Programming Applets How do Applets work This is
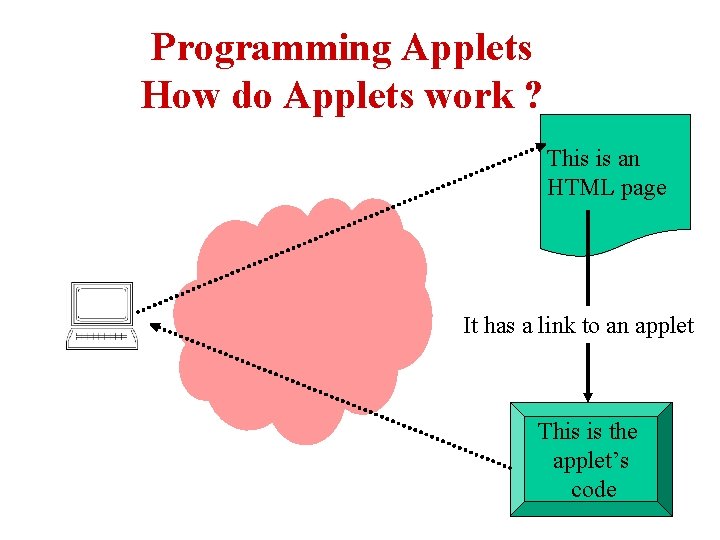
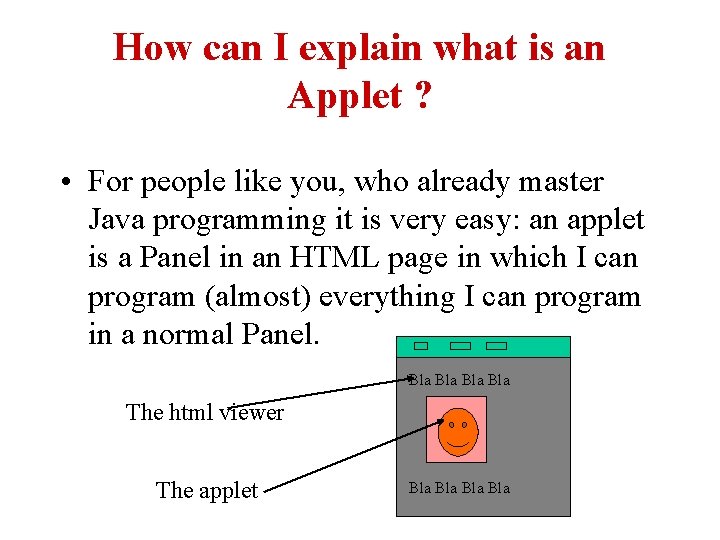
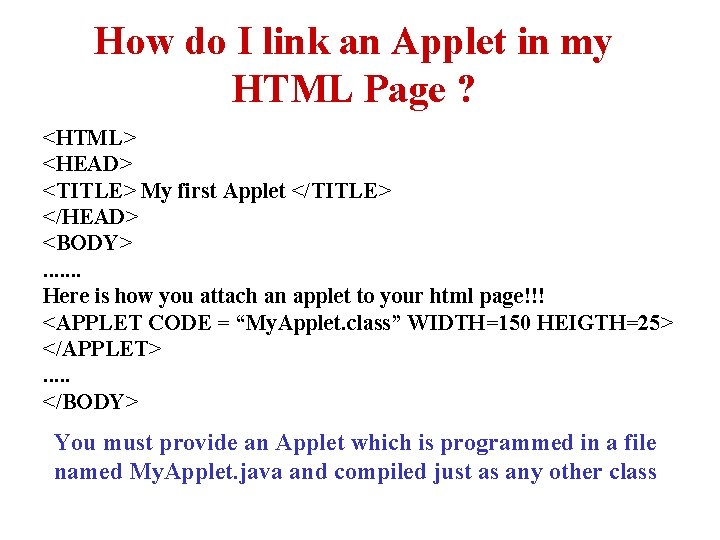
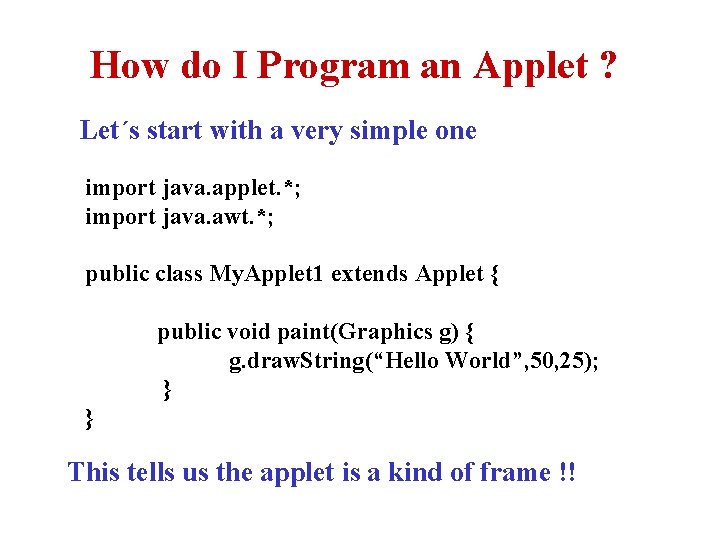
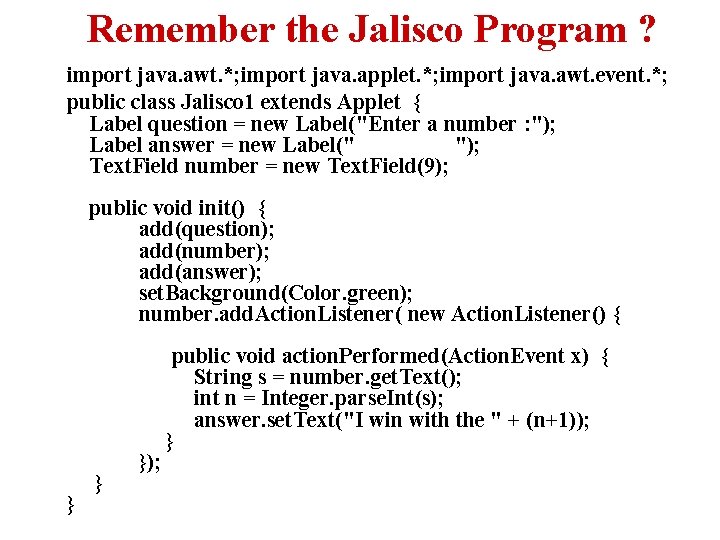
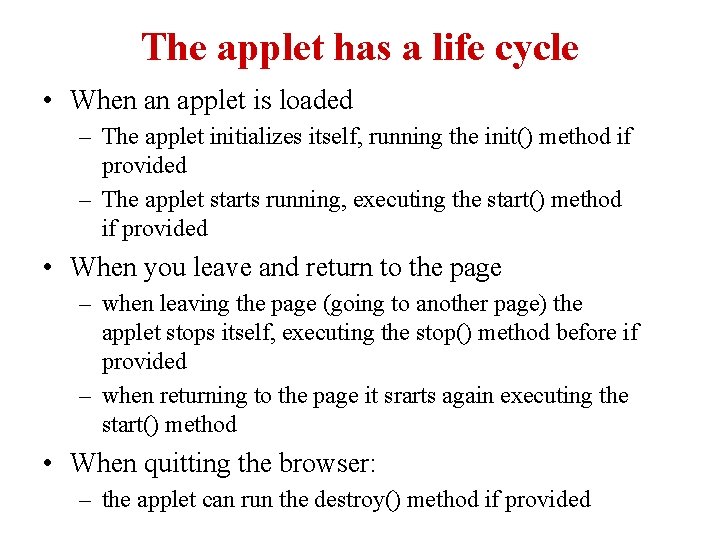
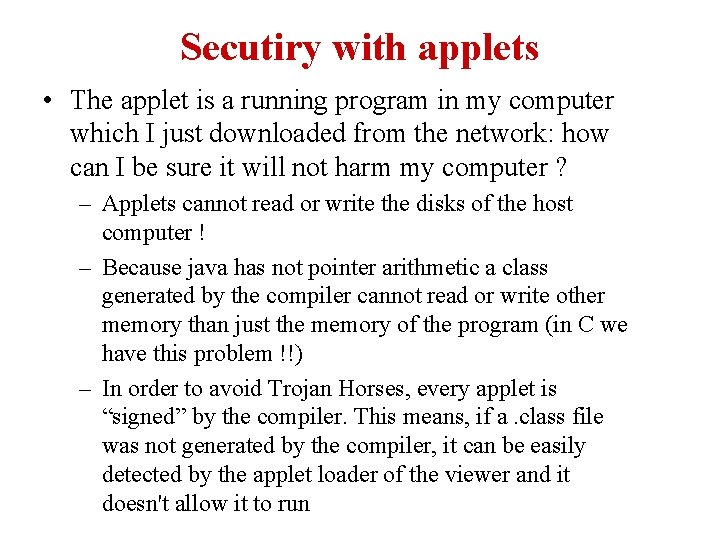
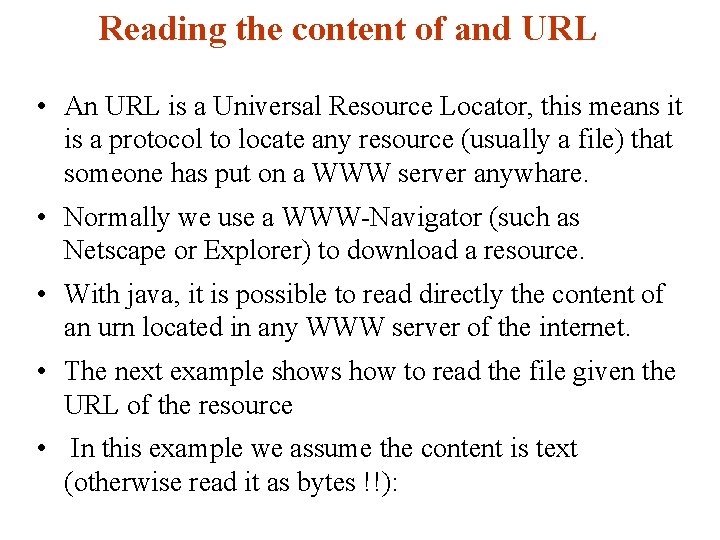
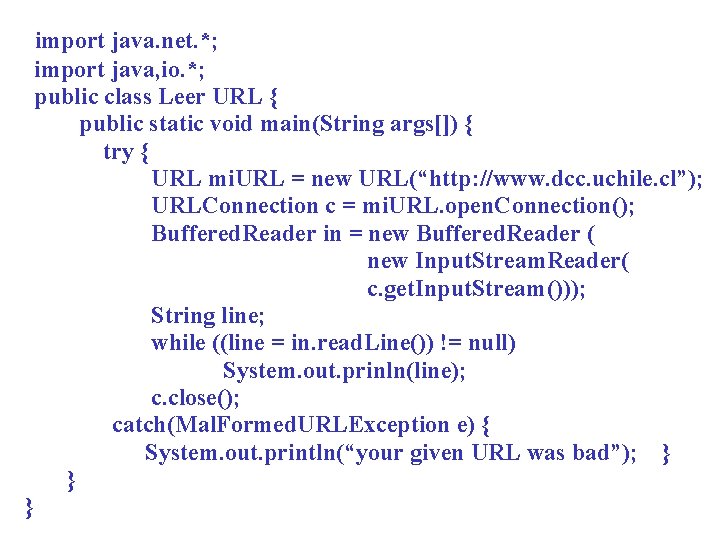
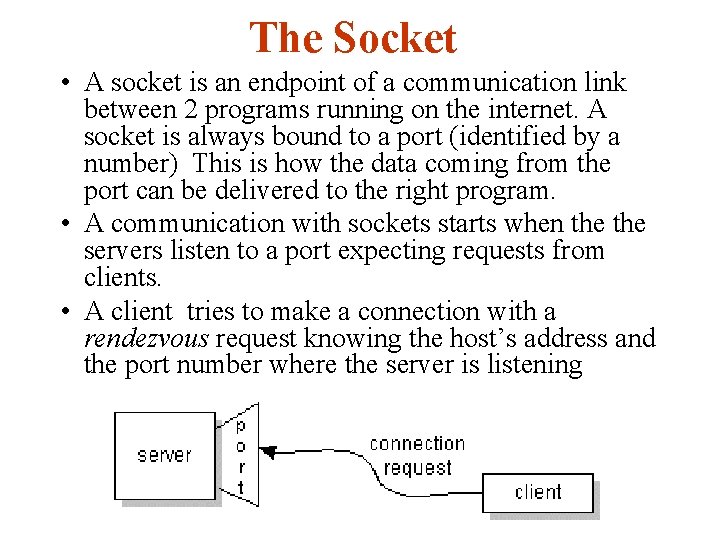
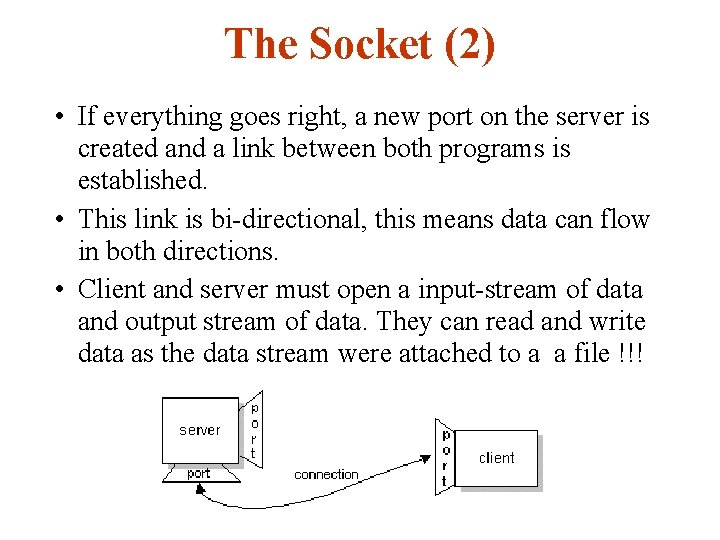
![Sockets on the Server side public class Arch. Servidor { public static void main(String[] Sockets on the Server side public class Arch. Servidor { public static void main(String[]](https://slidetodoc.com/presentation_image/e7d18a9892522900cf8e625e37764a51/image-12.jpg)
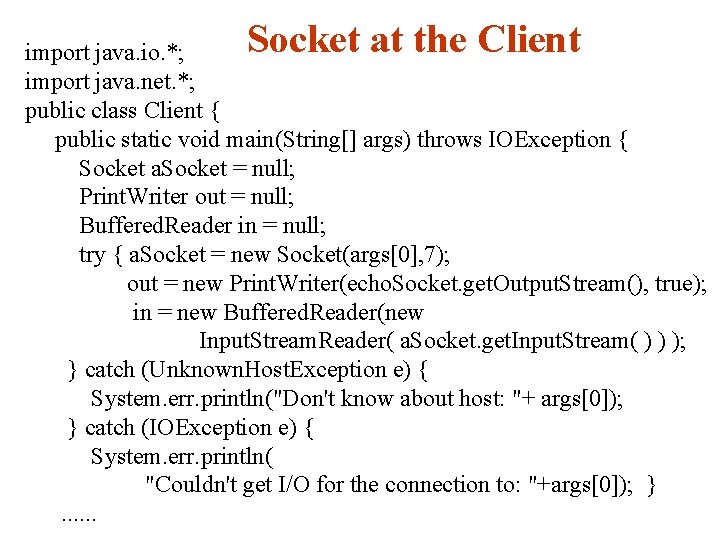
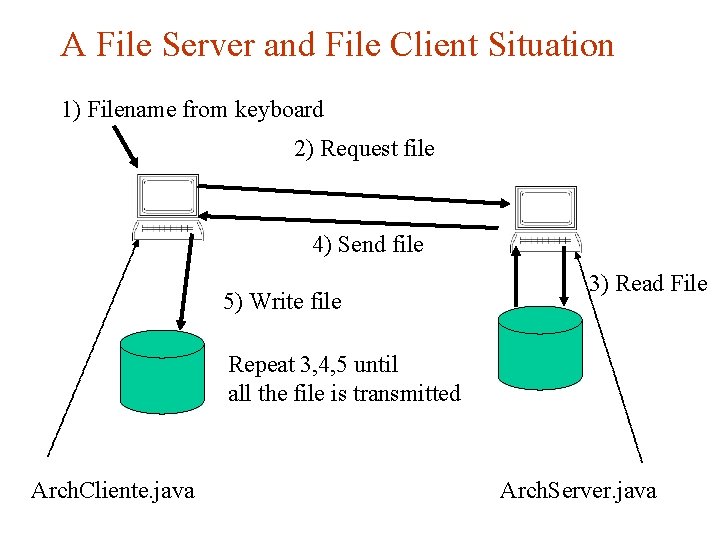
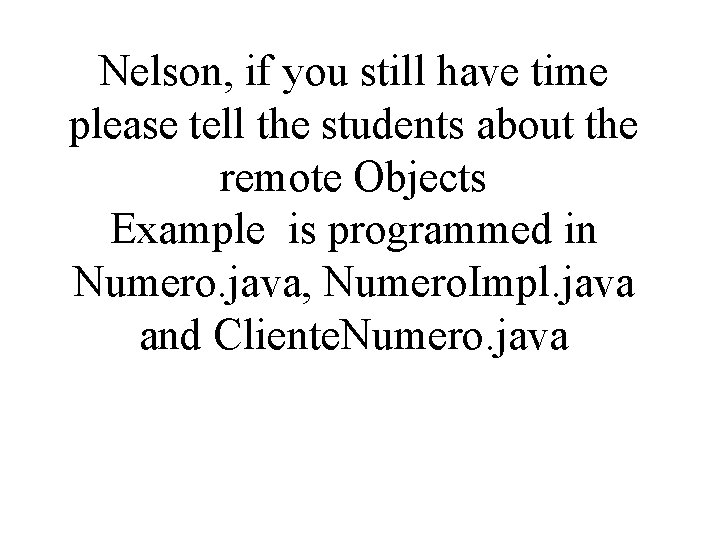
- Slides: 15
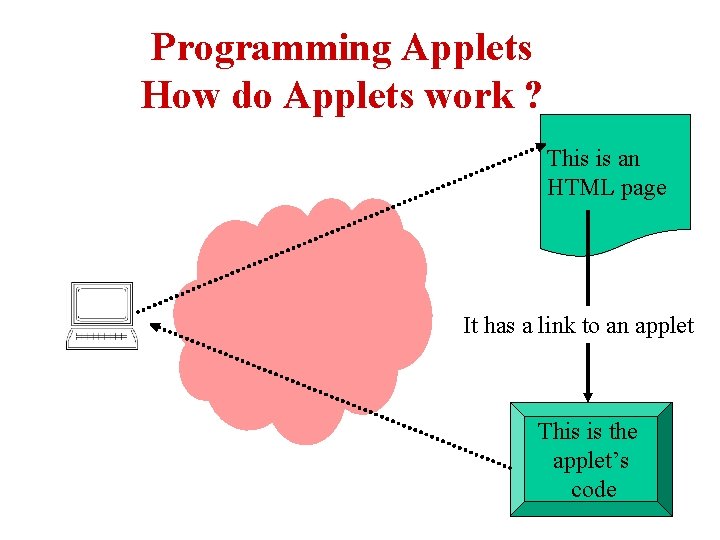
Programming Applets How do Applets work ? This is an HTML page It has a link to an applet This is the applet’s code
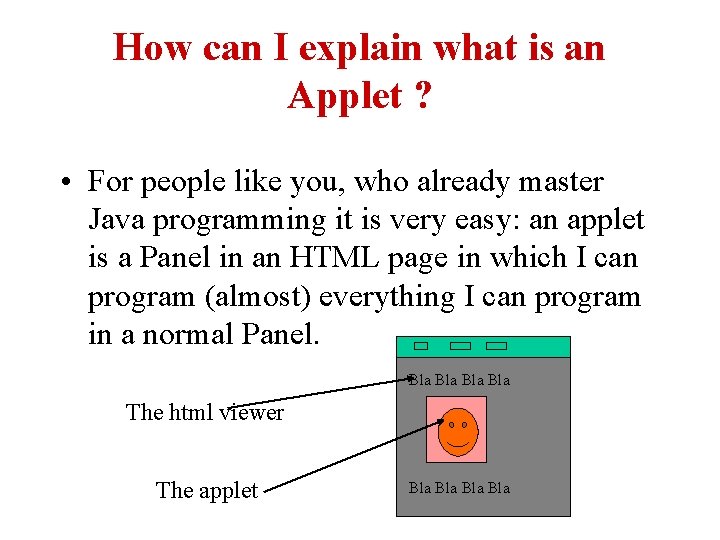
How can I explain what is an Applet ? • For people like you, who already master Java programming it is very easy: an applet is a Panel in an HTML page in which I can program (almost) everything I can program in a normal Panel. Bla Bla The html viewer The applet Bla Bla
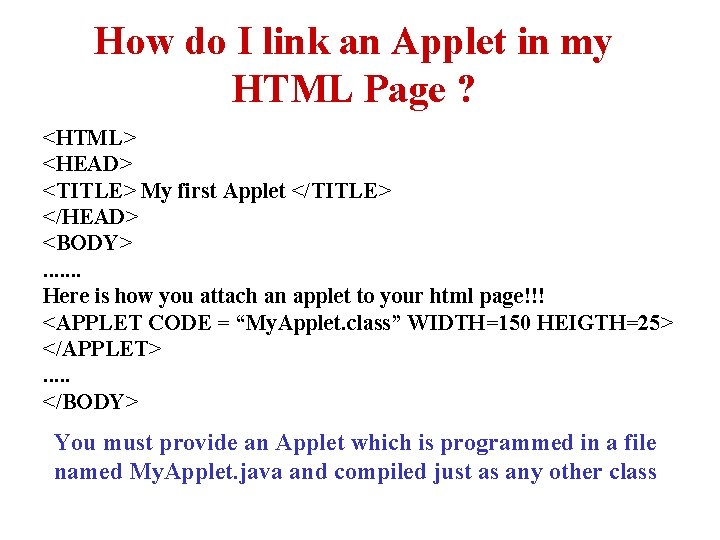
How do I link an Applet in my HTML Page ? <HTML> <HEAD> <TITLE> My first Applet </TITLE> </HEAD> <BODY>. . . . Here is how you attach an applet to your html page!!! <APPLET CODE = “My. Applet. class” WIDTH=150 HEIGTH=25> </APPLET>. . . </BODY> You must provide an Applet which is programmed in a file named My. Applet. java and compiled just as any other class
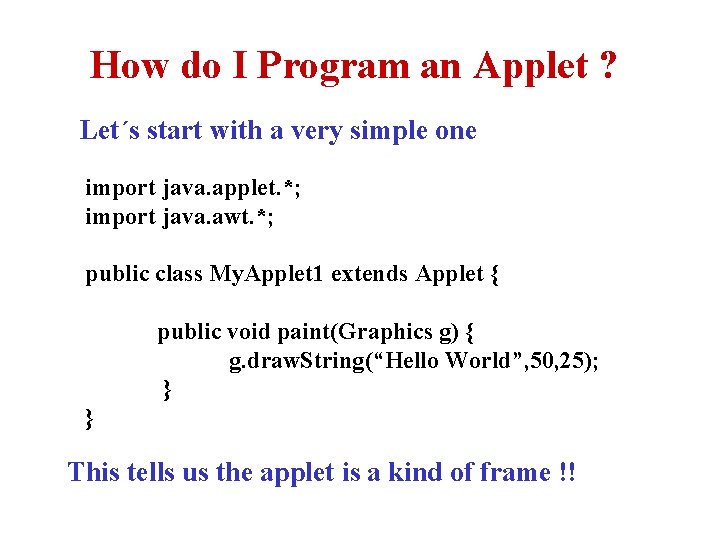
How do I Program an Applet ? Let´s start with a very simple one import java. applet. *; import java. awt. *; public class My. Applet 1 extends Applet { public void paint(Graphics g) { g. draw. String(“Hello World”, 50, 25); } } This tells us the applet is a kind of frame !!
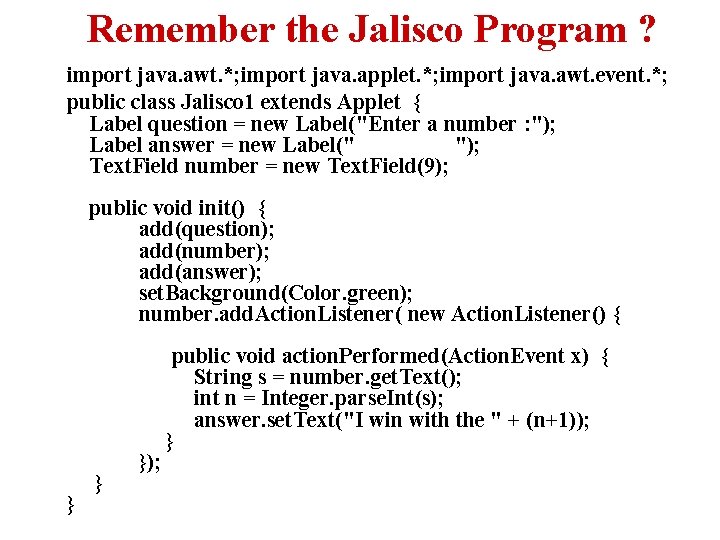
Remember the Jalisco Program ? import java. awt. *; import java. applet. *; import java. awt. event. *; public class Jalisco 1 extends Applet { Label question = new Label("Enter a number : "); Label answer = new Label(" "); Text. Field number = new Text. Field(9); public void init() { add(question); add(number); add(answer); set. Background(Color. green); number. add. Action. Listener( new Action. Listener() { } } }); public void action. Performed(Action. Event x) { String s = number. get. Text(); int n = Integer. parse. Int(s); answer. set. Text("I win with the " + (n+1)); }
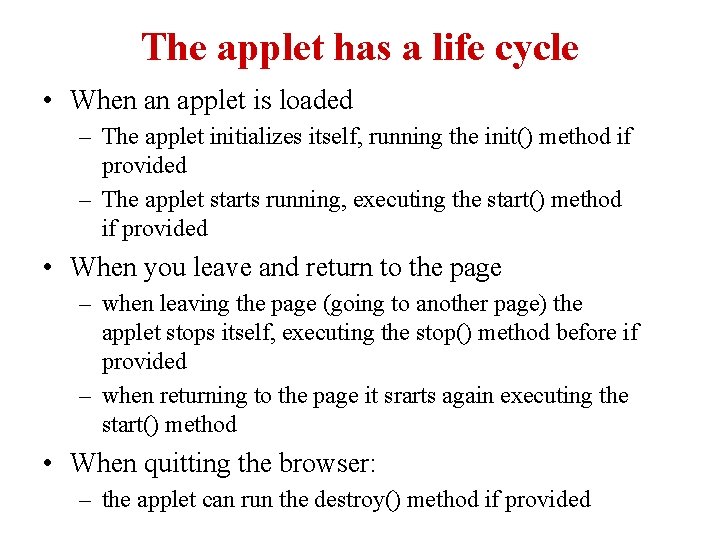
The applet has a life cycle • When an applet is loaded – The applet initializes itself, running the init() method if provided – The applet starts running, executing the start() method if provided • When you leave and return to the page – when leaving the page (going to another page) the applet stops itself, executing the stop() method before if provided – when returning to the page it srarts again executing the start() method • When quitting the browser: – the applet can run the destroy() method if provided
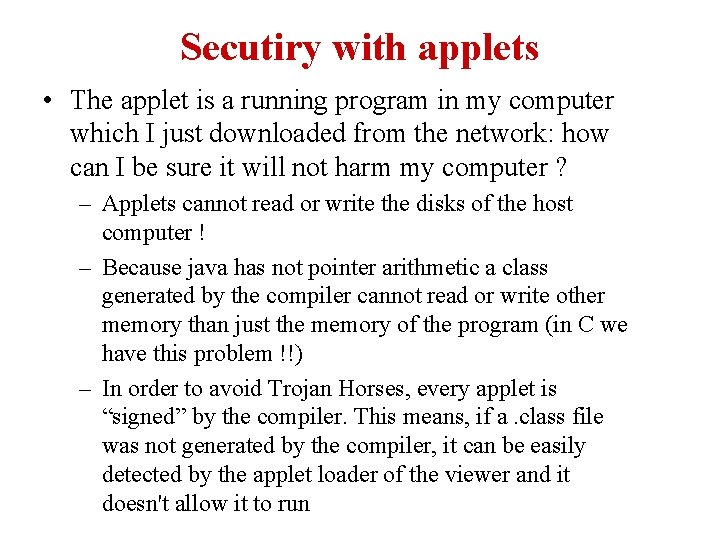
Secutiry with applets • The applet is a running program in my computer which I just downloaded from the network: how can I be sure it will not harm my computer ? – Applets cannot read or write the disks of the host computer ! – Because java has not pointer arithmetic a class generated by the compiler cannot read or write other memory than just the memory of the program (in C we have this problem !!) – In order to avoid Trojan Horses, every applet is “signed” by the compiler. This means, if a. class file was not generated by the compiler, it can be easily detected by the applet loader of the viewer and it doesn't allow it to run
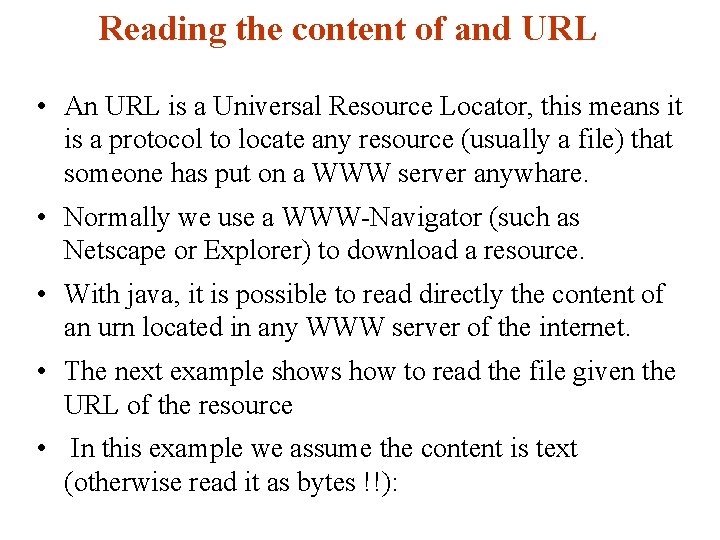
Reading the content of and URL • An URL is a Universal Resource Locator, this means it is a protocol to locate any resource (usually a file) that someone has put on a WWW server anywhare. • Normally we use a WWW-Navigator (such as Netscape or Explorer) to download a resource. • With java, it is possible to read directly the content of an urn located in any WWW server of the internet. • The next example shows how to read the file given the URL of the resource • In this example we assume the content is text (otherwise read it as bytes !!):
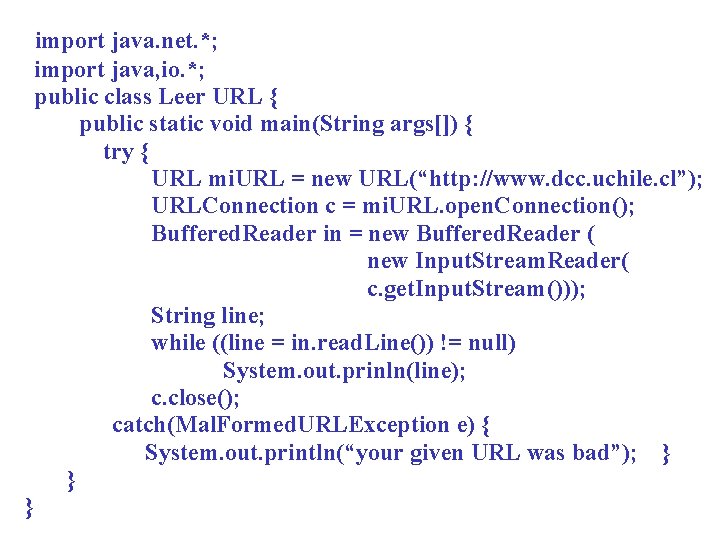
import java. net. *; import java, io. *; public class Leer URL { public static void main(String args[]) { try { URL mi. URL = new URL(“http: //www. dcc. uchile. cl”); URLConnection c = mi. URL. open. Connection(); Buffered. Reader in = new Buffered. Reader ( new Input. Stream. Reader( c. get. Input. Stream())); String line; while ((line = in. read. Line()) != null) System. out. prinln(line); c. close(); catch(Mal. Formed. URLException e) { System. out. println(“your given URL was bad”); } } }
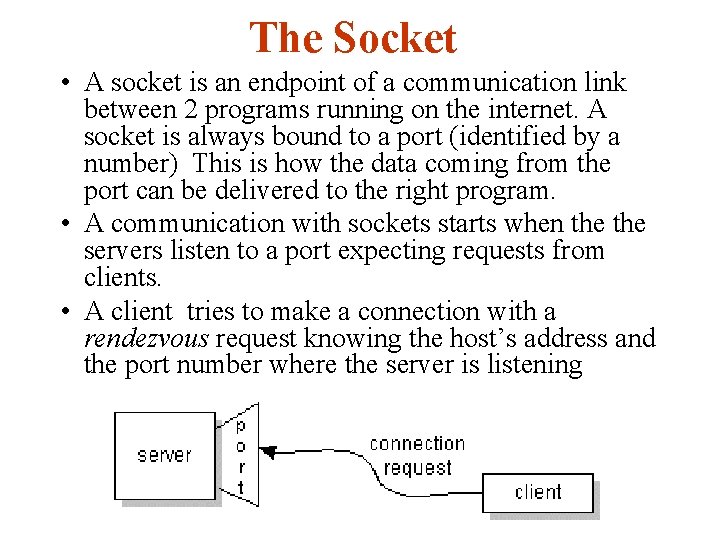
The Socket • A socket is an endpoint of a communication link between 2 programs running on the internet. A socket is always bound to a port (identified by a number) This is how the data coming from the port can be delivered to the right program. • A communication with sockets starts when the servers listen to a port expecting requests from clients. • A client tries to make a connection with a rendezvous request knowing the host’s address and the port number where the server is listening
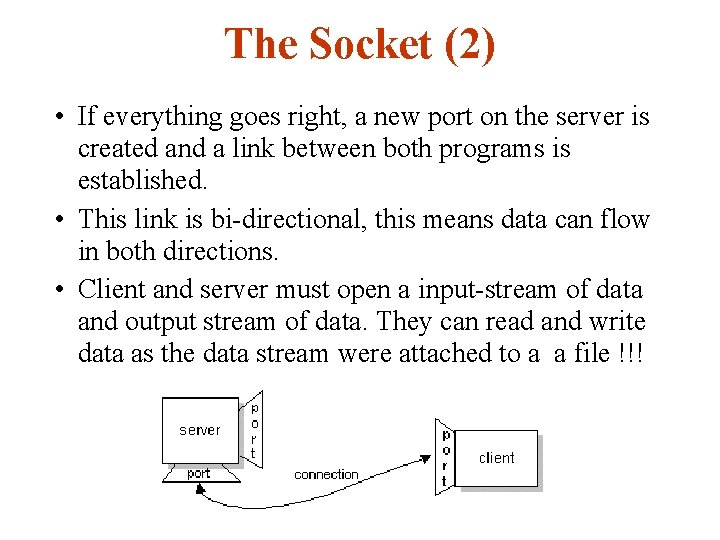
The Socket (2) • If everything goes right, a new port on the server is created and a link between both programs is established. • This link is bi-directional, this means data can flow in both directions. • Client and server must open a input-stream of data and output stream of data. They can read and write data as the data stream were attached to a a file !!!
![Sockets on the Server side public class Arch Servidor public static void mainString Sockets on the Server side public class Arch. Servidor { public static void main(String[]](https://slidetodoc.com/presentation_image/e7d18a9892522900cf8e625e37764a51/image-12.jpg)
Sockets on the Server side public class Arch. Servidor { public static void main(String[] args) throws IOException { Socket cs = null; Server. Socket ss = new Server. Socket(4444); System. out. println(”waiting for a client"); cs = ss. accept(); Buffered. Reader in. Socket = new Buffered. Reader( new Input. Stream. Reader(cs. get. Input. Stream())); Print. Writer out. Socket = new Print. Writer( cs. get. Output. Stream(), true); Now the program can read lines from the in. Socket and write lines to the out. Socket
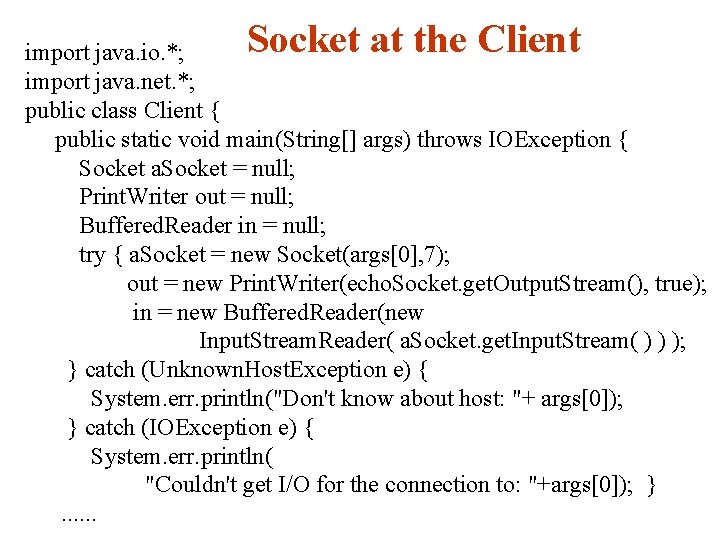
Socket at the Client import java. io. *; import java. net. *; public class Client { public static void main(String[] args) throws IOException { Socket a. Socket = null; Print. Writer out = null; Buffered. Reader in = null; try { a. Socket = new Socket(args[0], 7); out = new Print. Writer(echo. Socket. get. Output. Stream(), true); in = new Buffered. Reader(new Input. Stream. Reader( a. Socket. get. Input. Stream( ) ) ); } catch (Unknown. Host. Exception e) { System. err. println("Don't know about host: "+ args[0]); } catch (IOException e) { System. err. println( "Couldn't get I/O for the connection to: "+args[0]); }. . .
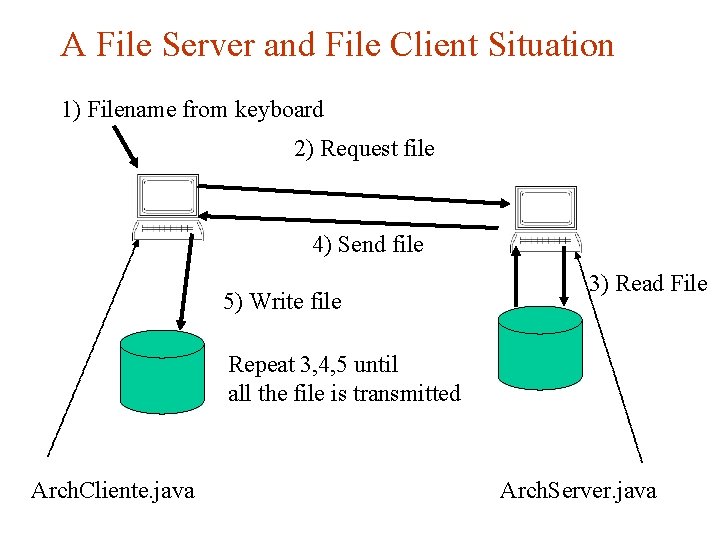
A File Server and File Client Situation 1) Filename from keyboard 2) Request file 4) Send file 5) Write file 3) Read File Repeat 3, 4, 5 until all the file is transmitted Arch. Cliente. java Arch. Server. java
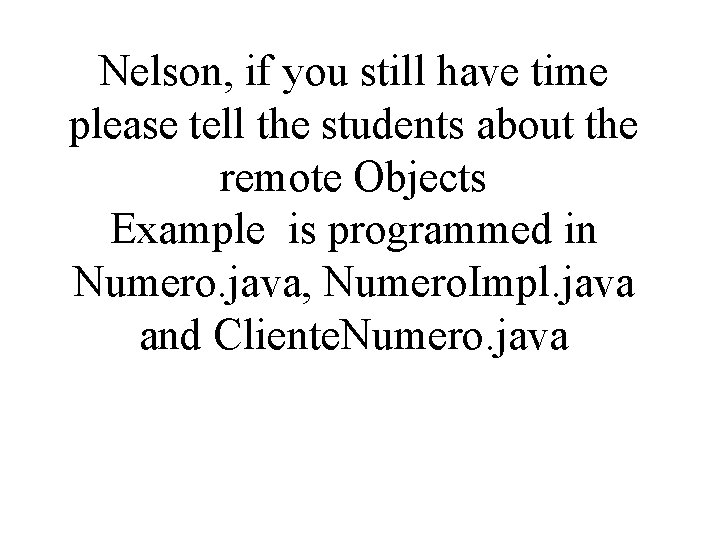
Nelson, if you still have time please tell the students about the remote Objects Example is programmed in Numero. java, Numero. Impl. java and Cliente. Numero. java