LOGIC OPERATIONS Logic operations We have already seen
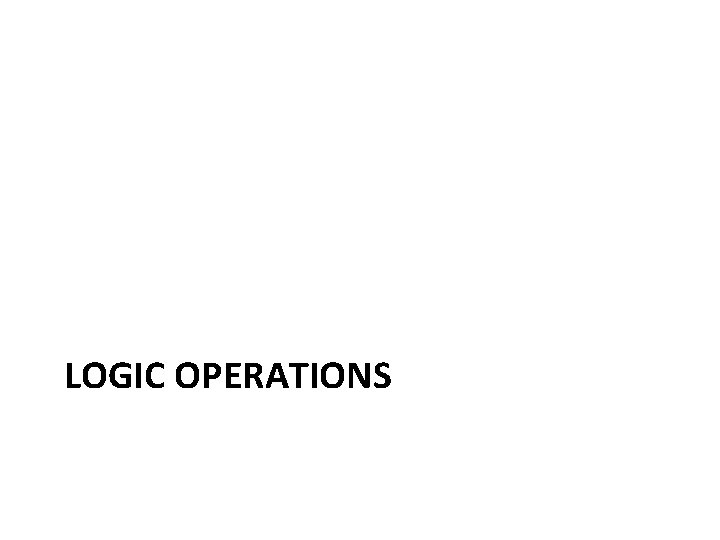
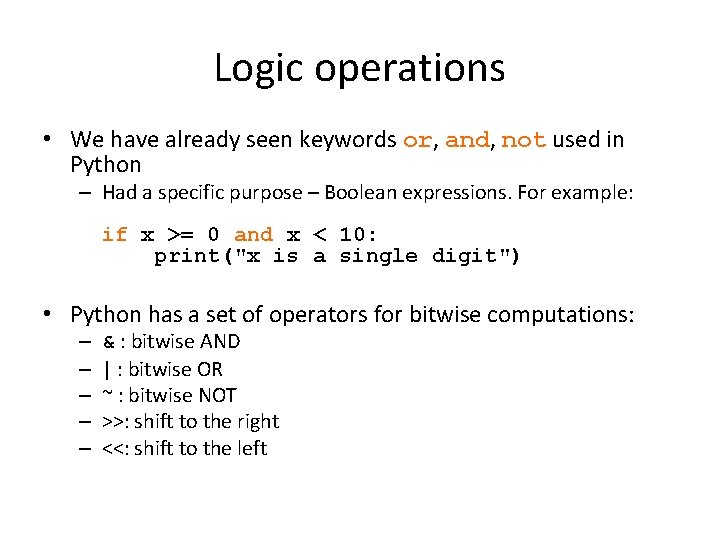
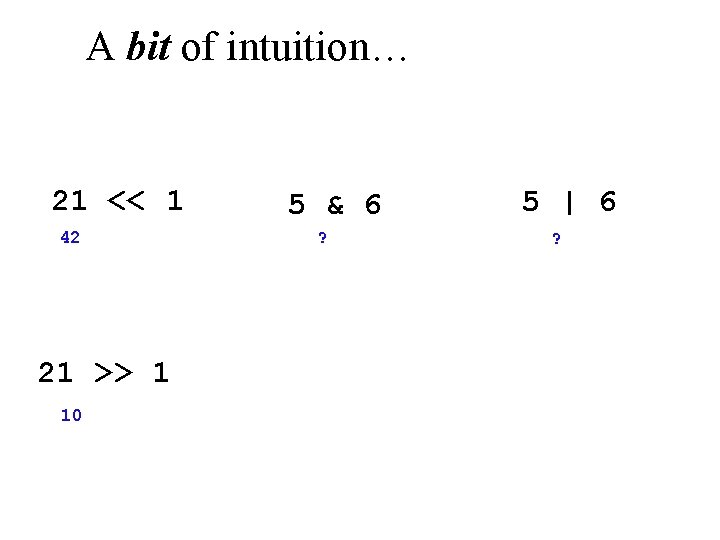
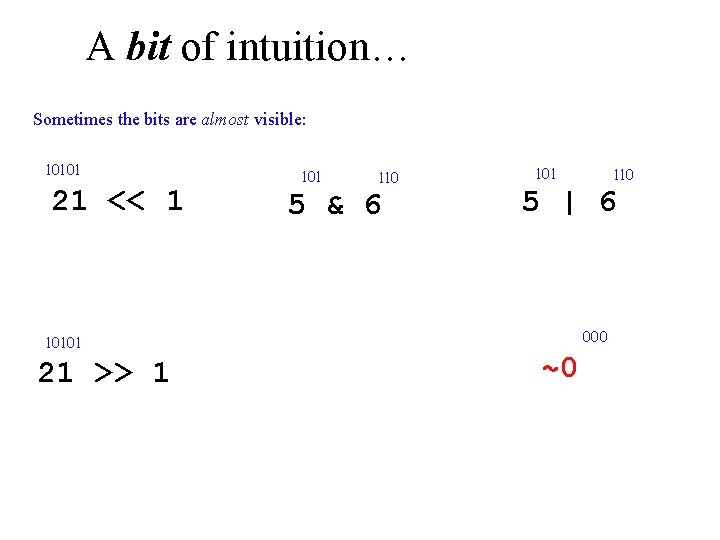
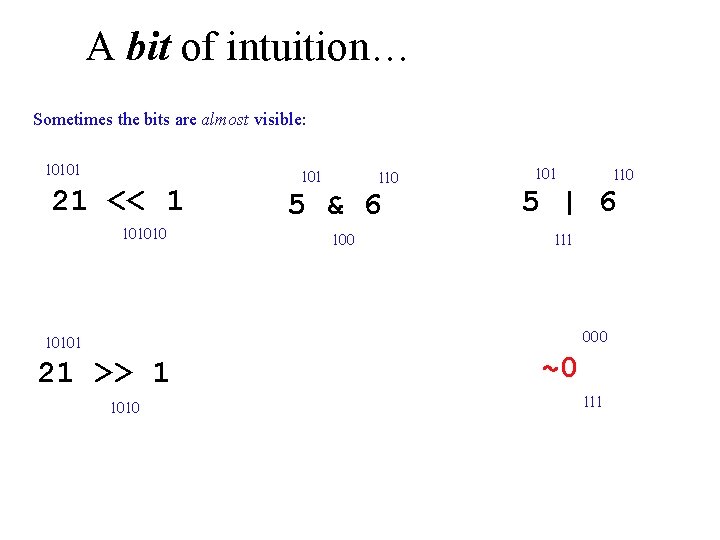
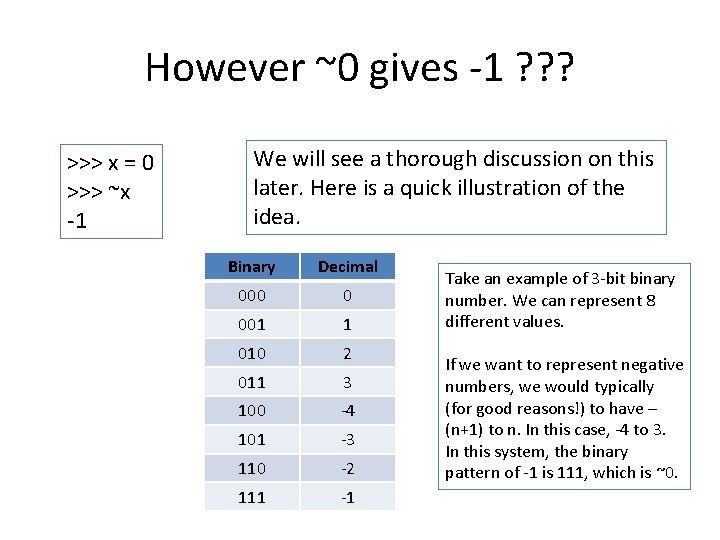
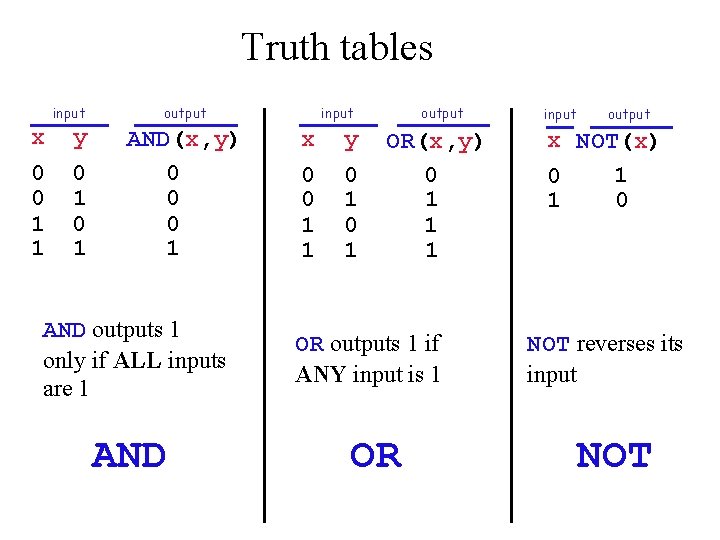
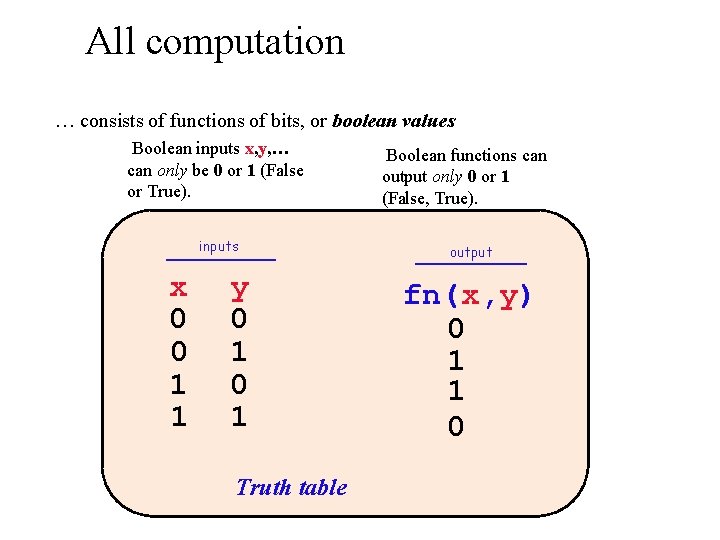
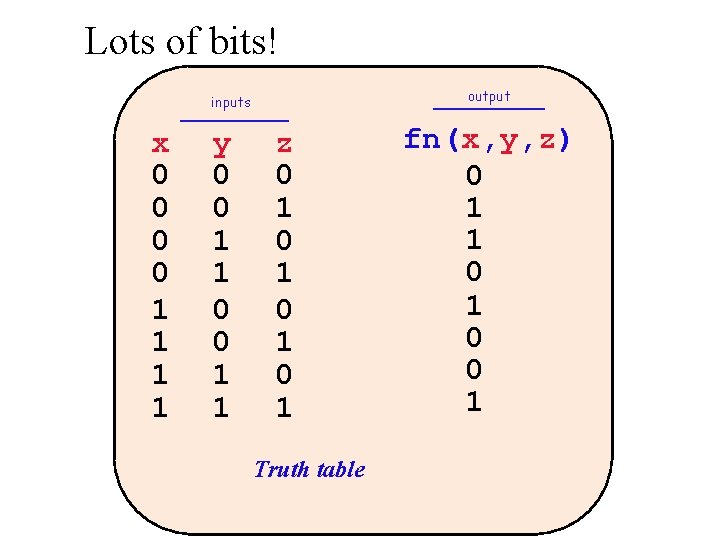
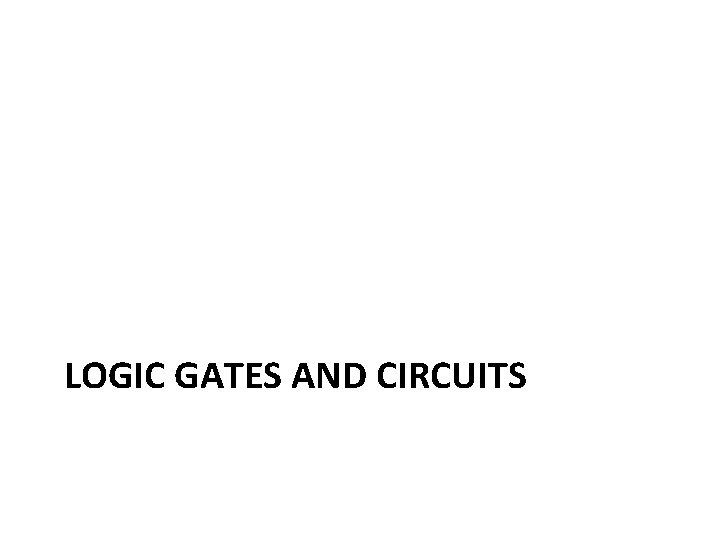
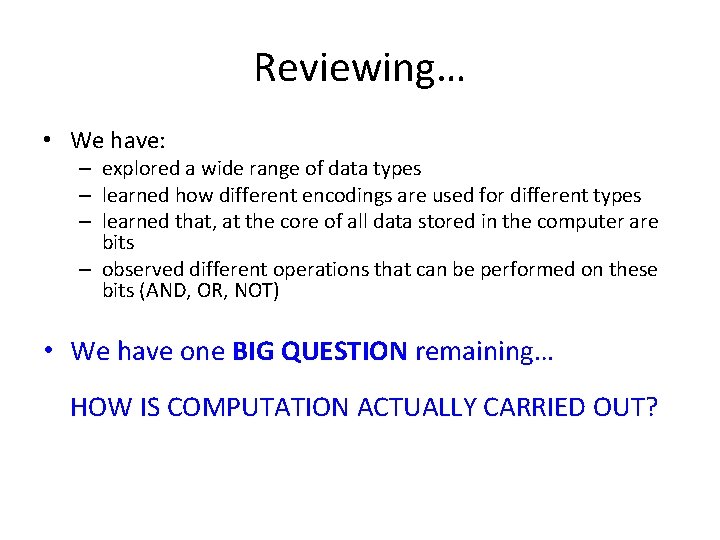
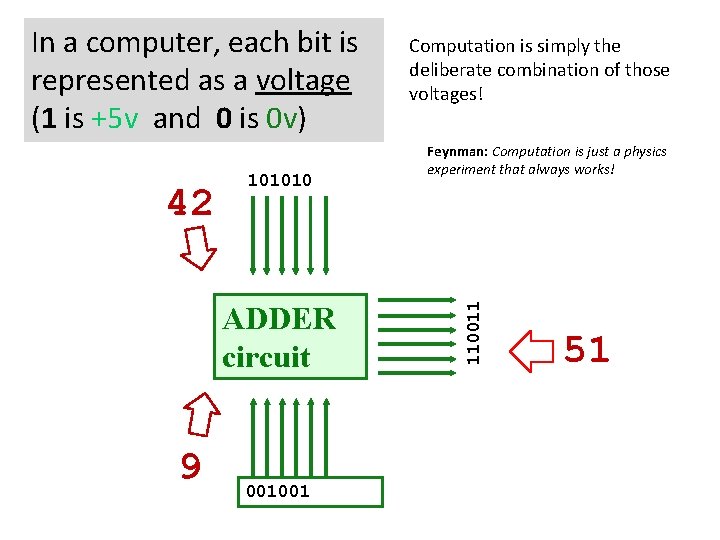
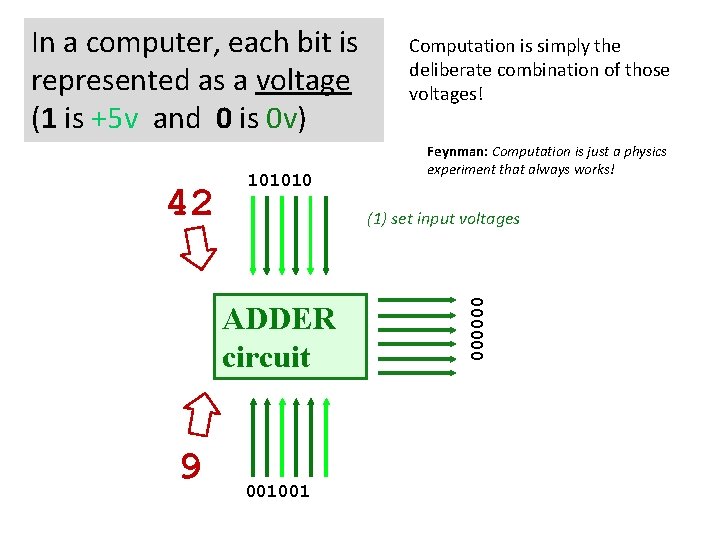
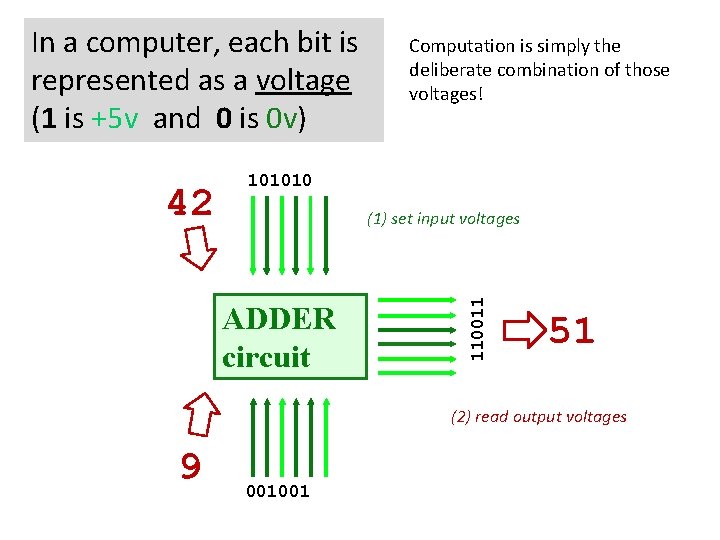
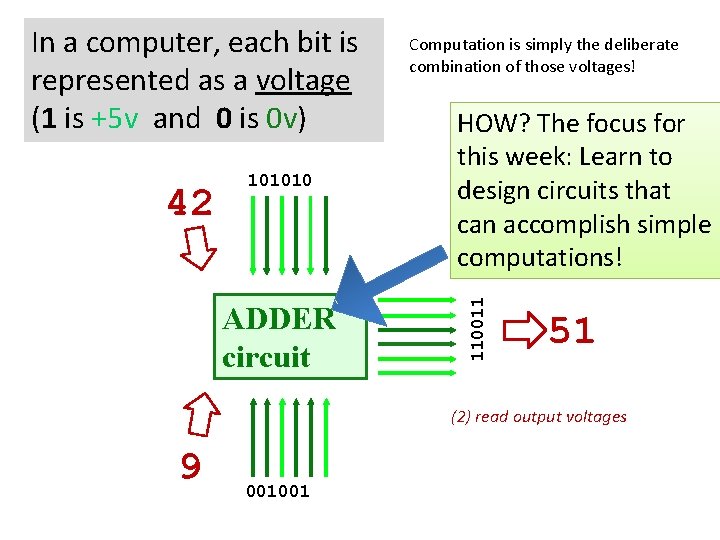
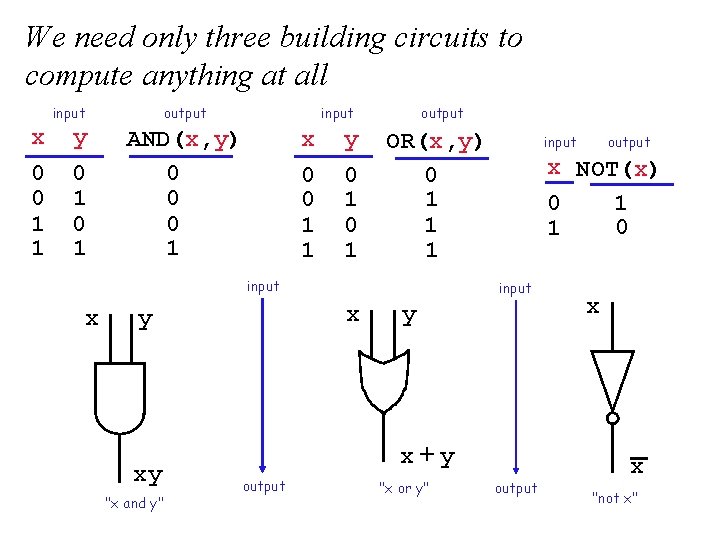
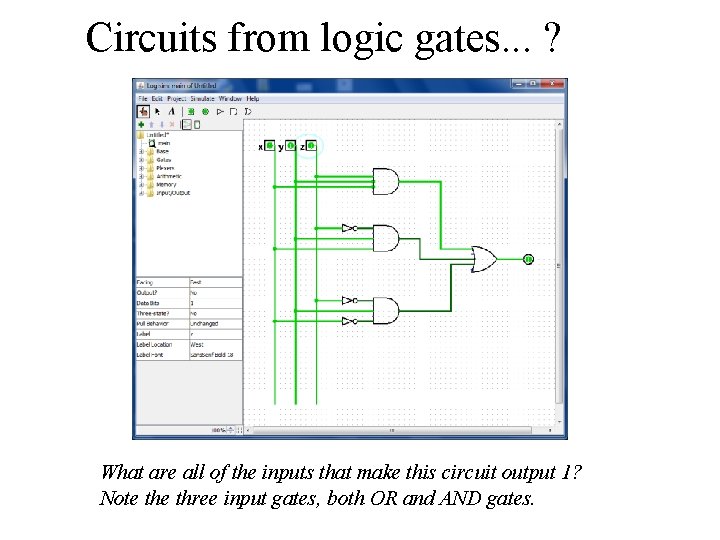
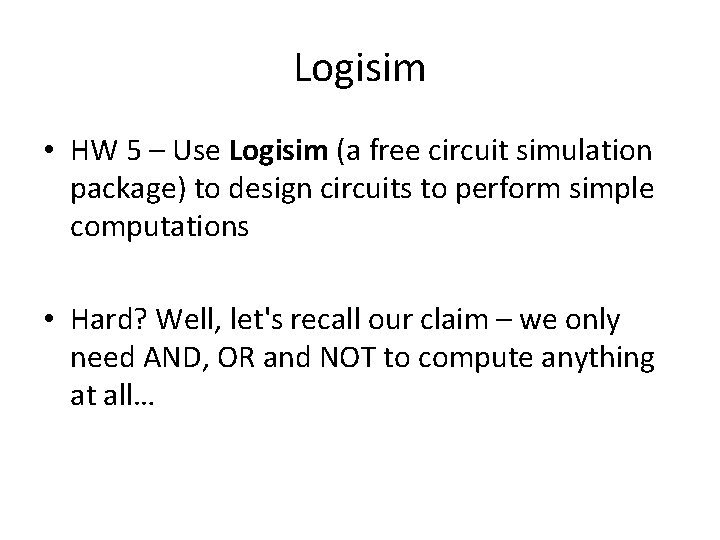
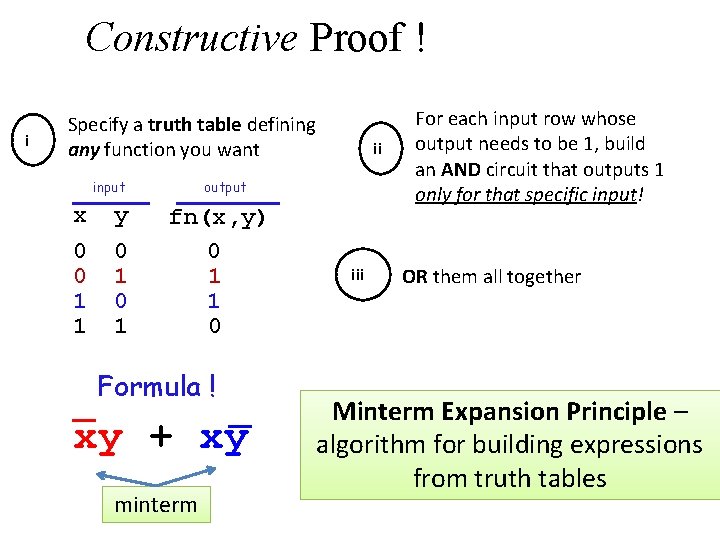
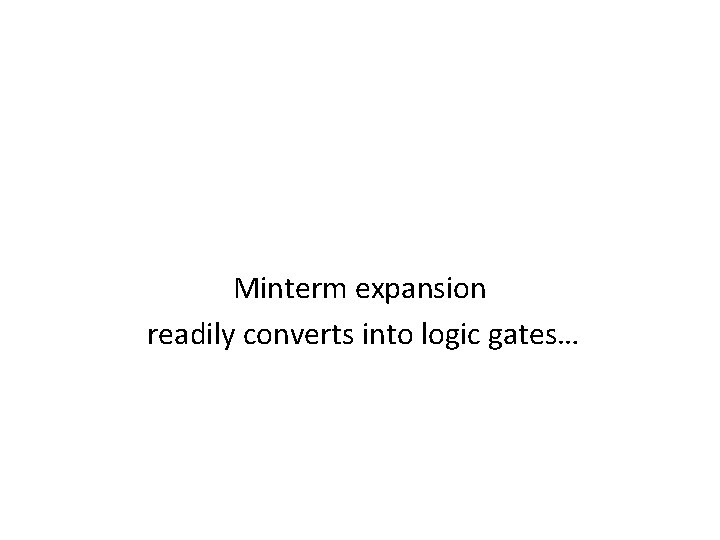
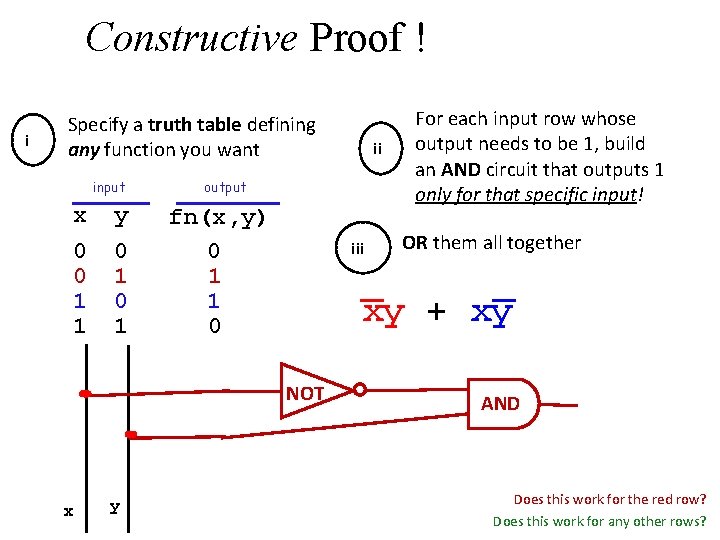
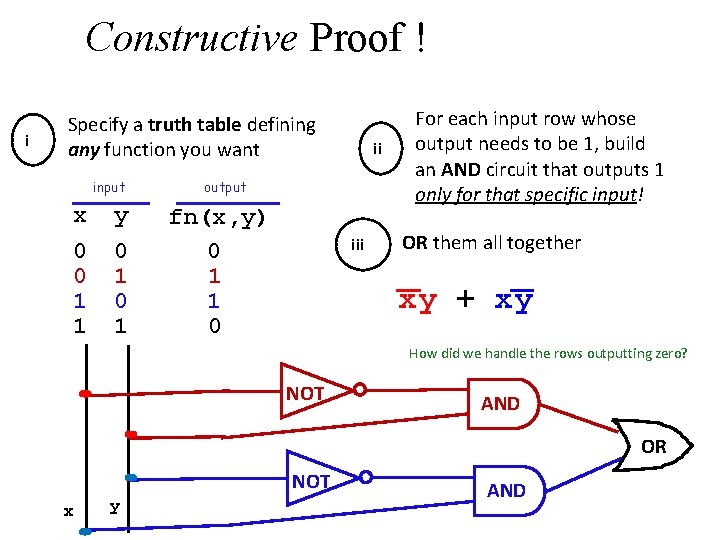
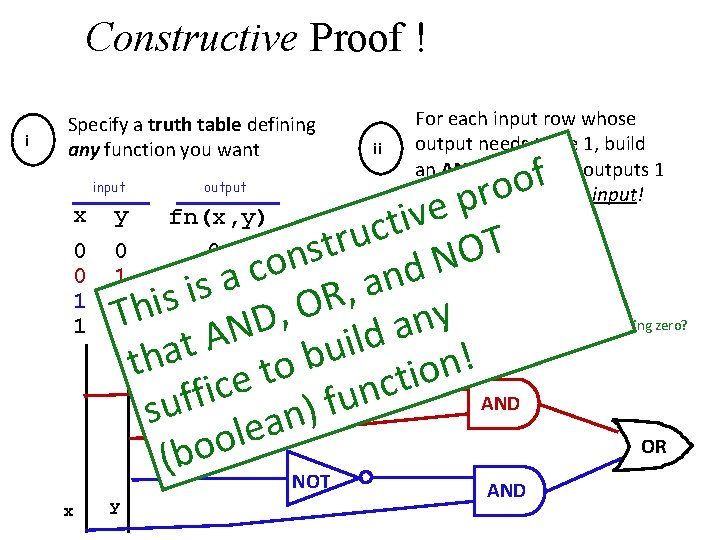
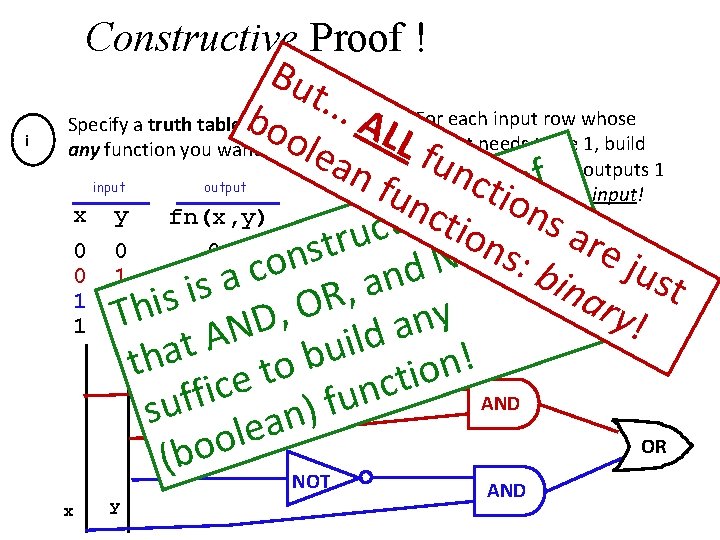
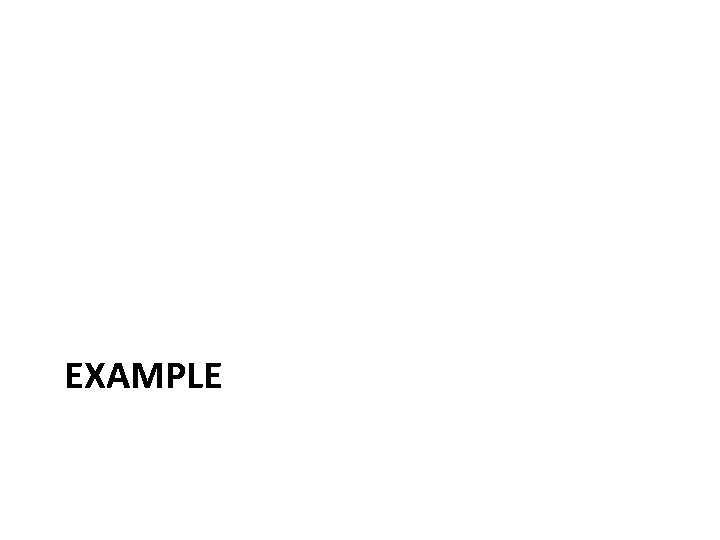
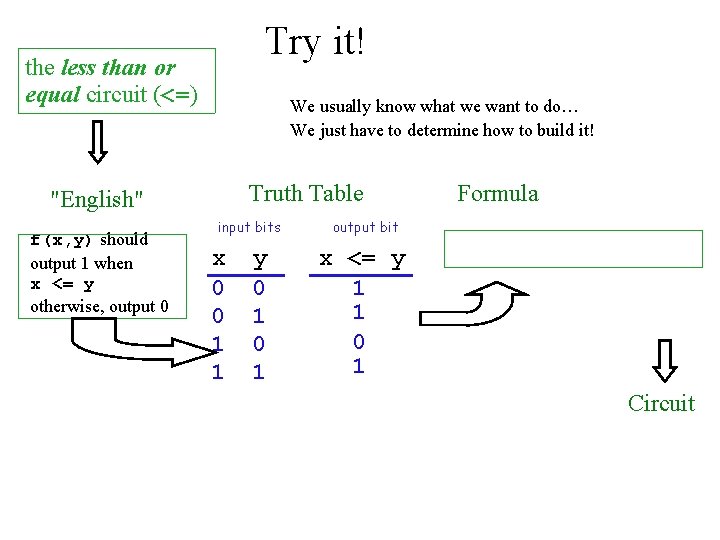
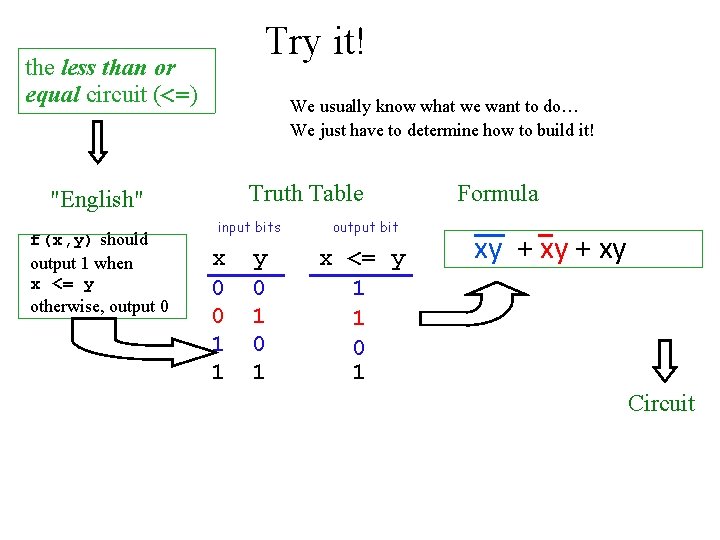
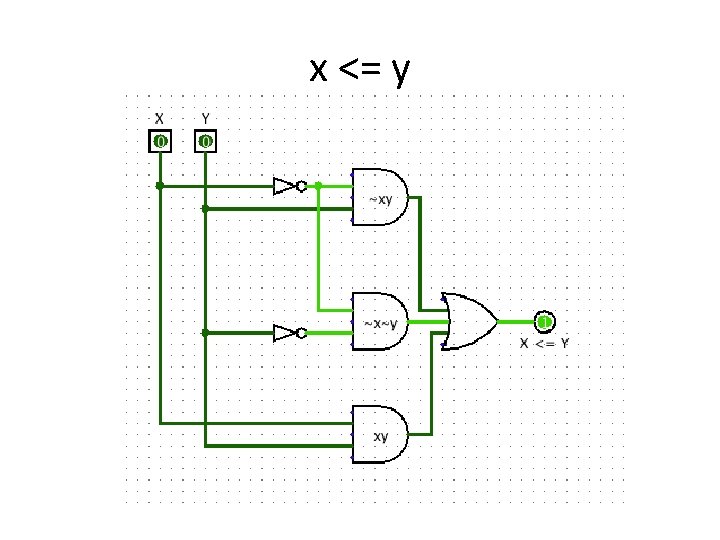
- Slides: 28
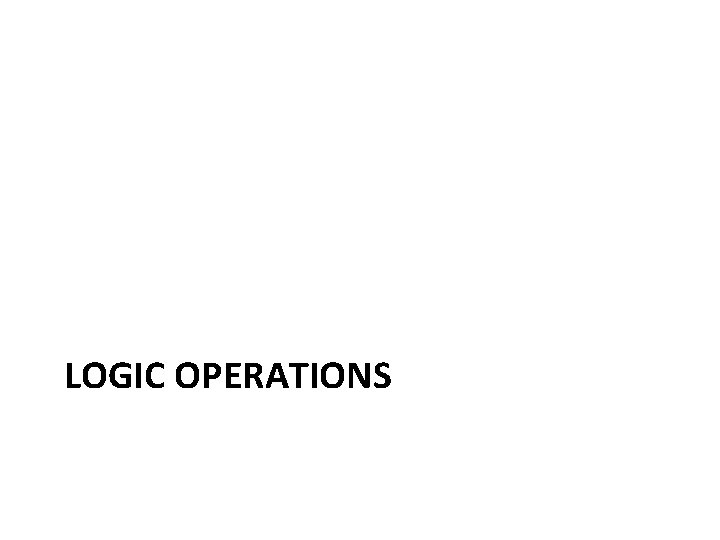
LOGIC OPERATIONS
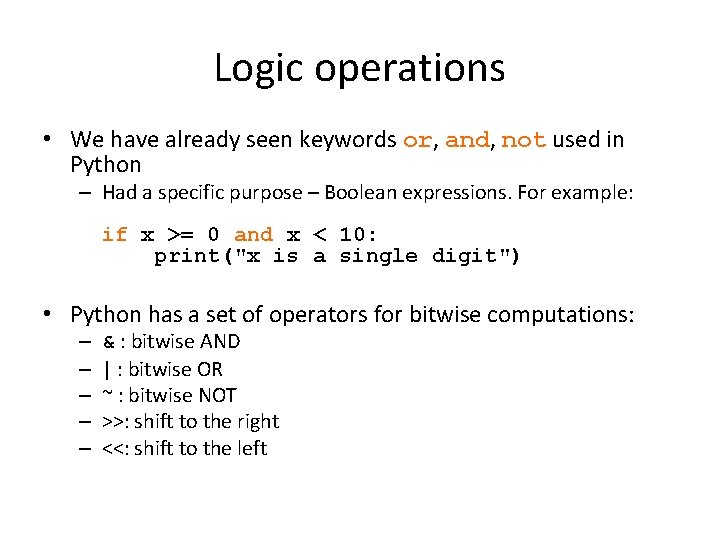
Logic operations • We have already seen keywords or, and, not used in Python – Had a specific purpose – Boolean expressions. For example: if x >= 0 and x < 10: print("x is a single digit") • Python has a set of operators for bitwise computations: – – – & : bitwise AND | : bitwise OR ~ : bitwise NOT >>: shift to the right <<: shift to the left
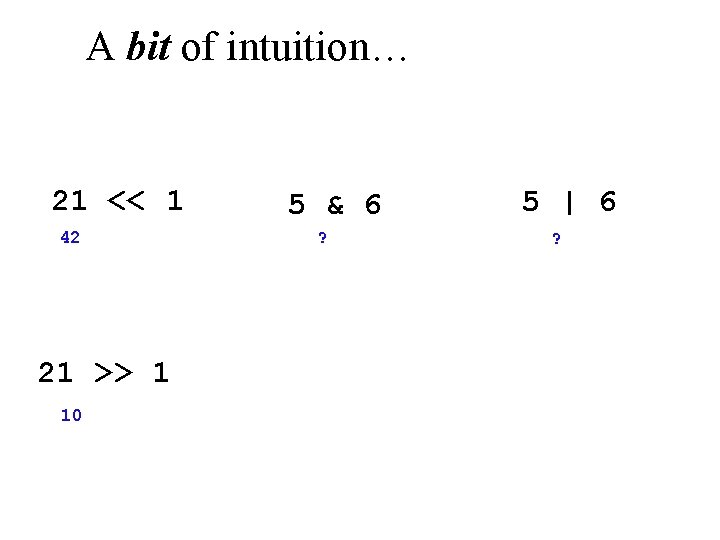
A bit of intuition… 21 << 1 42 21 >> 1 10 5 & 6 ? 5 | 6 ?
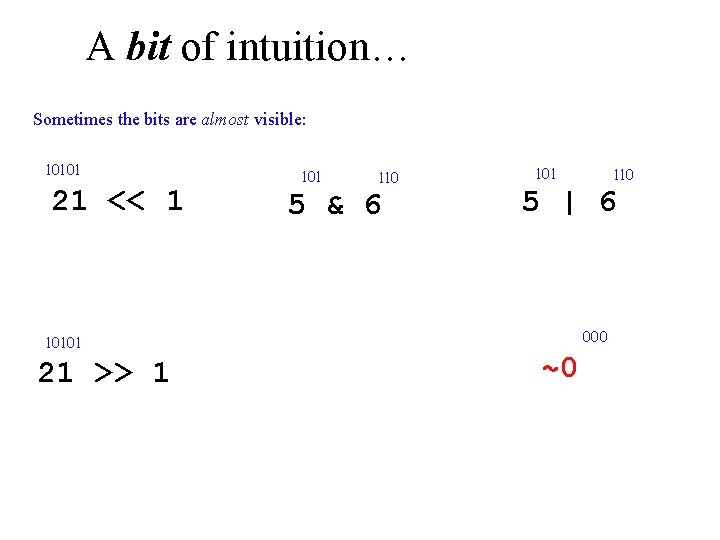
A bit of intuition… Sometimes the bits are almost visible: 10101 21 << 1 10101 21 >> 1 101 110 5 & 6 101 110 5 | 6 000 ~0
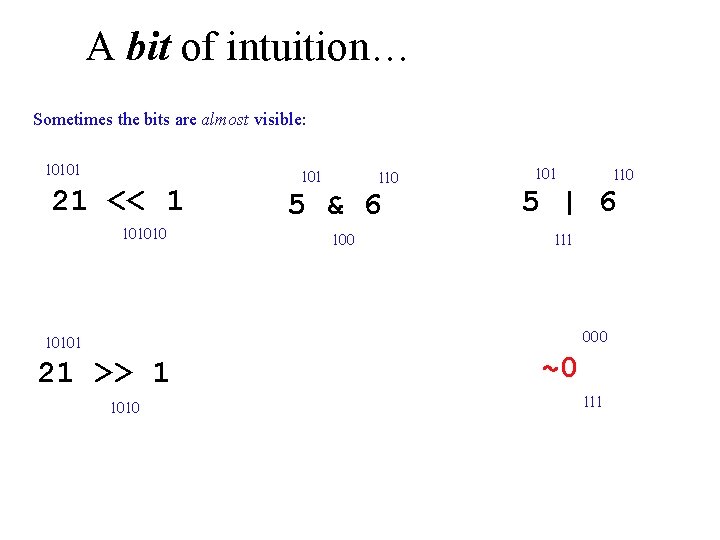
A bit of intuition… Sometimes the bits are almost visible: 10101 21 << 1 101010 101 110 5 & 6 100 101 110 5 | 6 111 000 10101 21 >> 1 1010 ~0 111
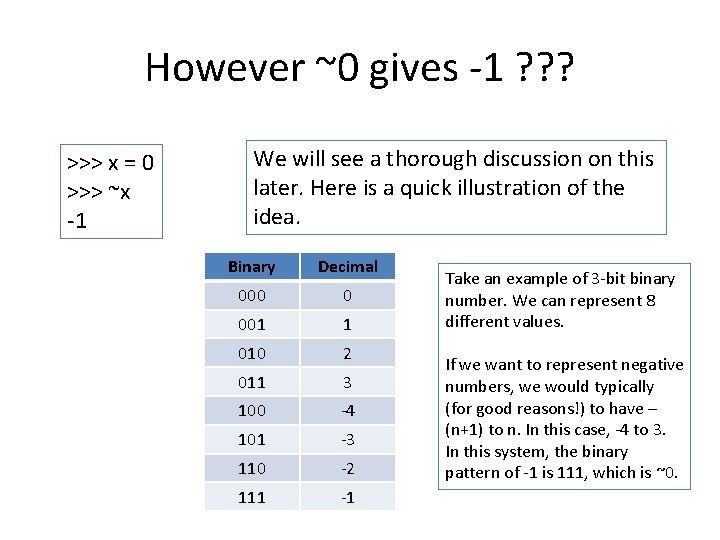
However ~0 gives -1 ? ? ? >>> x = 0 >>> ~x -1 We will see a thorough discussion on this later. Here is a quick illustration of the idea. Binary Decimal 000 0 001 1 010 2 011 3 100 -4 101 -3 110 -2 111 -1 Take an example of 3 -bit binary number. We can represent 8 different values. If we want to represent negative numbers, we would typically (for good reasons!) to have – (n+1) to n. In this case, -4 to 3. In this system, the binary pattern of -1 is 111, which is ~0.
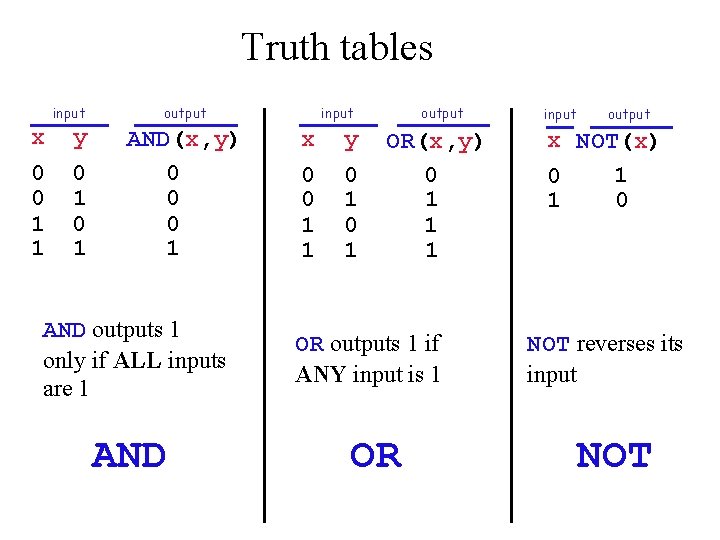
Truth tables input x y 0 0 1 1 0 1 output AND(x, y) 0 0 0 1 input x y 0 0 1 1 0 1 output OR(x, y) 0 1 1 1 AND outputs 1 only if ALL inputs are 1 OR outputs 1 if ANY input is 1 AND OR input output x NOT(x) 1 0 0 1 NOT reverses its input NOT
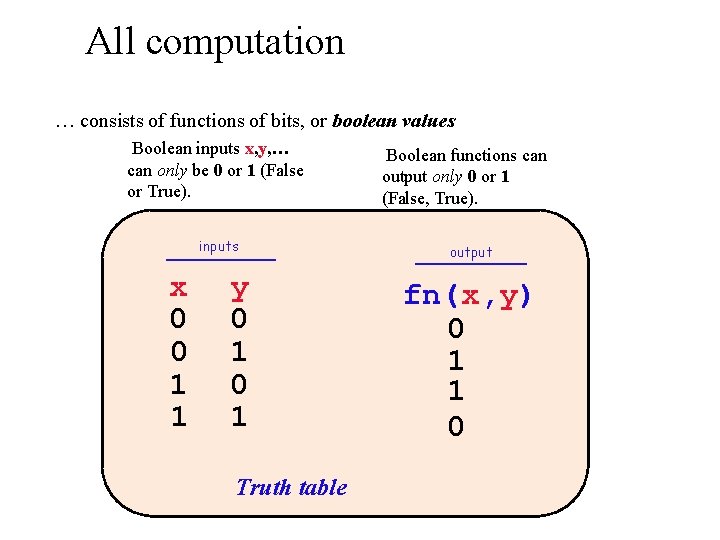
All computation … consists of functions of bits, or boolean values Boolean inputs x, y, … can only be 0 or 1 (False or True). inputs x 0 0 1 1 y 0 1 Truth table Boolean functions can output only 0 or 1 (False, True). output fn(x, y) 0 1 1 0
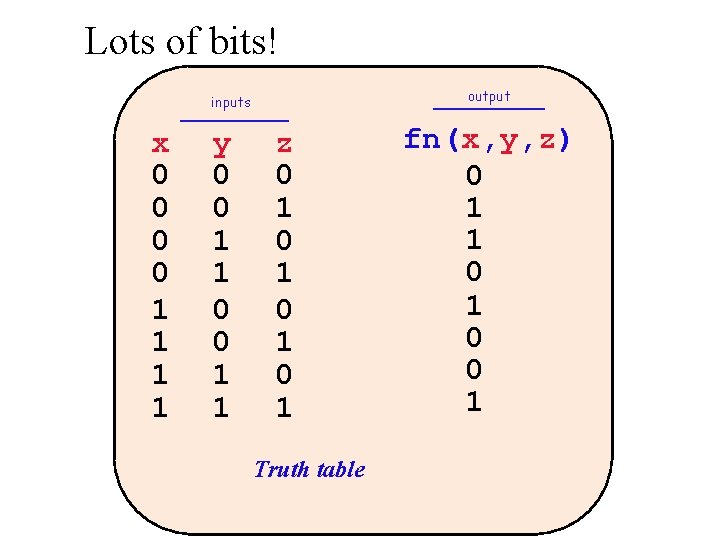
Lots of bits! output inputs x 0 0 1 1 y 0 0 1 1 z 0 1 0 1 Truth table fn(x, y, z) 0 1 1 0 0 1
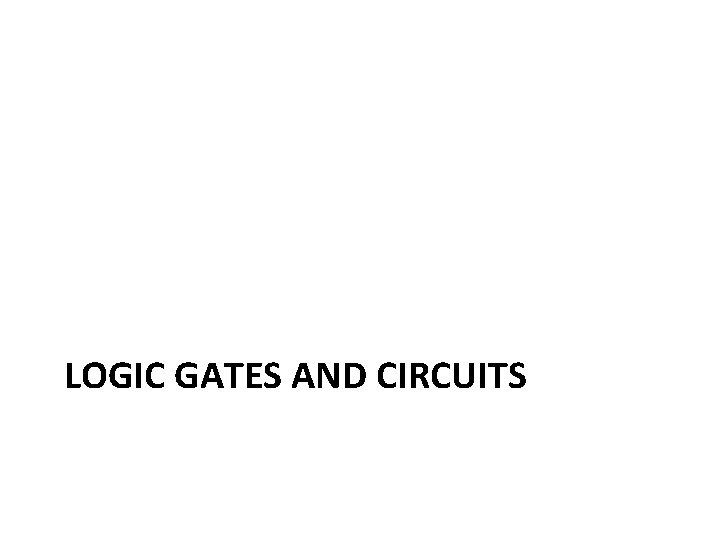
LOGIC GATES AND CIRCUITS
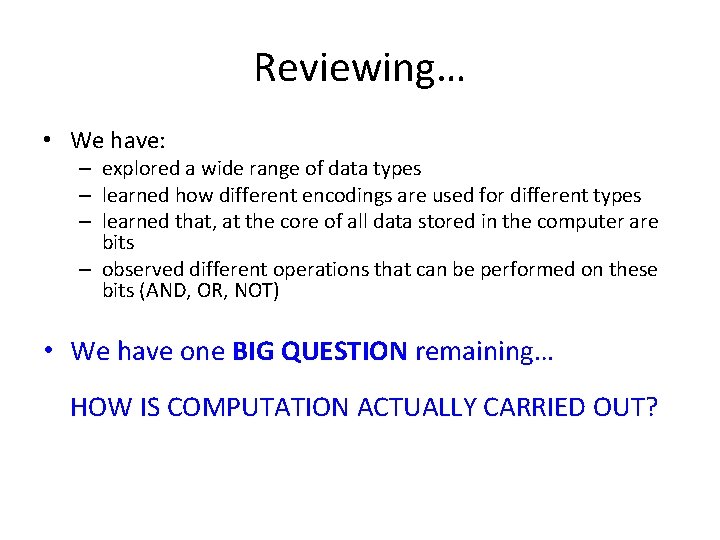
Reviewing… • We have: – explored a wide range of data types – learned how different encodings are used for different types – learned that, at the core of all data stored in the computer are bits – observed different operations that can be performed on these bits (AND, OR, NOT) • We have one BIG QUESTION remaining… HOW IS COMPUTATION ACTUALLY CARRIED OUT?
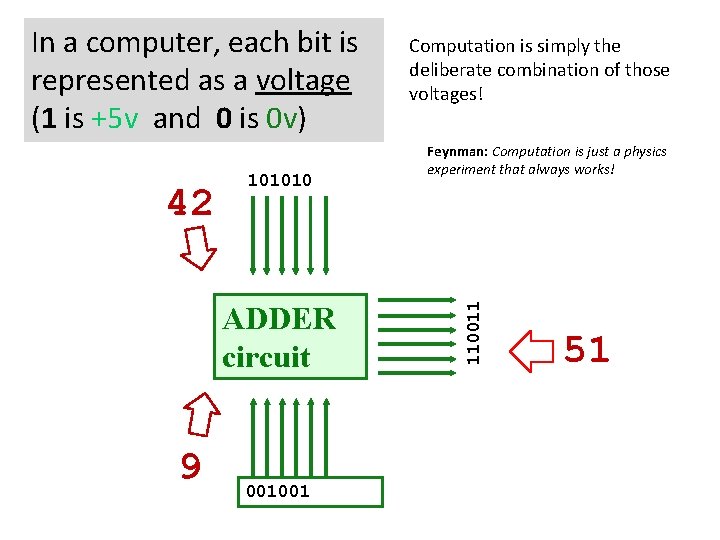
42 101010 ADDER circuit 9 001001 Computation is simply the deliberate combination of those voltages! Feynman: Computation is just a physics experiment that always works! 110011 In a computer, each bit is represented as a voltage (1 is +5 v and 0 is 0 v) 51
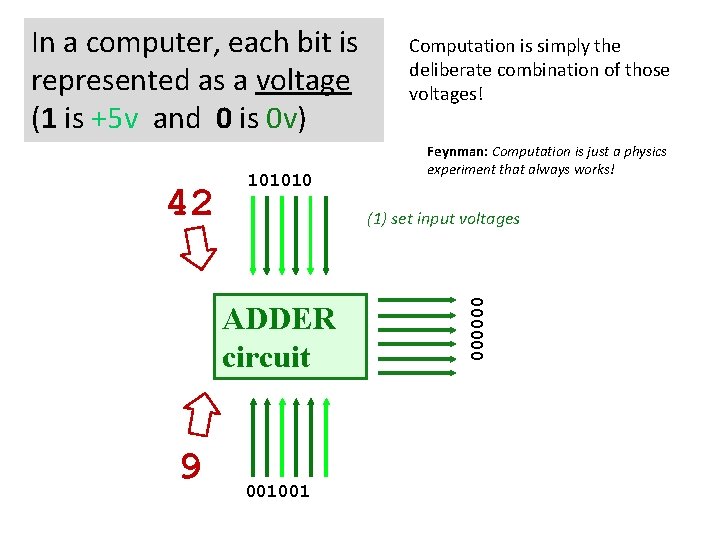
In a computer, each bit is represented as a voltage (1 is +5 v and 0 is 0 v) (1) set input voltages ADDER circuit 9 Feynman: Computation is just a physics experiment that always works! 001001 000000 42 101010 Computation is simply the deliberate combination of those voltages!
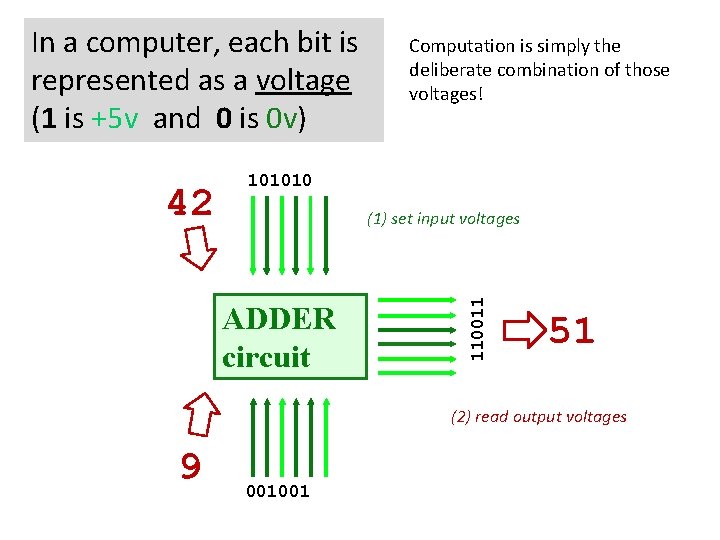
In a computer, each bit is represented as a voltage (1 is +5 v and 0 is 0 v) 101010 (1) set input voltages ADDER circuit 110011 42 Computation is simply the deliberate combination of those voltages! 51 (2) read output voltages 9 001001
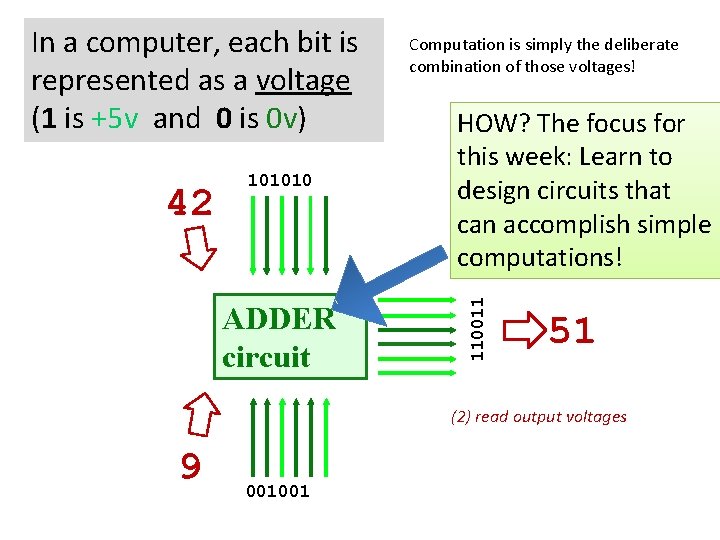
42 101010 ADDER circuit Computation is simply the deliberate combination of those voltages! HOW? The focus for this week: Learn to design circuits that can accomplish simple computations! 110011 In a computer, each bit is represented as a voltage (1 is +5 v and 0 is 0 v) 51 (2) read output voltages 9 001001
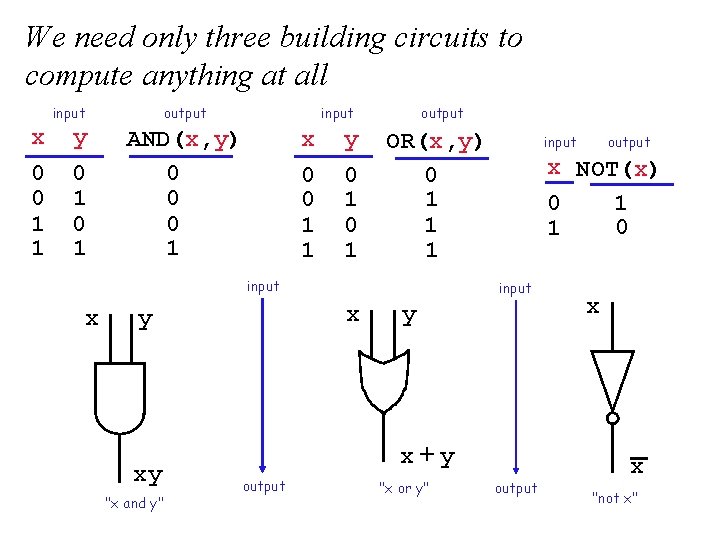
We need only three building circuits to compute anything at all input x y 0 0 1 1 0 1 output input AND(x, y) 0 0 0 1 x y 0 0 1 1 0 1 output OR(x, y) 0 1 1 1 input x xy "x and y" y x+y output "x or y" output x NOT(x) 1 0 0 1 input x y input output x x "not x"
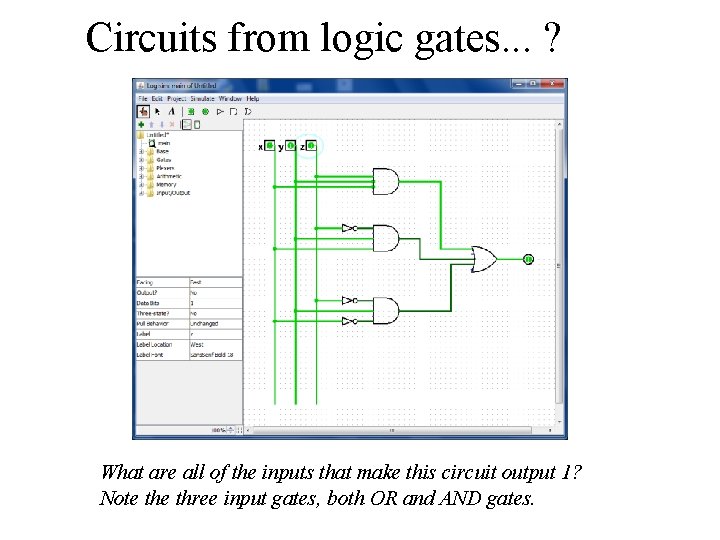
Circuits from logic gates. . . ? What are all of the inputs that make this circuit output 1? Note three input gates, both OR and AND gates.
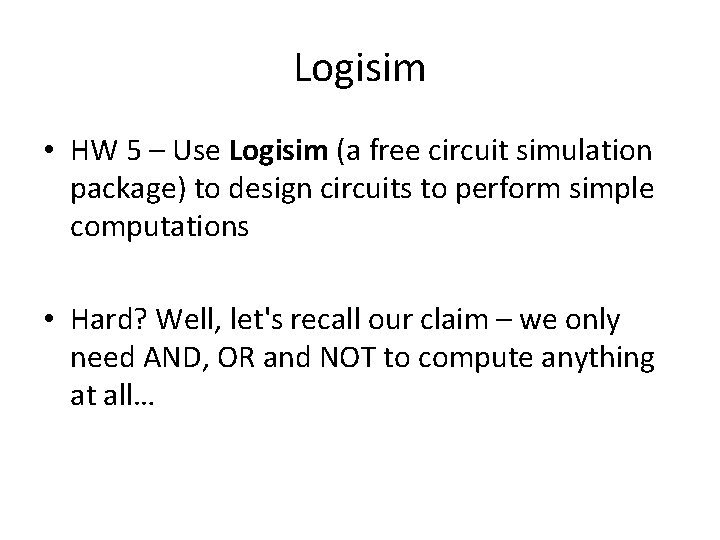
Logisim • HW 5 – Use Logisim (a free circuit simulation package) to design circuits to perform simple computations • Hard? Well, let's recall our claim – we only need AND, OR and NOT to compute anything at all…
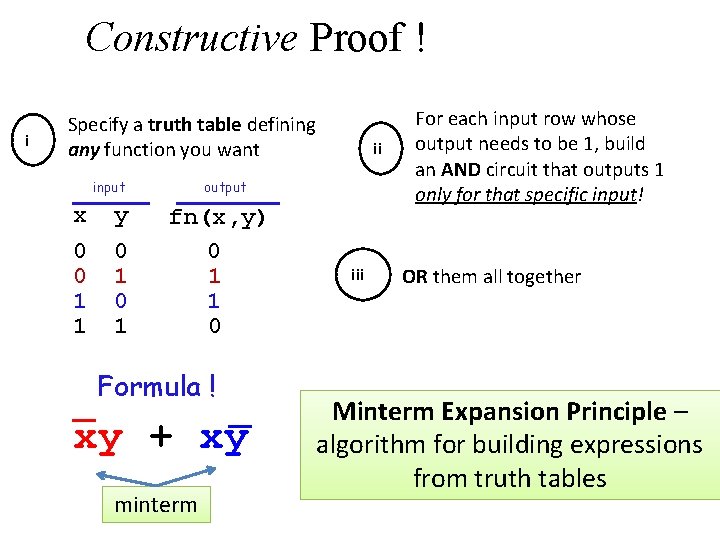
Constructive Proof ! i Specify a truth table defining any function you want input x 0 0 1 1 y 0 1 ii output fn(x, y) 0 1 1 0 Formula ! xy + xy minterm iii For each input row whose output needs to be 1, build an AND circuit that outputs 1 only for that specific input! OR them all together Minterm Expansion Principle – algorithm for building expressions from truth tables
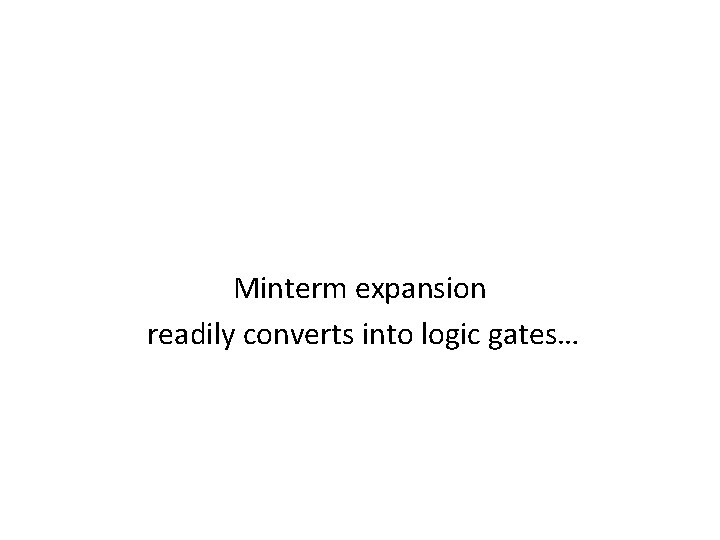
Minterm expansion readily converts into logic gates…
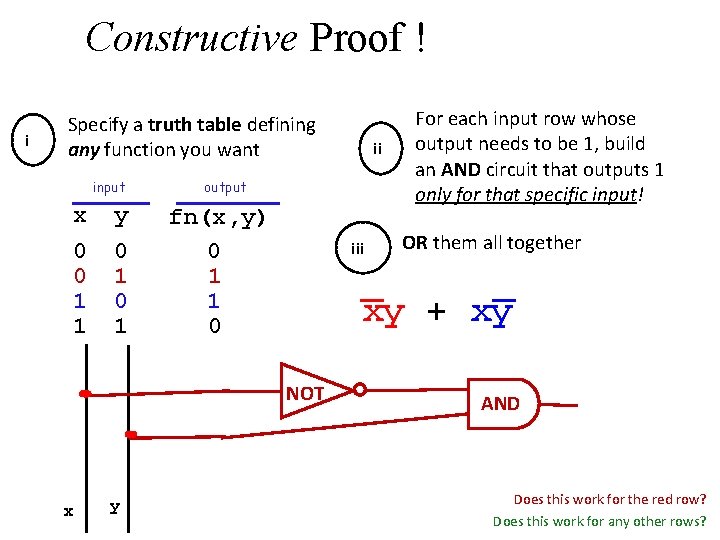
Constructive Proof ! i Specify a truth table defining any function you want input x 0 0 1 1 y 0 1 output fn(x, y) 0 1 1 0 iii y OR them all together xy + xy NOT x ii For each input row whose output needs to be 1, build an AND circuit that outputs 1 only for that specific input! AND Does this work for the red row? Does this work for any other rows?
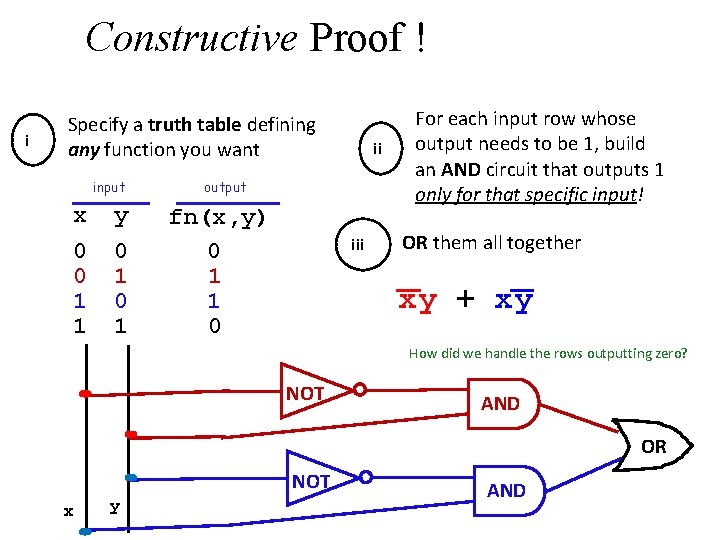
Constructive Proof ! i Specify a truth table defining any function you want input x 0 0 1 1 y 0 1 ii output fn(x, y) 0 1 1 0 iii For each input row whose output needs to be 1, build an AND circuit that outputs 1 only for that specific input! OR them all together xy + xy How did we handle the rows outputting zero? NOT AND OR NOT x y AND
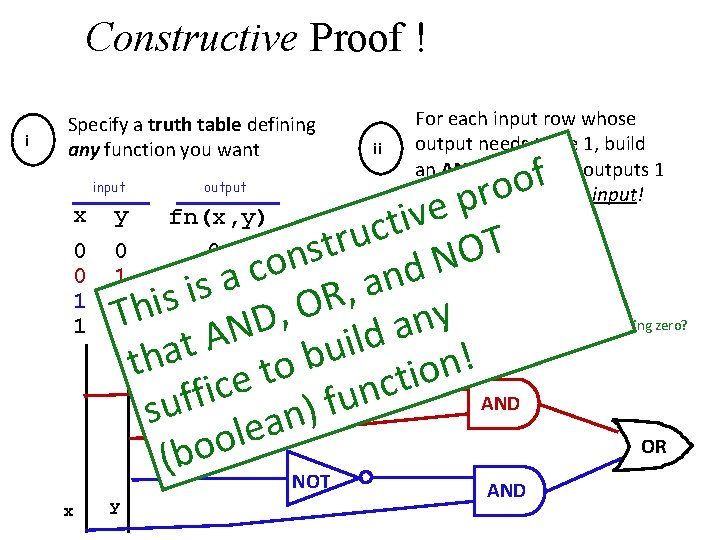
Constructive Proof ! i Specify a truth table defining any function you want f o o r p y e fn(x, y) v i t c u r T t 0 0 s O n N iii OR them all together 1 1 a co d n a , 0 is is 1 R O h , y T 1 0 ND n a d A l i t u a b ! h t n o o t i t e c NOT c i n f AND f u ) s n a e l o (bo NOT input x 0 0 1 1 x ii For each input row whose output needs to be 1, build an AND circuit that outputs 1 only for that specific input! output How did we handle the rows outputting zero? y AND OR
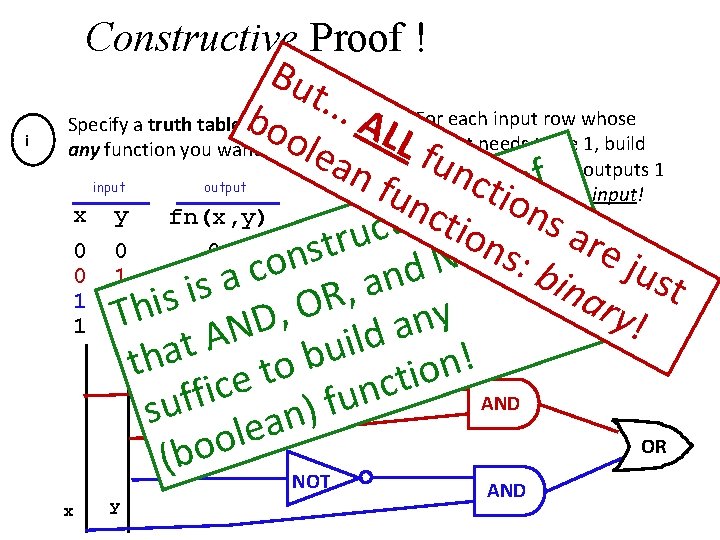
i Constructive Proof ! Bu t. . . For each input row whose A Specify a truth table b defining o L o needs to be 1, build ii any function you want lea L output f u. AND an circuit that outputs 1 nforctthat n f only f o oiospecific input! u r p n n x y e fn(x, y) c v i s t t i c a o u r r n T t 0 0 0 e s O s n N : j iii u OR them all together 0 1 1 a co d b n s i a n t s i , 1 0 is 1 a R r O h , y y T 1 1 0 ND n ! a d A l i t u ! tha e to b n o i t c NOT c i n f AND f u ) s n a e l OR o o b ( input output How did we handle the rows outputting zero? NOT x y AND
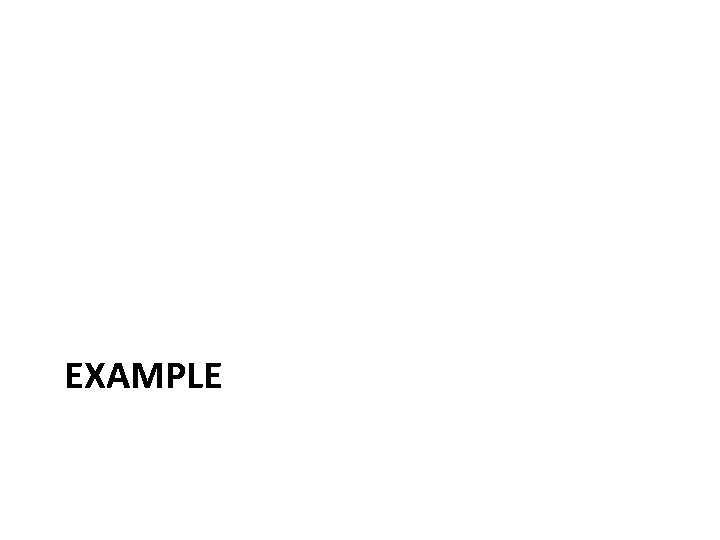
EXAMPLE
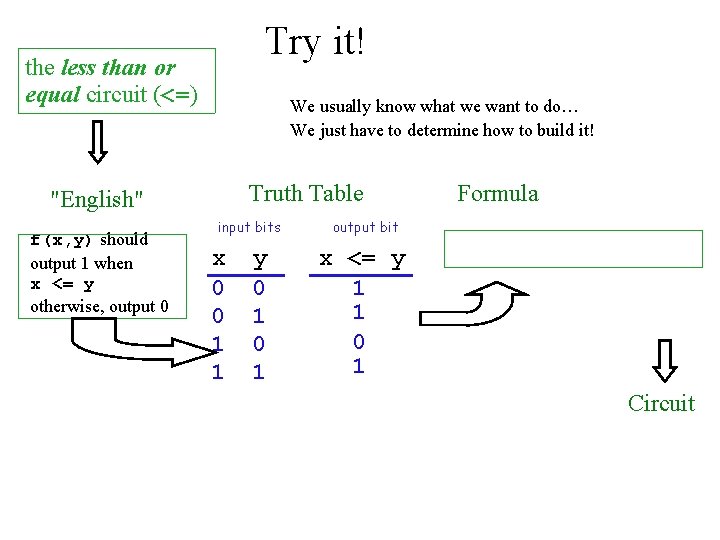
Try it! the less than or equal circuit (<=) We usually know what we want to do… We just have to determine how to build it! Truth Table "English" f(x, y) should output 1 when x <= y otherwise, output 0 input bits x 0 0 1 1 y 0 1 Formula output bit x <= y 1 1 0 1 Circuit
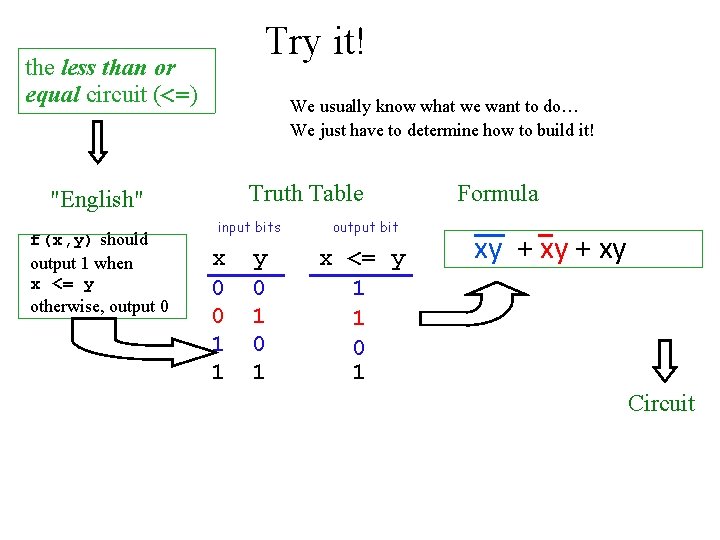
Try it! the less than or equal circuit (<=) We usually know what we want to do… We just have to determine how to build it! Truth Table "English" f(x, y) should output 1 when x <= y otherwise, output 0 input bits x 0 0 1 1 y 0 1 output bit x <= y 1 1 0 1 Formula xy + xy Circuit
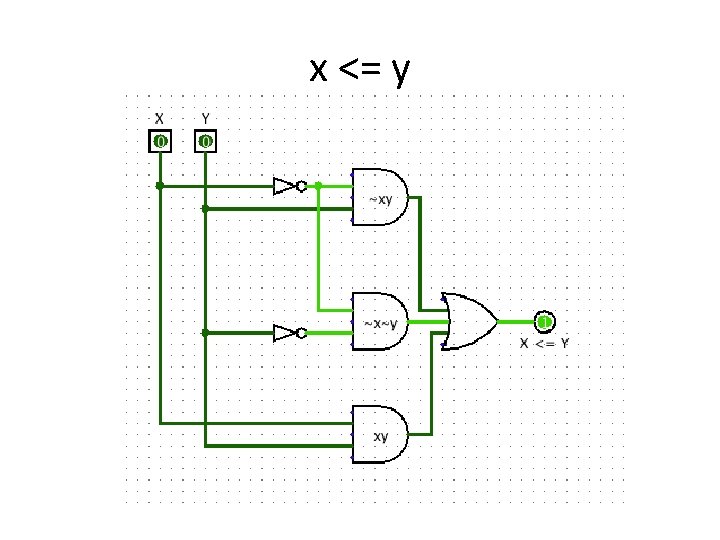
Already can or can already
I seen it already
Yo dejar dejaré correr correré invertir invertiré
L to have breakfast before i went to school
I have already learned
I have already thought
"if you have not already done so"
What have they seen in your house
My eyes have seen the glory of the coming of the lord
Have you ever seen a “greenhouse”?
Have you ever been to museum
My eyes have seen you lyrics
Go tell john what you see
Johnny told me, “i have seen this movie.”
What is a stanza in poetry
Have you ever seen a penguin come to tea
Interesting facts about gcina mhlophe
Reverse image search
Plural crocodile
Animals whose ears cannot see
I may have seen you
What is prufrock's problem
I have never seen a coward man like sohan
What have they seen in your house
What has 6 faces and 8 vertices
First order logic vs propositional logic
First order logic vs propositional logic
Third order logic
Combinational logic vs sequential logic