class Static Demo static int x int y
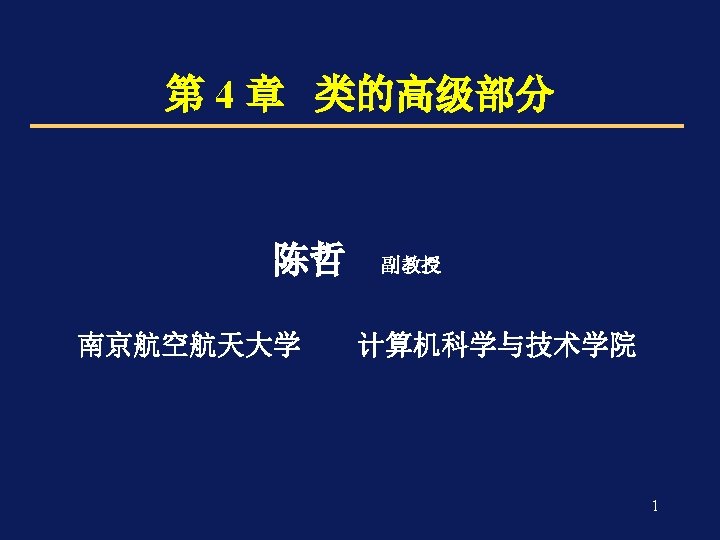
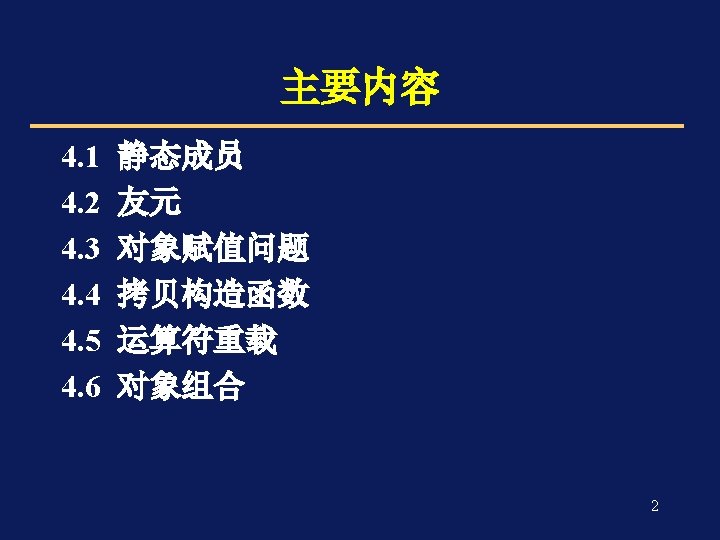
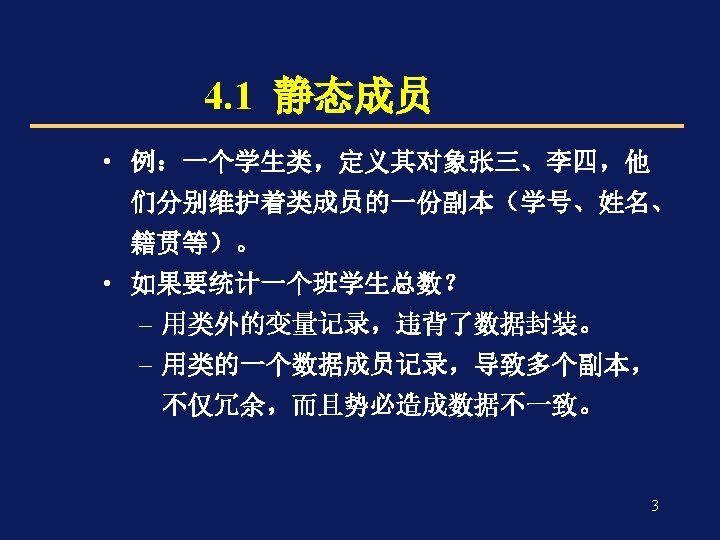
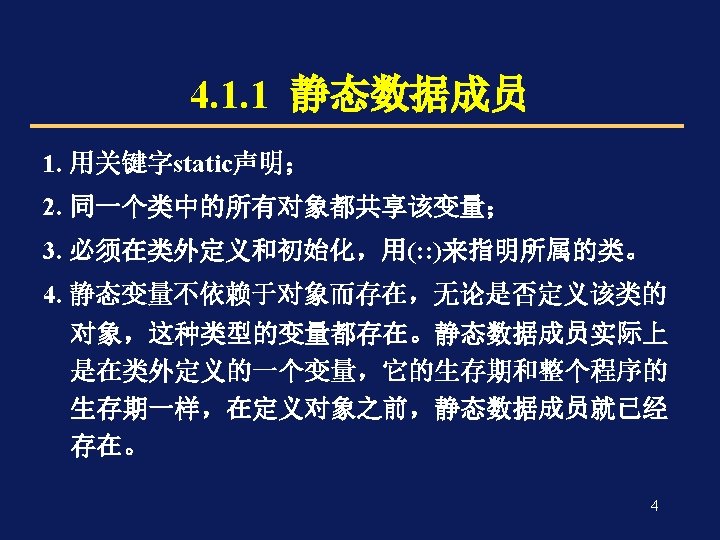
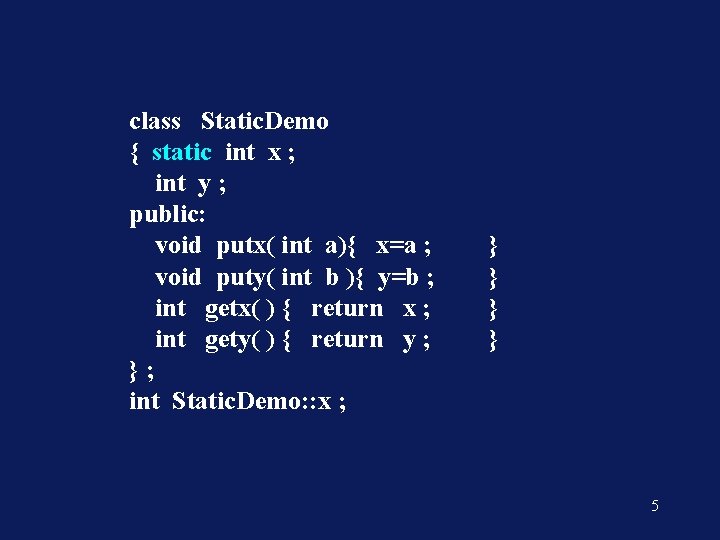
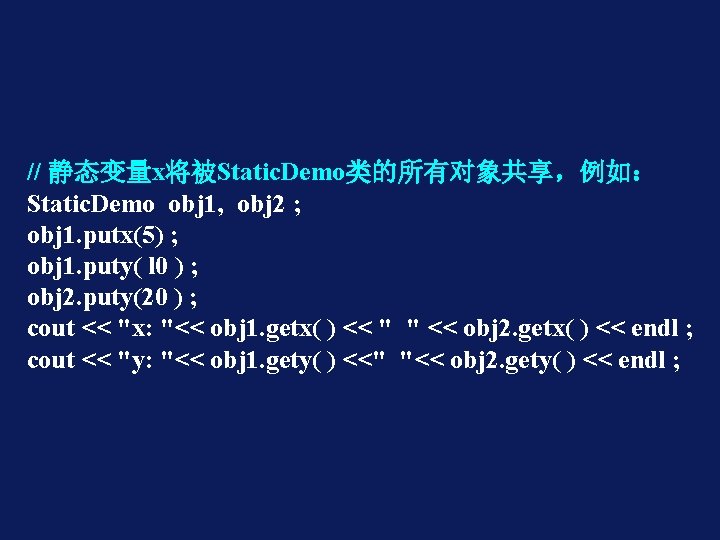
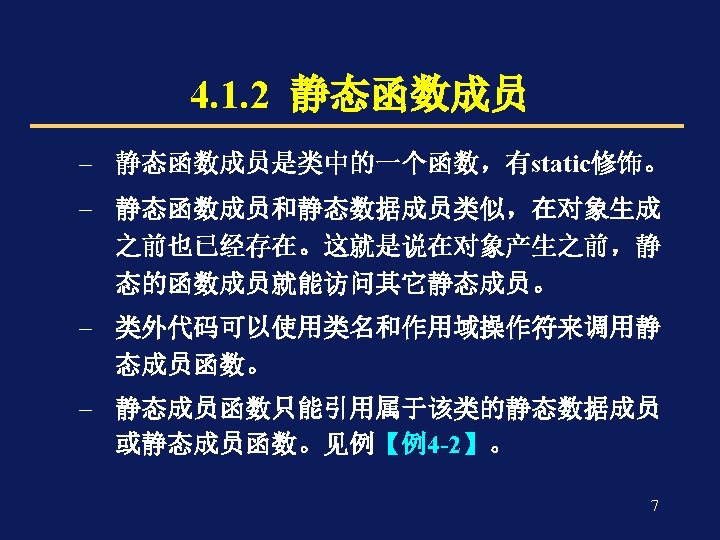
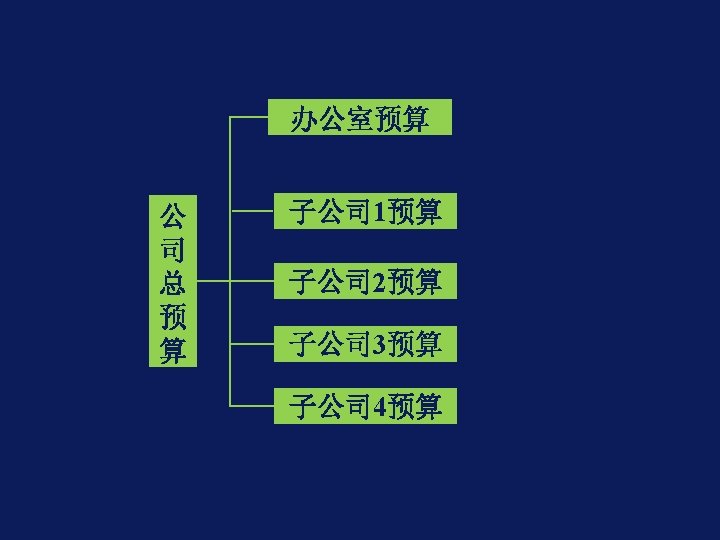
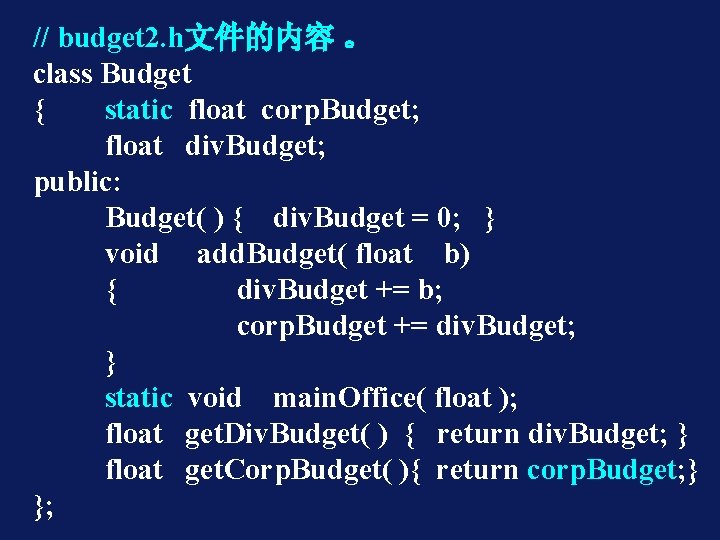
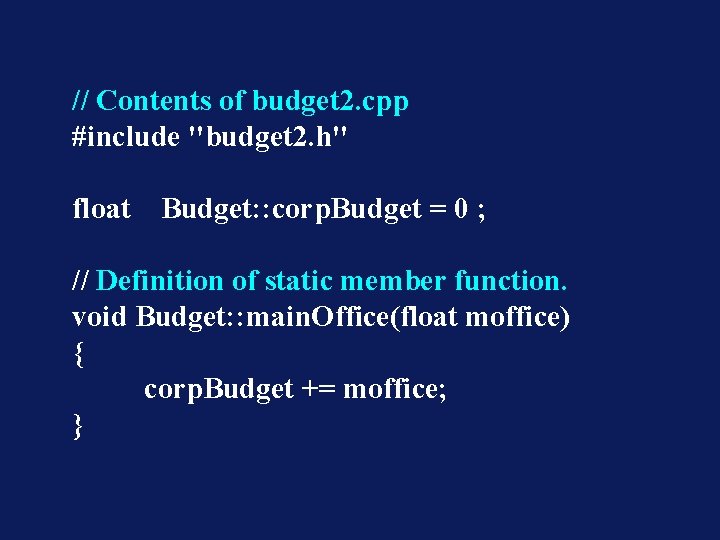
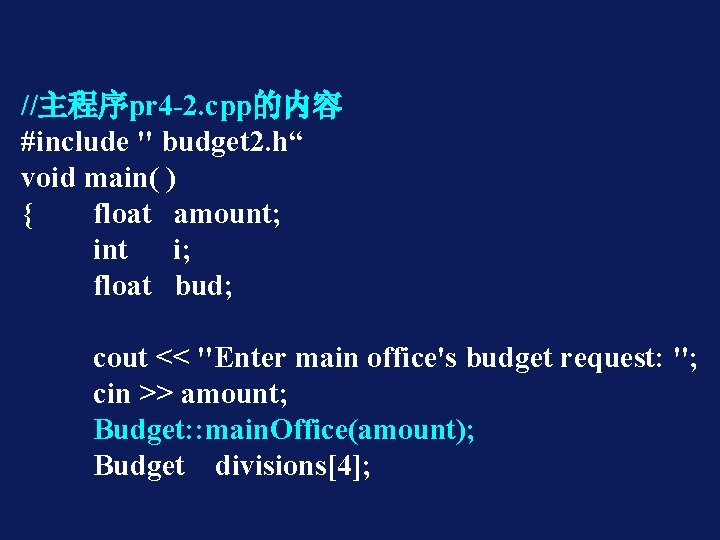
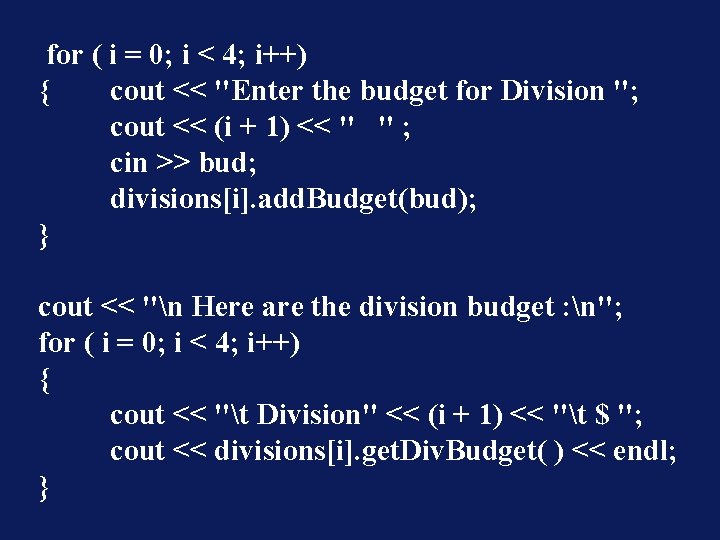
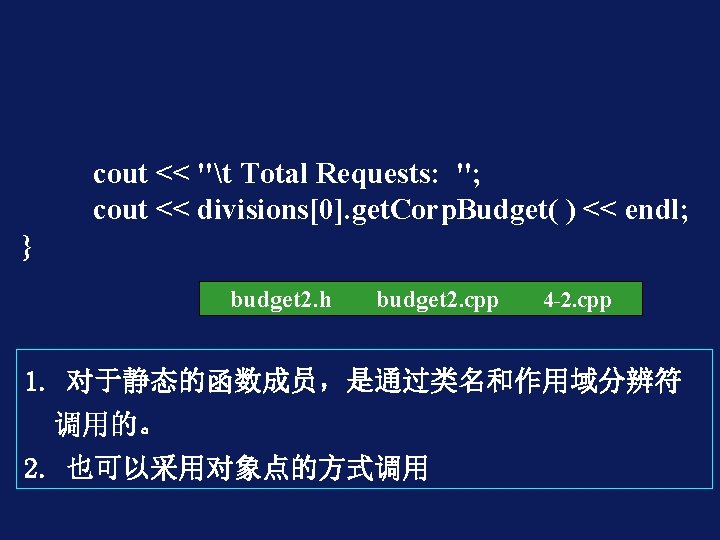
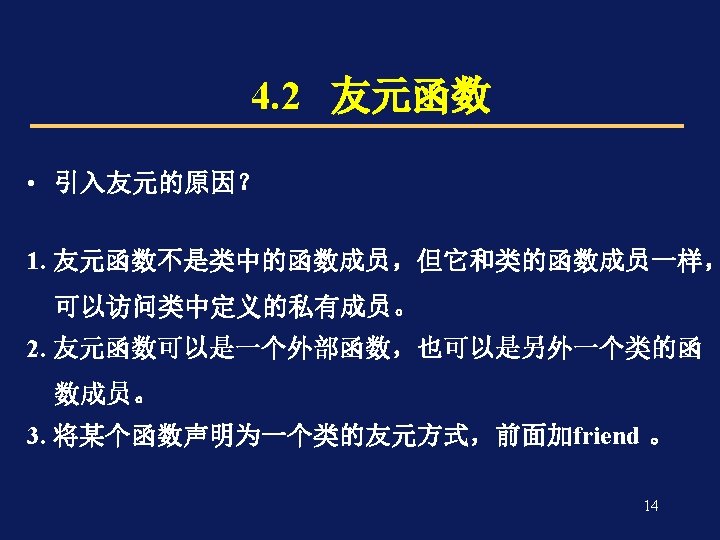
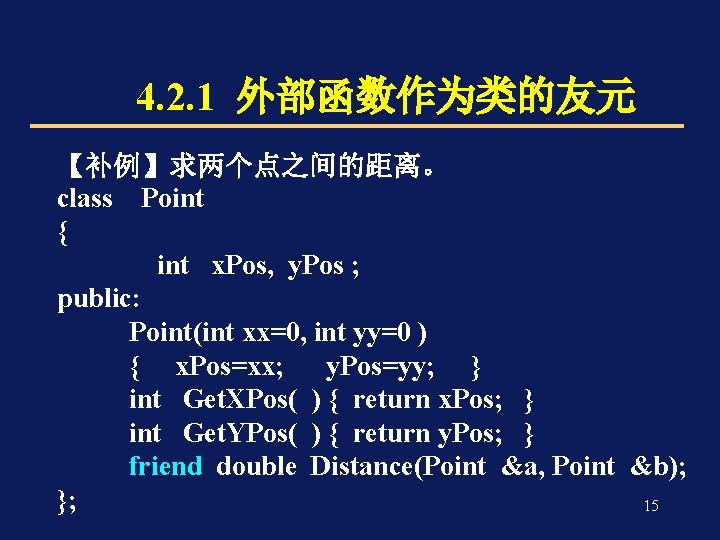
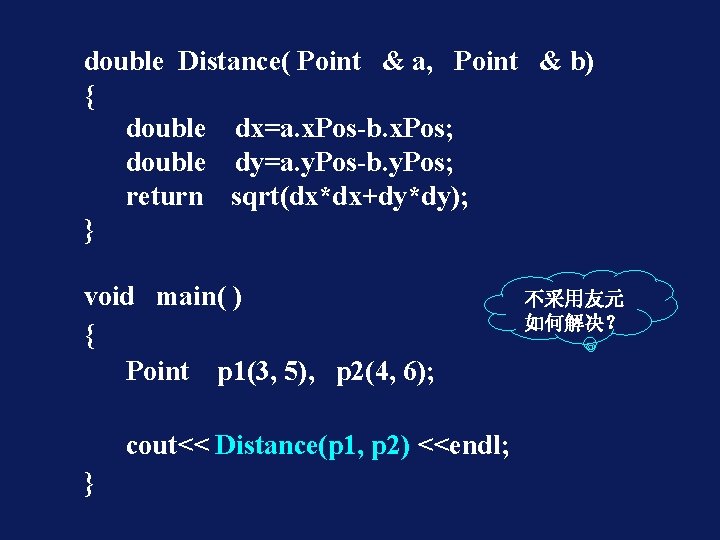
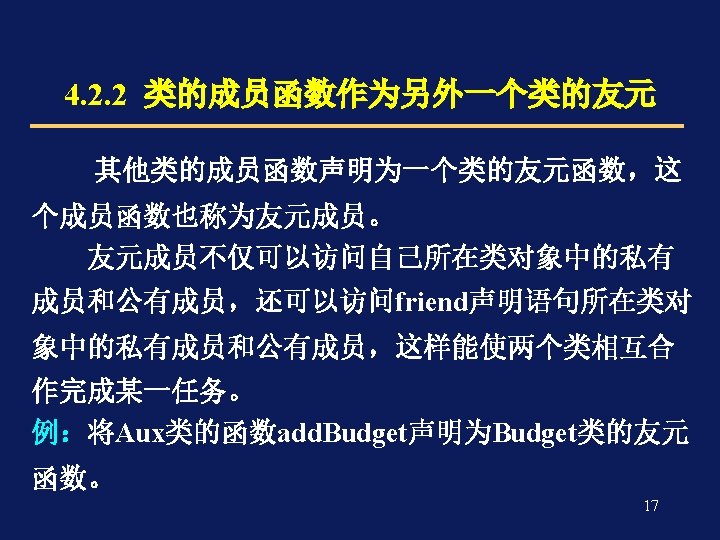
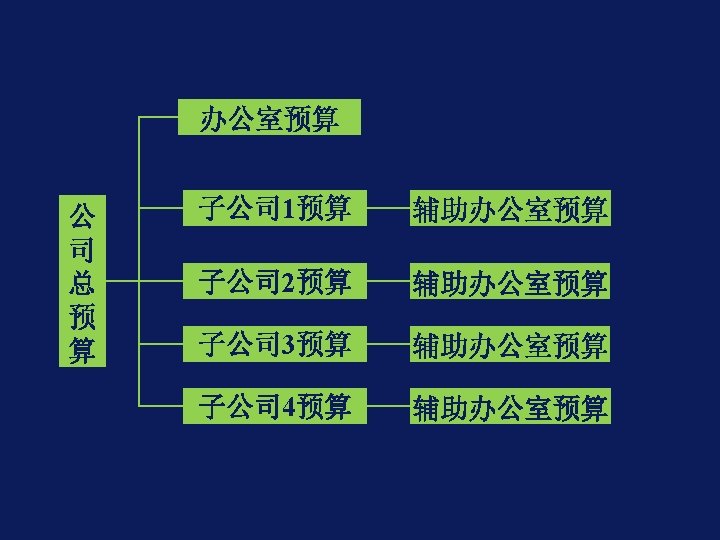
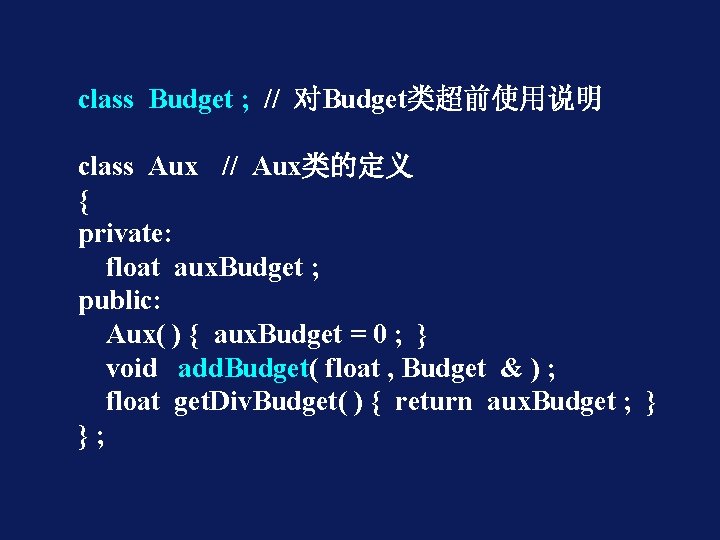
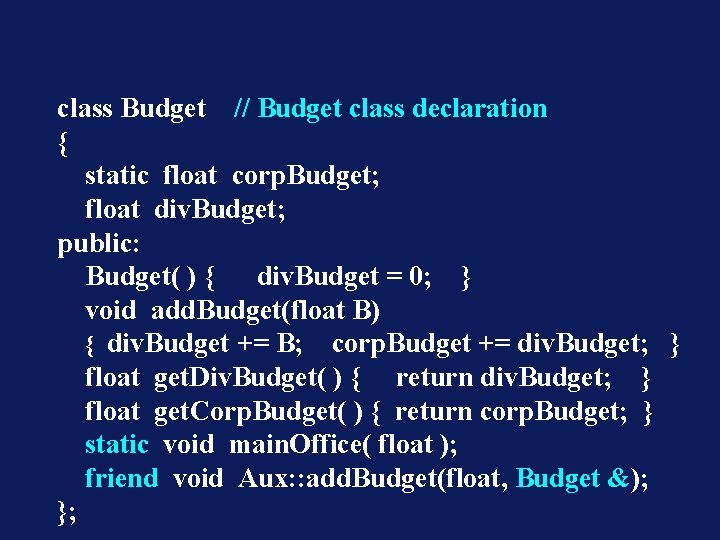
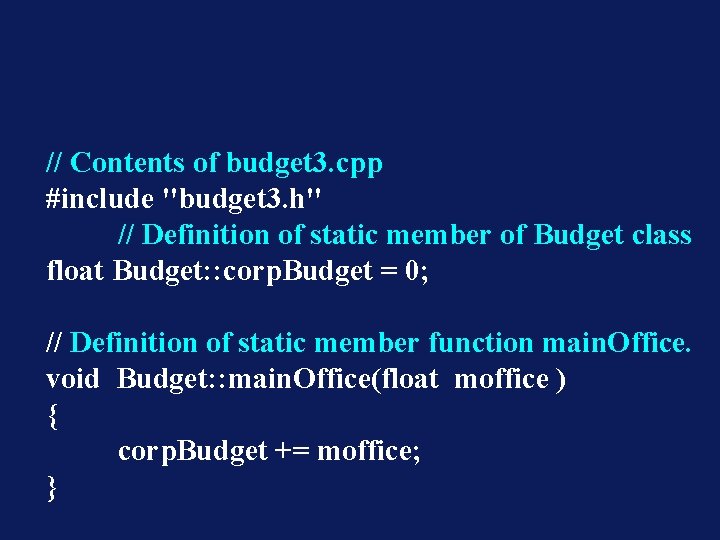
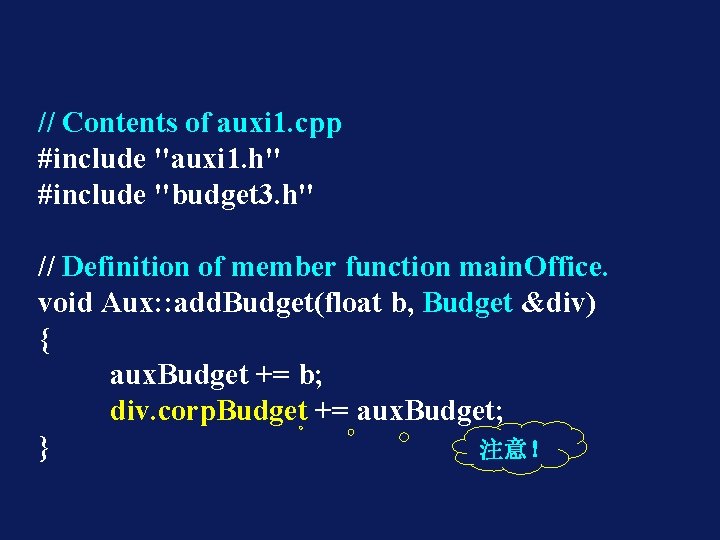
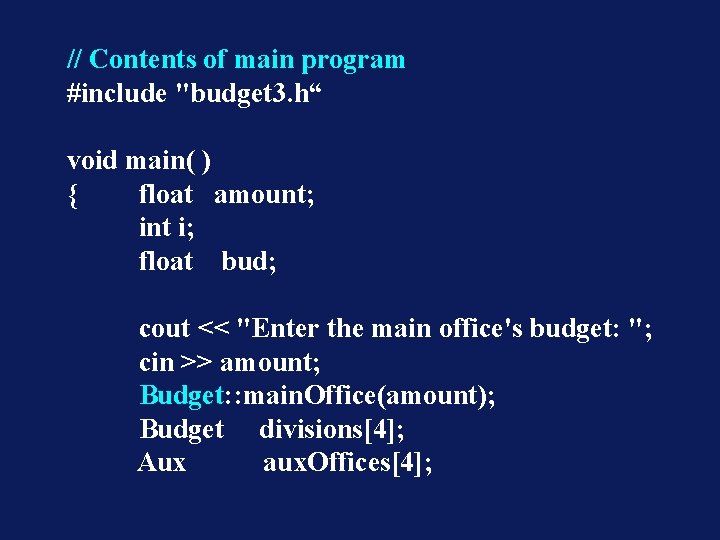
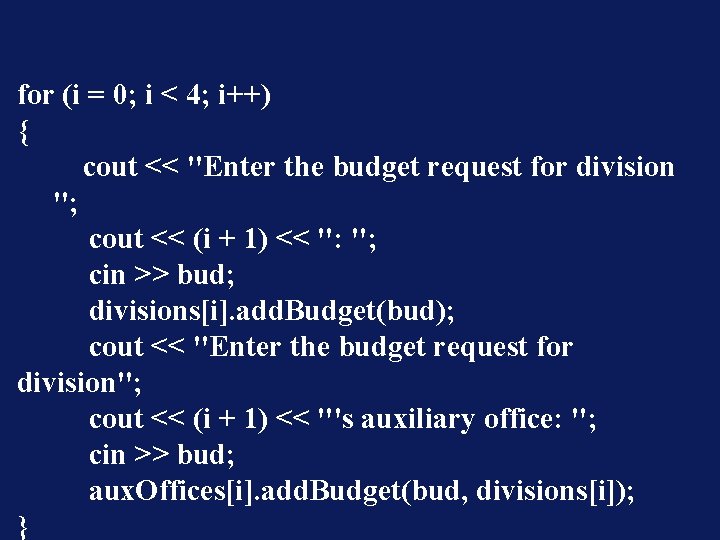
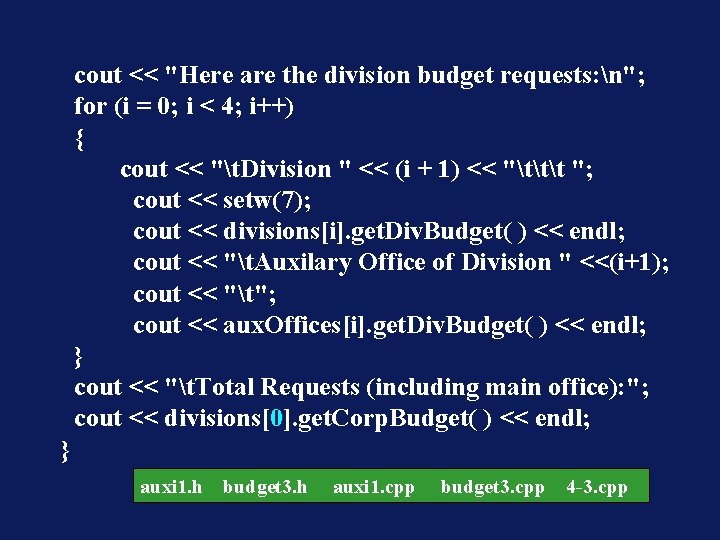
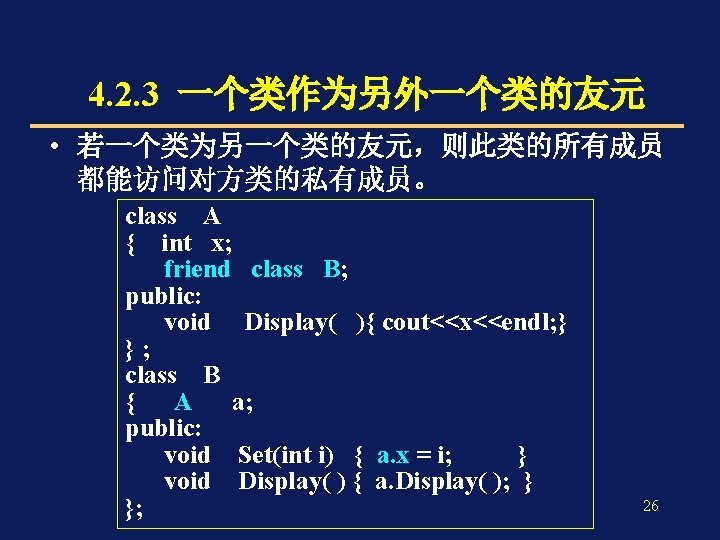
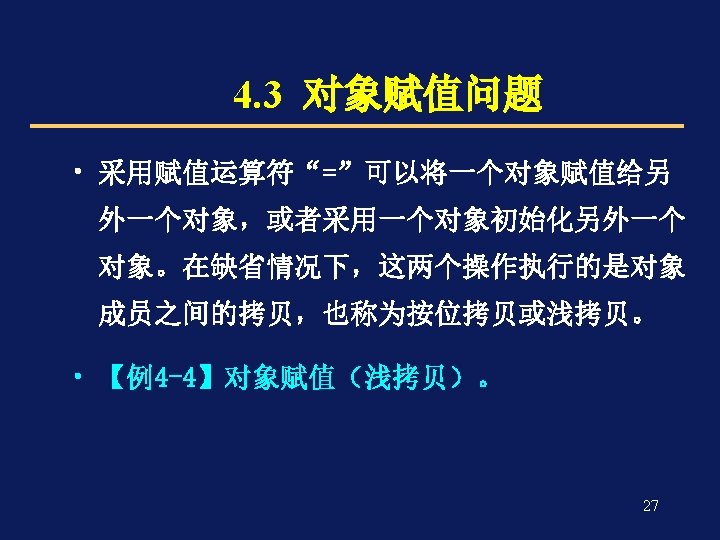
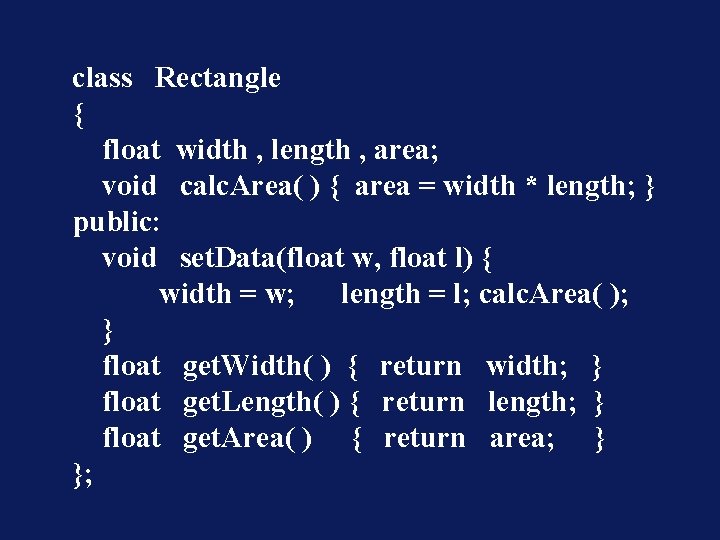
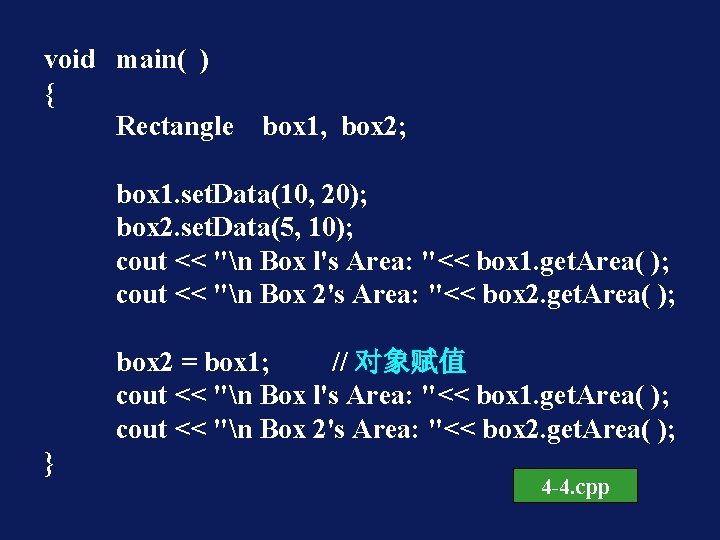
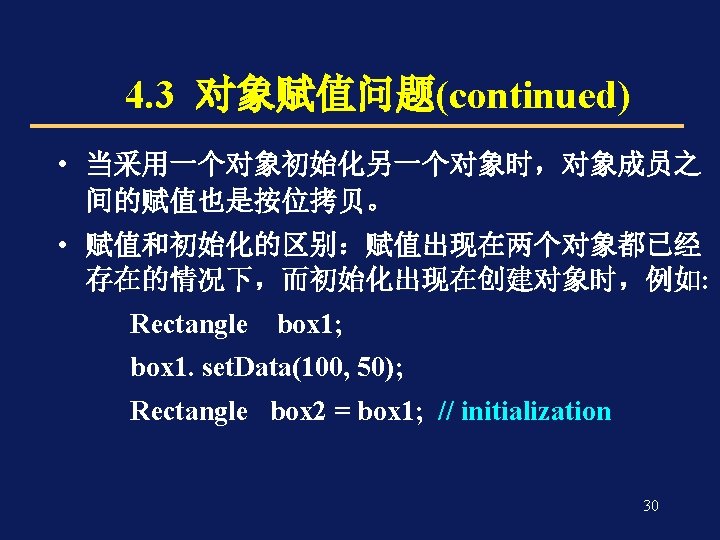
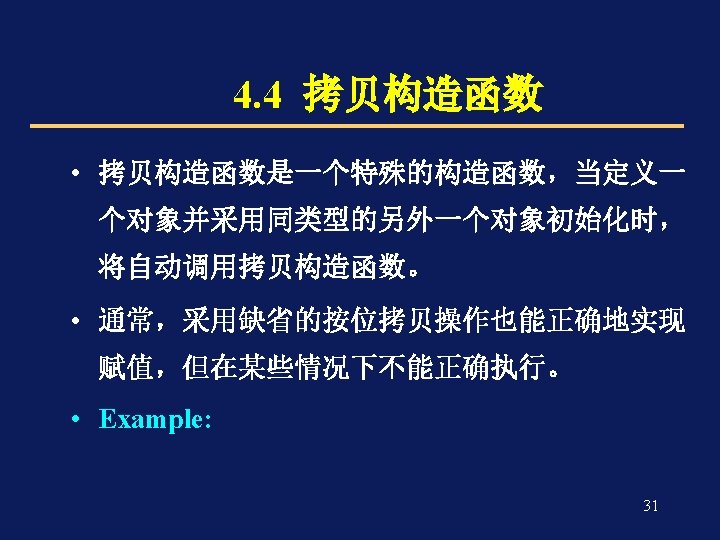
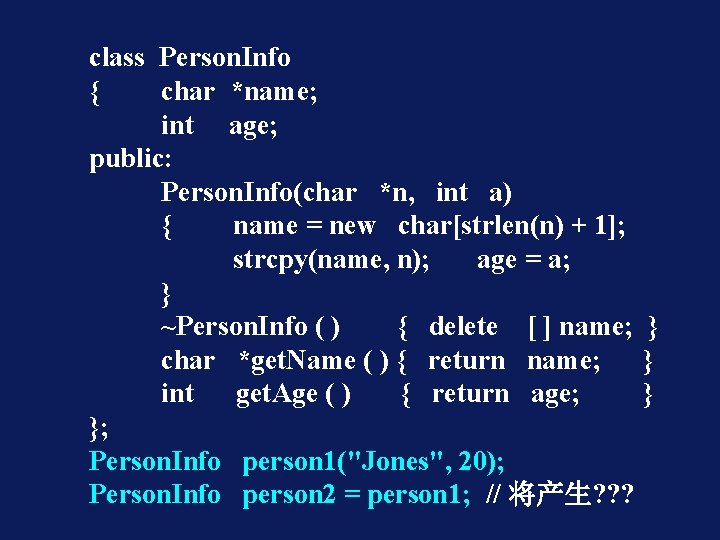
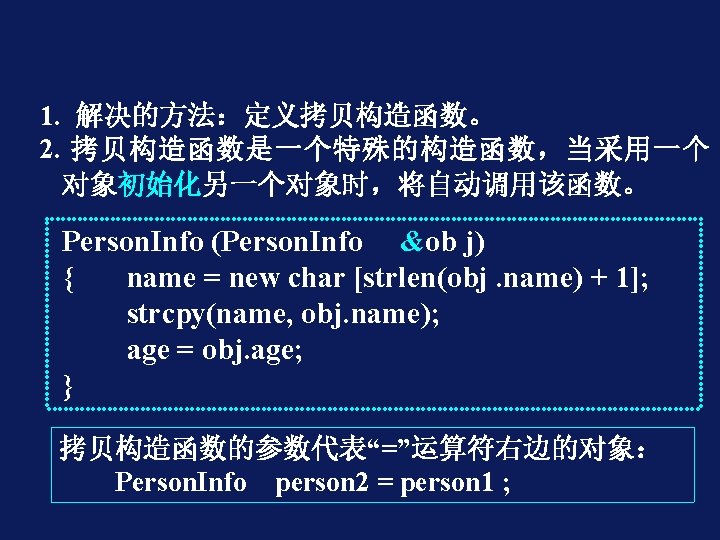
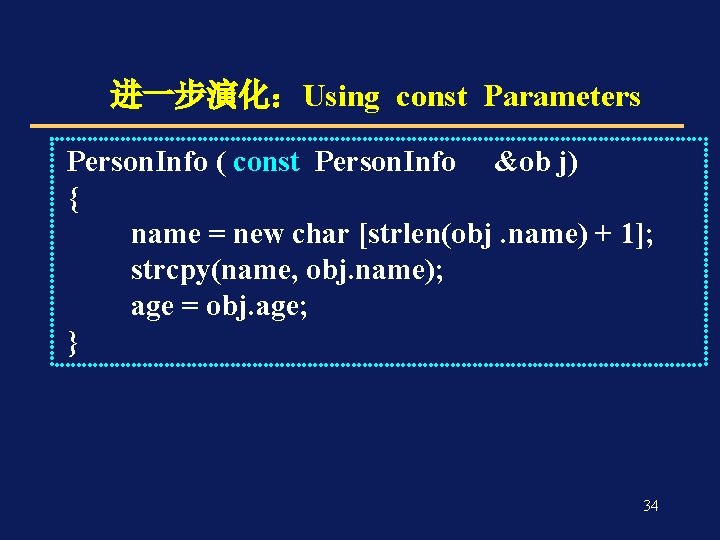
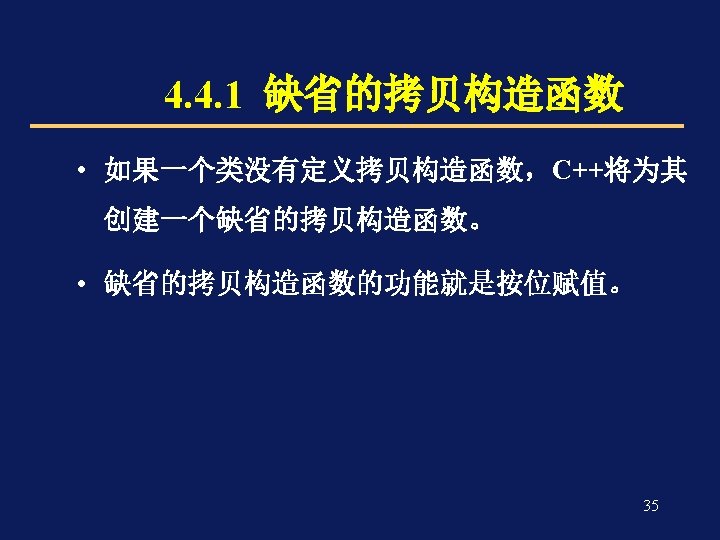
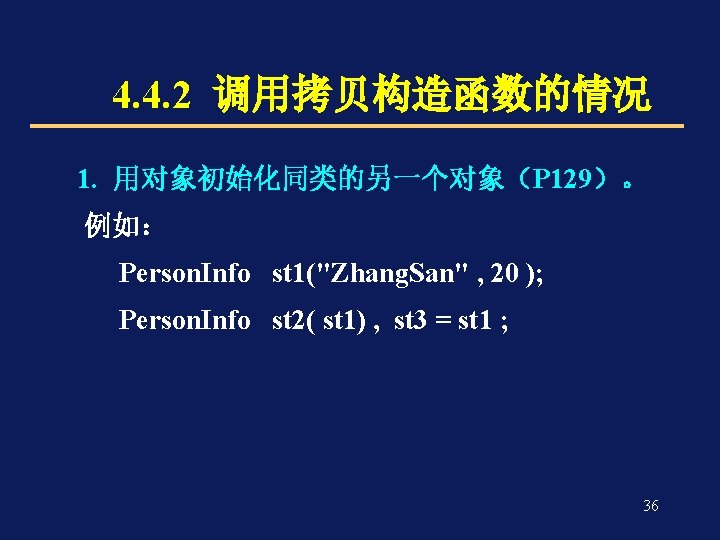
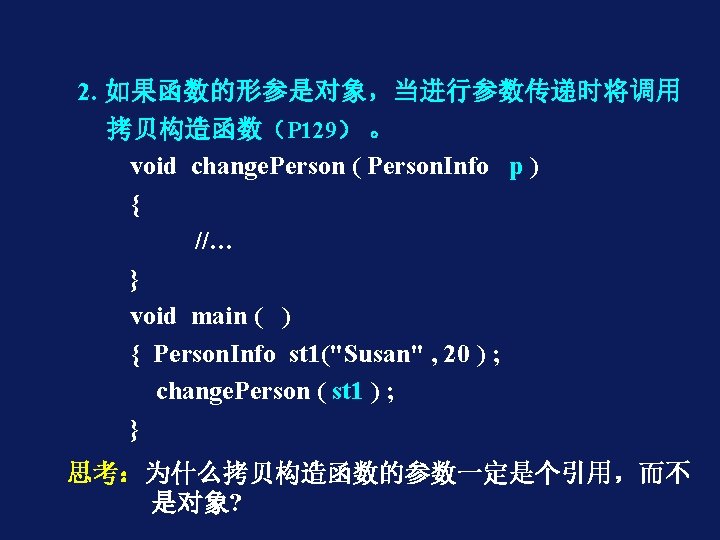
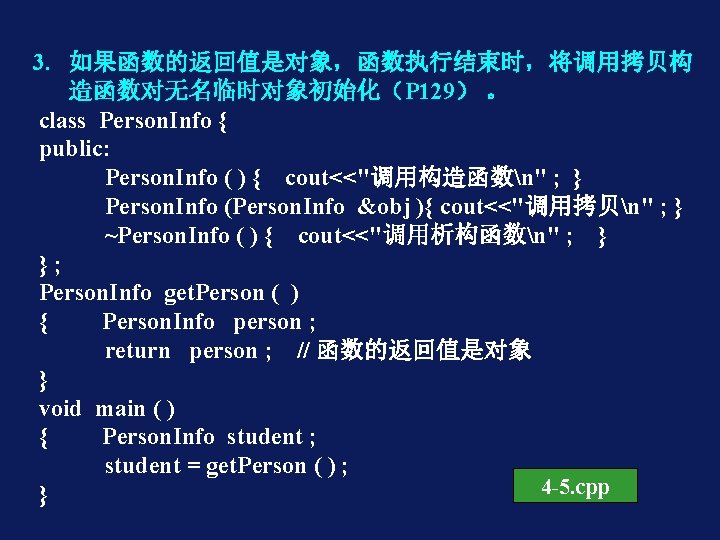
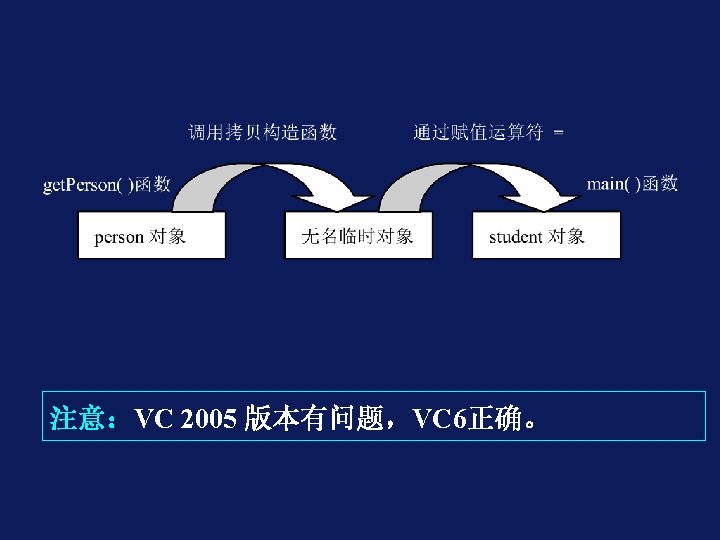
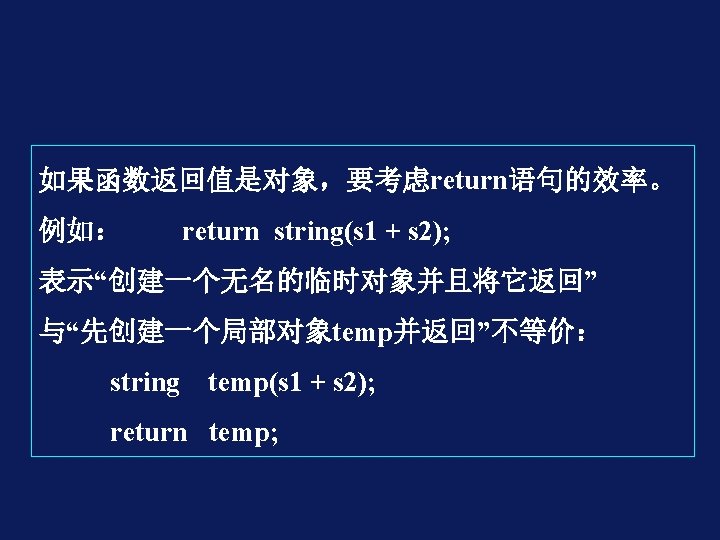
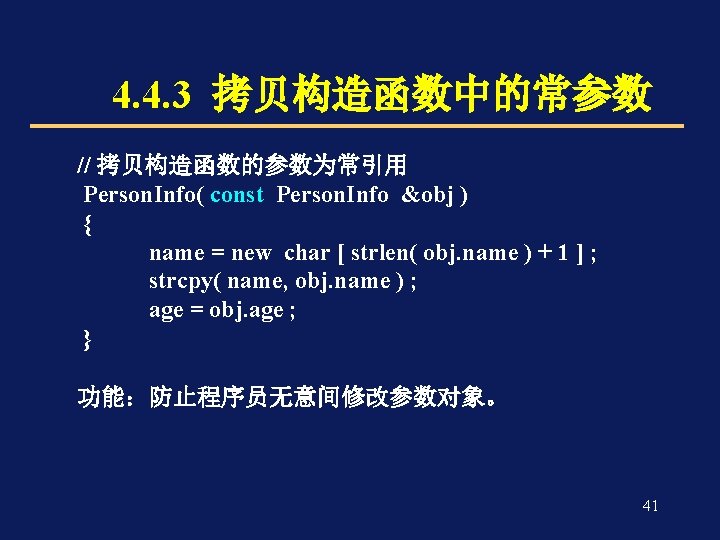
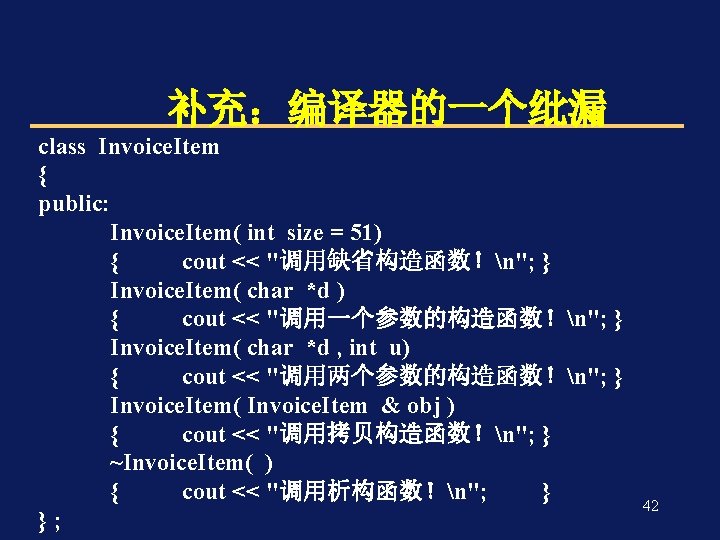
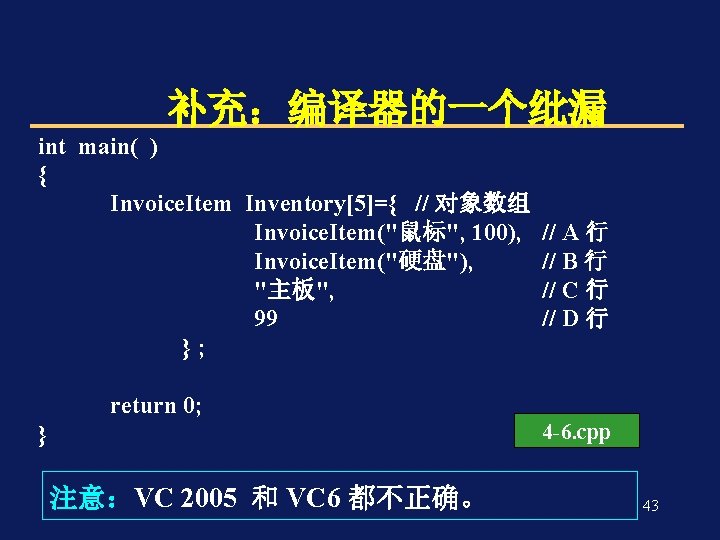
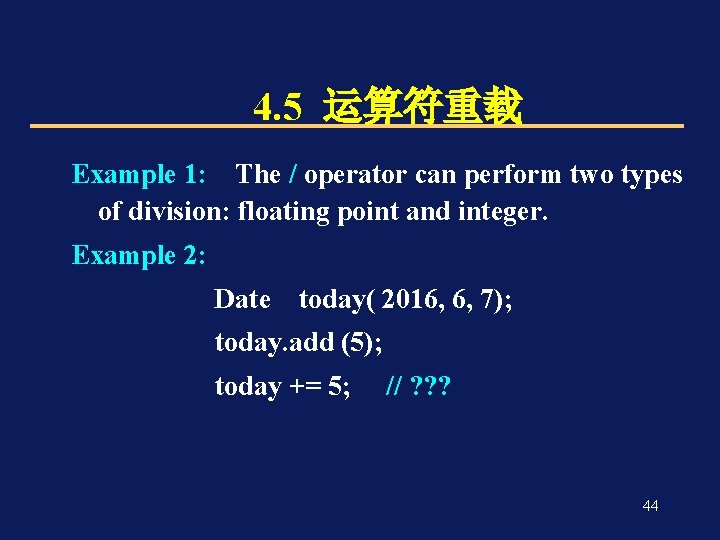
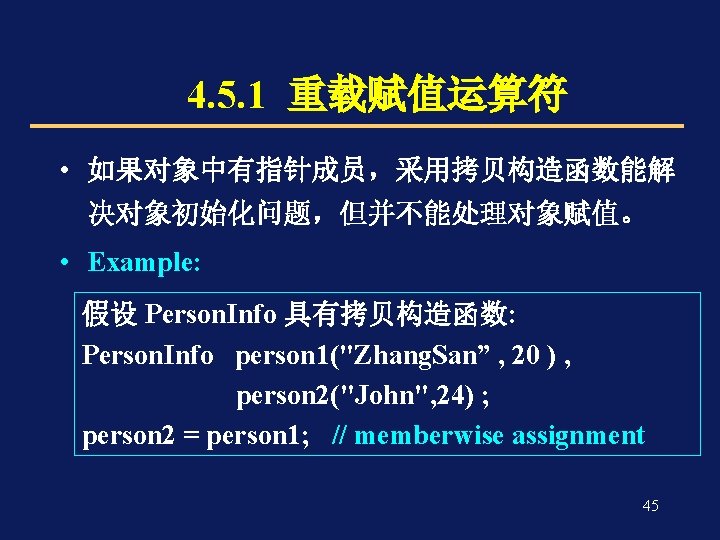
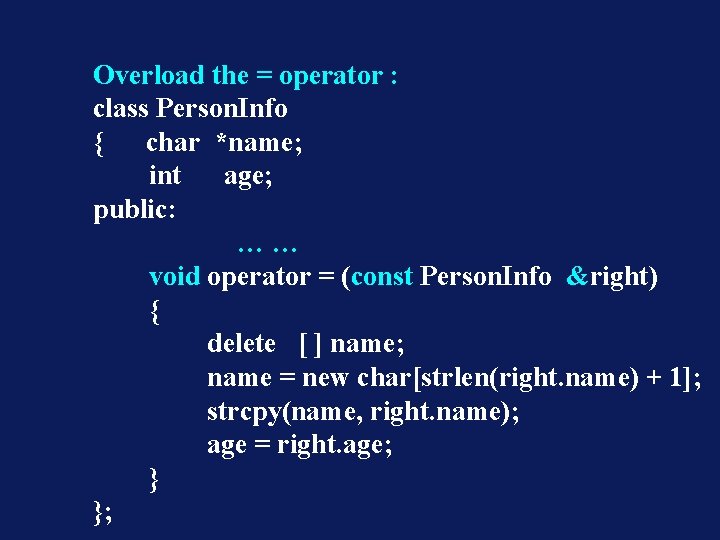
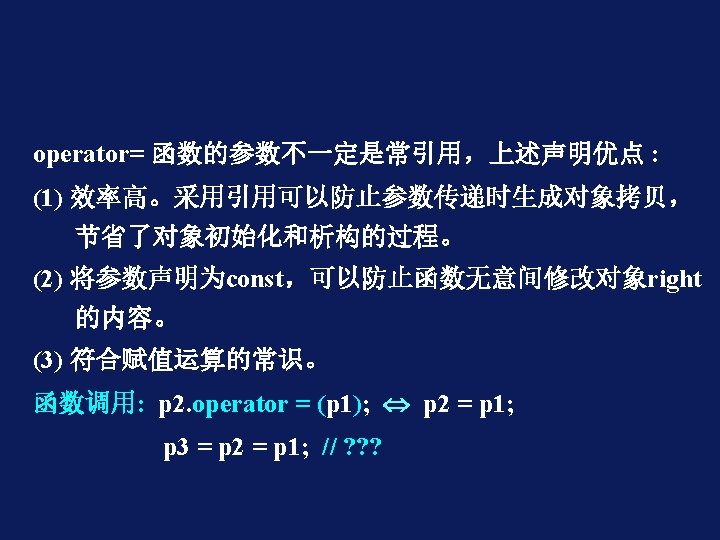
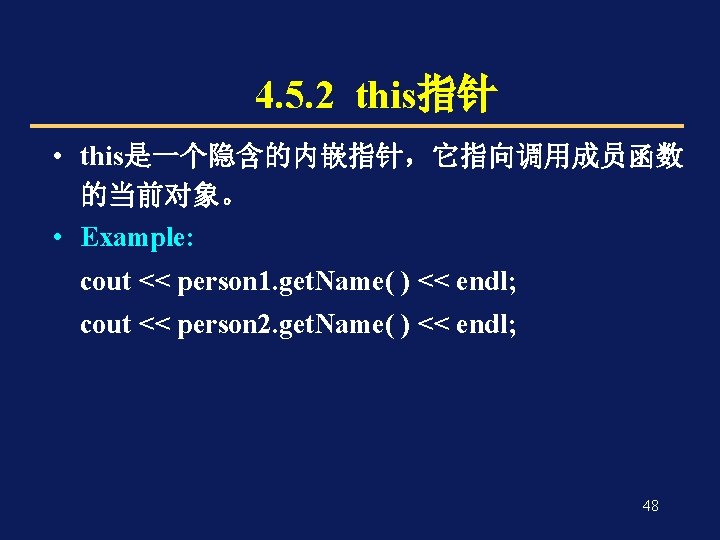
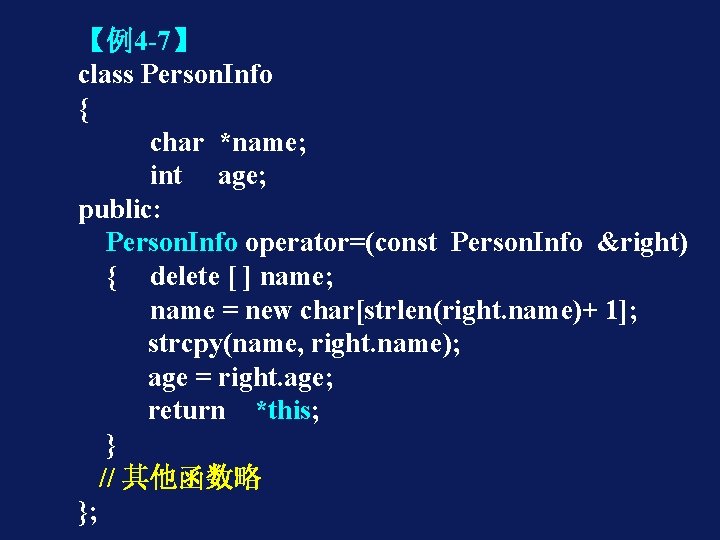
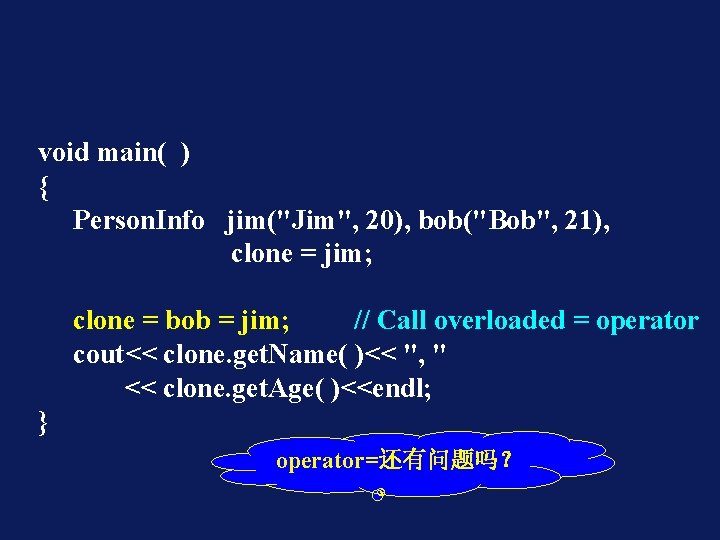
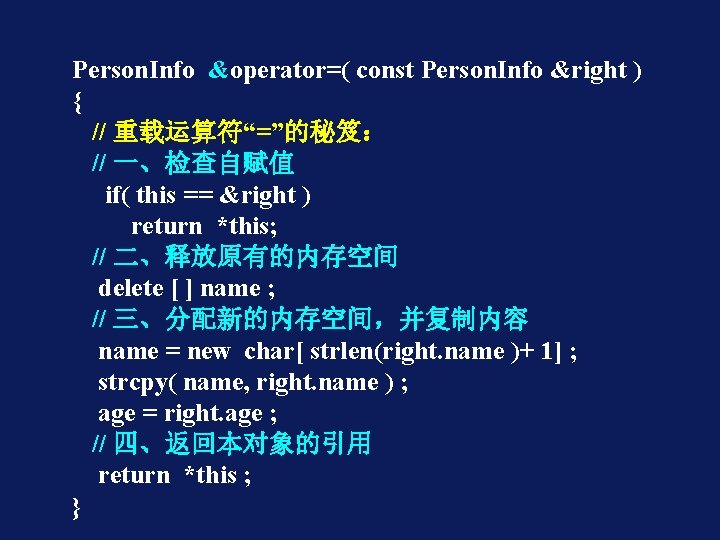
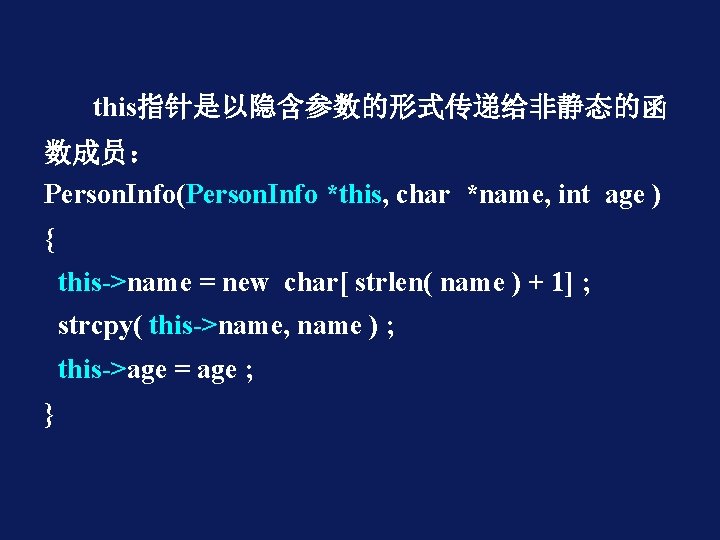
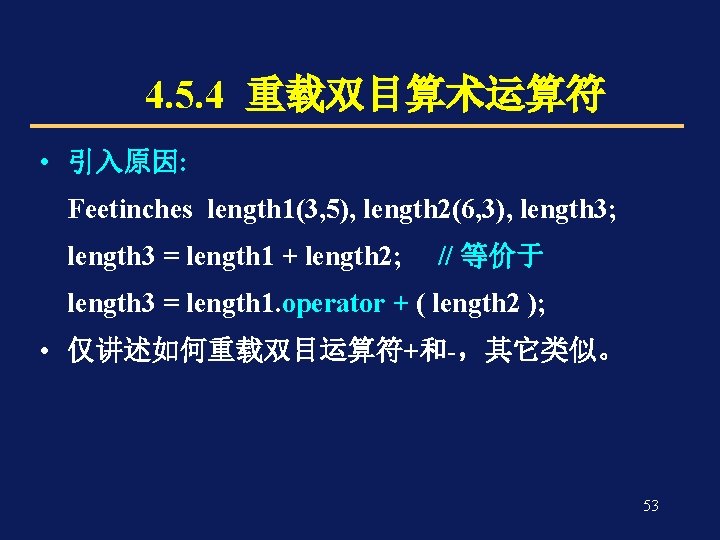
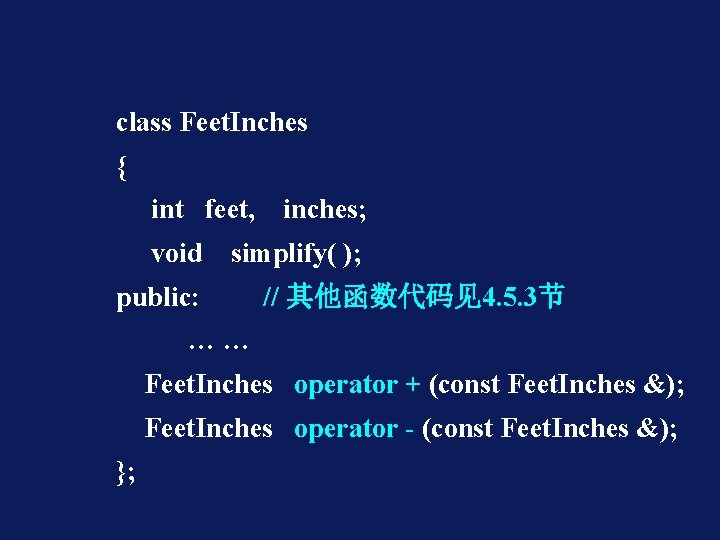
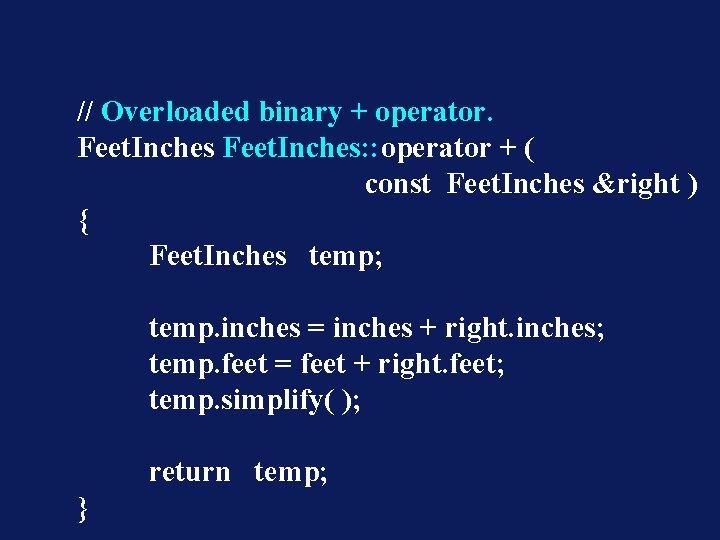
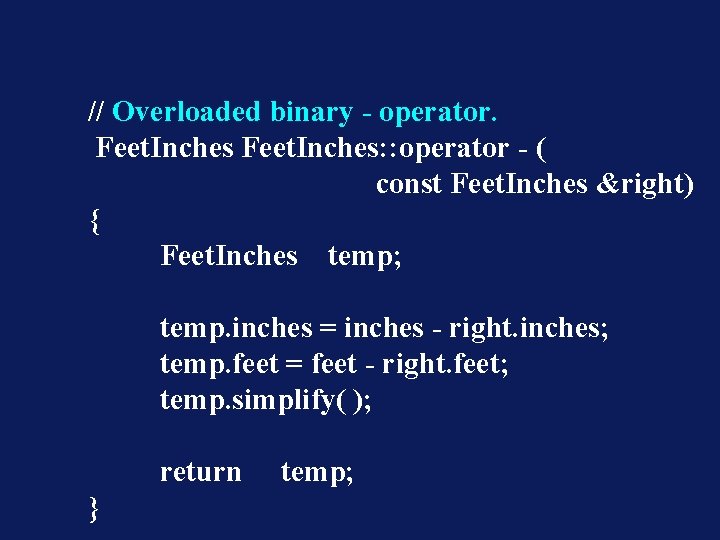
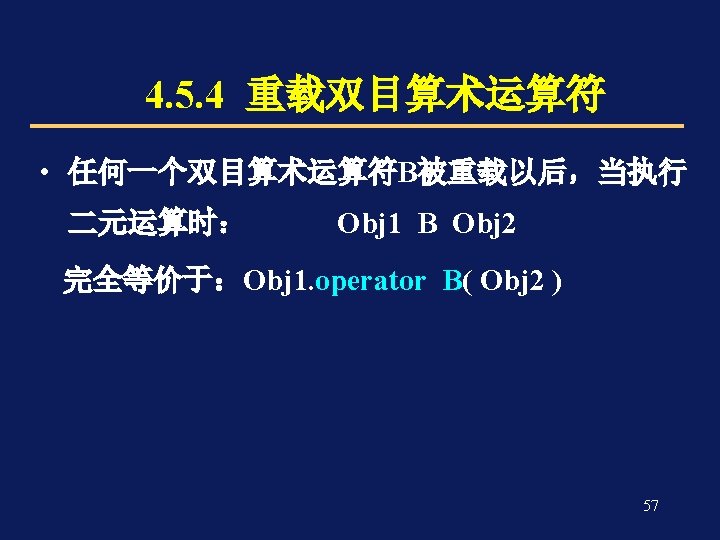
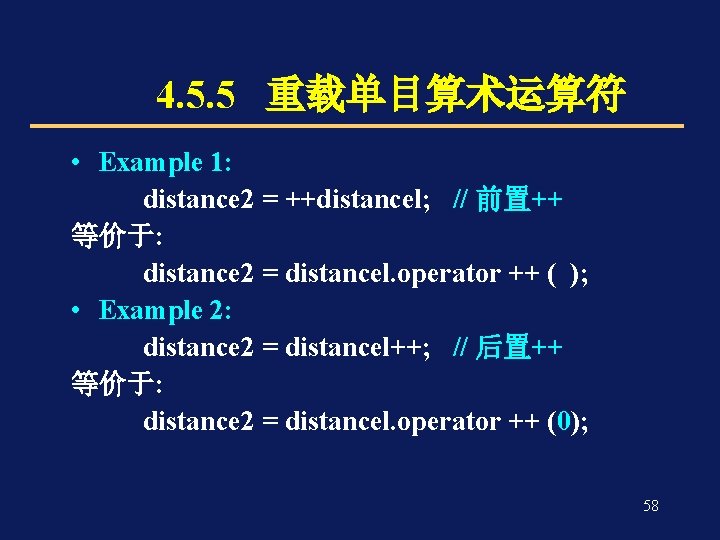
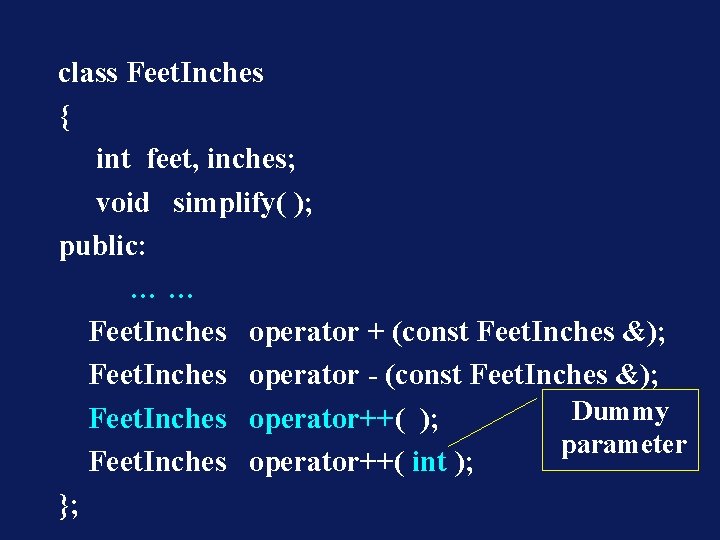
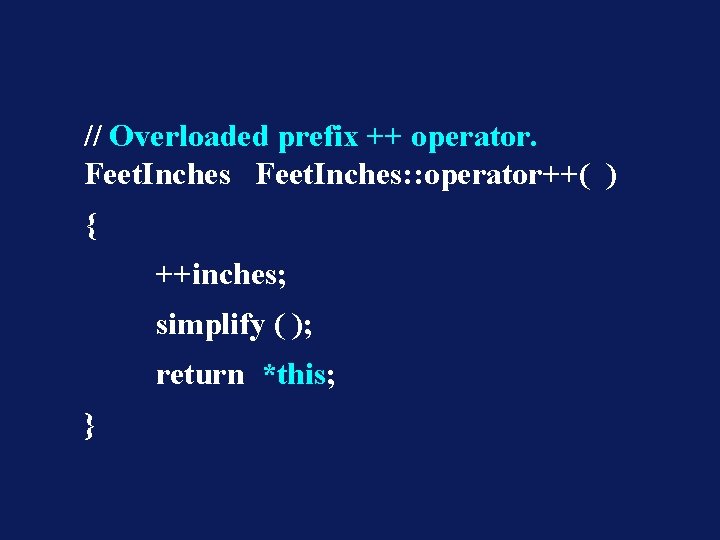
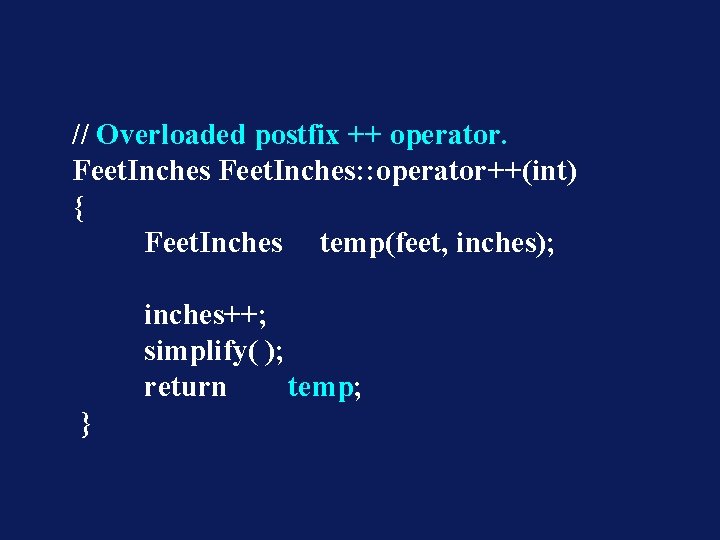
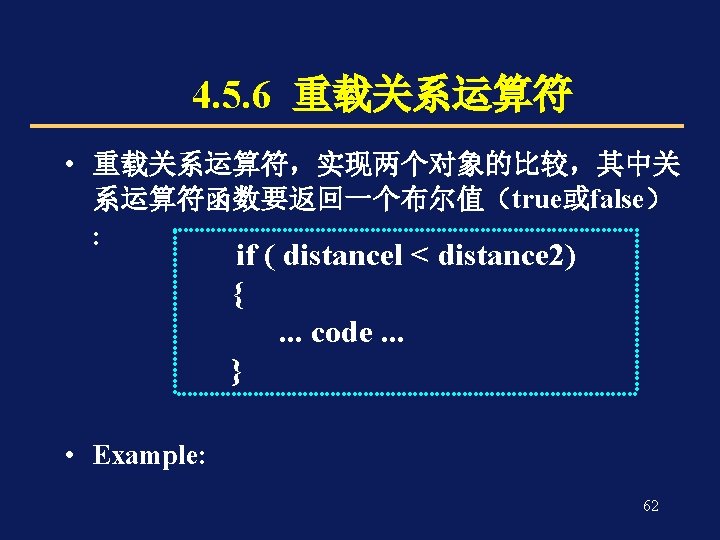
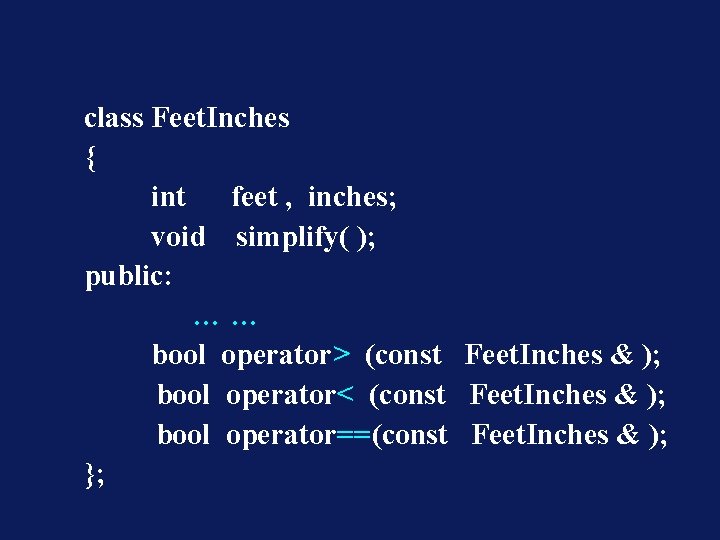
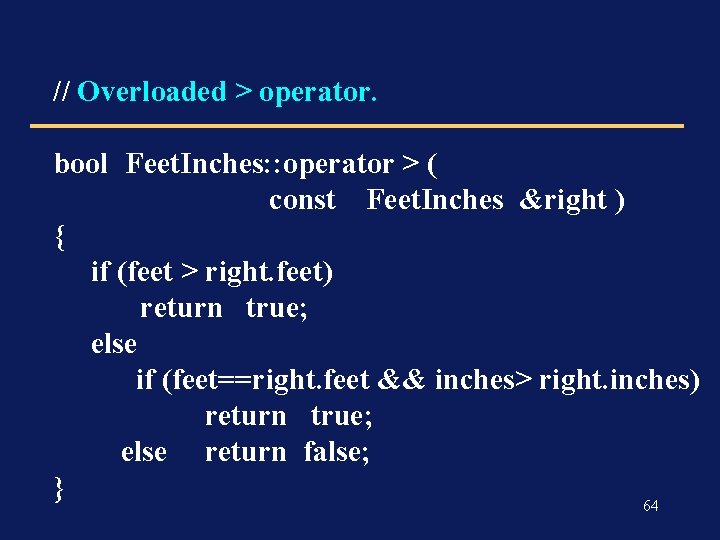
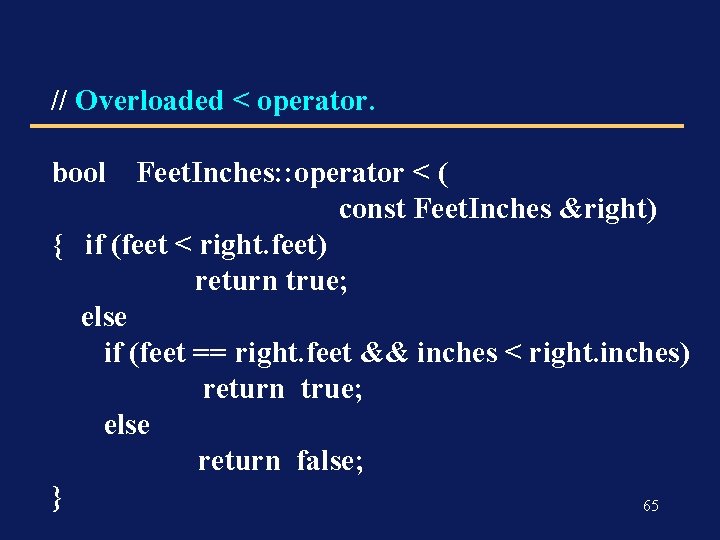
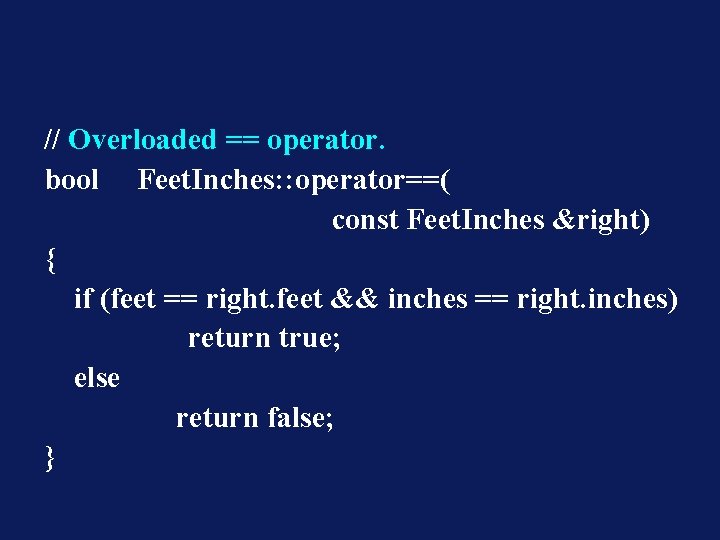
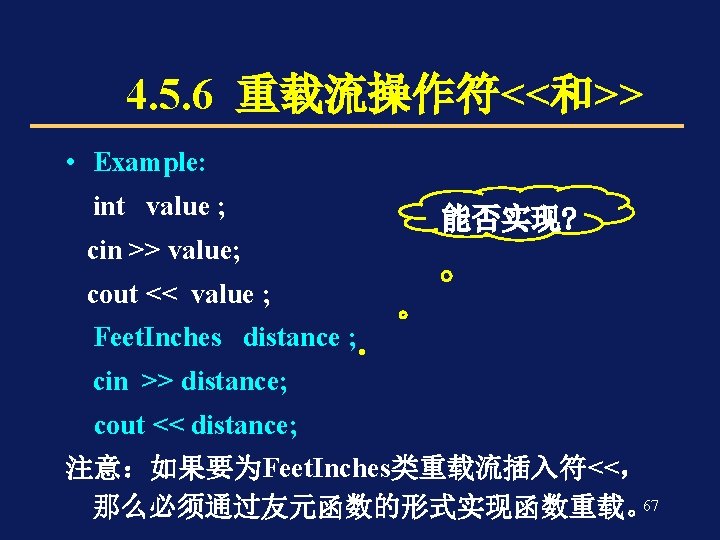
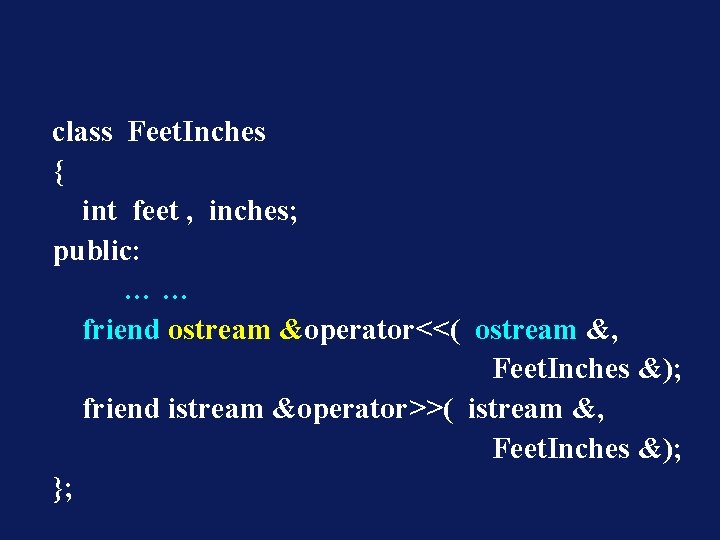
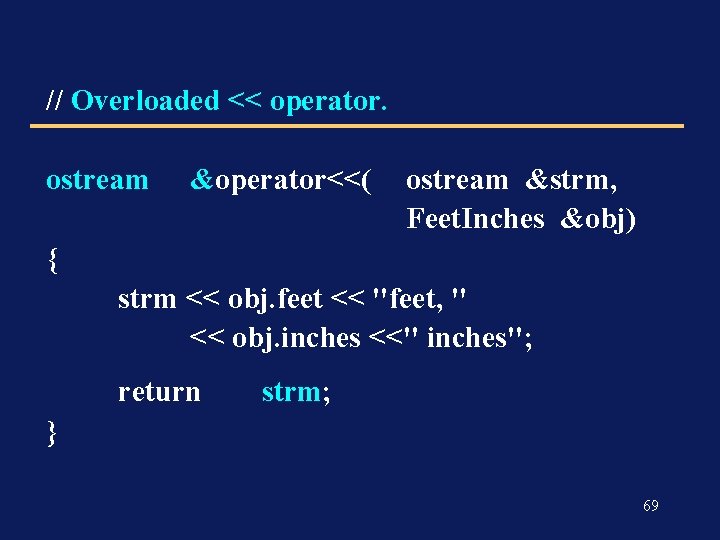
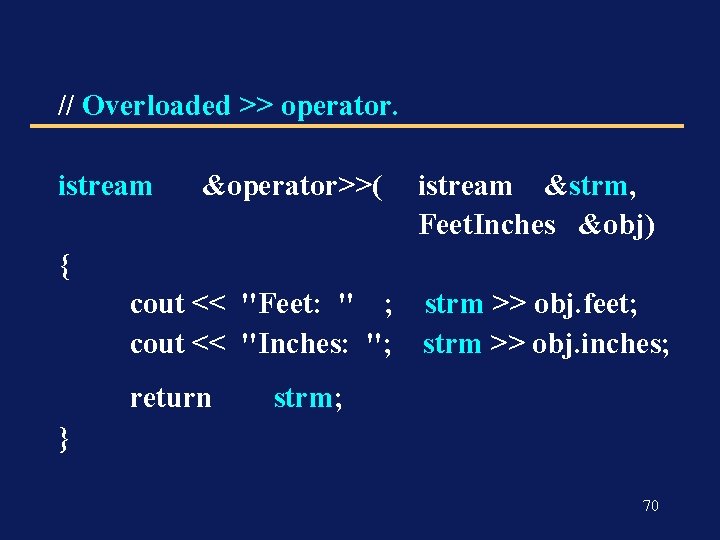
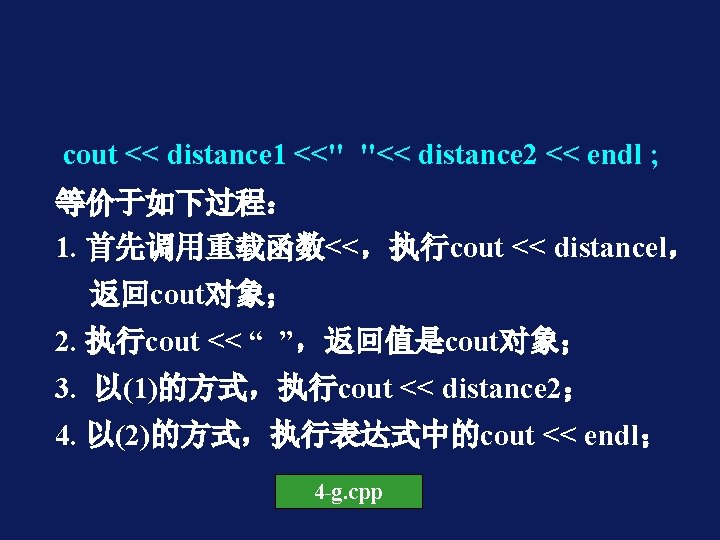
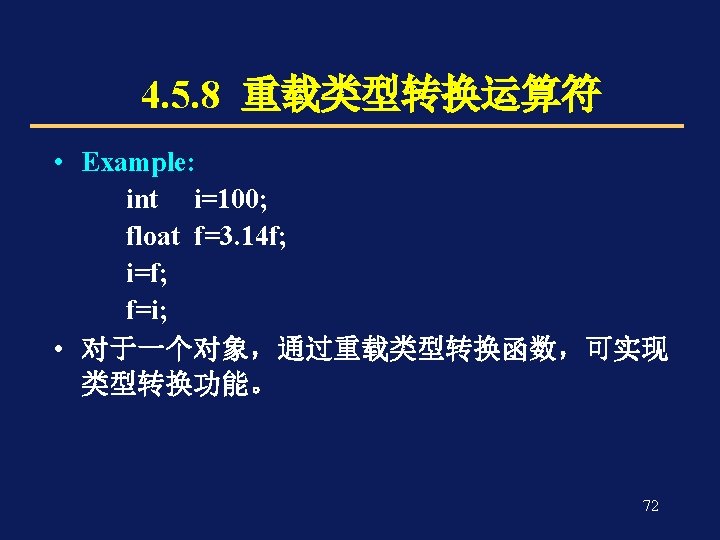
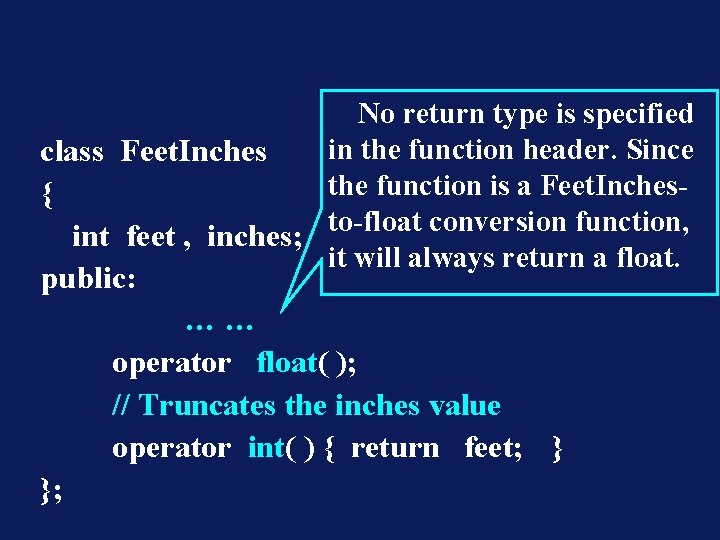
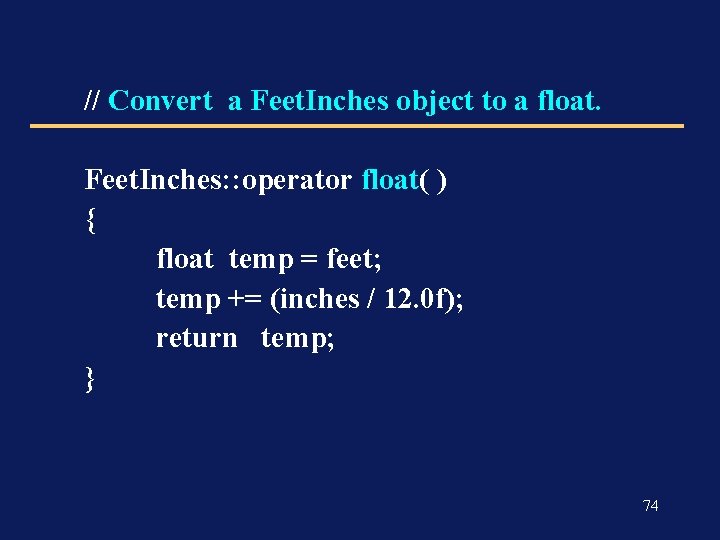
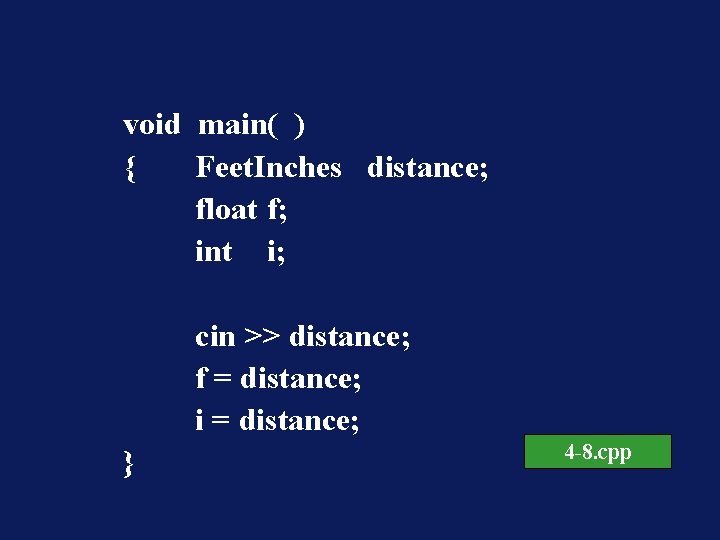
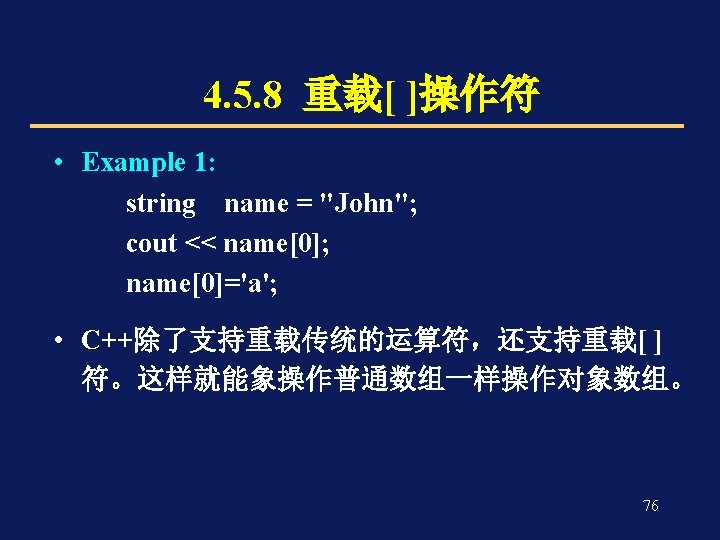
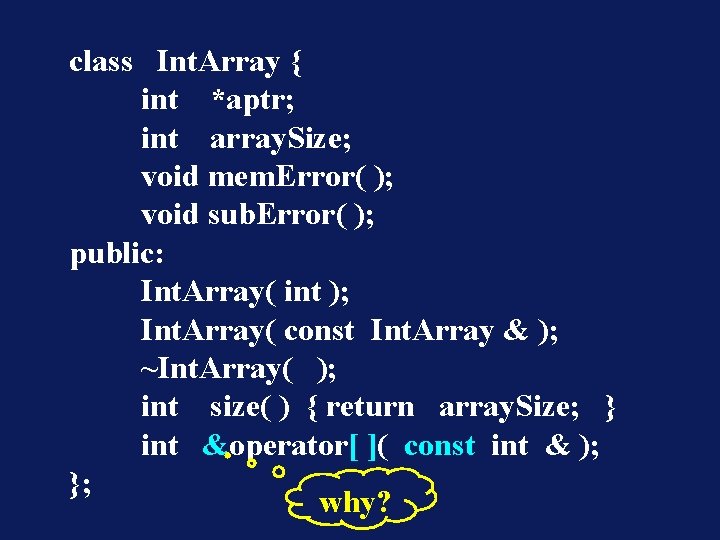
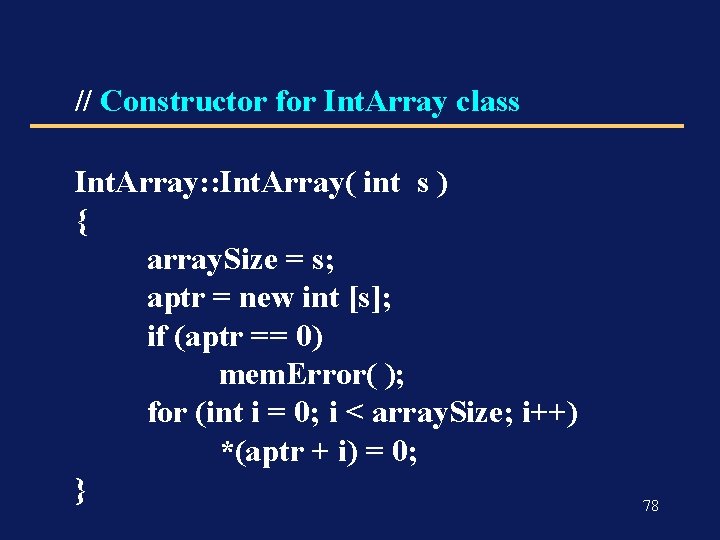
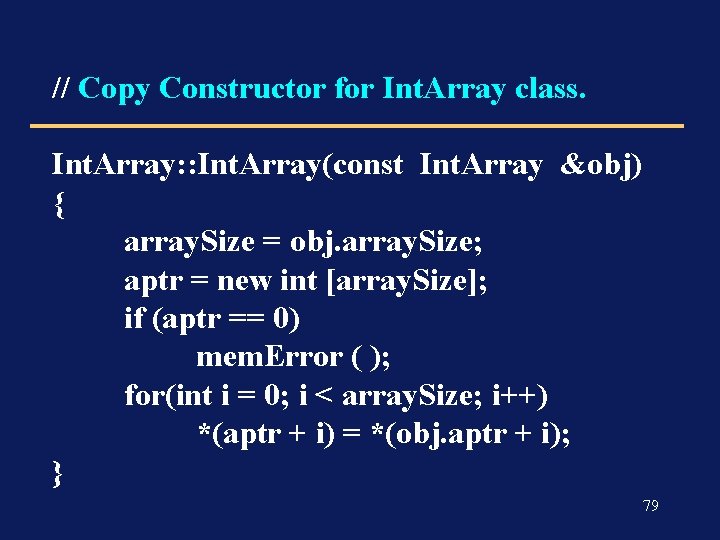
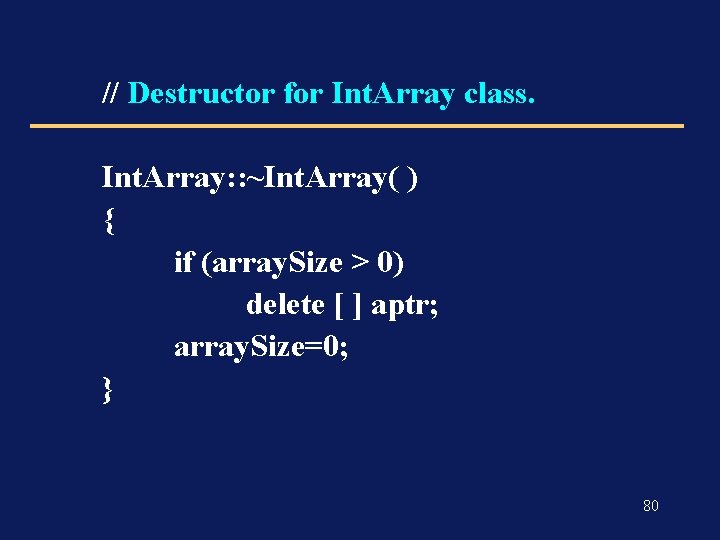
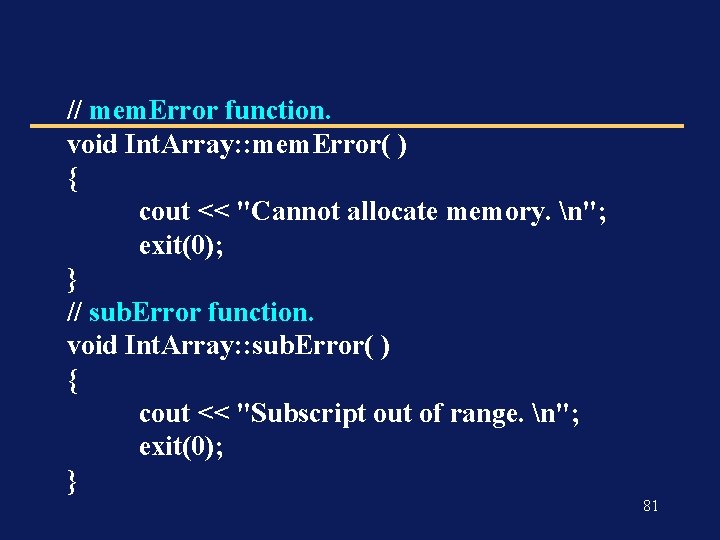
![// Overloaded [ ] operator. int & Int. Array: : operator[ ] (const int // Overloaded [ ] operator. int & Int. Array: : operator[ ] (const int](https://slidetodoc.com/presentation_image_h2/3116b27f6d5681323f4e291b9b95a294/image-82.jpg)
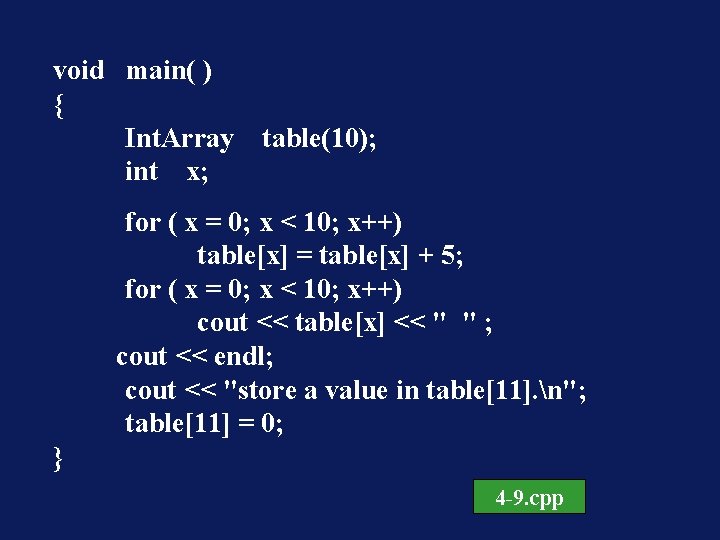
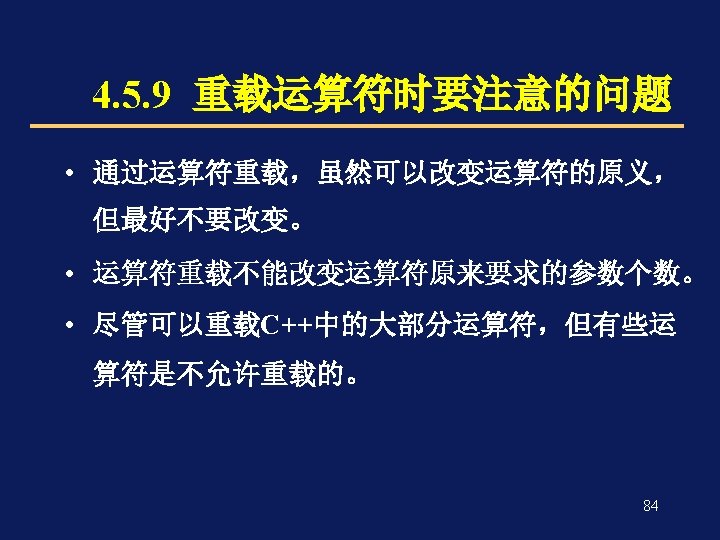
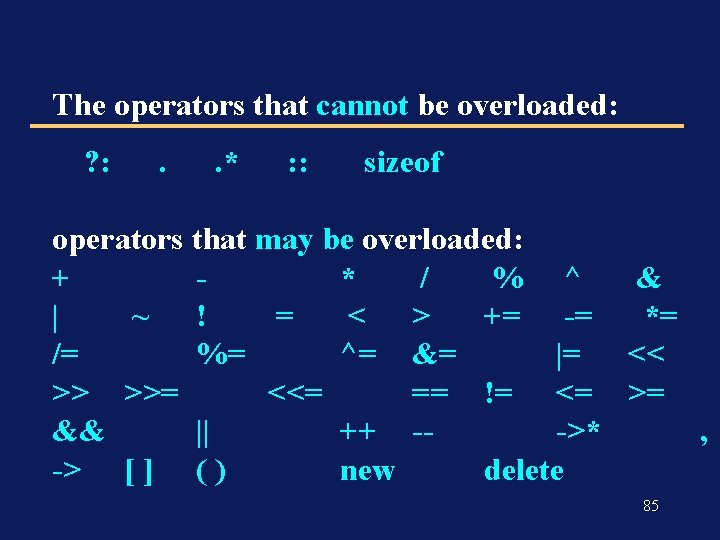
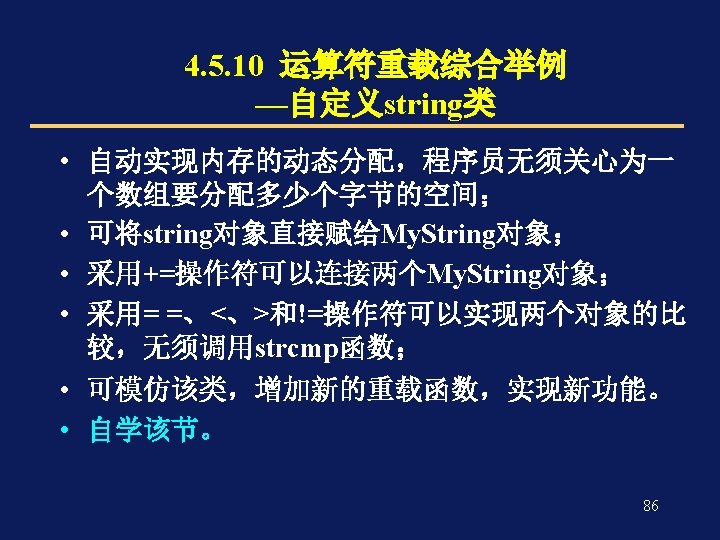
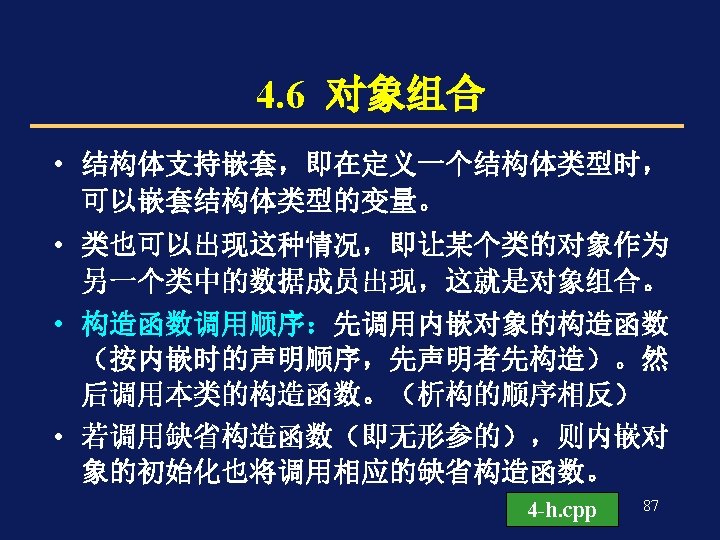
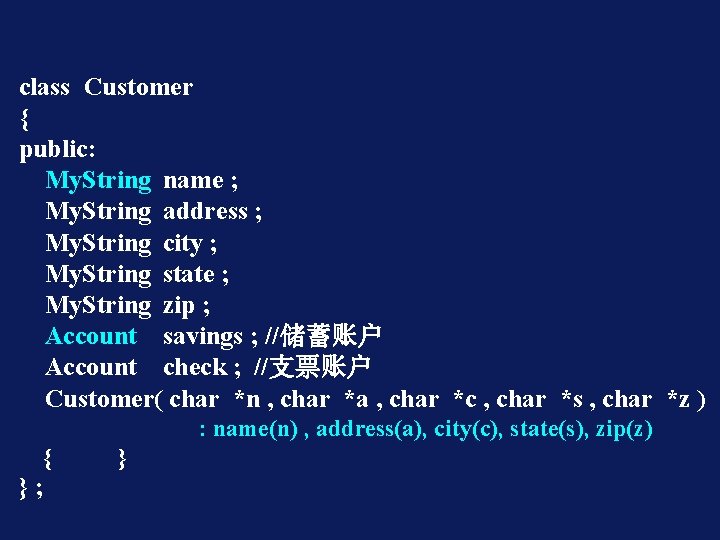
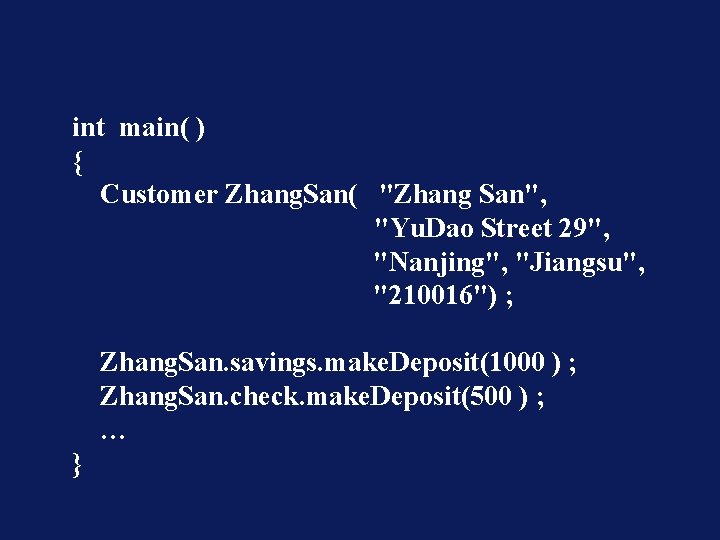
- Slides: 89
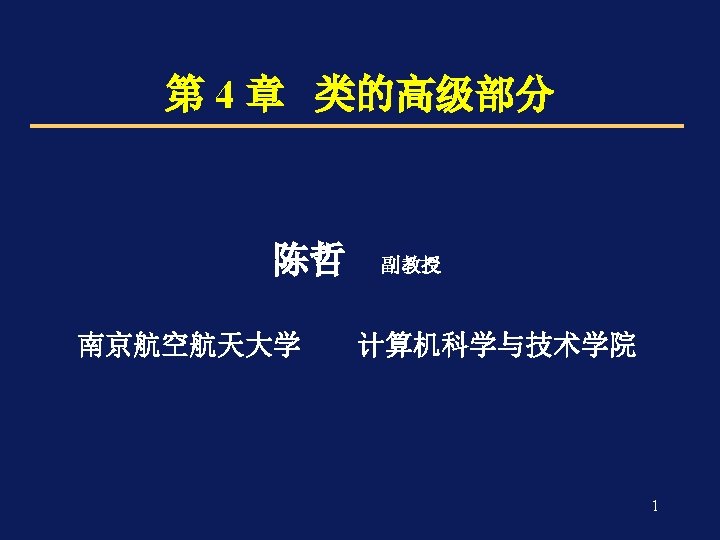
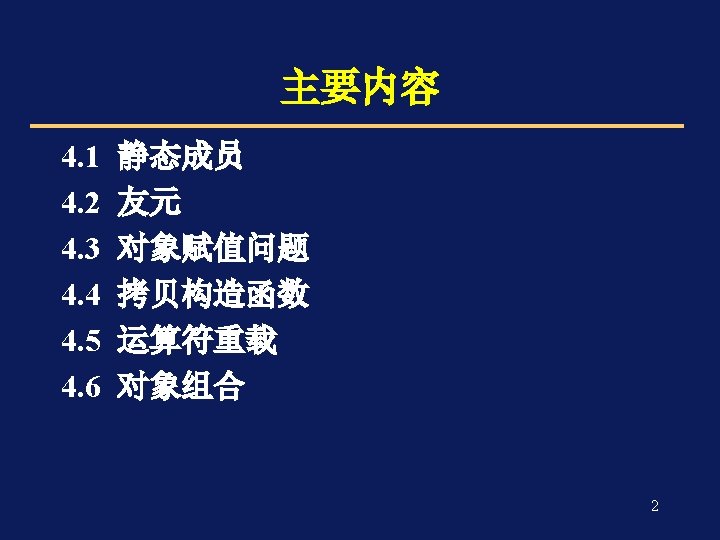
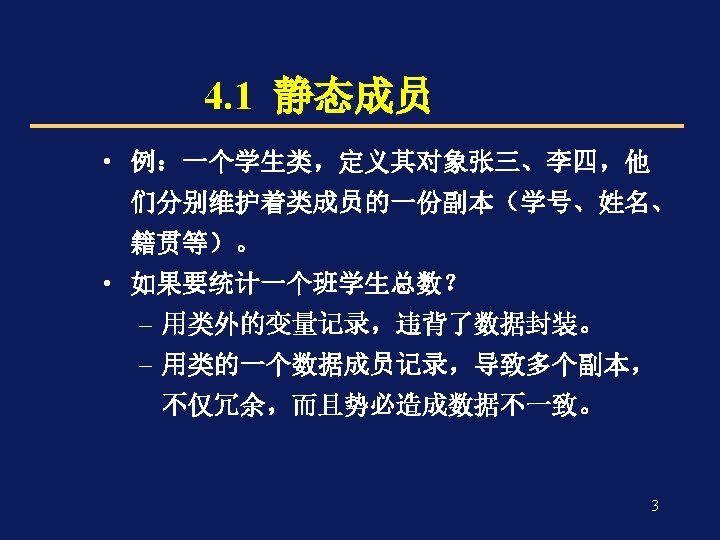
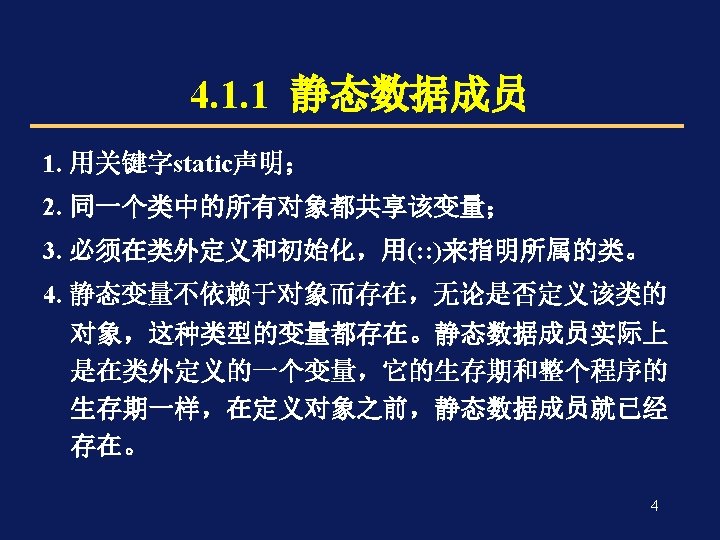
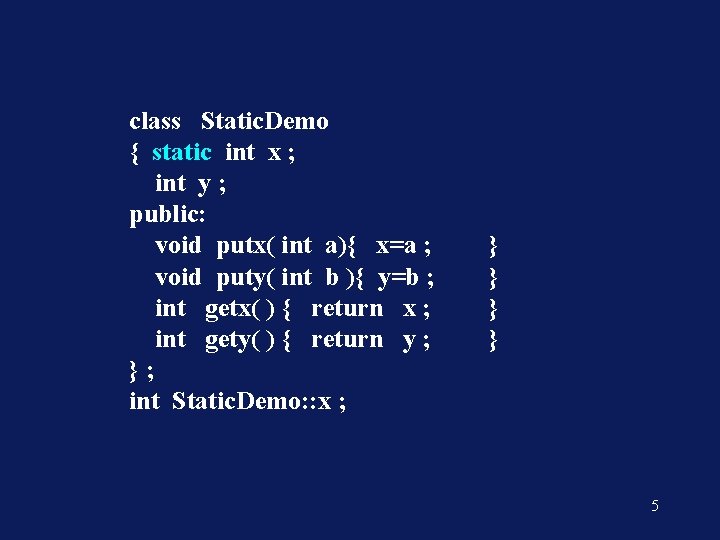
class Static. Demo { static int x ; int y ; public: void putx( int a){ x=a ; void puty( int b ){ y=b ; int getx( ) { return x ; int gety( ) { return y ; }; int Static. Demo: : x ; } } 5
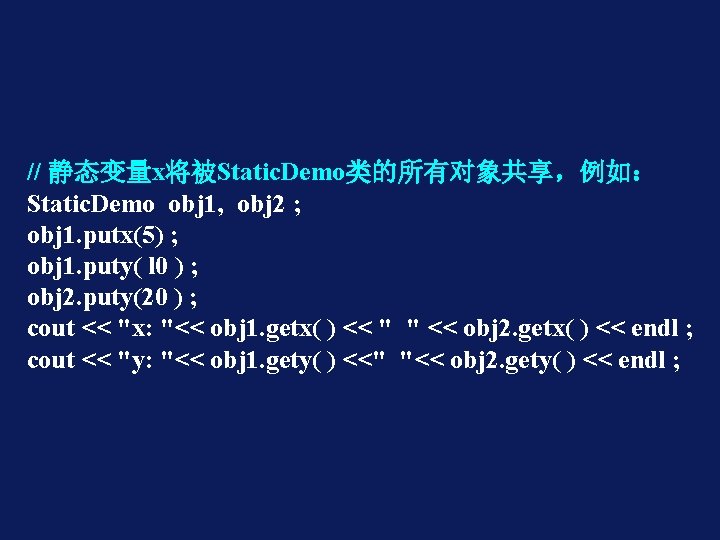
// 静态变量x将被Static. Demo类的所有对象共享,例如: Static. Demo obj 1, obj 2 ; obj 1. putx(5) ; obj 1. puty( l 0 ) ; obj 2. puty(20 ) ; cout << "x: "<< obj 1. getx( ) << " " << obj 2. getx( ) << endl ; cout << "y: "<< obj 1. gety( ) <<" "<< obj 2. gety( ) << endl ;
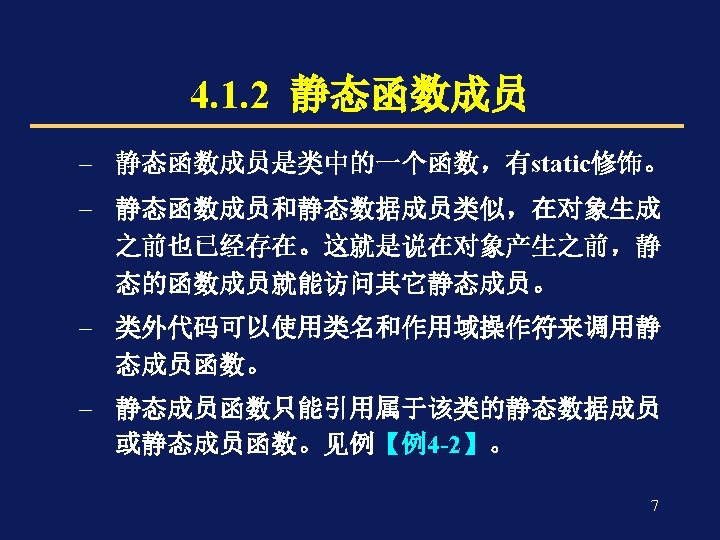
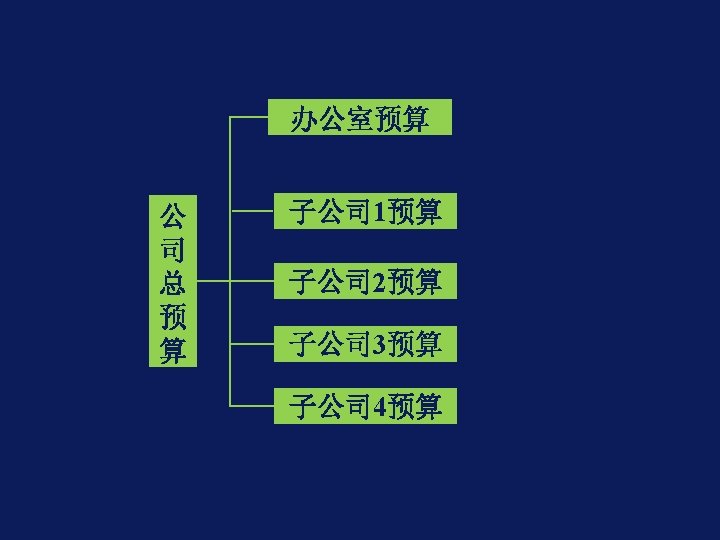
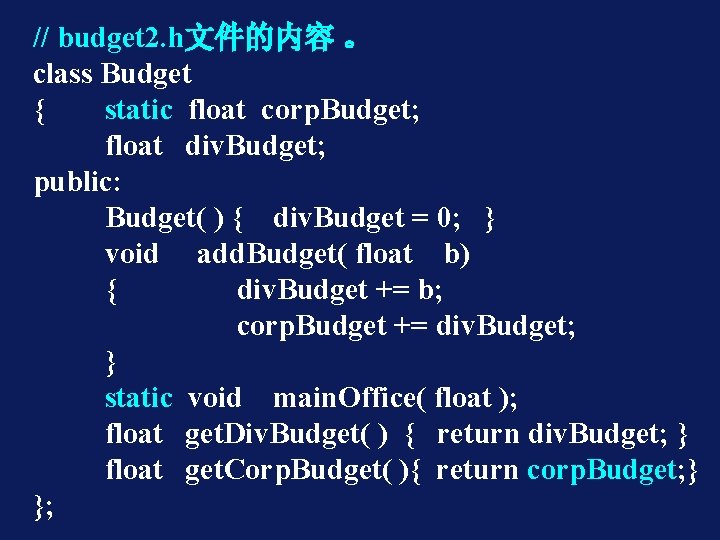
// budget 2. h文件的内容 。 class Budget { static float corp. Budget; float div. Budget; public: Budget( ) { div. Budget = 0; } void add. Budget( float b) { div. Budget += b; corp. Budget += div. Budget; } static void main. Office( float ); float get. Div. Budget( ) { return div. Budget; } float get. Corp. Budget( ){ return corp. Budget; } };
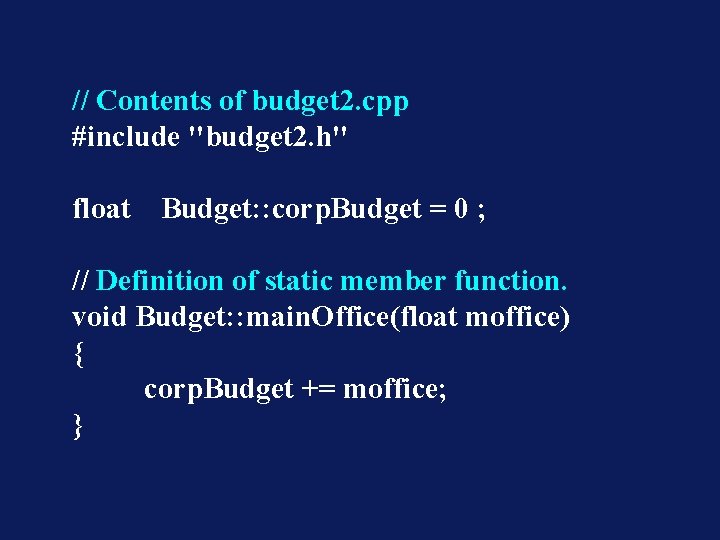
// Contents of budget 2. cpp #include "budget 2. h" float Budget: : corp. Budget = 0 ; // Definition of static member function. void Budget: : main. Office(float moffice) { corp. Budget += moffice; }
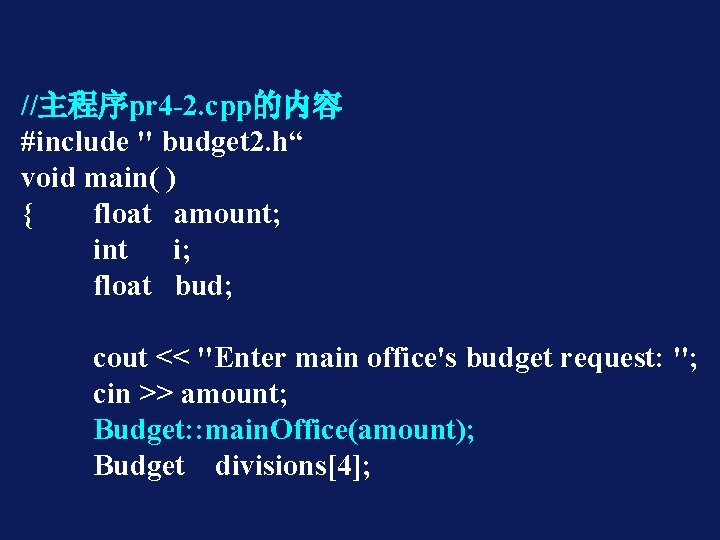
//主程序pr 4 -2. cpp的内容 #include " budget 2. h“ void main( ) { float amount; int i; float bud; cout << "Enter main office's budget request: "; cin >> amount; Budget: : main. Office(amount); Budget divisions[4];
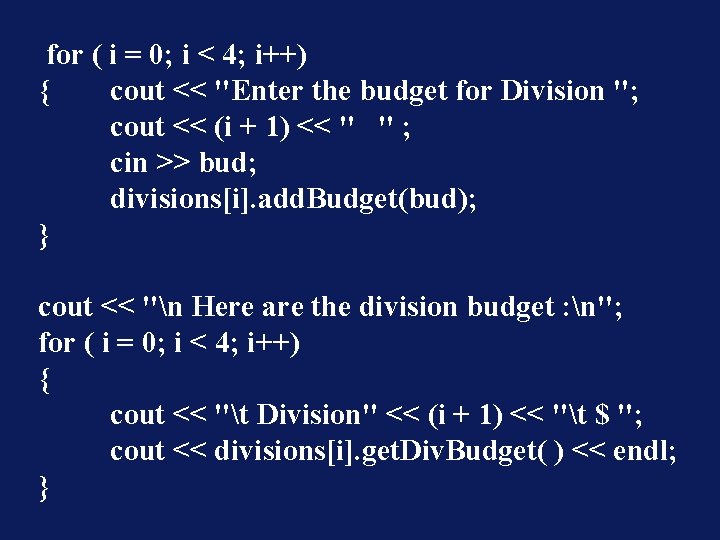
for ( i = 0; i < 4; i++) { cout << "Enter the budget for Division "; cout << (i + 1) << " " ; cin >> bud; divisions[i]. add. Budget(bud); } cout << "n Here are the division budget : n"; for ( i = 0; i < 4; i++) { cout << "t Division" << (i + 1) << "t $ "; cout << divisions[i]. get. Div. Budget( ) << endl; }
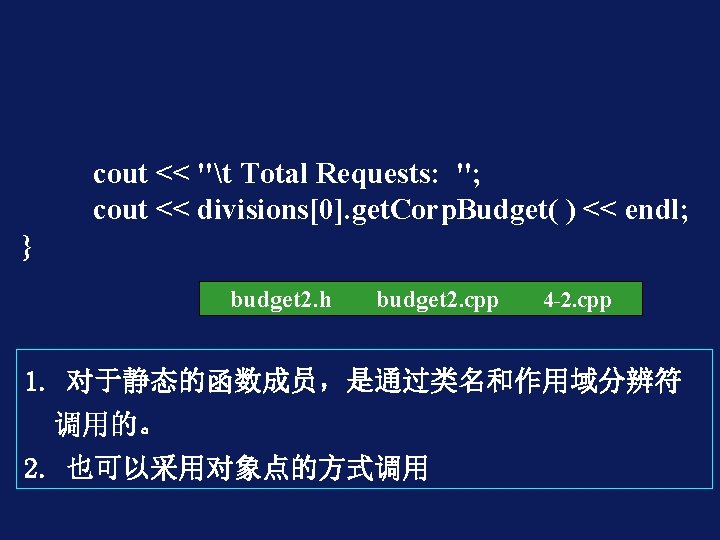
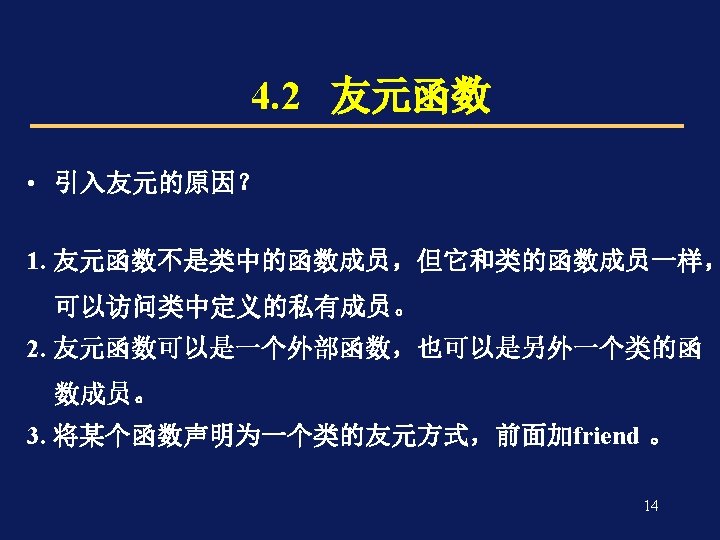
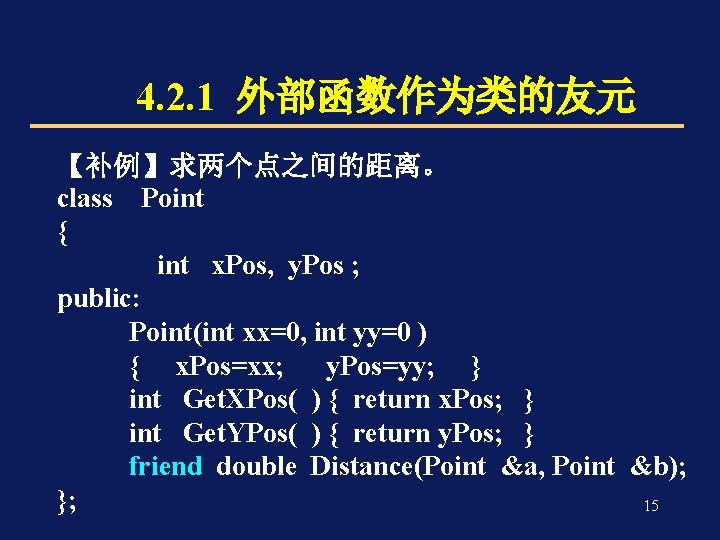
4. 2. 1 外部函数作为类的友元 【补例】求两个点之间的距离。 class Point { int x. Pos, y. Pos ; public: Point(int xx=0, int yy=0 ) { x. Pos=xx; y. Pos=yy; } int Get. XPos( ) { return x. Pos; } int Get. YPos( ) { return y. Pos; } friend double Distance(Point &a, Point &b); }; 15
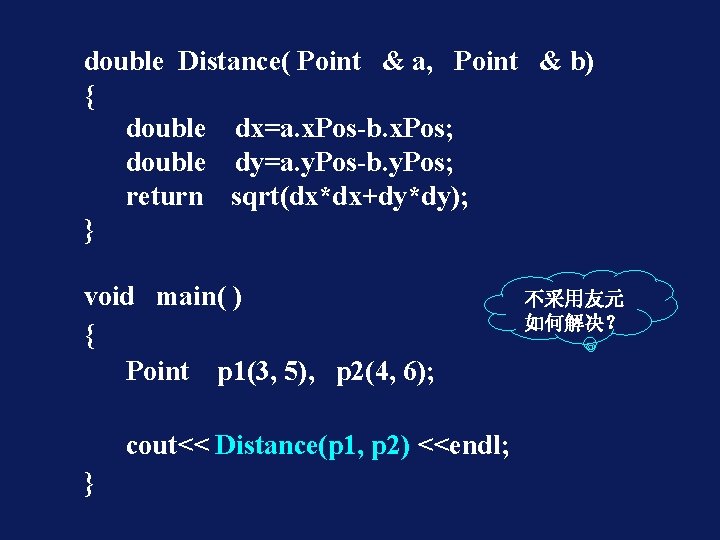
double Distance( Point & a, Point & b) { double dx=a. x. Pos-b. x. Pos; double dy=a. y. Pos-b. y. Pos; return sqrt(dx*dx+dy*dy); } void main( ) { Point p 1(3, 5), p 2(4, 6); cout<< Distance(p 1, p 2) <<endl; } 不采用友元 如何解决?
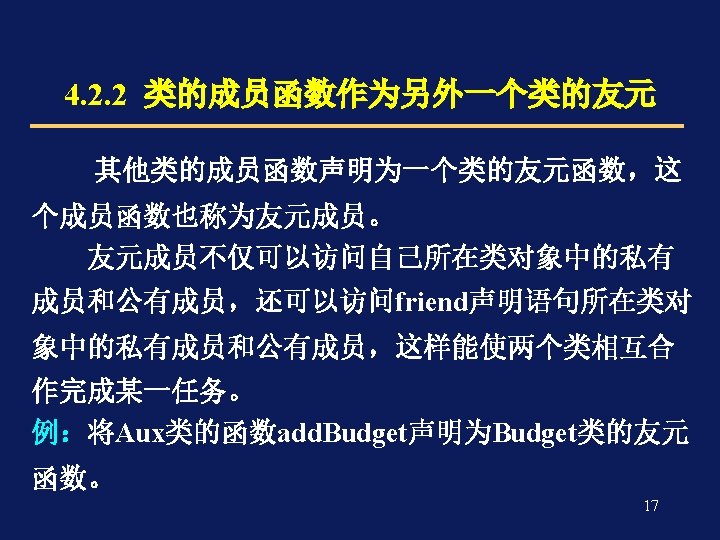
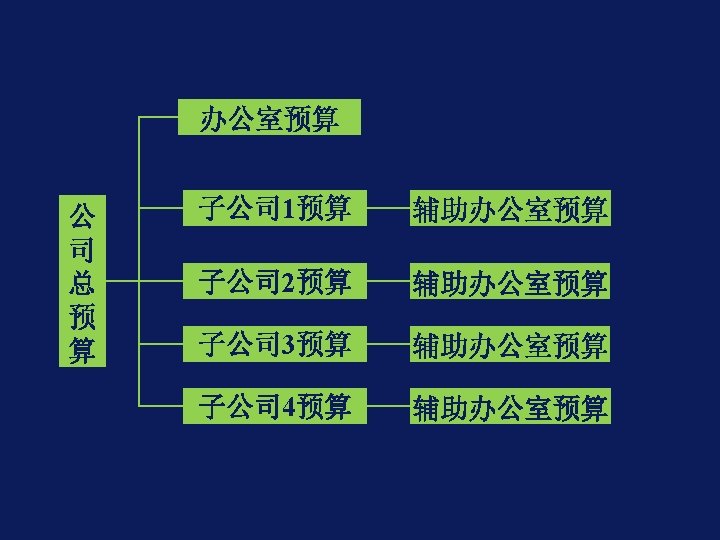
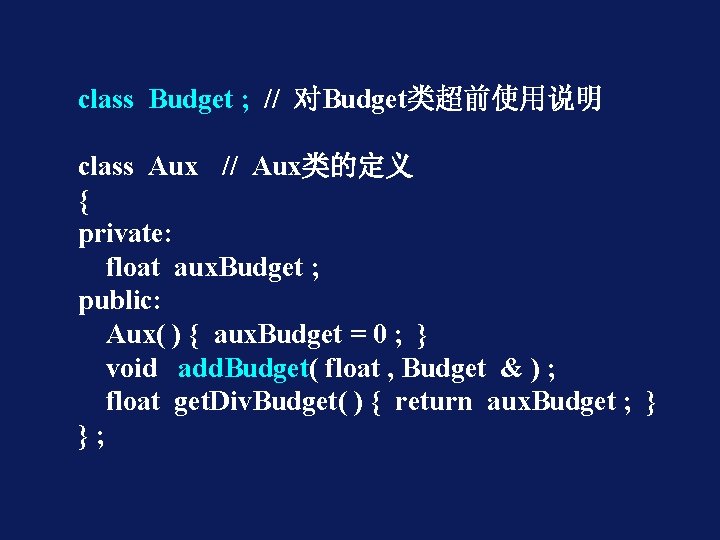
class Budget ; // 对Budget类超前使用说明 class Aux // Aux类的定义 { private: float aux. Budget ; public: Aux( ) { aux. Budget = 0 ; } void add. Budget( float , Budget & ) ; float get. Div. Budget( ) { return aux. Budget ; } };
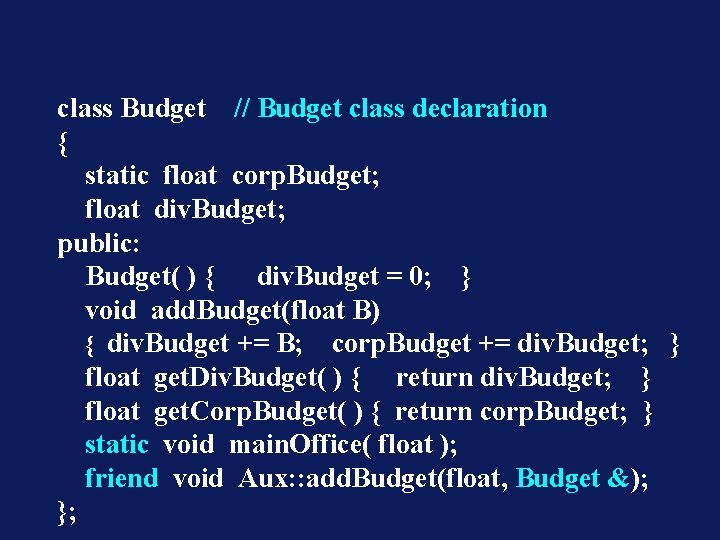
class Budget // Budget class declaration { static float corp. Budget; float div. Budget; public: Budget( ) { div. Budget = 0; } void add. Budget(float B) { div. Budget += B; corp. Budget += div. Budget; } float get. Div. Budget( ) { return div. Budget; } float get. Corp. Budget( ) { return corp. Budget; } static void main. Office( float ); friend void Aux: : add. Budget(float, Budget &); };
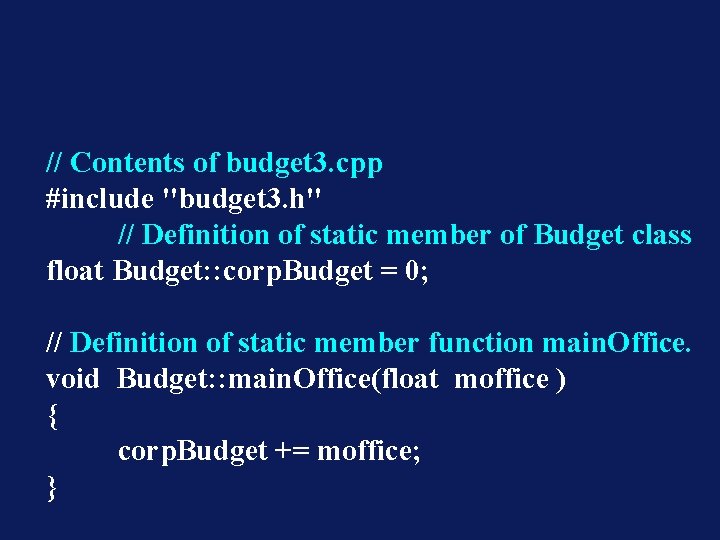
// Contents of budget 3. cpp #include "budget 3. h" // Definition of static member of Budget class float Budget: : corp. Budget = 0; // Definition of static member function main. Office. void Budget: : main. Office(float moffice ) { corp. Budget += moffice; }
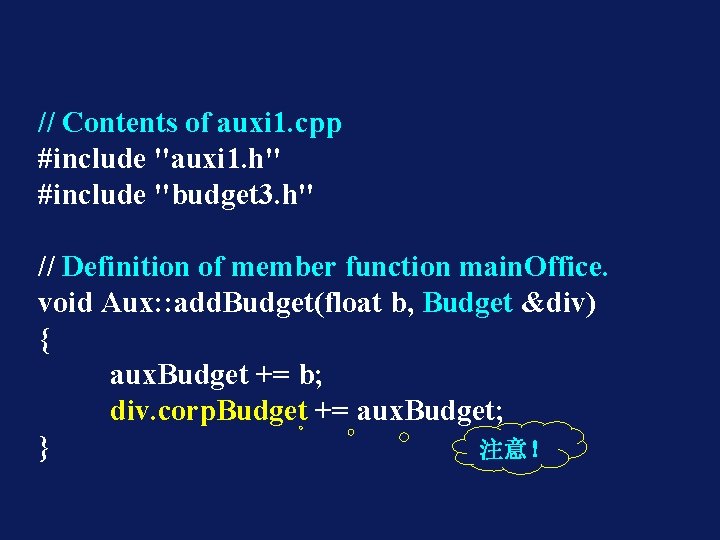
// Contents of auxi 1. cpp #include "auxi 1. h" #include "budget 3. h" // Definition of member function main. Office. void Aux: : add. Budget(float b, Budget &div) { aux. Budget += b; div. corp. Budget += aux. Budget; } 注意!
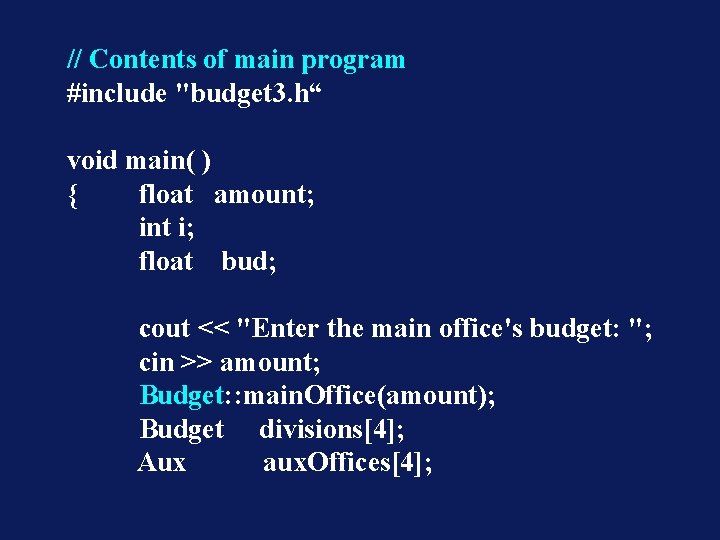
// Contents of main program #include "budget 3. h“ void main( ) { float amount; int i; float bud; cout << "Enter the main office's budget: "; cin >> amount; Budget: : main. Office(amount); Budget divisions[4]; Aux aux. Offices[4];
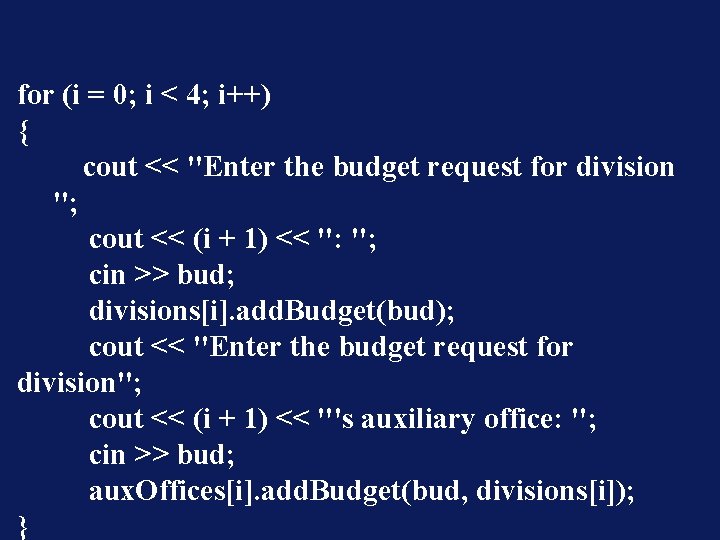
for (i = 0; i < 4; i++) { cout << "Enter the budget request for division "; cout << (i + 1) << ": "; cin >> bud; divisions[i]. add. Budget(bud); cout << "Enter the budget request for division"; cout << (i + 1) << "'s auxiliary office: "; cin >> bud; aux. Offices[i]. add. Budget(bud, divisions[i]); }
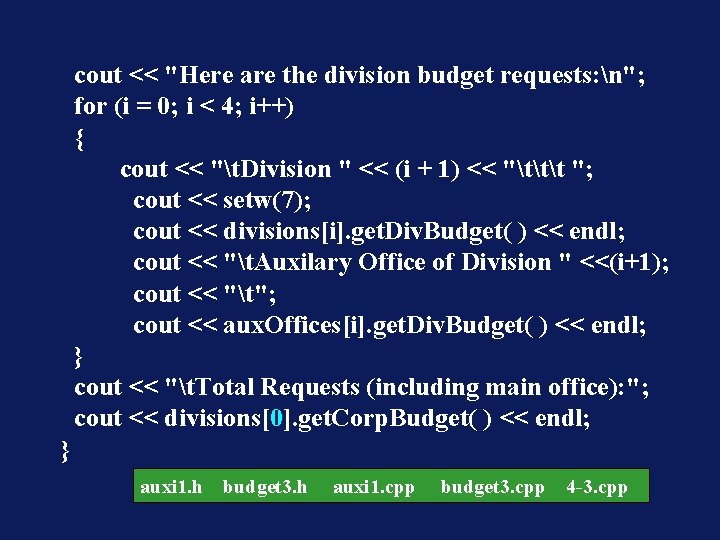
cout << "Here are the division budget requests: n"; for (i = 0; i < 4; i++) { cout << "t. Division " << (i + 1) << "ttt "; cout << setw(7); cout << divisions[i]. get. Div. Budget( ) << endl; cout << "t. Auxilary Office of Division " <<(i+1); cout << "t"; cout << aux. Offices[i]. get. Div. Budget( ) << endl; } cout << "t. Total Requests (including main office): "; cout << divisions[0]. get. Corp. Budget( ) << endl; } auxi 1. h budget 3. h auxi 1. cpp budget 3. cpp 4 -3. cpp
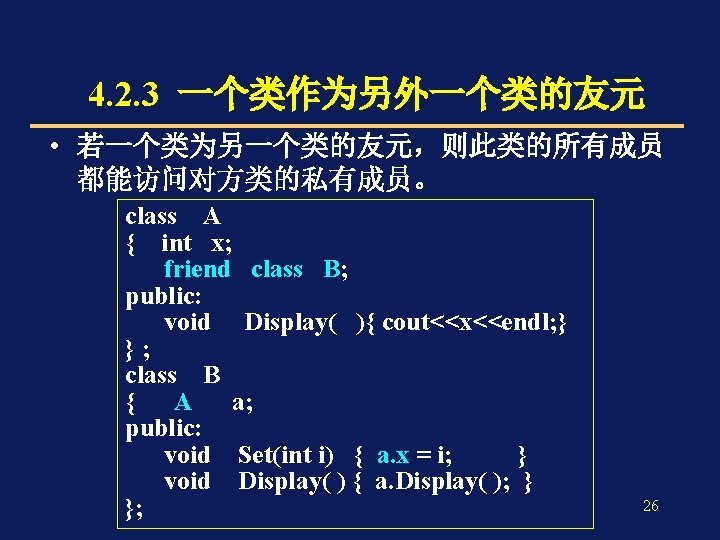
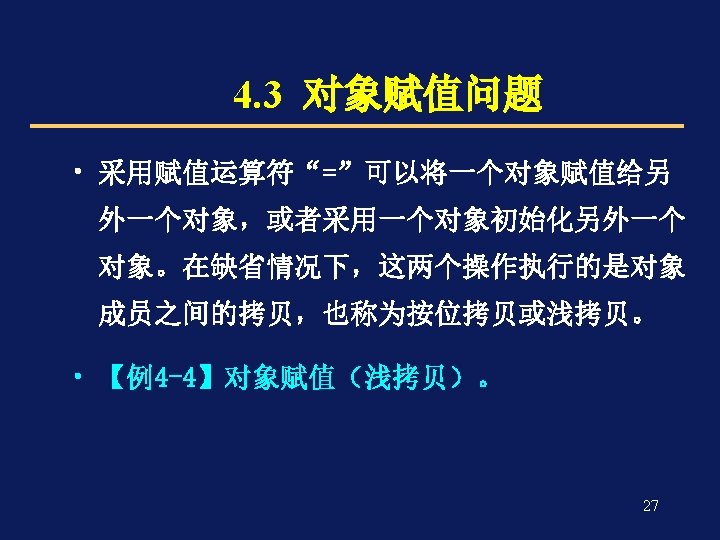
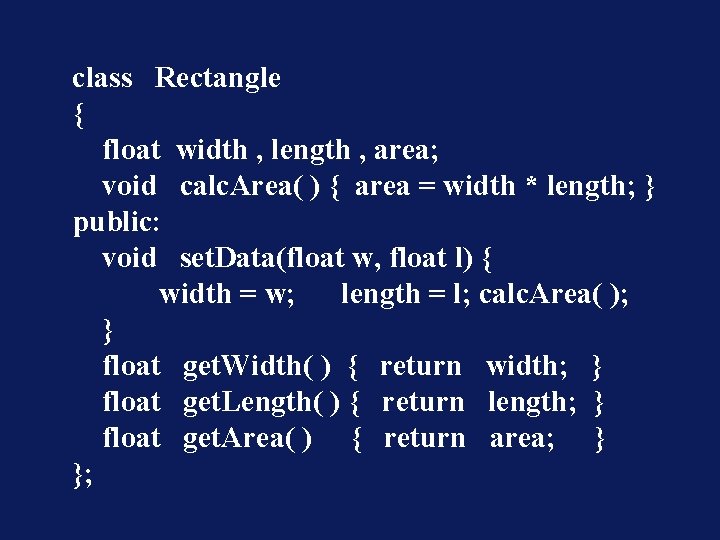
class Rectangle { float width , length , area; void calc. Area( ) { area = width * length; } public: void set. Data(float w, float l) { width = w; length = l; calc. Area( ); } float get. Width( ) { return width; } float get. Length( ) { return length; } float get. Area( ) { return area; } };
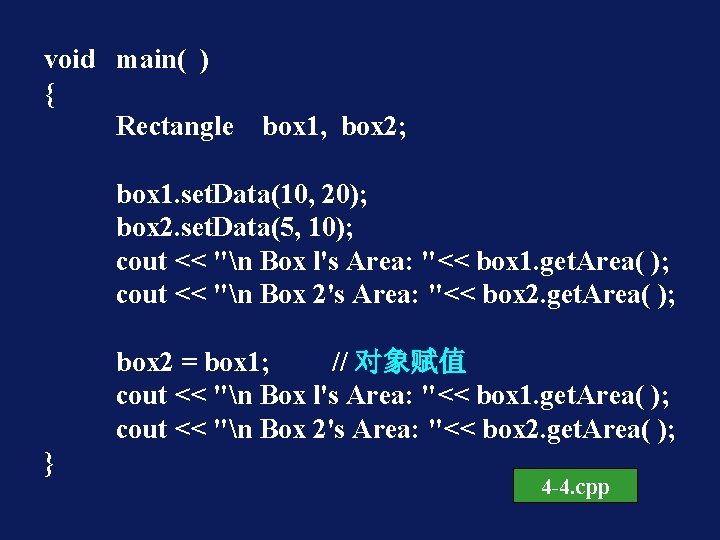
void main( ) { Rectangle box 1, box 2; box 1. set. Data(10, 20); box 2. set. Data(5, 10); cout << "n Box l's Area: "<< box 1. get. Area( ); cout << "n Box 2's Area: "<< box 2. get. Area( ); box 2 = box 1; // 对象赋值 cout << "n Box l's Area: "<< box 1. get. Area( ); cout << "n Box 2's Area: "<< box 2. get. Area( ); } 4 -4. cpp
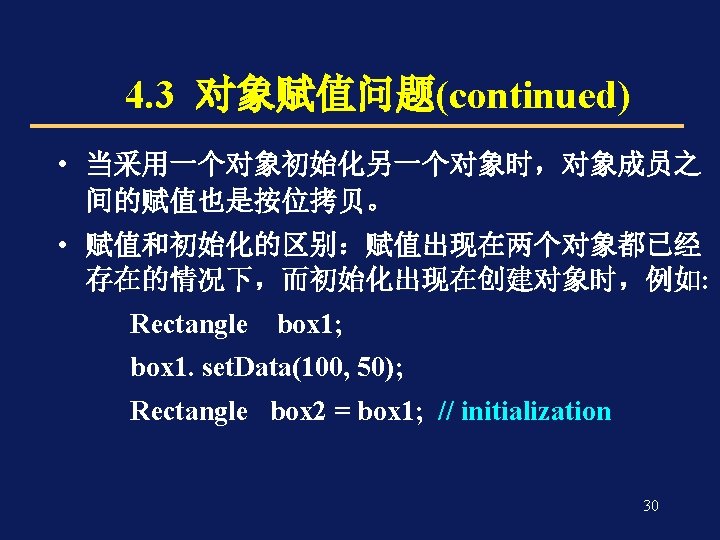
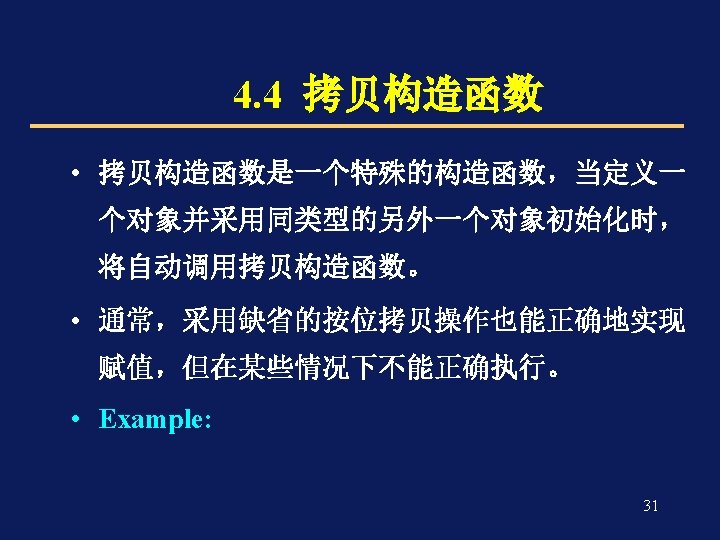
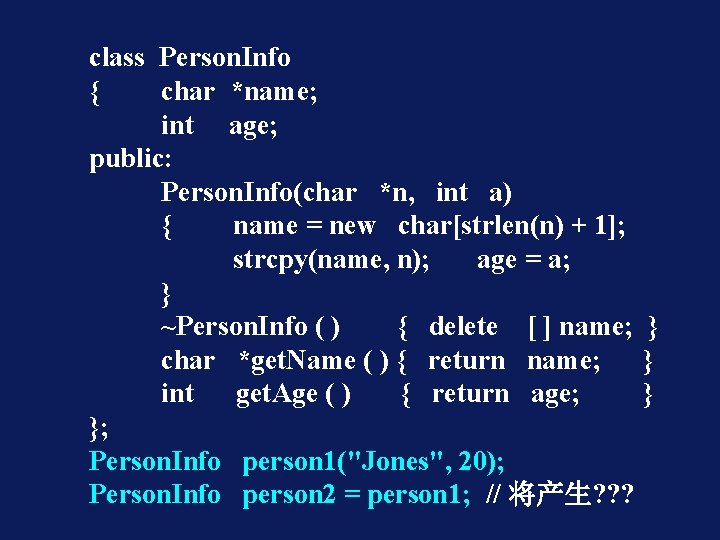
class Person. Info { char *name; int age; public: Person. Info(char *n, int a) { name = new char[strlen(n) + 1]; strcpy(name, n); age = a; } ~Person. Info ( ) { delete [ ] name; } char *get. Name ( ) { return name; } int get. Age ( ) { return age; } }; Person. Info person 1("Jones", 20); Person. Info person 2 = person 1; // 将产生? ? ?
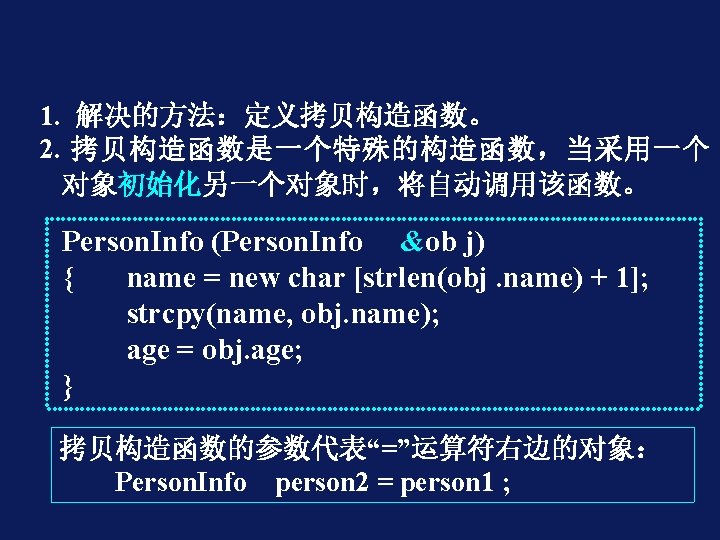
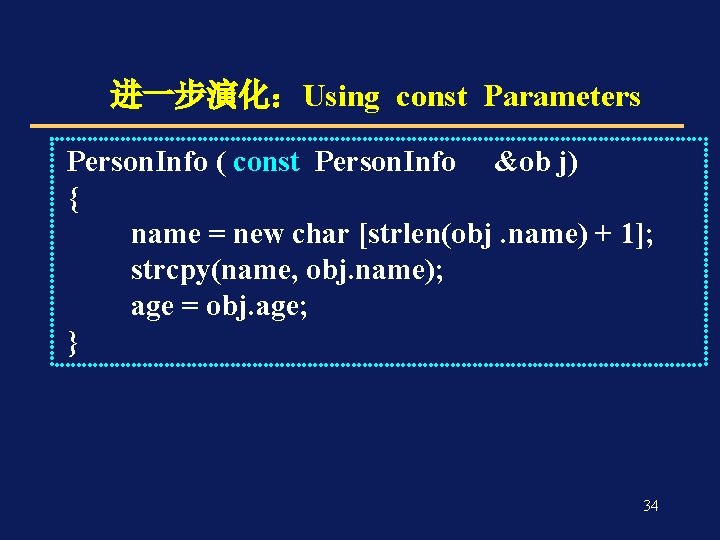
进一步演化:Using const Parameters Person. Info ( const Person. Info &ob j) { name = new char [strlen(obj. name) + 1]; strcpy(name, obj. name); age = obj. age; } 34
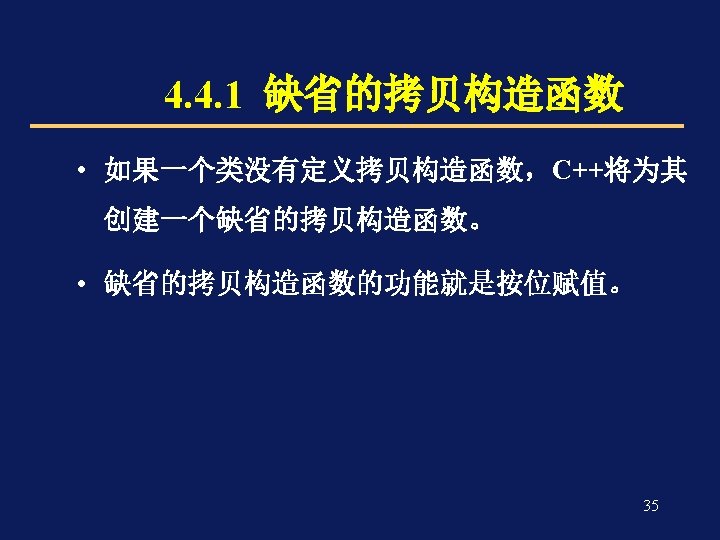
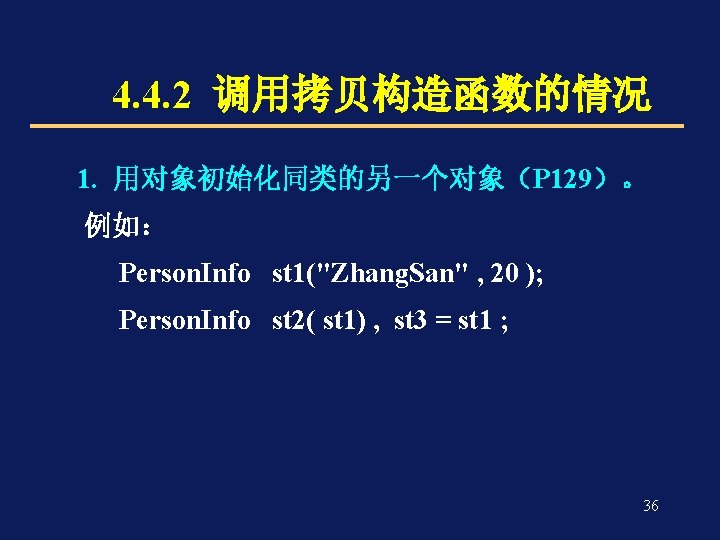
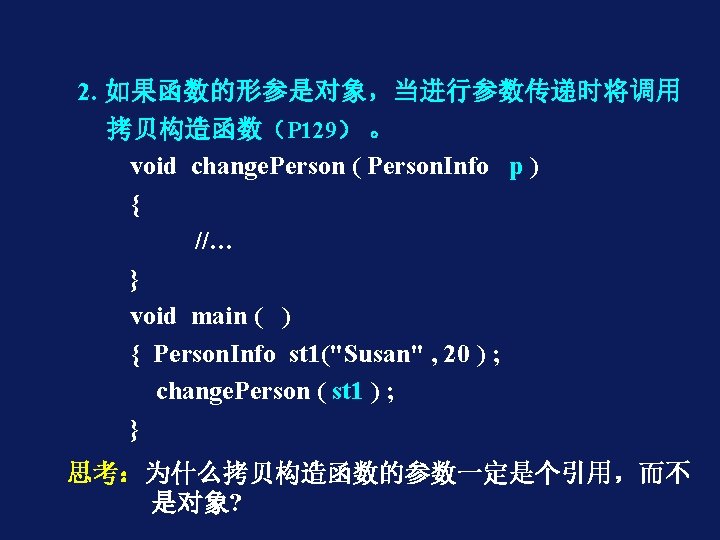
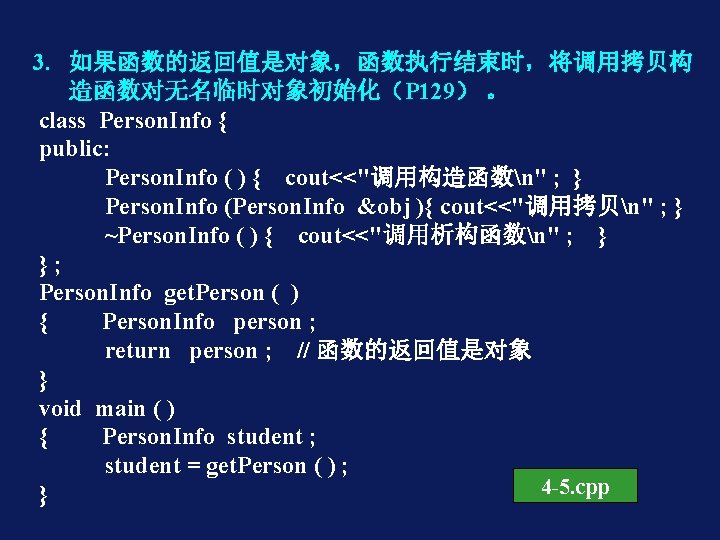
3. 如果函数的返回值是对象,函数执行结束时,将调用拷贝构 造函数对无名临时对象初始化(P 129) 。 class Person. Info { public: Person. Info ( ) { cout<<"调用构造函数n" ; } Person. Info (Person. Info &obj ){ cout<<"调用拷贝n" ; } ~Person. Info ( ) { cout<<"调用析构函数n" ; } }; Person. Info get. Person ( ) { Person. Info person ; return person ; // 函数的返回值是对象 } void main ( ) { Person. Info student ; student = get. Person ( ) ; 4 -5. cpp }
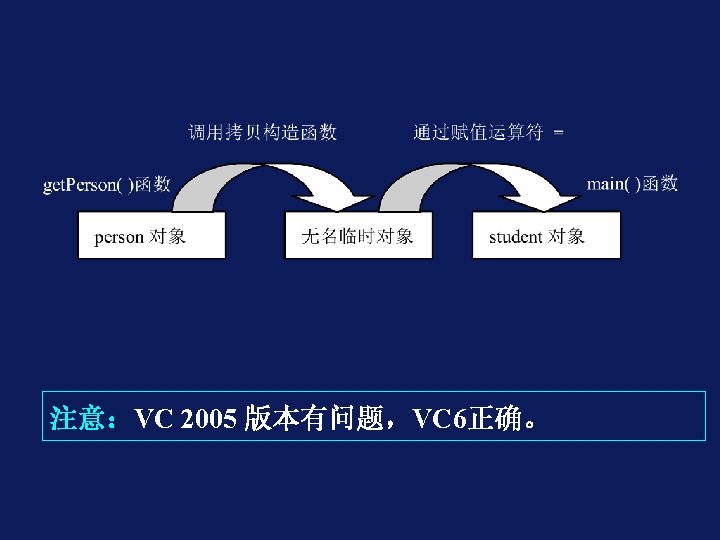
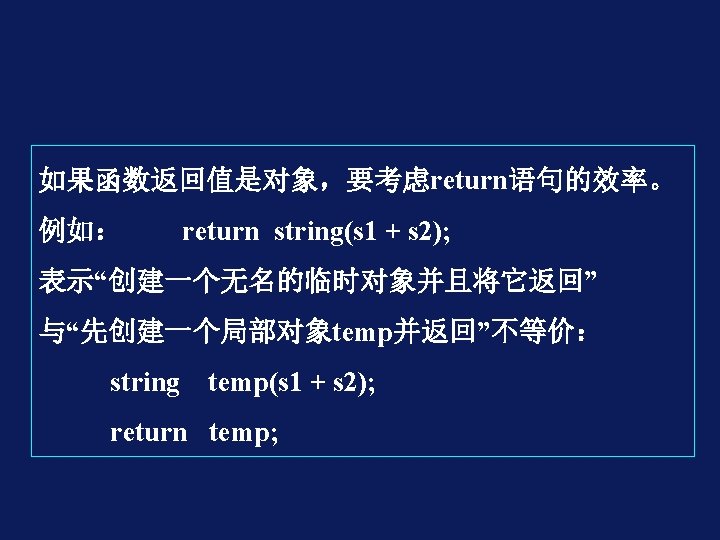
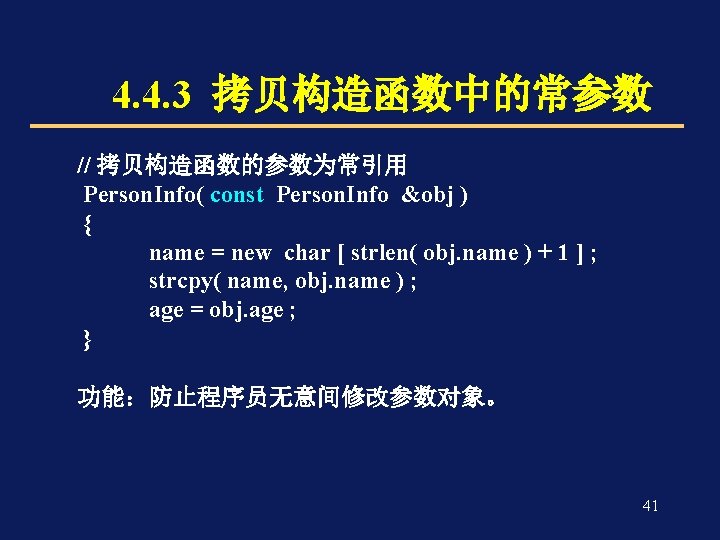
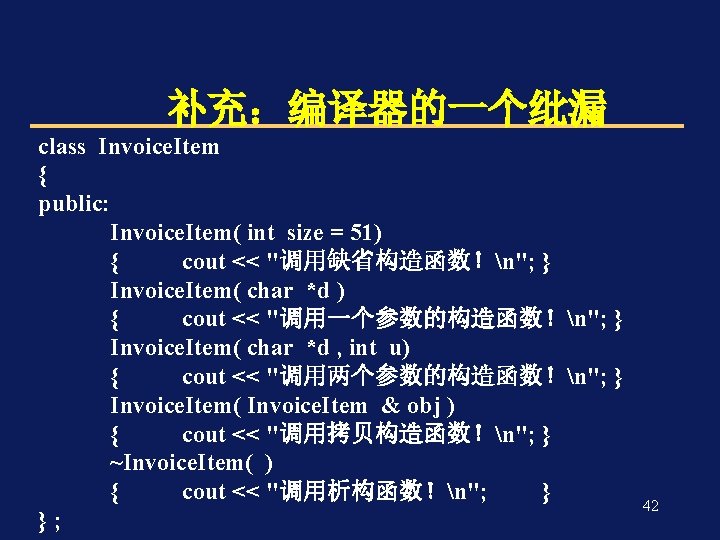
补充:编译器的一个纰漏 class Invoice. Item { public: Invoice. Item( int size = 51) { cout << "调用缺省构造函数!n"; } Invoice. Item( char *d ) { cout << "调用一个参数的构造函数!n"; } Invoice. Item( char *d , int u) { cout << "调用两个参数的构造函数!n"; } Invoice. Item( Invoice. Item & obj ) { cout << "调用拷贝构造函数!n"; } ~Invoice. Item( ) { cout << "调用析构函数!n"; } }; 42
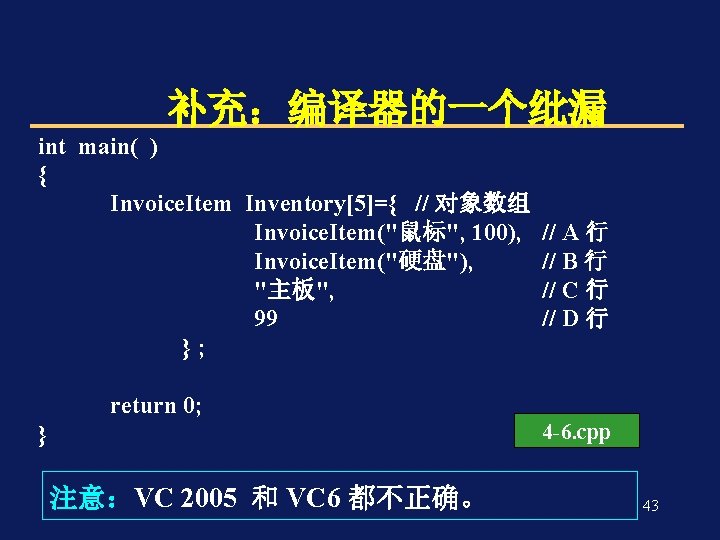
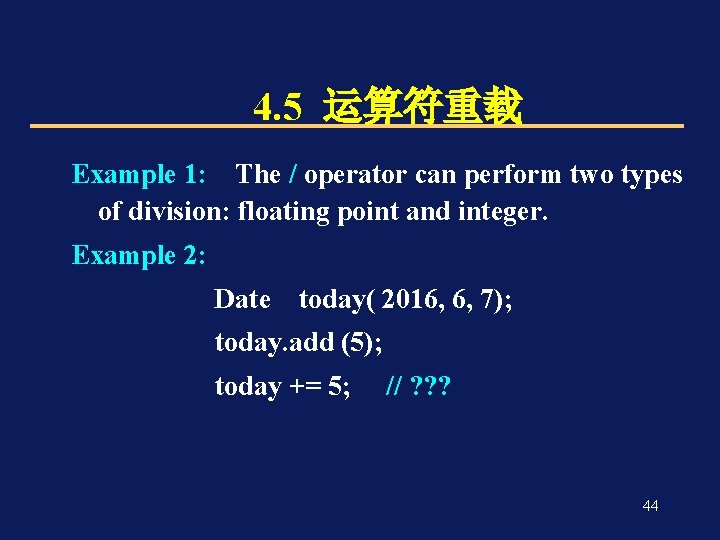
4. 5 运算符重载 Example 1: The / operator can perform two types of division: floating point and integer. Example 2: Date today( 2016, 6, 7); today. add (5); today += 5; // ? ? ? 44
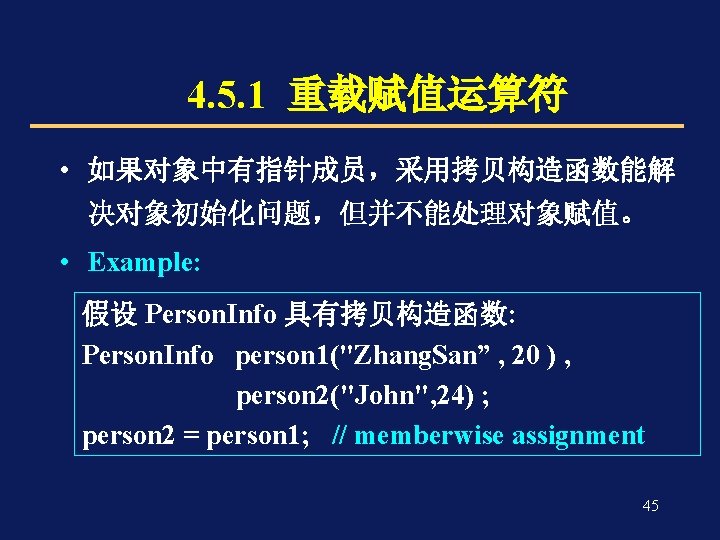
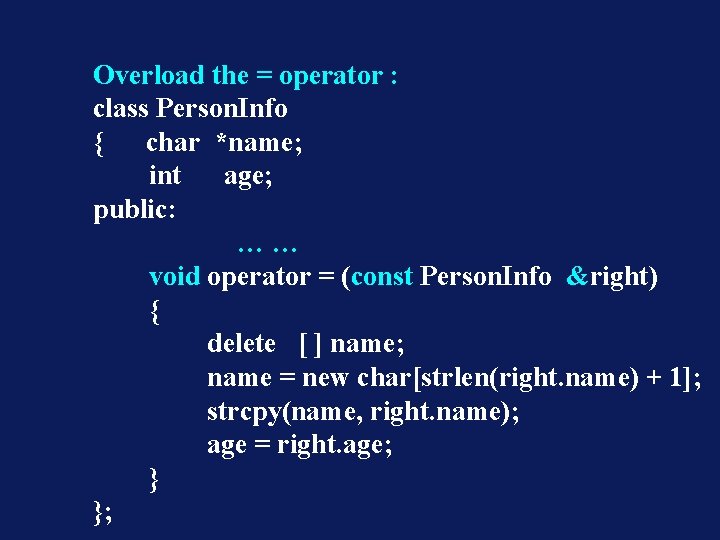
Overload the = operator : class Person. Info { char *name; int age; public: …… void operator = (const Person. Info &right) { delete [ ] name; name = new char[strlen(right. name) + 1]; strcpy(name, right. name); age = right. age; } };
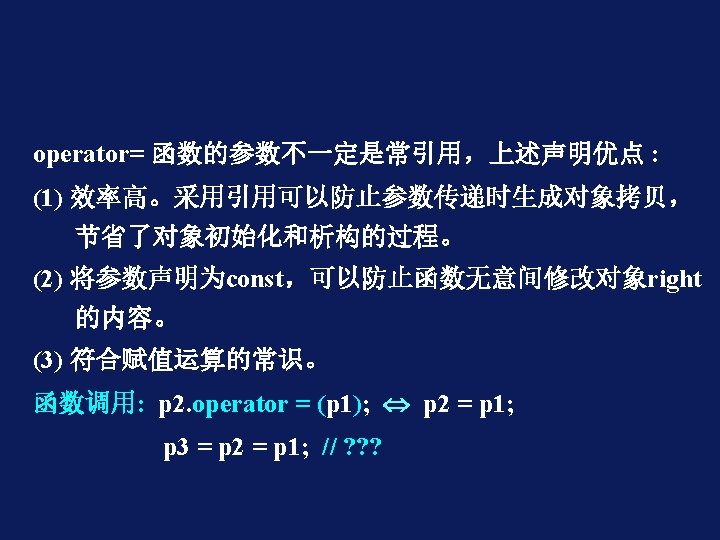
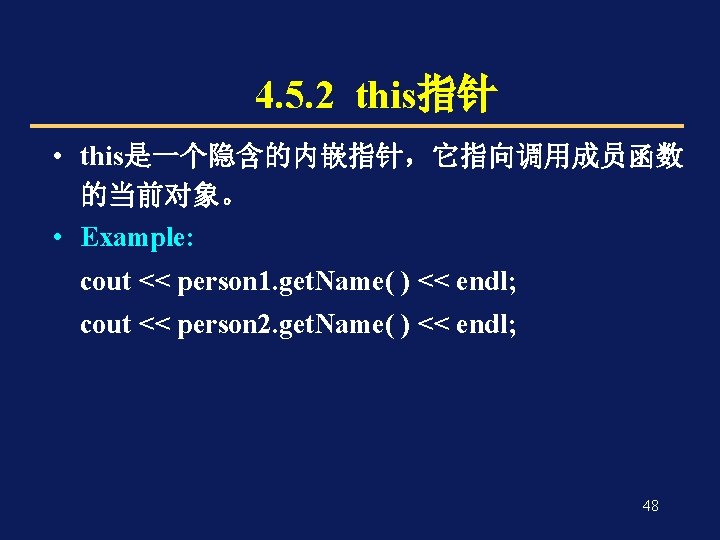
4. 5. 2 this指针 • this是一个隐含的内嵌指针,它指向调用成员函数 的当前对象。 • Example: cout << person 1. get. Name( ) << endl; cout << person 2. get. Name( ) << endl; 48
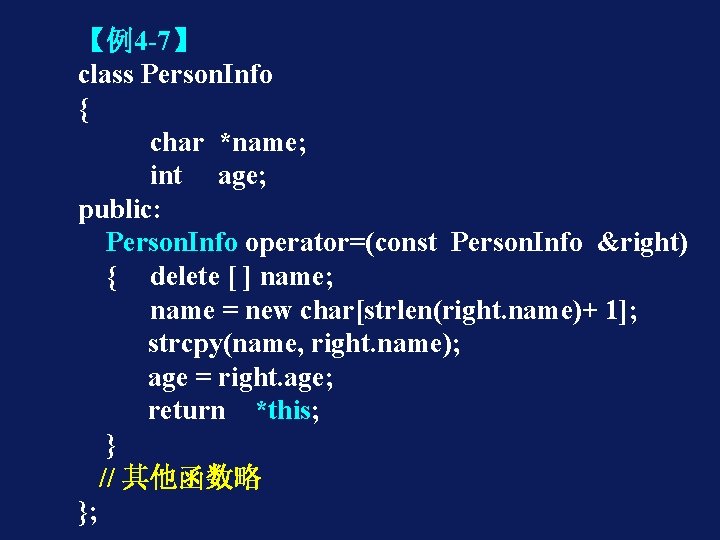
【例4 -7】 class Person. Info { char *name; int age; public: Person. Info operator=(const Person. Info &right) { delete [ ] name; name = new char[strlen(right. name)+ 1]; strcpy(name, right. name); age = right. age; return *this; } // 其他函数略 };
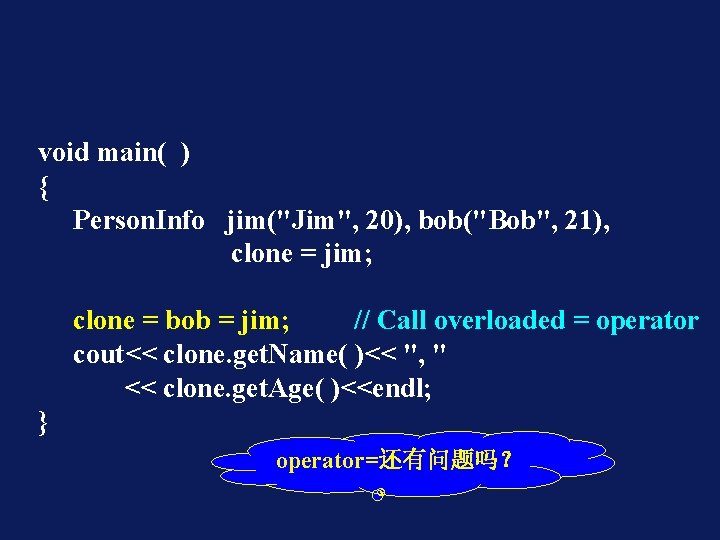
void main( ) { Person. Info jim("Jim", 20), bob("Bob", 21), clone = jim; clone = bob = jim; // Call overloaded = operator cout<< clone. get. Name( )<< ", " << clone. get. Age( )<<endl; } operator=还有问题吗?
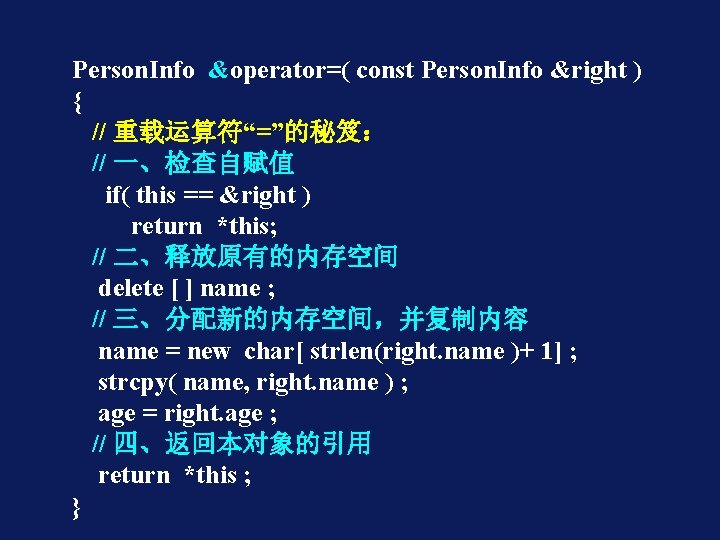
Person. Info &operator=( const Person. Info &right ) { // 重载运算符“=”的秘笈: // 一、检查自赋值 if( this == &right ) return *this; // 二、释放原有的内存空间 delete [ ] name ; // 三、分配新的内存空间,并复制内容 name = new char[ strlen(right. name )+ 1] ; strcpy( name, right. name ) ; age = right. age ; // 四、返回本对象的引用 return *this ; }
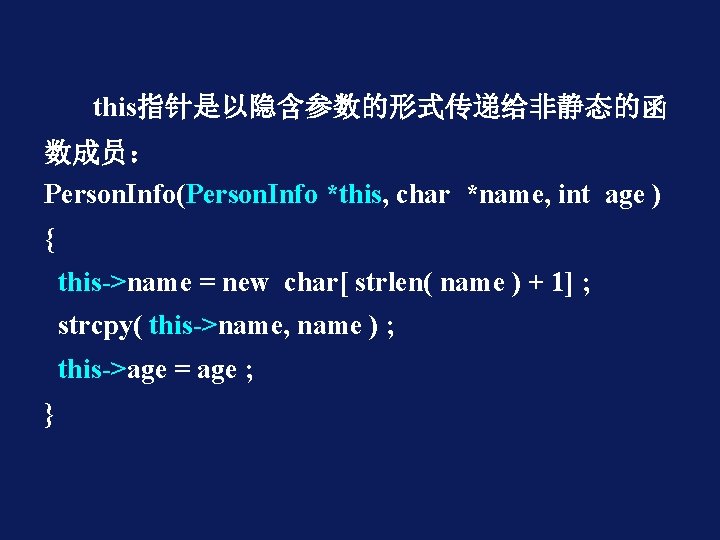
this指针是以隐含参数的形式传递给非静态的函 数成员: Person. Info(Person. Info *this, char *name, int age ) { this->name = new char[ strlen( name ) + 1] ; strcpy( this->name, name ) ; this->age = age ; }
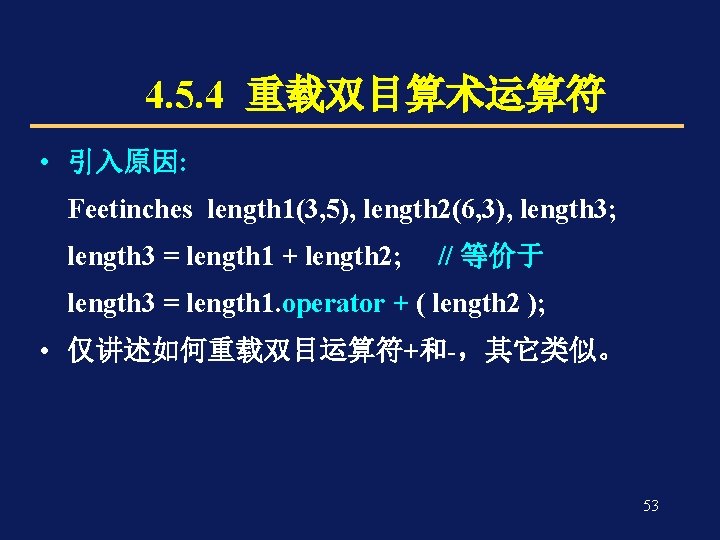
4. 5. 4 重载双目算术运算符 • 引入原因: Feetinches length 1(3, 5), length 2(6, 3), length 3; length 3 = length 1 + length 2; // 等价于 length 3 = length 1. operator + ( length 2 ); • 仅讲述如何重载双目运算符+和-,其它类似。 53
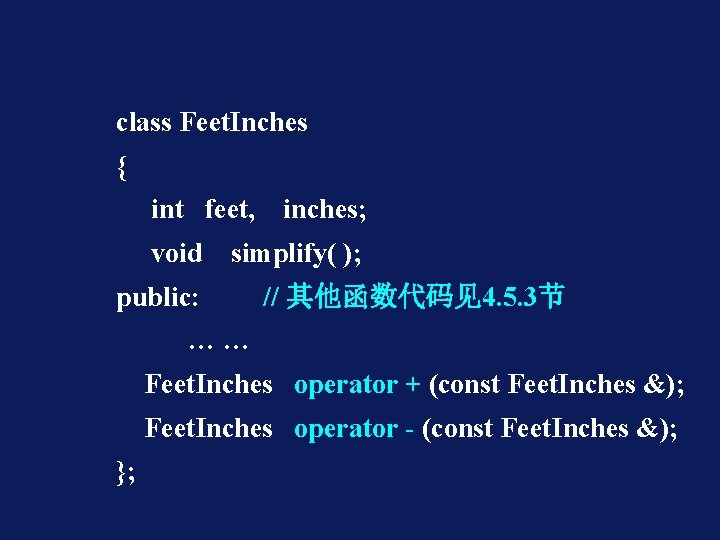
class Feet. Inches { int feet, inches; void simplify( ); public: // 其他函数代码见4. 5. 3节 …… Feet. Inches operator + (const Feet. Inches &); Feet. Inches operator - (const Feet. Inches &); };
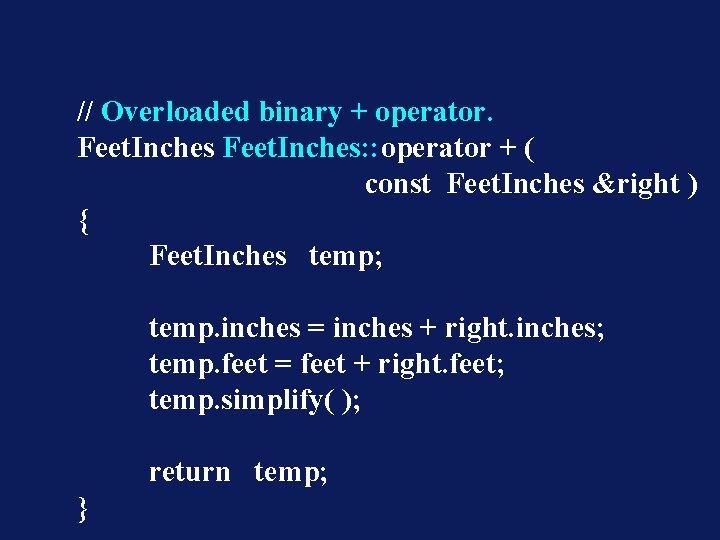
// Overloaded binary + operator. Feet. Inches: : operator + ( const Feet. Inches &right ) { Feet. Inches temp; temp. inches = inches + right. inches; temp. feet = feet + right. feet; temp. simplify( ); return temp; }
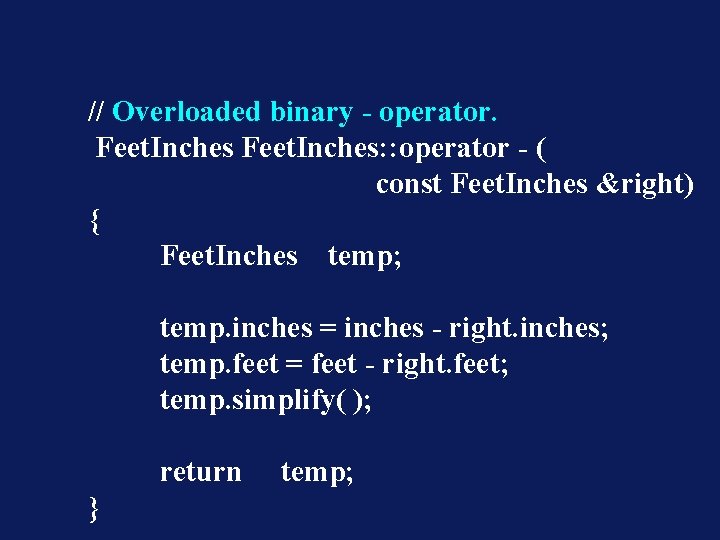
// Overloaded binary - operator. Feet. Inches: : operator - ( const Feet. Inches &right) { Feet. Inches temp; temp. inches = inches - right. inches; temp. feet = feet - right. feet; temp. simplify( ); return } temp;
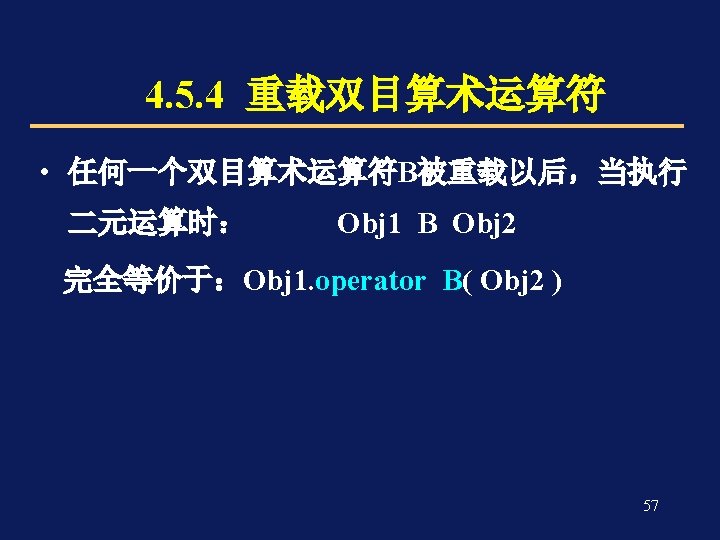
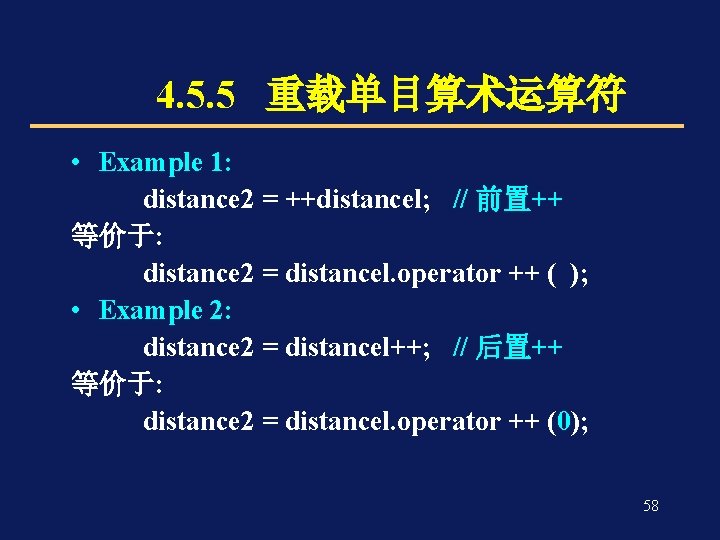
4. 5. 5 重载单目算术运算符 • Example 1: distance 2 = ++distancel; // 前置++ 等价于: distance 2 = distancel. operator ++ ( ); • Example 2: distance 2 = distancel++; // 后置++ 等价于: distance 2 = distancel. operator ++ (0); 58
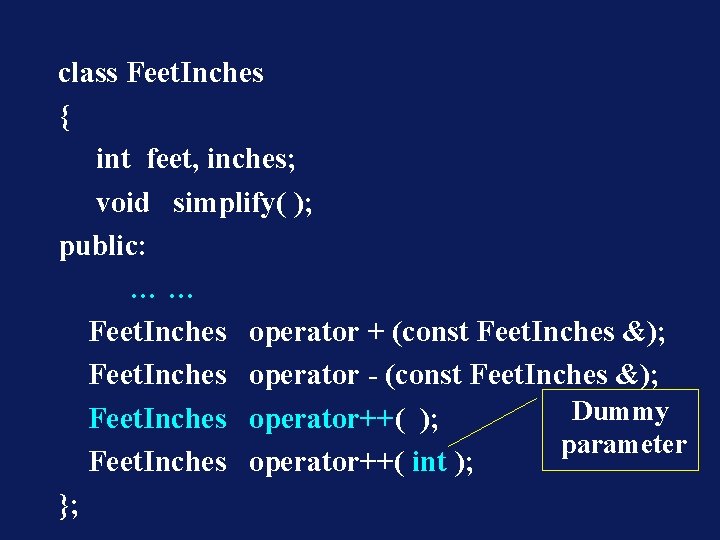
class Feet. Inches { int feet, inches; void simplify( ); public: …… Feet. Inches operator + (const Feet. Inches &); Feet. Inches operator - (const Feet. Inches &); Dummy Feet. Inches operator++( ); parameter Feet. Inches operator++( int ); };
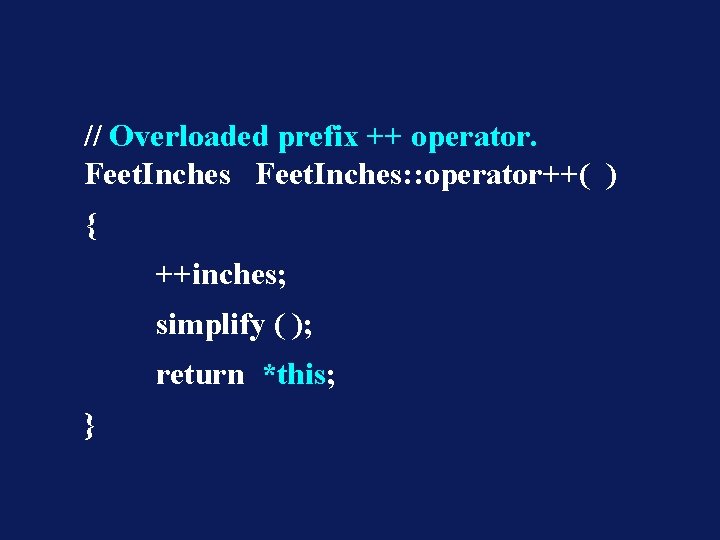
// Overloaded prefix ++ operator. Feet. Inches: : operator++( ) { ++inches; simplify ( ); return *this; }
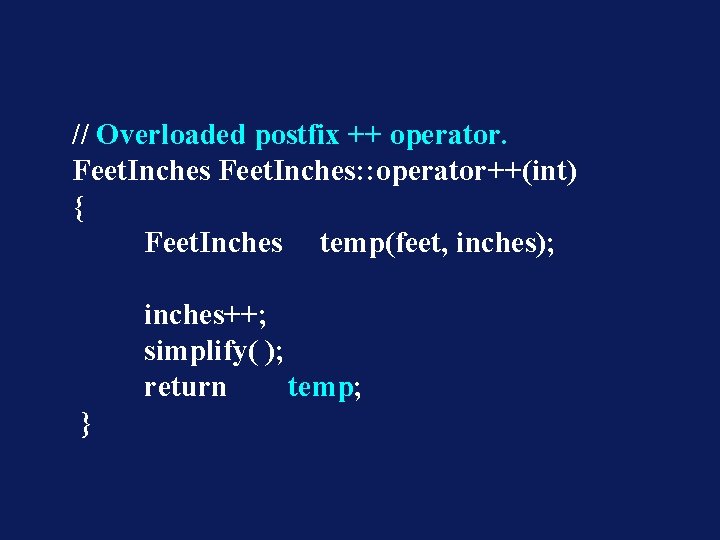
// Overloaded postfix ++ operator. Feet. Inches: : operator++(int) { Feet. Inches temp(feet, inches); inches++; simplify( ); return temp; }
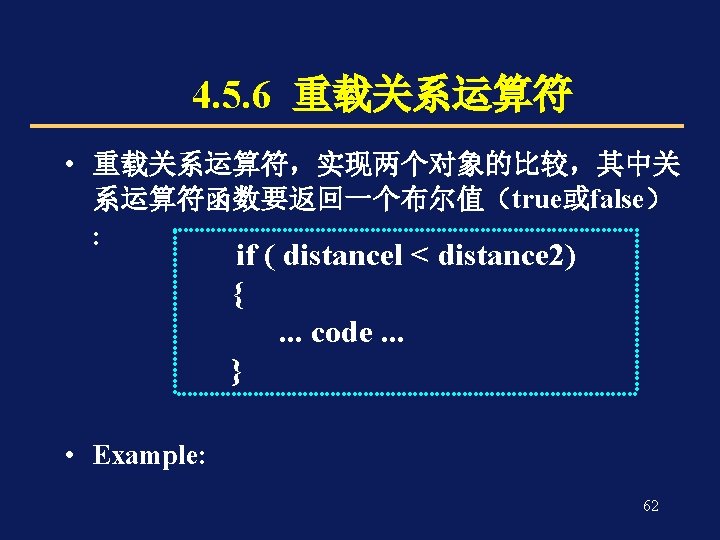
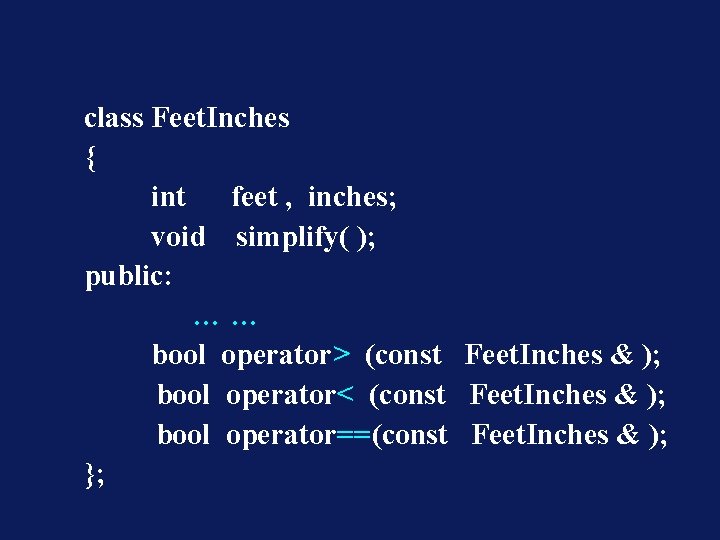
class Feet. Inches { int feet , inches; void simplify( ); public: …… bool operator> (const Feet. Inches & ); bool operator< (const Feet. Inches & ); bool operator==(const Feet. Inches & ); };
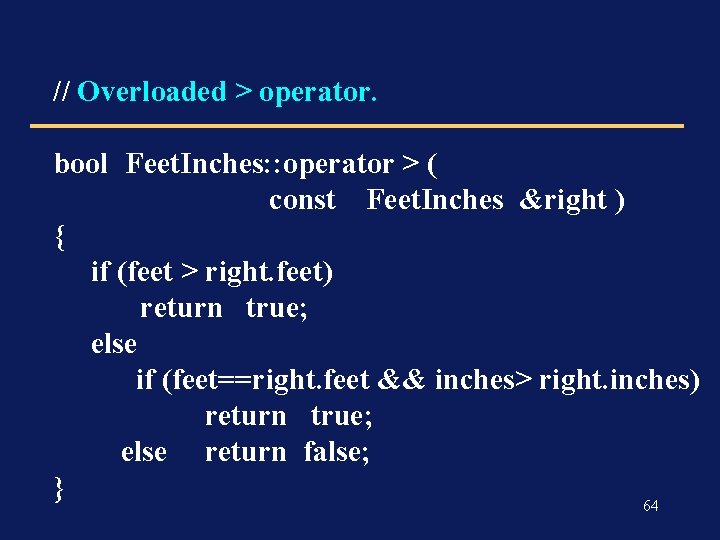
// Overloaded > operator. bool Feet. Inches: : operator > ( const Feet. Inches &right ) { if (feet > right. feet) return true; else if (feet==right. feet && inches> right. inches) return true; else return false; } 64
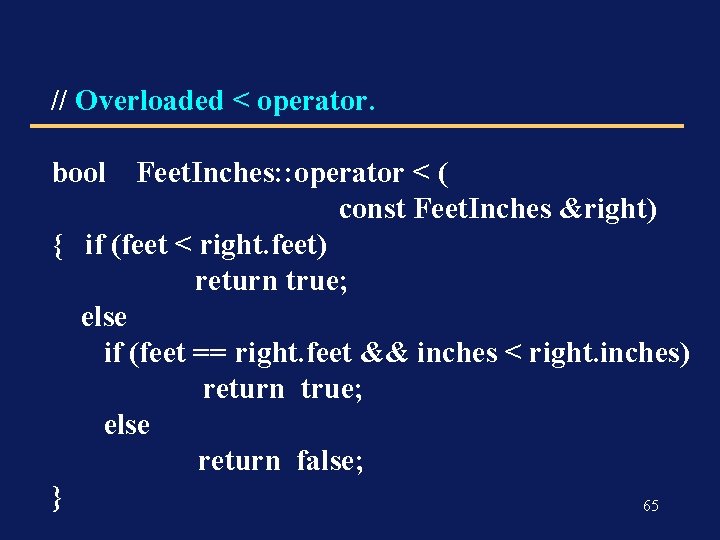
// Overloaded < operator. bool Feet. Inches: : operator < ( const Feet. Inches &right) { if (feet < right. feet) return true; else if (feet == right. feet && inches < right. inches) return true; else return false; } 65
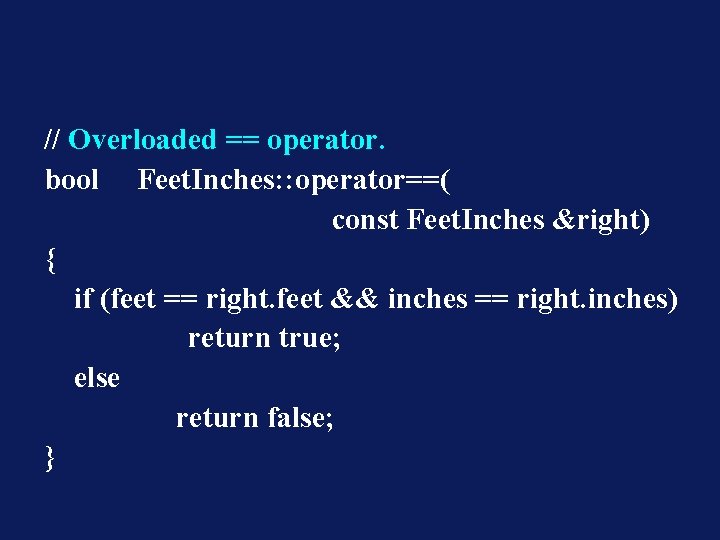
// Overloaded == operator. bool Feet. Inches: : operator==( const Feet. Inches &right) { if (feet == right. feet && inches == right. inches) return true; else return false; }
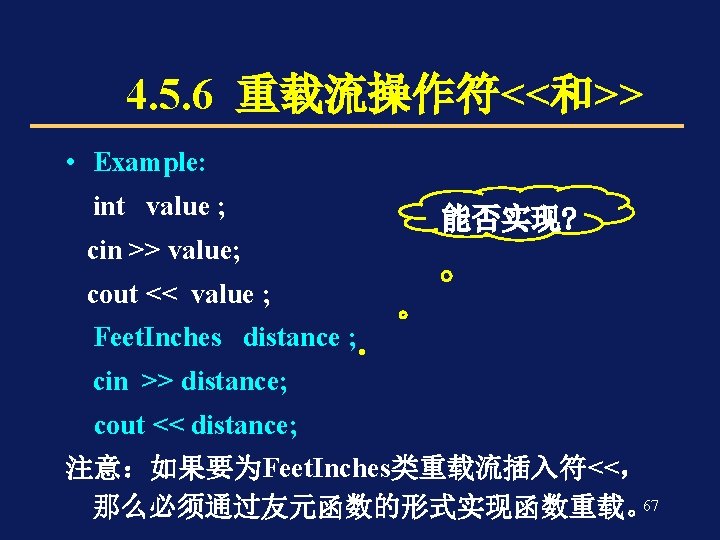
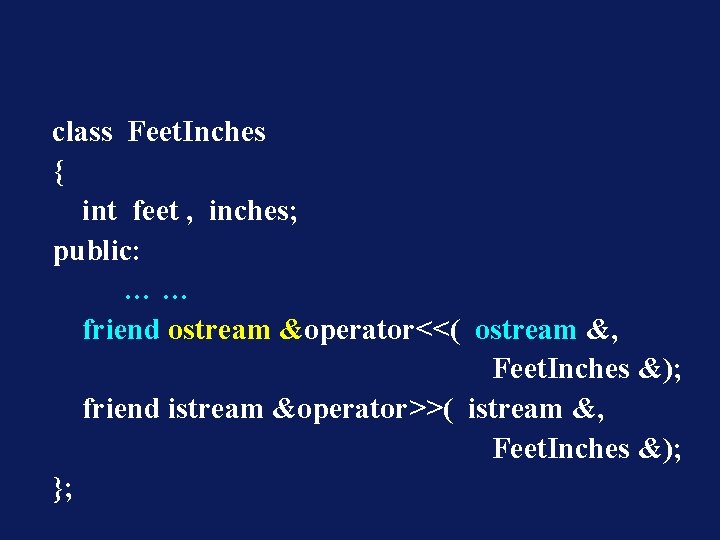
class Feet. Inches { int feet , inches; public: …… friend ostream &operator<<( ostream &, Feet. Inches &); friend istream &operator>>( istream &, Feet. Inches &); };
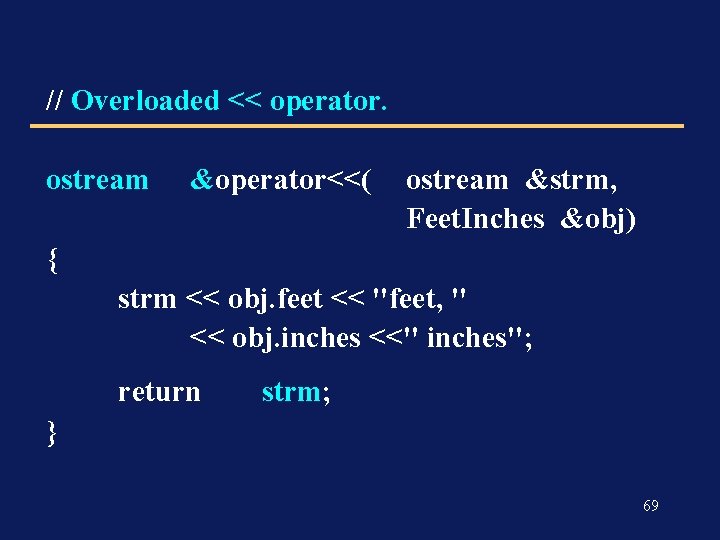
// Overloaded << operator. ostream &operator<<( ostream &strm, Feet. Inches &obj) { strm << obj. feet << "feet, " << obj. inches <<" inches"; return strm; } 69
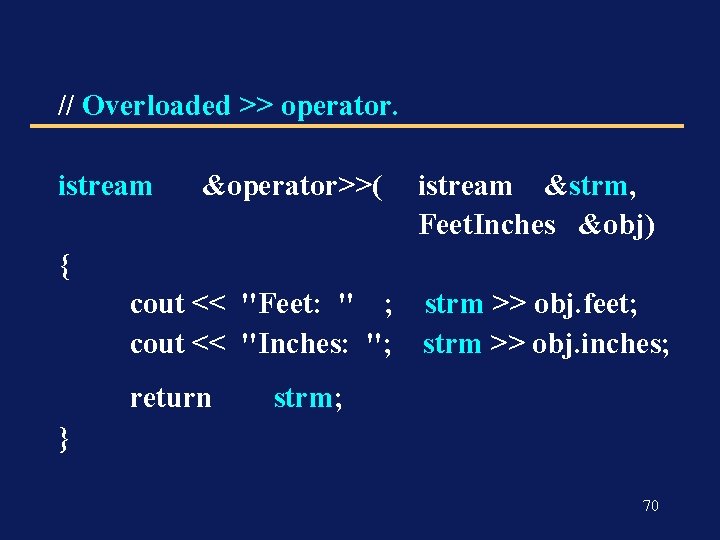
// Overloaded >> operator. istream &operator>>( istream &strm, Feet. Inches &obj) { cout << "Feet: " ; strm >> obj. feet; cout << "Inches: "; strm >> obj. inches; return strm; } 70
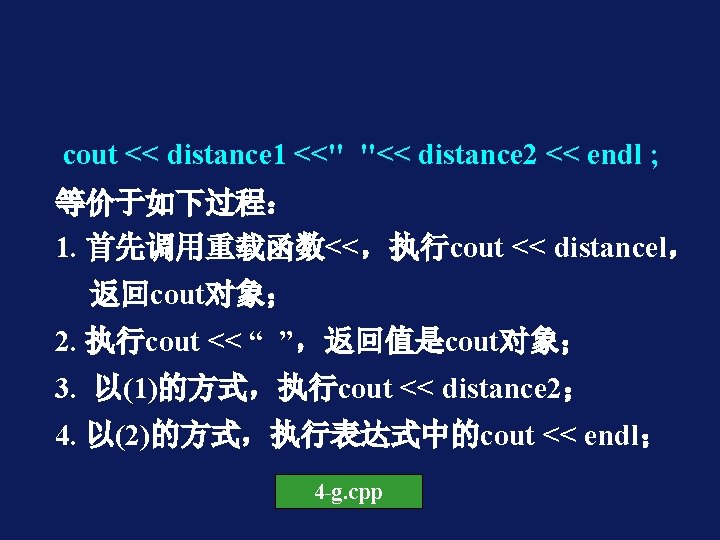
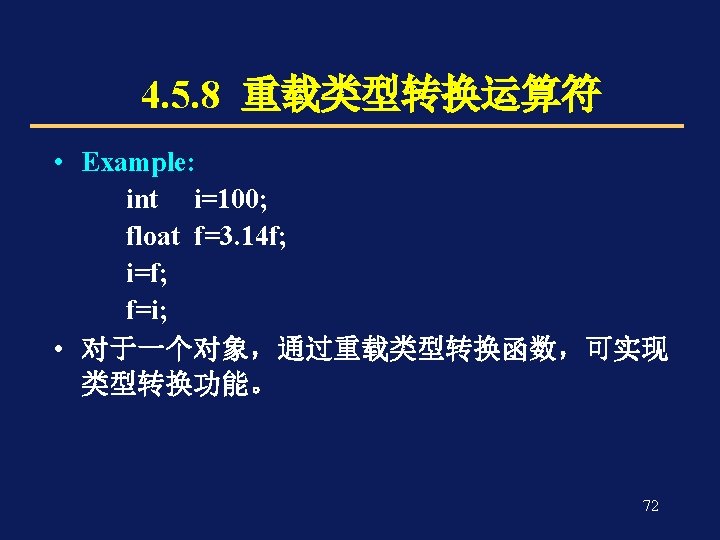
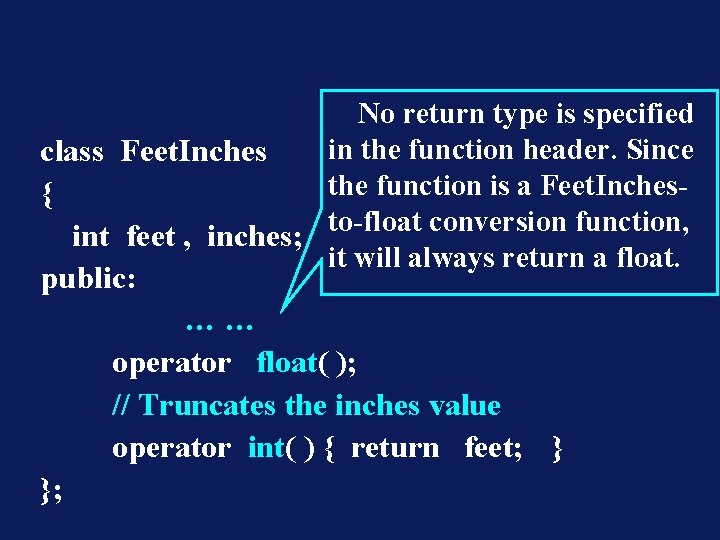
No return type is specified in the function header. Since class Feet. Inches the function is a Feet. Inches{ to-float conversion function, int feet , inches; it will always return a float. public: …… operator float( ); // Truncates the inches value operator int( ) { return feet; } };
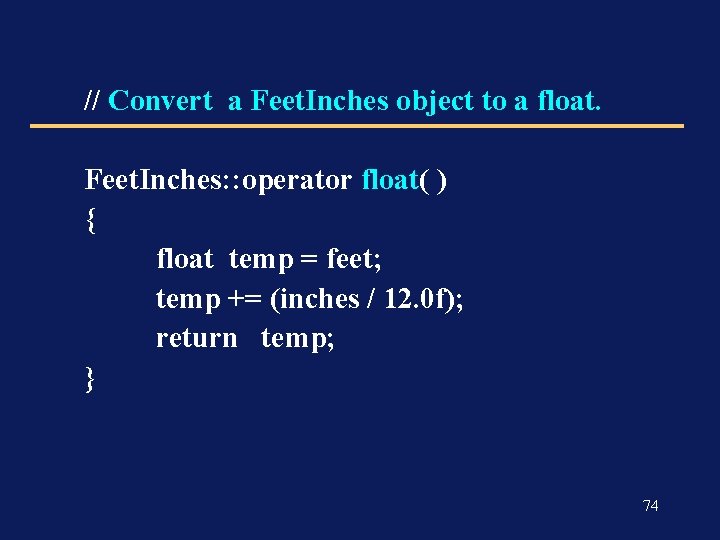
// Convert a Feet. Inches object to a float. Feet. Inches: : operator float( ) { float temp = feet; temp += (inches / 12. 0 f); return temp; } 74
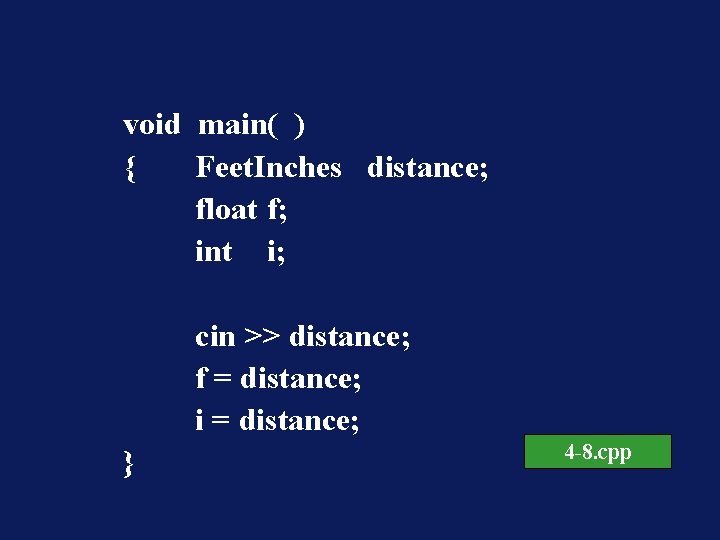
void main( ) { Feet. Inches distance; float f; int i; cin >> distance; f = distance; i = distance; } 4 -8. cpp
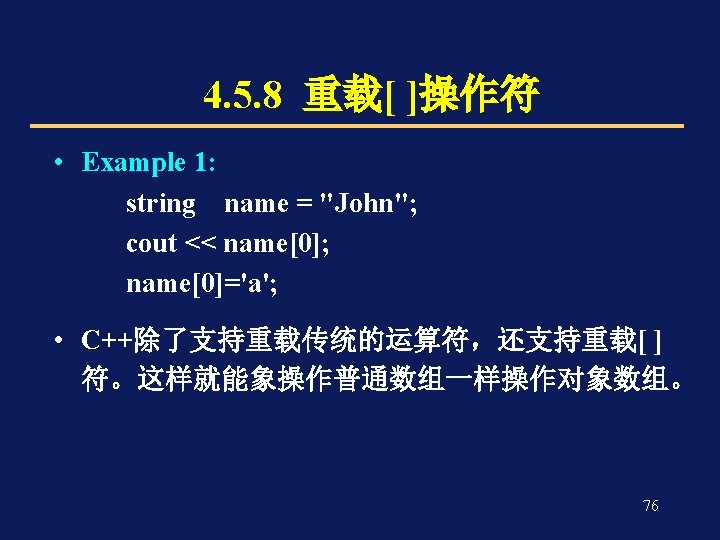
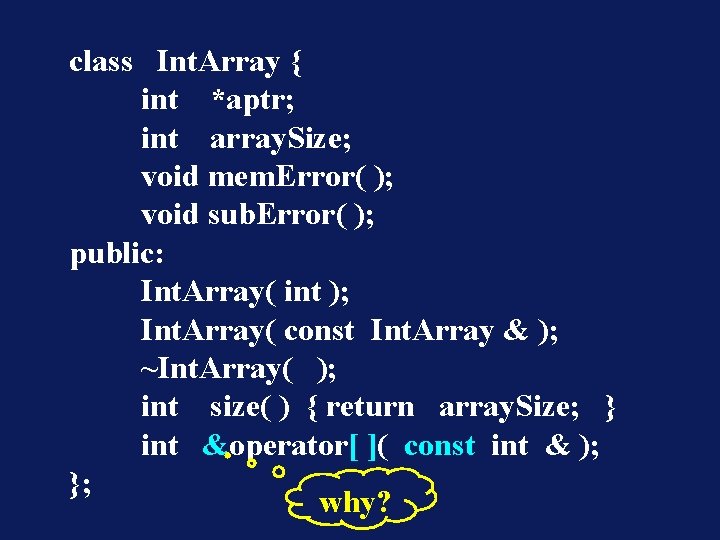
class Int. Array { int *aptr; int array. Size; void mem. Error( ); void sub. Error( ); public: Int. Array( int ); Int. Array( const Int. Array & ); ~Int. Array( ); int size( ) { return array. Size; } int &operator[ ]( const int & ); }; why?
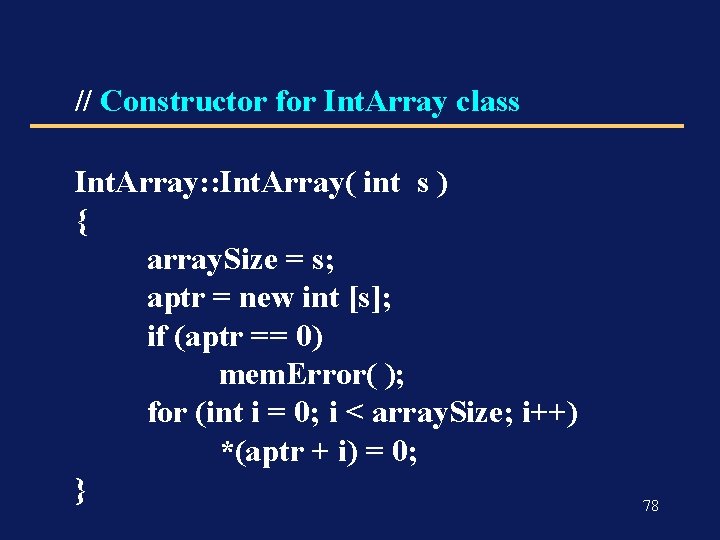
// Constructor for Int. Array class Int. Array: : Int. Array( int s ) { array. Size = s; aptr = new int [s]; if (aptr == 0) mem. Error( ); for (int i = 0; i < array. Size; i++) *(aptr + i) = 0; } 78
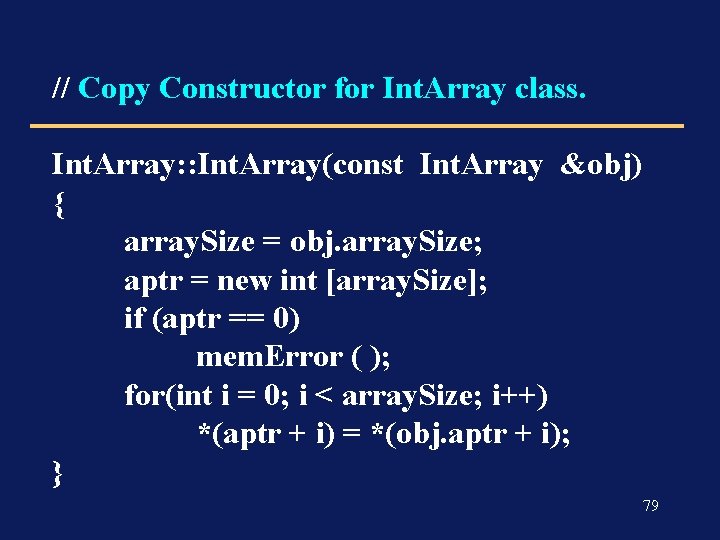
// Copy Constructor for Int. Array class. Int. Array: : Int. Array(const Int. Array &obj) { array. Size = obj. array. Size; aptr = new int [array. Size]; if (aptr == 0) mem. Error ( ); for(int i = 0; i < array. Size; i++) *(aptr + i) = *(obj. aptr + i); } 79
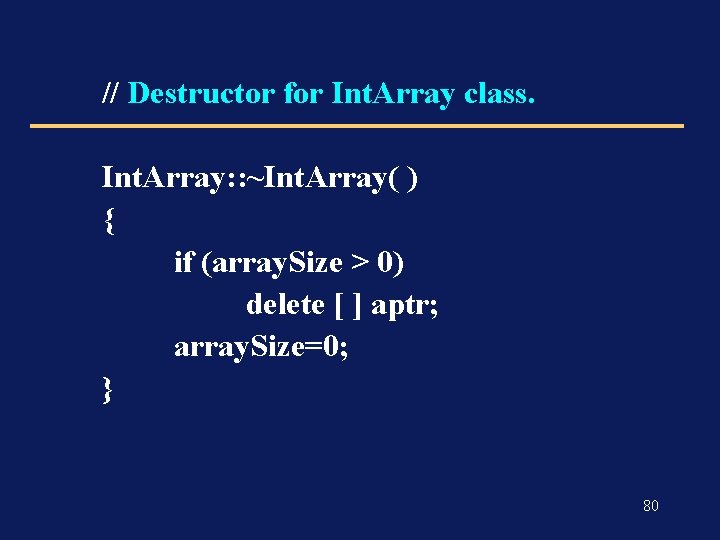
// Destructor for Int. Array class. Int. Array: : ~Int. Array( ) { if (array. Size > 0) delete [ ] aptr; array. Size=0; } 80
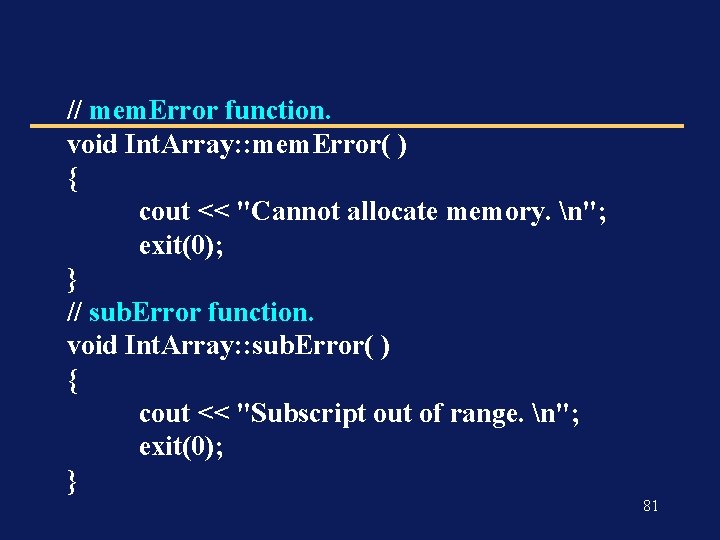
// mem. Error function. void Int. Array: : mem. Error( ) { cout << "Cannot allocate memory. n"; exit(0); } // sub. Error function. void Int. Array: : sub. Error( ) { cout << "Subscript out of range. n"; exit(0); } 81
![Overloaded operator int Int Array operator const int // Overloaded [ ] operator. int & Int. Array: : operator[ ] (const int](https://slidetodoc.com/presentation_image_h2/3116b27f6d5681323f4e291b9b95a294/image-82.jpg)
// Overloaded [ ] operator. int & Int. Array: : operator[ ] (const int &sub) { if ( sub<0 || sub >= array. Size) sub. Error( ); return aptr[sub]; } 82
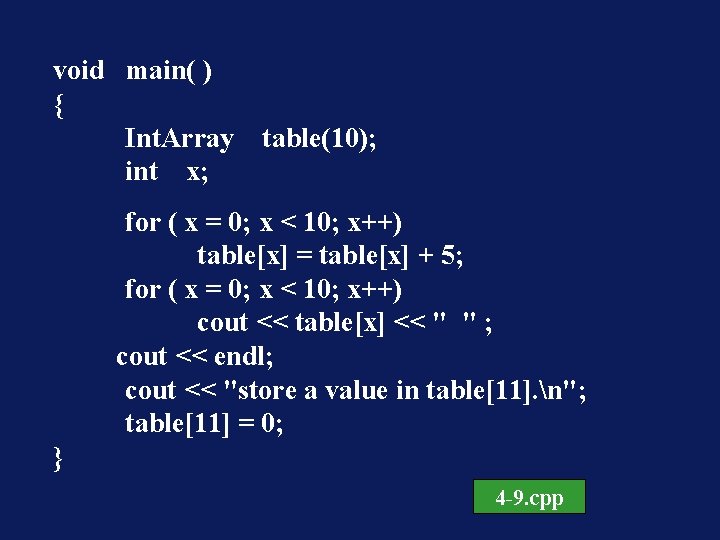
void main( ) { Int. Array int x; table(10); for ( x = 0; x < 10; x++) table[x] = table[x] + 5; for ( x = 0; x < 10; x++) cout << table[x] << " " ; cout << endl; cout << "store a value in table[11]. n"; table[11] = 0; } 4 -9. cpp
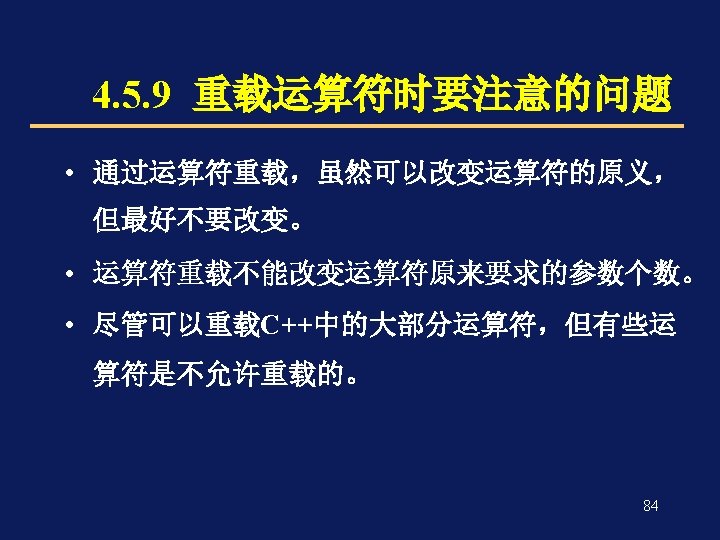
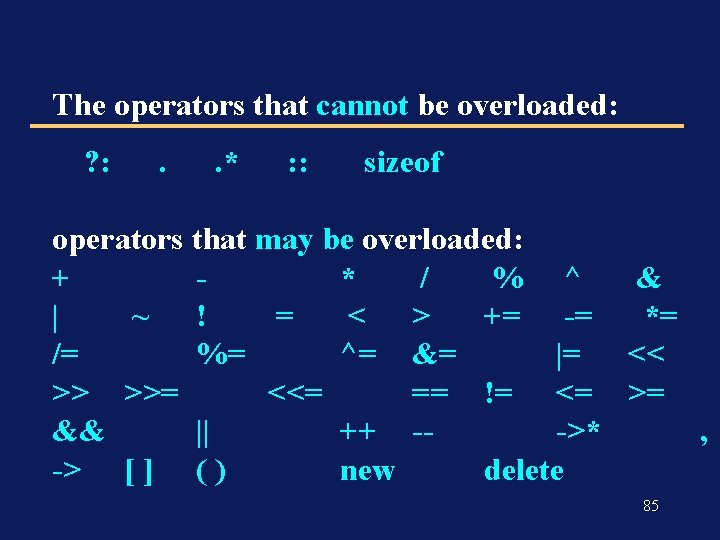
The operators that cannot be overloaded: ? : . . * : : sizeof operators that may be overloaded: + * / % ^ & | ~ ! = < > += -= *= /= %= ^= &= |= << >> >>= <<= == != <= >= && || ++ -->* , -> [ ] ( ) new delete 85
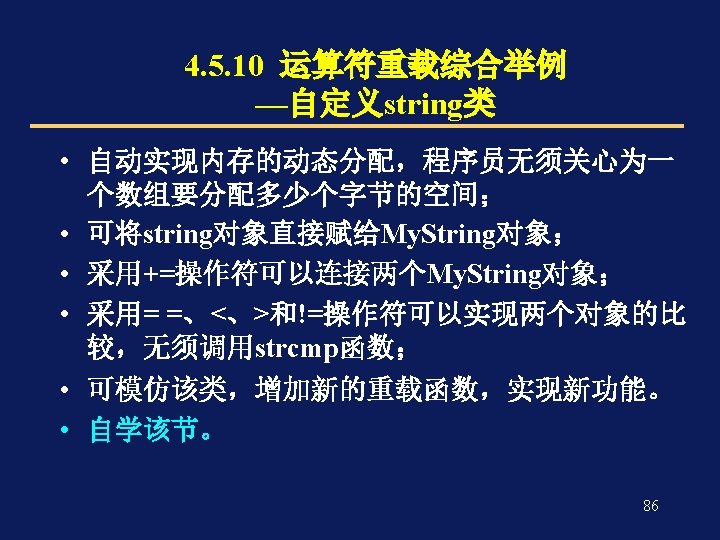
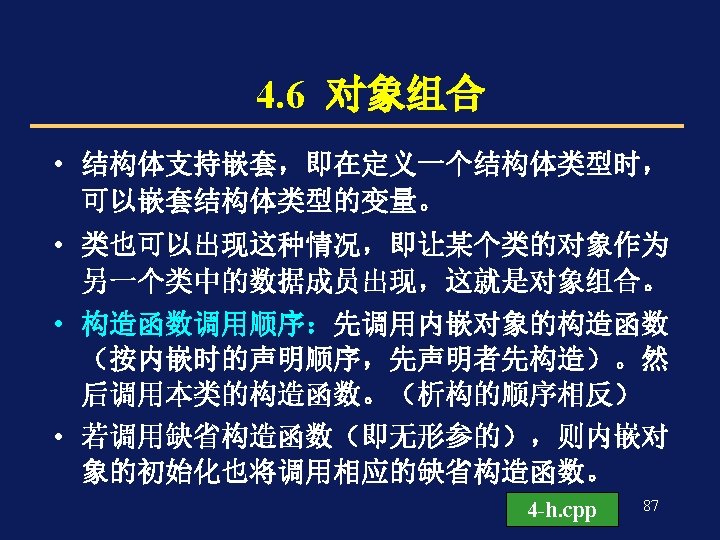
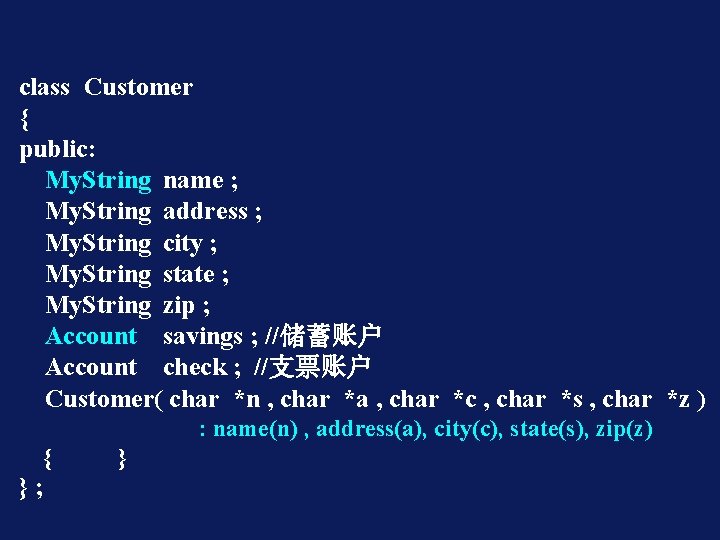
class Customer { public: My. String name ; My. String address ; My. String city ; My. String state ; My. String zip ; Account savings ; //储蓄账户 Account check ; //支票账户 Customer( char *n , char *a , char *c , char *s , char *z ) : name(n) , address(a), city(c), state(s), zip(z) { }; }
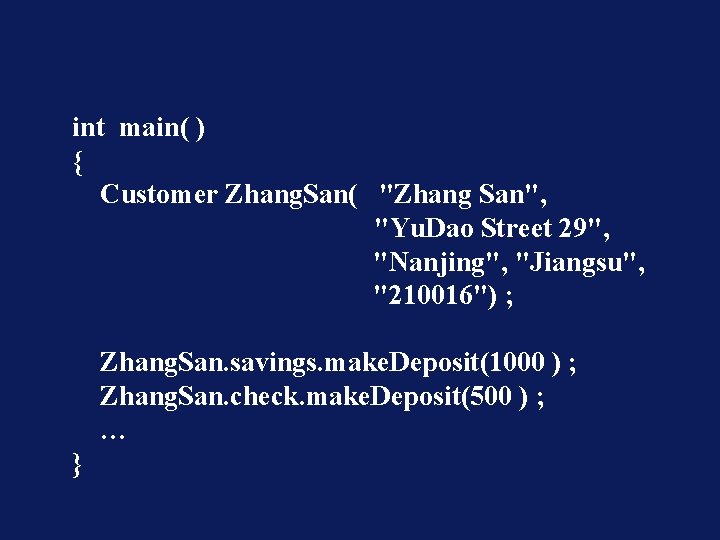
int main( ) { Customer Zhang. San( "Zhang San", "Yu. Dao Street 29", "Nanjing", "Jiangsu", "210016") ; Zhang. San. savings. make. Deposit(1000 ) ; Zhang. San. check. make. Deposit(500 ) ; … }