Tirgul 9 Exceptions Java Sockets Exceptions Java uses
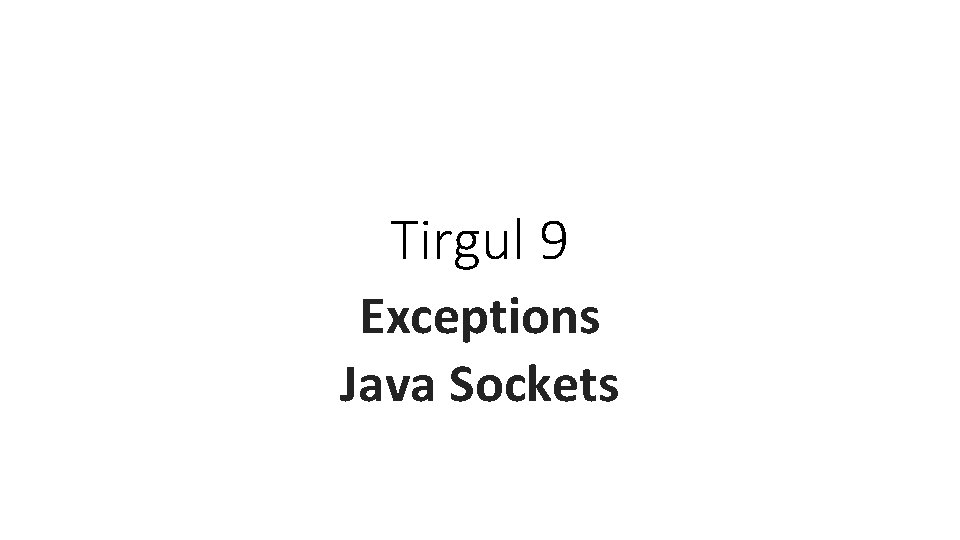
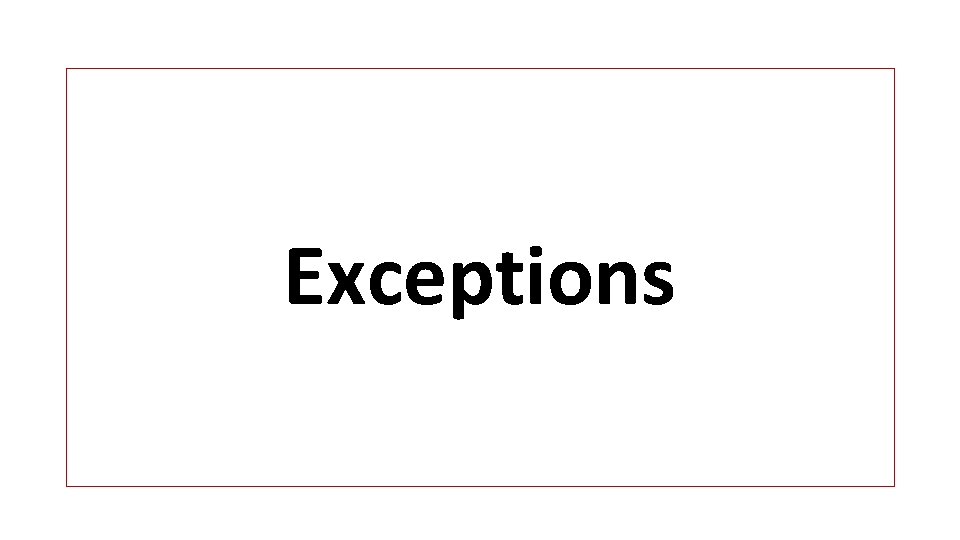
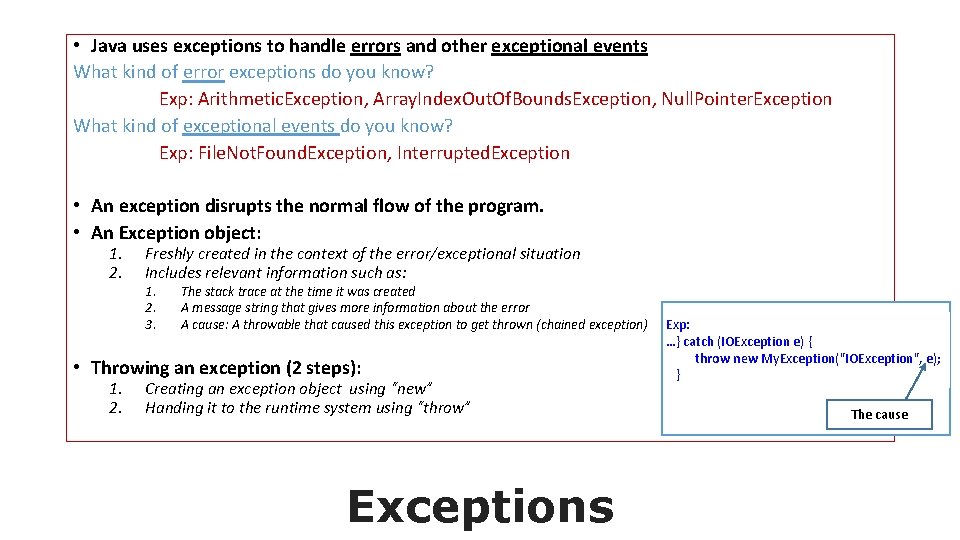
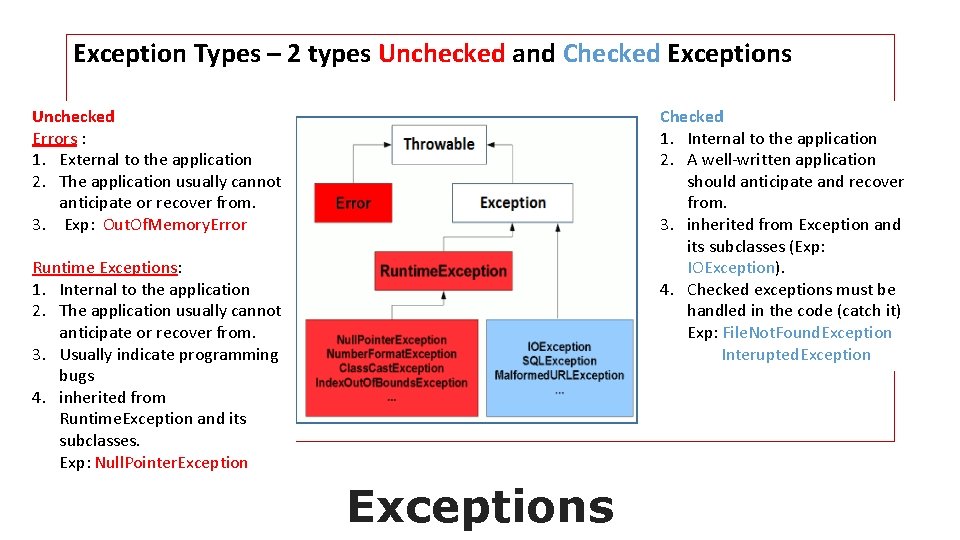
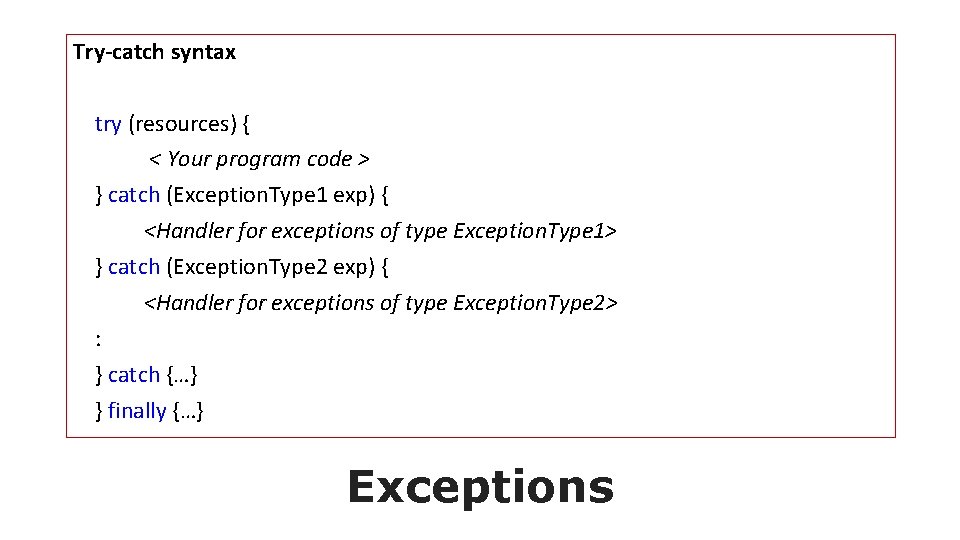
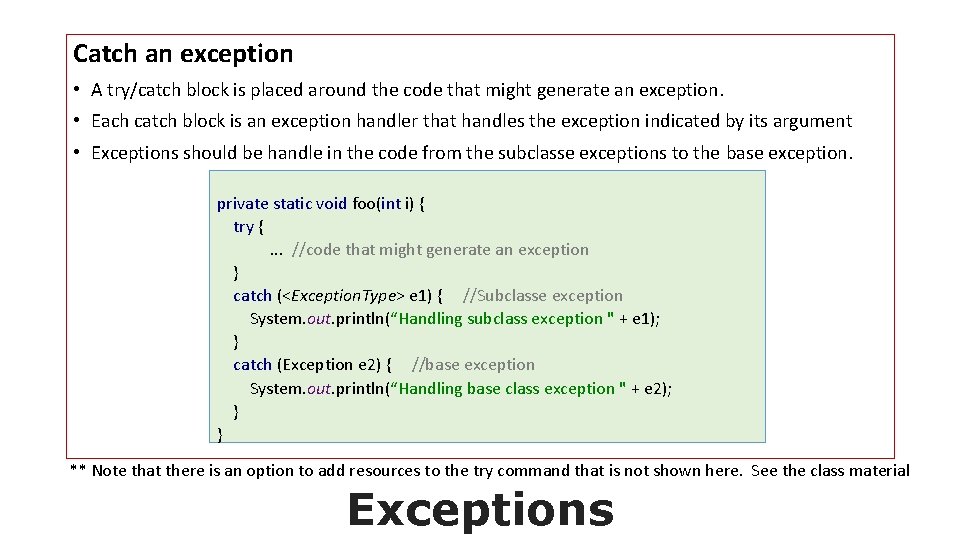
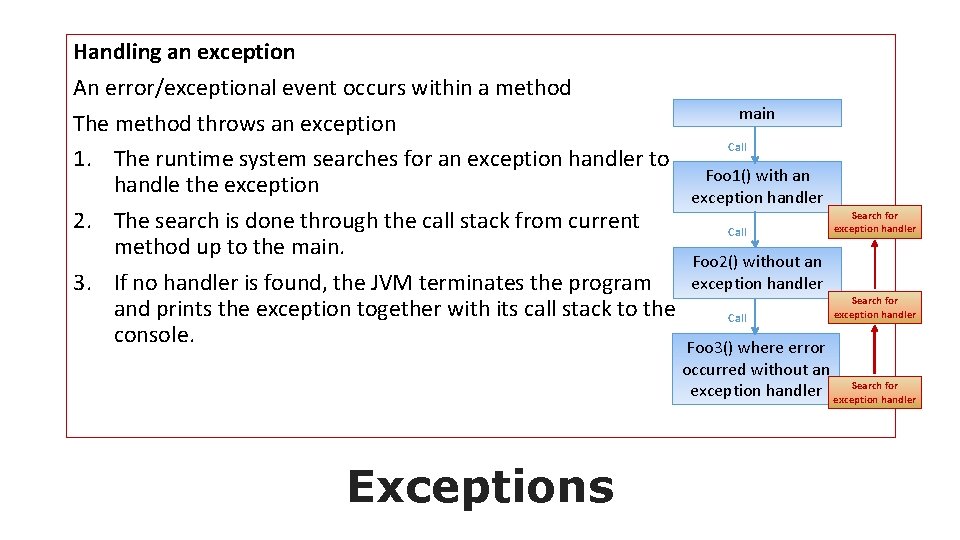
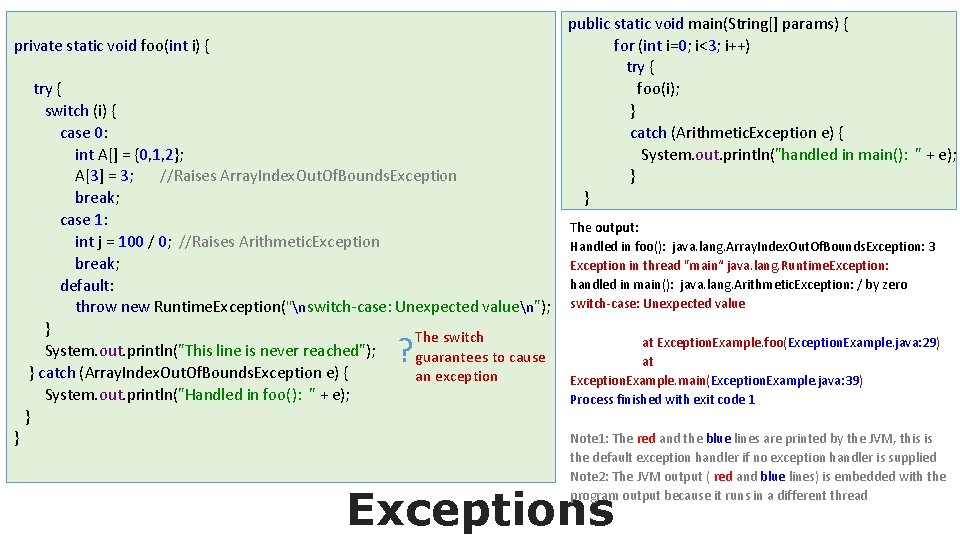
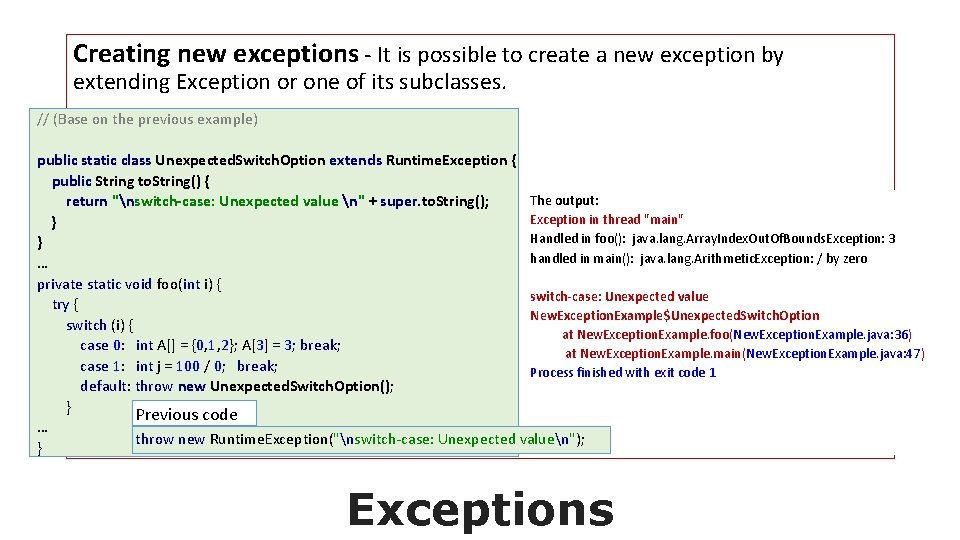
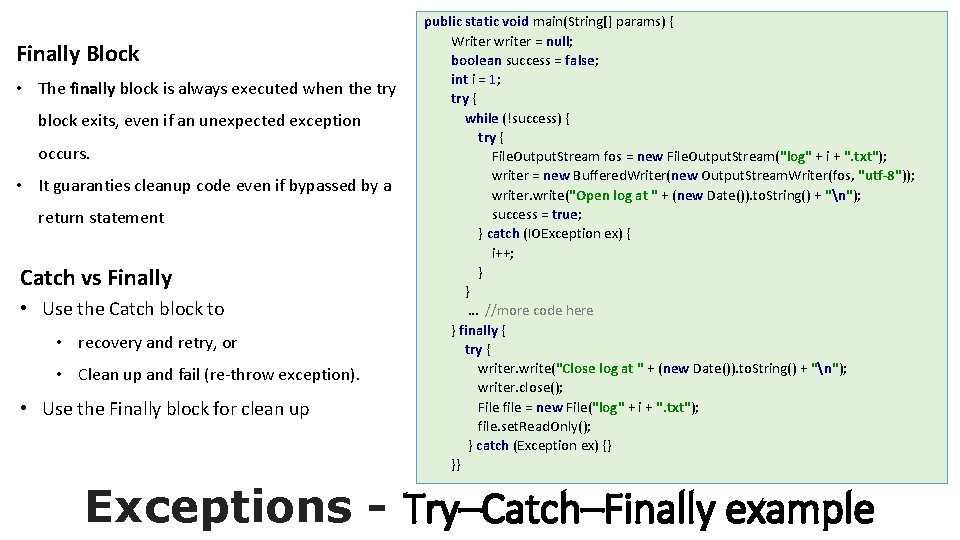
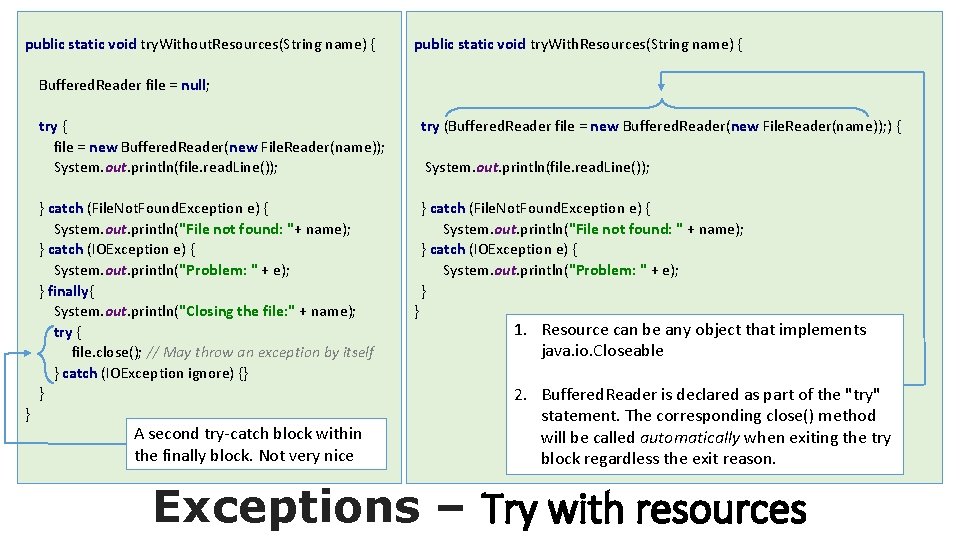
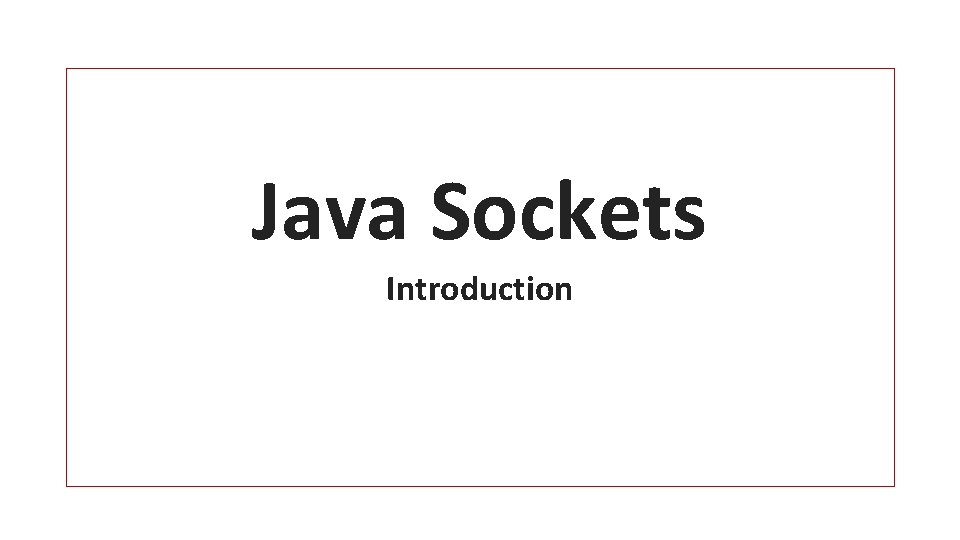
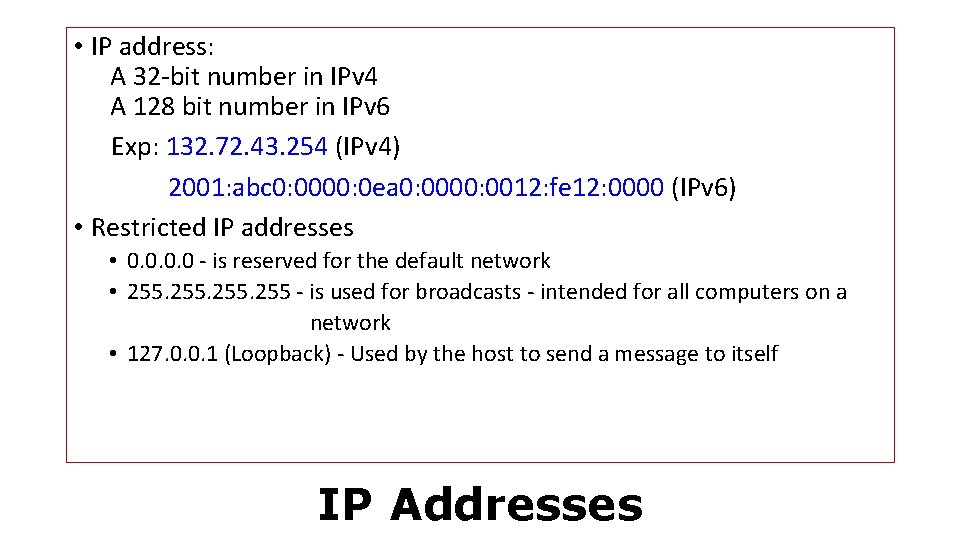
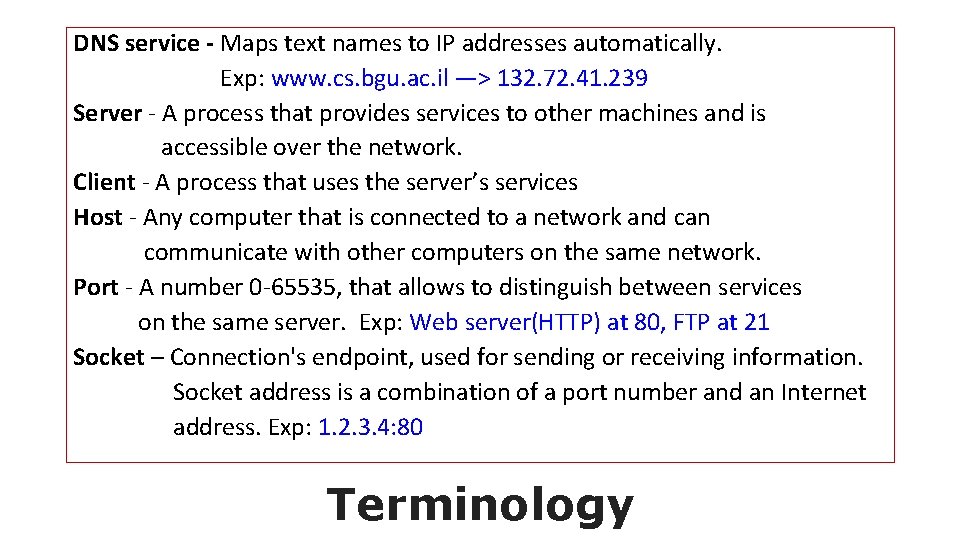
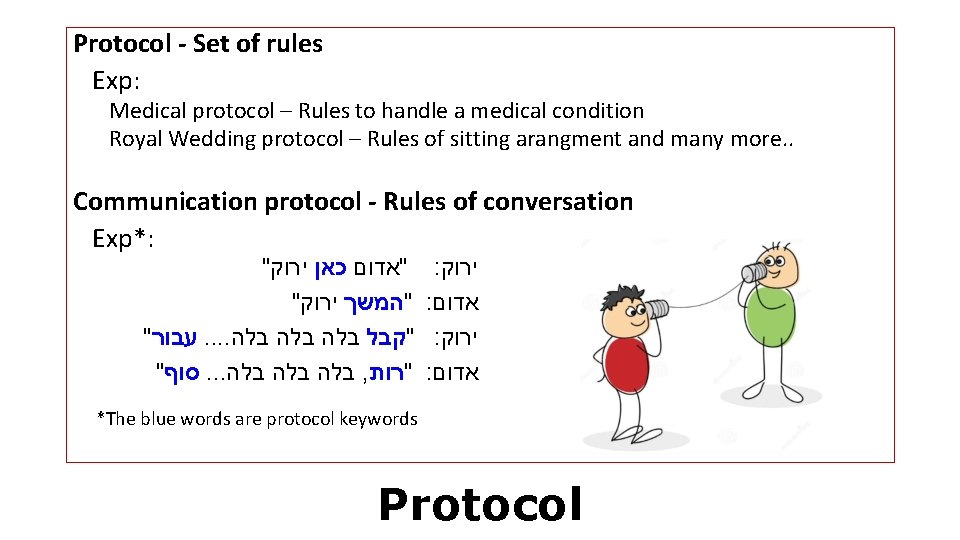
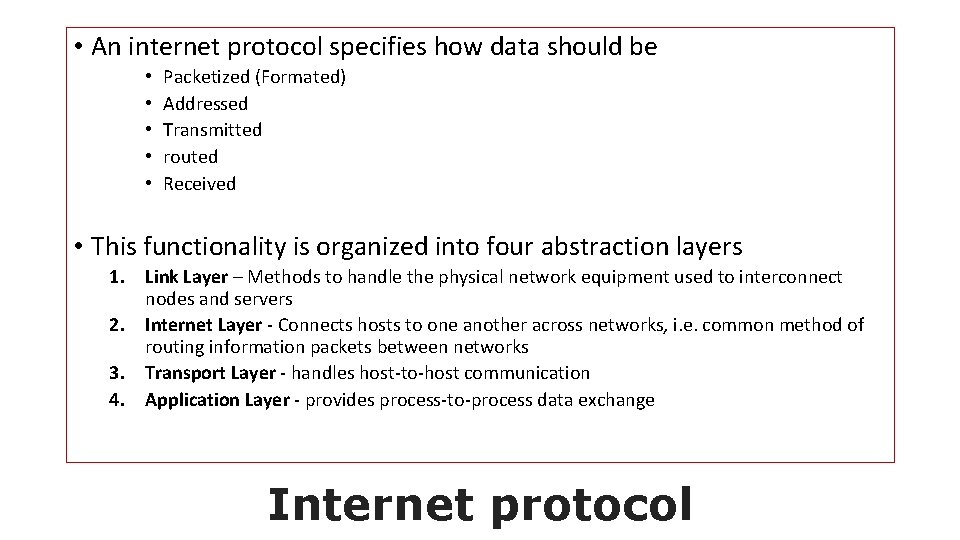
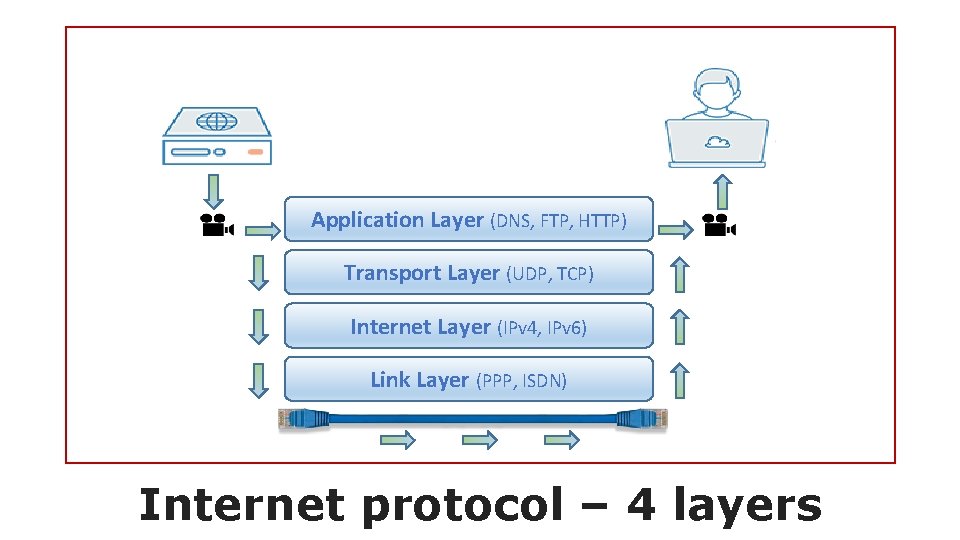
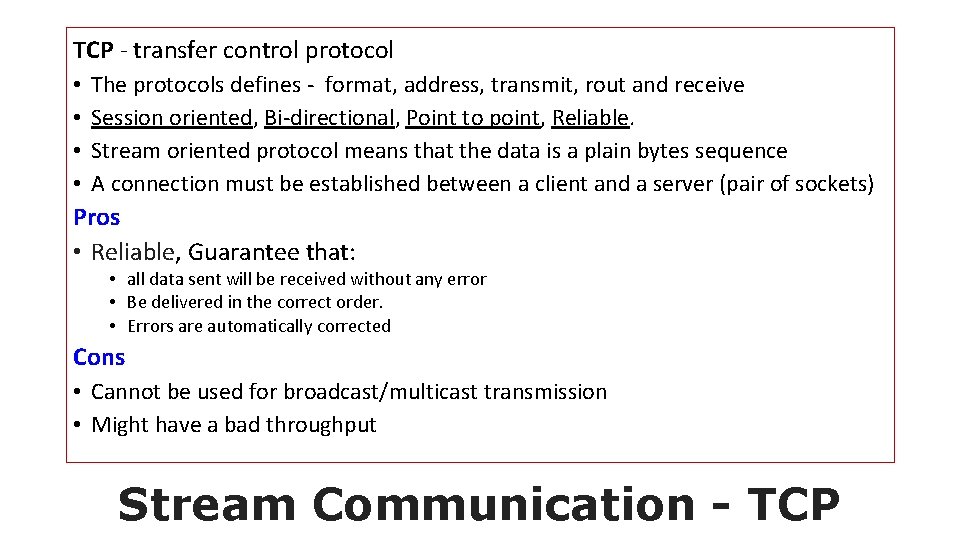
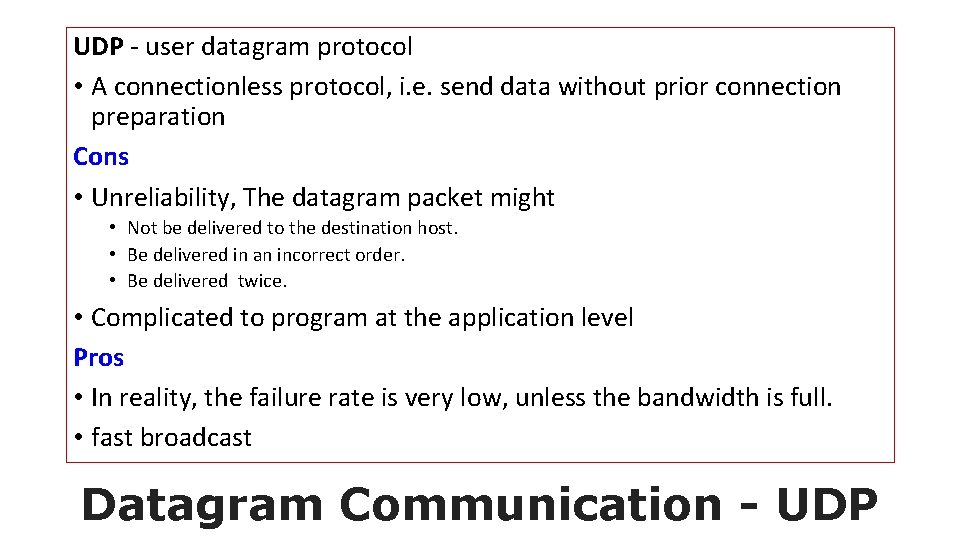
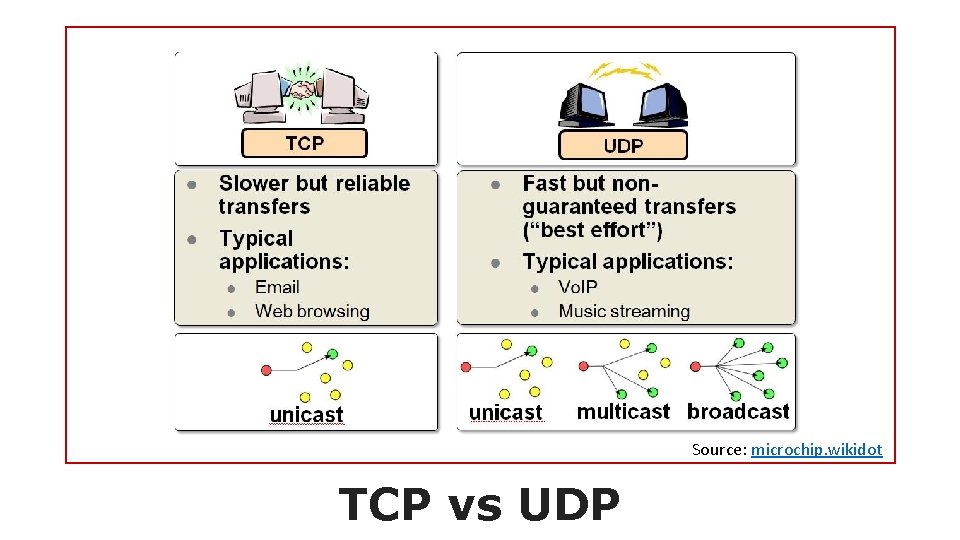
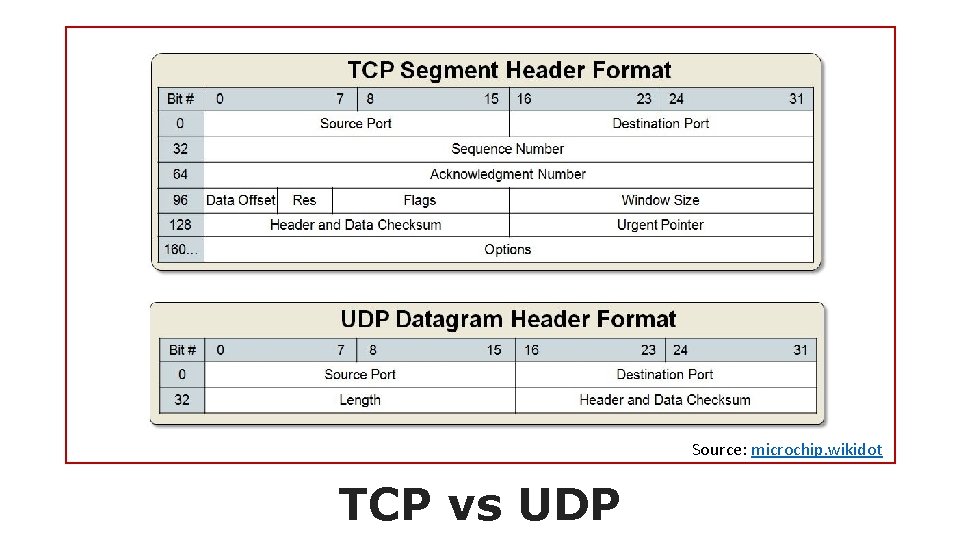
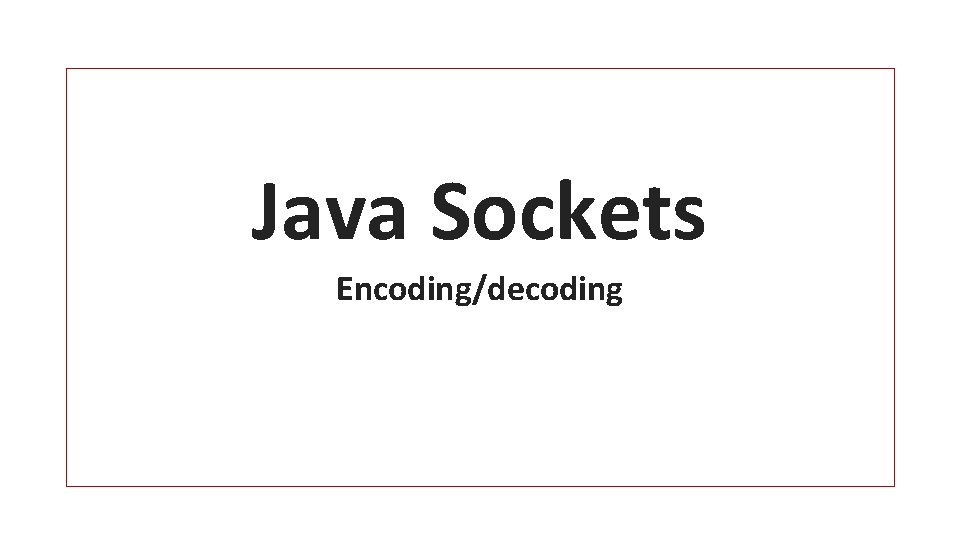
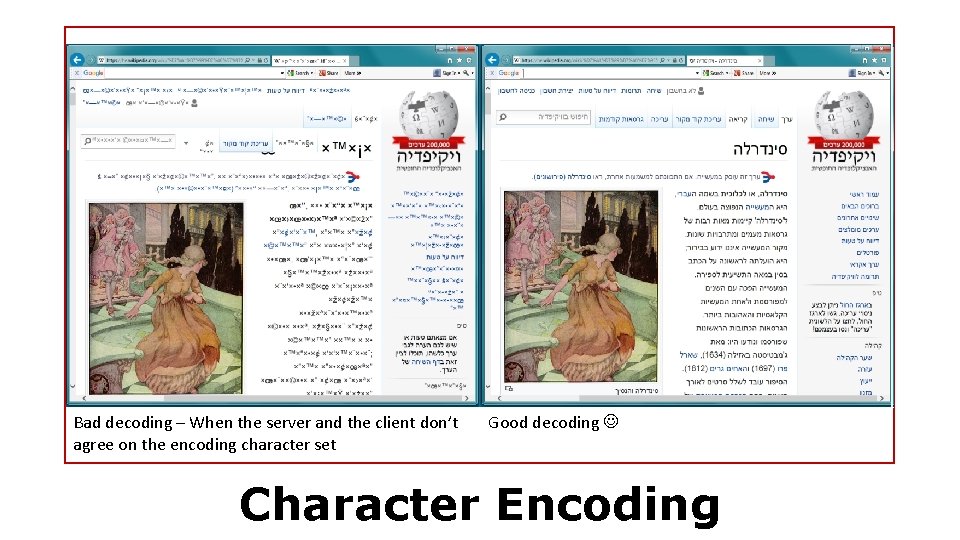
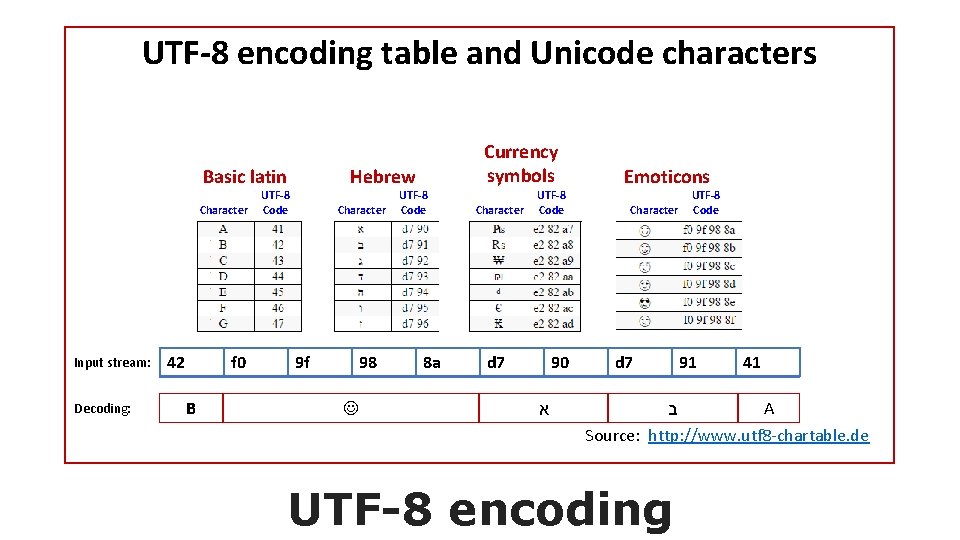
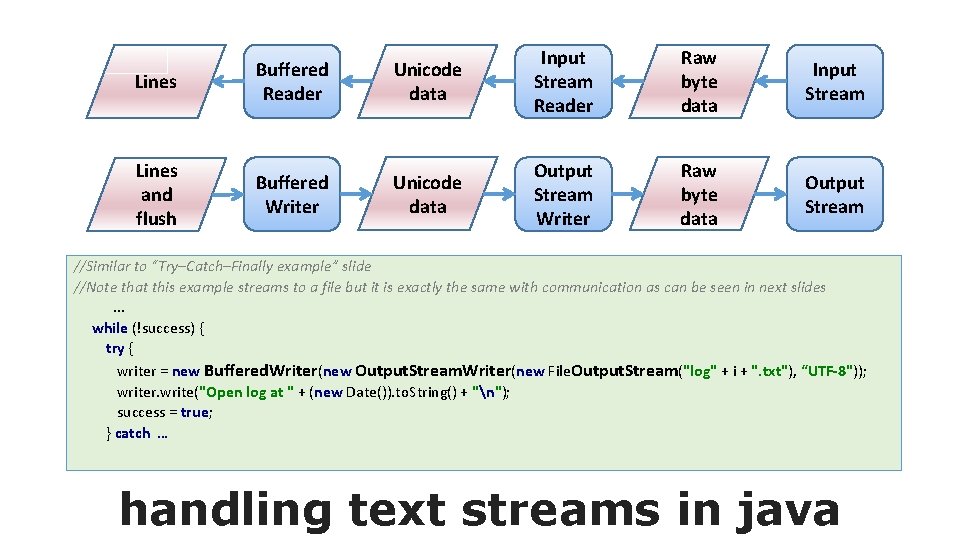
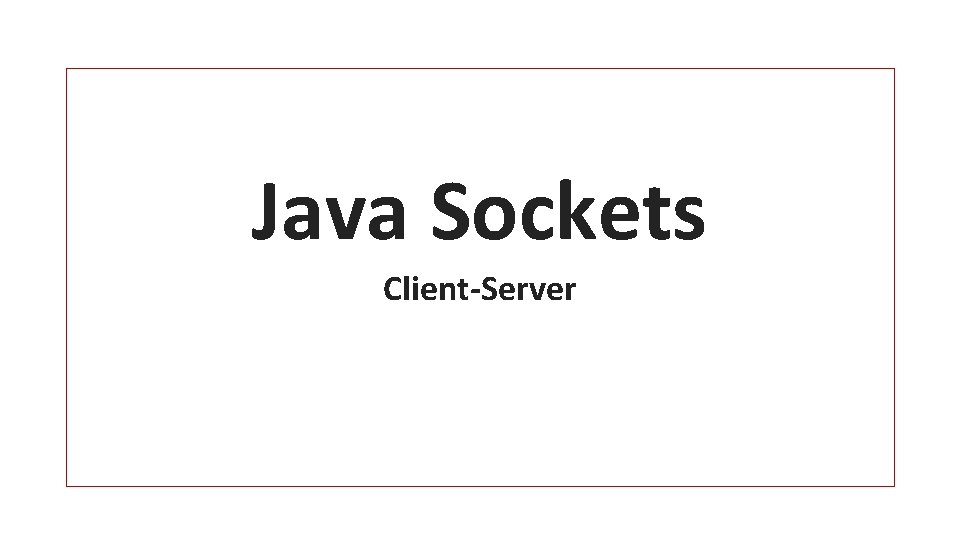
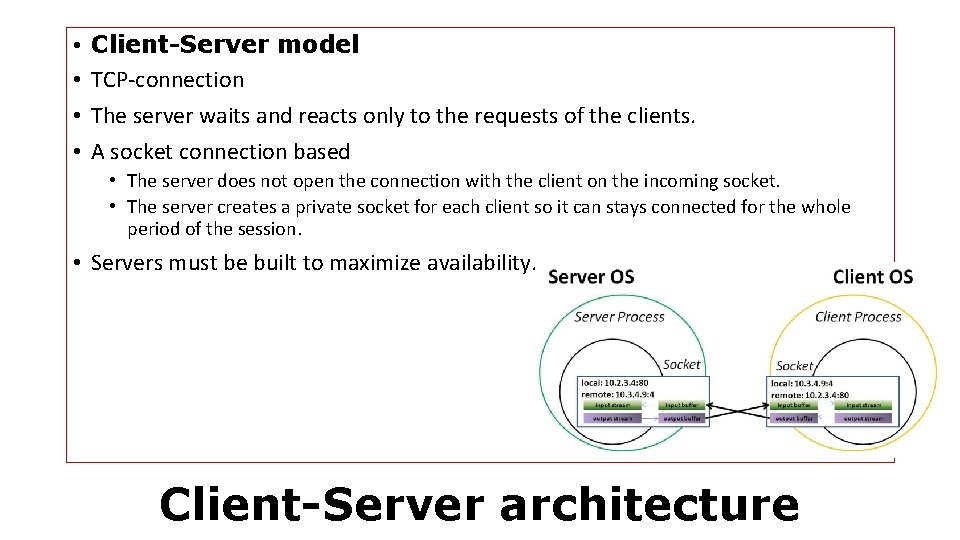
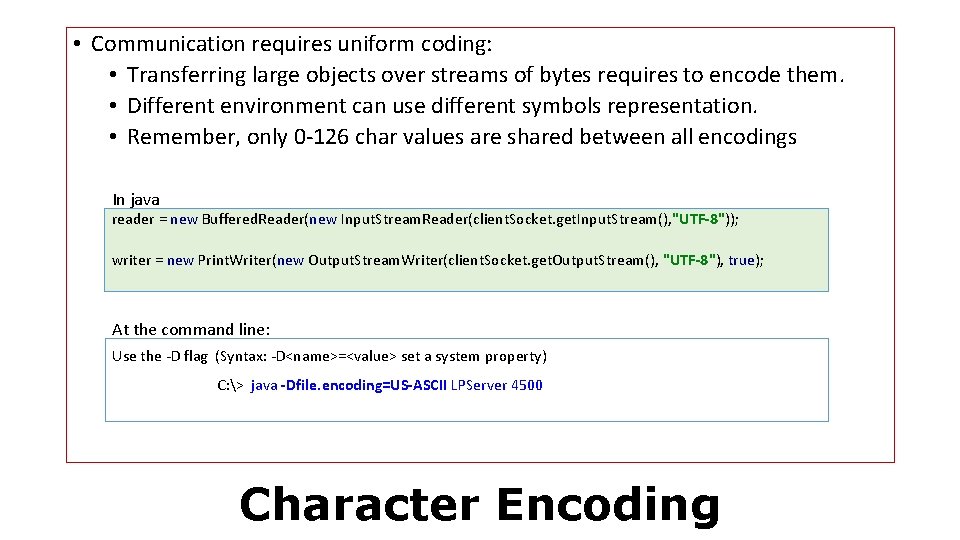
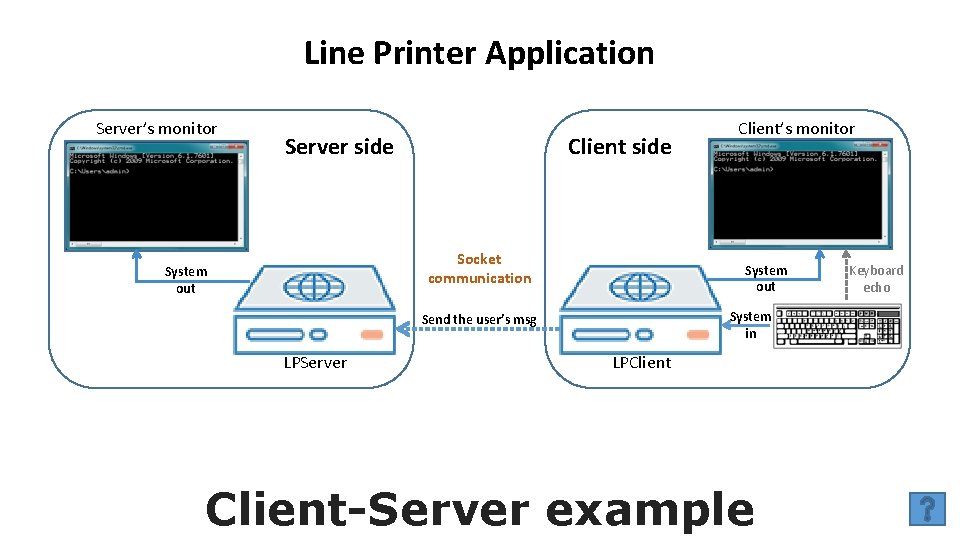
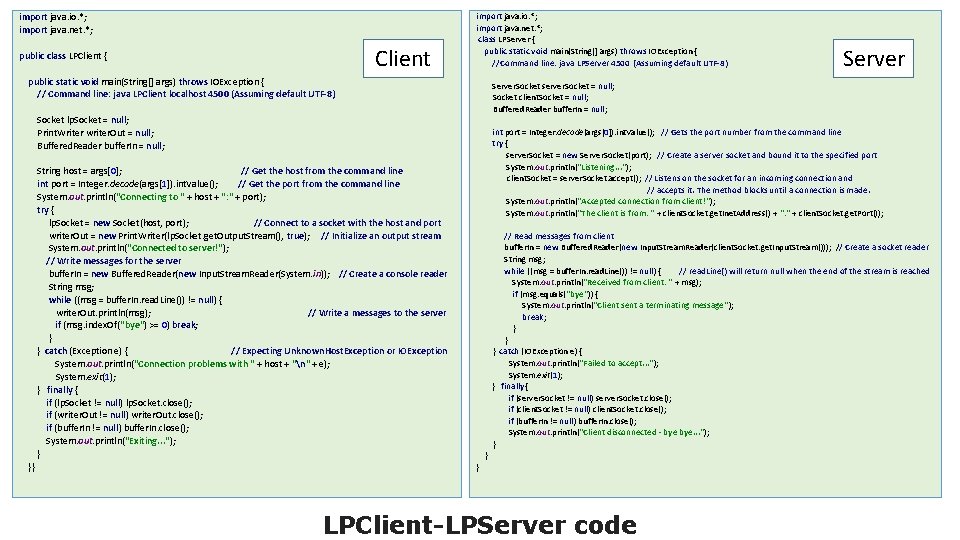
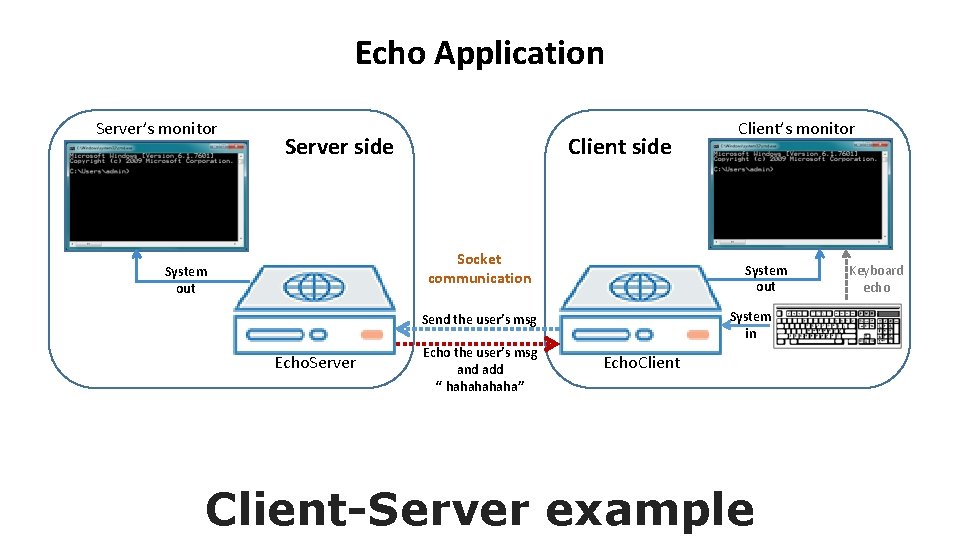
![public class Echo. Client { public static void main(String[] args) throws IOException { /* public class Echo. Client { public static void main(String[] args) throws IOException { /*](https://slidetodoc.com/presentation_image_h2/58f4fff8111cc83418332d0c4ba15468/image-32.jpg)
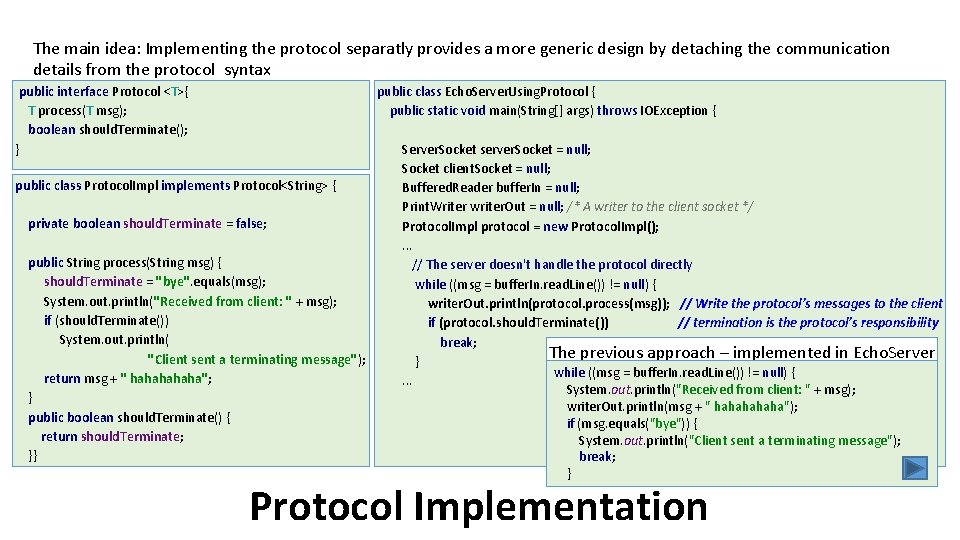
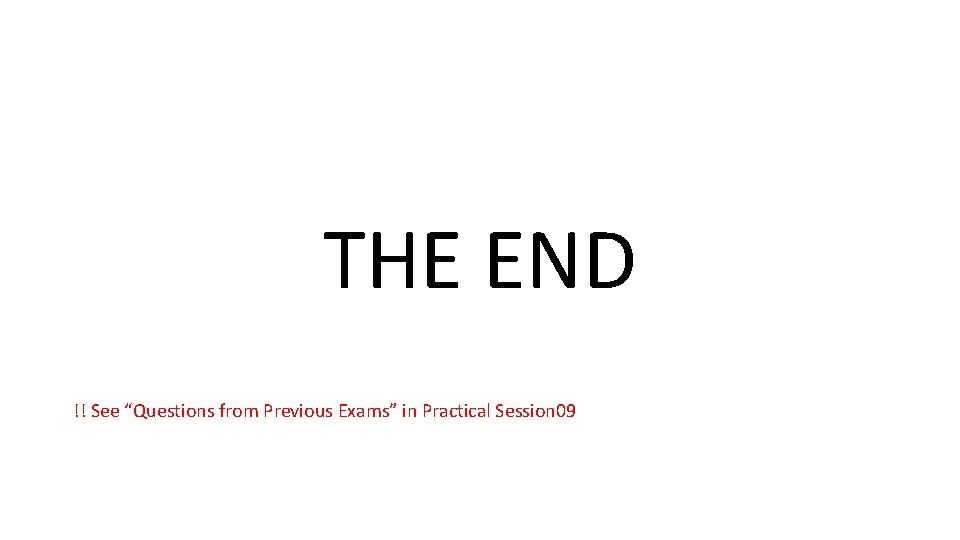
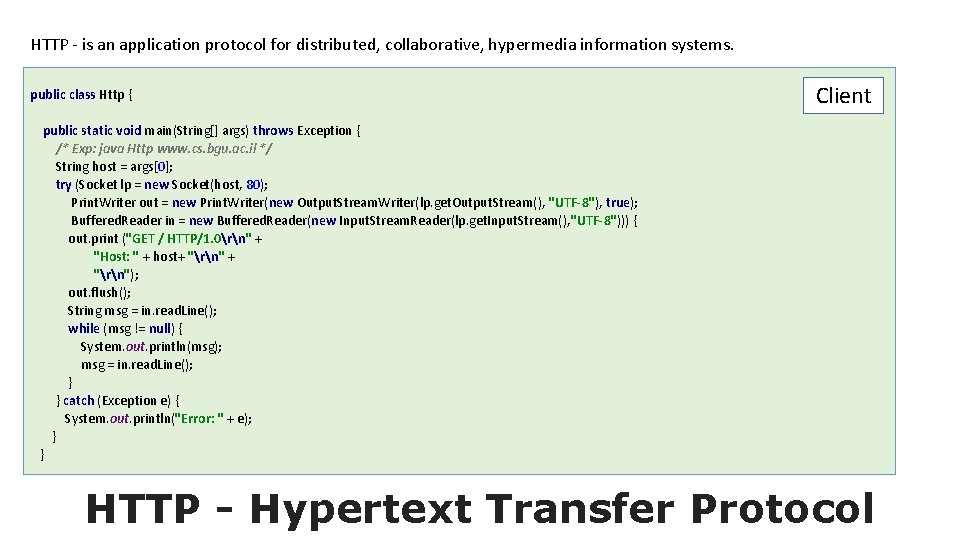
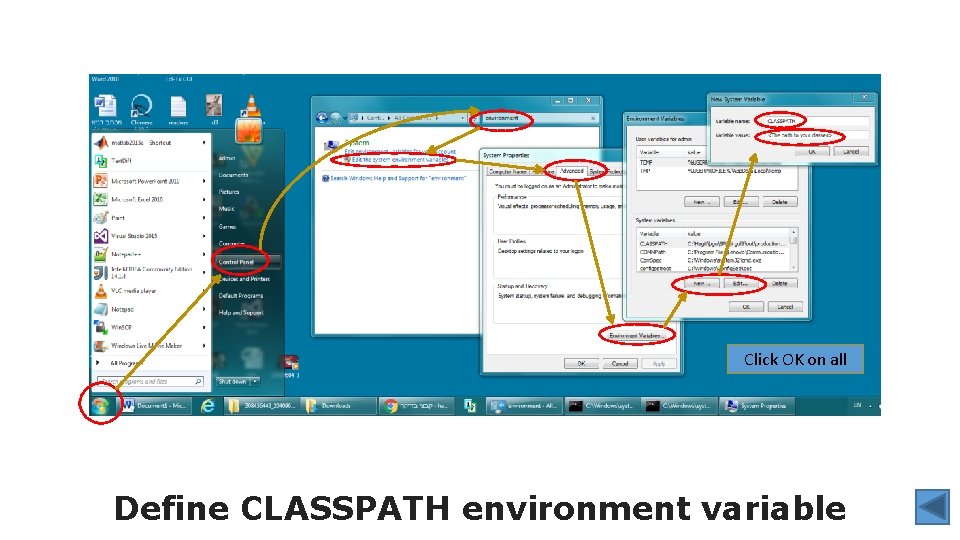
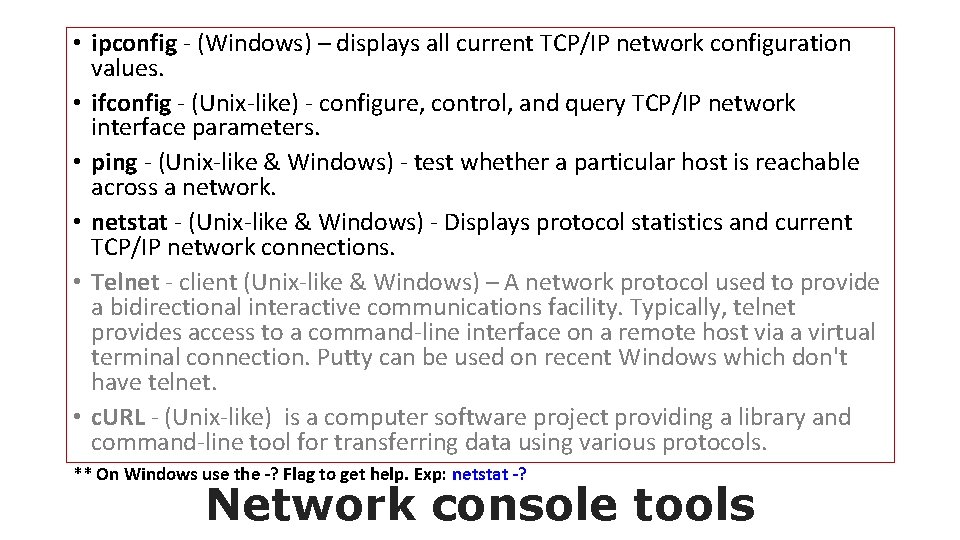
- Slides: 37
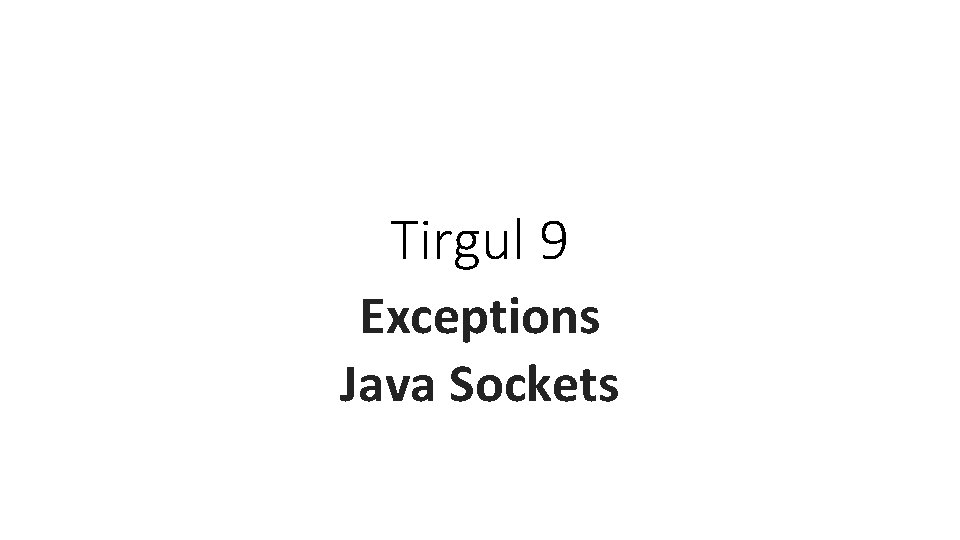
Tirgul 9 Exceptions Java Sockets
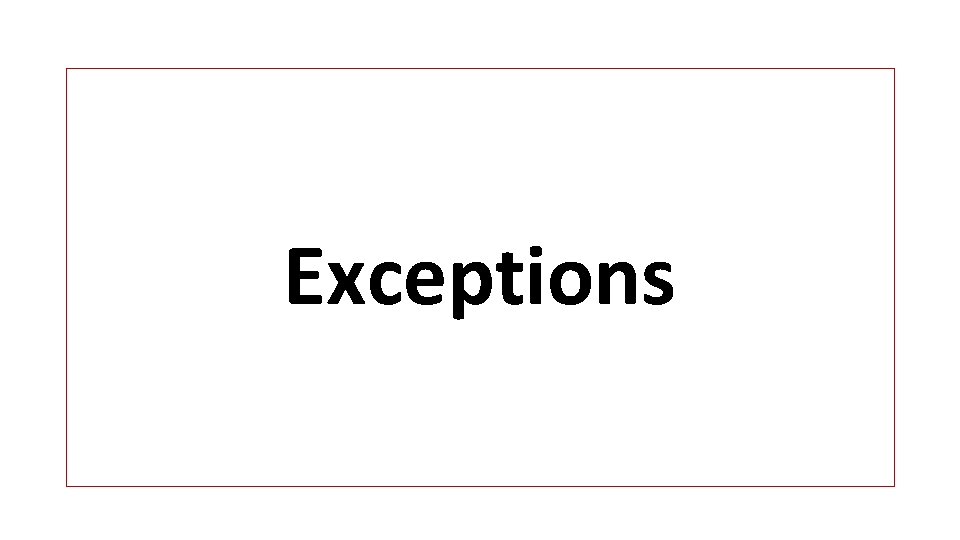
Exceptions
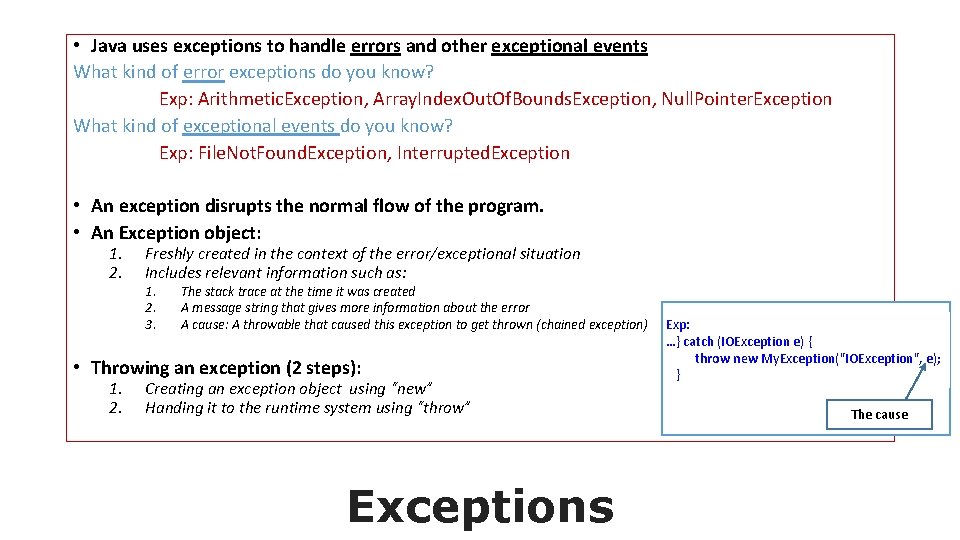
• Java uses exceptions to handle errors and other exceptional events What kind of error exceptions do you know? Exp: Arithmetic. Exception, Array. Index. Out. Of. Bounds. Exception, Null. Pointer. Exception What kind of exceptional events do you know? Exp: File. Not. Found. Exception, Interrupted. Exception • An exception disrupts the normal flow of the program. • An Exception object: 1. 2. Freshly created in the context of the error/exceptional situation Includes relevant information such as: 1. 2. 3. The stack trace at the time it was created A message string that gives more information about the error A cause: A throwable that caused this exception to get thrown (chained exception) • Throwing an exception (2 steps): 1. 2. Creating an exception object using “new” Handing it to the runtime system using “throw” Exceptions Exp: …} catch (IOException e) { throw new My. Exception("IOException", e); } The cause
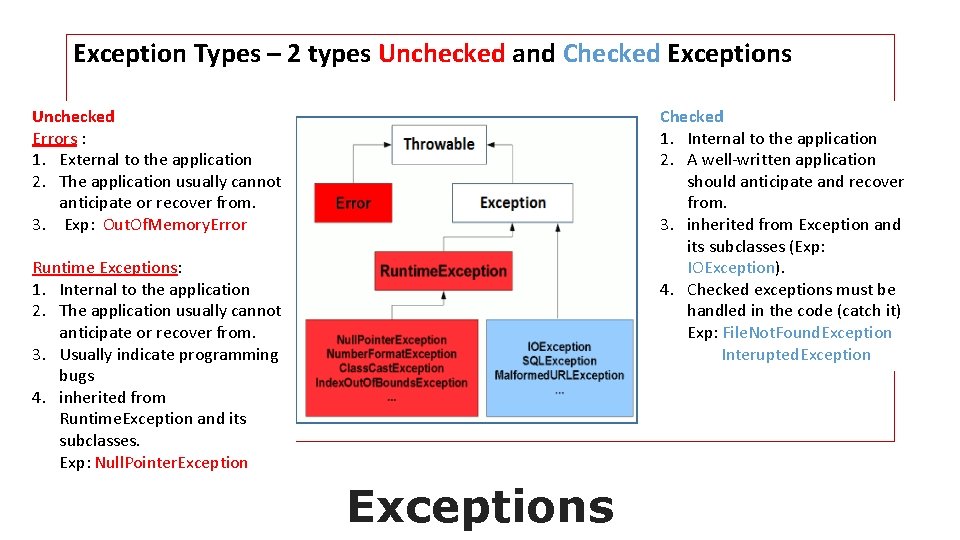
Exception Types – 2 types Unchecked and Checked Exceptions Unchecked Errors : 1. External to the application 2. The application usually cannot anticipate or recover from. 3. Exp: Out. Of. Memory. Error Checked 1. Internal to the application 2. A well-written application should anticipate and recover from. 3. inherited from Exception and its subclasses (Exp: IOException). 4. Checked exceptions must be handled in the code (catch it) Exp: File. Not. Found. Exception Interupted. Exception Runtime Exceptions: 1. Internal to the application 2. The application usually cannot anticipate or recover from. 3. Usually indicate programming bugs 4. inherited from Runtime. Exception and its subclasses. Exp: Null. Pointer. Exceptions
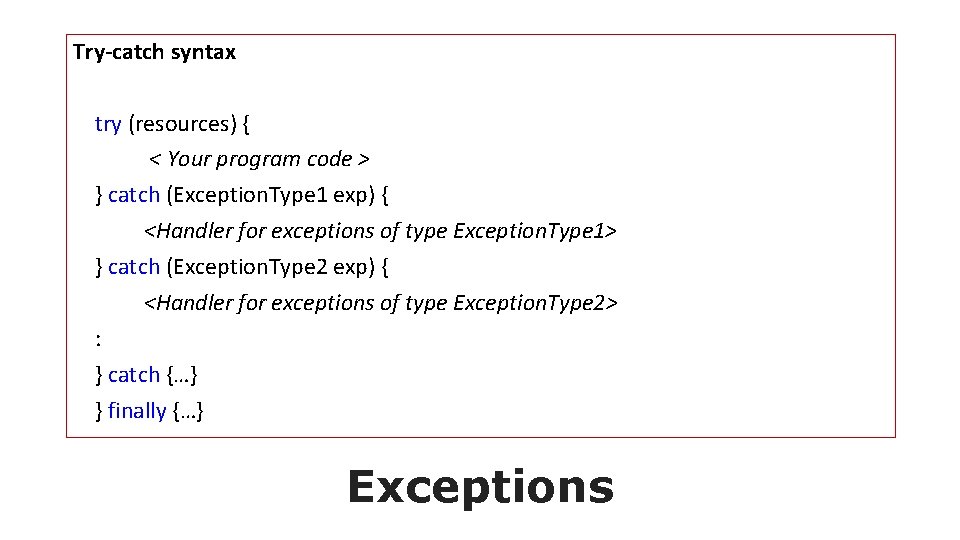
Try-catch syntax try (resources) { < Your program code > } catch (Exception. Type 1 exp) { <Handler for exceptions of type Exception. Type 1> } catch (Exception. Type 2 exp) { <Handler for exceptions of type Exception. Type 2> : } catch {…} } finally {…} Exceptions
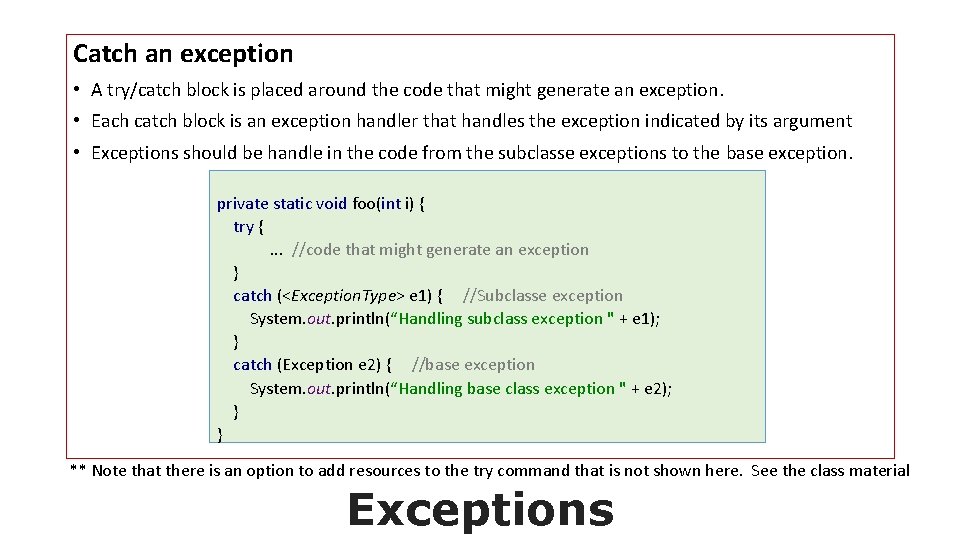
Catch an exception • A try/catch block is placed around the code that might generate an exception. • Each catch block is an exception handler that handles the exception indicated by its argument • Exceptions should be handle in the code from the subclasse exceptions to the base exception. private static void foo(int i) { try {. . . //code that might generate an exception } catch (<Exception. Type> e 1) { //Subclasse exception System. out. println(“Handling subclass exception " + e 1); } catch (Exception e 2) { //base exception System. out. println(“Handling base class exception " + e 2); } } ** Note that there is an option to add resources to the try command that is not shown here. See the class material Exceptions
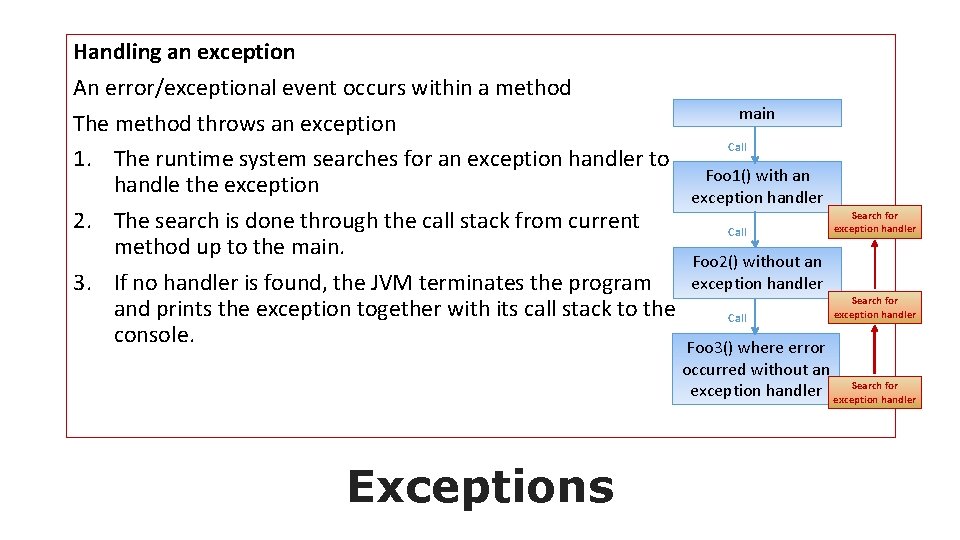
Handling an exception An error/exceptional event occurs within a method The method throws an exception 1. The runtime system searches for an exception handler to handle the exception 2. The search is done through the call stack from current method up to the main. 3. If no handler is found, the JVM terminates the program and prints the exception together with its call stack to the console. Exceptions main Call Foo 1() with an exception handler Call Search for exception handler Foo 2() without an exception handler Call Search for exception handler Foo 3() where error occurred without an Search for exception handler
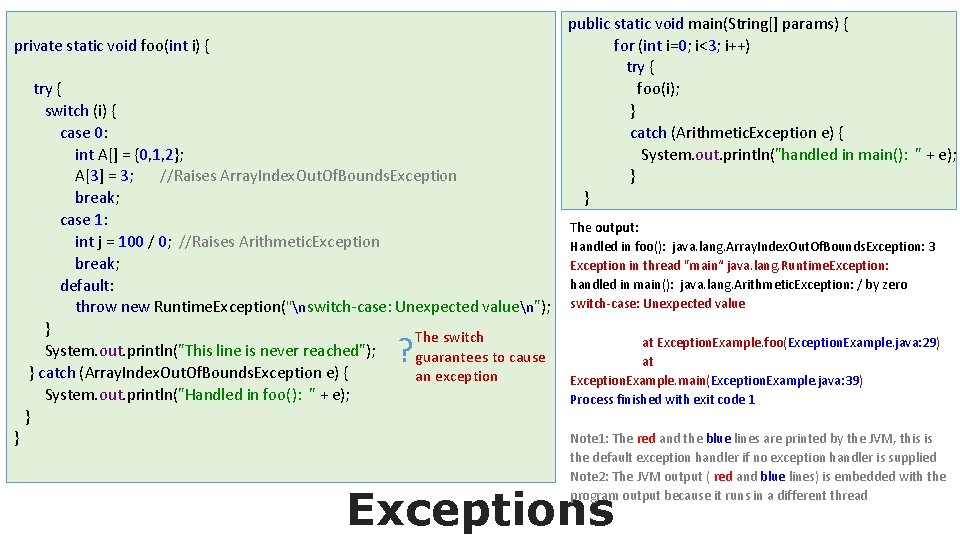
private static void foo(int i) { try { switch (i) { case 0: int A[] = {0, 1, 2}; A[3] = 3; //Raises Array. Index. Out. Of. Bounds. Exception break; case 1: int j = 100 / 0; //Raises Arithmetic. Exception break; default: throw new Runtime. Exception("nswitch-case: Unexpected valuen"); } The switch System. out. println("This line is never reached"); guarantees to cause } catch (Array. Index. Out. Of. Bounds. Exception e) { an exception System. out. println("Handled in foo(): " + e); } ? } public static void main(String[] params) { for (int i=0; i<3; i++) try { foo(i); } catch (Arithmetic. Exception e) { System. out. println("handled in main(): " + e); } } The output: Handled in foo(): java. lang. Array. Index. Out. Of. Bounds. Exception: 3 Exception in thread "main“ java. lang. Runtime. Exception: handled in main(): java. lang. Arithmetic. Exception: / by zero switch-case: Unexpected value at Exception. Example. foo(Exception. Example. java: 29) at Exception. Example. main(Exception. Example. java: 39) Process finished with exit code 1 Note 1: The red and the blue lines are printed by the JVM, this is the default exception handler if no exception handler is supplied Note 2: The JVM output ( red and blue lines) is embedded with the program output because it runs in a different thread Exceptions
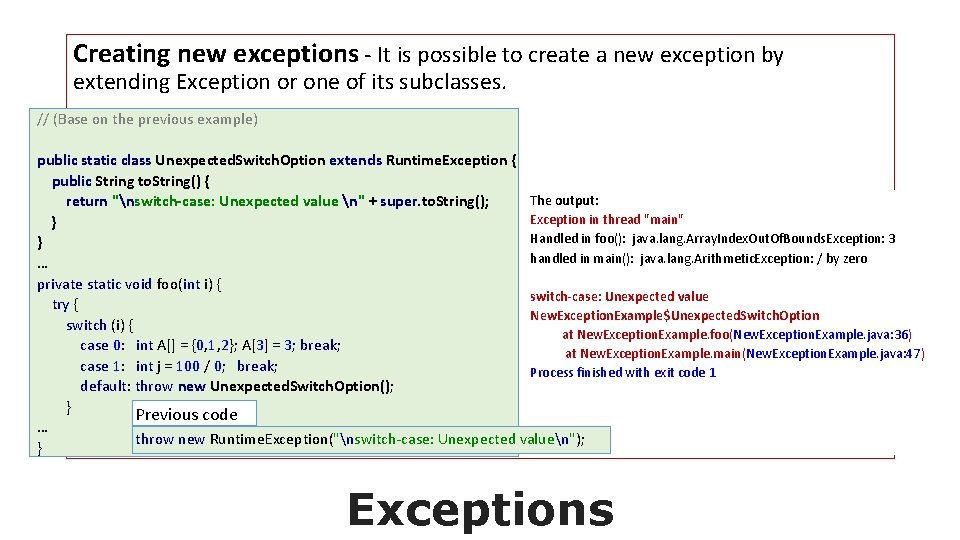
Creating new exceptions - It is possible to create a new exception by extending Exception or one of its subclasses. // (Base on the previous example) public static class Unexpected. Switch. Option extends Runtime. Exception { public String to. String() { The output: return "nswitch-case: Unexpected value n" + super. to. String(); Exception in thread "main" } Handled in foo(): java. lang. Array. Index. Out. Of. Bounds. Exception: 3 } handled in main(): java. lang. Arithmetic. Exception: / by zero … private static void foo(int i) { switch-case: Unexpected value try { New. Exception. Example$Unexpected. Switch. Option switch (i) { at New. Exception. Example. foo(New. Exception. Example. java: 36) case 0: int A[] = {0, 1, 2}; A[3] = 3; break; at New. Exception. Example. main(New. Exception. Example. java: 47) case 1: int j = 100 / 0; break; Process finished with exit code 1 default: throw new Unexpected. Switch. Option(); } Previous code … throw new Runtime. Exception("nswitch-case: Unexpected valuen"); } Exceptions
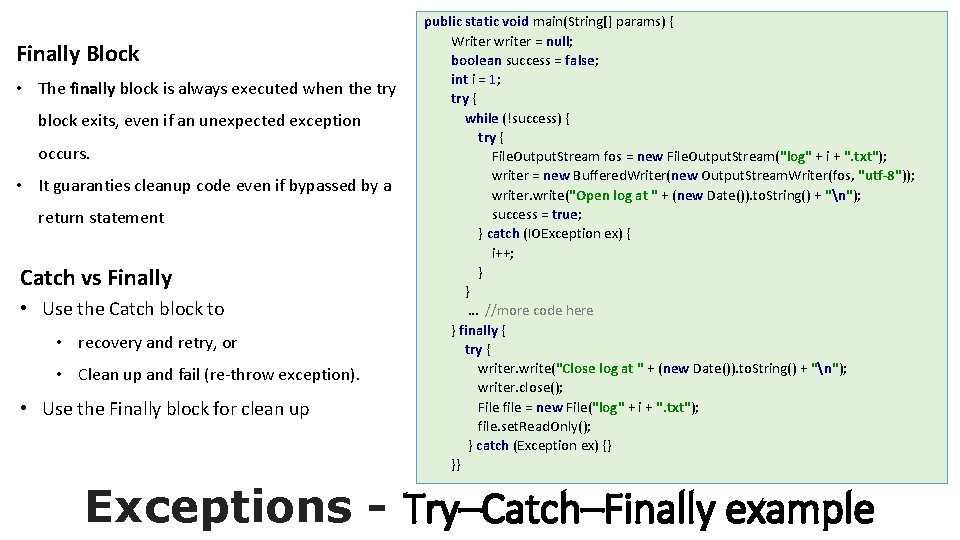
Finally Block • The finally block is always executed when the try block exits, even if an unexpected exception occurs. • It guaranties cleanup code even if bypassed by a return statement Catch vs Finally • Use the Catch block to • recovery and retry, or • Clean up and fail (re-throw exception). • Use the Finally block for clean up public static void main(String[] params) { Writer writer = null; boolean success = false; int i = 1; try { while (!success) { try { File. Output. Stream fos = new File. Output. Stream("log" + i + ". txt"); writer = new Buffered. Writer(new Output. Stream. Writer(fos, "utf-8")); writer. write("Open log at " + (new Date()). to. String() + "n"); success = true; } catch (IOException ex) { i++; } } … //more code here } finally { try { writer. write("Close log at " + (new Date()). to. String() + "n"); writer. close(); File file = new File("log" + i + ". txt"); file. set. Read. Only(); } catch (Exception ex) {} }} Exceptions - Try–Catch–Finally example
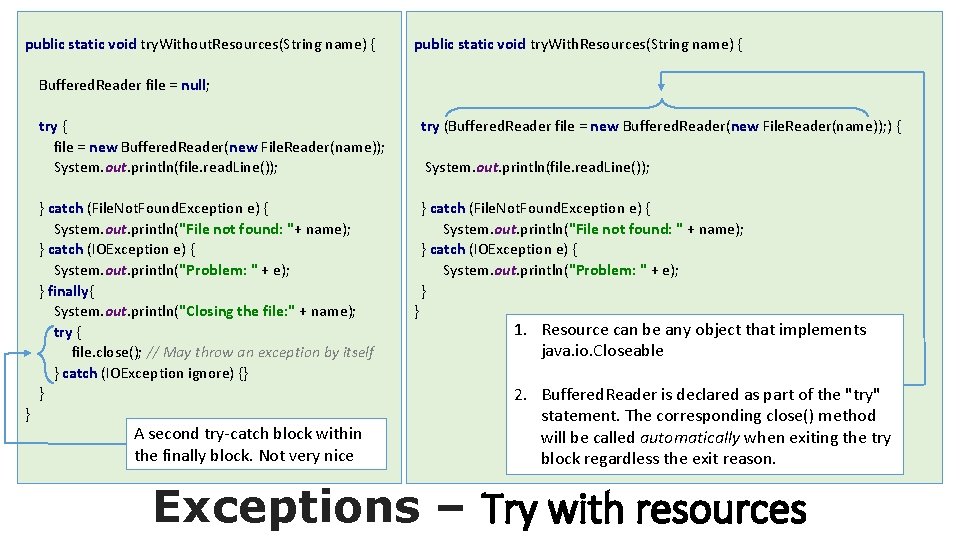
public static void try. Without. Resources(String name) { public static void try. With. Resources(String name) { Buffered. Reader file = null; } try { file = new Buffered. Reader(new File. Reader(name)); System. out. println(file. read. Line()); try (Buffered. Reader file = new Buffered. Reader(new File. Reader(name)); ) { } catch (File. Not. Found. Exception e) { System. out. println("File not found: "+ name); } catch (IOException e) { System. out. println("Problem: " + e); } finally{ System. out. println("Closing the file: " + name); try { file. close(); // May throw an exception by itself } catch (IOException ignore) {} } } catch (File. Not. Found. Exception e) { System. out. println("File not found: " + name); } catch (IOException e) { System. out. println("Problem: " + e); } A second try-catch block within the finally block. Not very nice System. out. println(file. read. Line()); } 1. Resource can be any object that implements java. io. Closeable 2. Buffered. Reader is declared as part of the "try" statement. The corresponding close() method will be called automatically when exiting the try block regardless the exit reason. Exceptions – Try with resources
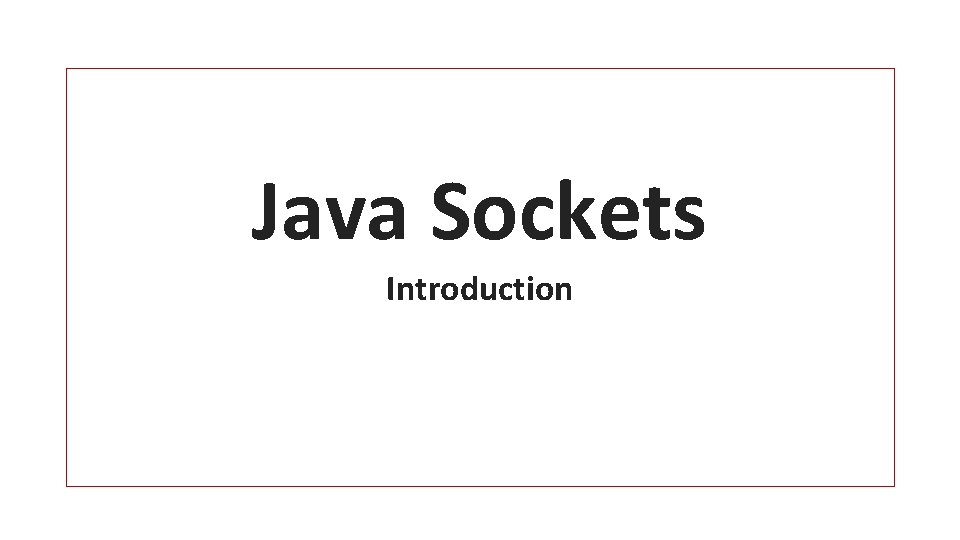
Java Sockets Introduction
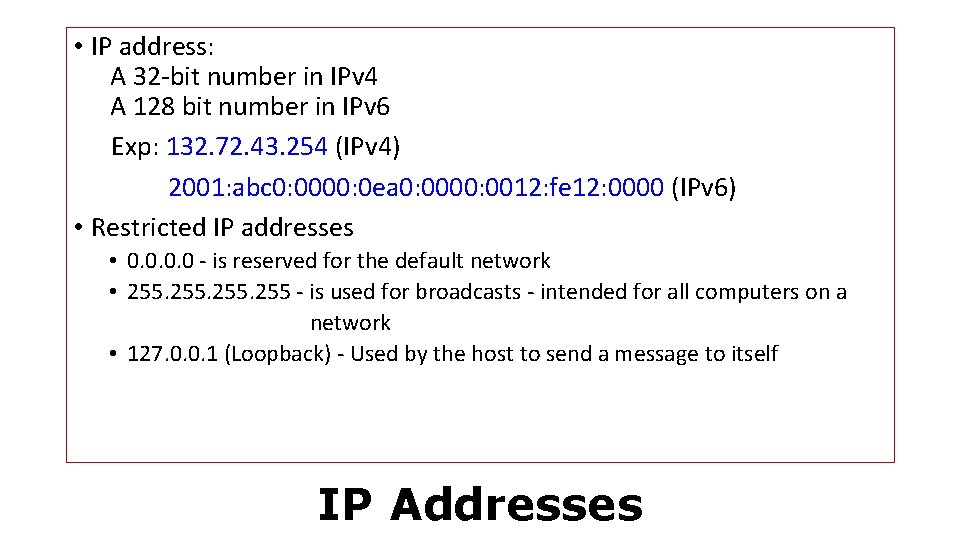
• IP address: A 32 -bit number in IPv 4 A 128 bit number in IPv 6 Exp: 132. 72. 43. 254 (IPv 4) 2001: abc 0: 0000: 0 ea 0: 0000: 0012: fe 12: 0000 (IPv 6) • Restricted IP addresses • 0. 0 - is reserved for the default network • 255 - is used for broadcasts - intended for all computers on a network • 127. 0. 0. 1 (Loopback) - Used by the host to send a message to itself IP Addresses
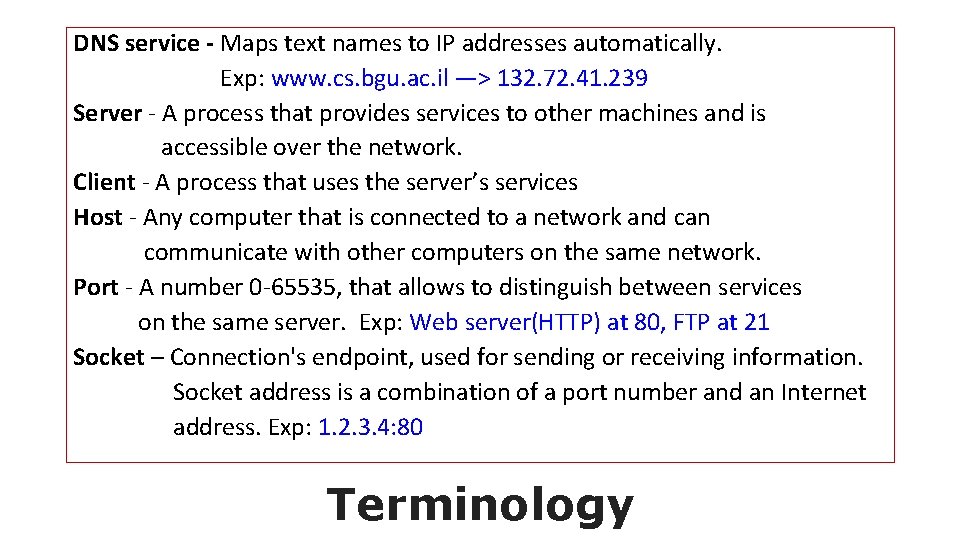
DNS service - Maps text names to IP addresses automatically. Exp: www. cs. bgu. ac. il —> 132. 72. 41. 239 Server - A process that provides services to other machines and is accessible over the network. Client - A process that uses the server’s services Host - Any computer that is connected to a network and can communicate with other computers on the same network. Port - A number 0 -65535, that allows to distinguish between services on the same server. Exp: Web server(HTTP) at 80, FTP at 21 Socket – Connection's endpoint, used for sending or receiving information. Socket address is a combination of a port number and an Internet address. Exp: 1. 2. 3. 4: 80 Terminology
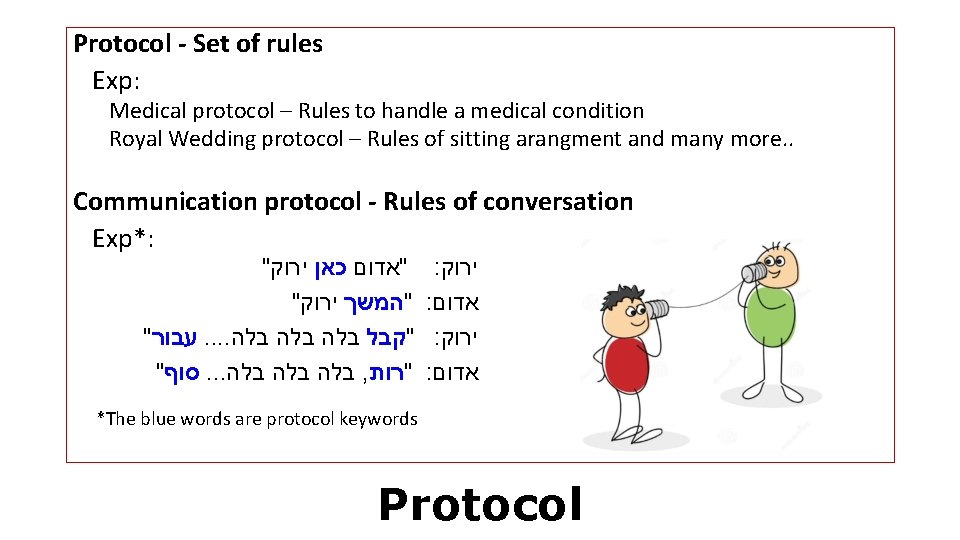
Protocol - Set of rules Exp: Medical protocol – Rules to handle a medical condition Royal Wedding protocol – Rules of sitting arangment and many more. . Communication protocol - Rules of conversation Exp*: " "אדום כאן ירוק " "המשך ירוק " עבור. . "קבל בלה בלה " סוף. . . בלה בלה , "רות : ירוק : אדום *The blue words are protocol keywords Protocol
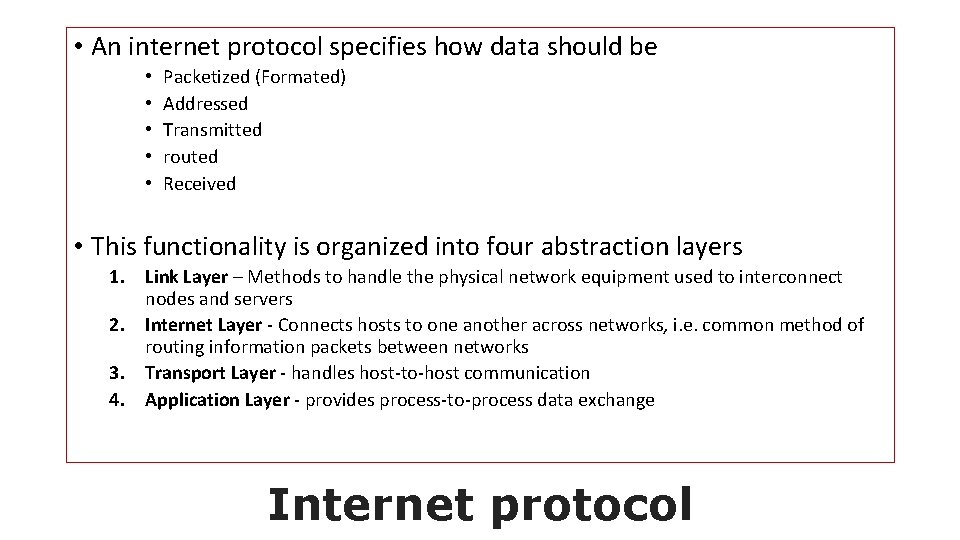
• An internet protocol specifies how data should be • • • Packetized (Formated) Addressed Transmitted routed Received • This functionality is organized into four abstraction layers 1. Link Layer – Methods to handle the physical network equipment used to interconnect nodes and servers 2. Internet Layer - Connects hosts to one another across networks, i. e. common method of routing information packets between networks 3. Transport Layer - handles host-to-host communication 4. Application Layer - provides process-to-process data exchange Internet protocol
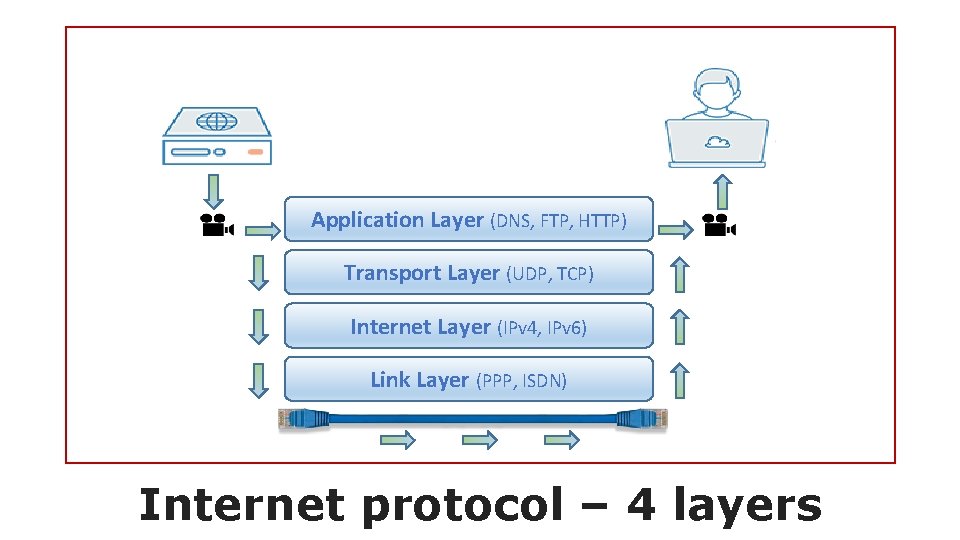
Application Layer (DNS, FTP, HTTP) Transport Layer (UDP, TCP) Internet Layer (IPv 4, IPv 6) Link Layer (PPP, ISDN) Internet protocol – 4 layers
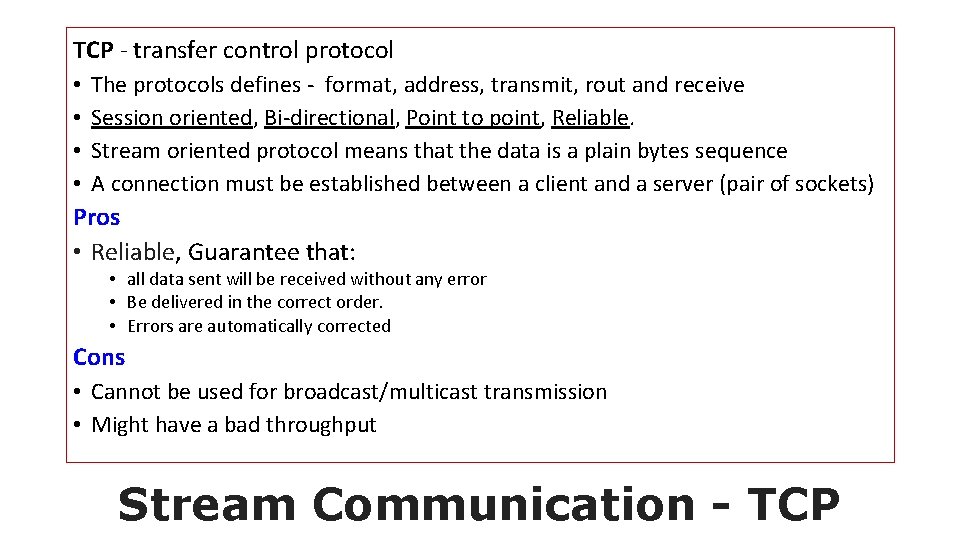
TCP - transfer control protocol • • The protocols defines - format, address, transmit, rout and receive Session oriented, Bi-directional, Point to point, Reliable. Stream oriented protocol means that the data is a plain bytes sequence A connection must be established between a client and a server (pair of sockets) Pros • Reliable, Guarantee that: • all data sent will be received without any error • Be delivered in the correct order. • Errors are automatically corrected Cons • Cannot be used for broadcast/multicast transmission • Might have a bad throughput Stream Communication - TCP
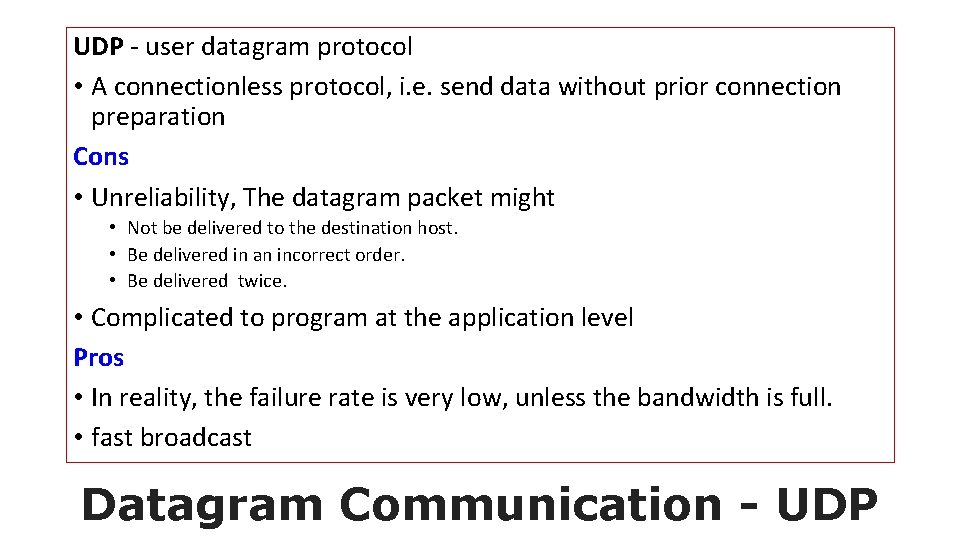
UDP - user datagram protocol • A connectionless protocol, i. e. send data without prior connection preparation Cons • Unreliability, The datagram packet might • Not be delivered to the destination host. • Be delivered in an incorrect order. • Be delivered twice. • Complicated to program at the application level Pros • In reality, the failure rate is very low, unless the bandwidth is full. • fast broadcast Datagram Communication - UDP
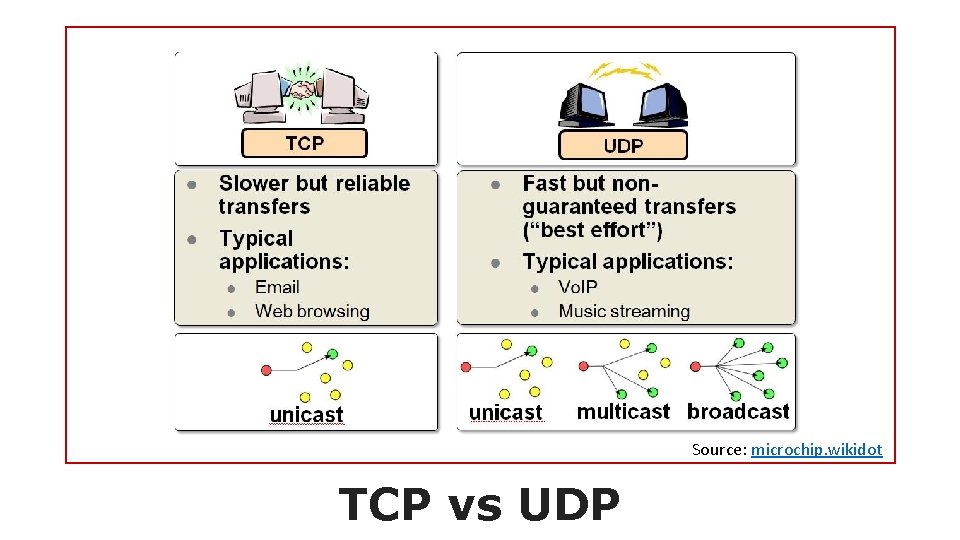
Source: microchip. wikidot TCP vs UDP
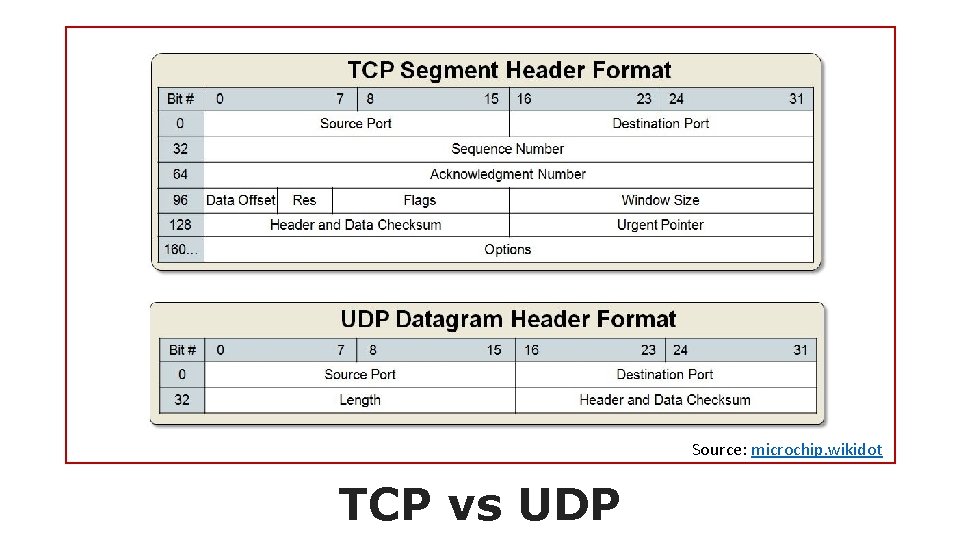
Source: microchip. wikidot TCP vs UDP
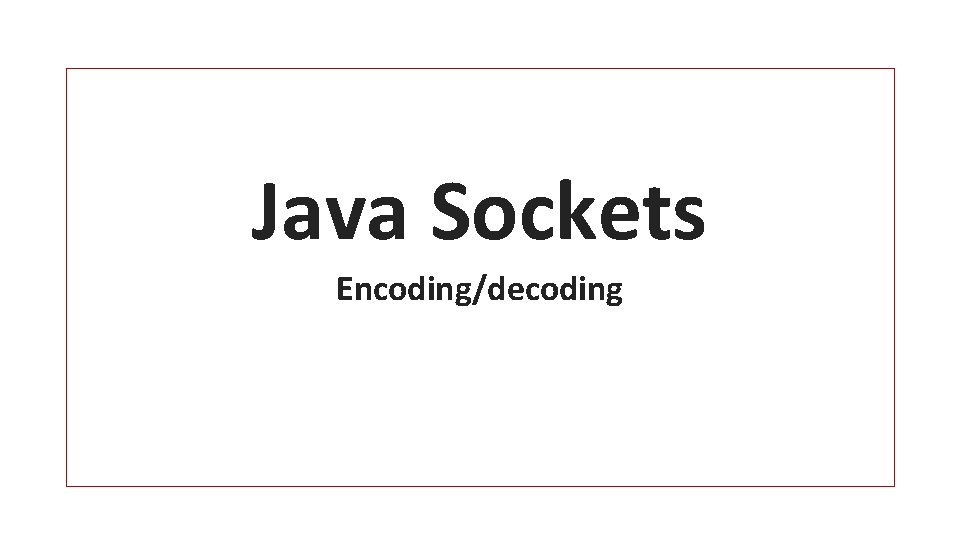
Java Sockets Encoding/decoding
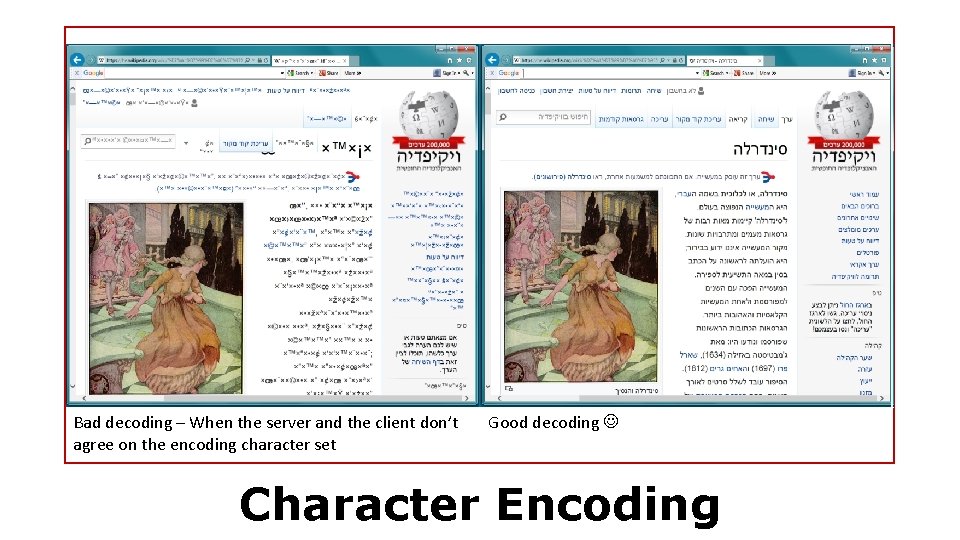
Bad decoding – When the server and the client don’t agree on the encoding character set Good decoding Character Encoding
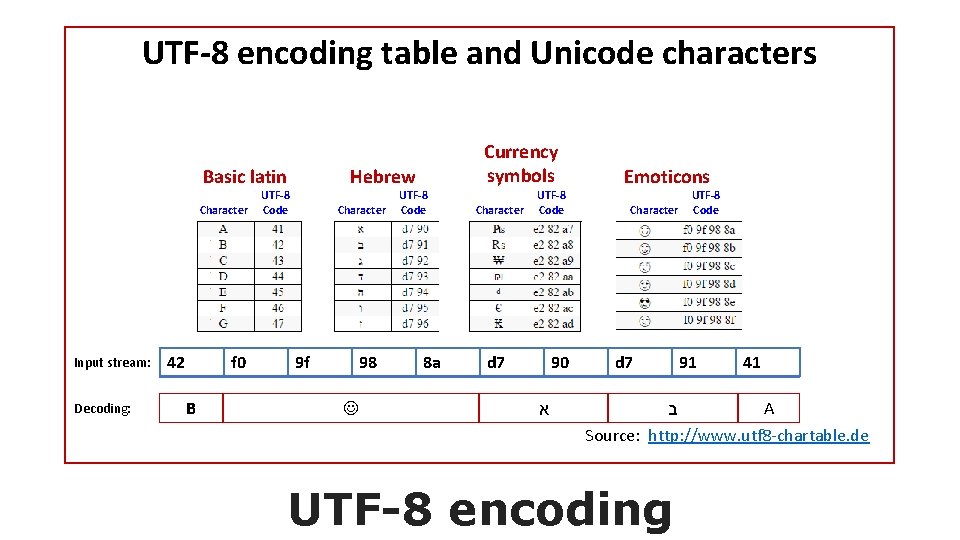
UTF-8 encoding table and Unicode characters Basic latin Character Input stream: Decoding: 42 f 0 B Currency symbols Hebrew UTF-8 Code Character 9 f 98 UTF-8 Code 8 a Character UTF-8 Code d 7 90 א Emoticons Character d 7 UTF-8 Code 91 41 A ב Source: http: //www. utf 8 -chartable. de UTF-8 encoding
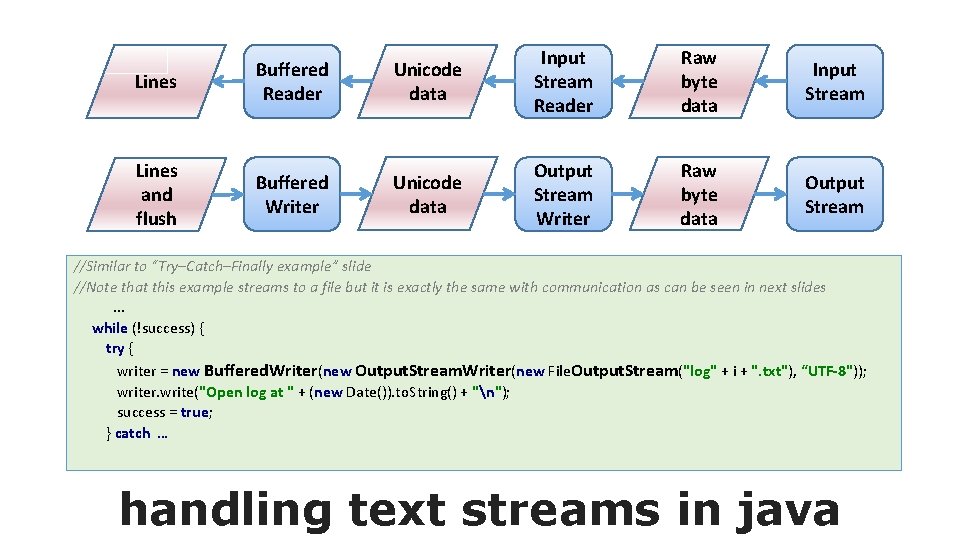
Lines Buffered Reader Unicode data Lines and flush Buffered Writer Unicode data Input Stream Reader Raw byte data Input Stream Output Stream Writer Raw byte data Output Stream //Similar to “Try–Catch–Finally example” slide //Note that this example streams to a file but it is exactly the same with communication as can be seen in next slides. . . while (!success) { try { writer = new Buffered. Writer(new Output. Stream. Writer(new File. Output. Stream("log" + i + ". txt"), “UTF-8")); writer. write("Open log at " + (new Date()). to. String() + "n"); success = true; } catch … handling text streams in java
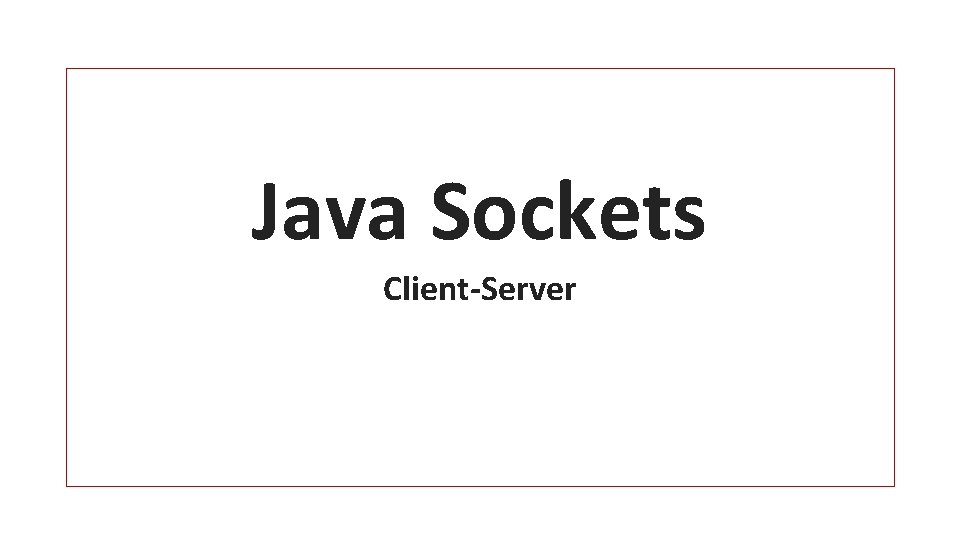
Java Sockets Client-Server
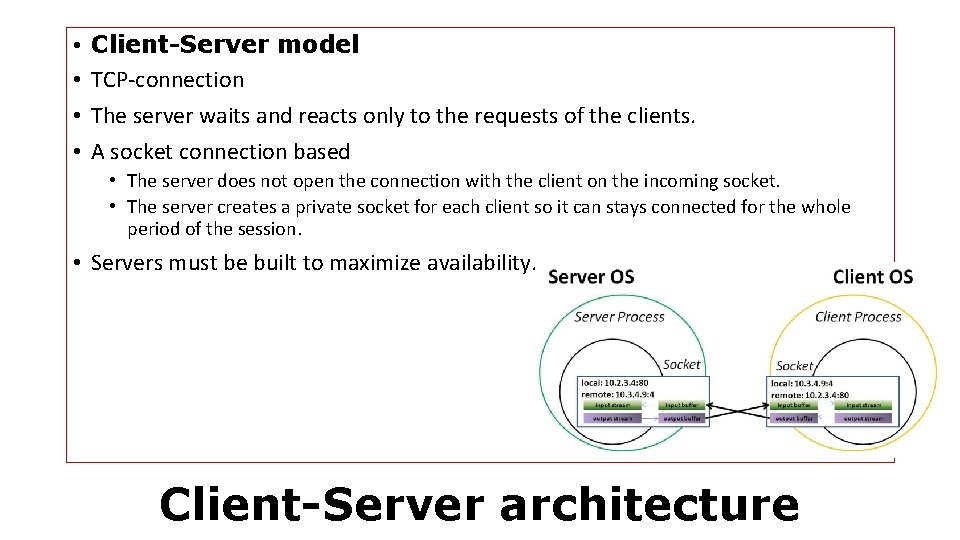
• • Client-Server model TCP-connection The server waits and reacts only to the requests of the clients. A socket connection based • The server does not open the connection with the client on the incoming socket. • The server creates a private socket for each client so it can stays connected for the whole period of the session. • Servers must be built to maximize availability. Client-Server architecture
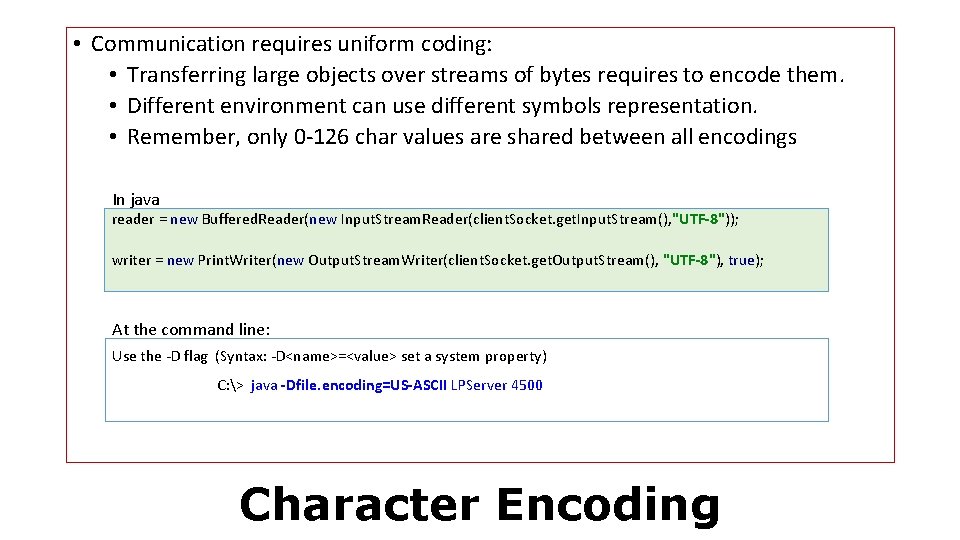
• Communication requires uniform coding: • Transferring large objects over streams of bytes requires to encode them. • Different environment can use different symbols representation. • Remember, only 0 -126 char values are shared between all encodings In java reader = new Buffered. Reader(new Input. Stream. Reader(client. Socket. get. Input. Stream(), "UTF-8")); writer = new Print. Writer(new Output. Stream. Writer(client. Socket. get. Output. Stream(), "UTF-8"), true); At the command line: Use the -D flag (Syntax: -D<name>=<value> set a system property) C: > java -Dfile. encoding=US-ASCII LPServer 4500 Character Encoding
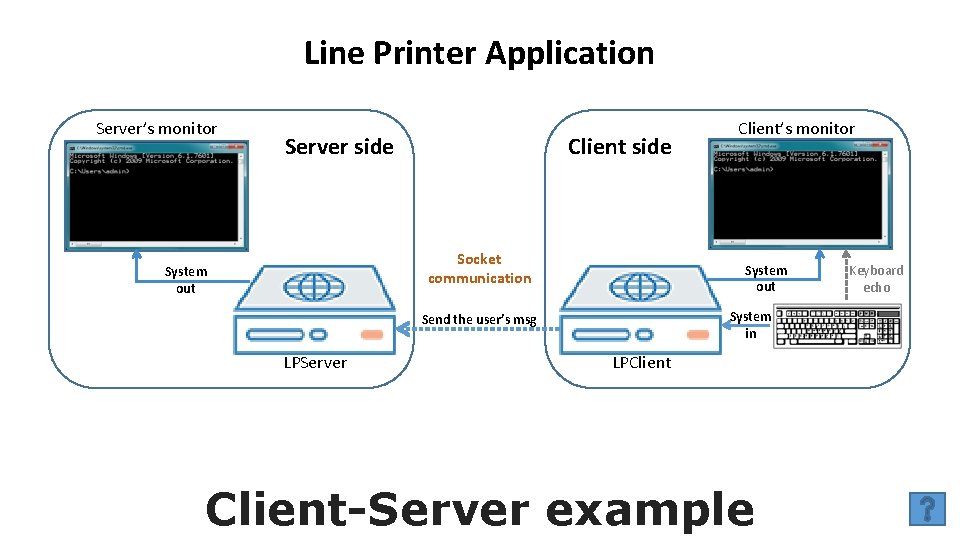
Line Printer Application Server’s monitor Server side Client side Socket communication System out System in Send the user’s msg LPServer Client’s monitor LPClient-Server example Keyboard echo
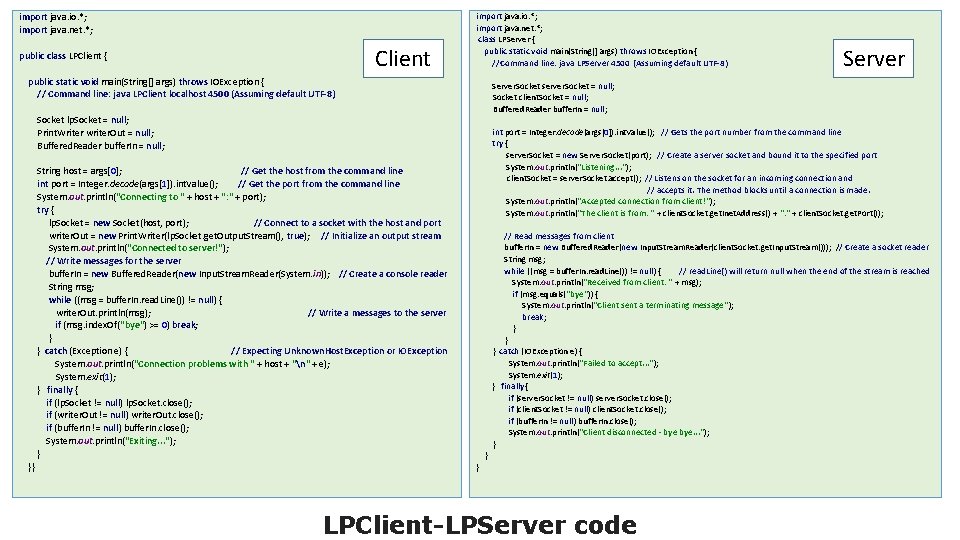
import java. io. *; import java. net. *; Client public class LPClient { import java. io. *; import java. net. *; class LPServer { public static void main(String[] args) throws IOException { //Command line: java LPServer 4500 (Assuming default UTF-8) public static void main(String[] args) throws IOException { // Command line: java LPClient localhost 4500 (Assuming default UTF-8) Server. Socket server. Socket = null; Socket client. Socket = null; Buffered. Reader buffer. In = null; Socket lp. Socket = null; Print. Writer writer. Out = null; Buffered. Reader buffer. In = null; int port = Integer. decode(args[0]). int. Value(); // Gets the port number from the command line try { server. Socket = new Server. Socket(port); // Create a server socket and bound it to the specified port System. out. println("Listening. . . "); client. Socket = server. Socket. accept(); // Listens on the socket for an incoming connection and // accepts it. The method blocks until a connection is made. System. out. println("Accepted connection from client!"); System. out. println("The client is from: " + client. Socket. get. Inet. Address() + ": " + client. Socket. get. Port()); String host = args[0]; // Get the host from the command line int port = Integer. decode(args[1]). int. Value(); // Get the port from the command line System. out. println("Connecting to " + host + ": " + port); try { lp. Socket = new Socket(host, port); // Connect to a socket with the host and port writer. Out = new Print. Writer(lp. Socket. get. Output. Stream(), true); // Initialize an output stream System. out. println("Connected to server!"); // Write messages for the server buffer. In = new Buffered. Reader(new Input. Stream. Reader(System. in)); // Create a console reader String msg; while ((msg = buffer. In. read. Line()) != null) { writer. Out. println(msg); // Write a messages to the server if (msg. index. Of("bye") >= 0) break; } } catch (Exception e) { // Expecting Unknown. Host. Exception or IOException System. out. println("Connection problems with " + host + "n" + e); System. exit(1); } finally { if (lp. Socket != null) lp. Socket. close(); if (writer. Out != null) writer. Out. close(); if (buffer. In != null) buffer. In. close(); System. out. println("Exiting. . . "); } }} Server // Read messages from client buffer. In = new Buffered. Reader(new Input. Stream. Reader(client. Socket. get. Input. Stream())); // Create a socket reader String msg; while ((msg = buffer. In. read. Line()) != null) { // read. Line() will return null when the end of the stream is reached System. out. println("Received from client: " + msg); if (msg. equals("bye")) { System. out. println("Client sent a terminating message"); break; } } } catch (IOException e) { System. out. println("Failed to accept. . . "); System. exit(1); } finally{ if (server. Socket != null) server. Socket. close(); if (client. Socket != null) client. Socket. close(); if (buffer. In != null) buffer. In. close(); System. out. println("Client disconnected - bye. . . "); } } } LPClient-LPServer code
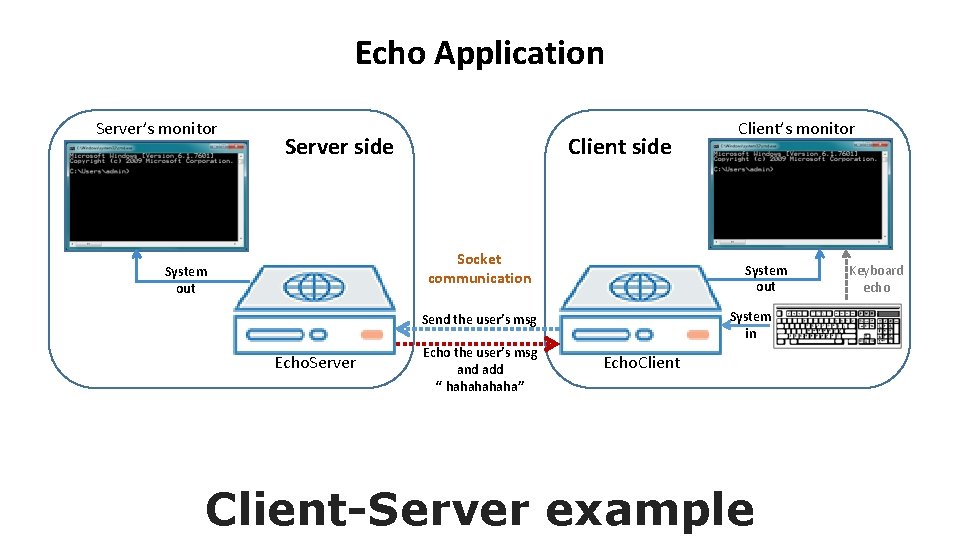
Echo Application Server’s monitor Server side Client side Socket communication System out System in Send the user’s msg Echo. Server Echo the user’s msg and add “ hahaha” Client’s monitor Echo. Client-Server example Keyboard echo
![public class Echo Client public static void mainString args throws IOException public class Echo. Client { public static void main(String[] args) throws IOException { /*](https://slidetodoc.com/presentation_image_h2/58f4fff8111cc83418332d0c4ba15468/image-32.jpg)
public class Echo. Client { public static void main(String[] args) throws IOException { /* Command line: java Echo. Client localhost 4500 (Assuming default UTF-8) */ Client Socket socket = null; Print. Writer writer. Out = null; Buffered. Reader buffer. In = null; Buffered. Reader echo. In = null; //A reader from the client socket } Server. Socket server. Socket = null; Socket client. Socket = null; Buffered. Reader buffer. In = null; Print. Writer writer. Out = null; //A writer to the client socket String host = args[0]; /* Get the host from the command line */ int port = Integer. decode(args[1]). int. Value(); /* Get the port from the command line */ System. out. println("Connecting to " + host + ": " + port); try { socket = new Socket(host, port); /* Connect to a socket with the host and port */ writer. Out = new Print. Writer(socket. get. Output. Stream(), true); /* Initialize an output stream */ System. out. println("Connected to server!"); /* Write messages for the server*/ buffer. In = new Buffered. Reader(new Input. Stream. Reader(System. in)); /* Create a console reader */ echo. In = new Buffered. Reader(new Input. Stream. Reader(socket. get. Input. Stream())); // Create the socket reader String msg; while ((msg = buffer. In. read. Line()) != null) { writer. Out. println(msg); /* Write a messages to the server */ String line = echo. In. read. Line(); // Read from the socket the incoming echo and print it System. out. println("message from server: " + line); if (msg. index. Of("bye") >= 0) break; } } catch (Exception e) { /* Expecting Unknown. Host. Exception or IOException */ System. out. println("Connection problems with " + host + "n" + e); System. exit(1); } finally { if (socket != null) socket. close(); if (writer. Out != null) writer. Out. close(); if (buffer. In != null) buffer. In. close(); if (echo. In != null) echo. In. close(); // Close the socket reader System. out. println("Exiting. . . "); } } import java. io. *; import java. net. *; public class Echo. Server { public static void main(String[] args) throws IOException { /* Command line: java LPServer 4500 */ int port = Integer. decode(args[0]). int. Value(); /* Gets the port number from the command line */ try { server. Socket = new Server. Socket(port); /* Create a server socket and bound it to the specified port */ System. out. println("Listening. . . "); client. Socket = server. Socket. accept(); /* Listens on the socket for an incoming connection and accepts it. The method blocks until a connection is made. . */ System. out. println("Accepted connection from client!"); System. out. println("The client is from: " + client. Socket. get. Inet. Address() + ": " + client. Socket. get. Port()); /* Read messages from client */ buffer. In = new Buffered. Reader(new Input. Stream. Reader(client. Socket. get. Input. Stream())); /* Create a socket reader */ writer. Out = new Print. Writer(client. Socket. get. Output. Stream(), true); // Create a socket writer String msg; while ((msg = buffer. In. read. Line()) != null) { /* read. Line() will return null when the end of the stream is reached */ System. out. println("Received from client: " + msg); writer. Out. println(msg + " hahaha"); // Write a messages to the client if (msg. equals("bye")) { System. out. println("Client sent a terminating message"); break; } } } catch (IOException e) { System. out. println("Communication Failed, port=" + port); System. exit(1); } finally{ if (server. Socket != null) server. Socket. close(); if (client. Socket != null) client. Socket. close(); if (buffer. In != null) buffer. In. close(); if (writer. Out != null) writer. Out. close(); // Close the writer System. out. println("Client disconnected - bye. . . "); }}} Echo. Client-Echo. Server code
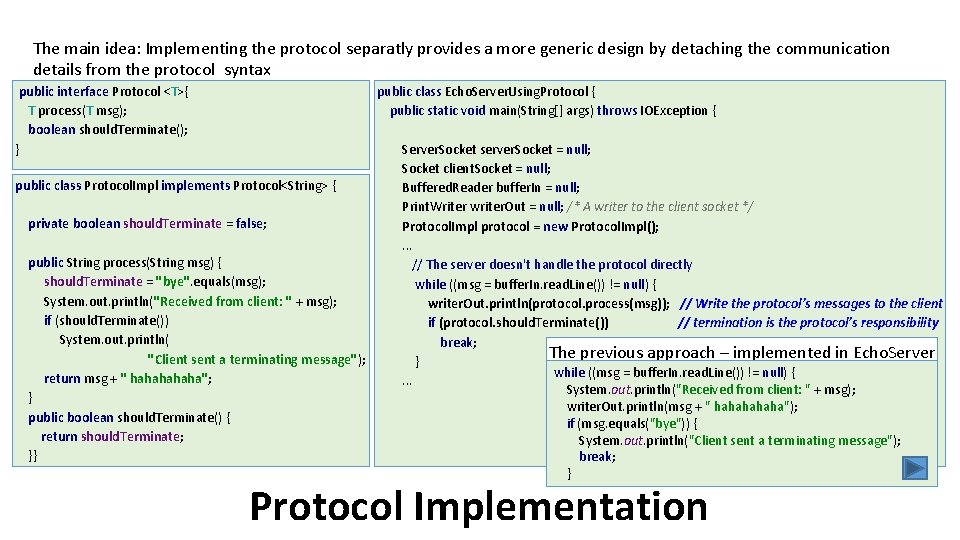
The main idea: Implementing the protocol separatly provides a more generic design by detaching the communication details from the protocol syntax public interface Protocol <T>{ T process(T msg); boolean should. Terminate(); } public class Echo. Server. Using. Protocol { public static void main(String[] args) throws IOException { public class Protocol. Impl implements Protocol<String> { private boolean should. Terminate = false; public String process(String msg) { should. Terminate = "bye". equals(msg); System. out. println("Received from client: " + msg); if (should. Terminate()) System. out. println( "Client sent a terminating message"); return msg + " hahaha"; } public boolean should. Terminate() { return should. Terminate; }} Server. Socket server. Socket = null; Socket client. Socket = null; Buffered. Reader buffer. In = null; Print. Writer writer. Out = null; /* A writer to the client socket */ Protocol. Impl protocol = new Protocol. Impl(); . . . // The server doesn't handle the protocol directly while ((msg = buffer. In. read. Line()) != null) { writer. Out. println(protocol. process(msg)); // Write the protocol’s messages to the client if (protocol. should. Terminate()) // termination is the protocol’s responsibility break; The previous approach – implemented in Echo. Server } while ((msg = buffer. In. read. Line()) != null) {. . . System. out. println("Received from client: " + msg); writer. Out. println(msg + " hahaha"); if (msg. equals("bye")) { System. out. println("Client sent a terminating message"); break; } Protocol Implementation
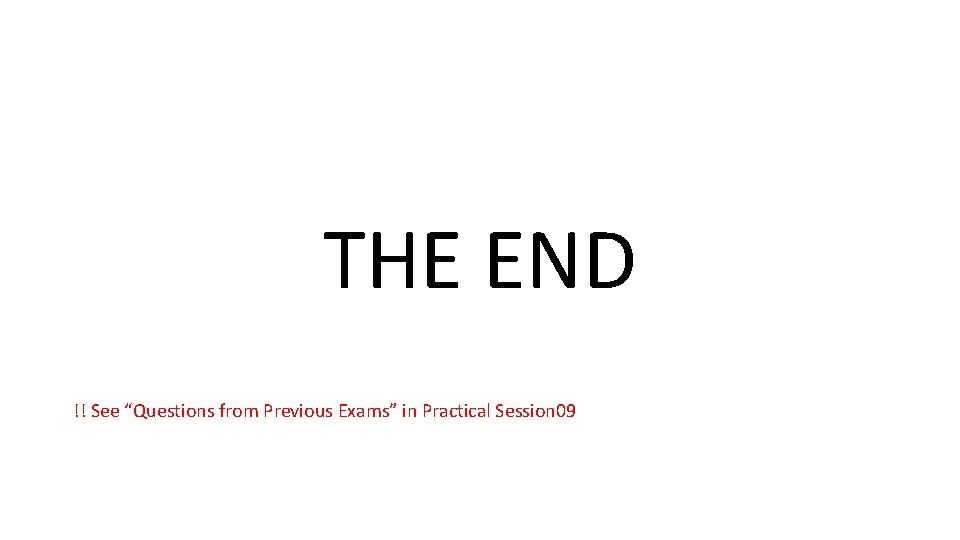
THE END !! See “Questions from Previous Exams” in Practical Session 09
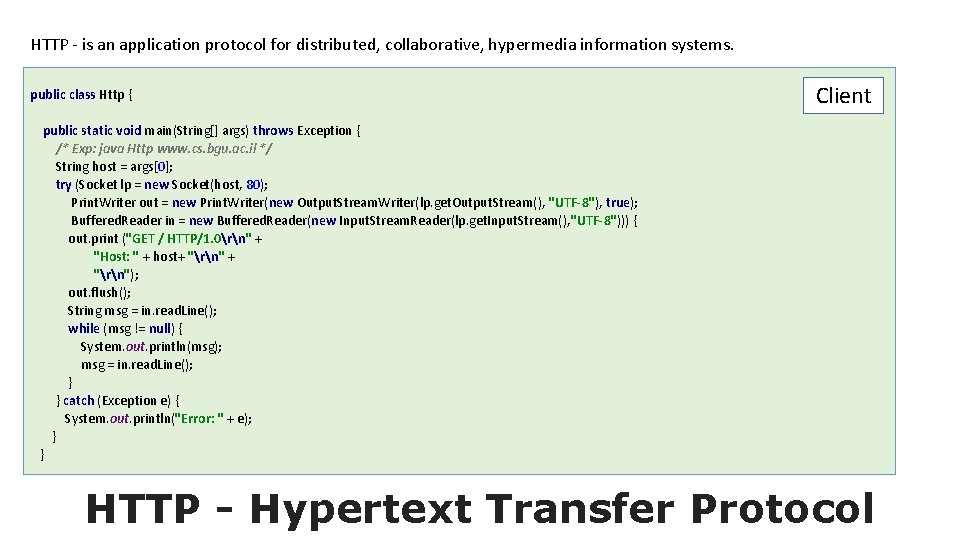
HTTP - is an application protocol for distributed, collaborative, hypermedia information systems. public class Http { Client public static void main(String[] args) throws Exception { /* Exp: java Http www. cs. bgu. ac. il */ String host = args[0]; try (Socket lp = new Socket(host, 80); Print. Writer out = new Print. Writer(new Output. Stream. Writer(lp. get. Output. Stream(), "UTF-8"), true); Buffered. Reader in = new Buffered. Reader(new Input. Stream. Reader(lp. get. Input. Stream(), "UTF-8"))) { out. print ("GET / HTTP/1. 0rn" + "Host: " + host+ "rn"); out. flush(); String msg = in. read. Line(); while (msg != null) { System. out. println(msg); msg = in. read. Line(); } } catch (Exception e) { System. out. println("Error: " + e); } } HTTP - Hypertext Transfer Protocol
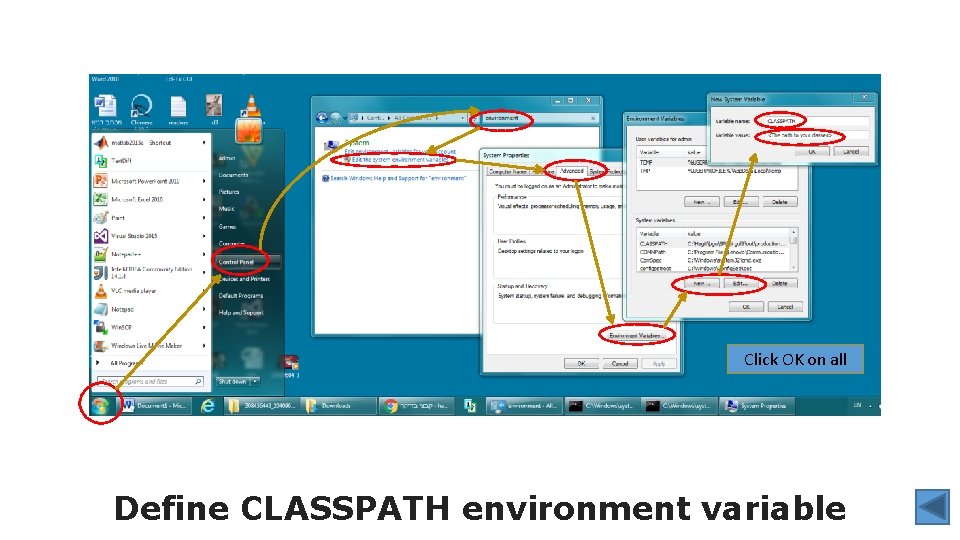
Click OK on all Define CLASSPATH environment variable
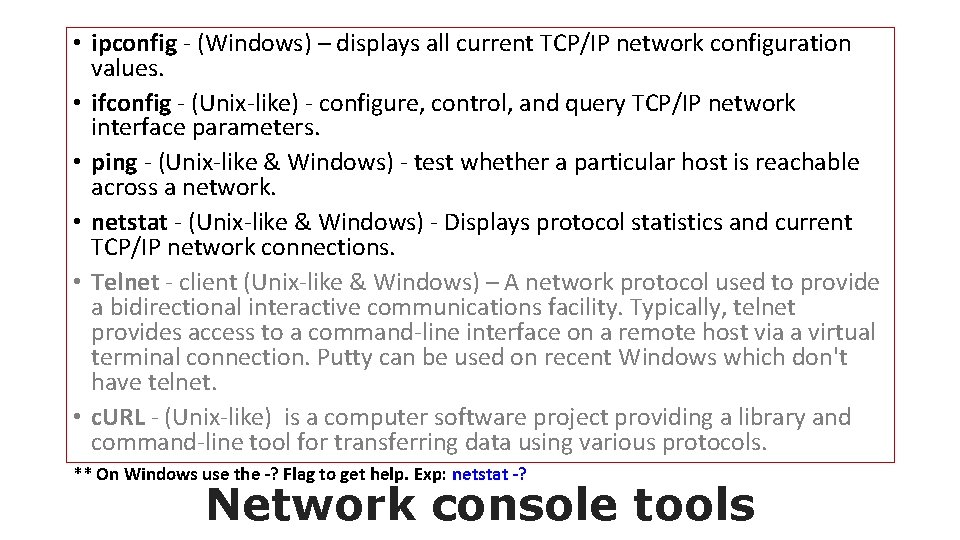
• ipconfig - (Windows) – displays all current TCP/IP network configuration values. • ifconfig - (Unix-like) - configure, control, and query TCP/IP network interface parameters. • ping - (Unix-like & Windows) - test whether a particular host is reachable across a network. • netstat - (Unix-like & Windows) - Displays protocol statistics and current TCP/IP network connections. • Telnet - client (Unix-like & Windows) – A network protocol used to provide a bidirectional interactive communications facility. Typically, telnet provides access to a command-line interface on a remote host via a virtual terminal connection. Putty can be used on recent Windows which don't have telnet. • c. URL - (Unix-like) is a computer software project providing a library and command-line tool for transferring data using various protocols. ** On Windows use the -? Flag to get help. Exp: netstat -? Network console tools
Tcp/ip sockets in java: practical guide for programmers
1c=142a=0 after try/catch blocks
Java array to list
Custom exceptions in java
Sockets of silver
Tcp ip sockets in c
Car steering components
Sockets direct protocol
Socket primitives in computer networks
Reliable datagram sockets
Stevens unix network programming
Socket raw
D3azeg_clwi -site:youtube.com
Sockets and threads
Cleanroom 380v sockets
R socket programming
Sockets linux
Secure sockets
Java raw socket
Datacenter fabric
Berkeley sockets
Spl exceptions
Stark law exceptions
Present continuous dragon
Exception handler php
Exception of koch postulates
Koch's postulates exceptions
Pasteurized milk meaning
Stark law exceptions
Predefined and non predefined exceptions are raised
Ionization potential
Octet exceptions
Superlatives exceptions
Koch's postulates exceptions
Shit rolls down hill
Exceptions to mendelian genetics
Different types of exceptions in c++
What are question tags