main cpp include iostream include string using namespace
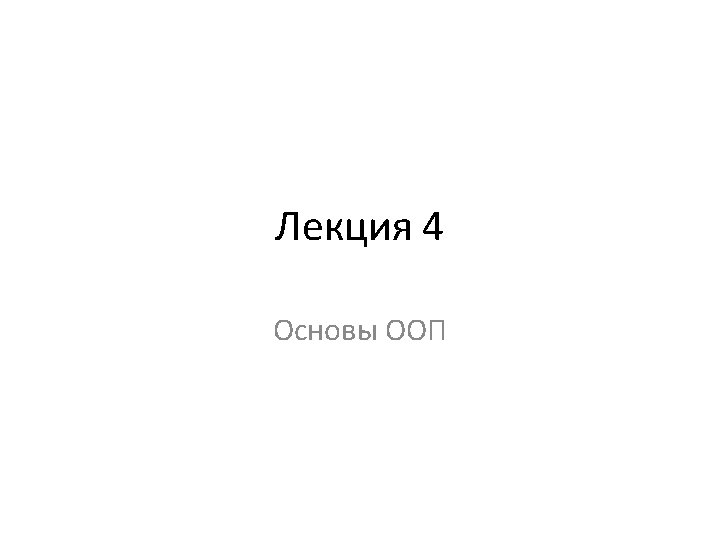
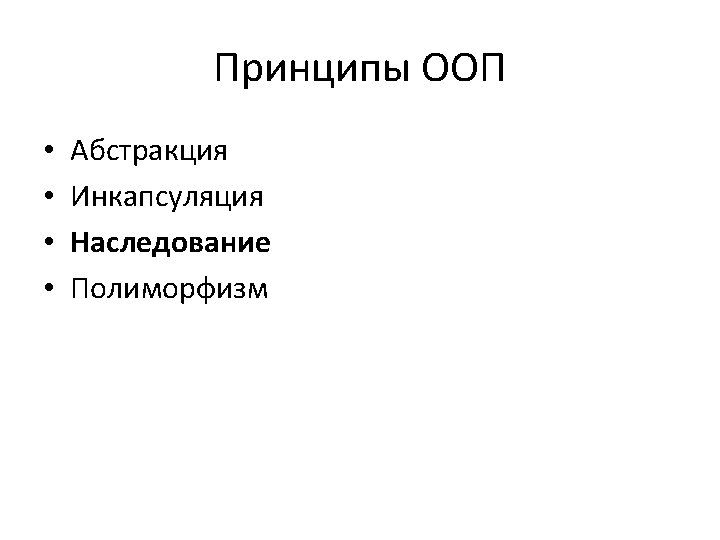
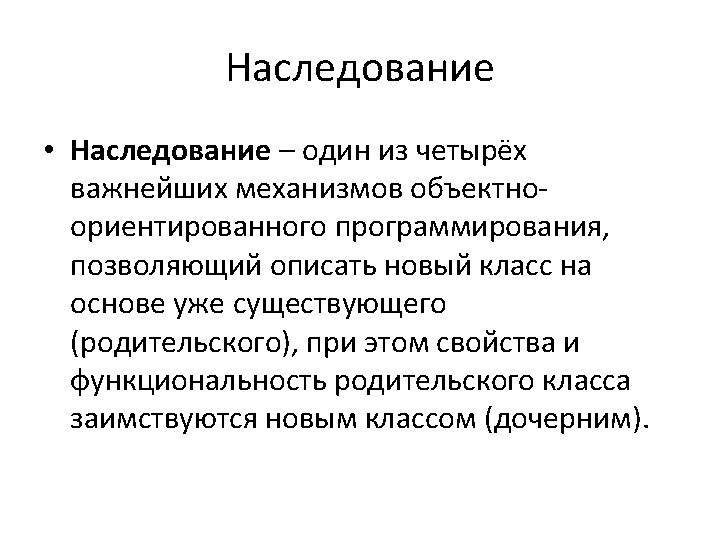
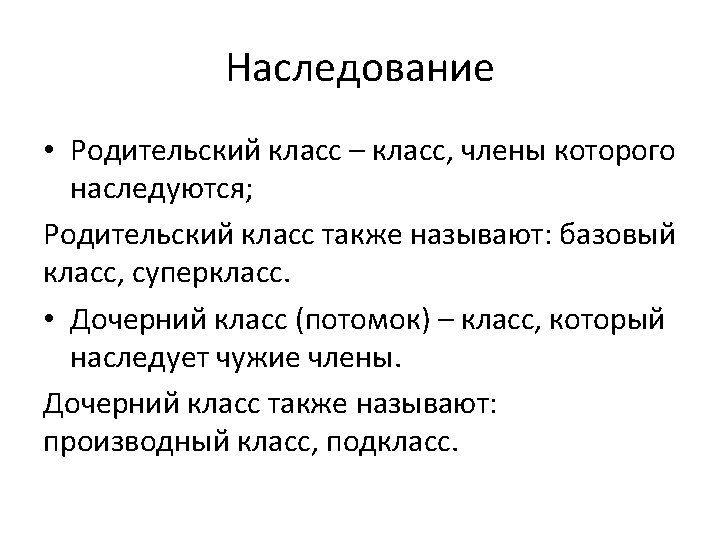
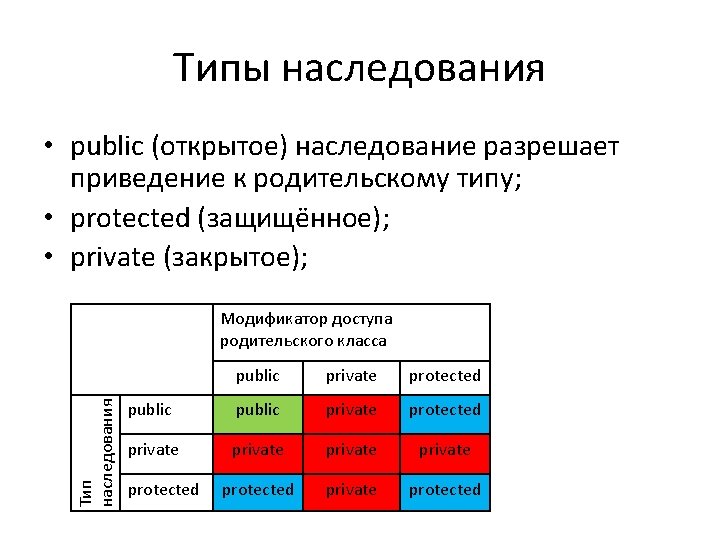
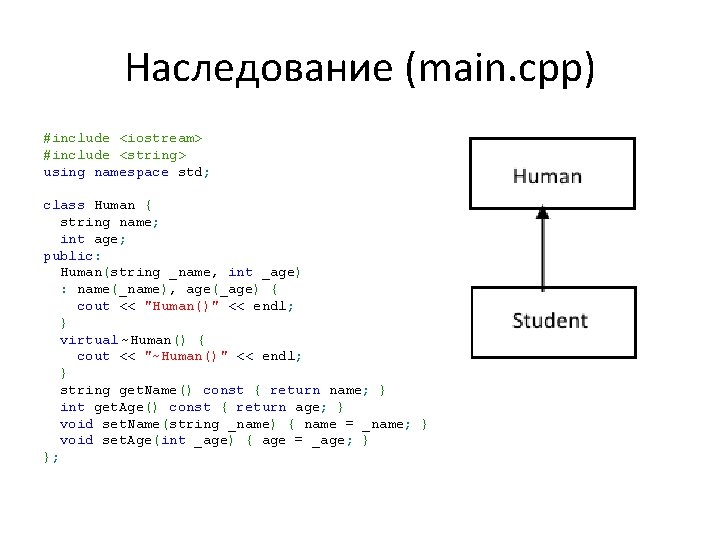
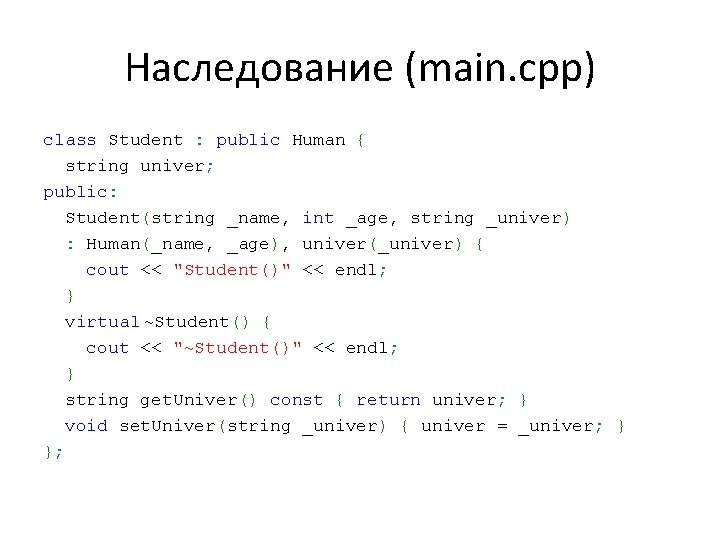
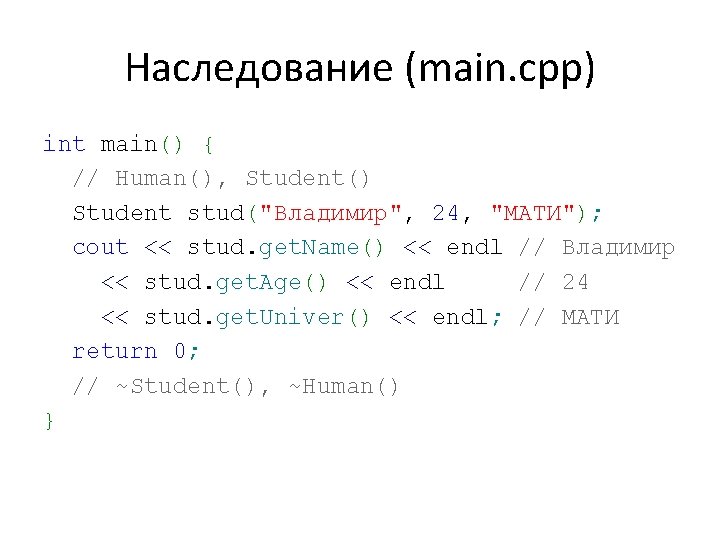
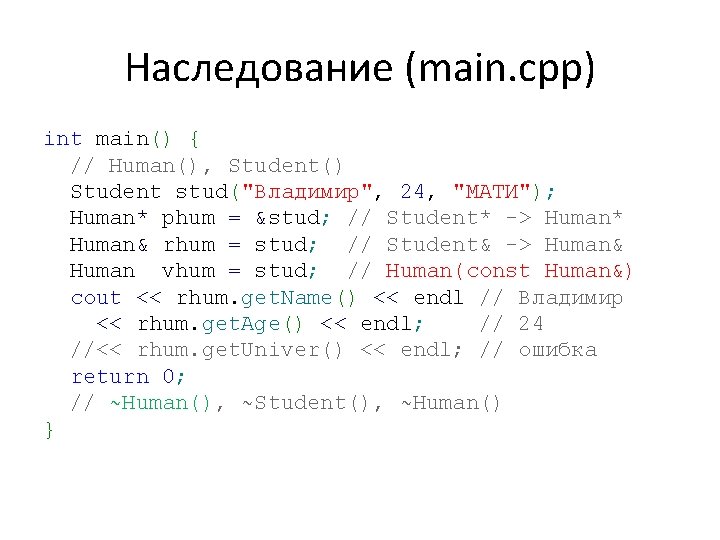
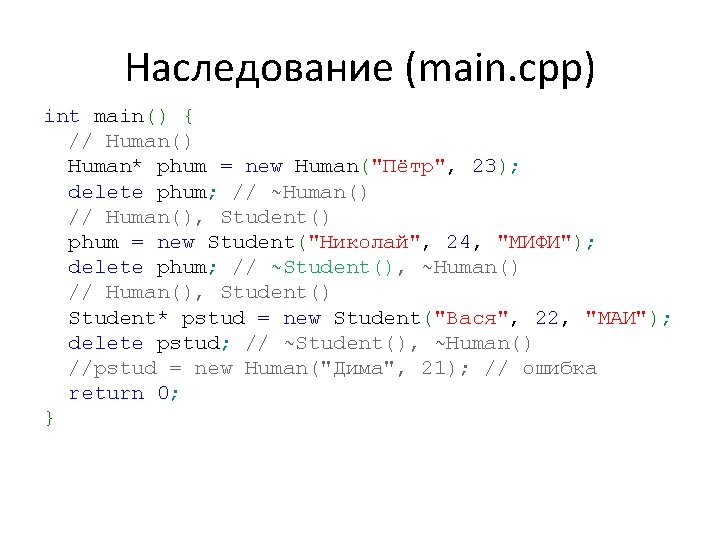
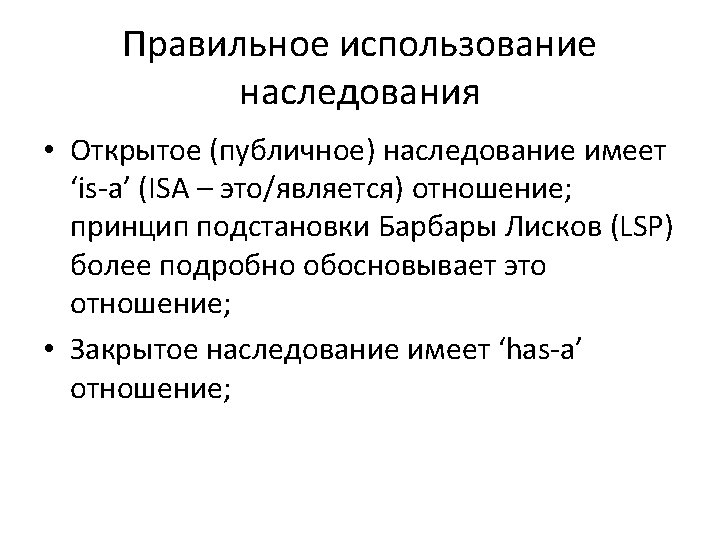
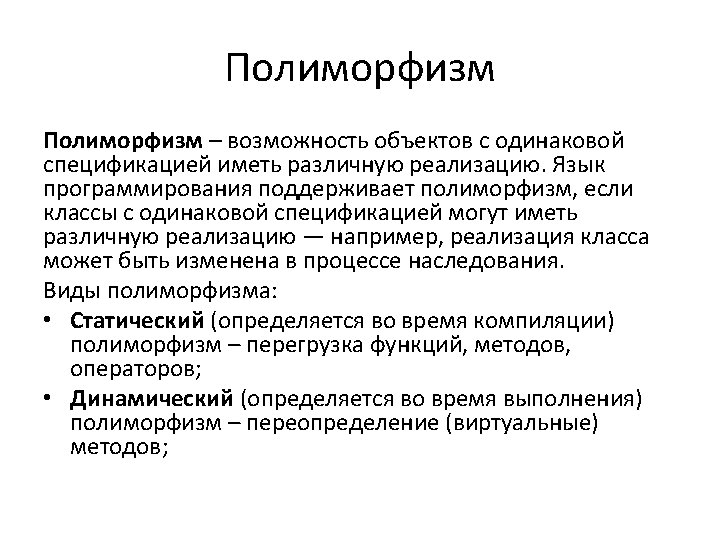
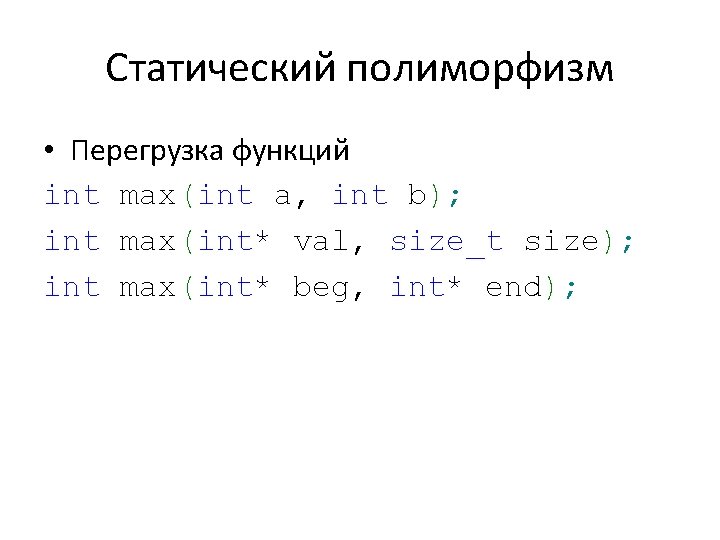
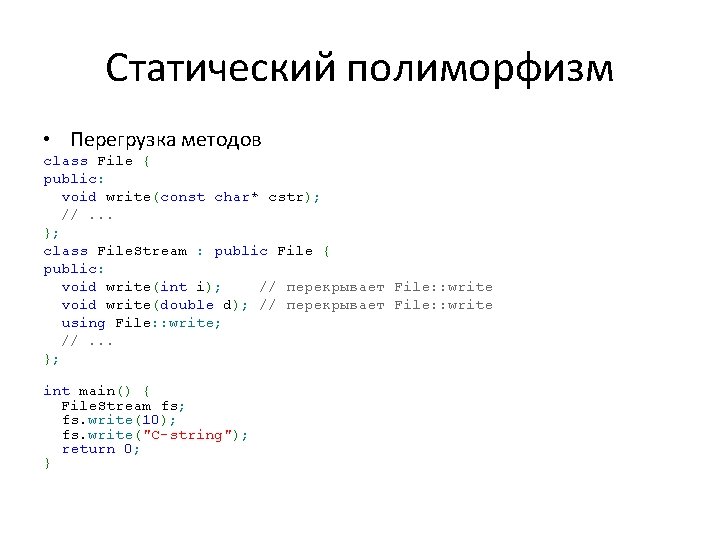
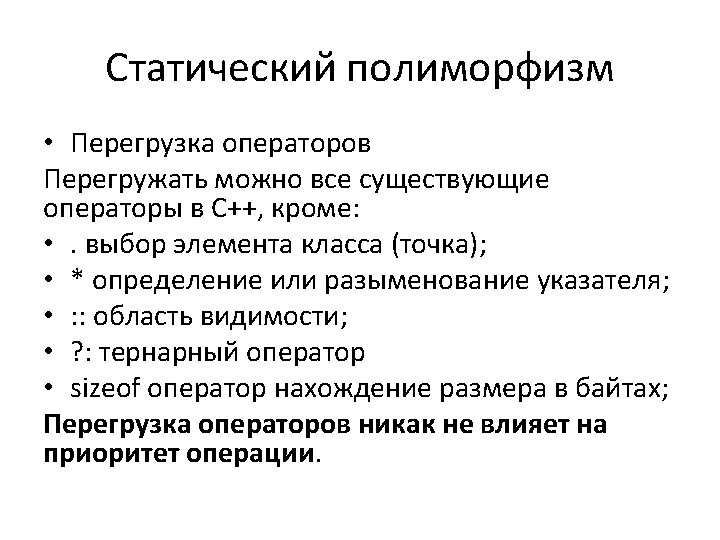
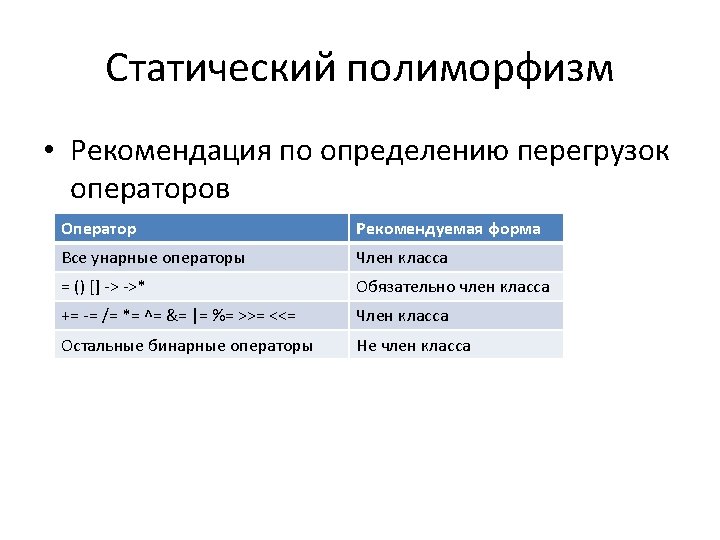
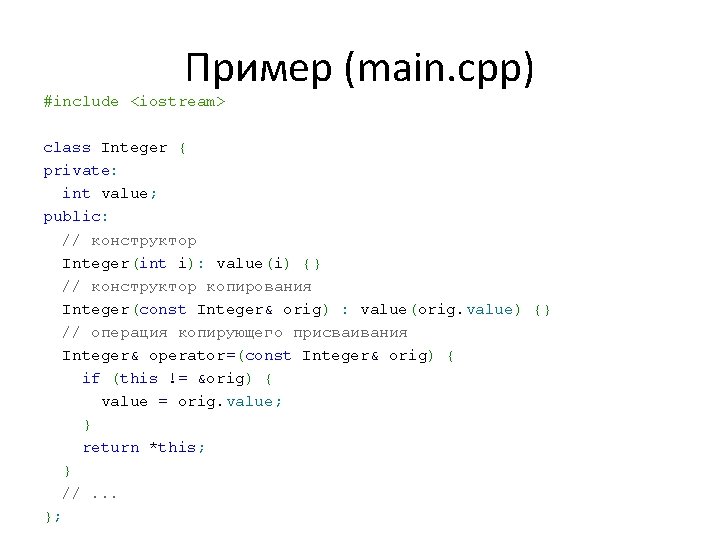
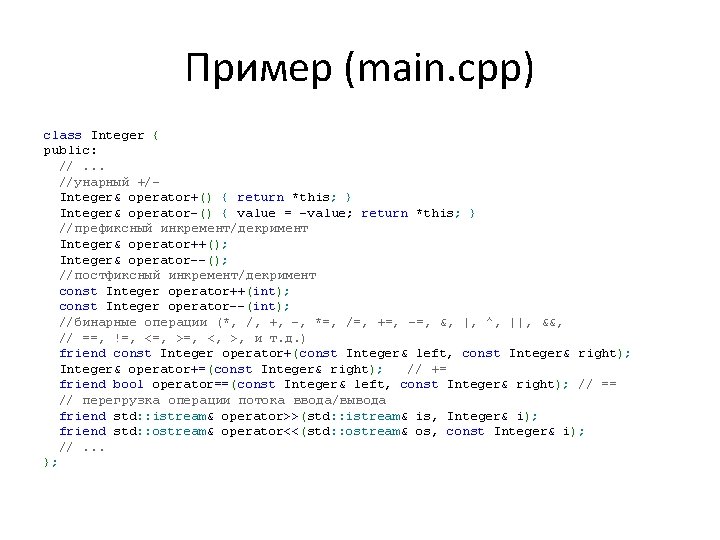
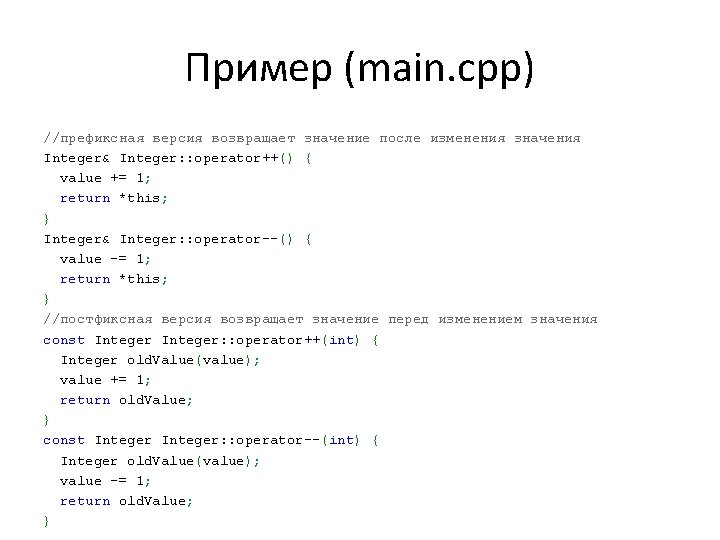
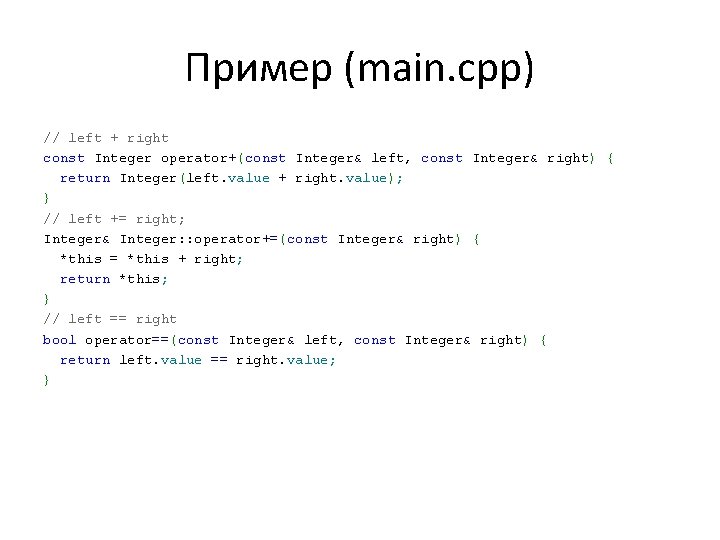
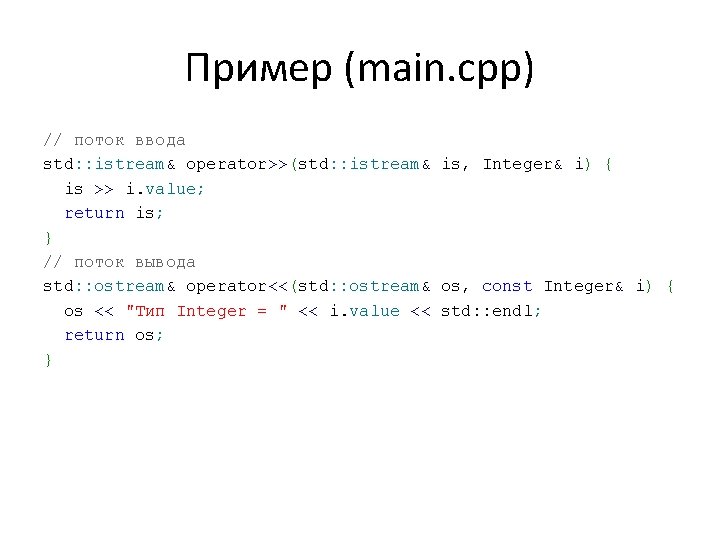
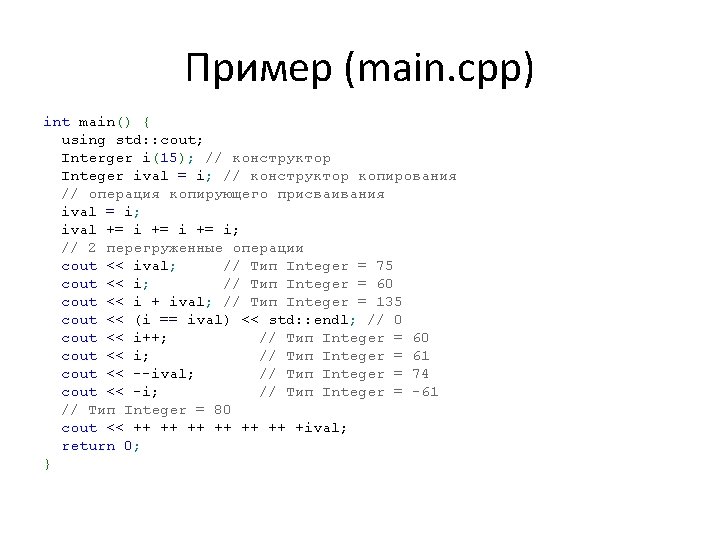
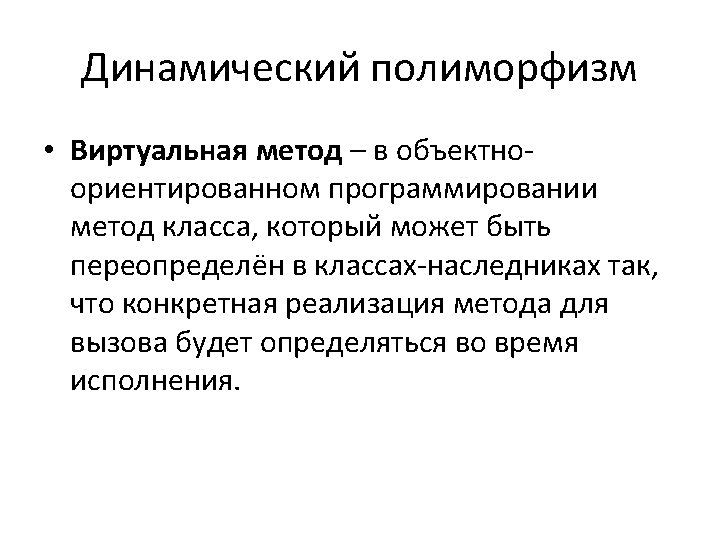
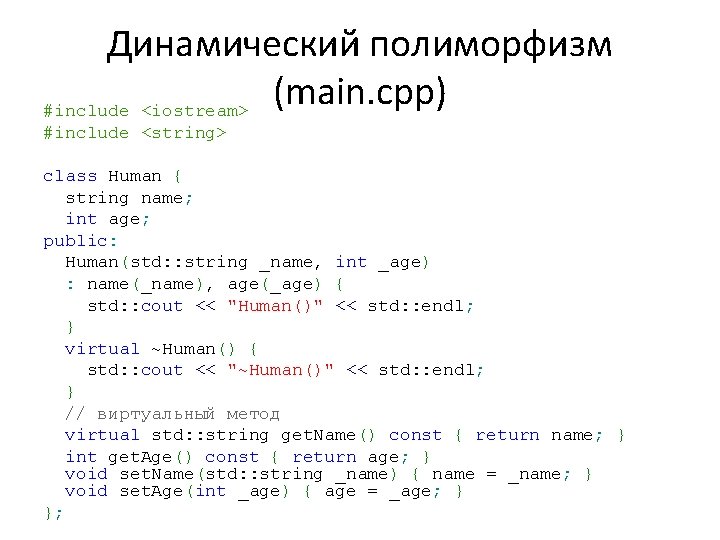
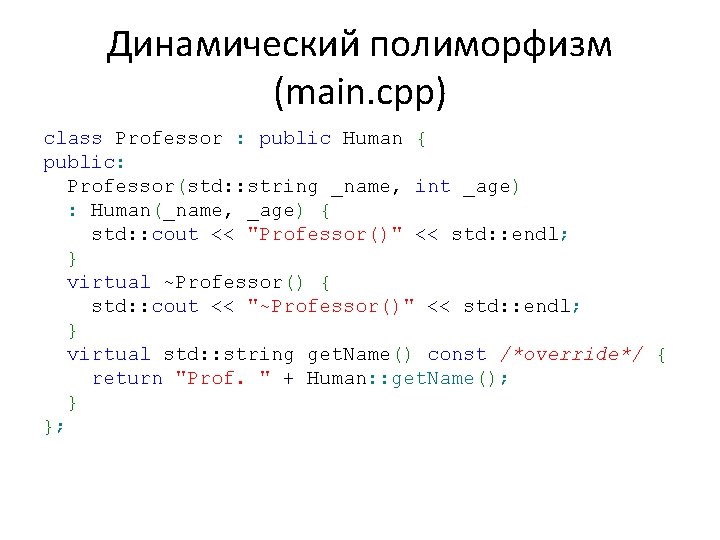
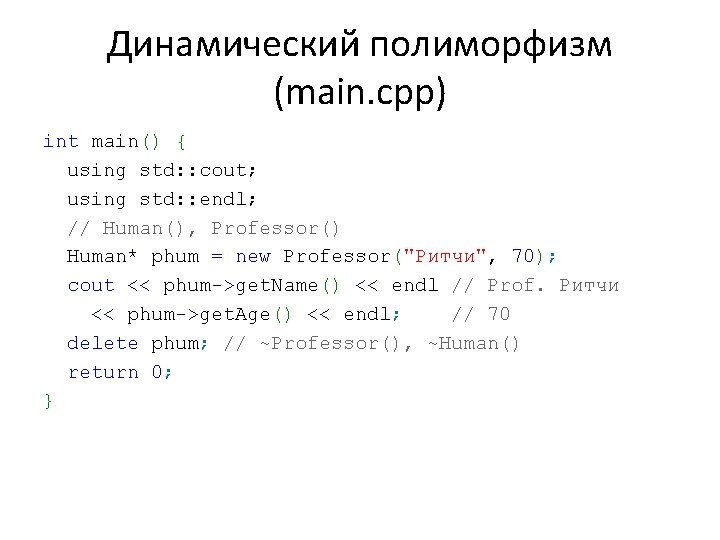
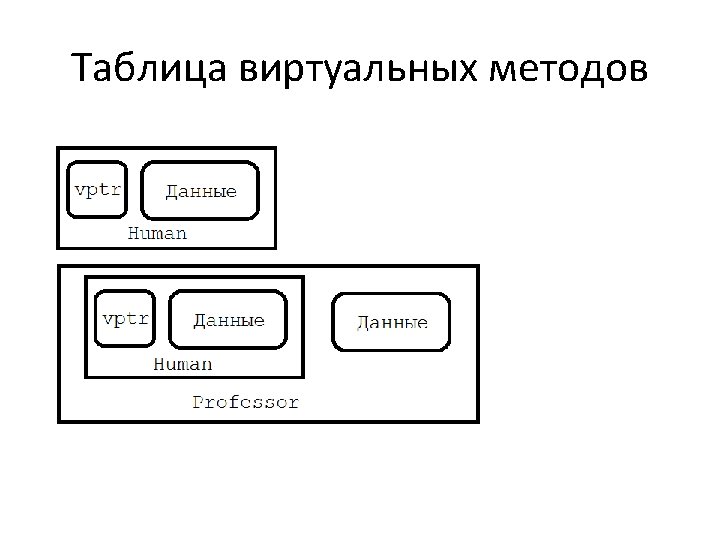
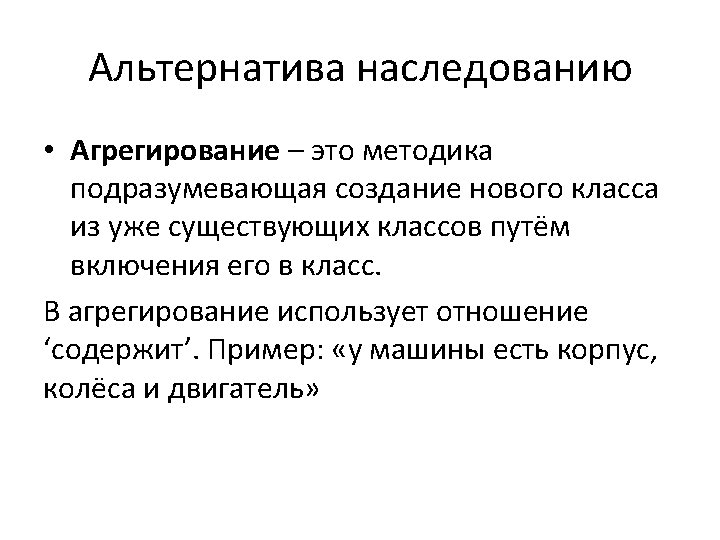
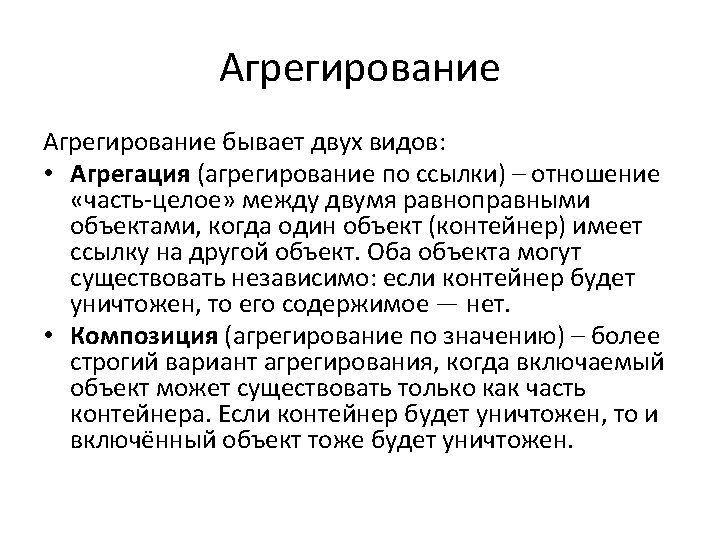
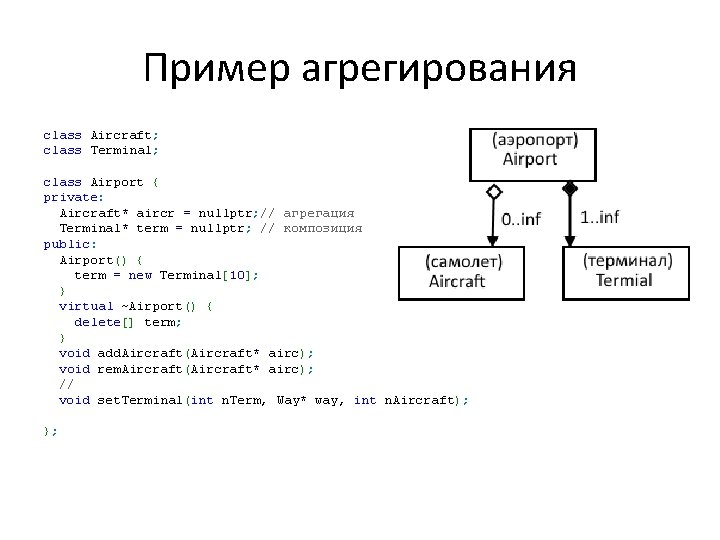
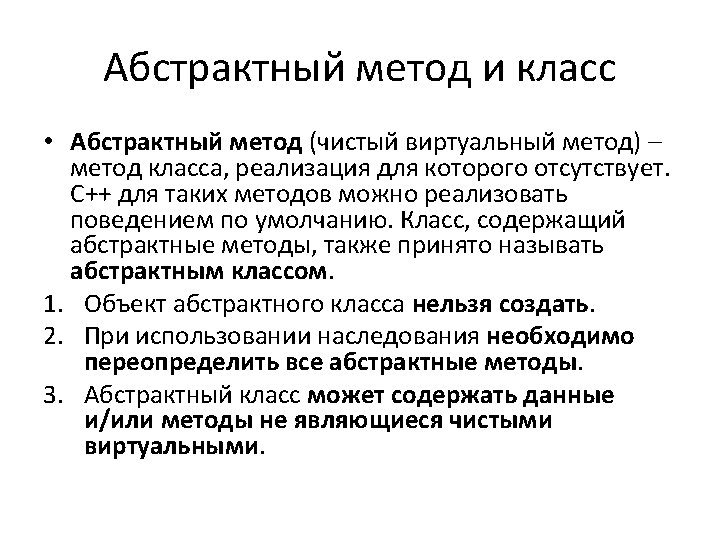
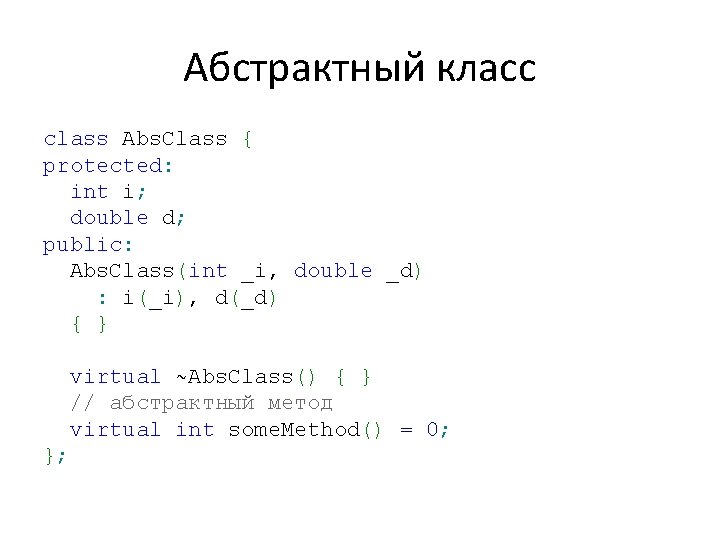
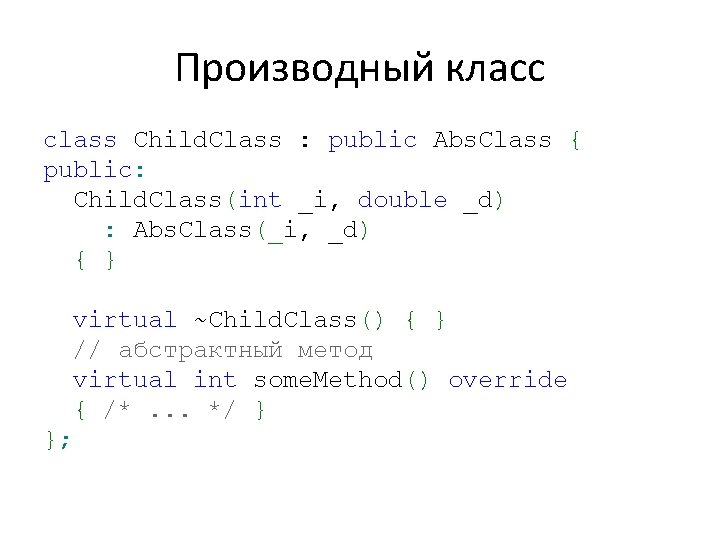
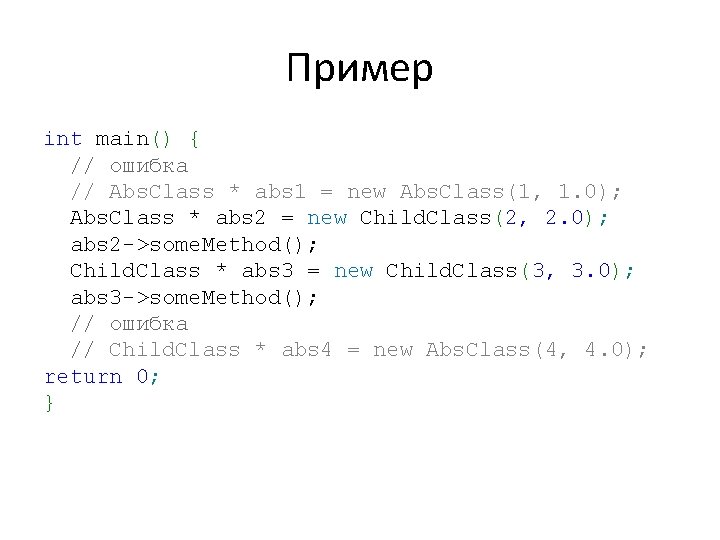
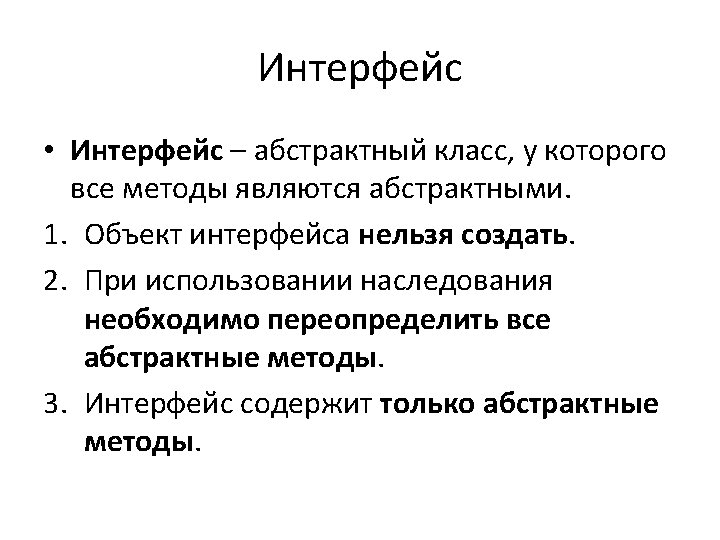
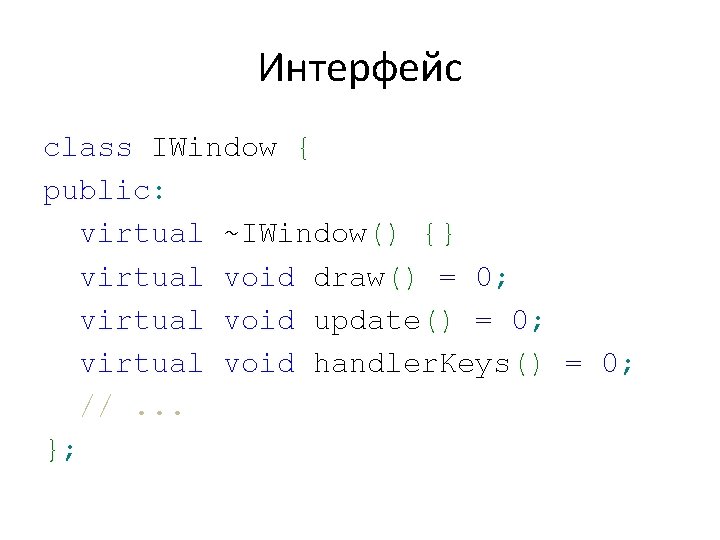
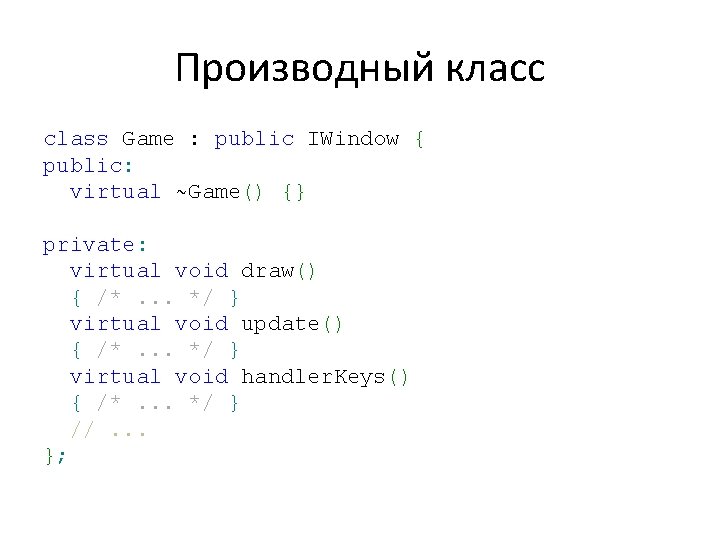
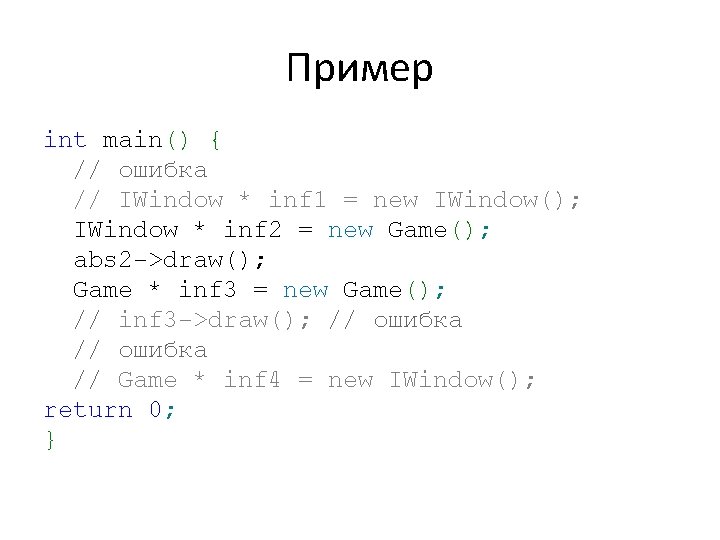
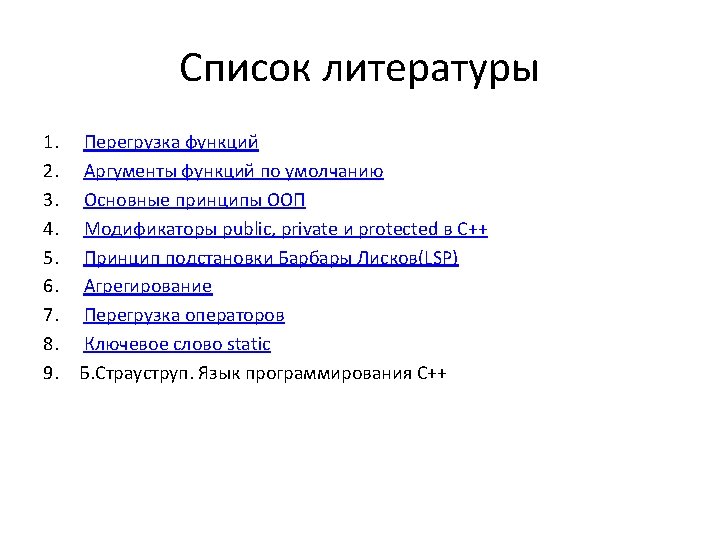
- Slides: 39
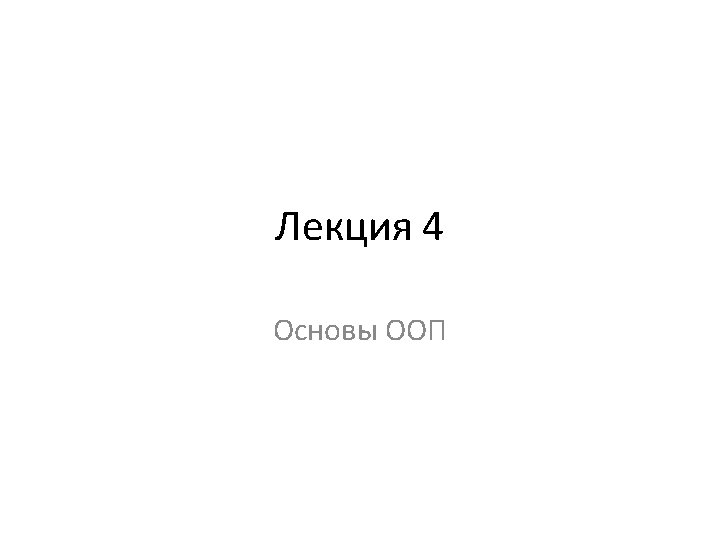
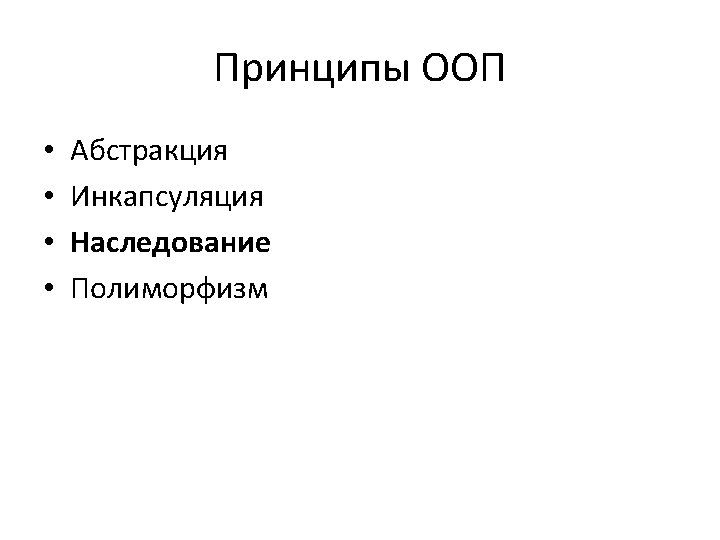
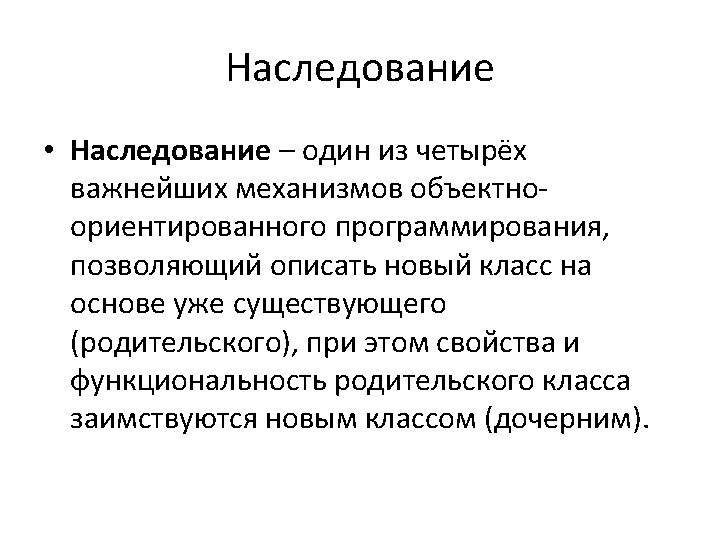
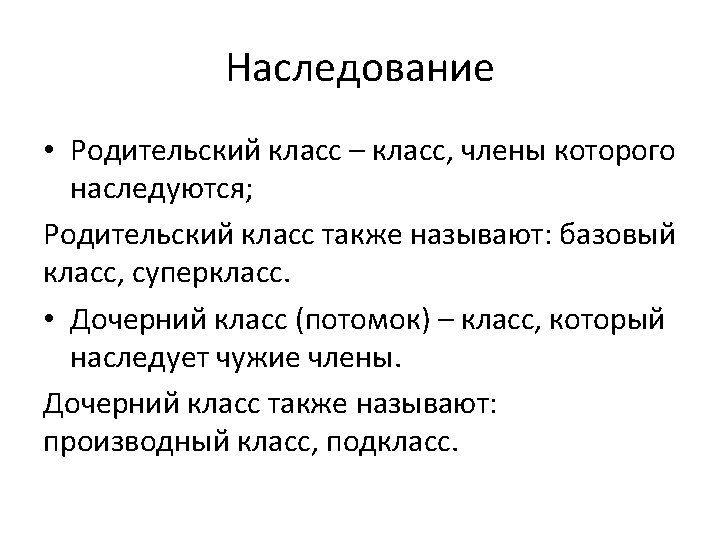
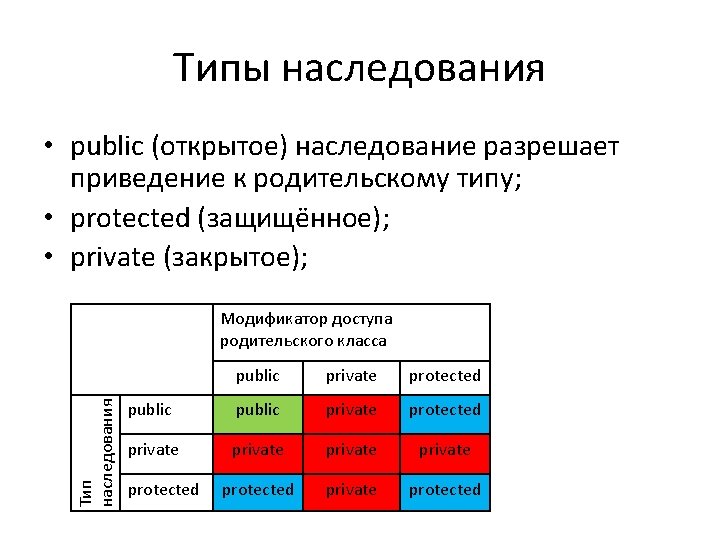
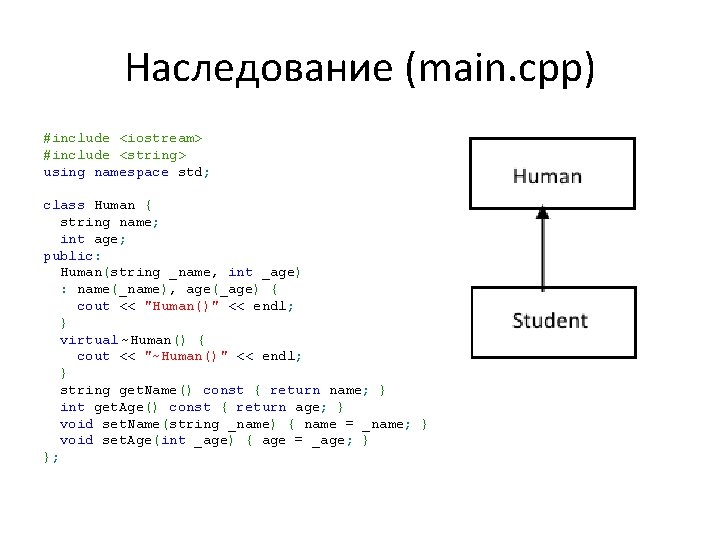
Наследование (main. cpp) #include <iostream> #include <string> using namespace std; class Human { string name; int age; public: Human(string _name, int _age) : name(_name), age(_age) { cout << "Human()" << endl; } virtual ~Human() { cout << "~Human()" << endl; } string get. Name() const { return name; } int get. Age() const { return age; } void set. Name(string _name) { name = _name; } void set. Age(int _age) { age = _age; } };
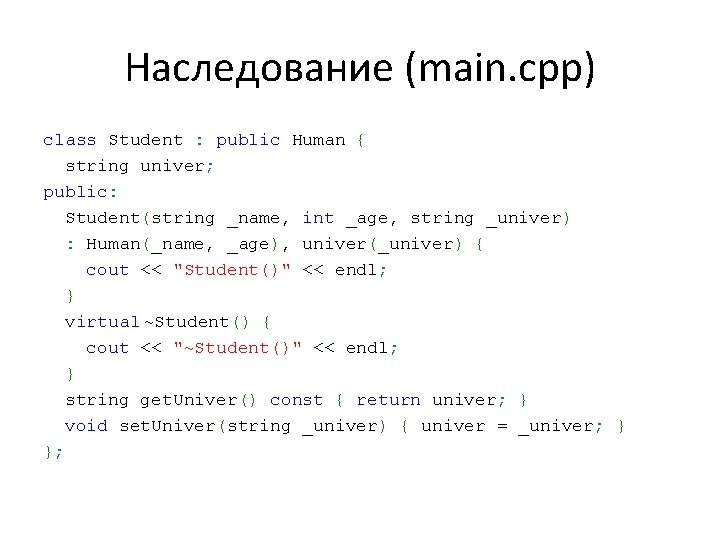
Наследование (main. cpp) class Student : public Human { string univer; public: Student(string _name, int _age, string _univer) : Human(_name, _age), univer(_univer) { cout << "Student()" << endl; } virtual ~Student() { cout << "~Student()" << endl; } string get. Univer() const { return univer; } void set. Univer(string _univer) { univer = _univer; } };
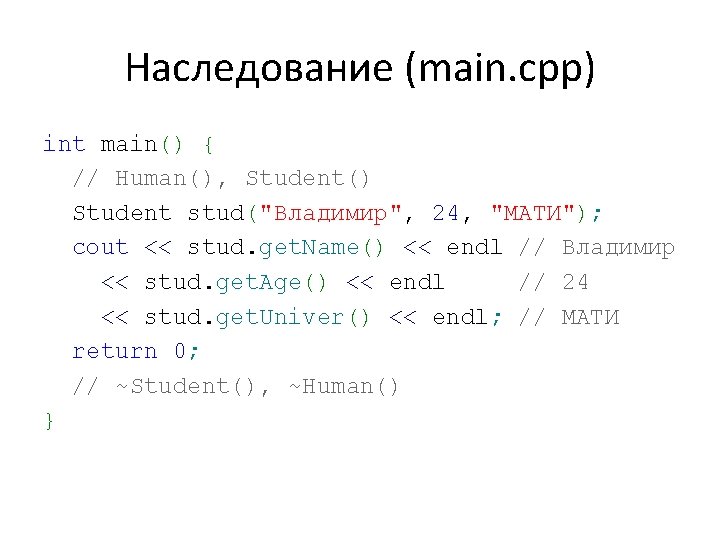
Наследование (main. cpp) int main() { // Human(), Student() Student stud("Владимир", 24, "МАТИ"); cout << stud. get. Name() << endl // Владимир << stud. get. Age() << endl // 24 << stud. get. Univer() << endl; // МАТИ return 0; // ~Student(), ~Human() }
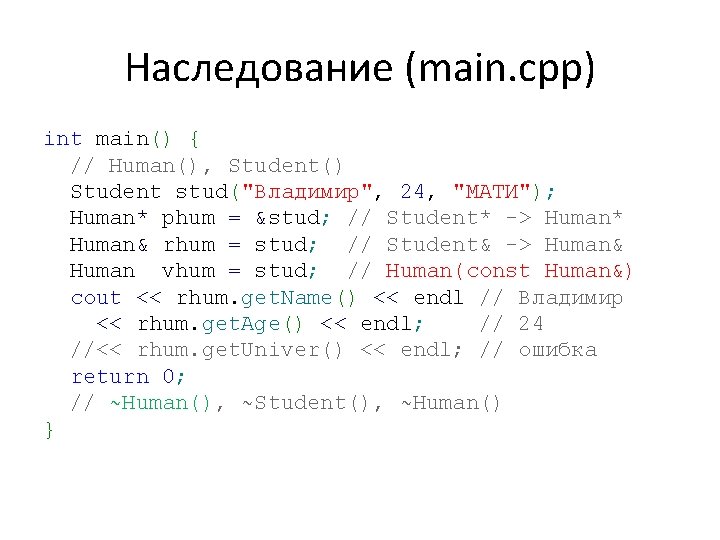
Наследование (main. cpp) int main() { // Human(), Student() Student stud("Владимир", 24, "МАТИ"); Human* phum = &stud; // Student* -> Human* Human& rhum = stud; // Student& -> Human& Human vhum = stud; // Human(const Human&) cout << rhum. get. Name() << endl // Владимир << rhum. get. Age() << endl; // 24 //<< rhum. get. Univer() << endl; // ошибка return 0; // ~Human(), ~Student(), ~Human() }
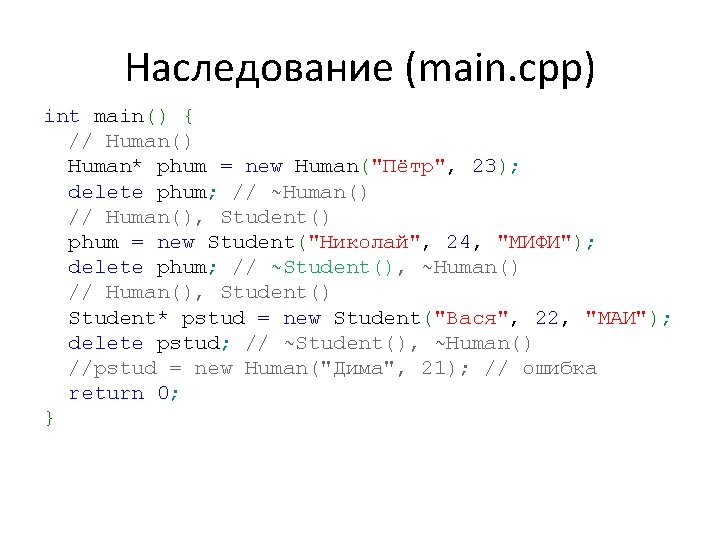
Наследование (main. cpp) int main() { // Human() Human* phum = new Human("Пётр", 23); delete phum; // ~Human() // Human(), Student() phum = new Student("Николай", 24, "МИФИ"); delete phum; // ~Student(), ~Human() // Human(), Student() Student* pstud = new Student("Вася", 22, "МАИ"); delete pstud; // ~Student(), ~Human() //pstud = new Human("Дима", 21); // ошибка return 0; }
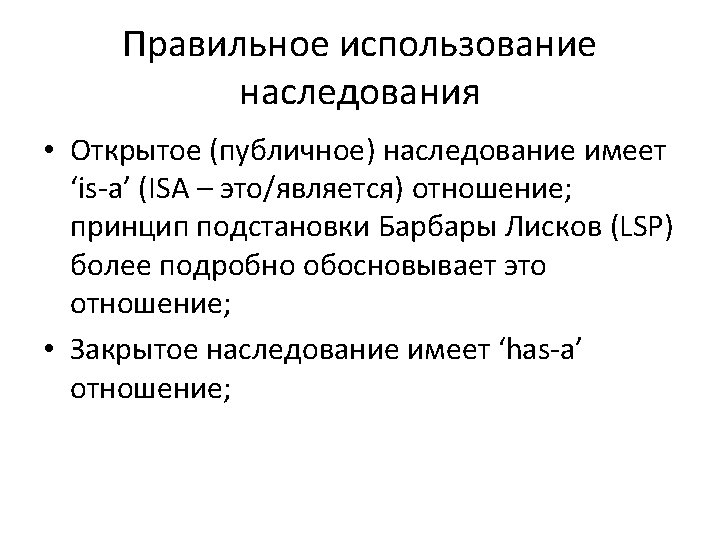
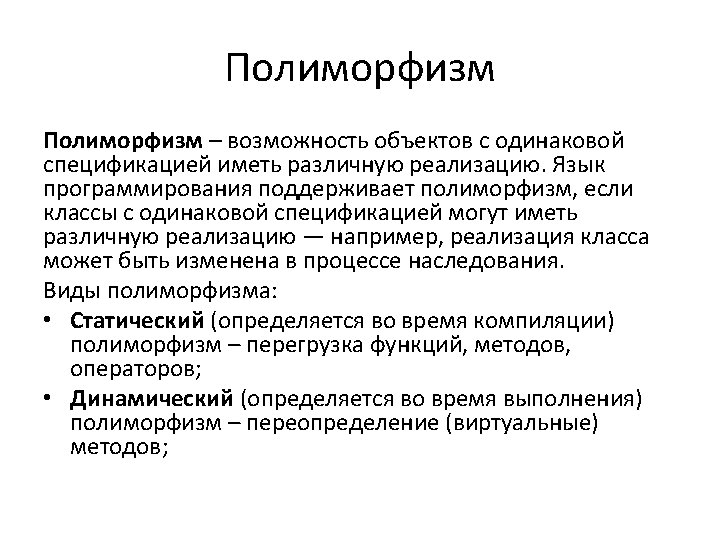
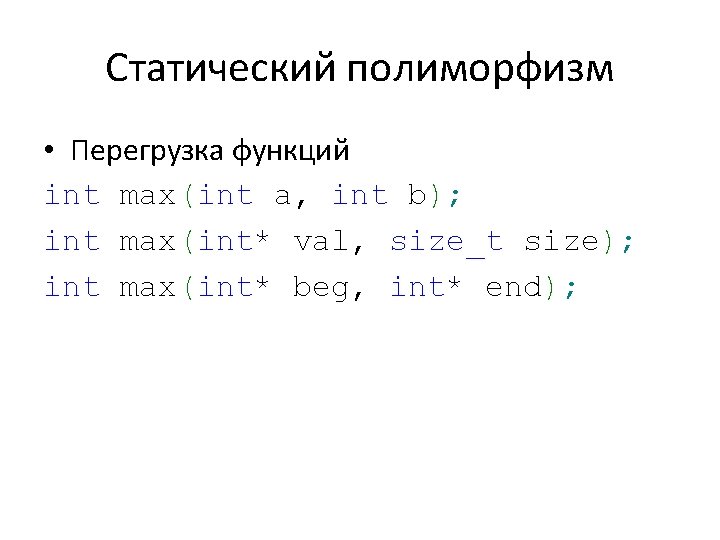
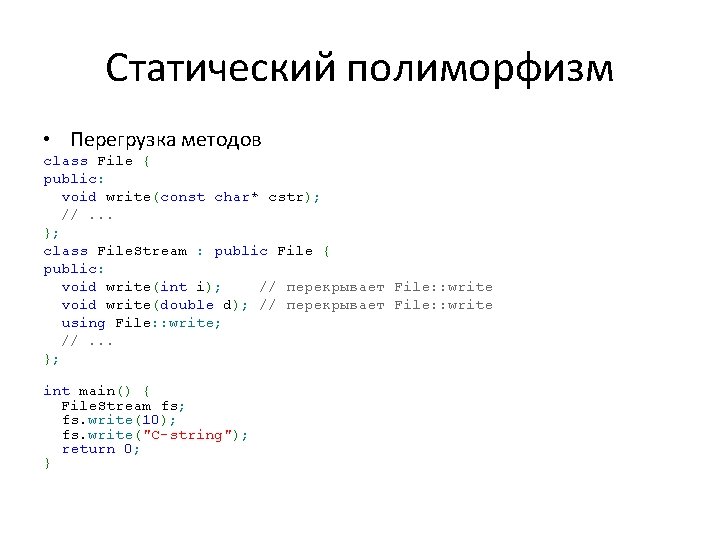
Статический полиморфизм • Перегрузка методов class File { public: void write(const char* cstr); //. . . }; class File. Stream : public File { public: void write(int i); // перекрывает File: : write void write(double d); // перекрывает File: : write using File: : write; //. . . }; int main() { File. Stream fs; fs. write(10); fs. write("C-string"); return 0; }
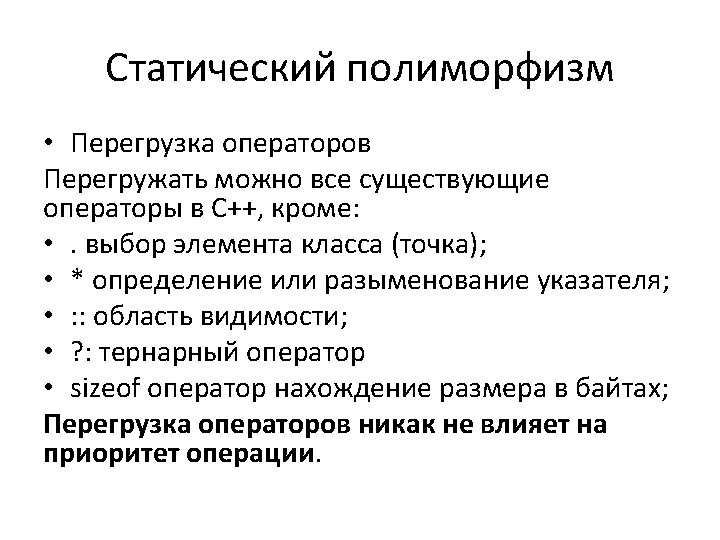
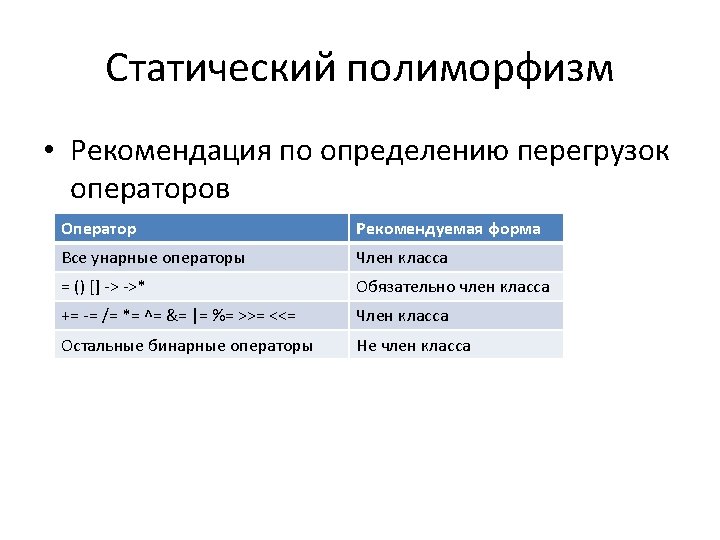
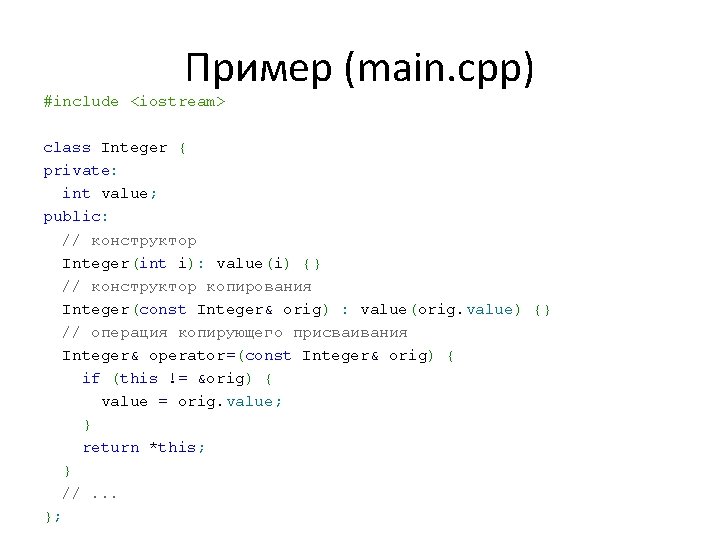
Пример (main. cpp) #include <iostream> class Integer { private: int value; public: // конструктор Integer(int i): value(i) {} // конструктор копирования Integer(const Integer& orig) : value(orig. value) {} // операция копирующего присваивания Integer& operator=(const Integer& orig) { if (this != &orig) { value = orig. value; } return *this; } //. . . };
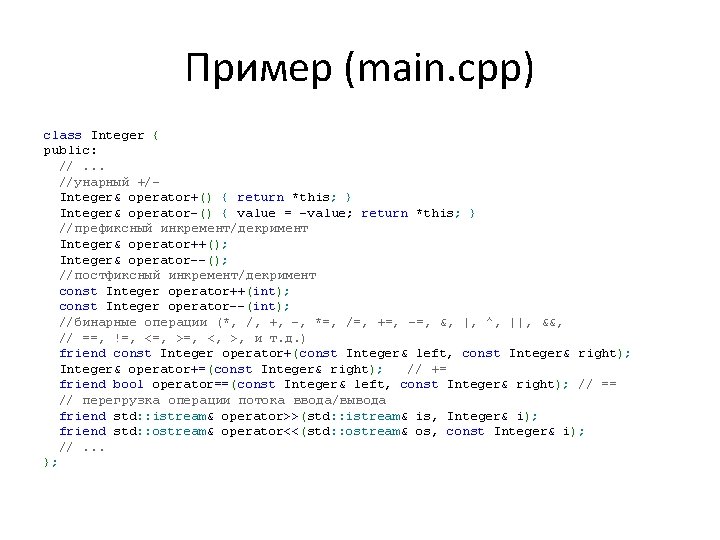
Пример (main. cpp) class Integer { public: //. . . //унарный +/Integer& operator+() { return *this; } Integer& operator-() { value = -value; return *this; } //префиксный инкремент/декримент Integer& operator++(); Integer& operator--(); //постфиксный инкремент/декримент const Integer operator++(int); const Integer operator--(int); //бинарные операции (*, /, +, -, *=, /=, +=, -=, &, |, ^, ||, &&, // ==, !=, <=, >=, <, >, и т. д. ) friend const Integer operator+(const Integer& left, const Integer& right); Integer& operator+=(const Integer& right); // += friend bool operator==(const Integer& left, const Integer& right); // == // перегрузка операции потока ввода/вывода friend std: : istream& operator>>(std: : istream& is, Integer& i); friend std: : ostream& operator<<(std: : ostream& os, const Integer& i); //. . . };
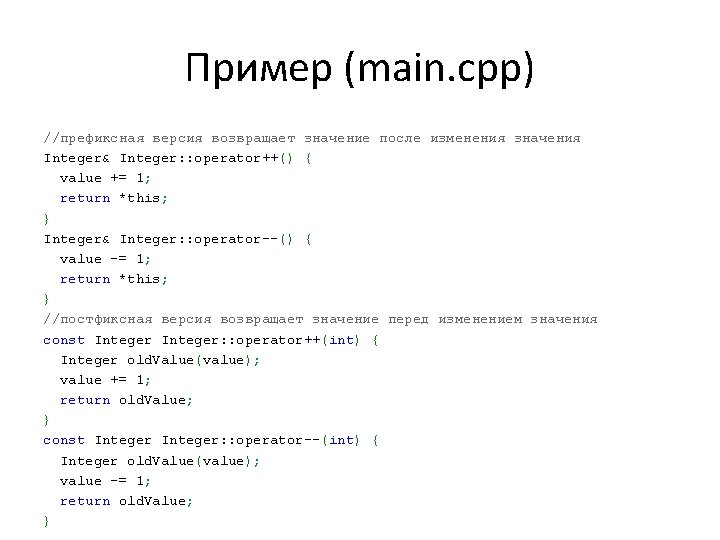
Пример (main. cpp) //префиксная версия возвращает значение после изменения значения Integer& Integer: : operator++() { value += 1; return *this; } Integer& Integer: : operator--() { value -= 1; return *this; } //постфиксная версия возвращает значение перед изменением значения const Integer: : operator++(int) { Integer old. Value(value); value += 1; return old. Value; } const Integer: : operator--(int) { Integer old. Value(value); value -= 1; return old. Value; }
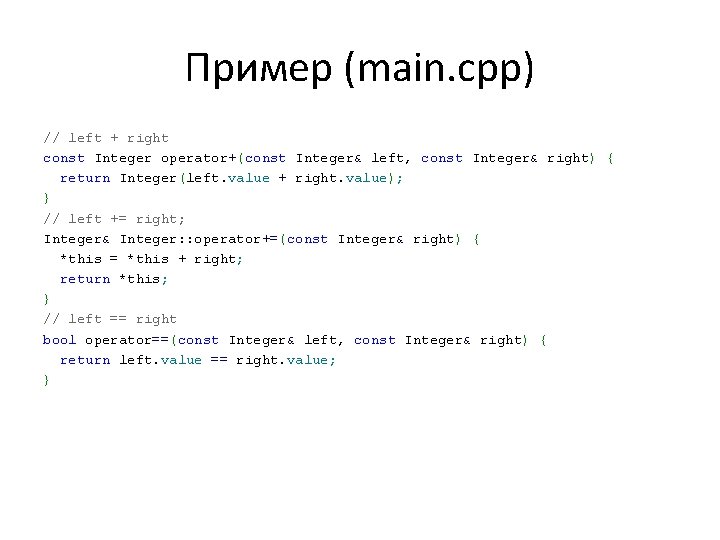
Пример (main. cpp) // left + right const Integer operator+(const Integer& left, const Integer& right) { return Integer(left. value + right. value); } // left += right; Integer& Integer: : operator+=(const Integer& right) { *this = *this + right; return *this; } // left == right bool operator==(const Integer& left, const Integer& right) { return left. value == right. value; }
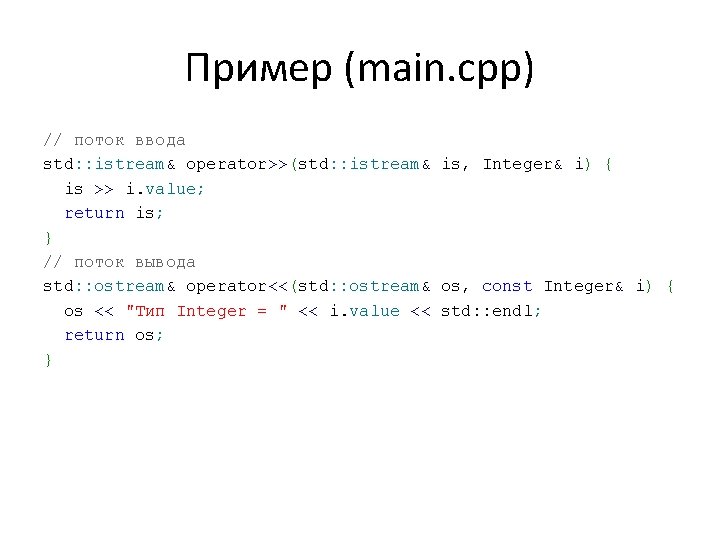
Пример (main. cpp) // поток ввода std: : istream& operator>>(std: : istream& is, Integer& i) { is >> i. value; return is; } // поток вывода std: : ostream& operator<<(std: : ostream& os, const Integer& i) { os << "Тип Integer = " << i. value << std: : endl; return os; }
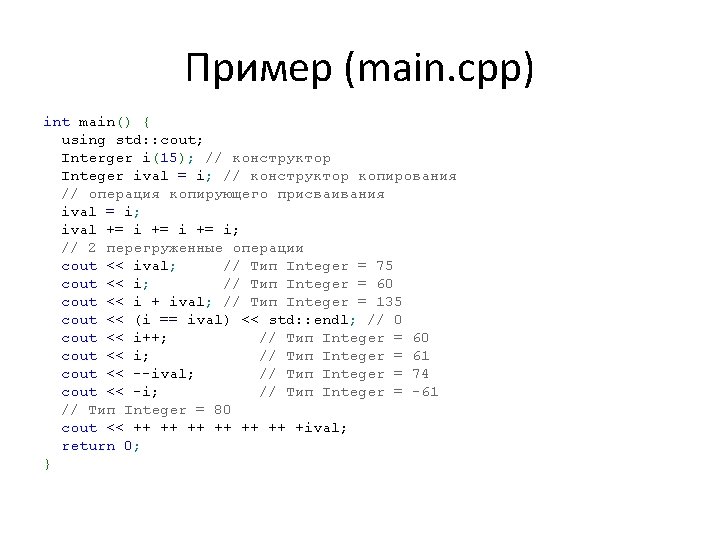
Пример (main. cpp) int main() { using std: : cout; Interger i(15); // конструктор Integer ival = i; // конструктор копирования // операция копирующего присваивания ival = i; ival += i; // 2 перегруженные операции cout << ival; // Тип Integer = 75 cout << i; // Тип Integer = 60 cout << i + ival; // Тип Integer = 135 cout << (i == ival) << std: : endl; // 0 cout << i++; // Тип Integer = 60 cout << i; // Тип Integer = 61 cout << --ival; // Тип Integer = 74 cout << -i; // Тип Integer = -61 // Тип Integer = 80 cout << ++ ++ ++ +ival; return 0; }
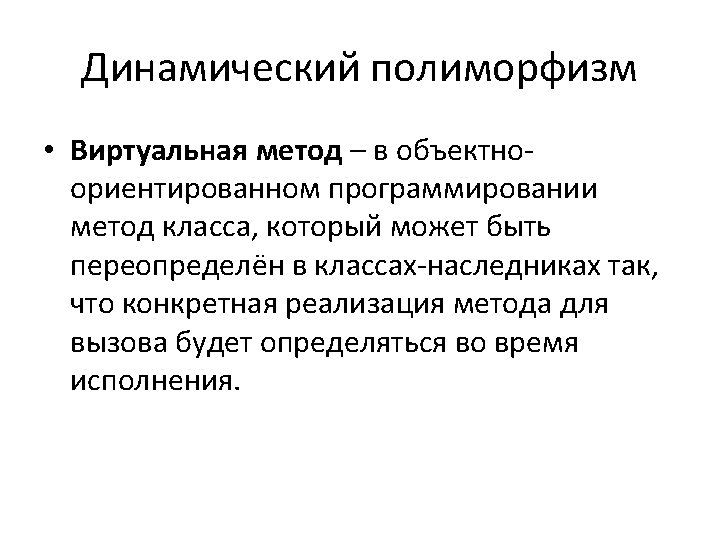
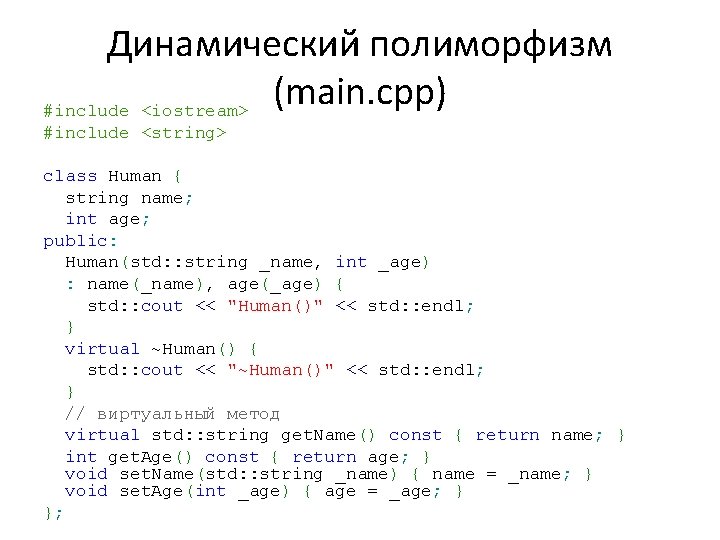
Динамический полиморфизм (main. cpp) #include <iostream> #include <string> class Human { string name; int age; public: Human(std: : string _name, int _age) : name(_name), age(_age) { std: : cout << "Human()" << std: : endl; } virtual ~Human() { std: : cout << "~Human()" << std: : endl; } // виртуальный метод virtual std: : string get. Name() const { return name; } int get. Age() const { return age; } void set. Name(std: : string _name) { name = _name; } void set. Age(int _age) { age = _age; } };
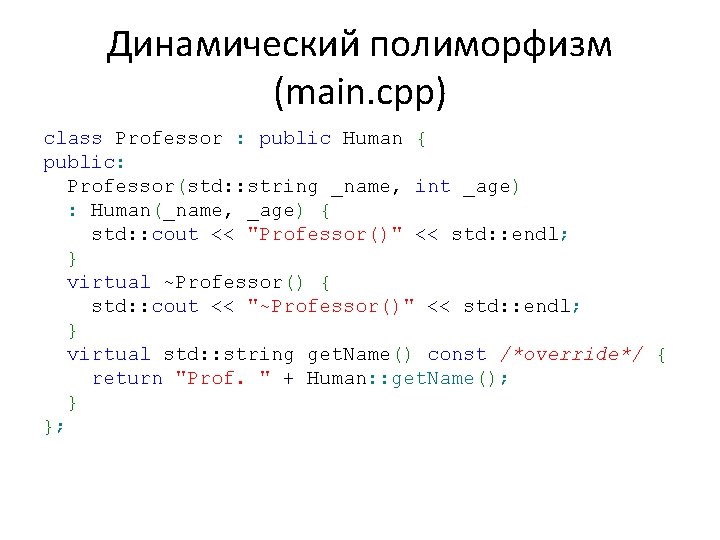
Динамический полиморфизм (main. cpp) class Professor : public Human { public: Professor(std: : string _name, int _age) : Human(_name, _age) { std: : cout << "Professor()" << std: : endl; } virtual ~Professor() { std: : cout << "~Professor()" << std: : endl; } virtual std: : string get. Name() const /*override*/ { return "Prof. " + Human: : get. Name(); } };
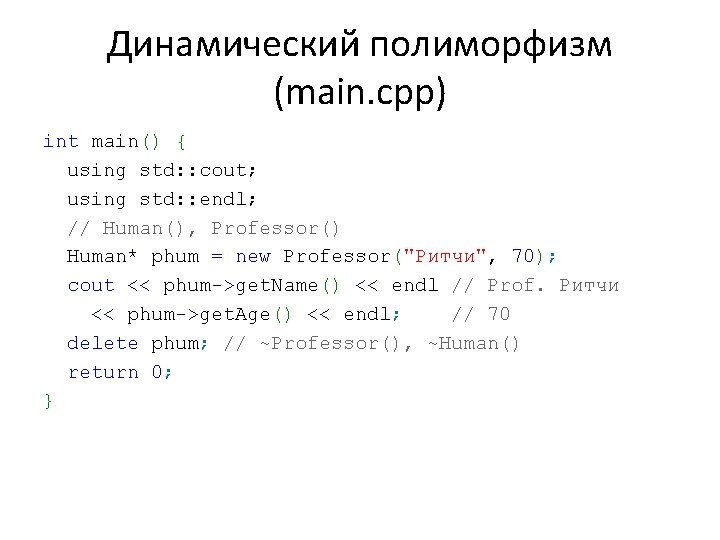
Динамический полиморфизм (main. cpp) int main() { using std: : cout; using std: : endl; // Human(), Professor() Human* phum = new Professor("Ритчи", 70); cout << phum->get. Name() << endl // Prof. Ритчи << phum->get. Age() << endl; // 70 delete phum; // ~Professor(), ~Human() return 0; }
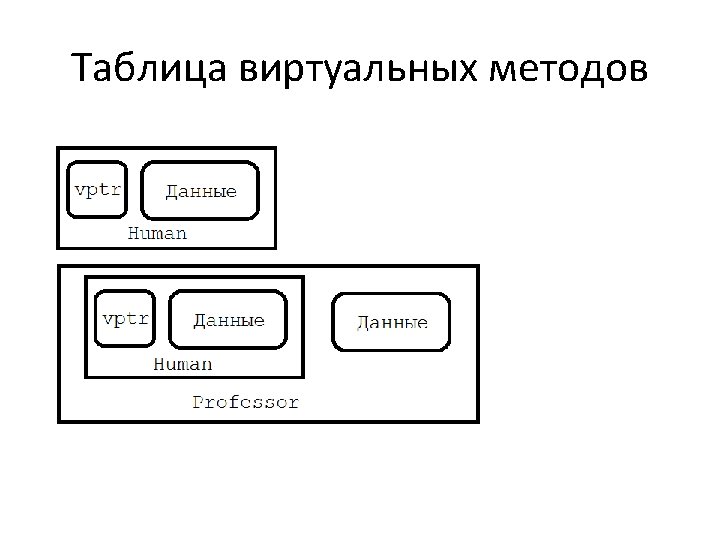
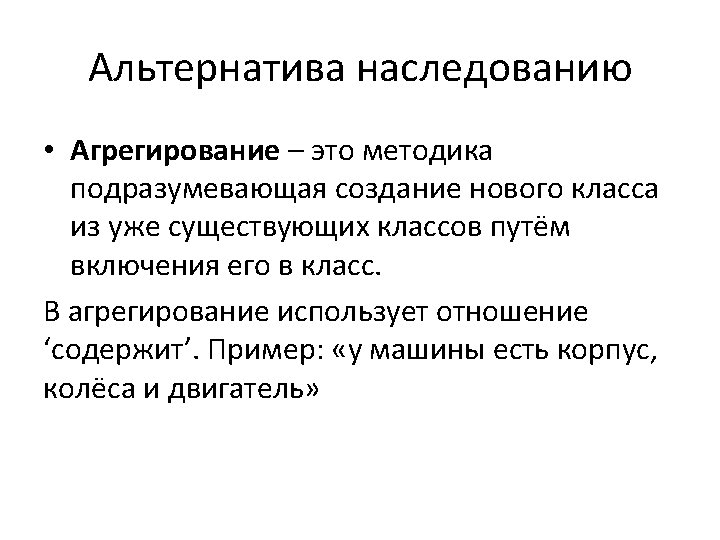
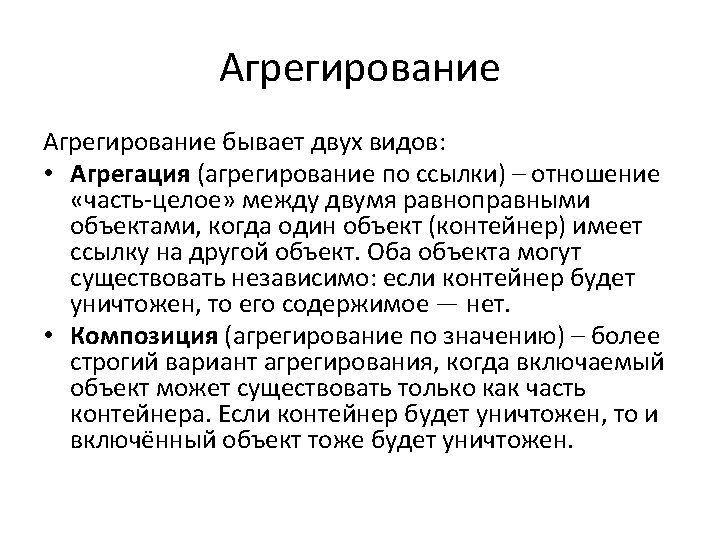
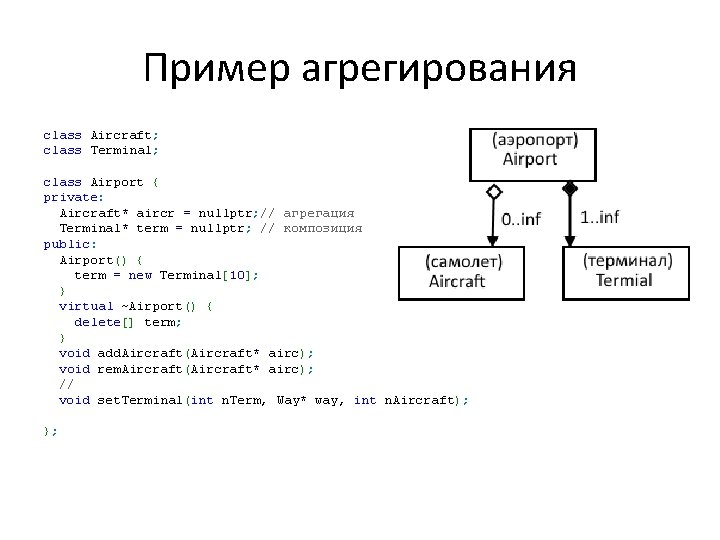
Пример агрегирования class Aircraft; class Terminal; class Airport { private: Aircraft* aircr = nullptr; // агрегация Terminal* term = nullptr; // композиция public: Airport() { term = new Terminal[10]; } virtual ~Airport() { delete[] term; } void add. Aircraft(Aircraft* airc); void rem. Aircraft(Aircraft* airc); // void set. Terminal(int n. Term, Way* way, int n. Aircraft); };
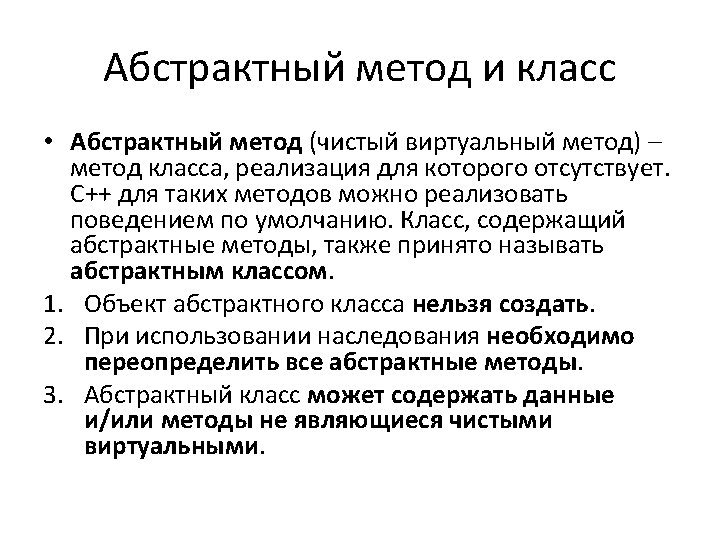
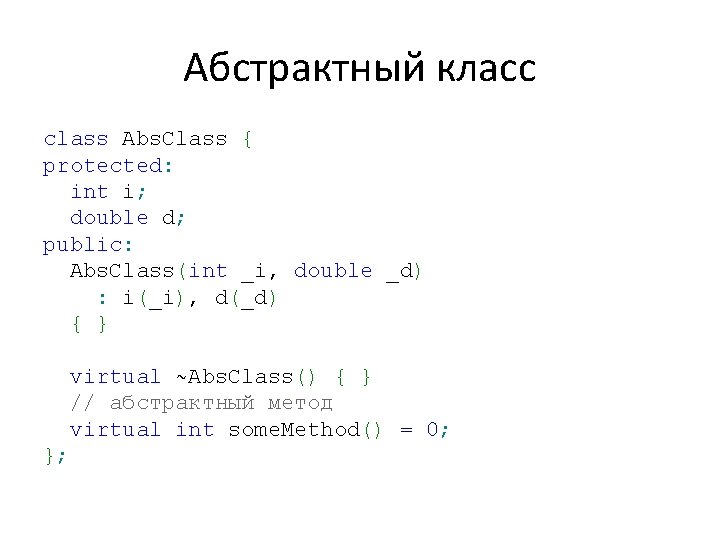
Абстрактный класс class Abs. Class { protected: int i; double d; public: Abs. Class(int _i, double _d) : i(_i), d(_d) { } virtual ~Abs. Class() { } // абстрактный метод virtual int some. Method() = 0; };
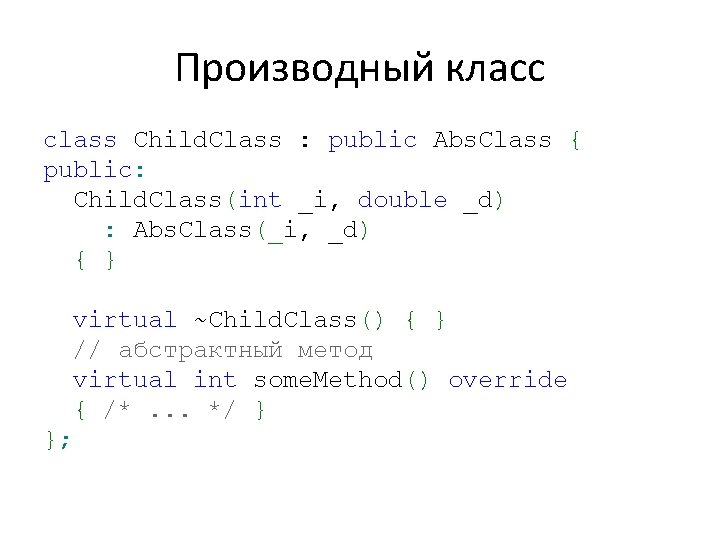
Производный класс class Child. Class : public Abs. Class { public: Child. Class(int _i, double _d) : Abs. Class(_i, _d) { } virtual ~Child. Class() { } // абстрактный метод virtual int some. Method() override { /*. . . */ } };
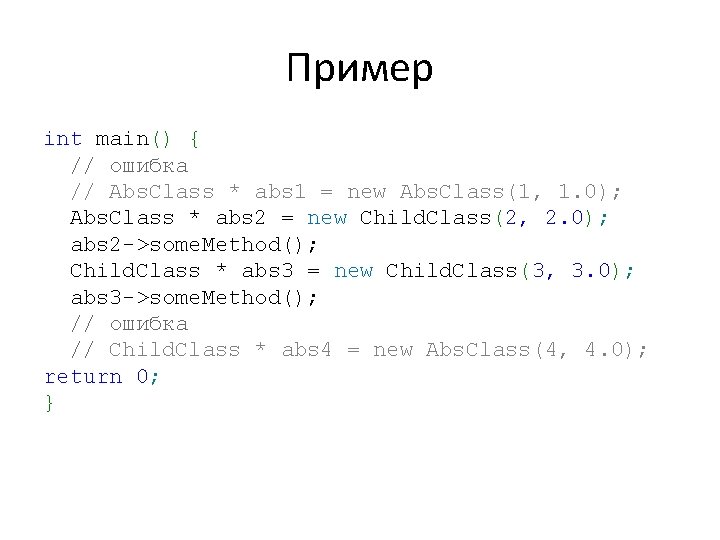
Пример int main() { // ошибка // Abs. Class * abs 1 = new Abs. Class(1, 1. 0); Abs. Class * abs 2 = new Child. Class(2, 2. 0); abs 2 ->some. Method(); Child. Class * abs 3 = new Child. Class(3, 3. 0); abs 3 ->some. Method(); // ошибка // Child. Class * abs 4 = new Abs. Class(4, 4. 0); return 0; }
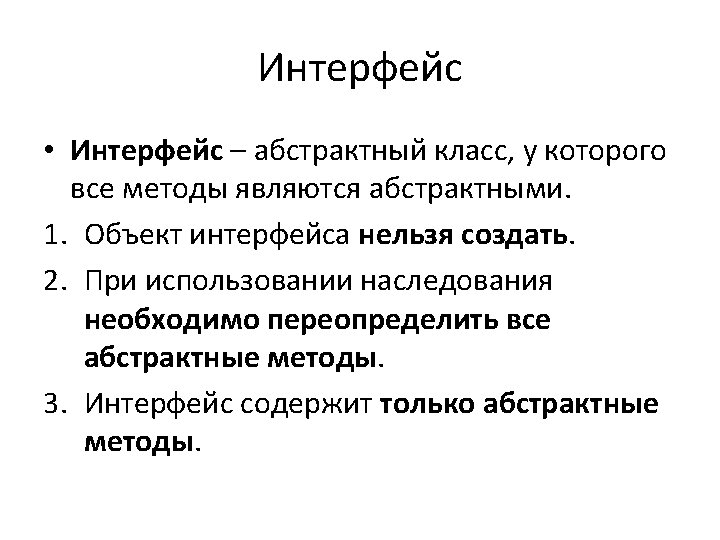
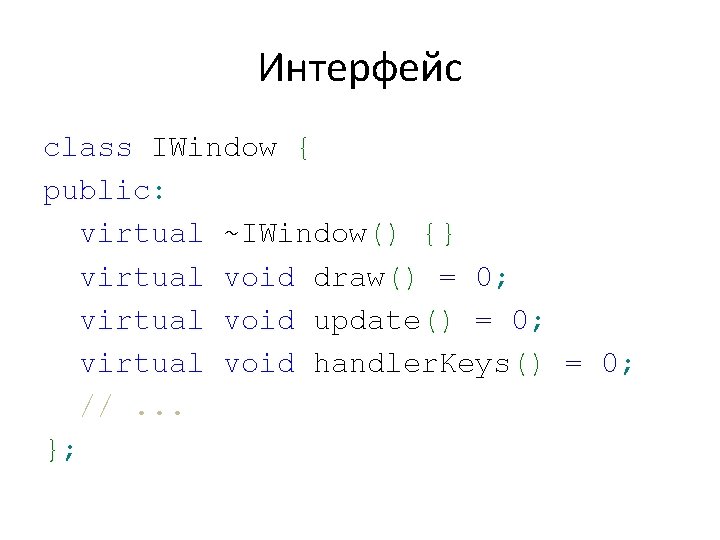
Интерфейс class IWindow { public: virtual ~IWindow() {} virtual void draw() = 0; virtual void update() = 0; virtual void handler. Keys() = 0; //. . . };
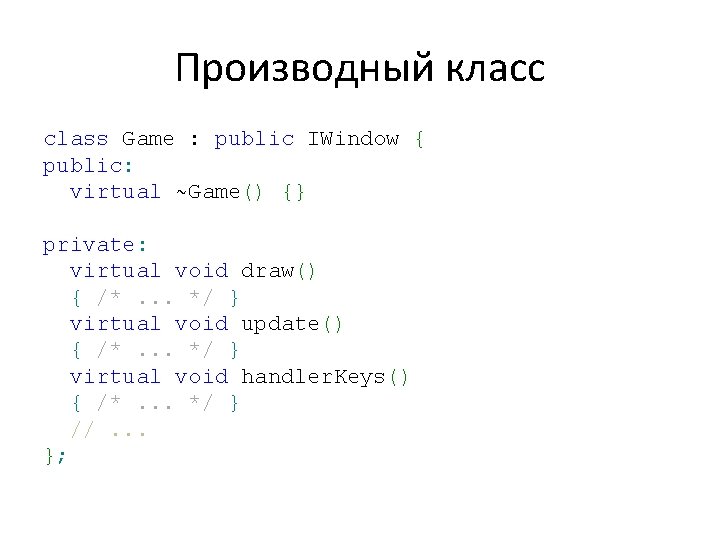
Производный класс class Game : public IWindow { public: virtual ~Game() {} private: virtual void draw() { /*. . . */ } virtual void update() { /*. . . */ } virtual void handler. Keys() { /*. . . */ } //. . . };
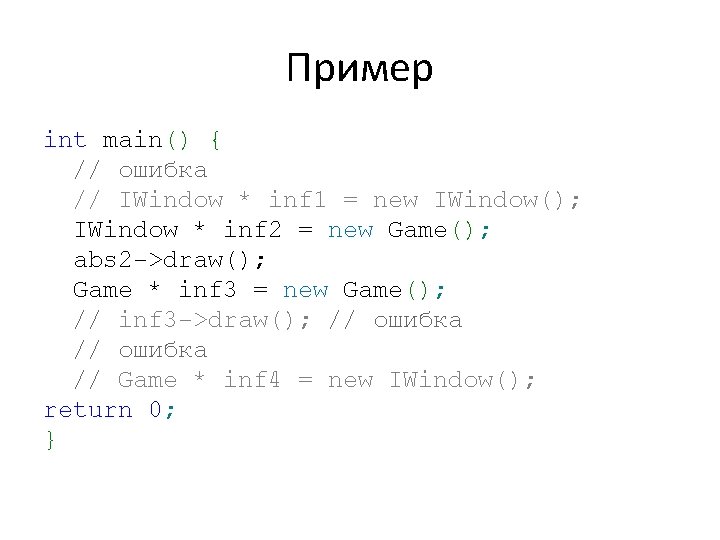
Пример int main() { // ошибка // IWindow * inf 1 = new IWindow(); IWindow * inf 2 = new Game(); abs 2 ->draw(); Game * inf 3 = new Game(); // inf 3 ->draw(); // ошибка // Game * inf 4 = new IWindow(); return 0; }
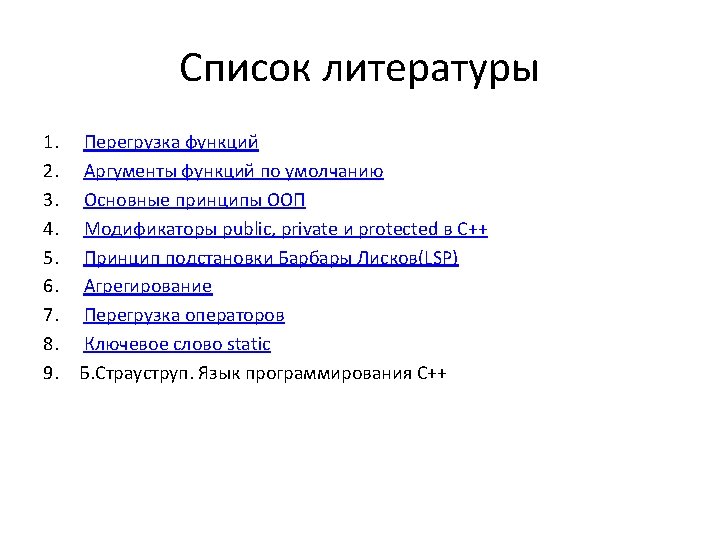
C++ include iostream
Cppinclude
Std::endl
Include
#include iostream using namespace std int main()
윤년 계산
#include using namespace std class base
Include iostream using namespace std
Using namespace std
#include iostream using namespace std
#include stdio.h #include stdlib.h #include string.h
String.h
Licenseid=string&content=string&/paramsxml=string
#include iostream #include cmath
#include iostream.h void main()
#include stdio.h #include conio.h #include stdlib.h
Fpos_t
Stdlib.h
Fungsi using namespace std
Using namespace std
Using namespace std
Using namespace std c++
Cstdlib
#include iostream adalah
#include iostream
Iostream h
Int
#iclude iostream
Include iostream h