Programming Fundamentals Loops Its Logic l l What
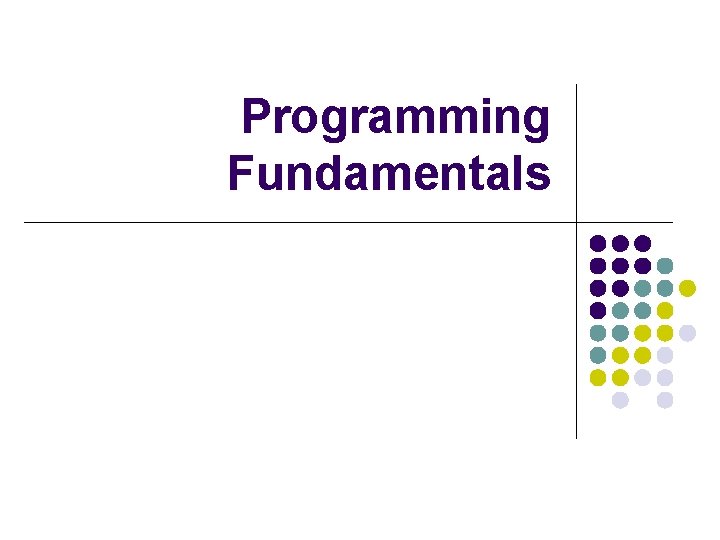
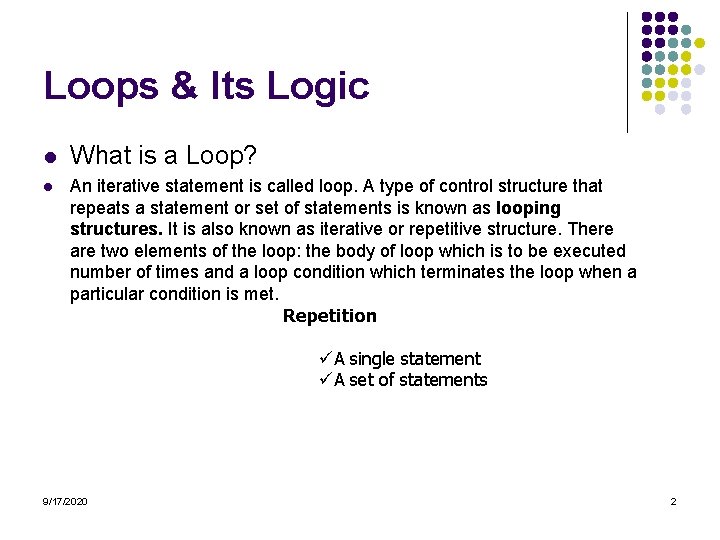
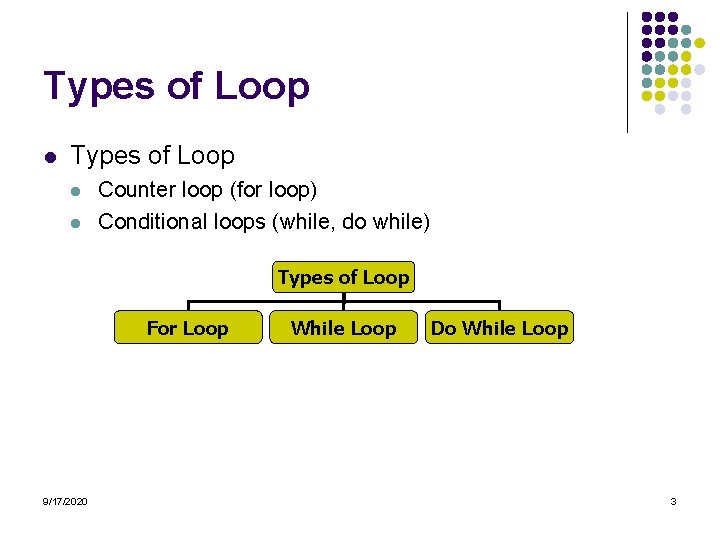
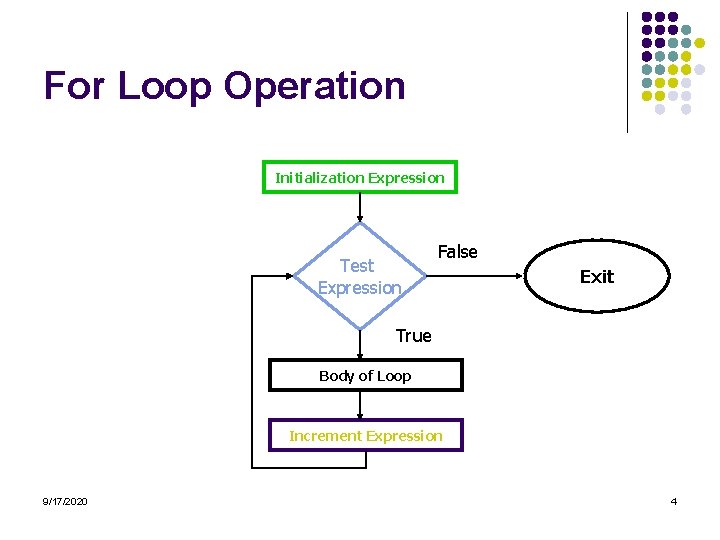
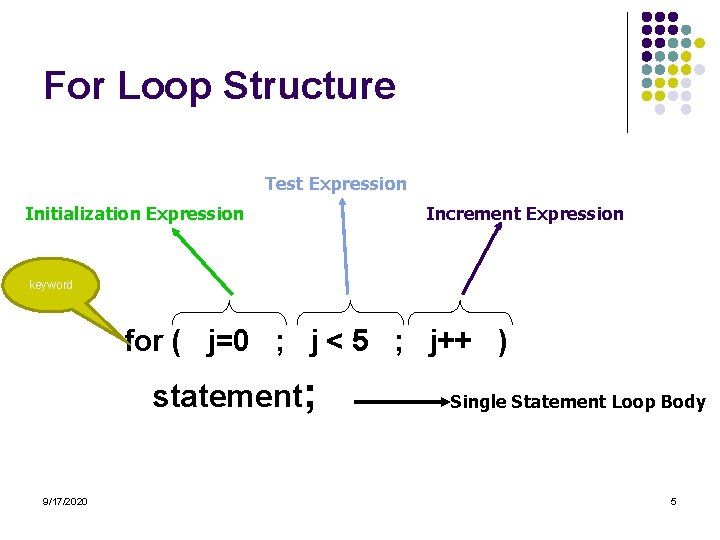
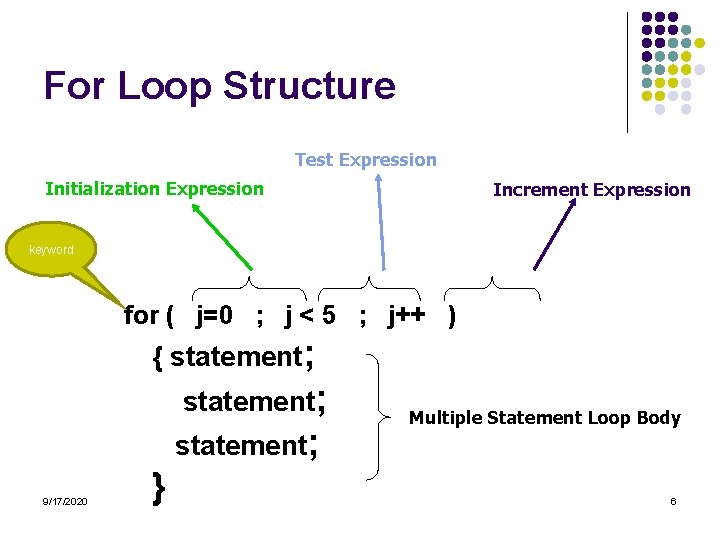
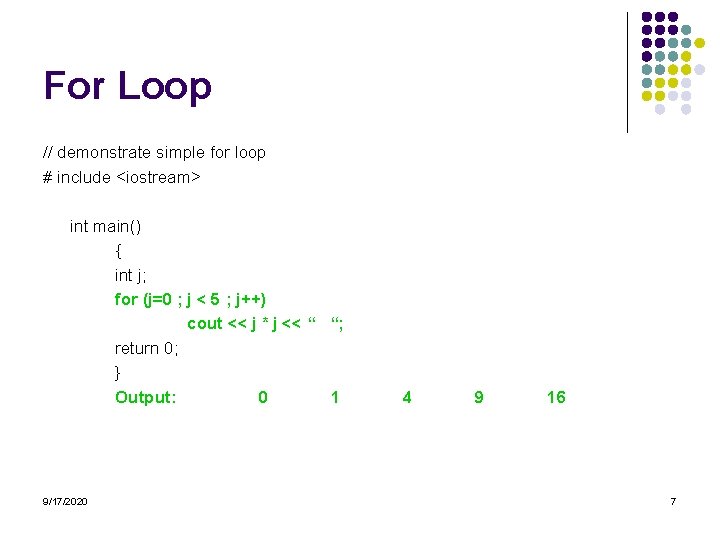
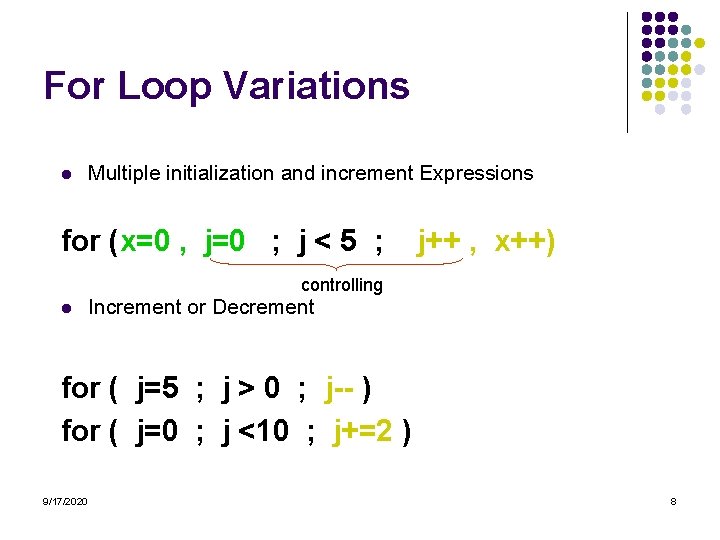
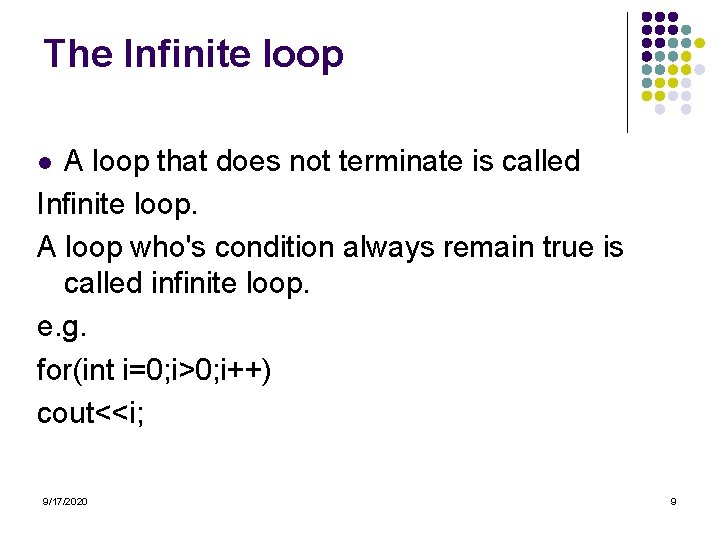
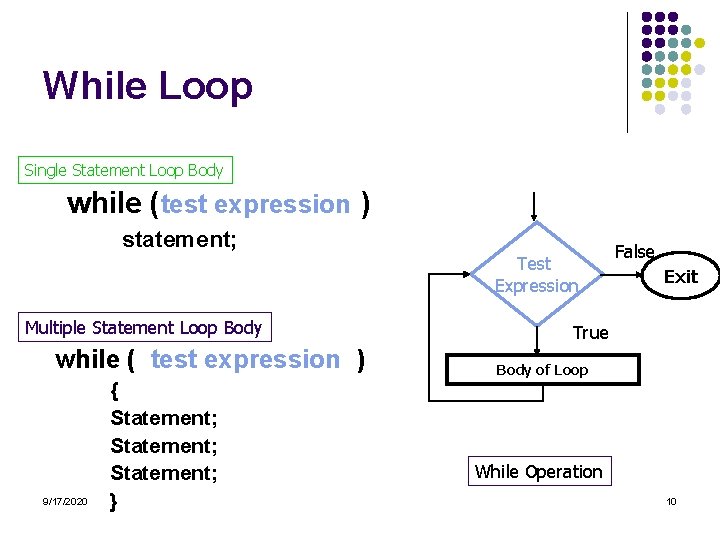
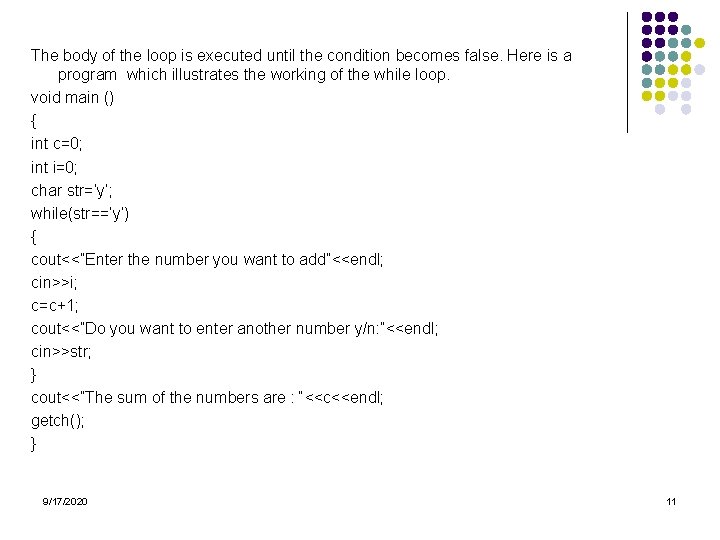
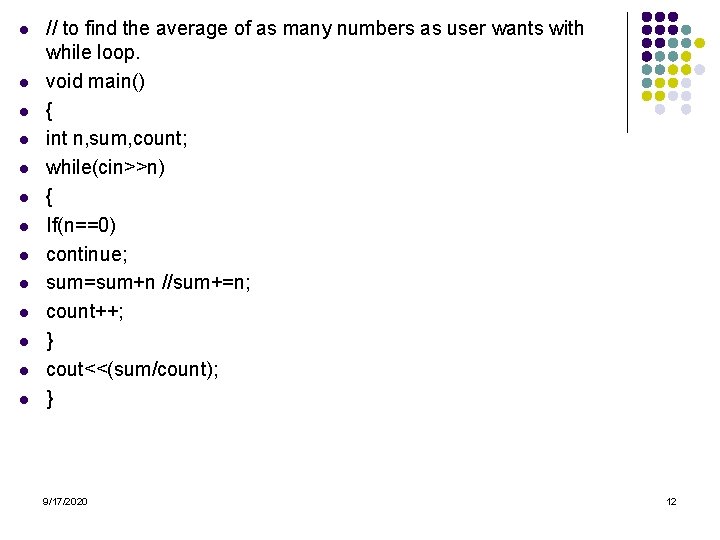
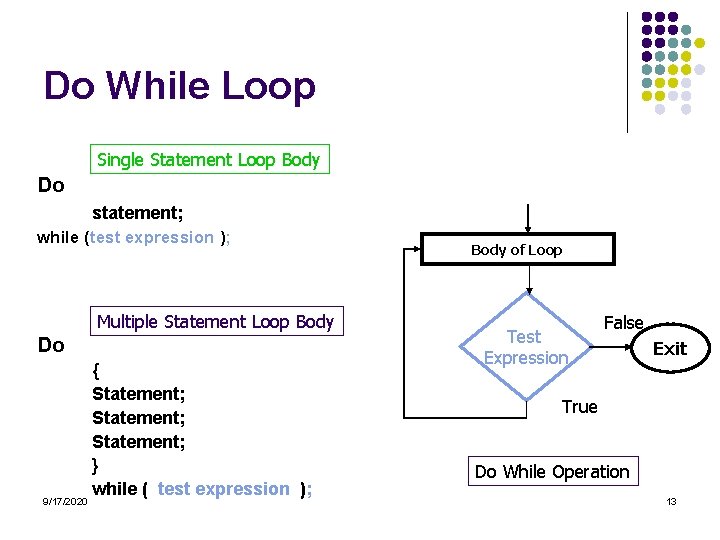
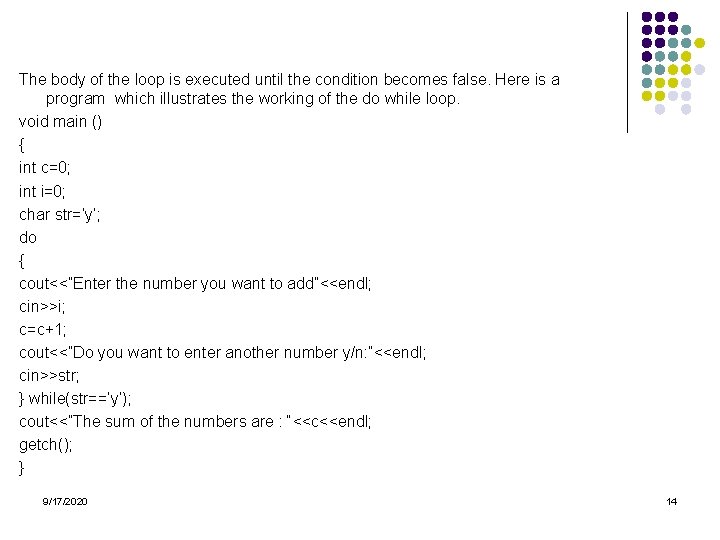
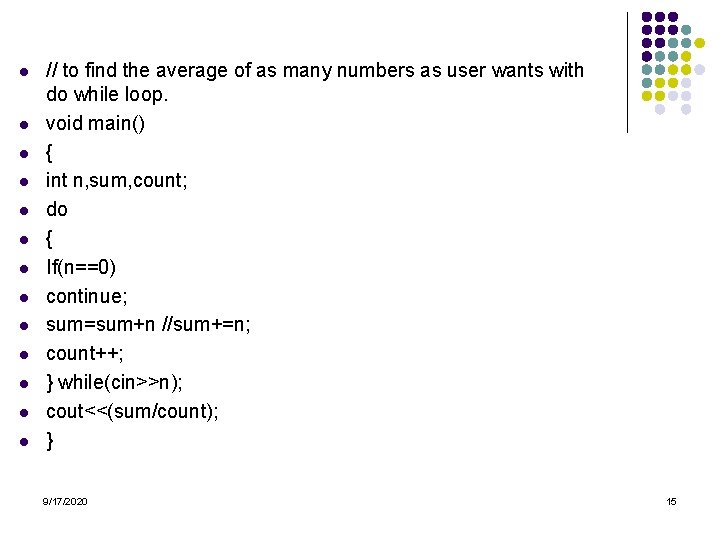
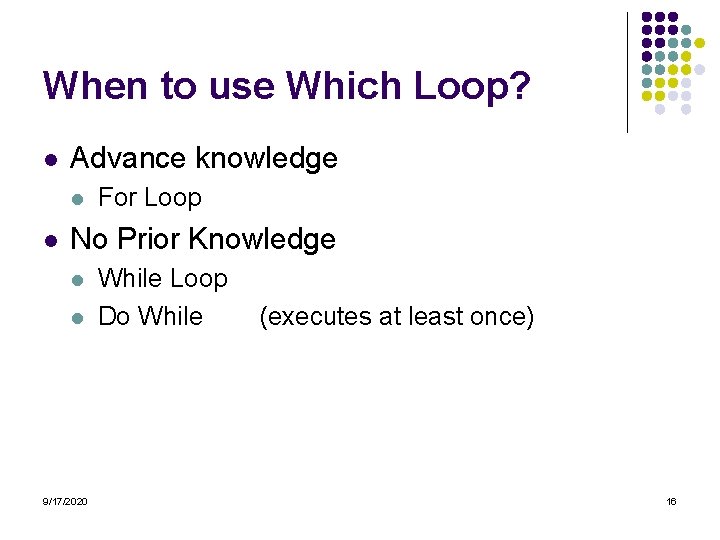
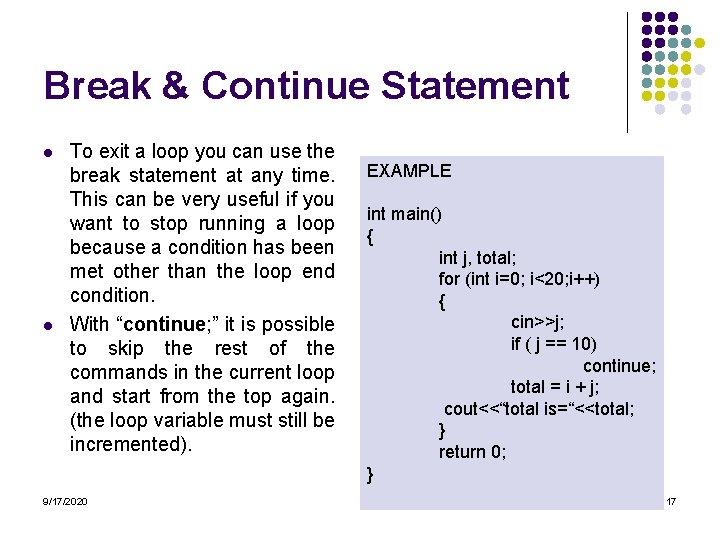
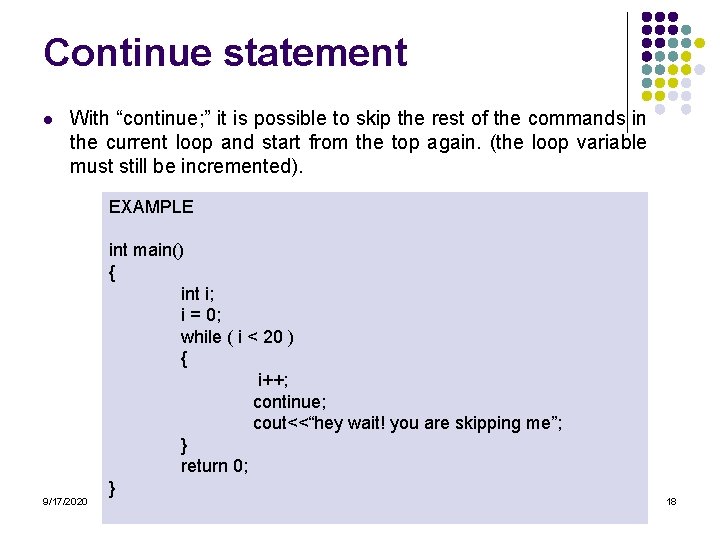
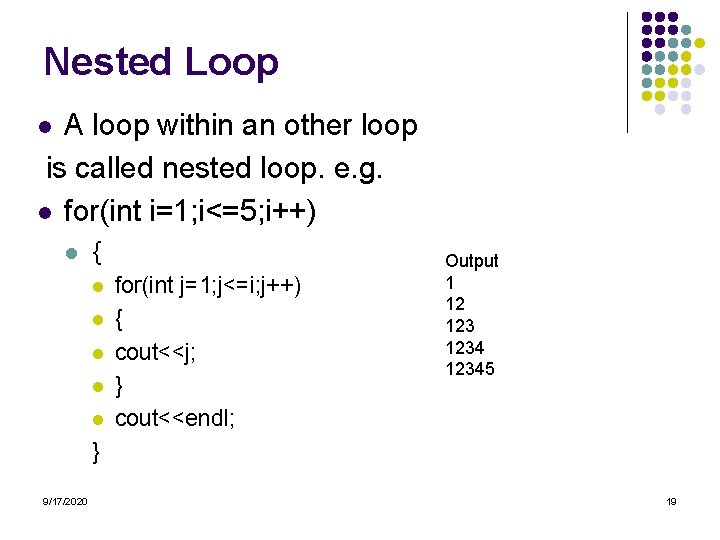
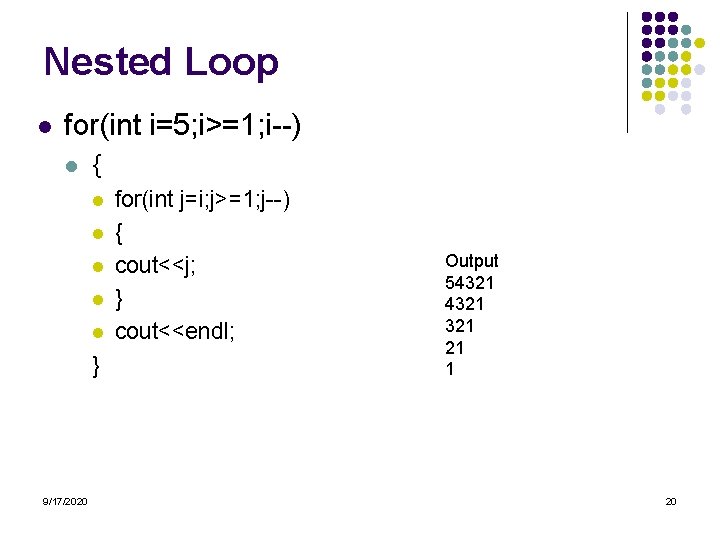
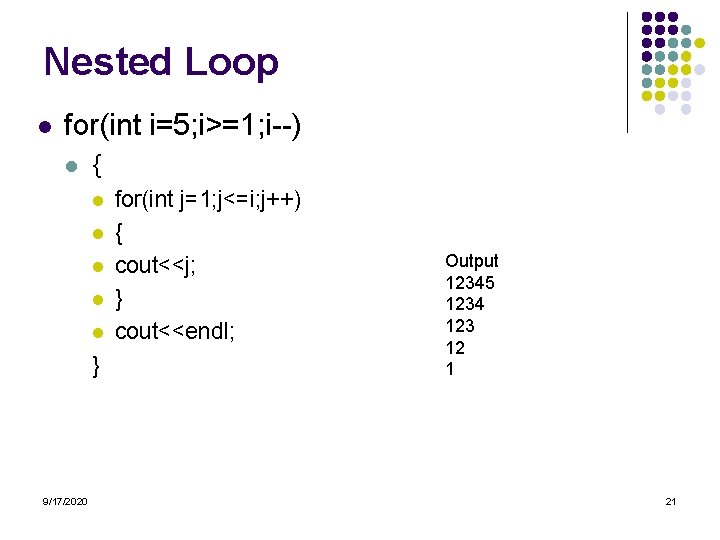
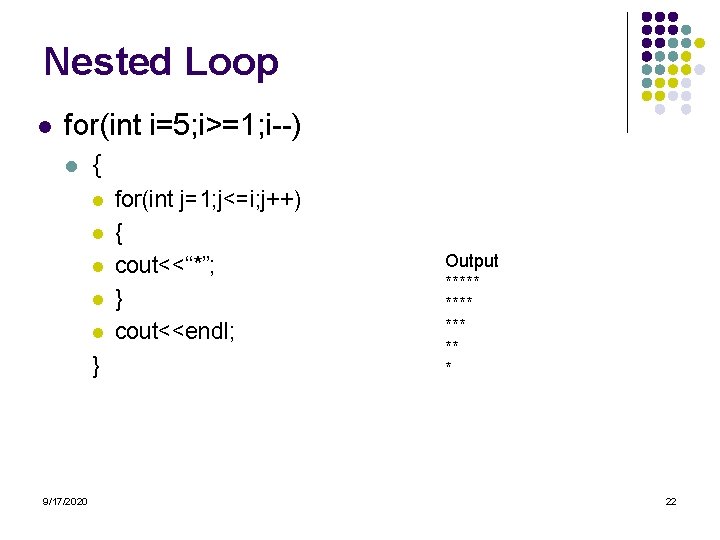
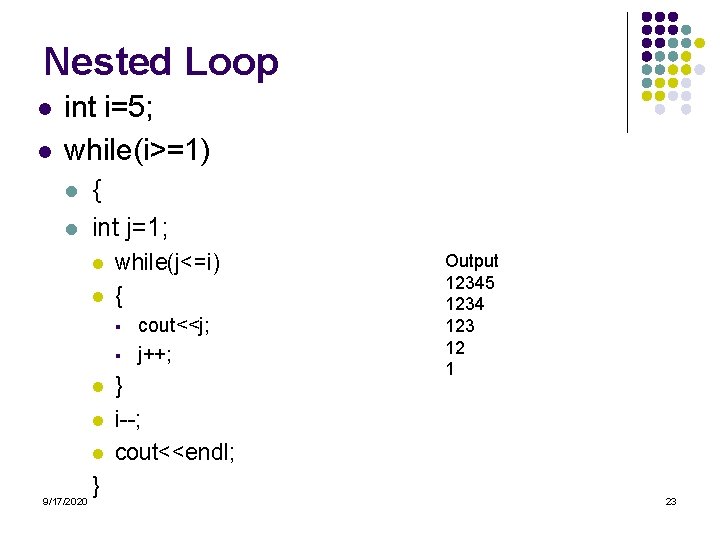
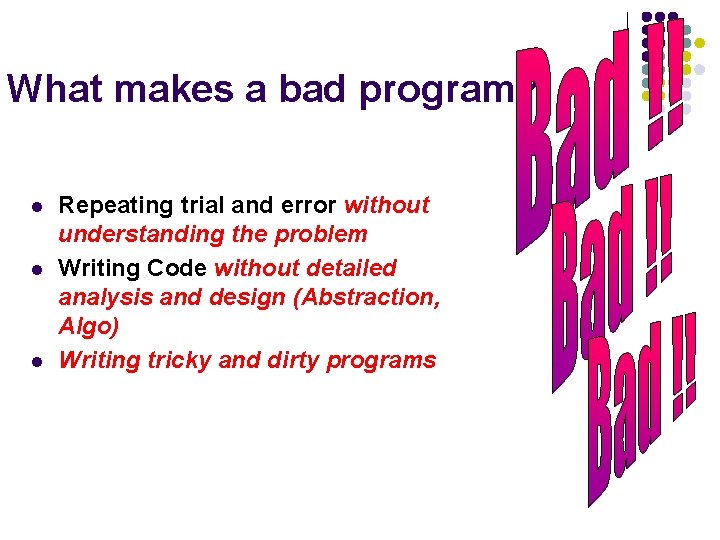
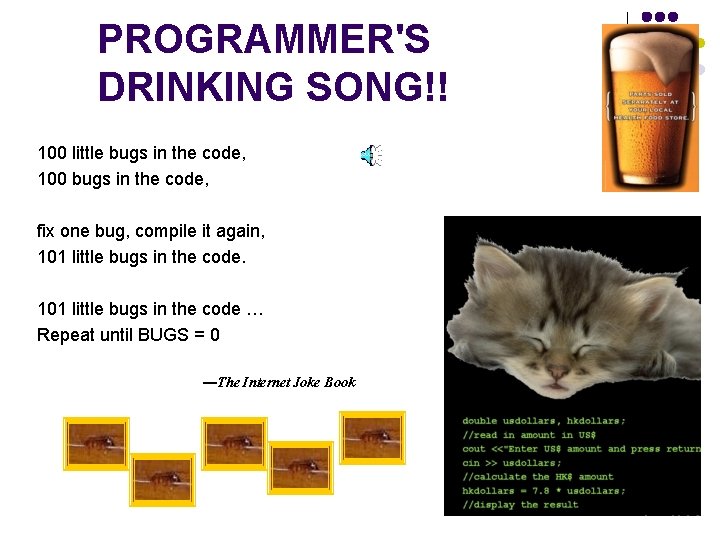
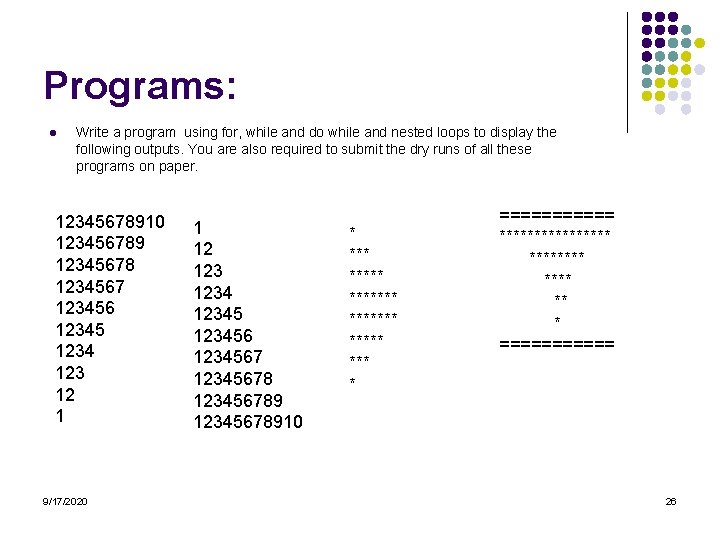
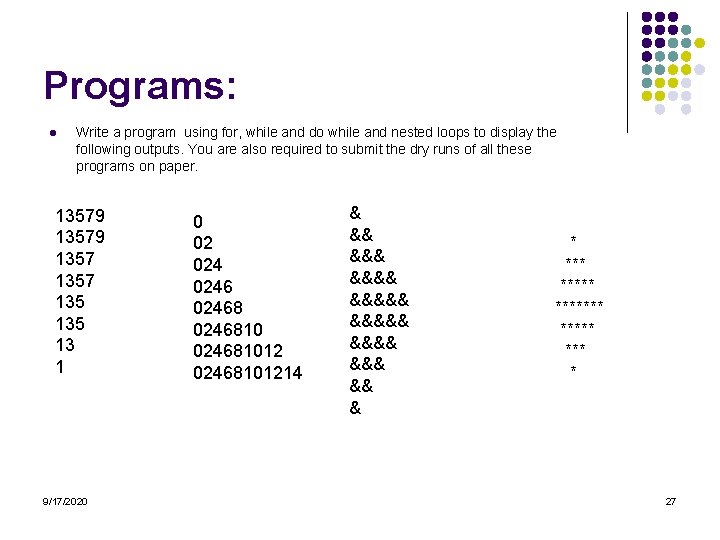
- Slides: 27
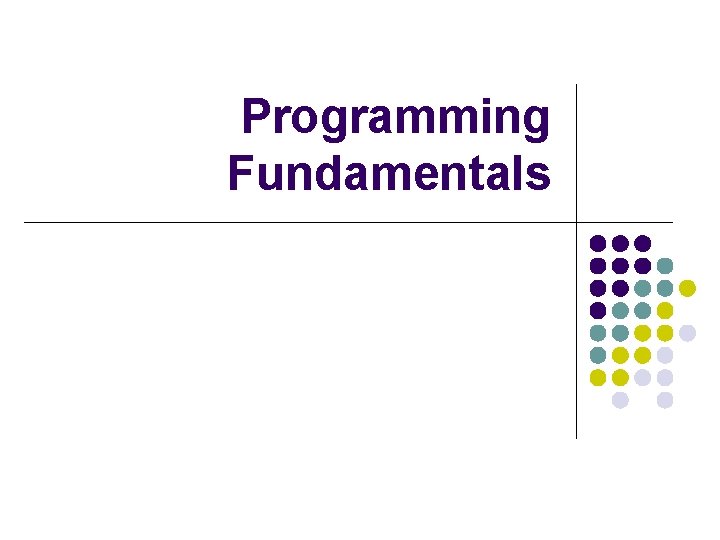
Programming Fundamentals
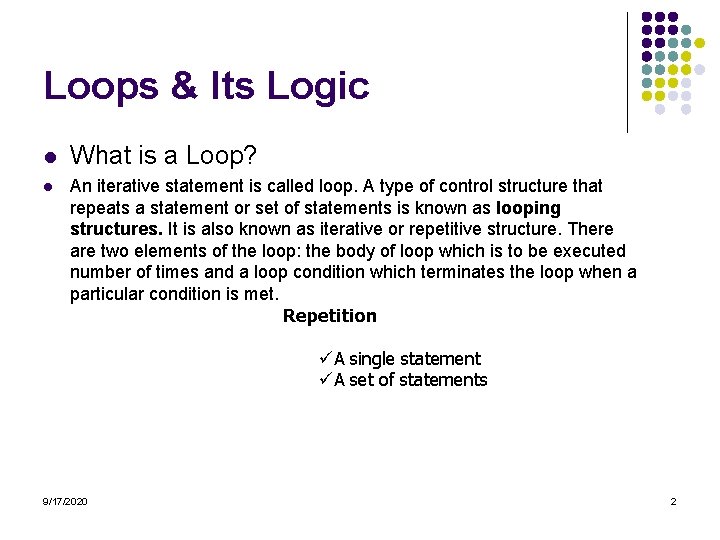
Loops & Its Logic l l What is a Loop? An iterative statement is called loop. A type of control structure that repeats a statement or set of statements is known as looping structures. It is also known as iterative or repetitive structure. There are two elements of the loop: the body of loop which is to be executed number of times and a loop condition which terminates the loop when a particular condition is met. Repetition üA single statement üA set of statements 9/17/2020 2
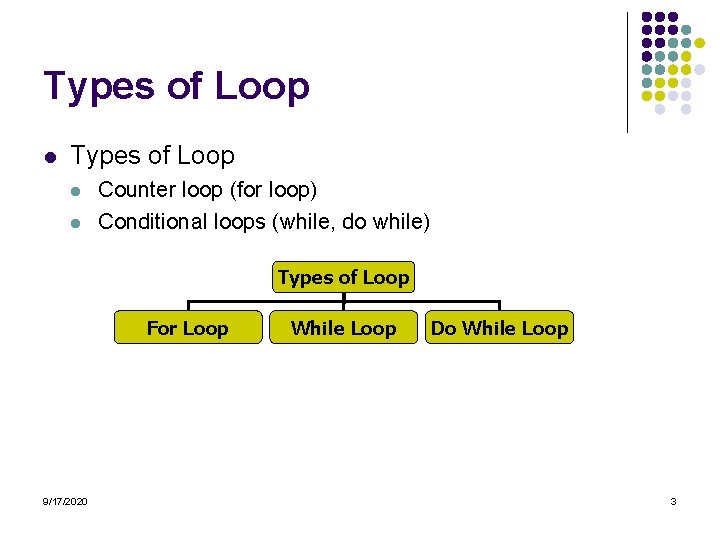
Types of Loop l l Counter loop (for loop) Conditional loops (while, do while) Types of Loop For Loop 9/17/2020 While Loop Do While Loop 3
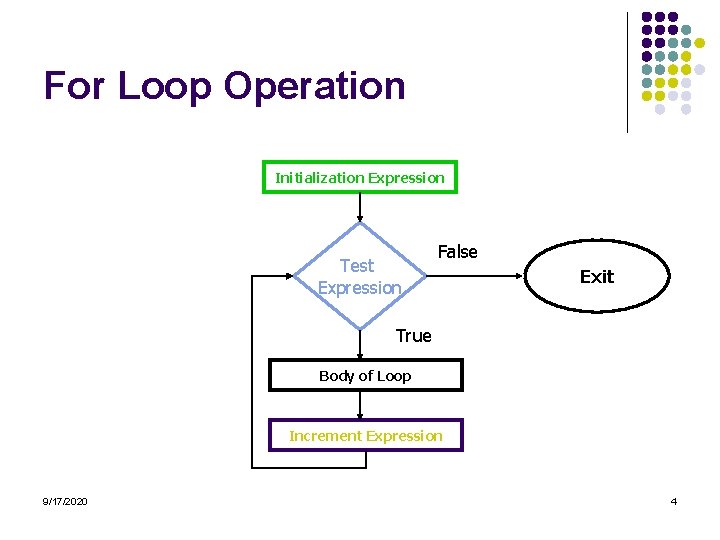
For Loop Operation Initialization Expression Test Expression False Exit True Body of Loop Increment Expression 9/17/2020 4
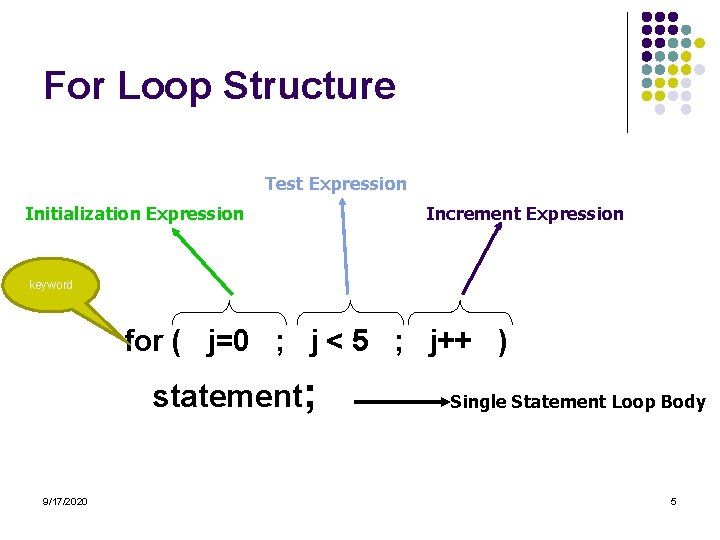
For Loop Structure Test Expression Initialization Expression Increment Expression keyword for ( j=0 ; j < 5 ; j++ ) statement; 9/17/2020 Single Statement Loop Body 5
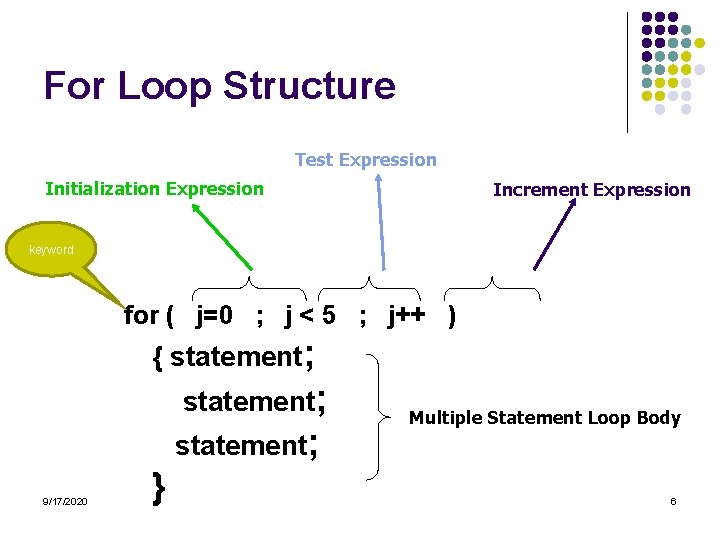
For Loop Structure Test Expression Initialization Expression Increment Expression keyword for ( j=0 ; j < 5 ; j++ ) { statement; 9/17/2020 } Multiple Statement Loop Body 6
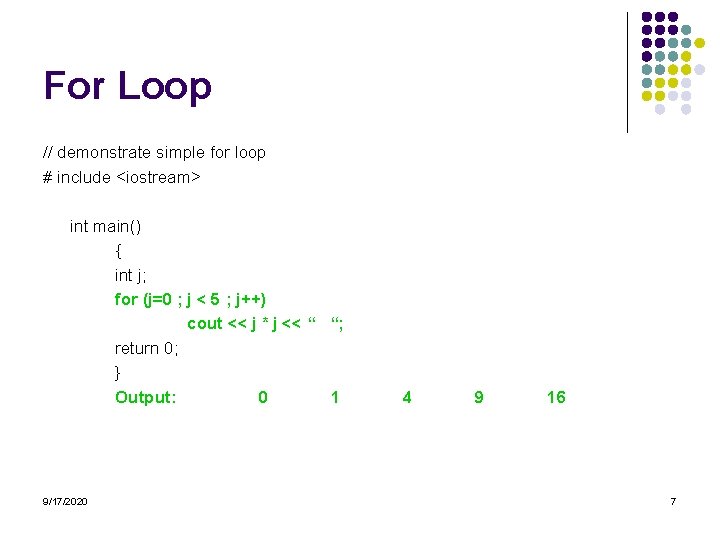
For Loop // demonstrate simple for loop # include <iostream> int main() { int j; for (j=0 ; j < 5 ; j++) cout << j * j << “ “; return 0; } Output: 0 1 9/17/2020 4 9 16 7
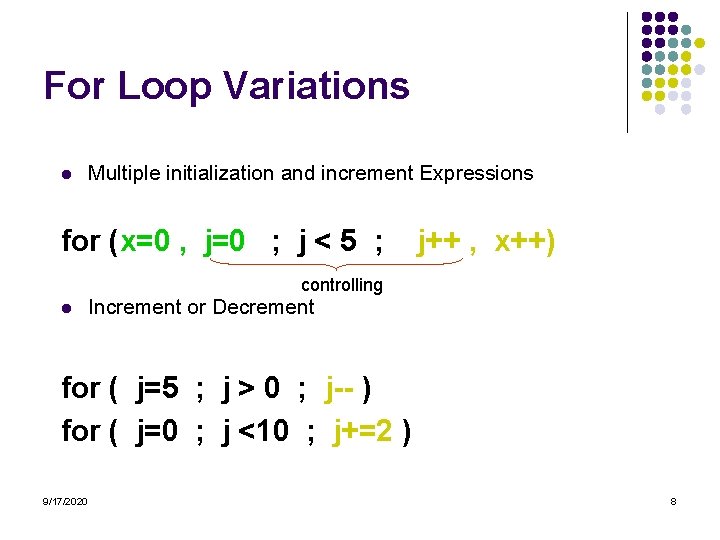
For Loop Variations l Multiple initialization and increment Expressions for (x=0 , j=0 ; j < 5 ; j++ , x++) controlling l Increment or Decrement for ( j=5 ; j > 0 ; j-- ) for ( j=0 ; j <10 ; j+=2 ) 9/17/2020 8
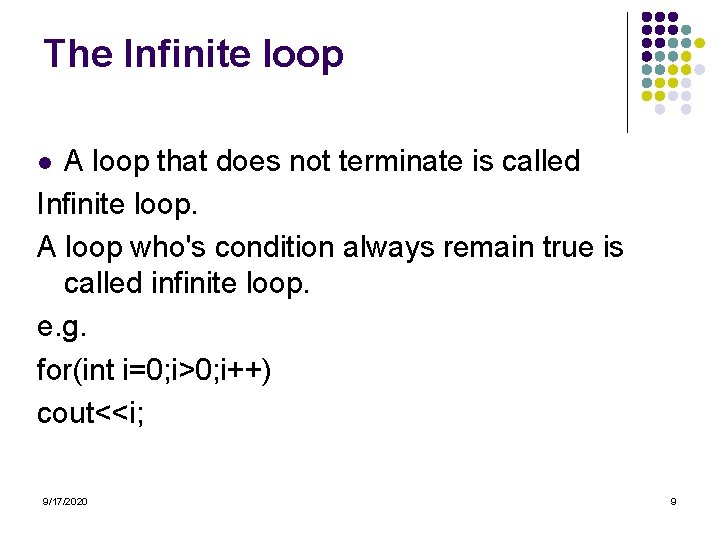
The Infinite loop A loop that does not terminate is called Infinite loop. A loop who's condition always remain true is called infinite loop. e. g. for(int i=0; i>0; i++) cout<<i; l 9/17/2020 9
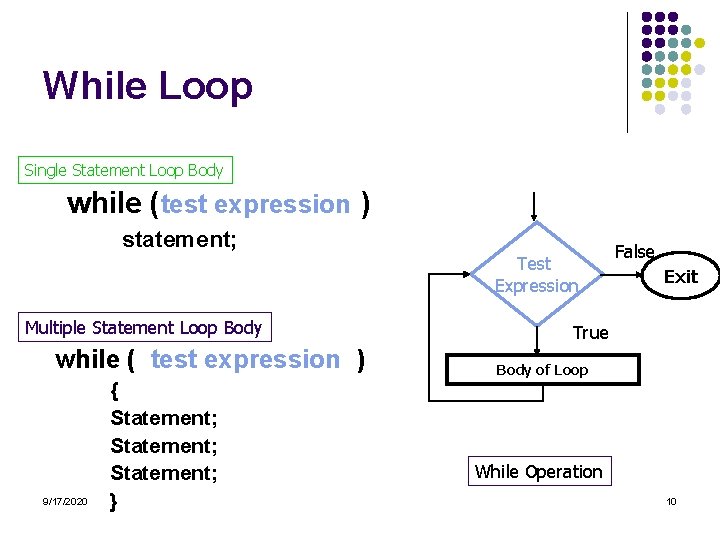
While Loop Single Statement Loop Body while (test expression ) statement; Test Expression Multiple Statement Loop Body while ( test expression ) 9/17/2020 { Statement; } False Exit True Body of Loop While Operation 10
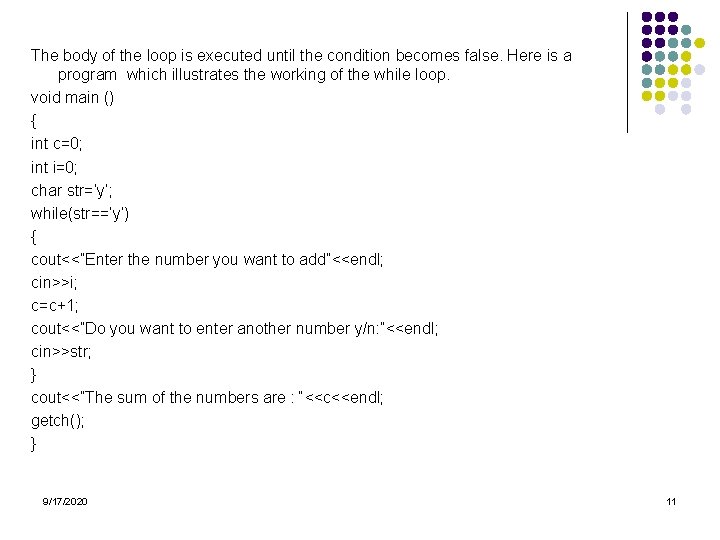
The body of the loop is executed until the condition becomes false. Here is a program which illustrates the working of the while loop. void main () { int c=0; int i=0; char str=‘y’; while(str==’y’) { cout<<”Enter the number you want to add”<<endl; cin>>i; c=c+1; cout<<”Do you want to enter another number y/n: ”<<endl; cin>>str; } cout<<”The sum of the numbers are : “<<c<<endl; getch(); } 9/17/2020 11
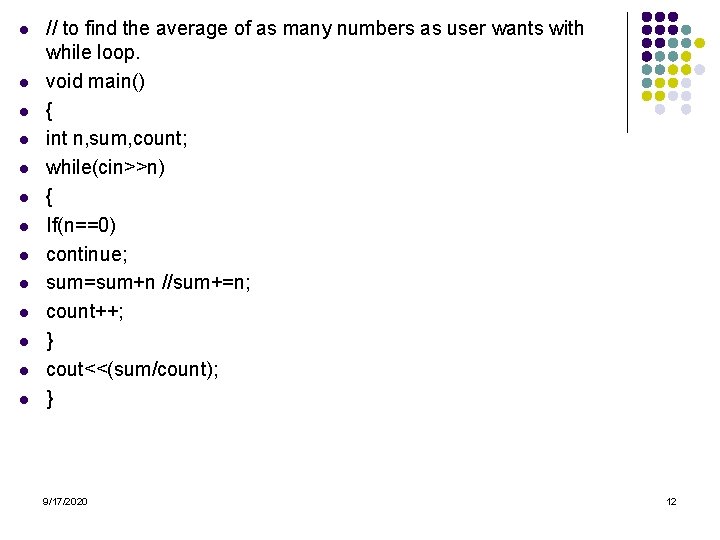
l l l l // to find the average of as many numbers as user wants with while loop. void main() { int n, sum, count; while(cin>>n) { If(n==0) continue; sum=sum+n //sum+=n; count++; } cout<<(sum/count); } 9/17/2020 12
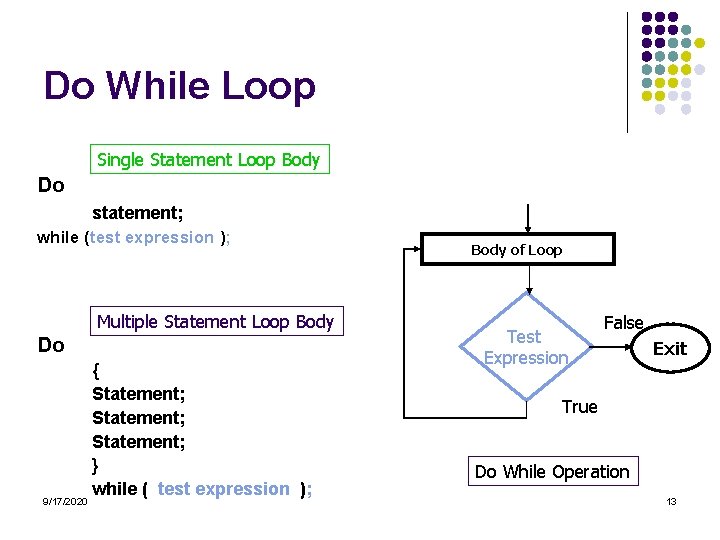
Do While Loop Single Statement Loop Body Do statement; while (test expression ); Multiple Statement Loop Body Do 9/17/2020 { Statement; } while ( test expression ); Body of Loop Test Expression False Exit True Do While Operation 13
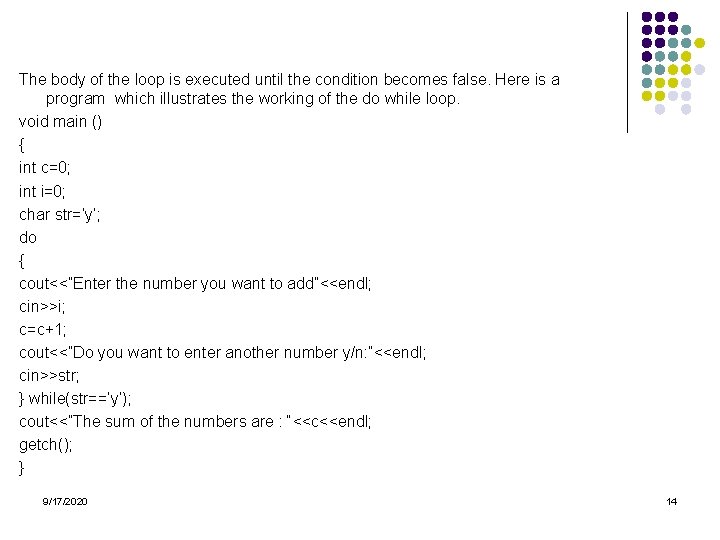
The body of the loop is executed until the condition becomes false. Here is a program which illustrates the working of the do while loop. void main () { int c=0; int i=0; char str=‘y’; do { cout<<”Enter the number you want to add”<<endl; cin>>i; c=c+1; cout<<”Do you want to enter another number y/n: ”<<endl; cin>>str; } while(str==’y’); cout<<”The sum of the numbers are : “<<c<<endl; getch(); } 9/17/2020 14
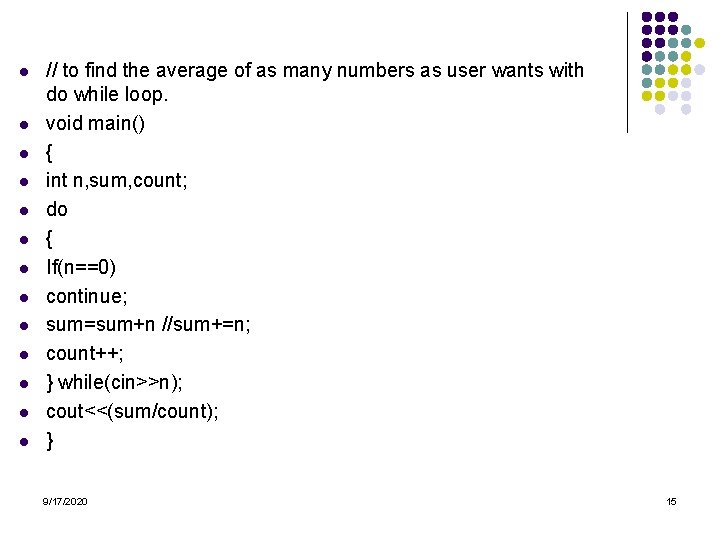
l l l l // to find the average of as many numbers as user wants with do while loop. void main() { int n, sum, count; do { If(n==0) continue; sum=sum+n //sum+=n; count++; } while(cin>>n); cout<<(sum/count); } 9/17/2020 15
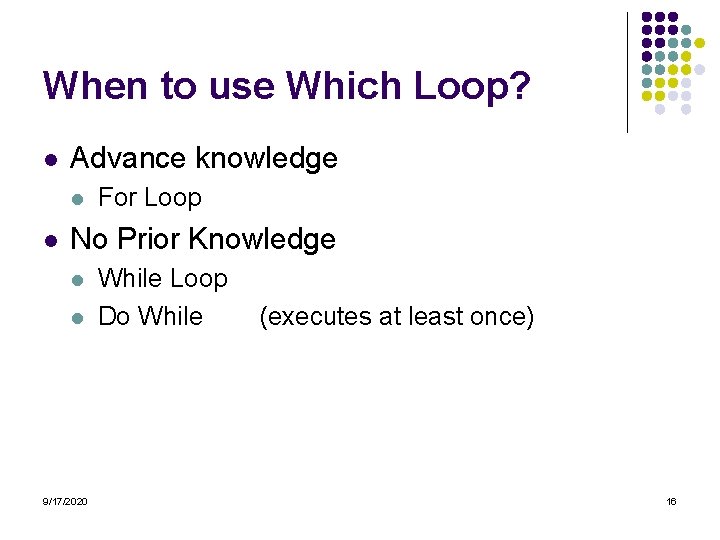
When to use Which Loop? l Advance knowledge l l For Loop No Prior Knowledge l l 9/17/2020 While Loop Do While (executes at least once) 16
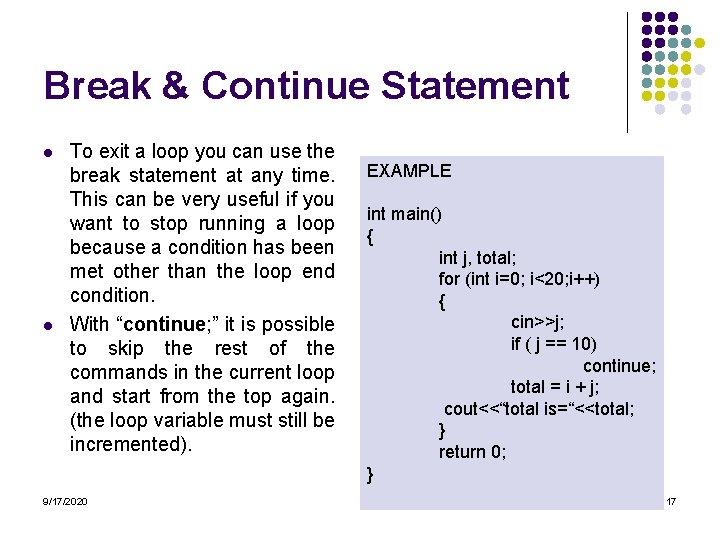
Break & Continue Statement l l To exit a loop you can use the break statement at any time. This can be very useful if you want to stop running a loop because a condition has been met other than the loop end condition. With “continue; ” it is possible to skip the rest of the commands in the current loop and start from the top again. (the loop variable must still be incremented). 9/17/2020 EXAMPLE int main() { int j, total; for (int i=0; i<20; i++) { cin>>j; if ( j == 10) continue; total = i + j; cout<<“total is=“<<total; } return 0; } 17
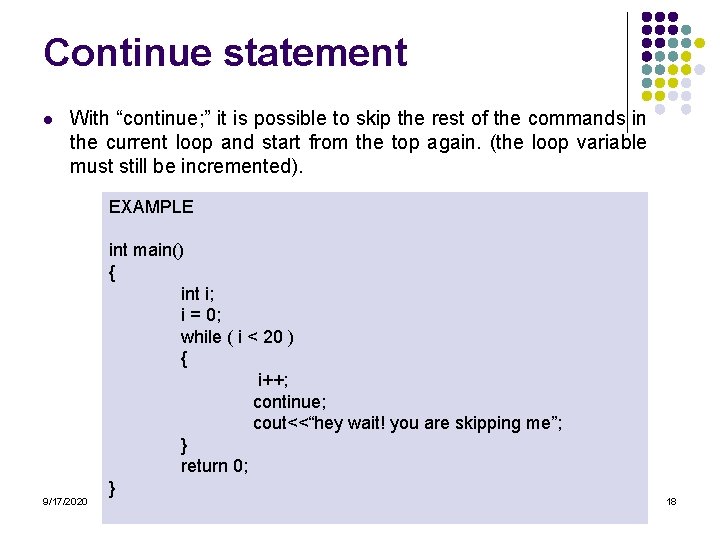
Continue statement l With “continue; ” it is possible to skip the rest of the commands in the current loop and start from the top again. (the loop variable must still be incremented). EXAMPLE 9/17/2020 int main() { int i; i = 0; while ( i < 20 ) { i++; continue; cout<<“hey wait! you are skipping me”; } return 0; } 18
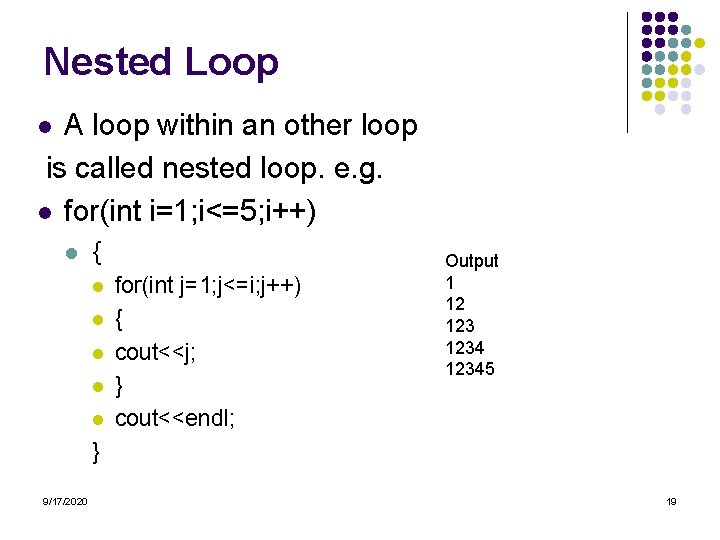
Nested Loop A loop within an other loop is called nested loop. e. g. l for(int i=1; i<=5; i++) l l { l l l for(int j=1; j<=i; j++) { cout<<j; } cout<<endl; Output 1 12 12345 } 9/17/2020 19
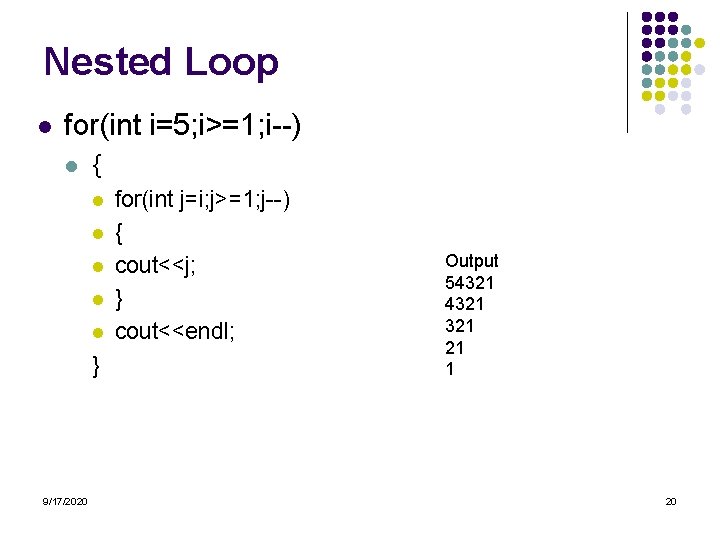
Nested Loop l for(int i=5; i>=1; i--) l { l l l } 9/17/2020 for(int j=i; j>=1; j--) { cout<<j; } cout<<endl; Output 54321 321 21 1 20
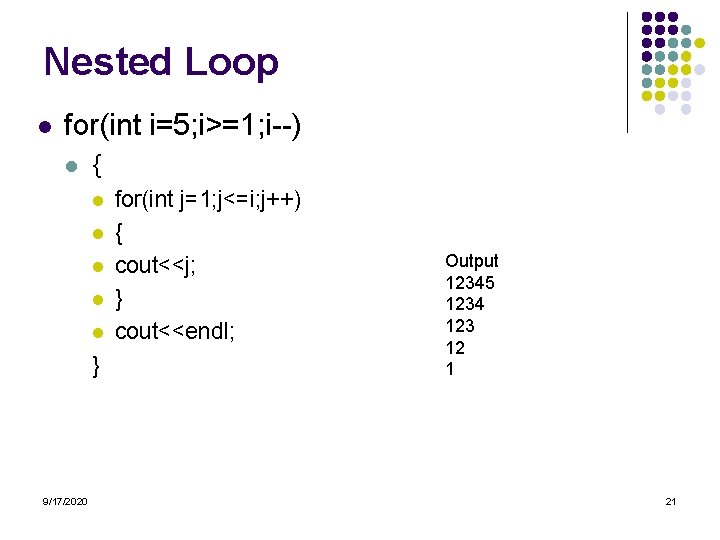
Nested Loop l for(int i=5; i>=1; i--) l { l l l } 9/17/2020 for(int j=1; j<=i; j++) { cout<<j; } cout<<endl; Output 12345 1234 123 12 1 21
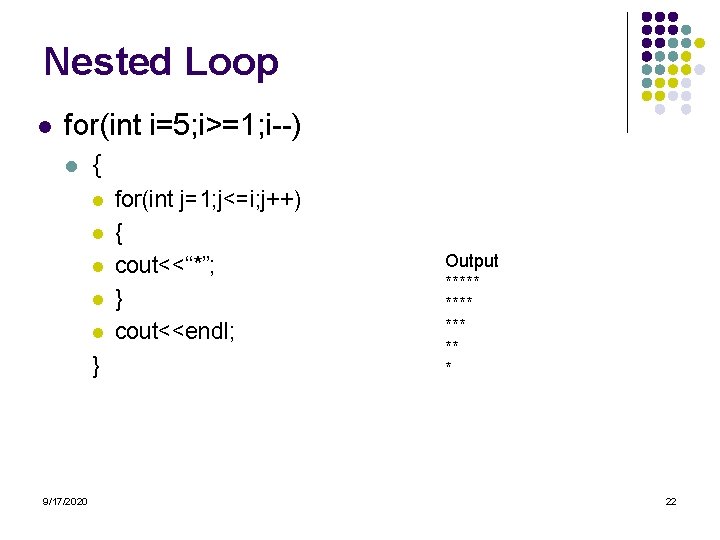
Nested Loop l for(int i=5; i>=1; i--) l { l l l } 9/17/2020 for(int j=1; j<=i; j++) { cout<<“*”; } cout<<endl; Output ***** *** ** * 22
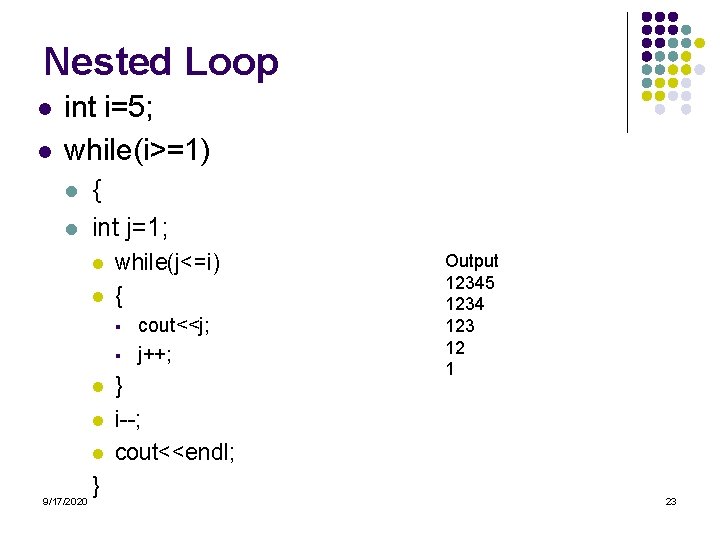
Nested Loop l l int i=5; while(i>=1) l l { int j=1; l l while(j<=i) { § § l l l 9/17/2020 } cout<<j; j++; } i--; cout<<endl; Output 12345 1234 123 12 1 23
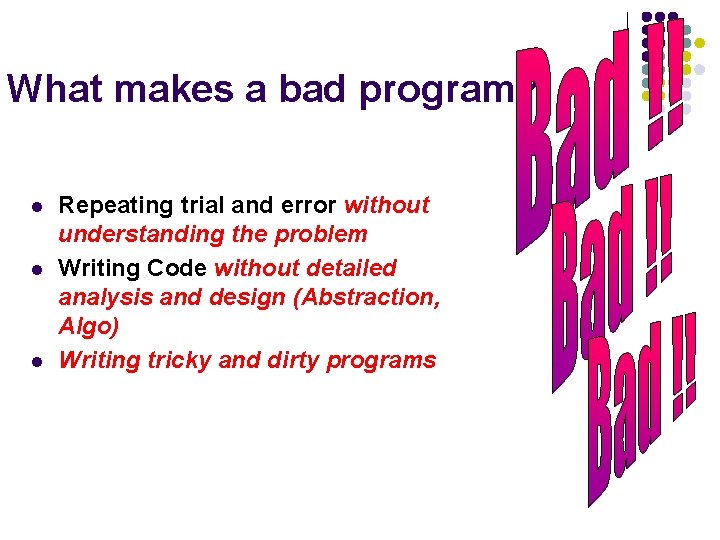
What makes a bad program? l l l Repeating trial and error without understanding the problem Writing Code without detailed analysis and design (Abstraction, Algo) Writing tricky and dirty programs
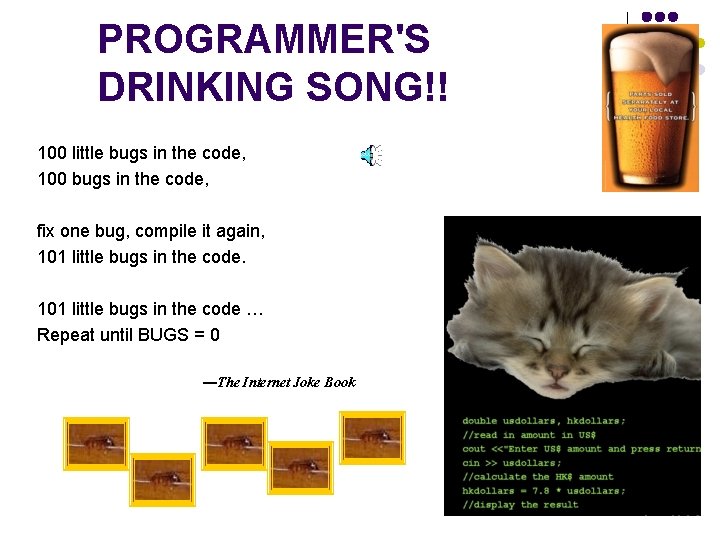
PROGRAMMER'S DRINKING SONG!! 100 little bugs in the code, 100 bugs in the code, fix one bug, compile it again, 101 little bugs in the code … Repeat until BUGS = 0 —The Internet Joke Book
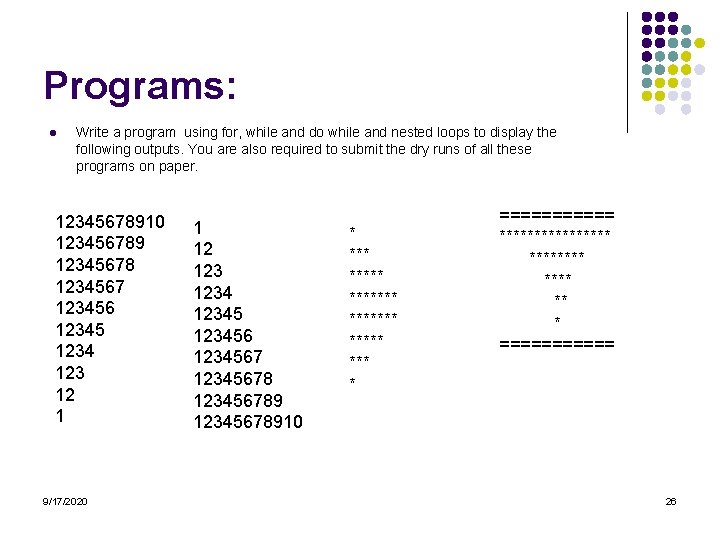
Programs: l Write a program using for, while and do while and nested loops to display the following outputs. You are also required to submit the dry runs of all these programs on paper. 12345678910 123456789 12345678 1234567 123456 12345 1234 123 12 1 9/17/2020 1 12 123456 12345678910 * ******* *** * ====== ******** **** ** * ====== 26
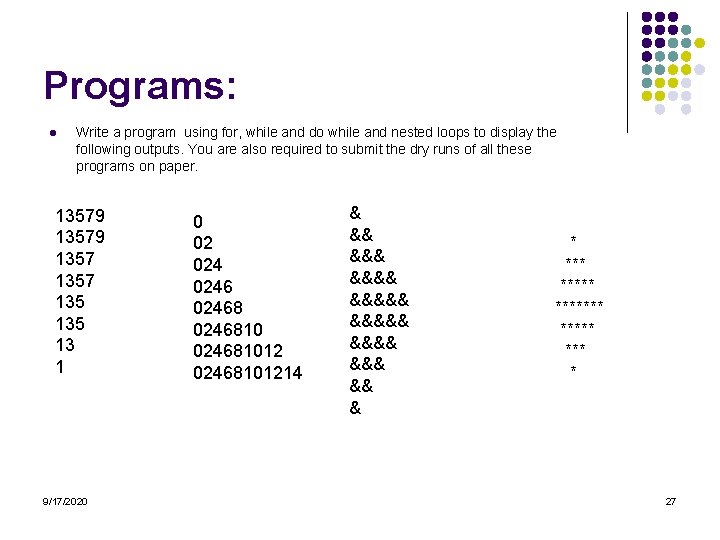
Programs: l Write a program using for, while and do while and nested loops to display the following outputs. You are also required to submit the dry runs of all these programs on paper. 13579 1357 135 13 1 9/17/2020 0 02 0246810 02468101214 & && &&&&& &&& && & * ***** *** * 27
Its platinum loops shrink to a wedding-ring
Word choice figurative language
Jk flip flop
Logic and computer design fundamentals
Logic and computer design fundamentals
Logic and computer design fundamentals
Logic & computer design fundamentals
Functional programming fundamentals
Cs 1101 programming fundamentals final exam
Fundamentals of functional programming language
Programming fundamentals 1
Salute report example
Cs 1101 programming fundamentals final exam
Cs 1101 programming fundamentals
Cs 1101 programming fundamentals
First order logic vs propositional logic
First order logic vs propositional logic
Third order logic
Concurrent vs sequential
Cryptarithmetic problem logic+logic=prolog
캠블리 단점
Majority circuit
Combinational logic sequential logic 차이
Combinational logic sequential logic
Logic programming tutorial
Looping algoritma
Plc ladder
Prolog language