XML files with LINQ Introduction to LINQ Language
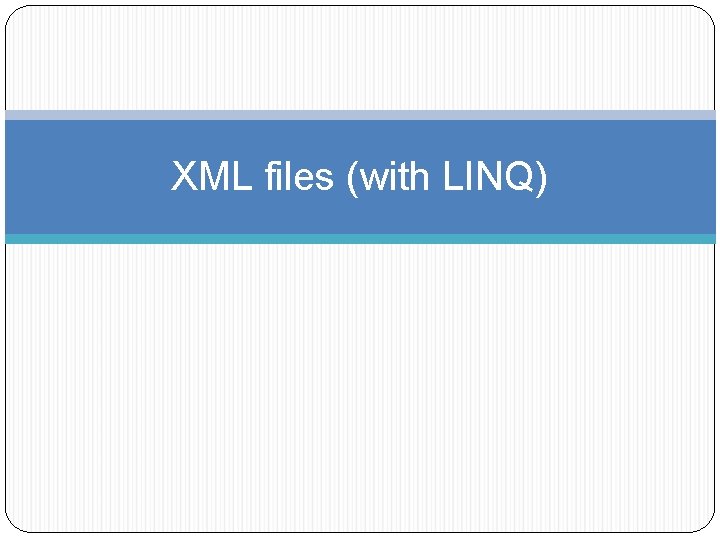
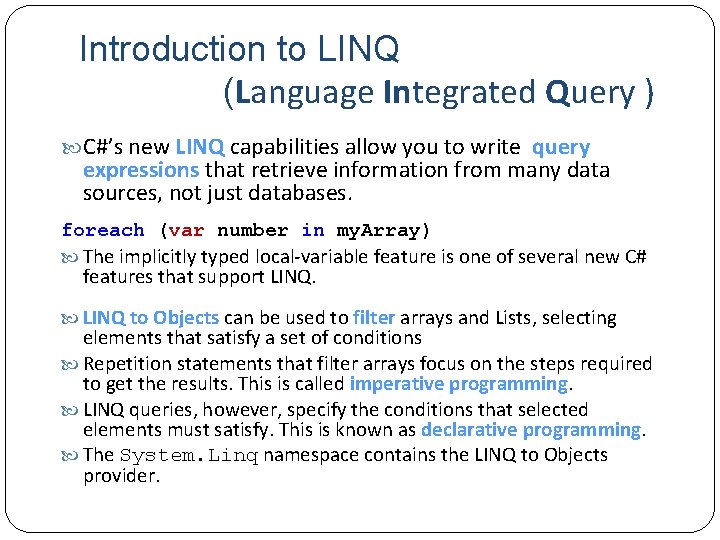
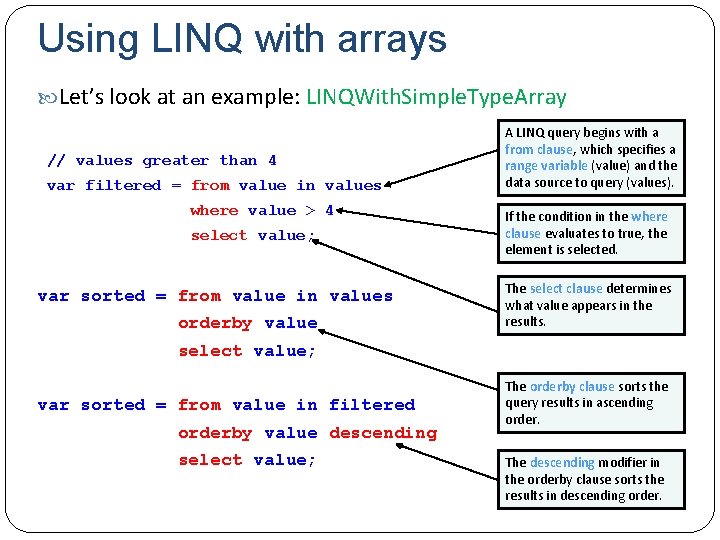
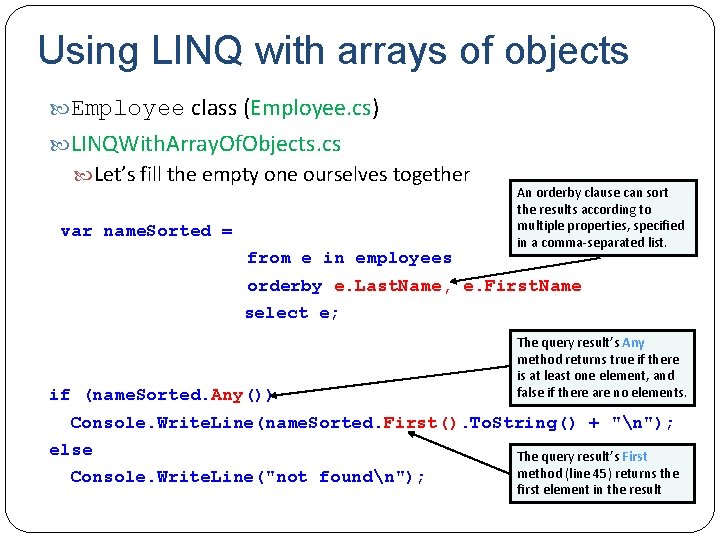
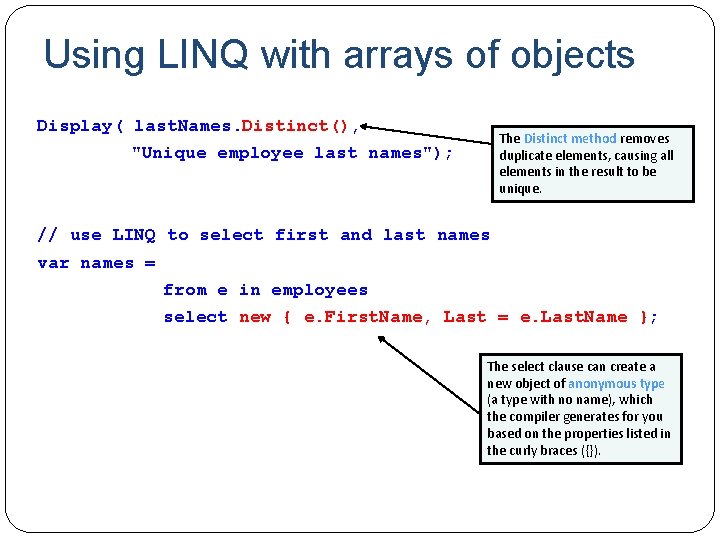
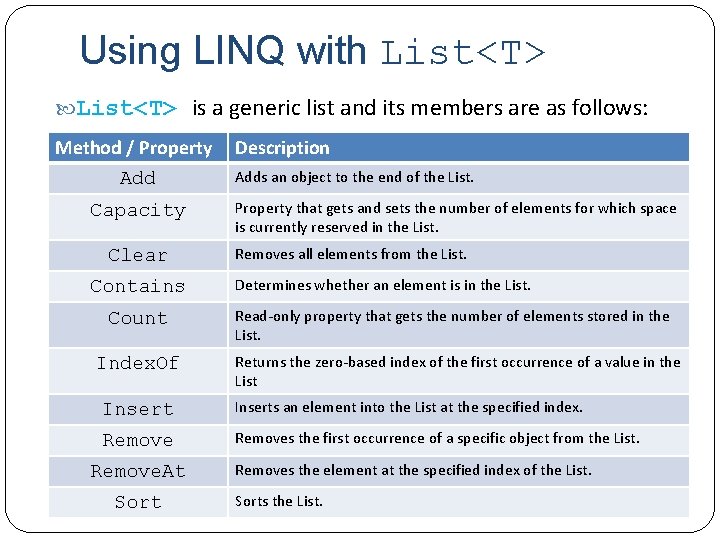
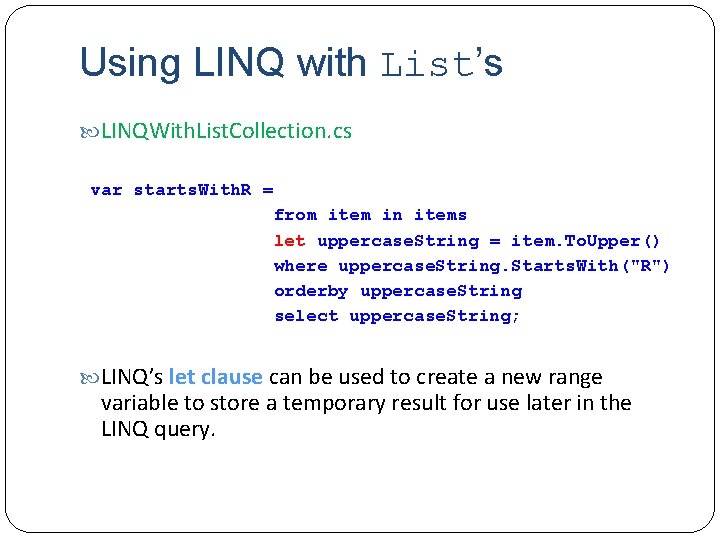
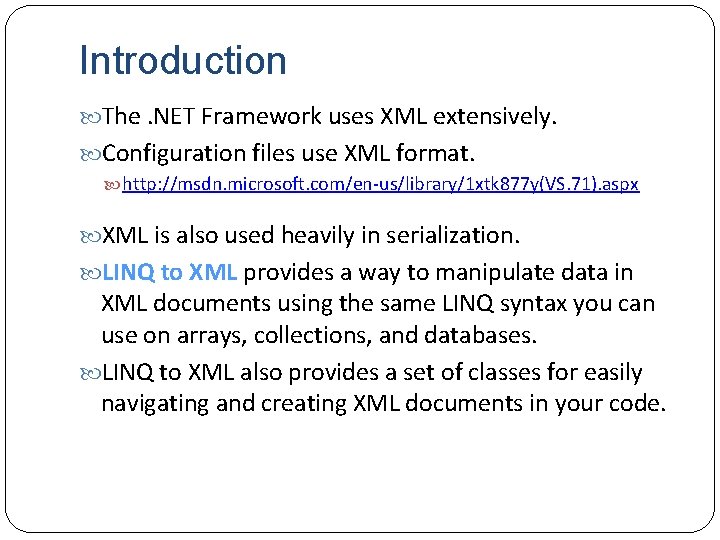
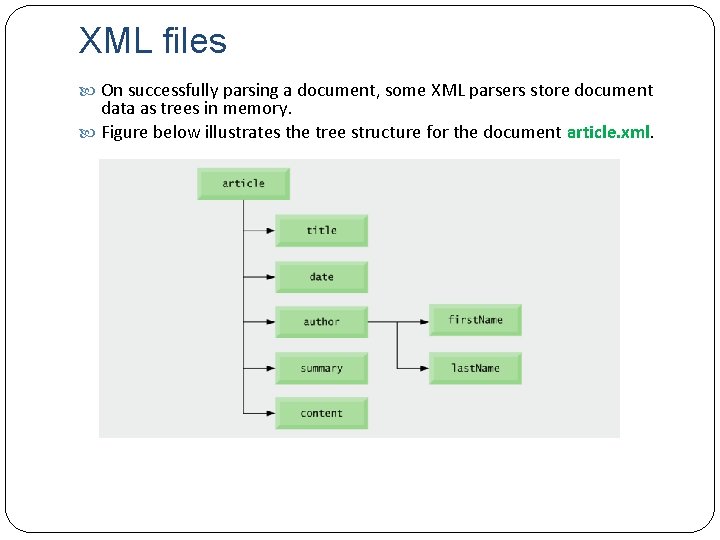
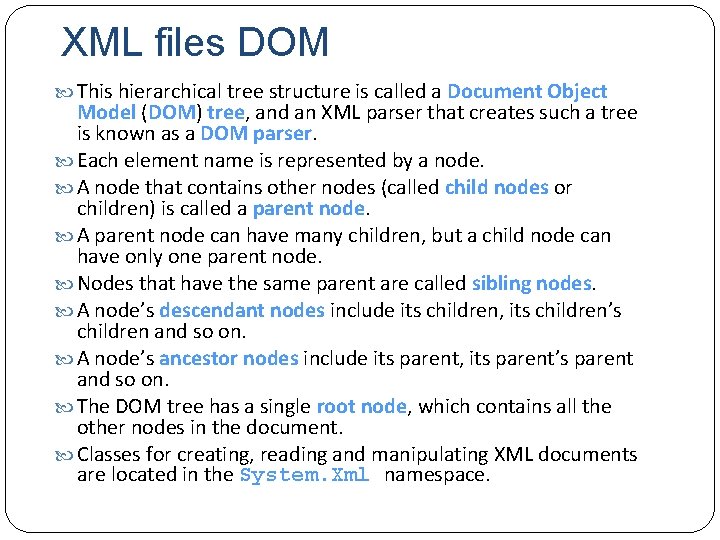
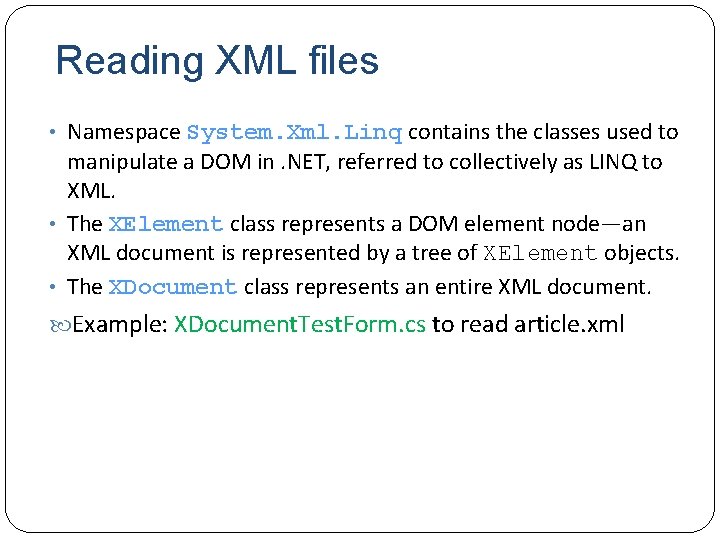
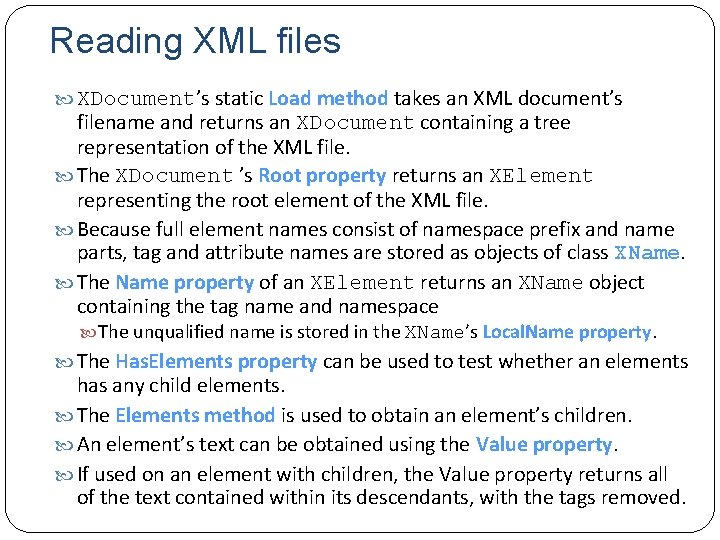
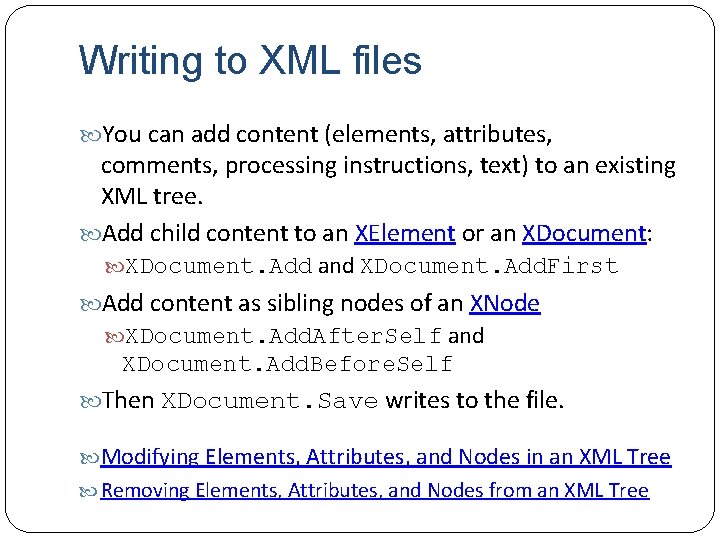
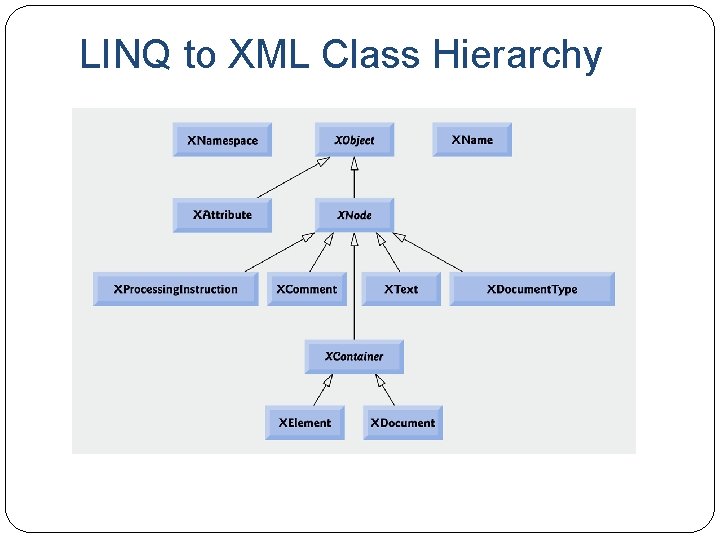
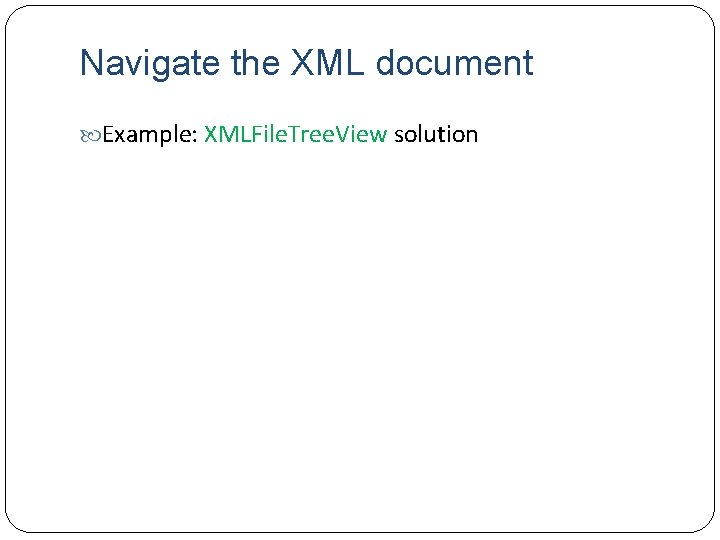
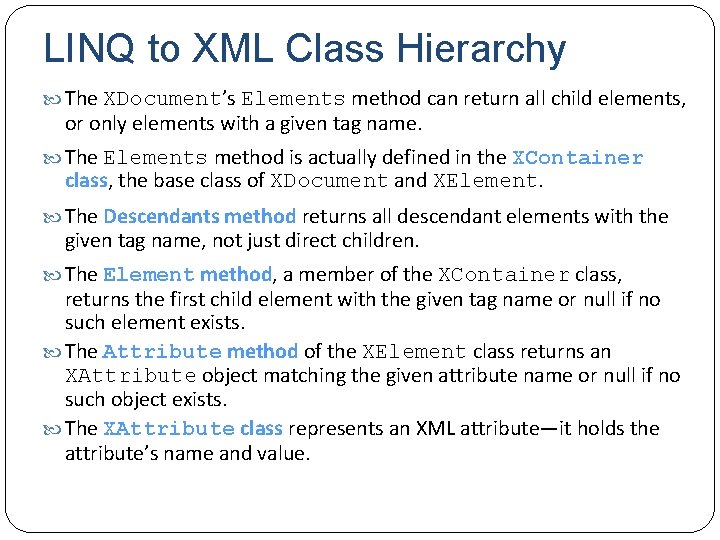
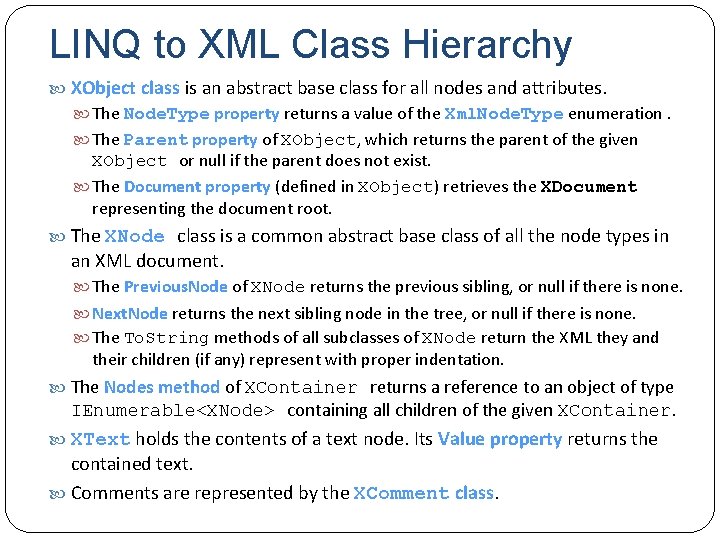
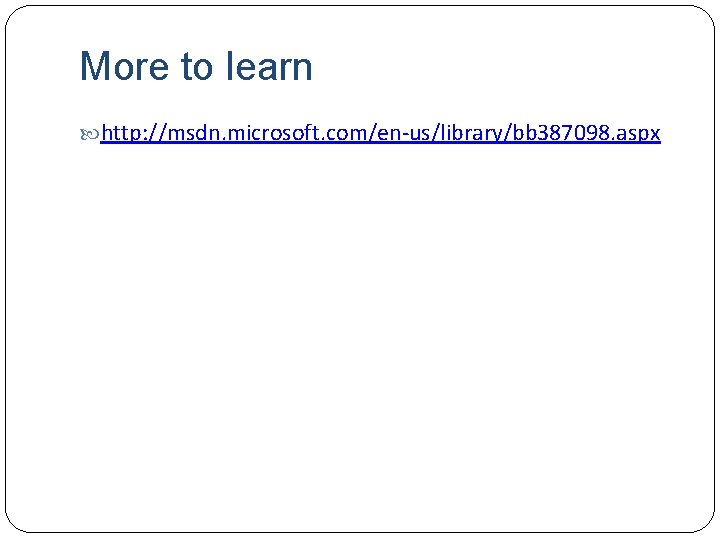
- Slides: 18
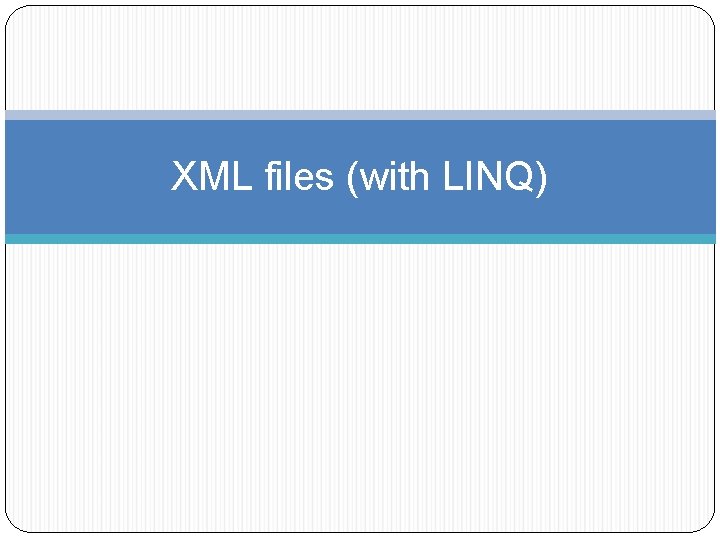
XML files (with LINQ)
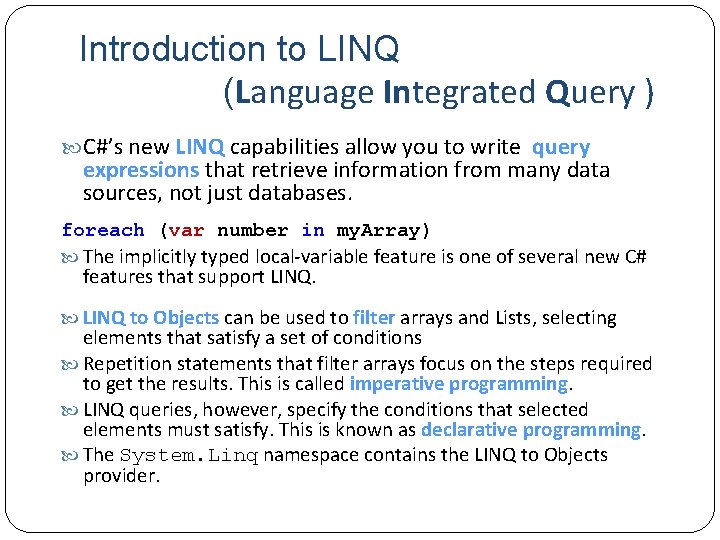
Introduction to LINQ (Language Integrated Query ) C#’s new LINQ capabilities allow you to write query expressions that retrieve information from many data sources, not just databases. foreach (var number in my. Array) The implicitly typed local-variable feature is one of several new C# features that support LINQ to Objects can be used to filter arrays and Lists, selecting elements that satisfy a set of conditions Repetition statements that filter arrays focus on the steps required to get the results. This is called imperative programming. LINQ queries, however, specify the conditions that selected elements must satisfy. This is known as declarative programming. The System. Linq namespace contains the LINQ to Objects provider.
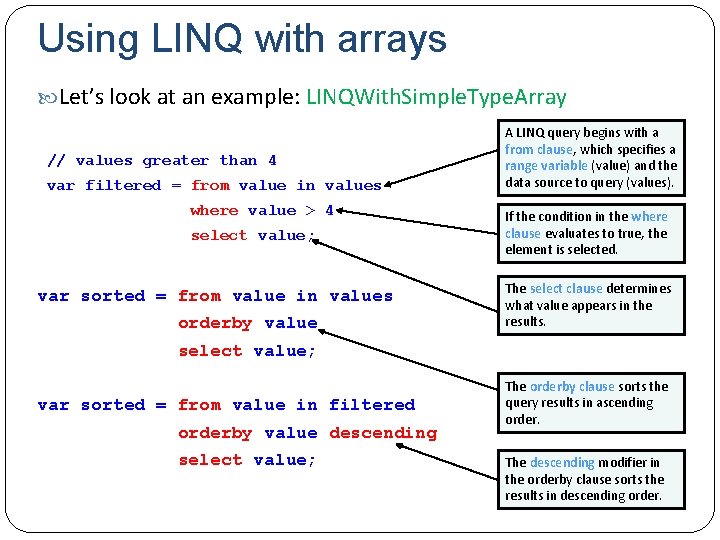
Using LINQ with arrays Let’s look at an example: LINQWith. Simple. Type. Array // values greater than 4 var filtered = from value in values where value > 4 select value; var sorted = from value in values orderby value A LINQ query begins with a from clause, which specifies a range variable (value) and the data source to query (values). If the condition in the where clause evaluates to true, the element is selected. The select clause determines what value appears in the results. select value; var sorted = from value in filtered orderby value descending select value; The orderby clause sorts the query results in ascending order. The descending modifier in the orderby clause sorts the results in descending order.
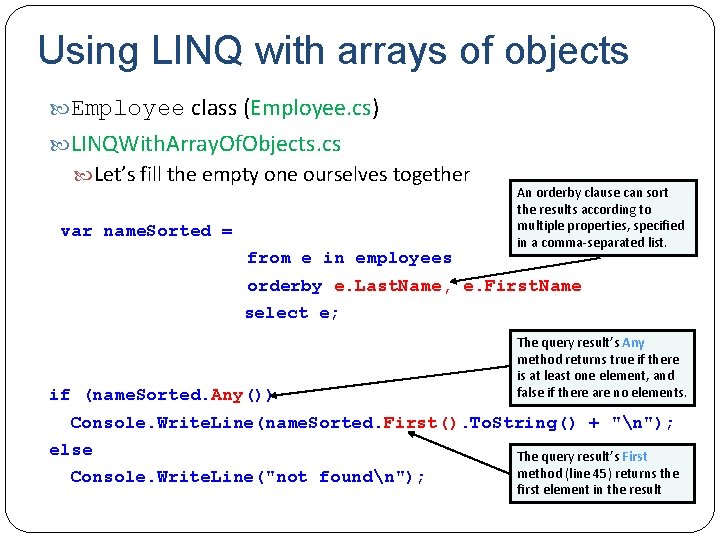
Using LINQ with arrays of objects Employee class (Employee. cs) LINQWith. Array. Of. Objects. cs Let’s fill the empty one ourselves together var name. Sorted = from e in employees An orderby clause can sort the results according to multiple properties, specified in a comma-separated list. orderby e. Last. Name, e. First. Name select e; if (name. Sorted. Any()) The query result’s Any method returns true if there is at least one element, and false if there are no elements. Console. Write. Line(name. Sorted. First(). To. String() + "n"); else Console. Write. Line("not foundn"); The query result’s First method (line 45) returns the first element in the result
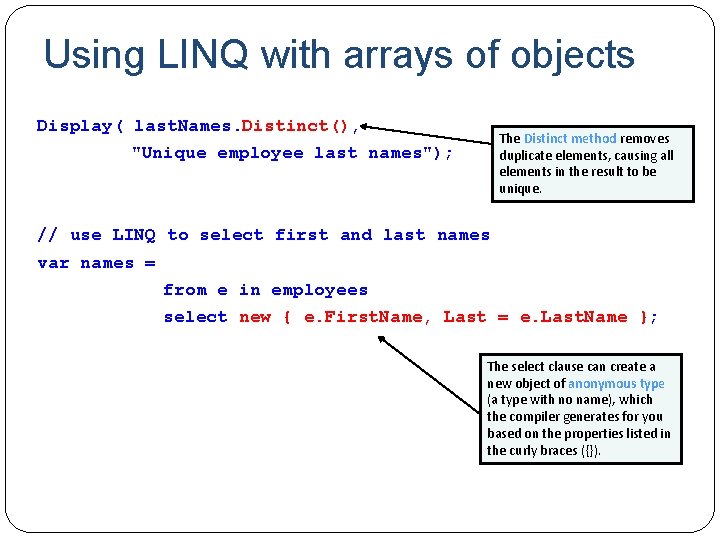
Using LINQ with arrays of objects Display( last. Names. Distinct(), The Distinct method removes duplicate elements, causing all elements in the result to be unique. "Unique employee last names"); // use LINQ to select first and last names var names = from e in employees select new { e. First. Name, Last = e. Last. Name }; The select clause can create a new object of anonymous type (a type with no name), which the compiler generates for you based on the properties listed in the curly braces ({}).
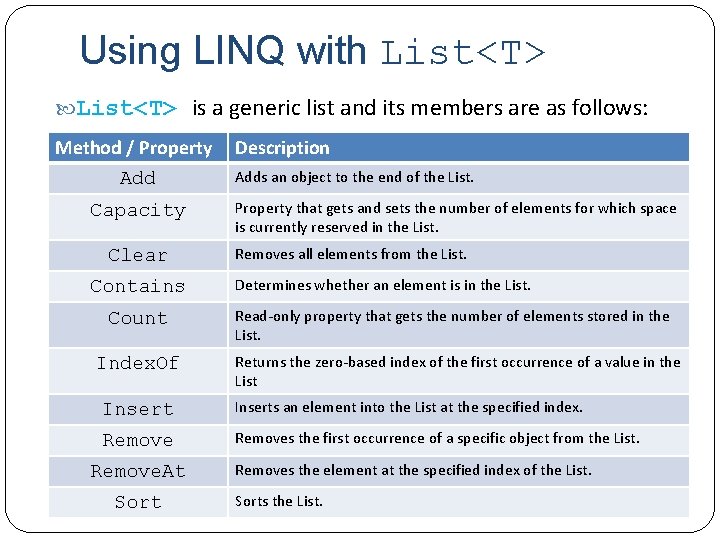
Using LINQ with List<T> is a generic list and its members are as follows: Method / Property Add Capacity Clear Contains Count Index. Of Description Adds an object to the end of the List. Property that gets and sets the number of elements for which space is currently reserved in the List. Removes all elements from the List. Determines whether an element is in the List. Read-only property that gets the number of elements stored in the List. Returns the zero-based index of the first occurrence of a value in the List Inserts an element into the List at the specified index. Removes the first occurrence of a specific object from the List. Remove. At Sort Removes the element at the specified index of the List. Sorts the List.
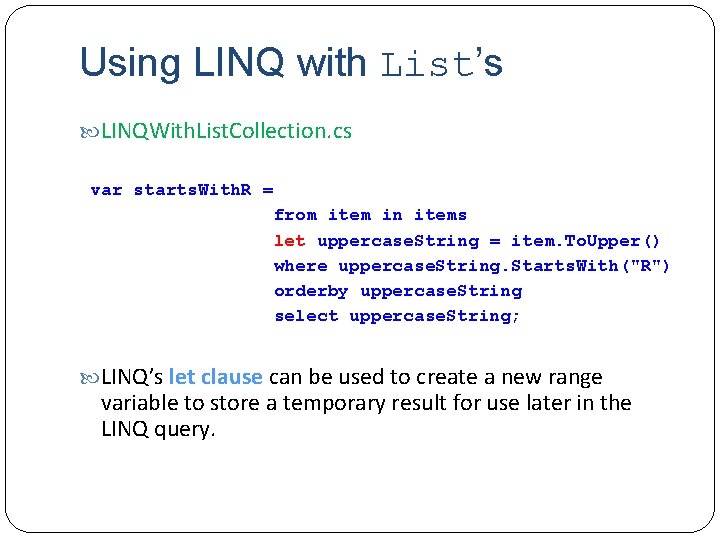
Using LINQ with List’s LINQWith. List. Collection. cs var starts. With. R = from item in items let uppercase. String = item. To. Upper() where uppercase. String. Starts. With("R") orderby uppercase. String select uppercase. String; LINQ’s let clause can be used to create a new range variable to store a temporary result for use later in the LINQ query.
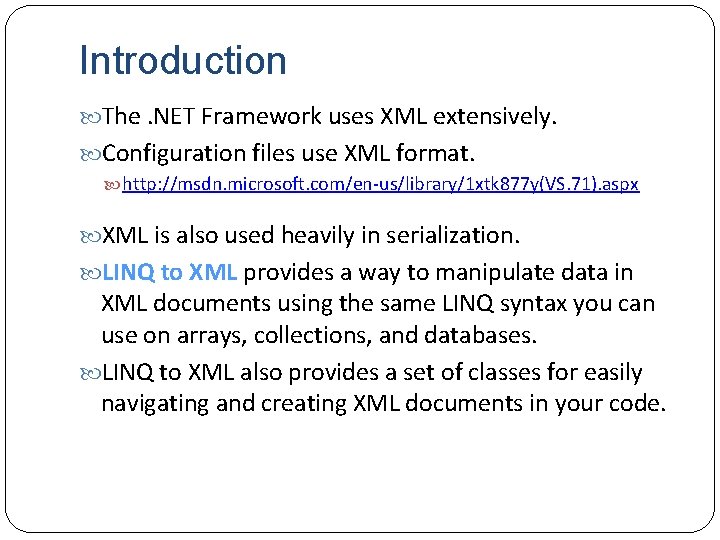
Introduction The. NET Framework uses XML extensively. Configuration files use XML format. http: //msdn. microsoft. com/en-us/library/1 xtk 877 y(VS. 71). aspx XML is also used heavily in serialization. LINQ to XML provides a way to manipulate data in XML documents using the same LINQ syntax you can use on arrays, collections, and databases. LINQ to XML also provides a set of classes for easily navigating and creating XML documents in your code.
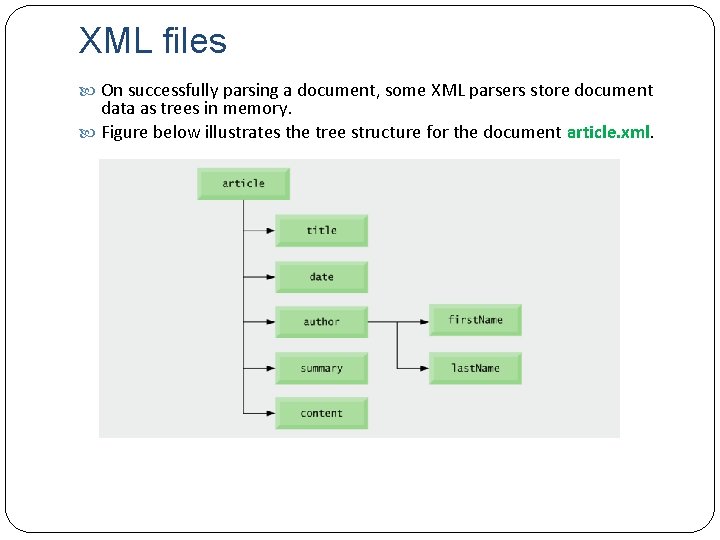
XML files On successfully parsing a document, some XML parsers store document data as trees in memory. Figure below illustrates the tree structure for the document article. xml.
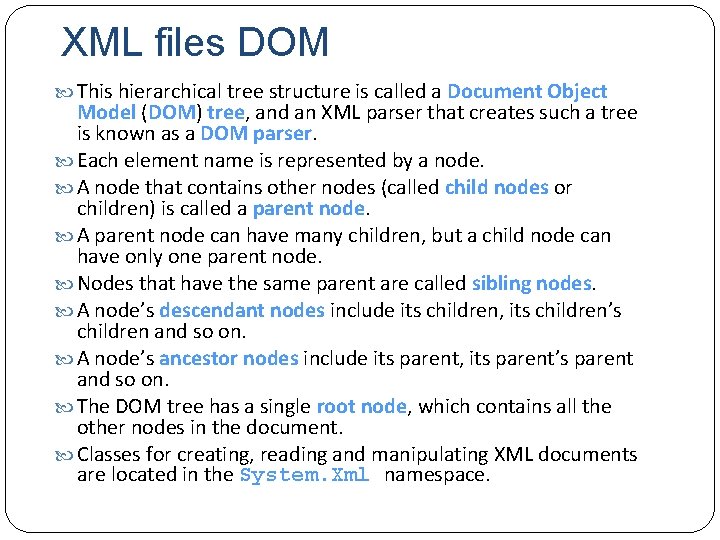
XML files DOM This hierarchical tree structure is called a Document Object Model (DOM) tree, and an XML parser that creates such a tree is known as a DOM parser. Each element name is represented by a node. A node that contains other nodes (called child nodes or children) is called a parent node. A parent node can have many children, but a child node can have only one parent node. Nodes that have the same parent are called sibling nodes. A node’s descendant nodes include its children, its children’s children and so on. A node’s ancestor nodes include its parent, its parent’s parent and so on. The DOM tree has a single root node, which contains all the other nodes in the document. Classes for creating, reading and manipulating XML documents are located in the System. Xml namespace.
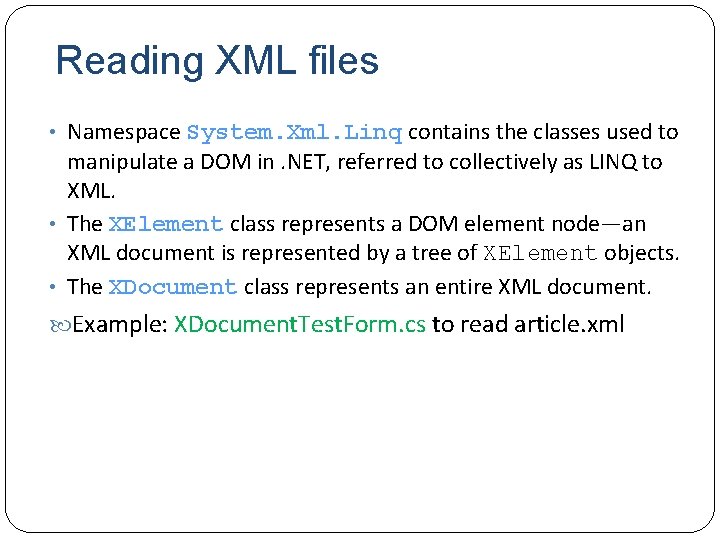
Reading XML files • Namespace System. Xml. Linq contains the classes used to manipulate a DOM in. NET, referred to collectively as LINQ to XML. • The XElement class represents a DOM element node—an XML document is represented by a tree of XElement objects. • The XDocument class represents an entire XML document. Example: XDocument. Test. Form. cs to read article. xml
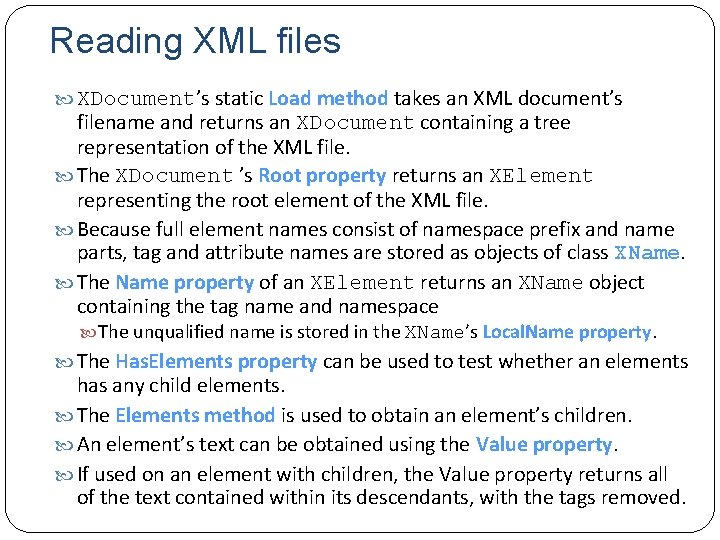
Reading XML files XDocument’s static Load method takes an XML document’s filename and returns an XDocument containing a tree representation of the XML file. The XDocument ’s Root property returns an XElement representing the root element of the XML file. Because full element names consist of namespace prefix and name parts, tag and attribute names are stored as objects of class XName. The Name property of an XElement returns an XName object containing the tag name and namespace The unqualified name is stored in the XName’s Local. Name property. The Has. Elements property can be used to test whether an elements has any child elements. The Elements method is used to obtain an element’s children. An element’s text can be obtained using the Value property. If used on an element with children, the Value property returns all of the text contained within its descendants, with the tags removed.
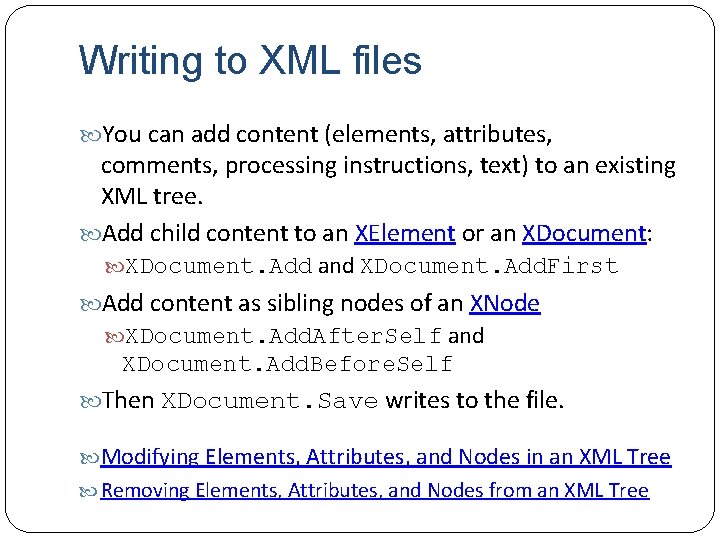
Writing to XML files You can add content (elements, attributes, comments, processing instructions, text) to an existing XML tree. Add child content to an XElement or an XDocument: XDocument. Add and XDocument. Add. First Add content as sibling nodes of an XNode XDocument. Add. After. Self and XDocument. Add. Before. Self Then XDocument. Save writes to the file. Modifying Elements, Attributes, and Nodes in an XML Tree Removing Elements, Attributes, and Nodes from an XML Tree
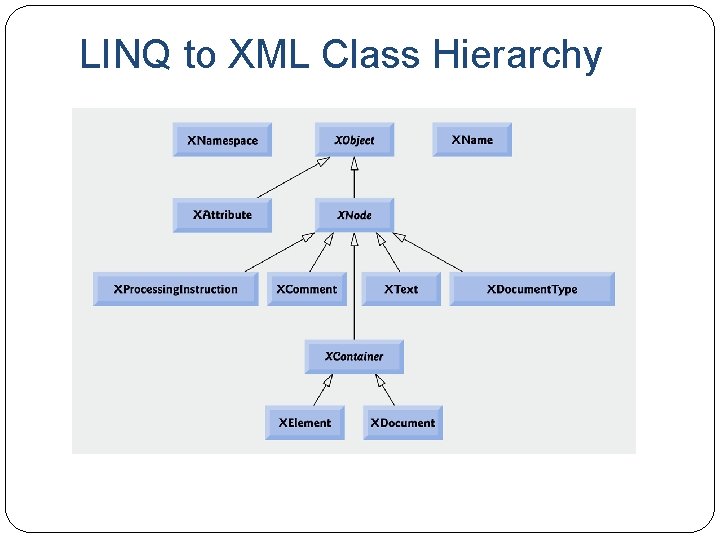
LINQ to XML Class Hierarchy
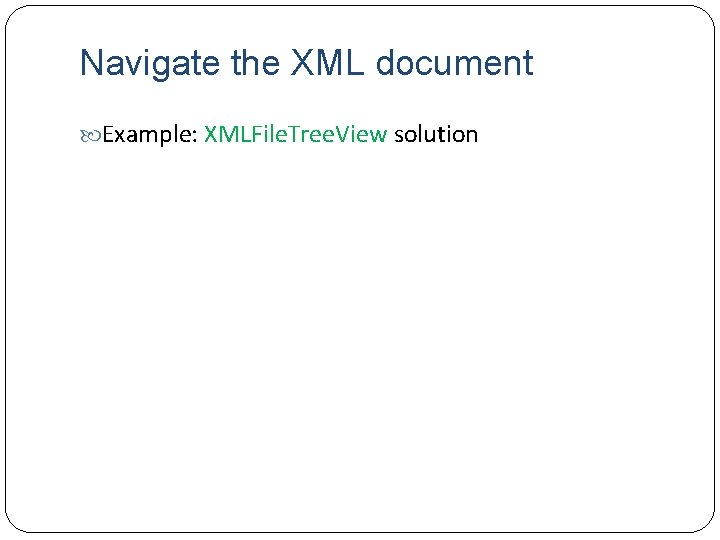
Navigate the XML document Example: XMLFile. Tree. View solution
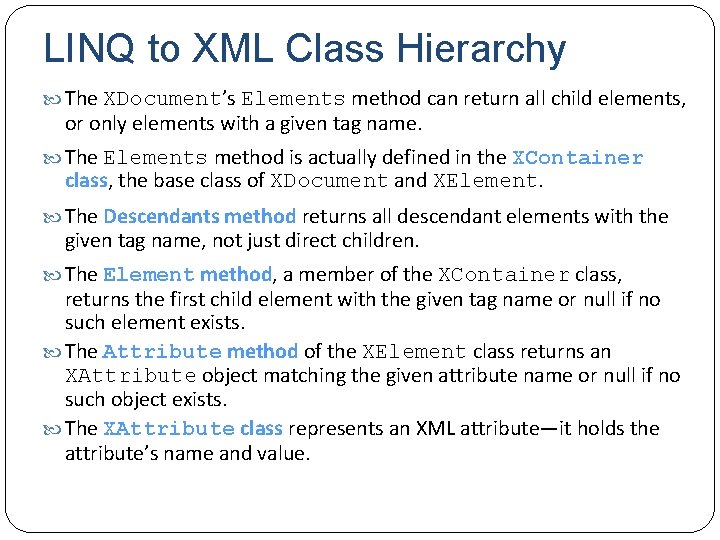
LINQ to XML Class Hierarchy The XDocument’s Elements method can return all child elements, or only elements with a given tag name. The Elements method is actually defined in the XContainer class, the base class of XDocument and XElement. The Descendants method returns all descendant elements with the given tag name, not just direct children. The Element method, a member of the XContainer class, returns the first child element with the given tag name or null if no such element exists. The Attribute method of the XElement class returns an XAttribute object matching the given attribute name or null if no such object exists. The XAttribute class represents an XML attribute—it holds the attribute’s name and value.
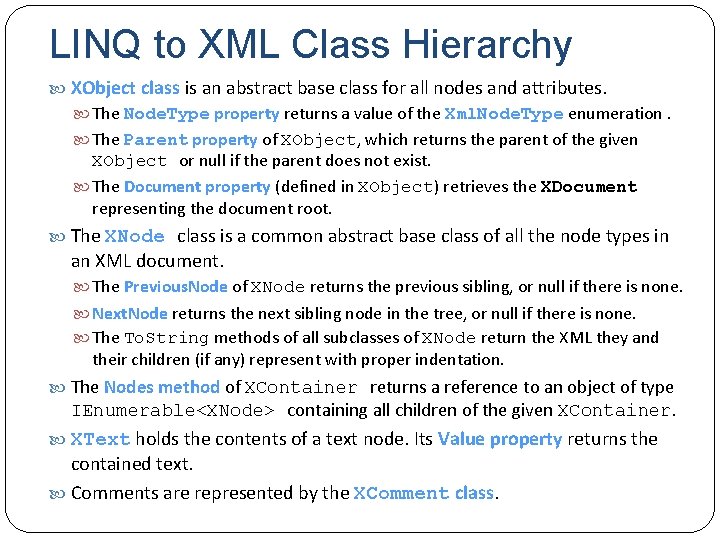
LINQ to XML Class Hierarchy XObject class is an abstract base class for all nodes and attributes. The Node. Type property returns a value of the Xml. Node. Type enumeration. The Parent property of XObject, which returns the parent of the given XObject or null if the parent does not exist. The Document property (defined in XObject) retrieves the XDocument representing the document root. The XNode class is a common abstract base class of all the node types in an XML document. The Previous. Node of XNode returns the previous sibling, or null if there is none. Next. Node returns the next sibling node in the tree, or null if there is none. The To. String methods of all subclasses of XNode return the XML they and their children (if any) represent with proper indentation. The Nodes method of XContainer returns a reference to an object of type IEnumerable<XNode> containing all children of the given XContainer. XText holds the contents of a text node. Its Value property returns the contained text. Comments are represented by the XComment class.
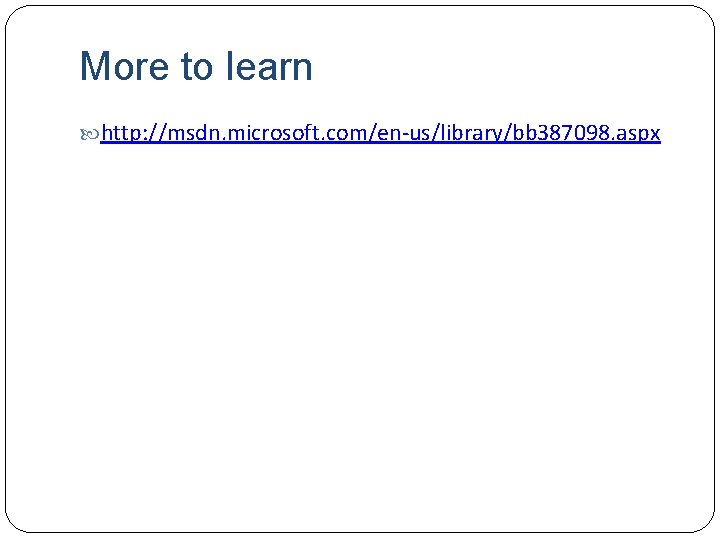
More to learn http: //msdn. microsoft. com/en-us/library/bb 387098. aspx