Programming Assignment 8 CS 113 Spring 2002 Programming
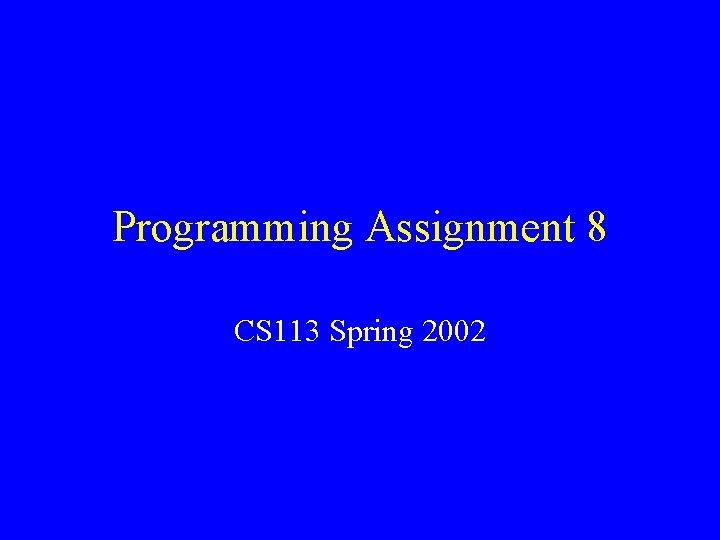
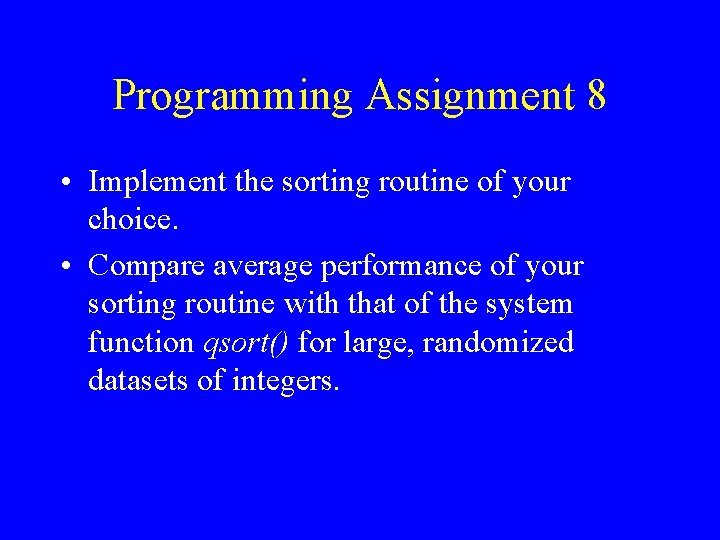
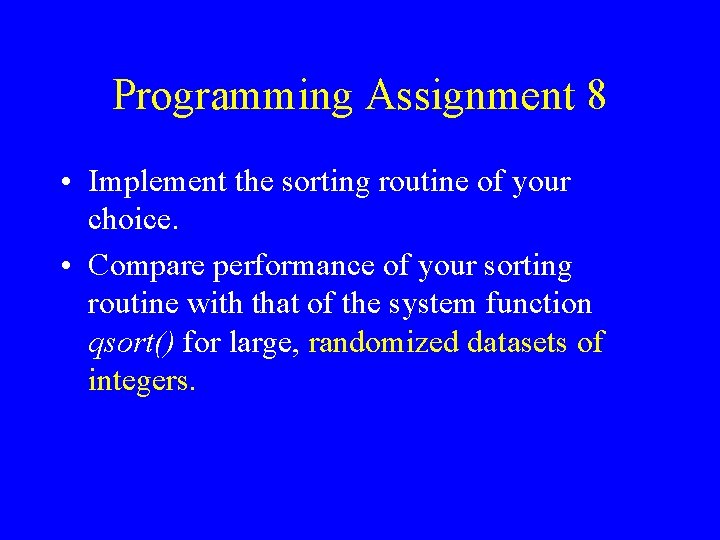
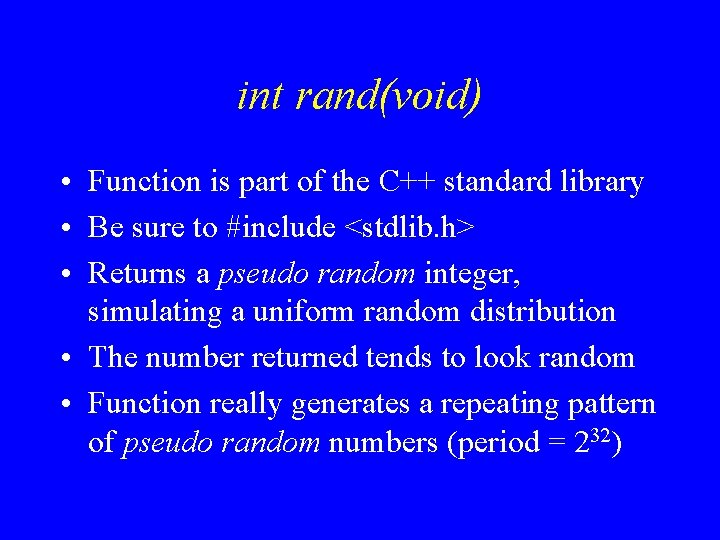
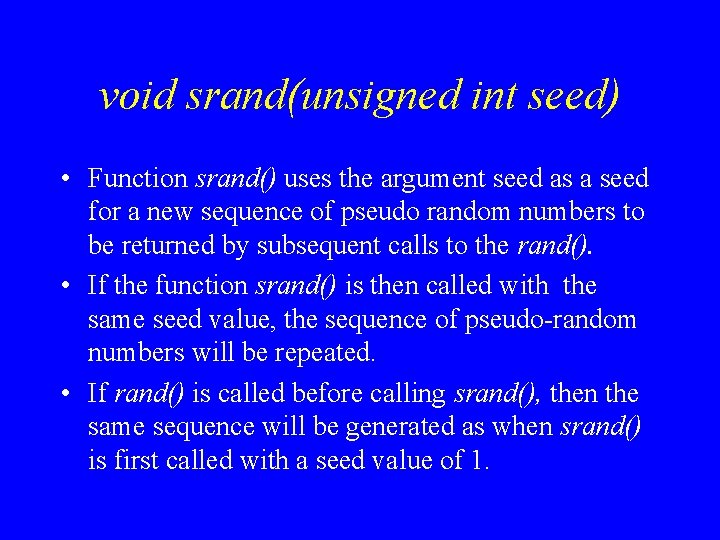
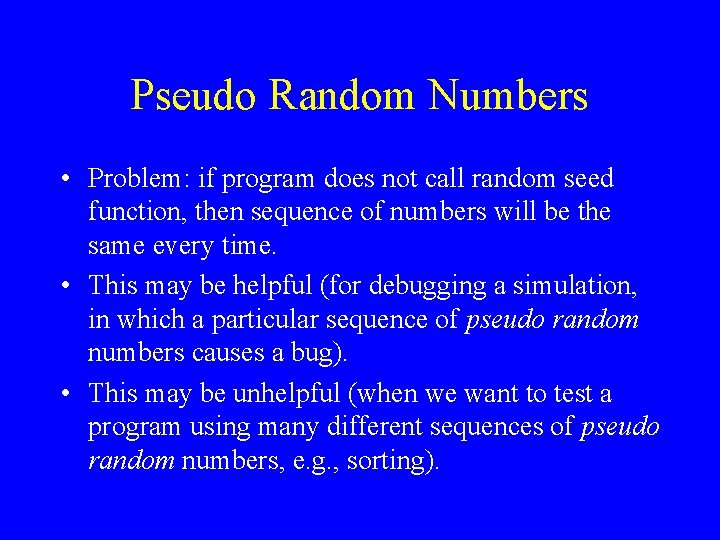
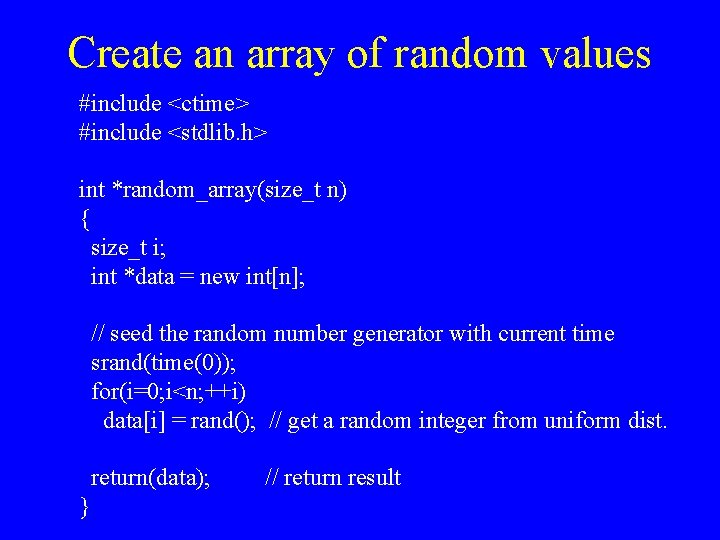
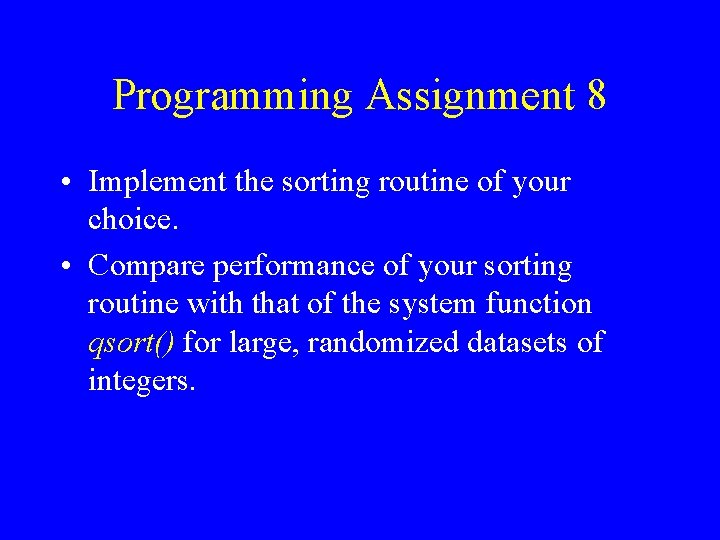
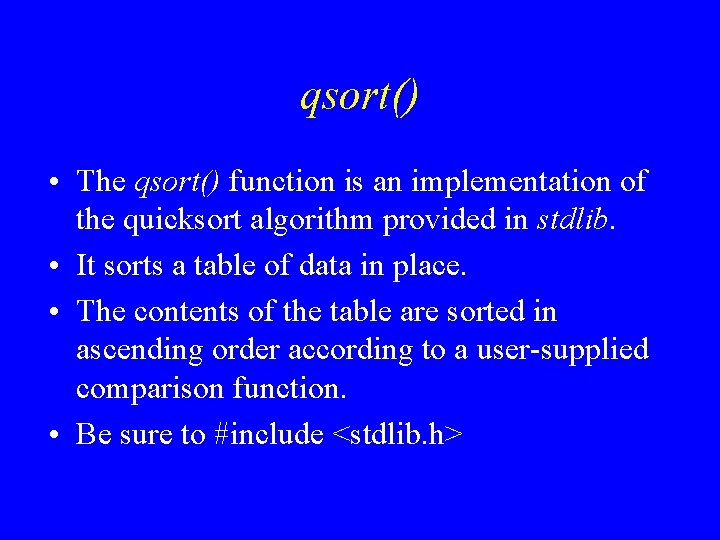
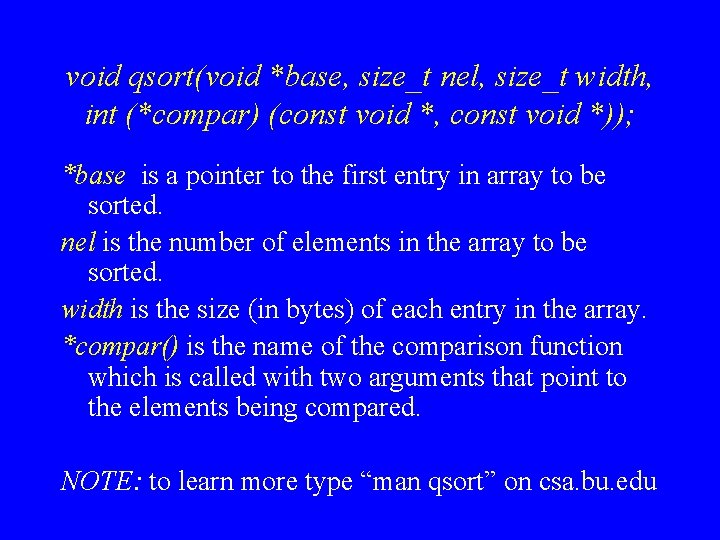
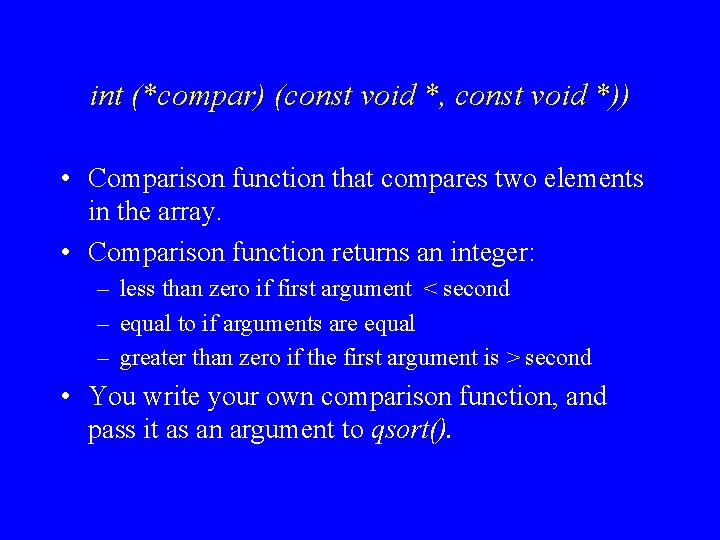
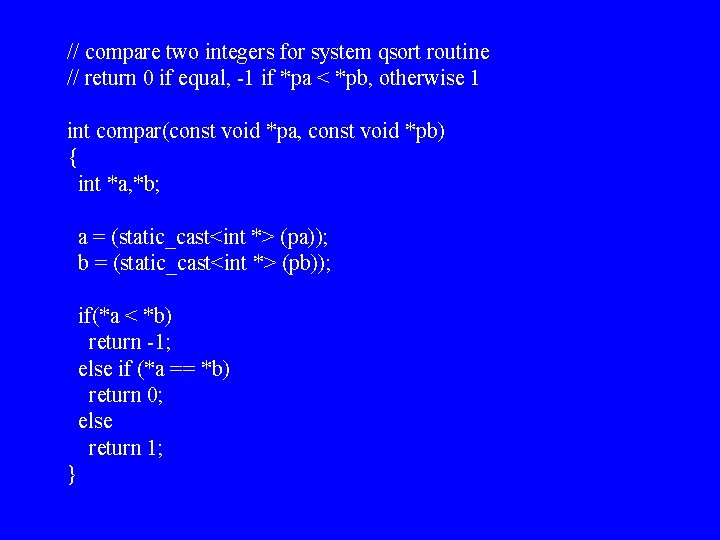
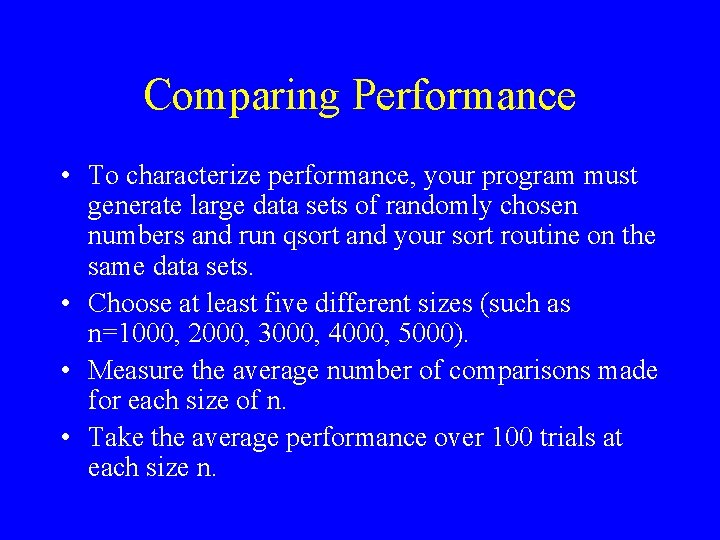
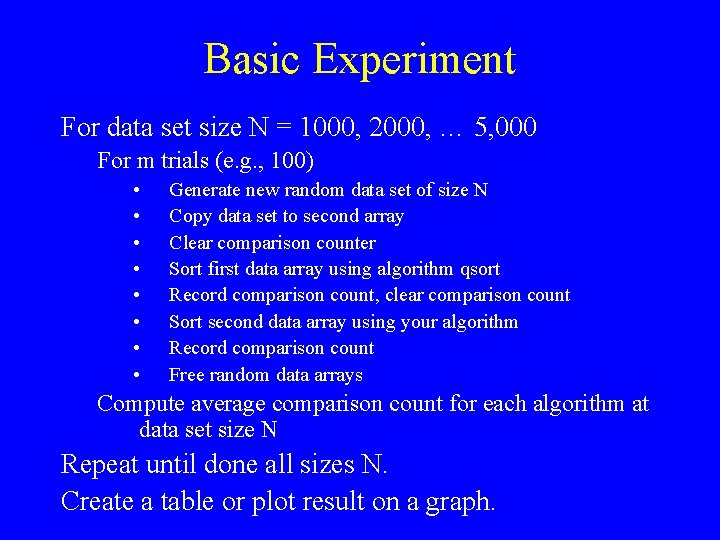
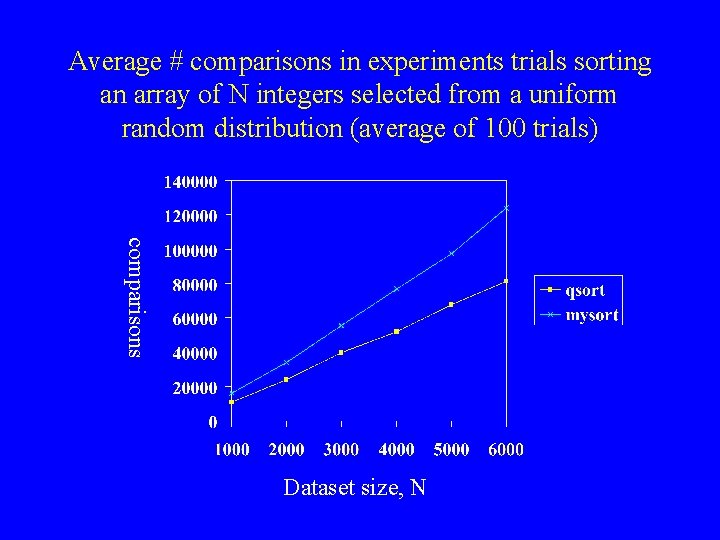
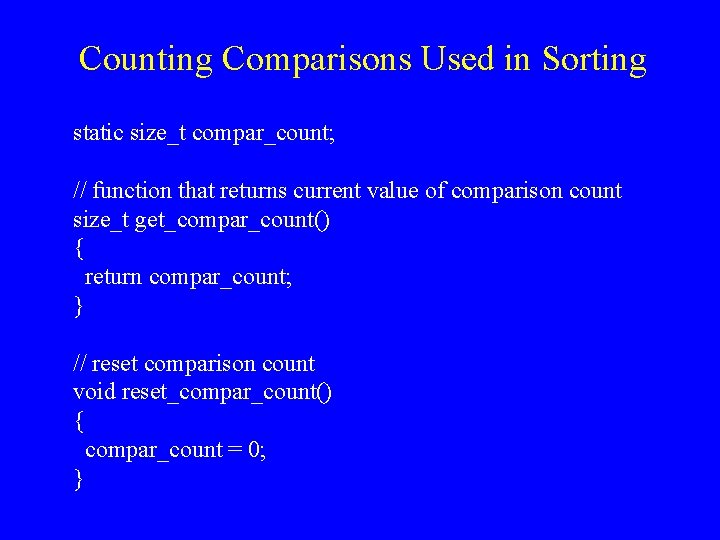
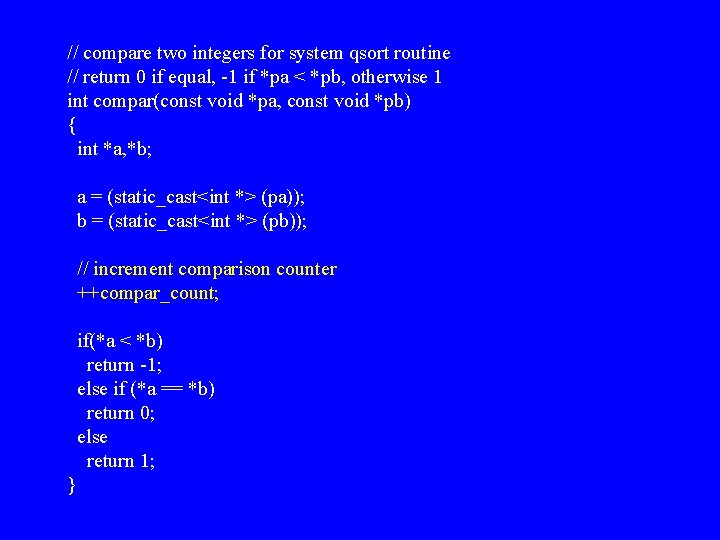
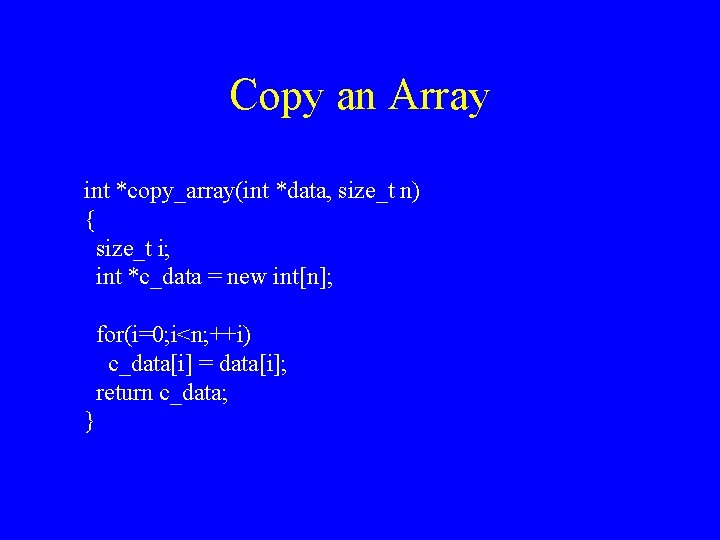
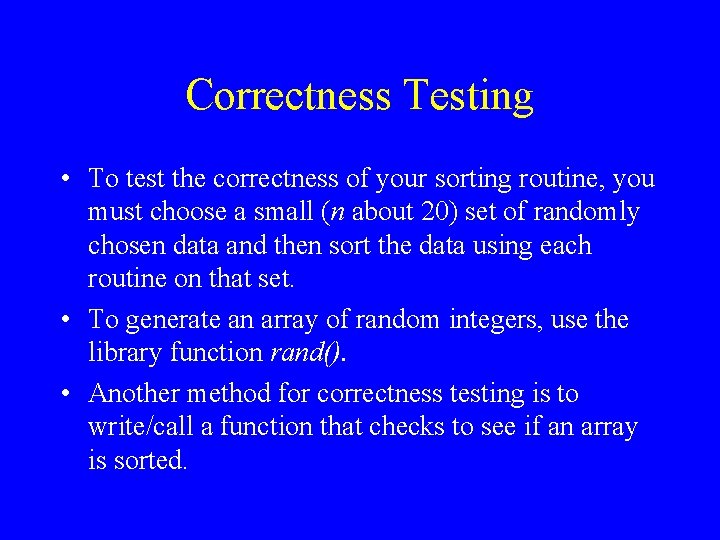
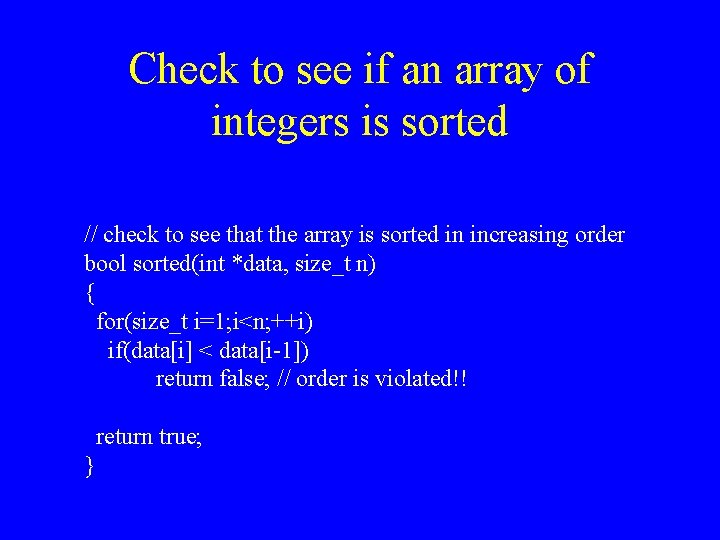
- Slides: 20
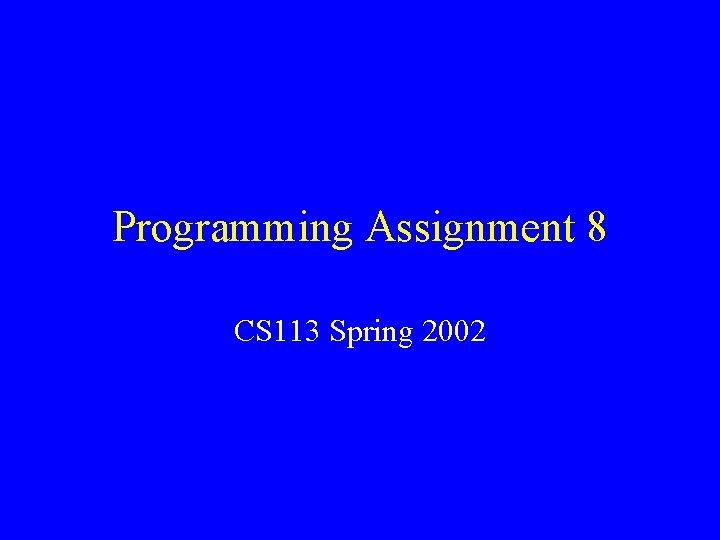
Programming Assignment 8 CS 113 Spring 2002
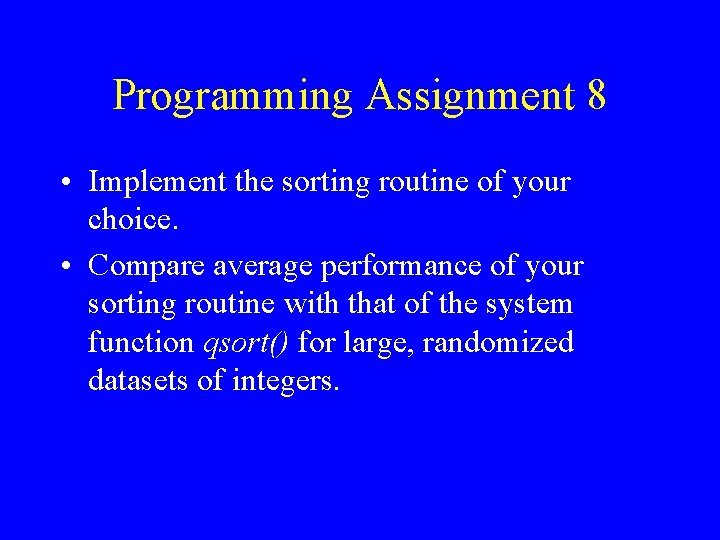
Programming Assignment 8 • Implement the sorting routine of your choice. • Compare average performance of your sorting routine with that of the system function qsort() for large, randomized datasets of integers.
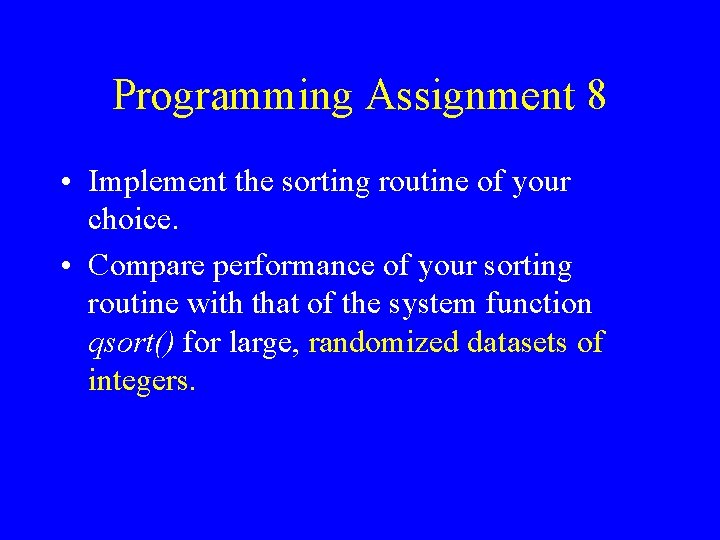
Programming Assignment 8 • Implement the sorting routine of your choice. • Compare performance of your sorting routine with that of the system function qsort() for large, randomized datasets of integers.
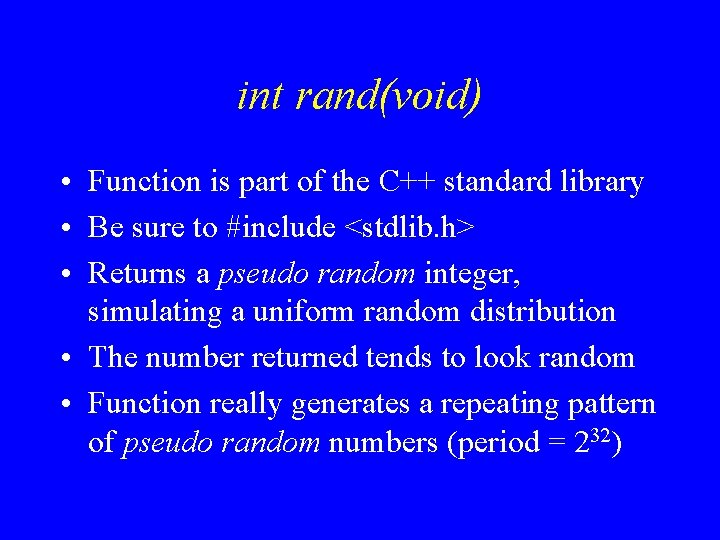
int rand(void) • Function is part of the C++ standard library • Be sure to #include <stdlib. h> • Returns a pseudo random integer, simulating a uniform random distribution • The number returned tends to look random • Function really generates a repeating pattern of pseudo random numbers (period = 232)
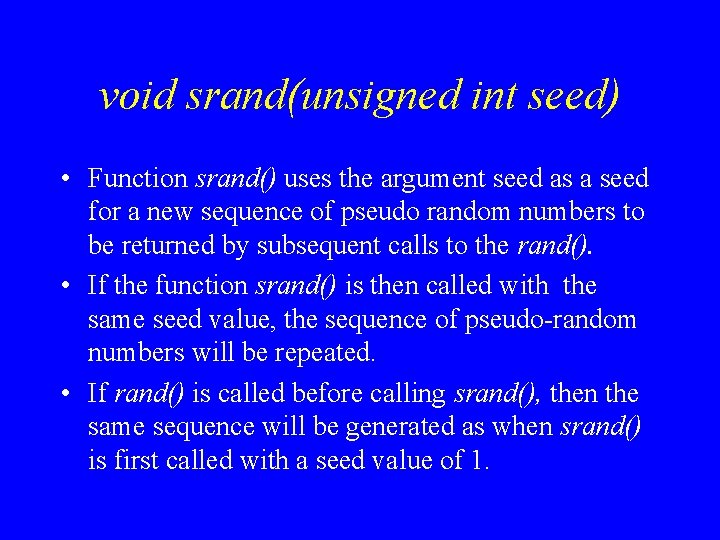
void srand(unsigned int seed) • Function srand() uses the argument seed as a seed for a new sequence of pseudo random numbers to be returned by subsequent calls to the rand(). • If the function srand() is then called with the same seed value, the sequence of pseudo-random numbers will be repeated. • If rand() is called before calling srand(), then the same sequence will be generated as when srand() is first called with a seed value of 1.
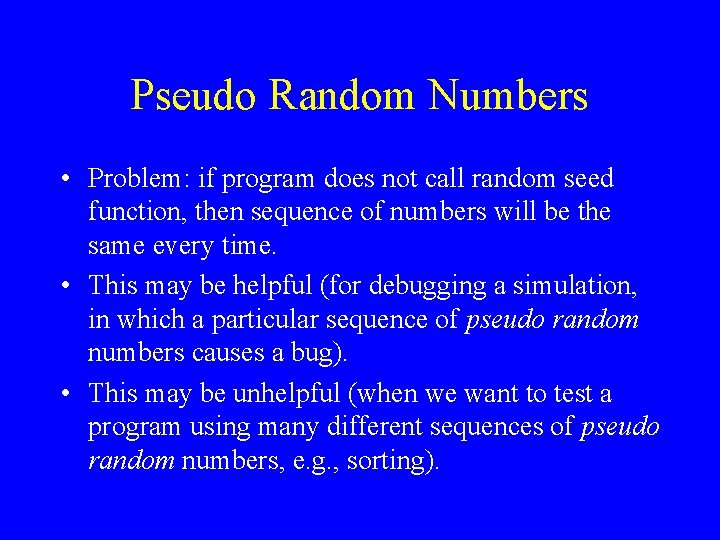
Pseudo Random Numbers • Problem: if program does not call random seed function, then sequence of numbers will be the same every time. • This may be helpful (for debugging a simulation, in which a particular sequence of pseudo random numbers causes a bug). • This may be unhelpful (when we want to test a program using many different sequences of pseudo random numbers, e. g. , sorting).
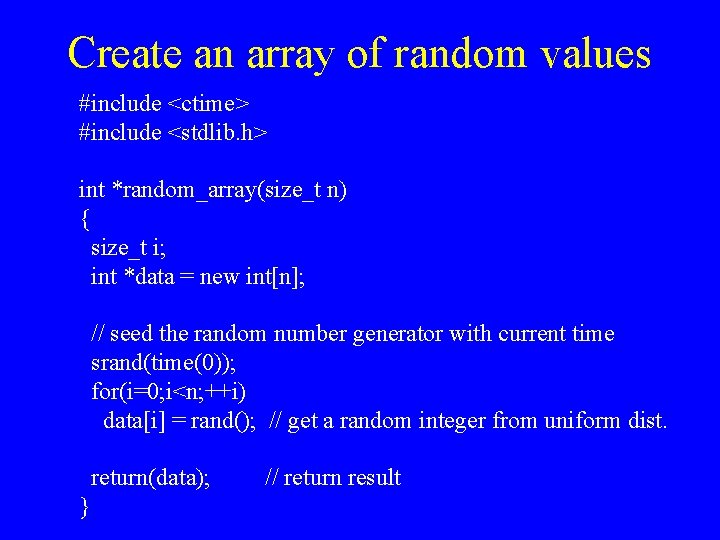
Create an array of random values #include <ctime> #include <stdlib. h> int *random_array(size_t n) { size_t i; int *data = new int[n]; // seed the random number generator with current time srand(time(0)); for(i=0; i<n; ++i) data[i] = rand(); // get a random integer from uniform dist. return(data); } // return result
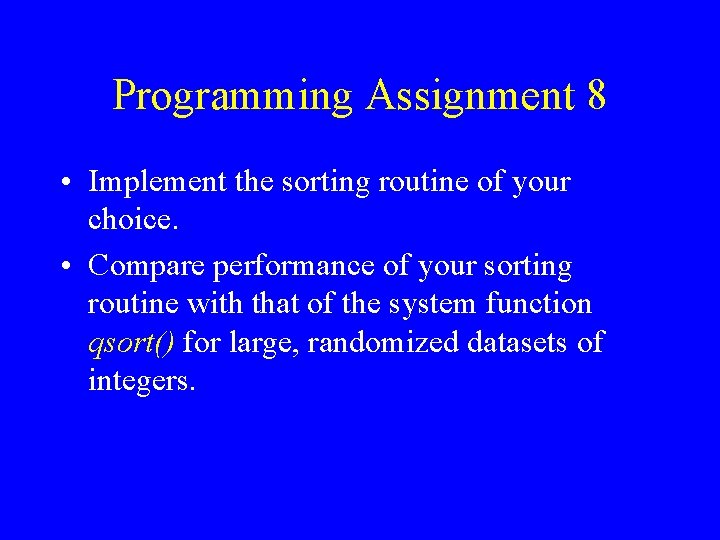
Programming Assignment 8 • Implement the sorting routine of your choice. • Compare performance of your sorting routine with that of the system function qsort() for large, randomized datasets of integers.
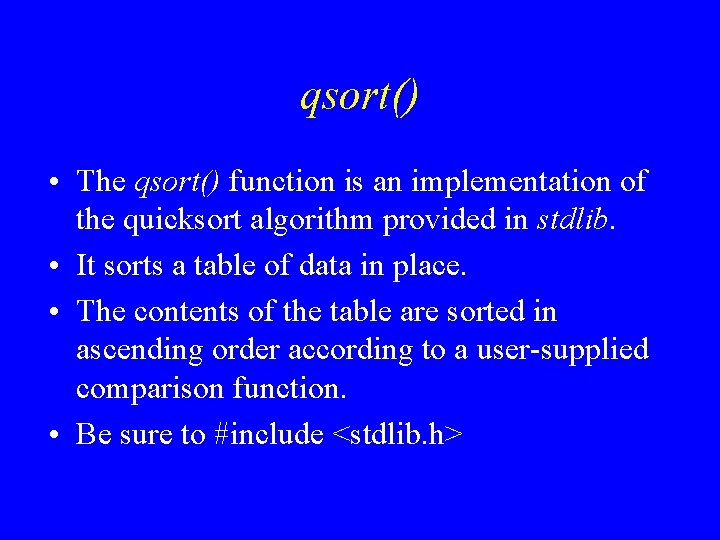
qsort() • The qsort() function is an implementation of the quicksort algorithm provided in stdlib. • It sorts a table of data in place. • The contents of the table are sorted in ascending order according to a user-supplied comparison function. • Be sure to #include <stdlib. h>
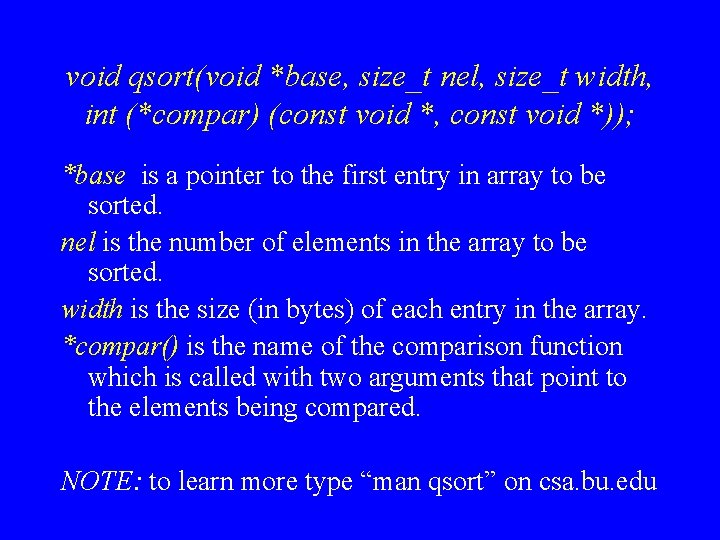
void qsort(void *base, size_t nel, size_t width, int (*compar) (const void *, const void *)); *base is a pointer to the first entry in array to be sorted. nel is the number of elements in the array to be sorted. width is the size (in bytes) of each entry in the array. *compar() is the name of the comparison function which is called with two arguments that point to the elements being compared. NOTE: to learn more type “man qsort” on csa. bu. edu
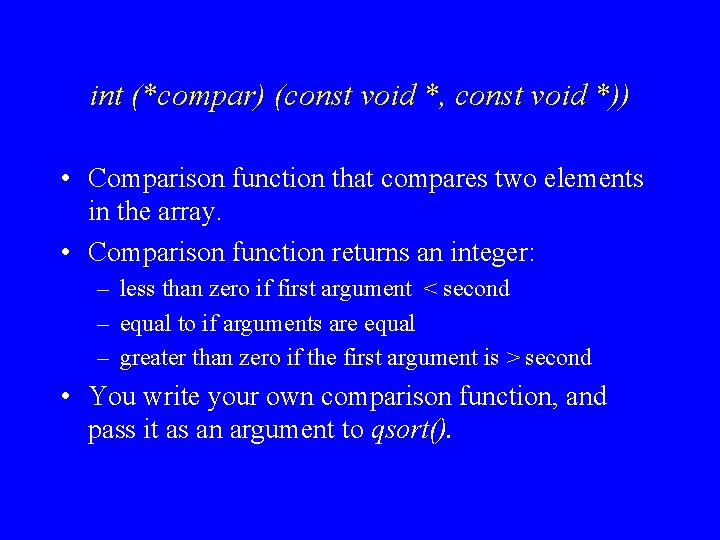
int (*compar) (const void *, const void *)) • Comparison function that compares two elements in the array. • Comparison function returns an integer: – less than zero if first argument < second – equal to if arguments are equal – greater than zero if the first argument is > second • You write your own comparison function, and pass it as an argument to qsort().
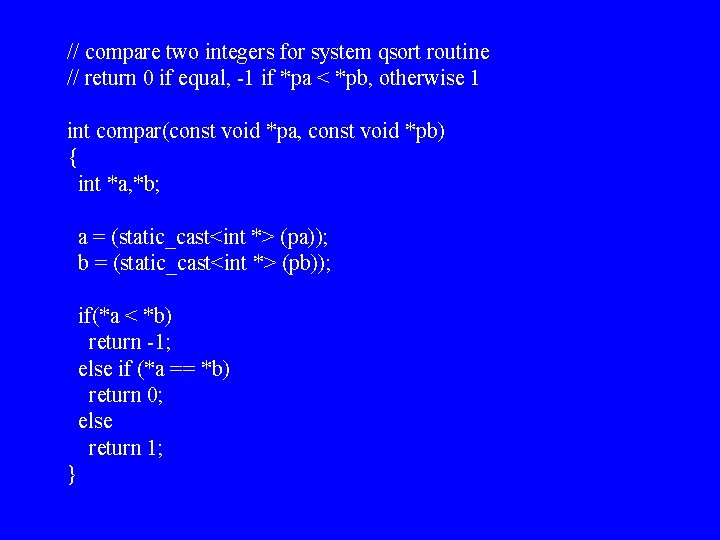
// compare two integers for system qsort routine // return 0 if equal, -1 if *pa < *pb, otherwise 1 int compar(const void *pa, const void *pb) { int *a, *b; a = (static_cast<int *> (pa)); b = (static_cast<int *> (pb)); if(*a < *b) return -1; else if (*a == *b) return 0; else return 1; }
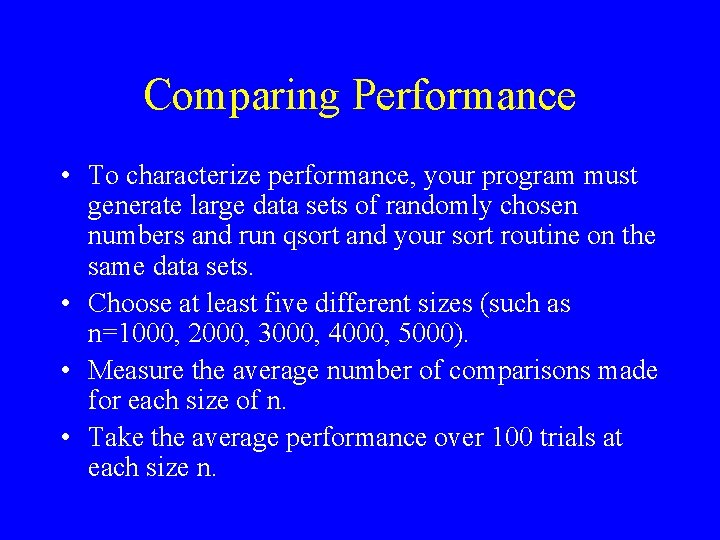
Comparing Performance • To characterize performance, your program must generate large data sets of randomly chosen numbers and run qsort and your sort routine on the same data sets. • Choose at least five different sizes (such as n=1000, 2000, 3000, 4000, 5000). • Measure the average number of comparisons made for each size of n. • Take the average performance over 100 trials at each size n.
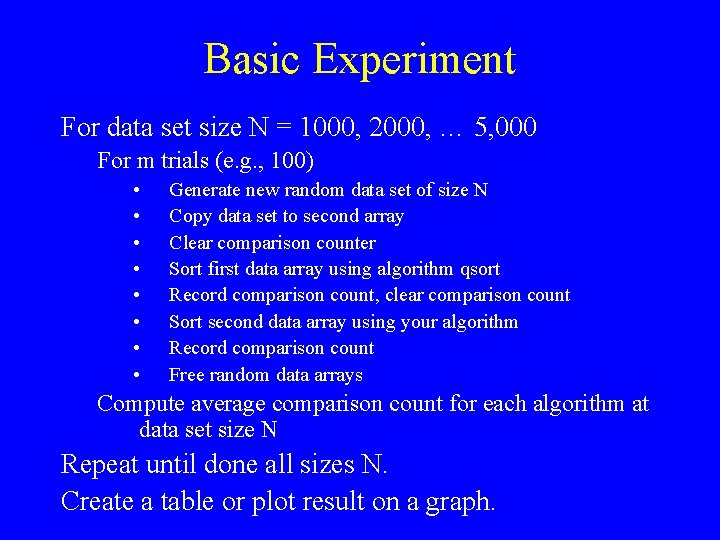
Basic Experiment For data set size N = 1000, 2000, … 5, 000 For m trials (e. g. , 100) • • Generate new random data set of size N Copy data set to second array Clear comparison counter Sort first data array using algorithm qsort Record comparison count, clear comparison count Sort second data array using your algorithm Record comparison count Free random data arrays Compute average comparison count for each algorithm at data set size N Repeat until done all sizes N. Create a table or plot result on a graph.
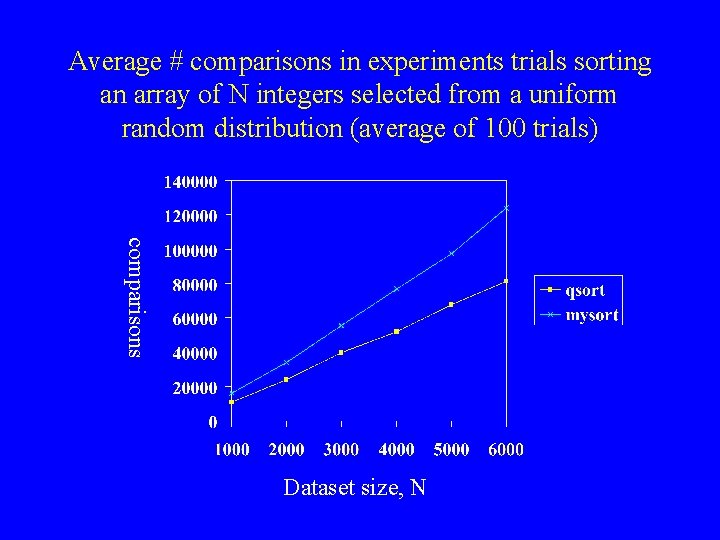
Average # comparisons in experiments trials sorting an array of N integers selected from a uniform random distribution (average of 100 trials) comparisons Dataset size, N
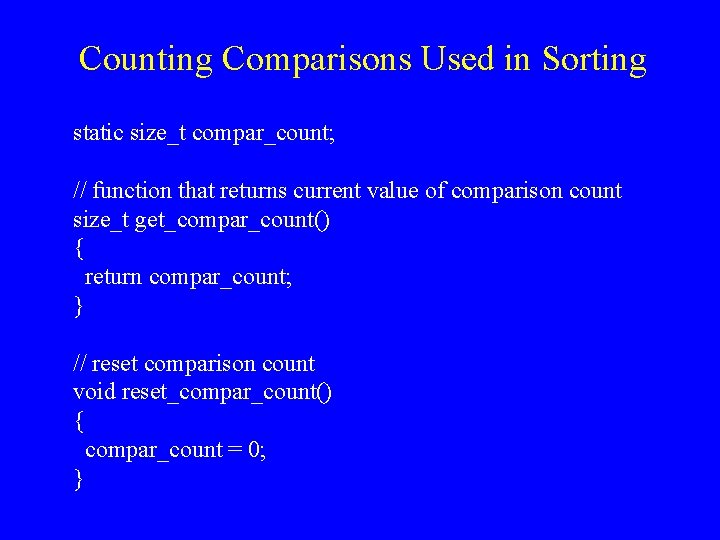
Counting Comparisons Used in Sorting static size_t compar_count; // function that returns current value of comparison count size_t get_compar_count() { return compar_count; } // reset comparison count void reset_compar_count() { compar_count = 0; }
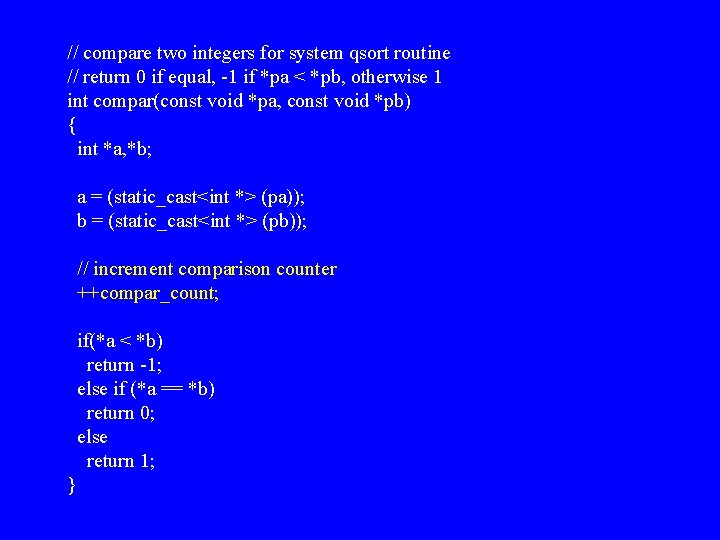
// compare two integers for system qsort routine // return 0 if equal, -1 if *pa < *pb, otherwise 1 int compar(const void *pa, const void *pb) { int *a, *b; a = (static_cast<int *> (pa)); b = (static_cast<int *> (pb)); // increment comparison counter ++compar_count; if(*a < *b) return -1; else if (*a == *b) return 0; else return 1; }
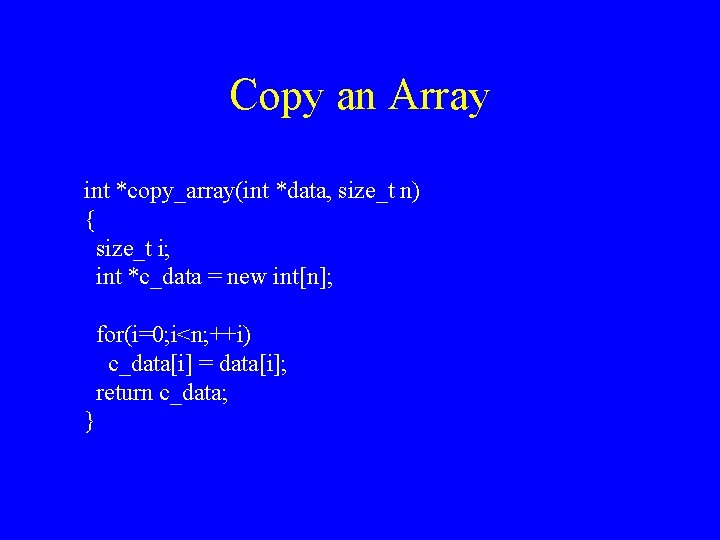
Copy an Array int *copy_array(int *data, size_t n) { size_t i; int *c_data = new int[n]; for(i=0; i<n; ++i) c_data[i] = data[i]; return c_data; }
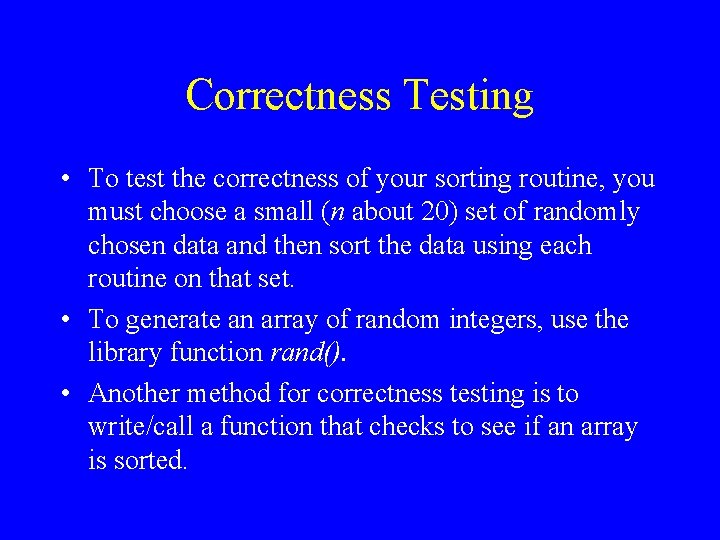
Correctness Testing • To test the correctness of your sorting routine, you must choose a small (n about 20) set of randomly chosen data and then sort the data using each routine on that set. • To generate an array of random integers, use the library function rand(). • Another method for correctness testing is to write/call a function that checks to see if an array is sorted.
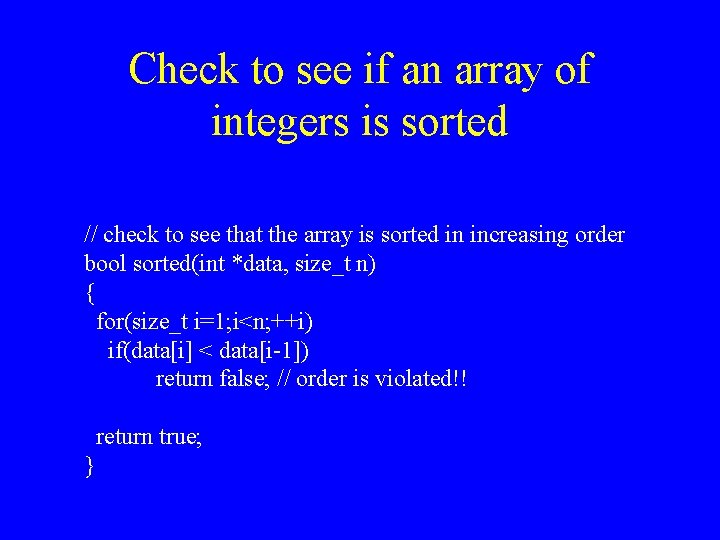
Check to see if an array of integers is sorted // check to see that the array is sorted in increasing order bool sorted(int *data, size_t n) { for(size_t i=1; i<n; ++i) if(data[i] < data[i-1]) return false; // order is violated!! return true; }