ME 171 Computer Programming Language Partha Kumar Das
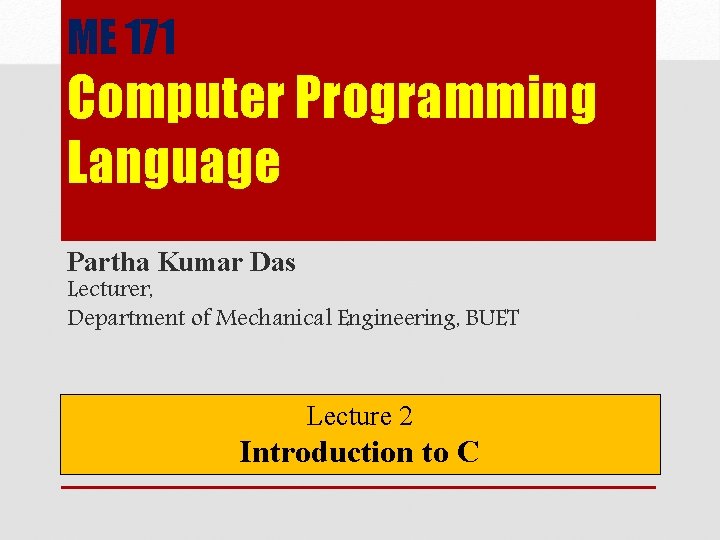
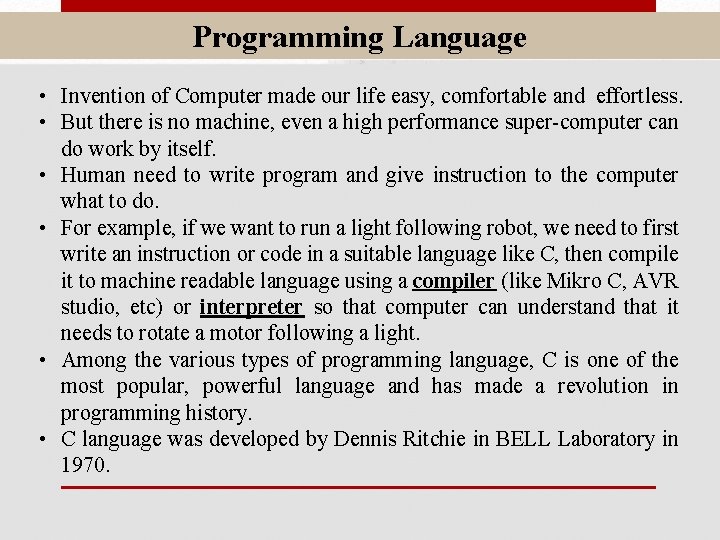
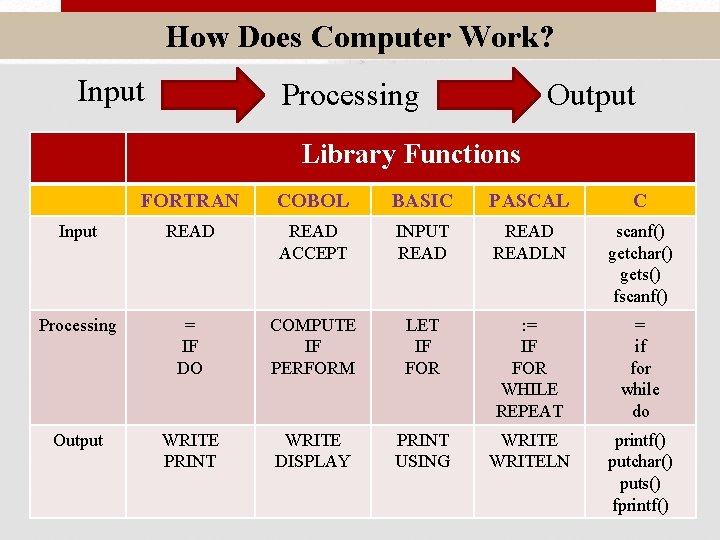
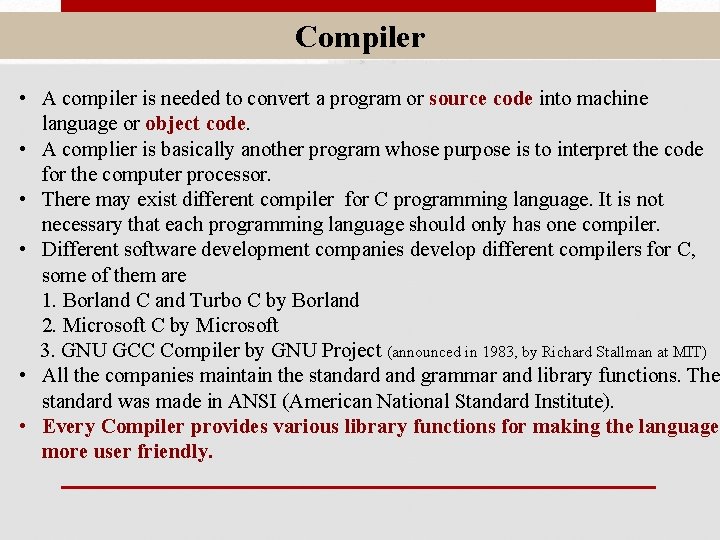
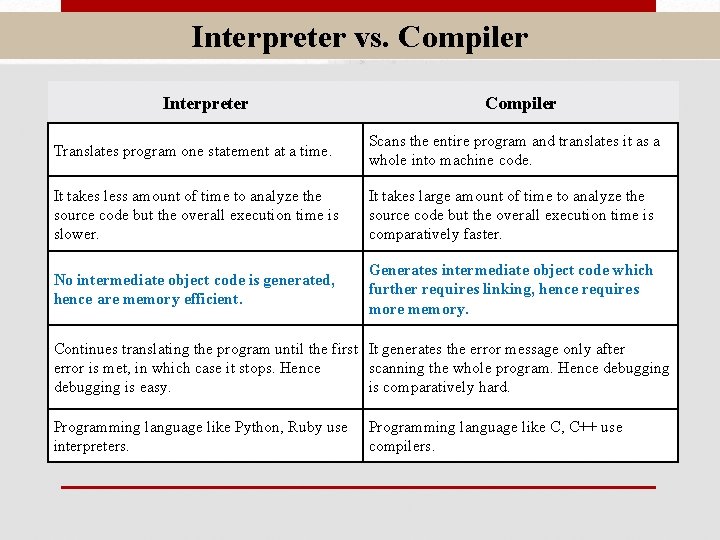
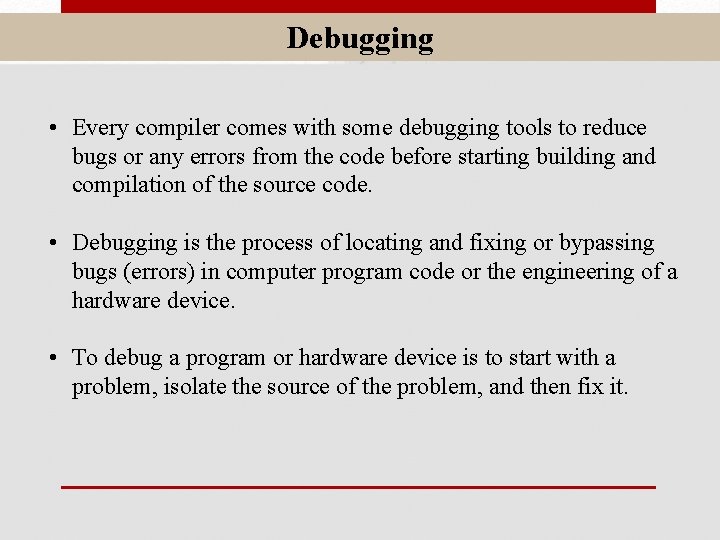
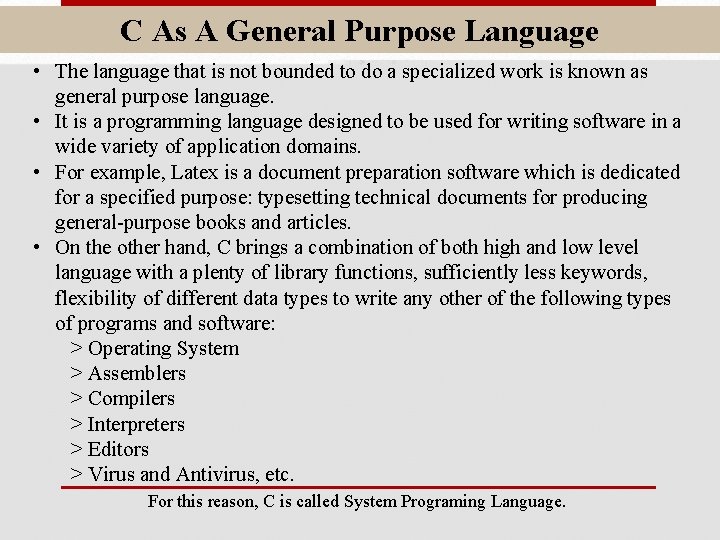
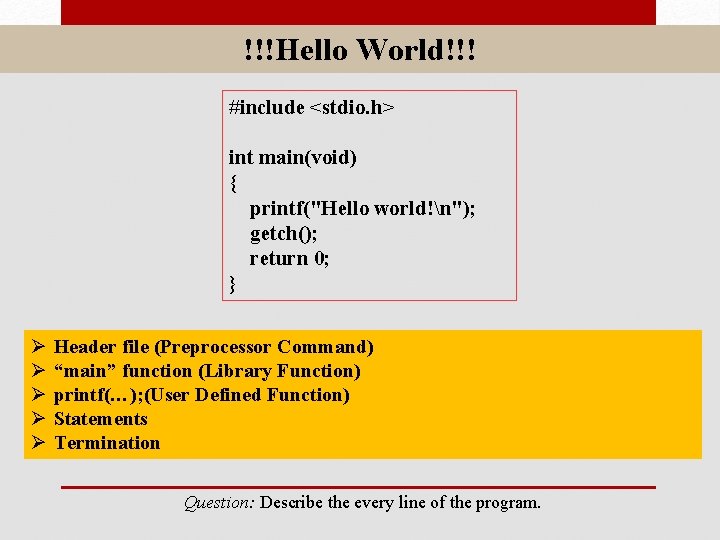
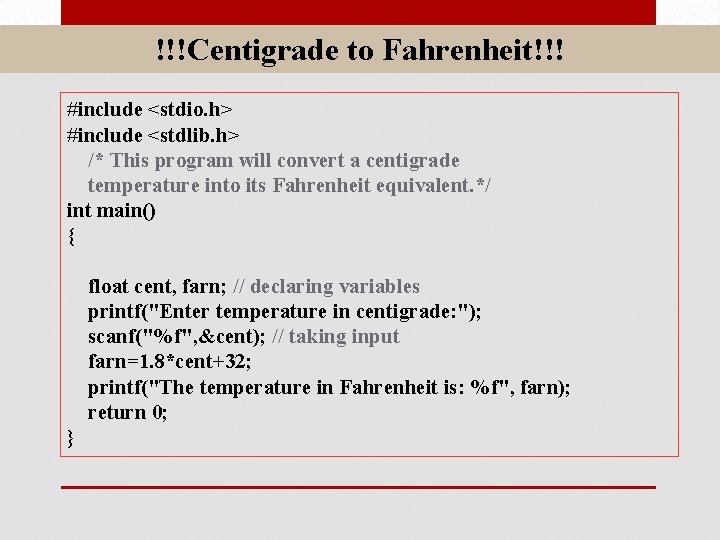
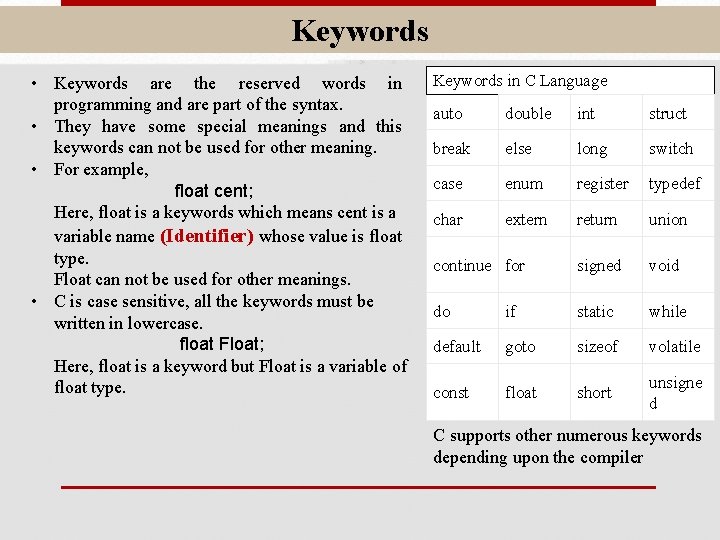
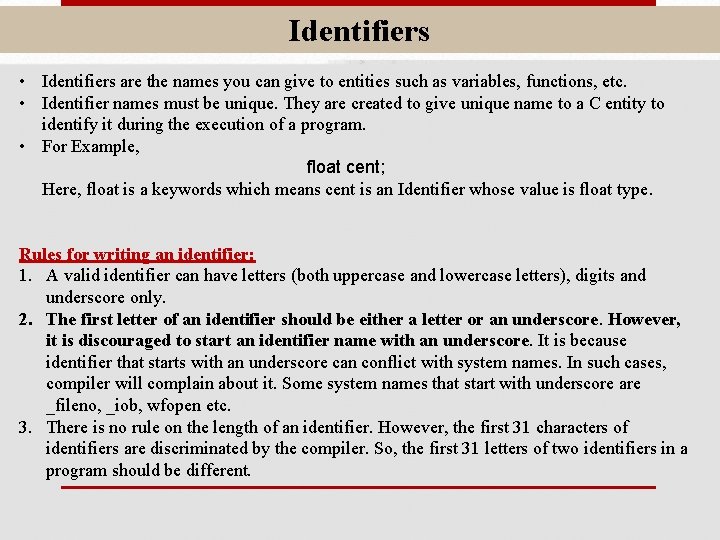
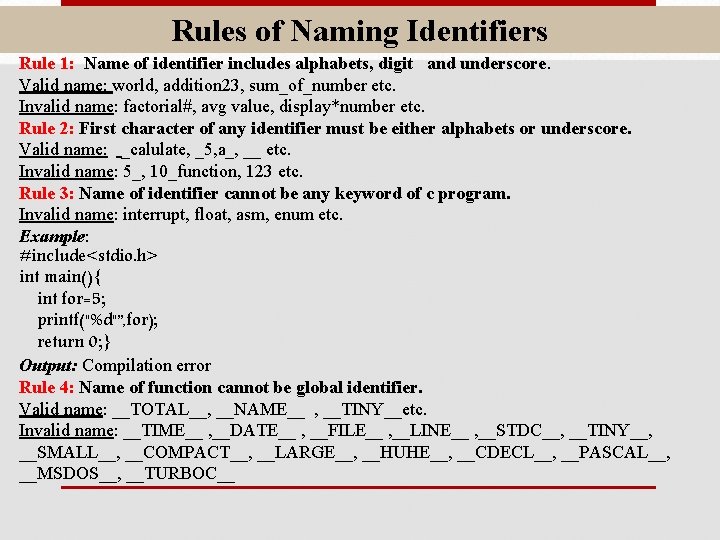
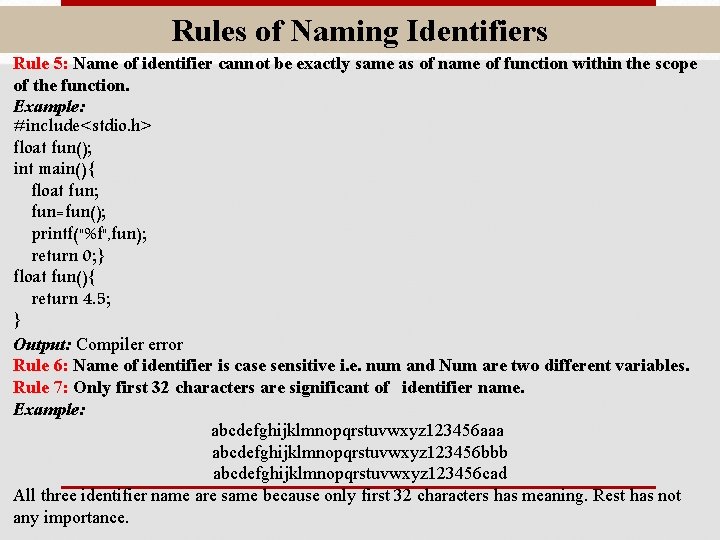
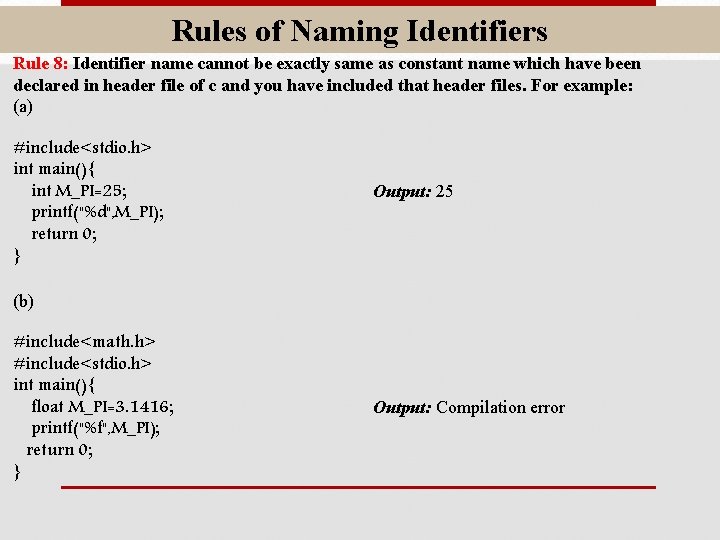
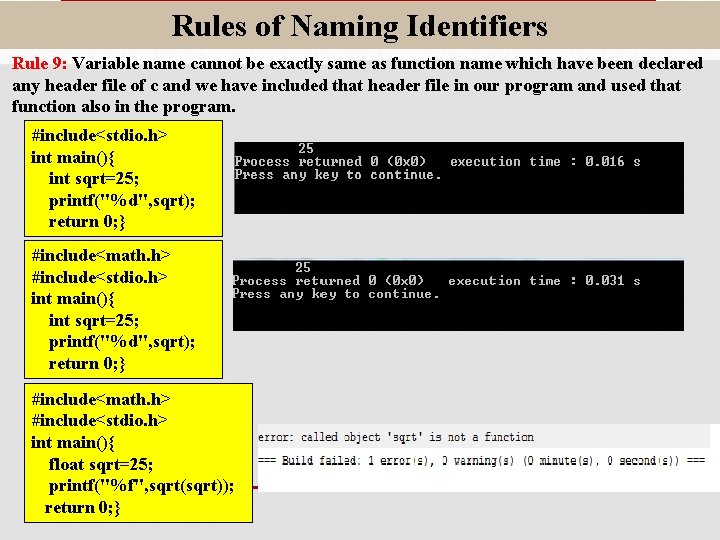
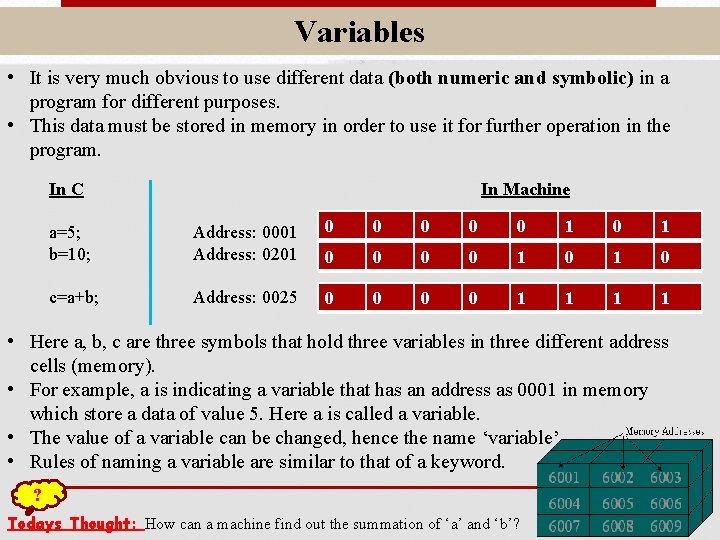
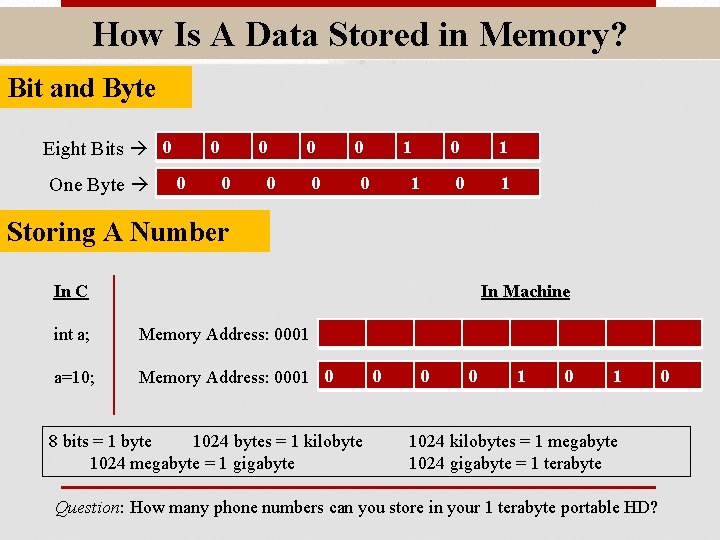
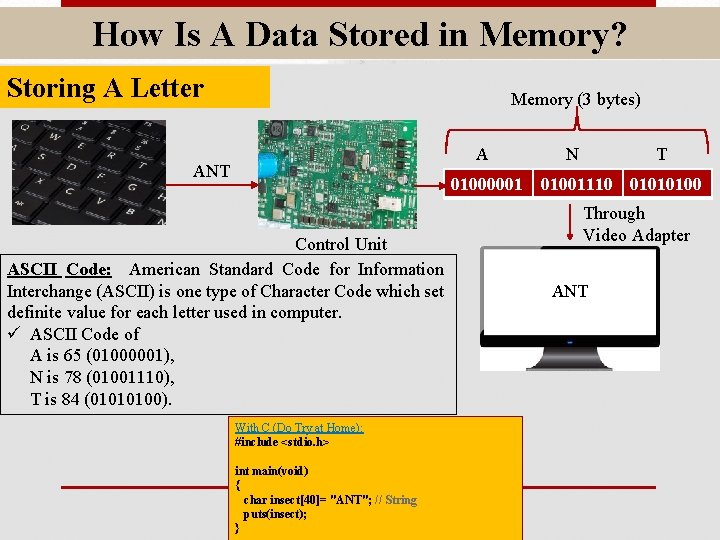
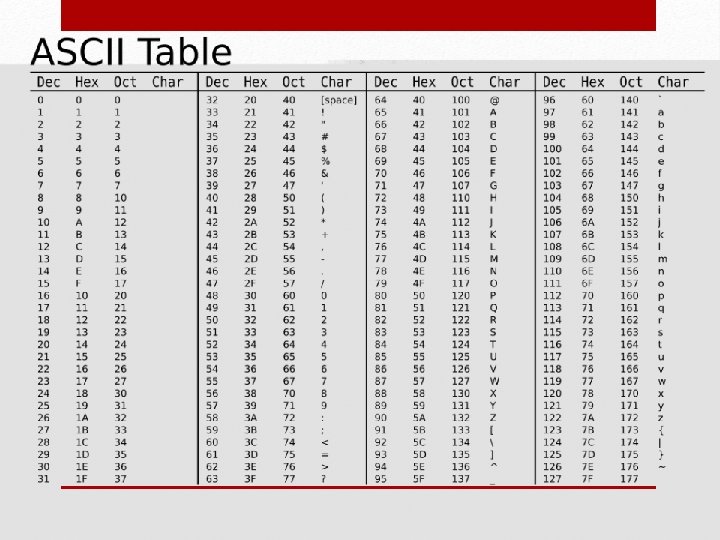
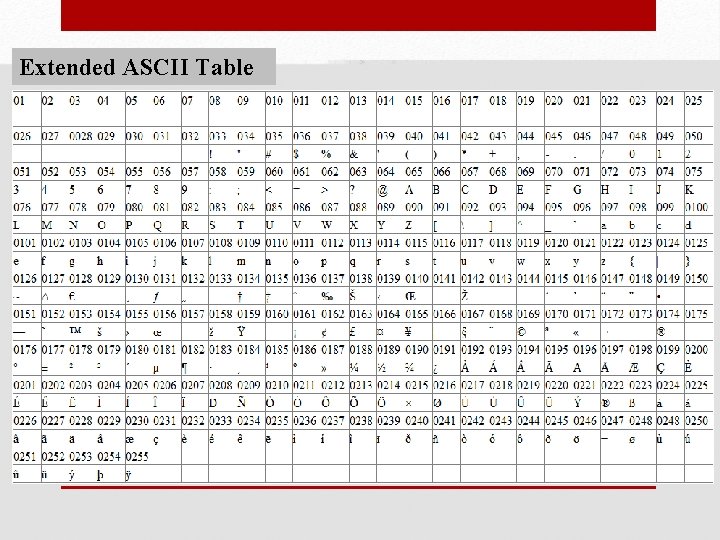
- Slides: 20
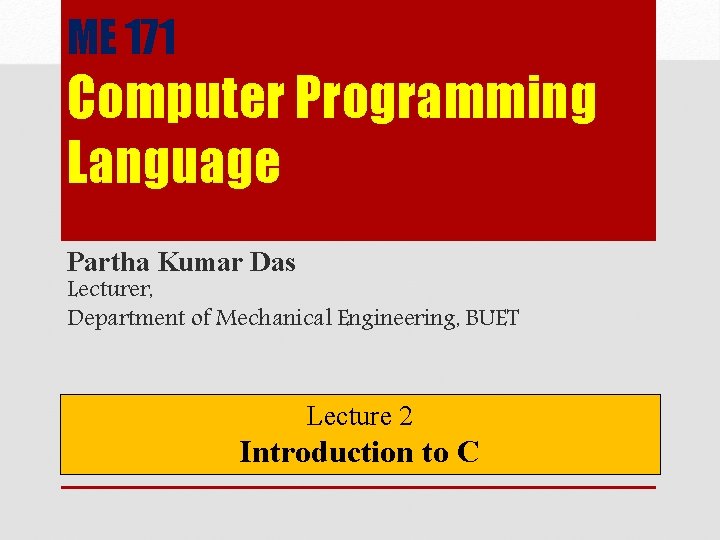
ME 171 Computer Programming Language Partha Kumar Das Lecturer, Department of Mechanical Engineering, BUET Lecture 2 Introduction to C
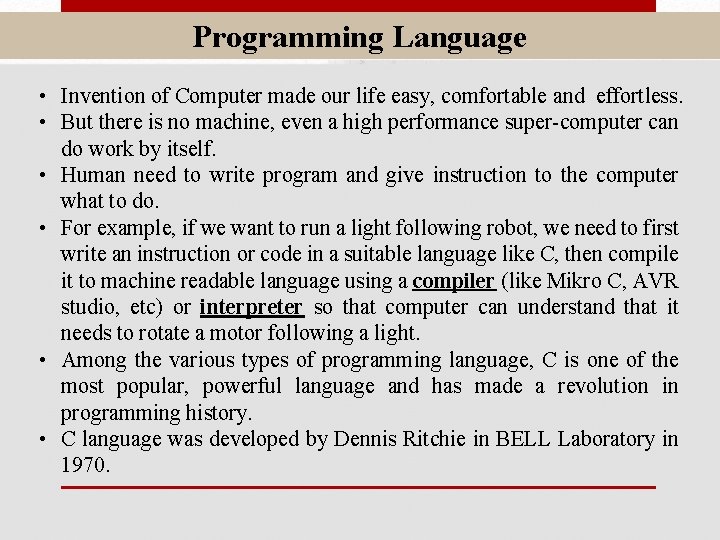
Programming Language • Invention of Computer made our life easy, comfortable and effortless. • But there is no machine, even a high performance super-computer can do work by itself. • Human need to write program and give instruction to the computer what to do. • For example, if we want to run a light following robot, we need to first write an instruction or code in a suitable language like C, then compile it to machine readable language using a compiler (like Mikro C, AVR studio, etc) or interpreter so that computer can understand that it needs to rotate a motor following a light. • Among the various types of programming language, C is one of the most popular, powerful language and has made a revolution in programming history. • C language was developed by Dennis Ritchie in BELL Laboratory in 1970.
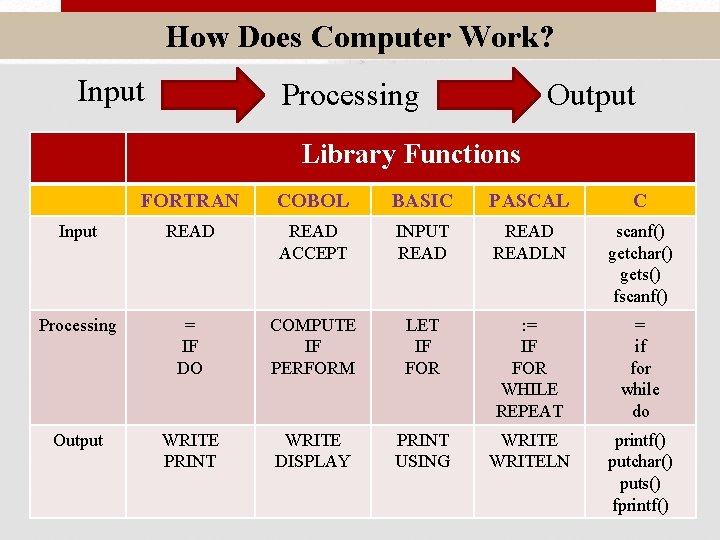
How Does Computer Work? Input Processing Output Library Functions FORTRAN COBOL BASIC PASCAL C Input READ ACCEPT INPUT READLN scanf() getchar() gets() fscanf() Processing = IF DO COMPUTE IF PERFORM LET IF FOR : = IF FOR WHILE REPEAT = if for while do Output WRITE PRINT WRITE DISPLAY PRINT USING WRITELN printf() putchar() puts() fprintf()
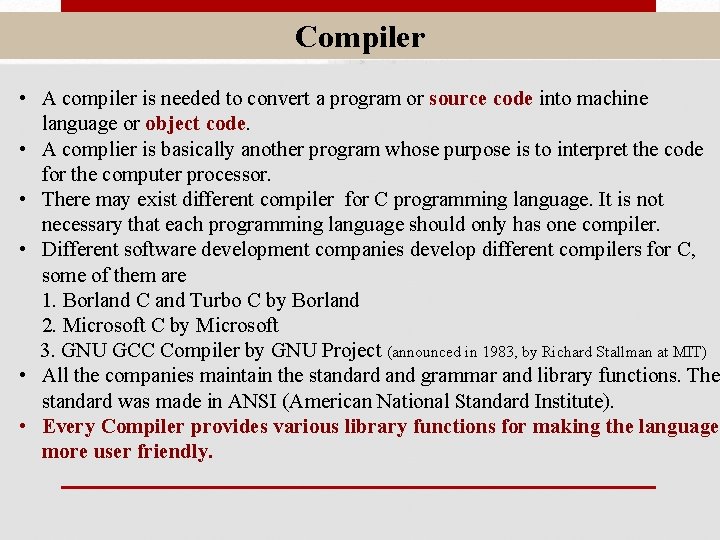
Compiler • A compiler is needed to convert a program or source code into machine language or object code. • A complier is basically another program whose purpose is to interpret the code for the computer processor. • There may exist different compiler for C programming language. It is not necessary that each programming language should only has one compiler. • Different software development companies develop different compilers for C, some of them are 1. Borland C and Turbo C by Borland 2. Microsoft C by Microsoft 3. GNU GCC Compiler by GNU Project (announced in 1983, by Richard Stallman at MIT) • All the companies maintain the standard and grammar and library functions. The standard was made in ANSI (American National Standard Institute). • Every Compiler provides various library functions for making the language more user friendly.
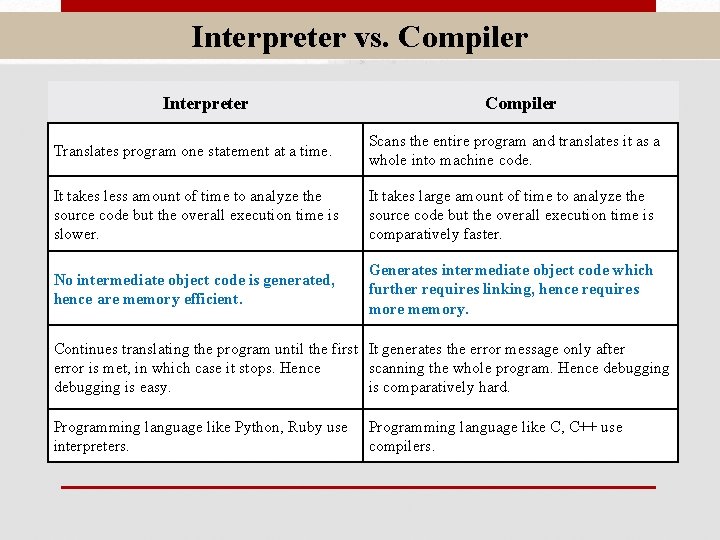
Interpreter vs. Compiler Interpreter Compiler Translates program one statement at a time. Scans the entire program and translates it as a whole into machine code. It takes less amount of time to analyze the source code but the overall execution time is slower. It takes large amount of time to analyze the source code but the overall execution time is comparatively faster. No intermediate object code is generated, hence are memory efficient. Generates intermediate object code which further requires linking, hence requires more memory. Continues translating the program until the first It generates the error message only after error is met, in which case it stops. Hence scanning the whole program. Hence debugging is easy. is comparatively hard. Programming language like Python, Ruby use interpreters. Programming language like C, C++ use compilers.
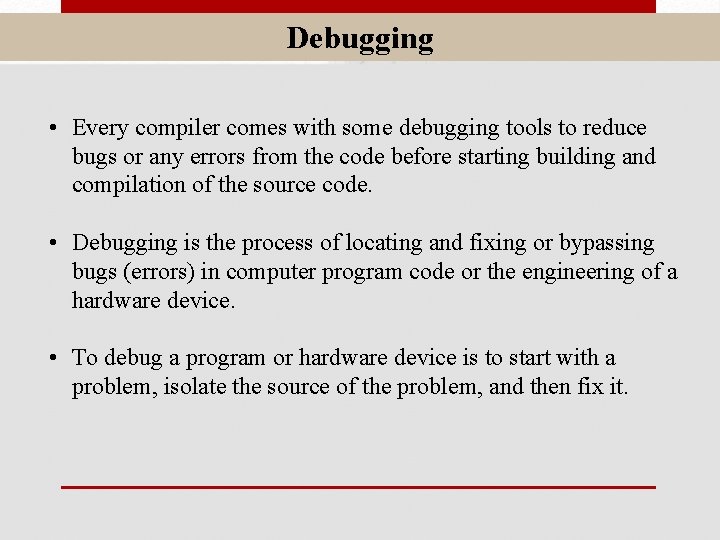
Debugging • Every compiler comes with some debugging tools to reduce bugs or any errors from the code before starting building and compilation of the source code. • Debugging is the process of locating and fixing or bypassing bugs (errors) in computer program code or the engineering of a hardware device. • To debug a program or hardware device is to start with a problem, isolate the source of the problem, and then fix it.
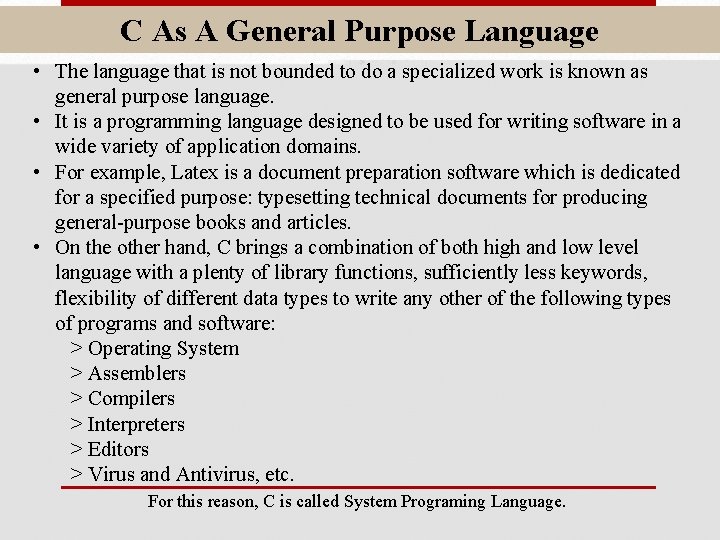
C As A General Purpose Language • The language that is not bounded to do a specialized work is known as general purpose language. • It is a programming language designed to be used for writing software in a wide variety of application domains. • For example, Latex is a document preparation software which is dedicated for a specified purpose: typesetting technical documents for producing general-purpose books and articles. • On the other hand, C brings a combination of both high and low level language with a plenty of library functions, sufficiently less keywords, flexibility of different data types to write any other of the following types of programs and software: > Operating System > Assemblers > Compilers > Interpreters > Editors > Virus and Antivirus, etc. For this reason, C is called System Programing Language.
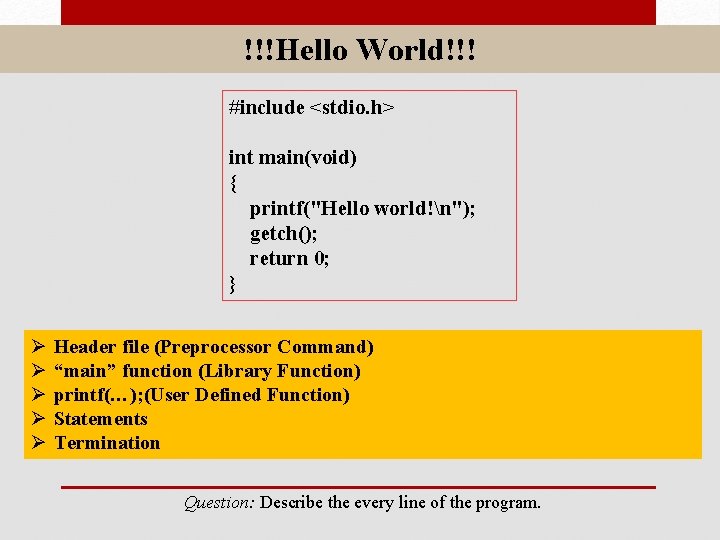
!!!Hello World!!! #include <stdio. h> int main(void) { printf("Hello world!n"); getch(); return 0; } Ø Ø Ø Header file (Preprocessor Command) “main” function (Library Function) printf(…); (User Defined Function) Statements Termination Question: Describe the every line of the program.
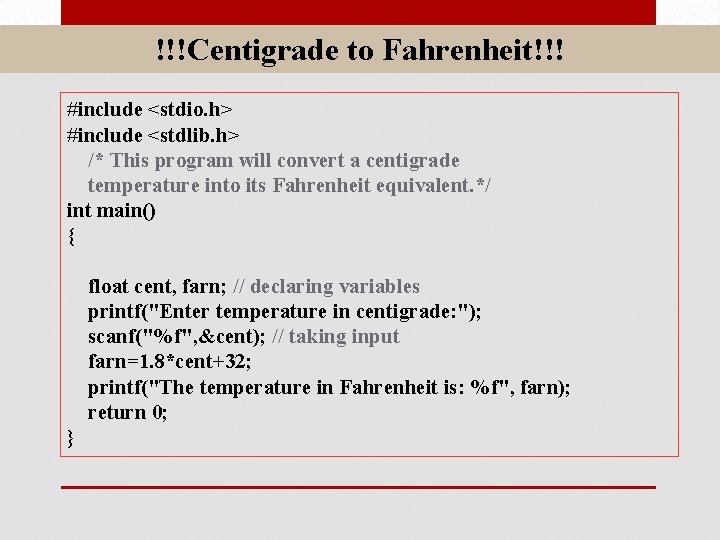
!!!Centigrade to Fahrenheit!!! #include <stdio. h> #include <stdlib. h> /* This program will convert a centigrade temperature into its Fahrenheit equivalent. */ int main() { float cent, farn; // declaring variables printf("Enter temperature in centigrade: "); scanf("%f", ¢); // taking input farn=1. 8*cent+32; printf("The temperature in Fahrenheit is: %f", farn); return 0; }
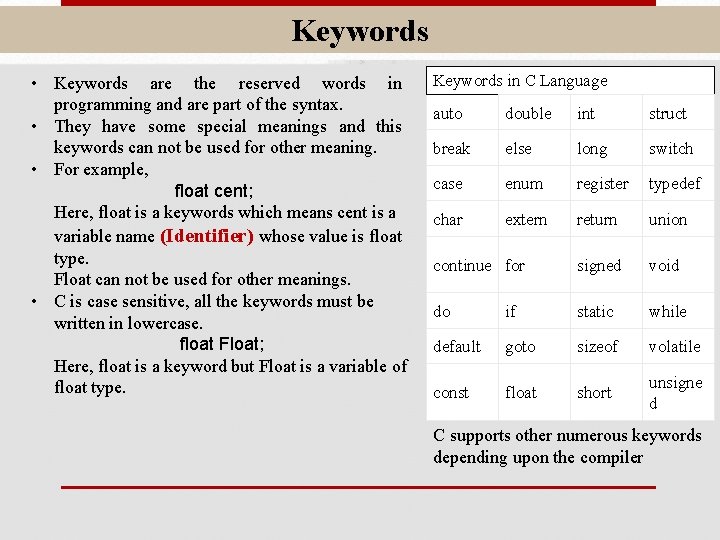
Keywords • Keywords are the reserved words in programming and are part of the syntax. • They have some special meanings and this keywords can not be used for other meaning. • For example, float cent; Here, float is a keywords which means cent is a variable name (Identifier) whose value is float type. Float can not be used for other meanings. • C is case sensitive, all the keywords must be written in lowercase. float Float; Here, float is a keyword but Float is a variable of float type. Keywords in C Language auto double int struct break else long switch case enum register typedef char extern return union continue for signed void do if static while default goto sizeof volatile const float short unsigne d C supports other numerous keywords depending upon the compiler
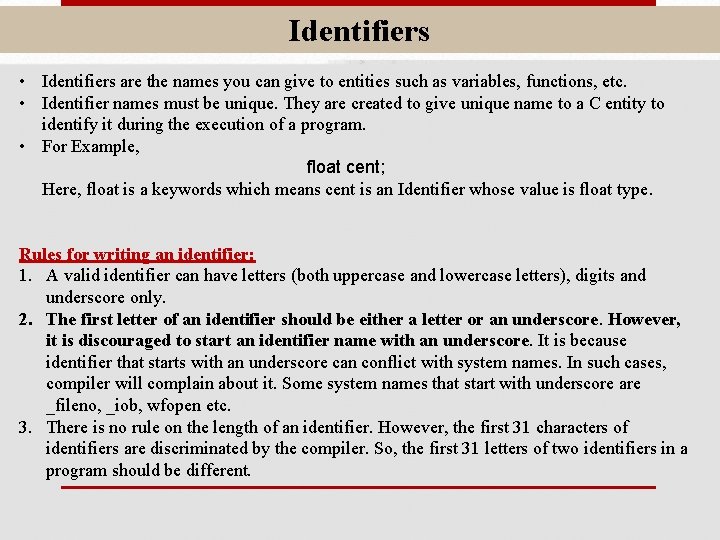
Identifiers • Identifiers are the names you can give to entities such as variables, functions, etc. • Identifier names must be unique. They are created to give unique name to a C entity to identify it during the execution of a program. • For Example, float cent; Here, float is a keywords which means cent is an Identifier whose value is float type. Rules for writing an identifier: 1. A valid identifier can have letters (both uppercase and lowercase letters), digits and underscore only. 2. The first letter of an identifier should be either a letter or an underscore. However, it is discouraged to start an identifier name with an underscore. It is because identifier that starts with an underscore can conflict with system names. In such cases, compiler will complain about it. Some system names that start with underscore are _fileno, _iob, wfopen etc. 3. There is no rule on the length of an identifier. However, the first 31 characters of identifiers are discriminated by the compiler. So, the first 31 letters of two identifiers in a program should be different.
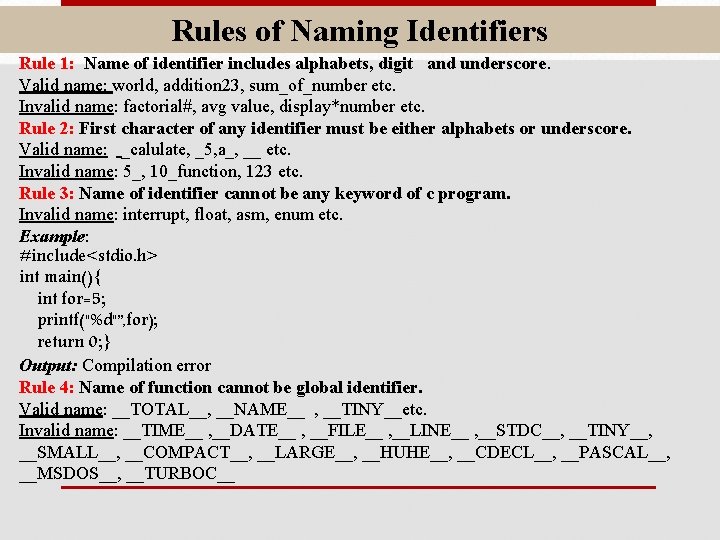
Rules of Naming Identifiers Rule 1: Name of identifier includes alphabets, digit and underscore. Valid name: world, addition 23, sum_of_number etc. Invalid name: factorial#, avg value, display*number etc. Rule 2: First character of any identifier must be either alphabets or underscore. Valid name: _calulate, _5, a_, __ etc. Invalid name: 5_, 10_function, 123 etc. Rule 3: Name of identifier cannot be any keyword of c program. Invalid name: interrupt, float, asm, enum etc. Example: #include<stdio. h> int main(){ int for=5; printf("%d"”, for); return 0; } Output: Compilation error Rule 4: Name of function cannot be global identifier. Valid name: __TOTAL__, __NAME__ , __TINY__etc. Invalid name: __TIME__ , __DATE__ , __FILE__ , __LINE__ , __STDC__, __TINY__, __SMALL__, __COMPACT__, __LARGE__, __HUHE__, __CDECL__, __PASCAL__, __MSDOS__, __TURBOC__
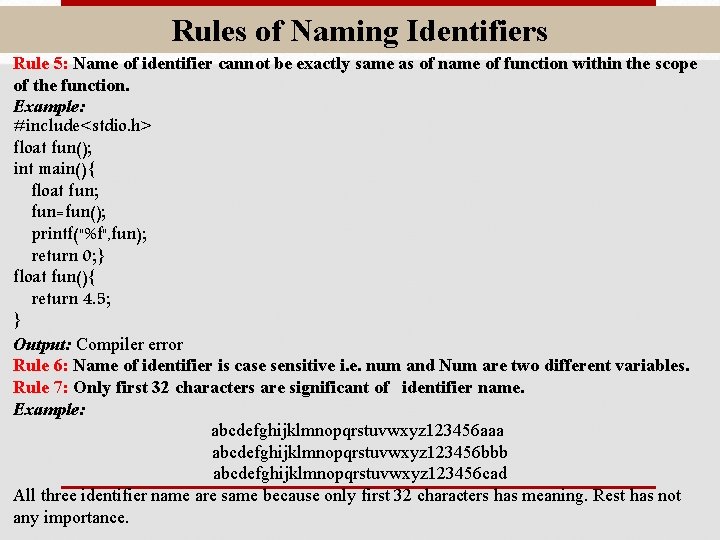
Rules of Naming Identifiers Rule 5: Name of identifier cannot be exactly same as of name of function within the scope of the function. Example: #include<stdio. h> float fun(); int main(){ float fun; fun=fun(); printf("%f", fun); return 0; } float fun(){ return 4. 5; } Output: Compiler error Rule 6: Name of identifier is case sensitive i. e. num and Num are two different variables. Rule 7: Only first 32 characters are significant of identifier name. Example: abcdefghijklmnopqrstuvwxyz 123456 aaa abcdefghijklmnopqrstuvwxyz 123456 bbb abcdefghijklmnopqrstuvwxyz 123456 cad All three identifier name are same because only first 32 characters has meaning. Rest has not any importance.
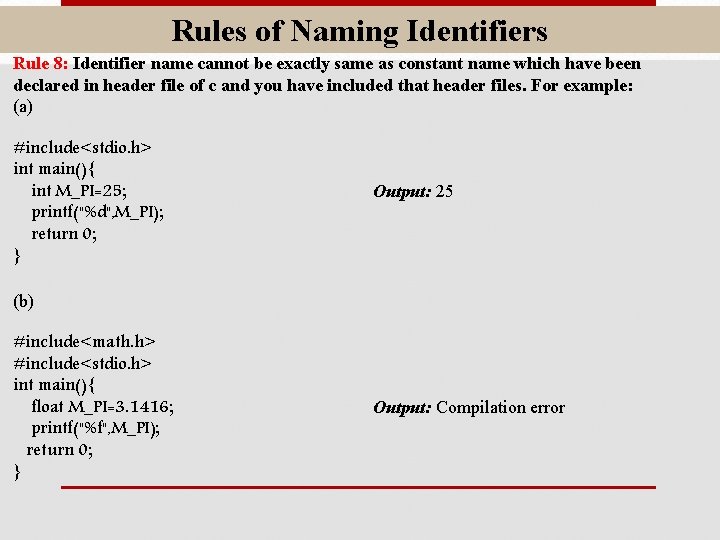
Rules of Naming Identifiers Rule 8: Identifier name cannot be exactly same as constant name which have been declared in header file of c and you have included that header files. For example: (a) #include<stdio. h> int main(){ int M_PI=25; printf("%d", M_PI); return 0; } Output: 25 (b) #include<math. h> #include<stdio. h> int main(){ float M_PI=3. 1416; printf("%f", M_PI); return 0; } Output: Compilation error
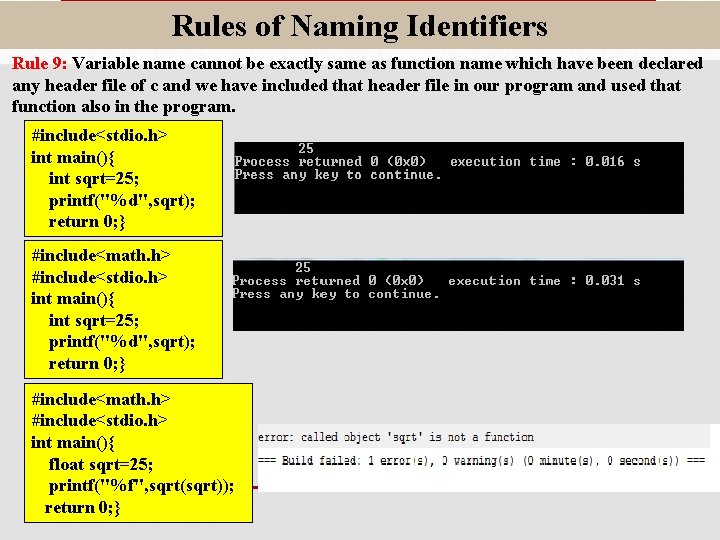
Rules of Naming Identifiers Rule 9: Variable name cannot be exactly same as function name which have been declared any header file of c and we have included that header file in our program and used that function also in the program. #include<stdio. h> int main(){ int sqrt=25; printf("%d", sqrt); return 0; } #include<math. h> #include<stdio. h> int main(){ float sqrt=25; printf("%f", sqrt(sqrt)); return 0; }
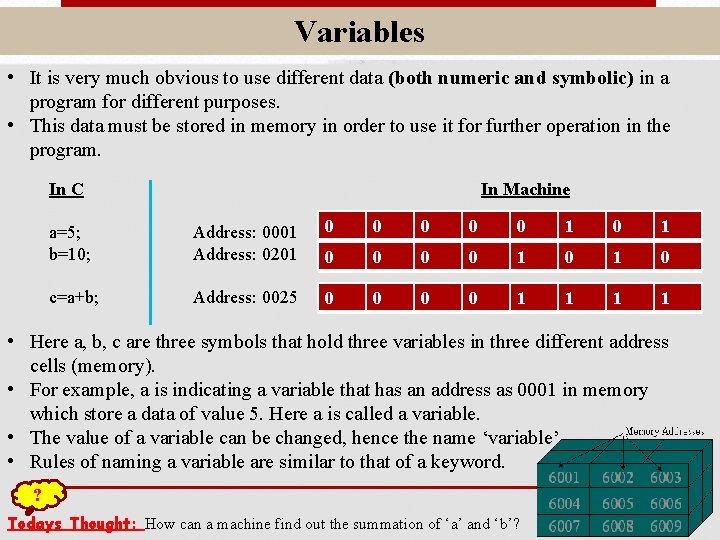
Variables • It is very much obvious to use different data (both numeric and symbolic) in a program for different purposes. • This data must be stored in memory in order to use it for further operation in the program. In C In Machine a=5; b=10; Address: 0001 Address: 0201 0 0 0 1 0 c=a+b; Address: 0025 0 0 1 1 • Here a, b, c are three symbols that hold three variables in three different address cells (memory). • For example, a is indicating a variable that has an address as 0001 in memory which store a data of value 5. Here a is called a variable. • The value of a variable can be changed, hence the name ‘variable’. • Rules of naming a variable are similar to that of a keyword. ? Todays Thought: How can a machine find out the summation of ‘a’ and ‘b’?
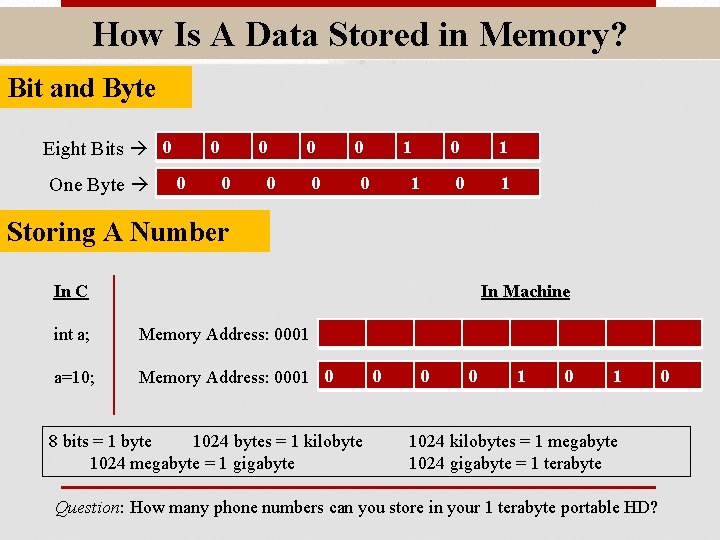
How Is A Data Stored in Memory? Bit and Byte Eight Bits 0 0 0 1 One Byte 0 0 0 1 0 1 Storing A Number In C In Machine int a; Memory Address: 0001 a=10; Memory Address: 0001 0 0 1 0 1 8 bits = 1 byte 1024 bytes = 1 kilobyte 1024 kilobytes = 1 megabyte 1024 megabyte = 1 gigabyte 1024 gigabyte = 1 terabyte Question: How many phone numbers can you store in your 1 terabyte portable HD? 0
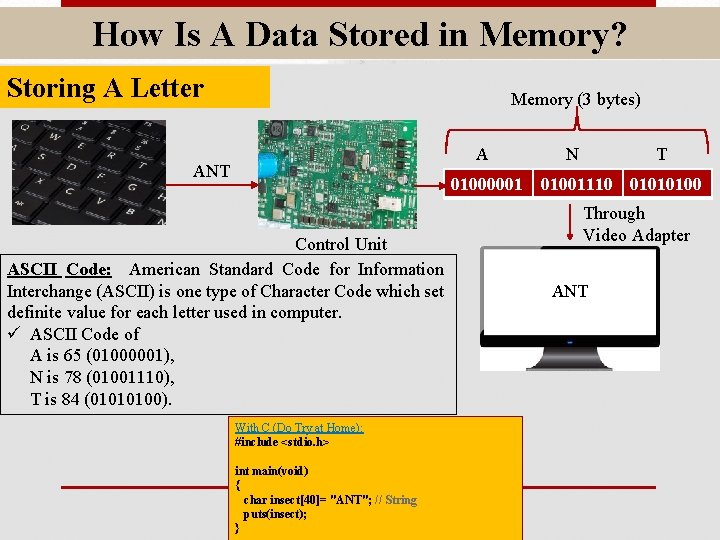
How Is A Data Stored in Memory? Storing A Letter Memory (3 bytes) A ANT N T 01000001 01001110 01010100 Control Unit ASCII Code: American Standard Code for Information Interchange (ASCII) is one type of Character Code which set definite value for each letter used in computer. ü ASCII Code of A is 65 (01000001), N is 78 (01001110), T is 84 (01010100). With C (Do Try at Home): #include <stdio. h> int main(void) { char insect[40]= "ANT"; // String puts(insect); } Through Video Adapter ANT
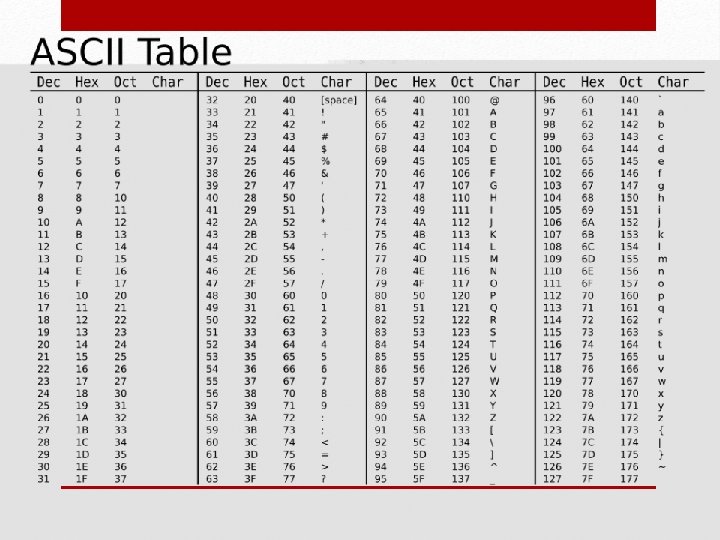
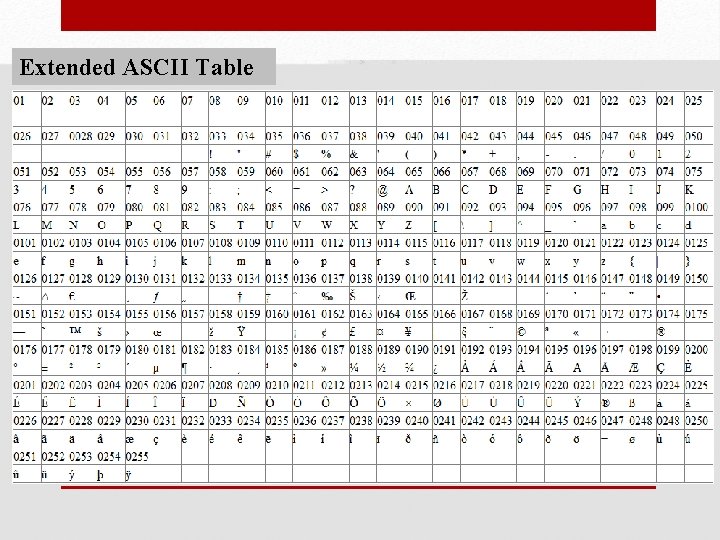
Extended ASCII Table