Todays topics Java Applications Graphics Upcoming Review for
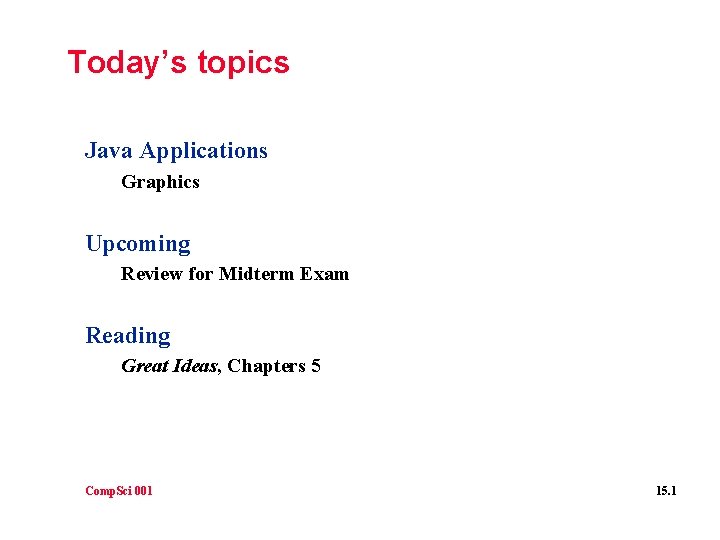
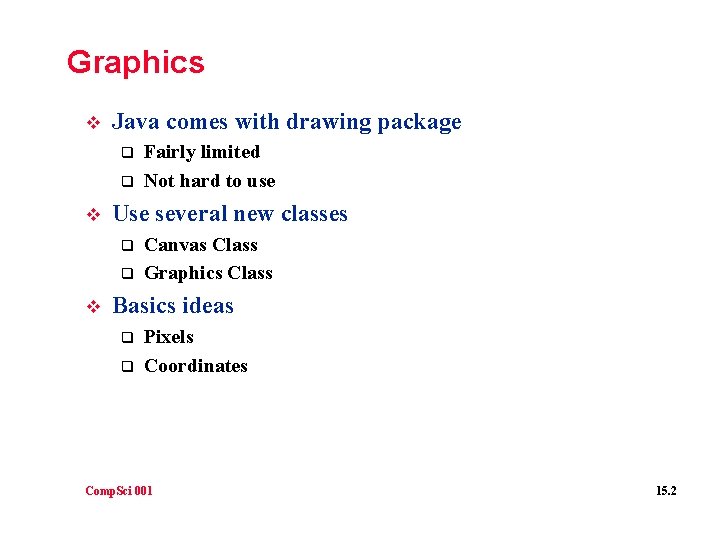
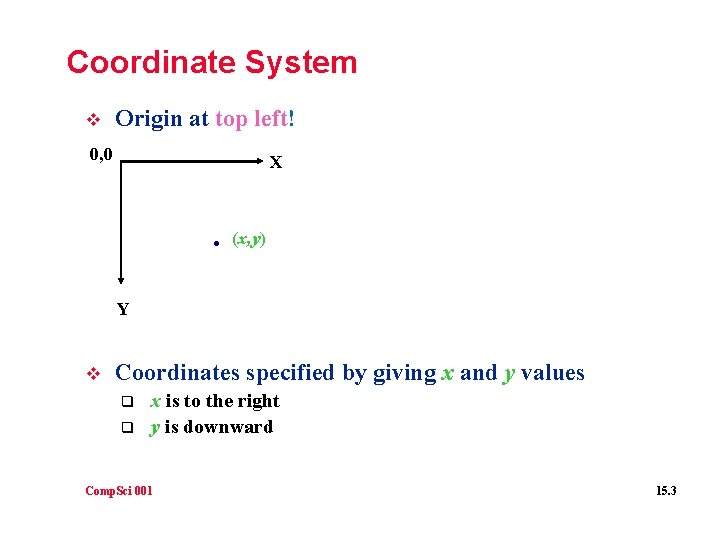
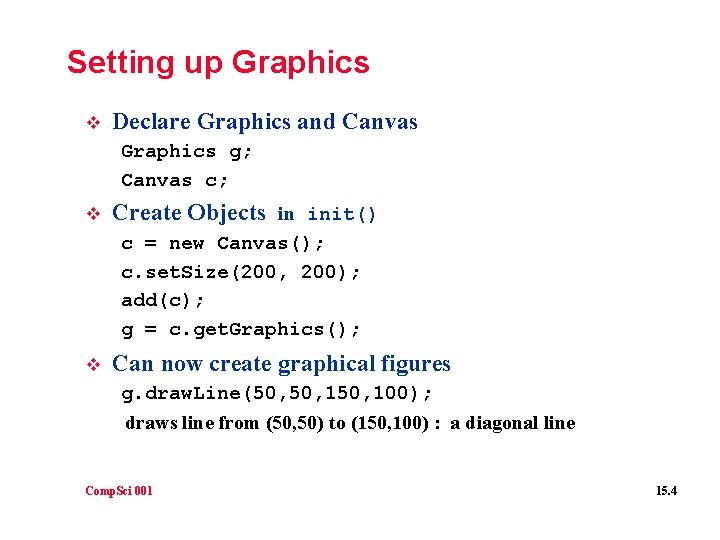
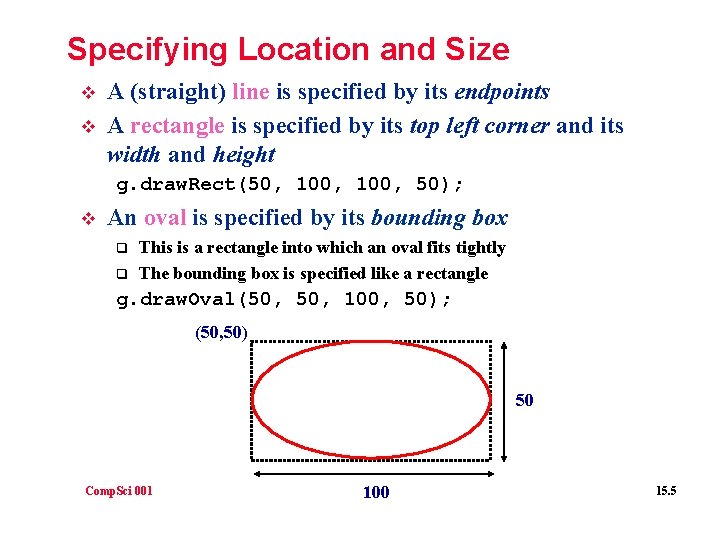
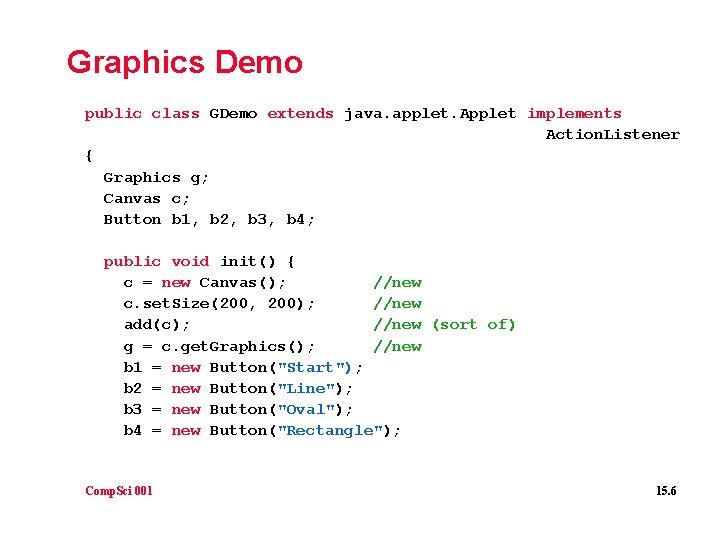
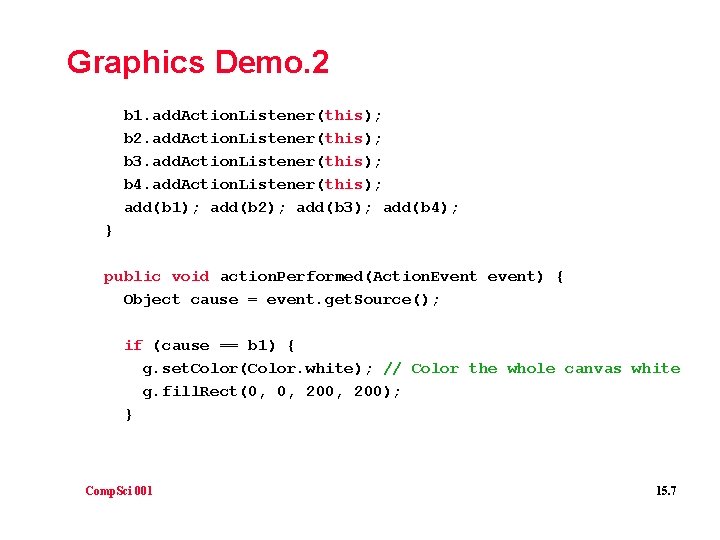
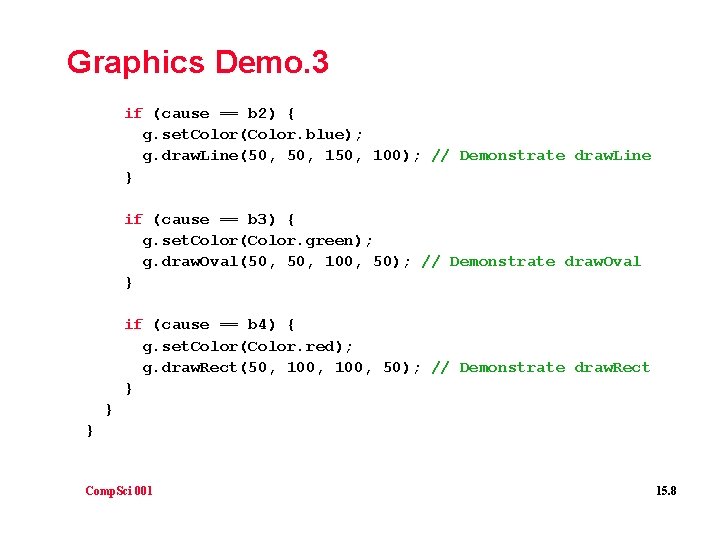
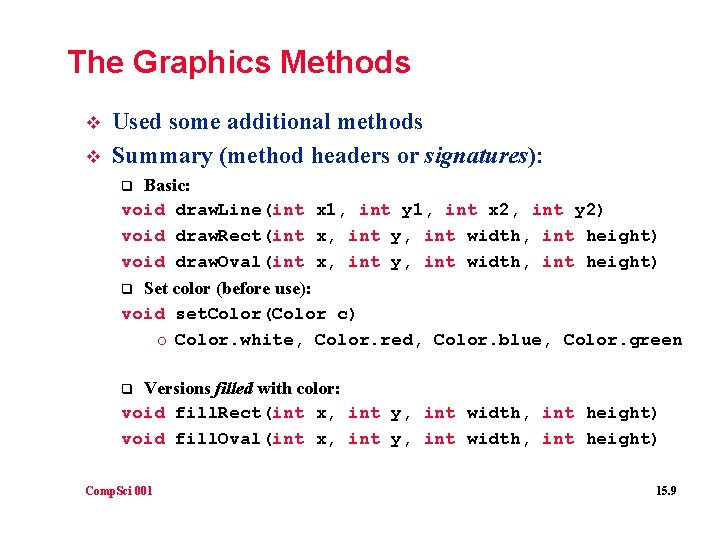
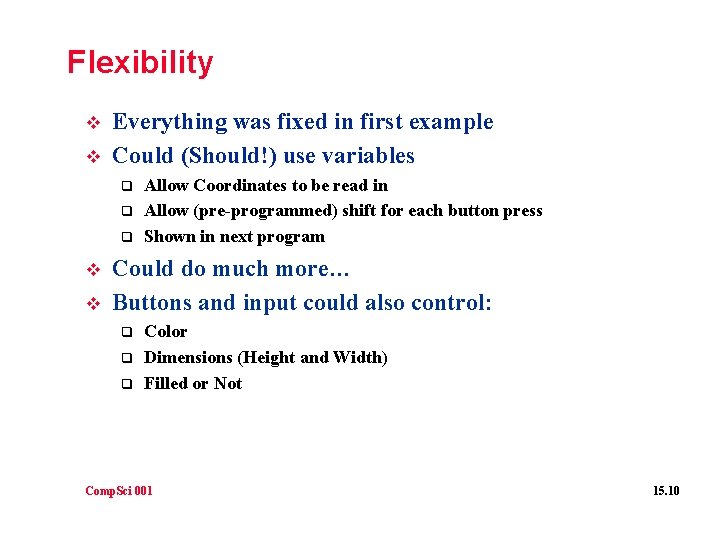
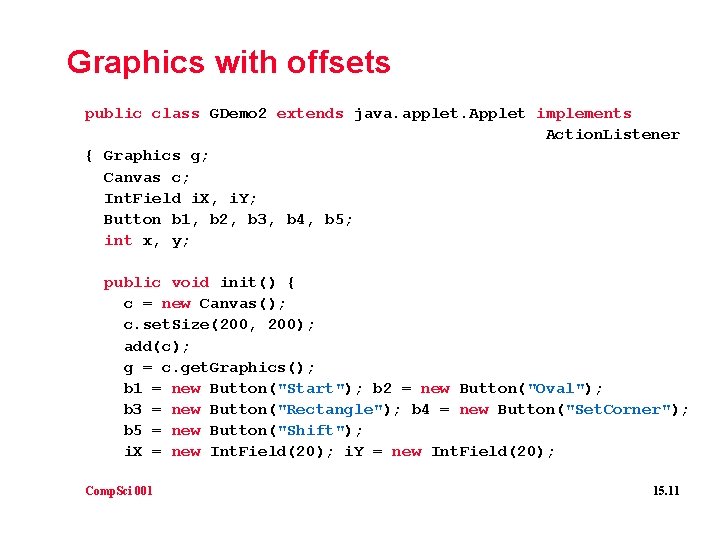
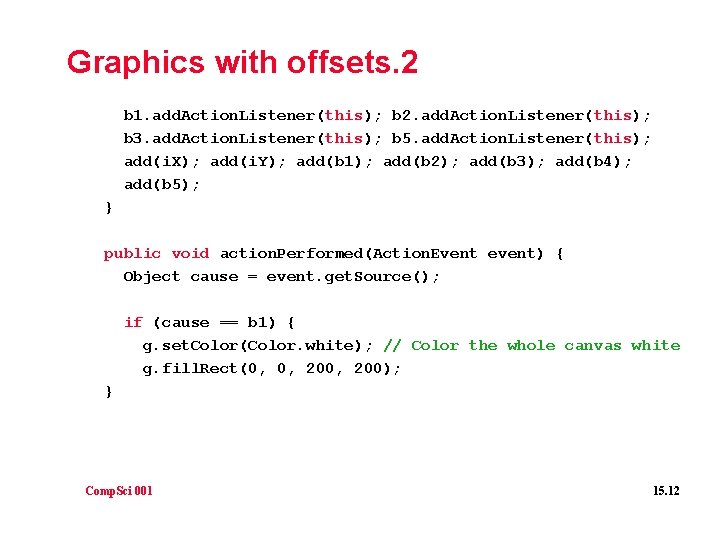
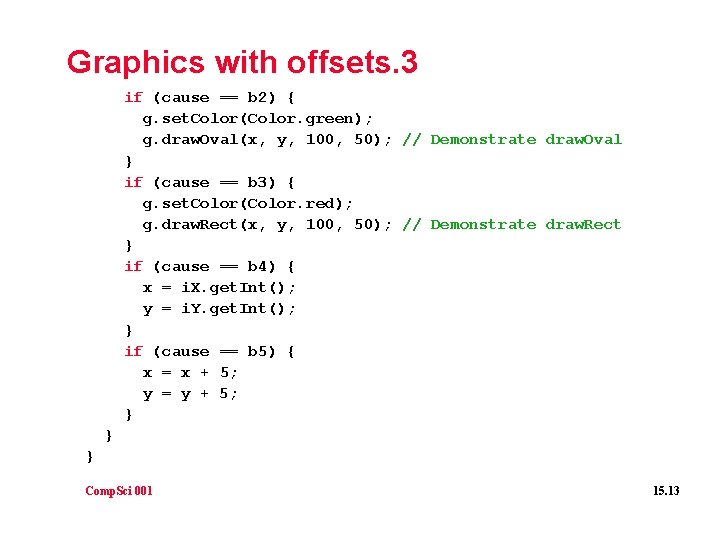
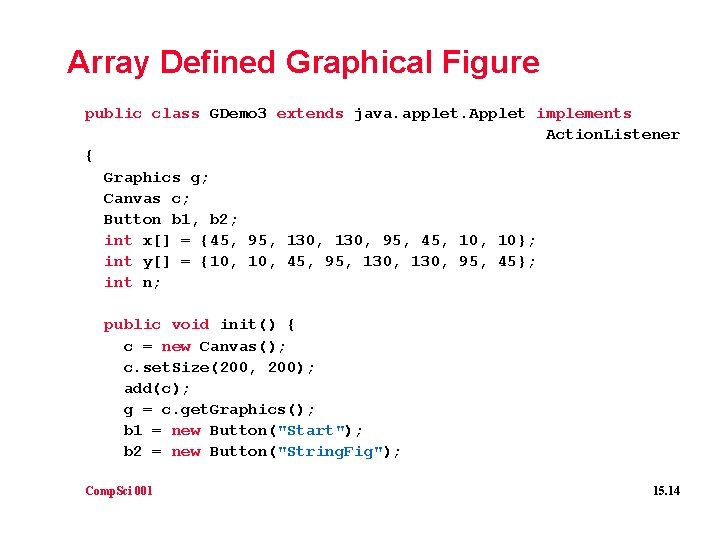
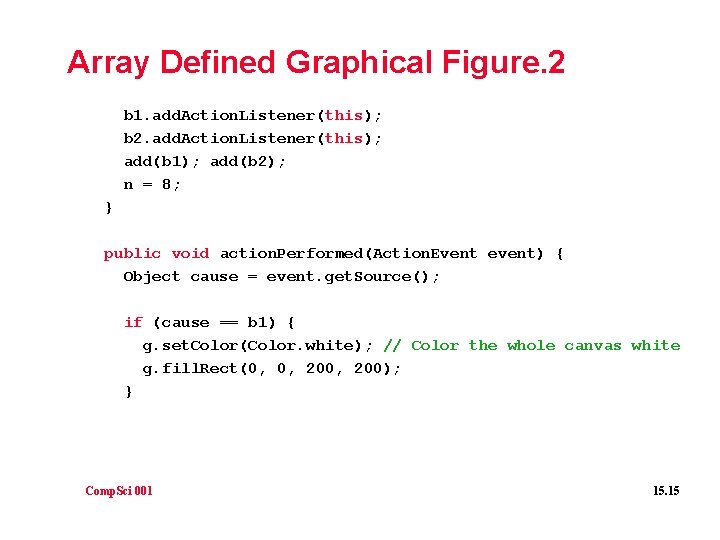
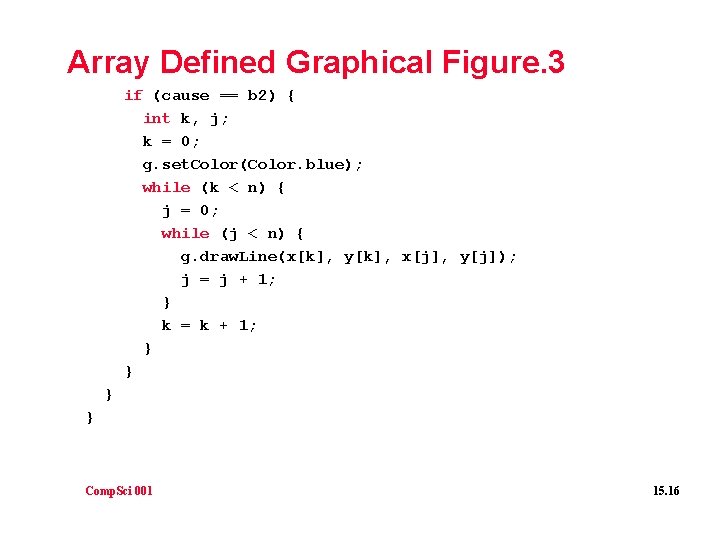
- Slides: 16
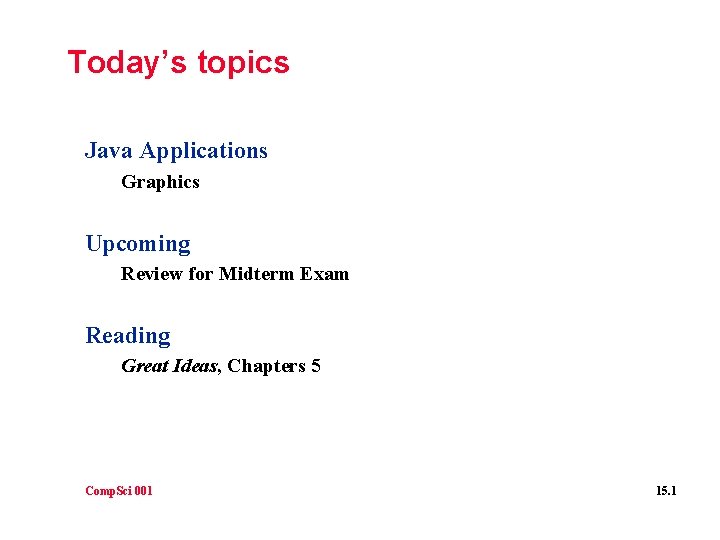
Today’s topics Java Applications Graphics Upcoming Review for Midterm Exam Reading Great Ideas, Chapters 5 Comp. Sci 001 15. 1
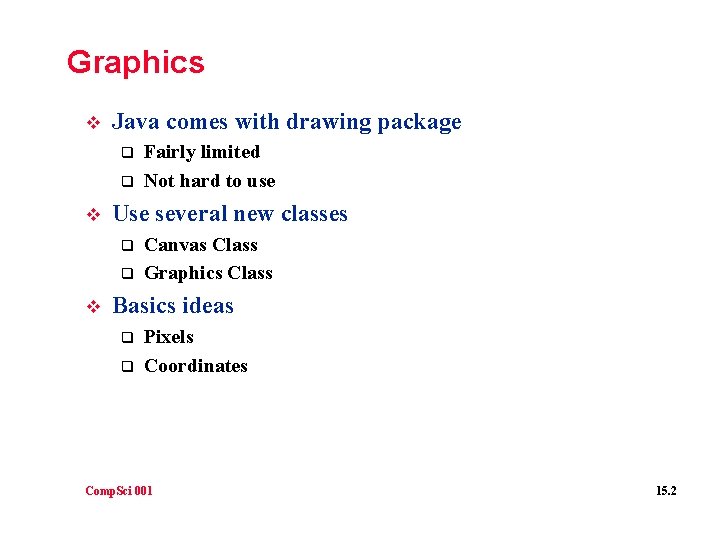
Graphics v Java comes with drawing package q q v Use several new classes q q v Fairly limited Not hard to use Canvas Class Graphics Class Basics ideas q q Pixels Coordinates Comp. Sci 001 15. 2
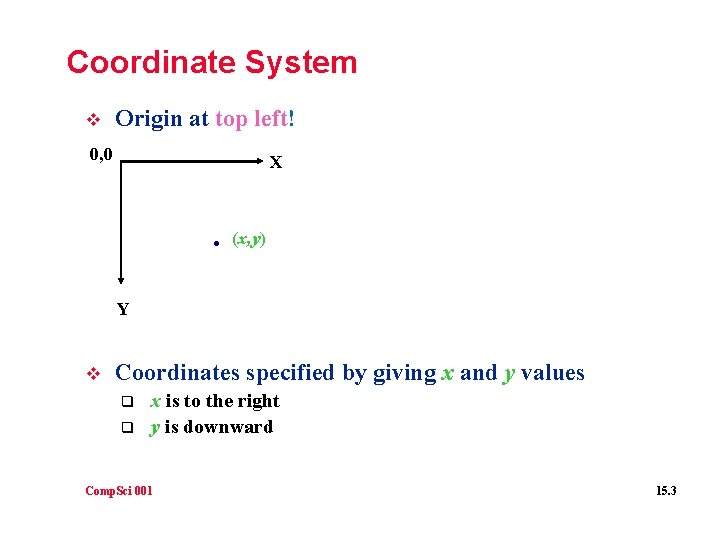
Coordinate System v Origin at top left! 0, 0 X . (x, y) Y v Coordinates specified by giving x and y values q q x is to the right y is downward Comp. Sci 001 15. 3
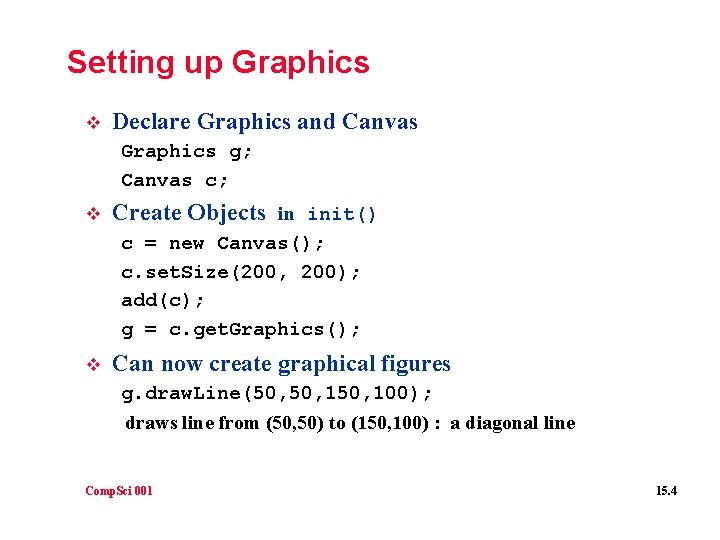
Setting up Graphics v Declare Graphics and Canvas Graphics g; Canvas c; v Create Objects in init() c = new Canvas(); c. set. Size(200, 200); add(c); g = c. get. Graphics(); v Can now create graphical figures g. draw. Line(50, 150, 100); draws line from (50, 50) to (150, 100) : a diagonal line Comp. Sci 001 15. 4
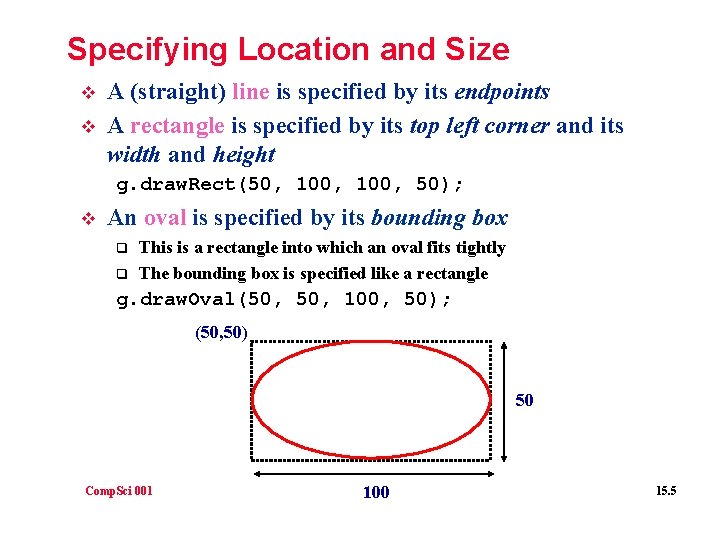
Specifying Location and Size v v A (straight) line is specified by its endpoints A rectangle is specified by its top left corner and its width and height g. draw. Rect(50, 100, 50); v An oval is specified by its bounding box q q This is a rectangle into which an oval fits tightly The bounding box is specified like a rectangle g. draw. Oval(50, 100, 50); (50, 50) 50 Comp. Sci 001 100 15. 5
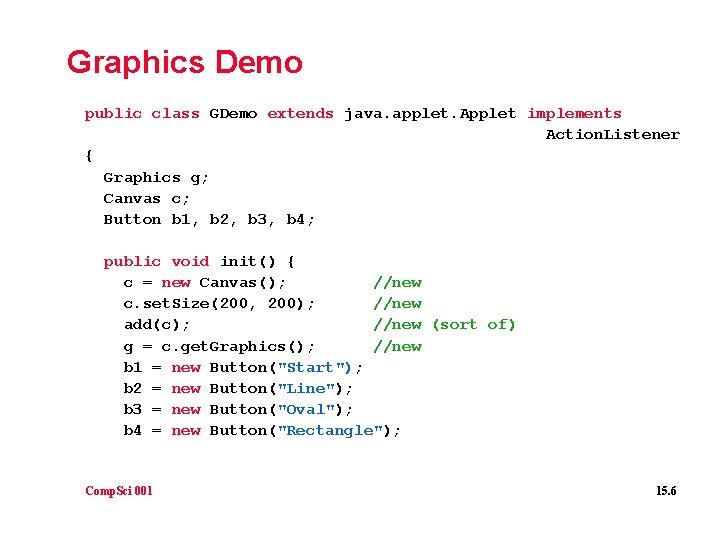
Graphics Demo public class GDemo extends java. applet. Applet implements Action. Listener { Graphics g; Canvas c; Button b 1, b 2, b 3, b 4; public void init() { c = new Canvas(); //new c. set. Size(200, 200); //new add(c); //new (sort of) g = c. get. Graphics(); //new b 1 = new Button("Start"); b 2 = new Button("Line"); b 3 = new Button("Oval"); b 4 = new Button("Rectangle"); Comp. Sci 001 15. 6
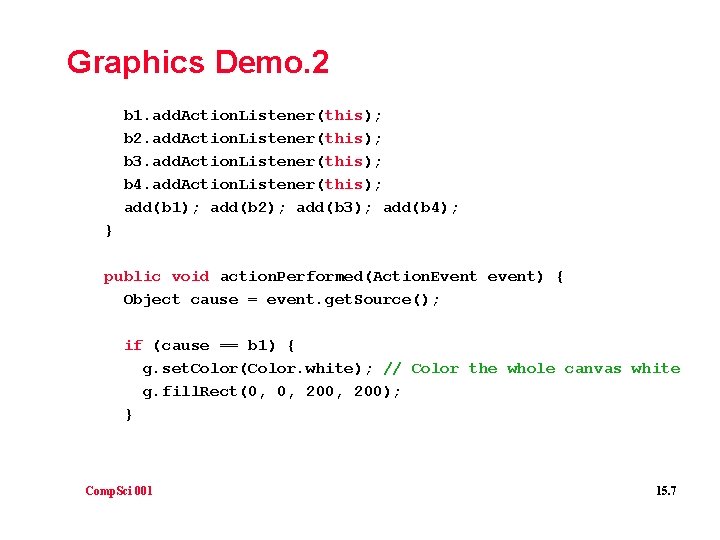
Graphics Demo. 2 b 1. add. Action. Listener(this); b 2. add. Action. Listener(this); b 3. add. Action. Listener(this); b 4. add. Action. Listener(this); add(b 1); add(b 2); add(b 3); add(b 4); } public void action. Performed(Action. Event event) { Object cause = event. get. Source(); if (cause == b 1) { g. set. Color(Color. white); // Color the whole canvas white g. fill. Rect(0, 0, 200); } Comp. Sci 001 15. 7
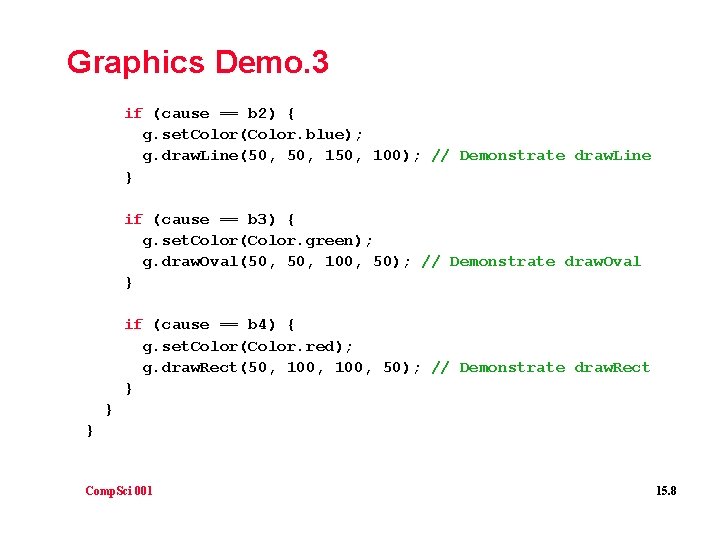
Graphics Demo. 3 if (cause == b 2) { g. set. Color(Color. blue); g. draw. Line(50, 150, 100); // Demonstrate draw. Line } if (cause == b 3) { g. set. Color(Color. green); g. draw. Oval(50, 100, 50); // Demonstrate draw. Oval } if (cause == b 4) { g. set. Color(Color. red); g. draw. Rect(50, 100, 50); // Demonstrate draw. Rect } } } Comp. Sci 001 15. 8
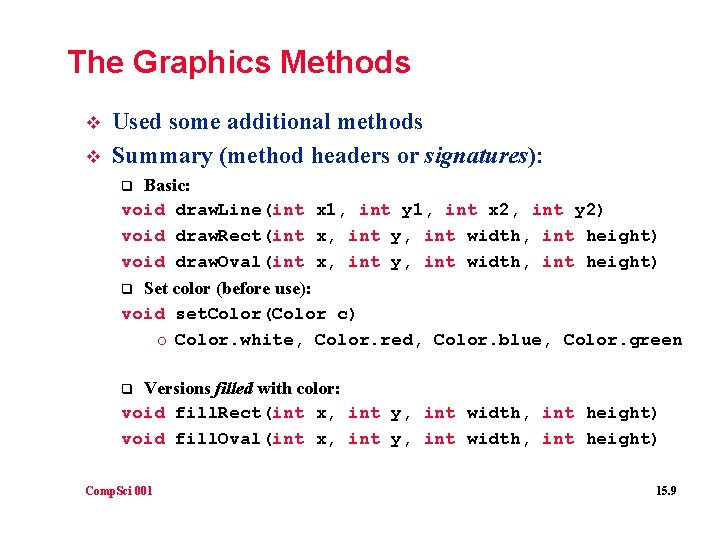
The Graphics Methods v v Used some additional methods Summary (method headers or signatures): Basic: void draw. Line(int x 1, int y 1, int x 2, int y 2) void draw. Rect(int x, int y, int width, int height) void draw. Oval(int x, int y, int width, int height) q Set color (before use): void set. Color(Color c) o Color. white, Color. red, Color. blue, Color. green q Versions filled with color: void fill. Rect(int x, int y, int width, int height) void fill. Oval(int x, int y, int width, int height) q Comp. Sci 001 15. 9
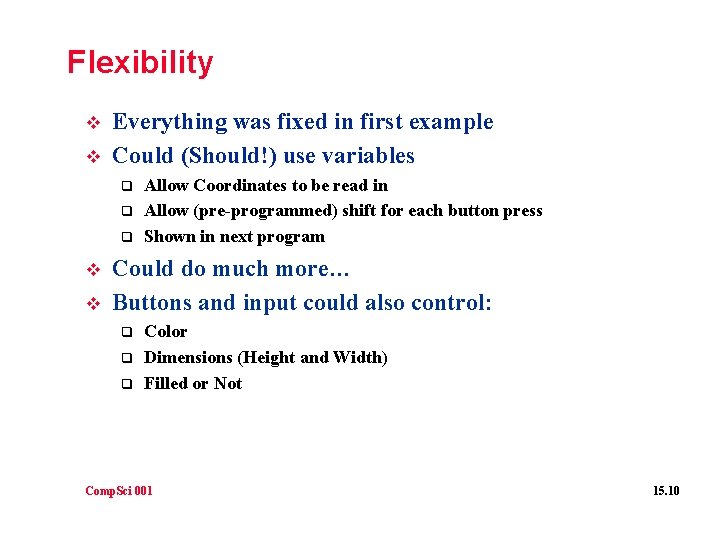
Flexibility v v Everything was fixed in first example Could (Should!) use variables q q q v v Allow Coordinates to be read in Allow (pre-programmed) shift for each button press Shown in next program Could do much more… Buttons and input could also control: q q q Color Dimensions (Height and Width) Filled or Not Comp. Sci 001 15. 10
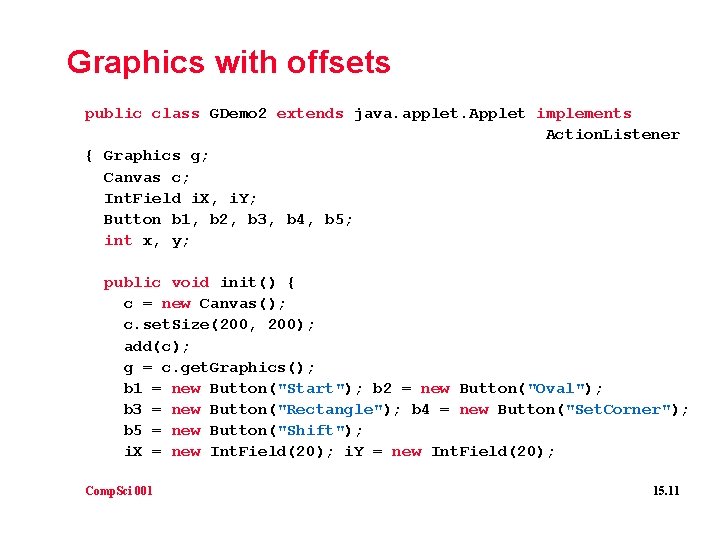
Graphics with offsets public class GDemo 2 extends java. applet. Applet implements Action. Listener { Graphics g; Canvas c; Int. Field i. X, i. Y; Button b 1, b 2, b 3, b 4, b 5; int x, y; public void init() { c = new Canvas(); c. set. Size(200, 200); add(c); g = c. get. Graphics(); b 1 = new Button("Start"); b 2 = new Button("Oval"); b 3 = new Button("Rectangle"); b 4 = new Button("Set. Corner"); b 5 = new Button("Shift"); i. X = new Int. Field(20); i. Y = new Int. Field(20); Comp. Sci 001 15. 11
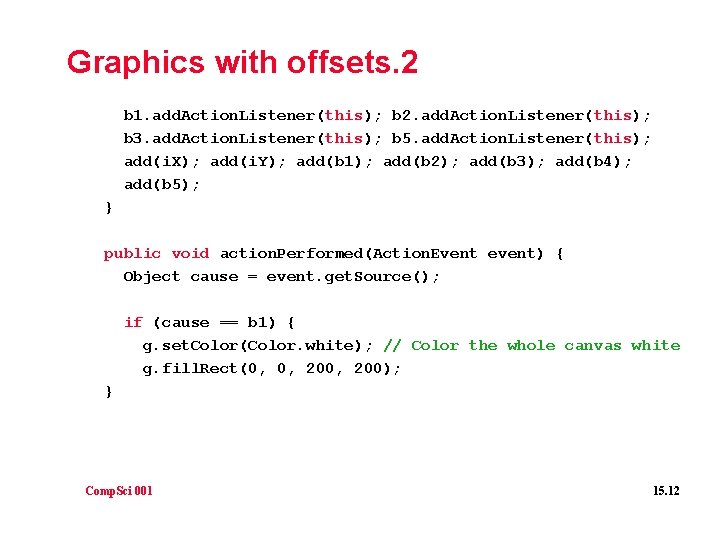
Graphics with offsets. 2 b 1. add. Action. Listener(this); b 2. add. Action. Listener(this); b 3. add. Action. Listener(this); b 5. add. Action. Listener(this); add(i. X); add(i. Y); add(b 1); add(b 2); add(b 3); add(b 4); add(b 5); } public void action. Performed(Action. Event event) { Object cause = event. get. Source(); if (cause == b 1) { g. set. Color(Color. white); // Color the whole canvas white g. fill. Rect(0, 0, 200); } Comp. Sci 001 15. 12
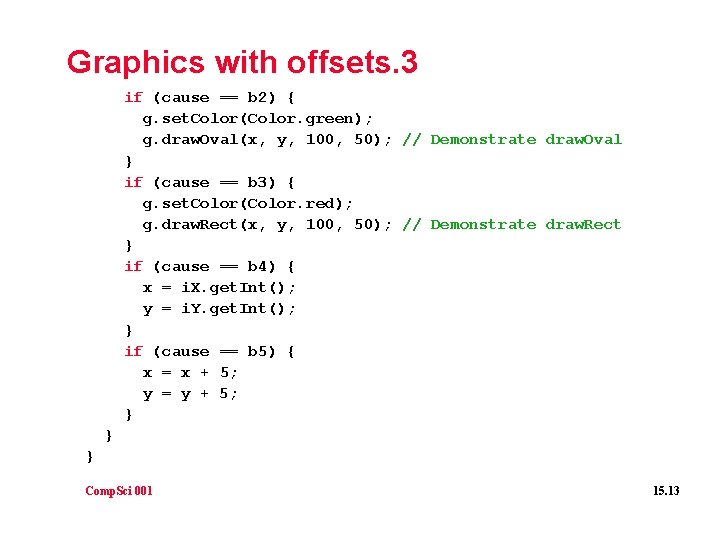
Graphics with offsets. 3 if (cause == b 2) { g. set. Color(Color. green); g. draw. Oval(x, y, 100, 50); // Demonstrate draw. Oval } if (cause == b 3) { g. set. Color(Color. red); g. draw. Rect(x, y, 100, 50); // Demonstrate draw. Rect } if (cause == b 4) { x = i. X. get. Int(); y = i. Y. get. Int(); } if (cause == b 5) { x = x + 5; y = y + 5; } } } Comp. Sci 001 15. 13
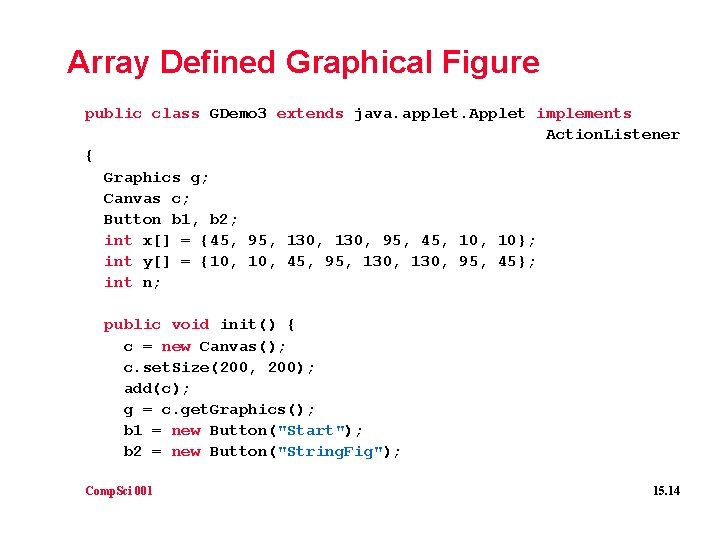
Array Defined Graphical Figure public class GDemo 3 extends java. applet. Applet implements Action. Listener { Graphics g; Canvas c; Button b 1, b 2; int x[] = {45, 95, 130, 95, 45, 10}; int y[] = {10, 45, 95, 130, 95, 45}; int n; public void init() { c = new Canvas(); c. set. Size(200, 200); add(c); g = c. get. Graphics(); b 1 = new Button("Start"); b 2 = new Button("String. Fig"); Comp. Sci 001 15. 14
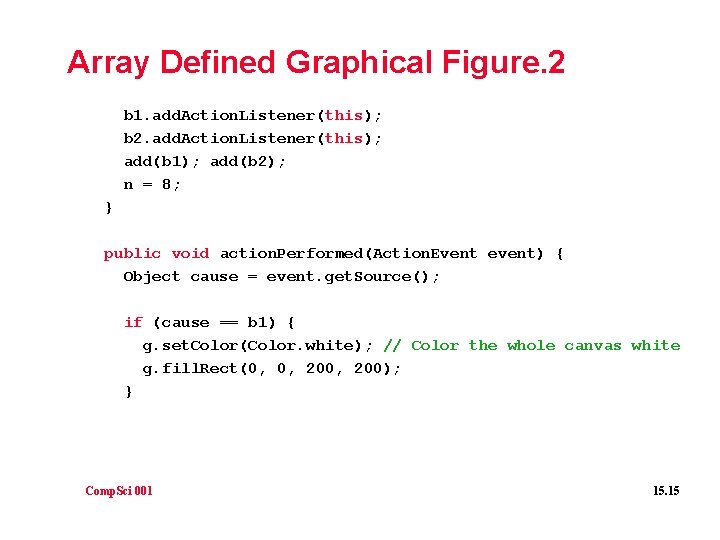
Array Defined Graphical Figure. 2 b 1. add. Action. Listener(this); b 2. add. Action. Listener(this); add(b 1); add(b 2); n = 8; } public void action. Performed(Action. Event event) { Object cause = event. get. Source(); if (cause == b 1) { g. set. Color(Color. white); // Color the whole canvas white g. fill. Rect(0, 0, 200); } Comp. Sci 001 15. 15
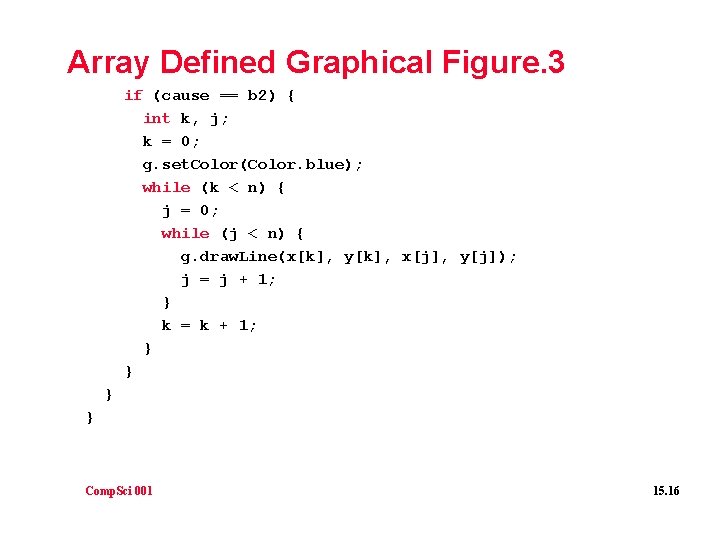
Array Defined Graphical Figure. 3 if (cause == b 2) { int k, j; k = 0; g. set. Color(Color. blue); while (k < n) { j = 0; while (j < n) { g. draw. Line(x[k], y[k], x[j], y[j]); j = j + 1; } k = k + 1; } } Comp. Sci 001 15. 16
Hand held computer
3d viewing devices in computer graphics ppt
Java graphics
Java graphics
Java graphics
Java graphics animation
Java graphics
Java graphics
Java graphics
Java swing graphics
Distributed applications in java
Lexical issues in java with example
Generations
We have class today
Multiple choice comma quiz
Todays worldld
Whats thermal energy