Nested conditional statements Previously discussed Conditional statements discussed
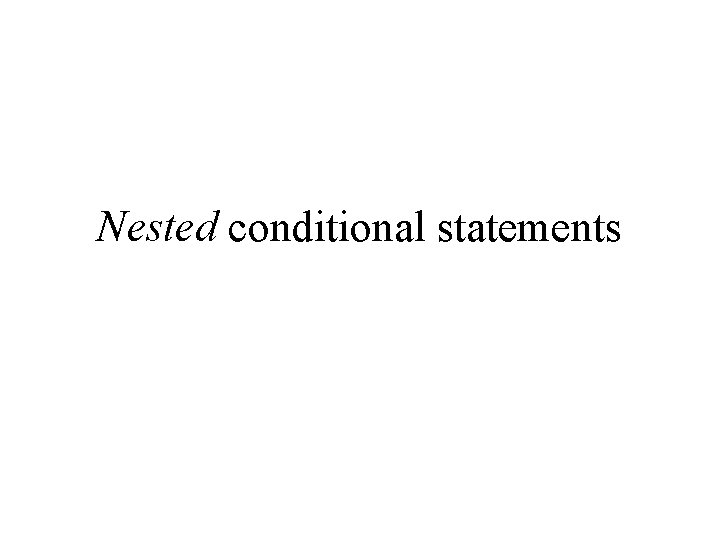
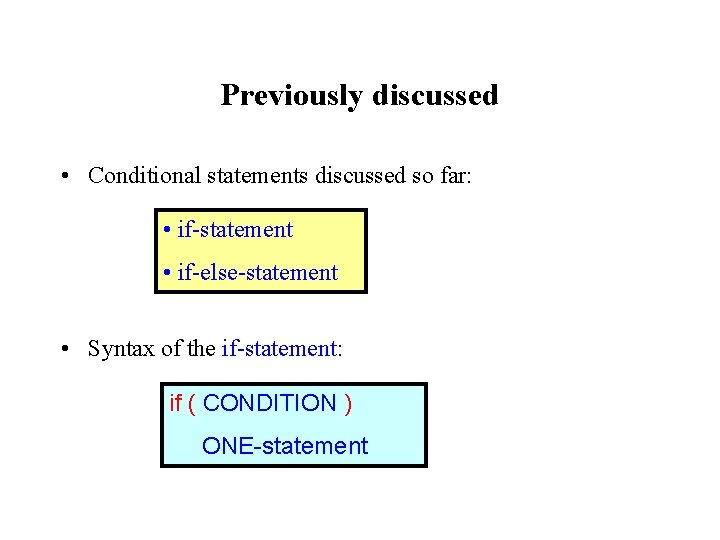
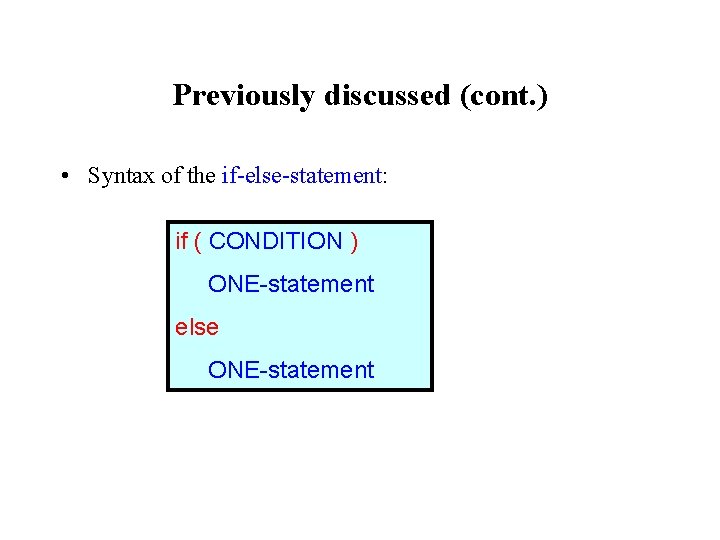
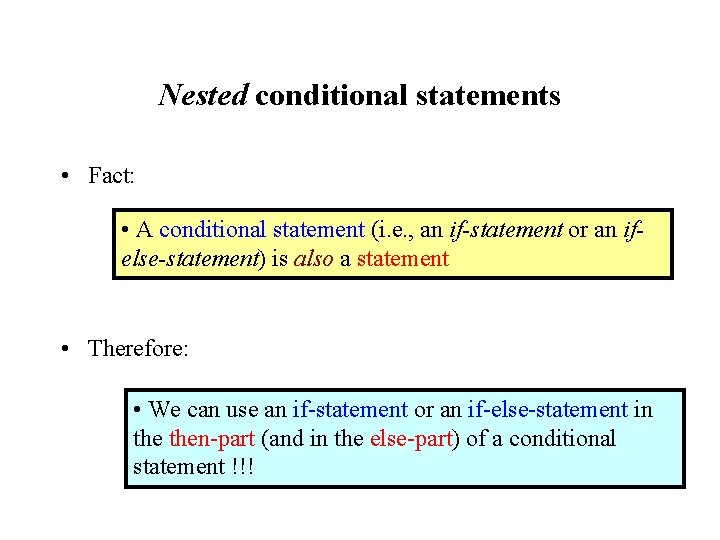
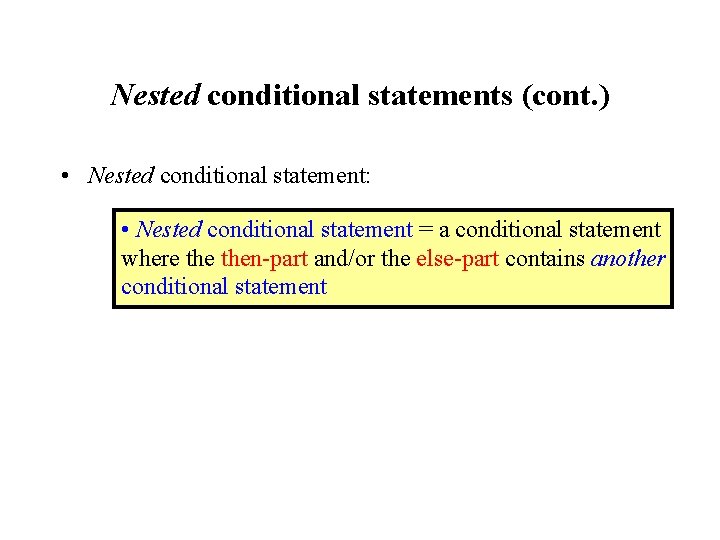
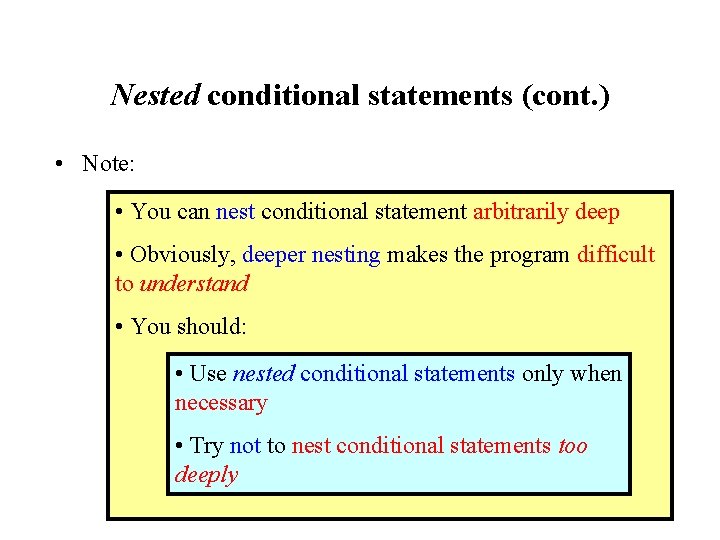
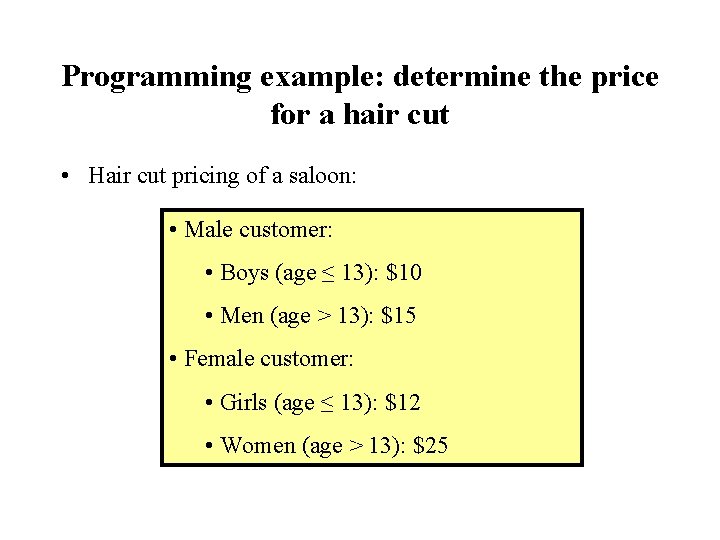
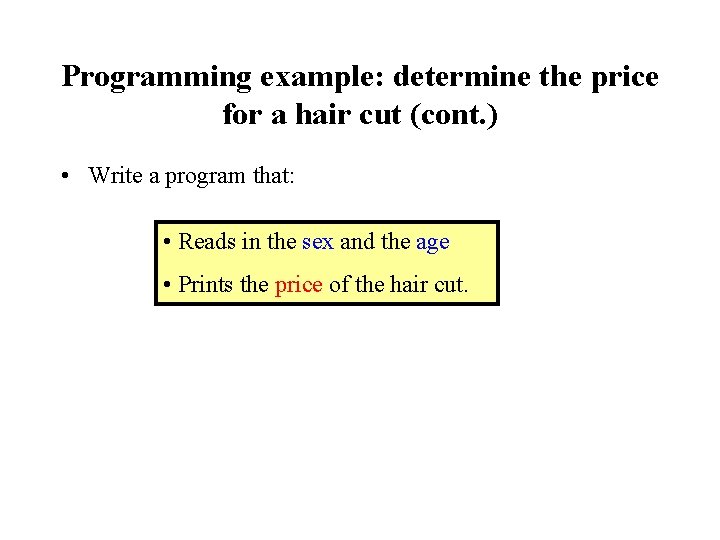
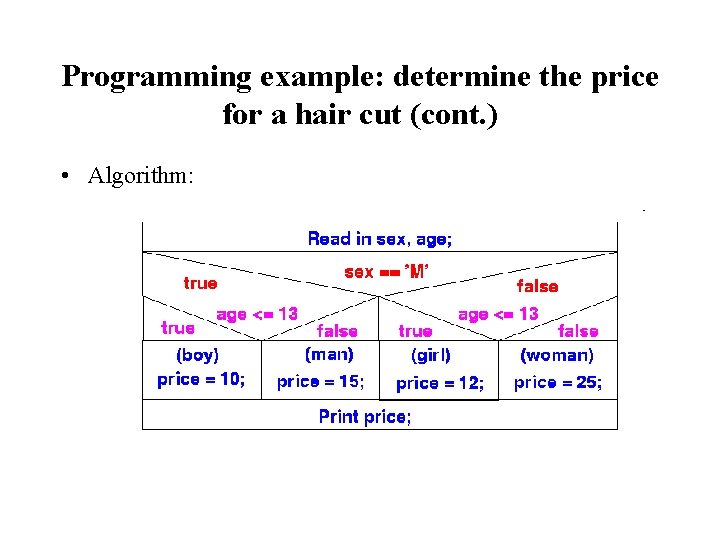
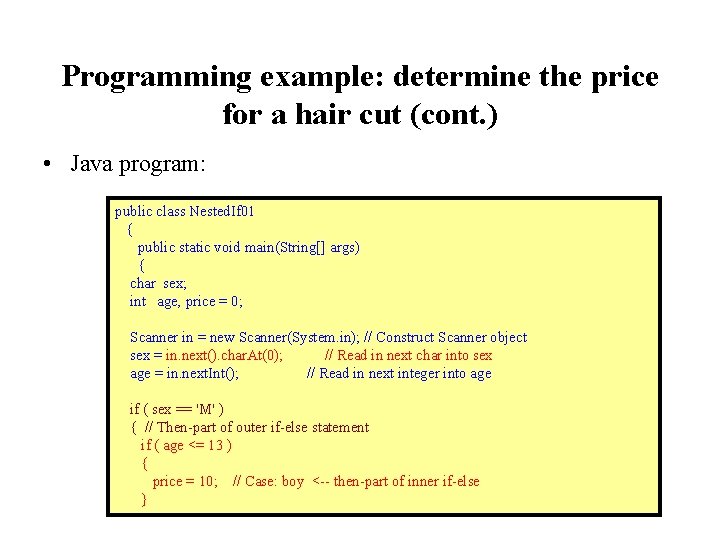
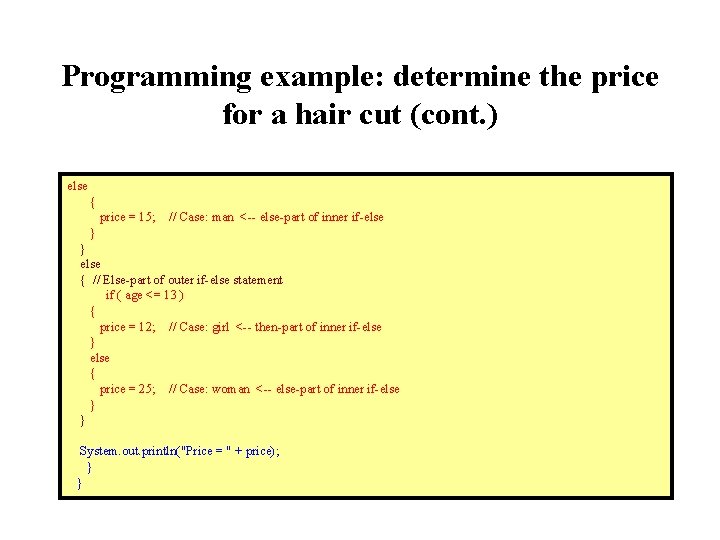
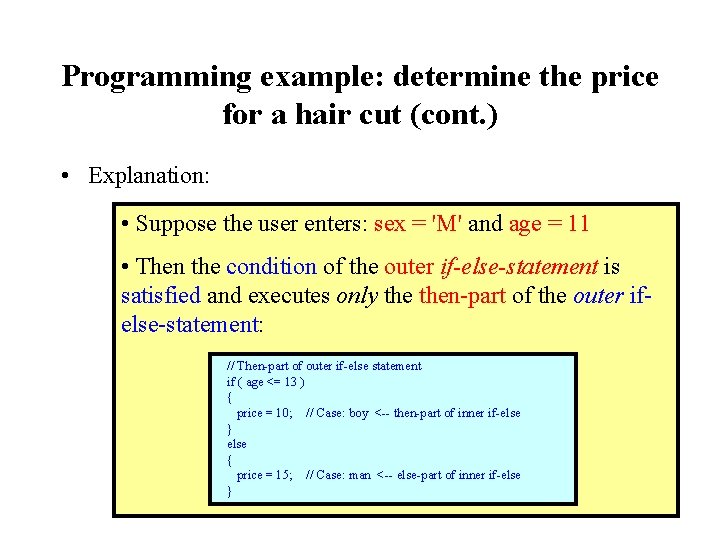
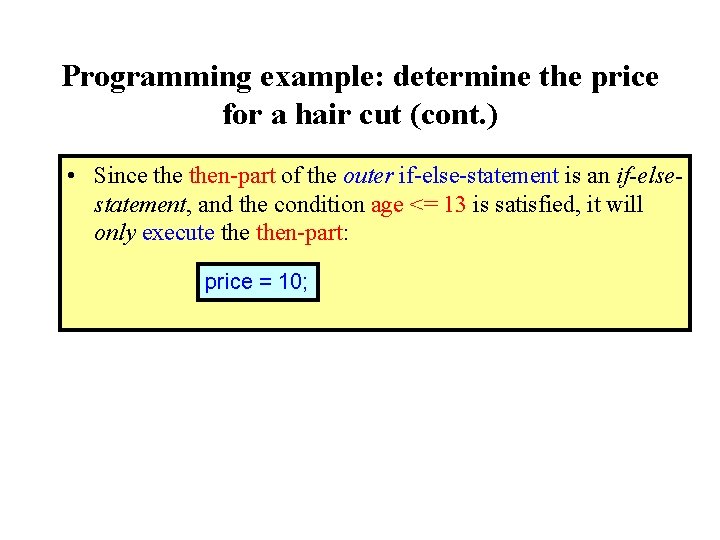
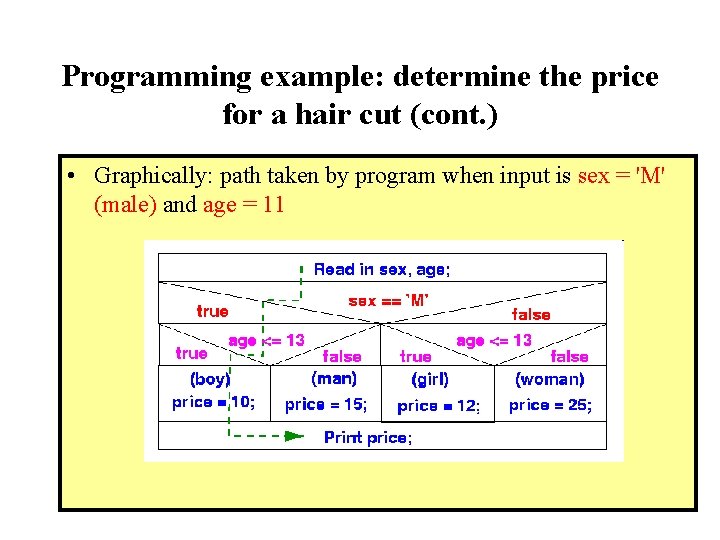
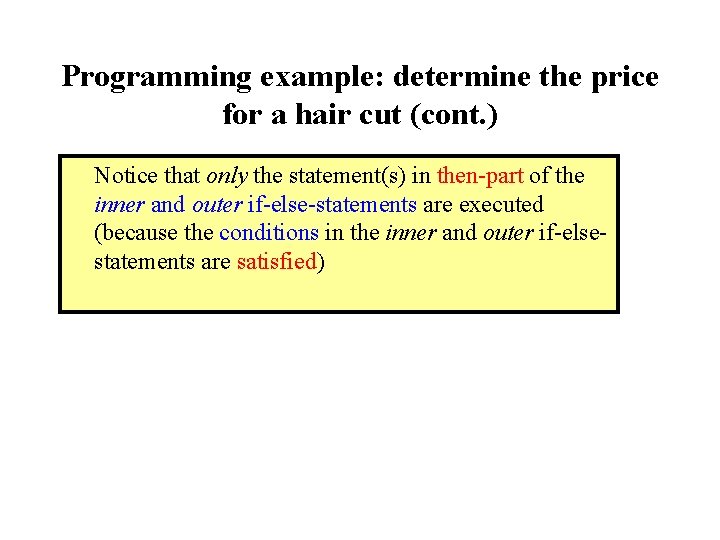
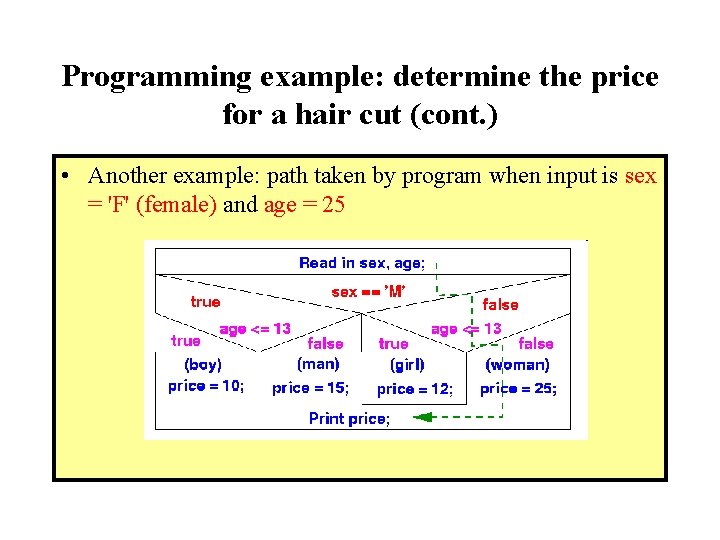
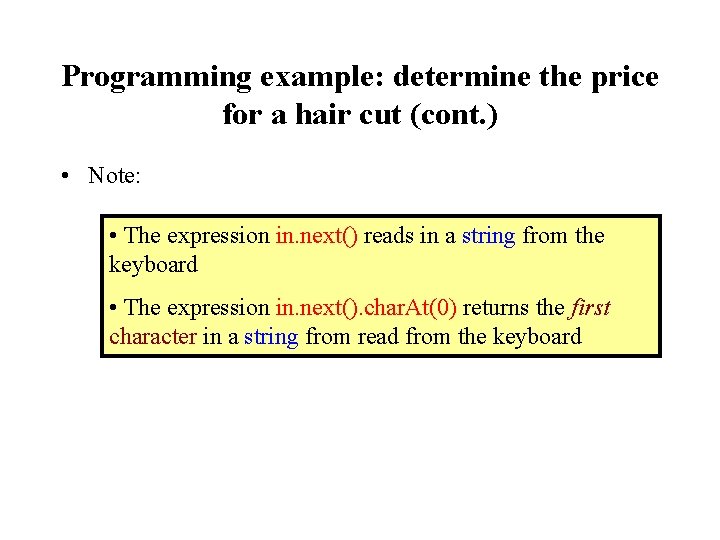
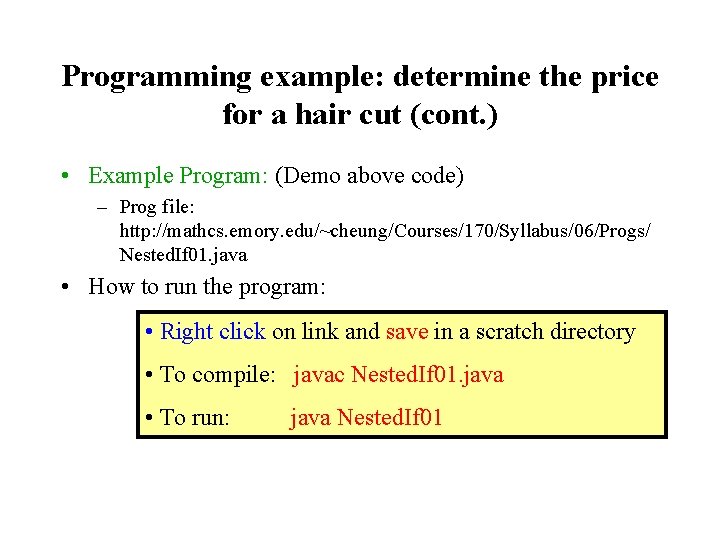
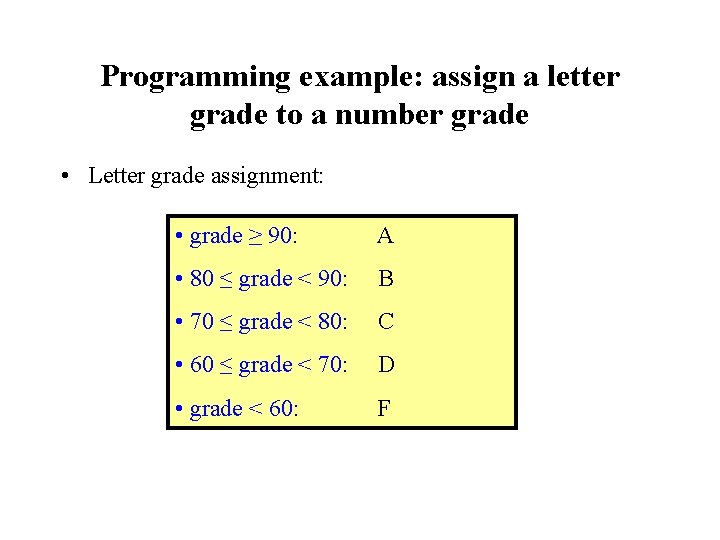
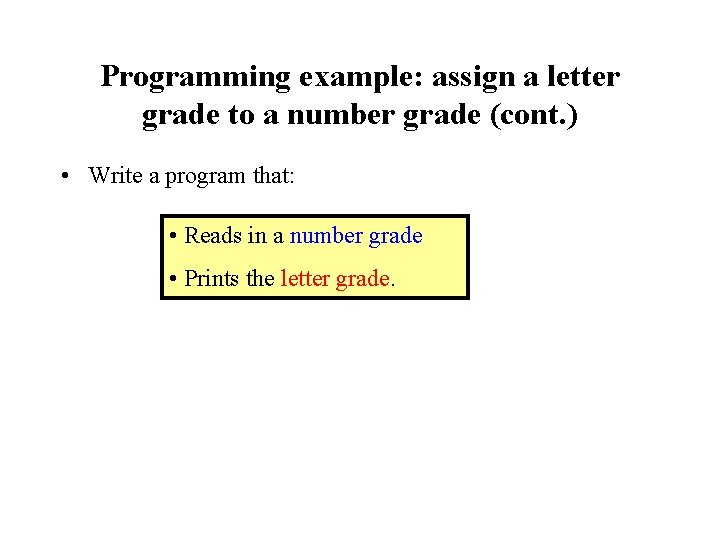
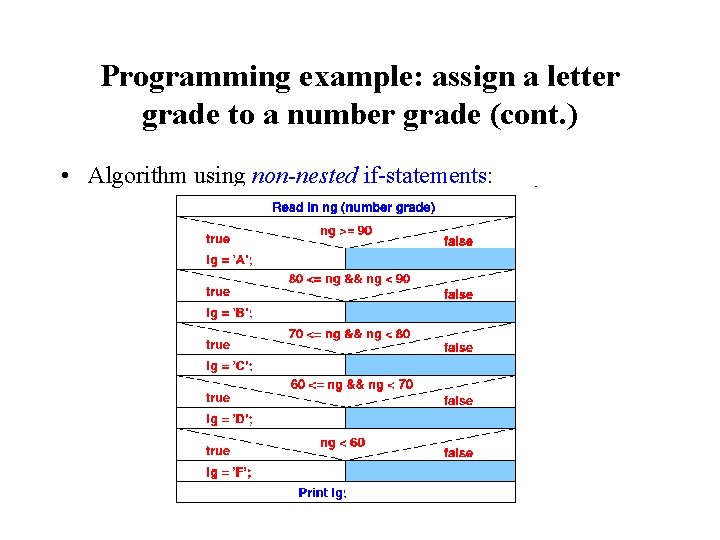
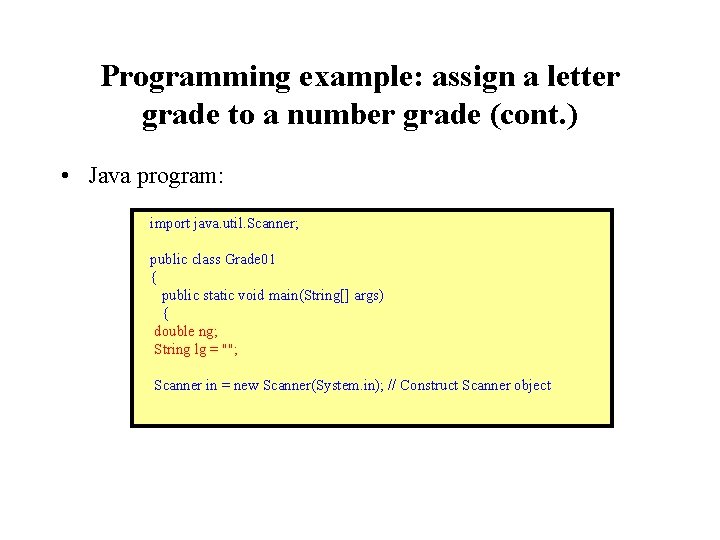
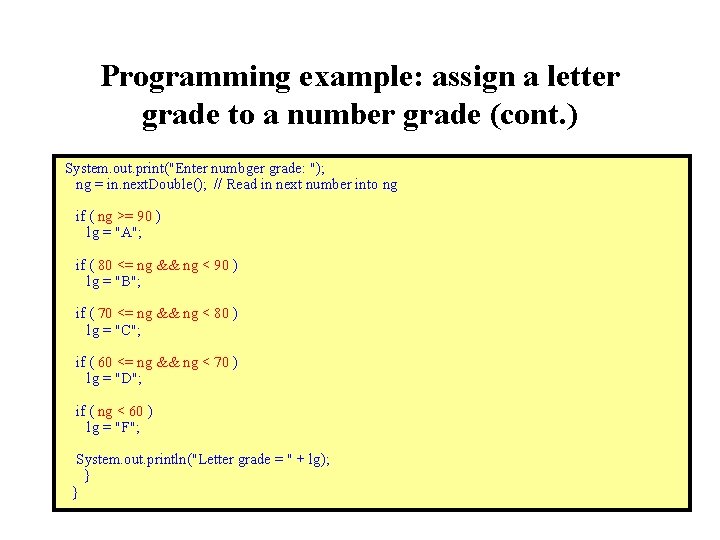
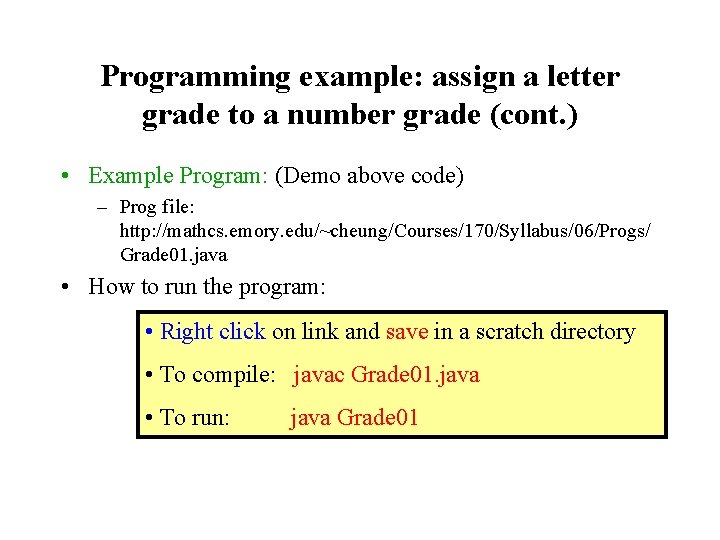
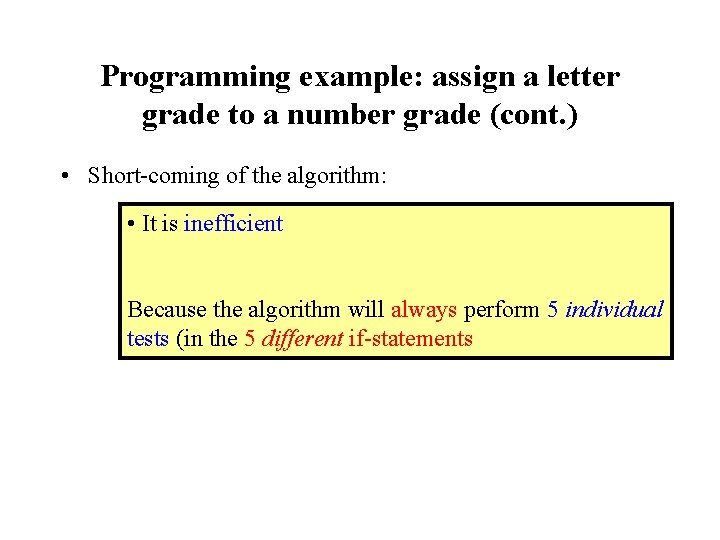
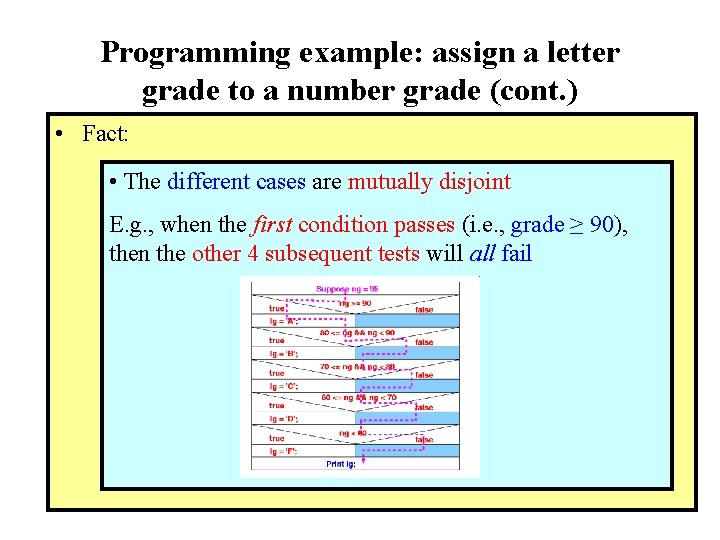
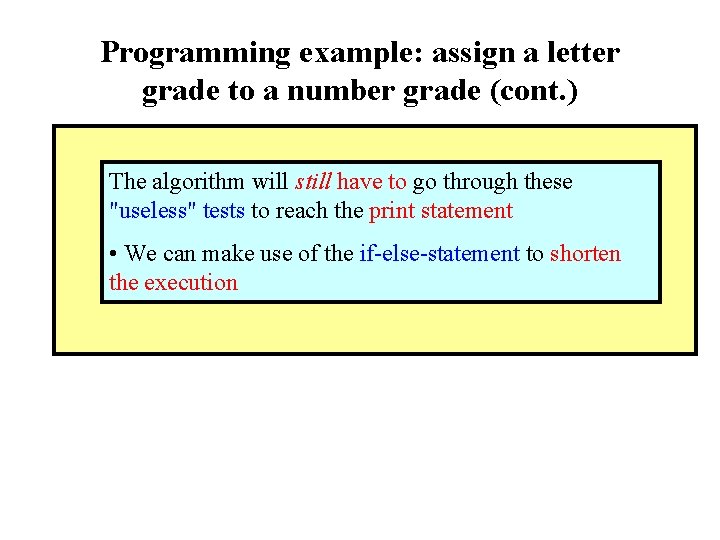
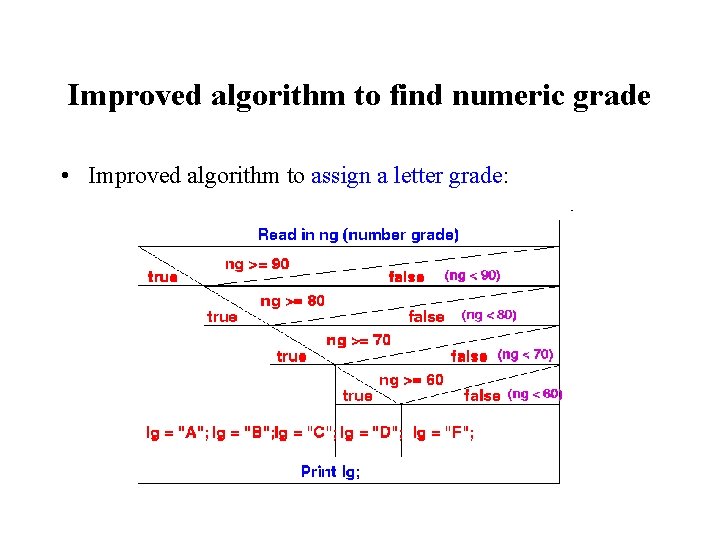
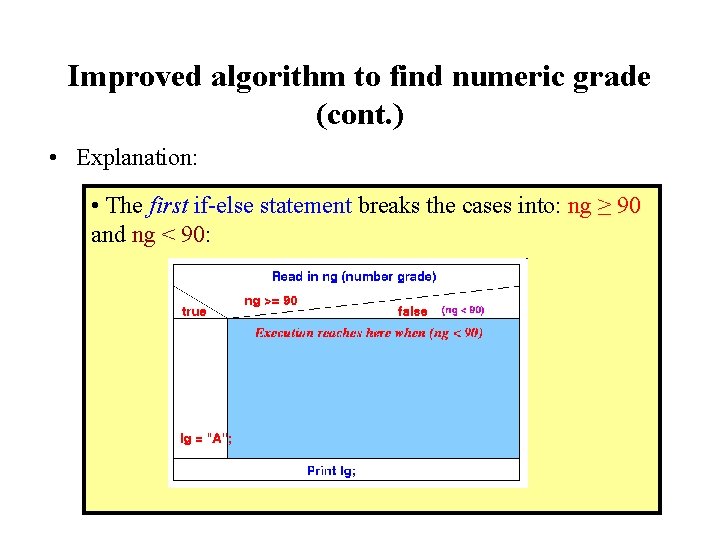
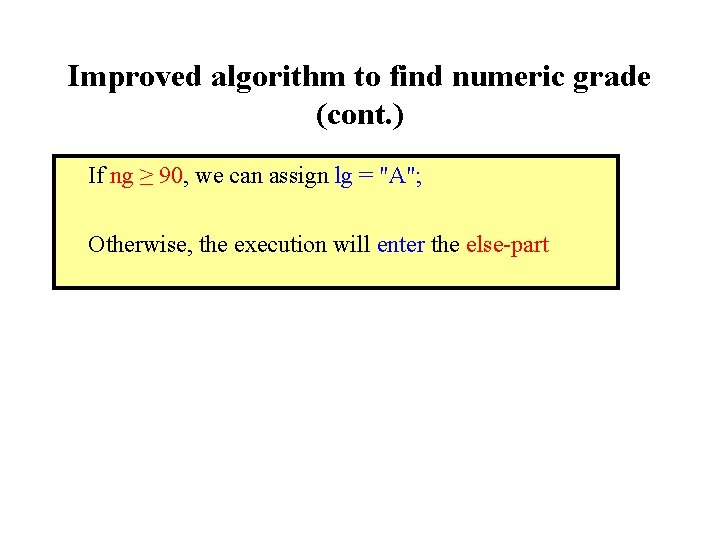
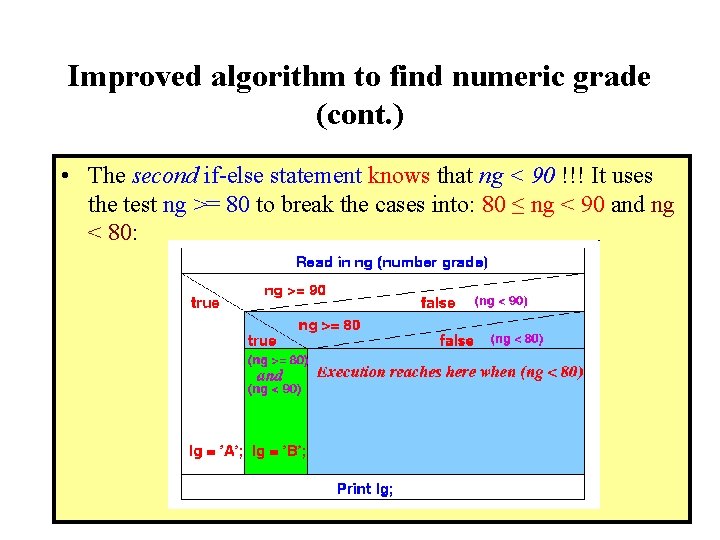
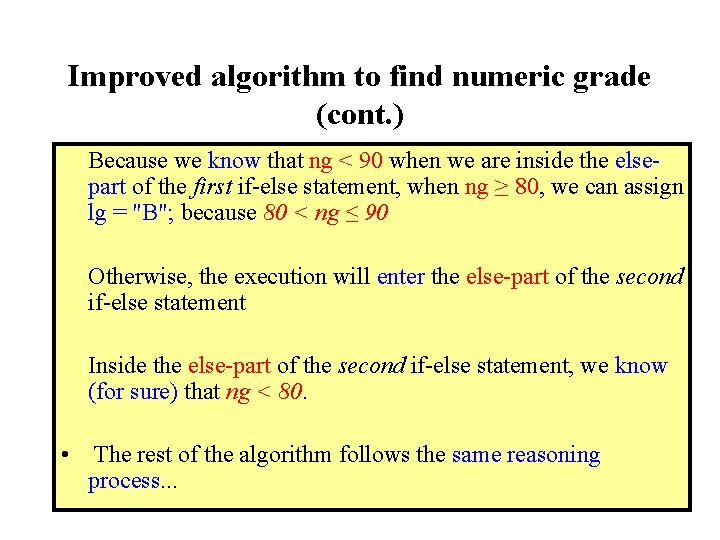
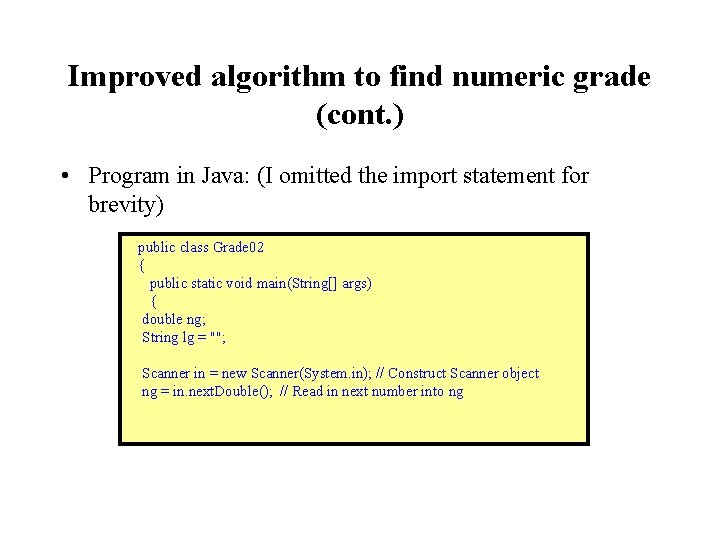
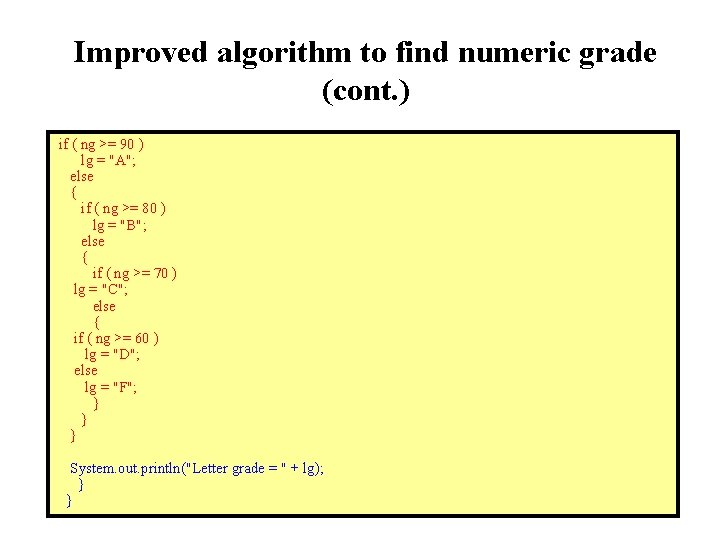
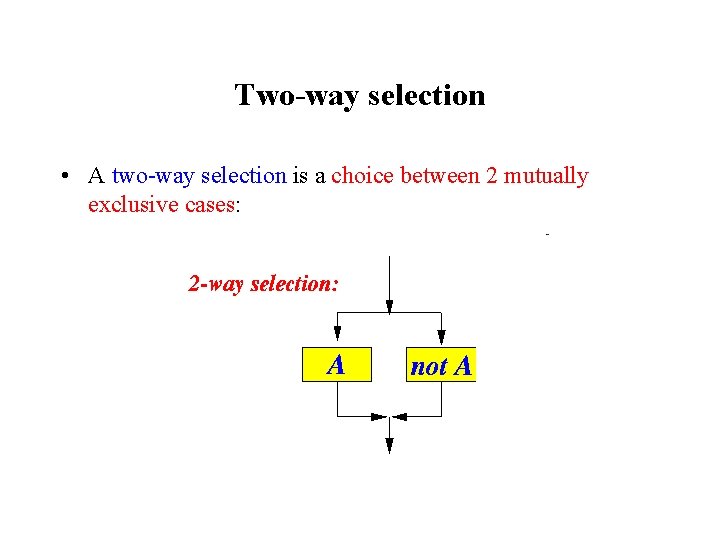
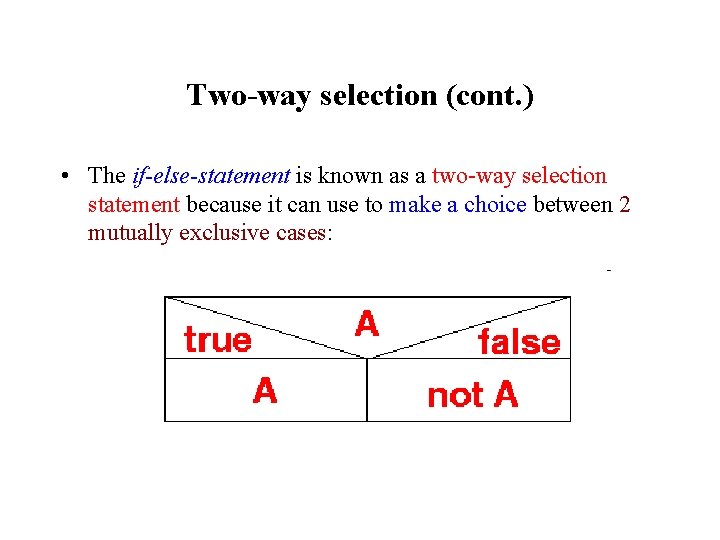
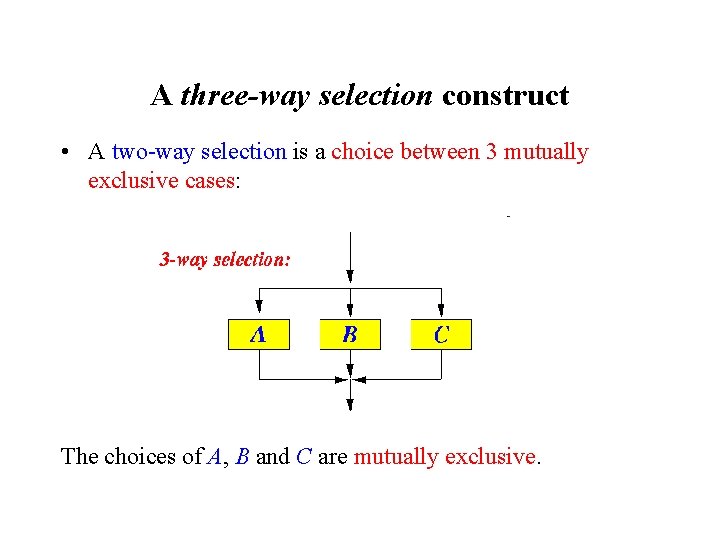
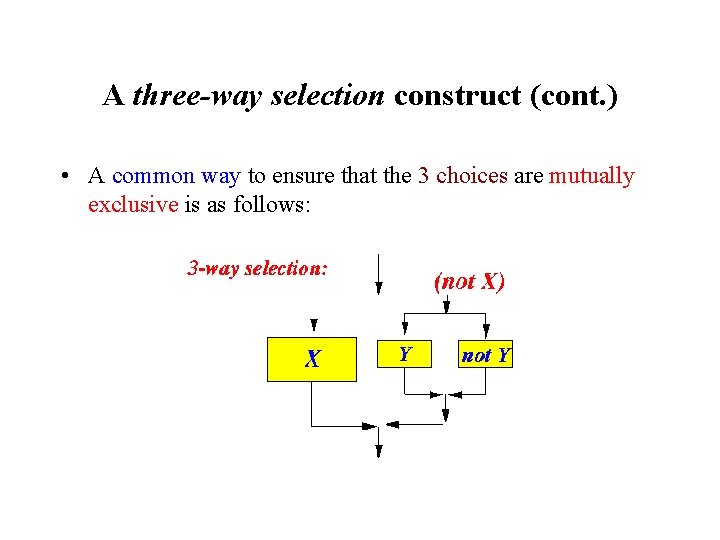
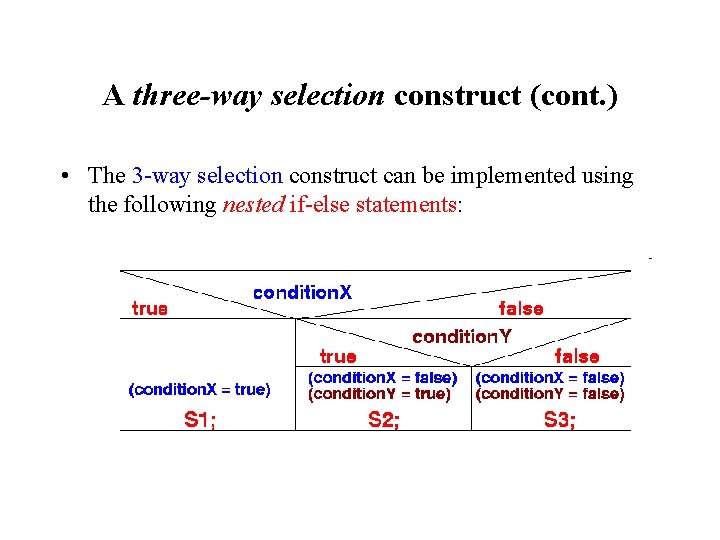
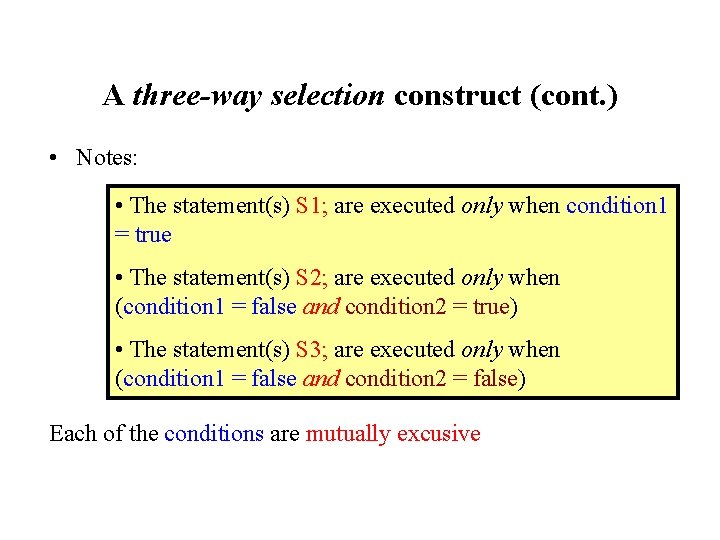
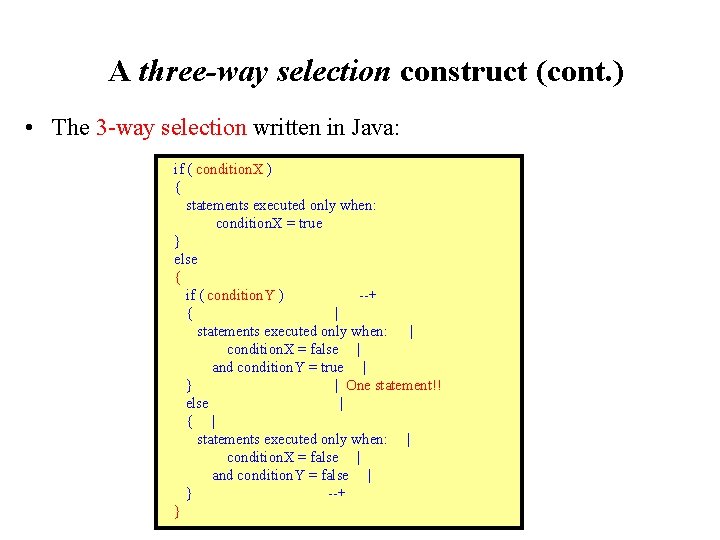
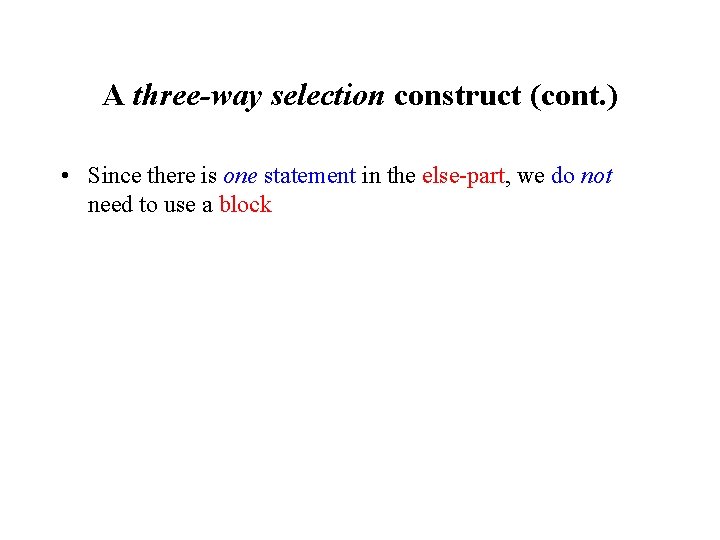
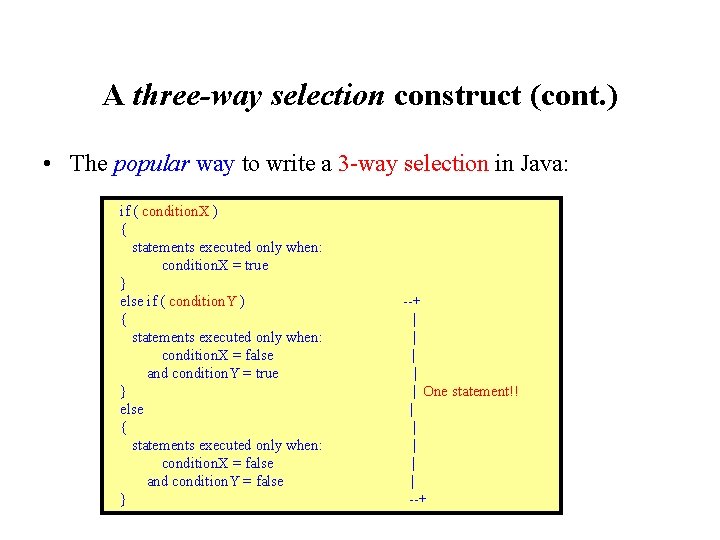
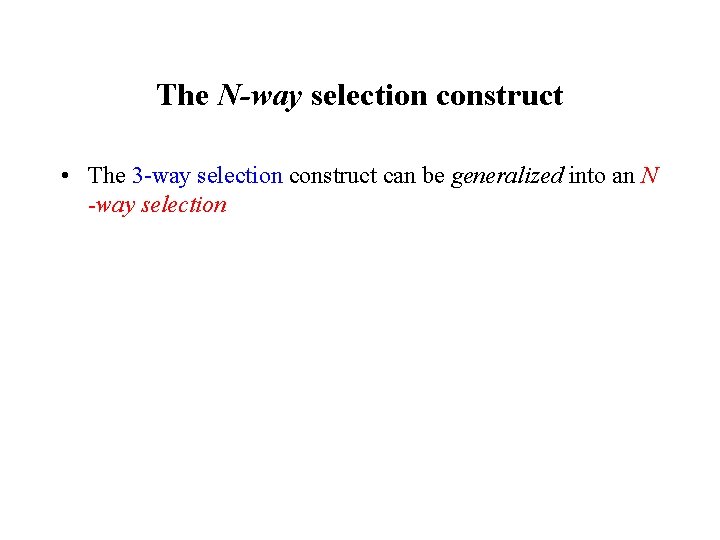
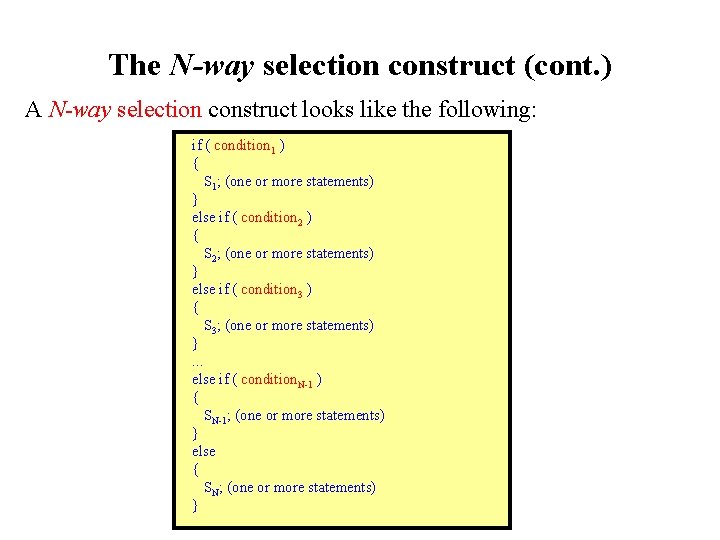
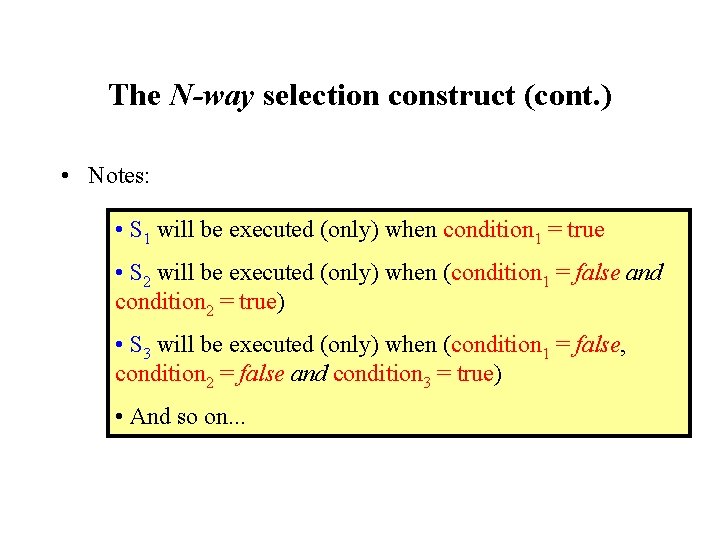
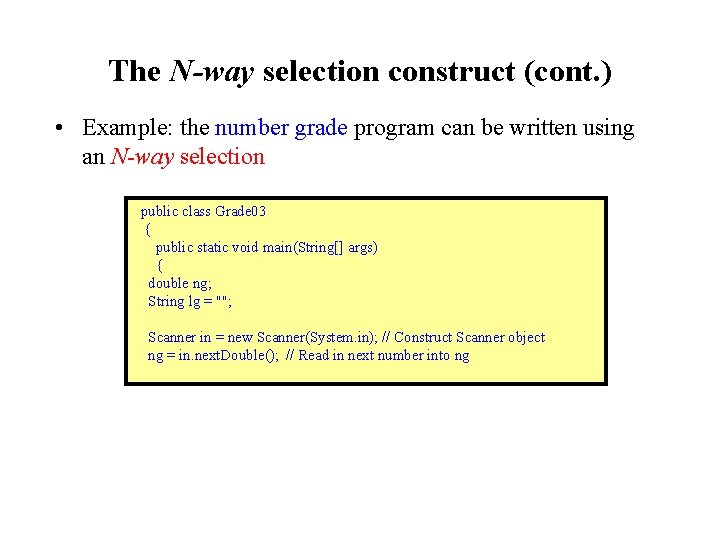
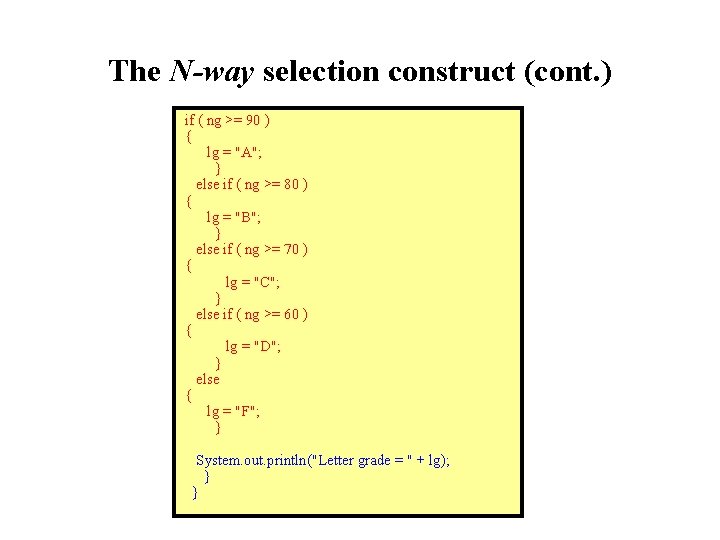
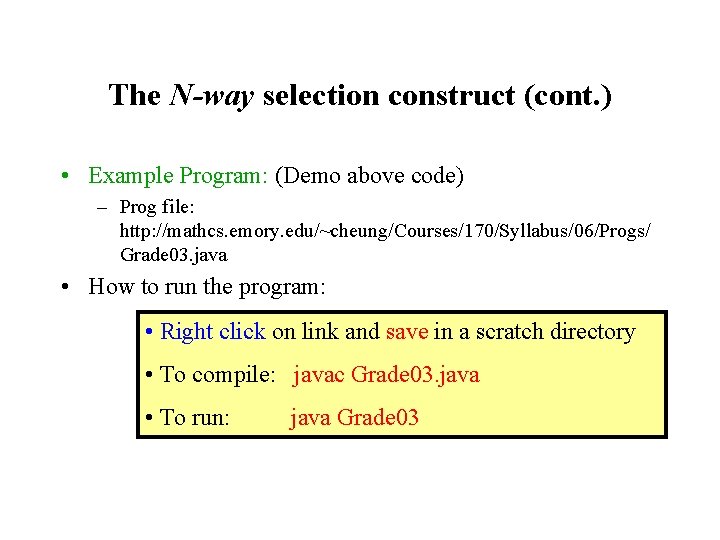
- Slides: 49
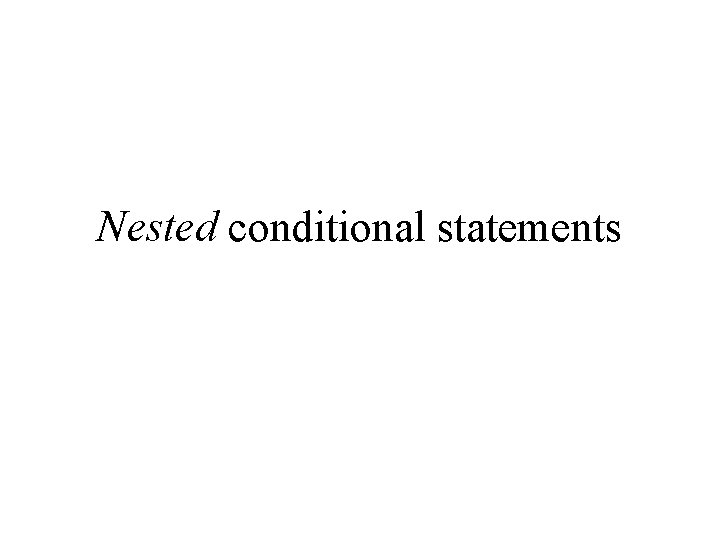
Nested conditional statements
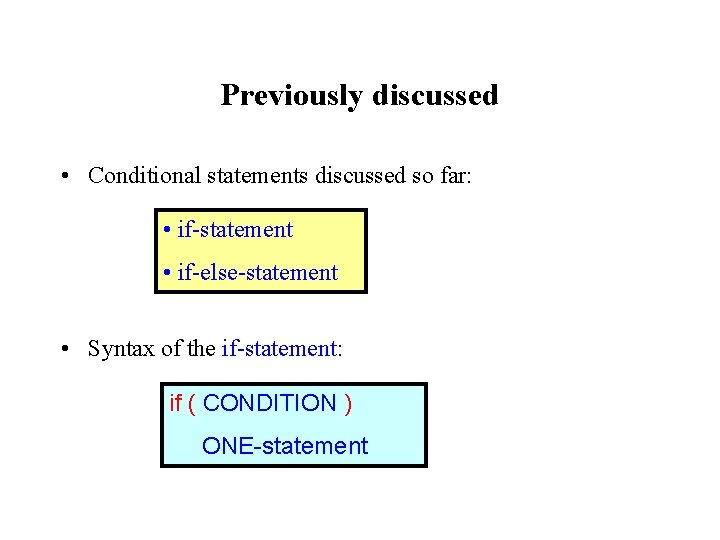
Previously discussed • Conditional statements discussed so far: • if-statement • if-else-statement • Syntax of the if-statement: if ( CONDITION ) ONE-statement
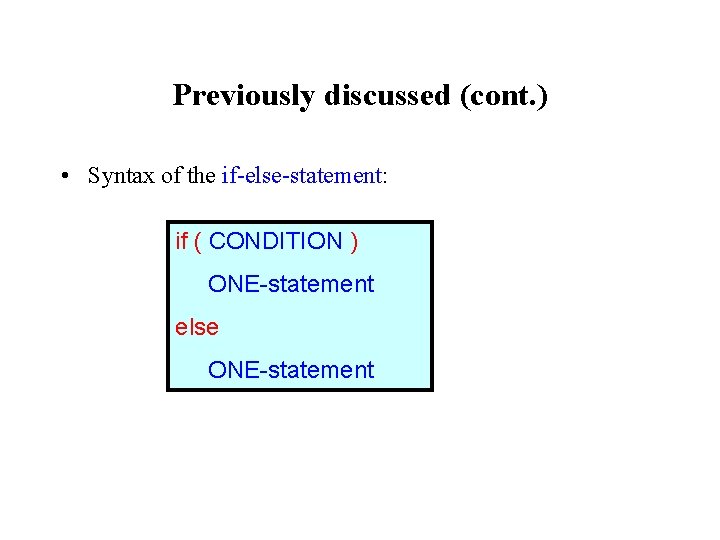
Previously discussed (cont. ) • Syntax of the if-else-statement: if ( CONDITION ) ONE-statement else ONE-statement
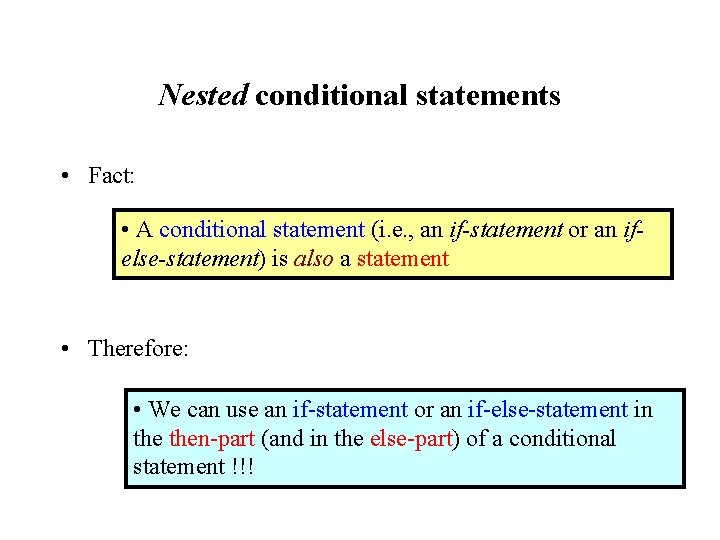
Nested conditional statements • Fact: • A conditional statement (i. e. , an if-statement or an ifelse-statement) is also a statement • Therefore: • We can use an if-statement or an if-else-statement in then-part (and in the else-part) of a conditional statement !!!
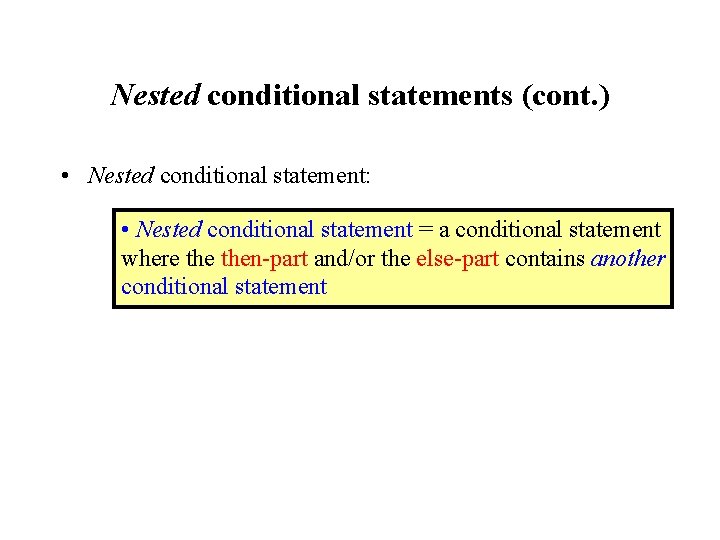
Nested conditional statements (cont. ) • Nested conditional statement: • Nested conditional statement = a conditional statement where then-part and/or the else-part contains another conditional statement
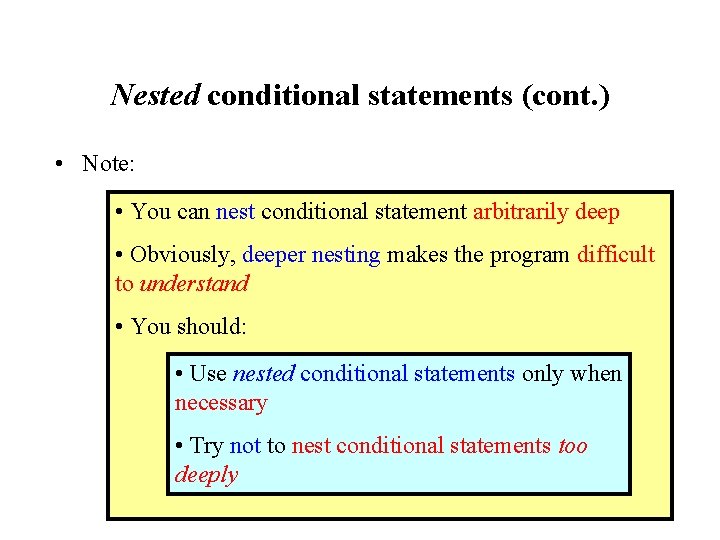
Nested conditional statements (cont. ) • Note: • You can nest conditional statement arbitrarily deep • Obviously, deeper nesting makes the program difficult to understand • You should: • Use nested conditional statements only when necessary • Try not to nest conditional statements too deeply
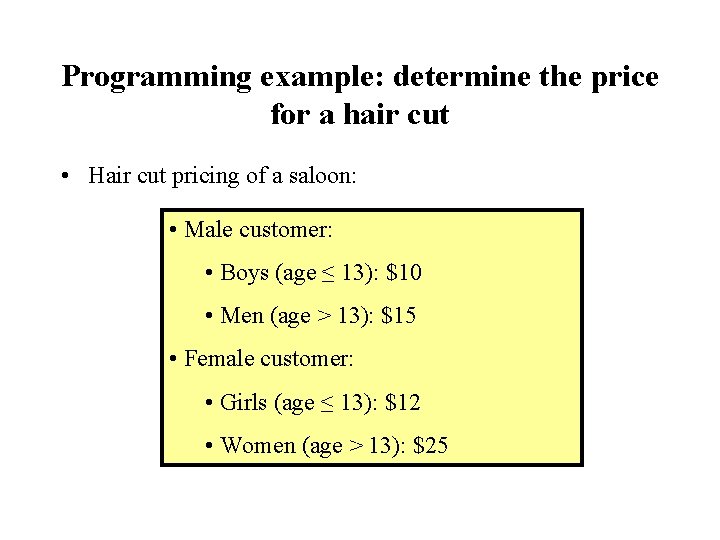
Programming example: determine the price for a hair cut • Hair cut pricing of a saloon: • Male customer: • Boys (age ≤ 13): $10 • Men (age > 13): $15 • Female customer: • Girls (age ≤ 13): $12 • Women (age > 13): $25
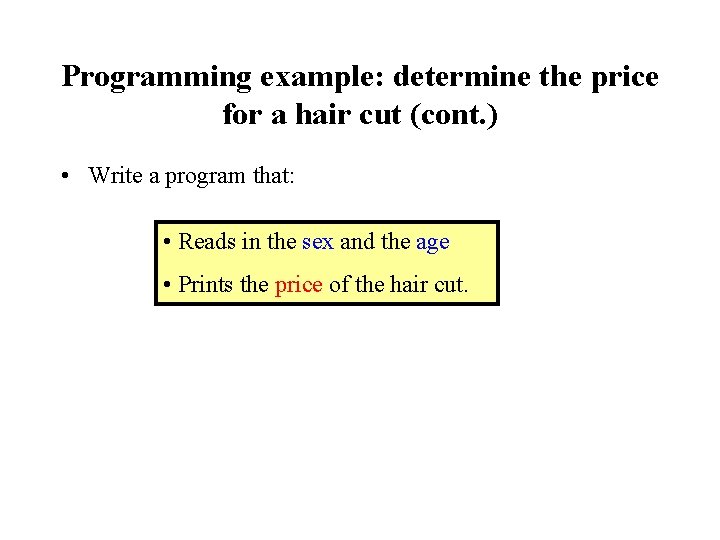
Programming example: determine the price for a hair cut (cont. ) • Write a program that: • Reads in the sex and the age • Prints the price of the hair cut.
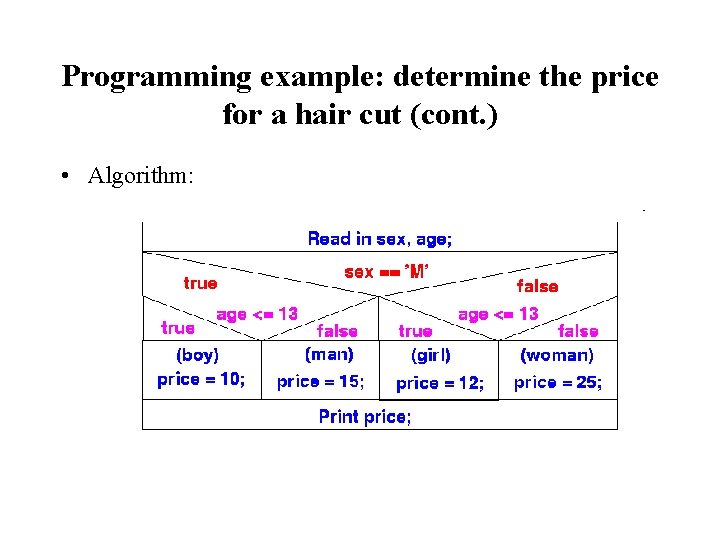
Programming example: determine the price for a hair cut (cont. ) • Algorithm:
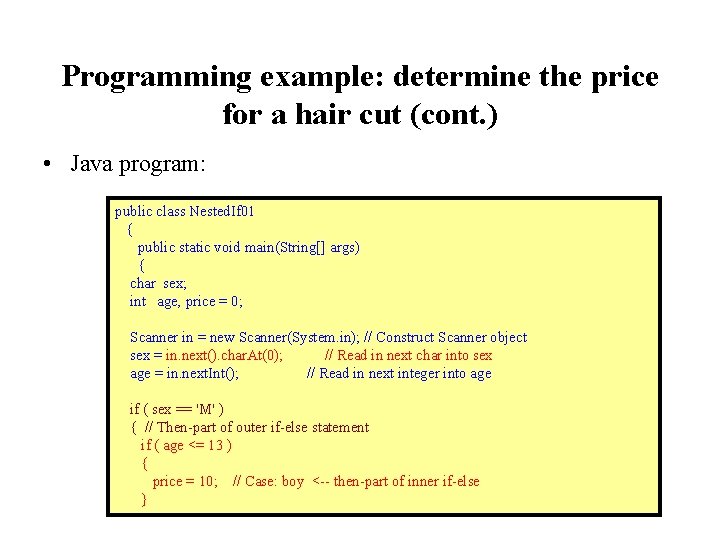
Programming example: determine the price for a hair cut (cont. ) • Java program: public class Nested. If 01 { public static void main(String[] args) { char sex; int age, price = 0; Scanner in = new Scanner(System. in); // Construct Scanner object sex = in. next(). char. At(0); // Read in next char into sex age = in. next. Int(); // Read in next integer into age if ( sex == 'M' ) { // Then-part of outer if-else statement if ( age <= 13 ) { price = 10; // Case: boy <-- then-part of inner if-else }
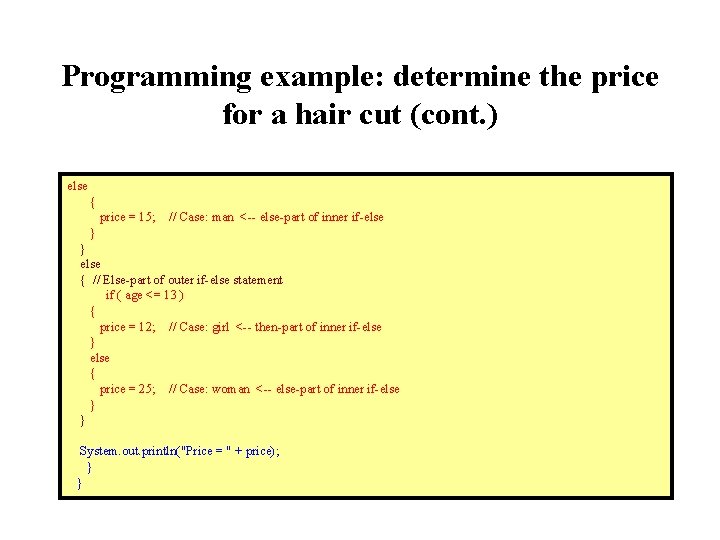
Programming example: determine the price for a hair cut (cont. ) else { price = 15; // Case: man <-- else-part of inner if-else } } else { // Else-part of outer if-else statement if ( age <= 13 ) { price = 12; // Case: girl <-- then-part of inner if-else } else { price = 25; // Case: woman <-- else-part of inner if-else } } System. out. println("Price = " + price); } }
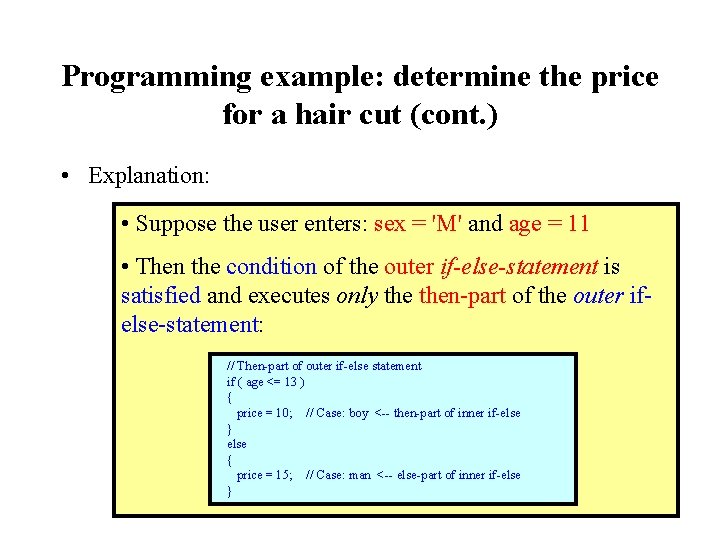
Programming example: determine the price for a hair cut (cont. ) • Explanation: • Suppose the user enters: sex = 'M' and age = 11 • Then the condition of the outer if-else-statement is satisfied and executes only then-part of the outer ifelse-statement: // Then-part of outer if-else statement if ( age <= 13 ) { price = 10; // Case: boy <-- then-part of inner if-else } else { price = 15; // Case: man <-- else-part of inner if-else }
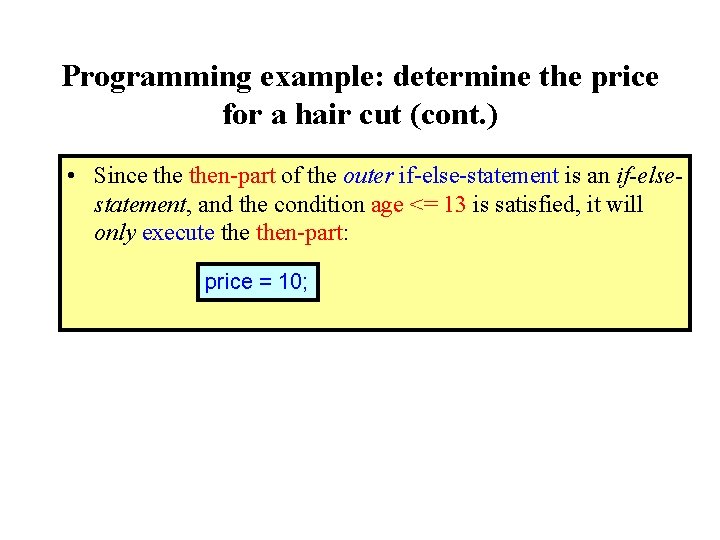
Programming example: determine the price for a hair cut (cont. ) • Since then-part of the outer if-else-statement is an if-elsestatement, and the condition age <= 13 is satisfied, it will only execute then-part: price = 10;
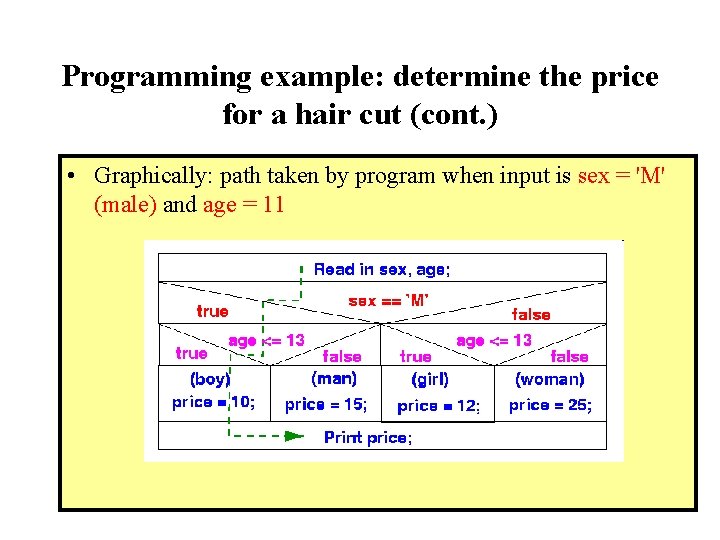
Programming example: determine the price for a hair cut (cont. ) • Graphically: path taken by program when input is sex = 'M' (male) and age = 11
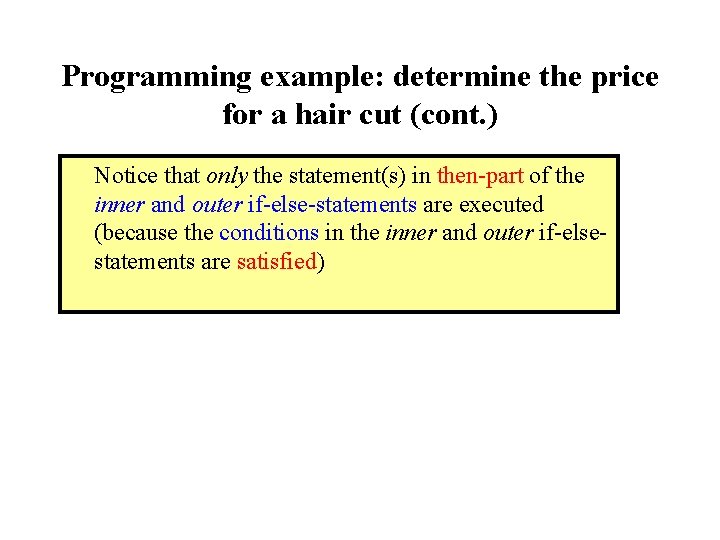
Programming example: determine the price for a hair cut (cont. ) Notice that only the statement(s) in then-part of the inner and outer if-else-statements are executed (because the conditions in the inner and outer if-elsestatements are satisfied)
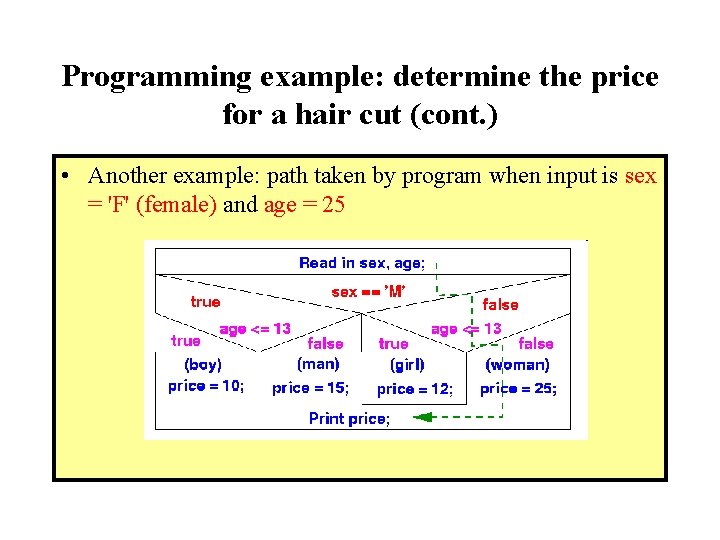
Programming example: determine the price for a hair cut (cont. ) • Another example: path taken by program when input is sex = 'F' (female) and age = 25
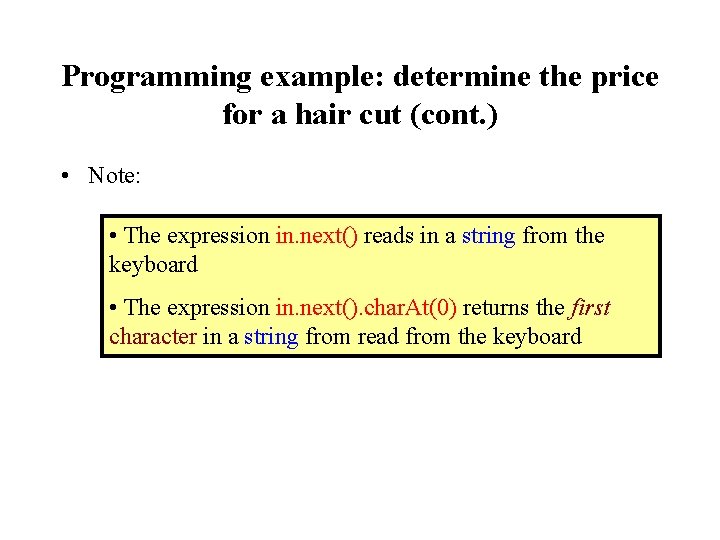
Programming example: determine the price for a hair cut (cont. ) • Note: • The expression in. next() reads in a string from the keyboard • The expression in. next(). char. At(0) returns the first character in a string from read from the keyboard
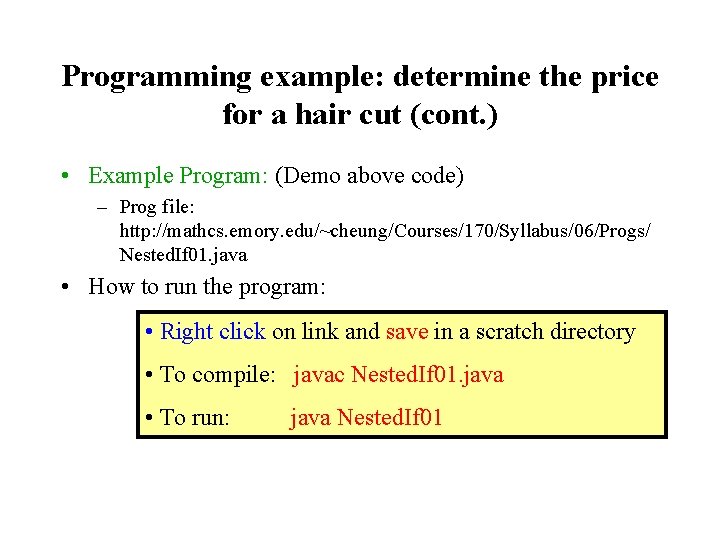
Programming example: determine the price for a hair cut (cont. ) • Example Program: (Demo above code) – Prog file: http: //mathcs. emory. edu/~cheung/Courses/170/Syllabus/06/Progs/ Nested. If 01. java • How to run the program: • Right click on link and save in a scratch directory • To compile: javac Nested. If 01. java • To run: java Nested. If 01
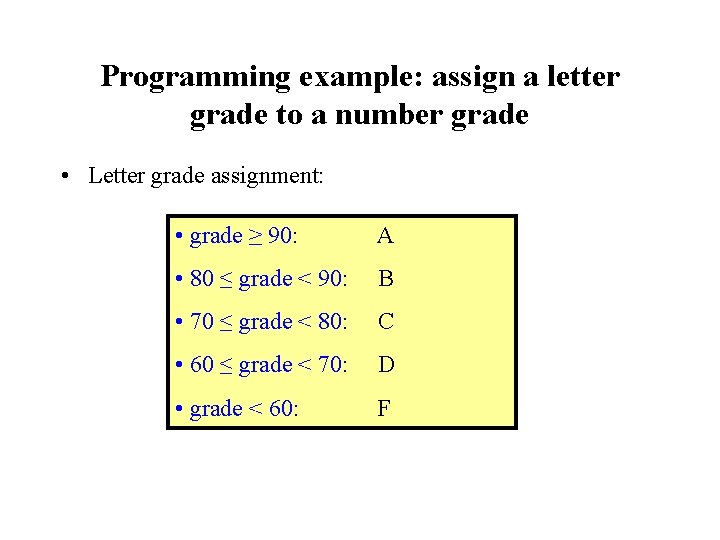
Programming example: assign a letter grade to a number grade • Letter grade assignment: • grade ≥ 90: A • 80 ≤ grade < 90: B • 70 ≤ grade < 80: C • 60 ≤ grade < 70: D • grade < 60: F
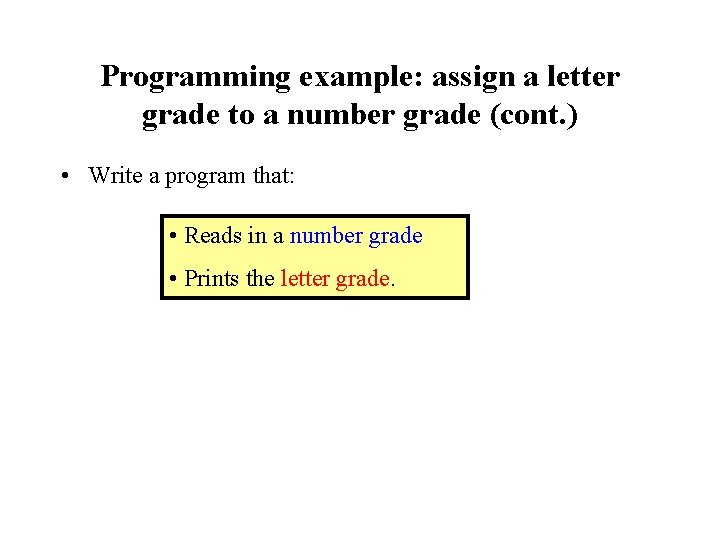
Programming example: assign a letter grade to a number grade (cont. ) • Write a program that: • Reads in a number grade • Prints the letter grade.
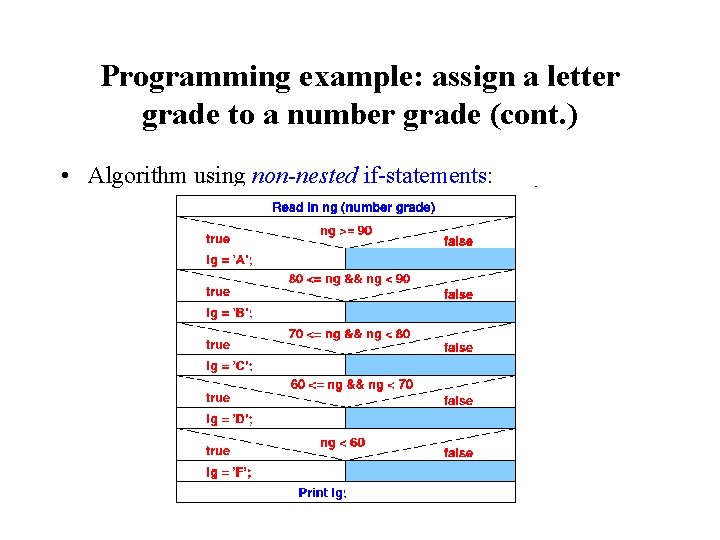
Programming example: assign a letter grade to a number grade (cont. ) • Algorithm using non-nested if-statements:
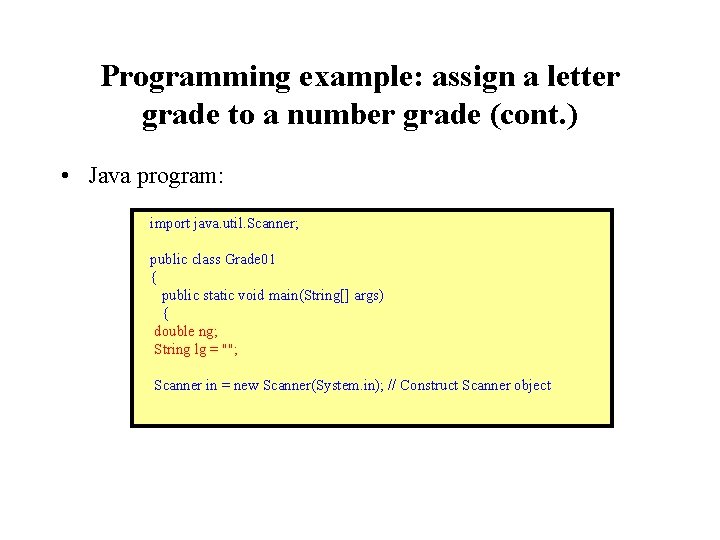
Programming example: assign a letter grade to a number grade (cont. ) • Java program: import java. util. Scanner; public class Grade 01 { public static void main(String[] args) { double ng; String lg = ""; Scanner in = new Scanner(System. in); // Construct Scanner object
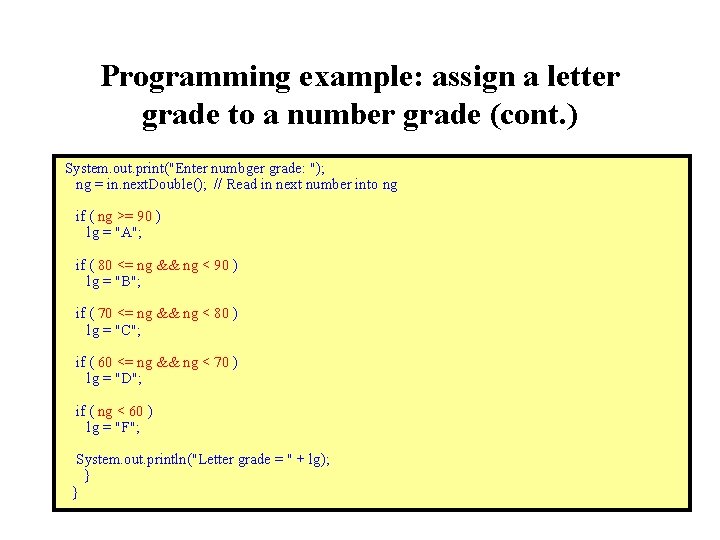
Programming example: assign a letter grade to a number grade (cont. ) System. out. print("Enter numbger grade: "); ng = in. next. Double(); // Read in next number into ng if ( ng >= 90 ) lg = "A"; if ( 80 <= ng && ng < 90 ) lg = "B"; if ( 70 <= ng && ng < 80 ) lg = "C"; if ( 60 <= ng && ng < 70 ) lg = "D"; if ( ng < 60 ) lg = "F"; System. out. println("Letter grade = " + lg); } }
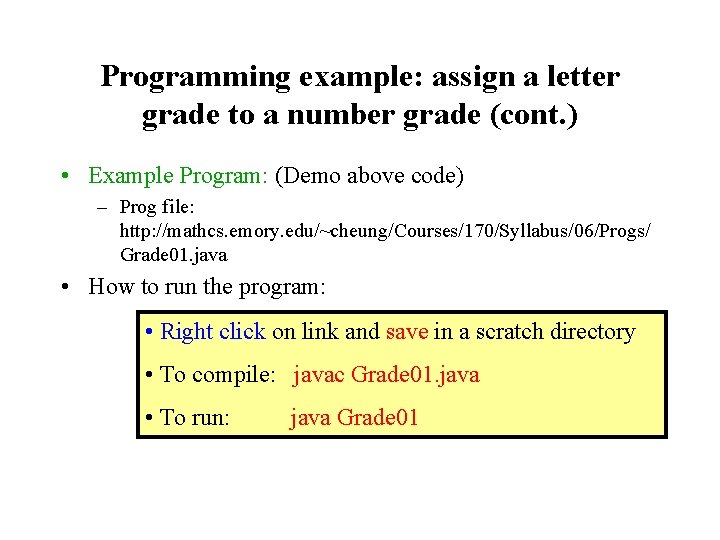
Programming example: assign a letter grade to a number grade (cont. ) • Example Program: (Demo above code) – Prog file: http: //mathcs. emory. edu/~cheung/Courses/170/Syllabus/06/Progs/ Grade 01. java • How to run the program: • Right click on link and save in a scratch directory • To compile: javac Grade 01. java • To run: java Grade 01
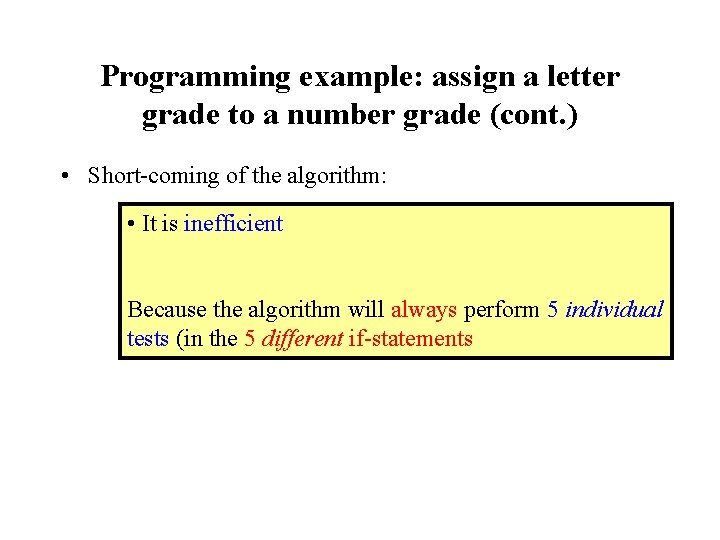
Programming example: assign a letter grade to a number grade (cont. ) • Short-coming of the algorithm: • It is inefficient Because the algorithm will always perform 5 individual tests (in the 5 different if-statements
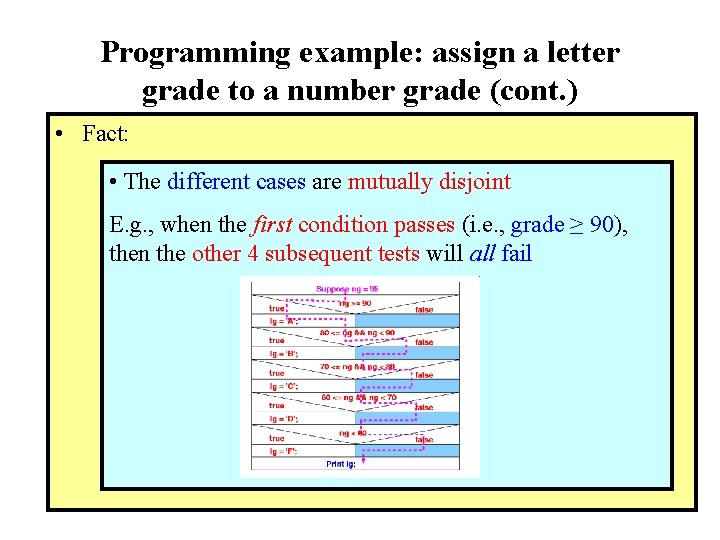
Programming example: assign a letter grade to a number grade (cont. ) • Fact: • The different cases are mutually disjoint E. g. , when the first condition passes (i. e. , grade ≥ 90), then the other 4 subsequent tests will all fail
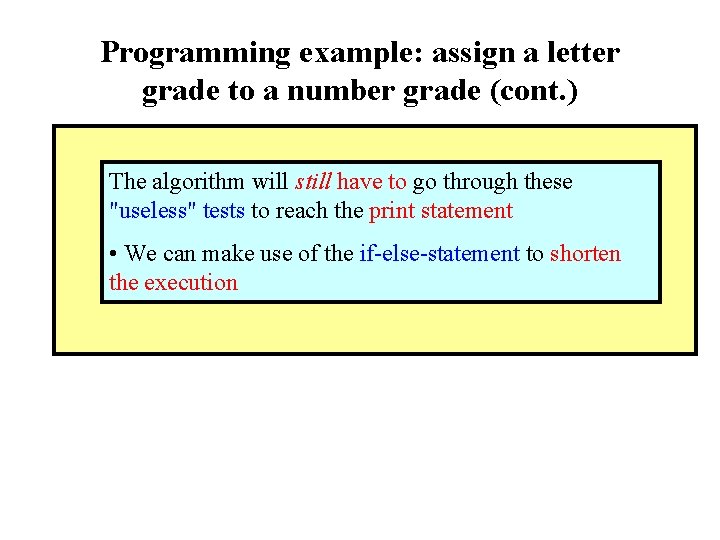
Programming example: assign a letter grade to a number grade (cont. ) The algorithm will still have to go through these "useless" tests to reach the print statement • We can make use of the if-else-statement to shorten the execution
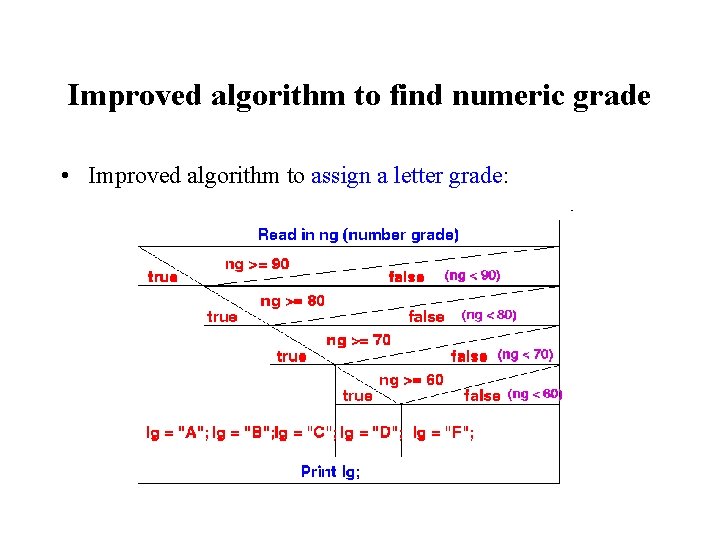
Improved algorithm to find numeric grade • Improved algorithm to assign a letter grade:
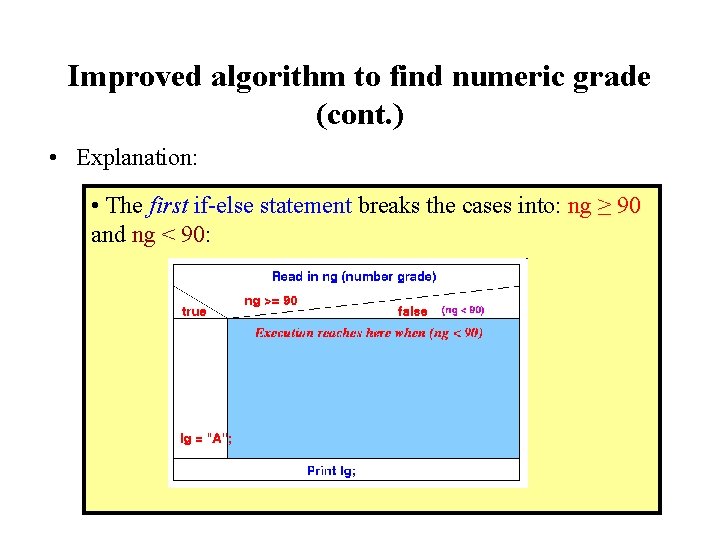
Improved algorithm to find numeric grade (cont. ) • Explanation: • The first if-else statement breaks the cases into: ng ≥ 90 and ng < 90:
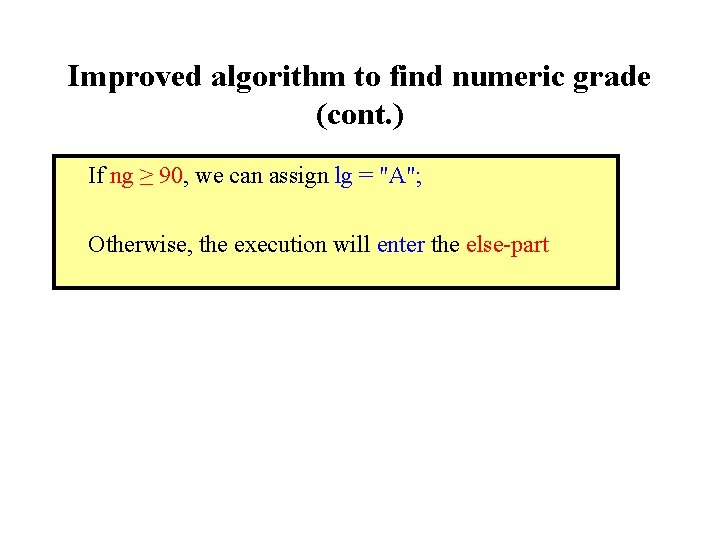
Improved algorithm to find numeric grade (cont. ) If ng ≥ 90, we can assign lg = "A"; Otherwise, the execution will enter the else-part
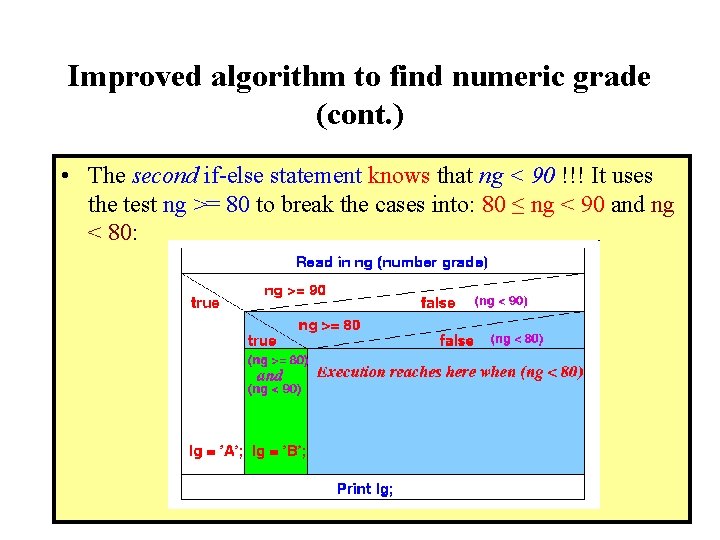
Improved algorithm to find numeric grade (cont. ) • The second if-else statement knows that ng < 90 !!! It uses the test ng >= 80 to break the cases into: 80 ≤ ng < 90 and ng < 80:
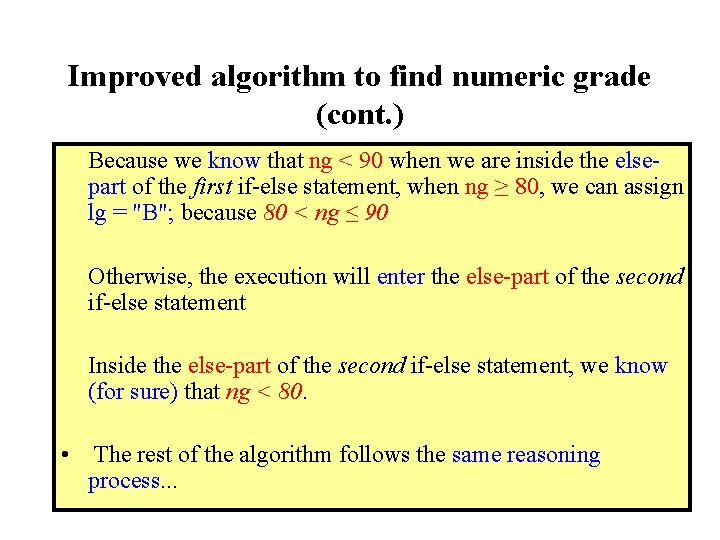
Improved algorithm to find numeric grade (cont. ) Because we know that ng < 90 when we are inside the elsepart of the first if-else statement, when ng ≥ 80, we can assign lg = "B"; because 80 < ng ≤ 90 Otherwise, the execution will enter the else-part of the second if-else statement Inside the else-part of the second if-else statement, we know (for sure) that ng < 80. • The rest of the algorithm follows the same reasoning process. . .
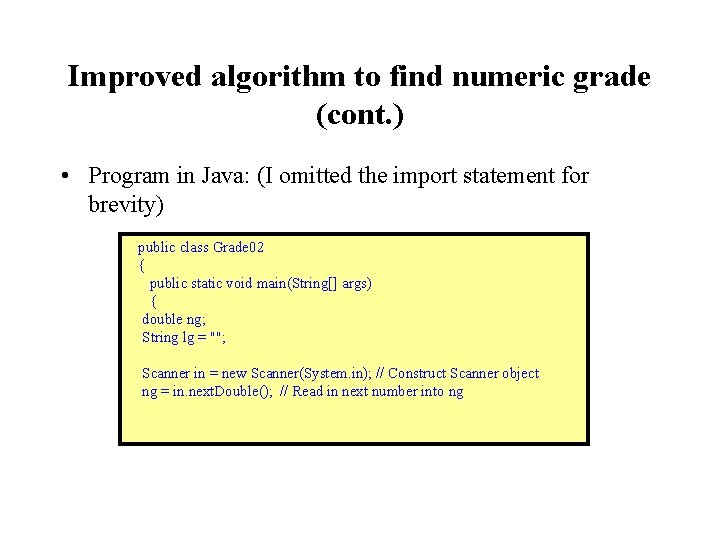
Improved algorithm to find numeric grade (cont. ) • Program in Java: (I omitted the import statement for brevity) public class Grade 02 { public static void main(String[] args) { double ng; String lg = ""; Scanner in = new Scanner(System. in); // Construct Scanner object ng = in. next. Double(); // Read in next number into ng
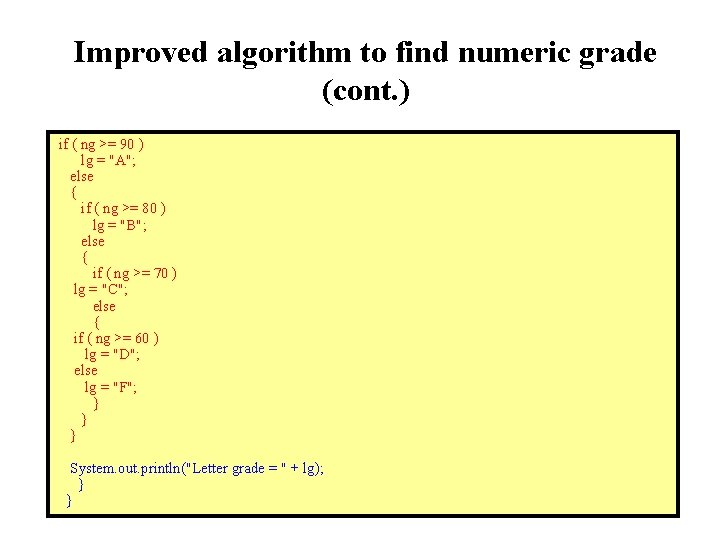
Improved algorithm to find numeric grade (cont. ) if ( ng >= 90 ) lg = "A"; else { if ( ng >= 80 ) lg = "B"; else { if ( ng >= 70 ) lg = "C"; else { if ( ng >= 60 ) lg = "D"; else lg = "F"; } } } System. out. println("Letter grade = " + lg); } }
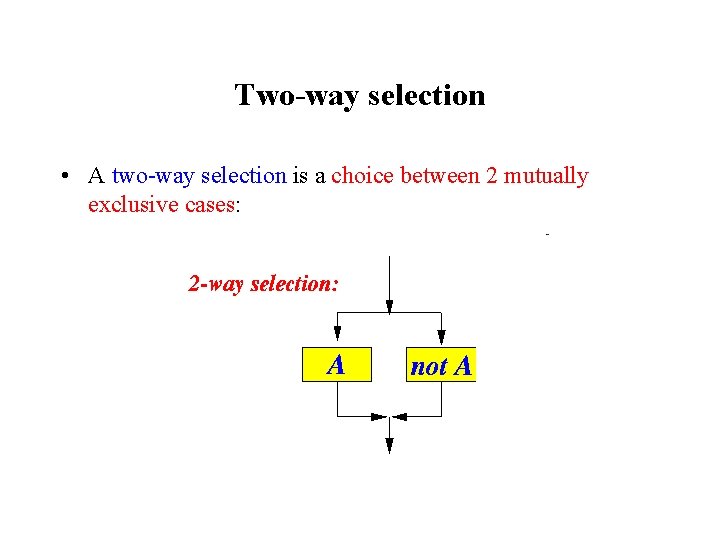
Two-way selection • A two-way selection is a choice between 2 mutually exclusive cases:
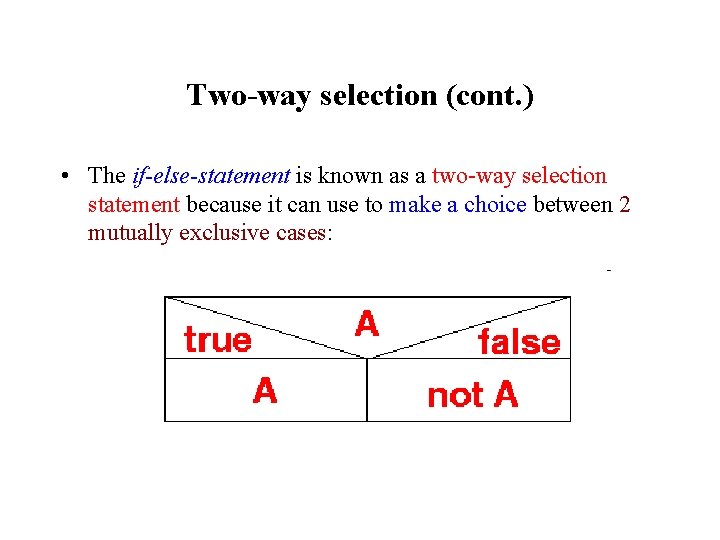
Two-way selection (cont. ) • The if-else-statement is known as a two-way selection statement because it can use to make a choice between 2 mutually exclusive cases:
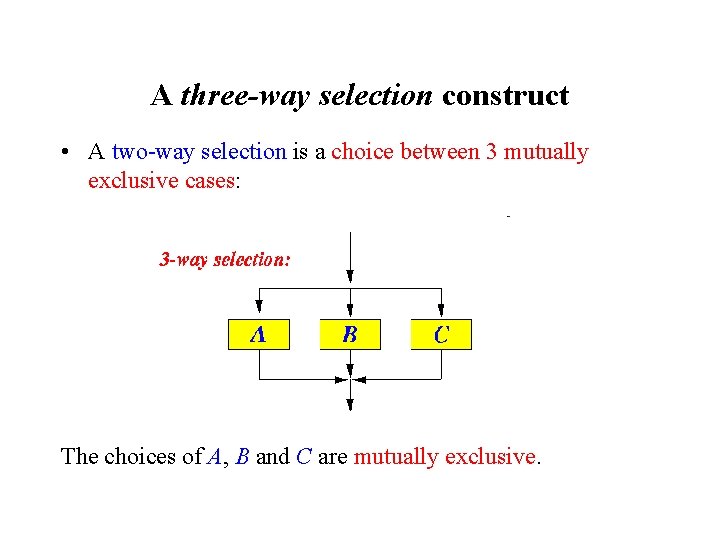
A three-way selection construct • A two-way selection is a choice between 3 mutually exclusive cases: The choices of A, B and C are mutually exclusive.
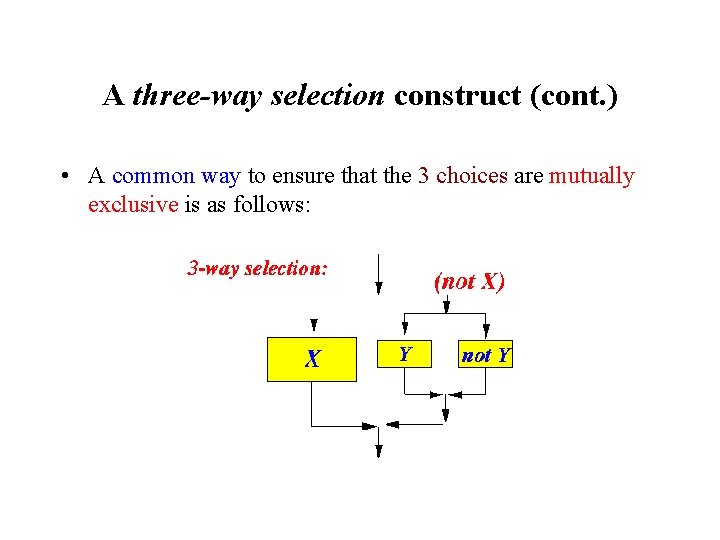
A three-way selection construct (cont. ) • A common way to ensure that the 3 choices are mutually exclusive is as follows:
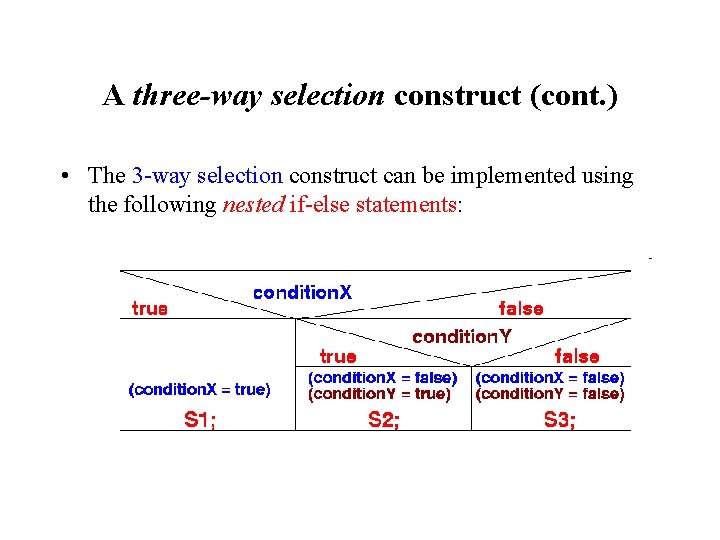
A three-way selection construct (cont. ) • The 3 -way selection construct can be implemented using the following nested if-else statements:
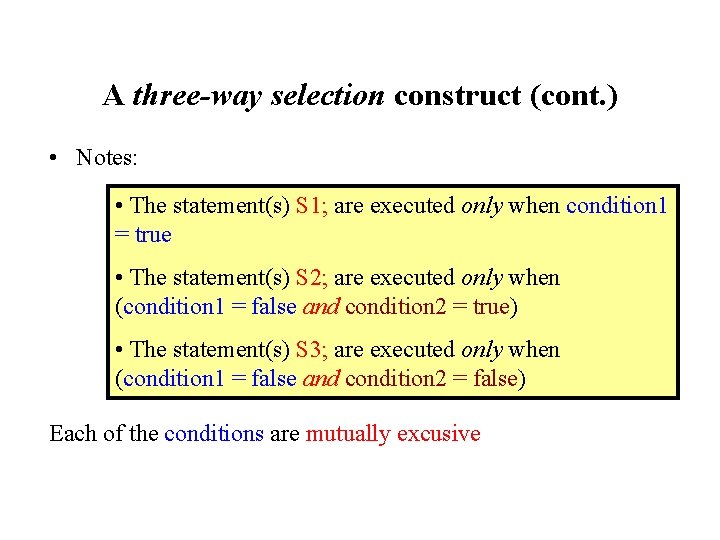
A three-way selection construct (cont. ) • Notes: • The statement(s) S 1; are executed only when condition 1 = true • The statement(s) S 2; are executed only when (condition 1 = false and condition 2 = true) • The statement(s) S 3; are executed only when (condition 1 = false and condition 2 = false) Each of the conditions are mutually excusive
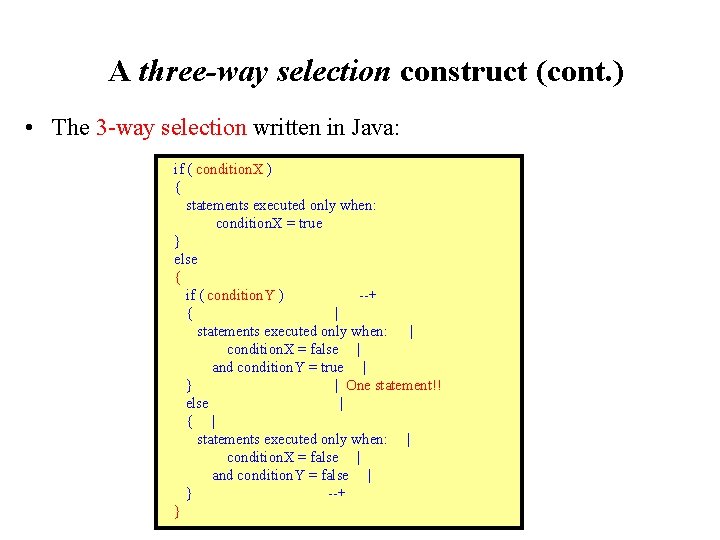
A three-way selection construct (cont. ) • The 3 -way selection written in Java: if ( condition. X ) { statements executed only when: condition. X = true } else { if ( condition. Y ) --+ { | statements executed only when: | condition. X = false | and condition. Y = true | } | One statement!! else | { | statements executed only when: | condition. X = false | and condition. Y = false | } --+ }
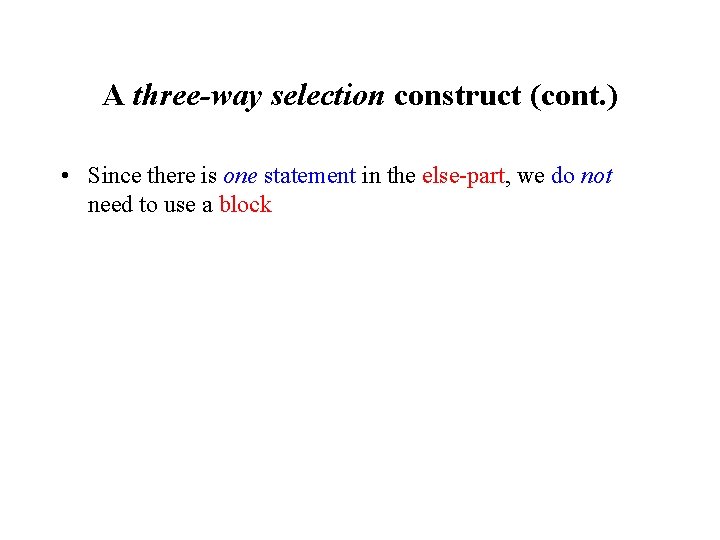
A three-way selection construct (cont. ) • Since there is one statement in the else-part, we do not need to use a block
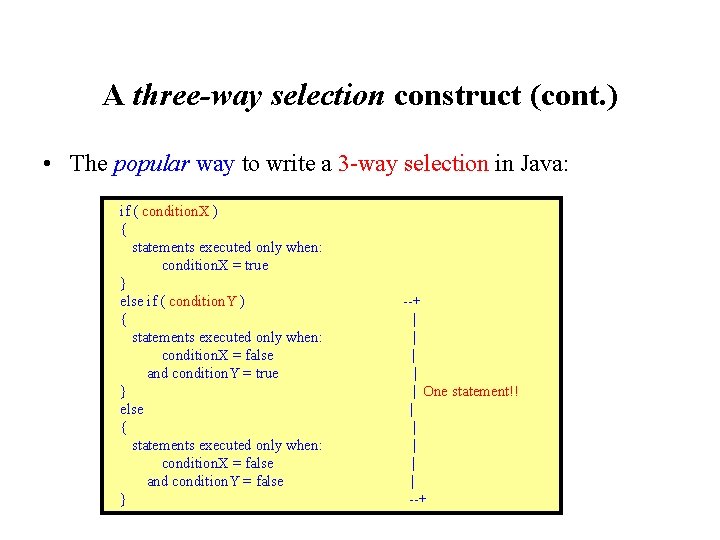
A three-way selection construct (cont. ) • The popular way to write a 3 -way selection in Java: if ( condition. X ) { statements executed only when: condition. X = true } else if ( condition. Y ) --+ { | statements executed only when: | condition. X = false | and condition. Y = true | } | One statement!! else | { | statements executed only when: | condition. X = false | and condition. Y = false | } --+
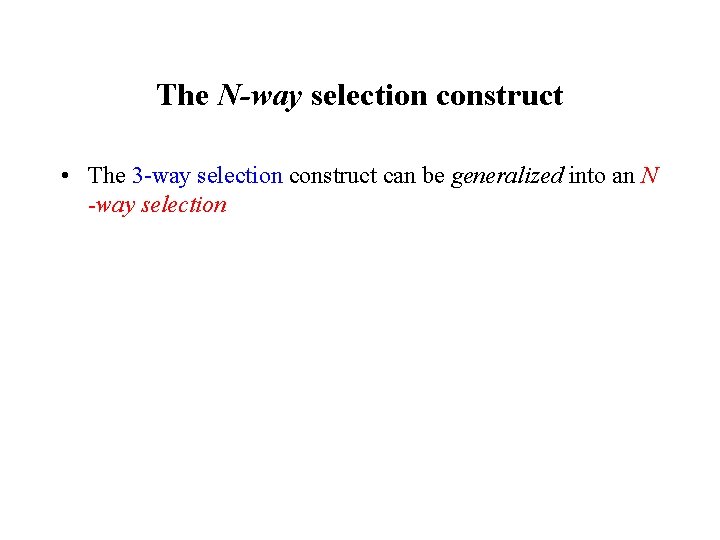
The N-way selection construct • The 3 -way selection construct can be generalized into an N -way selection
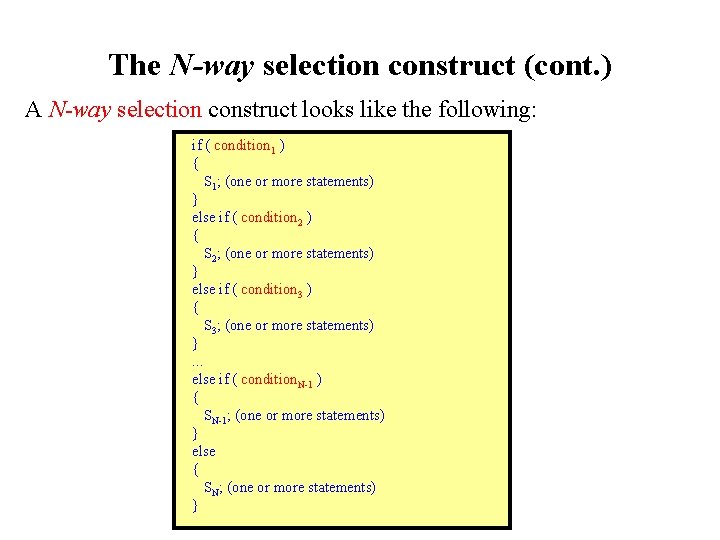
The N-way selection construct (cont. ) A N-way selection construct looks like the following: if ( condition 1 ) { S 1; (one or more statements) } else if ( condition 2 ) { S 2; (one or more statements) } else if ( condition 3 ) { S 3; (one or more statements) } . . . else if ( condition. N-1 ) { SN-1; (one or more statements) } else { SN; (one or more statements) }
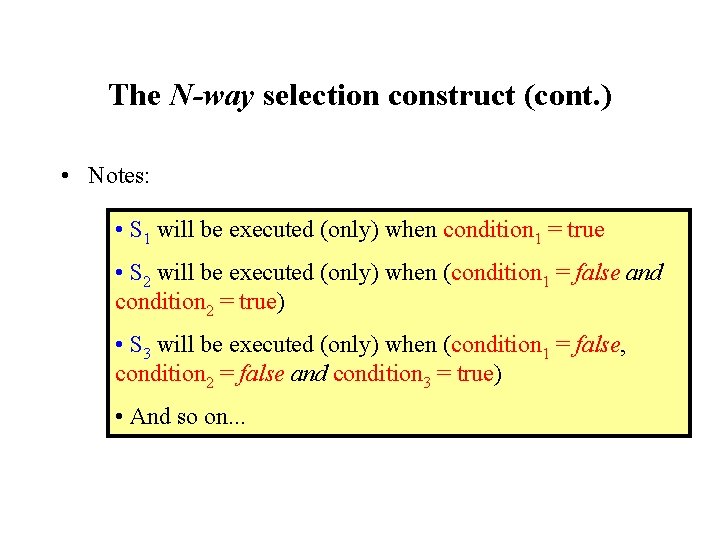
The N-way selection construct (cont. ) • Notes: • S 1 will be executed (only) when condition 1 = true • S 2 will be executed (only) when (condition 1 = false and condition 2 = true) • S 3 will be executed (only) when (condition 1 = false, condition 2 = false and condition 3 = true) • And so on. . .
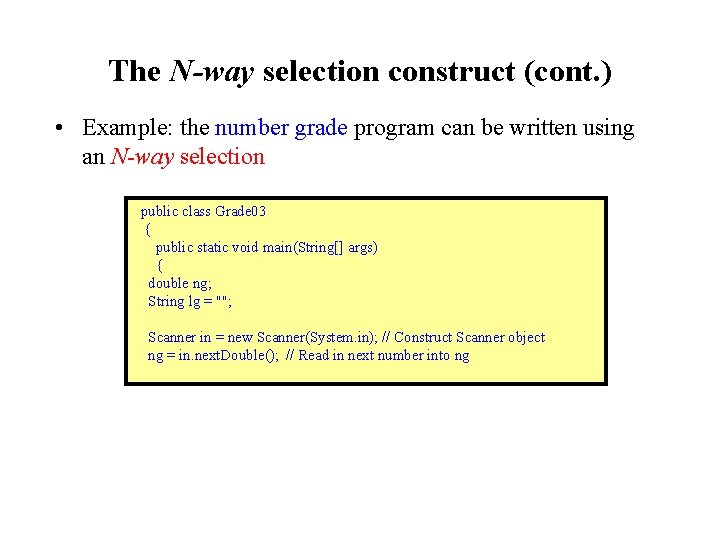
The N-way selection construct (cont. ) • Example: the number grade program can be written using an N-way selection public class Grade 03 { public static void main(String[] args) { double ng; String lg = ""; Scanner in = new Scanner(System. in); // Construct Scanner object ng = in. next. Double(); // Read in next number into ng
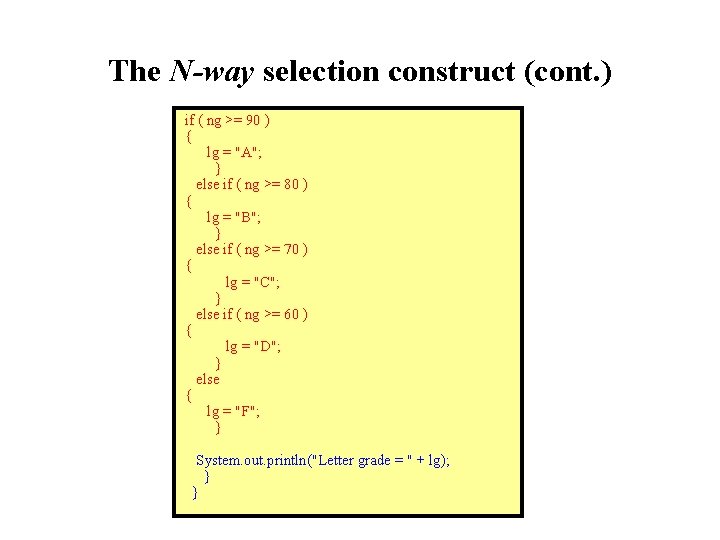
The N-way selection construct (cont. ) if ( ng >= 90 ) { lg = "A"; } else if ( ng >= 80 ) { lg = "B"; } else if ( ng >= 70 ) { lg = "C"; } else if ( ng >= 60 ) { lg = "D"; } else { lg = "F"; } System. out. println("Letter grade = " + lg); } }
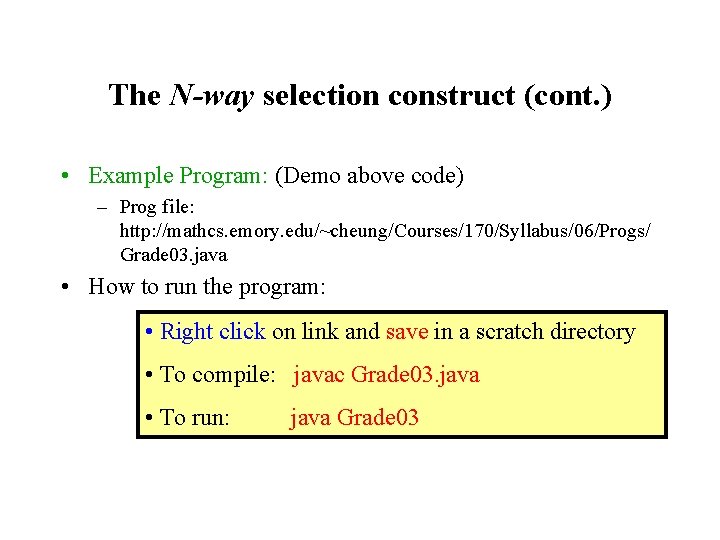
The N-way selection construct (cont. ) • Example Program: (Demo above code) – Prog file: http: //mathcs. emory. edu/~cheung/Courses/170/Syllabus/06/Progs/ Grade 03. java • How to run the program: • Right click on link and save in a scratch directory • To compile: javac Grade 03. java • To run: java Grade 03
Nested conditional statements definition
What thailand was previously known as
Ncaa rsro
Mse 227 csun
Kingdom archaebacteria (previously monera) nutrition
So far we have discussed
Current media and information technology
As discussed earlier meaning
Which of these is not a force discussed in this chapter?
We discussed the problem
As discussed
Having discussed
I all of the questions correctly since
As we discussed before
"datapath"
Categorical imperative formulations
Topics to be discussed
Lets recap
Last time we discussed
Which agency name belongs on the blank line
2-2 conditional statements
Unit 2 homework 3 conditional statements
Conditional and converse statements
Proving conditional statements
Lesson 2-2 conditional statements answer key
Conditional statements matlab
Conditional definition geometry
2-2 conditional statements
Conditional statements definition
Venn diagram conditional statement
Iterative statements in python
Unit 2 homework 3 conditional statements
The past conditional
Inverse of the conditional statement
First conditional rules
Latin subjunctive endings
Control statement
Conditional symbols
Lesson 1 conditional statements
Unit 3 lesson 1 conditional statements
Conditional symbolic notation
Arduino conditional statements
Conditional statement
Linkers for conditionals
Conditional statements examples
Conditional statement
Contoh soal rancangan tersarang
Block nested loop join cost
Algoritma nested loop
Nested loops python