A simple C program include iostream using namespace
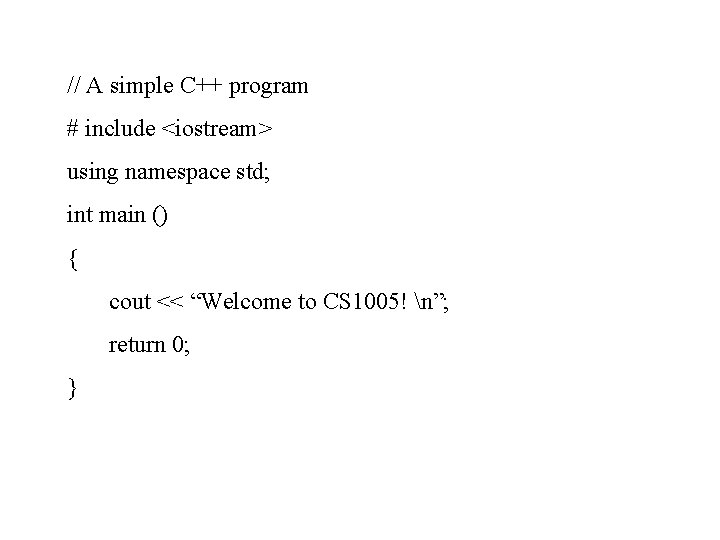
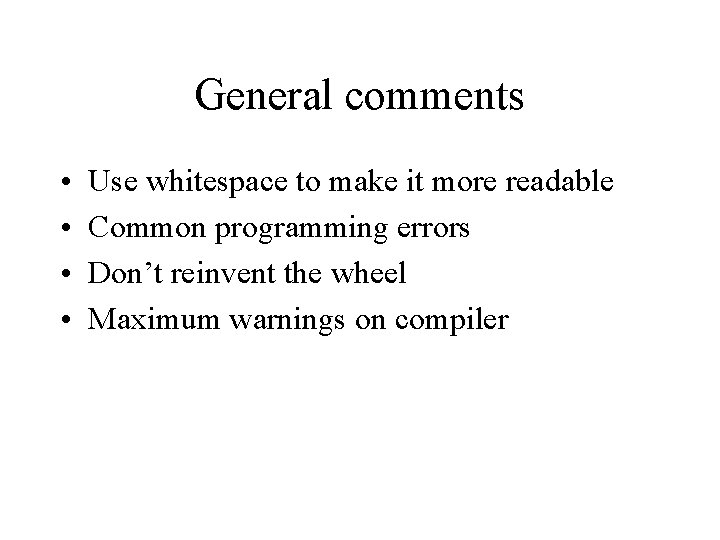
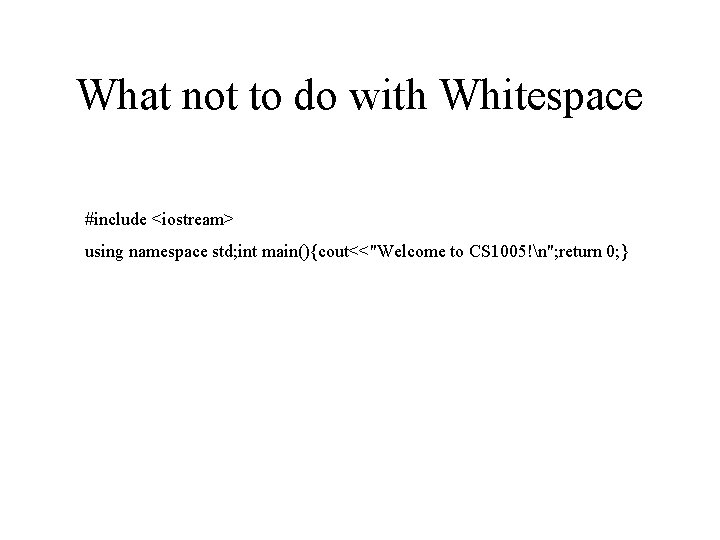
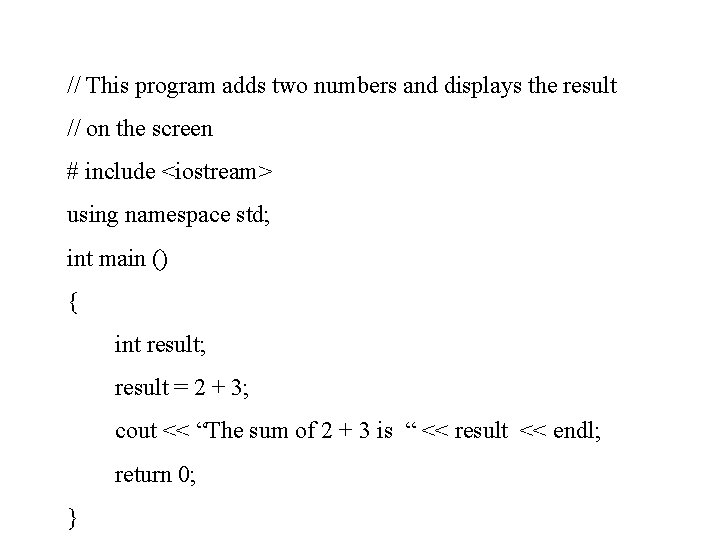
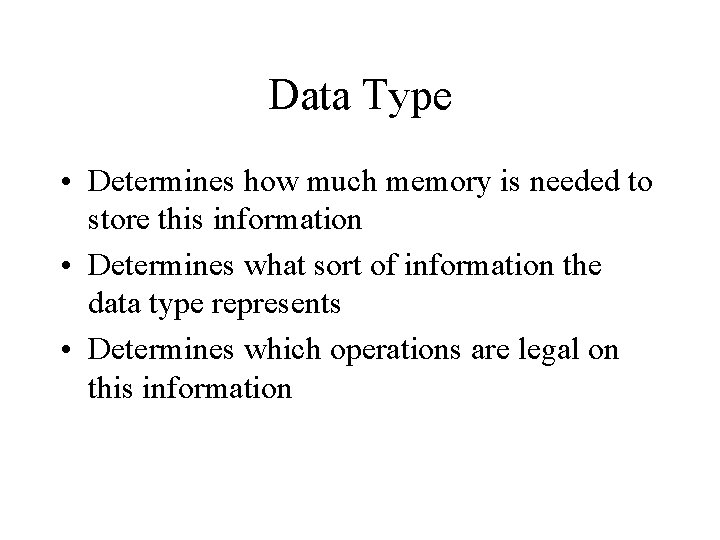
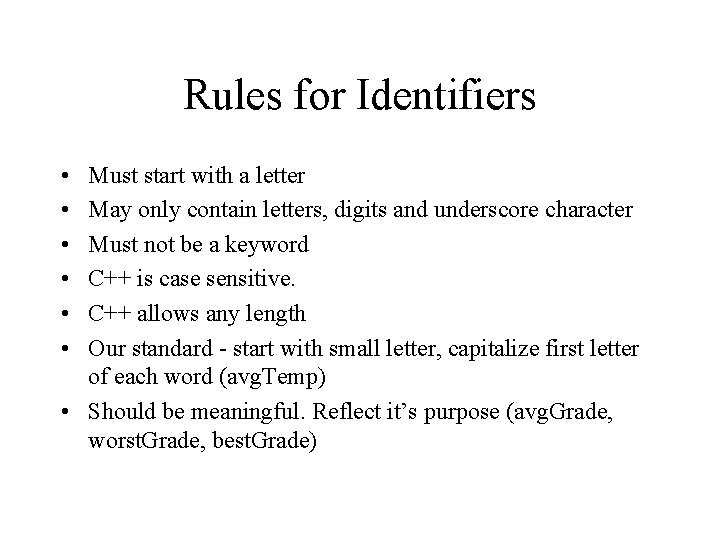
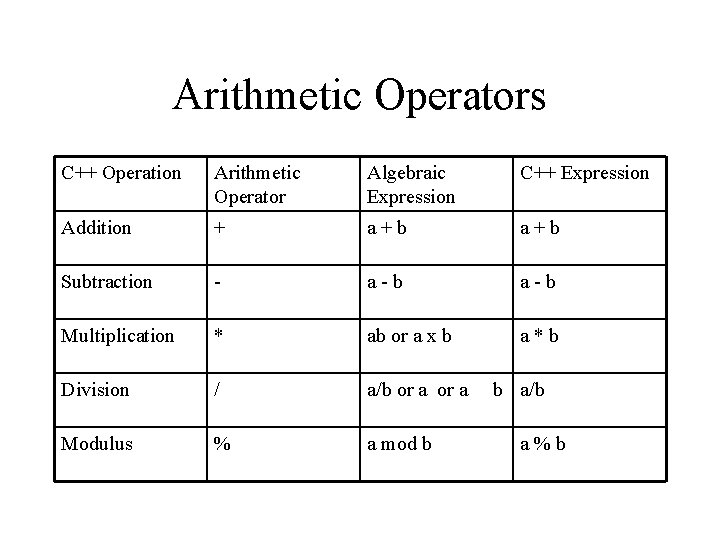
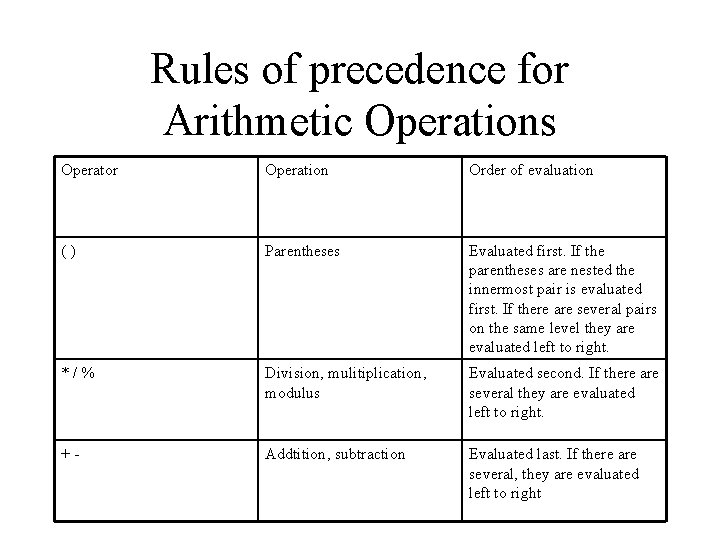
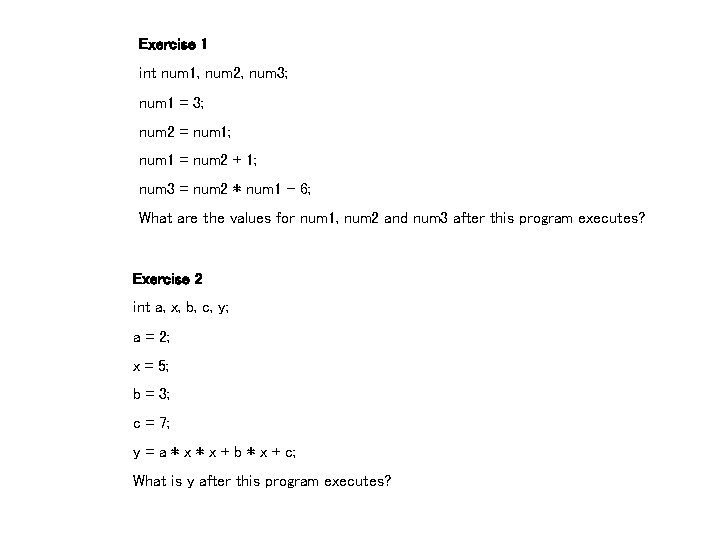
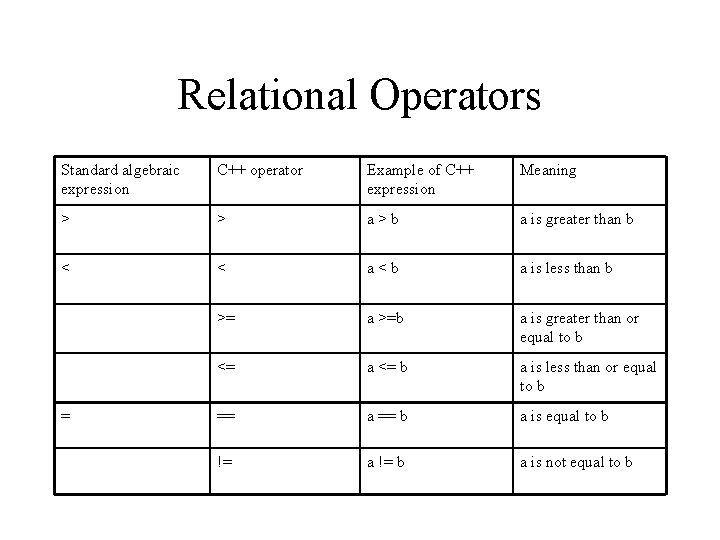
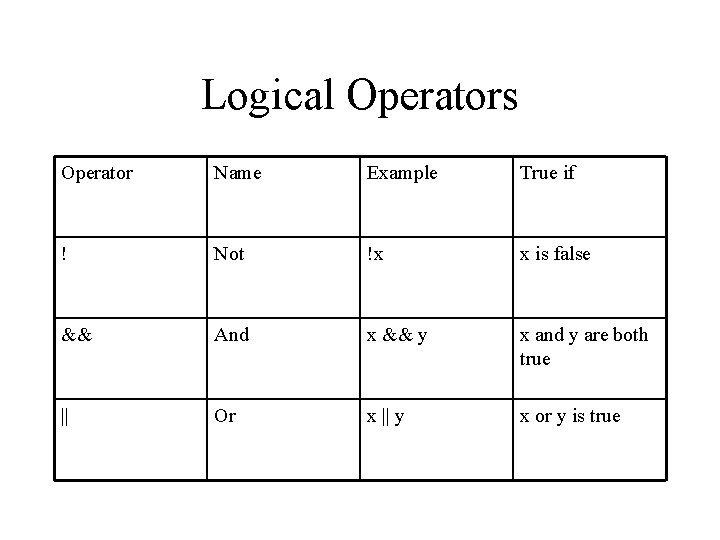
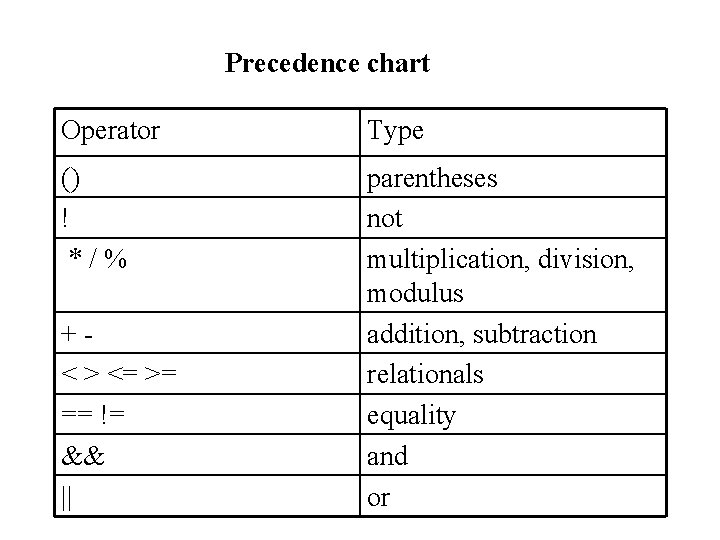
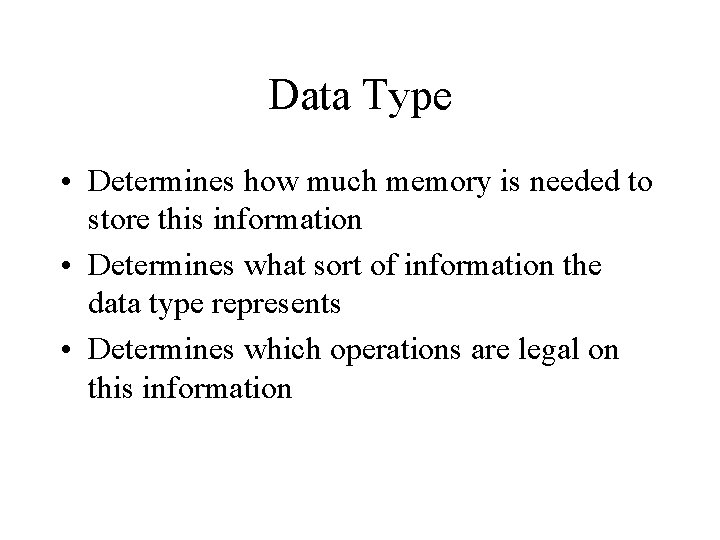
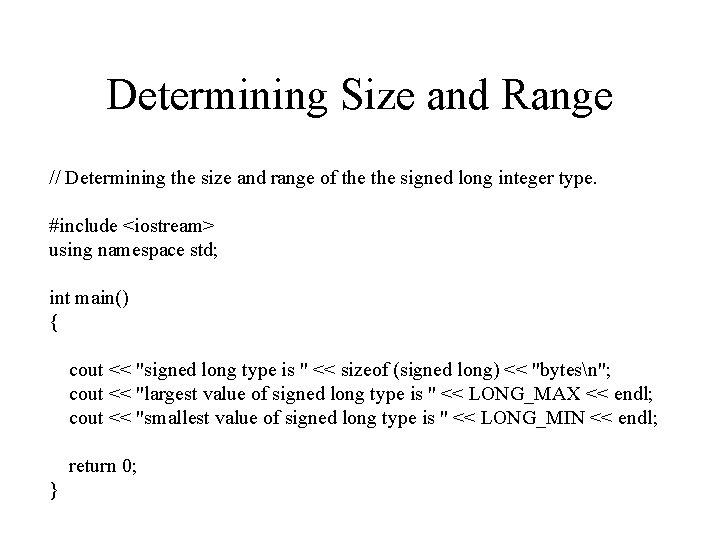
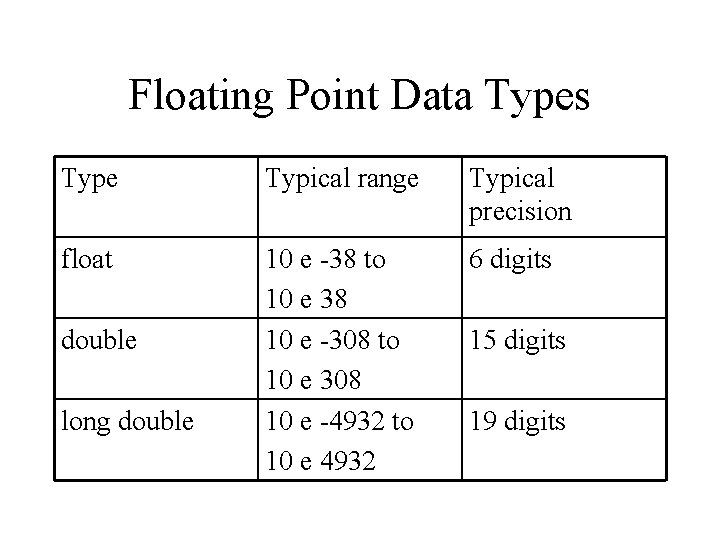
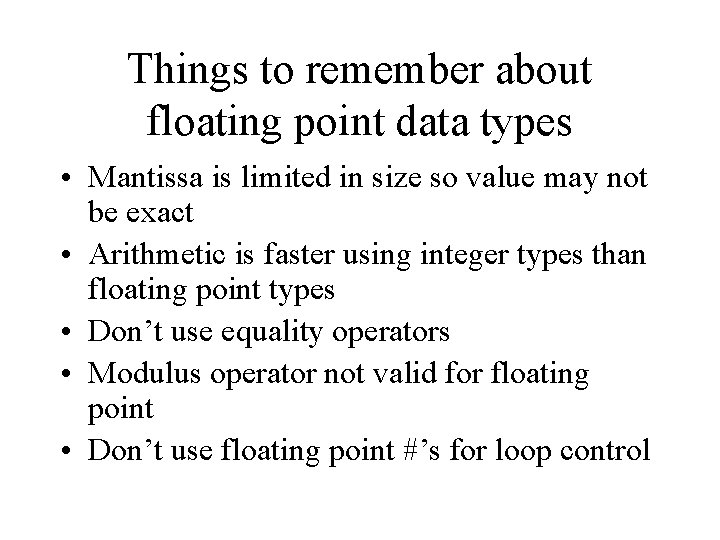
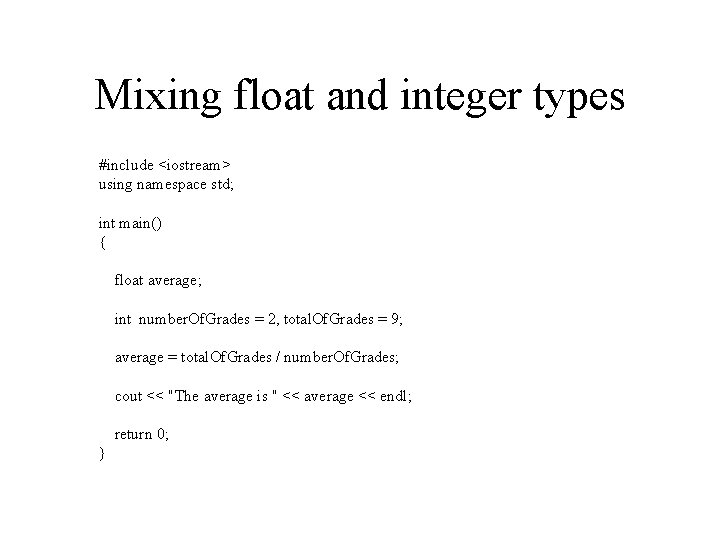
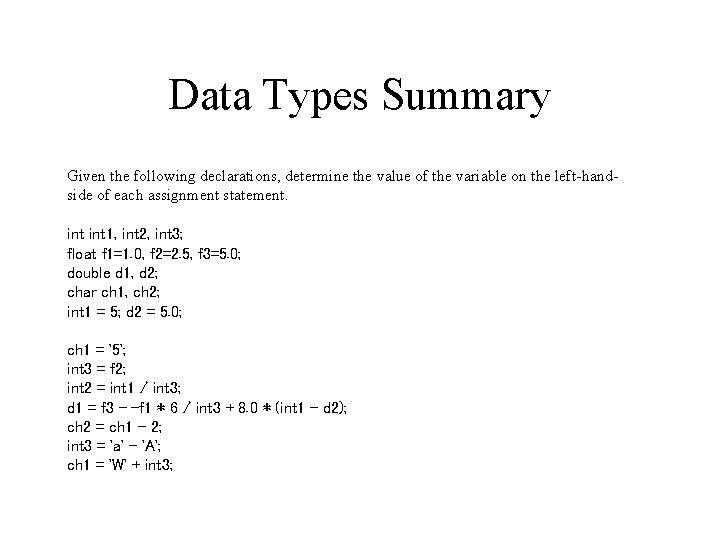
- Slides: 18
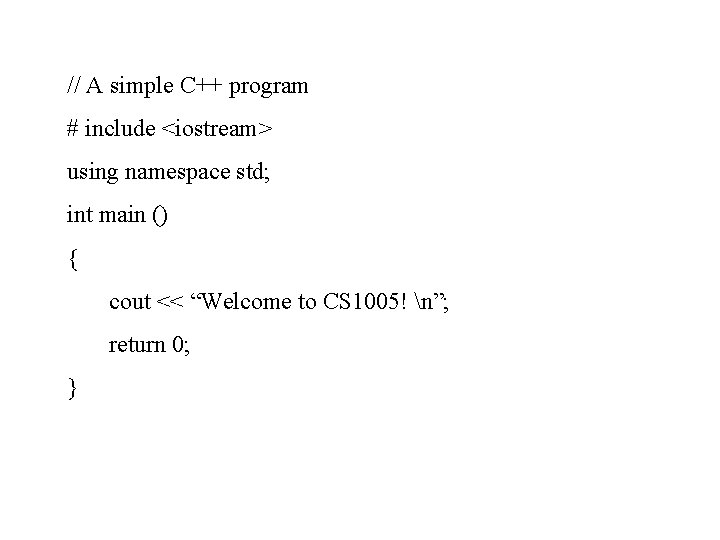
// A simple C++ program # include <iostream> using namespace std; int main () { cout << “Welcome to CS 1005! n”; return 0; }
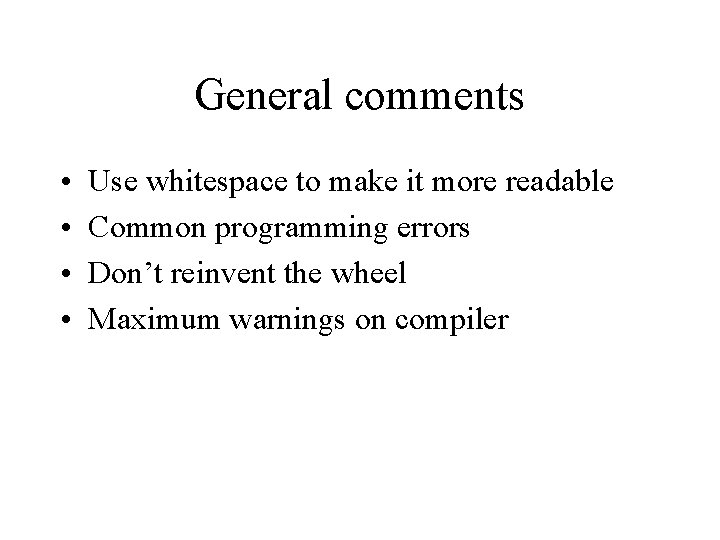
General comments • • Use whitespace to make it more readable Common programming errors Don’t reinvent the wheel Maximum warnings on compiler
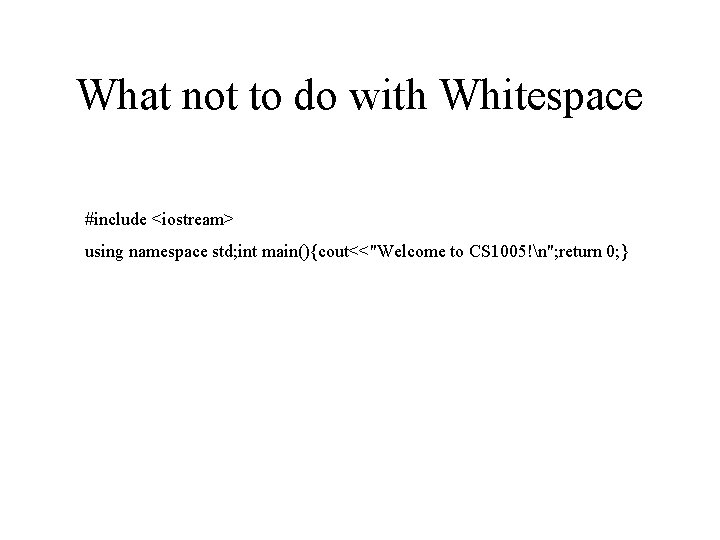
What not to do with Whitespace #include <iostream> using namespace std; int main(){cout<<"Welcome to CS 1005!n"; return 0; }
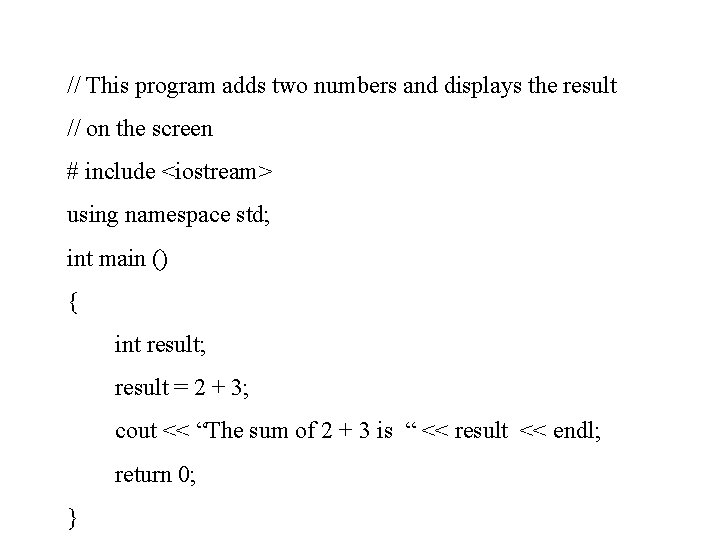
// This program adds two numbers and displays the result // on the screen # include <iostream> using namespace std; int main () { int result; result = 2 + 3; cout << “The sum of 2 + 3 is “ << result << endl; return 0; }
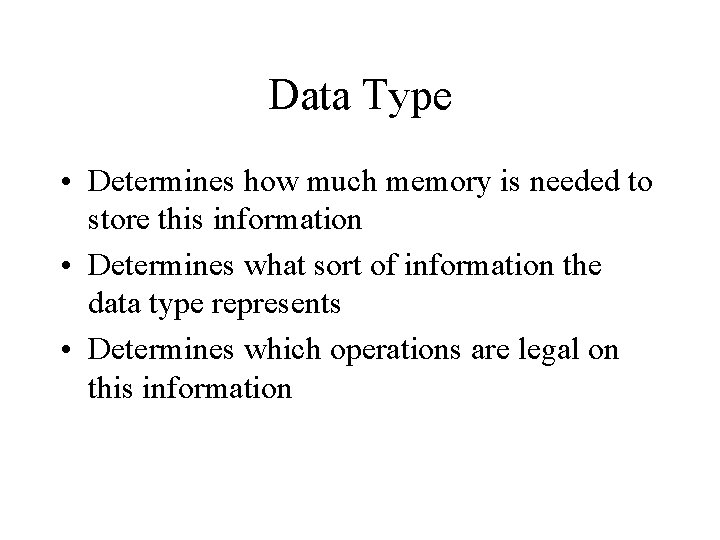
Data Type • Determines how much memory is needed to store this information • Determines what sort of information the data type represents • Determines which operations are legal on this information
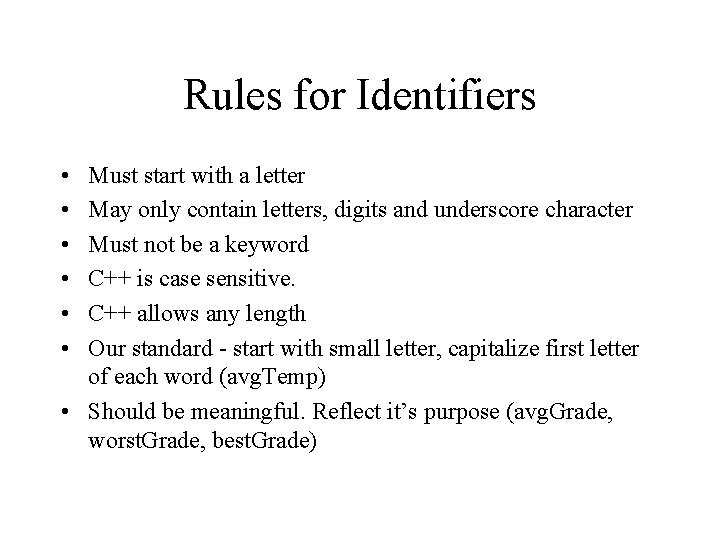
Rules for Identifiers • • • Must start with a letter May only contain letters, digits and underscore character Must not be a keyword C++ is case sensitive. C++ allows any length Our standard - start with small letter, capitalize first letter of each word (avg. Temp) • Should be meaningful. Reflect it’s purpose (avg. Grade, worst. Grade, best. Grade)
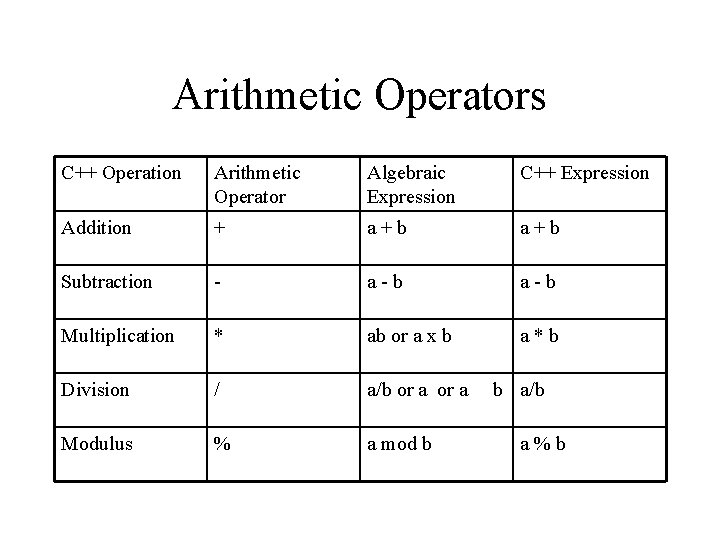
Arithmetic Operators C++ Operation Arithmetic Operator Algebraic Expression C++ Expression Addition + a+b Subtraction - a-b Multiplication * ab or a x b a*b Division / a/b or a Modulus % a mod b b a/b a%b
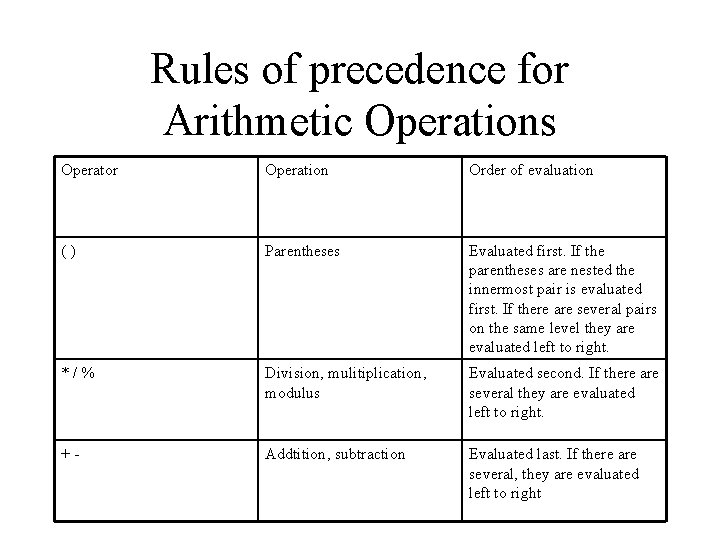
Rules of precedence for Arithmetic Operations Operator Operation Order of evaluation () Parentheses Evaluated first. If the parentheses are nested the innermost pair is evaluated first. If there are several pairs on the same level they are evaluated left to right. */% Division, mulitiplication, modulus Evaluated second. If there are several they are evaluated left to right. +- Addtition, subtraction Evaluated last. If there are several, they are evaluated left to right
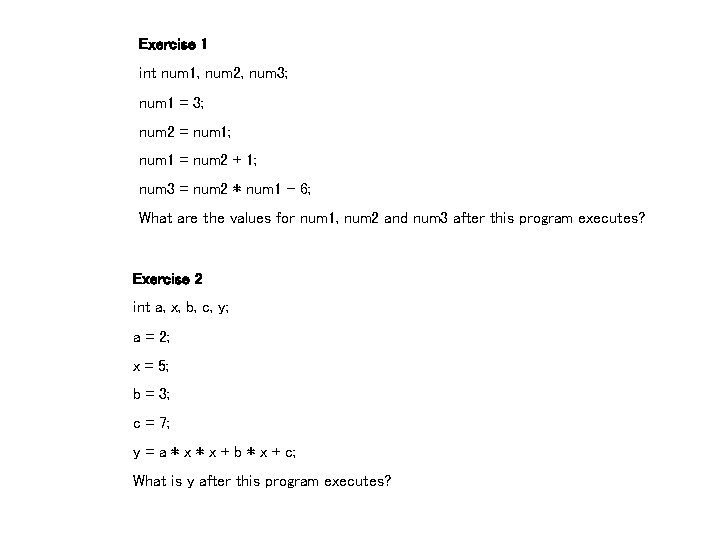
Exercise 1 int num 1, num 2, num 3; num 1 = 3; num 2 = num 1; num 1 = num 2 + 1; num 3 = num 2 * num 1 - 6; What are the values for num 1, num 2 and num 3 after this program executes? Exercise 2 int a, x, b, c, y; a = 2; x = 5; b = 3; c = 7; y = a * x + b * x + c; What is y after this program executes?
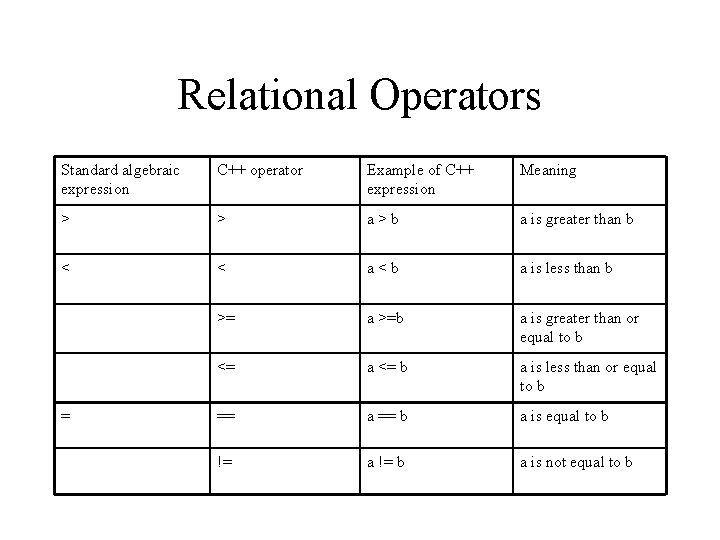
Relational Operators Standard algebraic expression C++ operator Example of C++ expression Meaning > > a>b a is greater than b < < a<b a is less than b >= a >=b a is greater than or equal to b <= a <= b a is less than or equal to b == a == b a is equal to b != a != b a is not equal to b =
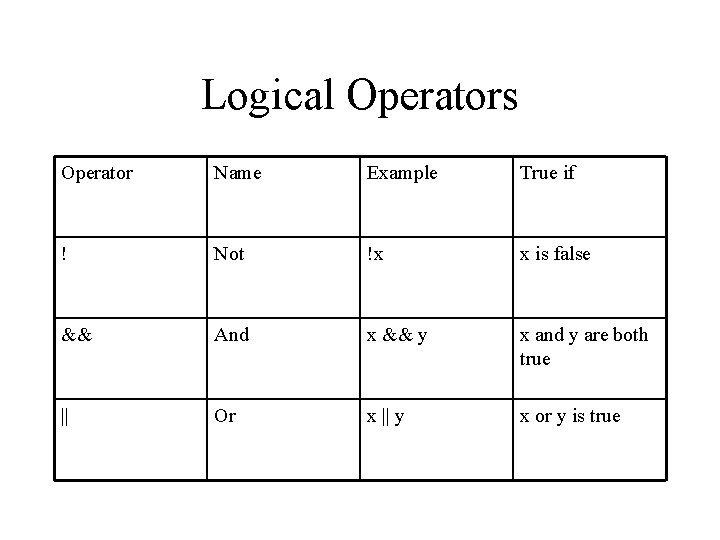
Logical Operators Operator Name Example True if ! Not !x x is false && And x && y x and y are both true || Or x || y x or y is true
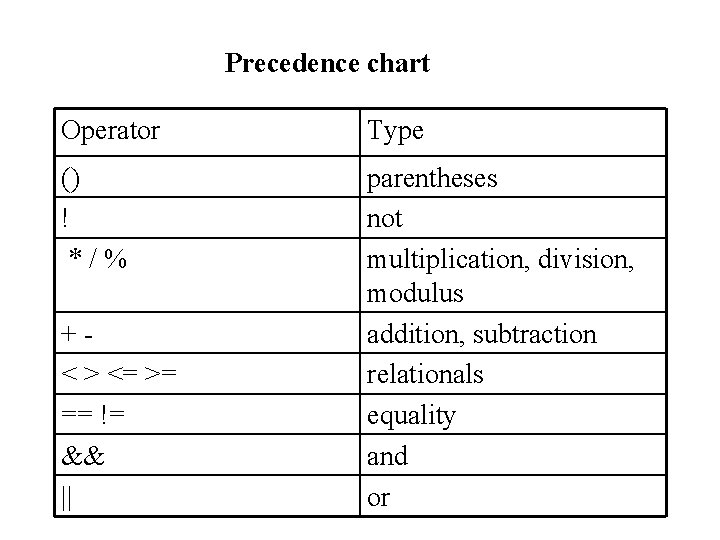
Precedence chart Operator Type () ! */% parentheses not multiplication, division, modulus addition, subtraction relationals equality and or +< > <= >= == != && ||
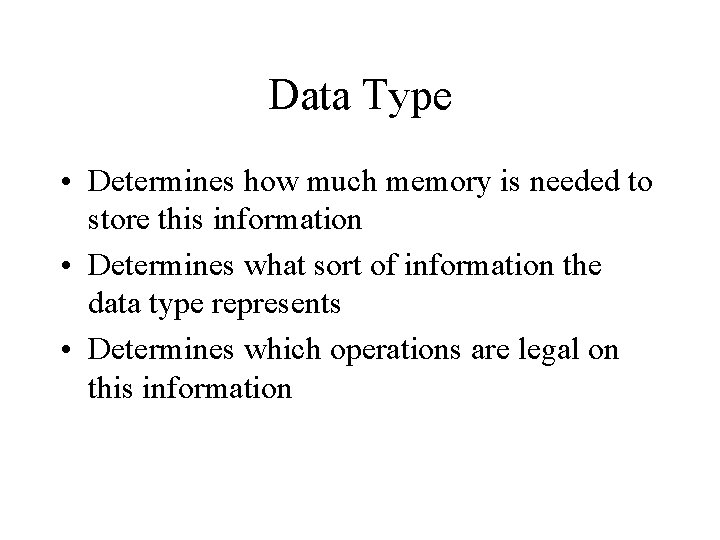
Data Type • Determines how much memory is needed to store this information • Determines what sort of information the data type represents • Determines which operations are legal on this information
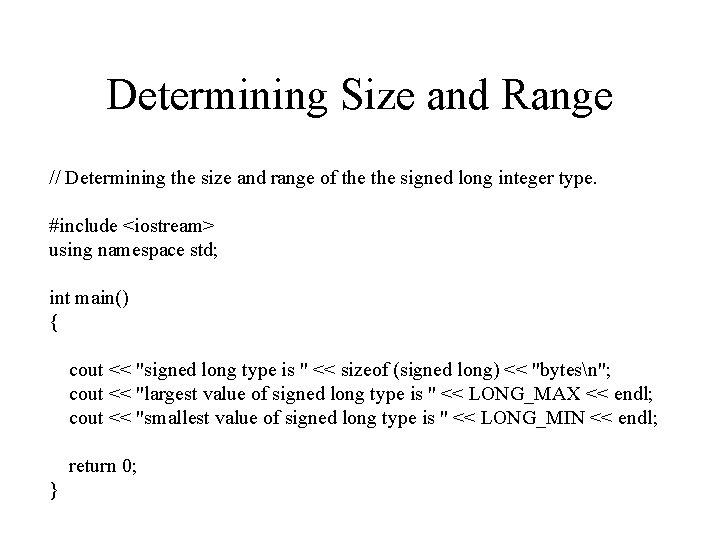
Determining Size and Range // Determining the size and range of the signed long integer type. #include <iostream> using namespace std; int main() { cout << "signed long type is " << sizeof (signed long) << "bytesn"; cout << "largest value of signed long type is " << LONG_MAX << endl; cout << "smallest value of signed long type is " << LONG_MIN << endl; return 0; }
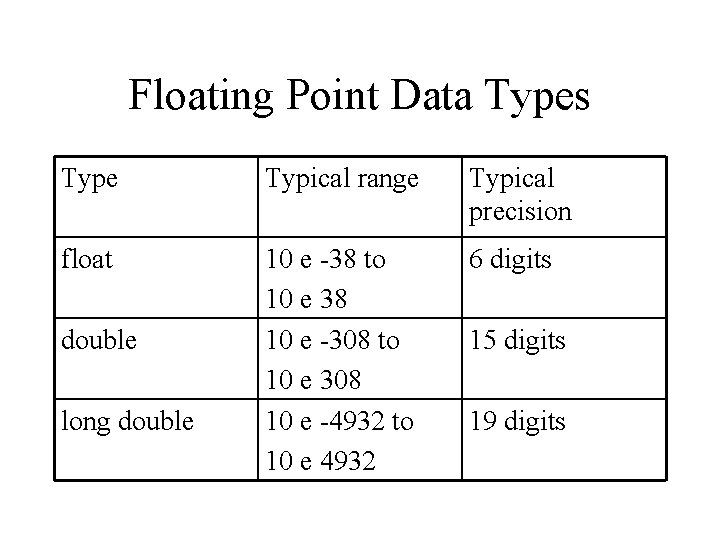
Floating Point Data Types Type Typical range Typical precision float 10 e -38 to 10 e 38 10 e -308 to 10 e 308 10 e -4932 to 10 e 4932 6 digits double long double 15 digits 19 digits
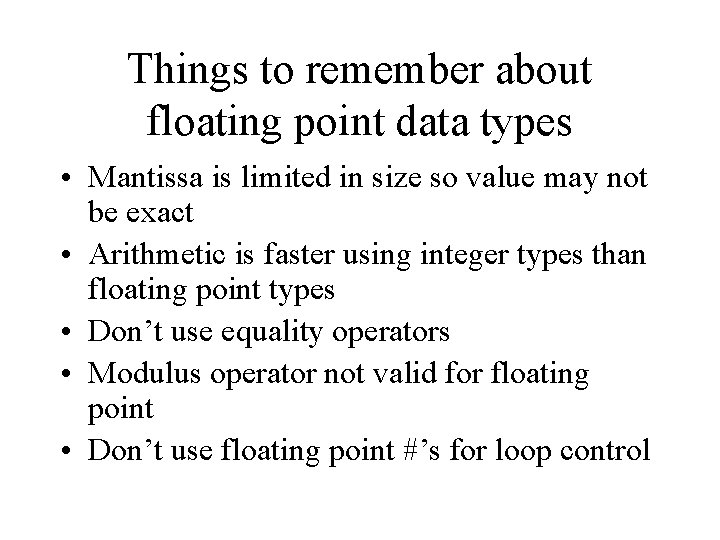
Things to remember about floating point data types • Mantissa is limited in size so value may not be exact • Arithmetic is faster using integer types than floating point types • Don’t use equality operators • Modulus operator not valid for floating point • Don’t use floating point #’s for loop control
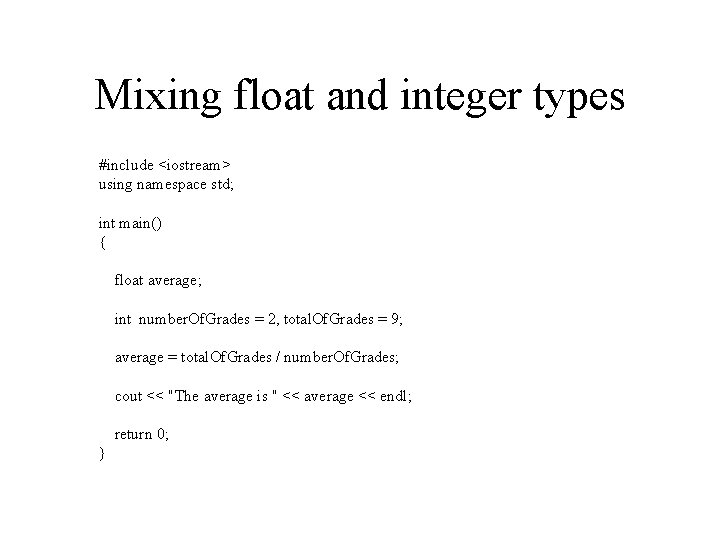
Mixing float and integer types #include <iostream> using namespace std; int main() { float average; int number. Of. Grades = 2, total. Of. Grades = 9; average = total. Of. Grades / number. Of. Grades; cout << "The average is " << average << endl; return 0; }
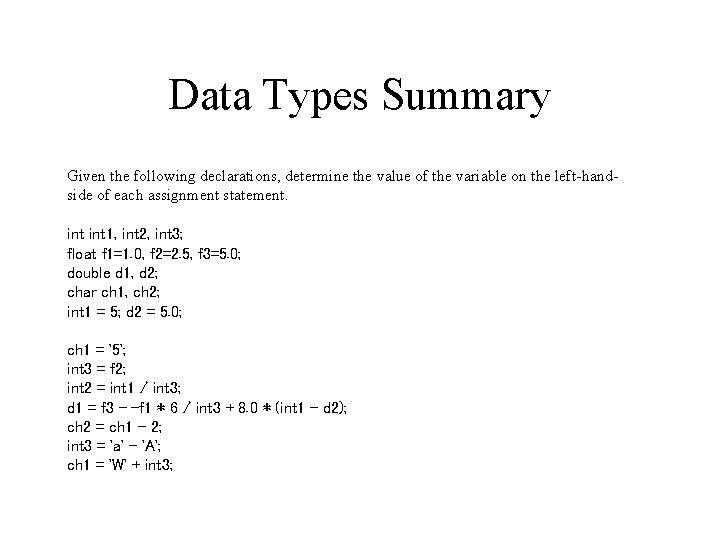
Data Types Summary Given the following declarations, determine the value of the variable on the left-handside of each assignment statement. int 1, int 2, int 3; float f 1=1. 0, f 2=2. 5, f 3=5. 0; double d 1, d 2; char ch 1, ch 2; int 1 = 5; d 2 = 5. 0; ch 1 = '5'; int 3 = f 2; int 2 = int 1 / int 3; d 1 = f 3 - -f 1 * 6 / int 3 + 8. 0 * (int 1 - d 2); ch 2 = ch 1 - 2; int 3 = 'a' - 'A'; ch 1 = 'W' + int 3;