Processes Creation and Threads Creation of Processes Creation
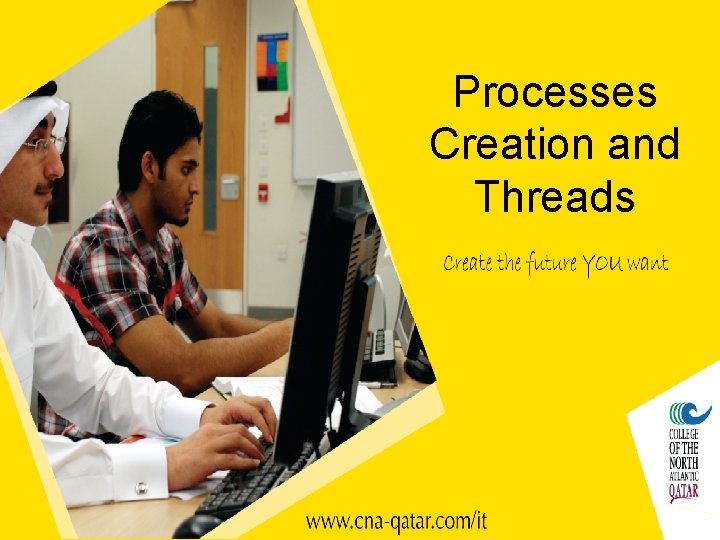
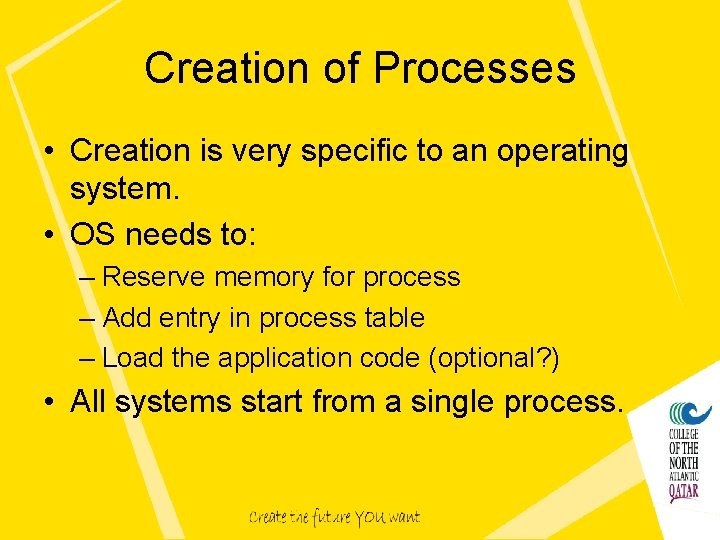
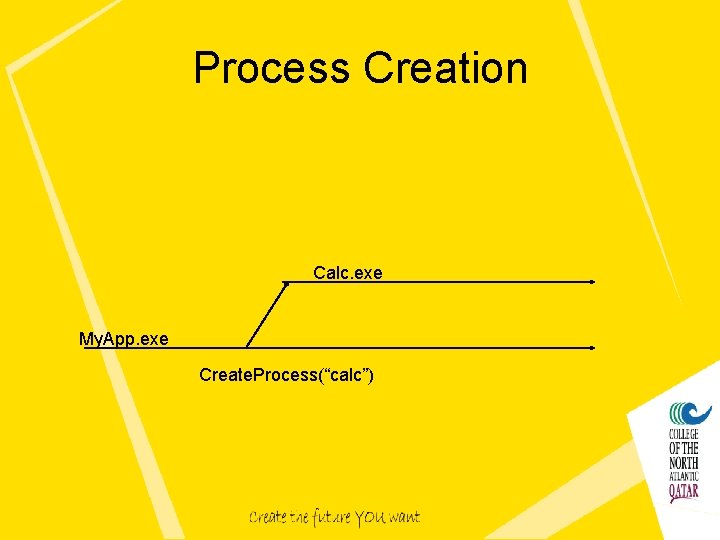
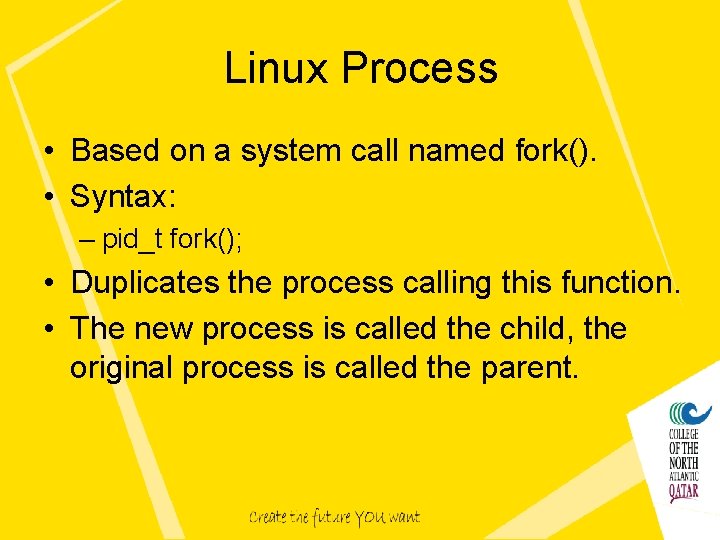
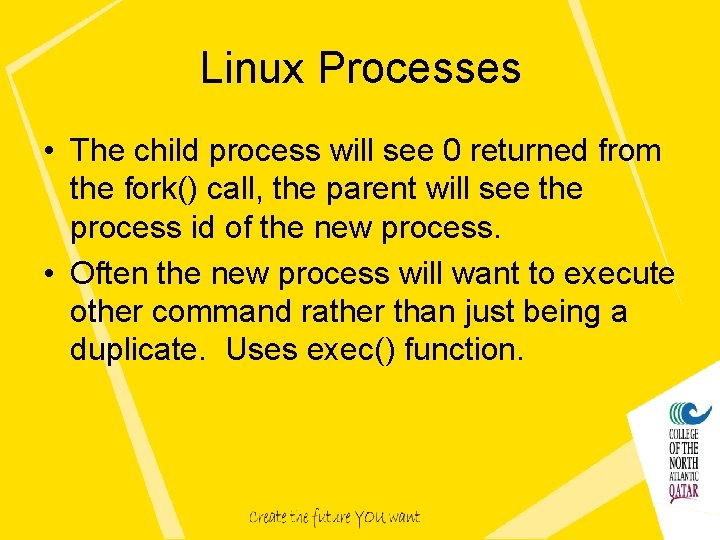
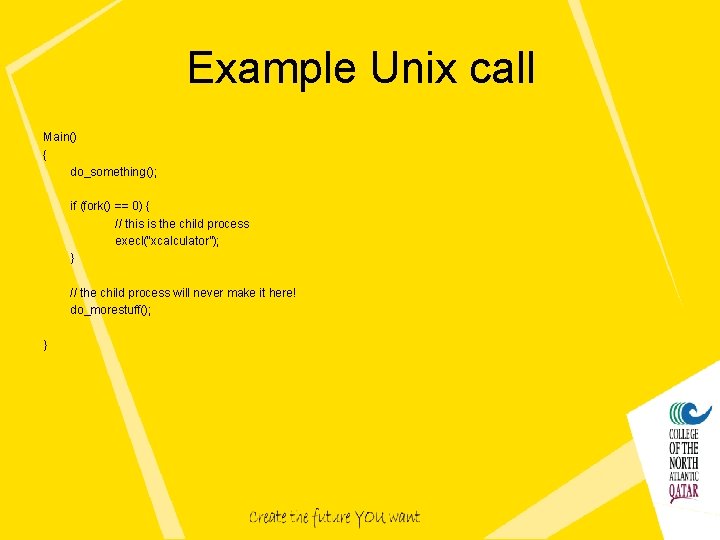
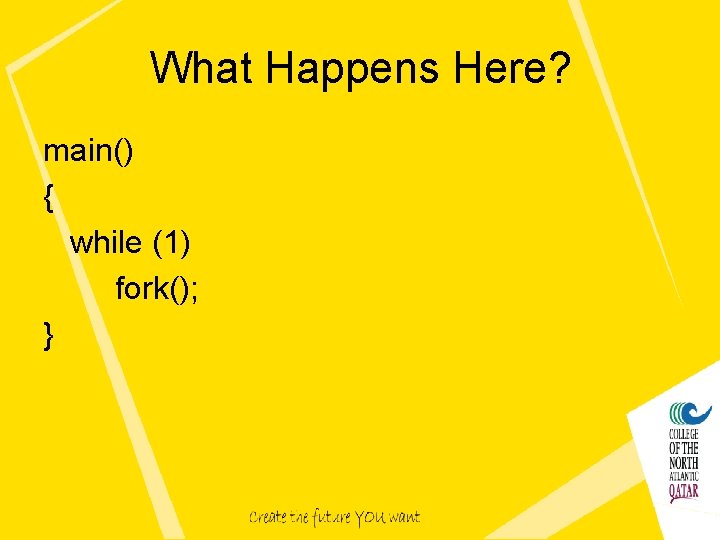
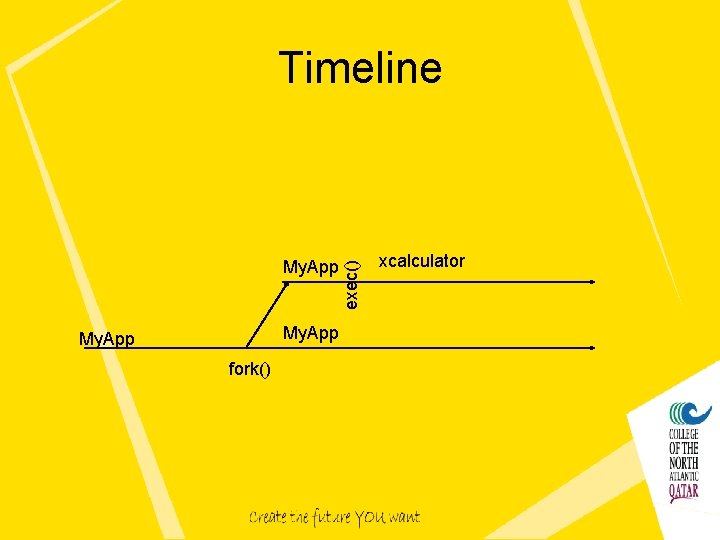
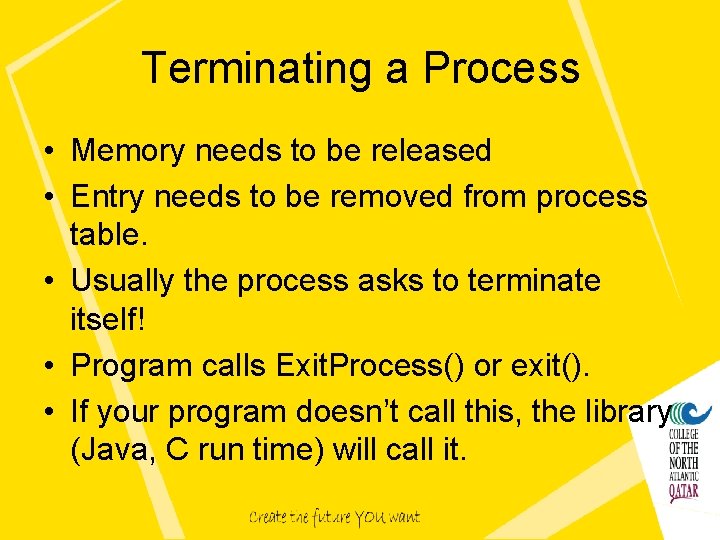
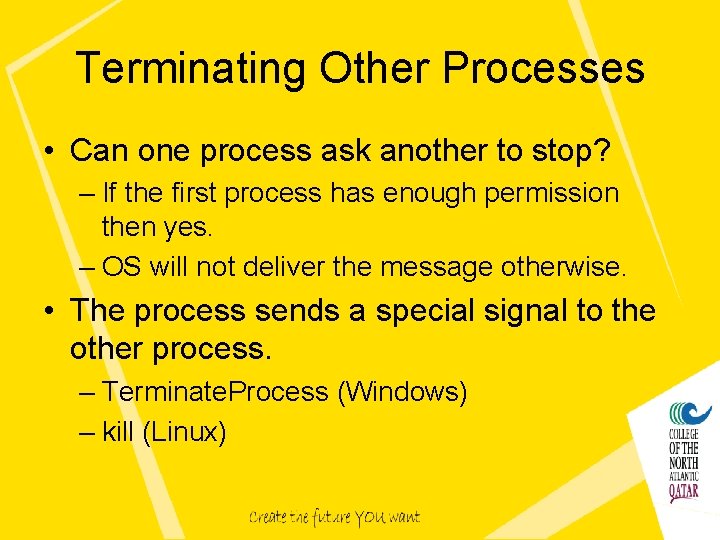
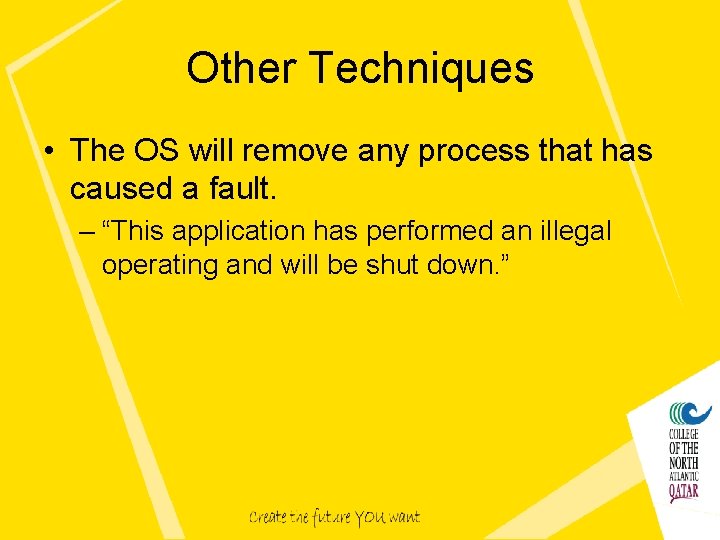
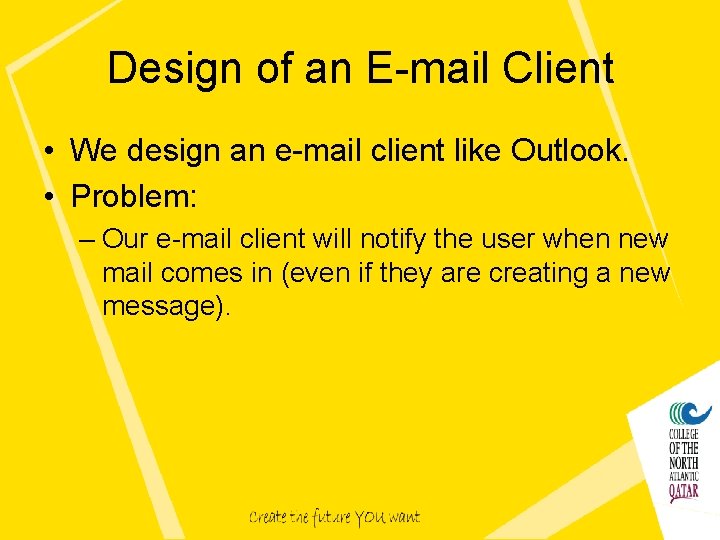
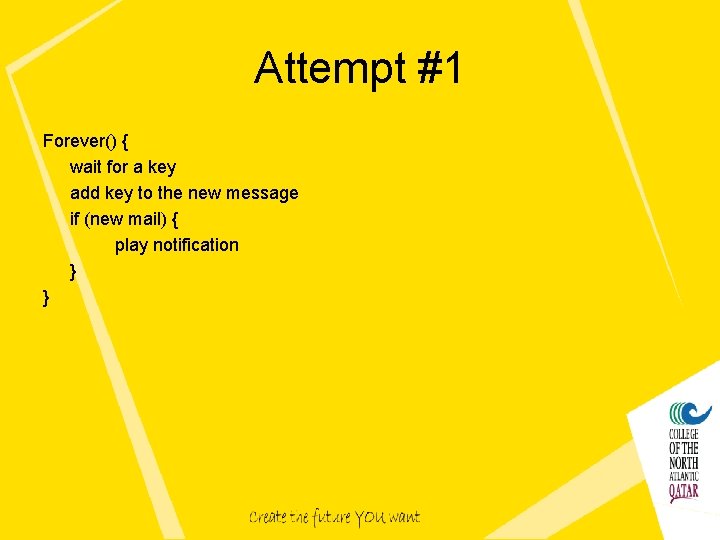
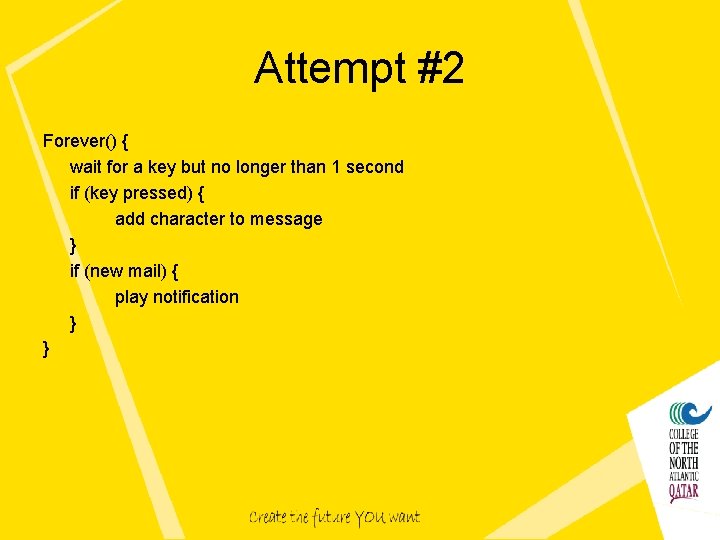
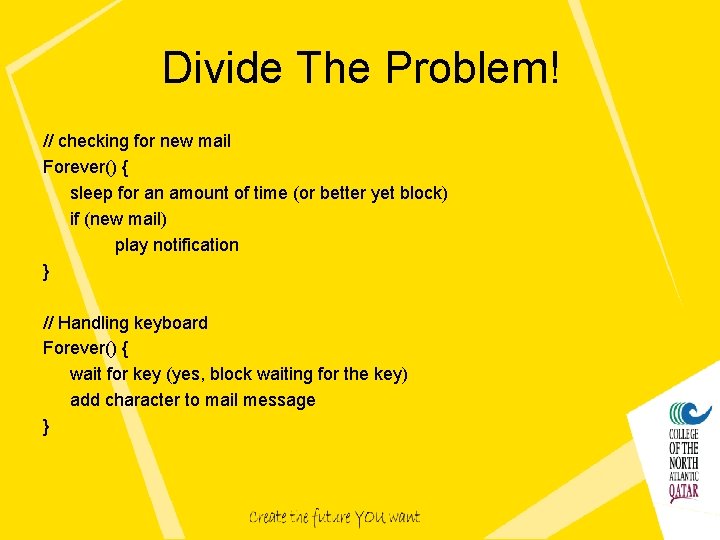
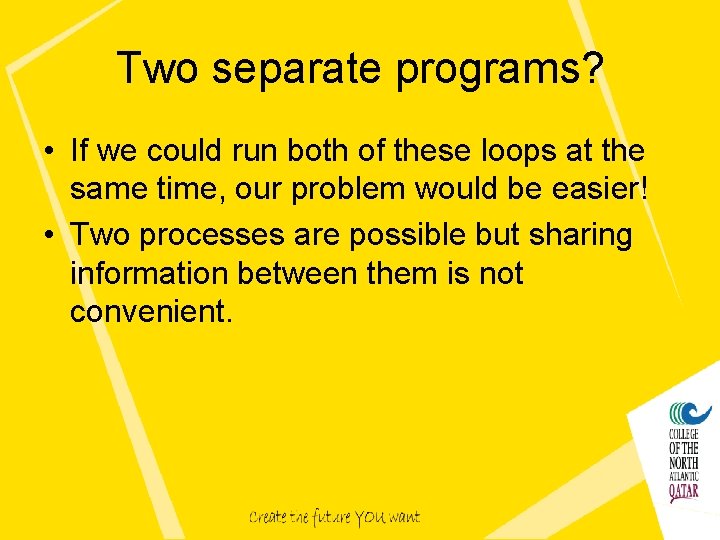
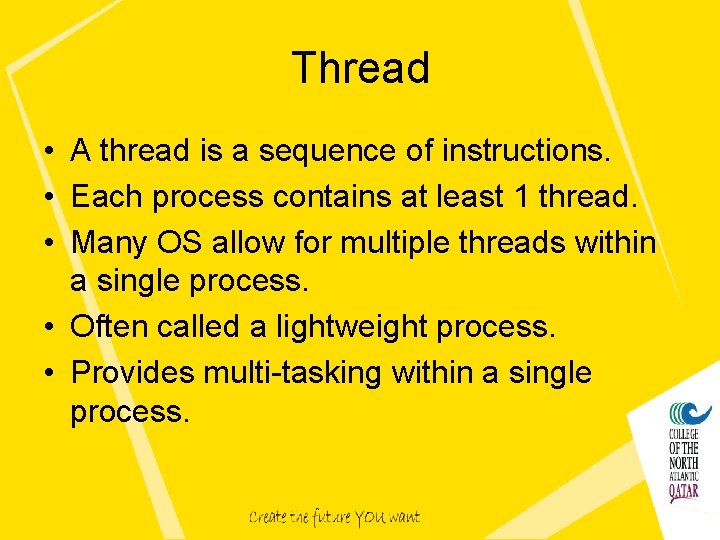
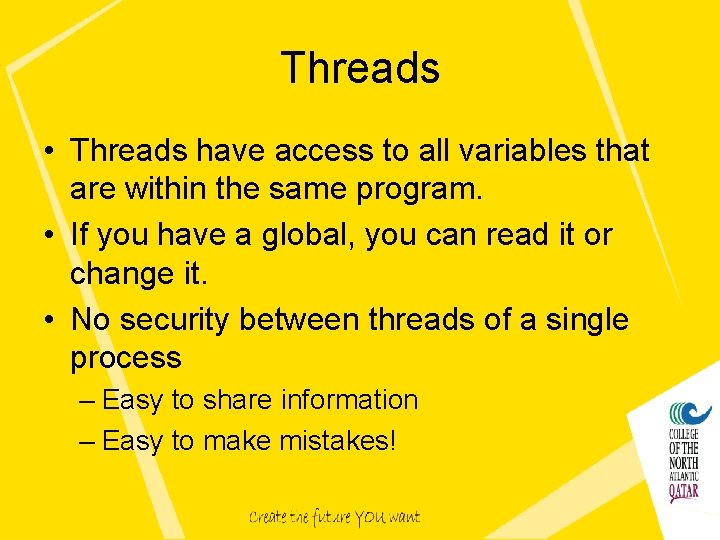
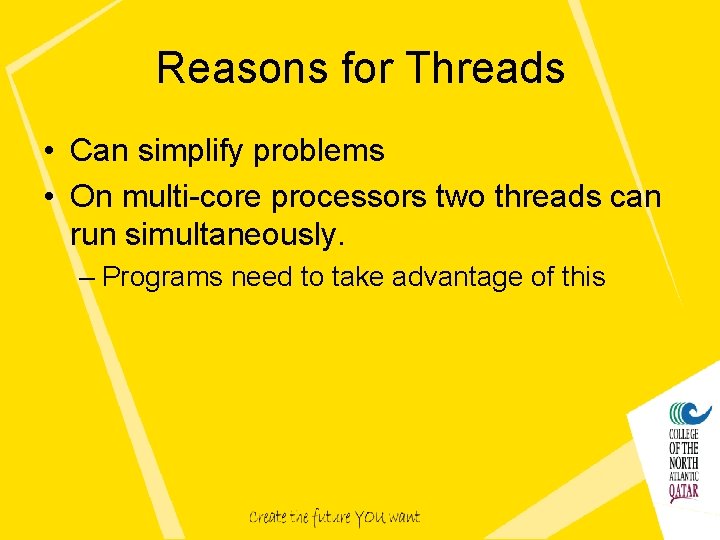
- Slides: 19
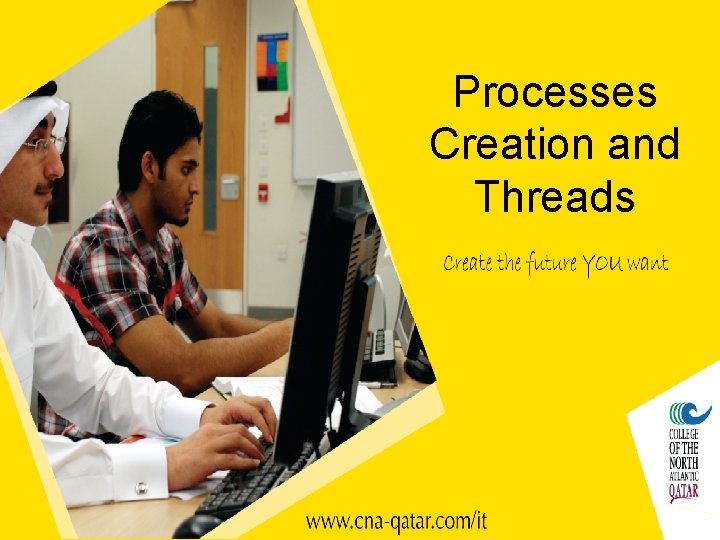
Processes Creation and Threads
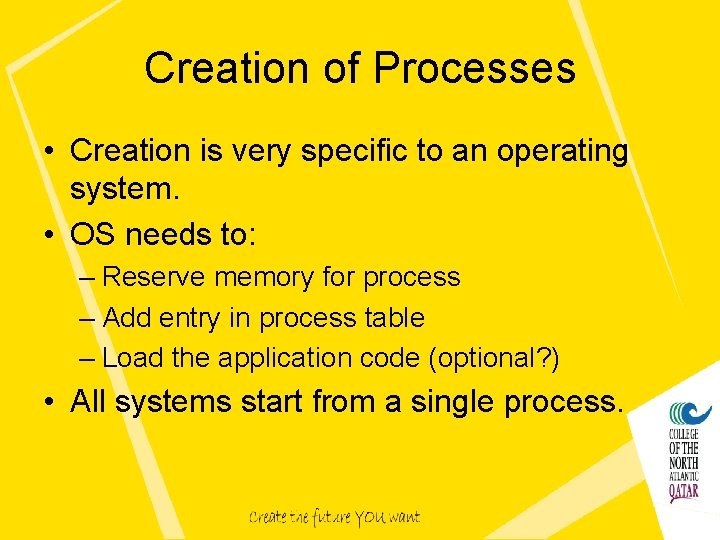
Creation of Processes • Creation is very specific to an operating system. • OS needs to: – Reserve memory for process – Add entry in process table – Load the application code (optional? ) • All systems start from a single process.
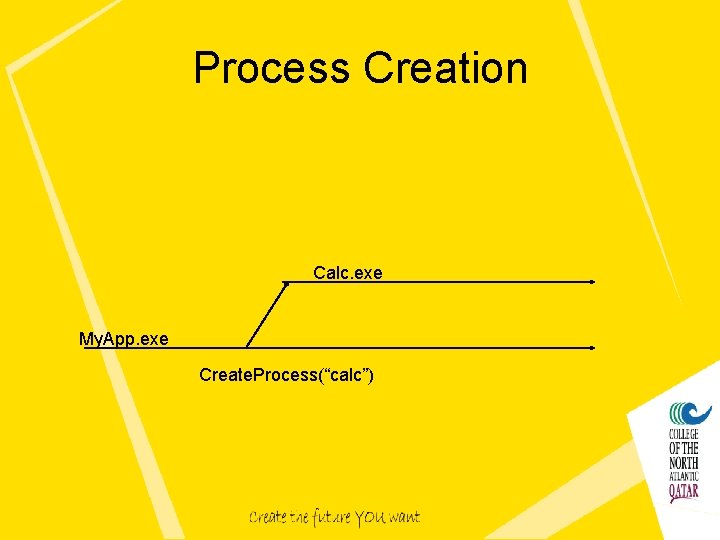
Process Creation Calc. exe My. App. exe Create. Process(“calc”)
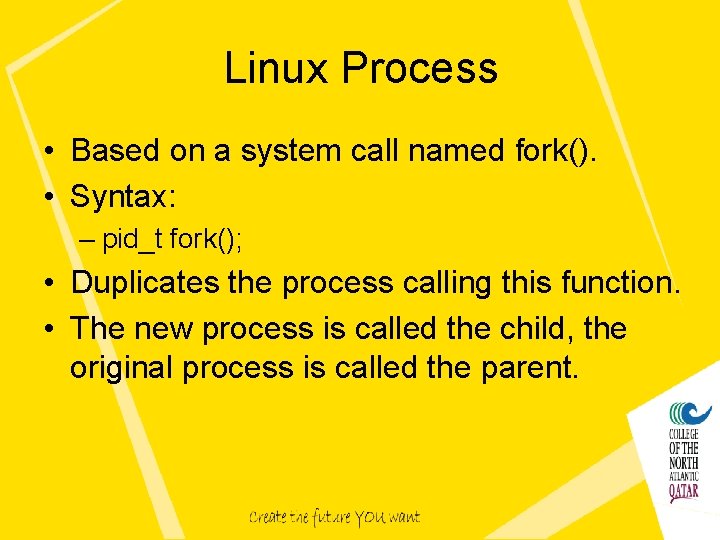
Linux Process • Based on a system call named fork(). • Syntax: – pid_t fork(); • Duplicates the process calling this function. • The new process is called the child, the original process is called the parent.
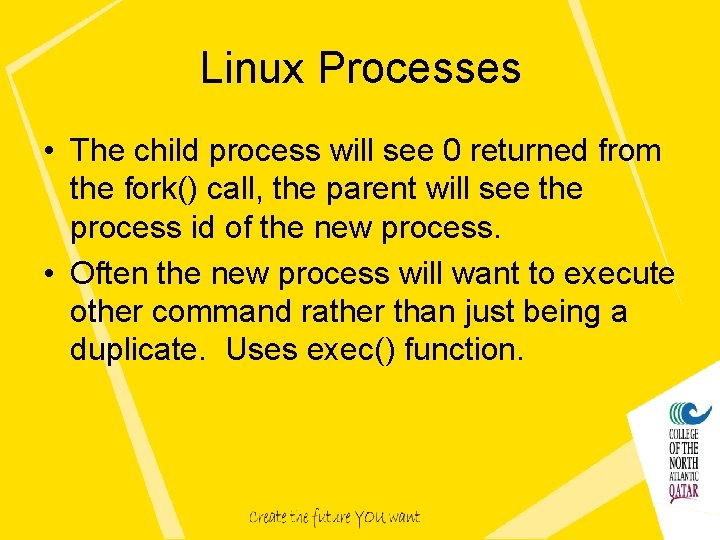
Linux Processes • The child process will see 0 returned from the fork() call, the parent will see the process id of the new process. • Often the new process will want to execute other command rather than just being a duplicate. Uses exec() function.
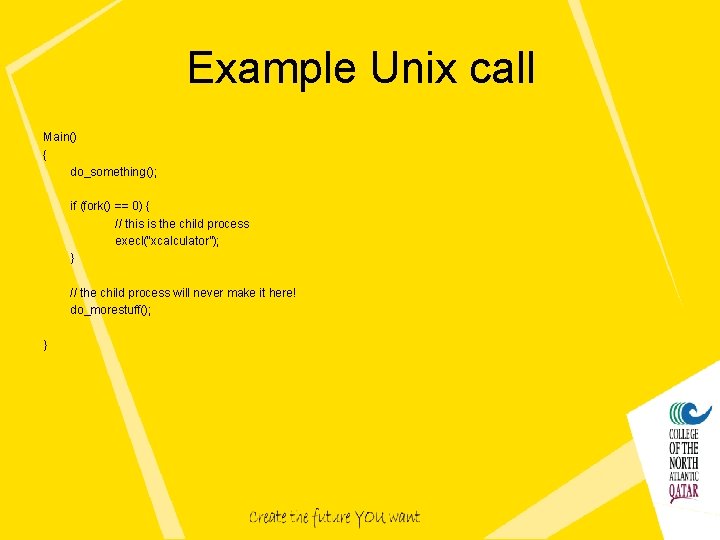
Example Unix call Main() { do_something(); if (fork() == 0) { // this is the child process execl(“xcalculator”); } // the child process will never make it here! do_morestuff(); }
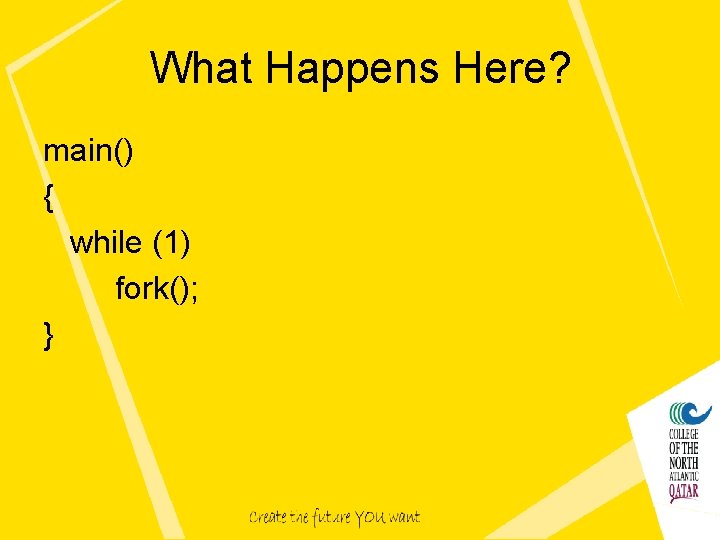
What Happens Here? main() { while (1) fork(); }
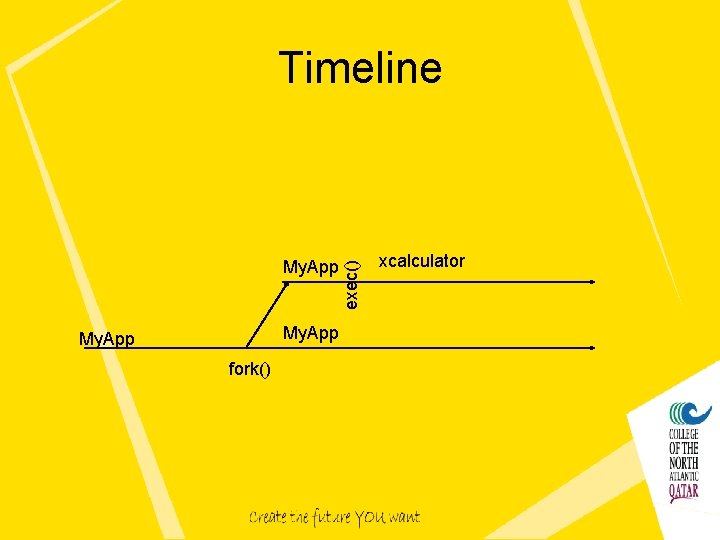
My. App fork() exec() Timeline xcalculator
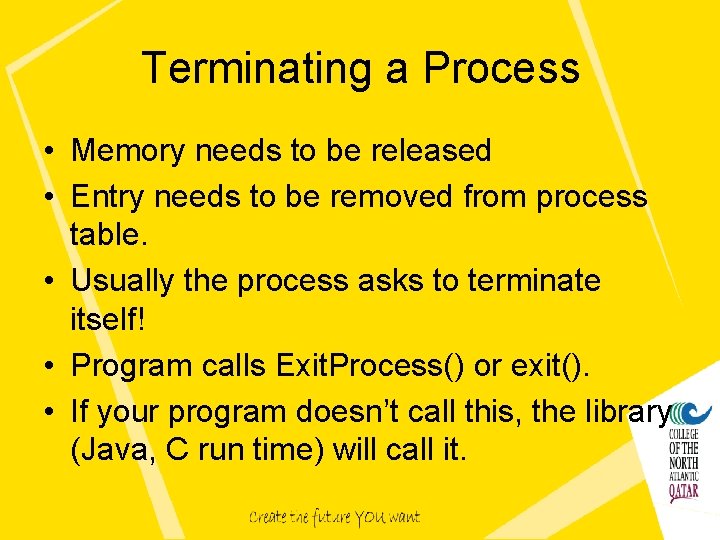
Terminating a Process • Memory needs to be released • Entry needs to be removed from process table. • Usually the process asks to terminate itself! • Program calls Exit. Process() or exit(). • If your program doesn’t call this, the library (Java, C run time) will call it.
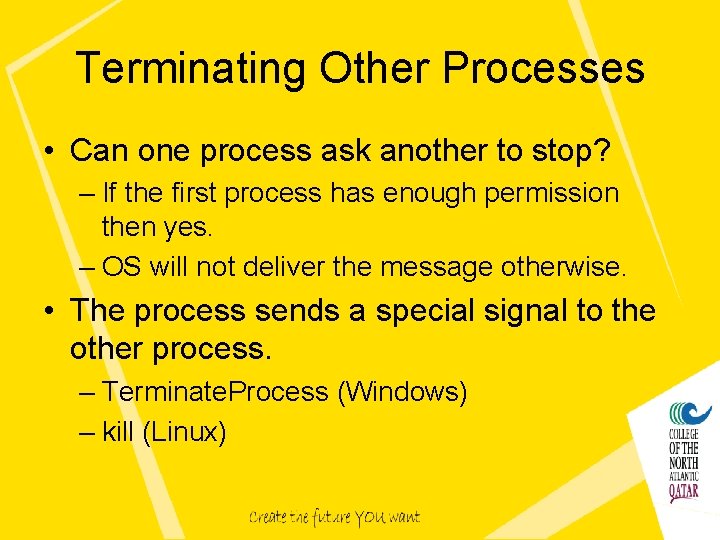
Terminating Other Processes • Can one process ask another to stop? – If the first process has enough permission then yes. – OS will not deliver the message otherwise. • The process sends a special signal to the other process. – Terminate. Process (Windows) – kill (Linux)
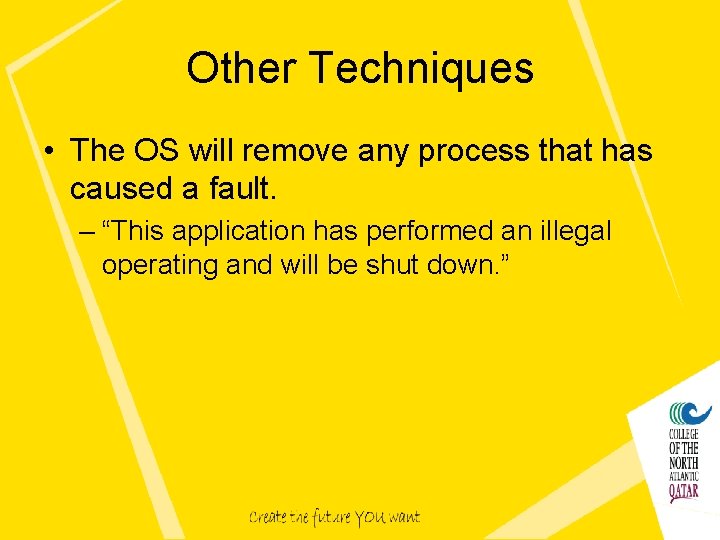
Other Techniques • The OS will remove any process that has caused a fault. – “This application has performed an illegal operating and will be shut down. ”
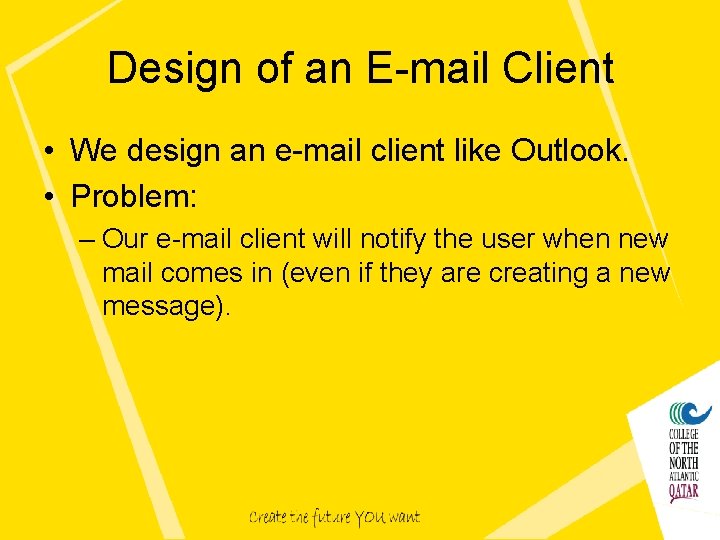
Design of an E-mail Client • We design an e-mail client like Outlook. • Problem: – Our e-mail client will notify the user when new mail comes in (even if they are creating a new message).
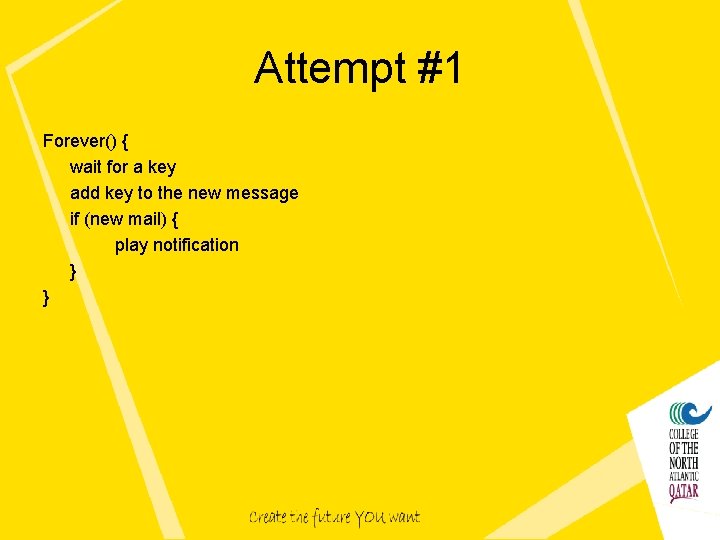
Attempt #1 Forever() { wait for a key add key to the new message if (new mail) { play notification } }
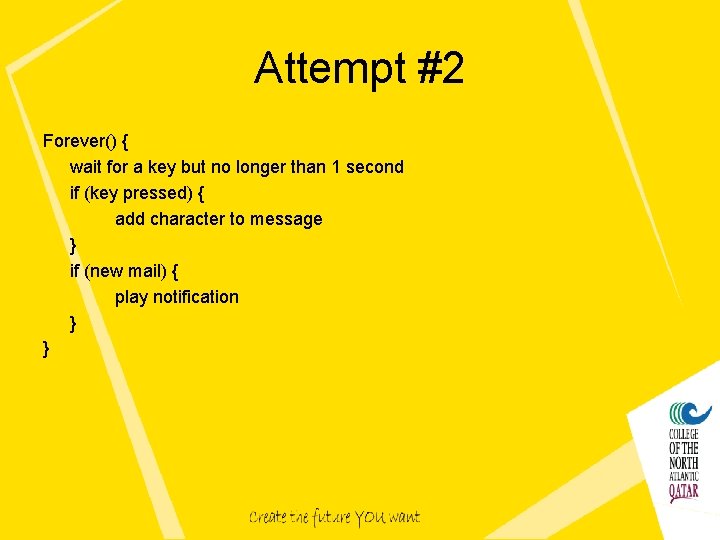
Attempt #2 Forever() { wait for a key but no longer than 1 second if (key pressed) { add character to message } if (new mail) { play notification } }
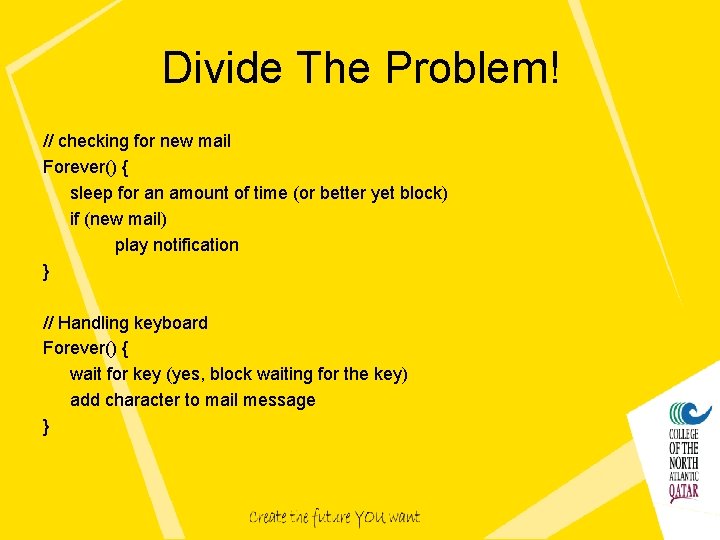
Divide The Problem! // checking for new mail Forever() { sleep for an amount of time (or better yet block) if (new mail) play notification } // Handling keyboard Forever() { wait for key (yes, block waiting for the key) add character to mail message }
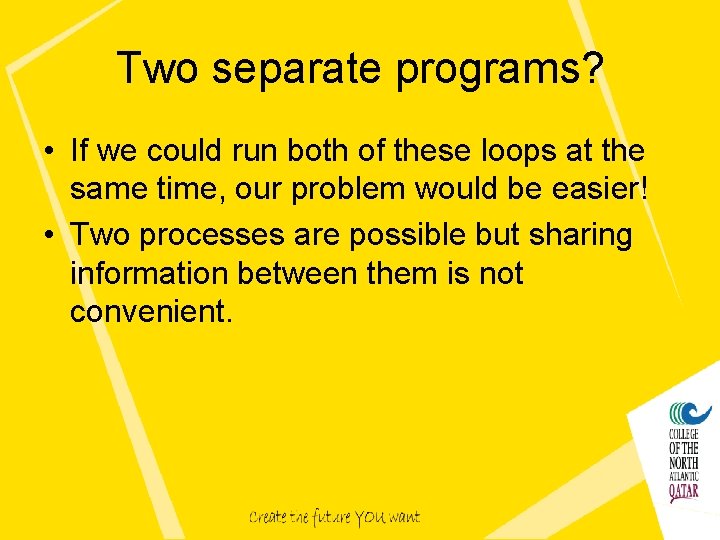
Two separate programs? • If we could run both of these loops at the same time, our problem would be easier! • Two processes are possible but sharing information between them is not convenient.
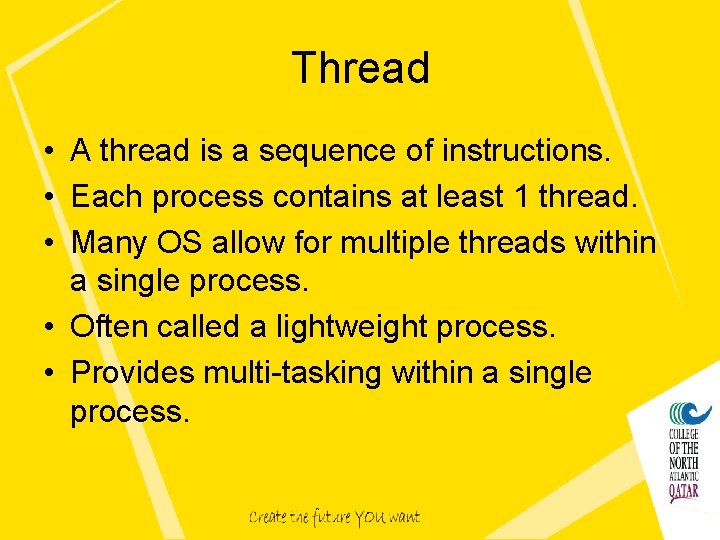
Thread • A thread is a sequence of instructions. • Each process contains at least 1 thread. • Many OS allow for multiple threads within a single process. • Often called a lightweight process. • Provides multi-tasking within a single process.
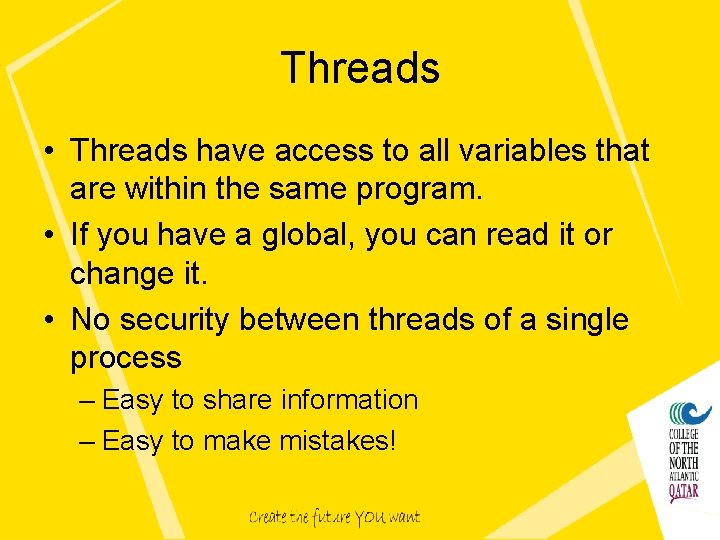
Threads • Threads have access to all variables that are within the same program. • If you have a global, you can read it or change it. • No security between threads of a single process – Easy to share information – Easy to make mistakes!
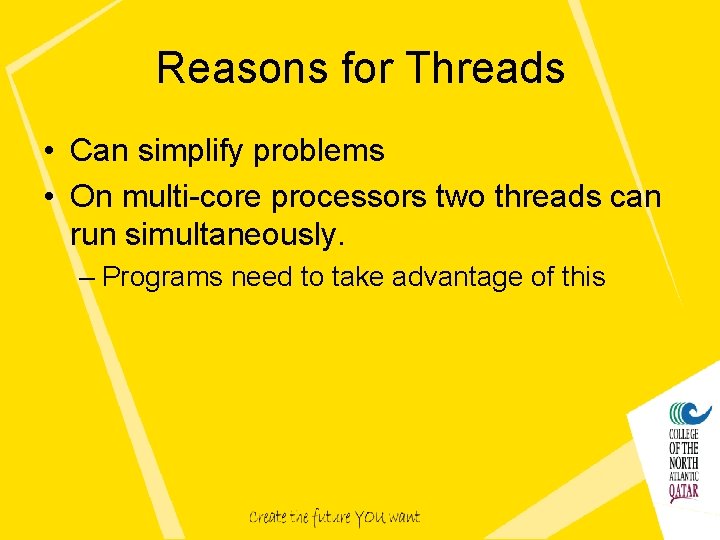
Reasons for Threads • Can simplify problems • On multi-core processors two threads can run simultaneously. – Programs need to take advantage of this
Threads vs processes
Threads vs processes
Concurrent processes are processes that
Process and threads
Process and threads
Sockets and threads
Process and thread management in operating system
C11 threads
Shared memory in java
Conventional representation of internal thread
Flexible flat material made by interlacing yarns
Escalonador de processos
Os threads
The vinaya pitaka is a sacred text of………….
Needle like threads of spongy bone
Helps press seams in tubes like sleeves
Golden thread strategy
Pintos priority scheduling
Posix threads in os
Threads java