Specification and Implementation Separating the specification from implementation
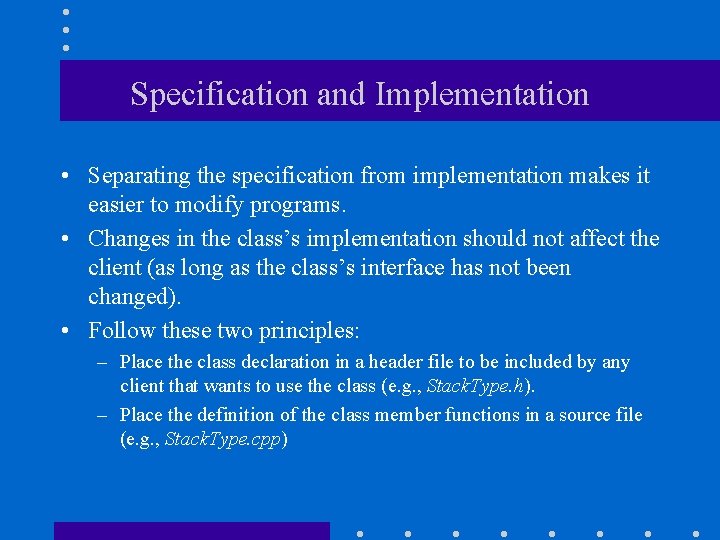
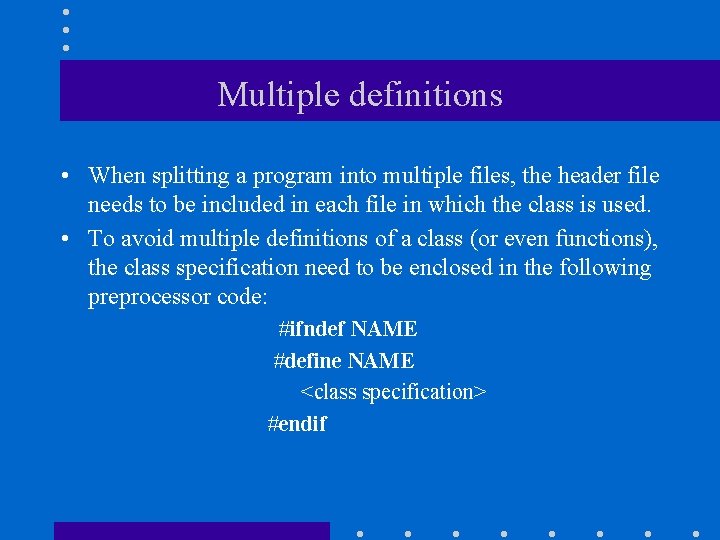
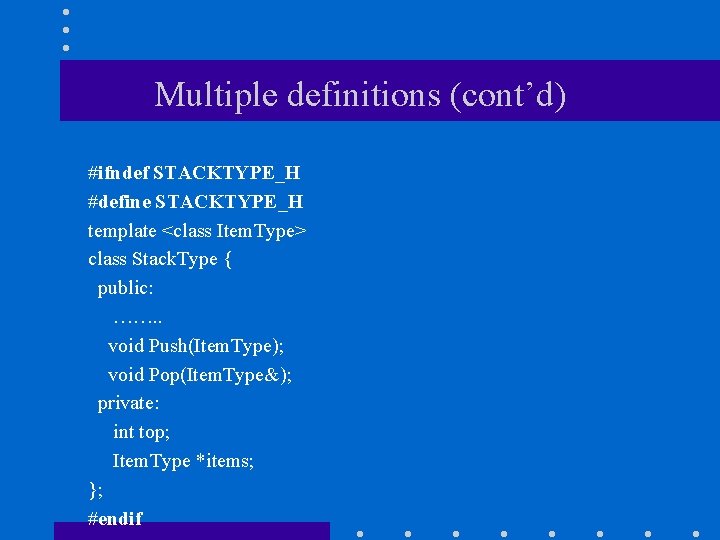
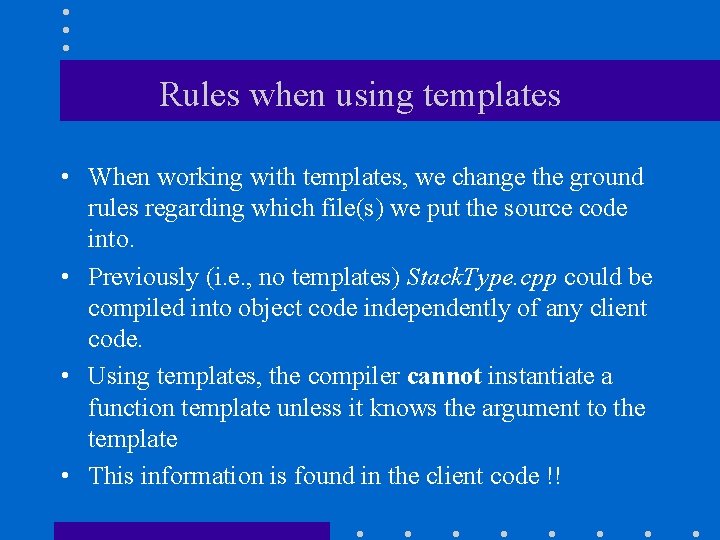
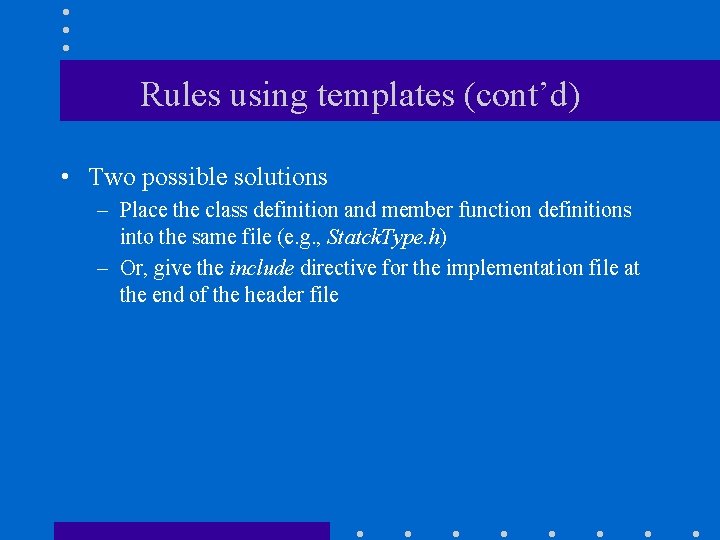
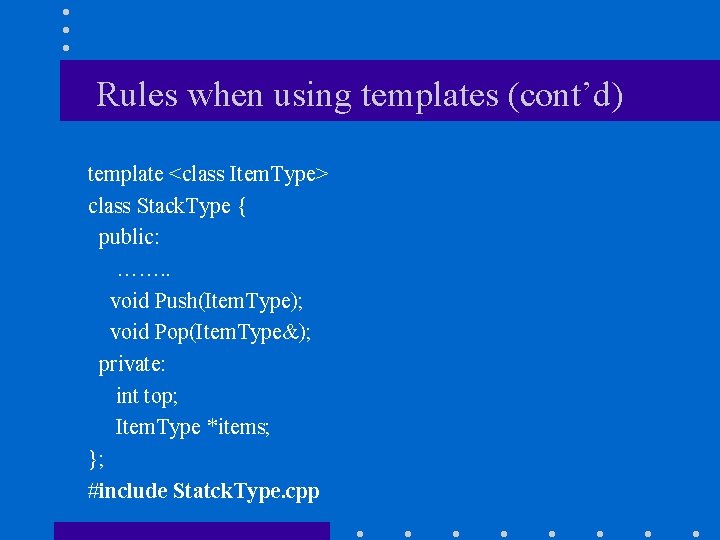
- Slides: 6
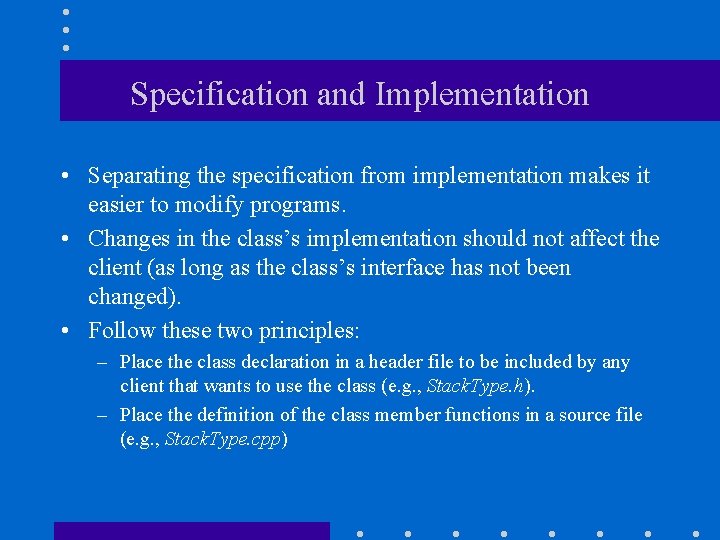
Specification and Implementation • Separating the specification from implementation makes it easier to modify programs. • Changes in the class’s implementation should not affect the client (as long as the class’s interface has not been changed). • Follow these two principles: – Place the class declaration in a header file to be included by any client that wants to use the class (e. g. , Stack. Type. h). – Place the definition of the class member functions in a source file (e. g. , Stack. Type. cpp)
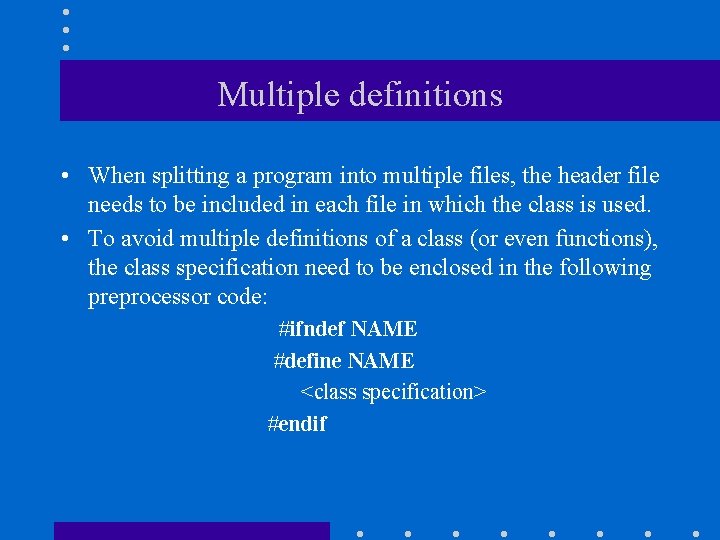
Multiple definitions • When splitting a program into multiple files, the header file needs to be included in each file in which the class is used. • To avoid multiple definitions of a class (or even functions), the class specification need to be enclosed in the following preprocessor code: #ifndef NAME #define NAME <class specification> #endif
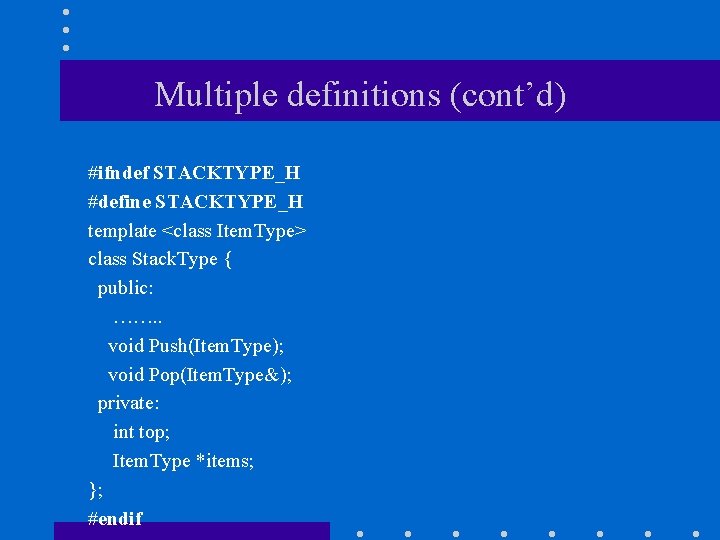
Multiple definitions (cont’d) #ifndef STACKTYPE_H #define STACKTYPE_H template <class Item. Type> class Stack. Type { public: ……. . void Push(Item. Type); void Pop(Item. Type&); private: int top; Item. Type *items; }; #endif
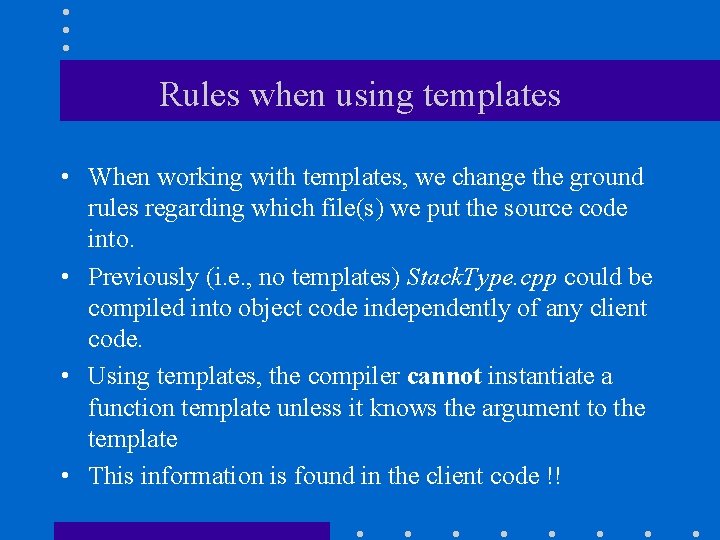
Rules when using templates • When working with templates, we change the ground rules regarding which file(s) we put the source code into. • Previously (i. e. , no templates) Stack. Type. cpp could be compiled into object code independently of any client code. • Using templates, the compiler cannot instantiate a function template unless it knows the argument to the template • This information is found in the client code !!
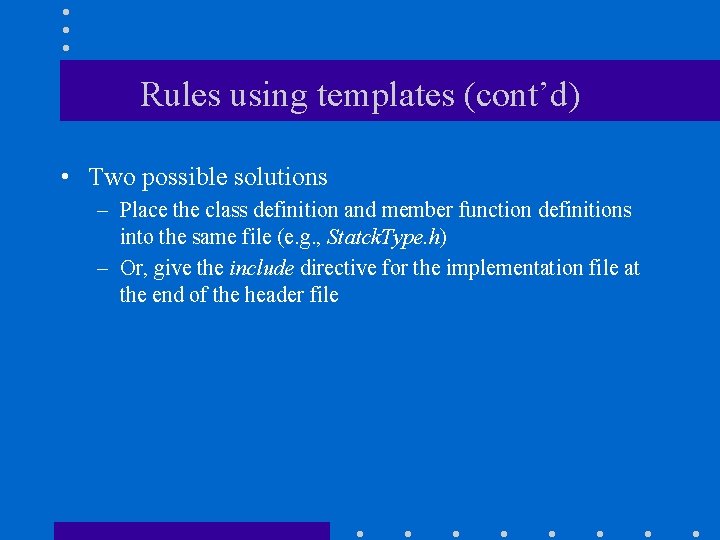
Rules using templates (cont’d) • Two possible solutions – Place the class definition and member function definitions into the same file (e. g. , Statck. Type. h) – Or, give the include directive for the implementation file at the end of the header file
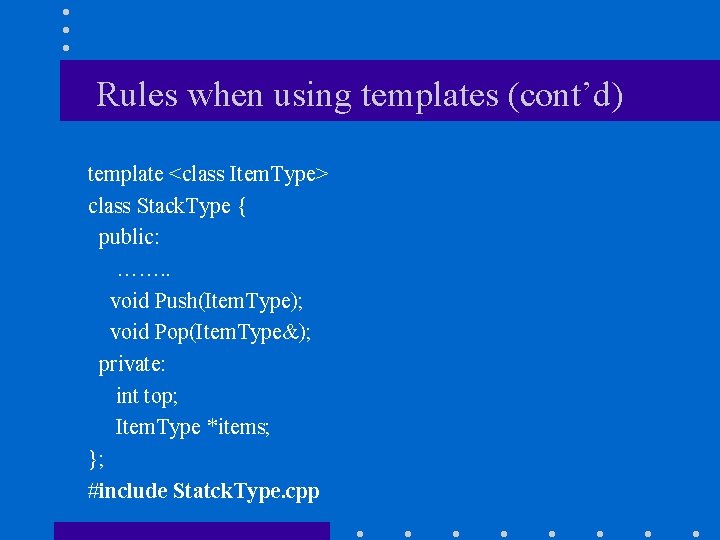
Rules when using templates (cont’d) template <class Item. Type> class Stack. Type { public: ……. . void Push(Item. Type); void Pop(Item. Type&); private: int top; Item. Type *items; }; #include Statck. Type. cpp