Decisions Decisions Conditional Statements In Java Conditional Statements
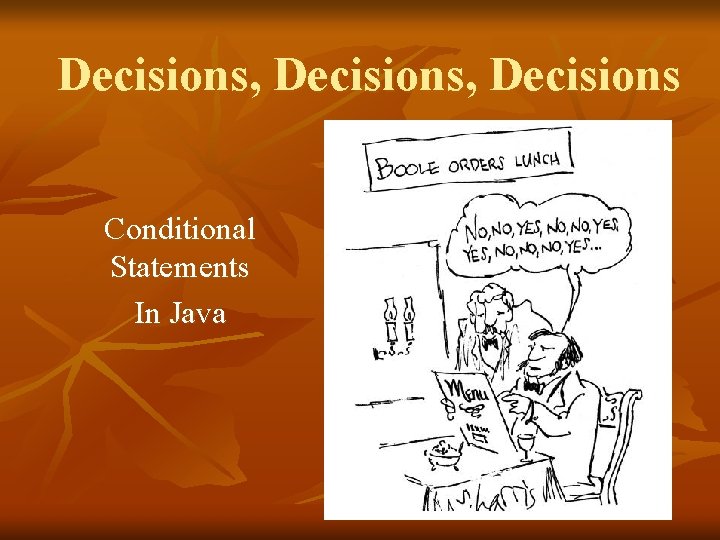
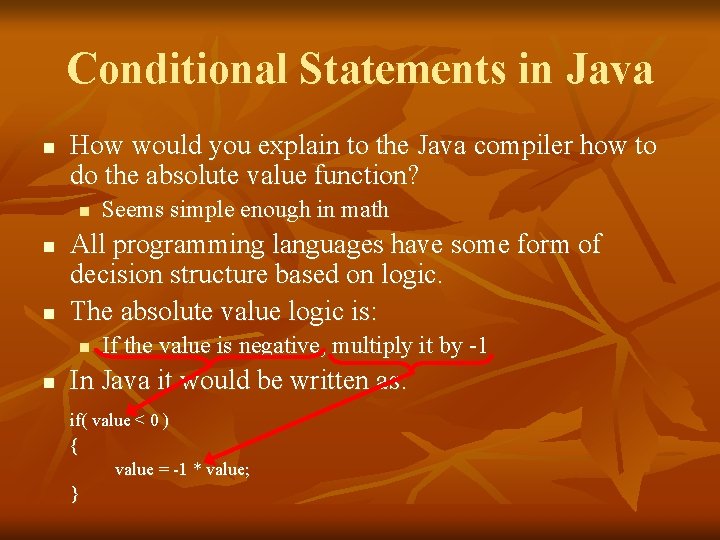
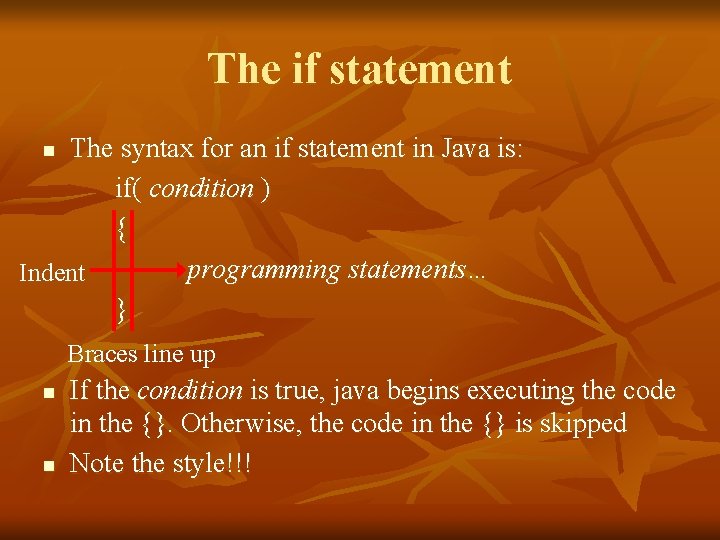
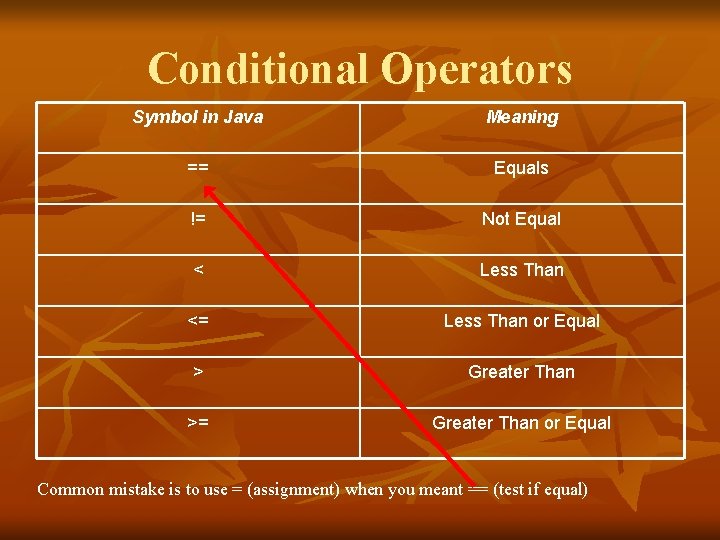
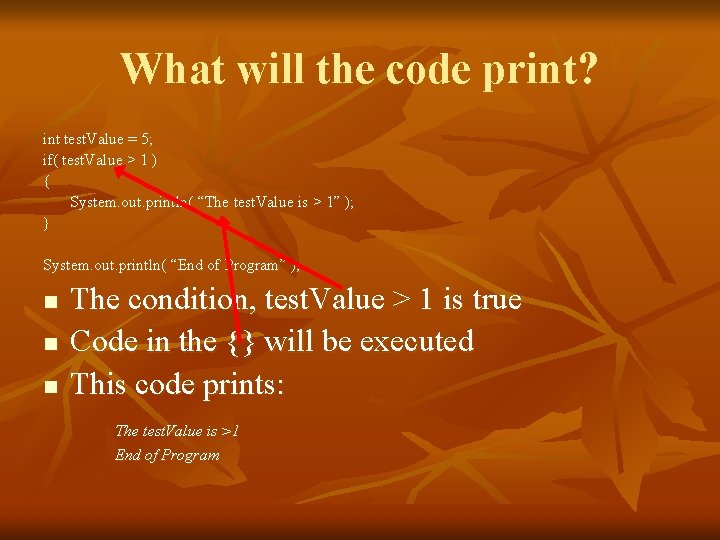
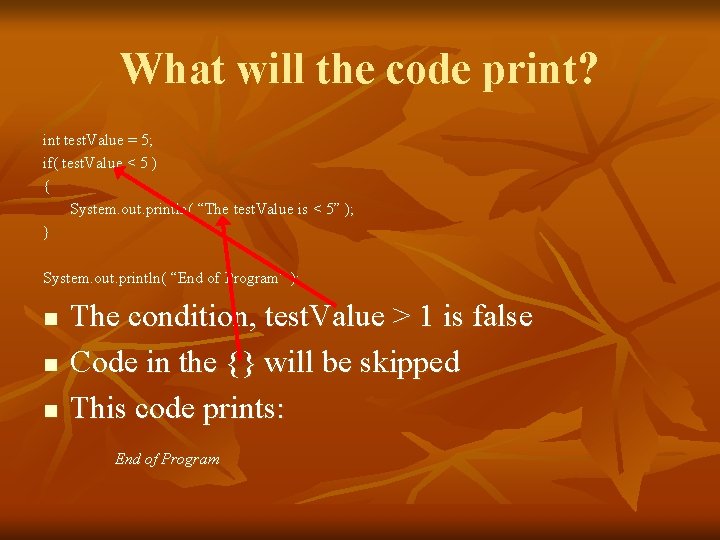
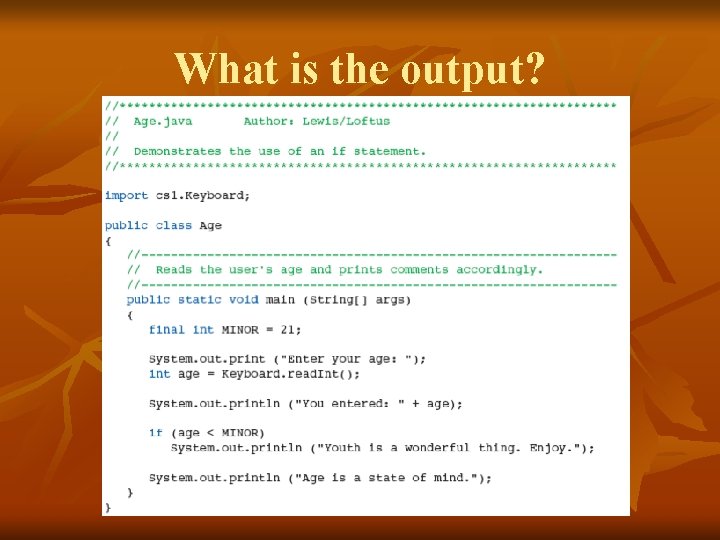
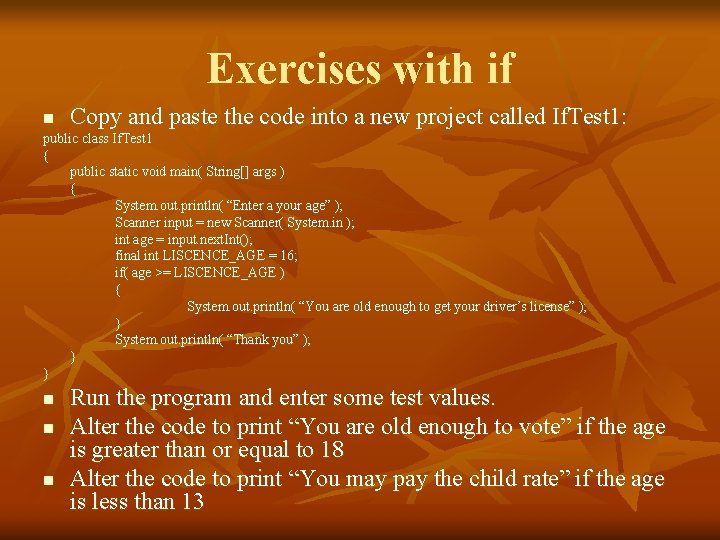
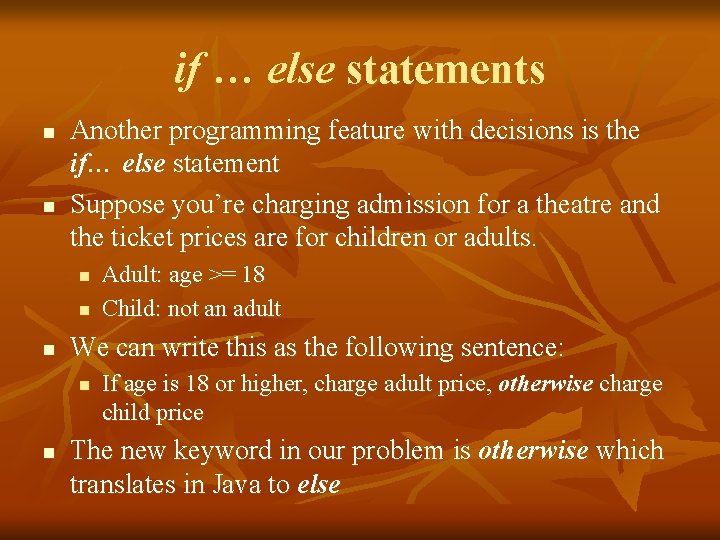
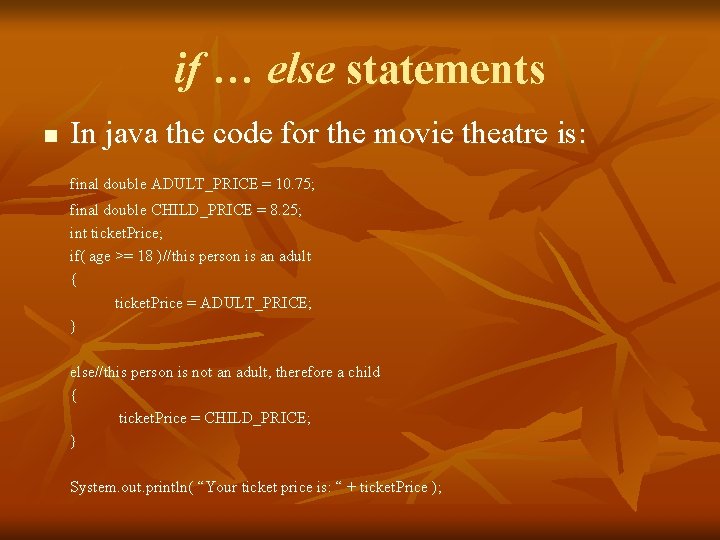
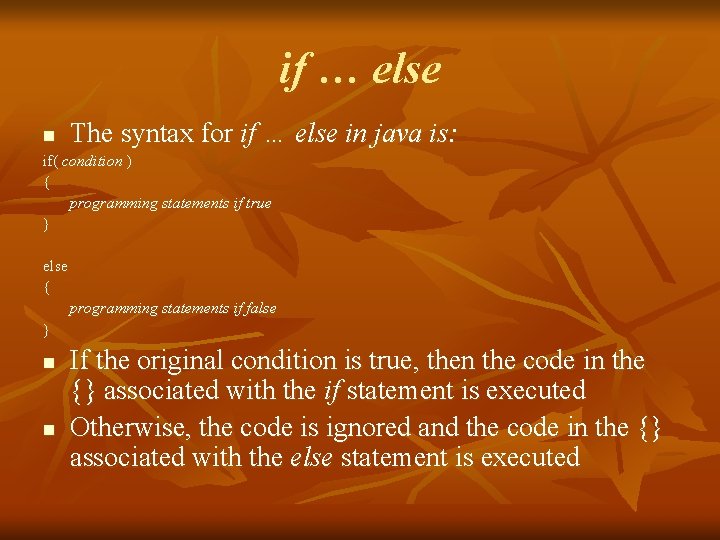
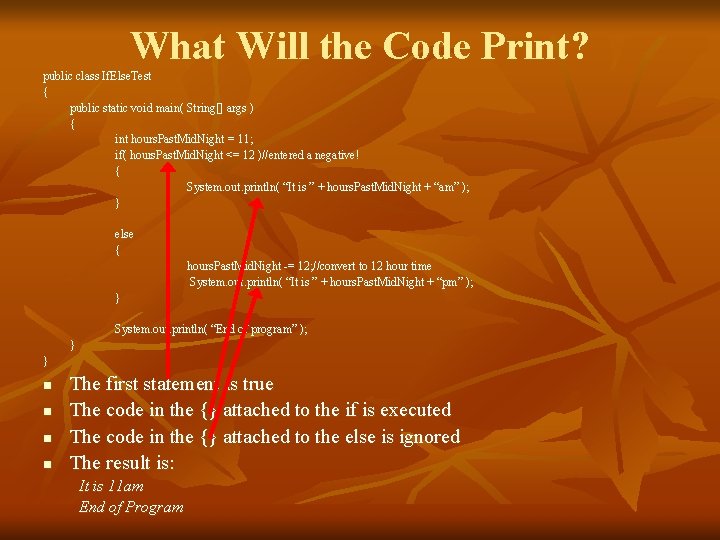
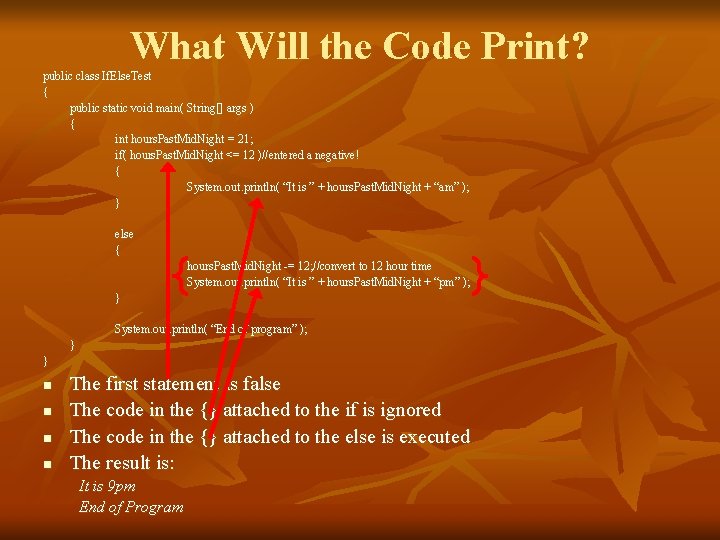
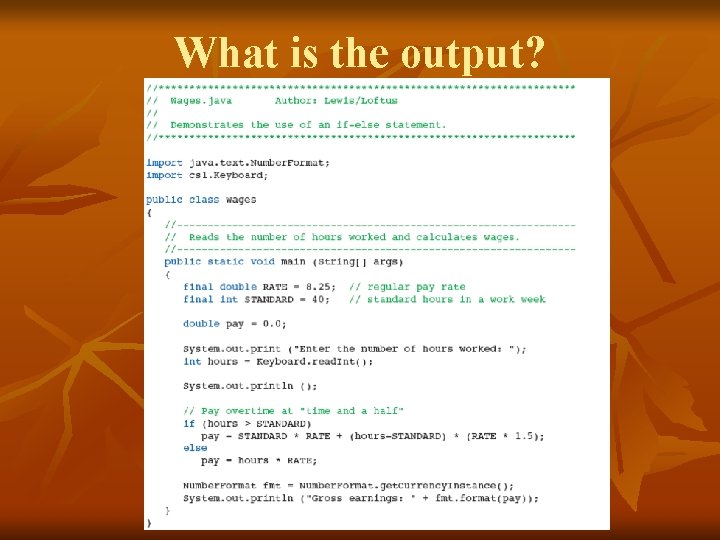
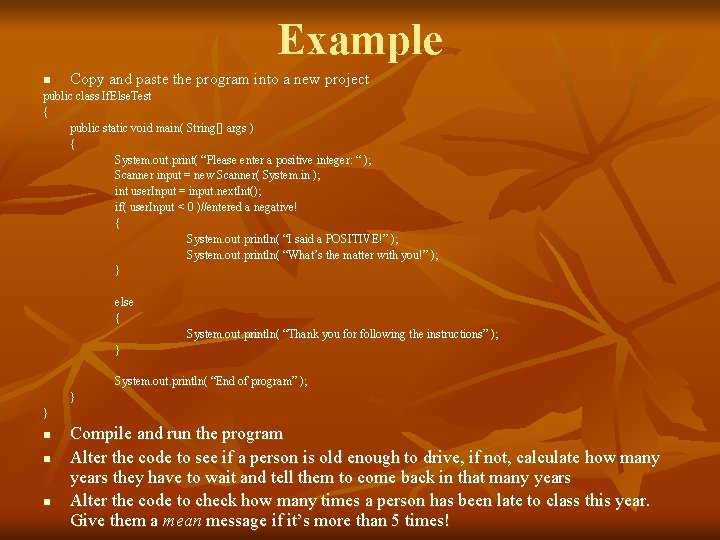
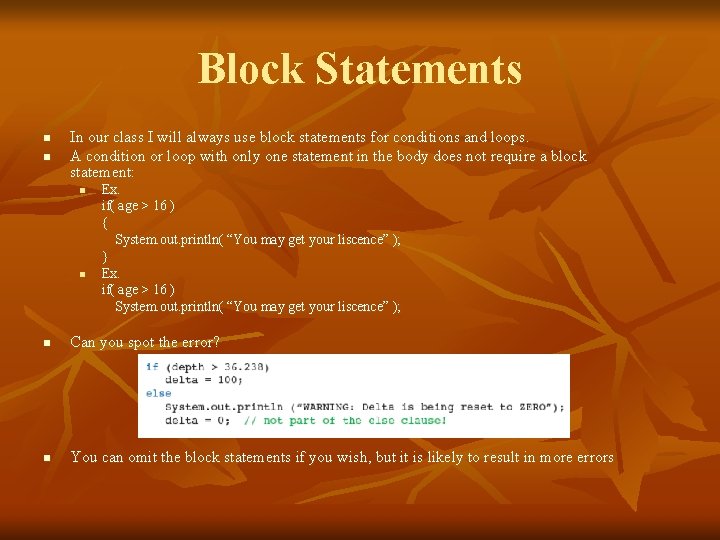
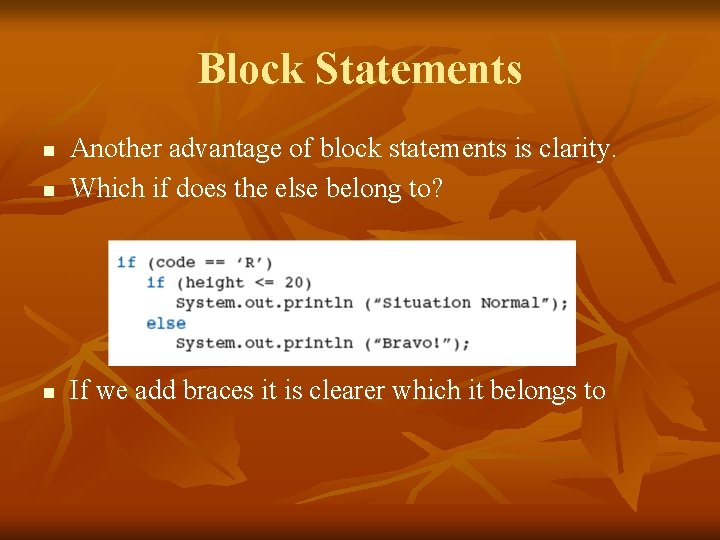
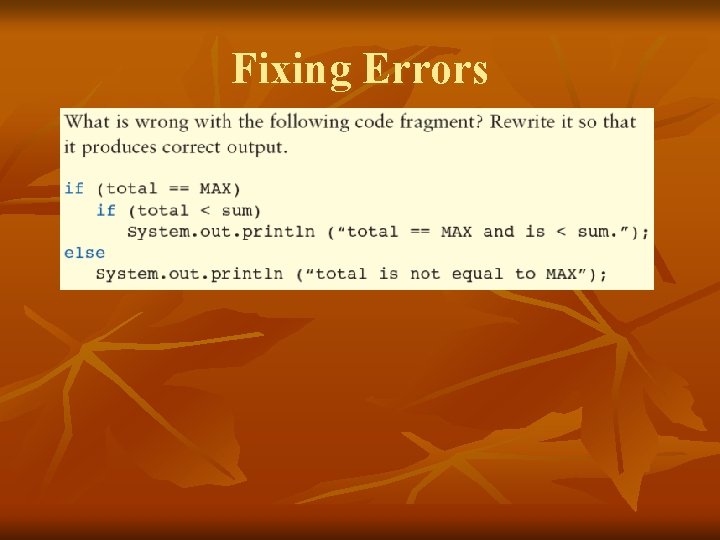
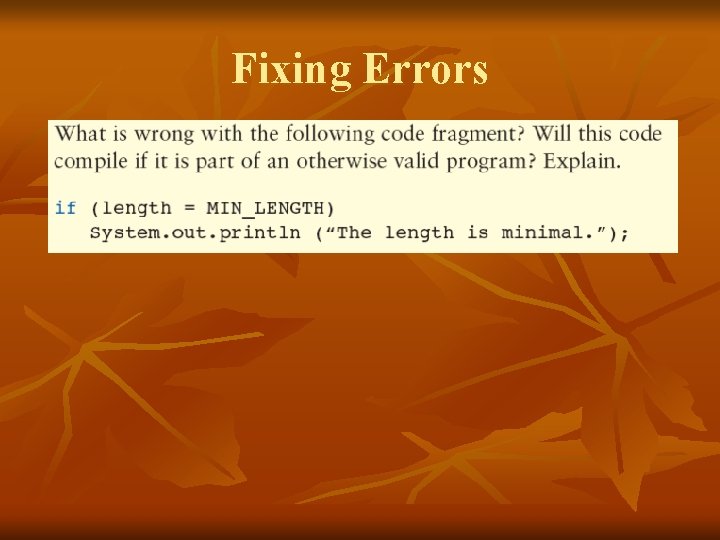
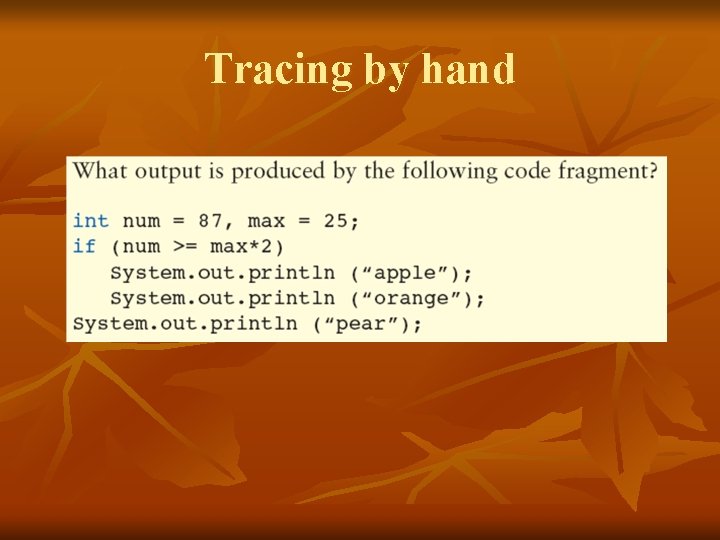
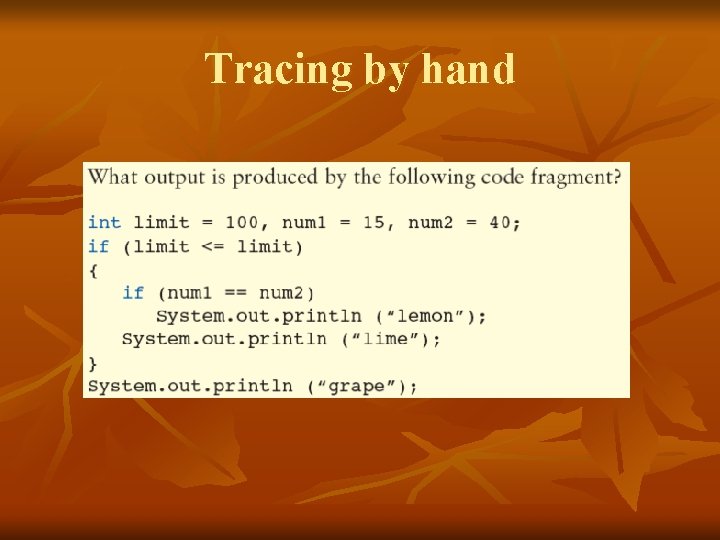
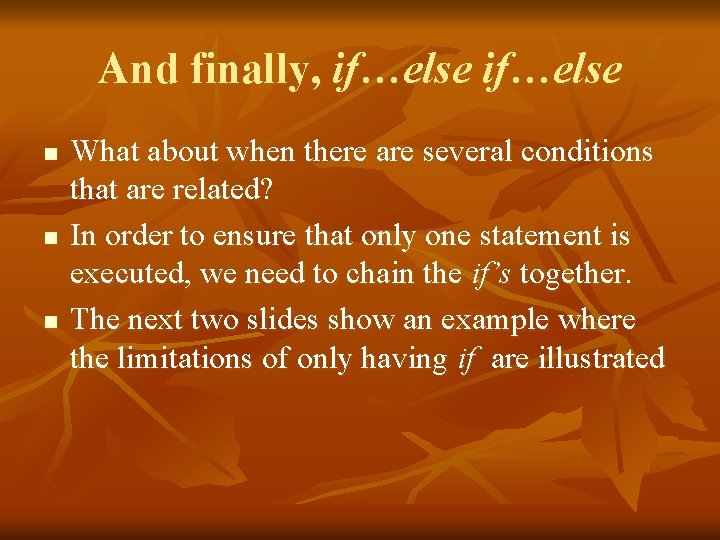
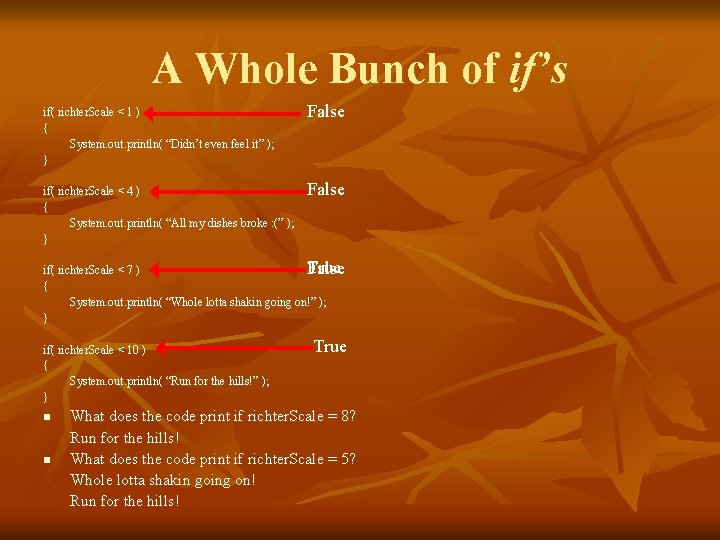
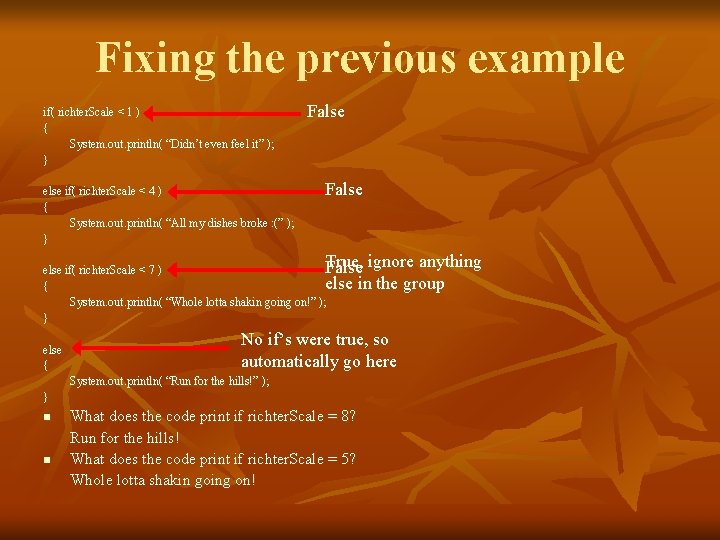
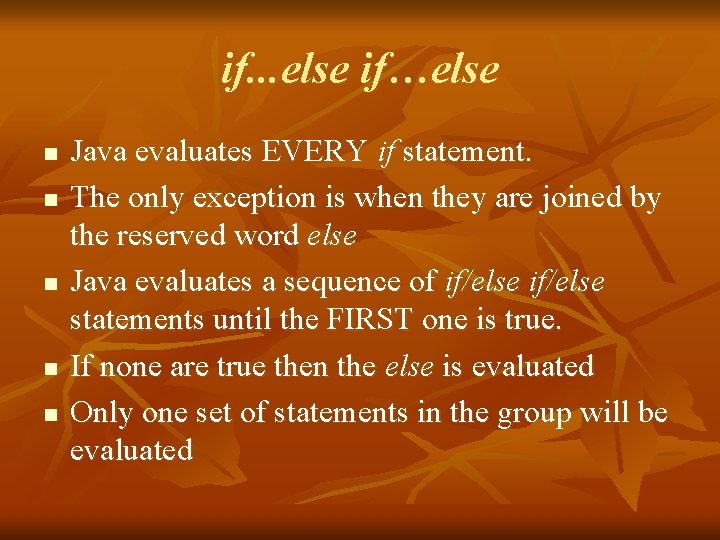
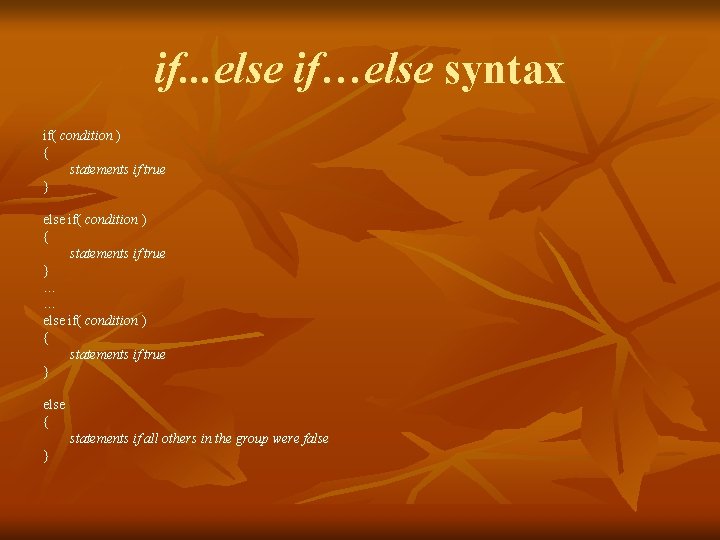
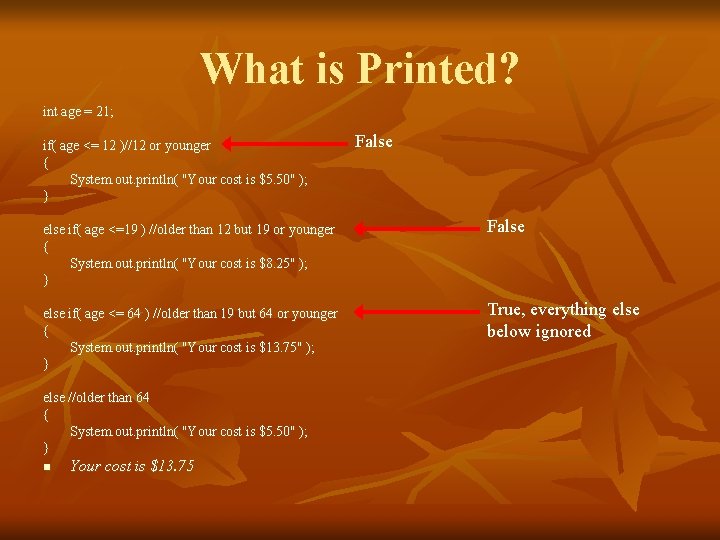
- Slides: 27
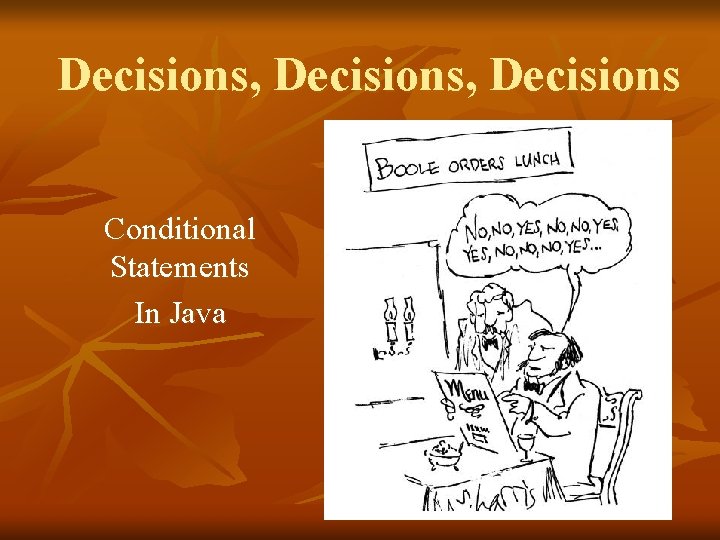
Decisions, Decisions Conditional Statements In Java
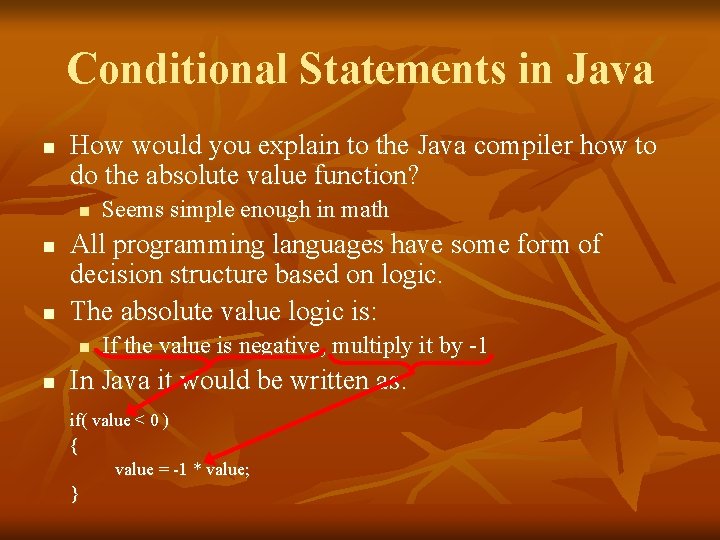
Conditional Statements in Java n How would you explain to the Java compiler how to do the absolute value function? n n n All programming languages have some form of decision structure based on logic. The absolute value logic is: n n Seems simple enough in math If the value is negative, multiply it by -1 In Java it would be written as: if( value < 0 ) { value = -1 * value; }
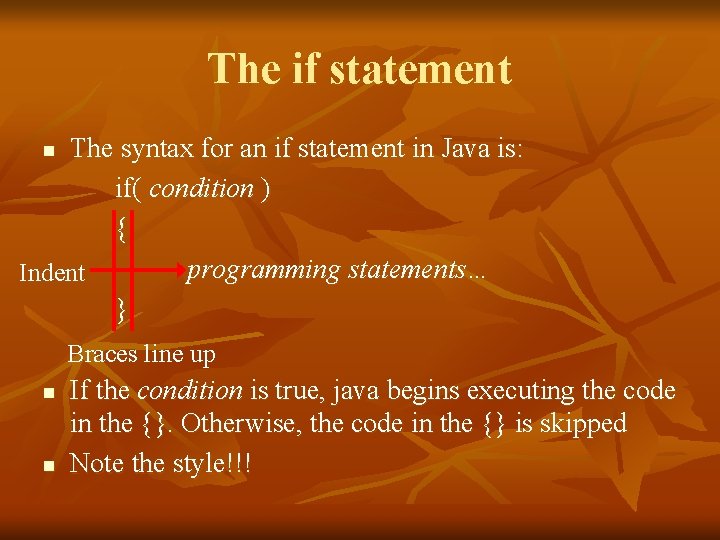
The if statement The syntax for an if statement in Java is: if( condition ) { programming statements… Indent } n Braces line up n n If the condition is true, java begins executing the code in the {}. Otherwise, the code in the {} is skipped Note the style!!!
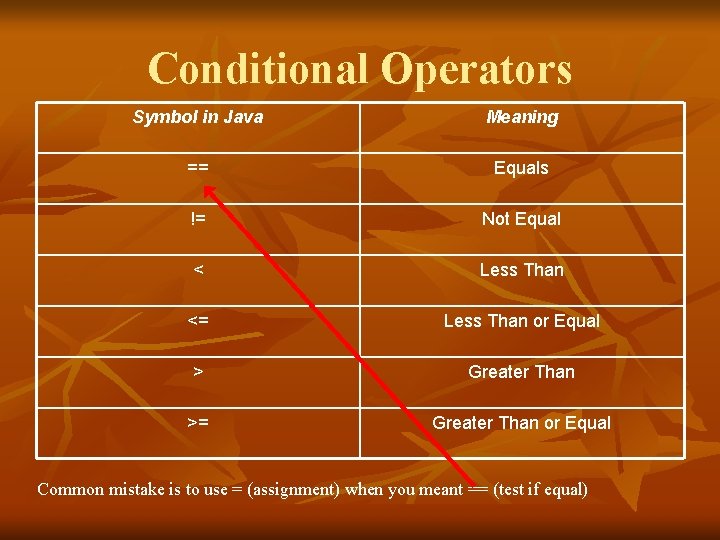
Conditional Operators Symbol in Java Meaning == Equals != Not Equal < Less Than <= Less Than or Equal > Greater Than >= Greater Than or Equal Common mistake is to use = (assignment) when you meant == (test if equal)
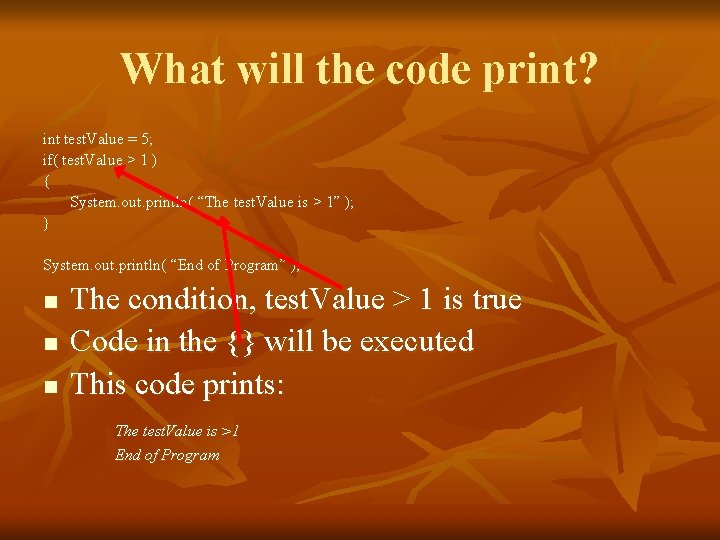
What will the code print? int test. Value = 5; if( test. Value > 1 ) { System. out. println( “The test. Value is > 1” ); } System. out. println( “End of Program” ); n n n The condition, test. Value > 1 is true Code in the {} will be executed This code prints: The test. Value is >1 End of Program
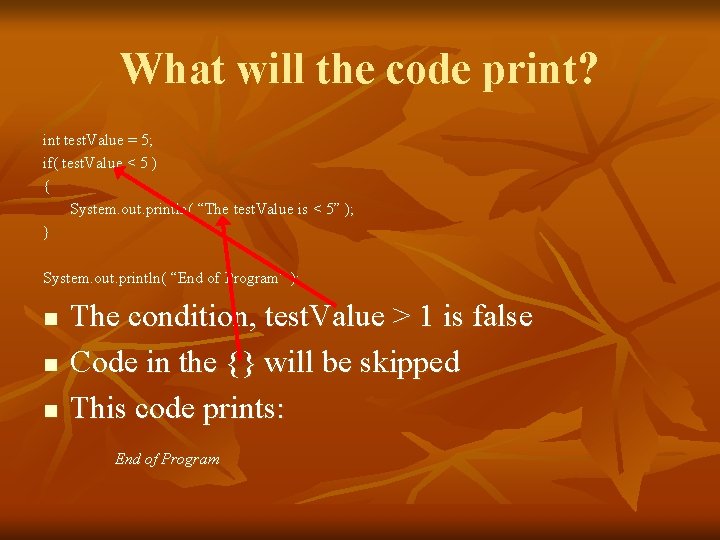
What will the code print? int test. Value = 5; if( test. Value < 5 ) { System. out. println( “The test. Value is < 5” ); } System. out. println( “End of Program” ); n n n The condition, test. Value > 1 is false Code in the {} will be skipped This code prints: End of Program
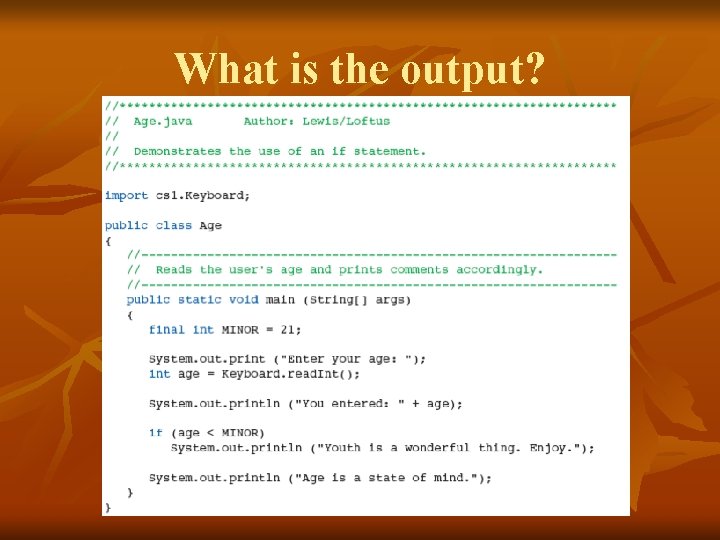
What is the output?
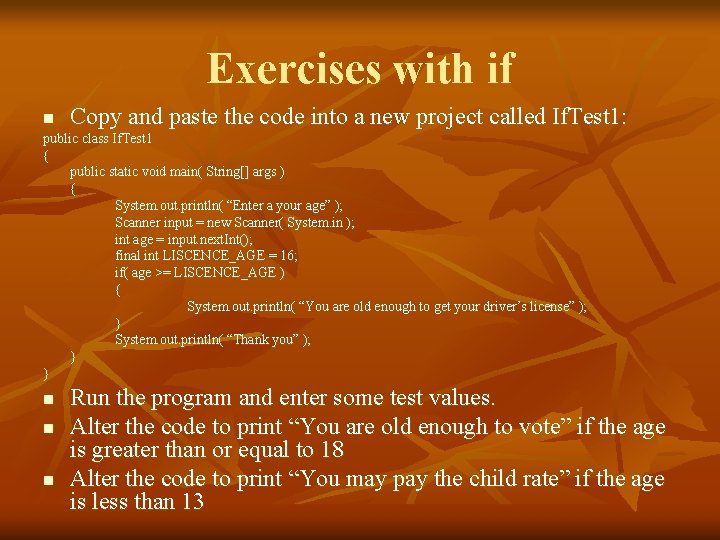
Exercises with if n Copy and paste the code into a new project called If. Test 1: public class If. Test 1 { public static void main( String[] args ) { System. out. println( “Enter a your age” ); Scanner input = new Scanner( System. in ); int age = input. next. Int(); final int LISCENCE_AGE = 16; if( age >= LISCENCE_AGE ) { System. out. println( “You are old enough to get your driver’s license” ); } System. out. println( “Thank you” ); } } n n n Run the program and enter some test values. Alter the code to print “You are old enough to vote” if the age is greater than or equal to 18 Alter the code to print “You may pay the child rate” if the age is less than 13
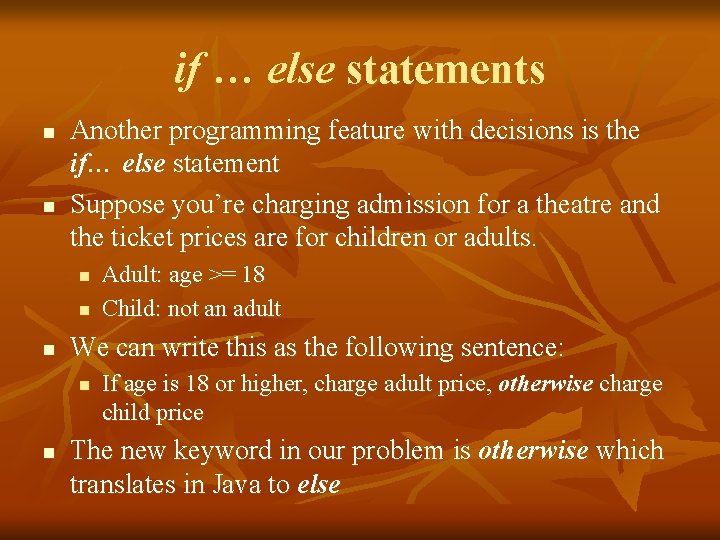
if … else statements n n Another programming feature with decisions is the if… else statement Suppose you’re charging admission for a theatre and the ticket prices are for children or adults. n n n We can write this as the following sentence: n n Adult: age >= 18 Child: not an adult If age is 18 or higher, charge adult price, otherwise charge child price The new keyword in our problem is otherwise which translates in Java to else
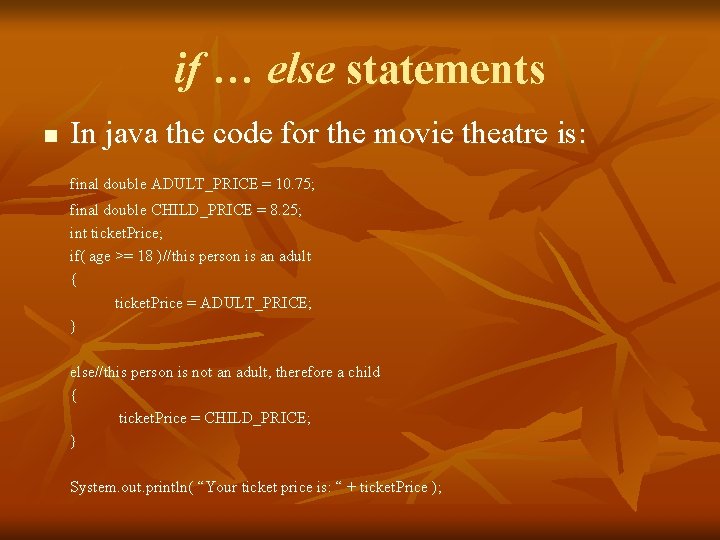
if … else statements n In java the code for the movie theatre is: final double ADULT_PRICE = 10. 75; final double CHILD_PRICE = 8. 25; int ticket. Price; if( age >= 18 )//this person is an adult { ticket. Price = ADULT_PRICE; } else//this person is not an adult, therefore a child { ticket. Price = CHILD_PRICE; } System. out. println( “Your ticket price is: “ + ticket. Price );
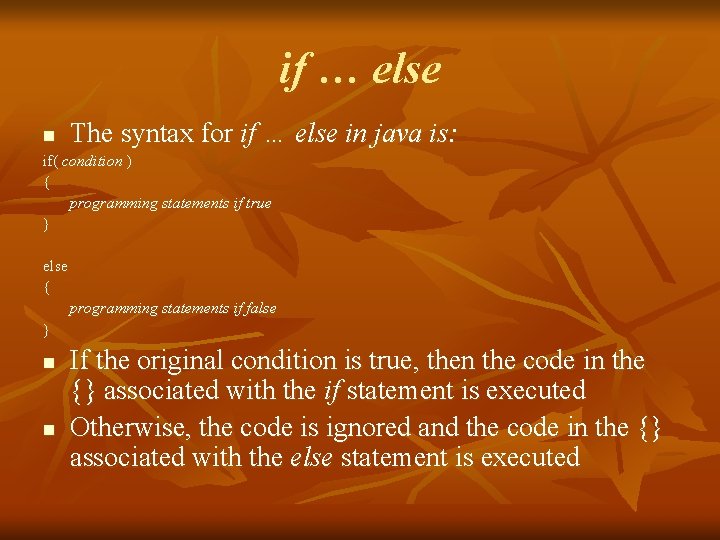
if … else n The syntax for if … else in java is: if( condition ) { programming statements if true } else { programming statements if false } n n If the original condition is true, then the code in the {} associated with the if statement is executed Otherwise, the code is ignored and the code in the {} associated with the else statement is executed
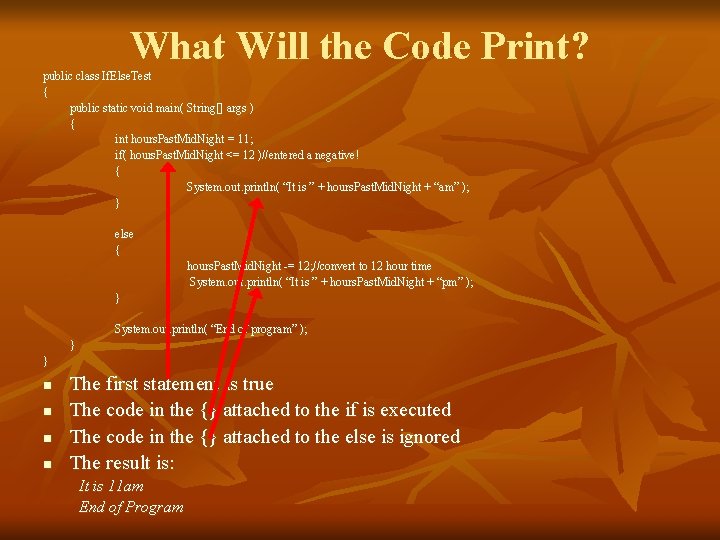
What Will the Code Print? public class If. Else. Test { public static void main( String[] args ) { int hours. Past. Mid. Night = 11; if( hours. Past. Mid. Night <= 12 )//entered a negative! { System. out. println( “It is ” + hours. Past. Mid. Night + “am” ); } else { hours. Past. Mid. Night -= 12; //convert to 12 hour time System. out. println( “It is ” + hours. Past. Mid. Night + “pm” ); } System. out. println( “End of program” ); } } n n The first statement is true The code in the {} attached to the if is executed The code in the {} attached to the else is ignored The result is: It is 11 am End of Program
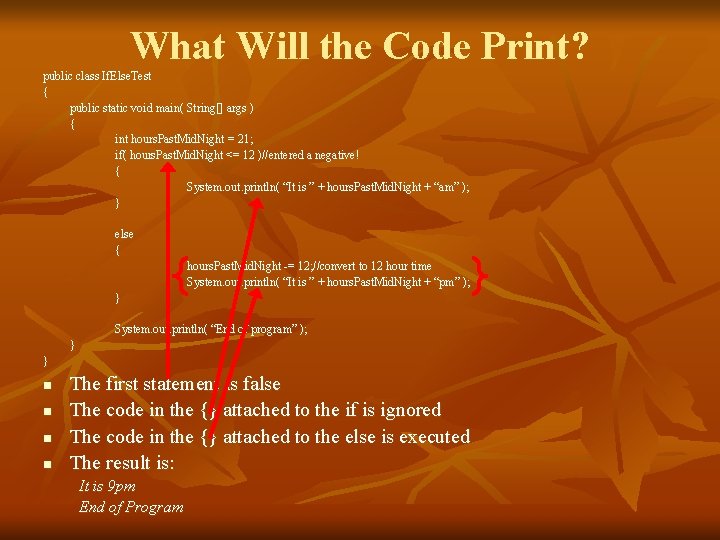
What Will the Code Print? public class If. Else. Test { public static void main( String[] args ) { int hours. Past. Mid. Night = 21; if( hours. Past. Mid. Night <= 12 )//entered a negative! { System. out. println( “It is ” + hours. Past. Mid. Night + “am” ); } else { hours. Past. Mid. Night -= 12; //convert to 12 hour time System. out. println( “It is ” + hours. Past. Mid. Night + “pm” ); } System. out. println( “End of program” ); } } n n The first statement is false The code in the {} attached to the if is ignored The code in the {} attached to the else is executed The result is: It is 9 pm End of Program
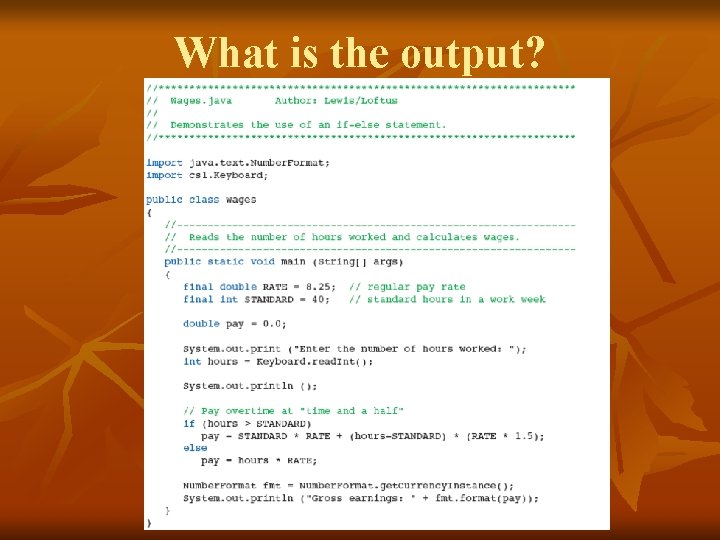
What is the output?
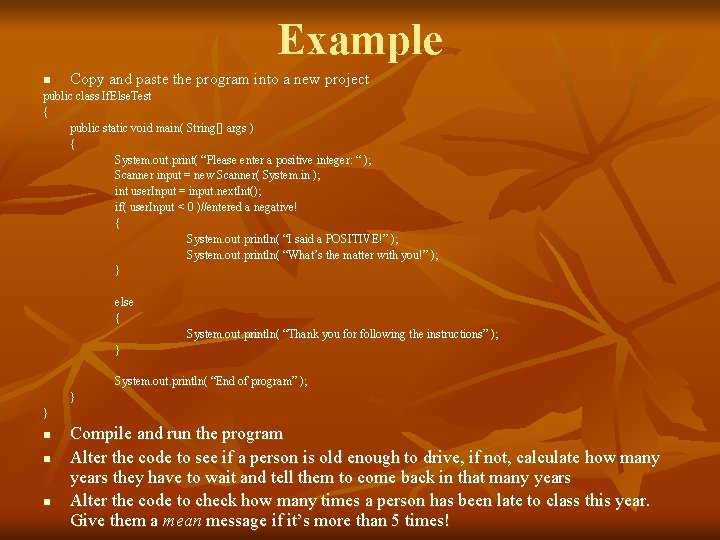
Example n Copy and paste the program into a new project public class If. Else. Test { public static void main( String[] args ) { System. out. print( “Please enter a positive integer: “ ); Scanner input = new Scanner( System. in ); int user. Input = input. next. Int(); if( user. Input < 0 )//entered a negative! { System. out. println( “I said a POSITIVE!” ); System. out. println( “What’s the matter with you!” ); } else { System. out. println( “Thank you for following the instructions” ); } System. out. println( “End of program” ); } } n n n Compile and run the program Alter the code to see if a person is old enough to drive, if not, calculate how many years they have to wait and tell them to come back in that many years Alter the code to check how many times a person has been late to class this year. Give them a mean message if it’s more than 5 times!
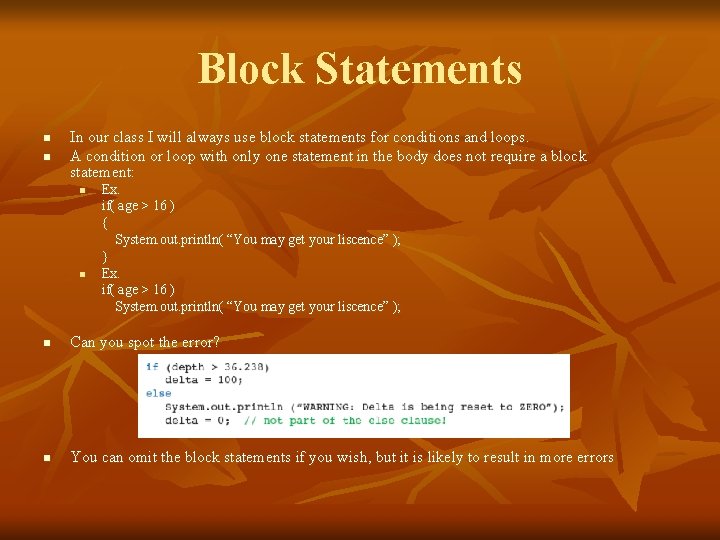
Block Statements n n In our class I will always use block statements for conditions and loops. A condition or loop with only one statement in the body does not require a block statement: n n Ex. if( age > 16 ) { System. out. println( “You may get your liscence” ); } Ex. if( age > 16 ) System. out. println( “You may get your liscence” ); n Can you spot the error? n You can omit the block statements if you wish, but it is likely to result in more errors
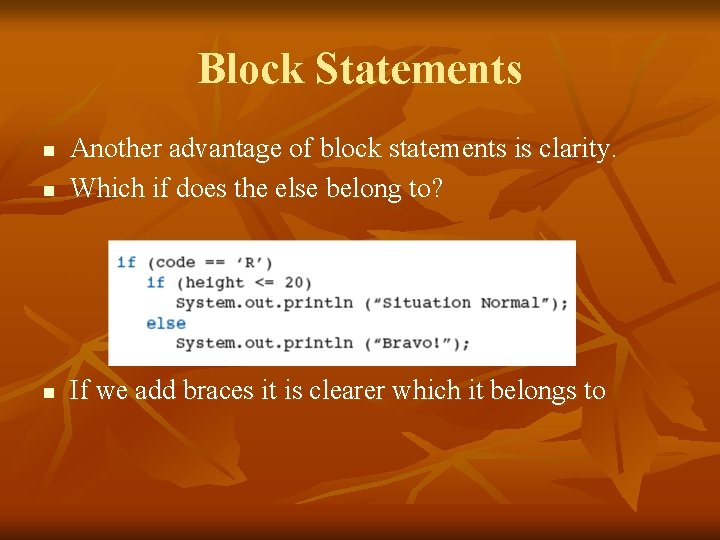
Block Statements n Another advantage of block statements is clarity. Which if does the else belong to? n If we add braces it is clearer which it belongs to n
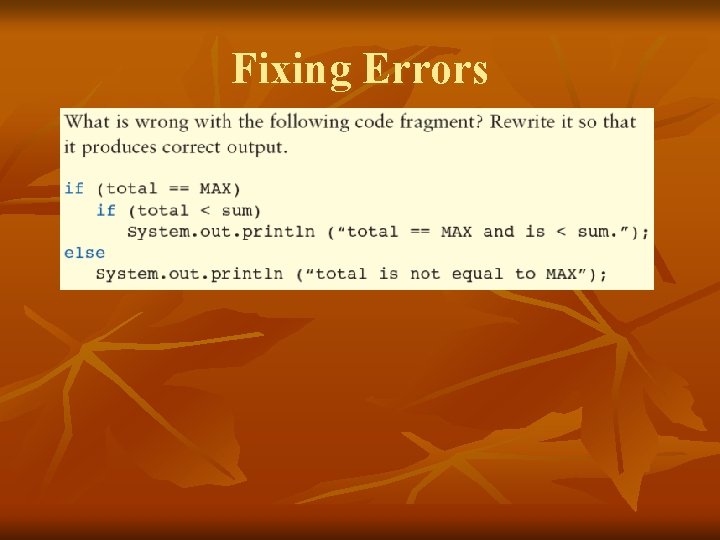
Fixing Errors
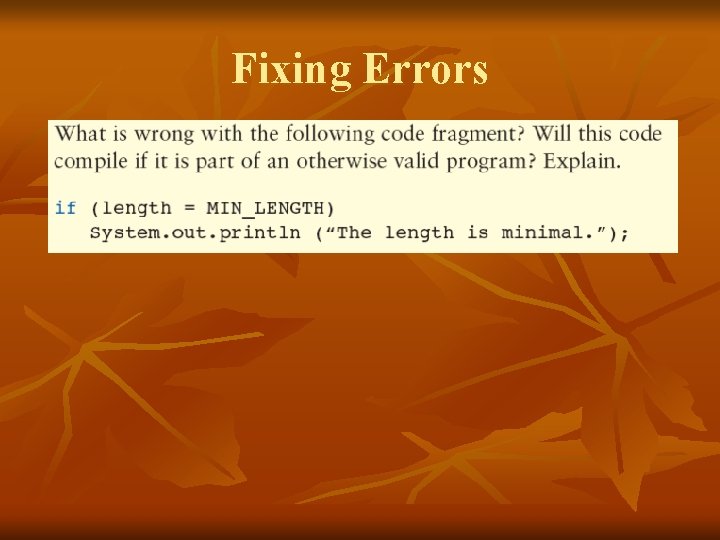
Fixing Errors
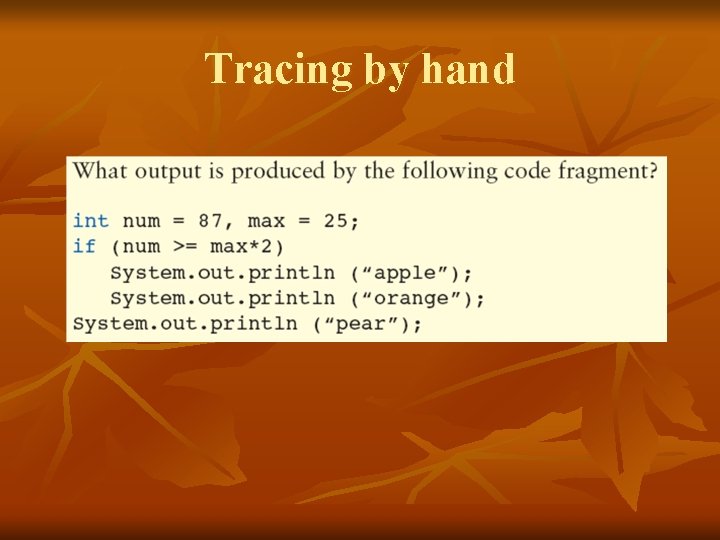
Tracing by hand
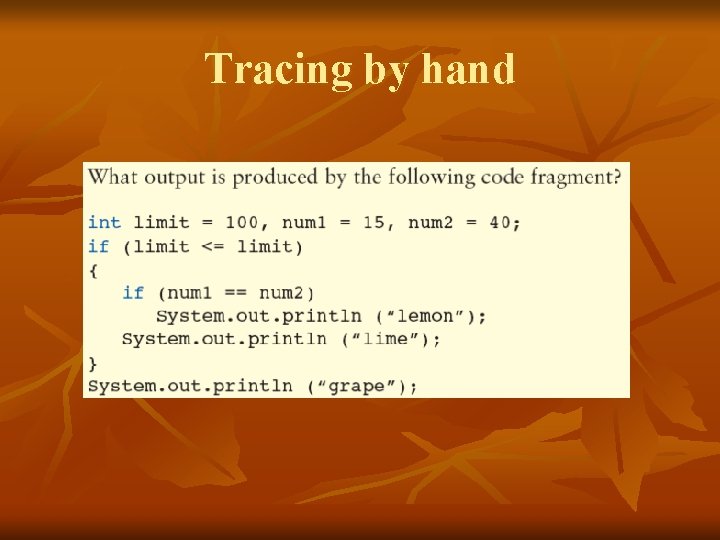
Tracing by hand
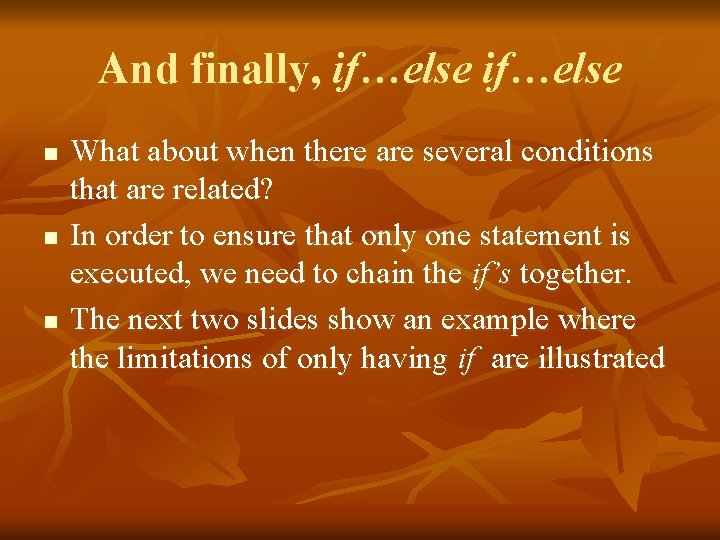
And finally, if…else n n n What about when there are several conditions that are related? In order to ensure that only one statement is executed, we need to chain the if’s together. The next two slides show an example where the limitations of only having if are illustrated
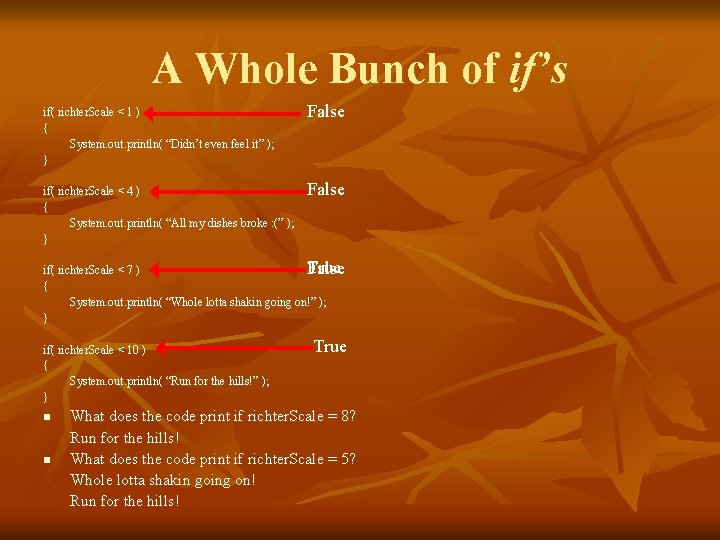
A Whole Bunch of if’s if( richter. Scale < 1 ) { System. out. println( “Didn’t even feel it” ); } False if( richter. Scale < 4 ) { System. out. println( “All my dishes broke : (” ); } False True False if( richter. Scale < 7 ) { System. out. println( “Whole lotta shakin going on!” ); } if( richter. Scale < 10 ) { System. out. println( “Run for the hills!” ); } n n True What does the code print if richter. Scale = 8? Run for the hills! What does the code print if richter. Scale = 5? Whole lotta shakin going on! Run for the hills!
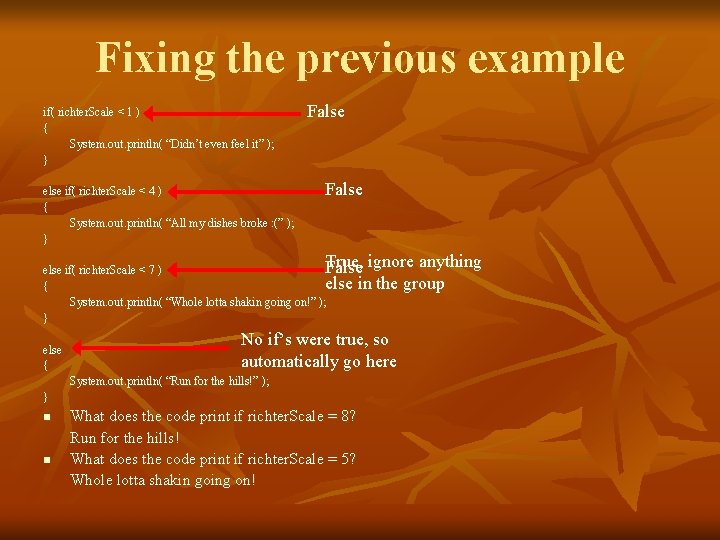
Fixing the previous example if( richter. Scale < 1 ) { System. out. println( “Didn’t even feel it” ); } else if( richter. Scale < 4 ) { System. out. println( “All my dishes broke : (” ); } False True, ignore anything the group False else if( richter. Scale < 7 ) else in { System. out. println( “Whole lotta shakin going on!” ); } else { No if’s were true, so automatically go here System. out. println( “Run for the hills!” ); } n n What does the code print if richter. Scale = 8? Run for the hills! What does the code print if richter. Scale = 5? Whole lotta shakin going on!
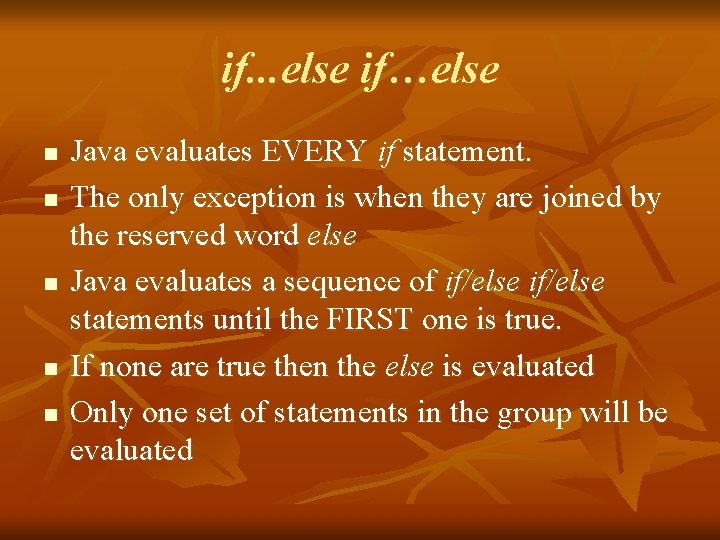
if. . . else if…else n n n Java evaluates EVERY if statement. The only exception is when they are joined by the reserved word else Java evaluates a sequence of if/else statements until the FIRST one is true. If none are true then the else is evaluated Only one set of statements in the group will be evaluated
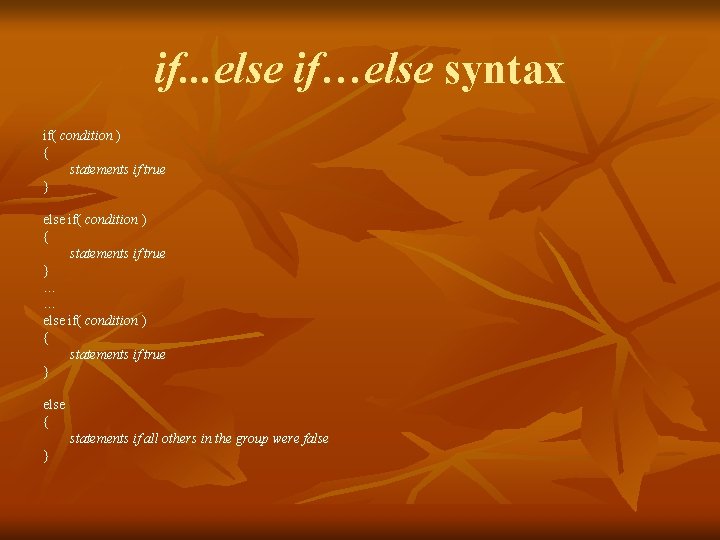
if. . . else if…else syntax if( condition ) { statements if true } else if( condition ) { statements if true } … … else if( condition ) { statements if true } else { statements if all others in the group were false }
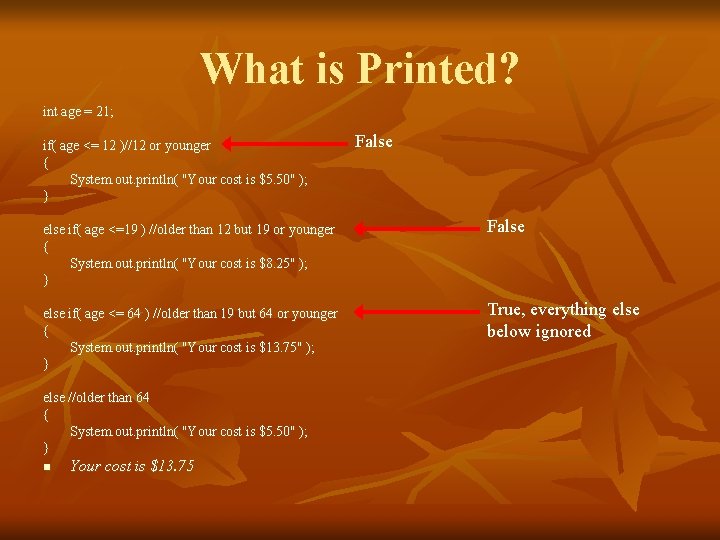
What is Printed? int age = 21; if( age <= 12 )//12 or younger { System. out. println( "Your cost is $5. 50" ); } False else if( age <=19 ) //older than 12 but 19 or younger { System. out. println( "Your cost is $8. 25" ); } False else if( age <= 64 ) //older than 19 but 64 or younger { System. out. println( "Your cost is $13. 75" ); } True, everything else below ignored else //older than 64 { System. out. println( "Your cost is $5. 50" ); } n Your cost is $13. 75
Decisions decisions decisions poster
Screening decisions and preference decisions
Lesson 2-2 conditional statements
Conditional statements geometry
First conditional rules
Converse geometry definition
2-2 practice conditional statements
Differentiate between conditional and iterative statements
Conditional statements examples
Bioconditional statement
Lesson 2-2 conditional statements
Third conditional past unreal
Nested conditional statements definition
First conditionals examples
Conditional statements matlab
Related conditionals
Unit 3 lesson 1 conditional statements
Unit 2 homework 3 conditional statements
Venn diagram or statement
Conditional control
If statement definition
Unit 2 logic and proof homework 3 conditional statements
Unit 2 homework 3 conditional statements
Conditional geometry
2-2 conditional statements
Conditional statements
Latin subjunctive endings
Arduino conditional statements