Stacks 1 Stacks l l A stack has
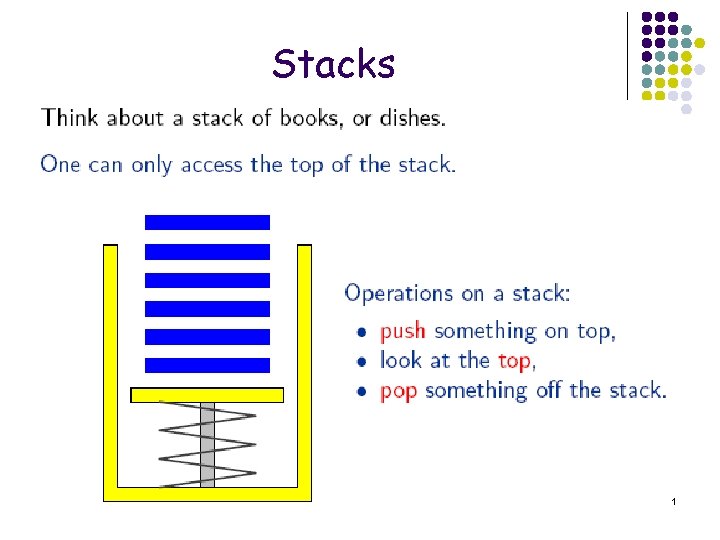
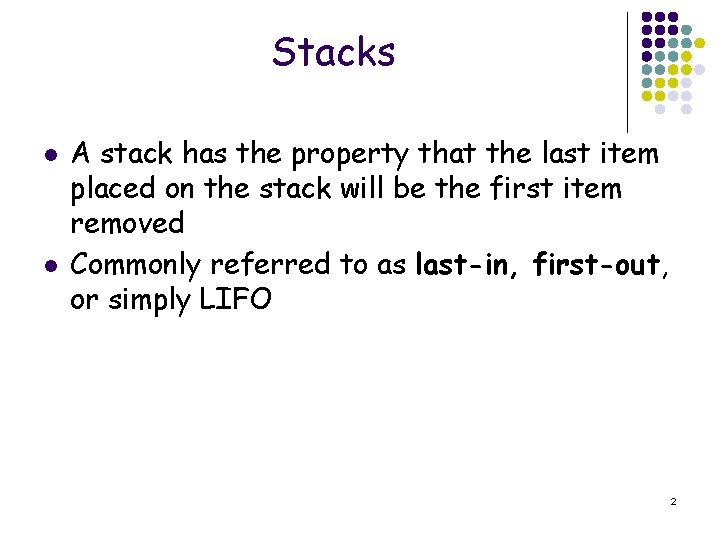
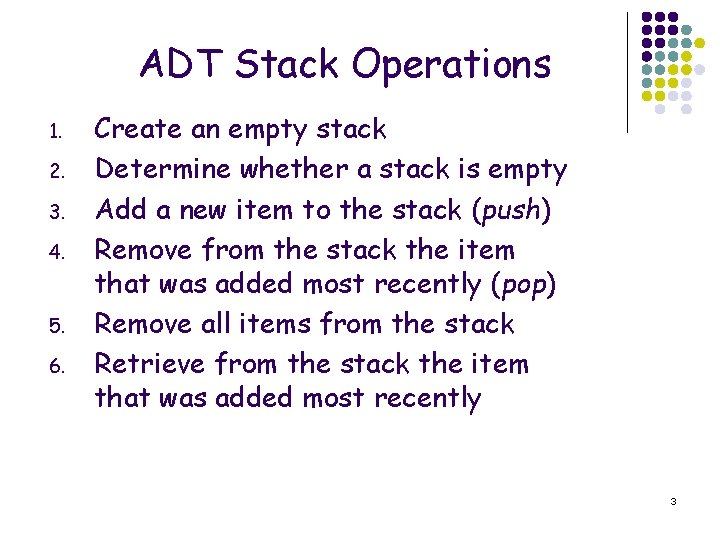
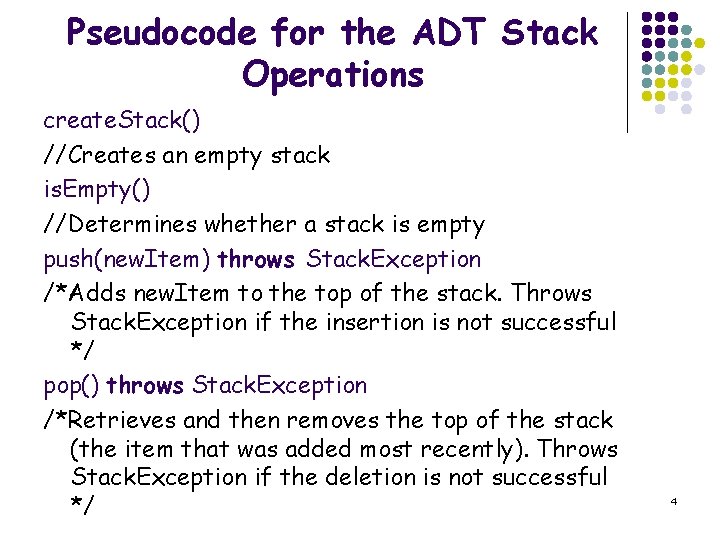
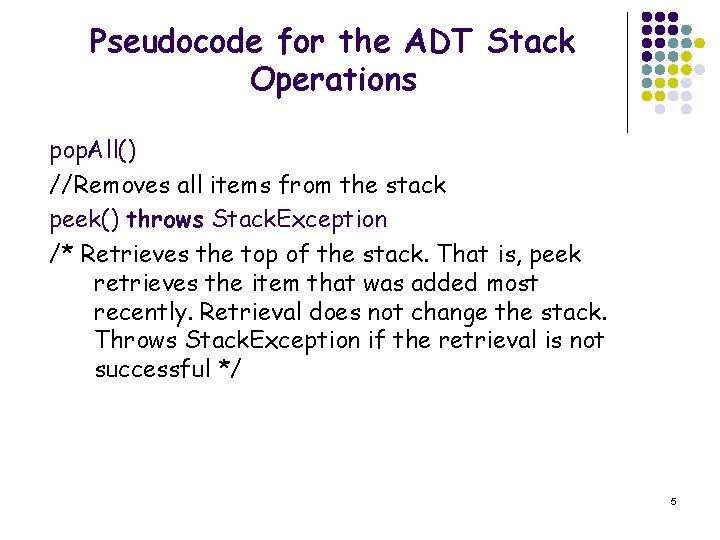
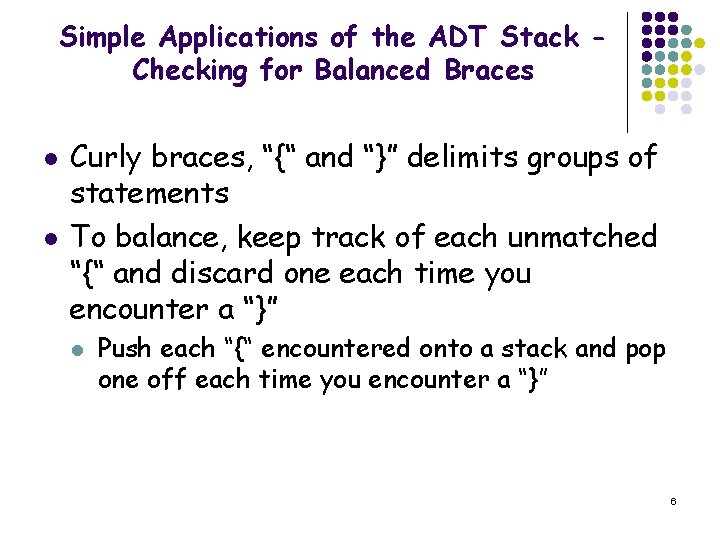
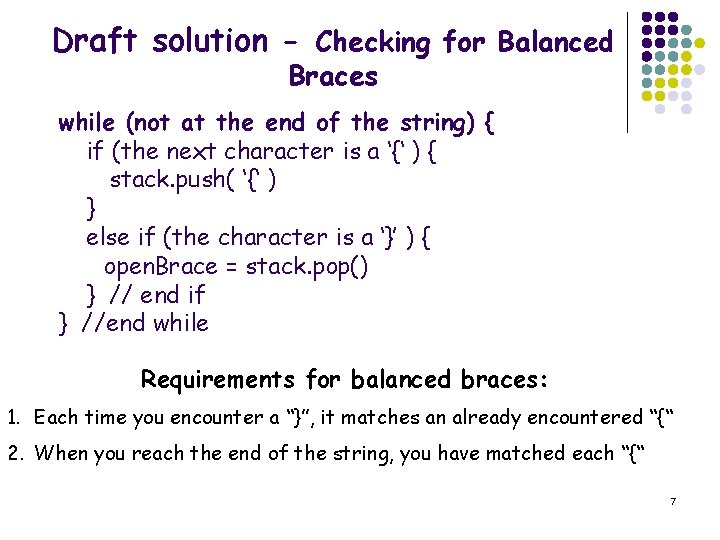
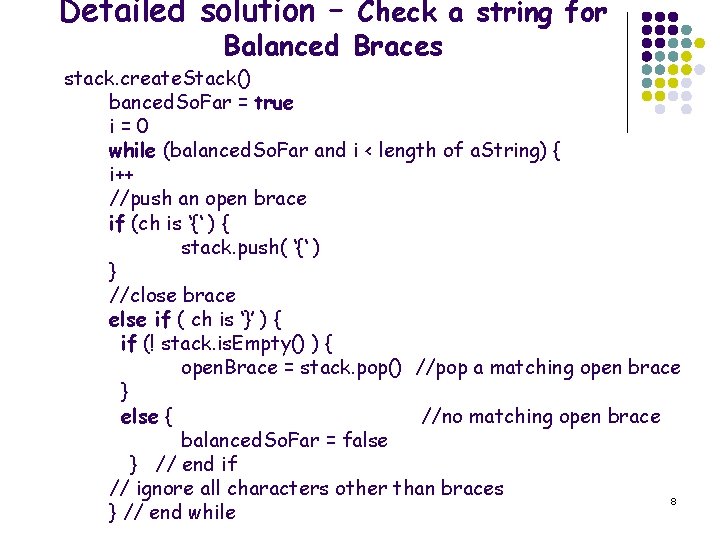
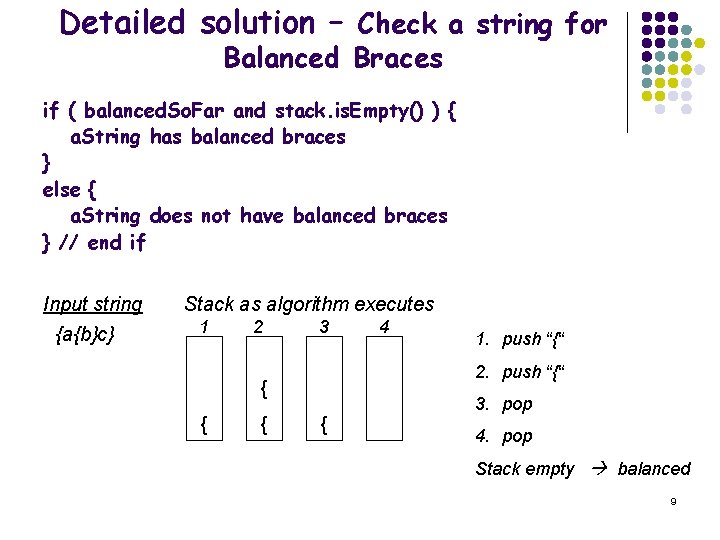
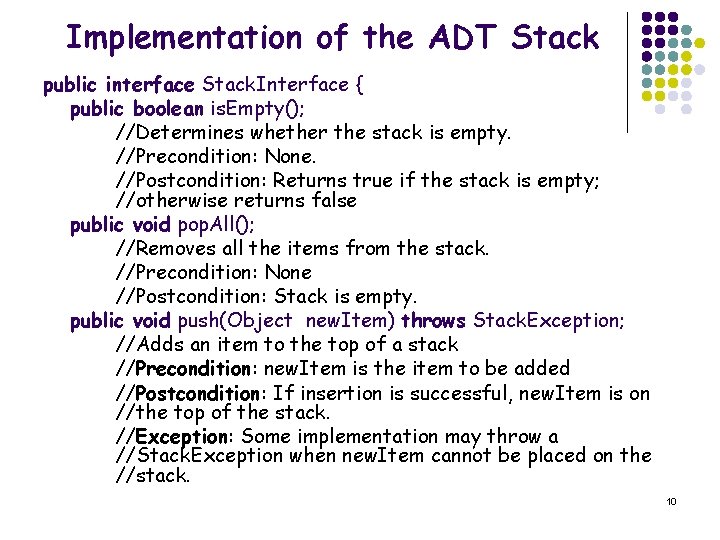
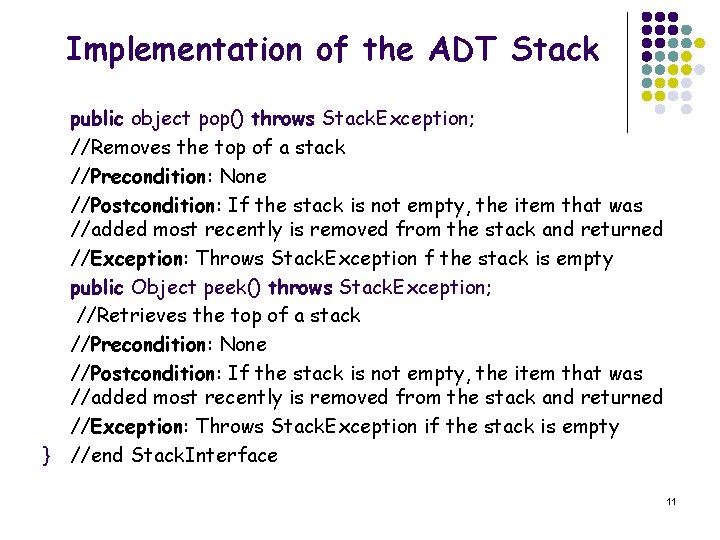
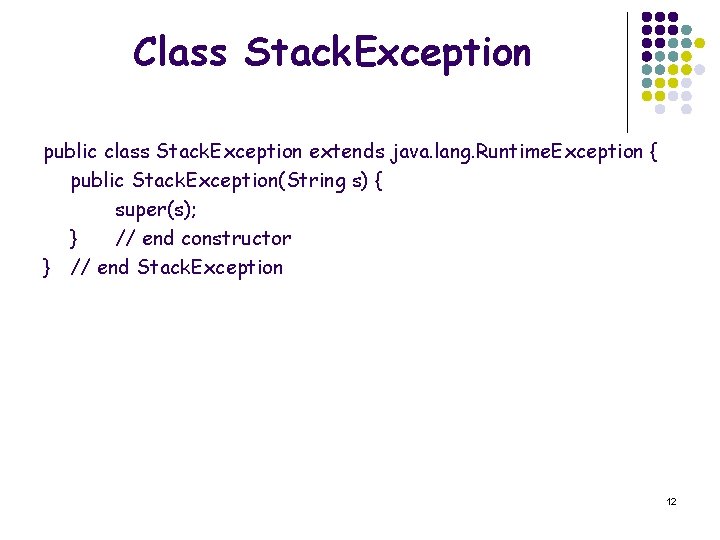
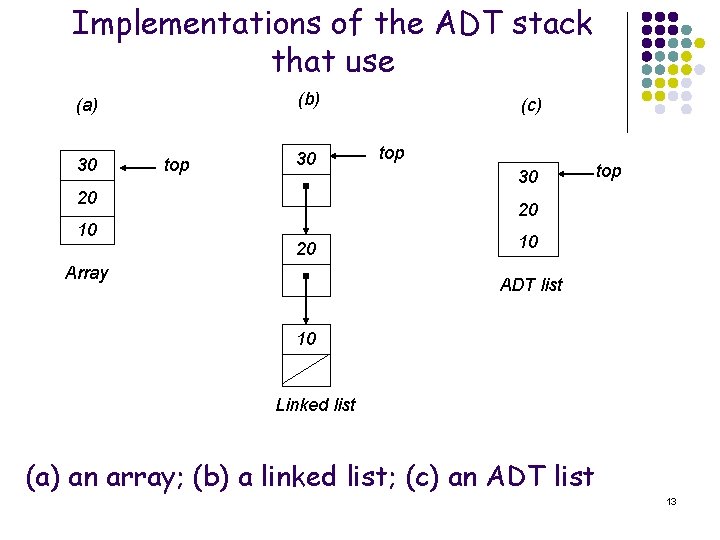
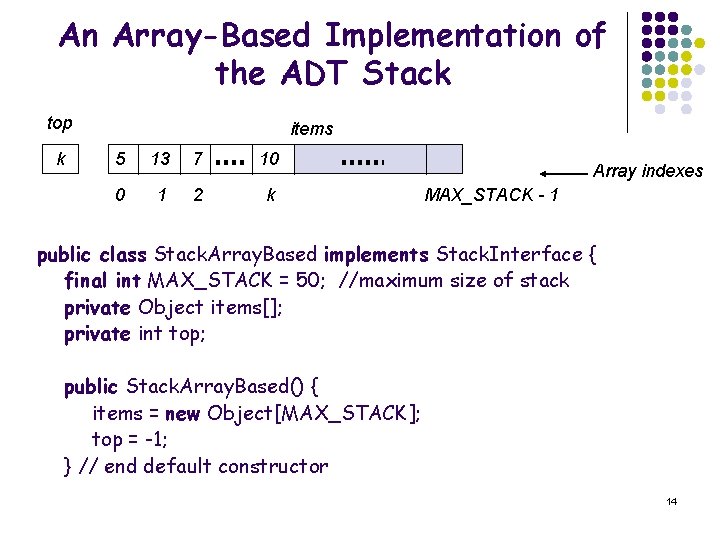
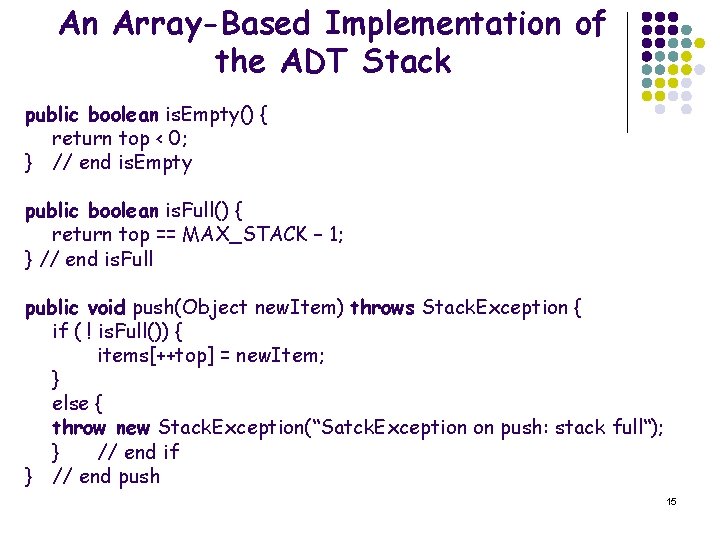
![public void pop. All() { items = new Object[MAX_STACK]; top = -1; } // public void pop. All() { items = new Object[MAX_STACK]; top = -1; } //](https://slidetodoc.com/presentation_image_h2/b32067e296dd225191404604150d4b9c/image-16.jpg)
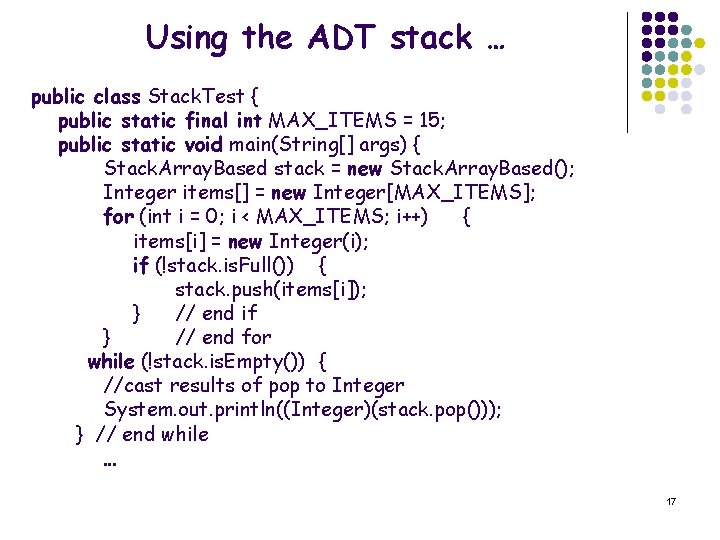
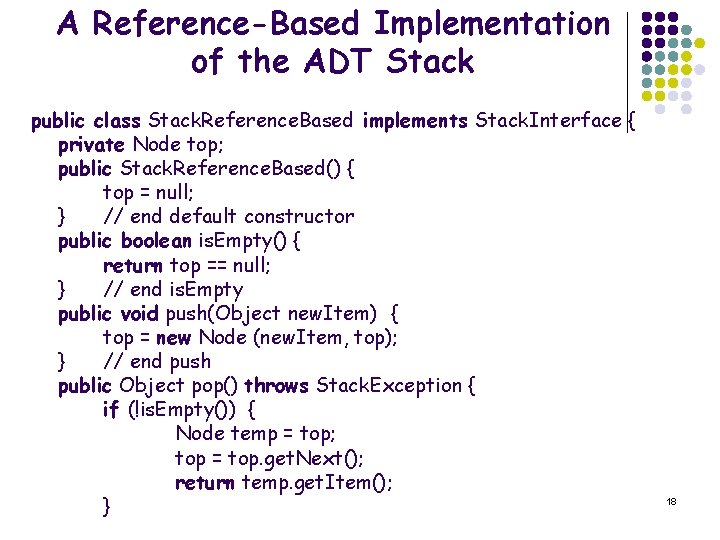
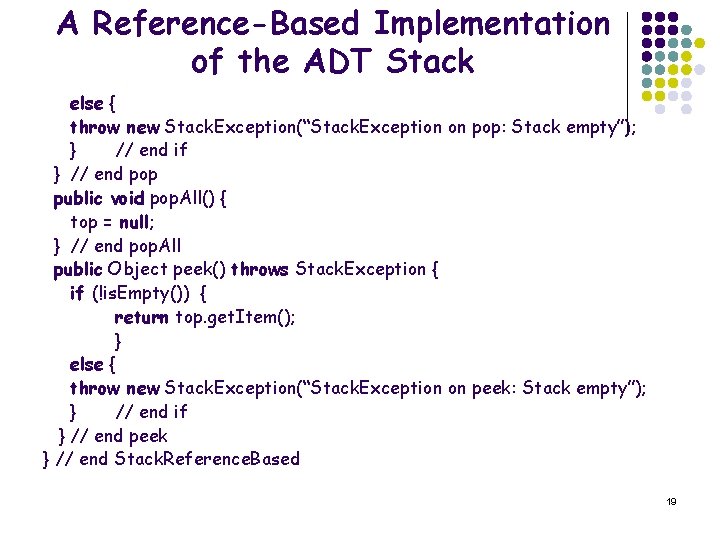
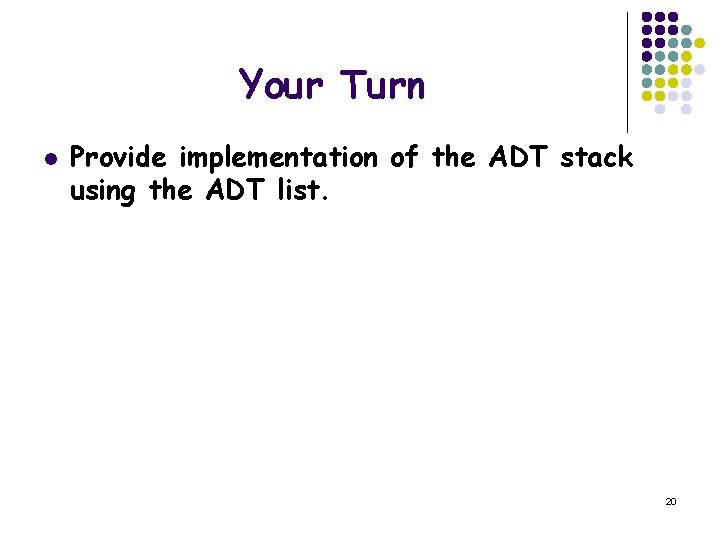
- Slides: 20
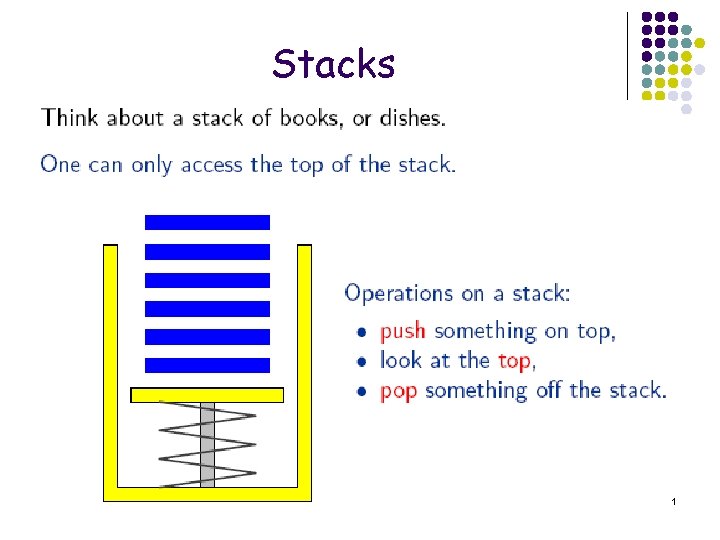
Stacks 1
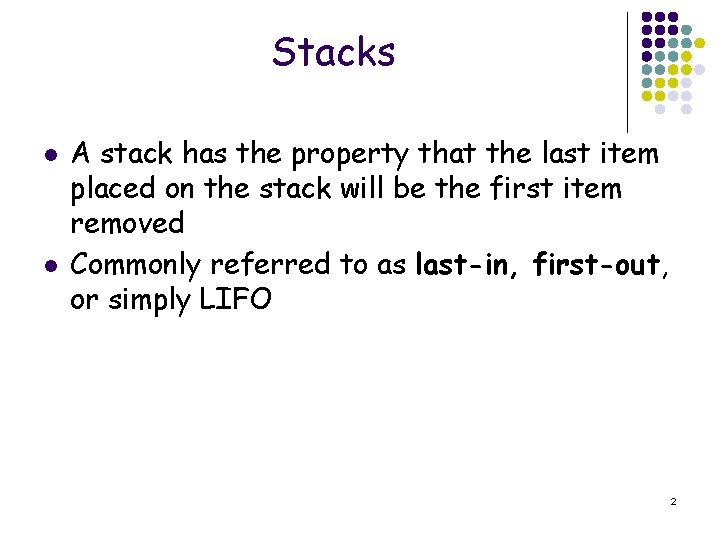
Stacks l l A stack has the property that the last item placed on the stack will be the first item removed Commonly referred to as last-in, first-out, or simply LIFO 2
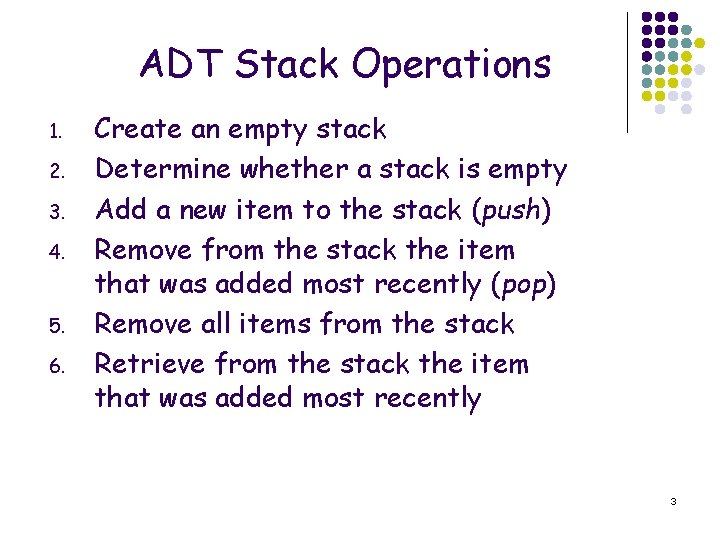
ADT Stack Operations 1. 2. 3. 4. 5. 6. Create an empty stack Determine whether a stack is empty Add a new item to the stack (push) Remove from the stack the item that was added most recently (pop) Remove all items from the stack Retrieve from the stack the item that was added most recently 3
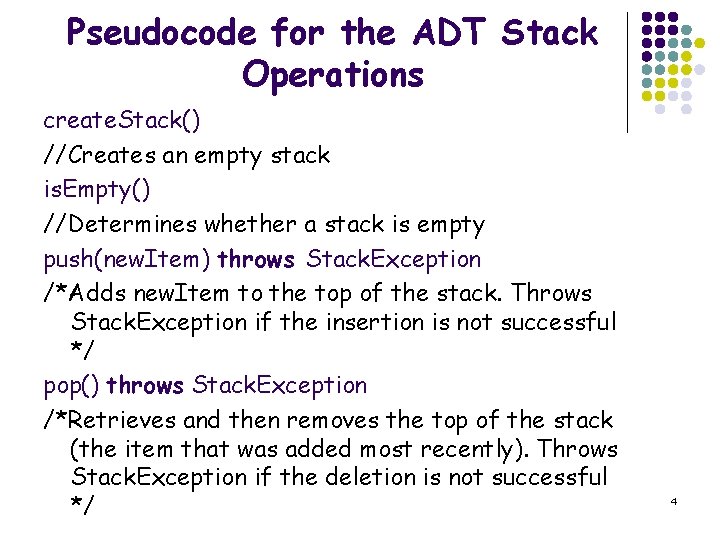
Pseudocode for the ADT Stack Operations create. Stack() //Creates an empty stack is. Empty() //Determines whether a stack is empty push(new. Item) throws Stack. Exception /*Adds new. Item to the top of the stack. Throws Stack. Exception if the insertion is not successful */ pop() throws Stack. Exception /*Retrieves and then removes the top of the stack (the item that was added most recently). Throws Stack. Exception if the deletion is not successful */ 4
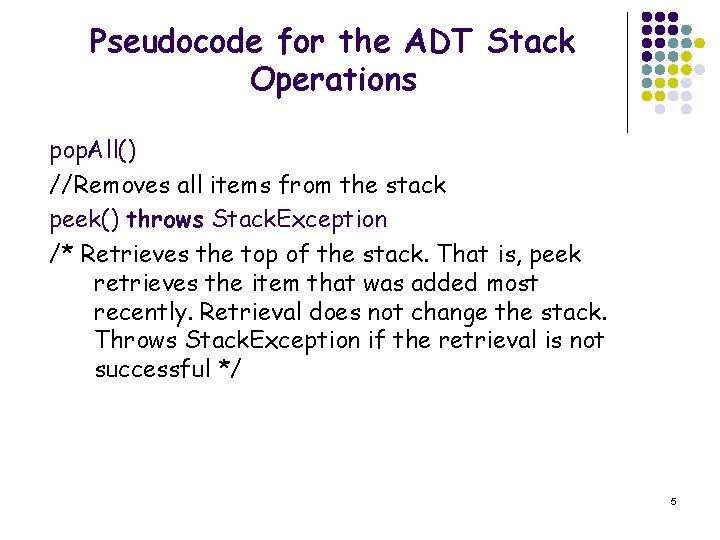
Pseudocode for the ADT Stack Operations pop. All() //Removes all items from the stack peek() throws Stack. Exception /* Retrieves the top of the stack. That is, peek retrieves the item that was added most recently. Retrieval does not change the stack. Throws Stack. Exception if the retrieval is not successful */ 5
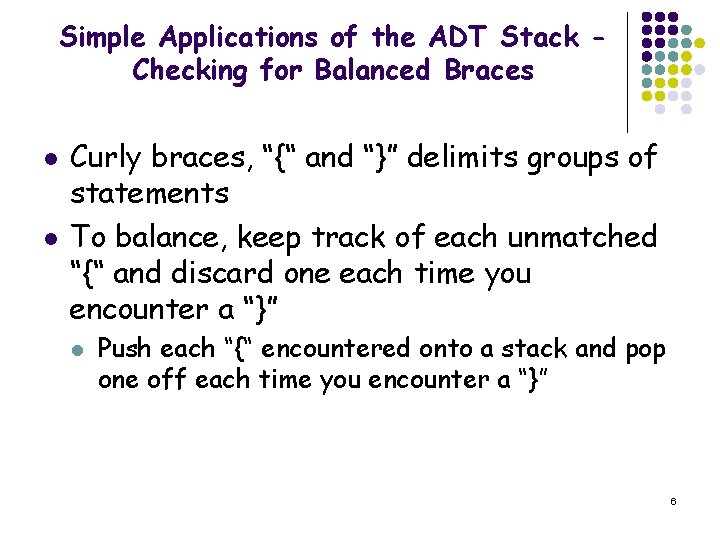
Simple Applications of the ADT Stack Checking for Balanced Braces l l Curly braces, “{“ and “}” delimits groups of statements To balance, keep track of each unmatched “{“ and discard one each time you encounter a “}” l Push each “{“ encountered onto a stack and pop one off each time you encounter a “}” 6
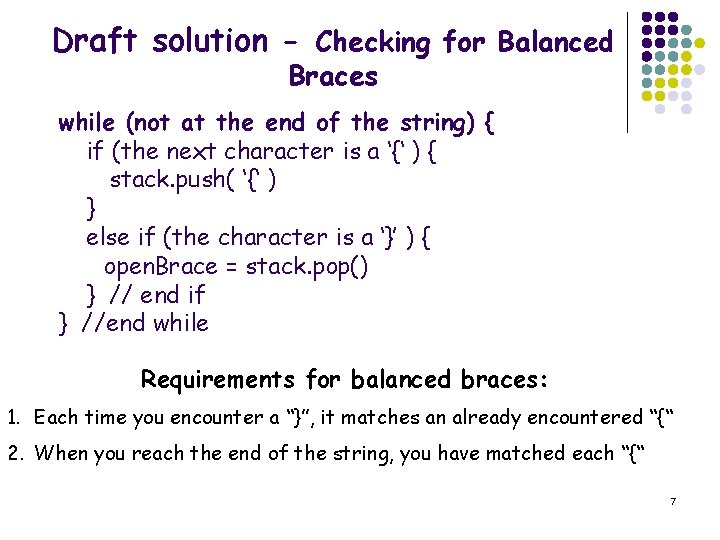
Draft solution - Checking for Balanced Braces while (not at the end of the string) { if (the next character is a ‘{‘ ) { stack. push( ‘{‘ ) } else if (the character is a ‘}’ ) { open. Brace = stack. pop() } // end if } //end while Requirements for balanced braces: 1. Each time you encounter a “}”, it matches an already encountered “{“ 2. When you reach the end of the string, you have matched each “{“ 7
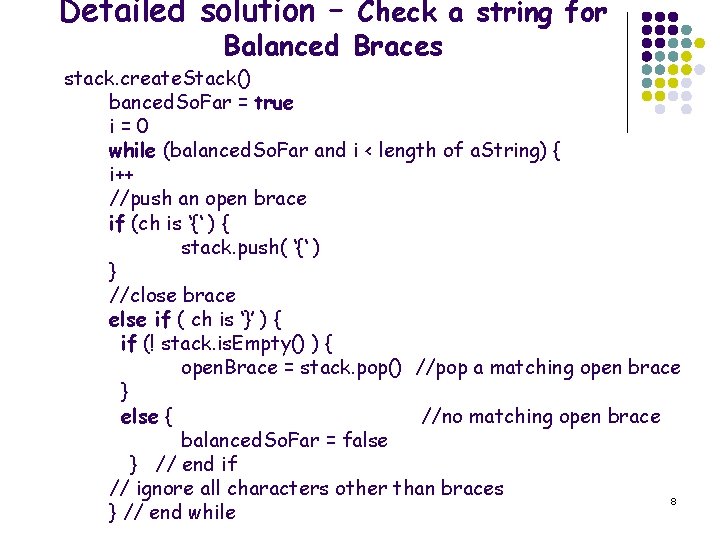
Detailed solution – Check a string for Balanced Braces stack. create. Stack() banced. So. Far = true i=0 while (balanced. So. Far and i < length of a. String) { i++ //push an open brace if (ch is ‘{‘ ) { stack. push( ‘{‘ ) } //close brace else if ( ch is ‘}’ ) { if (! stack. is. Empty() ) { open. Brace = stack. pop() //pop a matching open brace } else { //no matching open brace balanced. So. Far = false } // end if // ignore all characters other than braces 8 } // end while
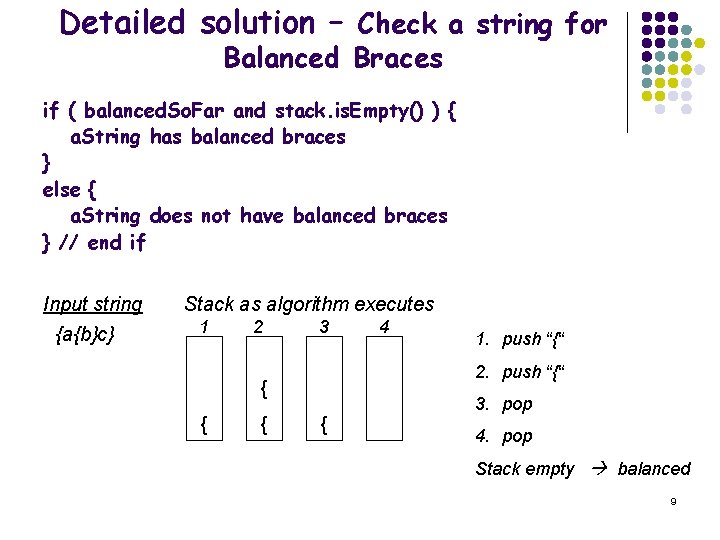
Detailed solution – Check a string for Balanced Braces if ( balanced. So. Far and stack. is. Empty() ) { a. String has balanced braces } else { a. String does not have balanced braces } // end if Input string {a{b}c} Stack as algorithm executes 1 2 3 { 1. push “{“ 2. push “{“ { { 4 { 3. pop 4. pop Stack empty balanced 9
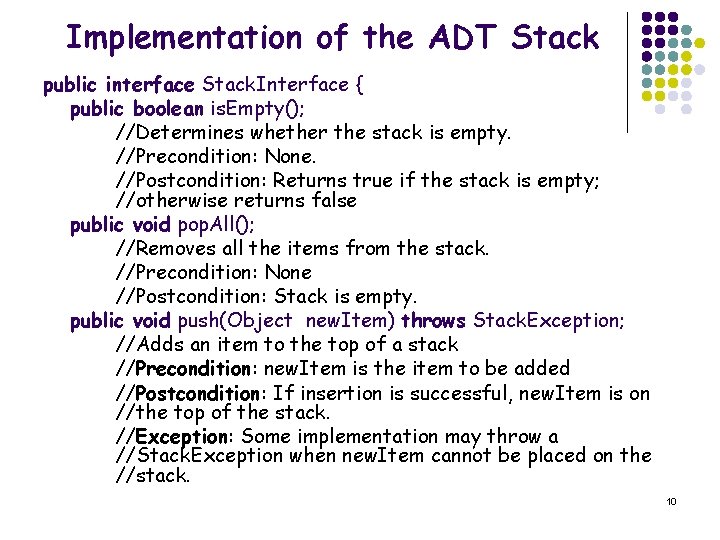
Implementation of the ADT Stack public interface Stack. Interface { public boolean is. Empty(); //Determines whether the stack is empty. //Precondition: None. //Postcondition: Returns true if the stack is empty; //otherwise returns false public void pop. All(); //Removes all the items from the stack. //Precondition: None //Postcondition: Stack is empty. public void push(Object new. Item) throws Stack. Exception; //Adds an item to the top of a stack //Precondition: new. Item is the item to be added //Postcondition: If insertion is successful, new. Item is on //the top of the stack. //Exception: Some implementation may throw a //Stack. Exception when new. Item cannot be placed on the //stack. 10
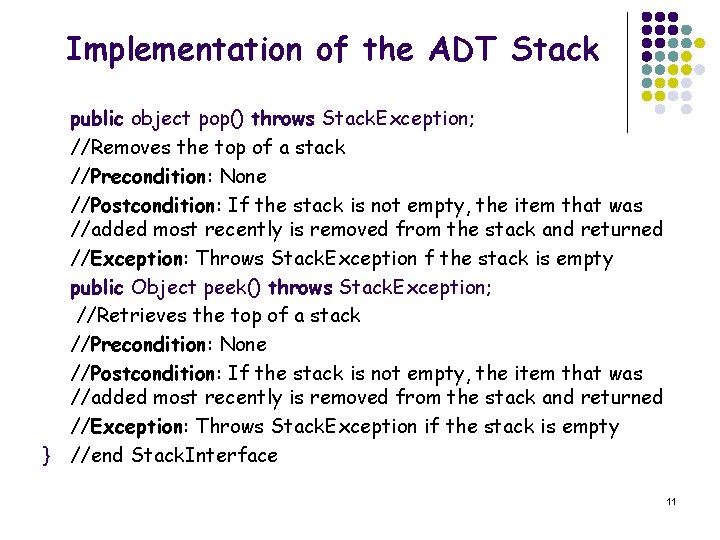
Implementation of the ADT Stack public object pop() throws Stack. Exception; //Removes the top of a stack //Precondition: None //Postcondition: If the stack is not empty, the item that was //added most recently is removed from the stack and returned //Exception: Throws Stack. Exception f the stack is empty public Object peek() throws Stack. Exception; //Retrieves the top of a stack //Precondition: None //Postcondition: If the stack is not empty, the item that was //added most recently is removed from the stack and returned //Exception: Throws Stack. Exception if the stack is empty } //end Stack. Interface 11
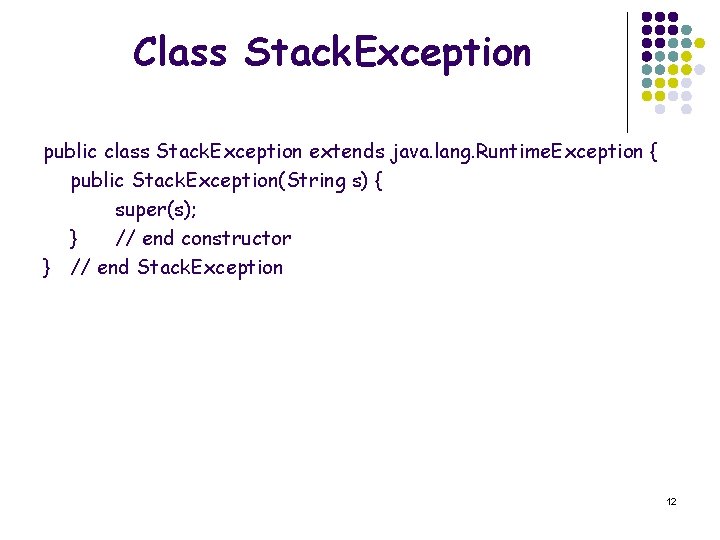
Class Stack. Exception public class Stack. Exception extends java. lang. Runtime. Exception { public Stack. Exception(String s) { super(s); } // end constructor } // end Stack. Exception 12
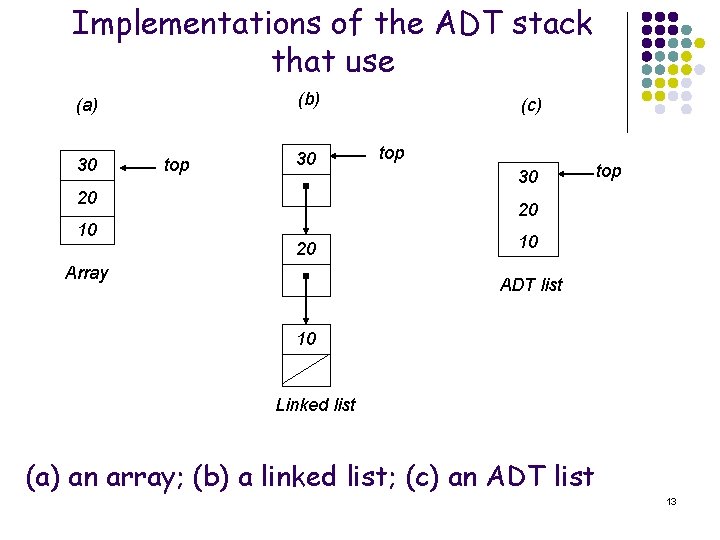
Implementations of the ADT stack that use (b) (a) 30 top 30 20 10 (c) top 30 top 20 20 Array 10 ADT list 10 Linked list (a) an array; (b) a linked list; (c) an ADT list 13
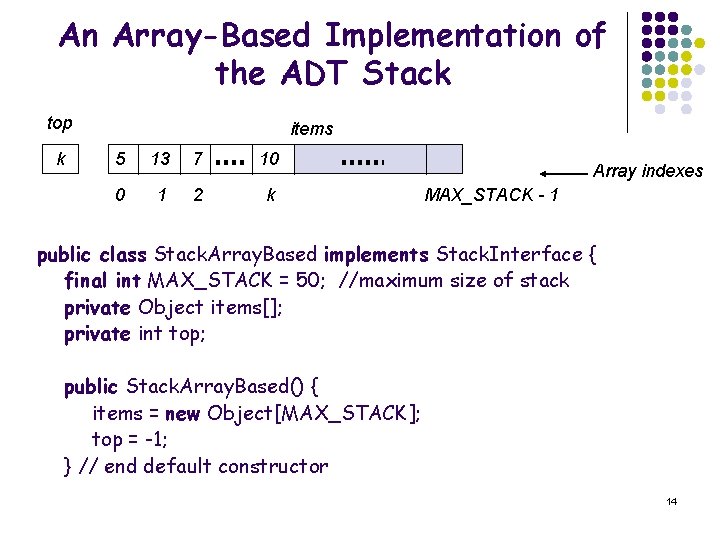
An Array-Based Implementation of the ADT Stack top k items 5 13 7 10 0 1 2 k … Array indexes MAX_STACK - 1 public class Stack. Array. Based implements Stack. Interface { final int MAX_STACK = 50; //maximum size of stack private Object items[]; private int top; public Stack. Array. Based() { items = new Object[MAX_STACK]; top = -1; } // end default constructor 14
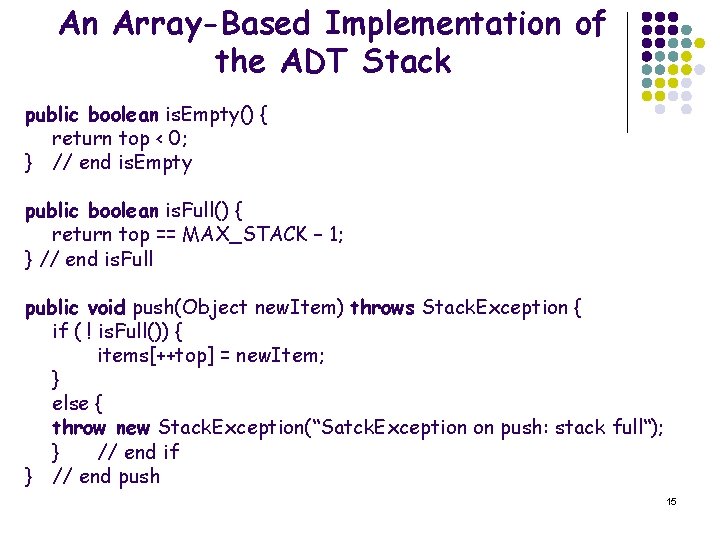
An Array-Based Implementation of the ADT Stack public boolean is. Empty() { return top < 0; } // end is. Empty public boolean is. Full() { return top == MAX_STACK – 1; } // end is. Full public void push(Object new. Item) throws Stack. Exception { if ( ! is. Full()) { items[++top] = new. Item; } else { throw new Stack. Exception(“Satck. Exception on push: stack full“); } // end if } // end push 15
![public void pop All items new ObjectMAXSTACK top 1 public void pop. All() { items = new Object[MAX_STACK]; top = -1; } //](https://slidetodoc.com/presentation_image_h2/b32067e296dd225191404604150d4b9c/image-16.jpg)
public void pop. All() { items = new Object[MAX_STACK]; top = -1; } // end pop. All public Object pop() throws Stack. Exception { if (!is. Empty()) { return items[top--]; } else { throws new Stack. Exception(“Stack. Exception on pop: Stack empty”); } // end if } // end pop public Object peek() throws Stack. Exception { if (!is. Empty()) { return items[top]; } else { throws new Stack. Exception(“Stack. Exception on peek: Stack empty”); } // end if } // end peek } // end Stack. Array. Based 16
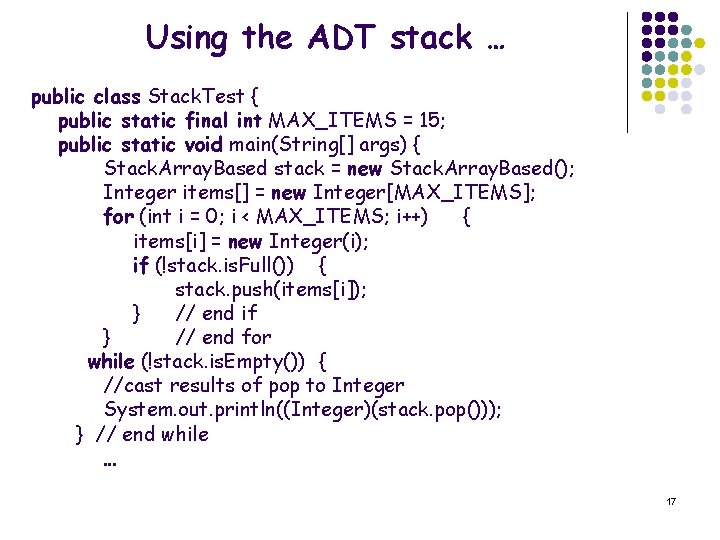
Using the ADT stack … public class Stack. Test { public static final int MAX_ITEMS = 15; public static void main(String[] args) { Stack. Array. Based stack = new Stack. Array. Based(); Integer items[] = new Integer[MAX_ITEMS]; for (int i = 0; i < MAX_ITEMS; i++) { items[i] = new Integer(i); if (!stack. is. Full()) { stack. push(items[i]); } // end if } // end for while (!stack. is. Empty()) { //cast results of pop to Integer System. out. println((Integer)(stack. pop())); } // end while … 17
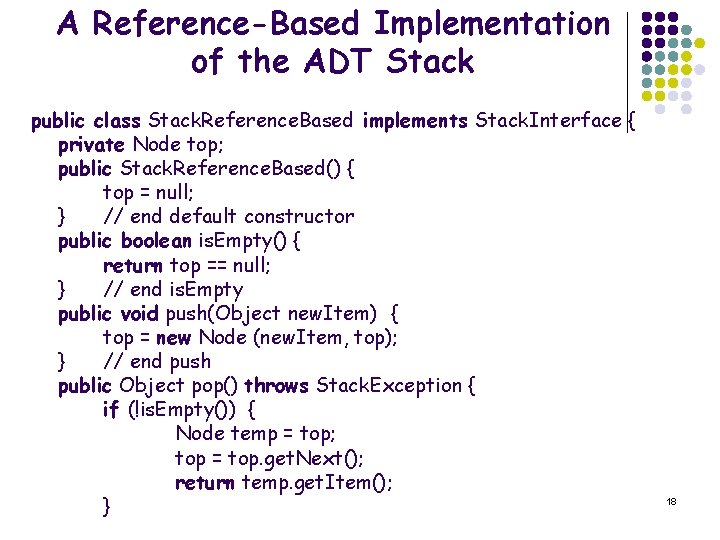
A Reference-Based Implementation of the ADT Stack public class Stack. Reference. Based implements Stack. Interface { private Node top; public Stack. Reference. Based() { top = null; } // end default constructor public boolean is. Empty() { return top == null; } // end is. Empty public void push(Object new. Item) { top = new Node (new. Item, top); } // end push public Object pop() throws Stack. Exception { if (!is. Empty()) { Node temp = top; top = top. get. Next(); return temp. get. Item(); } 18
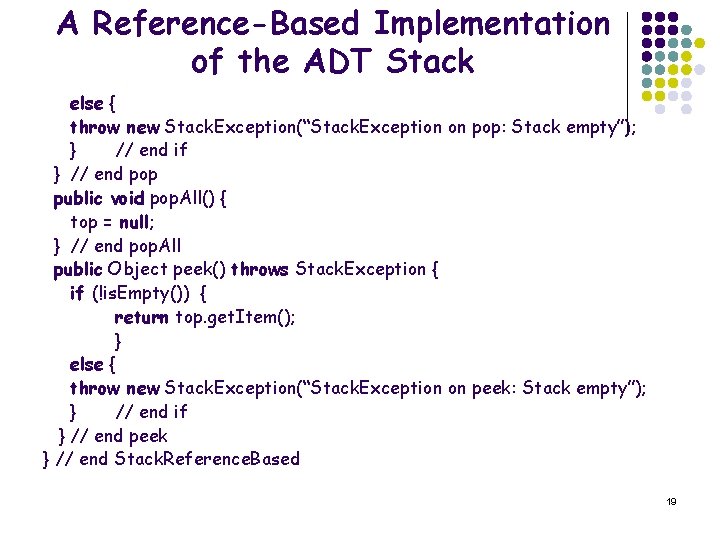
A Reference-Based Implementation of the ADT Stack else { throw new Stack. Exception(“Stack. Exception on pop: Stack empty”); } // end if } // end pop public void pop. All() { top = null; } // end pop. All public Object peek() throws Stack. Exception { if (!is. Empty()) { return top. get. Item(); } else { throw new Stack. Exception(“Stack. Exception on peek: Stack empty”); } // end if } // end peek } // end Stack. Reference. Based 19
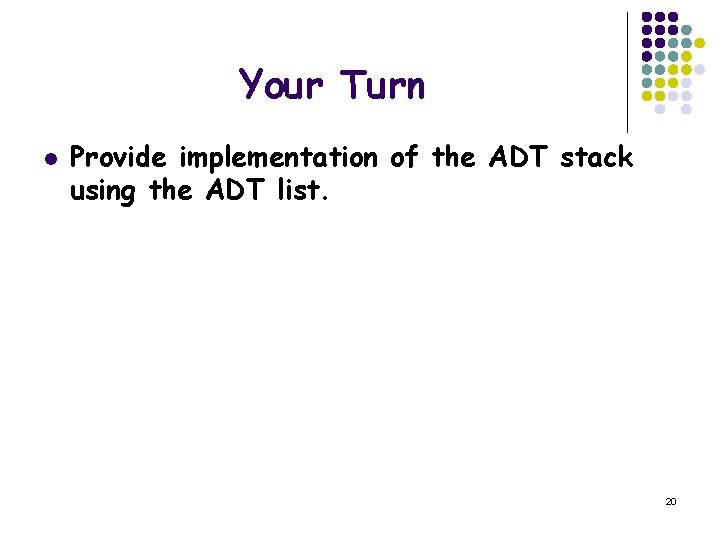
Your Turn l Provide implementation of the ADT stack using the ADT list. 20
Smash the stack
Characteristics of stack
Stack=[] digunakan untuk membuat stack dengan
Java stacks and queues
What are stacks
Two stack pda
Stacks internet
Plant cells
Types of stacks
Stacks+routined
Python stack and queue
Coastal landforms pictures
Data structures using java
6 stacks
Five ocean basins
Angle stacks
Exercises on stacks and queues
Speed stacks spreads nationally in 1998.
Density of golden syrup g/cm3
Every picture has a story and every story has a moment
A problem has been detected and windows o que é