Programming Languages and Paradigms The C Programming Language
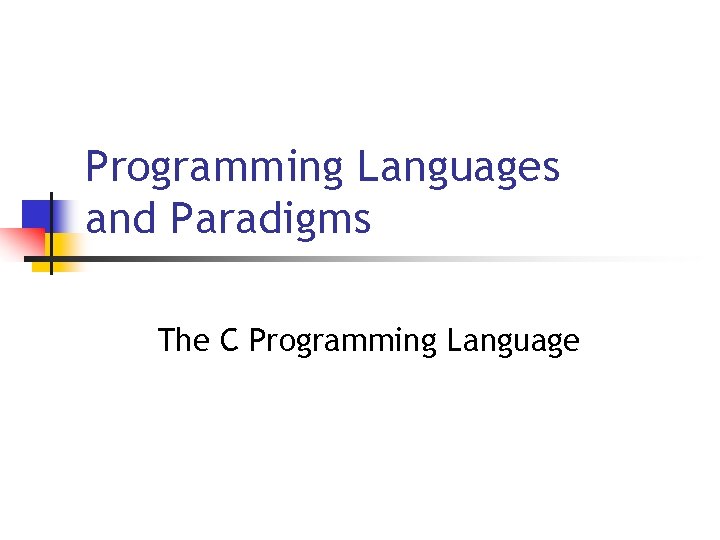
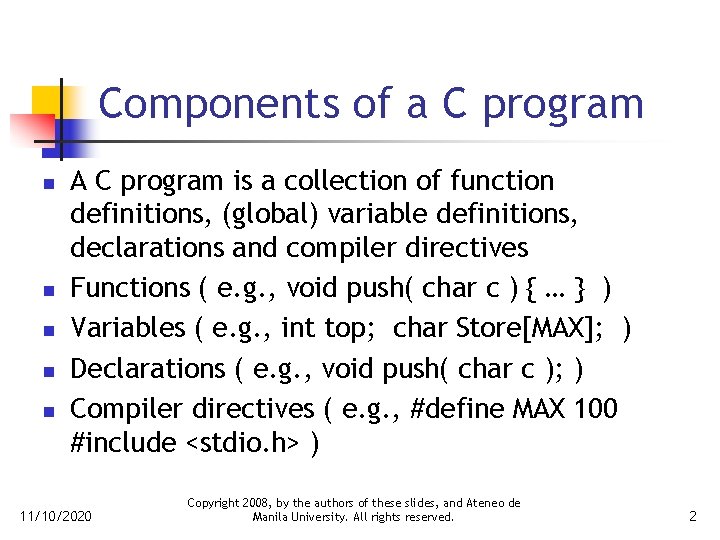
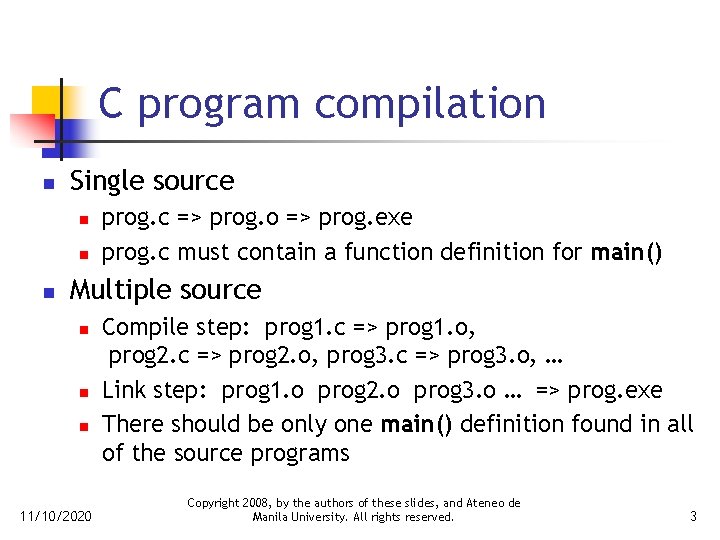
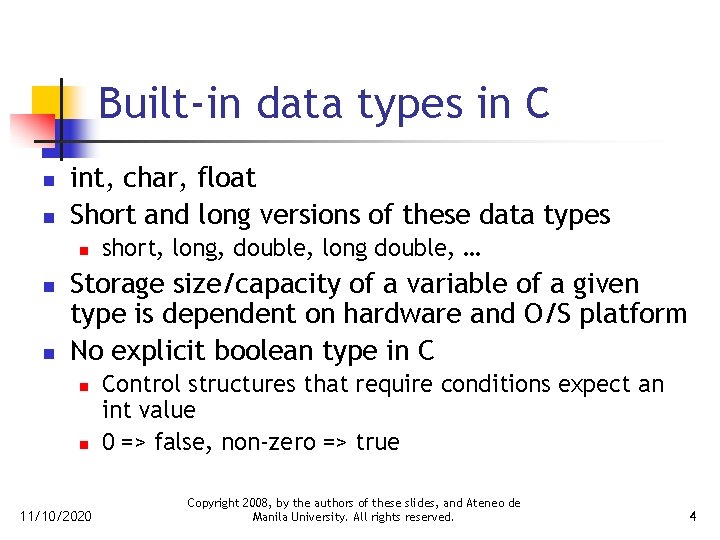
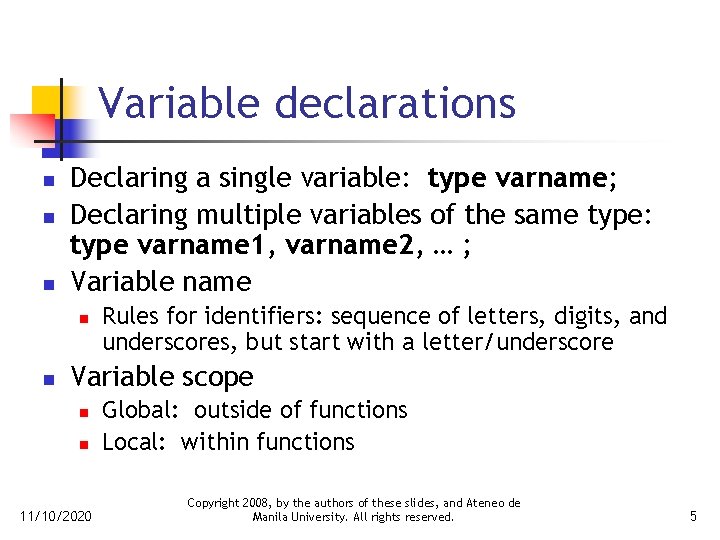
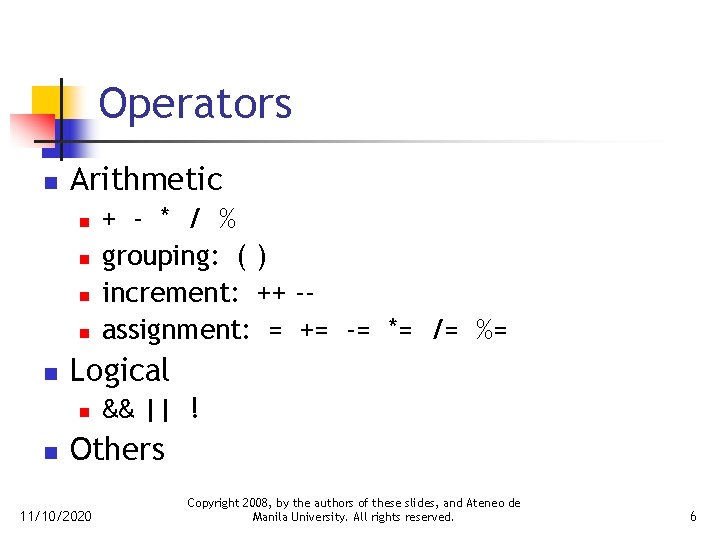
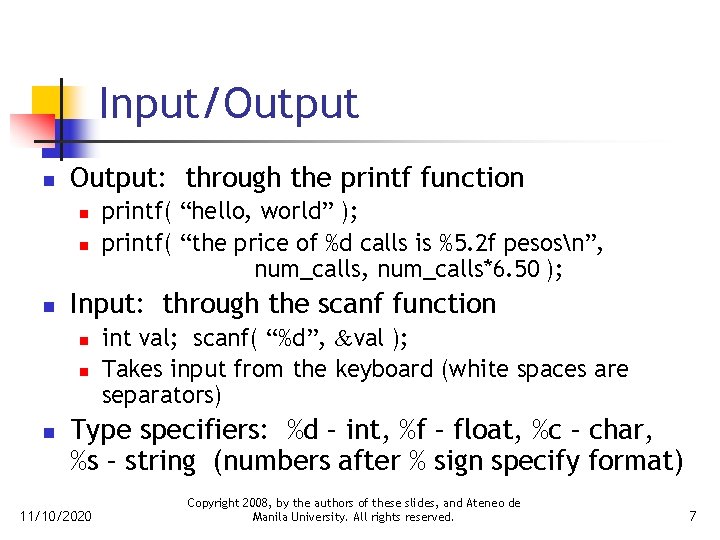
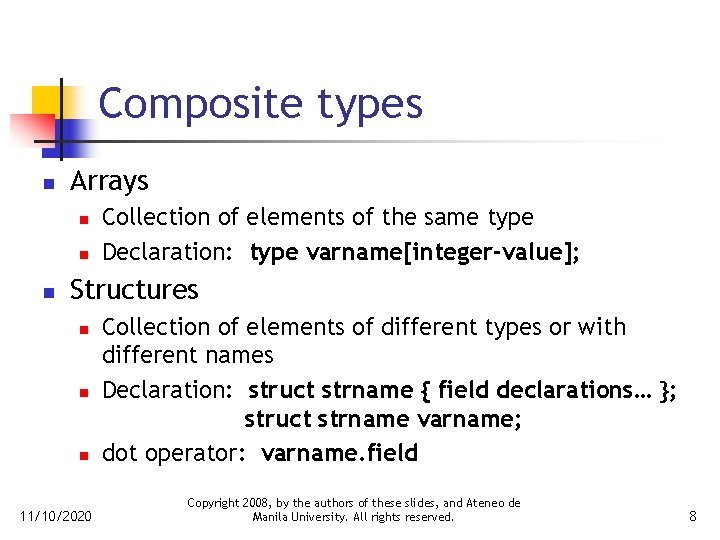
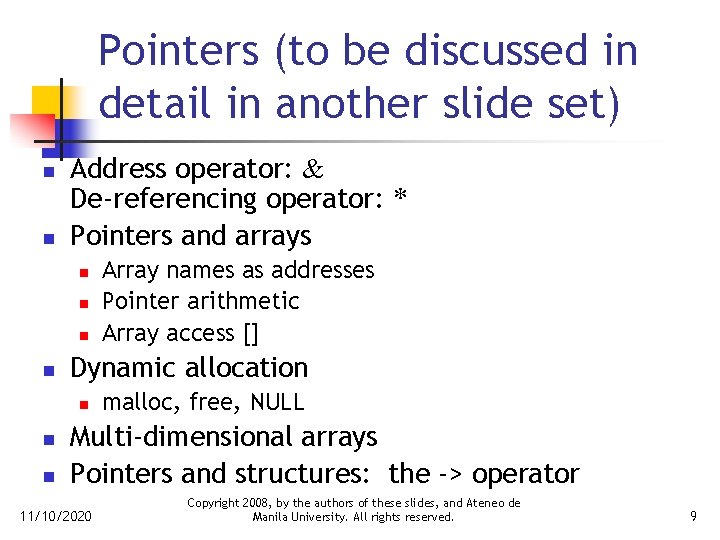
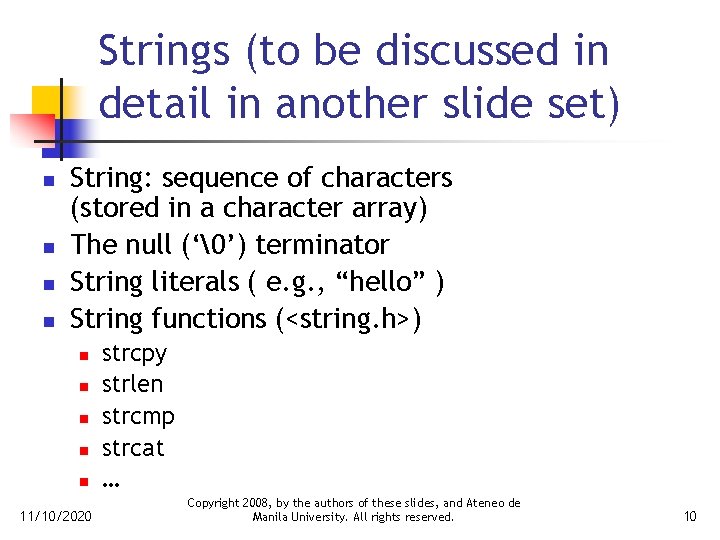
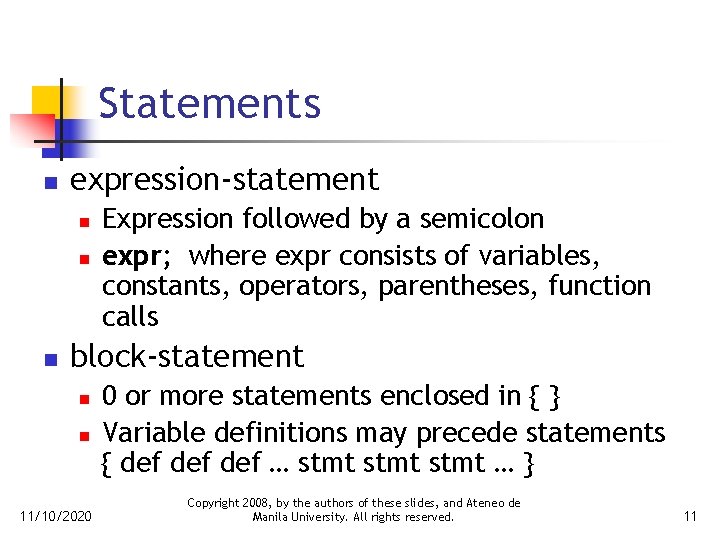
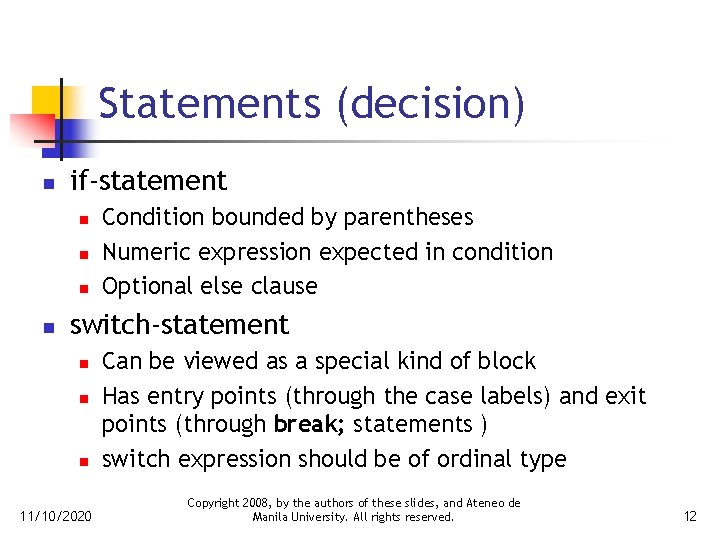
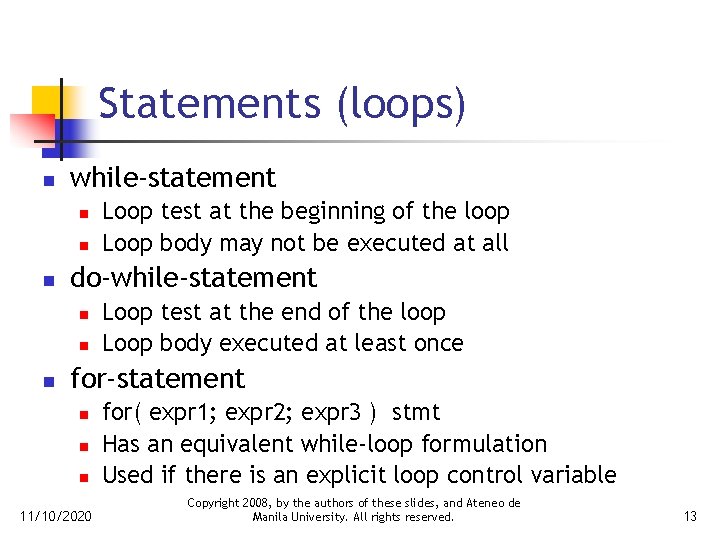
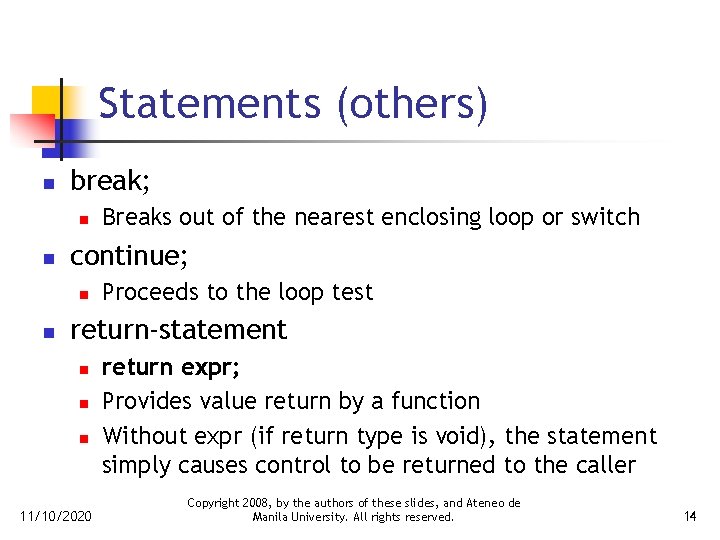
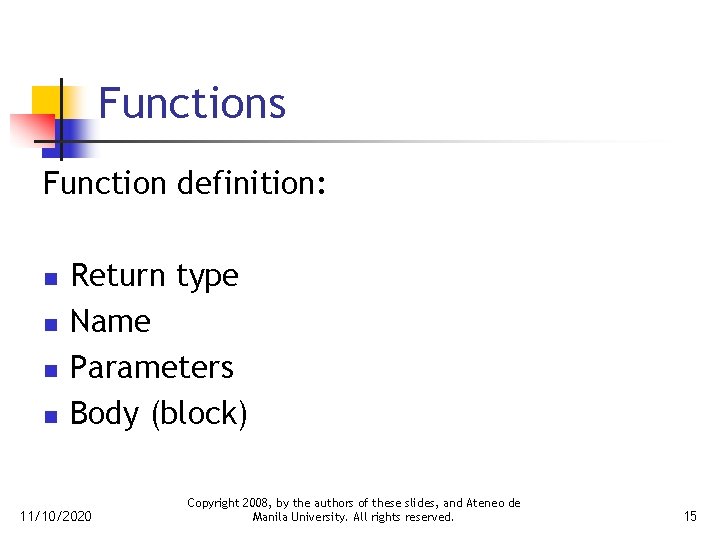
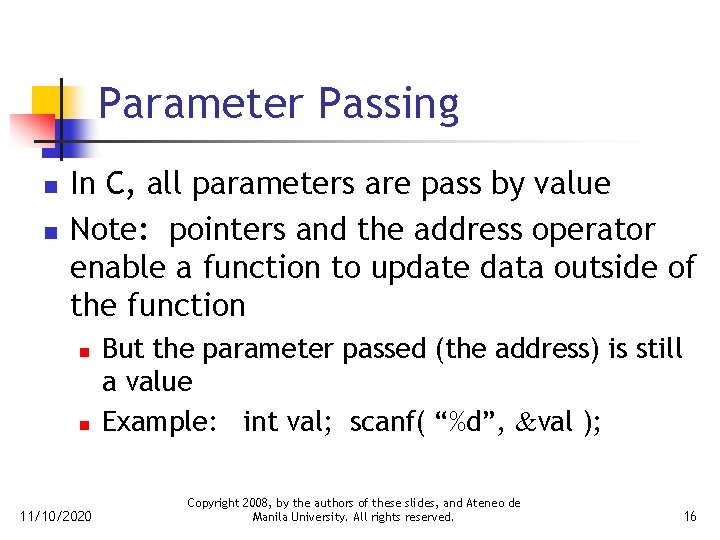
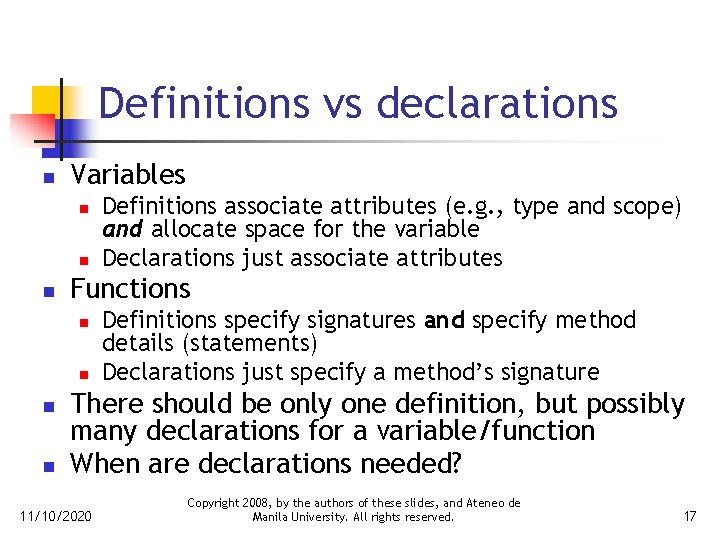
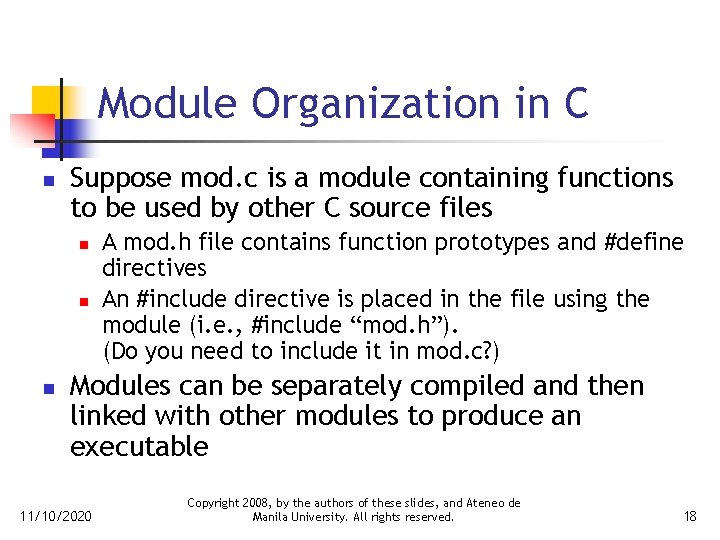
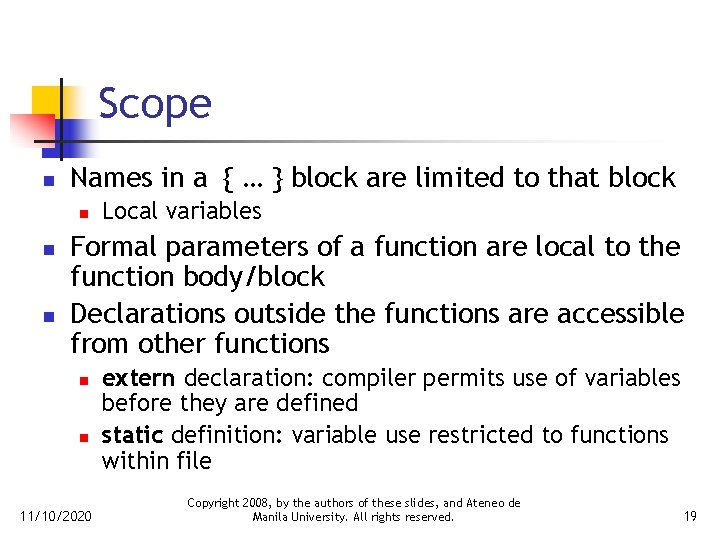
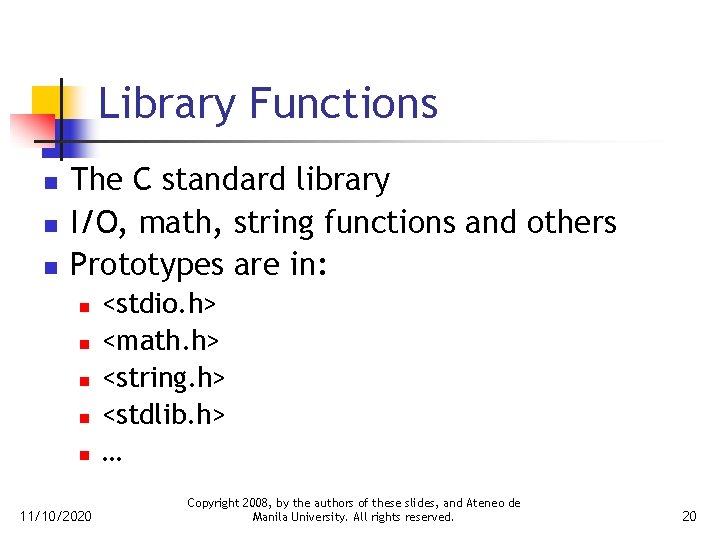
- Slides: 20
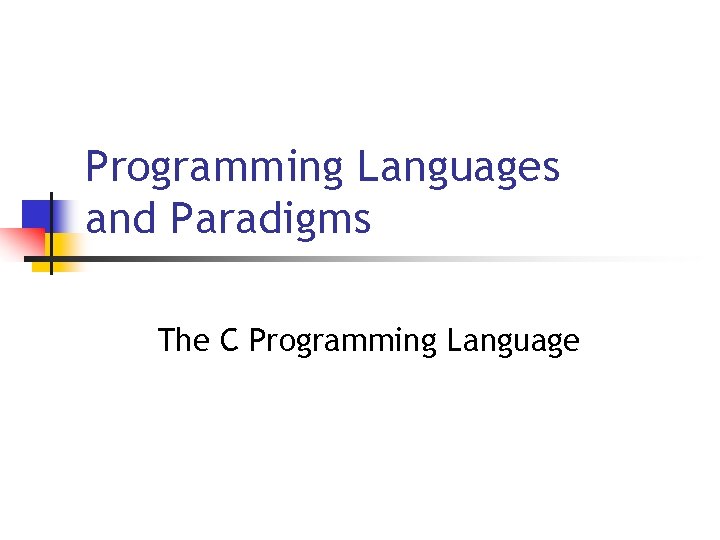
Programming Languages and Paradigms The C Programming Language
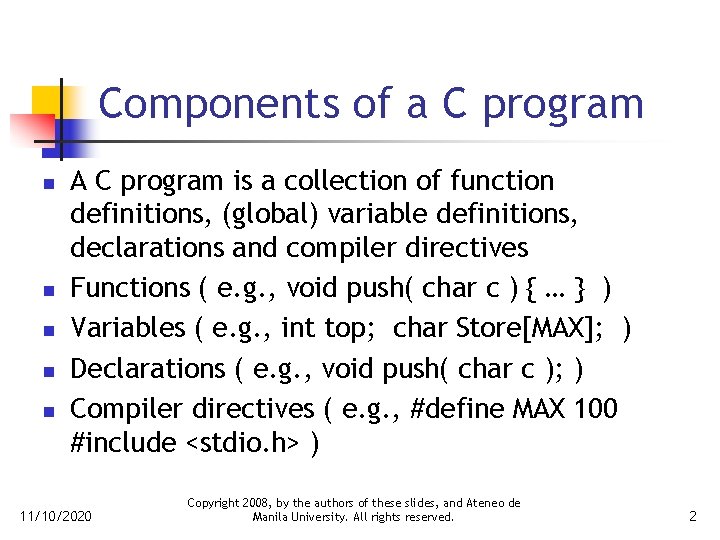
Components of a C program n n n A C program is a collection of function definitions, (global) variable definitions, declarations and compiler directives Functions ( e. g. , void push( char c ) { … } ) Variables ( e. g. , int top; char Store[MAX]; ) Declarations ( e. g. , void push( char c ); ) Compiler directives ( e. g. , #define MAX 100 #include <stdio. h> ) 11/10/2020 Copyright 2008, by the authors of these slides, and Ateneo de Manila University. All rights reserved. 2
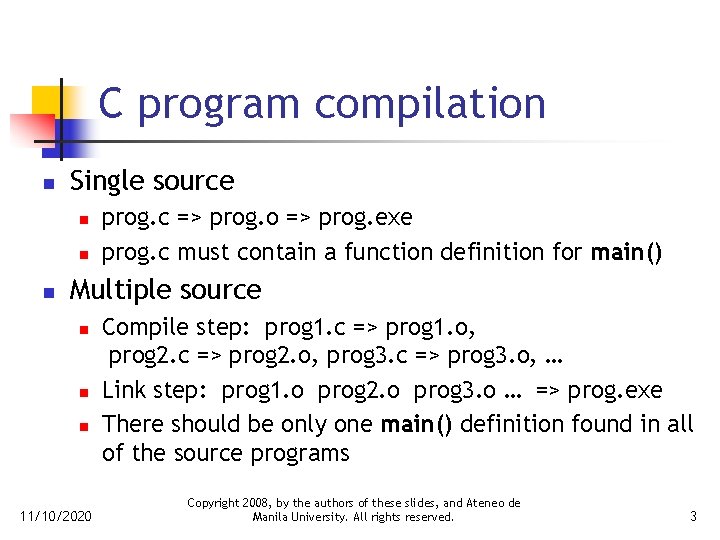
C program compilation n Single source n n n prog. c => prog. o => prog. exe prog. c must contain a function definition for main() Multiple source n n n 11/10/2020 Compile step: prog 1. c => prog 1. o, prog 2. c => prog 2. o, prog 3. c => prog 3. o, … Link step: prog 1. o prog 2. o prog 3. o … => prog. exe There should be only one main() definition found in all of the source programs Copyright 2008, by the authors of these slides, and Ateneo de Manila University. All rights reserved. 3
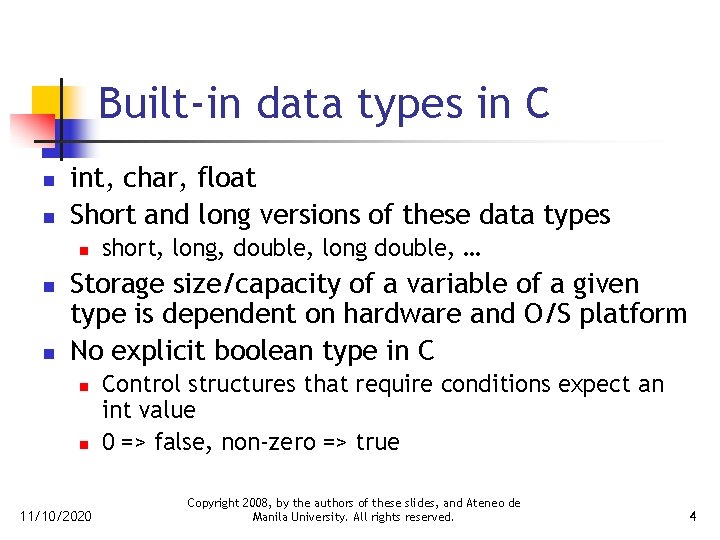
Built-in data types in C n n int, char, float Short and long versions of these data types n n n short, long, double, long double, … Storage size/capacity of a variable of a given type is dependent on hardware and O/S platform No explicit boolean type in C n n 11/10/2020 Control structures that require conditions expect an int value 0 => false, non-zero => true Copyright 2008, by the authors of these slides, and Ateneo de Manila University. All rights reserved. 4
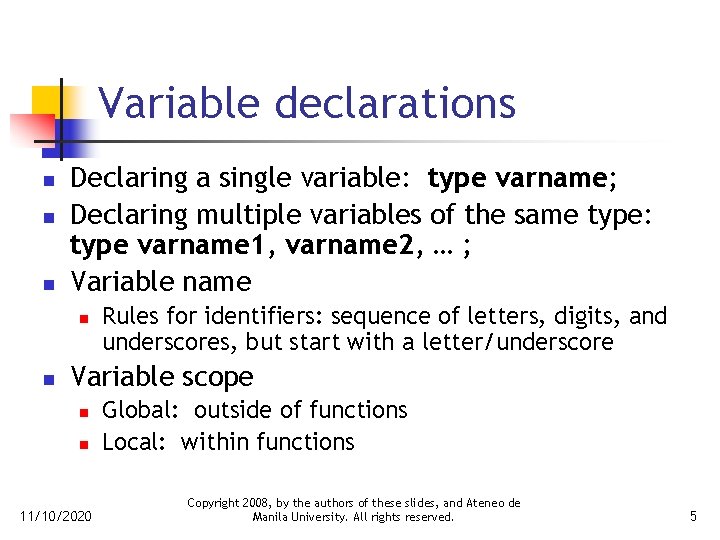
Variable declarations n n n Declaring a single variable: type varname; Declaring multiple variables of the same type: type varname 1, varname 2, … ; Variable name n n Rules for identifiers: sequence of letters, digits, and underscores, but start with a letter/underscore Variable scope n n 11/10/2020 Global: outside of functions Local: within functions Copyright 2008, by the authors of these slides, and Ateneo de Manila University. All rights reserved. 5
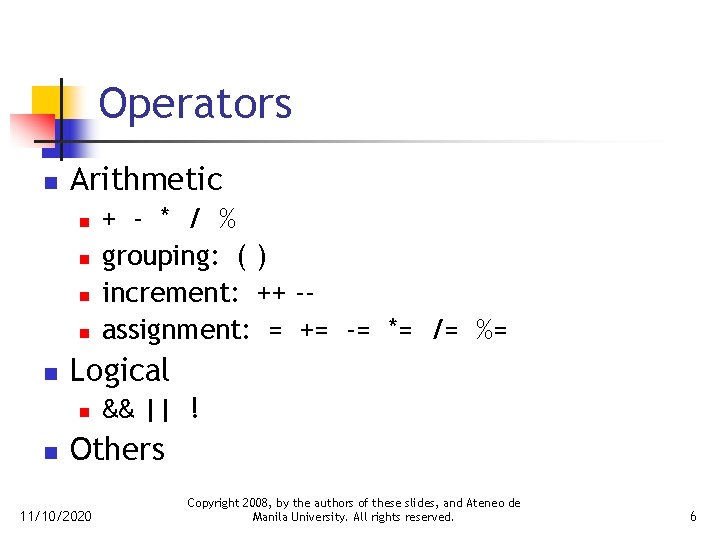
Operators n Arithmetic n n n Logical n n + - * / % grouping: ( ) increment: ++ -assignment: = += -= *= /= %= && || ! Others 11/10/2020 Copyright 2008, by the authors of these slides, and Ateneo de Manila University. All rights reserved. 6
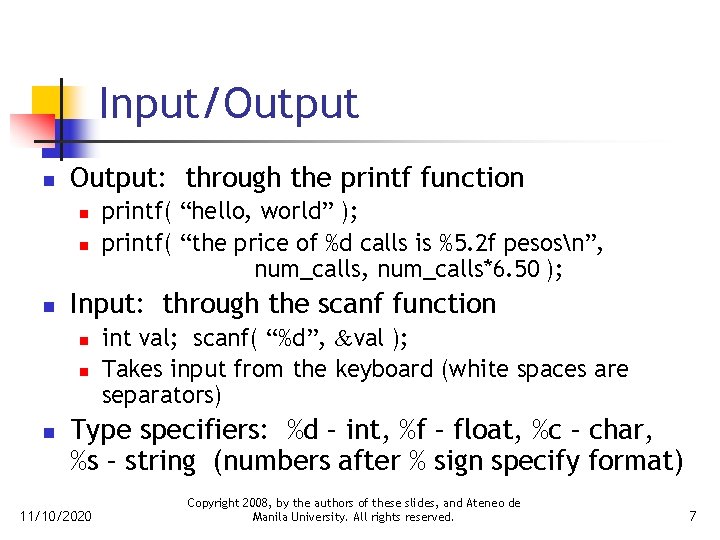
Input/Output n Output: through the printf function n Input: through the scanf function n printf( “hello, world” ); printf( “the price of %d calls is %5. 2 f pesosn”, num_calls*6. 50 ); int val; scanf( “%d”, &val ); Takes input from the keyboard (white spaces are separators) Type specifiers: %d – int, %f – float, %c – char, %s – string (numbers after % sign specify format) 11/10/2020 Copyright 2008, by the authors of these slides, and Ateneo de Manila University. All rights reserved. 7
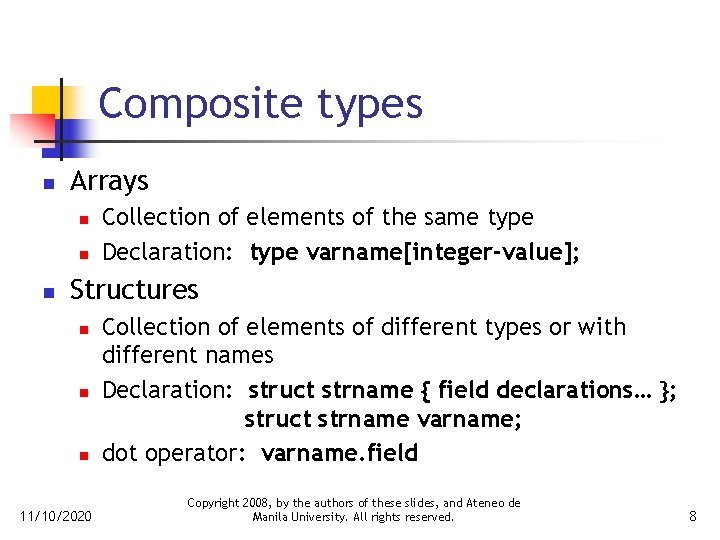
Composite types n Arrays n n n Collection of elements of the same type Declaration: type varname[integer-value]; Structures n n n 11/10/2020 Collection of elements of different types or with different names Declaration: struct strname { field declarations… }; struct strname varname; dot operator: varname. field Copyright 2008, by the authors of these slides, and Ateneo de Manila University. All rights reserved. 8
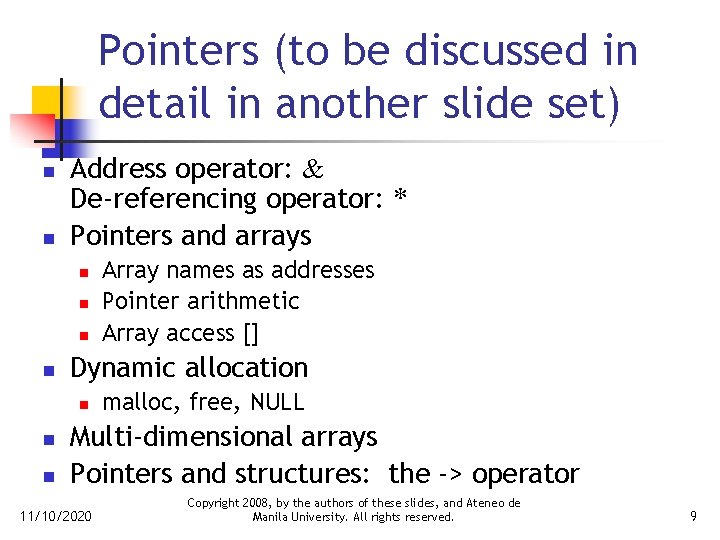
Pointers (to be discussed in detail in another slide set) n n Address operator: & De-referencing operator: * Pointers and arrays n n Dynamic allocation n Array names as addresses Pointer arithmetic Array access [] malloc, free, NULL Multi-dimensional arrays Pointers and structures: the -> operator 11/10/2020 Copyright 2008, by the authors of these slides, and Ateneo de Manila University. All rights reserved. 9
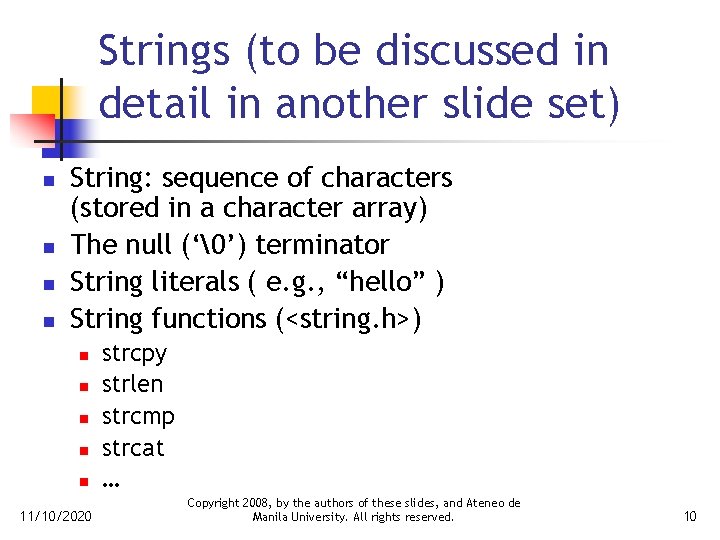
Strings (to be discussed in detail in another slide set) n n String: sequence of characters (stored in a character array) The null (‘ ’) terminator String literals ( e. g. , “hello” ) String functions (<string. h>) n n n 11/10/2020 strcpy strlen strcmp strcat … Copyright 2008, by the authors of these slides, and Ateneo de Manila University. All rights reserved. 10
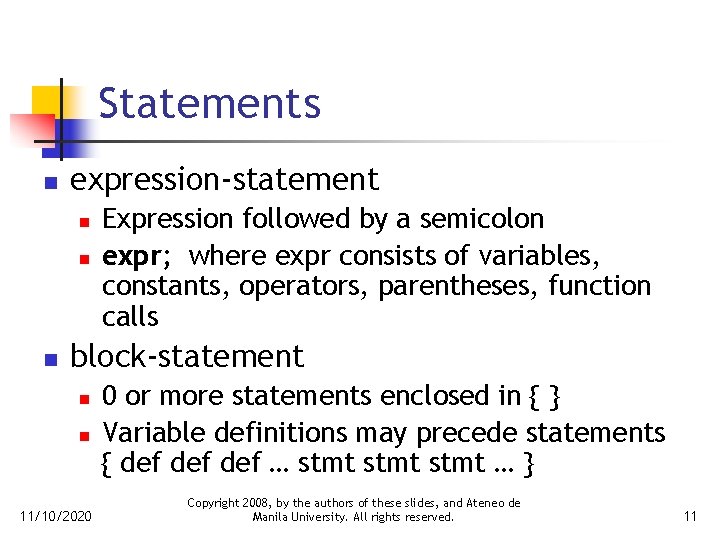
Statements n expression-statement n n n Expression followed by a semicolon expr; where expr consists of variables, constants, operators, parentheses, function calls block-statement n n 11/10/2020 0 or more statements enclosed in { } Variable definitions may precede statements { def def … stmt … } Copyright 2008, by the authors of these slides, and Ateneo de Manila University. All rights reserved. 11
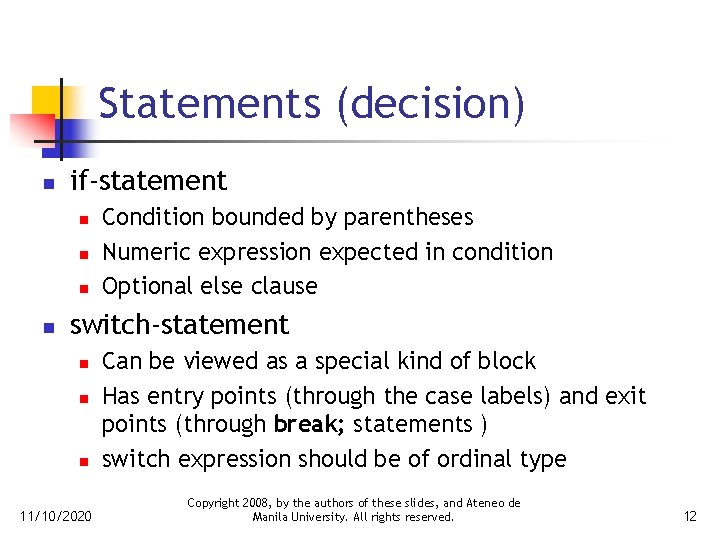
Statements (decision) n if-statement n n Condition bounded by parentheses Numeric expression expected in condition Optional else clause switch-statement n n n 11/10/2020 Can be viewed as a special kind of block Has entry points (through the case labels) and exit points (through break; statements ) switch expression should be of ordinal type Copyright 2008, by the authors of these slides, and Ateneo de Manila University. All rights reserved. 12
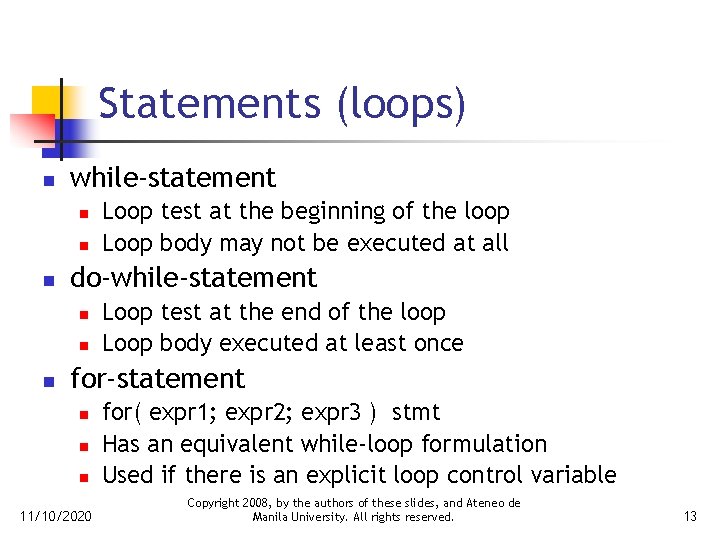
Statements (loops) n while-statement n n n do-while-statement n n n Loop test at the beginning of the loop Loop body may not be executed at all Loop test at the end of the loop Loop body executed at least once for-statement n n n 11/10/2020 for( expr 1; expr 2; expr 3 ) stmt Has an equivalent while-loop formulation Used if there is an explicit loop control variable Copyright 2008, by the authors of these slides, and Ateneo de Manila University. All rights reserved. 13
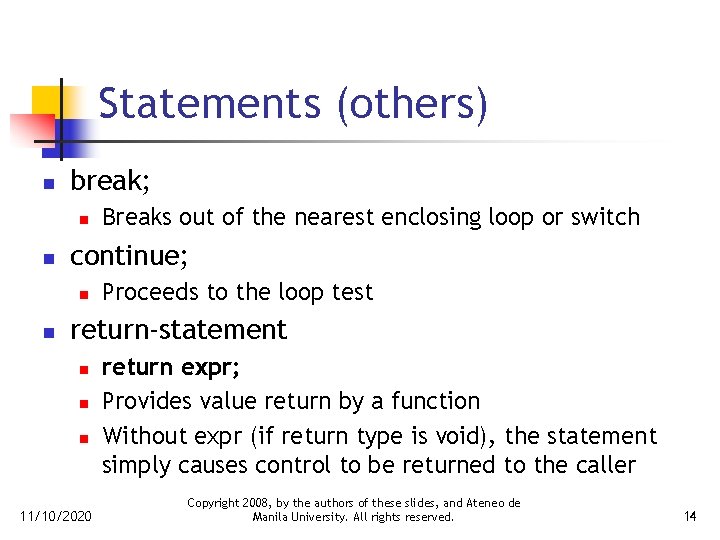
Statements (others) n break; n n continue; n n Breaks out of the nearest enclosing loop or switch Proceeds to the loop test return-statement n n n 11/10/2020 return expr; Provides value return by a function Without expr (if return type is void), the statement simply causes control to be returned to the caller Copyright 2008, by the authors of these slides, and Ateneo de Manila University. All rights reserved. 14
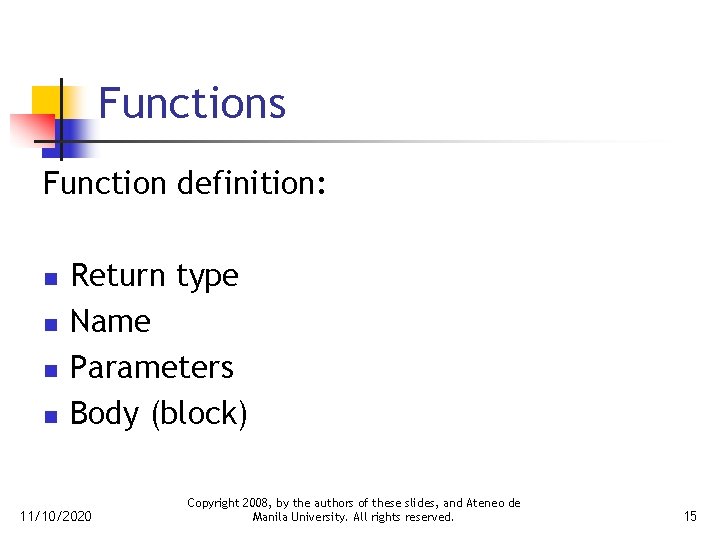
Functions Function definition: n n Return type Name Parameters Body (block) 11/10/2020 Copyright 2008, by the authors of these slides, and Ateneo de Manila University. All rights reserved. 15
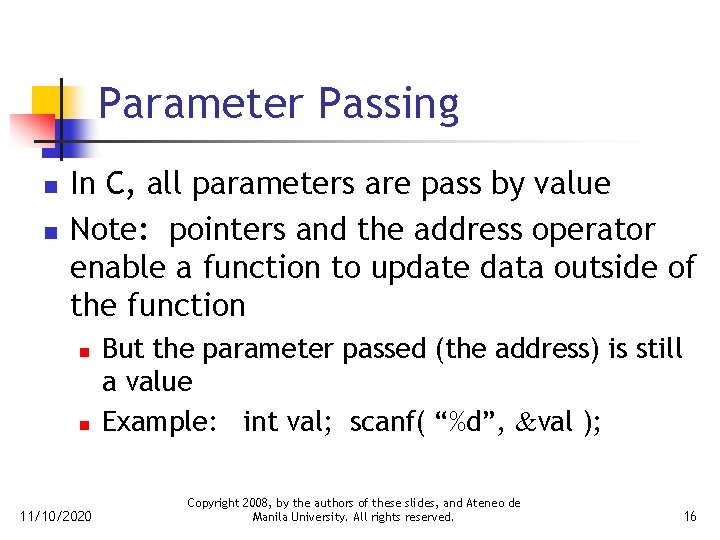
Parameter Passing n n In C, all parameters are pass by value Note: pointers and the address operator enable a function to update data outside of the function n n 11/10/2020 But the parameter passed (the address) is still a value Example: int val; scanf( “%d”, &val ); Copyright 2008, by the authors of these slides, and Ateneo de Manila University. All rights reserved. 16
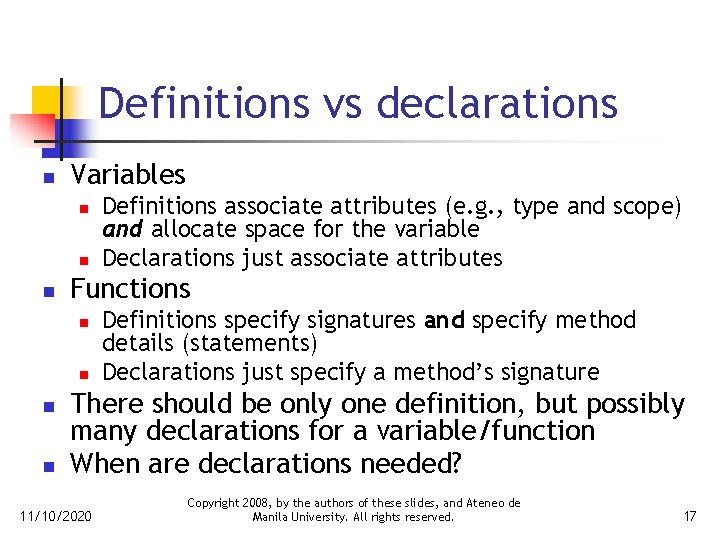
Definitions vs declarations n Variables n n n Functions n n Definitions associate attributes (e. g. , type and scope) and allocate space for the variable Declarations just associate attributes Definitions specify signatures and specify method details (statements) Declarations just specify a method’s signature There should be only one definition, but possibly many declarations for a variable/function When are declarations needed? 11/10/2020 Copyright 2008, by the authors of these slides, and Ateneo de Manila University. All rights reserved. 17
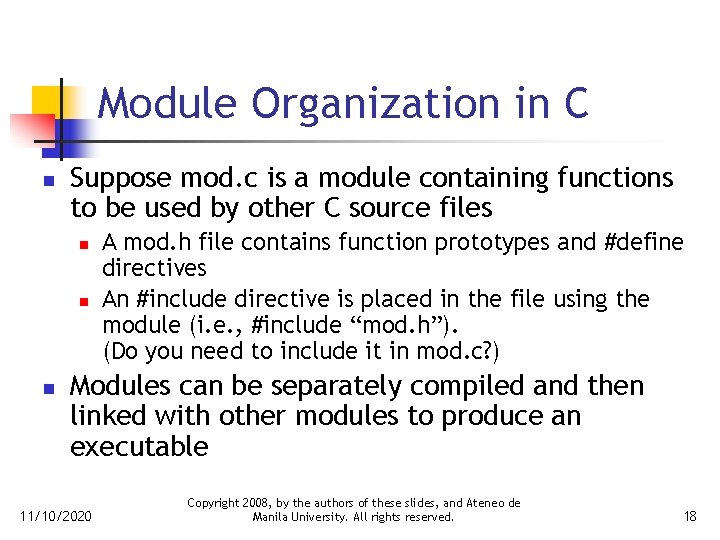
Module Organization in C n Suppose mod. c is a module containing functions to be used by other C source files n n n A mod. h file contains function prototypes and #define directives An #include directive is placed in the file using the module (i. e. , #include “mod. h”). (Do you need to include it in mod. c? ) Modules can be separately compiled and then linked with other modules to produce an executable 11/10/2020 Copyright 2008, by the authors of these slides, and Ateneo de Manila University. All rights reserved. 18
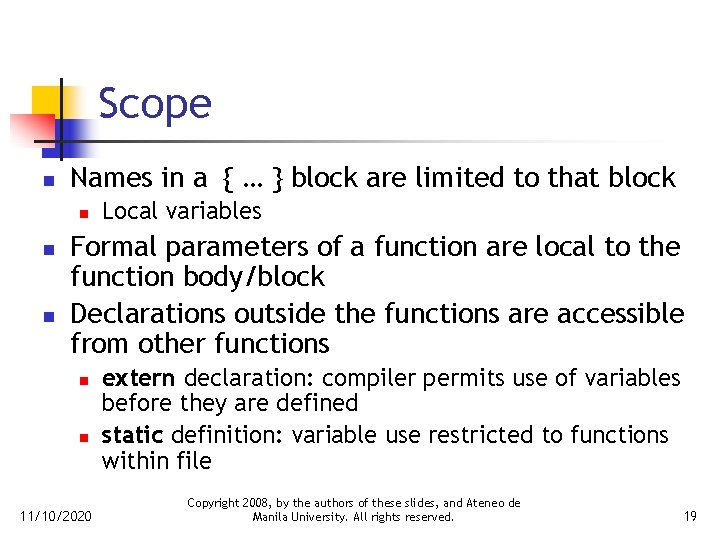
Scope n Names in a { … } block are limited to that block n n n Local variables Formal parameters of a function are local to the function body/block Declarations outside the functions are accessible from other functions n n 11/10/2020 extern declaration: compiler permits use of variables before they are defined static definition: variable use restricted to functions within file Copyright 2008, by the authors of these slides, and Ateneo de Manila University. All rights reserved. 19
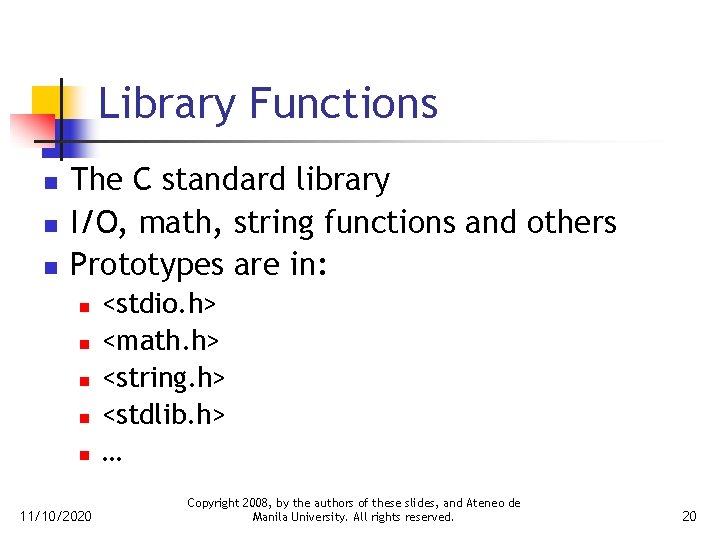
Library Functions n n n The C standard library I/O, math, string functions and others Prototypes are in: n n n 11/10/2020 <stdio. h> <math. h> <string. h> <stdlib. h> … Copyright 2008, by the authors of these slides, and Ateneo de Manila University. All rights reserved. 20