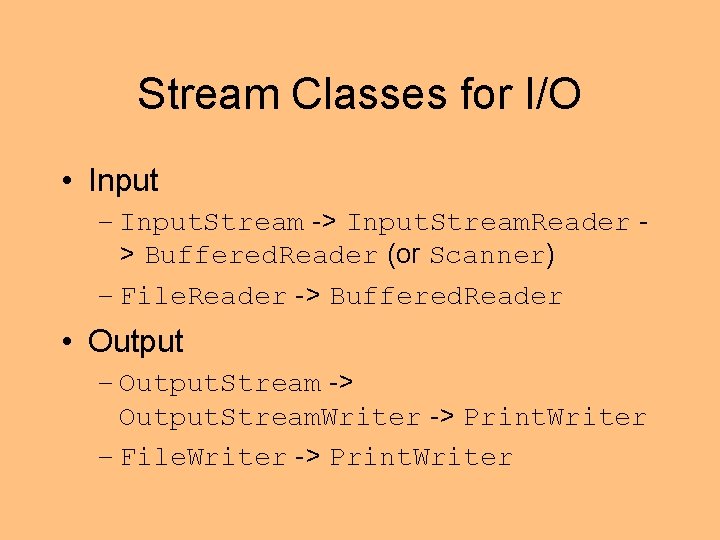
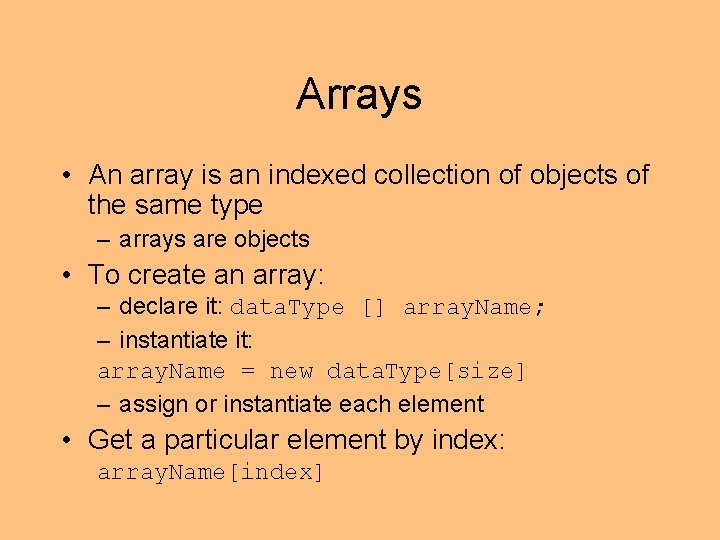
- Slides: 19
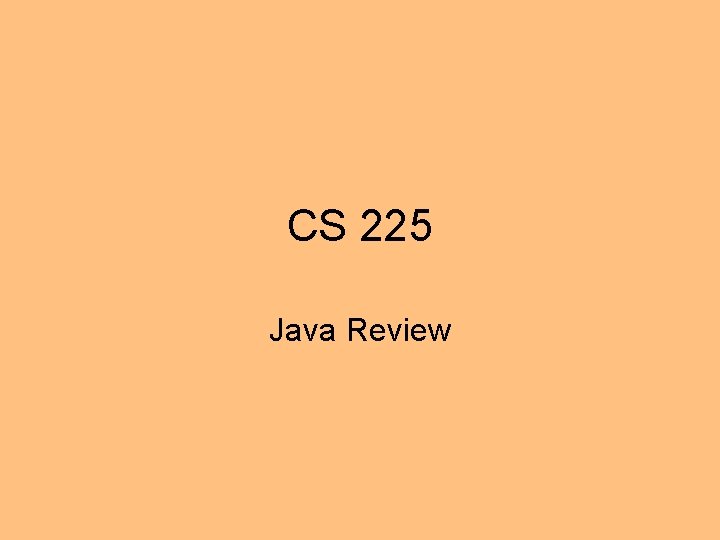
CS 225 Java Review
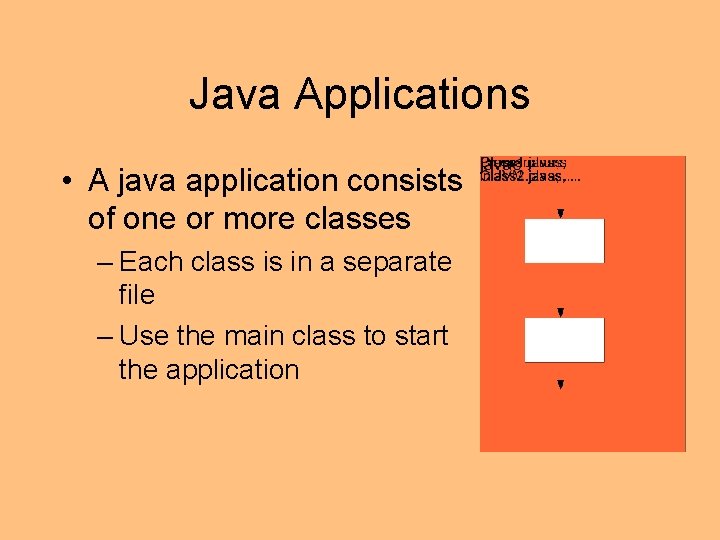
Java Applications • A java application consists of one or more classes – Each class is in a separate file – Use the main class to start the application
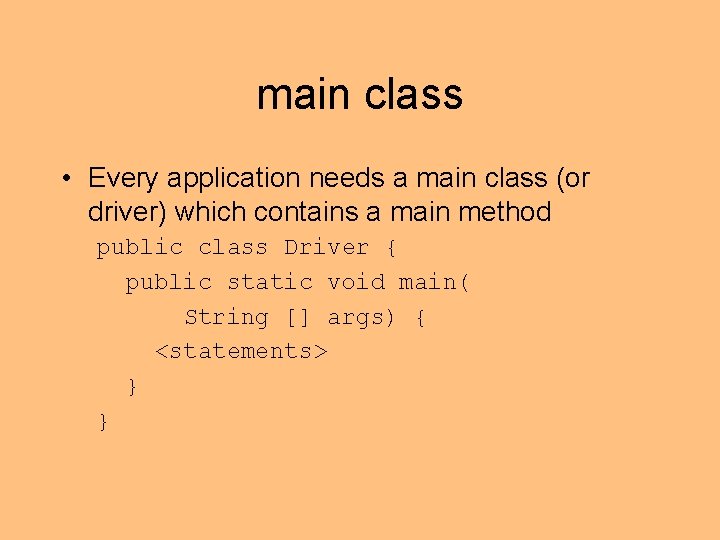
main class • Every application needs a main class (or driver) which contains a main method public class Driver { public static void main( String [] args) { <statements> } }
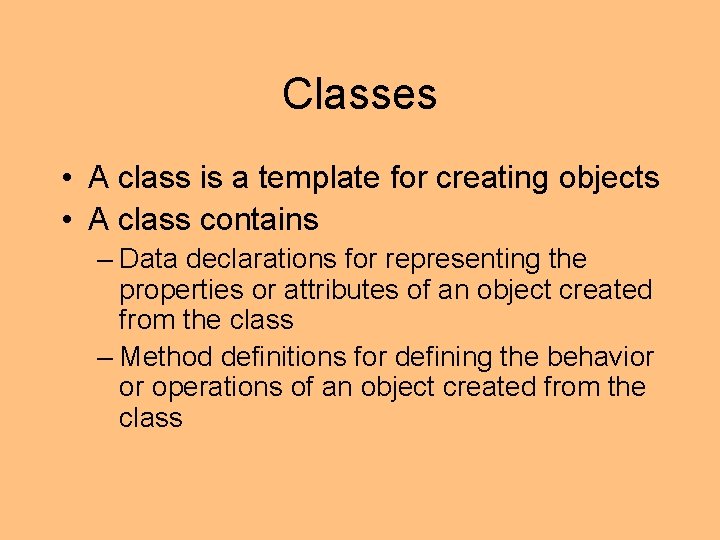
Classes • A class is a template for creating objects • A class contains – Data declarations for representing the properties or attributes of an object created from the class – Method definitions for defining the behavior or operations of an object created from the class
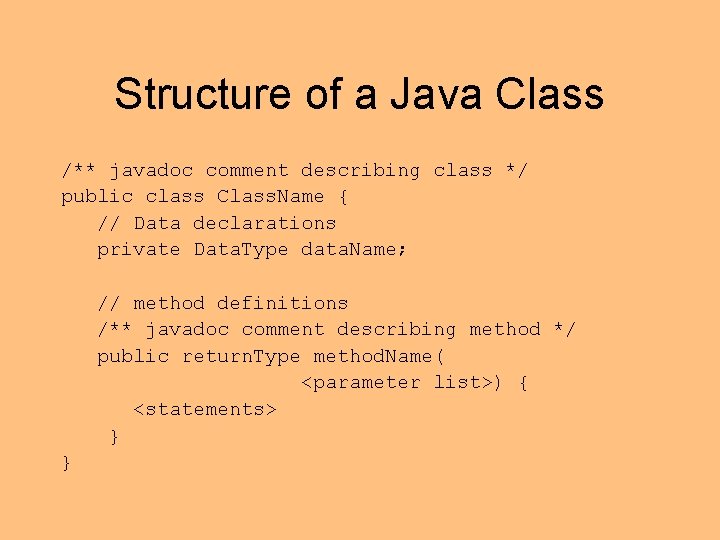
Structure of a Java Class /** javadoc comment describing class */ public class Class. Name { // Data declarations private Data. Type data. Name; // method definitions /** javadoc comment describing method */ public return. Type method. Name( <parameter list>) { <statements> } }
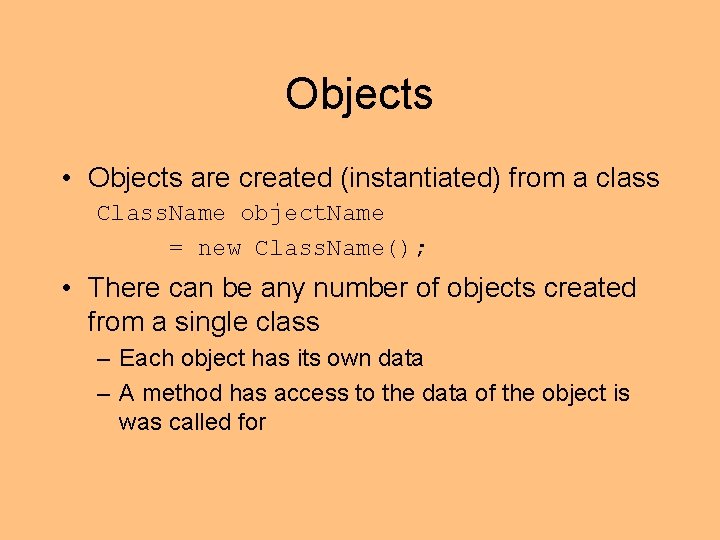
Objects • Objects are created (instantiated) from a class Class. Name object. Name = new Class. Name(); • There can be any number of objects created from a single class – Each object has its own data – A method has access to the data of the object is was called for
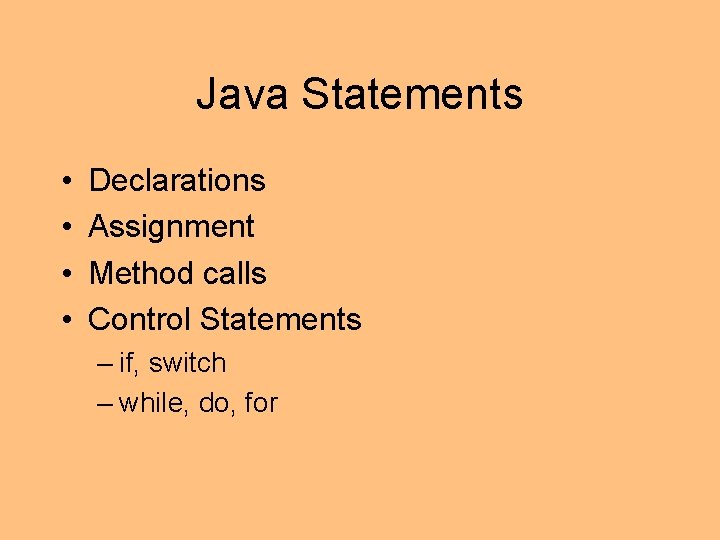
Java Statements • • Declarations Assignment Method calls Control Statements – if, switch – while, do, for
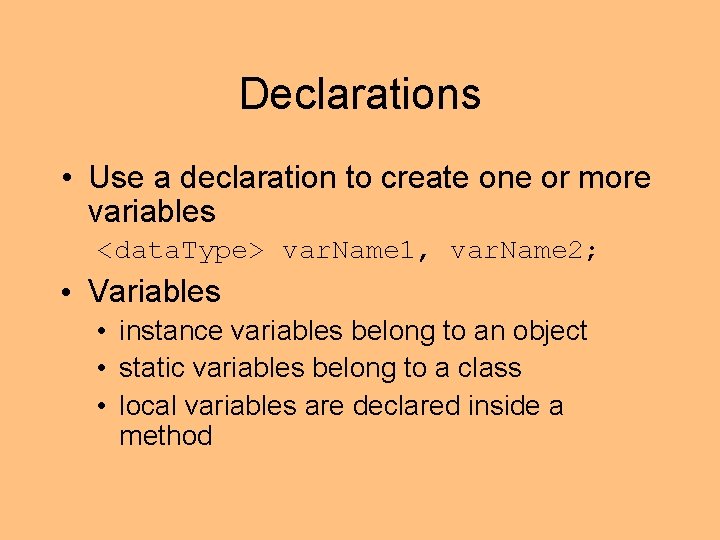
Declarations • Use a declaration to create one or more variables <data. Type> var. Name 1, var. Name 2; • Variables • instance variables belong to an object • static variables belong to a class • local variables are declared inside a method
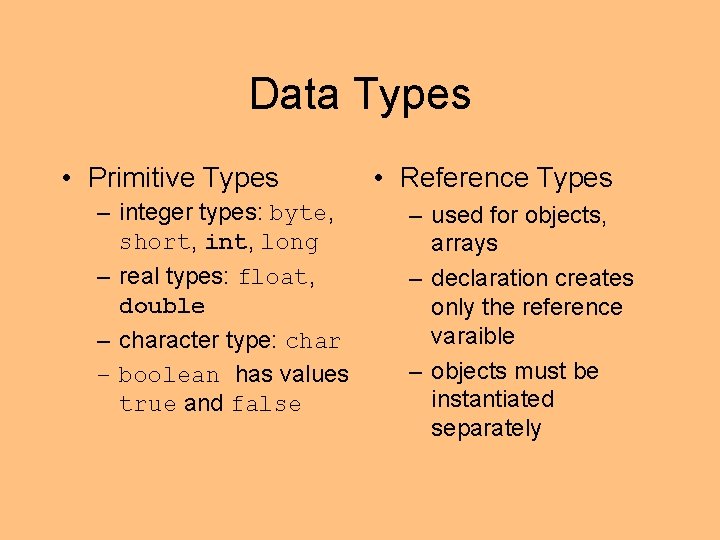
Data Types • Primitive Types – integer types: byte, short, int, long – real types: float, double – character type: char – boolean has values true and false • Reference Types – used for objects, arrays – declaration creates only the reference varaible – objects must be instantiated separately
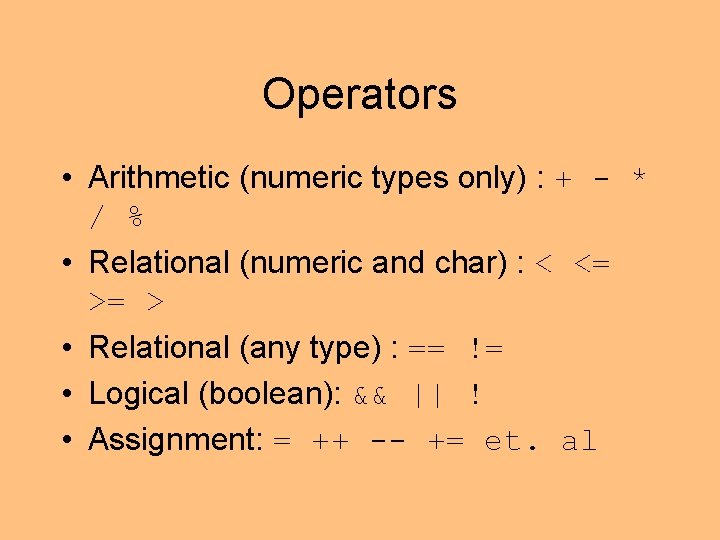
Operators • Arithmetic (numeric types only) : + - * / % • Relational (numeric and char) : < <= >= > • Relational (any type) : == != • Logical (boolean): && || ! • Assignment: = ++ -- += et. al
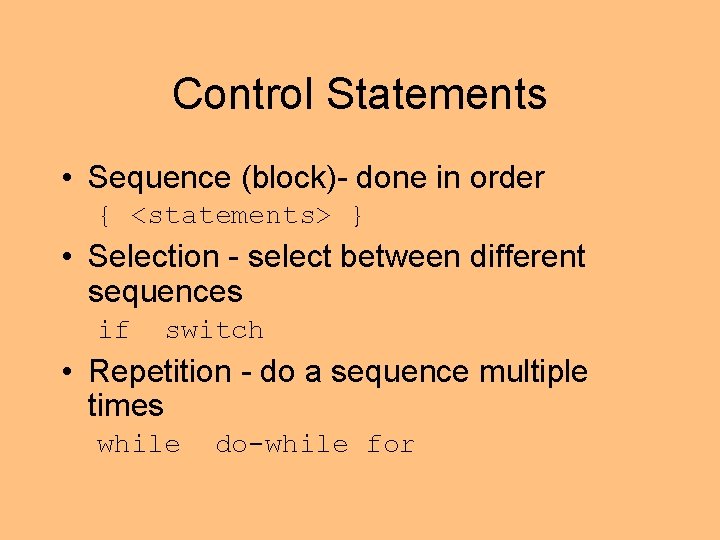
Control Statements • Sequence (block)- done in order { <statements> } • Selection - select between different sequences if switch • Repetition - do a sequence multiple times while do-while for
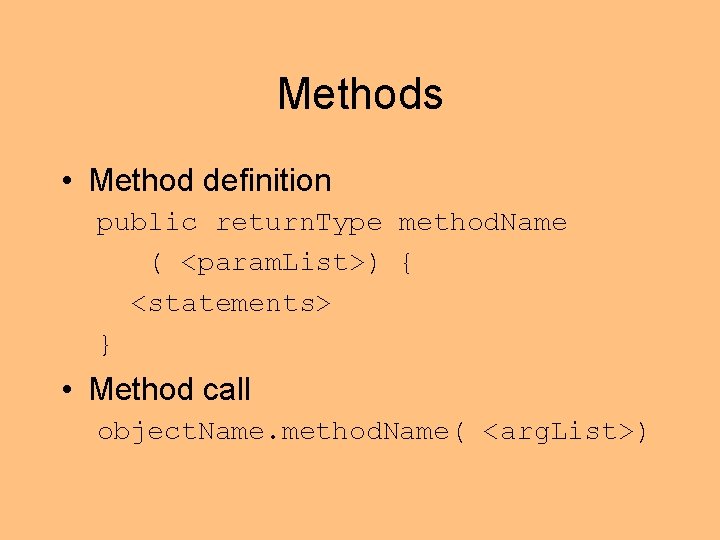
Methods • Method definition public return. Type method. Name ( <param. List>) { <statements> } • Method call object. Name. method. Name( <arg. List>)
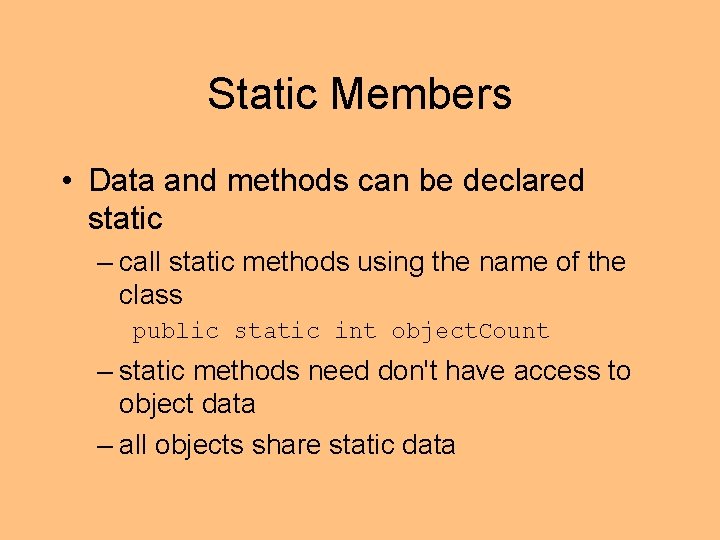
Static Members • Data and methods can be declared static – call static methods using the name of the class public static int object. Count – static methods need don't have access to object data – all objects share static data
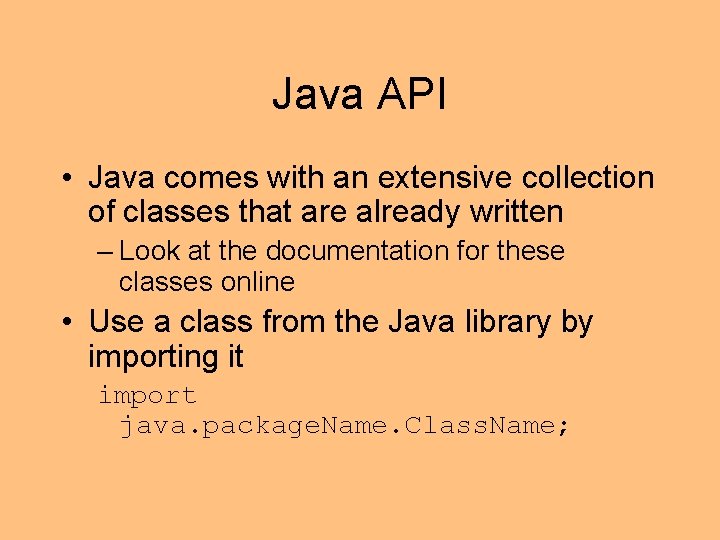
Java API • Java comes with an extensive collection of classes that are already written – Look at the documentation for these classes online • Use a class from the Java library by importing it import java. package. Name. Class. Name;
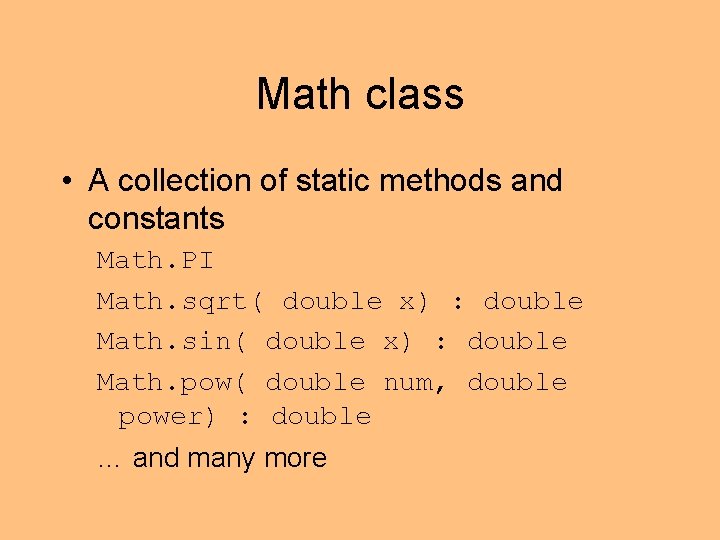
Math class • A collection of static methods and constants Math. PI Math. sqrt( double x) : double Math. sin( double x) : double Math. pow( double num, double power) : double … and many more
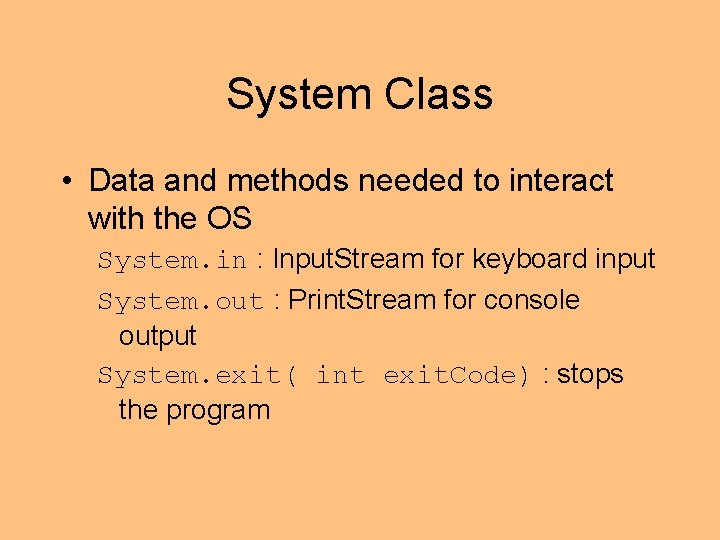
System Class • Data and methods needed to interact with the OS System. in : Input. Stream for keyboard input System. out : Print. Stream for console output System. exit( int exit. Code) : stops the program
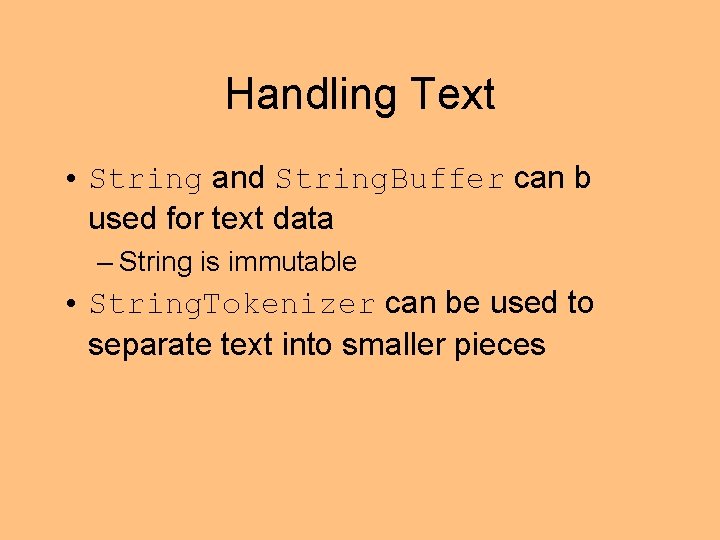
Handling Text • String and String. Buffer can b used for text data – String is immutable • String. Tokenizer can be used to separate text into smaller pieces
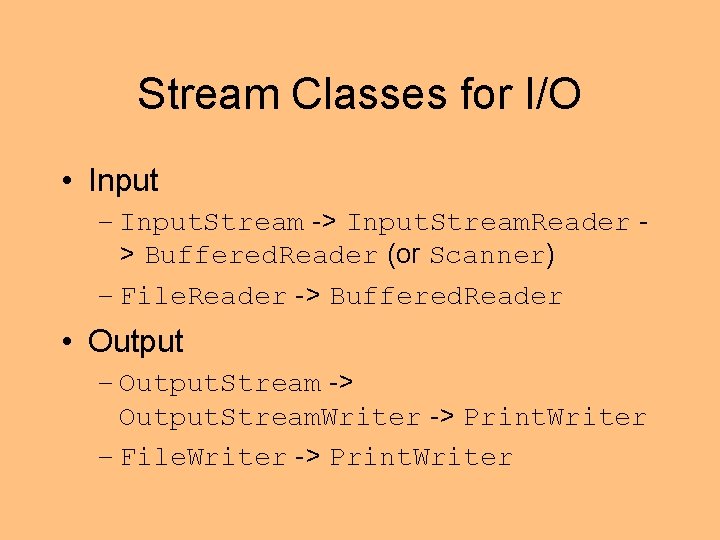
Stream Classes for I/O • Input – Input. Stream -> Input. Stream. Reader > Buffered. Reader (or Scanner) – File. Reader -> Buffered. Reader • Output – Output. Stream -> Output. Stream. Writer -> Print. Writer – File. Writer -> Print. Writer
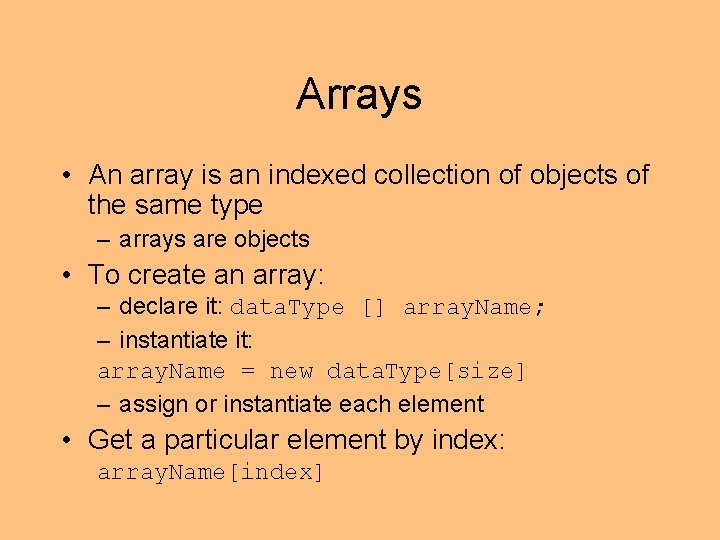
Arrays • An array is an indexed collection of objects of the same type – arrays are objects • To create an array: – declare it: data. Type [] array. Name; – instantiate it: array. Name = new data. Type[size] – assign or instantiate each element • Get a particular element by index: array. Name[index]
140 sayısının 25 eksiği
Sp 225 warszawa
Is 144 a cube number
Factor tree for 225
Types of prime numbers
Artigo 225
Artigo 225
Initial ray and terminal ray
Cmpt 225 sfu
225 binary
Calor sensivel
En una caja hay 75 canicas azules y 225 rojas
Rüzgar yönleri
Troop 225
Csc 225
Convert from degrees to radians 225 degrees
Jelaskan tentang konsep angka indeks
Dmca square root
Double hashing
Cmpt 225