Static Static Not dynamic class Widget static int
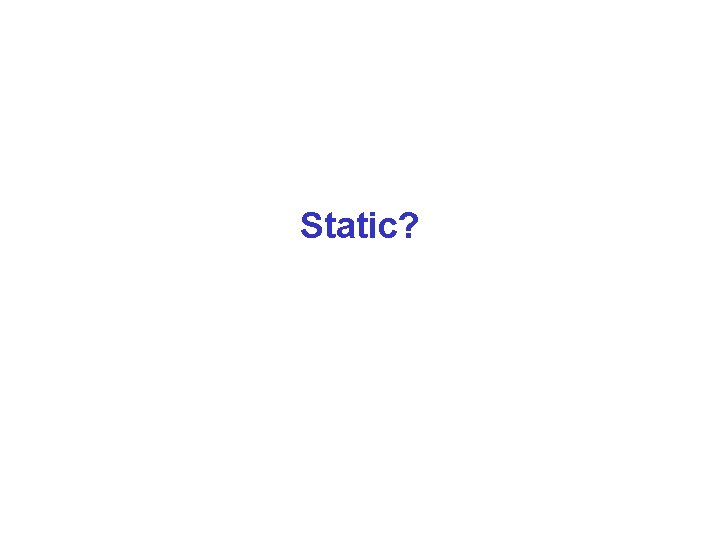
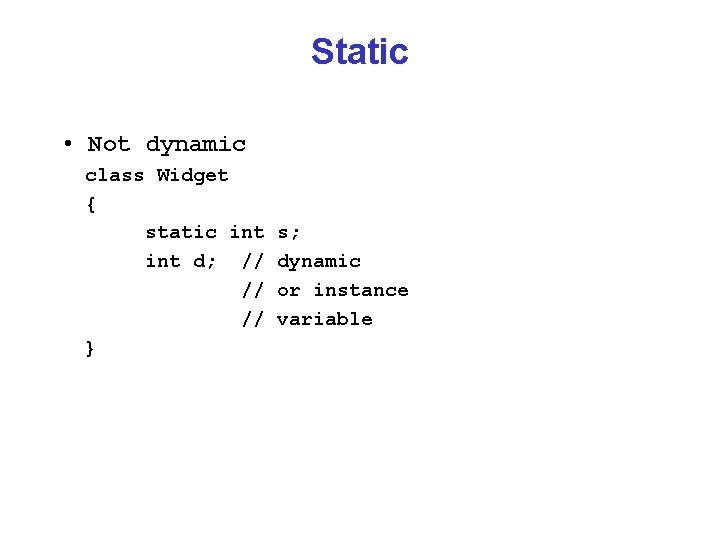
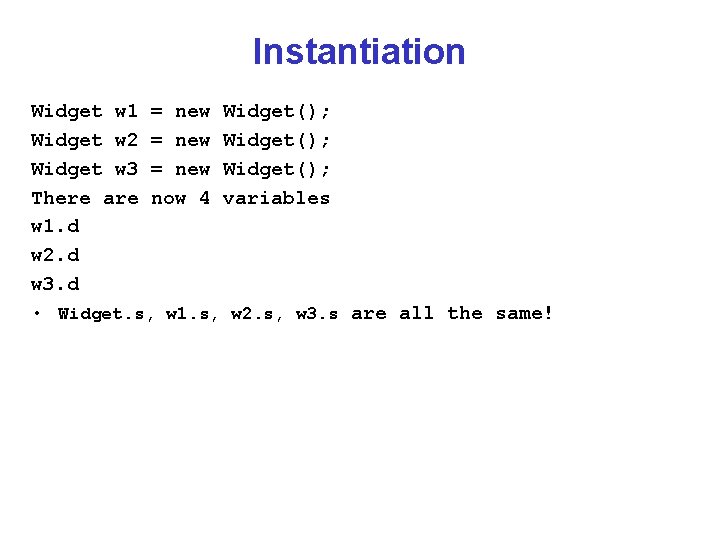
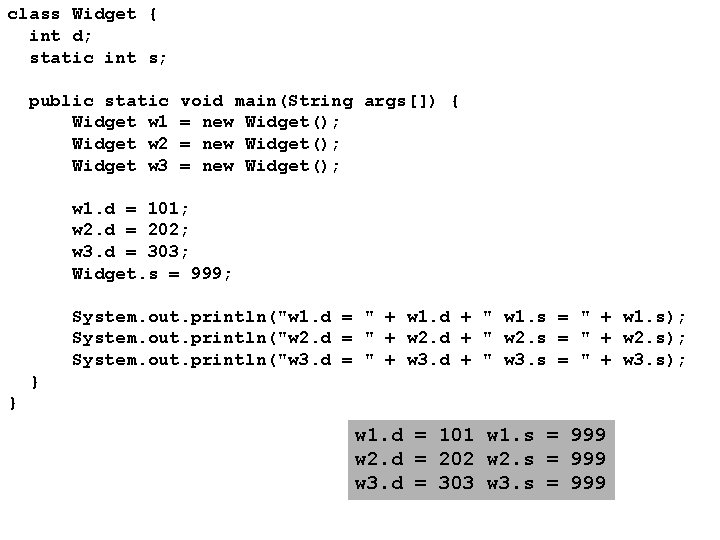
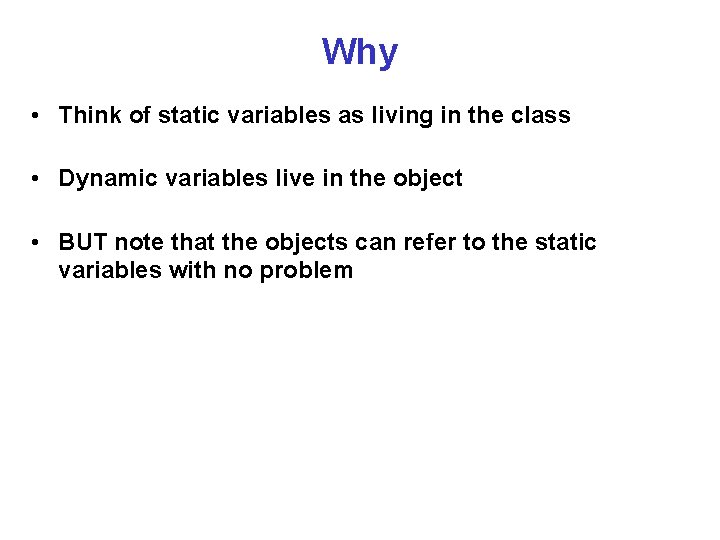
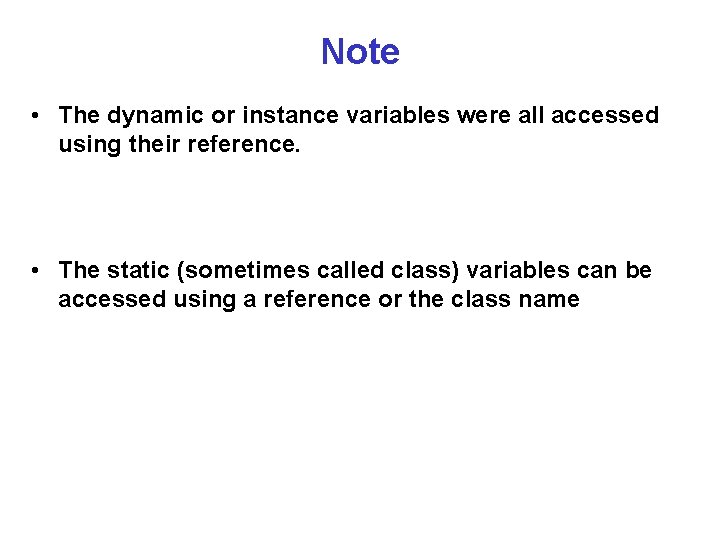
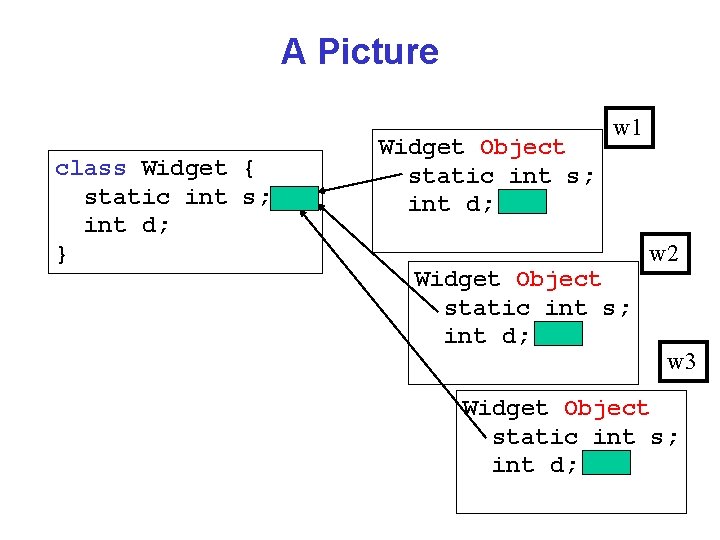
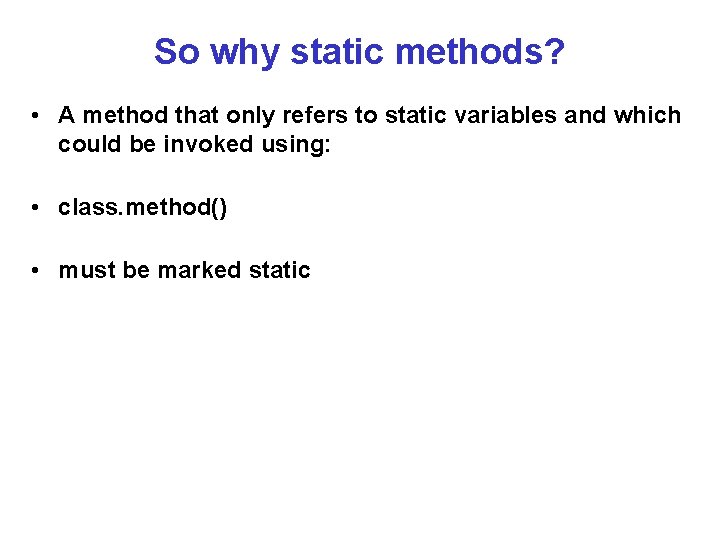
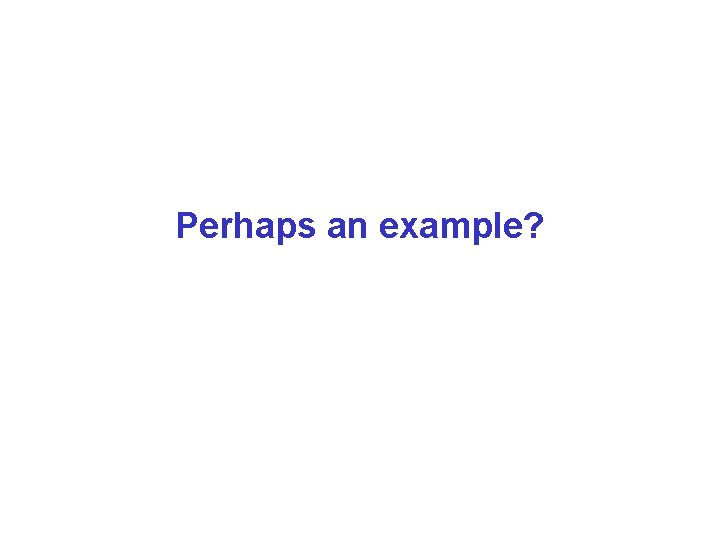
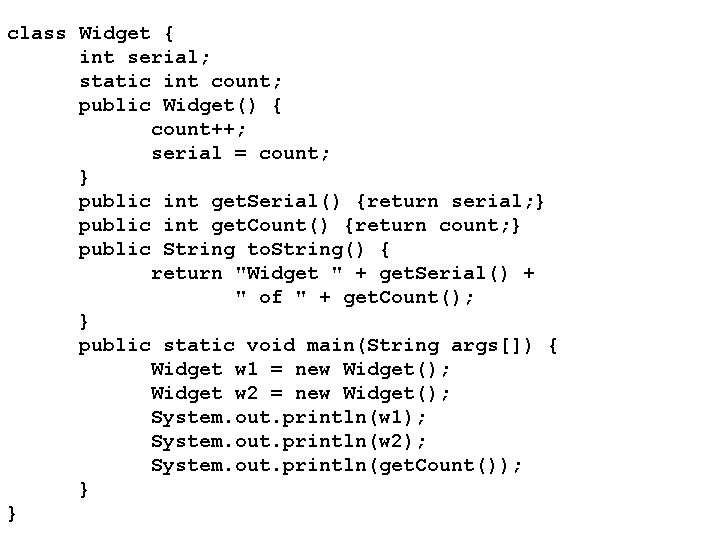
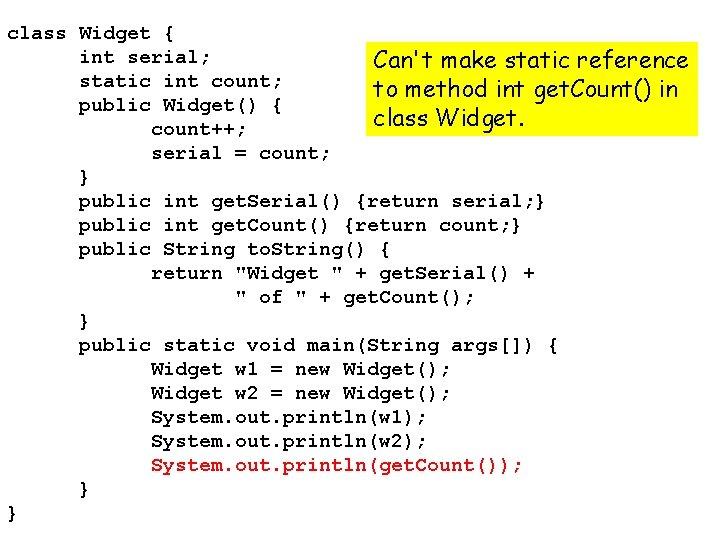
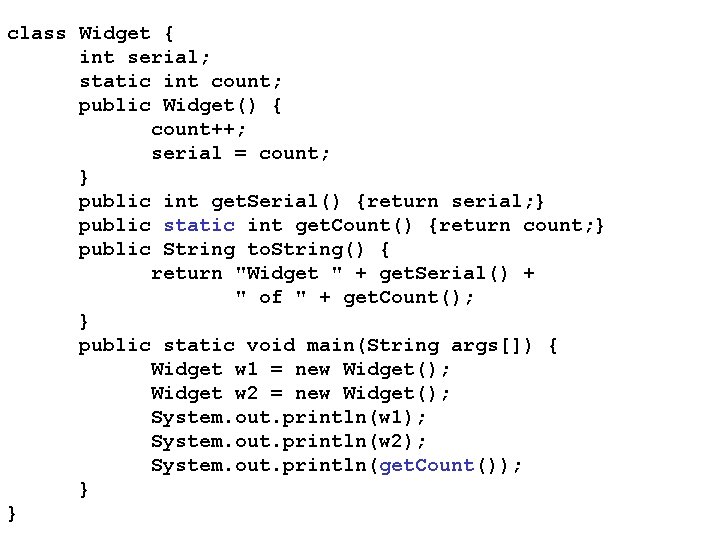
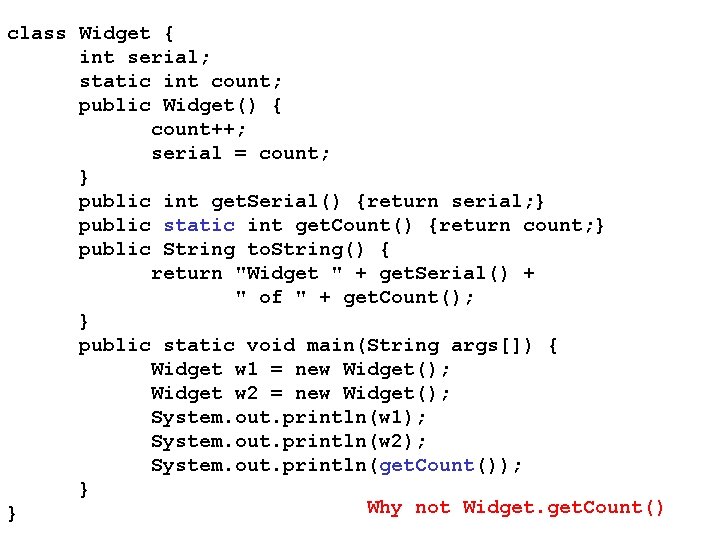
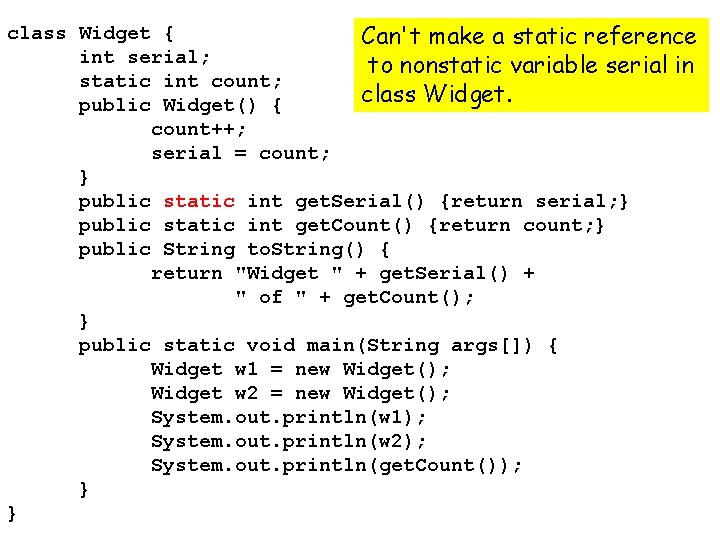
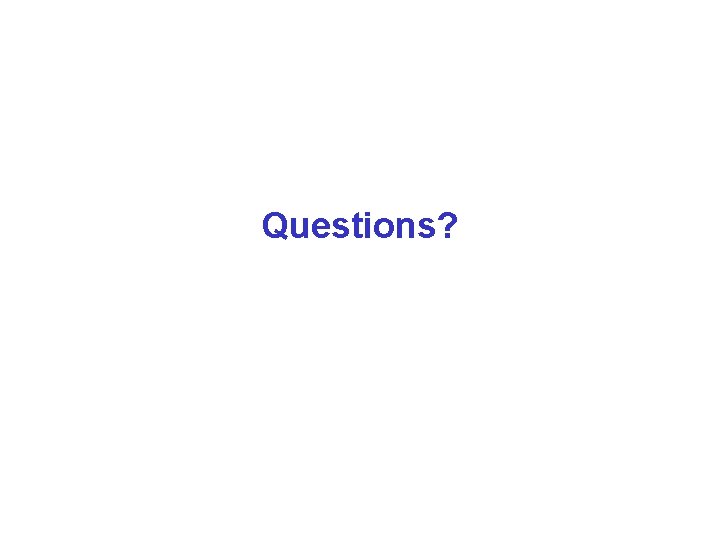
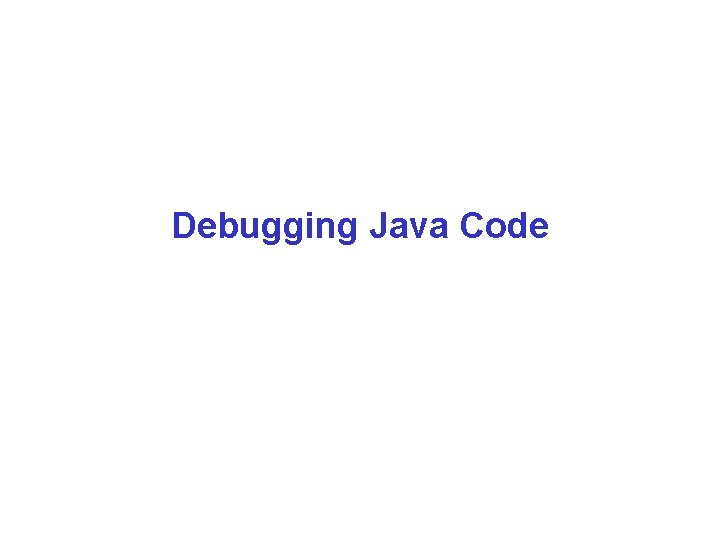
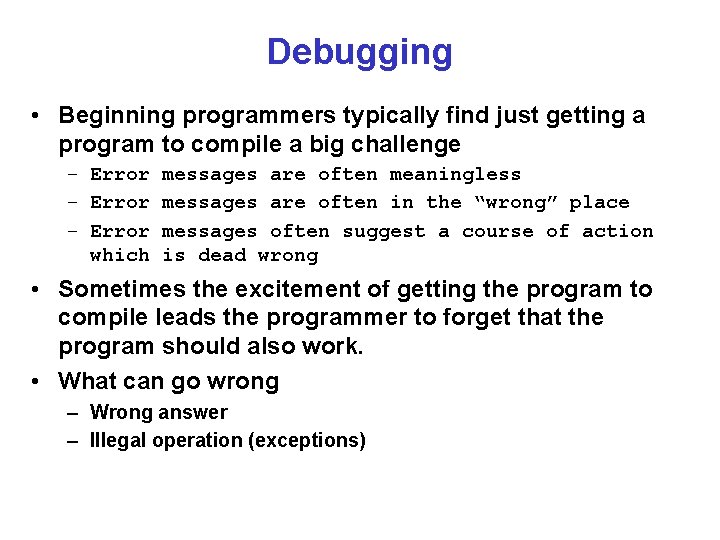
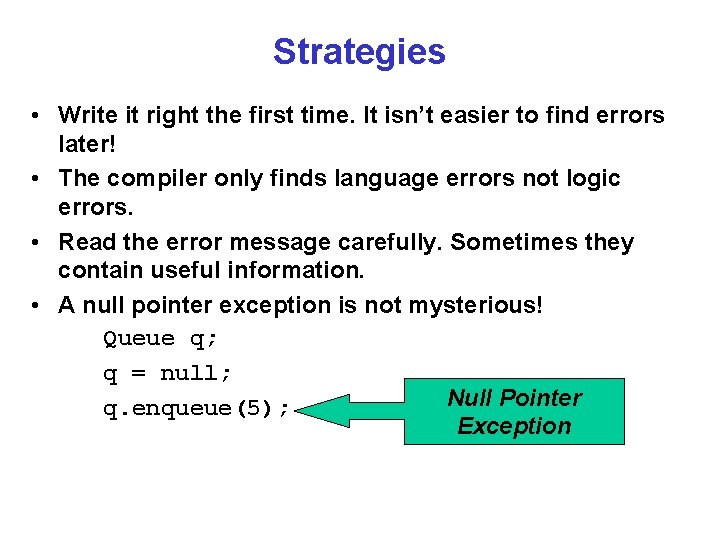
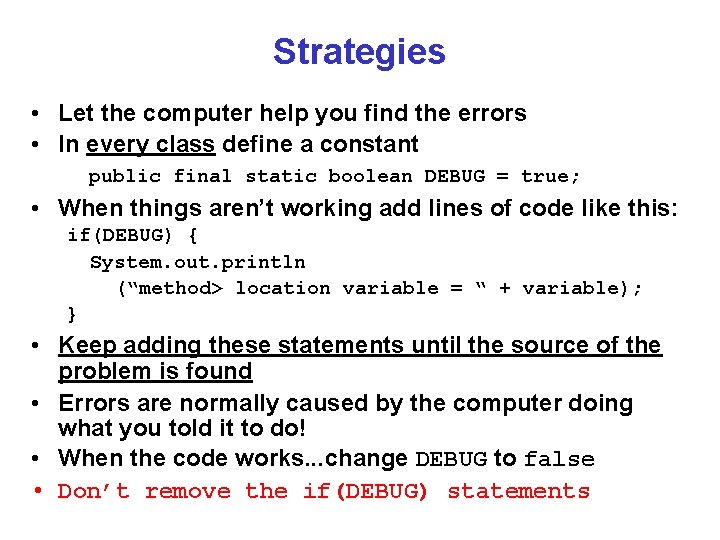
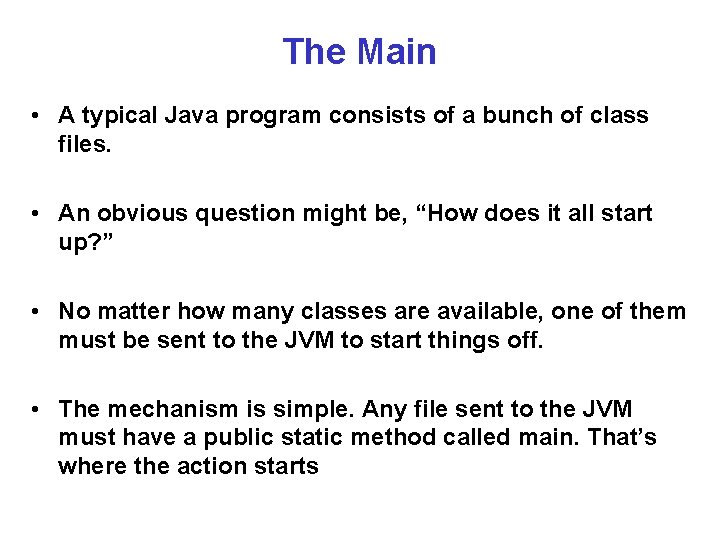
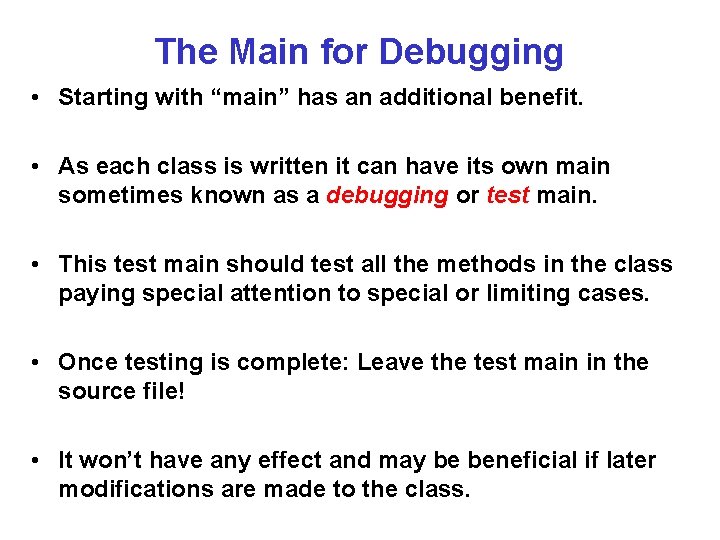
![The Main for Debugging class Driver {. . . public static void main(String args[]) The Main for Debugging class Driver {. . . public static void main(String args[])](https://slidetodoc.com/presentation_image/705e368433dc0b9052ad26b399f9d980/image-22.jpg)
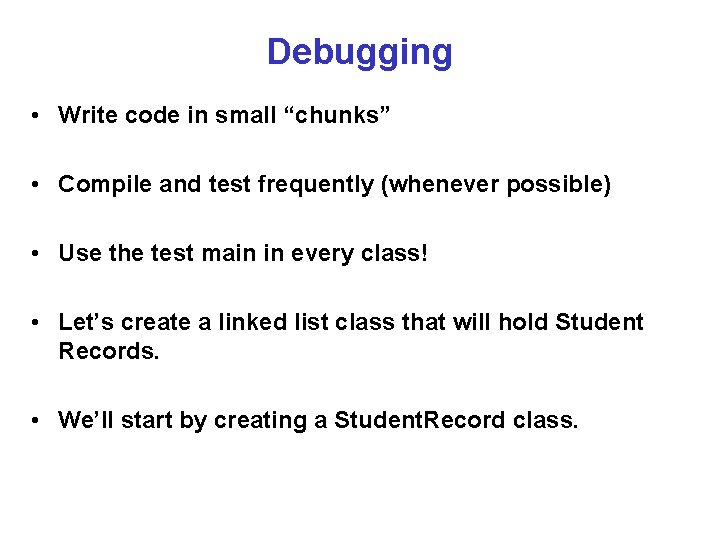
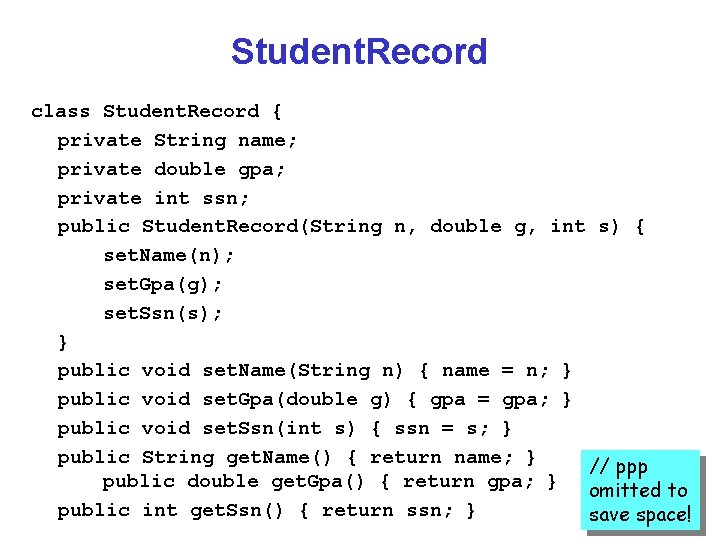
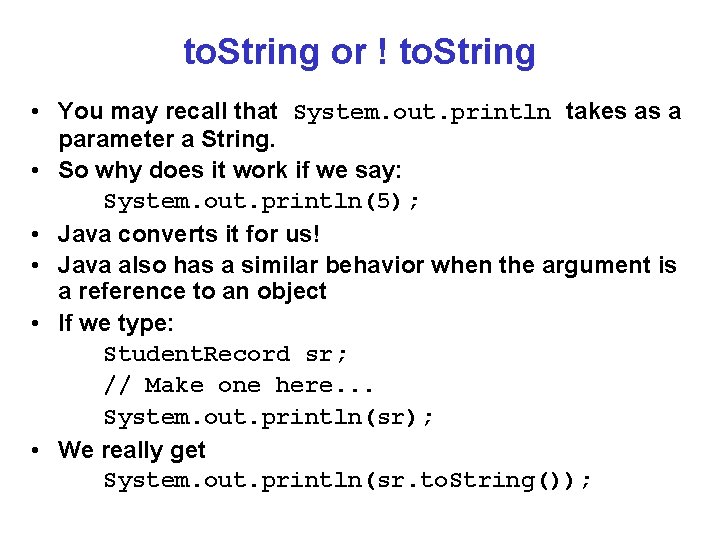
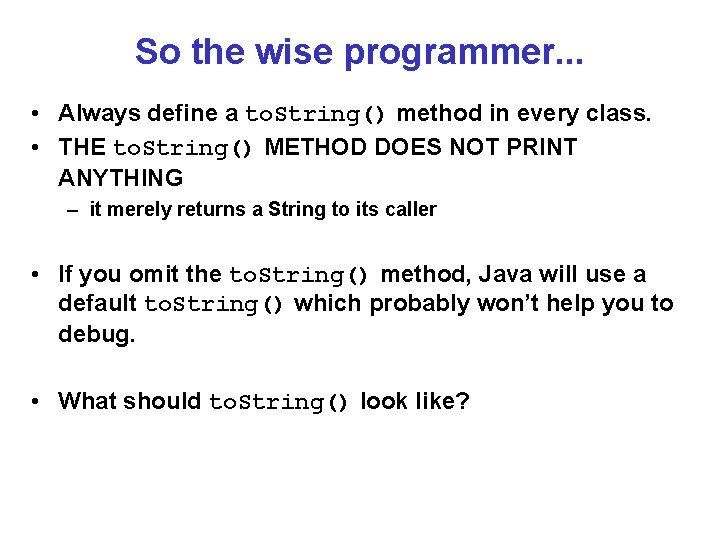
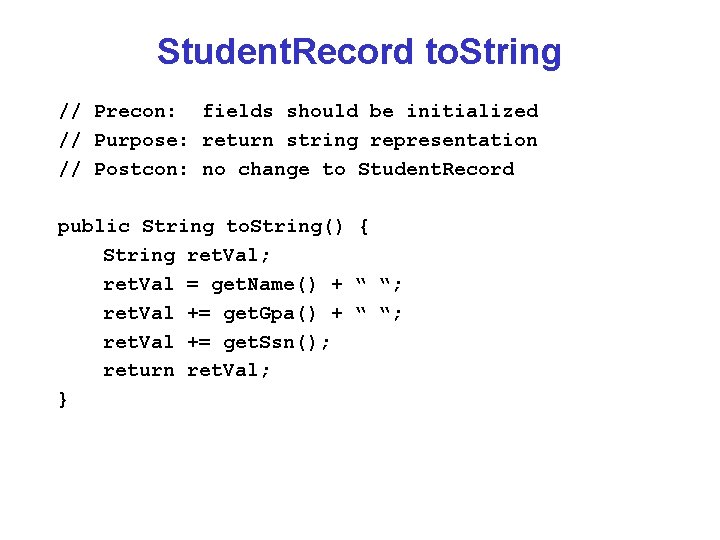
![Now the test main! // Purpose: test main public static void main(String args[]) { Now the test main! // Purpose: test main public static void main(String args[]) {](https://slidetodoc.com/presentation_image/705e368433dc0b9052ad26b399f9d980/image-28.jpg)
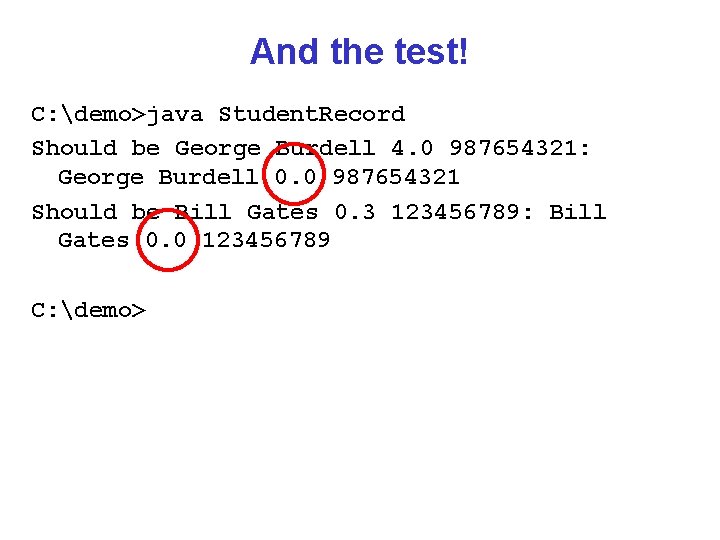
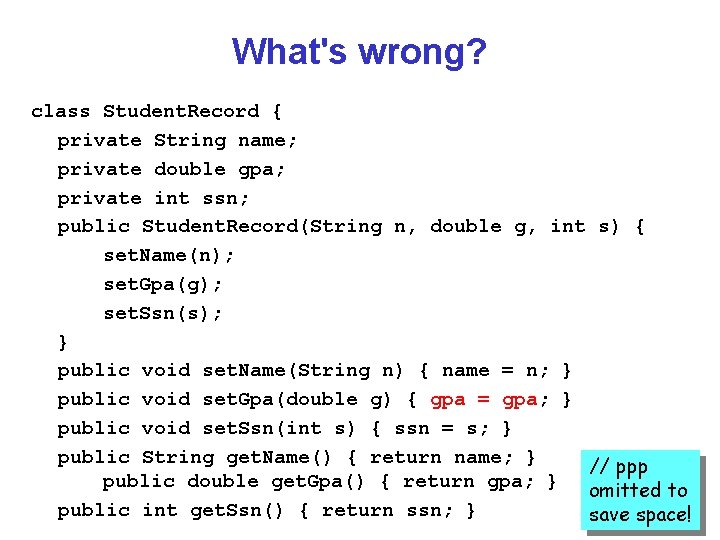
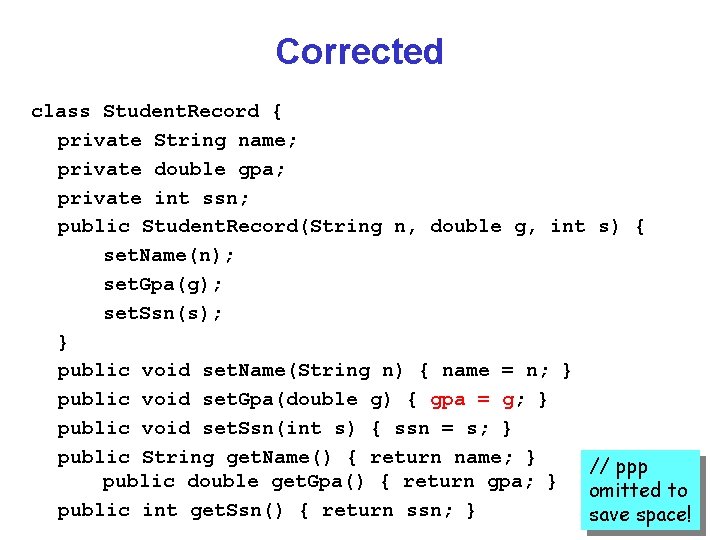
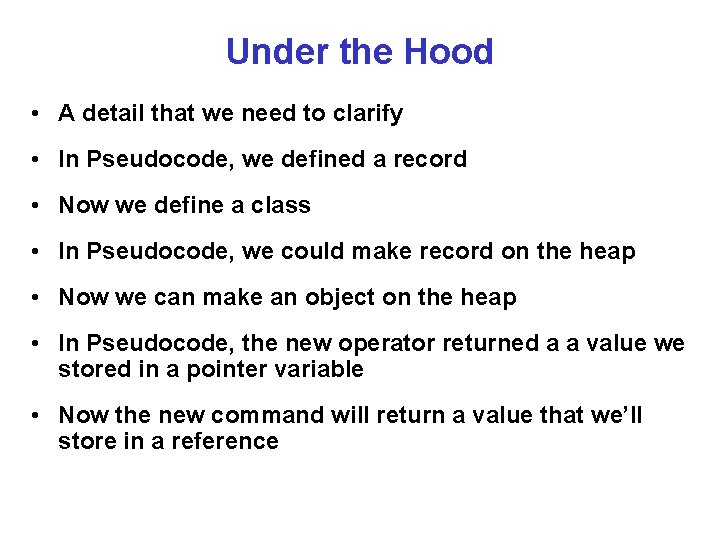
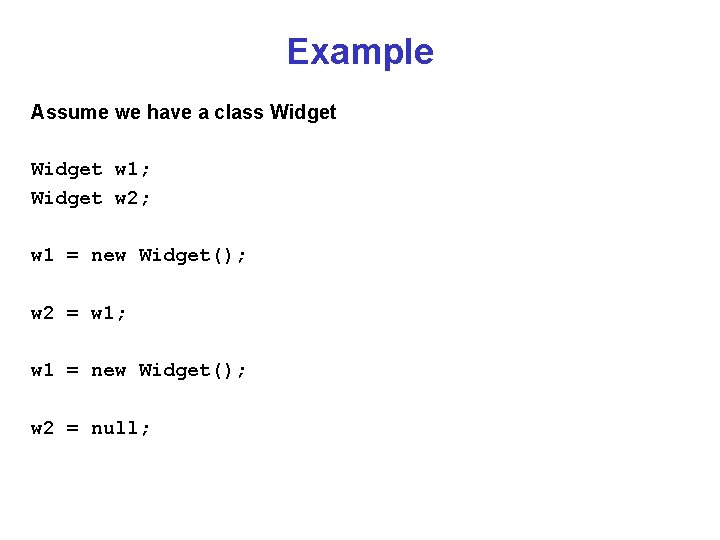
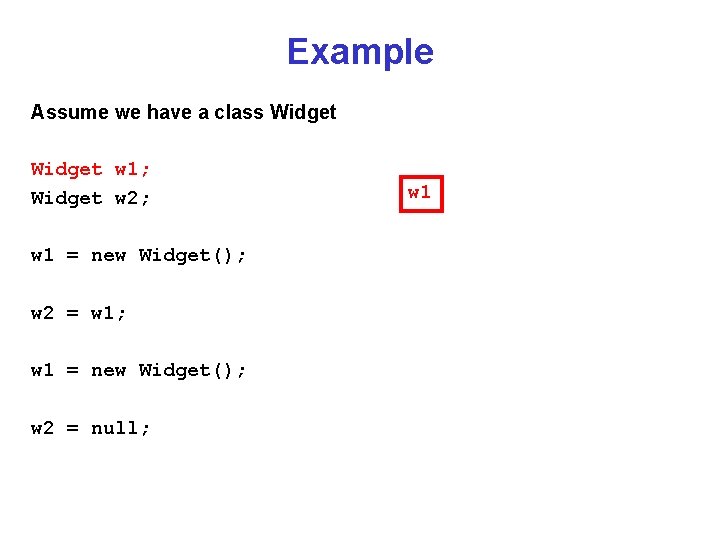
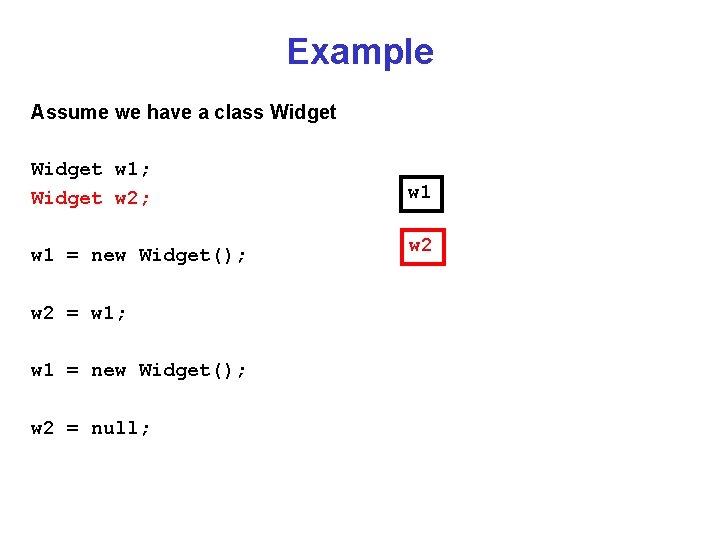
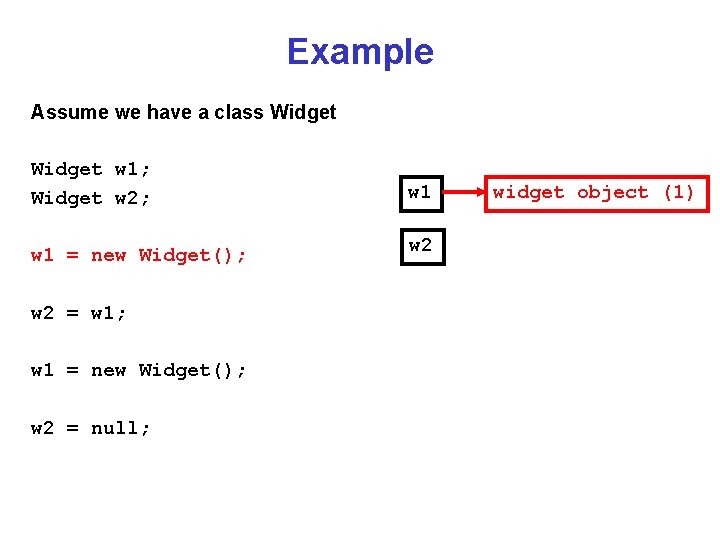
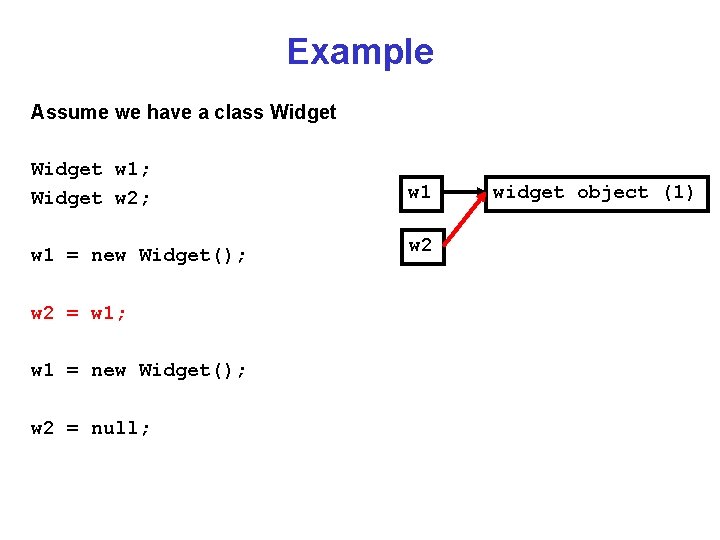
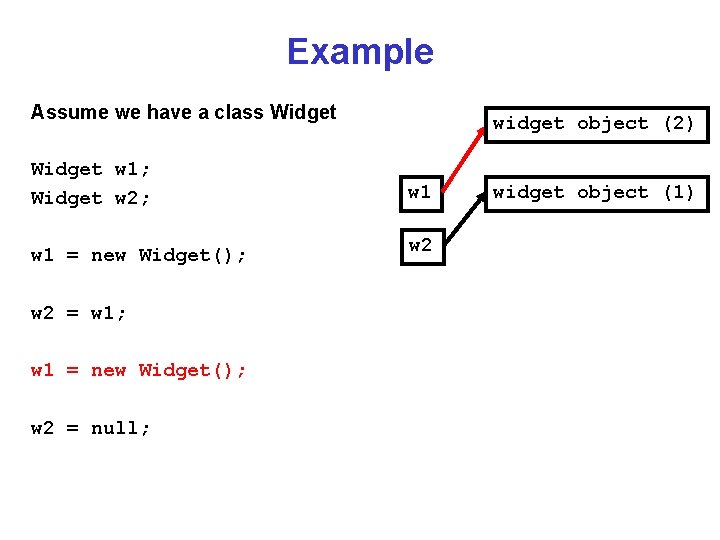
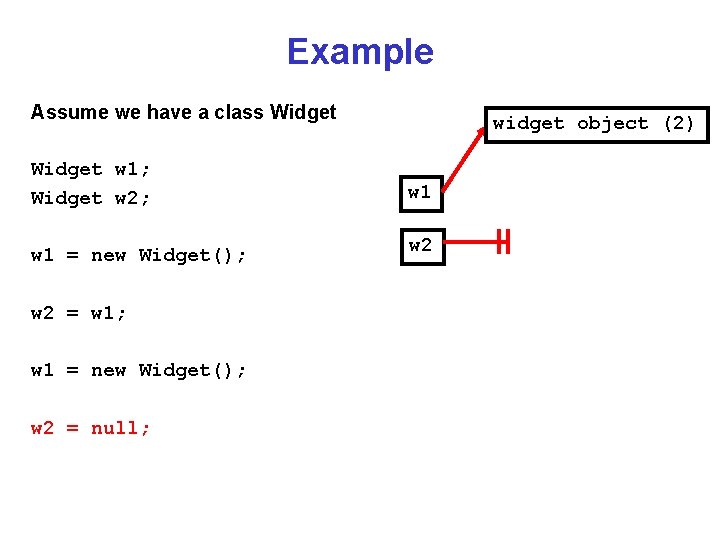
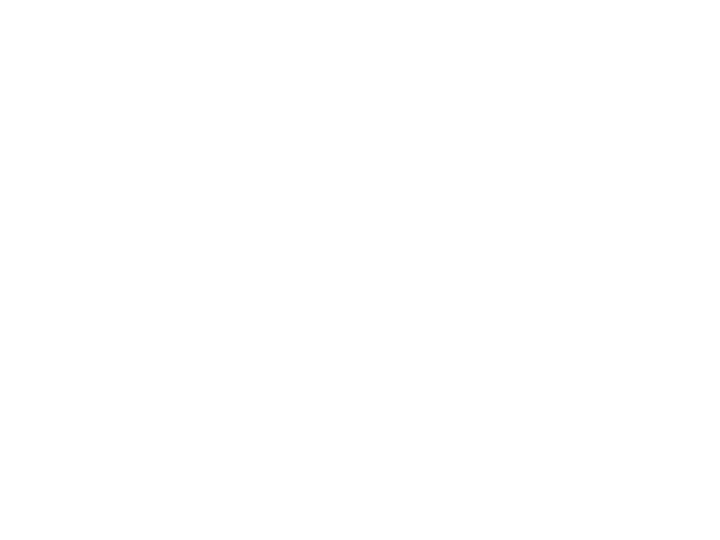
- Slides: 40
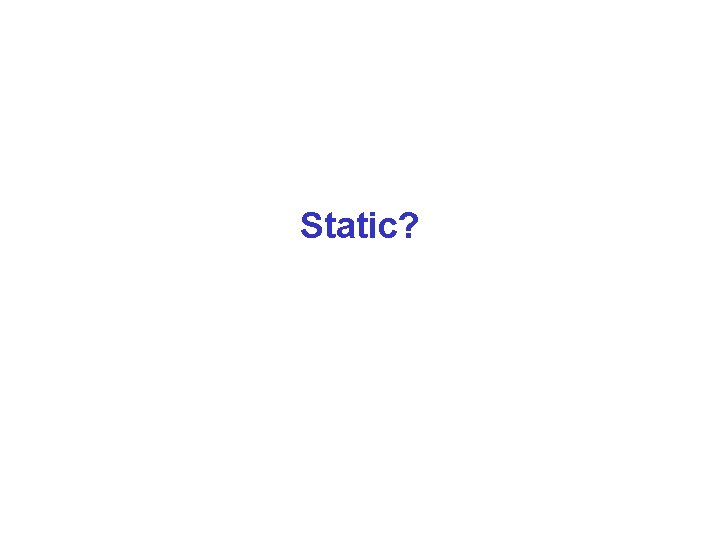
Static?
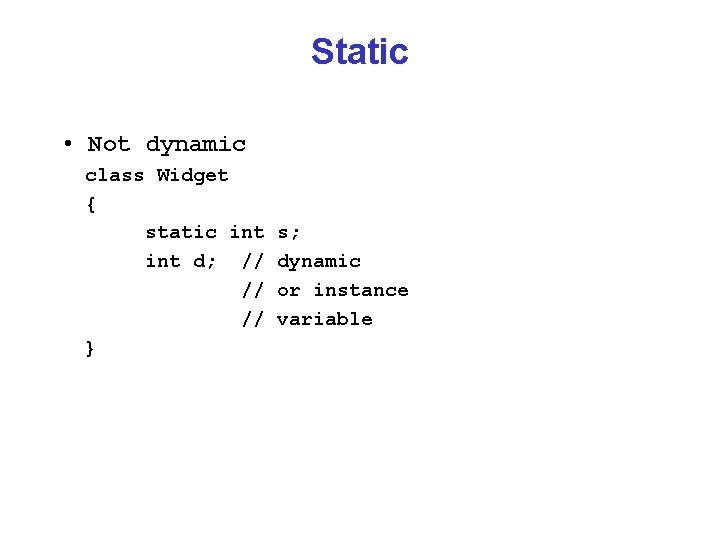
Static • Not dynamic class Widget { static int d; // // // } s; dynamic or instance variable
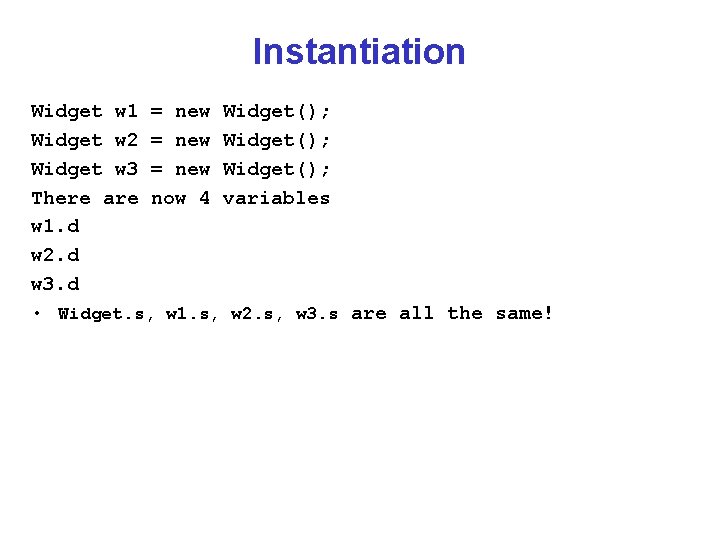
Instantiation Widget w 1 Widget w 2 Widget w 3 There are w 1. d w 2. d w 3. d = new now 4 Widget(); variables • Widget. s, w 1. s, w 2. s, w 3. s are all the same!
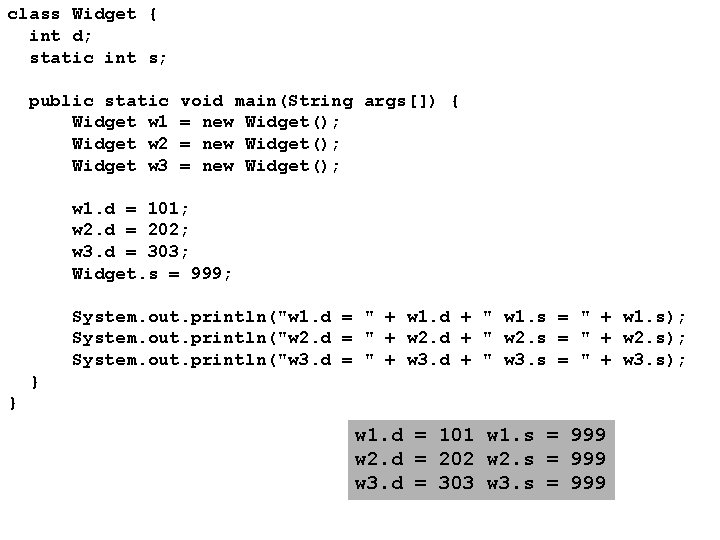
class Widget { int d; static int s; public static Widget w 1 Widget w 2 Widget w 3 void main(String args[]) { = new Widget(); w 1. d = 101; w 2. d = 202; w 3. d = 303; Widget. s = 999; System. out. println("w 1. d = " + w 1. d + " w 1. s = " + w 1. s); System. out. println("w 2. d = " + w 2. d + " w 2. s = " + w 2. s); System. out. println("w 3. d = " + w 3. d + " w 3. s = " + w 3. s); } } w 1. d = 101 w 1. s = 999 w 2. d = 202 w 2. s = 999 w 3. d = 303 w 3. s = 999
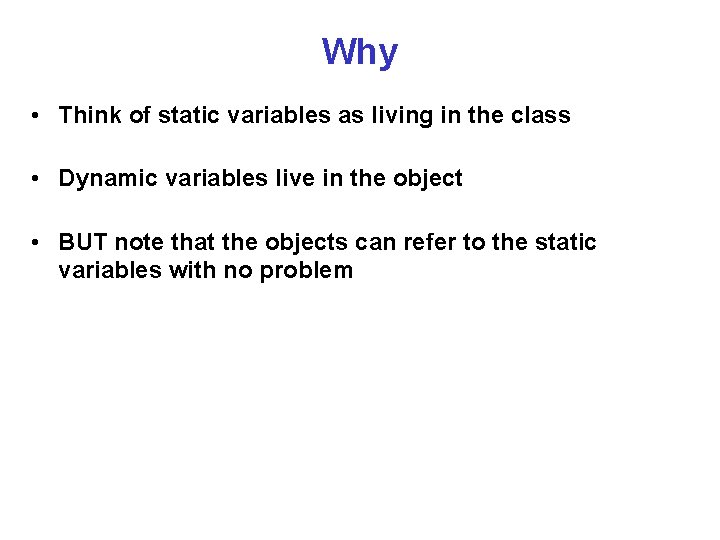
Why • Think of static variables as living in the class • Dynamic variables live in the object • BUT note that the objects can refer to the static variables with no problem
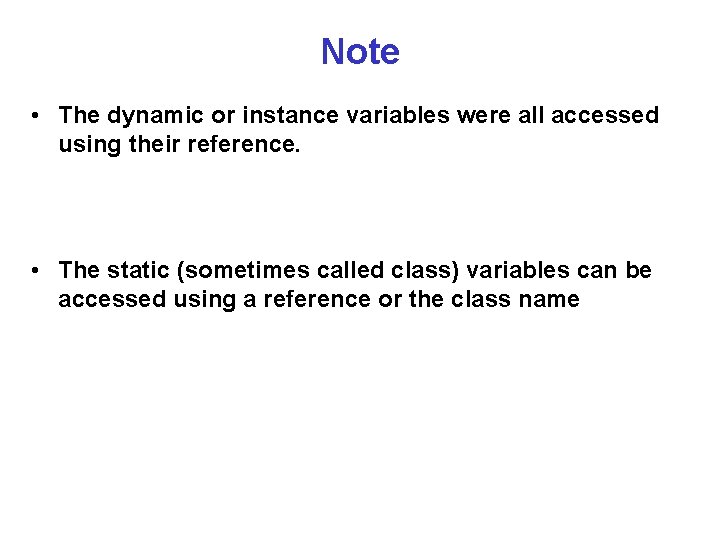
Note • The dynamic or instance variables were all accessed using their reference. • The static (sometimes called class) variables can be accessed using a reference or the class name
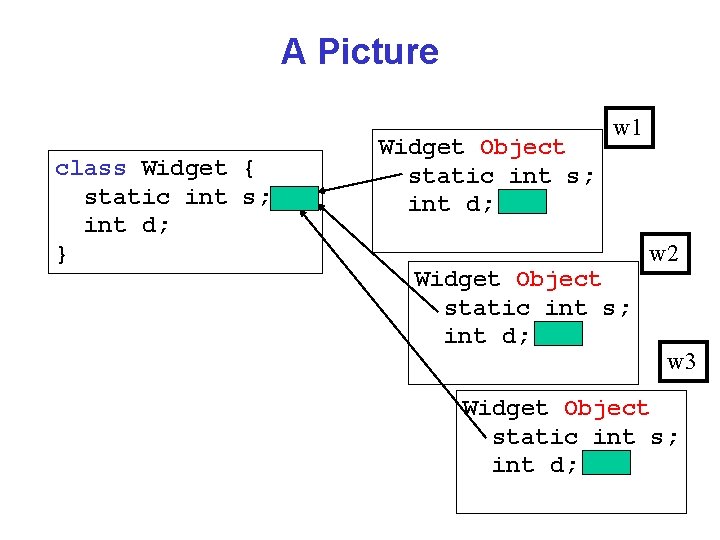
A Picture class Widget { static int s; int d; } Widget Object static int s; int d; w 1 Widget Object static int s; int d; w 2 w 3 Widget Object static int s; int d;
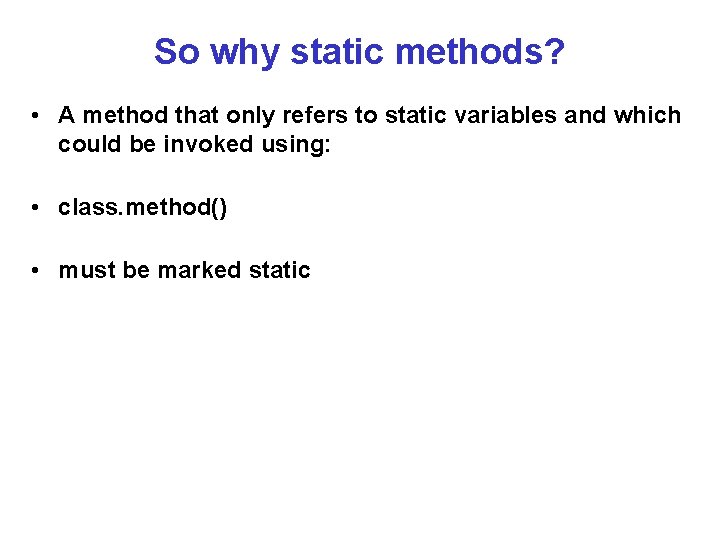
So why static methods? • A method that only refers to static variables and which could be invoked using: • class. method() • must be marked static
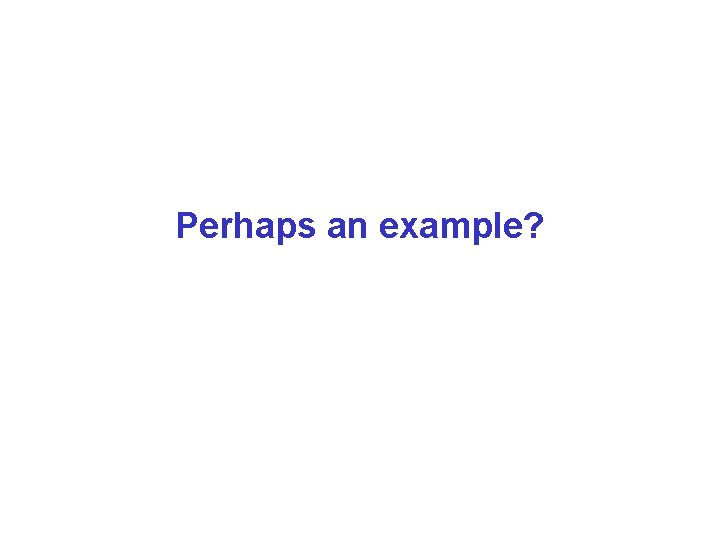
Perhaps an example?
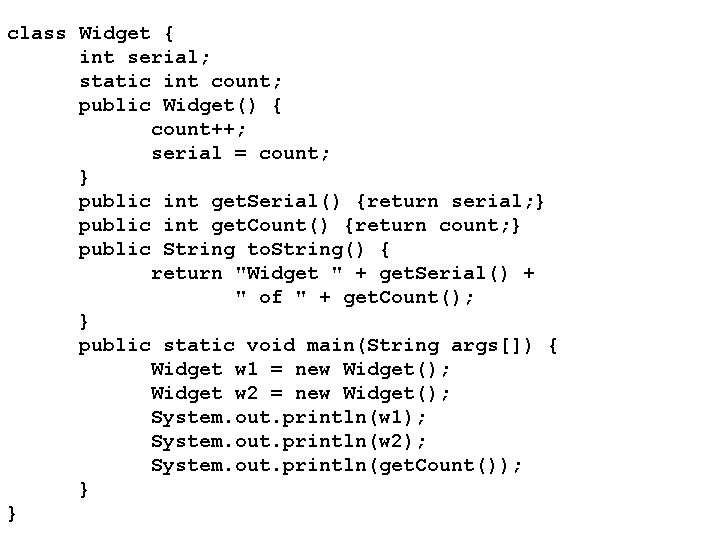
class Widget { int serial; static int count; public Widget() { count++; serial = count; } public int get. Serial() {return serial; } public int get. Count() {return count; } public String to. String() { return "Widget " + get. Serial() + " of " + get. Count(); } public static void main(String args[]) { Widget w 1 = new Widget(); Widget w 2 = new Widget(); System. out. println(w 1); System. out. println(w 2); System. out. println(get. Count()); } }
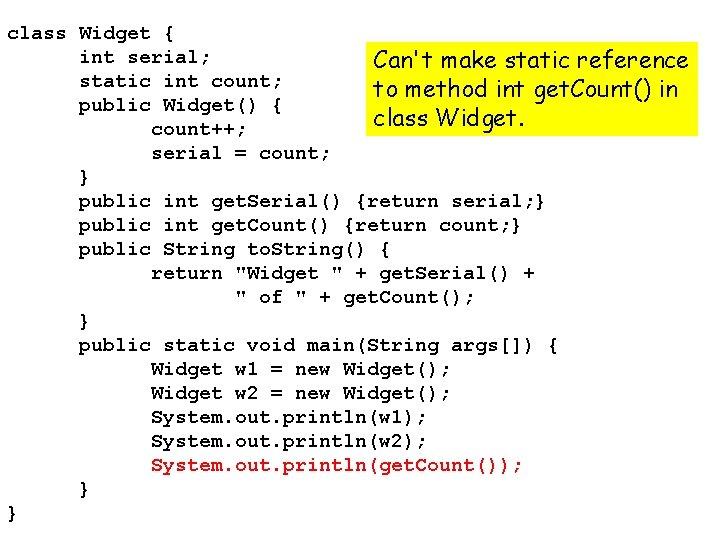
class Widget { int serial; Can't make static reference static int count; to method int get. Count() in public Widget() { class Widget. count++; serial = count; } public int get. Serial() {return serial; } public int get. Count() {return count; } public String to. String() { return "Widget " + get. Serial() + " of " + get. Count(); } public static void main(String args[]) { Widget w 1 = new Widget(); Widget w 2 = new Widget(); System. out. println(w 1); System. out. println(w 2); System. out. println(get. Count()); } }
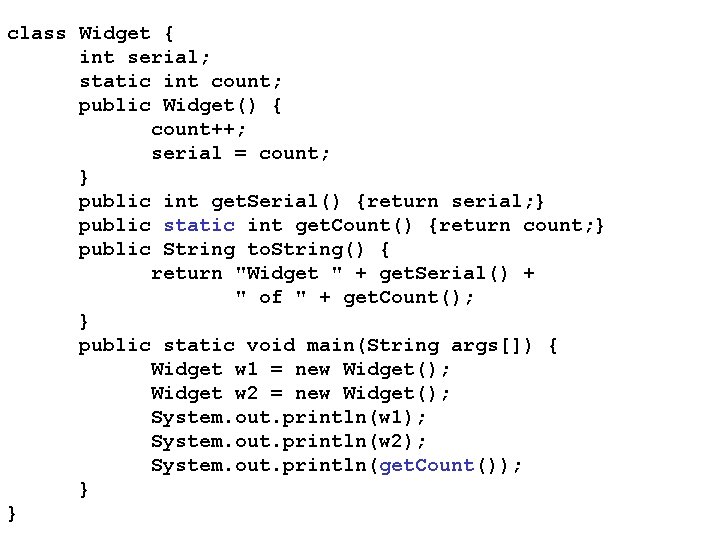
class Widget { int serial; static int count; public Widget() { count++; serial = count; } public int get. Serial() {return serial; } public static int get. Count() {return count; } public String to. String() { return "Widget " + get. Serial() + " of " + get. Count(); } public static void main(String args[]) { Widget w 1 = new Widget(); Widget w 2 = new Widget(); System. out. println(w 1); System. out. println(w 2); System. out. println(get. Count()); } }
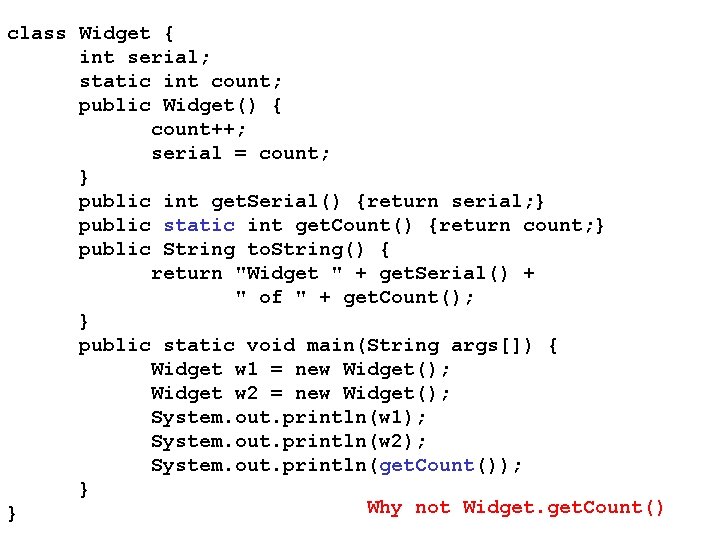
class Widget { int serial; static int count; public Widget() { count++; serial = count; } public int get. Serial() {return serial; } public static int get. Count() {return count; } public String to. String() { return "Widget " + get. Serial() + " of " + get. Count(); } public static void main(String args[]) { Widget w 1 = new Widget(); Widget w 2 = new Widget(); System. out. println(w 1); System. out. println(w 2); System. out. println(get. Count()); } Why not Widget. Count() }
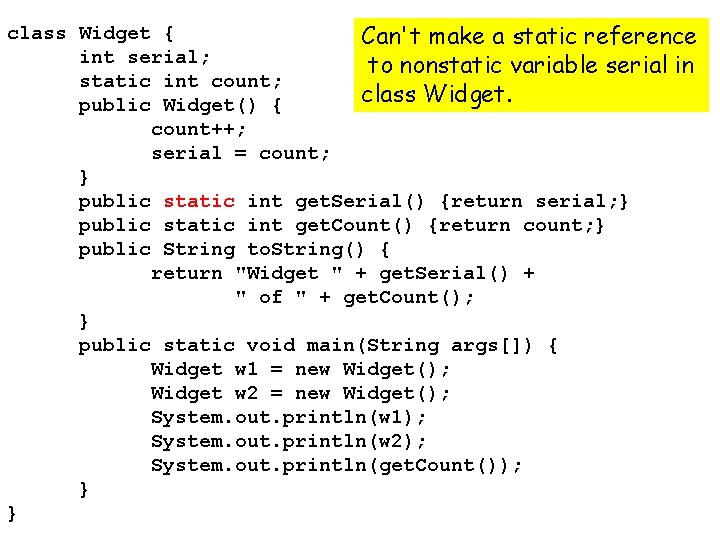
class Widget { Can't make a static reference int serial; to nonstatic variable serial in static int count; class Widget. public Widget() { count++; serial = count; } public static int get. Serial() {return serial; } public static int get. Count() {return count; } public String to. String() { return "Widget " + get. Serial() + " of " + get. Count(); } public static void main(String args[]) { Widget w 1 = new Widget(); Widget w 2 = new Widget(); System. out. println(w 1); System. out. println(w 2); System. out. println(get. Count()); } }
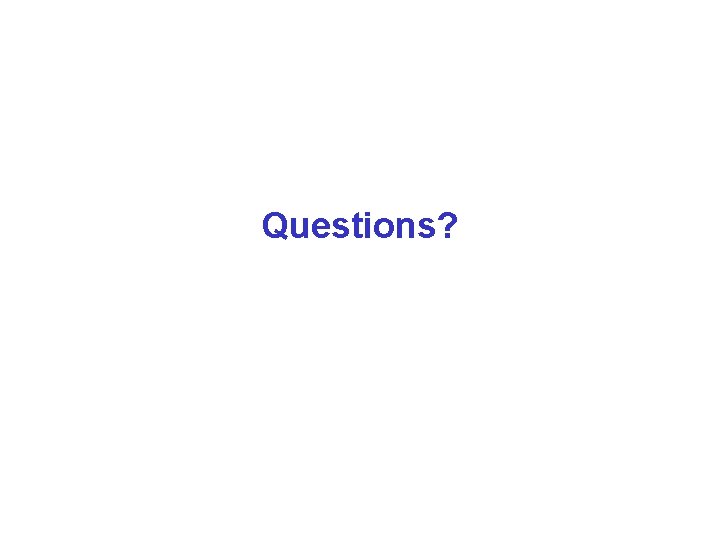
Questions?
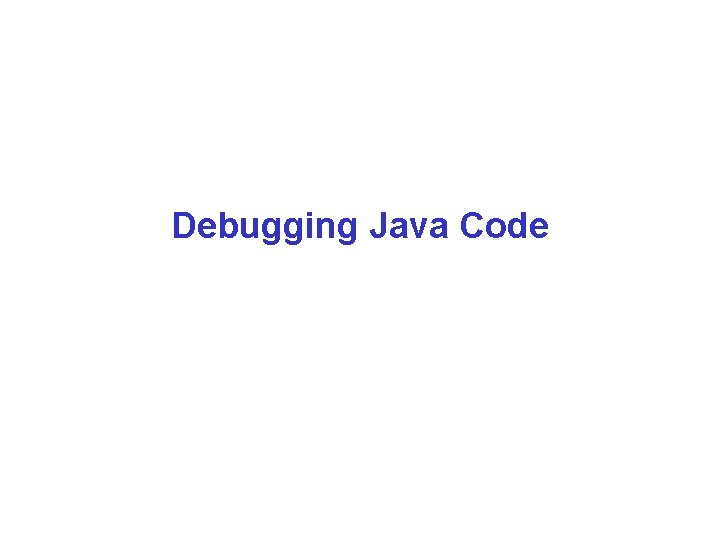
Debugging Java Code
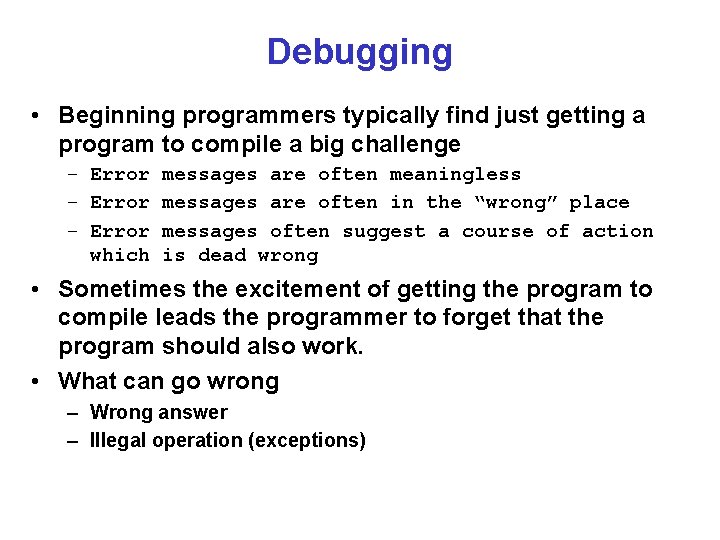
Debugging • Beginning programmers typically find just getting a program to compile a big challenge – Error messages are often meaningless – Error messages are often in the “wrong” place – Error messages often suggest a course of action which is dead wrong • Sometimes the excitement of getting the program to compile leads the programmer to forget that the program should also work. • What can go wrong – Wrong answer – Illegal operation (exceptions)
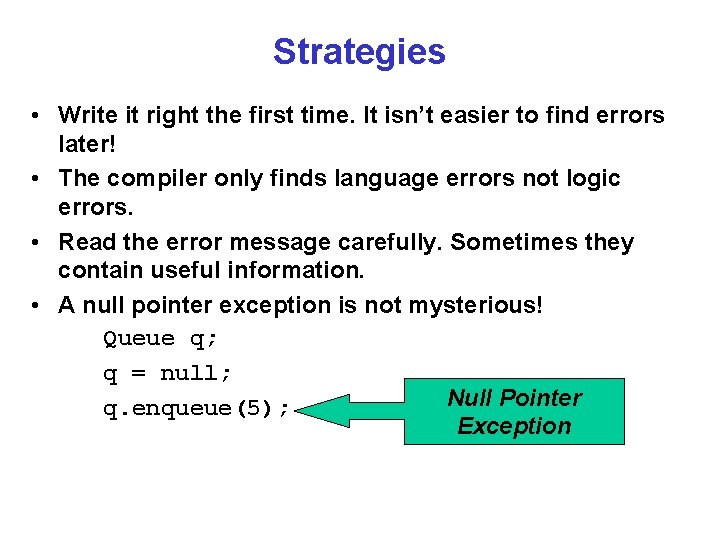
Strategies • Write it right the first time. It isn’t easier to find errors later! • The compiler only finds language errors not logic errors. • Read the error message carefully. Sometimes they contain useful information. • A null pointer exception is not mysterious! Queue q; q = null; Null Pointer q. enqueue(5); Exception
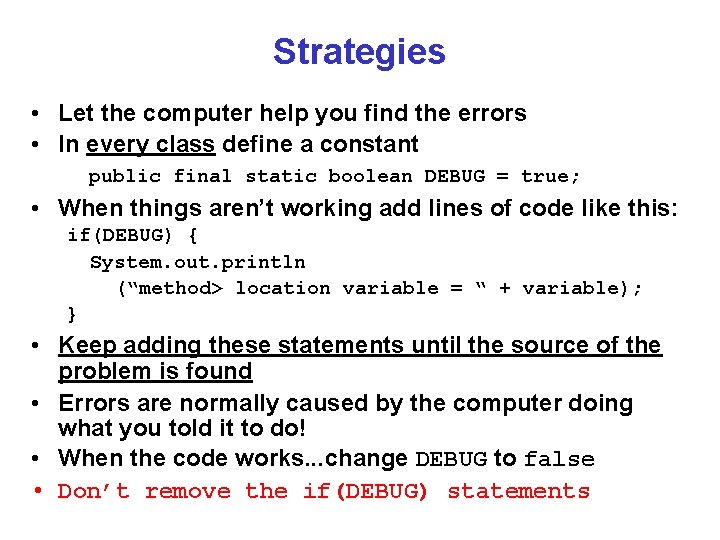
Strategies • Let the computer help you find the errors • In every class define a constant public final static boolean DEBUG = true; • When things aren’t working add lines of code like this: if(DEBUG) { System. out. println (“method> location variable = “ + variable); } • Keep adding these statements until the source of the problem is found • Errors are normally caused by the computer doing what you told it to do! • When the code works. . . change DEBUG to false • Don’t remove the if(DEBUG) statements
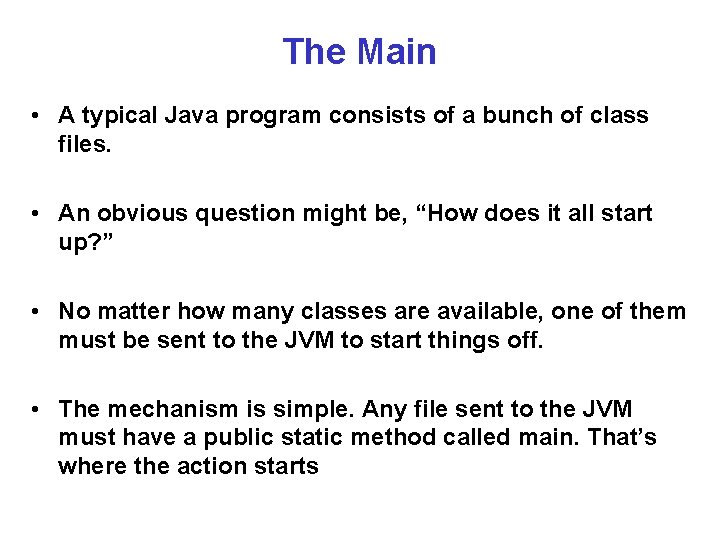
The Main • A typical Java program consists of a bunch of class files. • An obvious question might be, “How does it all start up? ” • No matter how many classes are available, one of them must be sent to the JVM to start things off. • The mechanism is simple. Any file sent to the JVM must have a public static method called main. That’s where the action starts
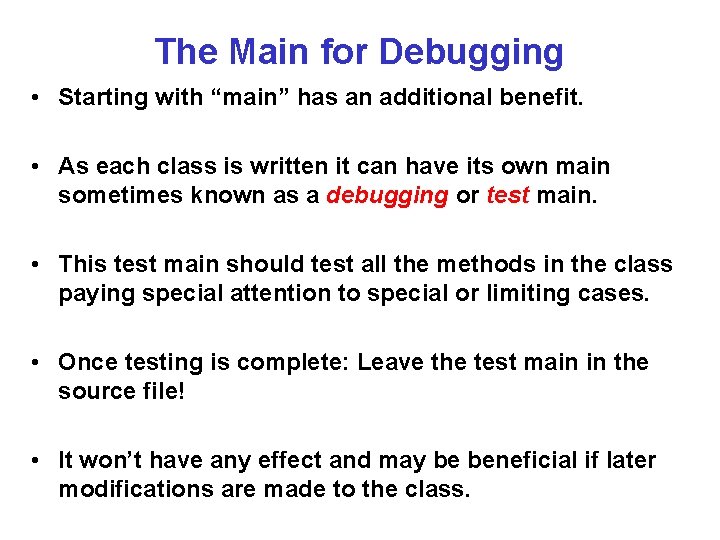
The Main for Debugging • Starting with “main” has an additional benefit. • As each class is written it can have its own main sometimes known as a debugging or test main. • This test main should test all the methods in the class paying special attention to special or limiting cases. • Once testing is complete: Leave the test main in the source file! • It won’t have any effect and may be beneficial if later modifications are made to the class.
![The Main for Debugging class Driver public static void mainString args The Main for Debugging class Driver {. . . public static void main(String args[])](https://slidetodoc.com/presentation_image/705e368433dc0b9052ad26b399f9d980/image-22.jpg)
The Main for Debugging class Driver {. . . public static void main(String args[]) {. . . } class Stack { }. . . public static void main(String args[]) {. . . } class Menu { }. . . public static void main(String args[]) {. . . } class Widget { } . . . public static void main(String args[]) {. . . } class Blivet { } . . . public static void main(String args[]) {. . . } }
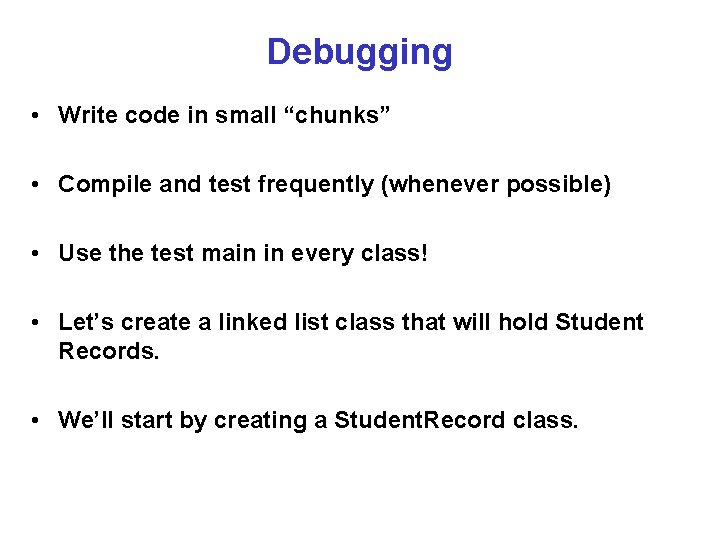
Debugging • Write code in small “chunks” • Compile and test frequently (whenever possible) • Use the test main in every class! • Let’s create a linked list class that will hold Student Records. • We’ll start by creating a Student. Record class.
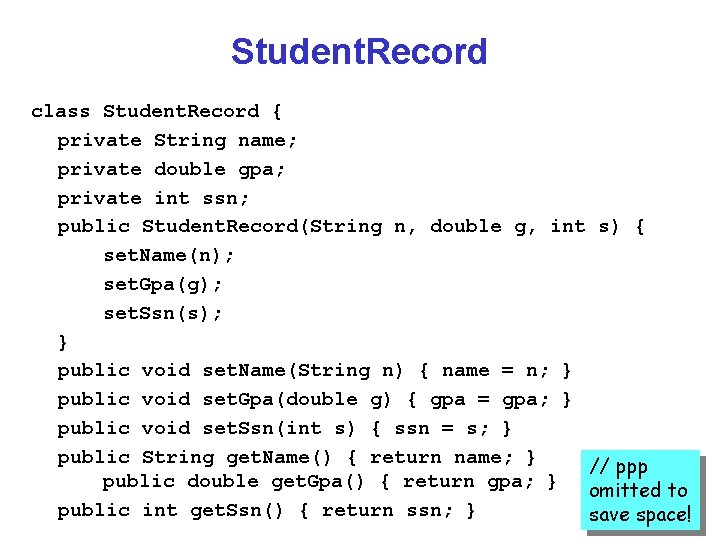
Student. Record class Student. Record { private String name; private double gpa; private int ssn; public Student. Record(String n, double g, int s) { set. Name(n); set. Gpa(g); set. Ssn(s); } public void set. Name(String n) { name = n; } public void set. Gpa(double g) { gpa = gpa; } public void set. Ssn(int s) { ssn = s; } public String get. Name() { return name; } // ppp public double get. Gpa() { return gpa; } omitted to public int get. Ssn() { return ssn; } save space!
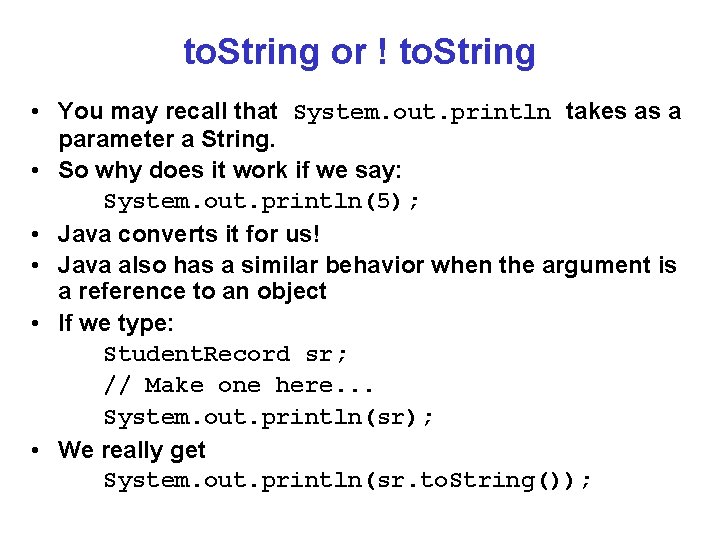
to. String or ! to. String • You may recall that System. out. println takes as a parameter a String. • So why does it work if we say: System. out. println(5); • Java converts it for us! • Java also has a similar behavior when the argument is a reference to an object • If we type: Student. Record sr; // Make one here. . . System. out. println(sr); • We really get System. out. println(sr. to. String());
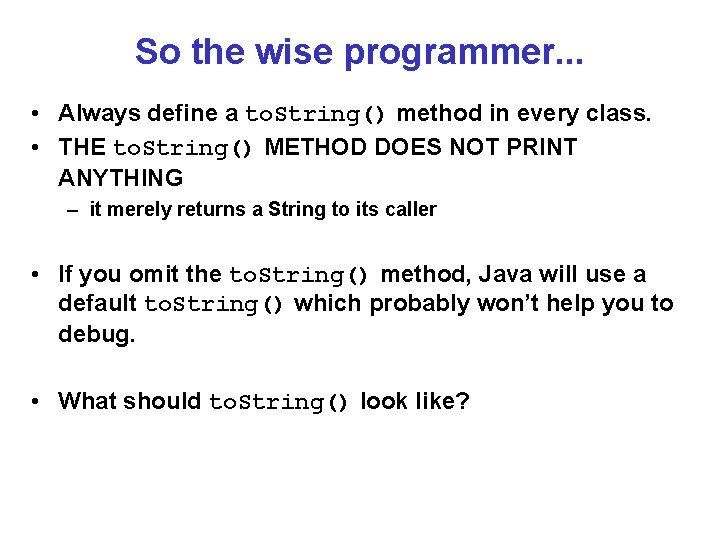
So the wise programmer. . . • Always define a to. String() method in every class. • THE to. String() METHOD DOES NOT PRINT ANYTHING – it merely returns a String to its caller • If you omit the to. String() method, Java will use a default to. String() which probably won’t help you to debug. • What should to. String() look like?
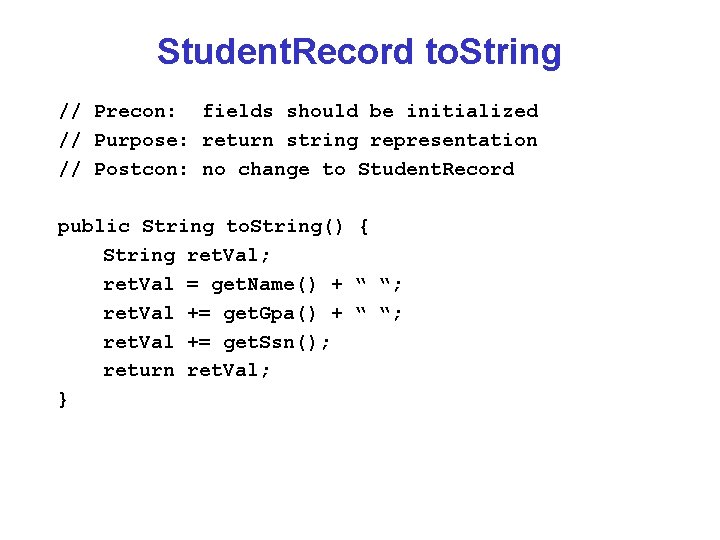
Student. Record to. String // Precon: fields should be initialized // Purpose: return string representation // Postcon: no change to Student. Record public String to. String() { String ret. Val; ret. Val = get. Name() + “ “; ret. Val += get. Gpa() + “ “; ret. Val += get. Ssn(); return ret. Val; }
![Now the test main Purpose test main public static void mainString args Now the test main! // Purpose: test main public static void main(String args[]) {](https://slidetodoc.com/presentation_image/705e368433dc0b9052ad26b399f9d980/image-28.jpg)
Now the test main! // Purpose: test main public static void main(String args[]) { Student. Record sr; sr = new Student. Record( "George Burdell", 4. 0, 987654321); System. out. println( "Should be George Burdell 4. 0 987654321: " + sr); sr. set. Name("Bill Gates"); sr. set. Ssn(123456789); sr. set. Gpa(0. 3); System. out. println( "Should be Bill Gates 0. 3 123456789: " + sr); } } // Studentrecord
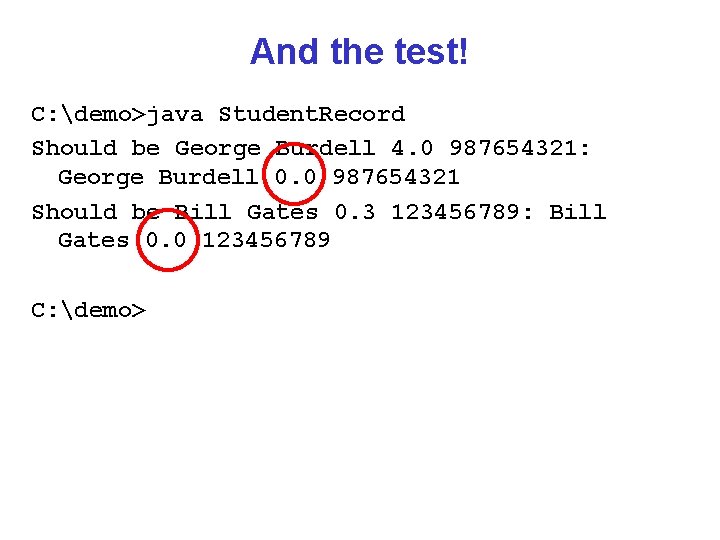
And the test! C: demo>java Student. Record Should be George Burdell 4. 0 987654321: George Burdell 0. 0 987654321 Should be Bill Gates 0. 3 123456789: Bill Gates 0. 0 123456789 C: demo>
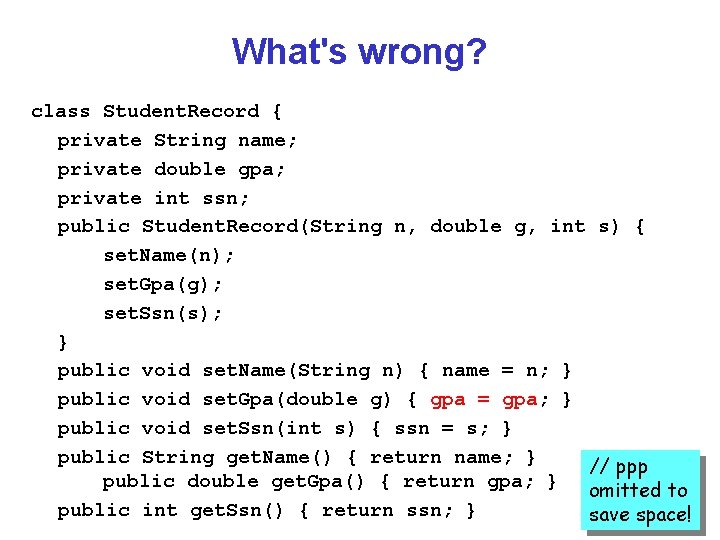
What's wrong? class Student. Record { private String name; private double gpa; private int ssn; public Student. Record(String n, double g, int s) { set. Name(n); set. Gpa(g); set. Ssn(s); } public void set. Name(String n) { name = n; } public void set. Gpa(double g) { gpa = gpa; } public void set. Ssn(int s) { ssn = s; } public String get. Name() { return name; } // ppp public double get. Gpa() { return gpa; } omitted to public int get. Ssn() { return ssn; } save space!
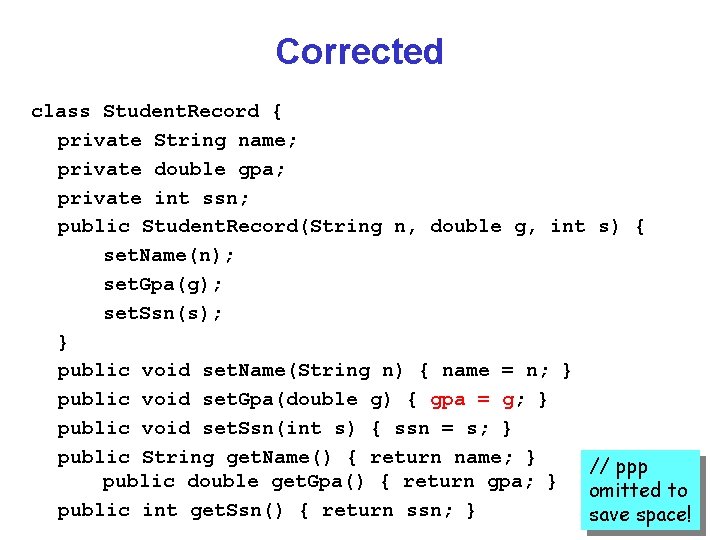
Corrected class Student. Record { private String name; private double gpa; private int ssn; public Student. Record(String n, double g, int s) { set. Name(n); set. Gpa(g); set. Ssn(s); } public void set. Name(String n) { name = n; } public void set. Gpa(double g) { gpa = g; } public void set. Ssn(int s) { ssn = s; } public String get. Name() { return name; } // ppp public double get. Gpa() { return gpa; } omitted to public int get. Ssn() { return ssn; } save space!
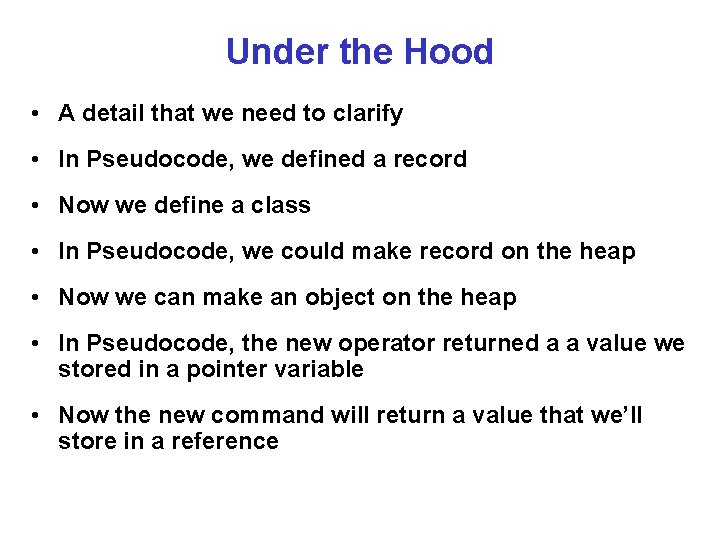
Under the Hood • A detail that we need to clarify • In Pseudocode, we defined a record • Now we define a class • In Pseudocode, we could make record on the heap • Now we can make an object on the heap • In Pseudocode, the new operator returned a a value we stored in a pointer variable • Now the new command will return a value that we’ll store in a reference
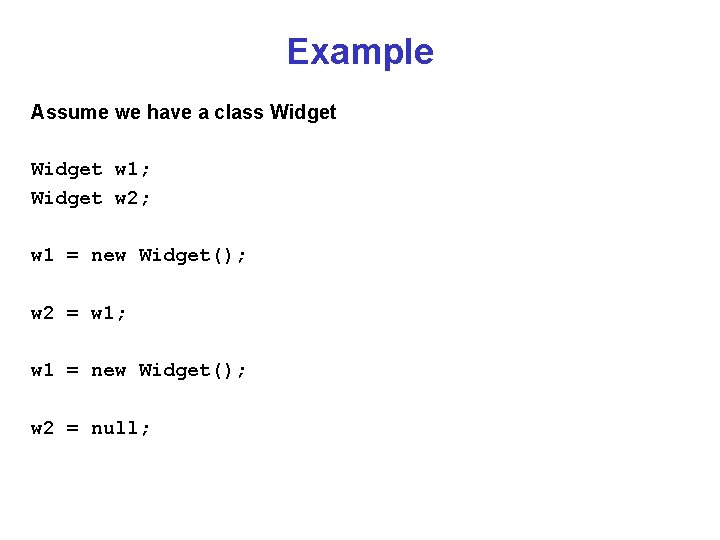
Example Assume we have a class Widget w 1; Widget w 2; w 1 = new Widget(); w 2 = w 1; w 1 = new Widget(); w 2 = null;
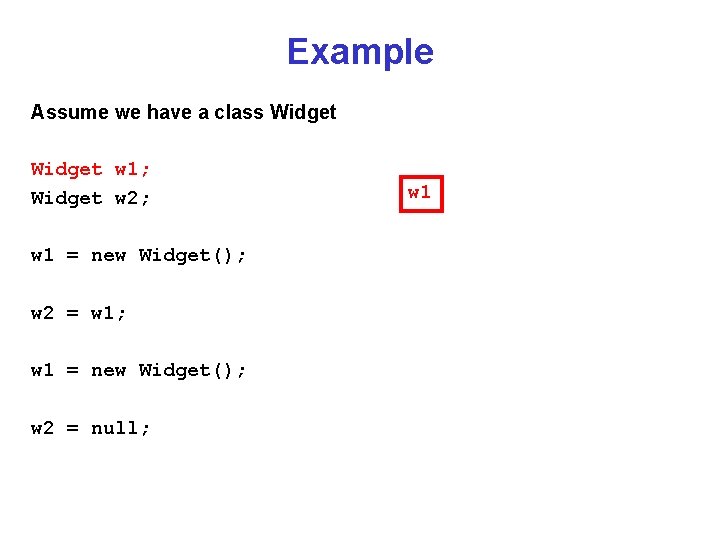
Example Assume we have a class Widget w 1; Widget w 2; w 1 = new Widget(); w 2 = w 1; w 1 = new Widget(); w 2 = null; w 1
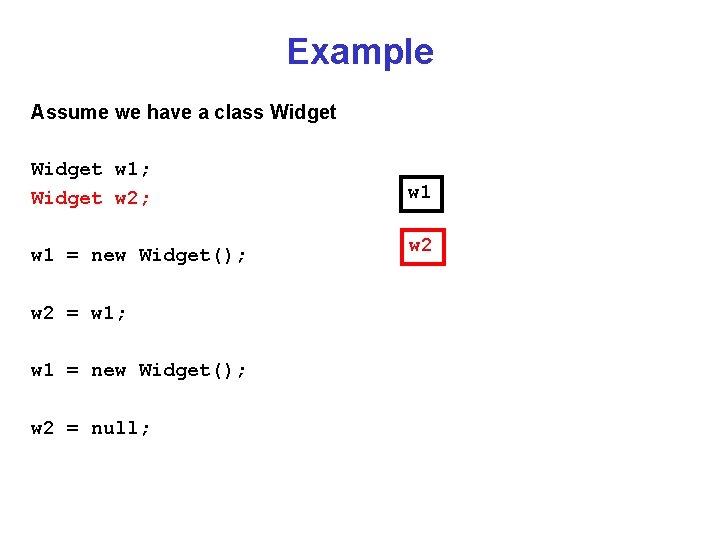
Example Assume we have a class Widget w 1; Widget w 2; w 1 = new Widget(); w 2 = w 1; w 1 = new Widget(); w 2 = null; w 1 w 2
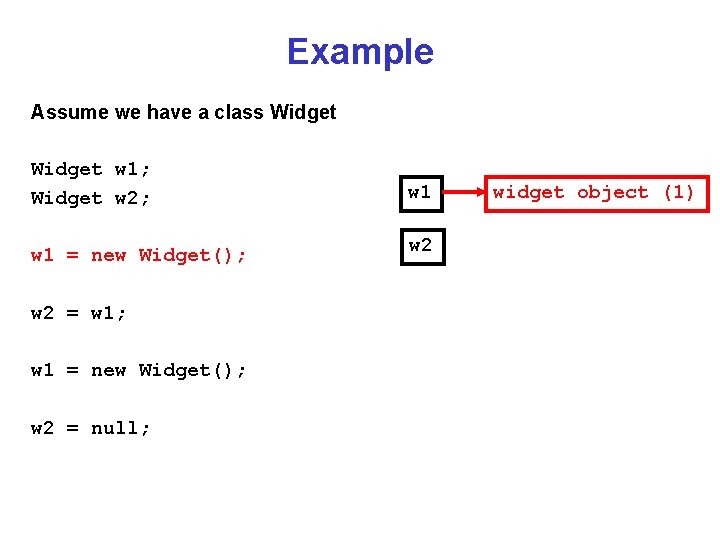
Example Assume we have a class Widget w 1; Widget w 2; w 1 = new Widget(); w 2 = w 1; w 1 = new Widget(); w 2 = null; w 1 w 2 widget object (1)
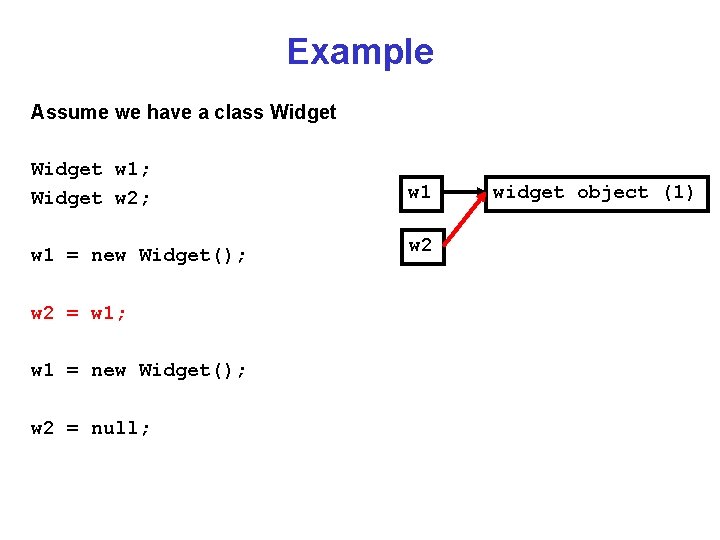
Example Assume we have a class Widget w 1; Widget w 2; w 1 = new Widget(); w 2 = w 1; w 1 = new Widget(); w 2 = null; w 1 w 2 widget object (1)
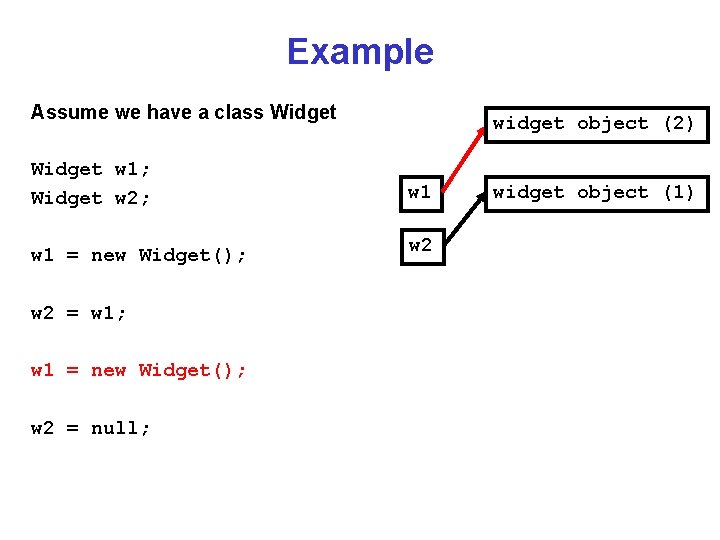
Example Assume we have a class Widget w 1; Widget w 2; w 1 = new Widget(); w 2 = w 1; w 1 = new Widget(); w 2 = null; widget object (2) w 1 w 2 widget object (1)
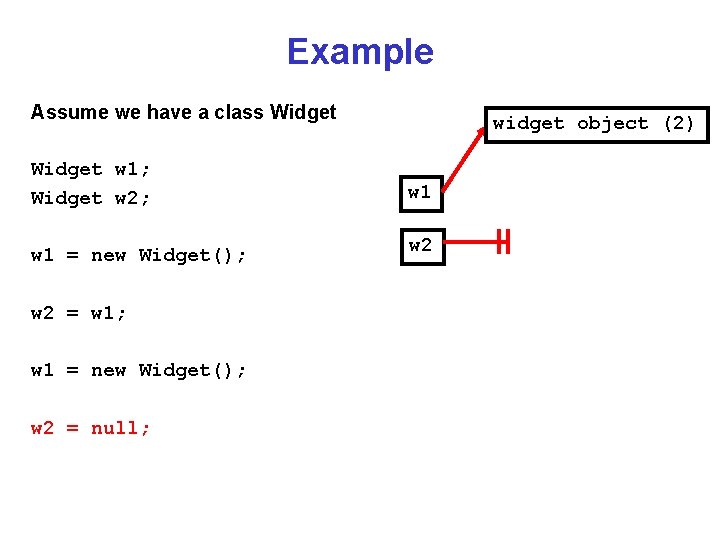
Example Assume we have a class Widget w 1; Widget w 2; w 1 = new Widget(); w 2 = w 1; w 1 = new Widget(); w 2 = null; widget object (2) w 1 w 2
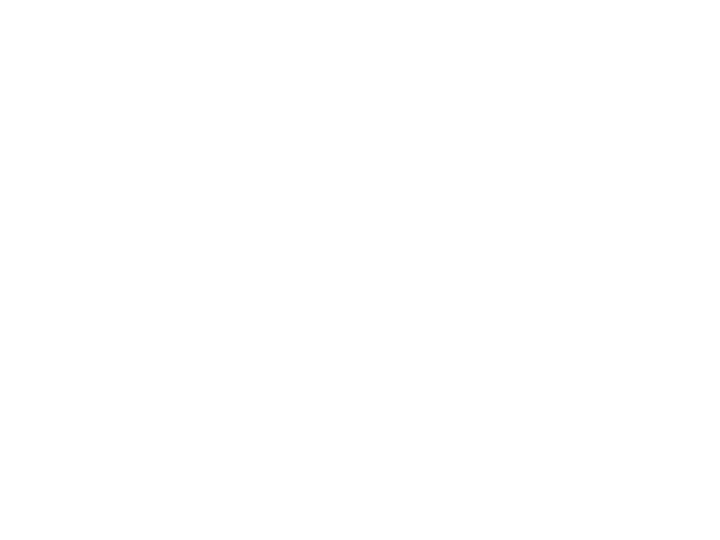
Int sum(int a int n) int sum=0 i
Interface calculator public int add class test
Int max(int x int y)
Public void drawsquare(int x, int y, int len)
Public int divide(int a int b)
Apdex
Static and dynamic class loading in java
Public class welcome public static int test
Class math static int x
Interface myinterface int foo(int x)
Int main(int argc, char** argv)
#include stdio.h int main() int i = 5
7팩토리얼
Const int arduino adalah
Int main int num 4
Void swap
Int f(int n)
Int.max
Int argc char argv
Vtkcontourwidget
Google desktop widget
A widget manufacturer currently produces
Jlv new user training quizlet
Widget toolkits
Shinywidgets
Gadget
Android widget toolbox
Uml static
Sadlier vocabulary workshop level d unit 1
Public static int
Static int
Public static void main int args
Transferered
Class bucket int capacity bucket()
Class person private int age
Inheritance types in java
Object diagram captures the behavior of a single use case
Transformer is static device
East egg west egg
Static antagonist
Dynamic vs static character