include fstream include iostream using namespace std int
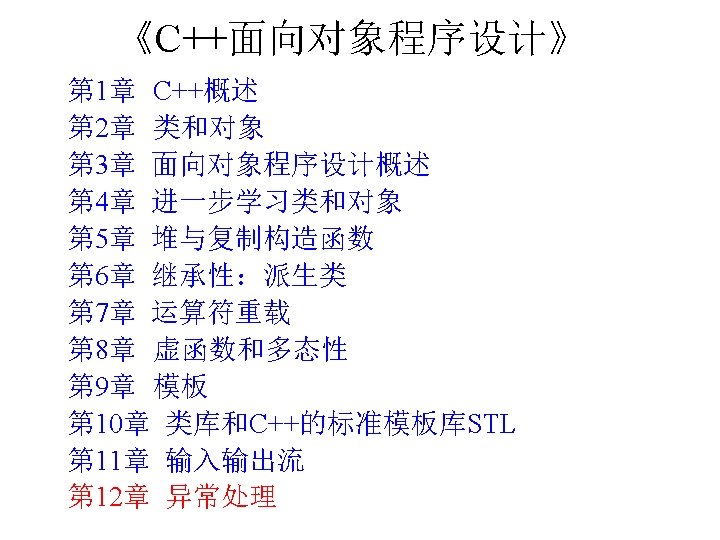
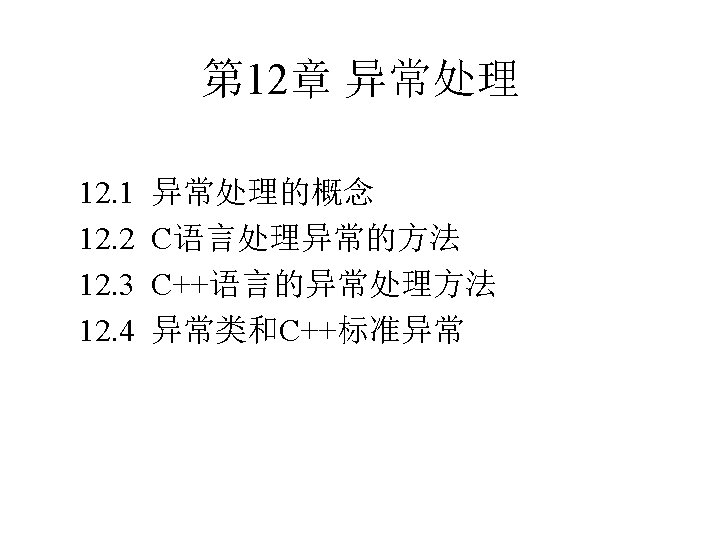
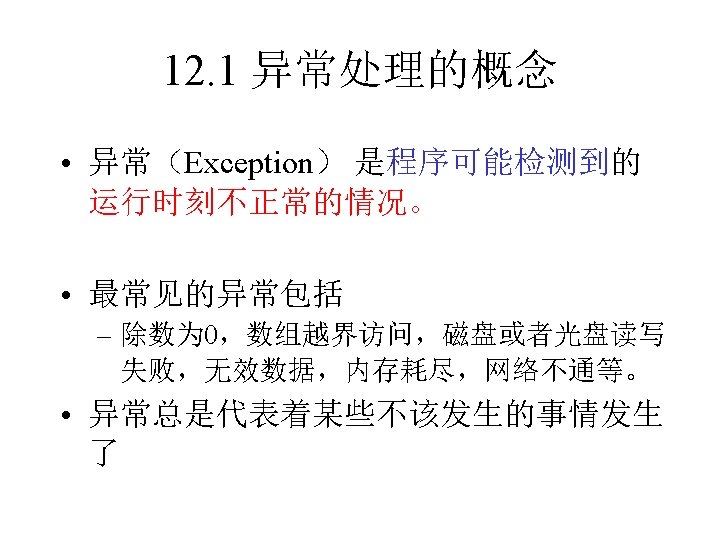
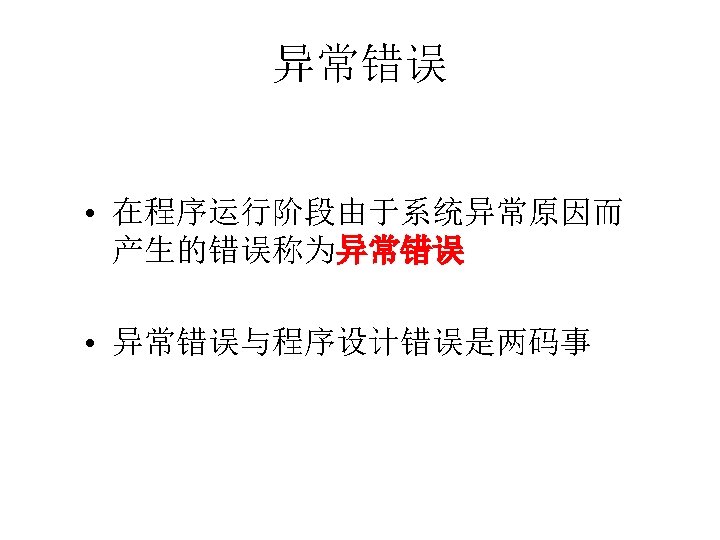
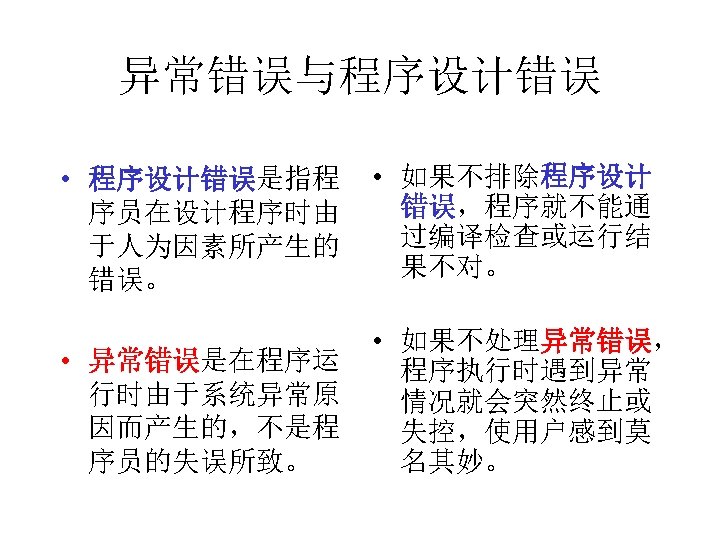
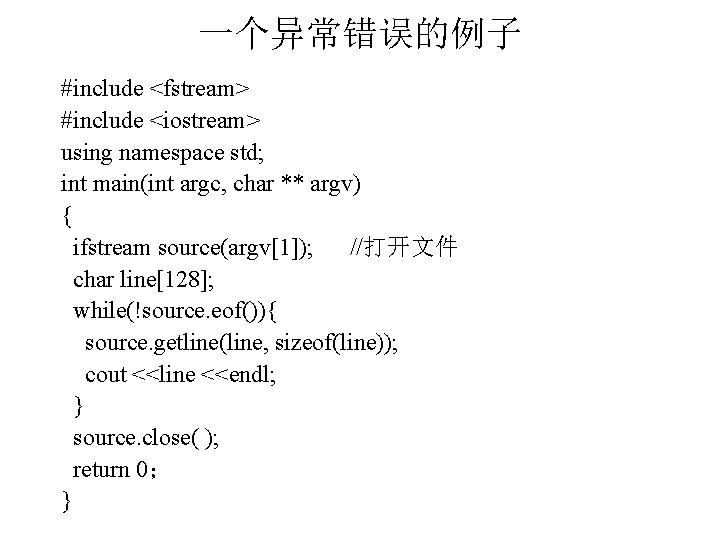
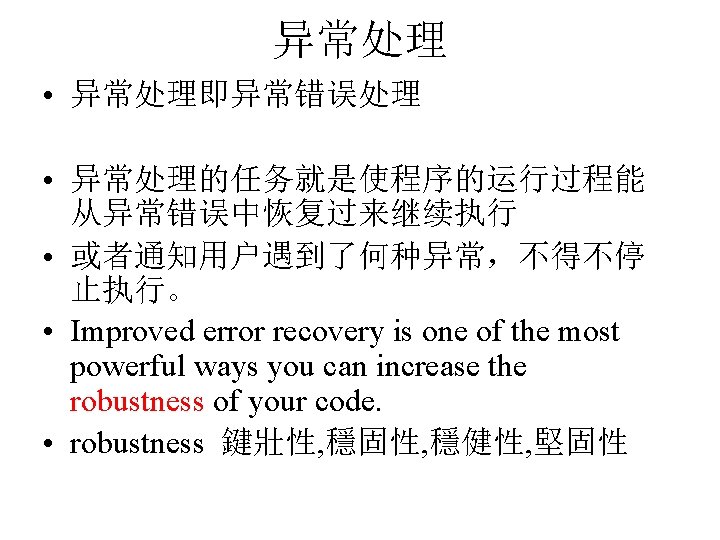
![异常处理的例子 int main(int argc, char ** argv) { ifstream source(argv[1]); //打开文件 char line[128]; if(source. 异常处理的例子 int main(int argc, char ** argv) { ifstream source(argv[1]); //打开文件 char line[128]; if(source.](https://slidetodoc.com/presentation_image_h/120c95e12a7ef0245e66475b7c050438/image-8.jpg)
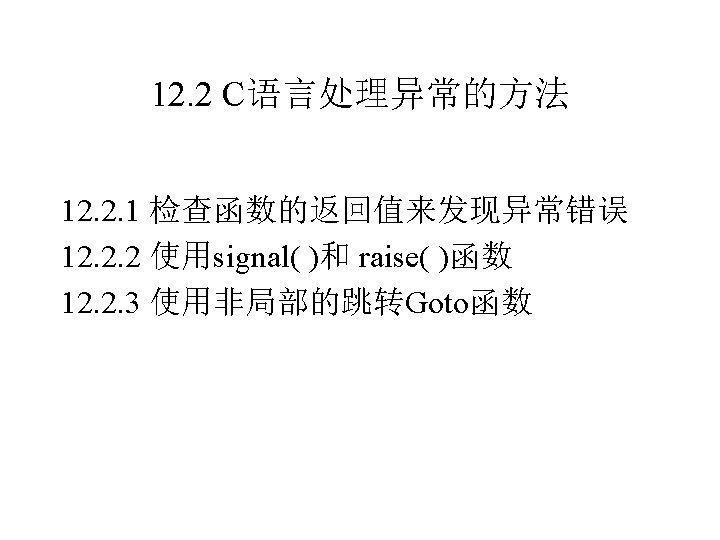
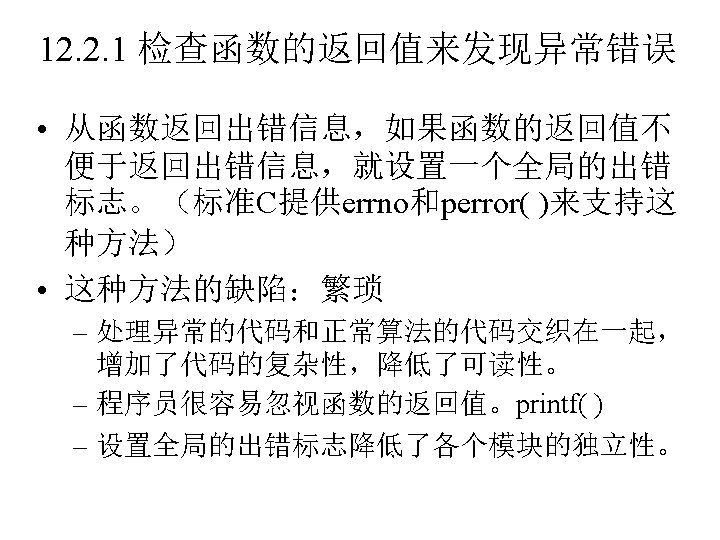
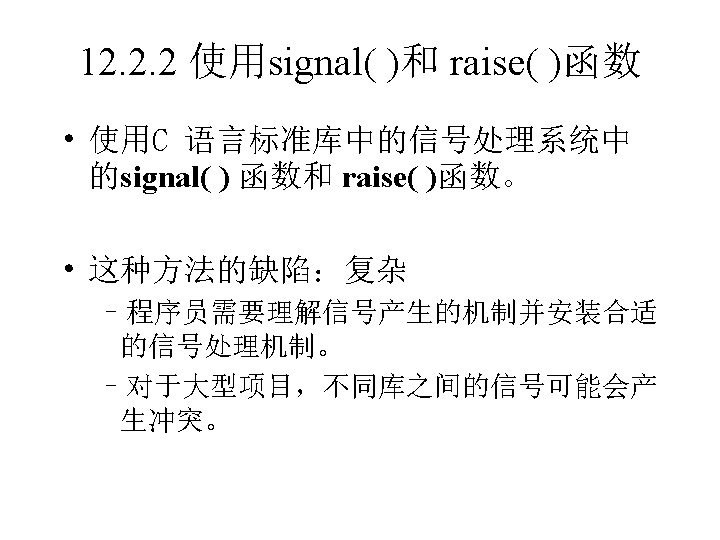
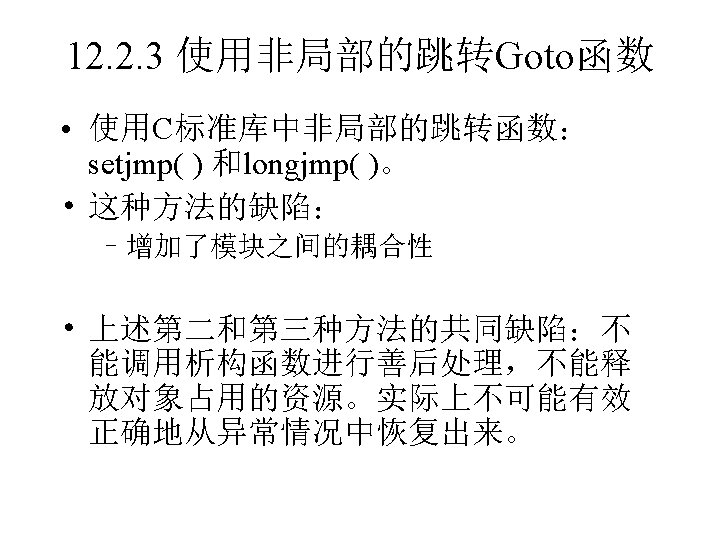
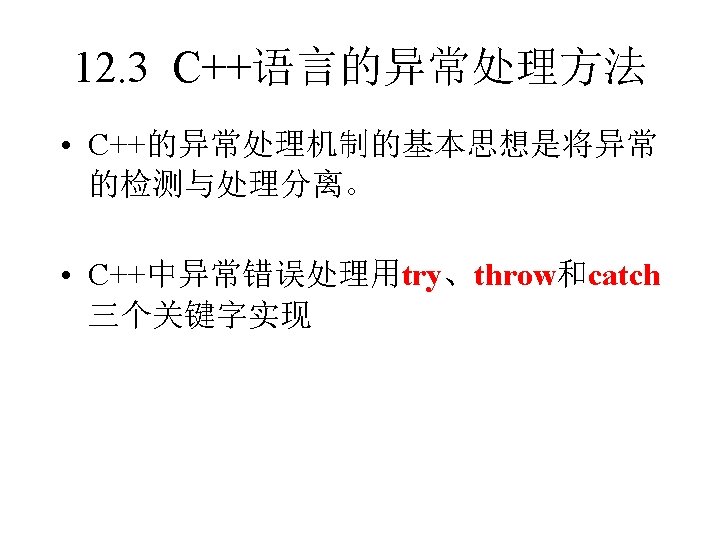
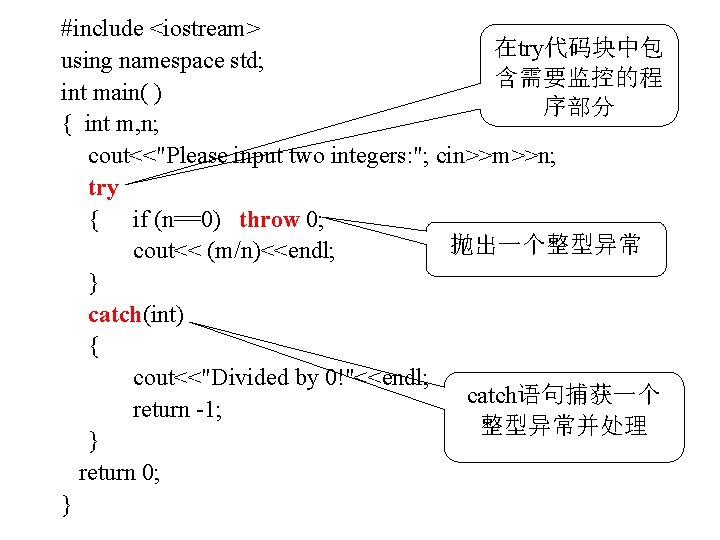
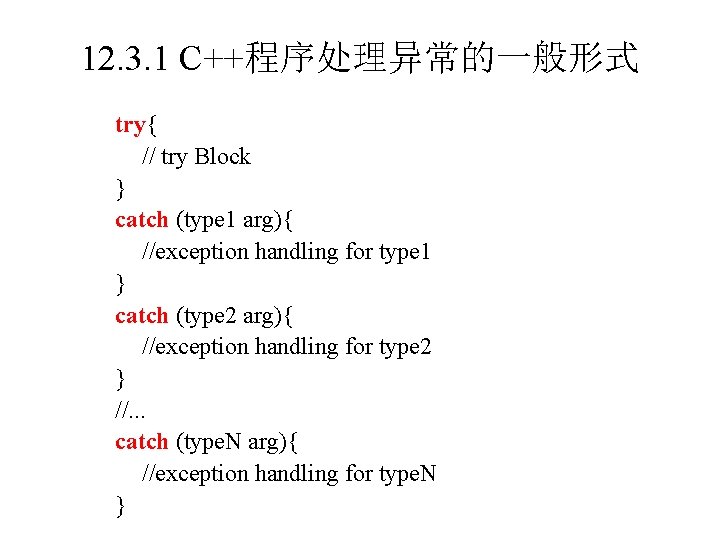
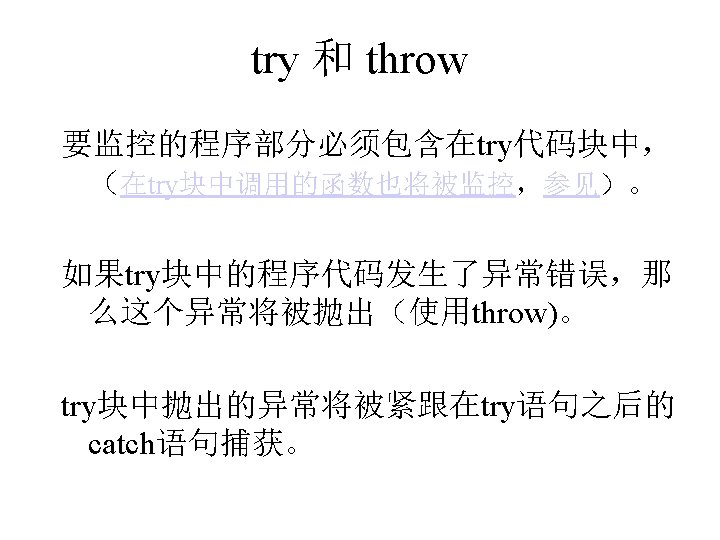
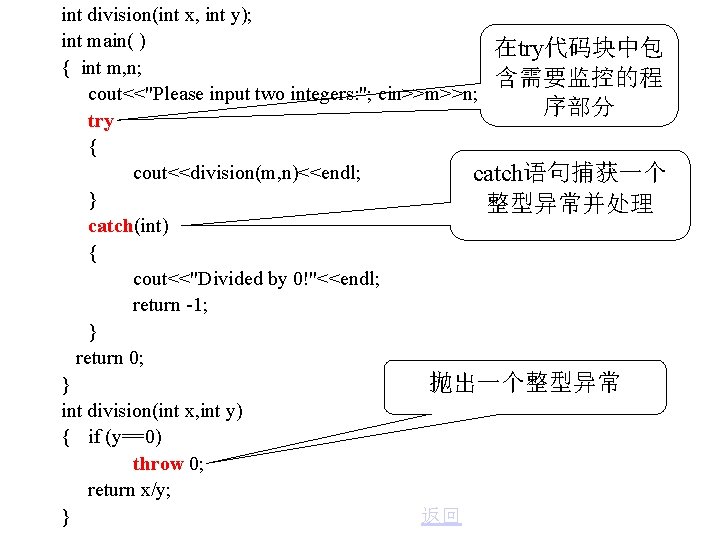
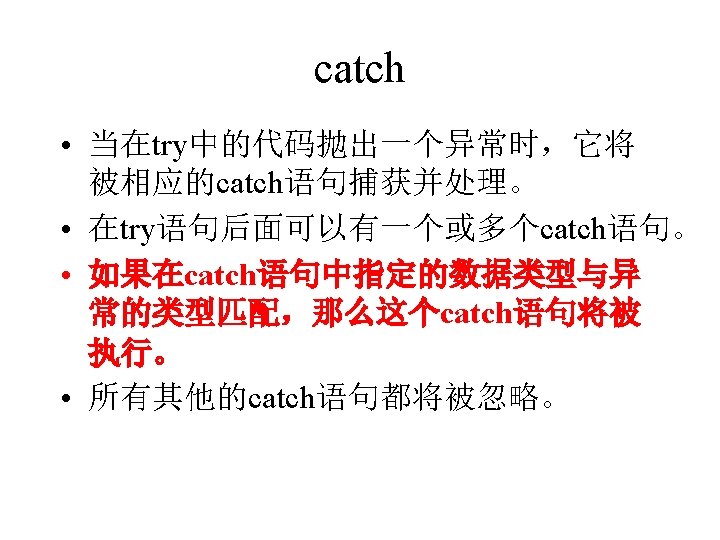
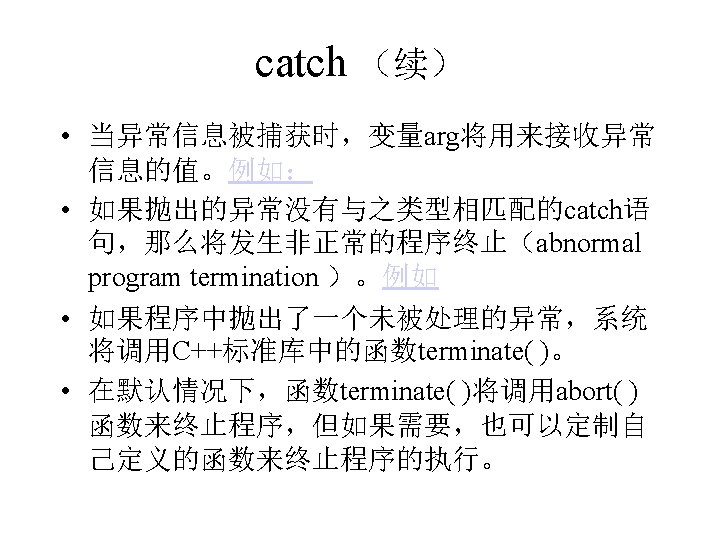
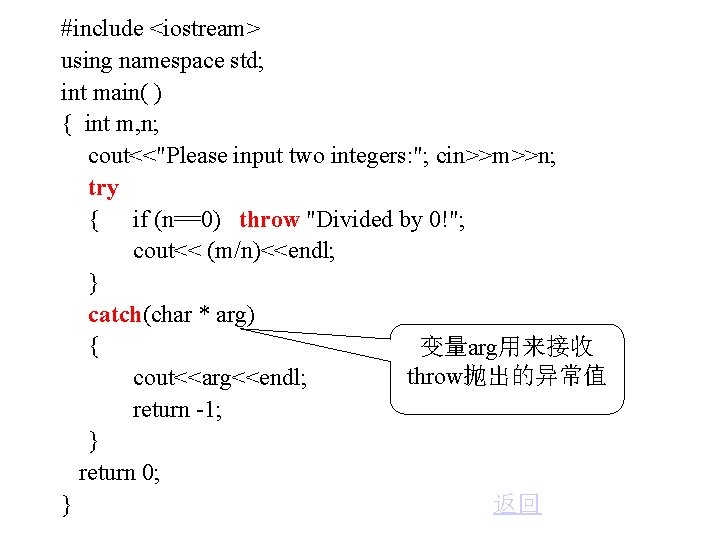
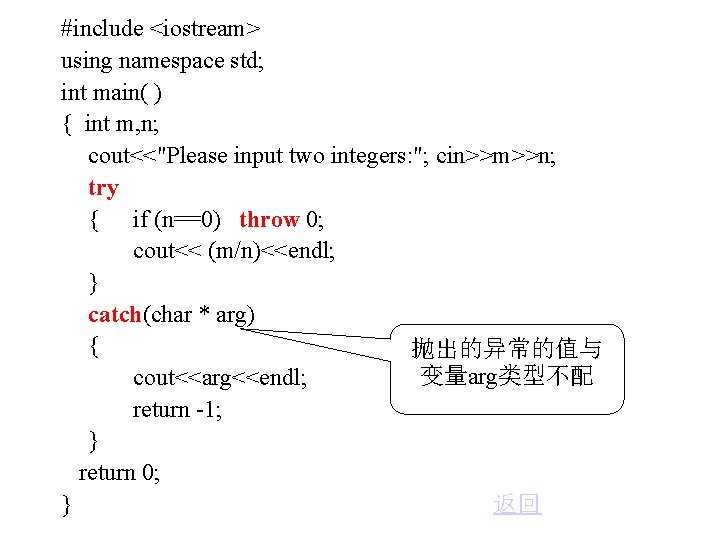
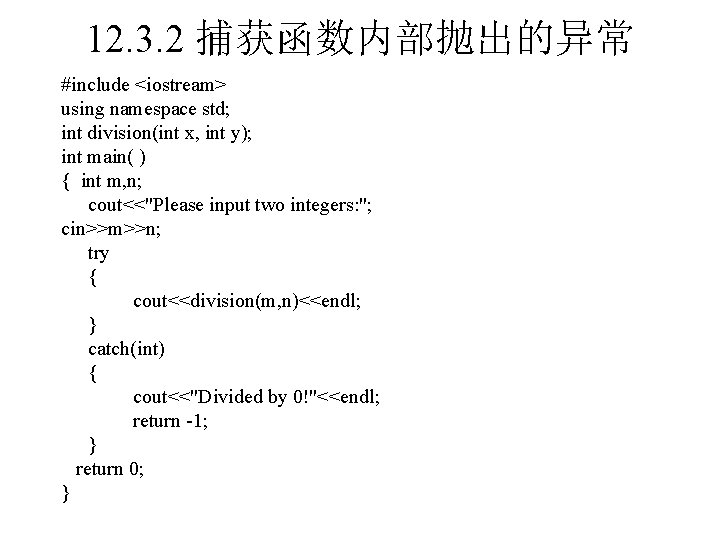
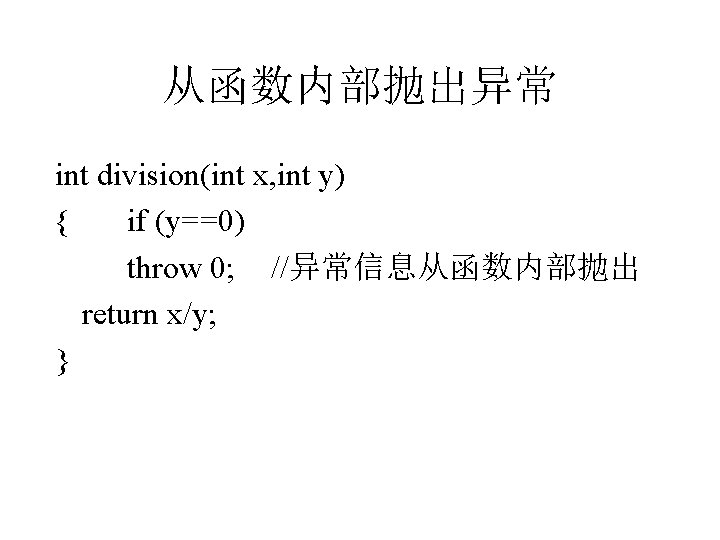
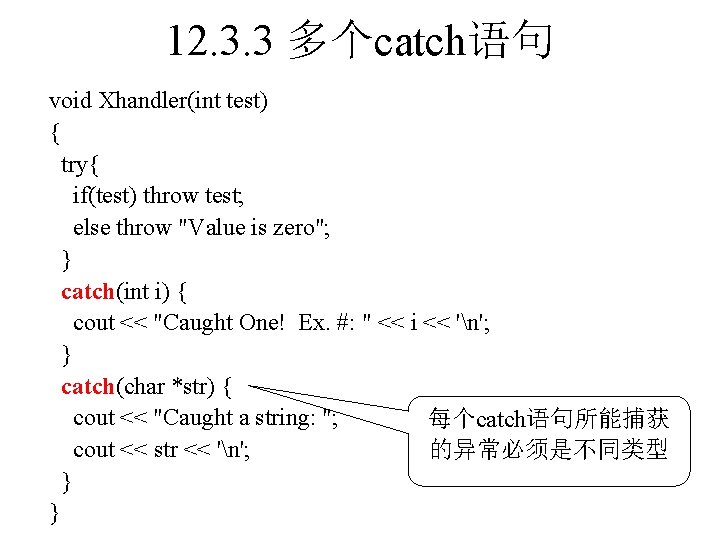
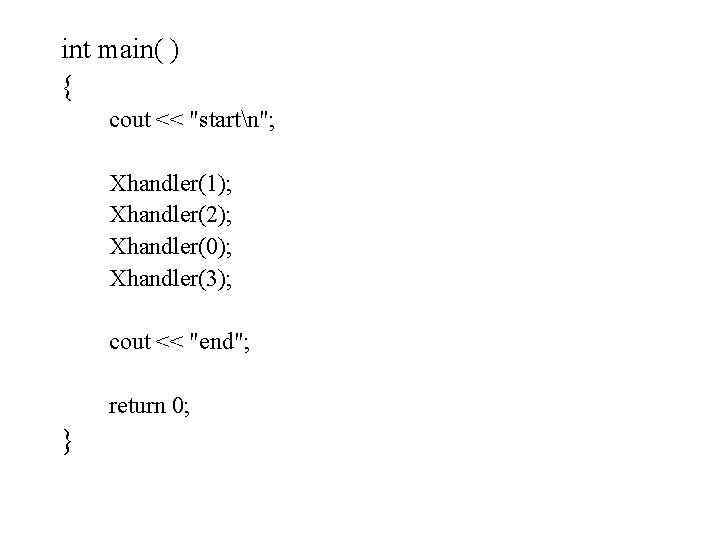
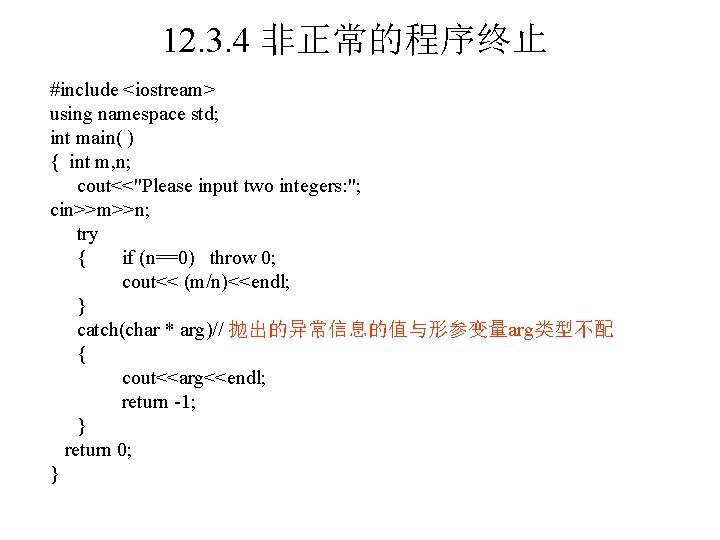
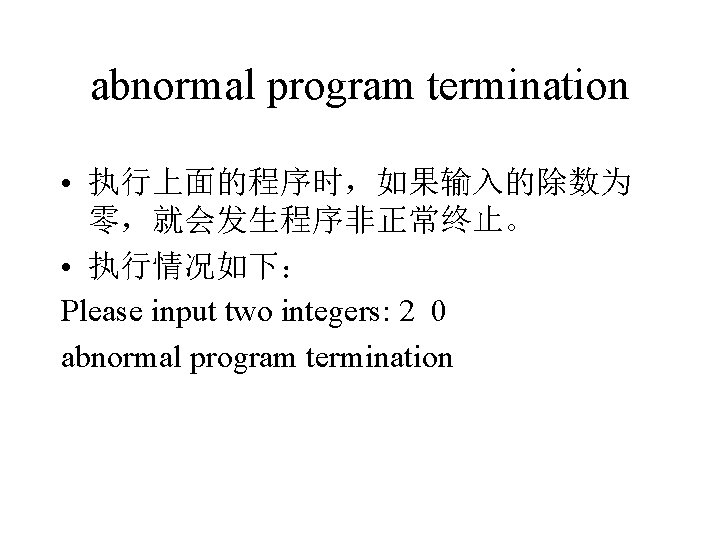
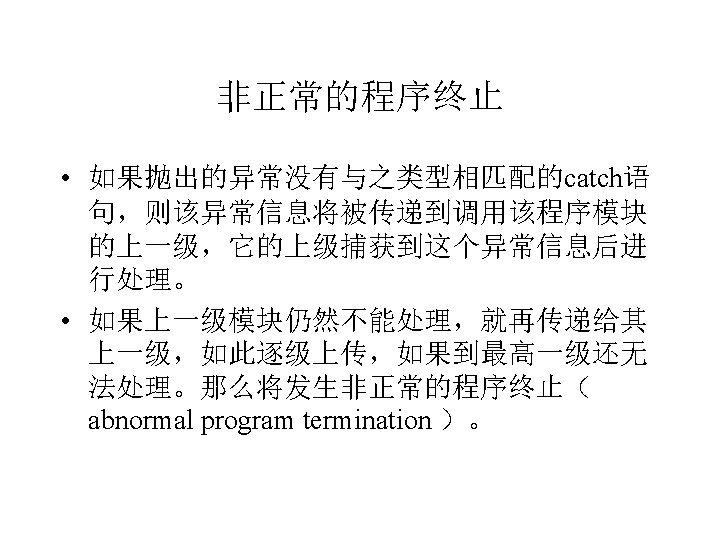
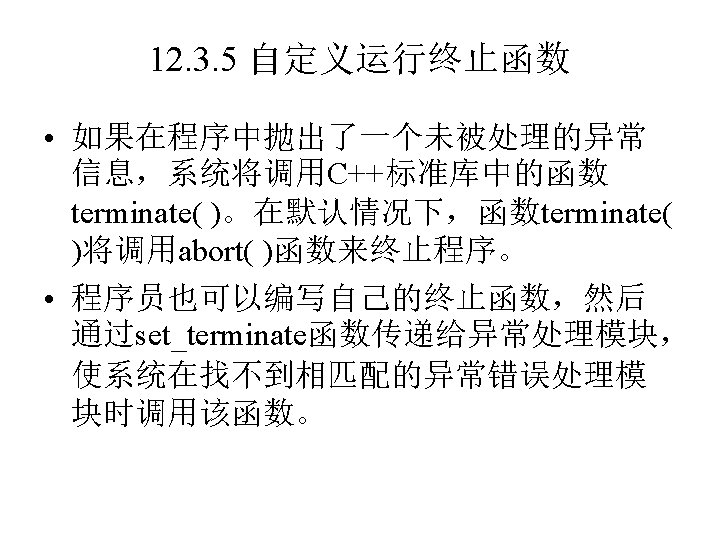
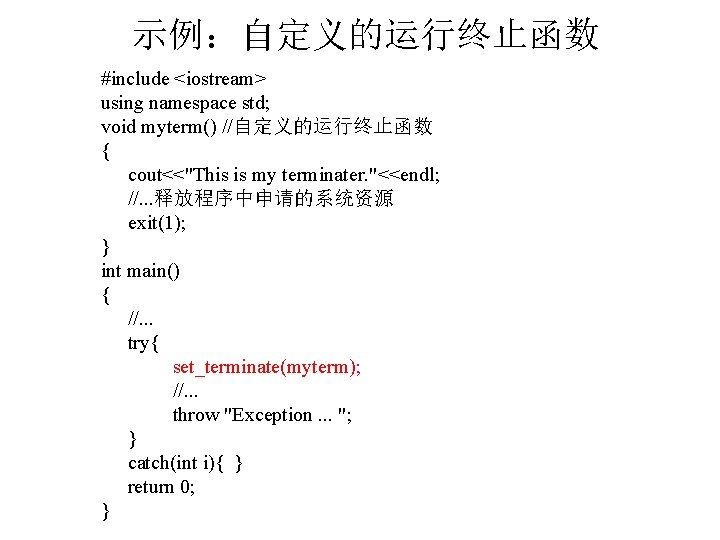
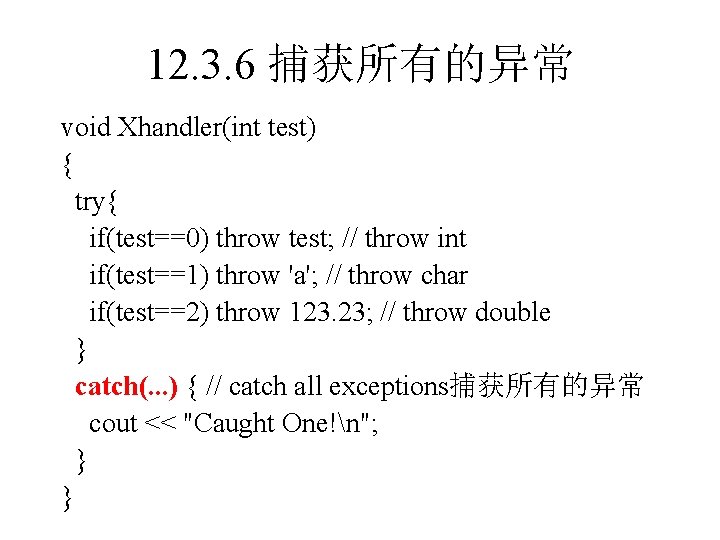
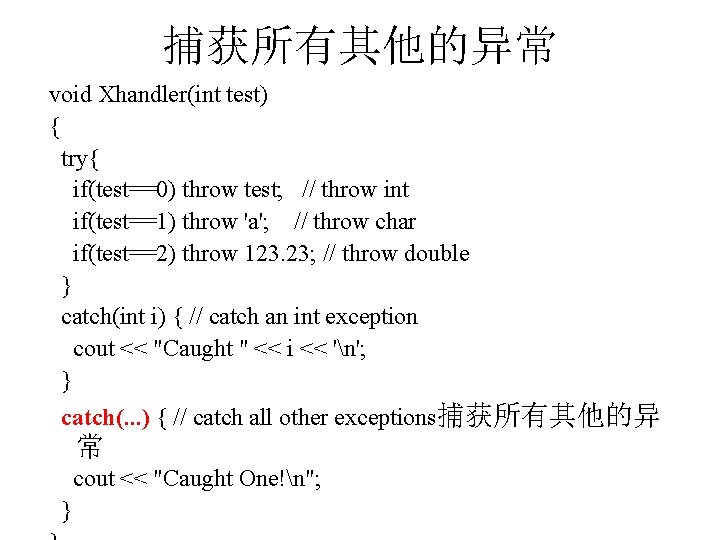
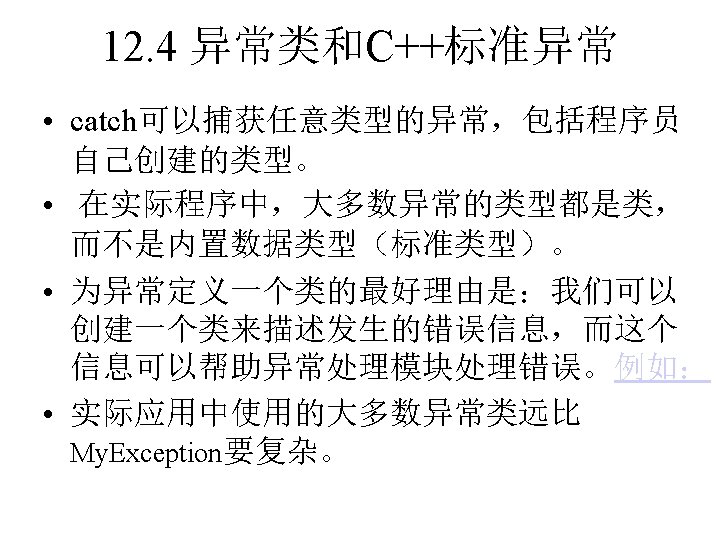
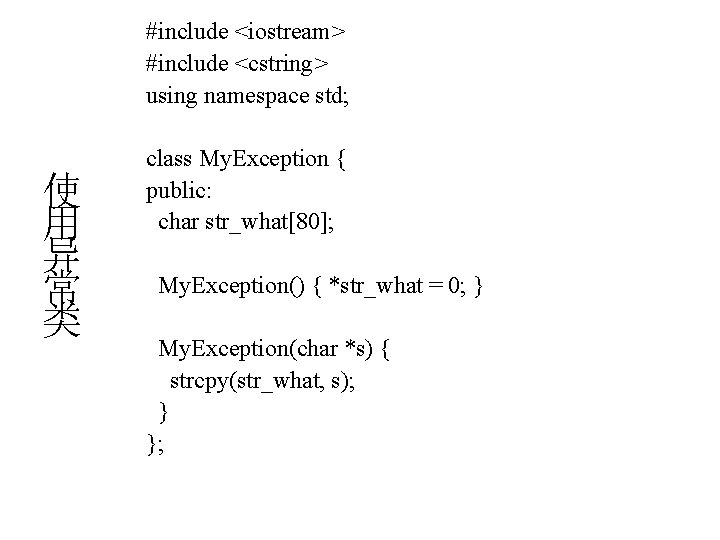
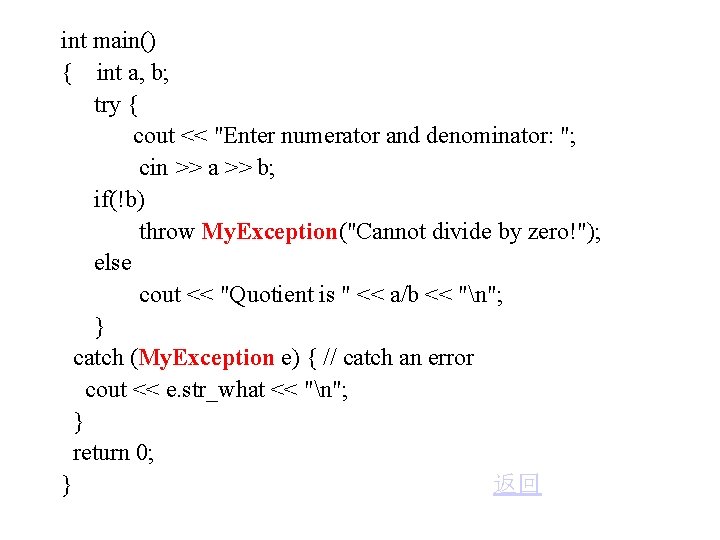
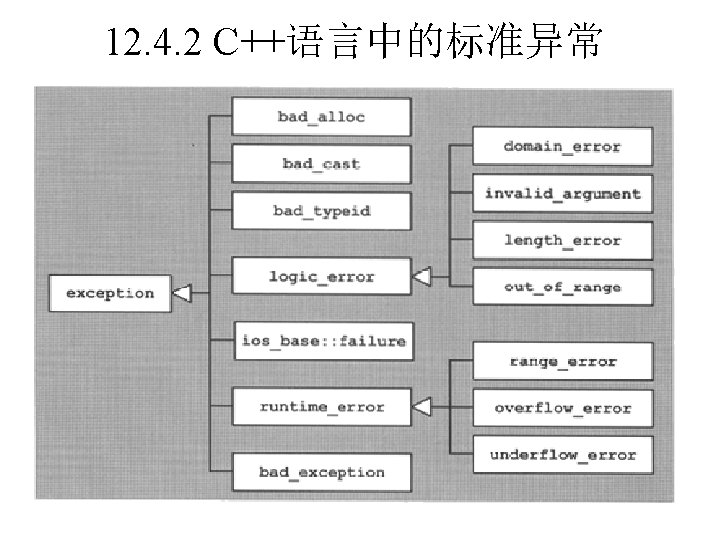
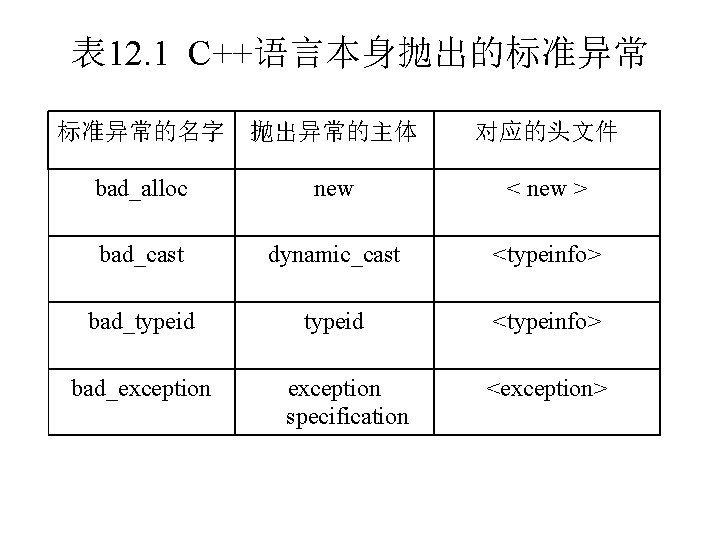
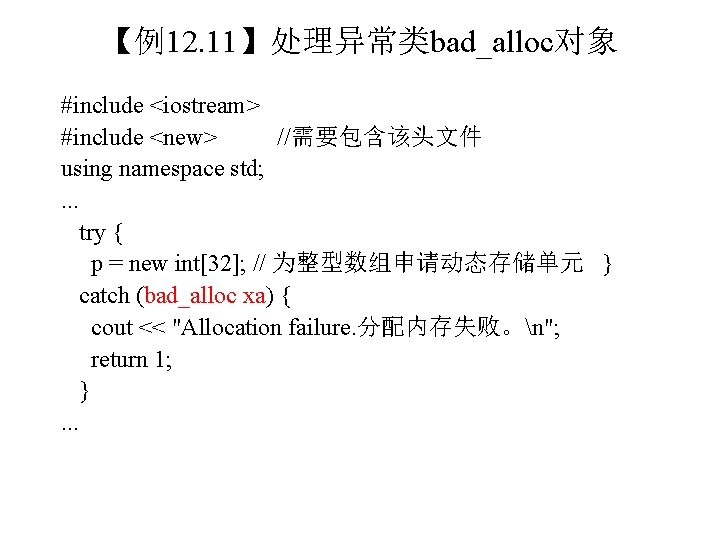
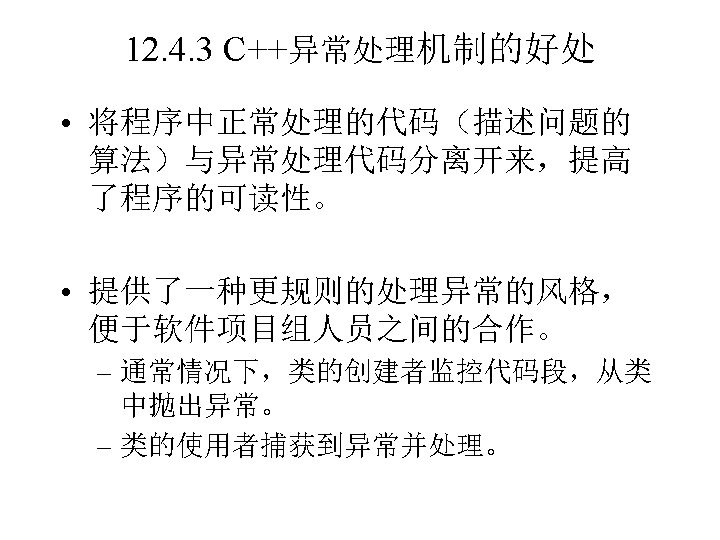
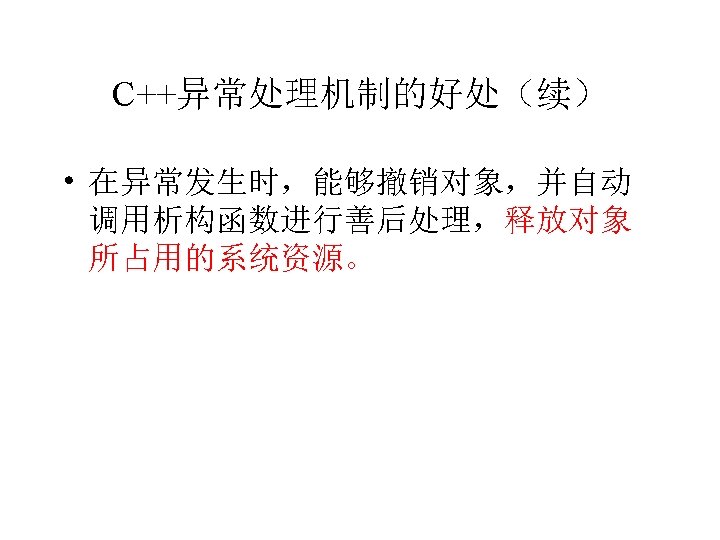
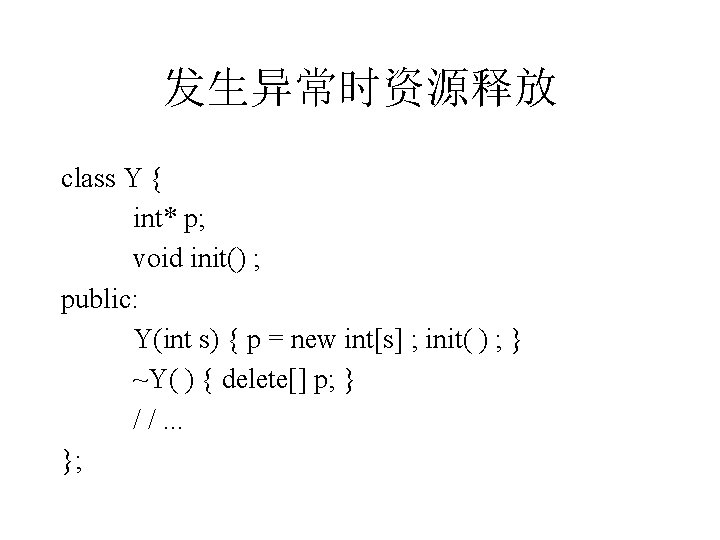
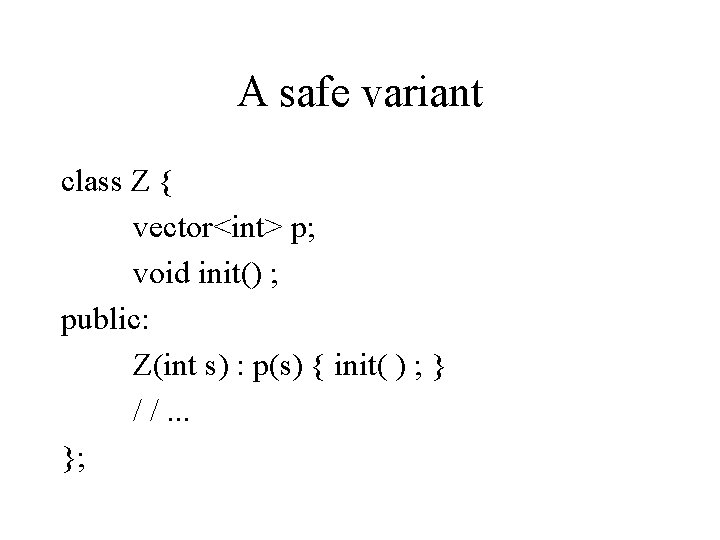
- Slides: 42
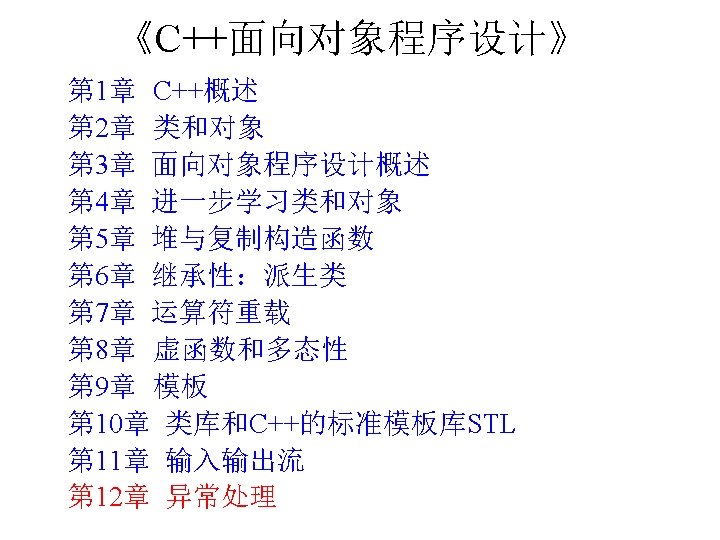
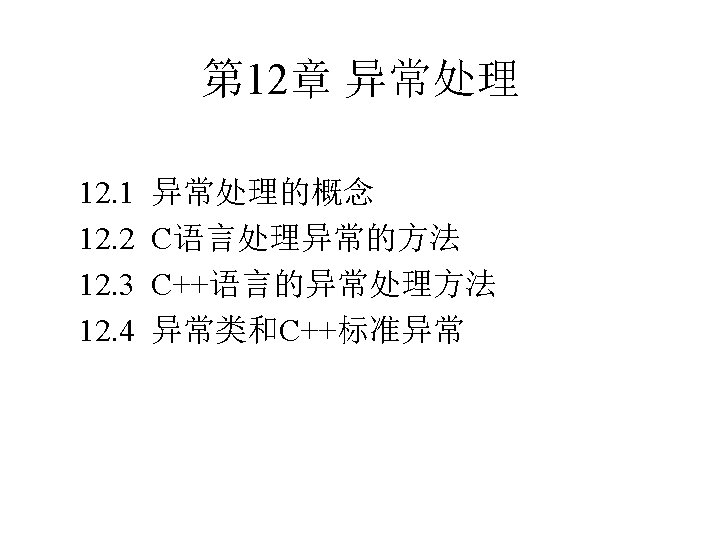
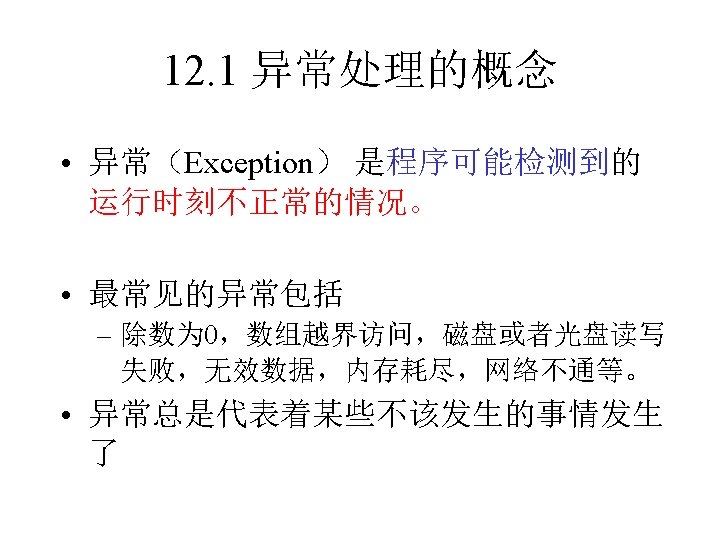
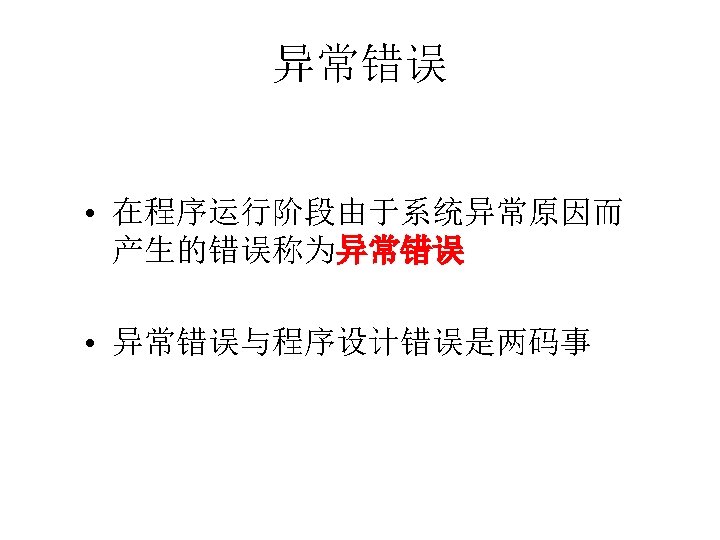
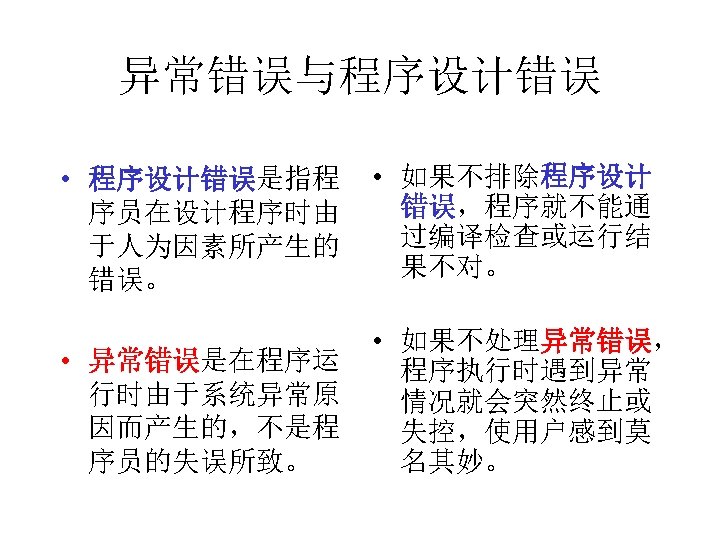
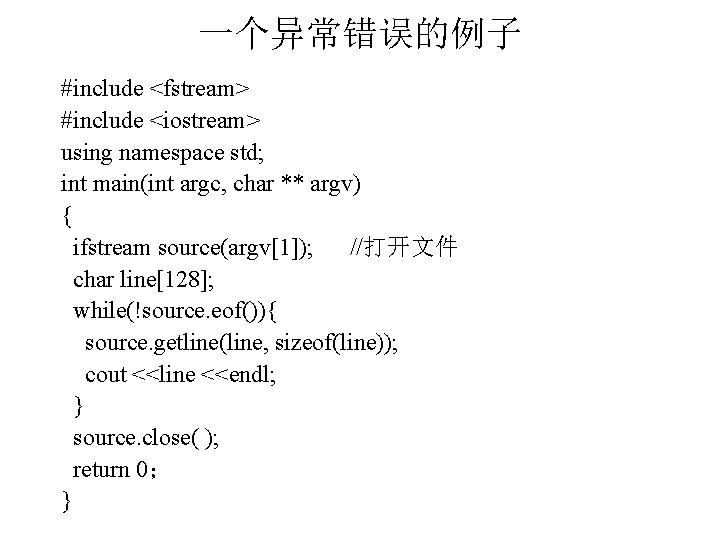
一个异常错误的例子 #include <fstream> #include <iostream> using namespace std; int main(int argc, char ** argv) { ifstream source(argv[1]); //打开文件 char line[128]; while(!source. eof()){ source. getline(line, sizeof(line)); cout <<line <<endl; } source. close( ); return 0; }
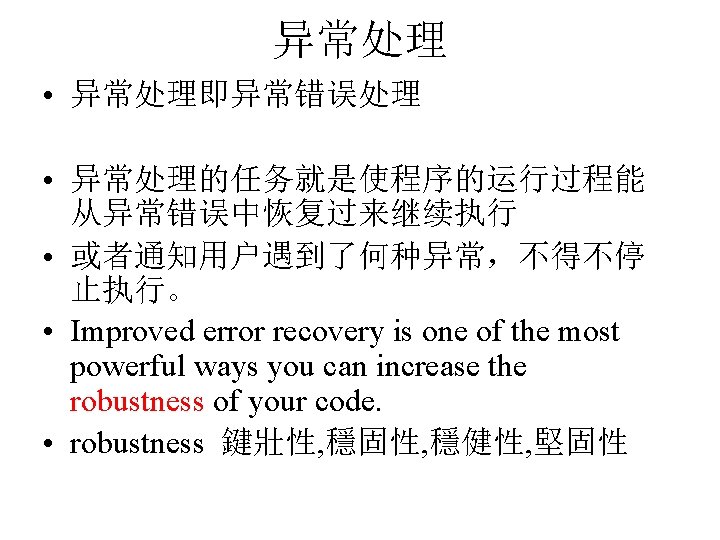
![异常处理的例子 int mainint argc char argv ifstream sourceargv1 打开文件 char line128 ifsource 异常处理的例子 int main(int argc, char ** argv) { ifstream source(argv[1]); //打开文件 char line[128]; if(source.](https://slidetodoc.com/presentation_image_h/120c95e12a7ef0245e66475b7c050438/image-8.jpg)
异常处理的例子 int main(int argc, char ** argv) { ifstream source(argv[1]); //打开文件 char line[128]; if(source. fail( )){ cout <<"error opening the file "<<argv[1] <<endl; exit(1); } while(!source. eof()){ source. getline(line, sizeof(line)); cout <<line <<endl; } source. close(); return 0; } 异常处理代码
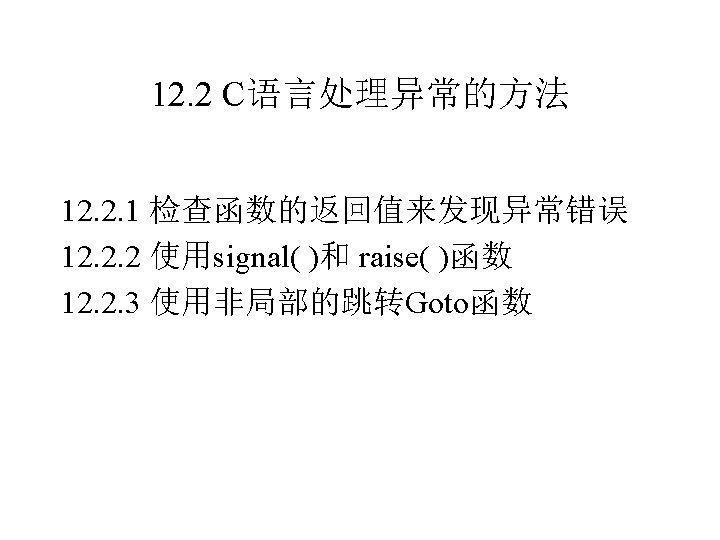
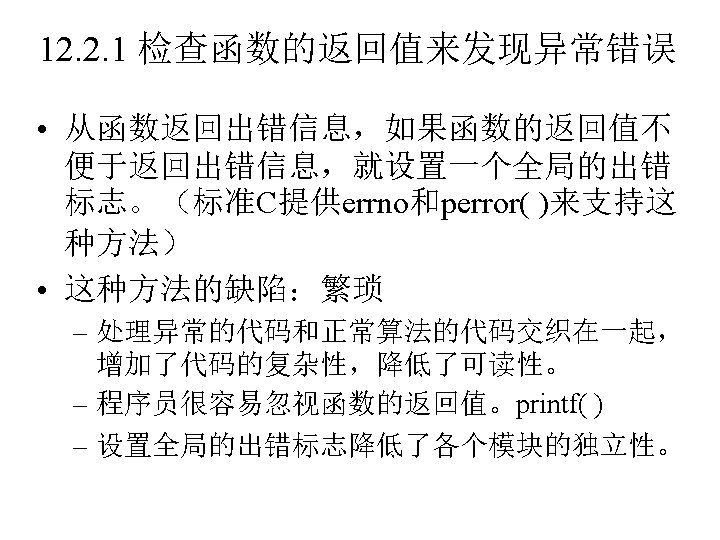
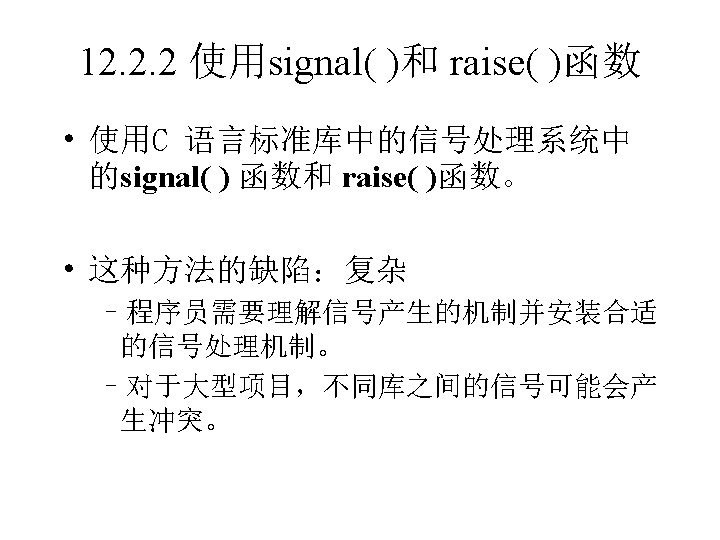
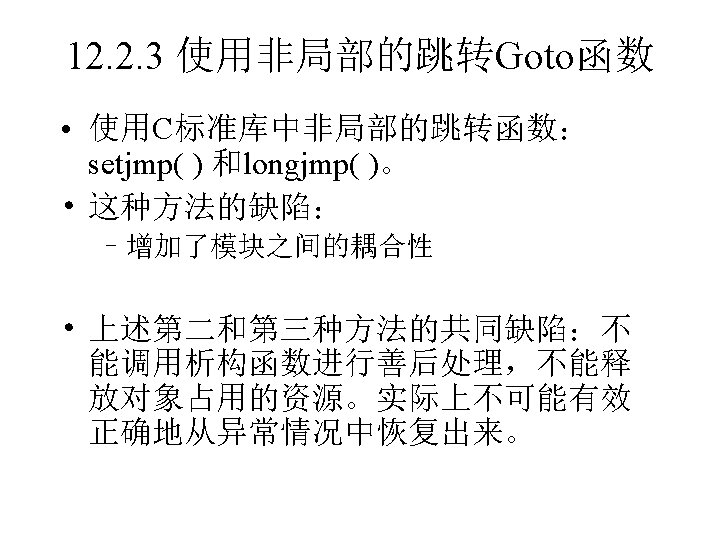
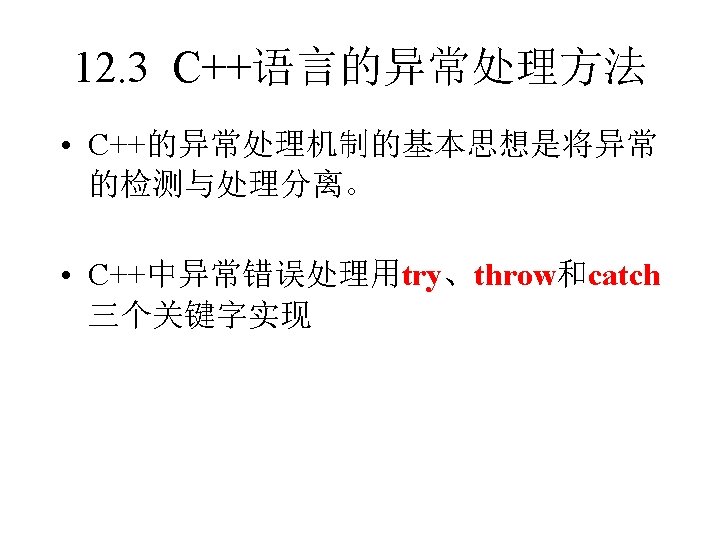
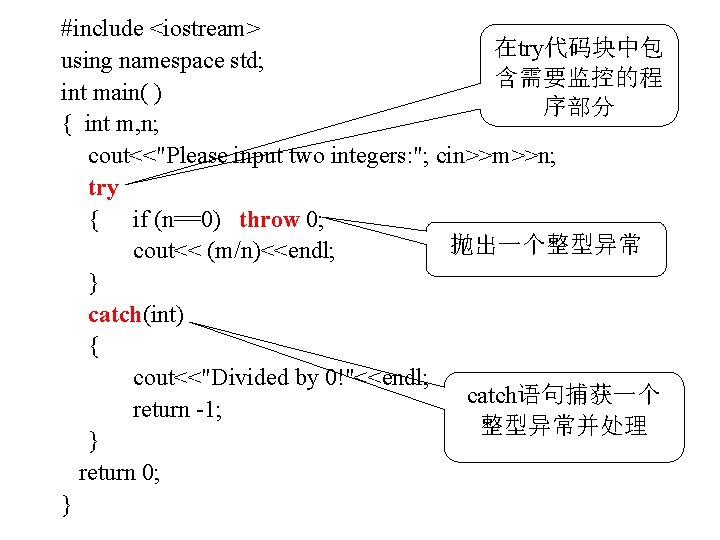
#include <iostream> 在try代码块中包 一个异常处理的简单例子 using namespace std; 含需要监控的程 int main( ) 序部分 { int m, n; cout<<"Please input two integers: "; cin>>m>>n; try { if (n==0) throw 0; 抛出一个整型异常 cout<< (m/n)<<endl; } catch(int) { cout<<"Divided by 0!"<<endl; catch语句捕获一个 return -1; 整型异常并处理 } return 0; }
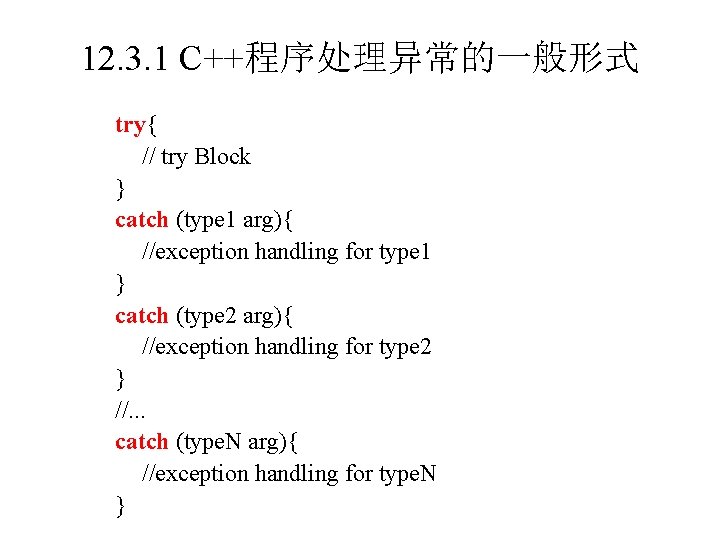
12. 3. 1 C++程序处理异常的一般形式 try{ // try Block } catch (type 1 arg){ //exception handling for type 1 } catch (type 2 arg){ //exception handling for type 2 } //. . . catch (type. N arg){ //exception handling for type. N }
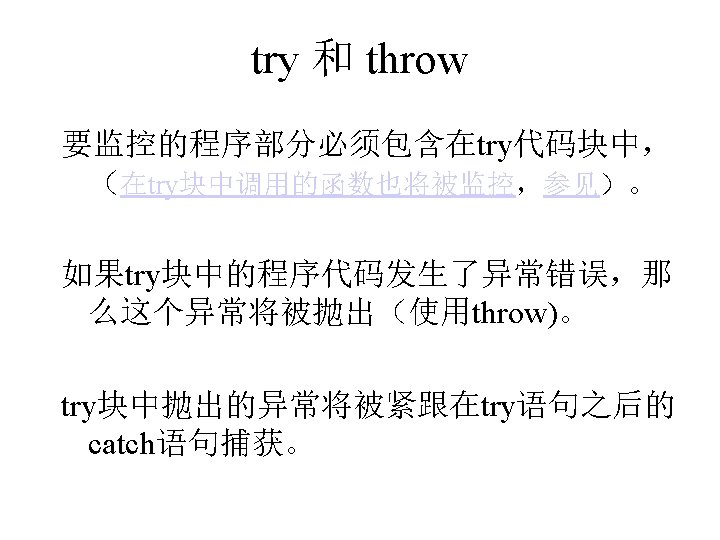
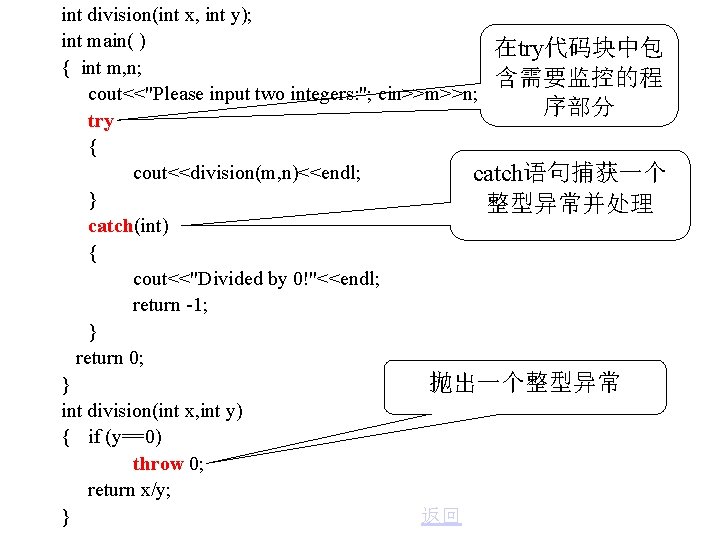
int division(int x, int y); int main( ) 在try代码块中包 { int m, n; 含需要监控的程 cout<<"Please input two integers: "; cin>>m>>n; 序部分 try { cout<<division(m, n)<<endl; catch语句捕获一个 } 整型异常并处理 catch(int) { cout<<"Divided by 0!"<<endl; return -1; } return 0; } 抛出一个整型异常 int division(int x, int y) { if (y==0) throw 0; return x/y; } 返回 另一个异常处理的简单例子
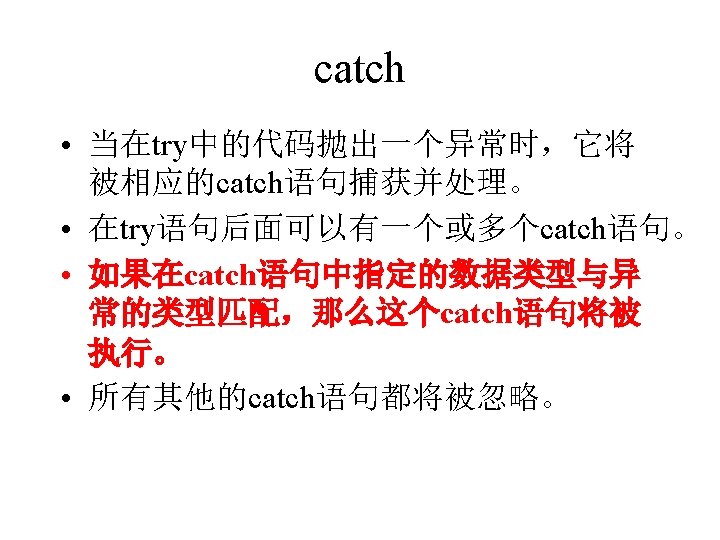
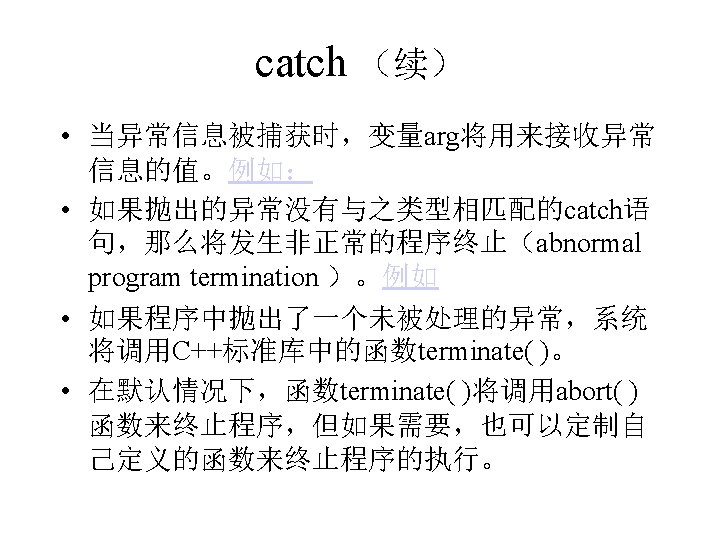
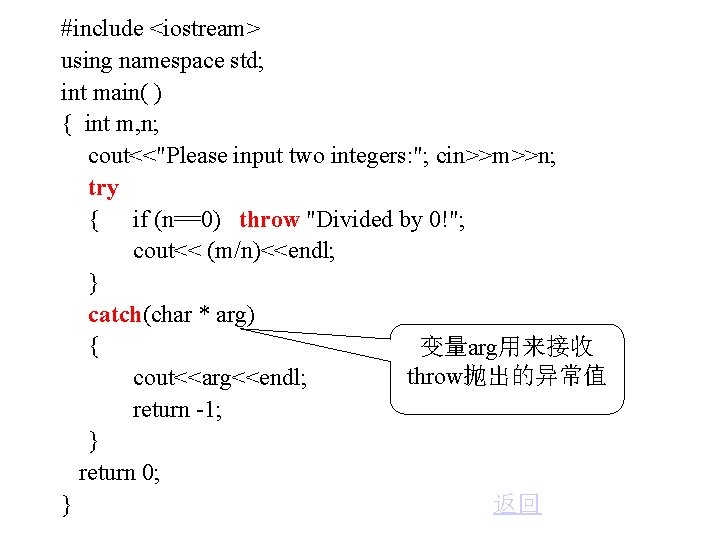
#include <iostream> 变量arg用来接收异常的值 using namespace std; int main( ) { int m, n; cout<<"Please input two integers: "; cin>>m>>n; try { if (n==0) throw "Divided by 0!"; cout<< (m/n)<<endl; } catch(char * arg) { 变量arg用来接收 throw抛出的异常值 cout<<arg<<endl; return -1; } return 0; } 返回
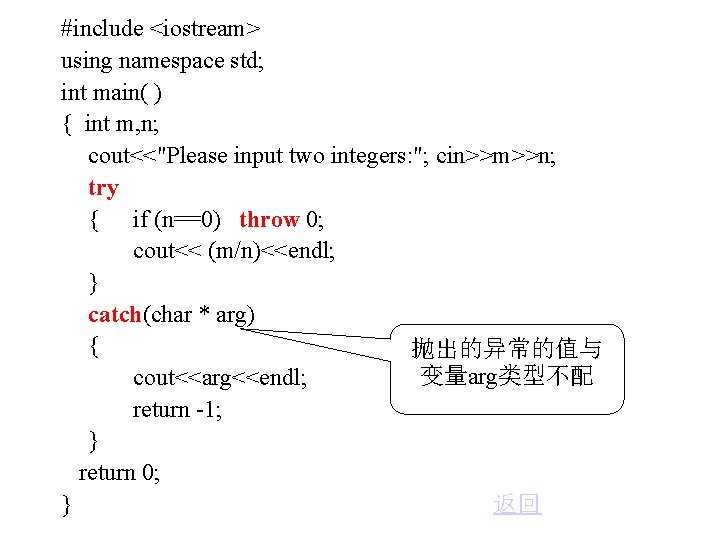
#include <iostream> 抛出的异常的值与变量arg类型不配 using namespace std; int main( ) { int m, n; cout<<"Please input two integers: "; cin>>m>>n; try { if (n==0) throw 0; cout<< (m/n)<<endl; } catch(char * arg) { 抛出的异常的值与 变量arg类型不配 cout<<arg<<endl; return -1; } return 0; } 返回
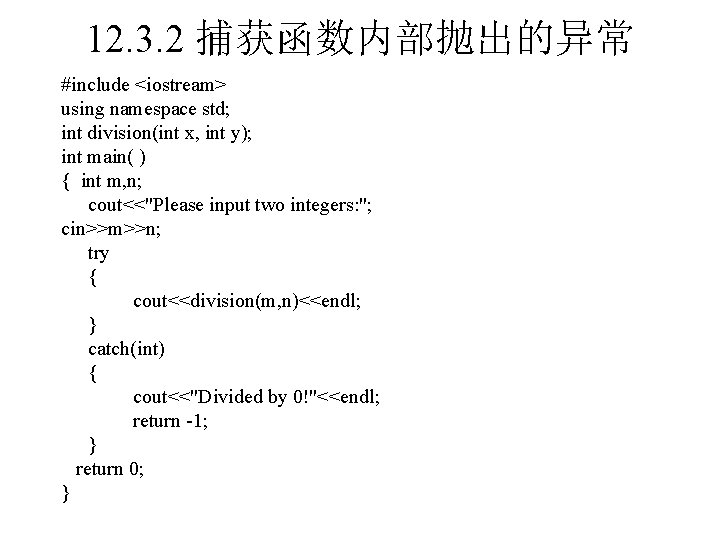
12. 3. 2 捕获函数内部抛出的异常 #include <iostream> using namespace std; int division(int x, int y); int main( ) { int m, n; cout<<"Please input two integers: "; cin>>m>>n; try { cout<<division(m, n)<<endl; } catch(int) { cout<<"Divided by 0!"<<endl; return -1; } return 0; }
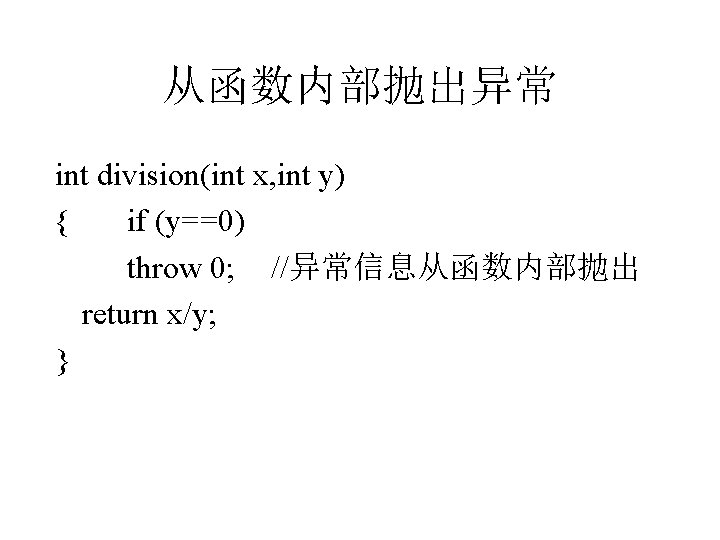
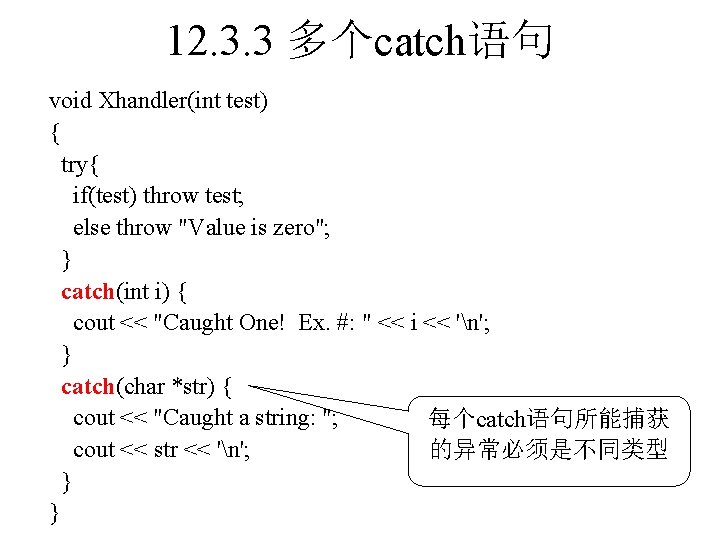
12. 3. 3 多个catch语句 void Xhandler(int test) { try{ if(test) throw test; else throw "Value is zero"; } catch(int i) { cout << "Caught One! Ex. #: " << i << 'n'; } catch(char *str) { cout << "Caught a string: "; 每个catch语句所能捕获 cout << str << 'n'; 的异常必须是不同类型 } }
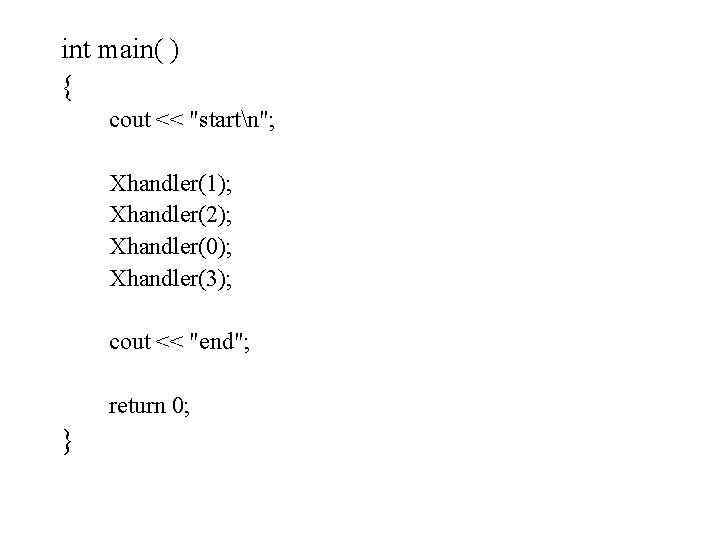
int main( ) { 使用多个catch语句(续) cout << "startn"; Xhandler(1); Xhandler(2); Xhandler(0); Xhandler(3); cout << "end"; return 0; }
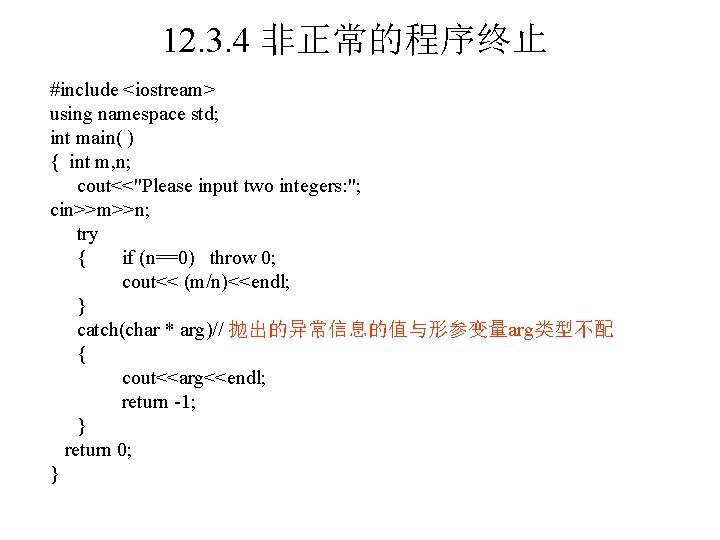
12. 3. 4 非正常的程序终止 #include <iostream> using namespace std; int main( ) { int m, n; cout<<"Please input two integers: "; cin>>m>>n; try { if (n==0) throw 0; cout<< (m/n)<<endl; } catch(char * arg)// 抛出的异常信息的值与形参变量arg类型不配 { cout<<arg<<endl; return -1; } return 0; }
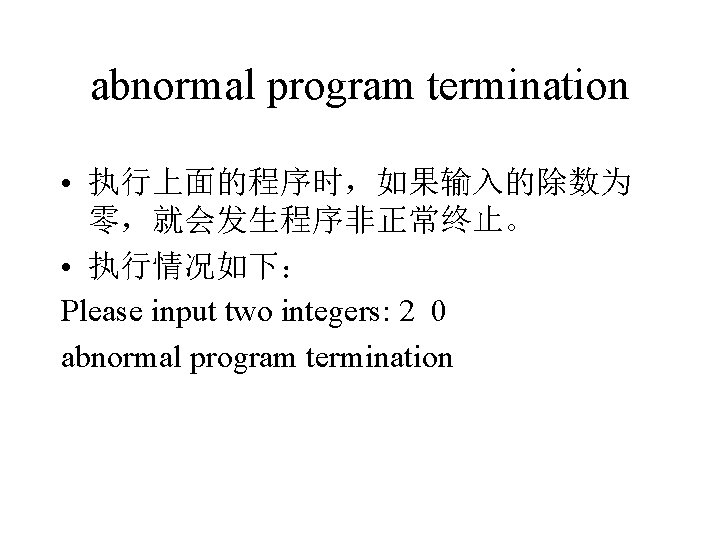
abnormal program termination • 执行上面的程序时,如果输入的除数为 零,就会发生程序非正常终止。 • 执行情况如下: Please input two integers: 2 0 abnormal program termination
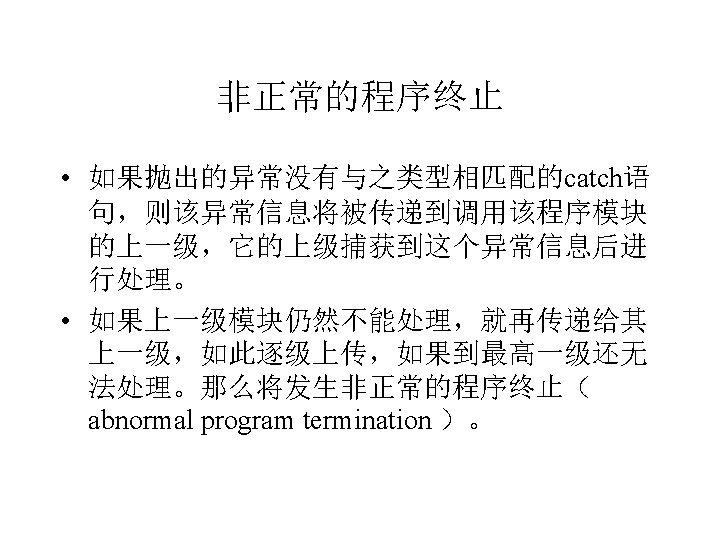
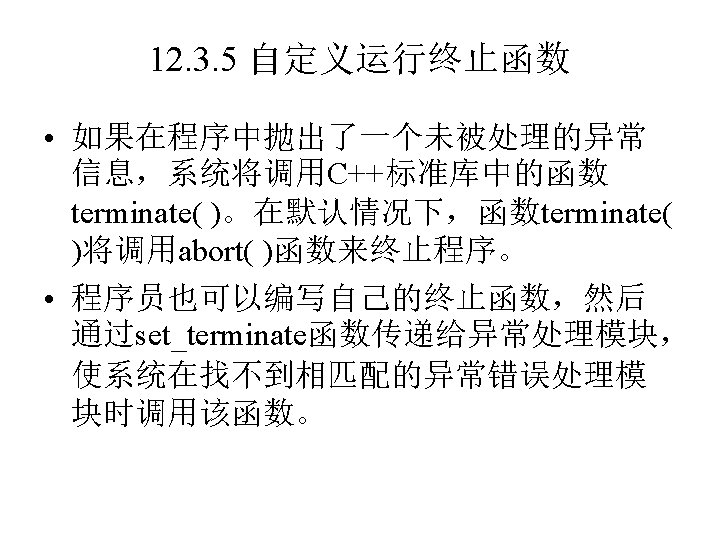
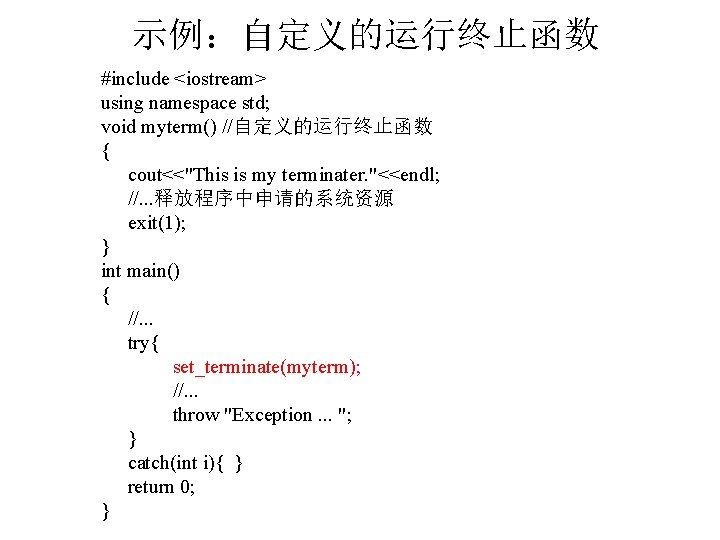
示例:自定义的运行终止函数 #include <iostream> using namespace std; void myterm() //自定义的运行终止函数 { cout<<"This is my terminater. "<<endl; //. . . 释放程序中申请的系统资源 exit(1); } int main() { //. . . try{ set_terminate(myterm); //. . . throw "Exception. . . "; } catch(int i){ } return 0; }
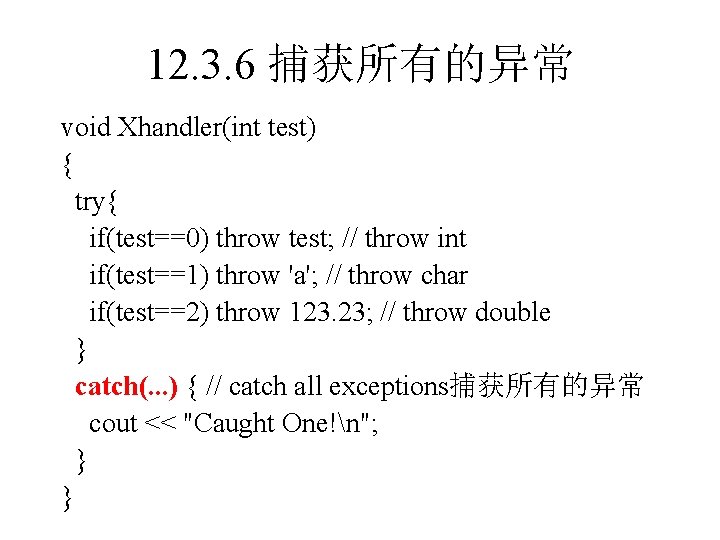
12. 3. 6 捕获所有的异常 void Xhandler(int test) { try{ if(test==0) throw test; // throw int if(test==1) throw 'a'; // throw char if(test==2) throw 123. 23; // throw double } catch(. . . ) { // catch all exceptions捕获所有的异常 cout << "Caught One!n"; } }
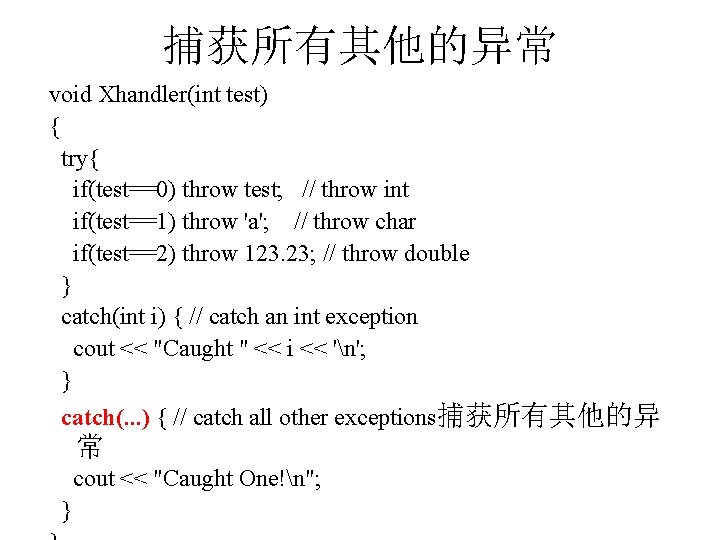
捕获所有其他的异常 void Xhandler(int test) { try{ if(test==0) throw test; // throw int if(test==1) throw 'a'; // throw char if(test==2) throw 123. 23; // throw double } catch(int i) { // catch an int exception cout << "Caught " << i << 'n'; } catch(. . . ) { // catch all other exceptions捕获所有其他的异 常 cout << "Caught One!n"; }
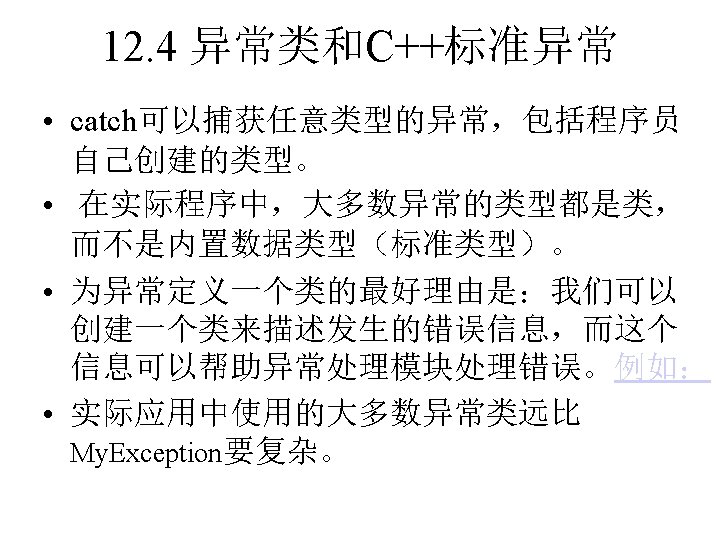
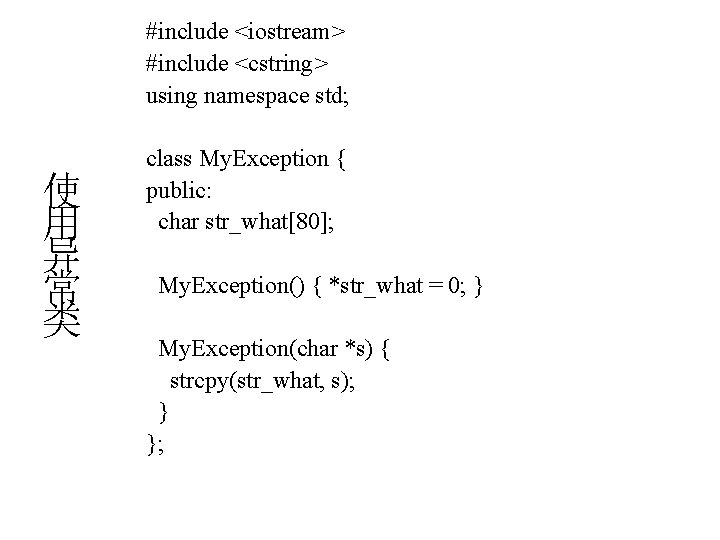
#include <iostream> #include <cstring> using namespace std; 12. 4. 1 使用异常类 使 用 异 常 类 class My. Exception { public: char str_what[80]; My. Exception() { *str_what = 0; } My. Exception(char *s) { strcpy(str_what, s); } };
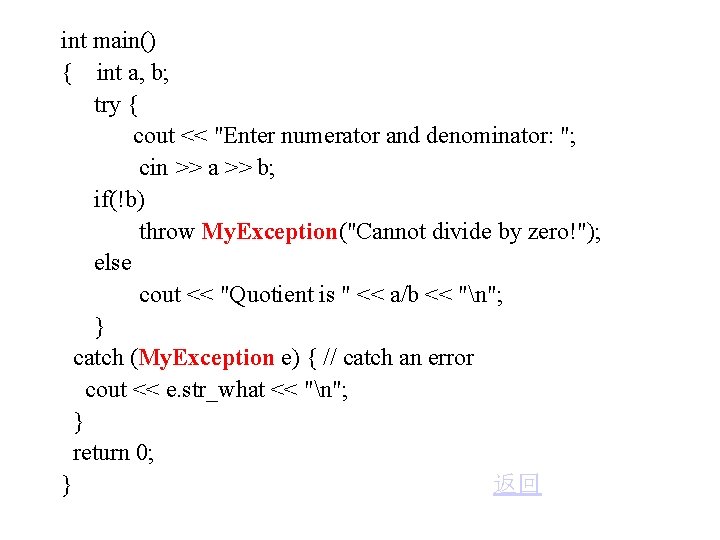
int main() { int a, b; try { cout << "Enter numerator and denominator: "; cin >> a >> b; if(!b) throw My. Exception("Cannot divide by zero!"); else cout << "Quotient is " << a/b << "n"; } catch (My. Exception e) { // catch an error cout << e. str_what << "n"; } return 0; } 返回 使用异常类(续)
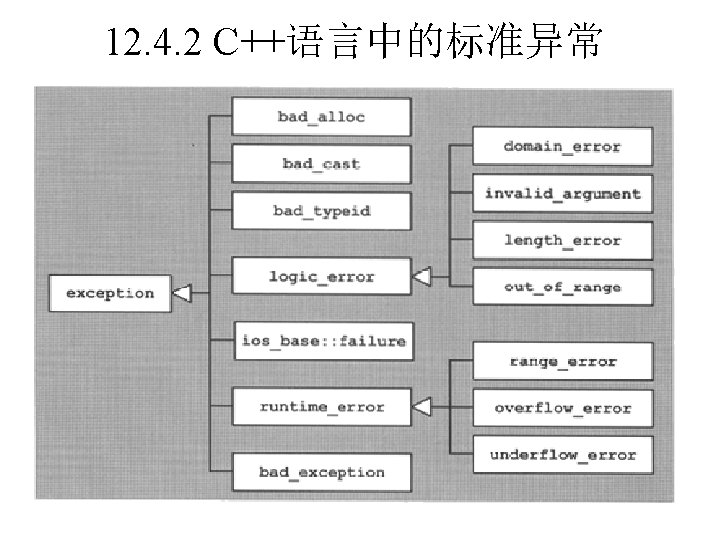
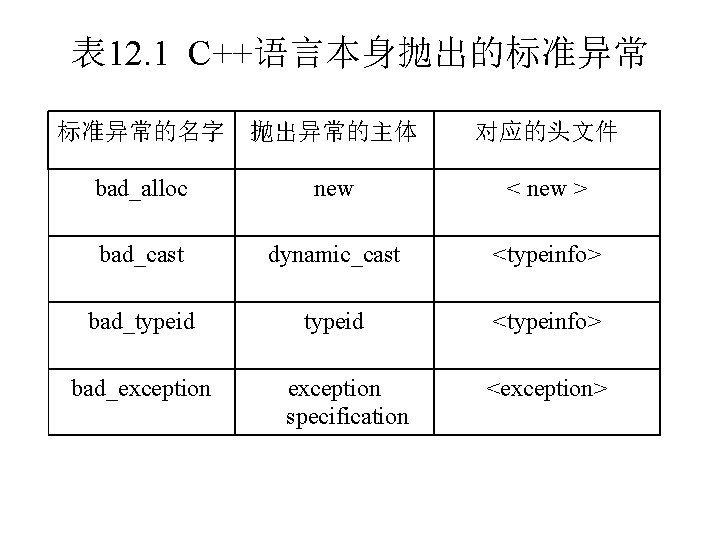
表 12. 1 C++语言本身抛出的标准异常的名字 抛出异常的主体 对应的头文件 bad_alloc new < new > bad_cast dynamic_cast <typeinfo> bad_typeid <typeinfo> bad_exception specification <exception>
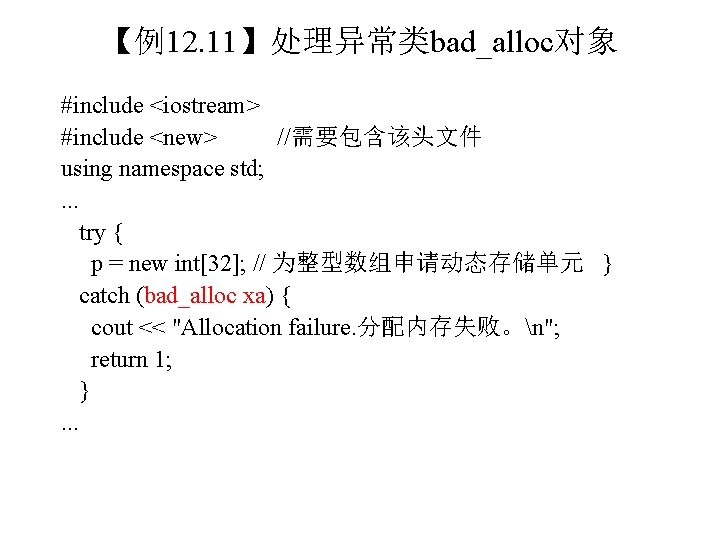
【例12. 11】处理异常类bad_alloc对象 #include <iostream> #include <new> //需要包含该头文件 using namespace std; . . . try { p = new int[32]; // 为整型数组申请动态存储单元 } catch (bad_alloc xa) { cout << "Allocation failure. 分配内存失败。n"; return 1; }. . .
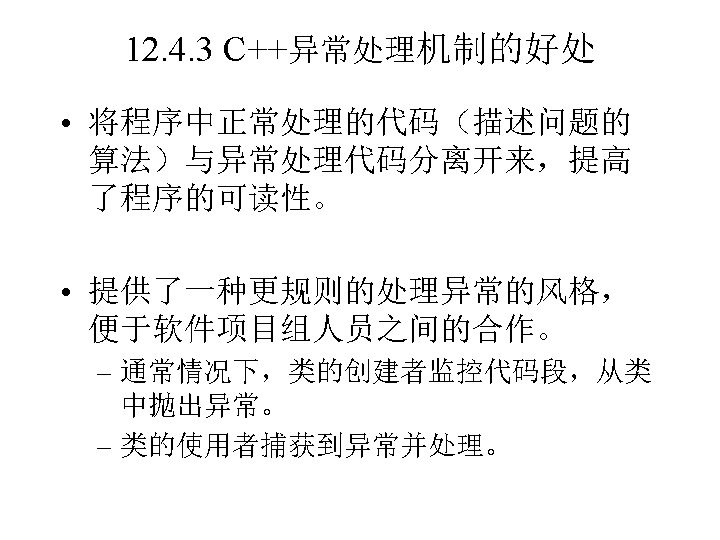
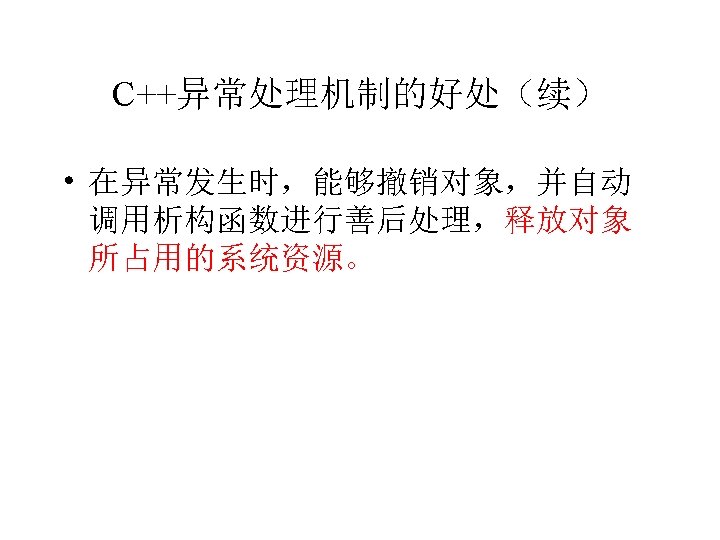
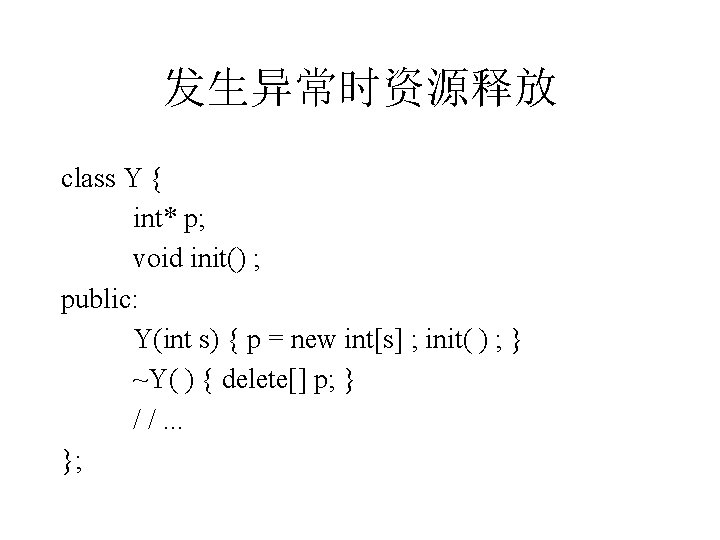
发生异常时资源释放 class Y { int* p; void init() ; public: Y(int s) { p = new int[s] ; init( ) ; } ~Y( ) { delete[] p; } / /. . . };
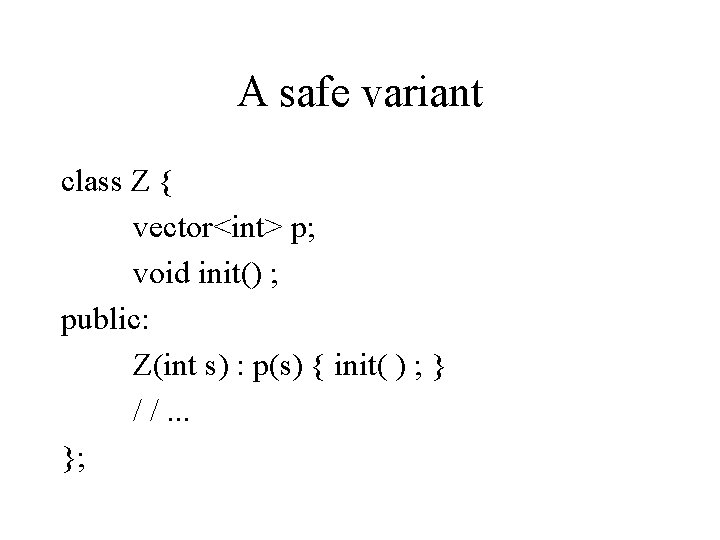
A safe variant class Z { vector<int> p; void init() ; public: Z(int s) : p(s) { init( ) ; } / /. . . };