RM 102 DSA Refresher Module C Refresher Module
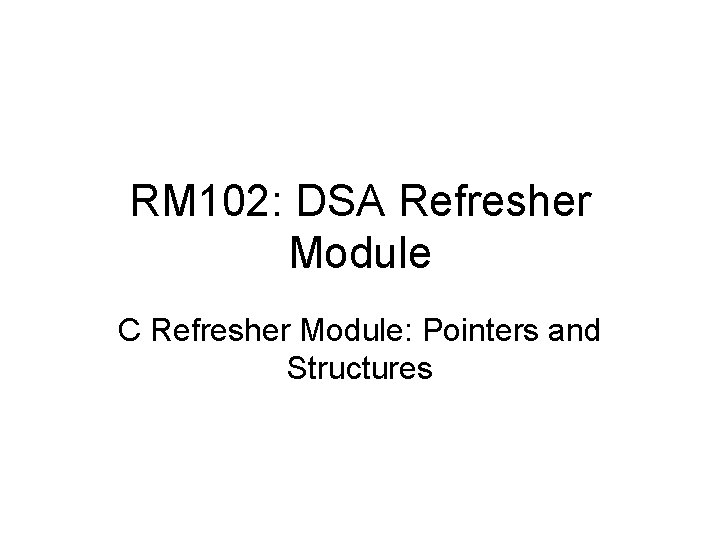
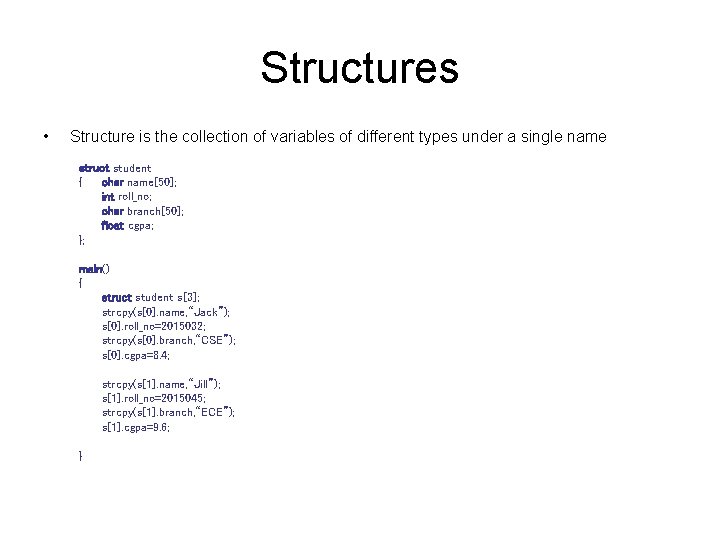
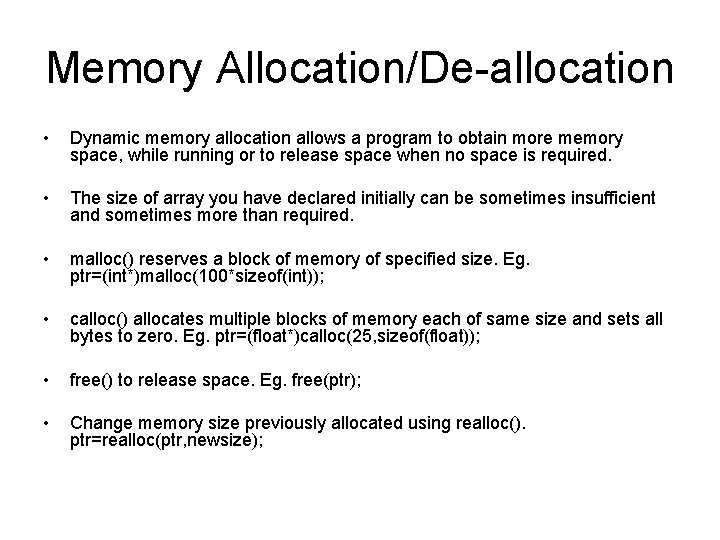
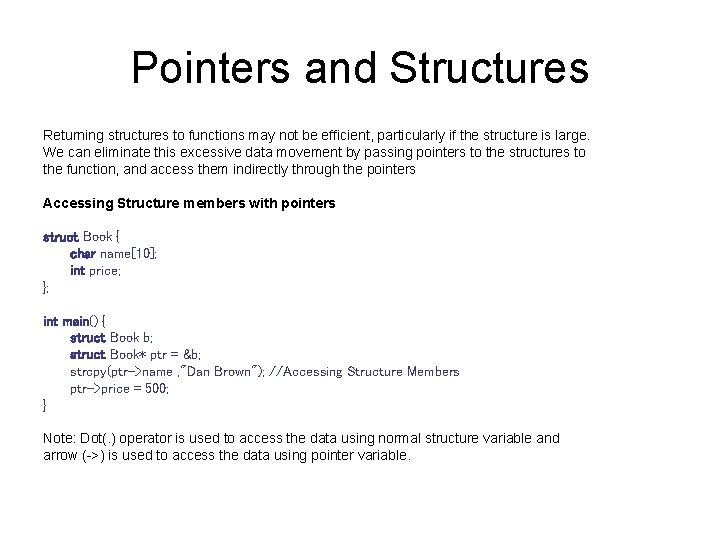
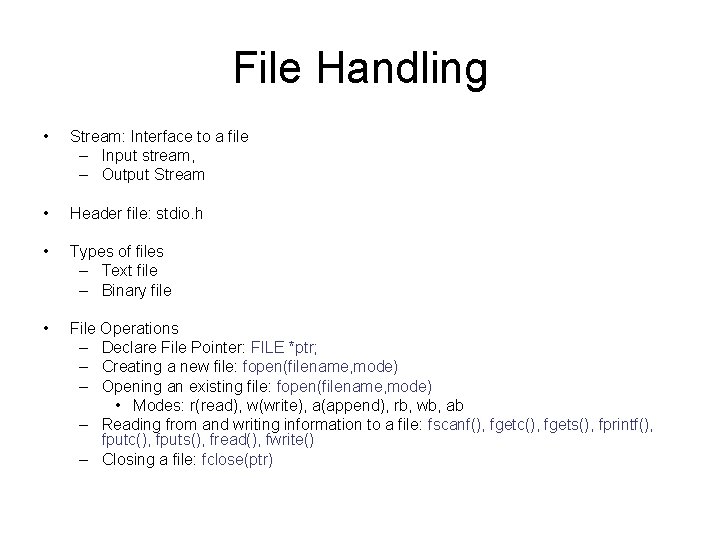
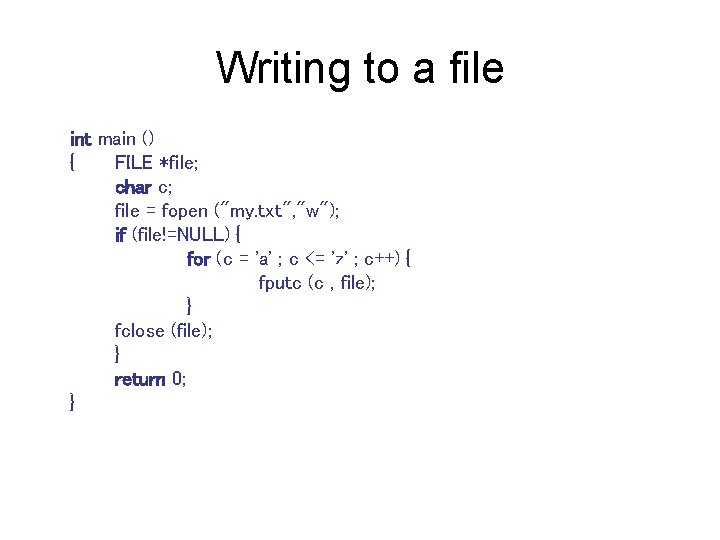
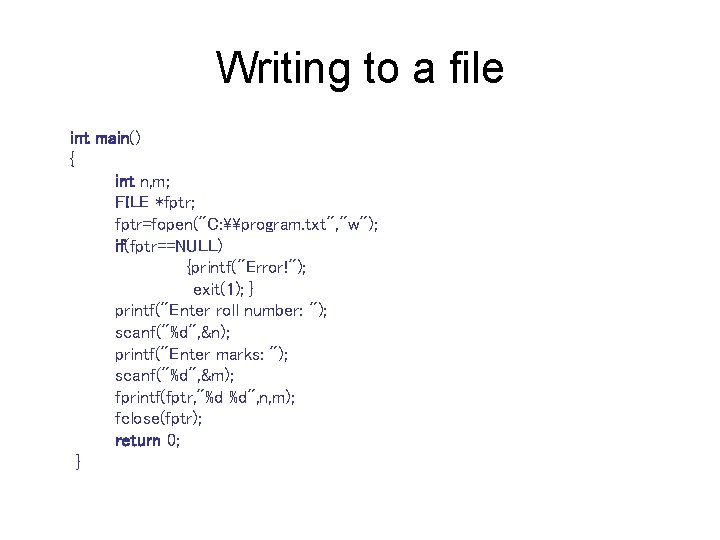
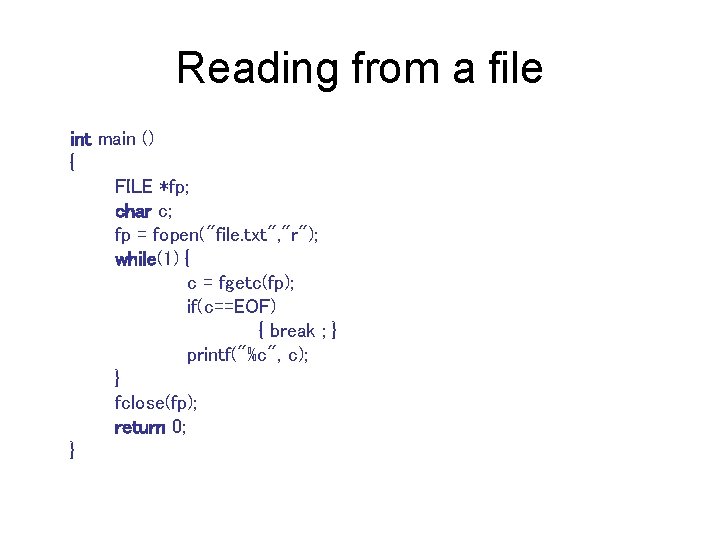
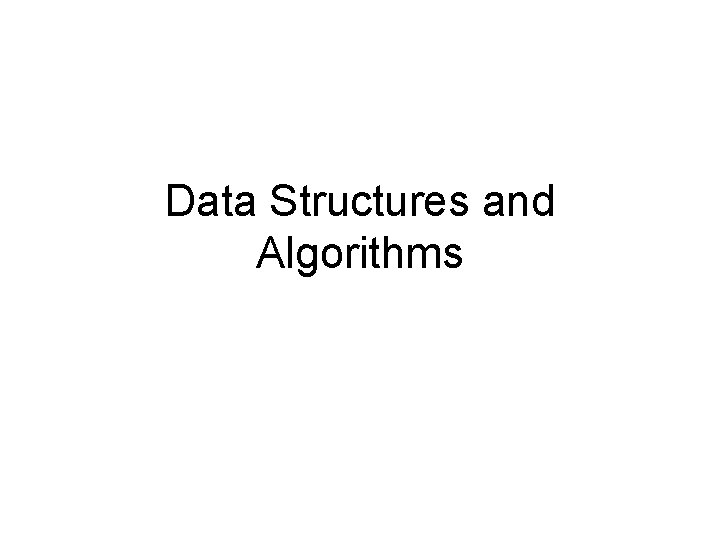
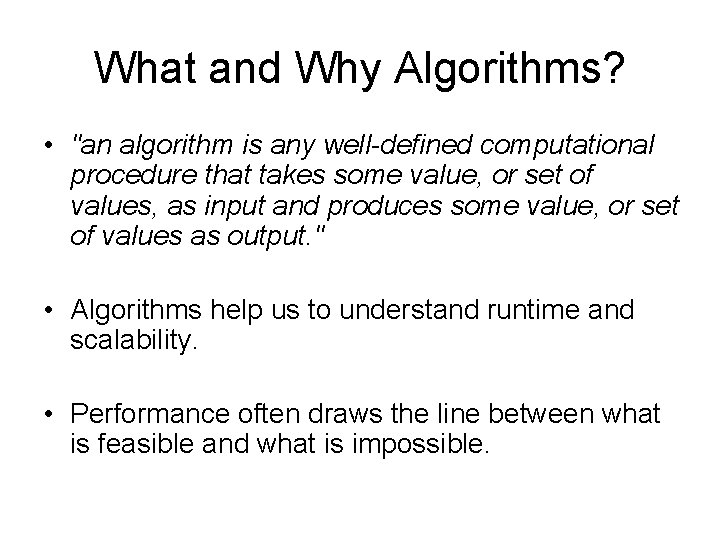
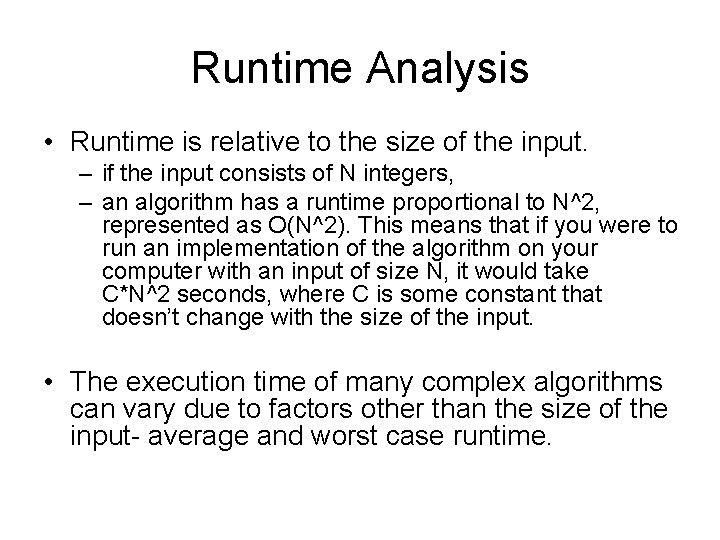
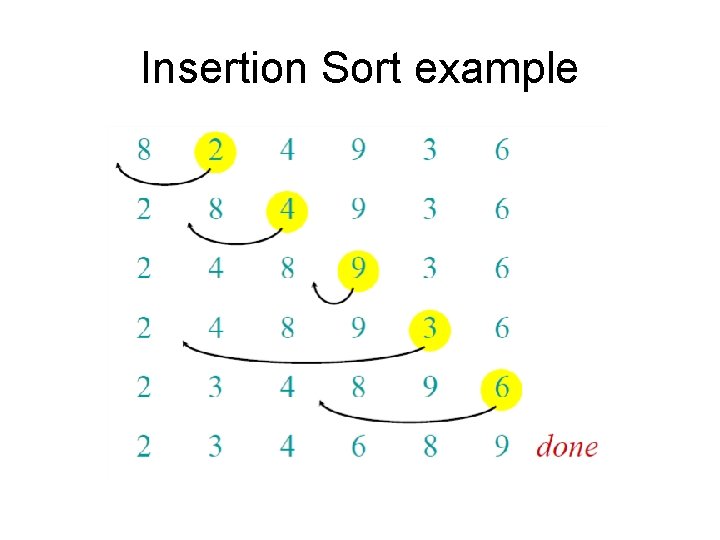
![Insertion Sort INSERTION-SORT(A, n) forj ← 2 to n key ←A[ j] i ←j Insertion Sort INSERTION-SORT(A, n) forj ← 2 to n key ←A[ j] i ←j](https://slidetodoc.com/presentation_image_h2/72a35e801e8cdad4af7dadd13c72532a/image-13.jpg)
- Slides: 13
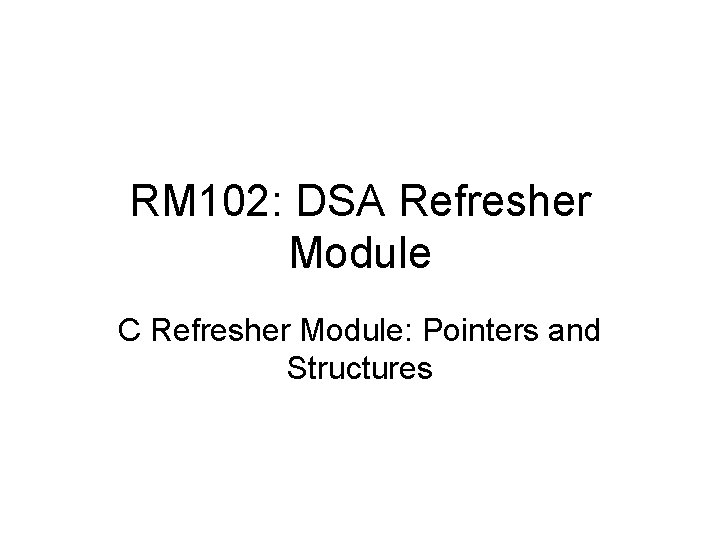
RM 102: DSA Refresher Module C Refresher Module: Pointers and Structures
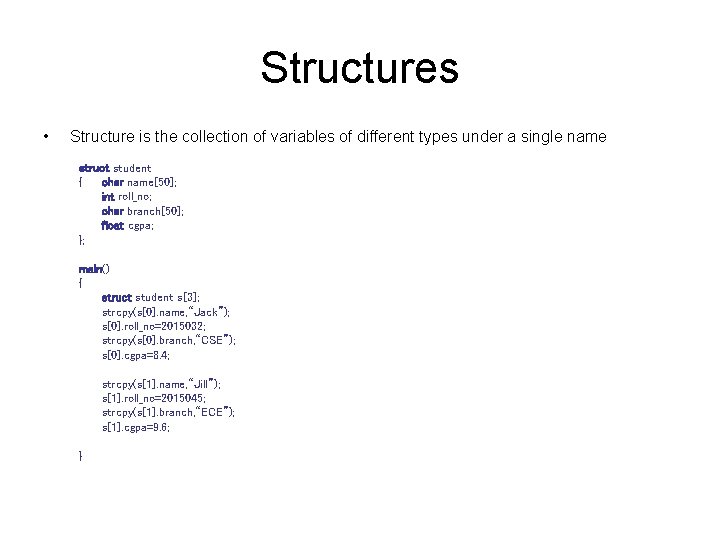
Structures • Structure is the collection of variables of different types under a single name struct student { char name[50]; int roll_no; char branch[50]; float cgpa; }; main() { struct student s[3]; strcpy(s[0]. name, “Jack”); s[0]. roll_no=2015032; strcpy(s[0]. branch, “CSE”); s[0]. cgpa=8. 4; strcpy(s[1]. name, “Jill”); s[1]. roll_no=2015045; strcpy(s[1]. branch, “ECE”); s[1]. cgpa=9. 6; }
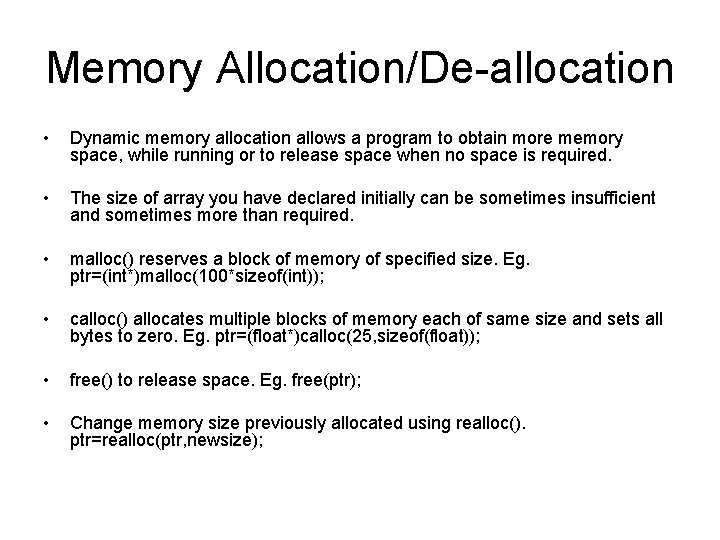
Memory Allocation/De-allocation • Dynamic memory allocation allows a program to obtain more memory space, while running or to release space when no space is required. • The size of array you have declared initially can be sometimes insufficient and sometimes more than required. • malloc() reserves a block of memory of specified size. Eg. ptr=(int*)malloc(100*sizeof(int)); • calloc() allocates multiple blocks of memory each of same size and sets all bytes to zero. Eg. ptr=(float*)calloc(25, sizeof(float)); • free() to release space. Eg. free(ptr); • Change memory size previously allocated using realloc(). ptr=realloc(ptr, newsize);
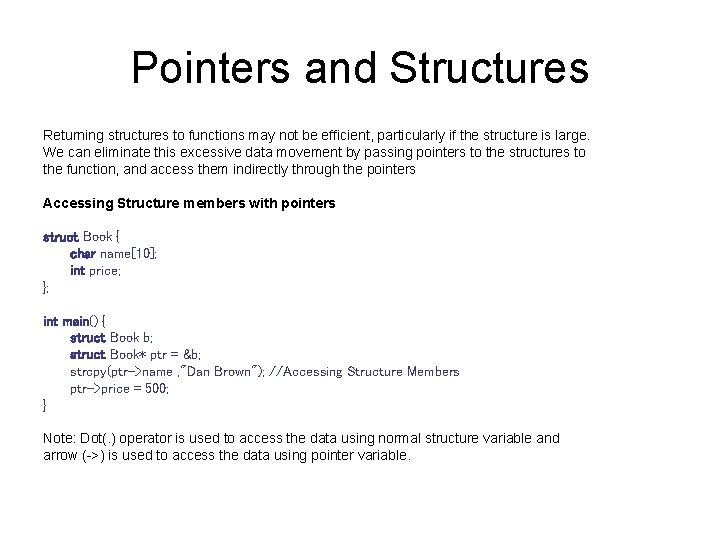
Pointers and Structures Returning structures to functions may not be efficient, particularly if the structure is large. We can eliminate this excessive data movement by passing pointers to the structures to the function, and access them indirectly through the pointers Accessing Structure members with pointers struct Book { char name[10]; int price; }; int main() { struct Book b; struct Book* ptr = &b; strcpy(ptr->name , "Dan Brown"); //Accessing Structure Members ptr->price = 500; } Note: Dot(. ) operator is used to access the data using normal structure variable and arrow (->) is used to access the data using pointer variable.
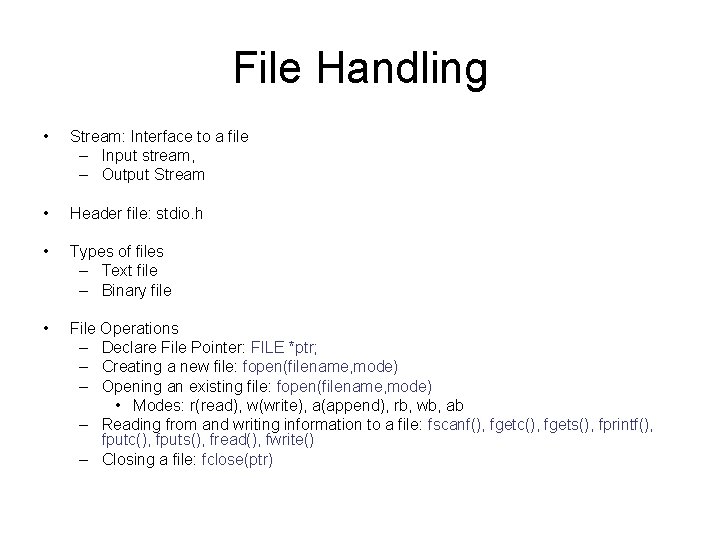
File Handling • Stream: Interface to a file – Input stream, – Output Stream • Header file: stdio. h • Types of files – Text file – Binary file • File Operations – Declare File Pointer: FILE *ptr; – Creating a new file: fopen(filename, mode) – Opening an existing file: fopen(filename, mode) • Modes: r(read), w(write), a(append), rb, wb, ab – Reading from and writing information to a file: fscanf(), fgetc(), fgets(), fprintf(), fputc(), fputs(), fread(), fwrite() – Closing a file: fclose(ptr)
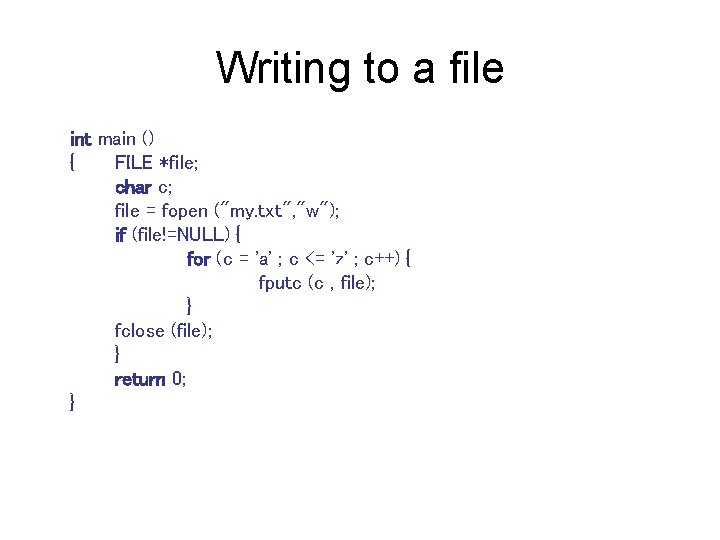
Writing to a file int main () { FILE *file; char c; file = fopen ("my. txt", "w"); if (file!=NULL) { for (c = 'a' ; c <= 'z' ; c++) { fputc (c , file); } fclose (file); } return 0; }
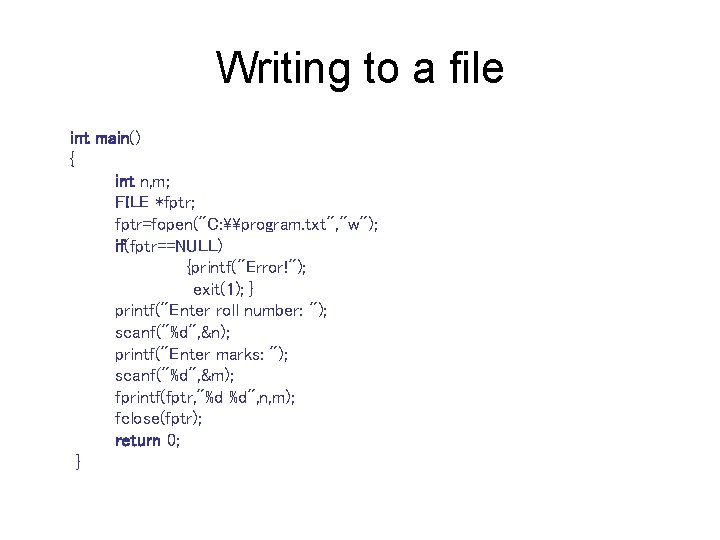
Writing to a file int main() { int n, m; FILE *fptr; fptr=fopen("C: \program. txt", "w"); if(fptr==NULL) {printf("Error!"); exit(1); } printf("Enter roll number: "); scanf("%d", &n); printf("Enter marks: "); scanf("%d", &m); fprintf(fptr, "%d %d", n, m); fclose(fptr); return 0; }
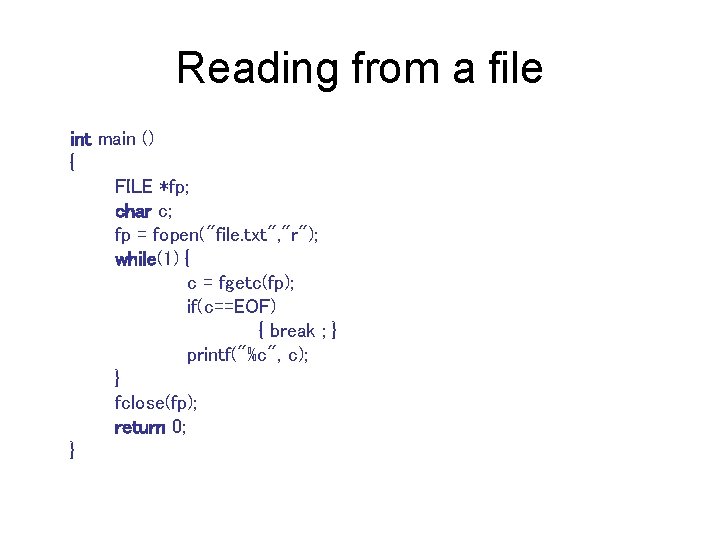
Reading from a file int main () { FILE *fp; char c; fp = fopen("file. txt", "r"); while(1) { c = fgetc(fp); if(c==EOF) { break ; } printf("%c", c); } fclose(fp); return 0; }
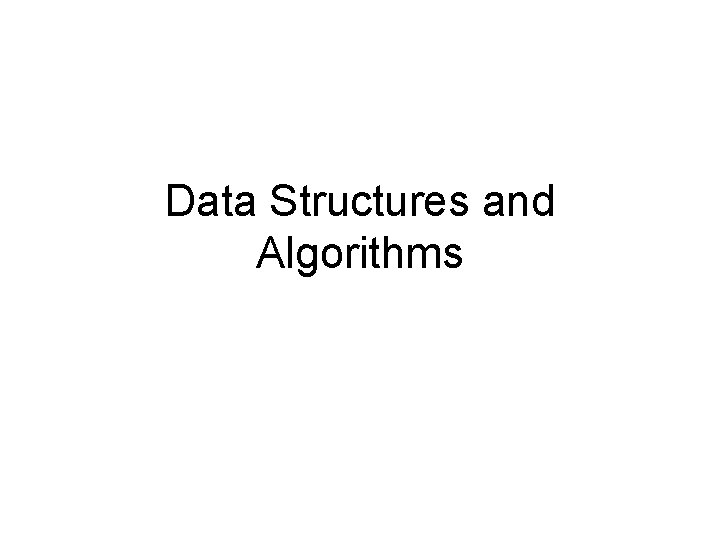
Data Structures and Algorithms
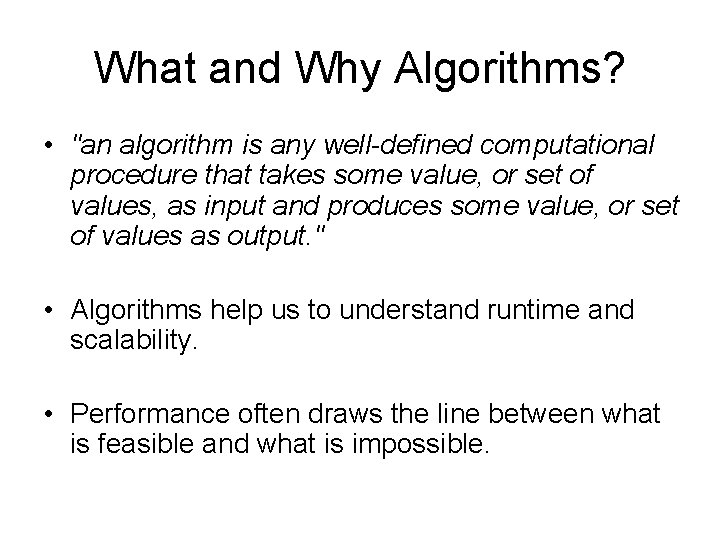
What and Why Algorithms? • "an algorithm is any well-defined computational procedure that takes some value, or set of values, as input and produces some value, or set of values as output. " • Algorithms help us to understand runtime and scalability. • Performance often draws the line between what is feasible and what is impossible.
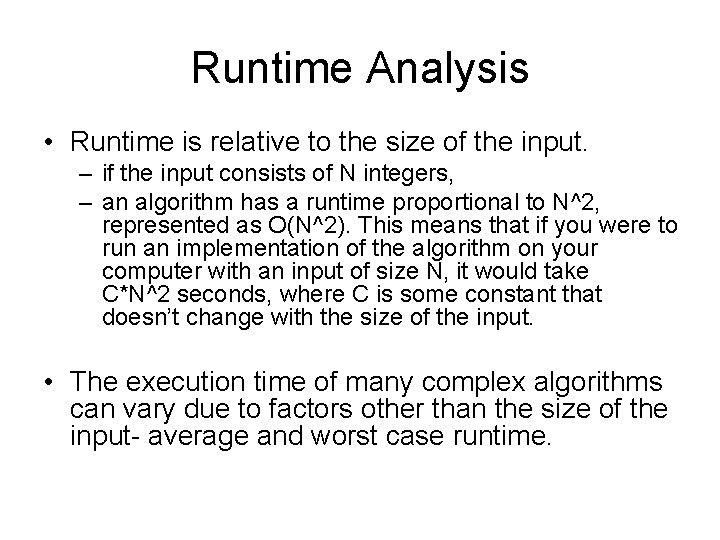
Runtime Analysis • Runtime is relative to the size of the input. – if the input consists of N integers, – an algorithm has a runtime proportional to N^2, represented as O(N^2). This means that if you were to run an implementation of the algorithm on your computer with an input of size N, it would take C*N^2 seconds, where C is some constant that doesn’t change with the size of the input. • The execution time of many complex algorithms can vary due to factors other than the size of the input- average and worst case runtime.
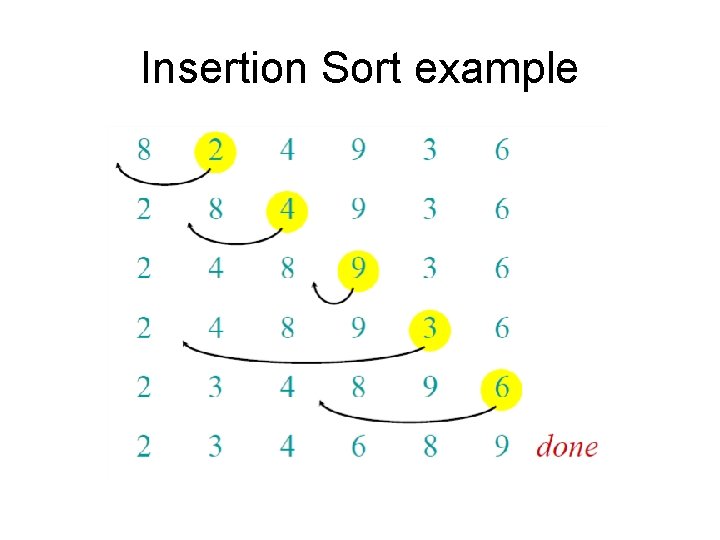
Insertion Sort example
![Insertion Sort INSERTIONSORTA n forj 2 to n key A j i j Insertion Sort INSERTION-SORT(A, n) forj ← 2 to n key ←A[ j] i ←j](https://slidetodoc.com/presentation_image_h2/72a35e801e8cdad4af7dadd13c72532a/image-13.jpg)
Insertion Sort INSERTION-SORT(A, n) forj ← 2 to n key ←A[ j] i ←j – 1 whilei > 0 and A[i] > key A[i+1] ←A[i] i ←i – 1 A[i+1] = key cost C 1 C 2 C 3 times N N-1 C 4 C 5 C 6 C 7 ∑t ∑(t-1) N-1 Run time: C 1*N + C 2*(N-1) + C 3*(N-1)+ C 4*(∑t) + C 5*(∑(t-1)) + C 6*(∑(t-1)) + C 7*(N-1) = A*N^2 + B*N + C