Programming Languages and Paradigms Activation Records in Java
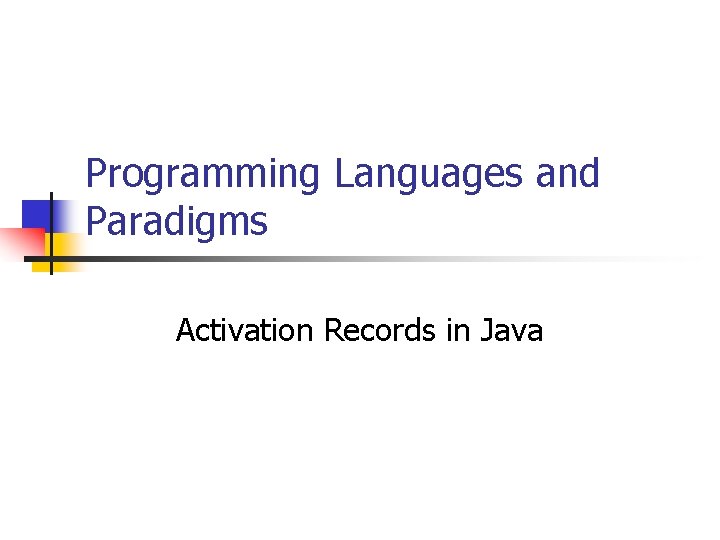
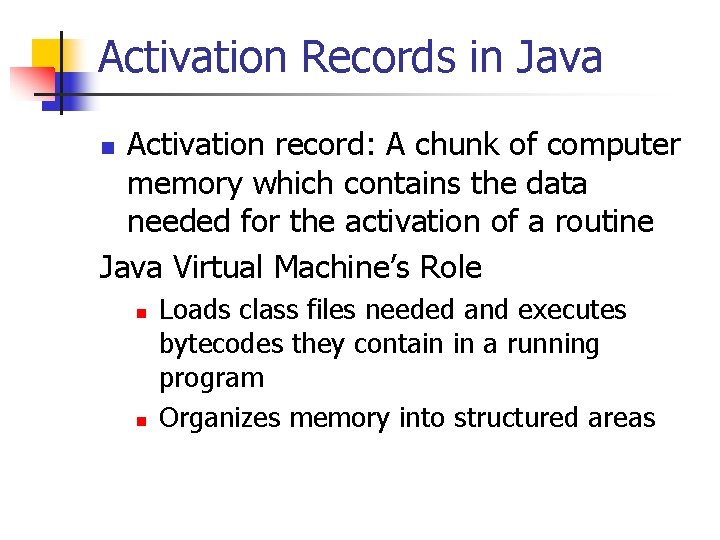
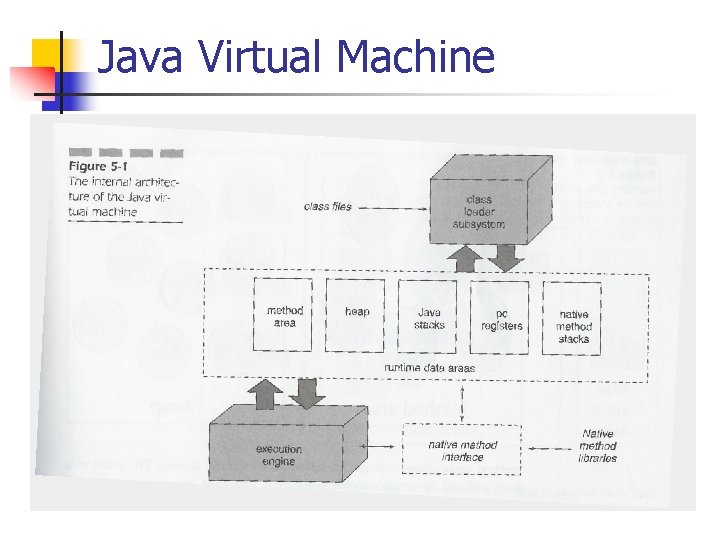
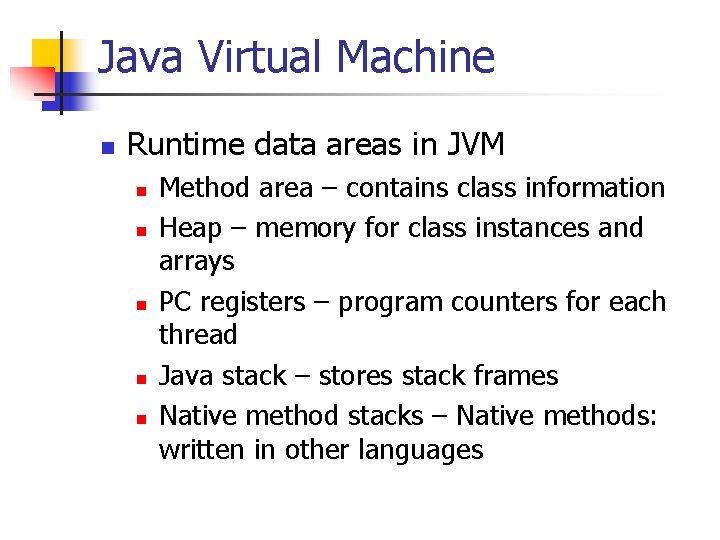
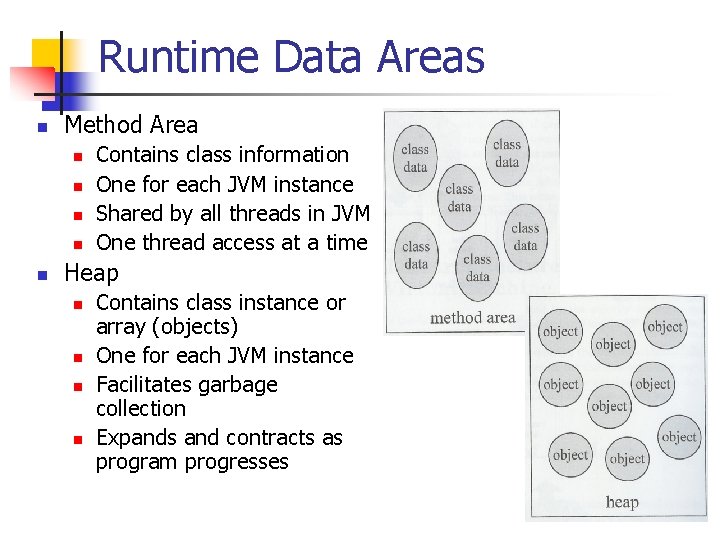
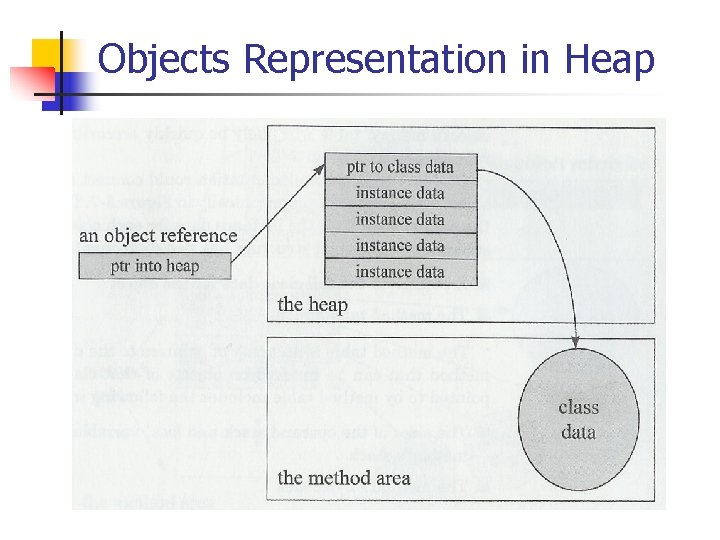
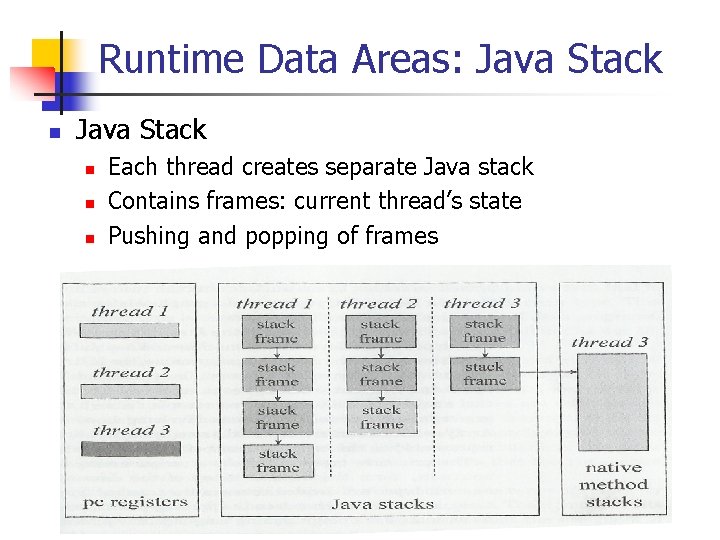
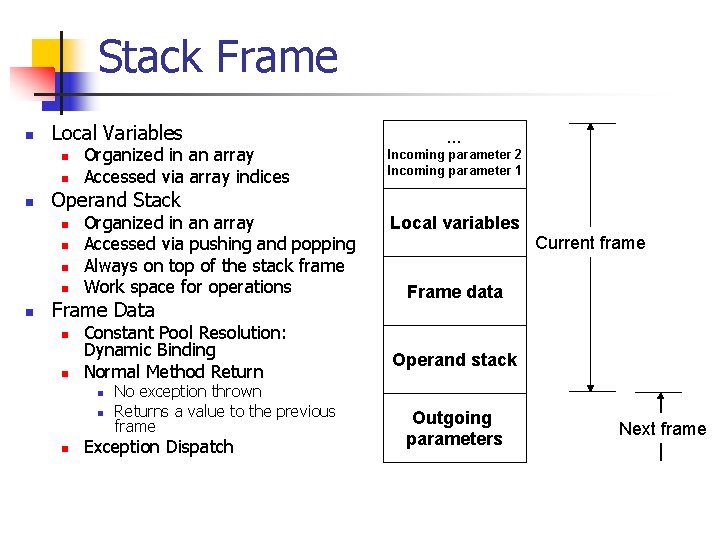
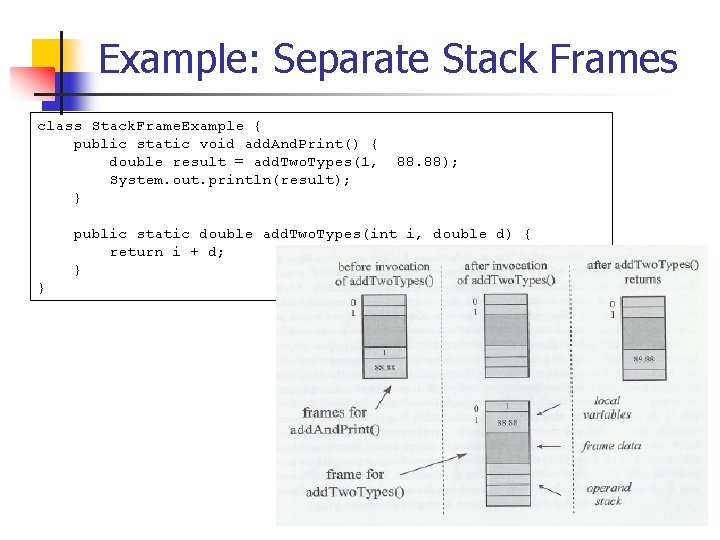
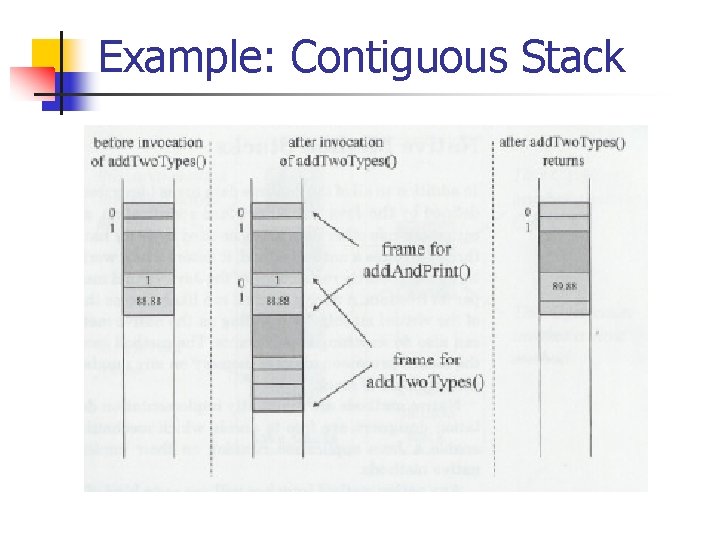
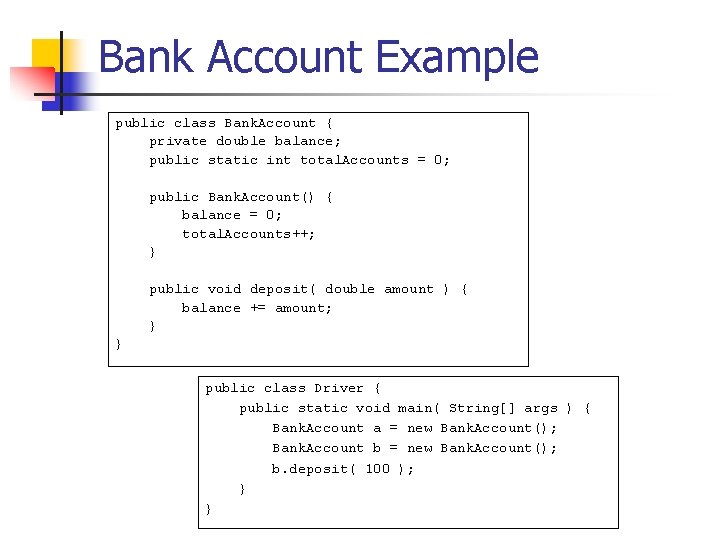
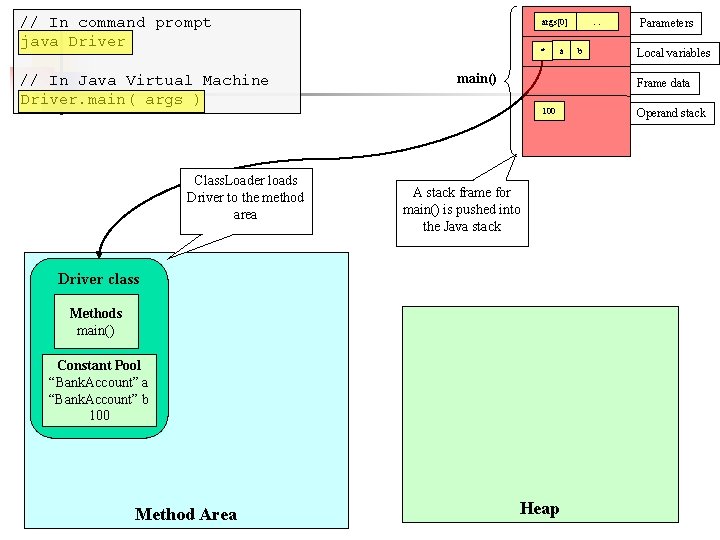
![// Driver. java public static void main( String[] args ) { Bank. Account a // Driver. java public static void main( String[] args ) { Bank. Account a](https://slidetodoc.com/presentation_image_h/dc3eae9bcf270dc8fa8da6e6fceb7926/image-13.jpg)
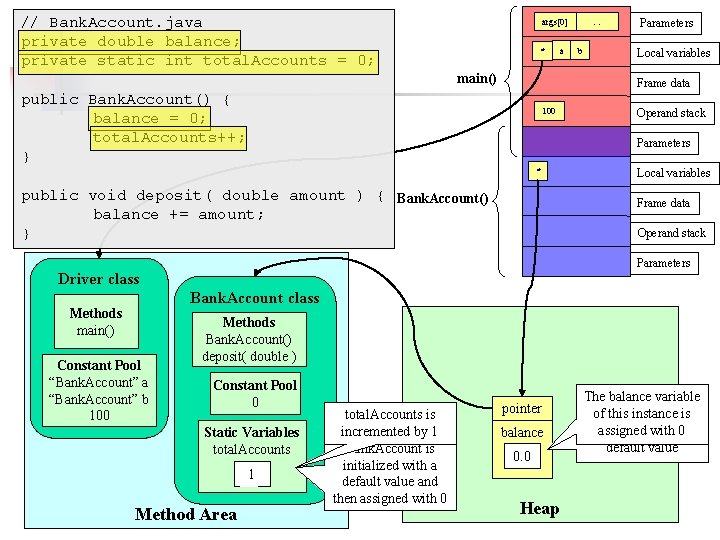
![// Driver. java public static void main( String[] args ) { Bank. Account a // Driver. java public static void main( String[] args ) { Bank. Account a](https://slidetodoc.com/presentation_image_h/dc3eae9bcf270dc8fa8da6e6fceb7926/image-15.jpg)
![// Driver. java public static void main( String[] args ) { Bank. Account a // Driver. java public static void main( String[] args ) { Bank. Account a](https://slidetodoc.com/presentation_image_h/dc3eae9bcf270dc8fa8da6e6fceb7926/image-16.jpg)
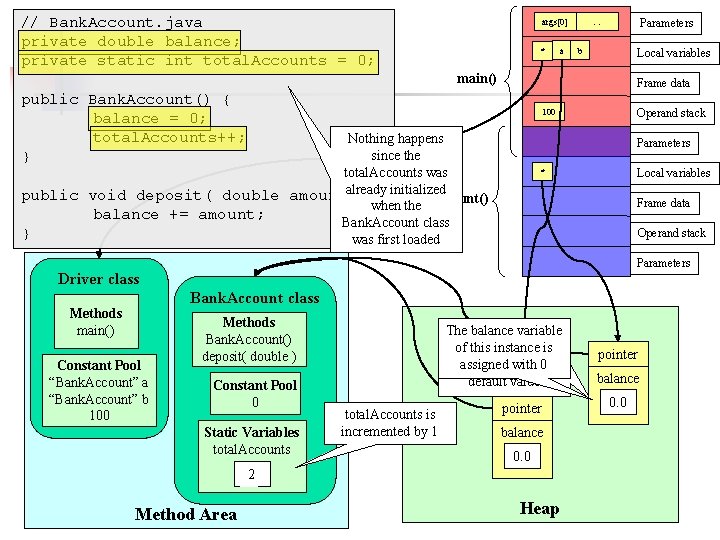
![// Driver. java public static void main( String[] args ) { Bank. Account a // Driver. java public static void main( String[] args ) { Bank. Account a](https://slidetodoc.com/presentation_image_h/dc3eae9bcf270dc8fa8da6e6fceb7926/image-18.jpg)
![// Driver. java public static void main( String[] args ) { Bank. Account a // Driver. java public static void main( String[] args ) { Bank. Account a](https://slidetodoc.com/presentation_image_h/dc3eae9bcf270dc8fa8da6e6fceb7926/image-19.jpg)
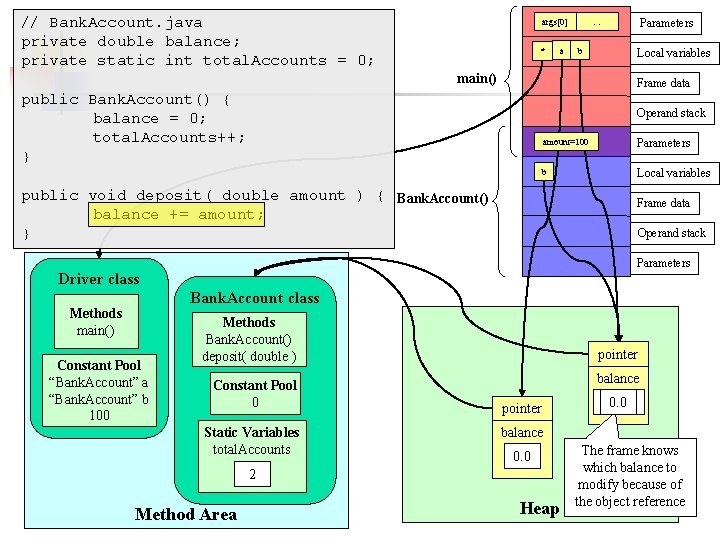
- Slides: 20
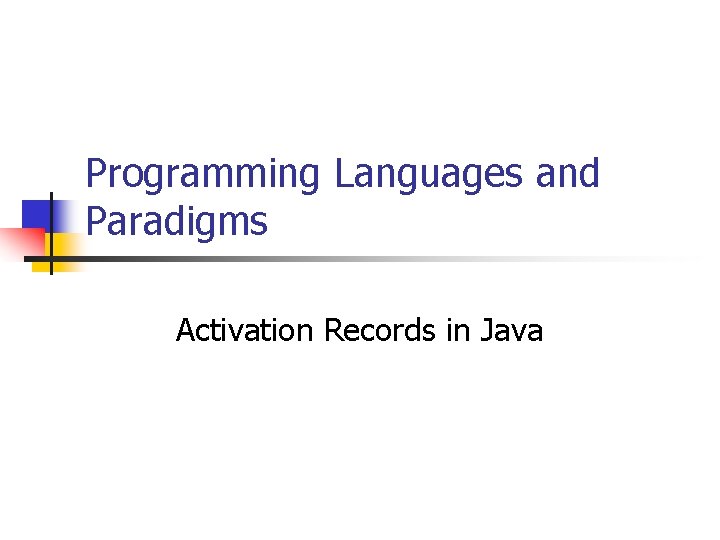
Programming Languages and Paradigms Activation Records in Java
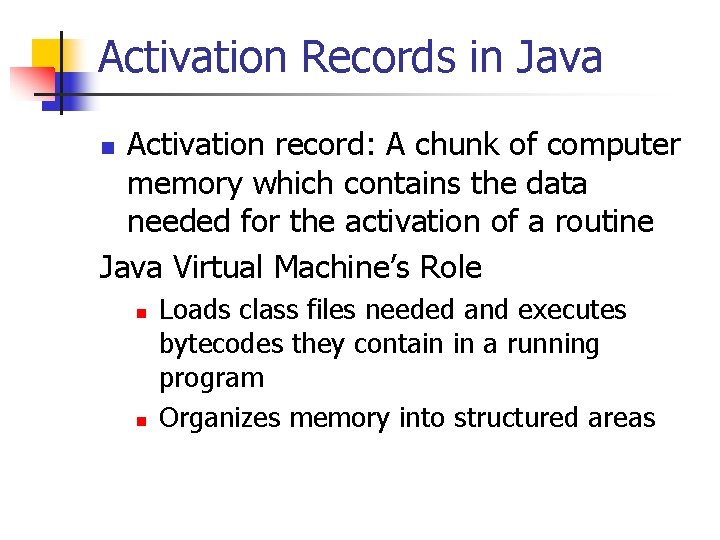
Activation Records in Java Activation record: A chunk of computer memory which contains the data needed for the activation of a routine Java Virtual Machine’s Role n n n Loads class files needed and executes bytecodes they contain in a running program Organizes memory into structured areas
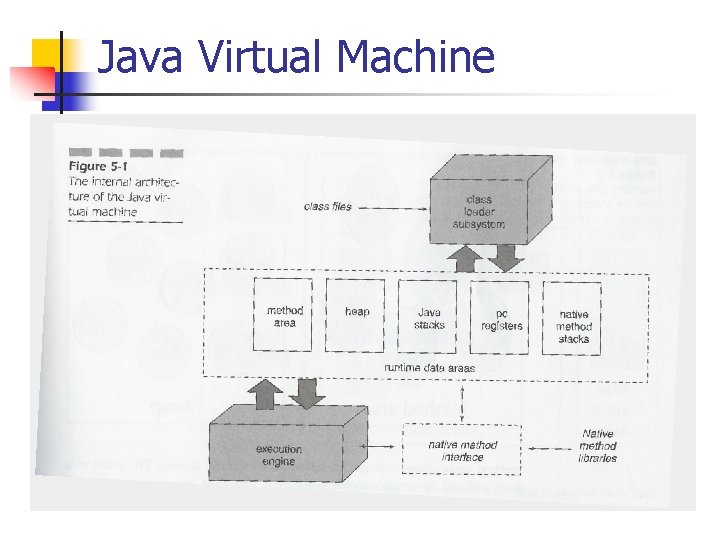
Java Virtual Machine
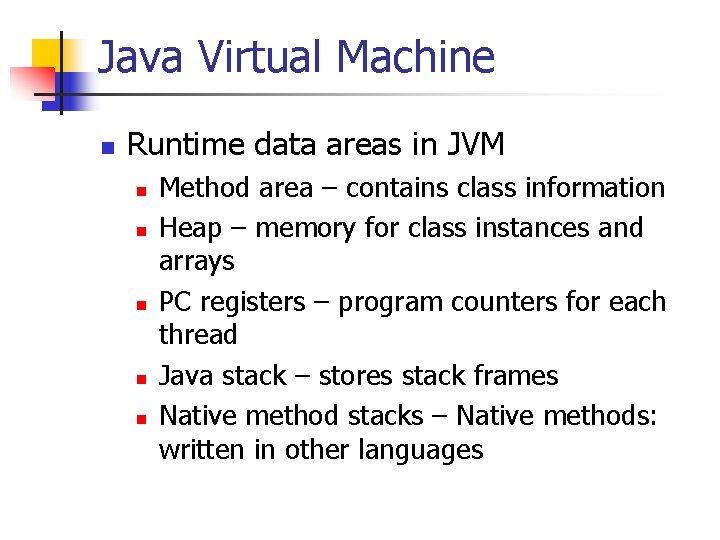
Java Virtual Machine n Runtime data areas in JVM n n n Method area – contains class information Heap – memory for class instances and arrays PC registers – program counters for each thread Java stack – stores stack frames Native method stacks – Native methods: written in other languages
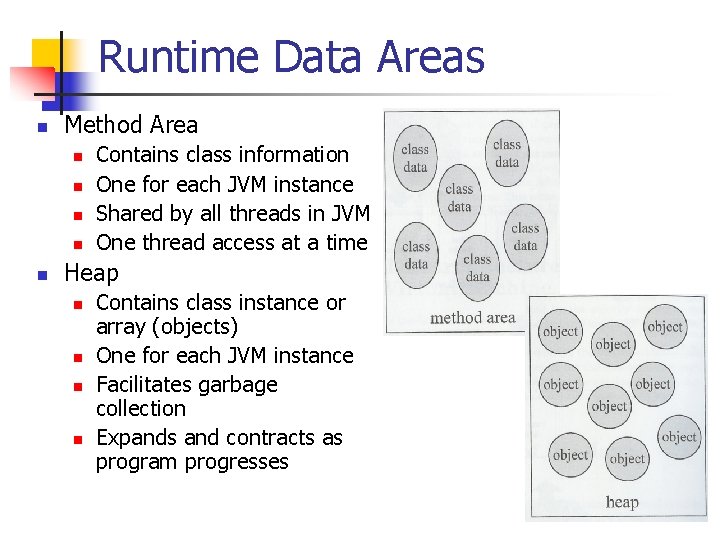
Runtime Data Areas n Method Area n n n Contains class information One for each JVM instance Shared by all threads in JVM One thread access at a time Heap n n Contains class instance or array (objects) One for each JVM instance Facilitates garbage collection Expands and contracts as program progresses
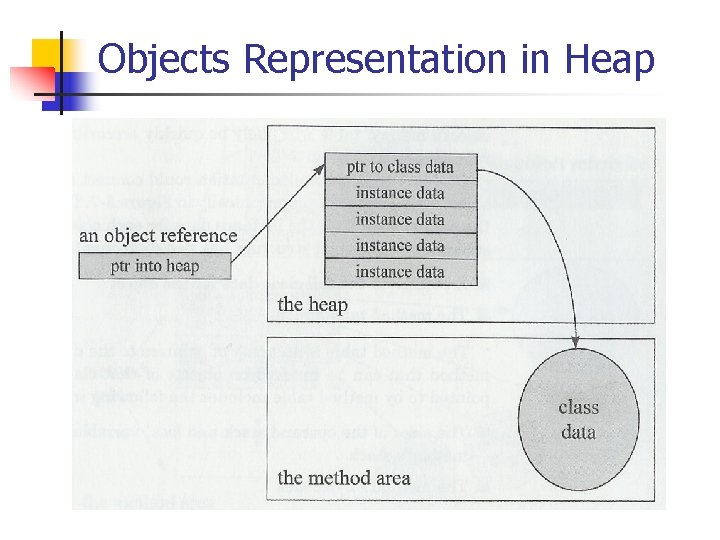
Objects Representation in Heap
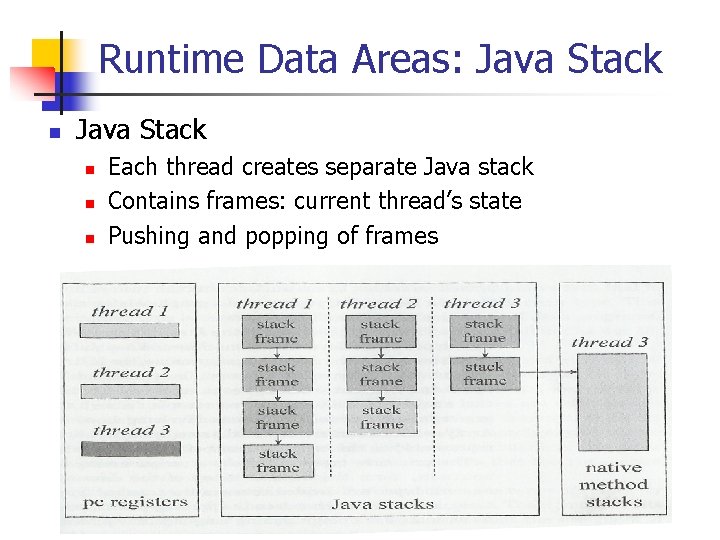
Runtime Data Areas: Java Stack n n n Each thread creates separate Java stack Contains frames: current thread’s state Pushing and popping of frames
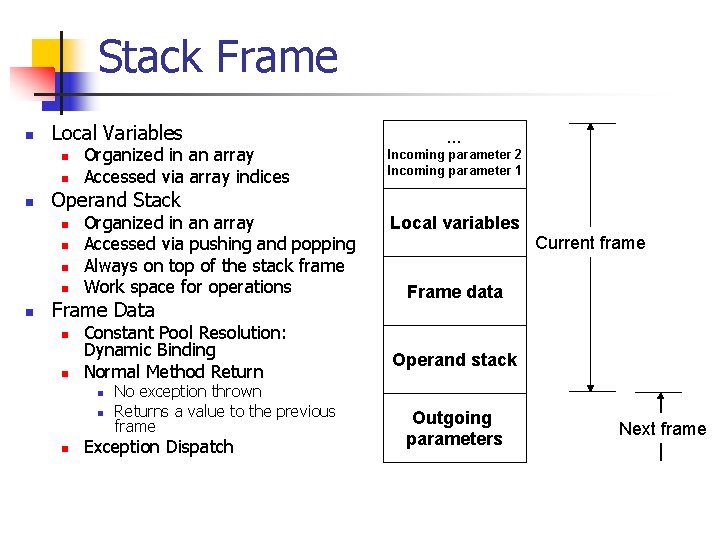
Stack Frame n Local Variables n n n Operand Stack n n n Organized in an array Accessed via array indices … Incoming parameter 2 Incoming parameter 1 Organized in an array Accessed via pushing and popping Always on top of the stack frame Work space for operations Frame Data n n Constant Pool Resolution: Dynamic Binding Normal Method Return n No exception thrown Returns a value to the previous frame Exception Dispatch Local variables Current frame Frame data Operand stack Outgoing parameters Next frame
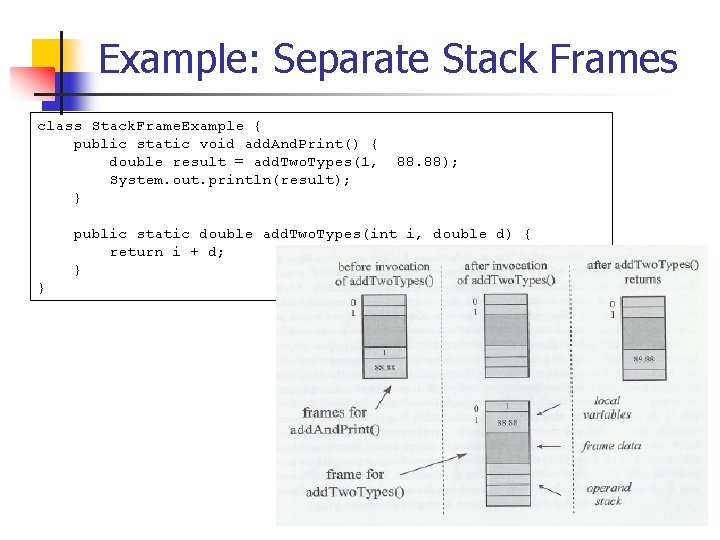
Example: Separate Stack Frames class Stack. Frame. Example { public static void add. And. Print() { double result = add. Two. Types(1, System. out. println(result); } 88. 88); public static double add. Two. Types(int i, double d) { return i + d; } }
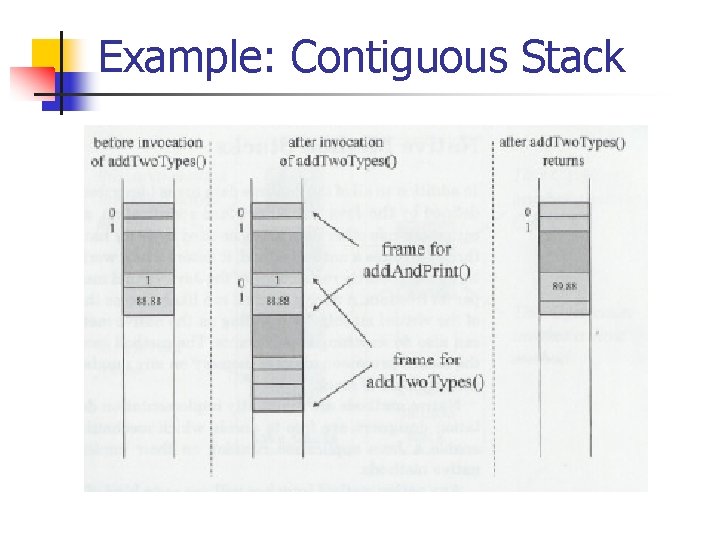
Example: Contiguous Stack
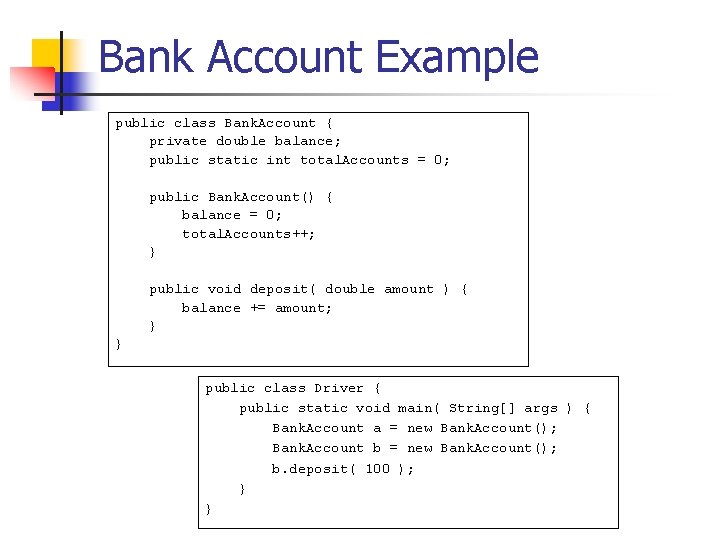
Bank Account Example public class Bank. Account { private double balance; public static int total. Accounts = 0; public Bank. Account() { balance = 0; total. Accounts++; } public void deposit( double amount ) { balance += amount; } } public class Driver { public static void main( String[] args ) { Bank. Account a = new Bank. Account(); Bank. Account b = new Bank. Account(); b. deposit( 100 ); } }
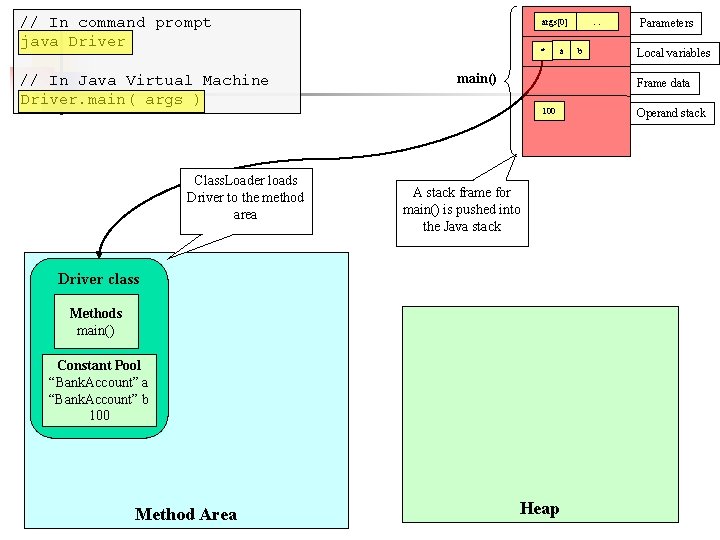
// In command prompt java Driver // In Java Virtual Machine Driver. main( args ) Class. Loader loads Driver to the method area args[0] * a main() b Parameters Local variables Frame data 100 A stack frame for main() is pushed into the Java stack Driver class Methods main() Constant Pool “Bank. Account” a “Bank. Account” b 100 Method Area … Heap Operand stack
![Driver java public static void main String args Bank Account a // Driver. java public static void main( String[] args ) { Bank. Account a](https://slidetodoc.com/presentation_image_h/dc3eae9bcf270dc8fa8da6e6fceb7926/image-13.jpg)
// Driver. java public static void main( String[] args ) { Bank. Account a = new Bank. Account(); Bank. Account b = new Bank. Account(); b. deposit( 100 ); } args[0] * a main() 100 * Class. Loader loads Bank. Account() Bank. Account to the method area … b Parameters Local variables A pointer to the Frame data Bank. Account pointer in the heap is Operand stack created (Constant Pool Resolution) Parameters Local variables Frame data Operand stack Parameters Driver class Bank. Account class Methods main() Constant Pool “Bank. Account” a “Bank. Account” b 100 A stack frame for the Bank. Account constructor is pushed into the Java stack Methods Bank. Account() deposit( double ) Constant Pool 0 Method Area pointer A pointer to the Bank. Account class data is created Heap
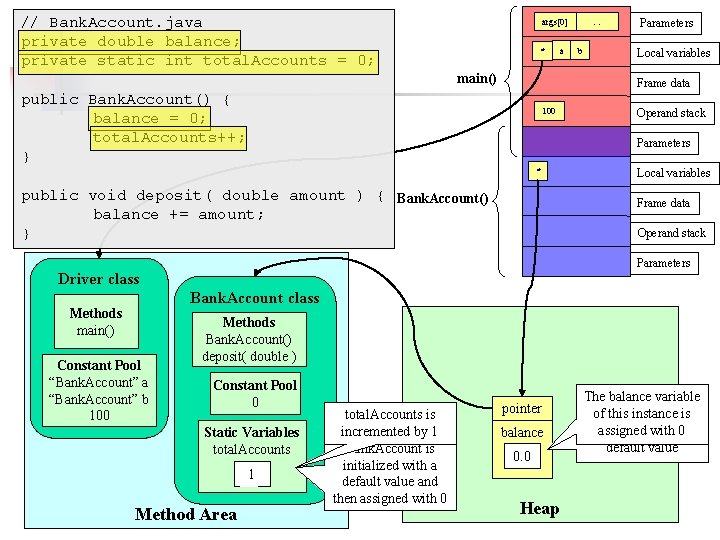
// Bank. Account. java private double balance; private static int total. Accounts = 0; args[0] * a main() … b Parameters Local variables Frame data public Bank. Account() { balance = 0; total. Accounts++; } 100 Operand stack Parameters * public void deposit( double amount ) { Bank. Account() balance += amount; } Local variables Frame data Operand stack Parameters Driver class Bank. Account class Methods main() Constant Pool “Bank. Account” a “Bank. Account” b 100 Methods Bank. Account() deposit( double ) Constant Pool 0 Static Variables total. Accounts 10 Method Area The total. Accounts is incremented static variable byof 1 Bank. Account is initialized with a default value and then assigned with 0 pointer balance 0. 0 Heap The balance variable of this instance is initialized assigned with 0 a default value
![Driver java public static void main String args Bank Account a // Driver. java public static void main( String[] args ) { Bank. Account a](https://slidetodoc.com/presentation_image_h/dc3eae9bcf270dc8fa8da6e6fceb7926/image-15.jpg)
// Driver. java public static void main( String[] args ) { Bank. Account a = new Bank. Account(); Bank. Account b = new Bank. Account(); b. deposit( 100 ); } * a Frame data 100 Methods main() Constant Pool “Bank. Account” a “Bank. Account” b 100 Methods Bank. Account() deposit( double ) Constant Pool 0 Static Variables total. Accounts * Operand stack Local variables Frame data Operand stack The stack frame for the Bank. Account constructor is popped from the Java stack Parameters pointer balance 0. 0 1 Method Area * Parameters Bank. Account() Bank. Account class b main() The pointer is popped from the operand stack and assigned to a Driver class Parameters pointer is returned to the calling. Local framevariables …The args[0] Heap
![Driver java public static void main String args Bank Account a // Driver. java public static void main( String[] args ) { Bank. Account a](https://slidetodoc.com/presentation_image_h/dc3eae9bcf270dc8fa8da6e6fceb7926/image-16.jpg)
// Driver. java public static void main( String[] args ) { Bank. Account a = new Bank. Account(); Bank. Account b = new Bank. Account(); b. deposit( 100 ); } Since the Bank. Account class was already loaded in the method area, no other loading happens args[0] * a main() 100 * Bank. Account() … b Parameters Local variables A pointer to the Frame data Bank. Account pointer in the heap is Operand stack created (Constant Pool Resolution) Parameters Local variables Frame data Operand stack Parameters Driver class Bank. Account class Methods main() Constant Pool “Bank. Account” a “Bank. Account” b 100 A stack frame for the Bank. Account Constructor is pointer pushed into the Java stack Methods Bank. Account() deposit( double ) Constant Pool 0 Static Variables total. Accounts pointer balance 0. 0 1 Method Area Heap A pointer to the Bank. Account class data is created
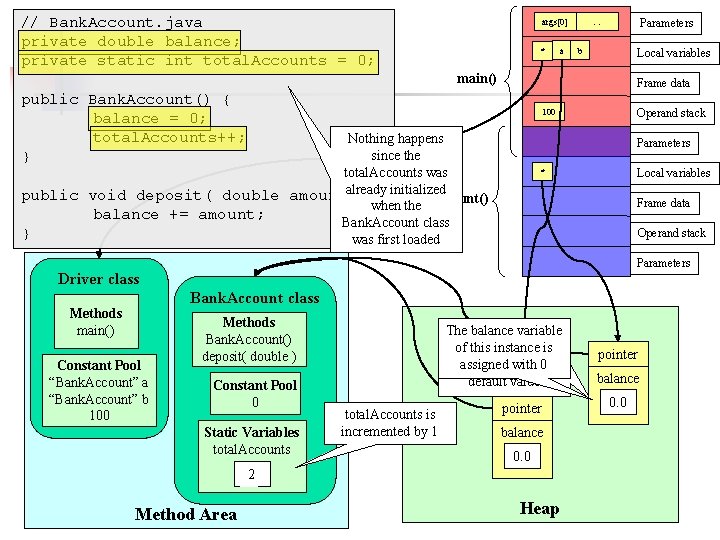
// Bank. Account. java private double balance; private static int total. Accounts = 0; args[0] * a Parameters … b Local variables main() Frame data public Bank. Account() { balance = 0; total. Accounts++; } Operand stack 100 Nothing happens since the total. Accounts was public void deposit( double amountalready ) { initialized Bank. Account() when the balance += amount; Bank. Account class } was first loaded Parameters Local variables * Frame data Operand stack Parameters Driver class Bank. Account class Methods main() Constant Pool “Bank. Account” a “Bank. Account” b 100 Methods Bank. Account() deposit( double ) Constant Pool 0 Static Variables total. Accounts The balance variable of this instance is initialized assigned with 0 a default value total. Accounts is incremented by 1 pointer balance 0. 0 21 Method Area Heap pointer balance 0. 0
![Driver java public static void main String args Bank Account a // Driver. java public static void main( String[] args ) { Bank. Account a](https://slidetodoc.com/presentation_image_h/dc3eae9bcf270dc8fa8da6e6fceb7926/image-18.jpg)
// Driver. java public static void main( String[] args ) { Bank. Account a = new Bank. Account(); Bank. Account b = new Bank. Account(); b. deposit( 100 ); } args[0] * Parameters … a b Local variables main() Frame data 100 The pointer is popped from the operand stack and assigned to b Operand stack * Parameters * Bank. Account() The pointer is Local variables returned to the calling frame Frame data Operand stack Driver class Bank. Account class Methods main() Constant Pool “Bank. Account” a “Bank. Account” b 100 Methods Bank. Account() deposit( double ) Constant Pool 0 Static Variables total. Accounts The stack frame for the Bank. Account Constructor is popped from the Java stack pointer balance 0. 0 2 Method Area Parameters Heap 0. 0
![Driver java public static void main String args Bank Account a // Driver. java public static void main( String[] args ) { Bank. Account a](https://slidetodoc.com/presentation_image_h/dc3eae9bcf270dc8fa8da6e6fceb7926/image-19.jpg)
// Driver. java public static void main( String[] args ) { Bank. Account a = new Bank. Account(); Bank. Account b = new Bank. Account(); b. deposit( 100 ); } args[0] * a Methods main() Constant Pool “Bank. Account” a “Bank. Account” b 100 Static Variables total. Accounts Operand stack amount=100 Parameters The object reference to the instance is always put as the first local variable of a stack frame Local variables 100 is popped from the operand Framestack data and put into the next frame’s Operand parameters stack Parameters pointer balance 0. 0 2 Method Area Local variables 100 Methods Bank. Account() deposit( double ) Constant Pool 0 Parameters Frame data deposit( double ) Bank. Account class b main() b Driver class … Heap A stack frame for the deposit method of instance ‘b’ is pointer pushed into the Java balance stack 0. 0
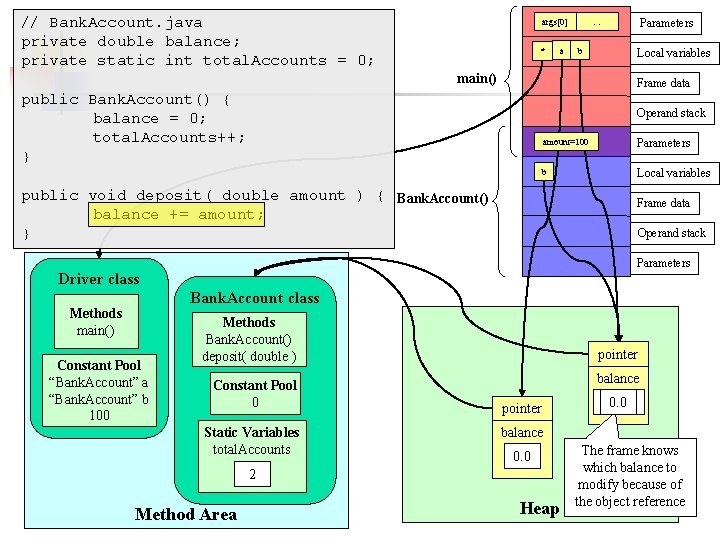
// Bank. Account. java private double balance; private static int total. Accounts = 0; args[0] * a Parameters … b Local variables main() Frame data public Bank. Account() { balance = 0; total. Accounts++; } Operand stack amount=100 Parameters b Local variables public void deposit( double amount ) { Bank. Account() balance += amount; } Frame data Operand stack Parameters Driver class Bank. Account class Methods main() Constant Pool “Bank. Account” a “Bank. Account” b 100 Methods Bank. Account() deposit( double ) Constant Pool 0 Static Variables total. Accounts pointer balance 0. 0 2 Method Area 100. 0 Heap The frame knows which balance to modify because of the object reference
Activation tree and activation record
Binding in programming paradigms
Ktu programming paradigms notes
R programming language paradigms
Activation record java
Activation record java
Real-time systems and programming languages
Cs 421 programming languages and compilers
Advantages and disadvantages of programming languages
Real time programming language
Cs 421 uiuc
Multithreading program in java
Programming languages levels
Introduction to programming languages
Plc programming languages
Procedural programming languages
Imperative programming languages
Alternative programming languages
Strongly typed vs weakly typed
Transmission programming languages
Adam doupe cse 340